Python Create Directory If Not Exists
One common task that Python developers frequently encounter is the need to create directories programmatically. Creating a directory in Python is a straightforward process, but before creating a new directory, it is essential to check if the directory already exists. In this article, we will explore different methods of checking directory existence and creating directories if they do not already exist. We will also discuss handling exceptions and error conditions, performing additional checks, using conditional statements, and handling edge cases and special situations.
Checking if a Directory Exists
Before proceeding with the creation of a new directory, it is important to check whether the directory already exists. We can determine directory existence using either the `os.path.exists()` function or the `Path.exists()` method from the `pathlib` module.
Using the `os.path.exists()` function:
The `os.path.exists()` function returns `True` if the path passed as an argument exists, whether it is a directory or a file. Here is an example of using `os.path.exists()` to check for directory existence:
“`python
import os
if os.path.exists(‘/path/to/directory’):
print(‘Directory exists’)
else:
print(‘Directory does not exist’)
“`
Implementing the `pathlib` module’s `Path.exists()` method:
The `Path.exists()` method is provided by the `pathlib` module, which provides an object-oriented approach to working with filesystem paths. Here is an example of using `Path.exists()` to check for directory existence:
“`python
from pathlib import Path
directory_path = Path(‘/path/to/directory’)
if directory_path.exists():
print(‘Directory exists’)
else:
print(‘Directory does not exist’)
“`
Creating a Directory if It Does Not Exist
Once we have determined that a directory does not exist, we can proceed with creating it. Python provides different methods for creating directories, such as the `os.makedirs()` function and the `Path.mkdir()` method from the `pathlib` module. Additionally, we can specify directory permissions while creating a new directory.
Utilizing the `os.makedirs()` function:
The `os.makedirs()` function creates directories recursively. That means it creates any necessary parent directories in the given path if they do not already exist. Here is an example of using `os.makedirs()` to create a directory:
“`python
import os
os.makedirs(‘/path/to/new_directory’)
“`
Using the `pathlib` module’s `Path.mkdir()` method:
The `Path.mkdir()` method from the `pathlib` module creates a new directory at the given path. By default, it raises a `FileExistsError` exception if the directory already exists. Here is an example of using `Path.mkdir()` to create a directory:
“`python
from pathlib import Path
directory_path = Path(‘/path/to/new_directory’)
directory_path.mkdir()
“`
Specifying directory permissions while creating:
Both the `os.makedirs()` function and the `Path.mkdir()` method allow us to specify directory permissions while creating a new directory. We can use the `mode` parameter to define the permissions as an octal value. Here is an example of creating a directory with specific permissions:
“`python
import os
os.makedirs(‘/path/to/new_directory’, mode=0o755)
“`
Handling Exceptions and Error Conditions
During the directory creation process, we may encounter various exceptions and error conditions. It is important to handle these exceptions gracefully to ensure the robustness of our code. Let’s explore some common scenarios and how to deal with them.
Handling `FileExistsError` exception:
If a directory already exists at the specified path, both `os.makedirs()` and `Path.mkdir()` raise a `FileExistsError` exception. We can catch this exception and handle it accordingly. Here is an example of handling the `FileExistsError` exception:
“`python
import os
try:
os.makedirs(‘/path/to/new_directory’)
except FileExistsError:
print(‘Directory already exists’)
“`
Dealing with `PermissionError` exception:
If we do not have permission to create a directory at the specified path, both `os.makedirs()` and `Path.mkdir()` raise a `PermissionError` exception. We can catch this exception and handle it appropriately. Here is an example of handling the `PermissionError` exception:
“`python
import os
try:
os.makedirs(‘/path/to/new_directory’)
except PermissionError:
print(‘Permission denied’)
“`
Addressing other potential errors:
In addition to `FileExistsError` and `PermissionError`, other exceptions may occur during the directory creation process. It is a good practice to catch any unexpected exceptions and handle them gracefully. Here is an example of catching generic exceptions:
“`python
import os
try:
os.makedirs(‘/path/to/new_directory’)
except Exception as e:
print(f’An error occurred: {str(e)}’)
“`
Checking Directory Existence on a Specific Path
In some situations, we may need to check whether a given path is a directory or a file. Python provides methods to verify folder existence, such as `os.path.isdir()` and the `is_dir()` method from the `pathlib` module.
Verifying folder existence with `os.path.isdir()` function:
The `os.path.isdir()` function returns `True` if the specified path exists and is a directory. Here is an example of using `os.path.isdir()` to check directory existence:
“`python
import os
if os.path.isdir(‘/path/to/directory’):
print(‘Folder exists’)
else:
print(‘Folder does not exist’)
“`
Utilizing the `is_dir()` method in `pathlib` module:
The `is_dir()` method from the `pathlib` module returns `True` if the path exists and is a directory. Here is an example of using `is_dir()` to check directory existence:
“`python
from pathlib import Path
directory_path = Path(‘/path/to/directory’)
if directory_path.is_dir():
print(‘Folder exists’)
else:
print(‘Folder does not exist’)
“`
Adding More Robustness to the Directory Creation Process
To make the directory creation process more robust, we can perform additional checks before creating a new directory. Let’s explore some techniques to ensure the reliability of our code.
Checking for directory existence with `os.path.islink()` function:
The `os.path.islink()` function returns `True` if the specified path exists and is a symbolic link. We can use this function to determine if the given path points to a symbolic link instead of a directory. Here is an example of using `os.path.islink()` to check for symbolic links:
“`python
import os
if os.path.islink(‘/path/to/directory’):
print(‘Path is a symbolic link’)
else:
print(‘Path is not a symbolic link’)
“`
Validating that a path is a directory using `pathlib` module’s `is_dir()` method:
The `is_dir()` method from the `pathlib` module returns `True` if the path exists and is a directory. By using this method, we can ensure that the given path is indeed a directory before creating a new one. Here is an example of validating path as a directory:
“`python
from pathlib import Path
directory_path = Path(‘/path/to/new_directory’)
if not directory_path.is_dir():
directory_path.mkdir()
“`
Performing Additional Checks before Directory Creation
Before creating a new directory, we can perform additional checks to validate the directory path and ensure proper accessibility and permissions. Let’s explore a few techniques to enhance our code reliability.
Verifying directory path validity with `os.path.isabs()` function:
The `os.path.isabs()` function returns `True` if the specified path is an absolute path. This can be useful to ensure that the directory path we are working with is a valid absolute path. Here is an example of using `os.path.isabs()` for path validation:
“`python
import os
if os.path.isabs(‘/path/to/directory’):
print(‘Path is absolute’)
else:
print(‘Path is not absolute’)
“`
Checking path accessibility and permissions using `os.access()` function:
The `os.access()` function allows us to check whether the current process has access permissions to the specified path. We can use this function to ensure that the directory path is accessible before creating a new directory. Here is an example of using `os.access()` for path accessibility check:
“`python
import os
if os.access(‘/path/to/directory’, os.W_OK):
print(‘Path is writable’)
else:
print(‘Path is not writable’)
“`
Using Conditional Statements for Directory Creation
To create a directory only if certain conditions are met, we can employ conditional statements. By combining checks with logical operators, we can create complex conditions for directory creation.
Employing `if` statements to check directory existence before creation:
We can use an `if` statement to check if a directory already exists before creating it. By using the `os.path.exists()` function or the `Path.exists()` method, we can conditionally create a directory based on its existence. Here is an example of using an `if` statement for directory creation:
“`python
import os
if not os.path.exists(‘/path/to/new_directory’):
os.makedirs(‘/path/to/new_directory’)
“`
Combining checks with logical operators for complex conditions:
By combining multiple checks using logical operators such as `and` and `or`, we can create more complex conditions for directory creation. Here is an example of combining checks using logical operators:
“`python
import os
if not os.path.exists(‘/path/to/new_directory’) or not os.path.isdir(‘/path/to/new_directory’):
os.makedirs(‘/path/to/new_directory’)
“`
Handling Edge Cases and Special Situations
In certain scenarios, we may encounter edge cases or special situations regarding directory creation. Let’s explore a few potential challenges and how to address them.
Handling relative paths and ensuring correct working directory:
If we are using relative paths to specify the directory, it is important to ensure that the correct working directory is set. We can achieve this by using the `os.chdir()` function to change the current working directory before creating the new directory. Here is an example of handling relative paths:
“`python
import os
os.chdir(‘/path/to/current_directory’)
os.makedirs(‘new_directory’)
“`
Dealing with potential symbolic link conflicts:
When creating a new directory, there might be situations where the specified path coincides with an existing symbolic link. In such cases, we can validate the path as a directory using `os.path.islink()` before creating it. Here is an example of handling symbolic link conflicts:
“`python
import os
if not os.path.islink(‘/path/to/new_directory’):
os.makedirs(‘/path/to/new_directory’)
“`
Addressing platform-specific differences in directory creation behavior:
There might be platform-specific differences in how directories are created, such as variations in default permissions or behavior when creating directories on network drives. It is important to consider such differences and take appropriate measures to ensure consistent and reliable directory creation across different platforms.
FAQs
Q: Can I create a file if the directory does not exist?
A: Yes, you can create a file if the directory does not already exist. You can use the same directory existence checks and creation methods mentioned in this article to create both directories and files.
Q: How can I copy a file and automatically create the destination directory if it does not exist?
A: To copy a file and automatically create the destination directory if it does not exist, you can use the `shutil.copy()` function from the `shutil` module. This function creates any necessary parent directories in the destination path if they do not already exist.
Q: How can I check if a folder exists in Python and create it if it does not?
A: To check if a folder exists in Python, you can use the `os.path.exists()` function or the `Path.exists()` method from the `pathlib` module. If the folder does not exist, you can create it using the `os.makedirs()` function or the `Path.mkdir()` method, specifying the folder path as the argument.
Create Directory If Not Exist In Python 😀
Keywords searched by users: python create directory if not exists Os mkdir if not exists, Python create file if not exists, Path mkdir if not exists, Python check directory exists if not create, Os mkdir exist ok, Create folder if it doesn t exist Python, Python copy file auto create directory if not exist, Check folder exist Python
Categories: Top 55 Python Create Directory If Not Exists
See more here: nhanvietluanvan.com
Os Mkdir If Not Exists
Introduction:
In Python, the `os` (Operating System) module provides a range of functions to interact with the underlying operating system. Among these functions, `os.mkdir()` stands out as a powerful tool for creating directories. However, there is a common scenario where performing a directory creation operation might result in an error if the specified directory already exists. To address this issue, Python offers a handy solution – `os.mkdir if not exists`, which intelligently checks for the existence of a directory before attempting to create it. In this article, we will explore this functionality in depth, explaining its syntax, usage, and answering frequently asked questions.
An Overview of `os.mkdir`:
Before diving into `os.mkdir if not exists`, let us briefly explore the basic functionality of `os.mkdir`. This function is used to create a single directory path specified as an argument. However, if the directory already exists, it raises a `FileExistsError` exception, preventing overwriting of existing directories.
Syntax and Usage of `os.mkdir if not exists`:
The `os.mkdir if not exists` pattern enables us to gracefully handle directory creation by checking if it exists before attempting to create it. Here’s the syntax of how it is applied in Python:
“`python
import os
path = ‘path/to/directory’
if not os.path.exists(path):
os.mkdir(path)
“`
Explanation of the Syntax:
1. We begin by importing the `os` module to utilize its functions.
2. Next, we specify the `path` variable, representing the desired directory path to be created.
3. Using an `if` statement, we check if the directory doesn’t exist by invoking `os.path.exists()` with the `path` as an argument.
4. If the condition is true (i.e., the directory doesn’t exist), we call `os.mkdir()` with `path` as the argument to create the directory.
Explanation of the Logic:
By employing this method, we can safely create a directory without encountering any errors associated with existing directories. If the specified directory path already exists, the condition in the `if` statement returns `False`, and hence, `os.mkdir()` is not executed. Conversely, if the directory path doesn’t exist, the condition evaluates to `True`, prompting the creation of the directory using `os.mkdir()`.
FAQs:
Q1. What happens if the specified directory already exists?
A1. In the case of standard usage without using `os.mkdir if not exists`, the `os.mkdir()` function raises a `FileExistsError` exception, indicating that the directory already exists. However, using `os.mkdir if not exists` pattern allows us to avoid such exceptions by checking for directory existence before attempting to create it.
Q2. Does `os.mkdir if not exists` work only for a single-directory depth?
A2. No, `os.mkdir if not exists` can be employed to create a directory at any depth, including subdirectories. The functionality inherently checks for the existence of the complete directory path provided.
Q3. Are there any alternative methods to `os.mkdir if not exists`?
A3. Indeed, an alternative approach is to use `os.makedirs()` instead of `os.mkdir()`. While `os.mkdir()` only creates a single directory, `os.makedirs()` can handle the creation of multiple subdirectories. In this case, if any intermediate directories already exist, they are not affected, ensuring a safe creation of the desired directory.
Q4. What if the creation of the directory fails due to insufficient permissions?
A4. Whether you use `os.mkdir()` or `os.mkdir if not exists`, both functions will raise a `PermissionError` if the user does not have sufficient permissions to create directories in the specified location.
Q5. Can `os.mkdir if not exists` be used for dynamic directory creation?
A5. Yes, absolutely. The condition within the `if` statement can be expanded to include dynamic logic to determine whether a directory should be created based on specific conditions or user input.
Conclusion:
In this article, we explored the `os.mkdir if not exists` pattern in Python. We now understand how this useful functionality allows us to safely create directories without encountering errors caused by existing directories. By using this approach, we can efficiently handle directory creation and avoid exceptions. Remember, it is always advisable to check for directory existence before attempting to create it, especially when dealing with unpredictable scenarios.
Python Create File If Not Exists
Python, being one of the most popular programming languages today, offers a wide range of functionalities to meet various programming needs. File operations are an essential aspect of any programming language, and Python provides seamless ways to interact with files. One common requirement is to create a file if it does not already exist. In this article, we will explore how to achieve this in Python, delve into the intricacies of working with files, and answer frequently asked questions related to this topic.
Creating a file in Python can be accomplished using the built-in `open()` function. However, if the file already exists, opening it with the `w` or `x` mode provided by `open()` will override the existing file. To create a file only if it does not already exist, we need to perform additional checks.
One way to accomplish this is by using the `os` module. The `os.path` sub-module provides methods that allow us to perform operations on paths, such as checking if a file or directory exists. By combining the functionalities of `os` with `open()`, we can create a file if it does not exist. Here’s an example:
“`python
import os
def create_file_if_not_exists(file_path):
if not os.path.exists(file_path):
with open(file_path, ‘w’) as file:
# Perform necessary operations on the file
file.write(“Hello, World!”)
print(“File created.”)
else:
print(“File already exists.”)
# Usage:
create_file_if_not_exists(“example.txt”)
“`
In the above code, we import the `os` module and define a function `create_file_if_not_exists` that takes the `file_path` as an argument. We use `os.path.exists(file_path)` to check if the file already exists. If it does not exist, we create the file using `open()` with the `w` mode and perform the desired operations. On the other hand, if the file already exists, we print a message indicating that it is already present.
It’s important to note that when creating a file, we typically specify the mode as `w`, which represents write mode. This mode truncates the file if it already exists or creates a new file if it doesn’t. If we want to append to an existing file instead of overriding it, we can use the `a` mode.
Frequently Asked Questions:
Q1. Can we achieve the same result with pure Python, without using the `os` module?
Yes, we can also create a file if it doesn’t exist without using the `os` module. By utilizing the exception handling mechanism in Python, we can catch a specific error `FileNotFoundError` that occurs when a file is not found, and create the file within the exception block.
Here’s an example:
“`python
def create_file_if_not_exists(file_path):
try:
with open(file_path, ‘r’):
pass
print(“File already exists.”)
except FileNotFoundError:
with open(file_path, ‘w’) as file:
# Perform necessary operations on the file
file.write(“Hello, World!”)
print(“File created.”)
# Usage:
create_file_if_not_exists(“example.txt”)
“`
In the above code, we attempt to open the file in `r` mode, which throws a `FileNotFoundError` if the file does not exist. If the exception occurs, we create the file within the `except` block using `open()` with `w` mode.
Q2. Is there any performance difference between the two approaches?
Performance-wise, using the `os` module is generally more efficient when checking if a file exists, particularly in scenarios where you are frequently performing file operations. However, for occasional file creations, the performance difference may be negligible.
Q3. What if we want to create directories as well as files?
If you need to create directories along with files, you can use the `os.makedirs()` function. This function creates any intermediate-level directories if they don’t already exist.
Here’s an example:
“`python
import os
def create_file_with_directories_if_not_exists(file_path):
file_dir = os.path.dirname(file_path)
os.makedirs(file_dir, exist_ok=True)
if not os.path.exists(file_path):
with open(file_path, ‘w’) as file:
# Perform necessary operations on the file
file.write(“Hello, World!”)
print(“File created.”)
else:
print(“File already exists.”)
# Usage:
create_file_with_directories_if_not_exists(“path/to/example.txt”)
“`
In the code snippet above, we use `os.makedirs(file_dir, exist_ok=True)` to create the parent directories of the file if they don’t already exist.
In conclusion, creating a file if it doesn’t already exist in Python can be achieved by using the `os` module or by utilizing the exception handling mechanism. Both approaches offer efficient solutions, and the choice depends on the specific requirements of the project. By understanding these techniques, you can seamlessly handle file operations in Python while ensuring file integrity and adherence to program logic.
Path Mkdir If Not Exists
In the world of programming, the creation of directories is an essential task that developers often have to deal with. The process typically involves creating a new directory, but before doing so, it is important to ensure the directory does not already exist. This is where the “Path mkdir if not exists” concept comes into play.
The “Path mkdir if not exists” function is specifically designed to create a new directory only if it does not already exist. It is widely used in programming languages like Python, Java, and C++, allowing developers to efficiently manage directories without encountering any errors or overwriting existing directories unintentionally.
When using this function, programmers can be confident that the code will perform as expected, even if the directory already exists. This eliminates the need for additional checks and improves the overall efficiency of the program. Let’s delve deeper into how this concept works and its significance in programming.
How does it work?
The “Path mkdir if not exists” function uses the Path class, which represents a file or directory path in a file system. It provides various methods to manipulate paths, ensuring the smooth management of directories. The specific method, “mkdir if not exists,” checks if the directory already exists. If it does, the function simply returns without creating a new directory. However, if the directory does not exist, the function creates it.
Essentially, it combines checking the existence of a directory and creating one in a single method call, simplifying the code and preventing unnecessary duplication or errors. Developers can focus on other aspects of their program while this function takes care of directory management efficiently.
Why is it important?
The importance of the “Path mkdir if not exists” concept lies in its ability to streamline directory management. Without this functionality, developers would have to manually check for the existence of a directory before attempting to create a new one. This manual check often involves additional lines of code, making the program more complex and increasing the likelihood of introducing errors.
Moreover, relying solely on manual checks can introduce race conditions, where two threads simultaneously detect that a directory does not exist and attempt to create it. This can lead to conflicts and inconsistent behavior within the program. With “Path mkdir if not exists” functionality, race conditions are avoided entirely, ensuring the integrity and stability of the code.
FAQs:
Q: Does the “Path mkdir if not exists” function create nested directories?
A: Yes, the function can create nested directories. If any of the directories in the path do not exist, the function will create them recursively.
Q: Can I specify custom permissions while creating a directory with this function?
A: Yes, most programming languages that support the “Path mkdir if not exists” function allow you to specify custom permissions for the newly created directory.
Q: Is this function limited to a specific operating system?
A: No, the “Path mkdir if not exists” function is supported across multiple operating systems, including Windows, macOS, and Linux.
Q: What happens if there are permission issues while creating a directory?
A: If there are permission issues, such as insufficient rights to create a directory, the function will typically throw an exception or return an error, depending on the programming language being used.
Q: Can I use this function to create files instead of directories?
A: No, the “Path mkdir if not exists” function is specifically designed for directory creation. To create files, you would need to use other file-specific functions or methods provided by the programming language.
In conclusion, the “Path mkdir if not exists” concept simplifies directory management in programming languages, allowing developers to create directories only if they do not already exist. By combining the check for directory existence and creation into a single method call, potential errors are minimized and code efficiency is enhanced. This functionality is crucial for maintaining the integrity and stability of programs, eliminating race conditions, and ensuring smooth directory management across multiple operating systems.
Images related to the topic python create directory if not exists
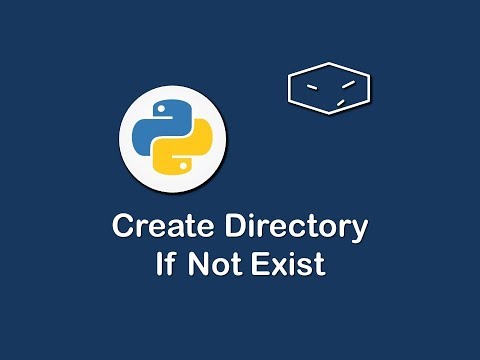
Found 15 images related to python create directory if not exists theme
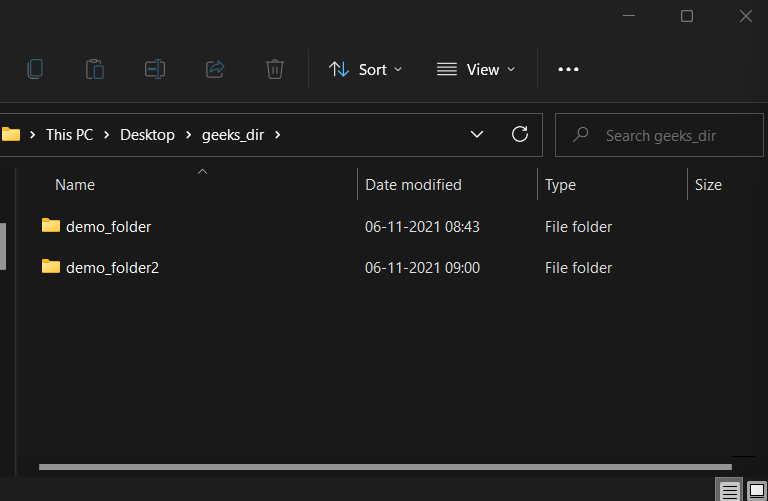
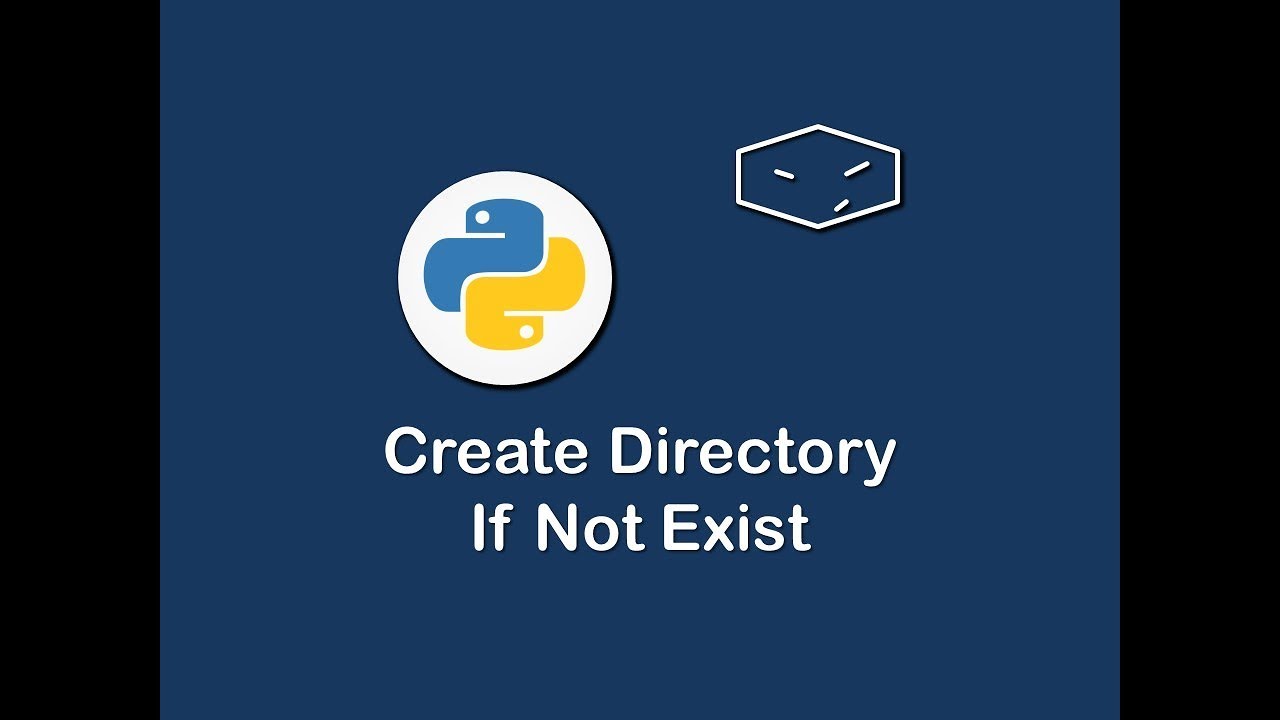
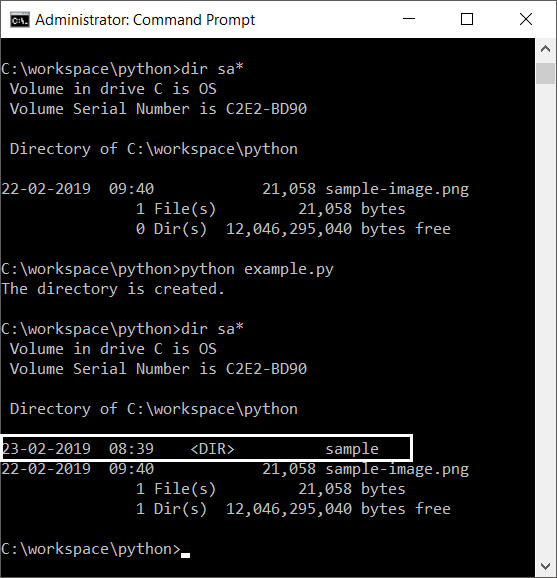

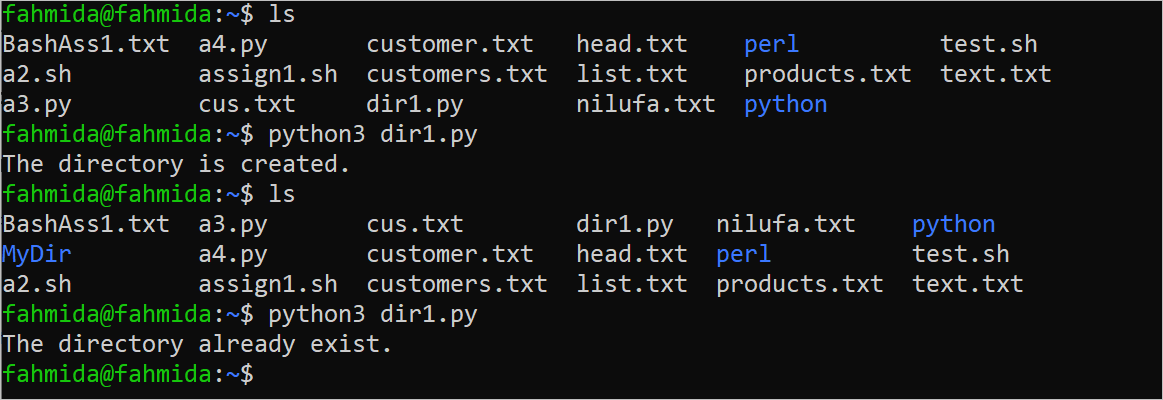
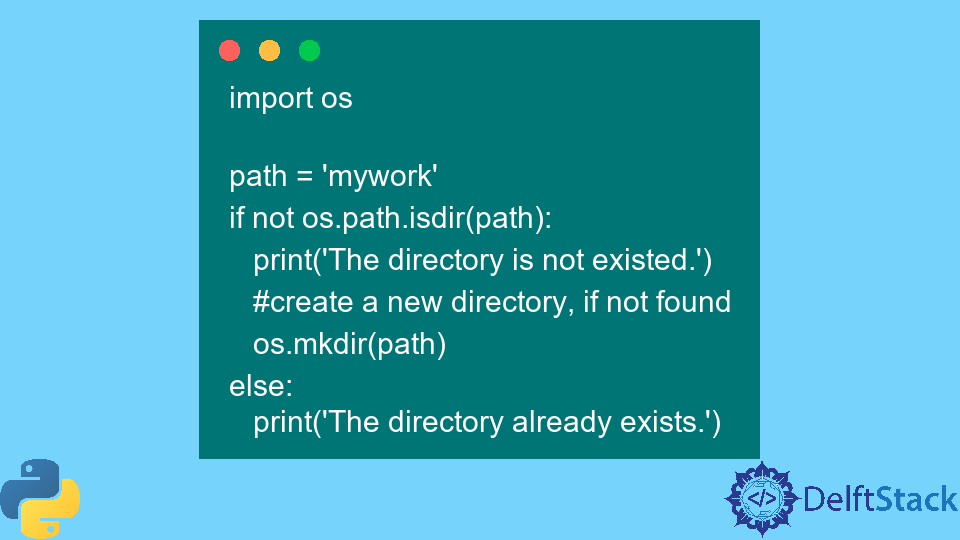
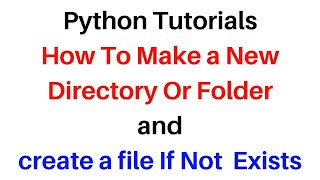
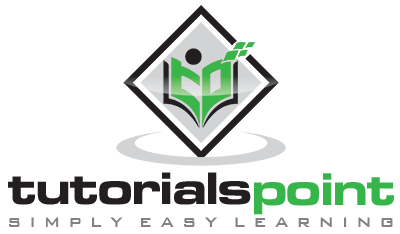
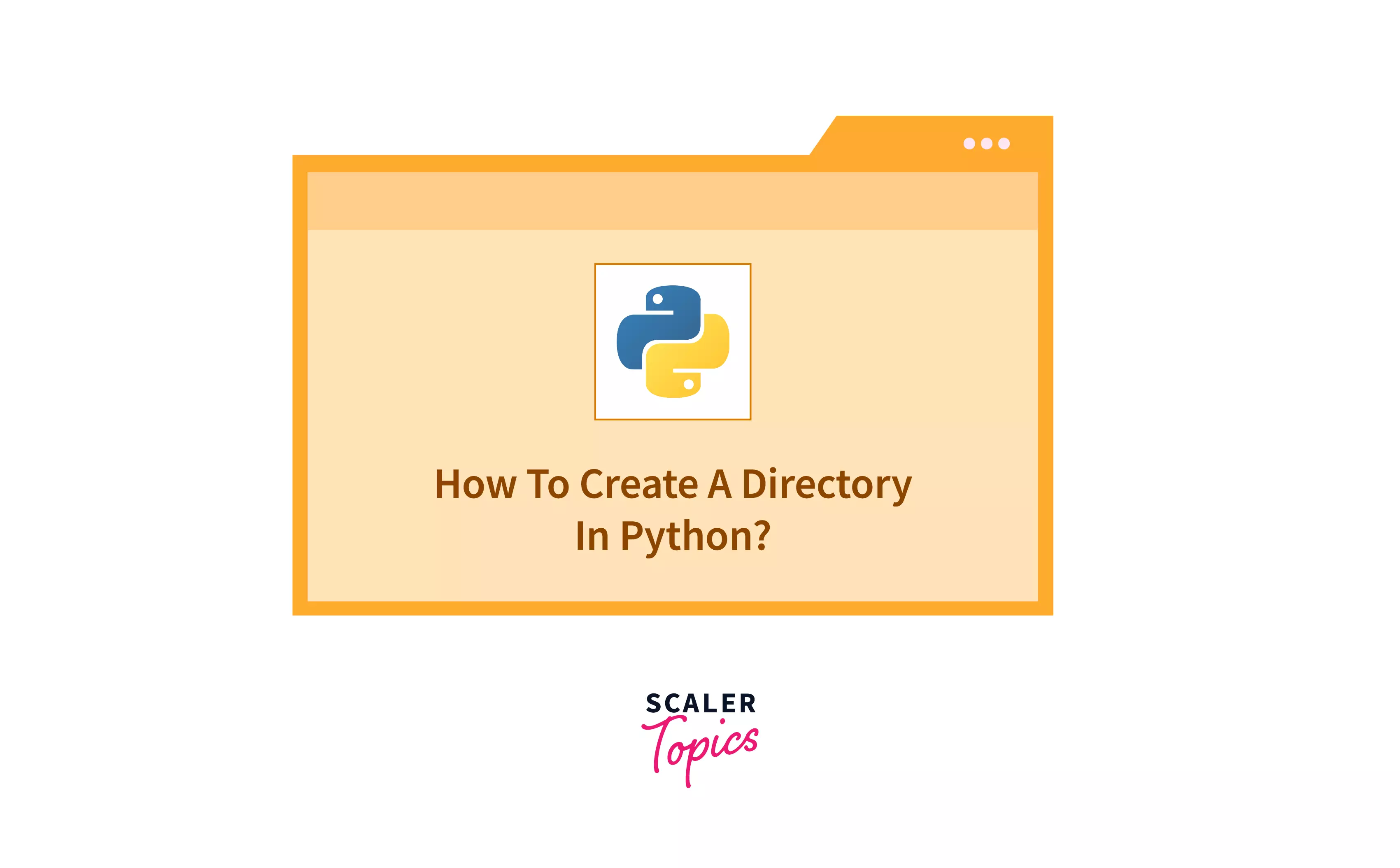
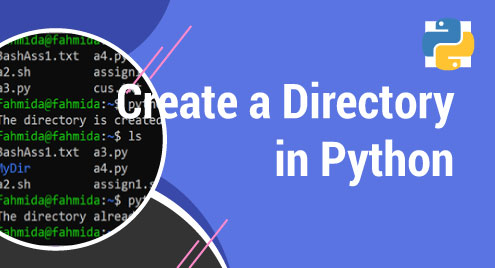
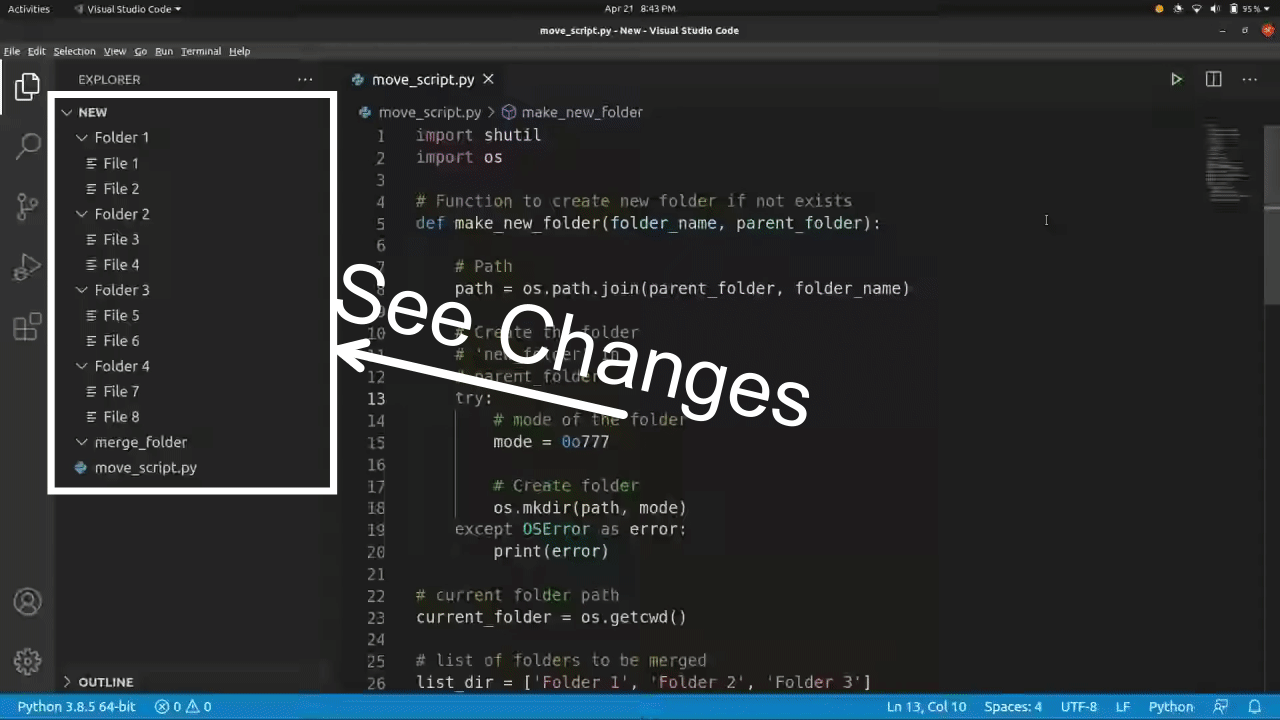
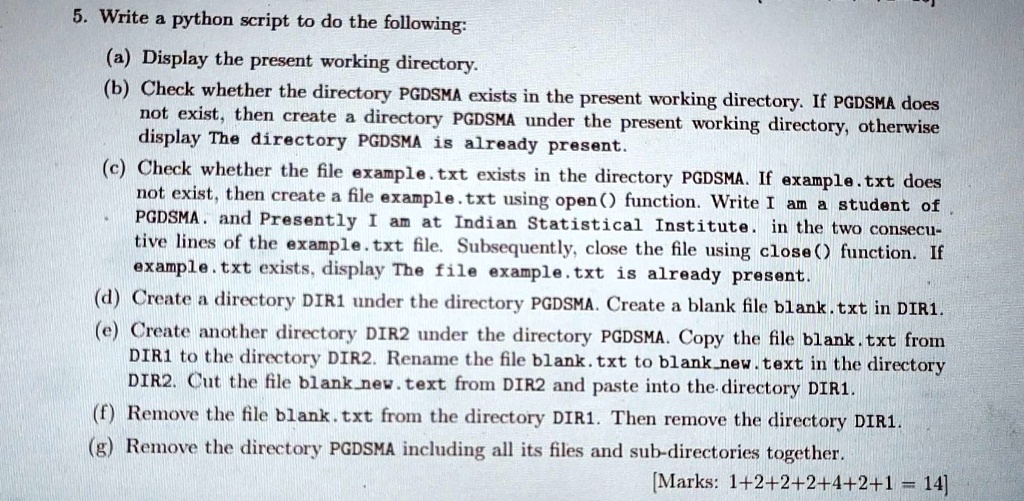
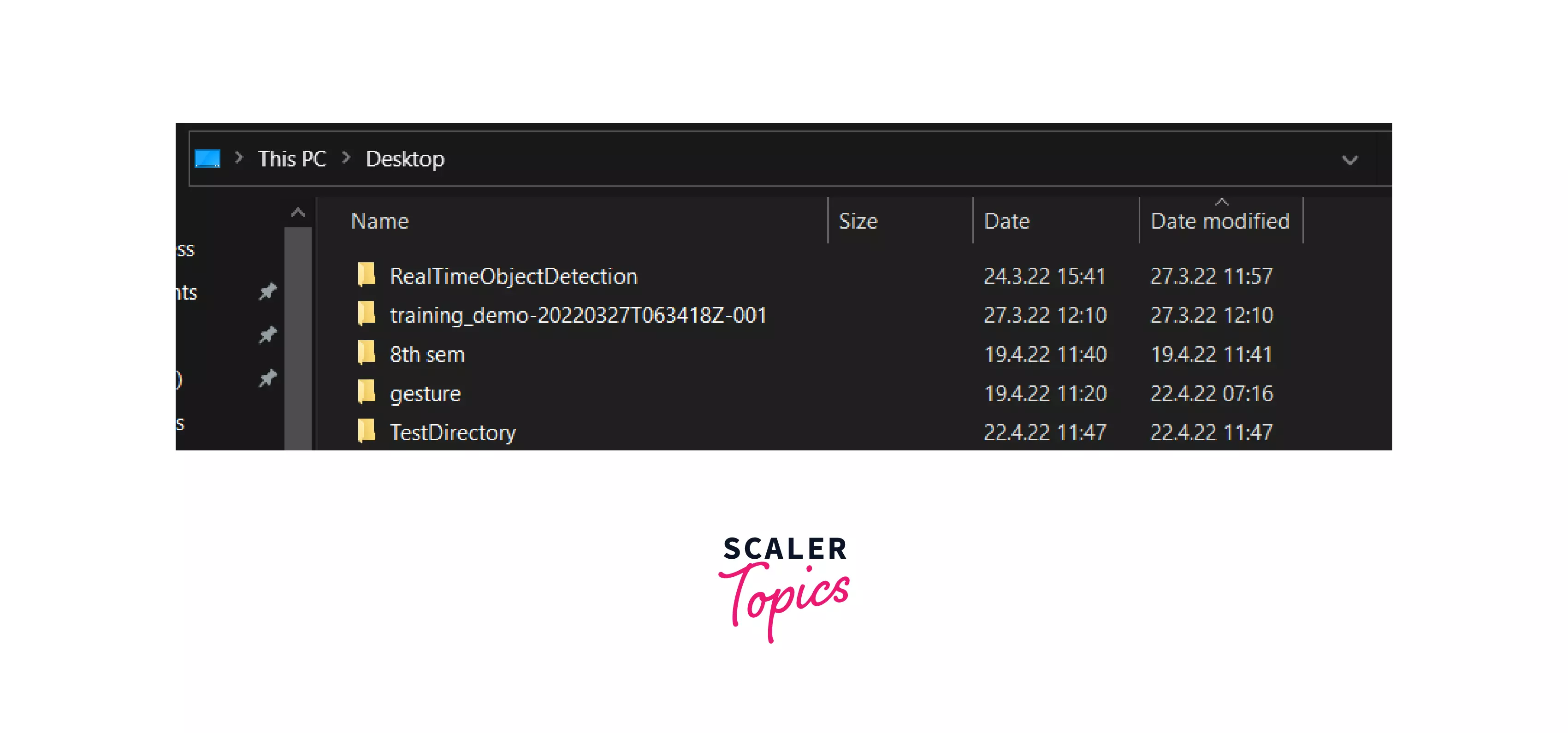
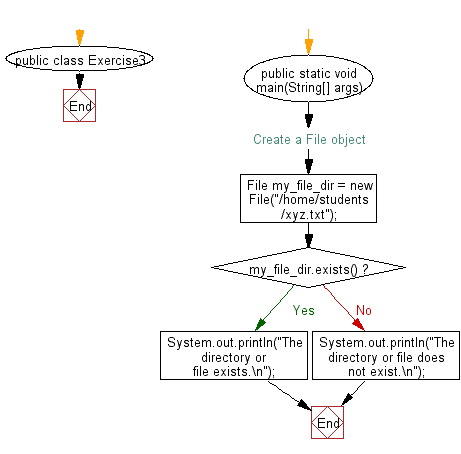
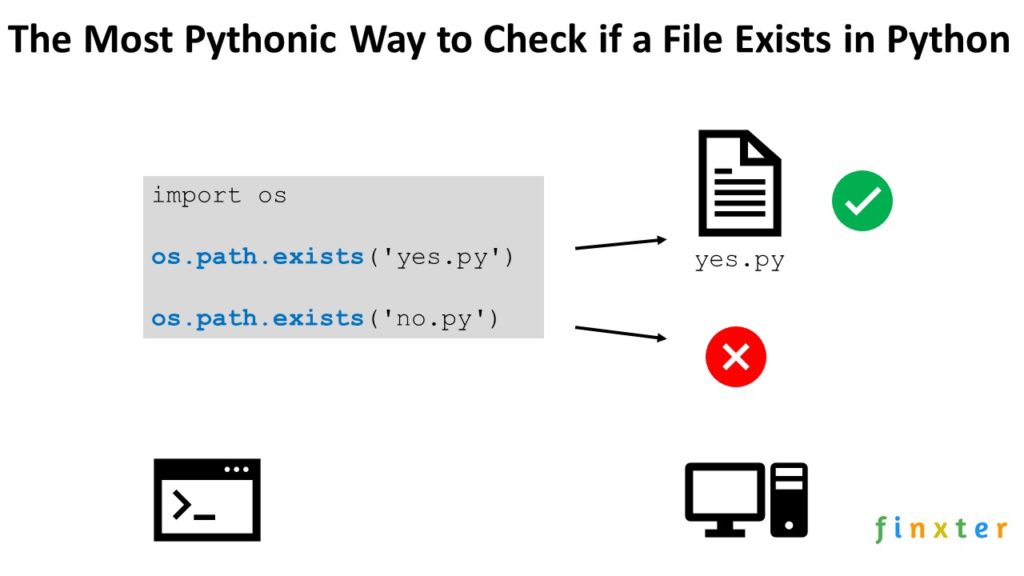
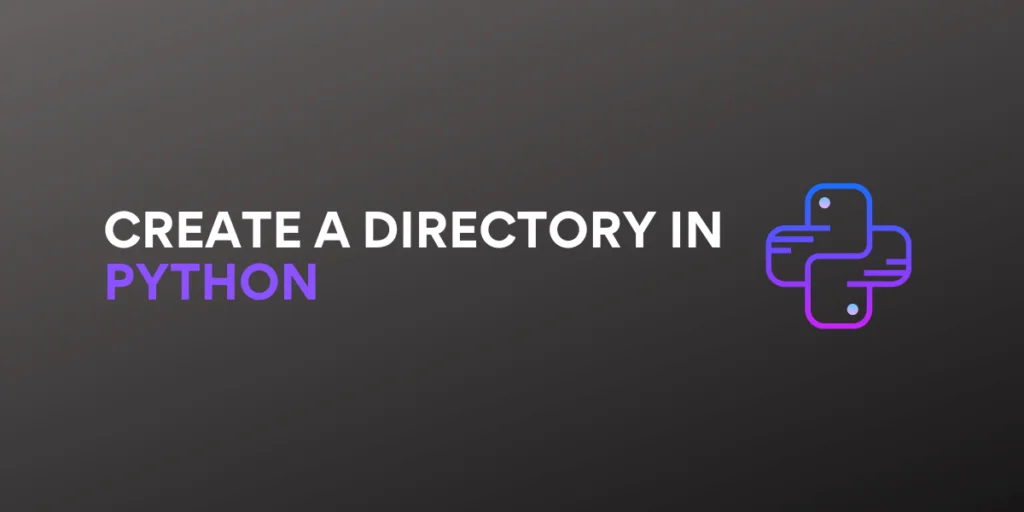
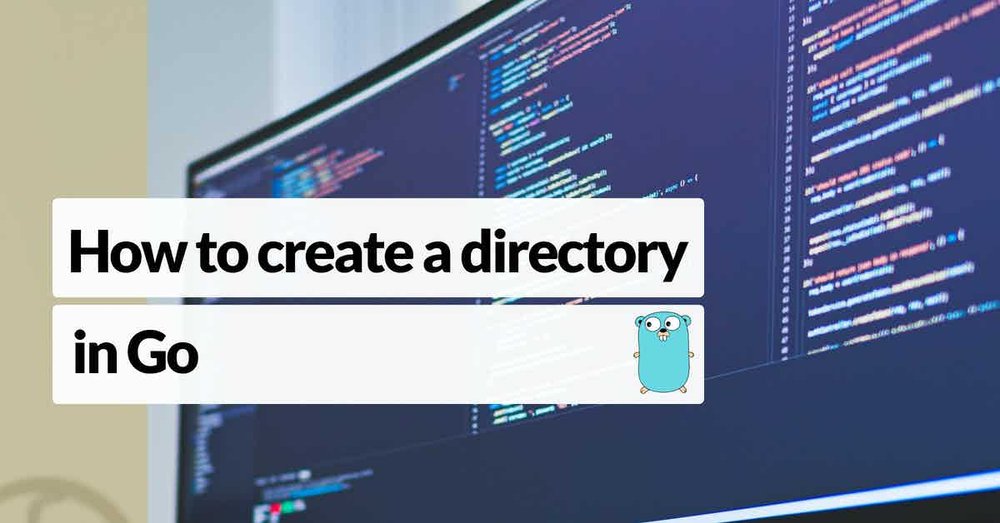
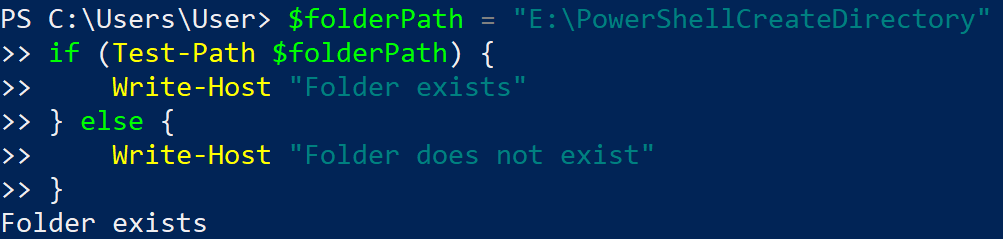
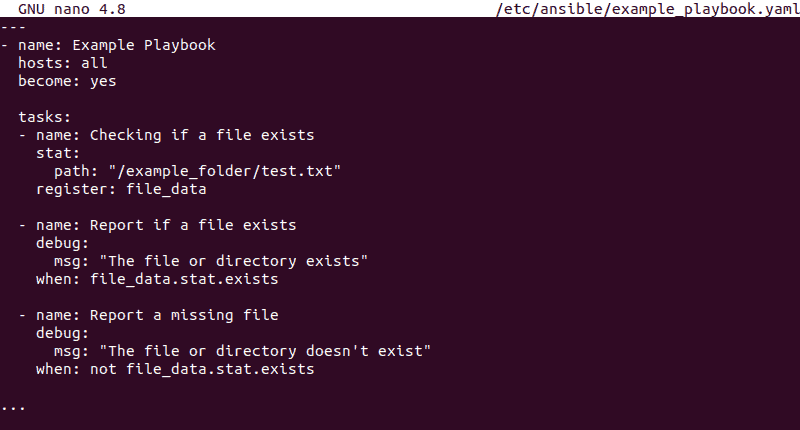
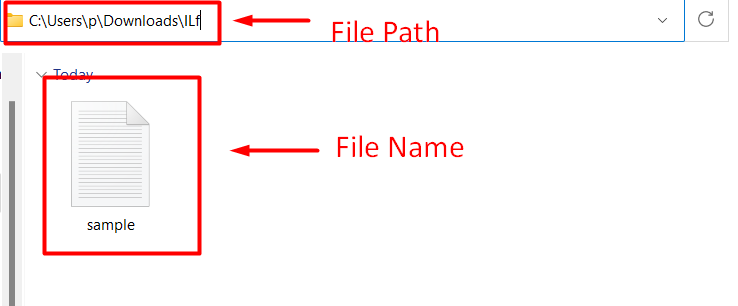
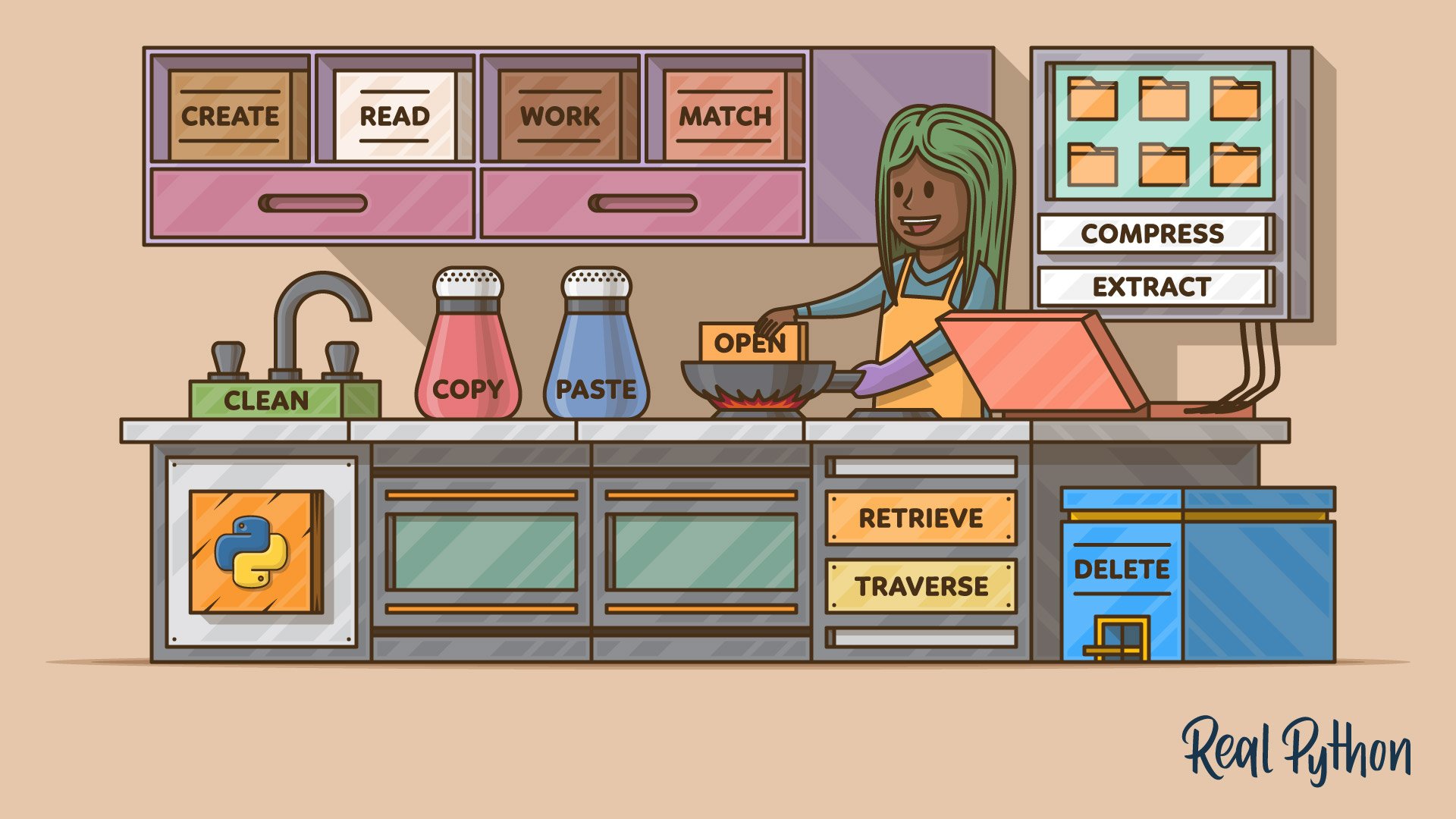
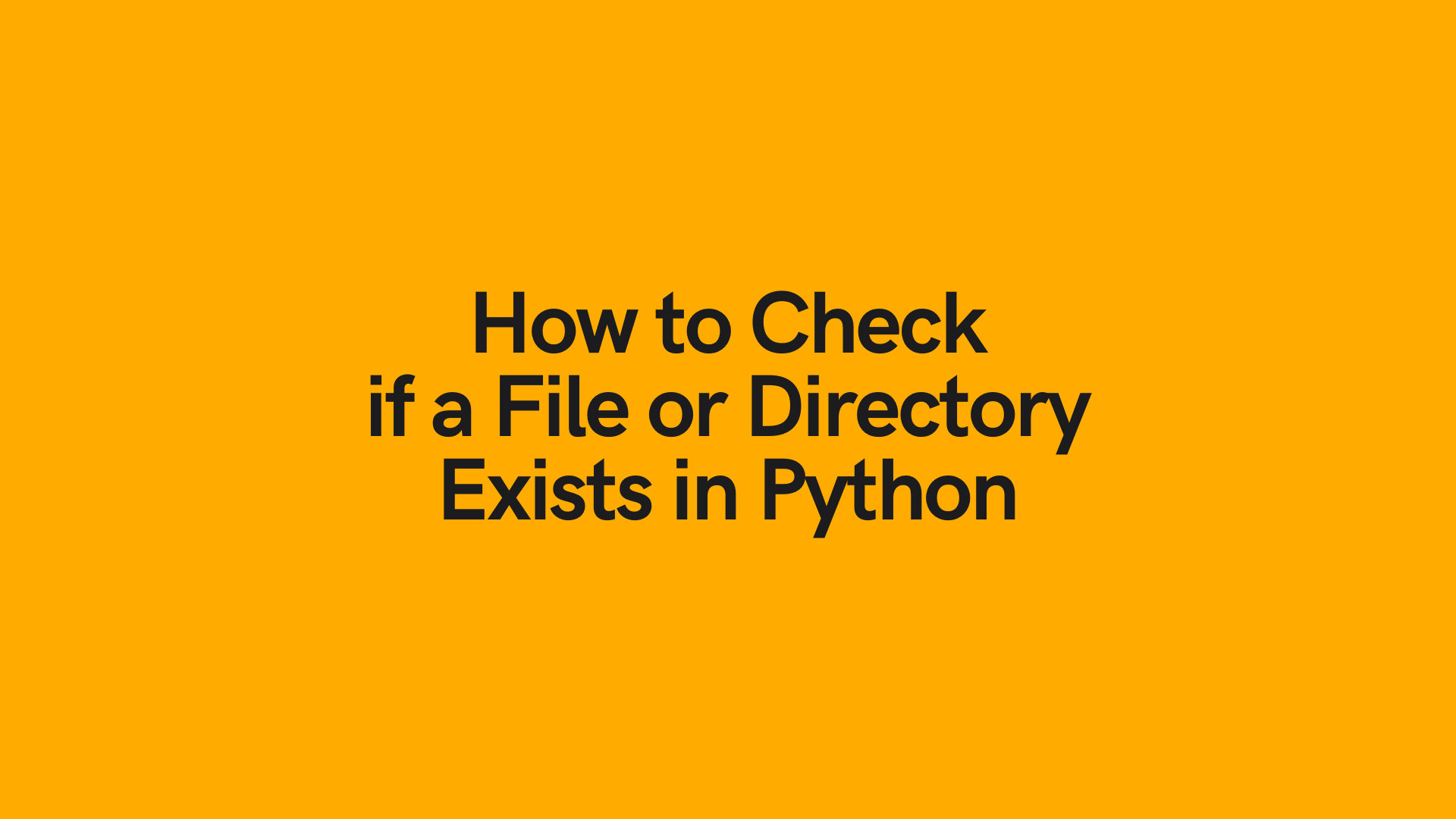
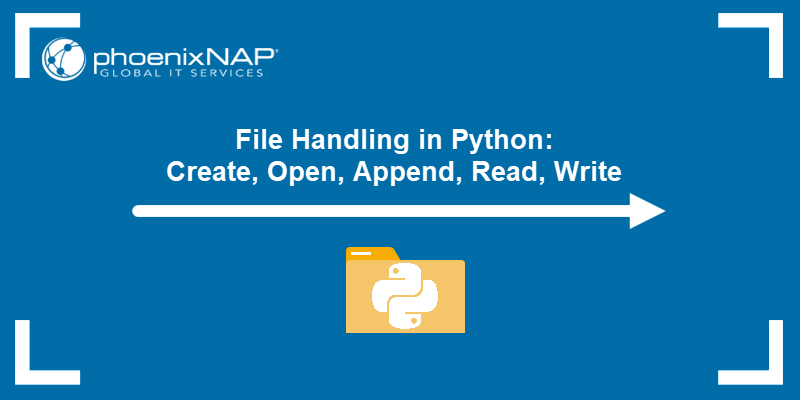
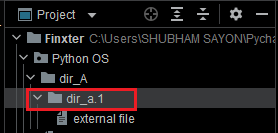
![FileExistsError: [Errno 17] File exists in Python [Solved] | bobbyhadz Fileexistserror: [Errno 17] File Exists In Python [Solved] | Bobbyhadz](https://bobbyhadz.com/images/blog/python-fileexistserror-errno-17-file-exists/banner.webp)
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/all-fs-methods-1.png)
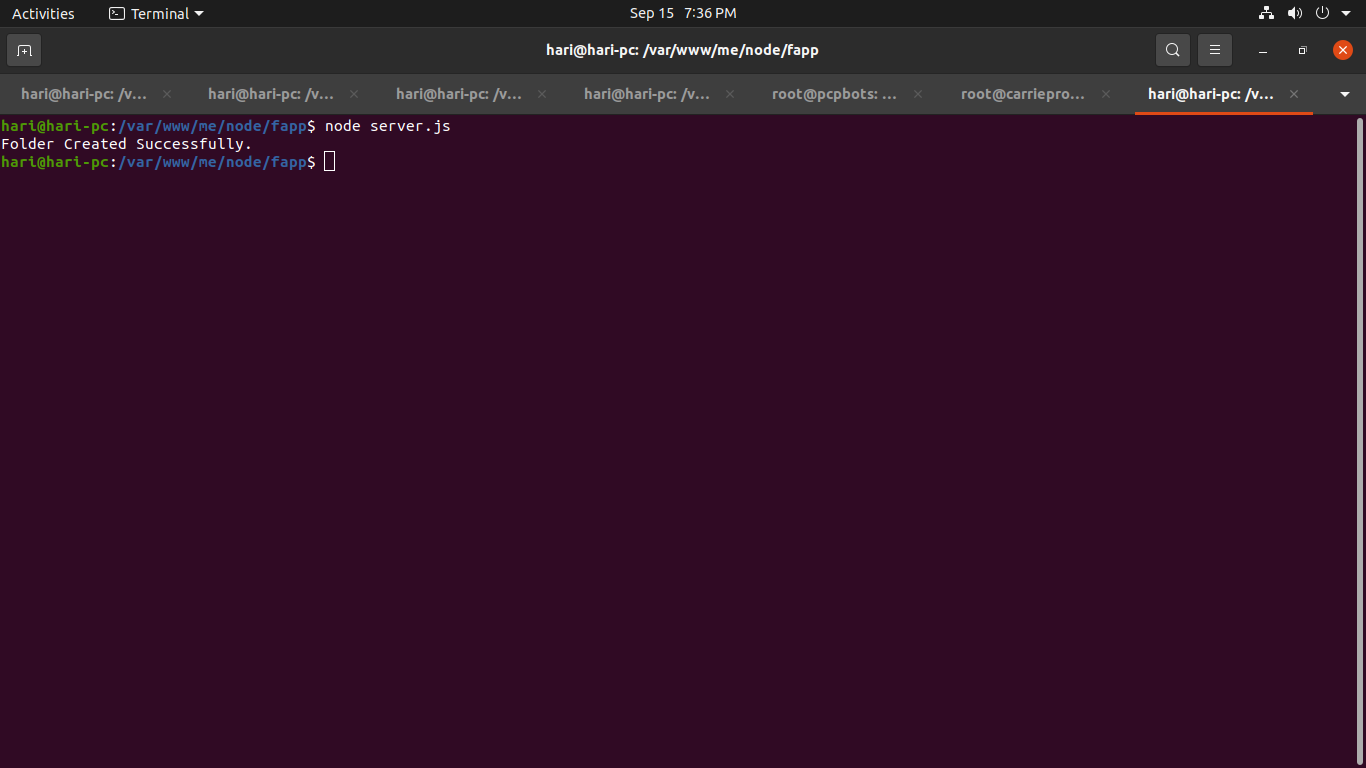
![Create File in Python [4 Ways] – PYnative Create File In Python [4 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/06/created-files.png)
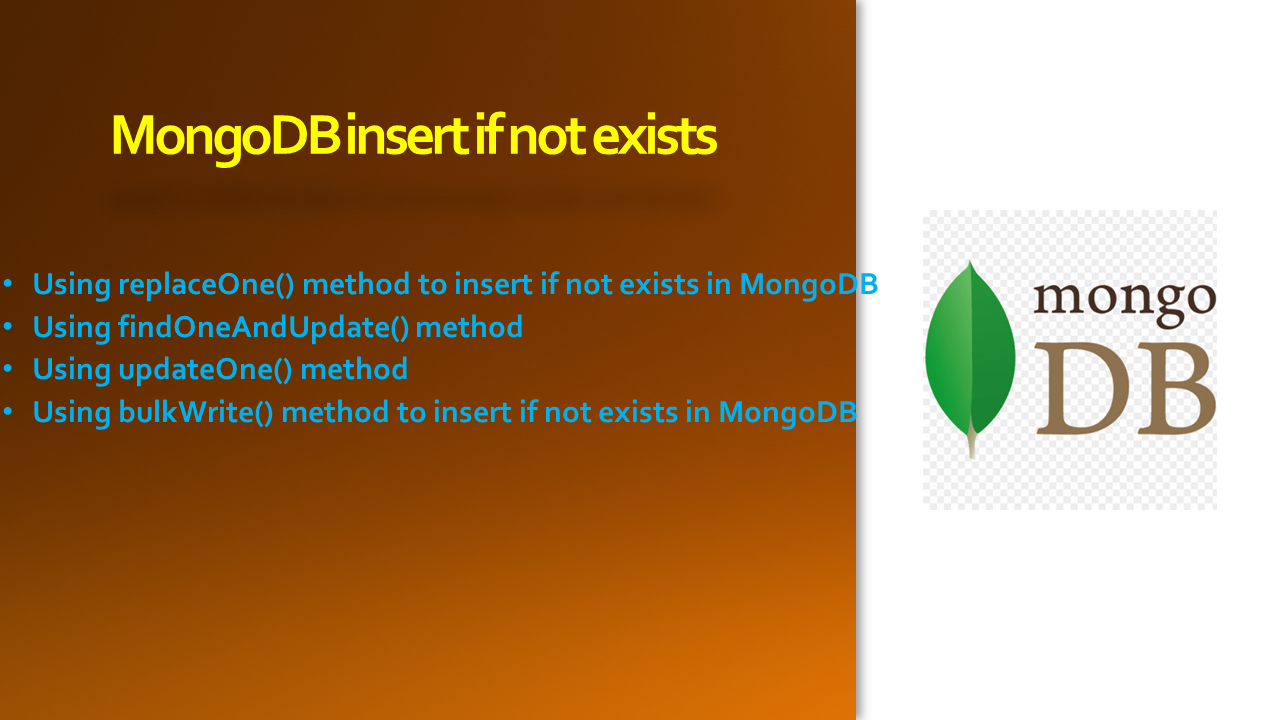
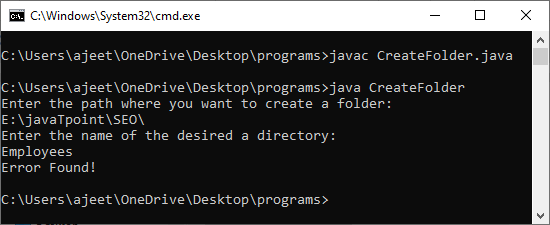

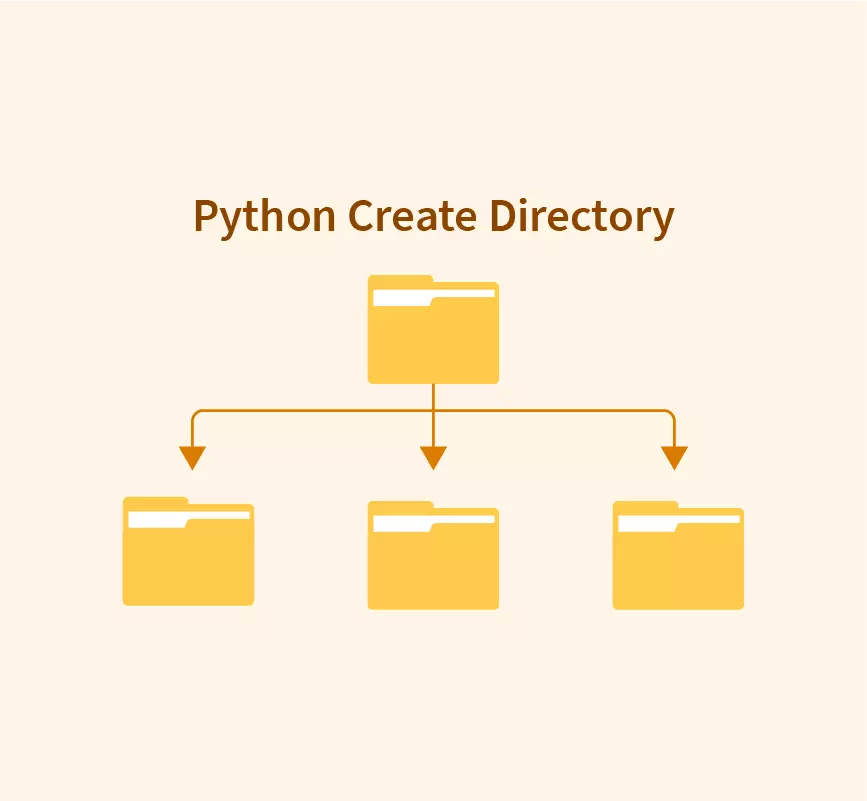
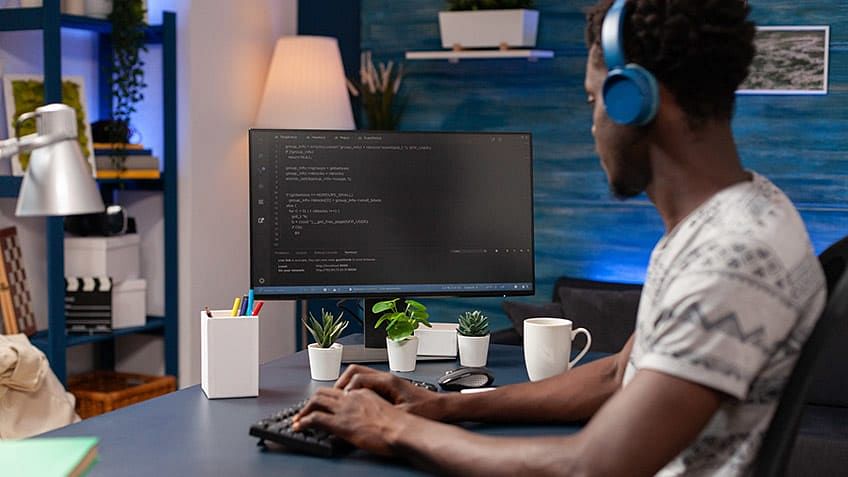
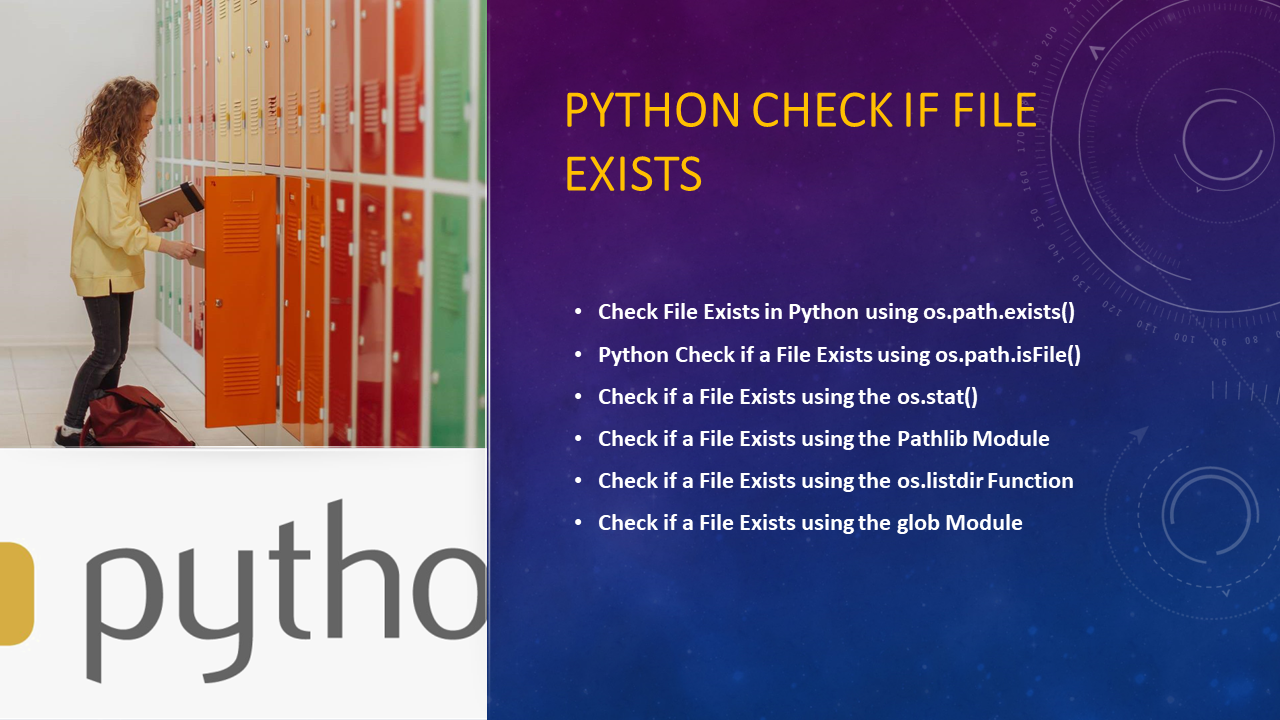
![FileExistsError: [Errno 17] File exists in Python [Solved] | bobbyhadz Fileexistserror: [Errno 17] File Exists In Python [Solved] | Bobbyhadz](https://bobbyhadz.com/images/blog/python-fileexistserror-errno-17-file-exists/directory-already-exists.webp)
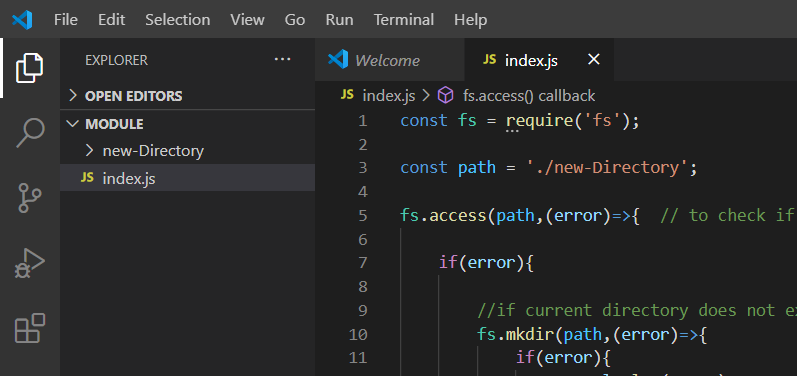

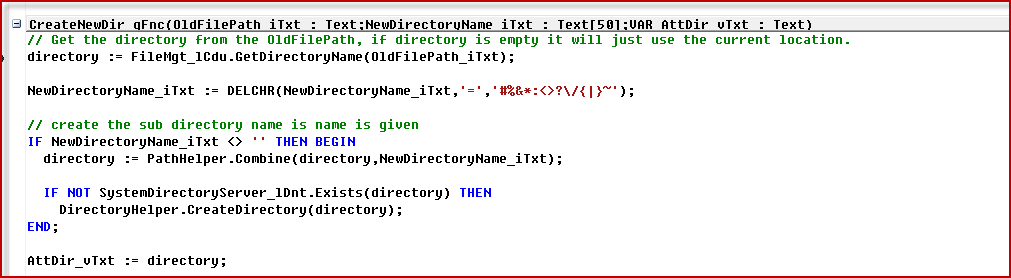
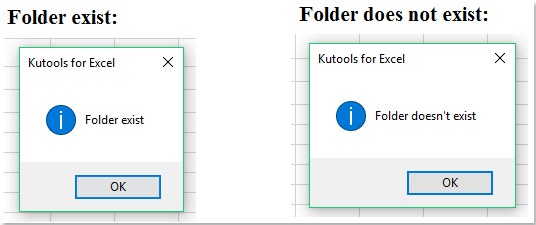
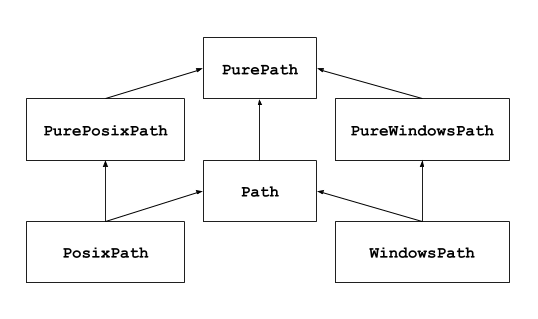
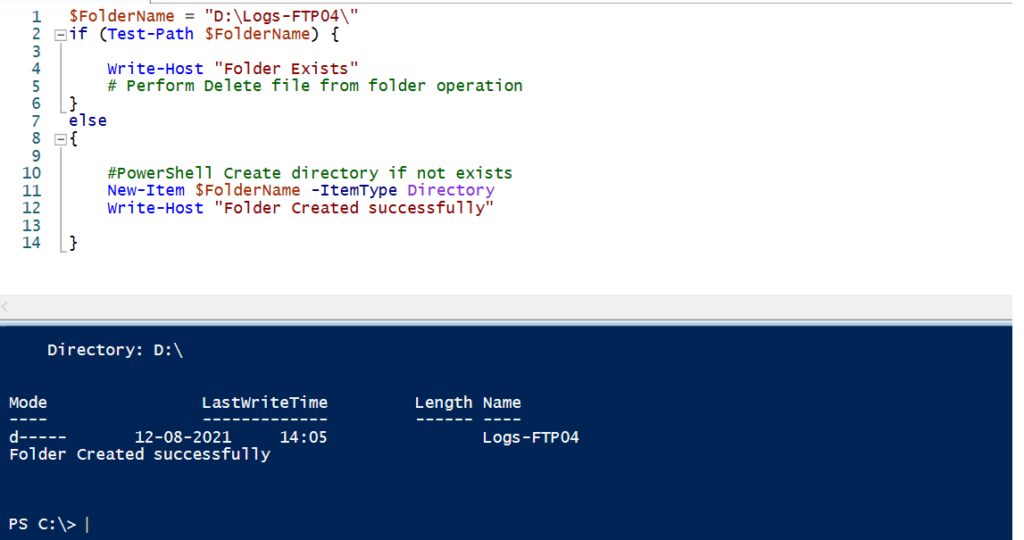
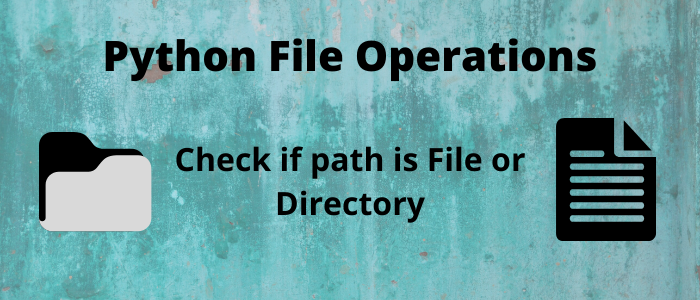
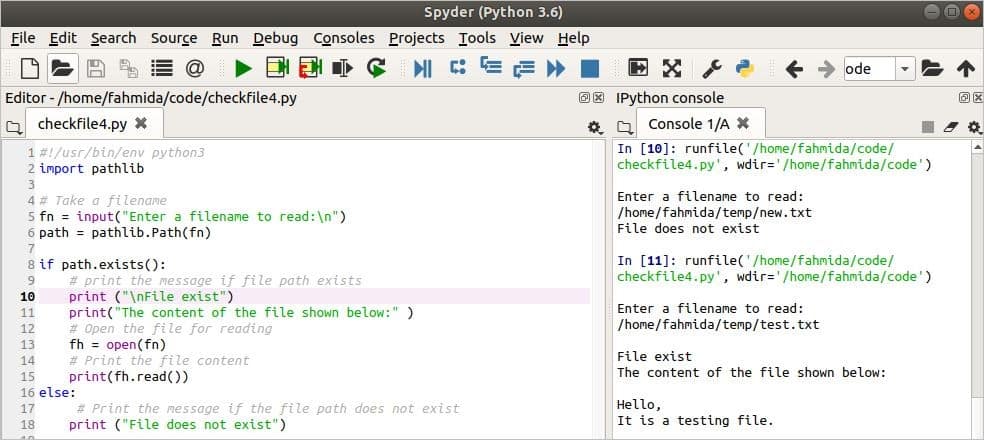
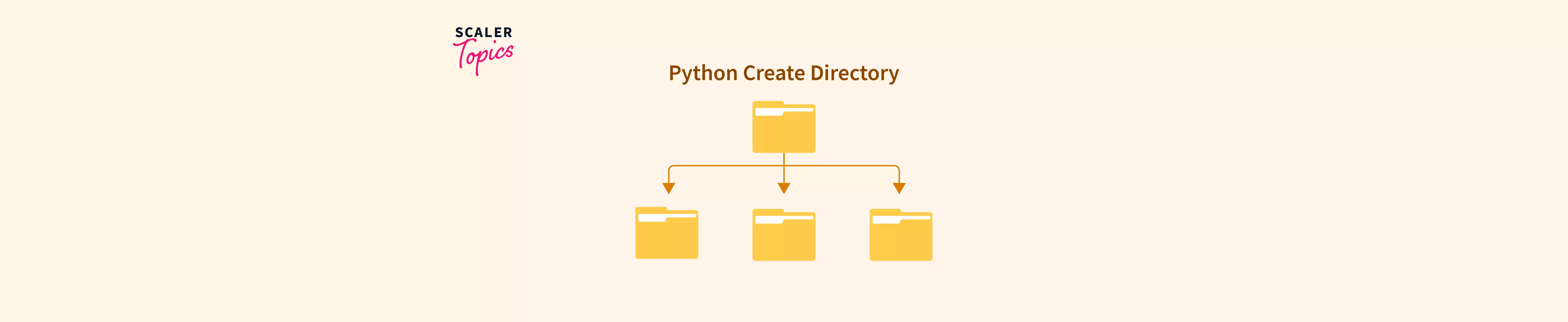

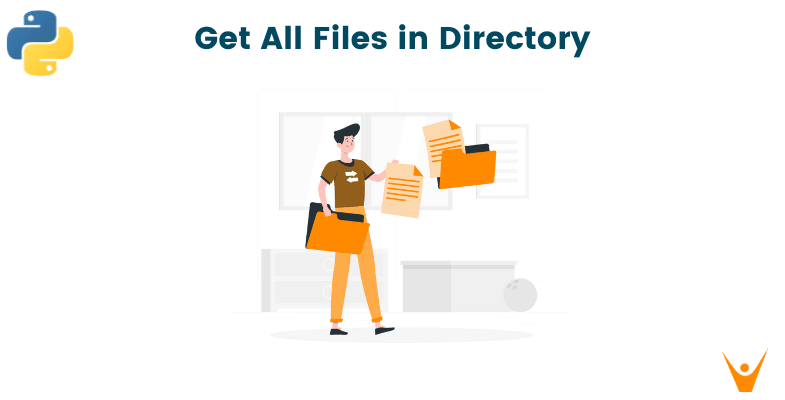

![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/recursive-directory-creation.png)
Article link: python create directory if not exists.
Learn more about the topic python create directory if not exists.
- How can I create a directory if it does not exist using Python
- How to Create Directory If Not Exists in Python – AppDividend
- How can I create a directory in Python using ‘mkdir if not exists’?
- How do I create a directory, and any missing parent directories?
- How to Create Directory If it Does Not Exist using Python?
- Python: Create a Directory if it Doesn’t Exist – Datagy
- How To Create a Directory If Not Exist In Python – pythonpip.com
- Creating a Directory in Python – How to Create a Folder
- How to check if a file or directory exists in Python
- Create a directory with mkdir(), makedirs() in Python – nkmk note
See more: nhanvietluanvan.com/luat-hoc