Python Create Directory If Not Exist
Creating directories is a common task in programming, especially when dealing with file operations. In Python, there are several ways to check if a directory exists and create one if it doesn’t. This article will guide you through the various methods, including using the `os` module, `os.path` module, and the `pathlib` module. We will also provide code examples and address some frequently asked questions about creating directories in Python.
Checking if a Directory Exists in Python
Before creating a directory, it is essential to check if it already exists. There are a few ways to perform this check in Python. Let’s explore each of these methods.
1. Using the `os` module:
The `os` module in Python provides a method called `path.exists(path)` that checks if a given path exists. In our case, we will pass the directory path to this method to determine its existence. Here’s an example:
“`
import os
directory_path = ‘/path/to/directory’
if os.path.exists(directory_path):
print(‘Directory exists’)
else:
print(‘Directory does not exist’)
“`
2. Using the `os.path` module:
The `os.path` module offers more specific options for dealing with paths, including directories. We can use the `os.path.isdir(path)` function to check if a given path is a directory. Here’s an example:
“`
import os.path
directory_path = ‘/path/to/directory’
if os.path.isdir(directory_path):
print(‘Directory exists’)
else:
print(‘Directory does not exist’)
“`
3. Using the `pathlib` module:
Python’s `pathlib` module provides an object-oriented approach for dealing with paths. We can use the `Path` class and the `exists()` method to check if a directory exists. Here’s an example:
“`
from pathlib import Path
directory_path = Path(‘/path/to/directory’)
if directory_path.exists():
print(‘Directory exists’)
else:
print(‘Directory does not exist’)
“`
Creating a Directory if it Doesn’t Exist using the `os` module
Once we have checked whether a directory exists or not, we can take the necessary steps to create it if it doesn’t. The `os` module provides the `mkdir(path)` function to create a directory at the specified path. Here’s an example of creating a directory using the `os` module:
“`
import os
directory_path = ‘/path/to/new/directory’
if not os.path.exists(directory_path):
os.mkdir(directory_path)
print(‘Directory created successfully’)
else:
print(‘Directory already exists’)
“`
Creating a Directory if it Doesn’t Exist using the `pathlib` module
Similarly, the `pathlib` module offers a convenient method to create a directory if it doesn’t already exist. We can use the `mkdir()` method of the `Path` class. Here’s an example:
“`
from pathlib import Path
directory_path = Path(‘/path/to/new/directory’)
if not directory_path.exists():
directory_path.mkdir()
print(‘Directory created successfully’)
else:
print(‘Directory already exists’)
“`
FAQs (Frequently Asked Questions)
Q1. How can I create a file if it doesn’t exist in Python?
To create a file if it doesn’t exist, you can use the `open()` function with the `x` mode specifier. For example:
“`
file_path = ‘/path/to/new/file.txt’
try:
f = open(file_path, ‘x’)
print(‘File created successfully’)
except FileExistsError:
print(‘File already exists’)
“`
Q2. Can I create a folder while opening a file if it doesn’t exist?
Yes, you can create a folder while opening a file if it doesn’t exist by specifying the folder path in the file path. For example:
“`
file_path = ‘/path/to/new/directory/new_file.txt’
try:
f = open(file_path, ‘x’)
print(‘File created successfully’)
except FileExistsError:
print(‘File already exists’)
“`
Q3. How can I copy a file and automatically create the necessary directories if they don’t exist?
You can use the `shutil` module to copy files and create directories if they don’t exist. The `copy2()` function handles both file copying and directory creation. Here’s an example:
“`
import shutil
source_file = ‘/path/to/source/file.txt’
destination_file = ‘/path/to/destination/file.txt’
shutil.copy2(source_file, destination_file)
print(‘File copied successfully’)
“`
Q4. Is it possible to create a directory and its parent directories if they don’t exist?
Yes, you can use the `os.makedirs(path)` function to create a directory and its parent directories if they don’t exist. For example:
“`
import os
directory_path = ‘/path/to/new/directory’
os.makedirs(directory_path, exist_ok=True)
print(‘Directory created successfully’)
“`
In conclusion, creating directories if they don’t exist is a common task in Python programming. By using the methods provided by the `os` module, `os.path` module, and the `pathlib` module, you can easily check for directory existence and create directories when necessary. Remember to handle exceptions and consider the functionalities offered by other modules, such as `shutil`.
Create Directory If Not Exist In Python 😀
Keywords searched by users: python create directory if not exist Os mkdir if not exists, Python create file if not exists, Python open file create folder if not exist, Path mkdir if not exists, Python copy file auto create directory if not exist, Python check directory exists if not create, Os mkdir exist ok, Create folder if it doesn t exist Python
Categories: Top 49 Python Create Directory If Not Exist
See more here: nhanvietluanvan.com
Os Mkdir If Not Exists
In the realm of computer programming, the creation of directories or folders is an essential task. However, there may be instances where we want to create a directory only if it does not already exist. To cater to this requirement, the programming language Python provides the `os.mkdir()` function with the option to check for the existence of a directory before creating it. In this article, we will dive into the world of `os.mkdir()` if not exists, exploring its functionality, implementation, and addressing common questions and concerns.
Understanding `os.mkdir()`
Before we discuss the specifics of `os.mkdir()` if not exists, let’s first gain a comprehensive understanding of the `os.mkdir()` function itself. The `os.mkdir()` function in Python is used to create a new directory. Its basic syntax is as follows:
“`
os.mkdir(path, mode)
“`
Here, `path` represents the path or name of the directory to be created, while `mode` determines the permissions assigned to the new directory. However, if we attempt to use `os.mkdir()` to create a directory that already exists, an error will be raised, obstructing the program’s execution.
The Need for `os.mkdir()` If Not Exists
To overcome the challenge of erroneously attempting to create a directory that already exists, the `if not exists` condition is employed. By implementing this condition, we can check whether a directory exists before invoking `os.mkdir()`, thereby preventing any errors from arising.
Implementation of `os.mkdir()` If Not Exists
To use `os.mkdir()` with the `if not exists` condition, a typical implementation follows these steps:
1. Import the `os` module:
“`python
import os
“`
2. Define the path for the directory to be created:
“`python
path = “/path/to/new_directory”
“`
3. Check if the directory already exists using the `os.path.isdir()` function. If it does not exist, create the directory using `os.mkdir()`:
“`python
if not os.path.isdir(path):
os.mkdir(path)
“`
4. Alternatively, to create intermediate directories (if required) while using `os.mkdir()`, the `os.makedirs()` function can be used:
“`python
if not os.path.isdir(path):
os.makedirs(path)
“`
By employing this implementation, we can dynamically create directories only when they do not already exist, significantly improving the efficiency and reliability of our programs.
FAQs
Q1: What happens if I use `os.mkdir()` on an existing directory without checking whether it exists?
If you attempt to create a directory that already exists without checking its existence, your program will raise an error. This error, commonly known as a “FileExistsError,” halts the program’s execution and may cause unintended consequences if not handled properly.
Q2: Can I specify the permissions while using `os.mkdir()` with the `if not exists` condition?
Yes, the `mode` parameter of the `os.mkdir()` function allows you to set the permissions of the new directory. By default, the permissions are set according to the system’s umask.
Q3: How does the `os.makedirs()` function differ from `os.mkdir()`?
The `os.makedirs()` function is used to create directories recursively, even if intermediate directories do not exist. On the other hand, `os.mkdir()` can only create a single directory and throws an error if the path contains non-existent intermediate directories.
Q4: How can I ensure platform independence while using `os.mkdir()` if not exists?
To ensure platform independence, the `os.path` module provides several functions, such as `os.path.sep` to retrieve the path separator specific to the operating system, and `os.path.join()` to join path components using the appropriate separator.
Q5: Can I delete a directory if it already exists using `os.rmdir()` with the `if not exists` condition?
No, `os.rmdir()` cannot be used for this purpose. `os.rmdir()` is specifically designed to remove an existing and empty directory, but it will raise an error if the directory does not exist.
Conclusion
The `os.mkdir()` if not exists feature in Python empowers programmers to create directories dynamically, without encountering errors when attempting to create directories that already exist. By incorporating this functionality into your programs, you ensure smoother execution and avoid unexpected interruptions. The ability to check for the existence of a directory before creation provides greater control, flexibility, and efficiency in directory management.
Python Create File If Not Exists
Python is a versatile programming language that offers numerous functionalities for file handling. When working with files in Python, it is often required to create a file if it does not already exist. In this article, we will explore different approaches to achieve this and provide insights into the related concepts.
Creating a file in Python is a straightforward process, but before doing so, it is crucial to understand the concept of file existence. Python provides various methods and modules that can be utilized to determine whether a file exists or not.
One of the most common approaches to create a file if it does not exist is by using the `os` module. The `os.path` module provides functions for file-related operations and includes a convenient method, `os.path.exists(path)`, which allows checking whether a file exists. By utilizing this method, we can easily determine if a file exists before creating it.
Here is an example that demonstrates how to use the `os.path.exists()` method to check for file existence and create the file if necessary:
“`python
import os
filename = “example.txt”
if not os.path.exists(filename):
open(filename, ‘w’).close()
“`
In the above example, we check if the file named “example.txt” exists using the `os.path.exists()` method. If the file does not exist, a new file with the specified filename is created using the `open()` function and immediately closed using the `close()` method. This ensures that an empty file is created.
Another way to create a file if it does not exist is by using the `Path` module provided by the `pathlib` library. `Path` provides a high-level interface for file path manipulation and operation. By invoking the `Path.touch()` method, we can create a file if it doesn’t already exist.
Here’s an example illustrating the usage of `Path.touch()` method:
“`python
from pathlib import Path
filename = Path(“example.txt”)
if not filename.exists():
filename.touch()
“`
In this case, we create a `Path` object with the desired filename. The existence of the file is checked using `filename.exists()`. If the file doesn’t exist, we create it using `filename.touch()`. The `Path.touch()` method creates an empty file by default.
Now, let’s address some frequently asked questions related to creating files if they don’t exist in Python.
## FAQs
**Q: How do I specify the file path while creating a file if it doesn’t exist?**
A: When using the `open()` function, you can specify the file path and filename within the parentheses, for example: `open(“/path/to/file/example.txt”, ‘w’)`.
**Q: How can I handle errors if the file creation fails?**
A: In Python, you can make use of exception handling to catch any errors that may occur during file creation. Enclosing the file creation code within a `try-except` block allows you to handle the exceptions gracefully and take appropriate actions.
**Q: Is it possible to create a file with specific content if it doesn’t exist?**
A: Yes, it is possible using the `open()` function with the ‘w’ mode. After creating the file, you can write content to it by using the `write()` method. However, please note that this approach will overwrite any existing content if the file already exists.
**Q: How can I create directories along with the file if they don’t exist?**
A: Python provides the `os.makedirs()` function, which allows you to create directories recursively. You can utilize this function to create directories along with the file if they do not already exist.
**Q: Are there any other libraries for file handling in Python?**
A: Yes, Python provides several other libraries for more advanced file handling operations. Some popular ones include `shutil`, `glob`, and `os.path`. These libraries offer additional functionality for copying, moving, searching, and manipulating files and directories.
In conclusion, creating a file if it does not exist in Python is a common task when working with file handling. By leveraging modules like `os` or `pathlib`, developers can easily check for file existence and create files on demand. Furthermore, exception handling can be employed to ensure error-free file creation. Understanding these concepts provides a solid foundation for working with files in Python efficiently.
Python Open File Create Folder If Not Exist
## Opening a File in Python
Before we dive into the folder creation aspect, let’s first understand how to open a file in Python. The `open()` function is used for this purpose, which takes in the file path and a mode as parameters. The mode can be “r” for reading, “w” for writing, “a” for appending, or “x” for exclusive creation.
To open a file in read mode and print its contents, you can use the following code snippet:
“`python
file_path = ‘path/to/your/file.txt’
file = open(file_path, ‘r’)
content = file.read()
print(content)
file.close()
“`
Here, we open the file located at `file_path` in read mode (‘r’) using the `open()` function. Then, we read the content using the `read()` method and store it in the `content` variable. Finally, we print the contents and close the file using the `close()` method. Closing the file is essential to free up system resources.
## Creating a Folder in Python
To create a new folder/directory in Python, you can use the `os` module, which provides a range of useful functions for working with the operating system. Specifically, we will utilize the `os.makedirs()` function, which creates nested directories recursively. This function takes the directory path as a parameter and automatically creates any missing directories along that path.
Here’s an example that demonstrates how to create a folder:
“`python
import os
folder_path = ‘path/to/your/folder’
if not os.path.exists(folder_path):
os.makedirs(folder_path)
print(f”Successfully created folder at: {folder_path}”)
else:
print(f”Folder already exists at: {folder_path}”)
“`
In this example, we first import the `os` module. Then, we set the desired folder path in the `folder_path` variable. The `os.path.exists()` function is used to check if the folder already exists. If it doesn’t, we create the folder using `os.makedirs()` and print a success message. If the folder already exists, we simply print a message stating that it is already present.
## Combining File Opening and Folder Creation
Now that we understand how to open files and create folders individually, let’s bring them together by implementing a solution that creates a folder if it doesn’t already exist and opens the file.
“`python
import os
folder_path = ‘path/to/your/folder’
file_name = ‘file.txt’
if not os.path.exists(folder_path):
os.makedirs(folder_path)
print(f”Successfully created folder at: {folder_path}”)
file_path = os.path.join(folder_path, file_name)
file = open(file_path, ‘w’)
file.write(“Hello, World!”)
file.close()
“`
In this example, we first create the folder using the same code we discussed earlier. Then, we concatenate the `folder_path` and `file_name` variables using the `os.path.join()` function to create the complete file path. After that, we open the file in write mode (‘w’), write a simple string to it, and close the file as before.
## FAQs
**Q: What happens if the folder creation fails?**\
A: If the folder creation fails due to insufficient permissions or any other reason, Python will raise an exception. You can handle this exception using a try-except block to provide a fallback or display an appropriate error message.
**Q: What are the different modes used when opening a file?**\
A: There are various modes available when opening a file in Python:
– “r”: Read mode, which allows reading from a file.
– “w”: Write mode, which allows writing to a file. Creates a new file if it doesn’t exist or truncates an existing file to zero length.
– “a”: Append mode, which allows writing to a file. However, it appends the content at the end of the file, preserving its existing content.
– “x”: Exclusive creation mode, which creates a new file but raises an error if the file already exists.
**Q: Is it necessary to close the file after opening it?**\
A: Yes, it is considered good practice to close the file after you have finished working with it. This helps free up system resources and prevents any potential issues that might arise from keeping the file open for an extended period.
**Q: Can I create nested folders using the `os.makedirs()` function?**\
A: Yes, the `os.makedirs()` function can create nested folders by automatically creating any missing directories along the provided path. This makes it easy to create complex folder structures within a single function call.
In conclusion, Python provides us with convenient methods and modules to handle file operations, including creating folders if they do not already exist. By utilizing functions such as `open()` and `os.makedirs()`, we can efficiently open files and ensure the existence of folders as needed. Understanding these concepts is crucial for successfully working with files and directories in Python.
Images related to the topic python create directory if not exist
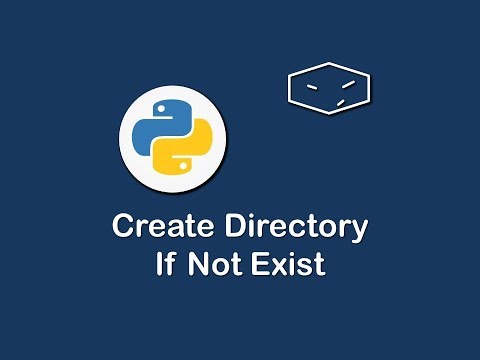
Found 39 images related to python create directory if not exist theme
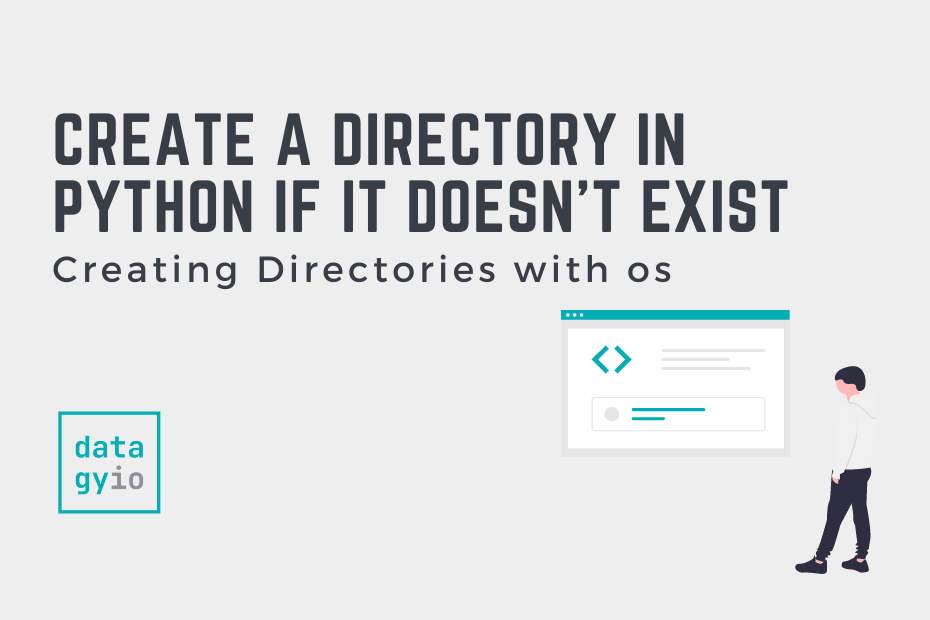
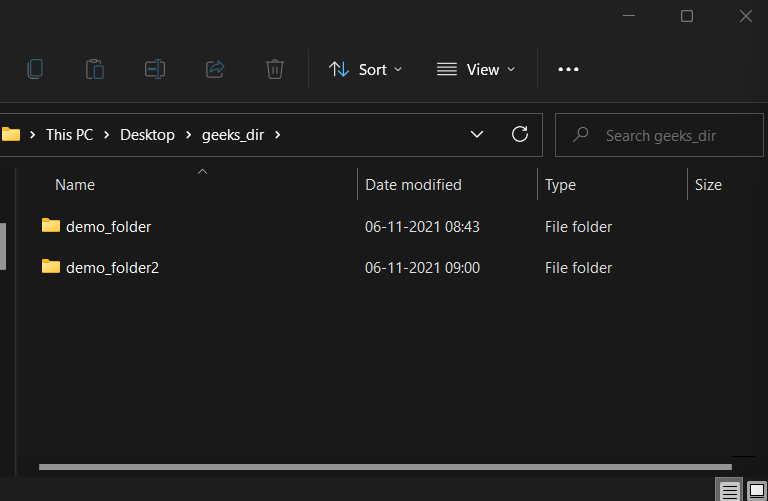
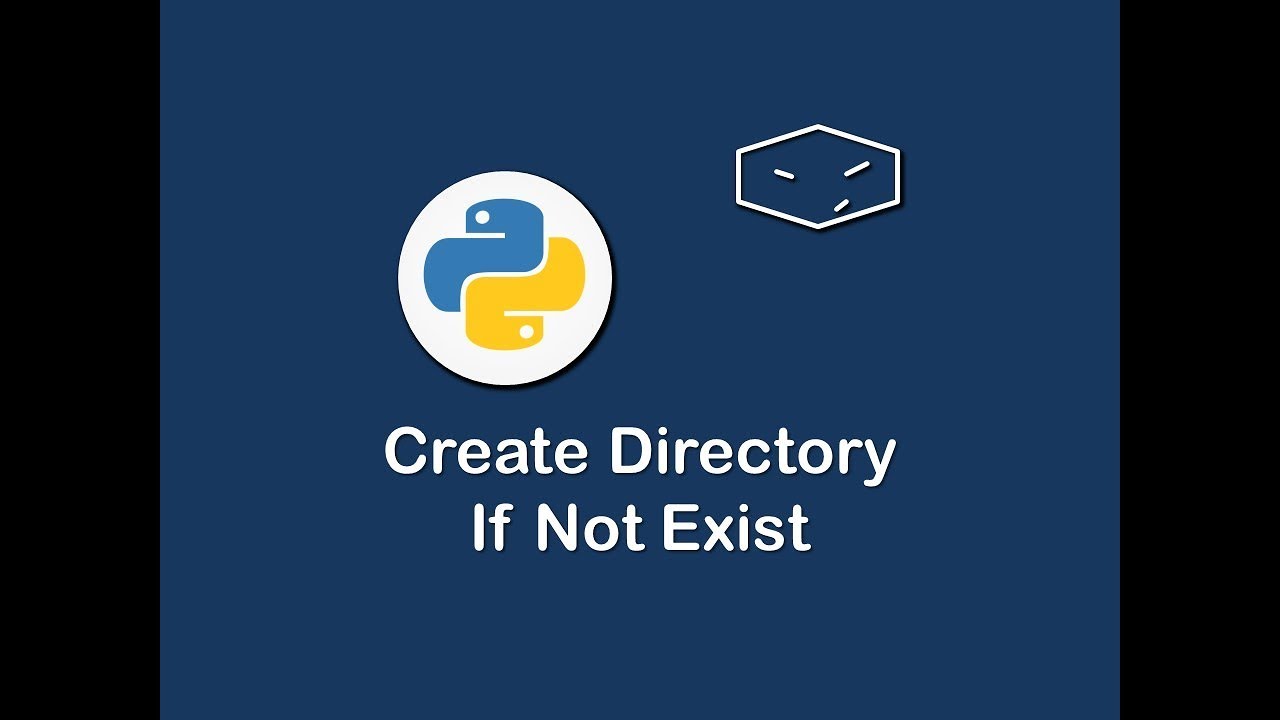
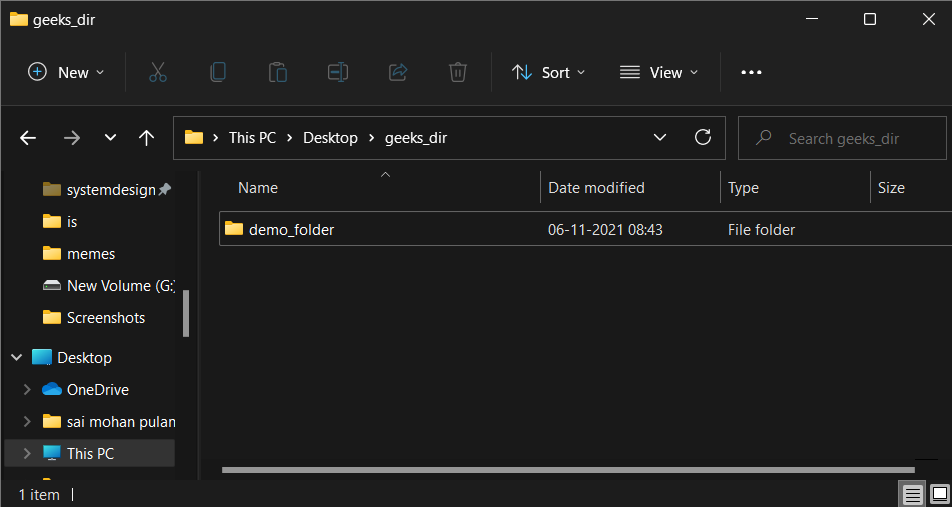
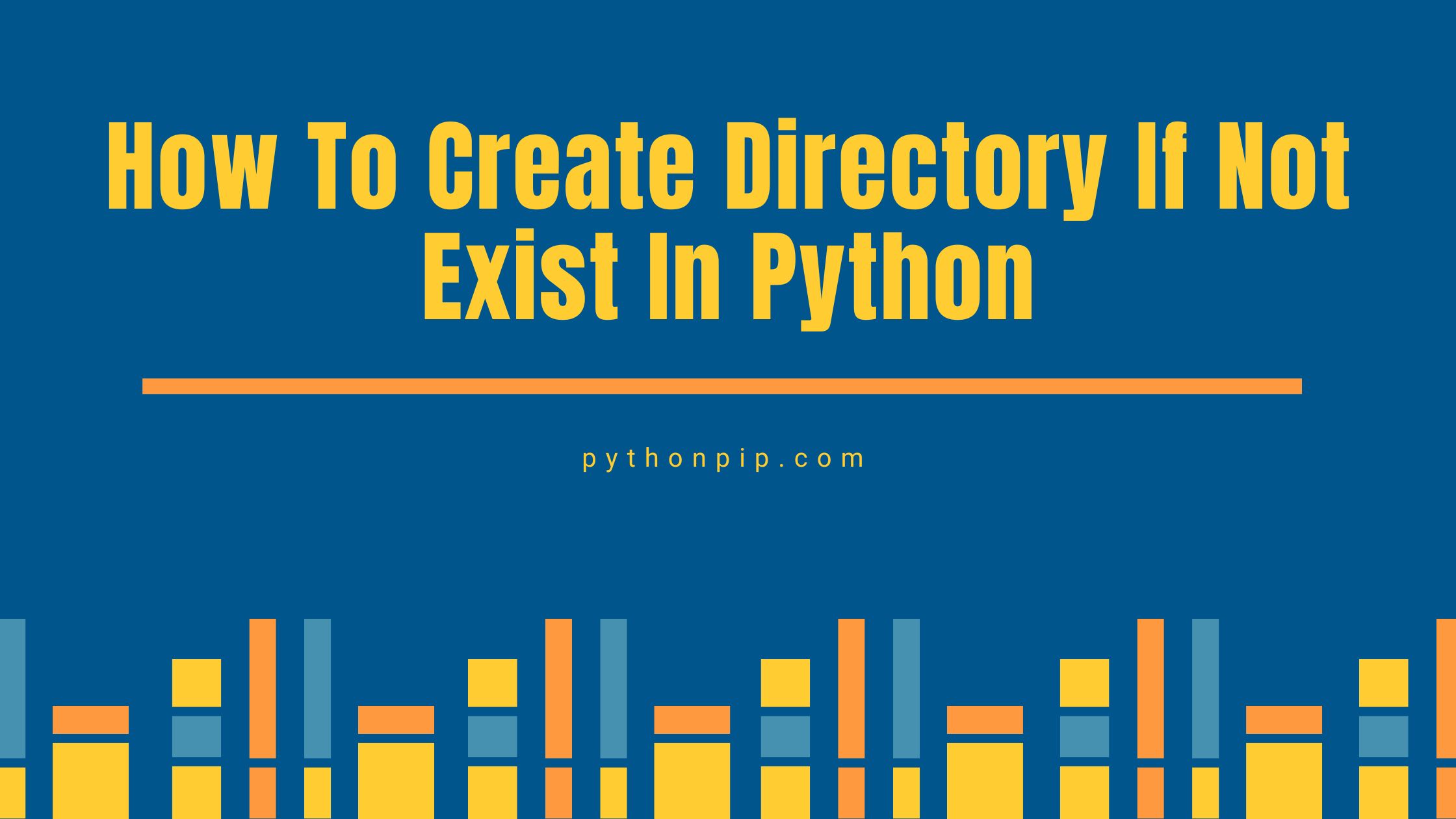
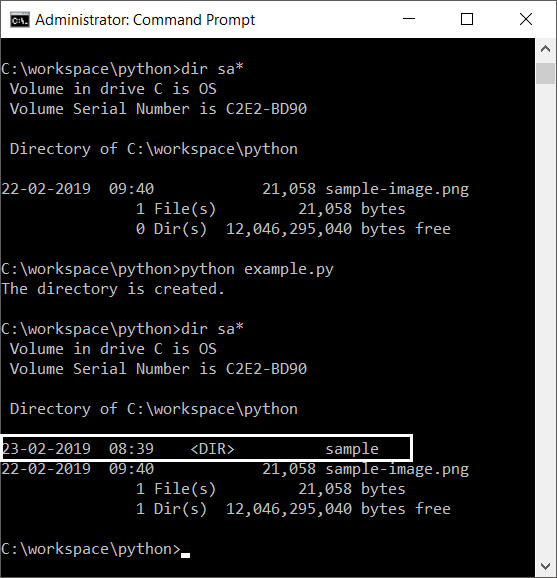

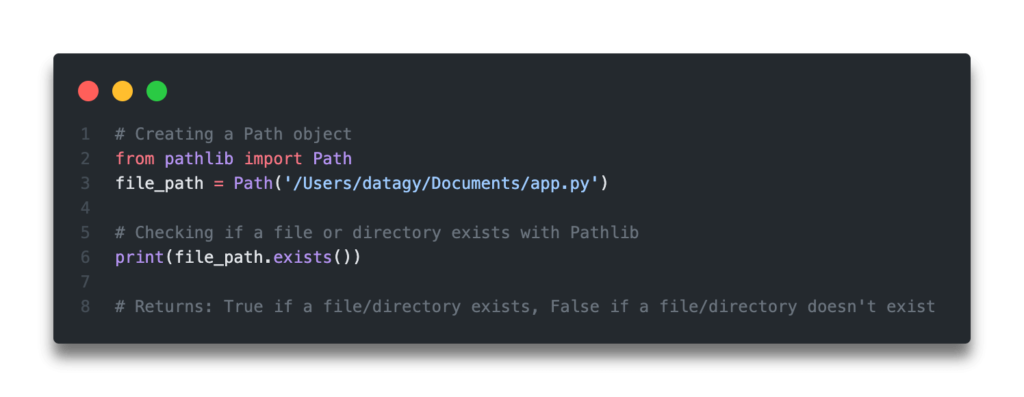
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/output-2.png)
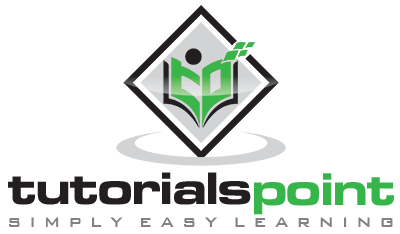
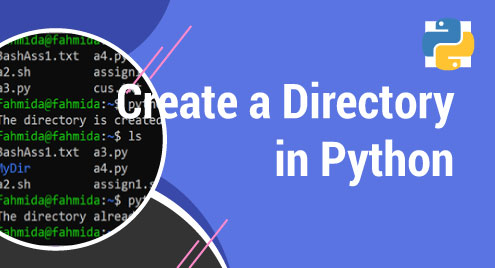
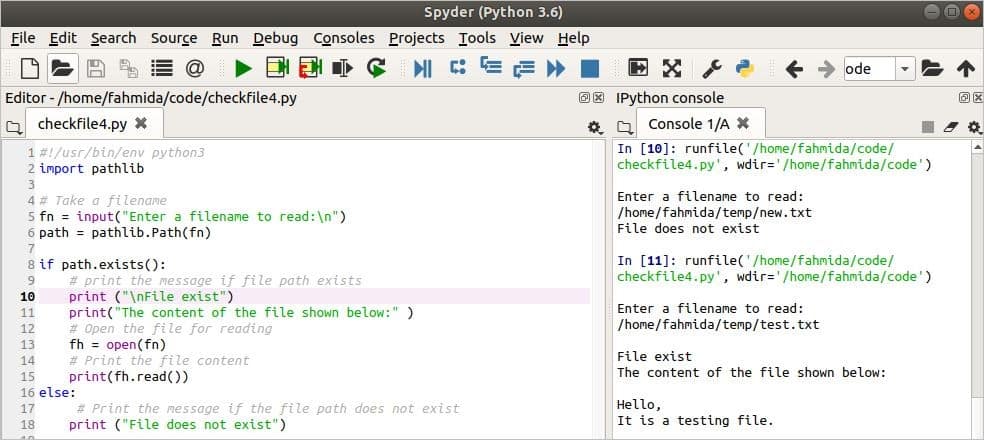
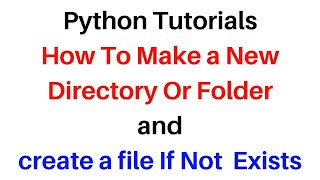
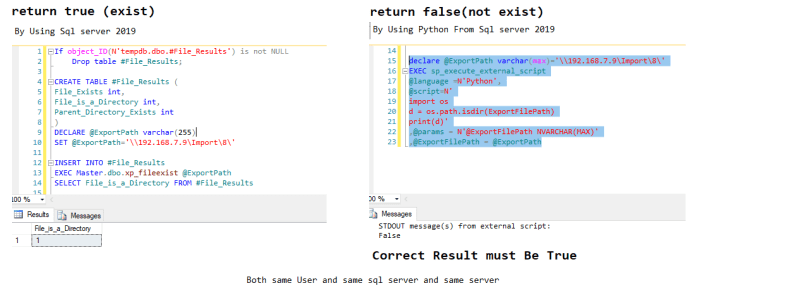
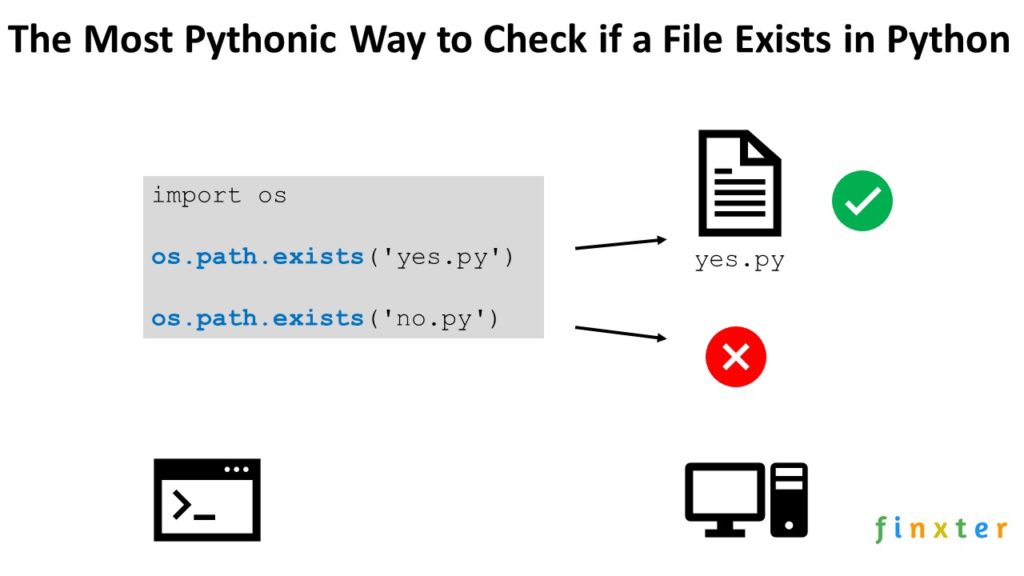
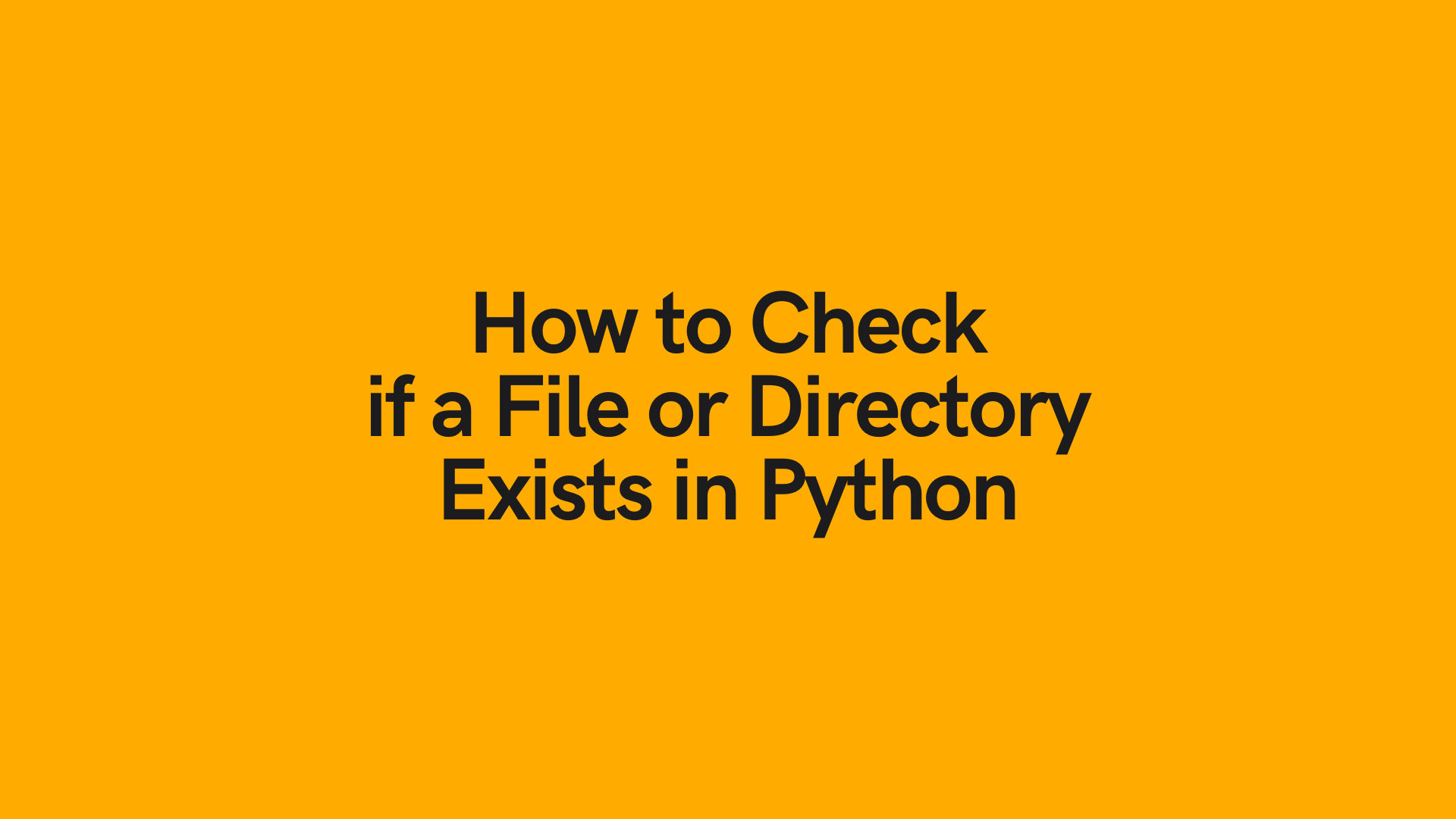
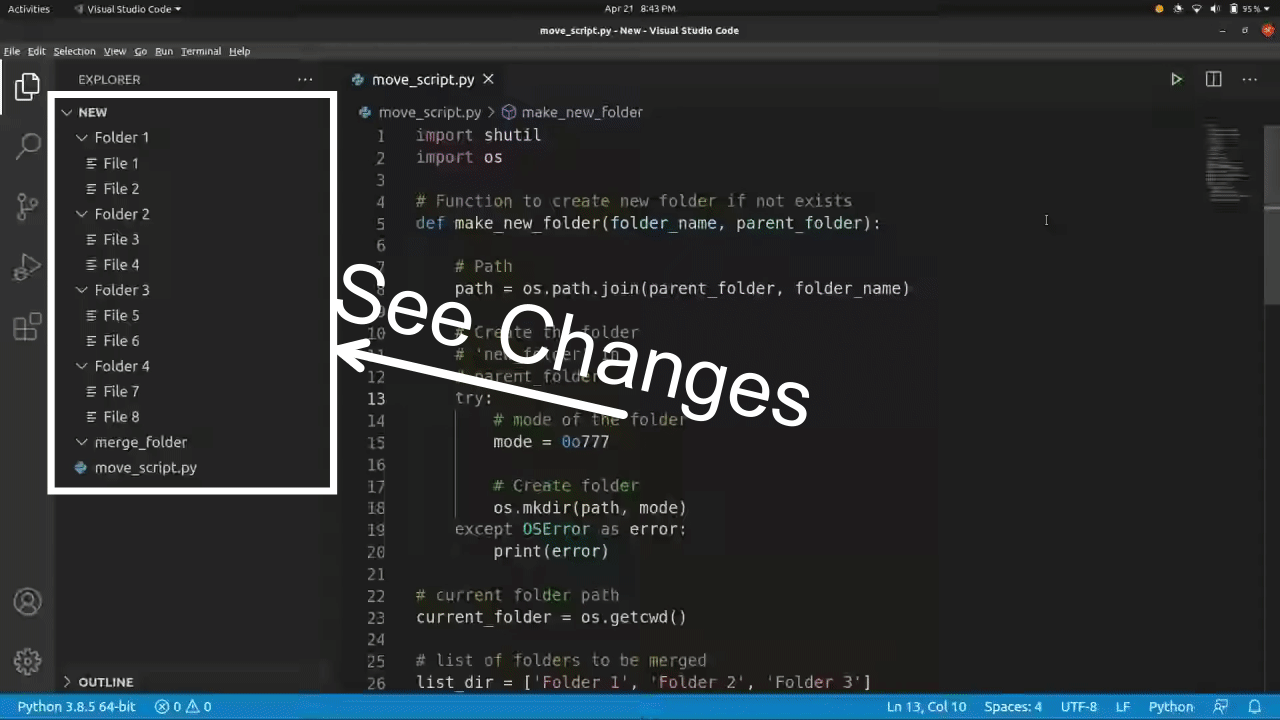

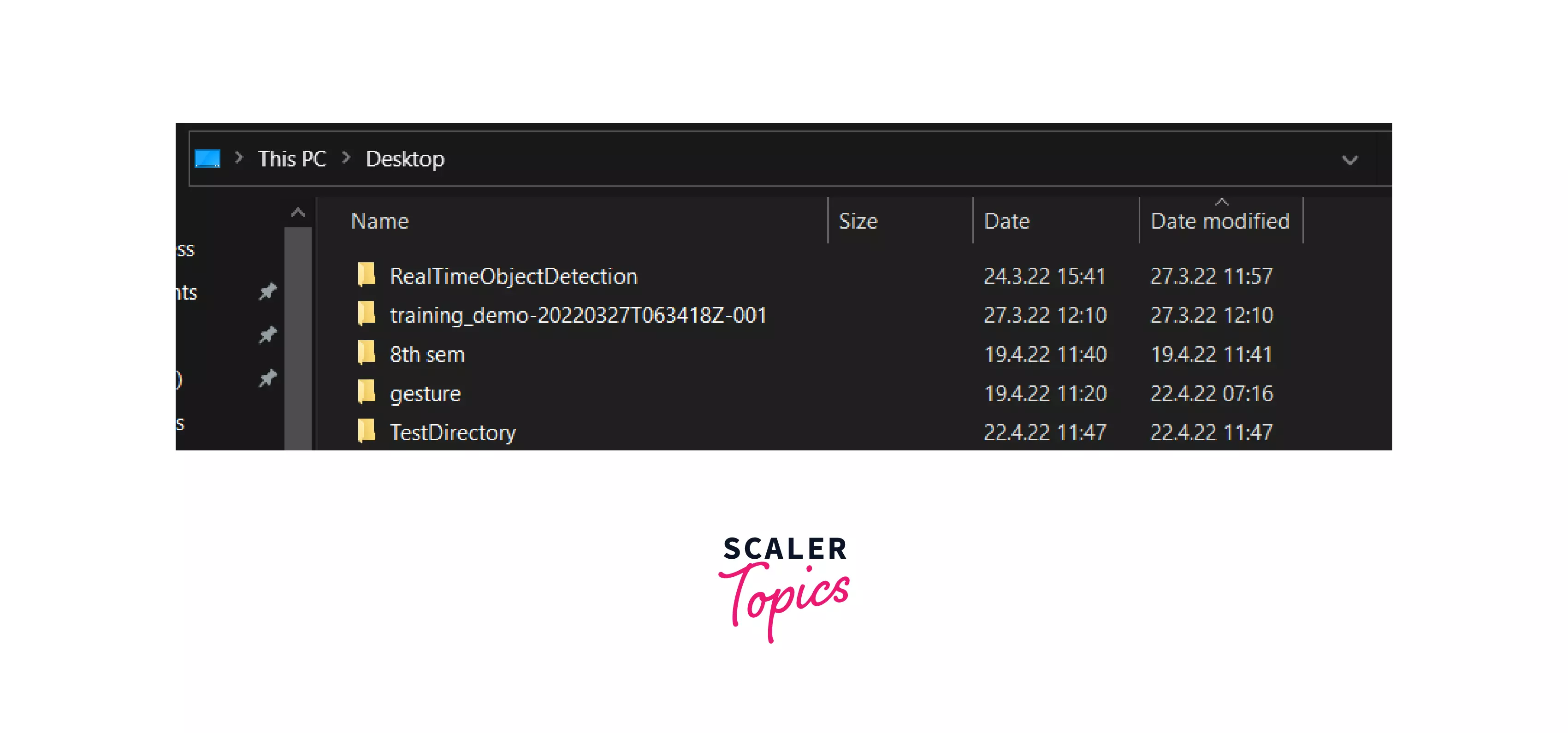
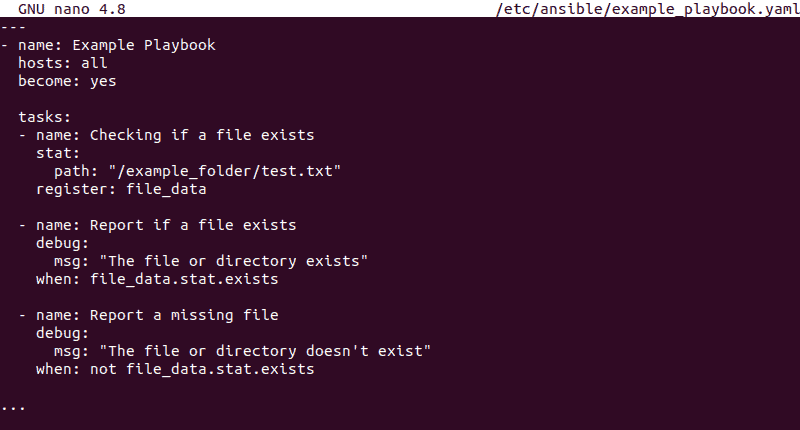

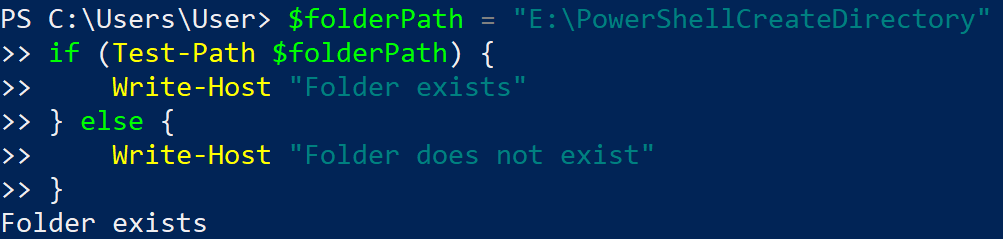
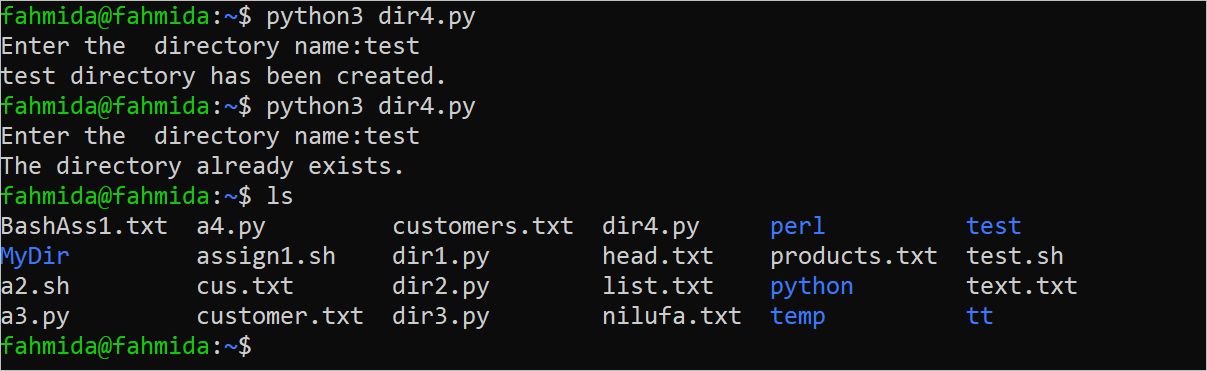
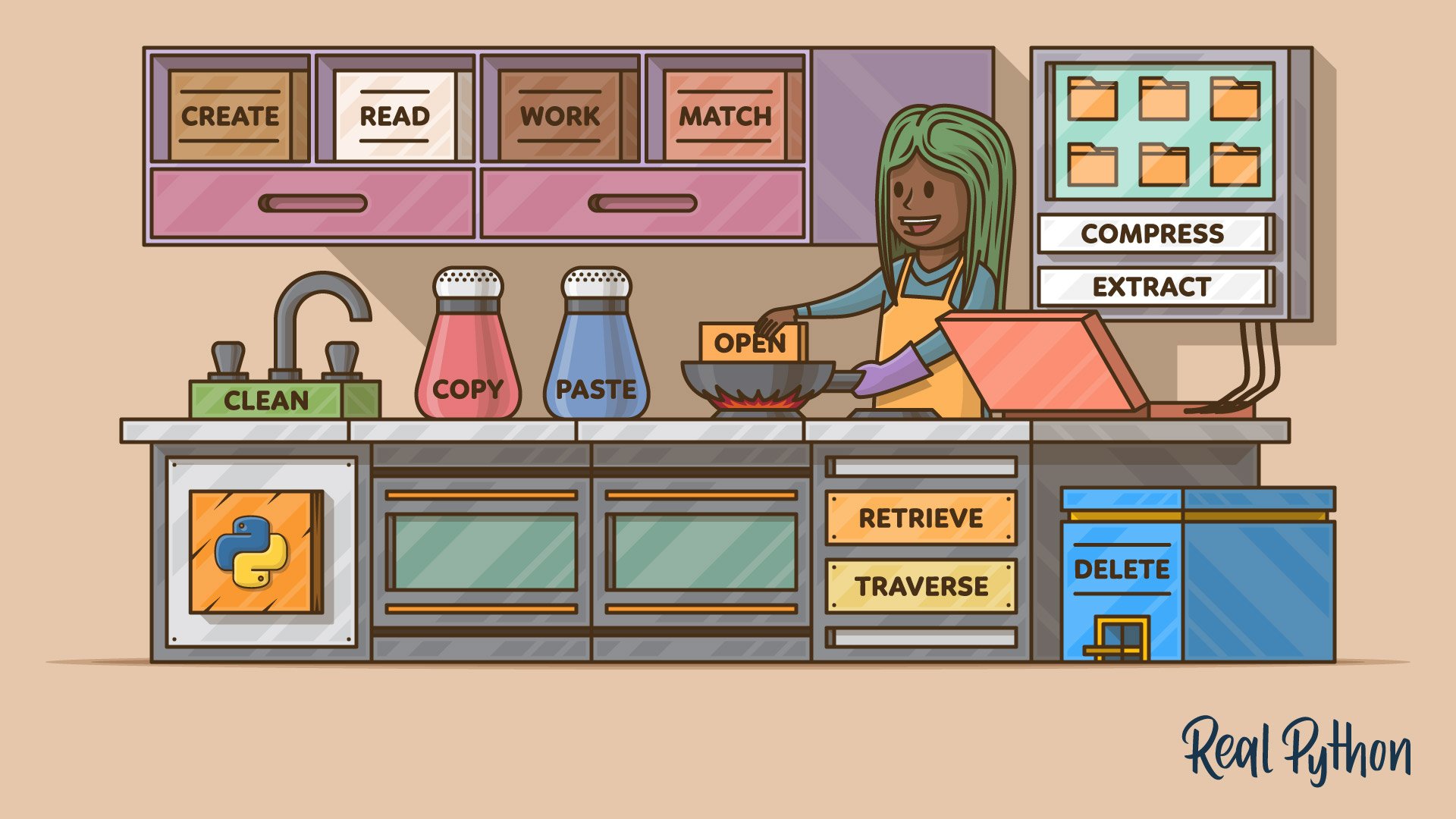
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/all-fs-methods-1.png)
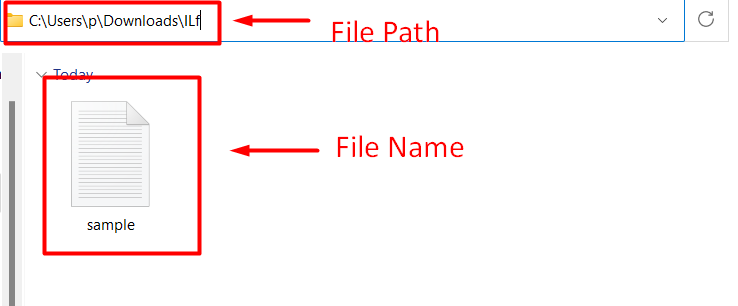
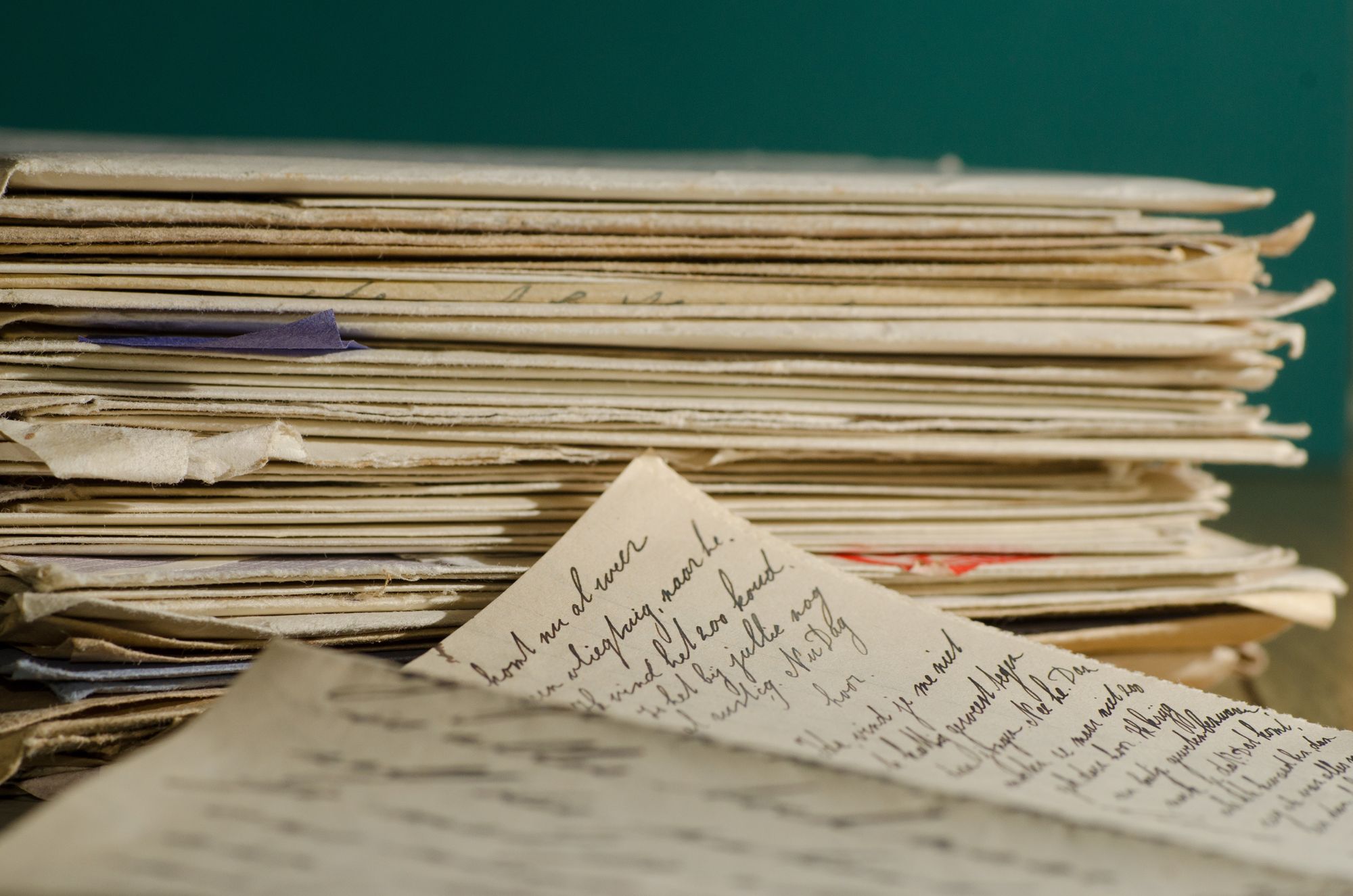
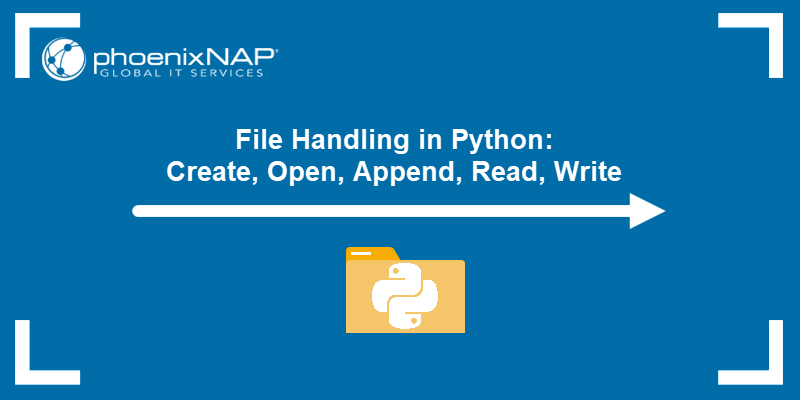
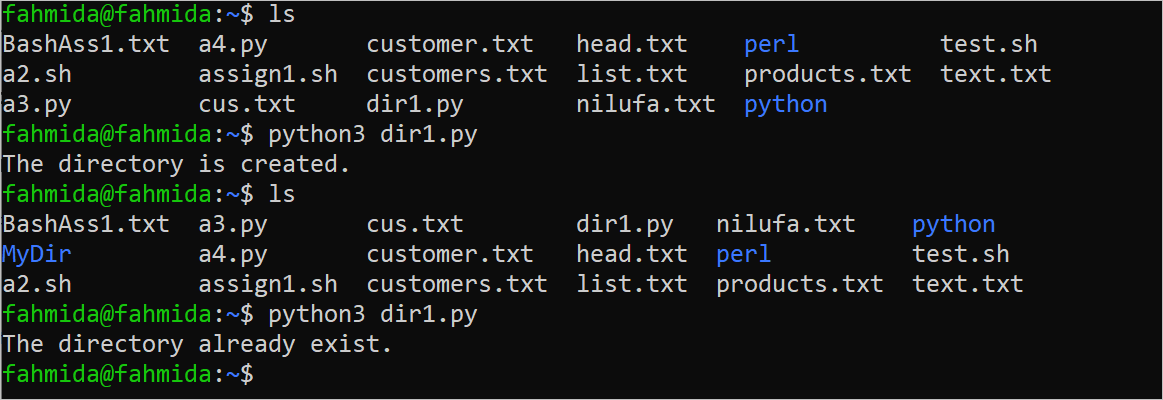
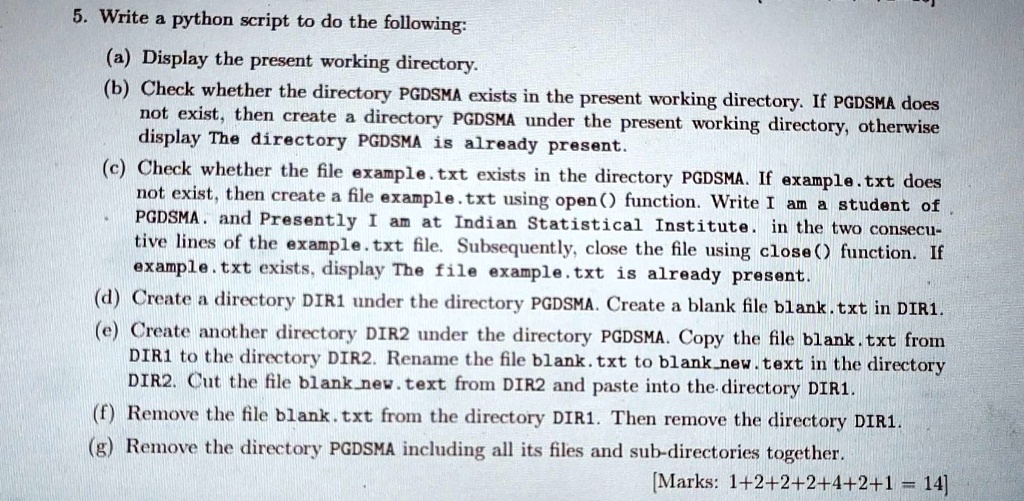
![FileExistsError: [Errno 17] File exists in Python [Solved] | bobbyhadz Fileexistserror: [Errno 17] File Exists In Python [Solved] | Bobbyhadz](https://bobbyhadz.com/images/blog/python-fileexistserror-errno-17-file-exists/banner.webp)


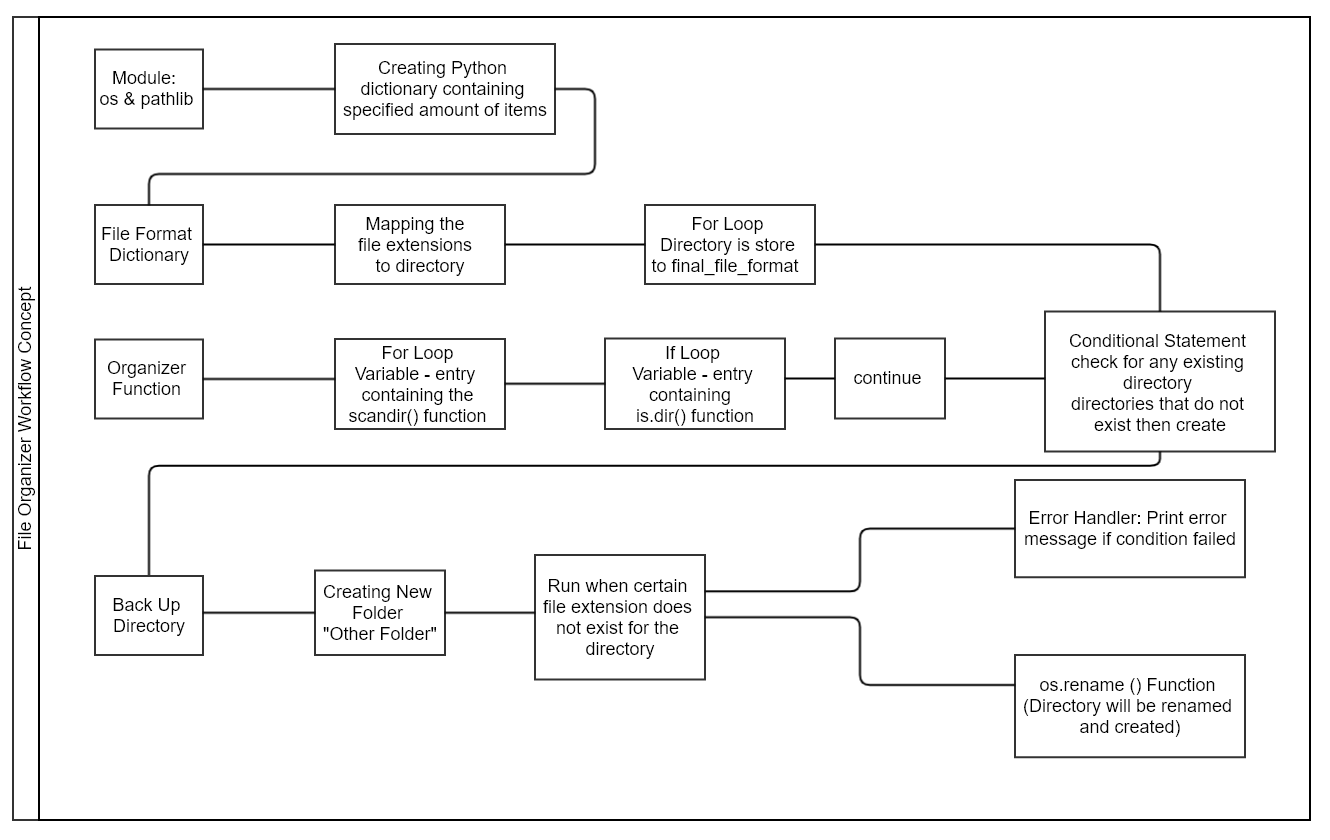
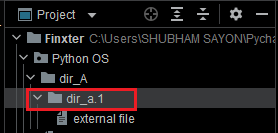
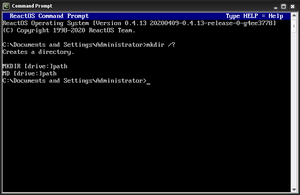
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/recursive-directory-creation.png)
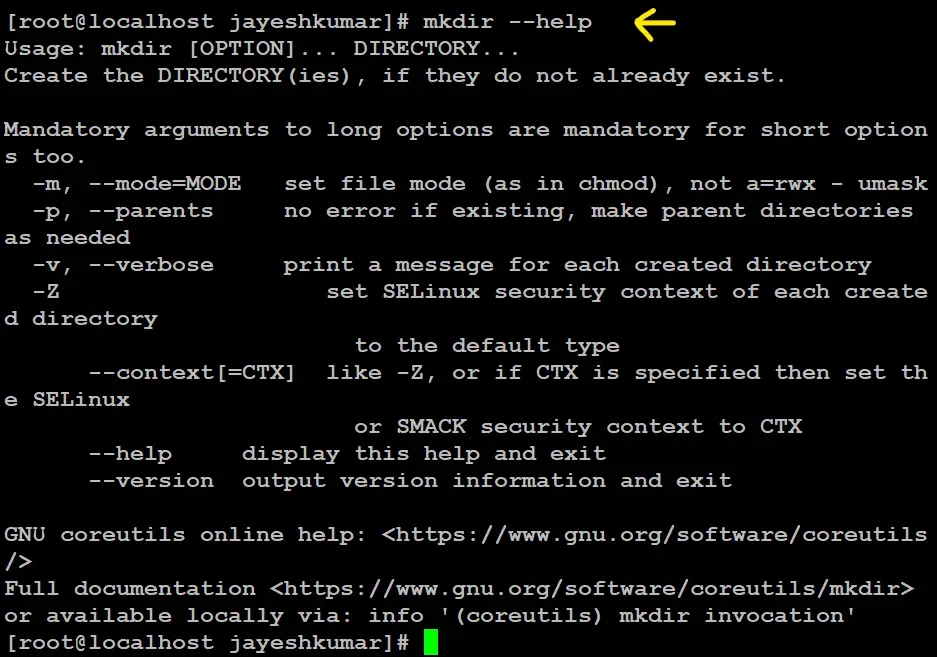
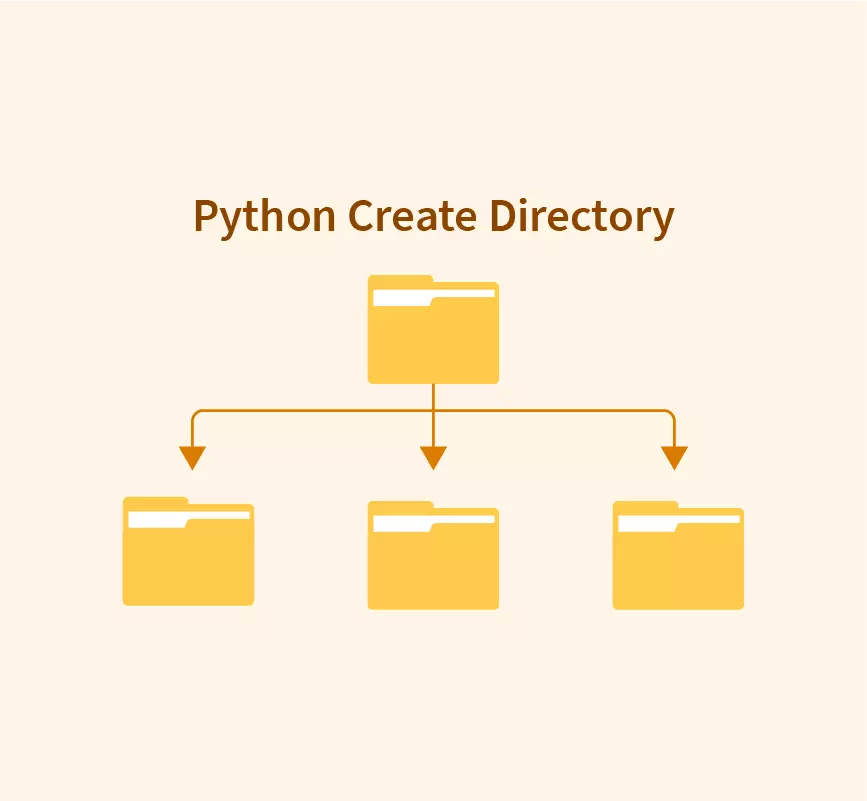
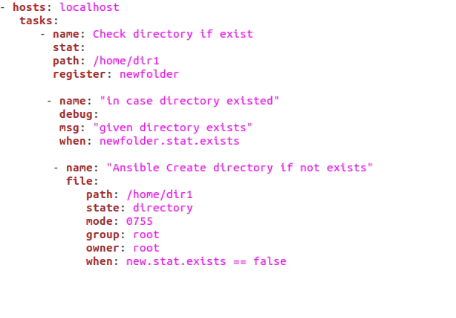
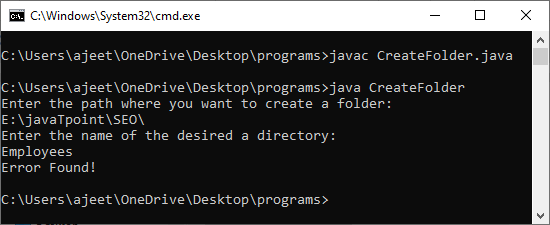
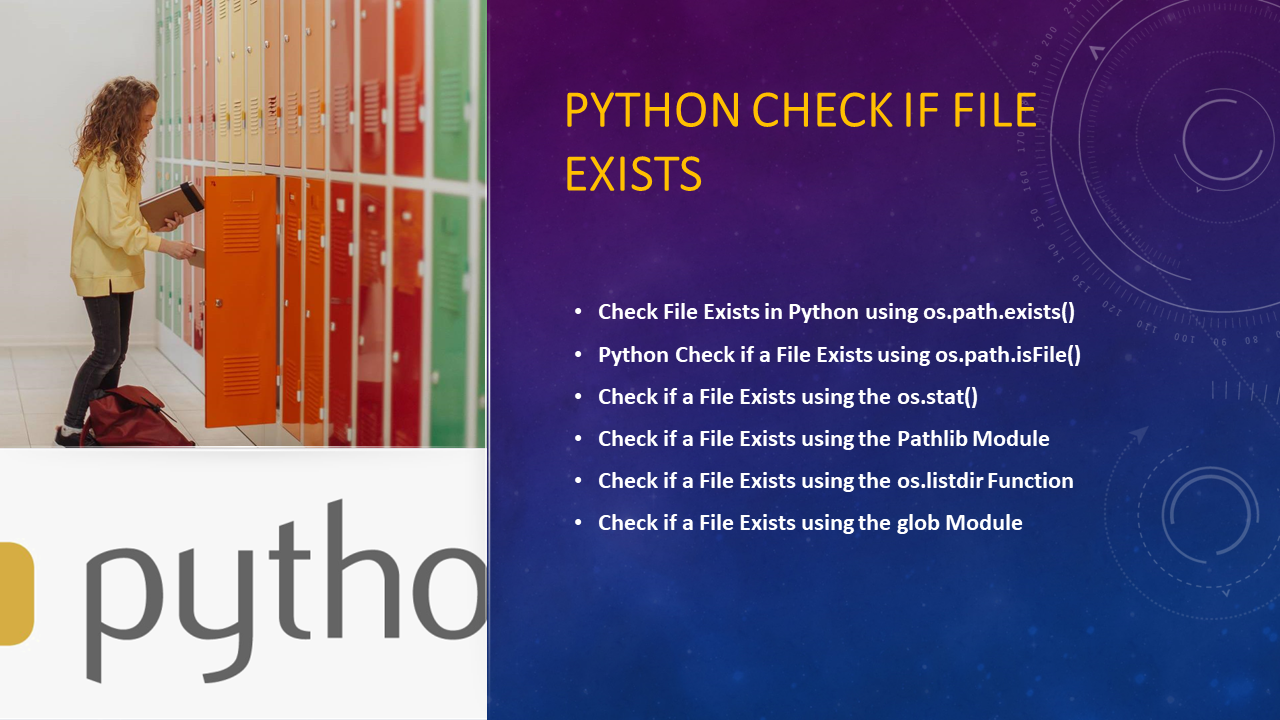
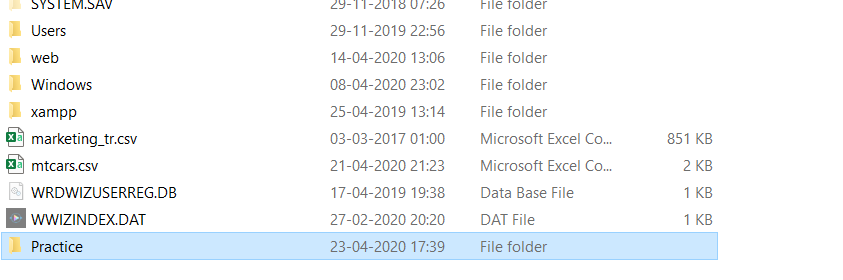
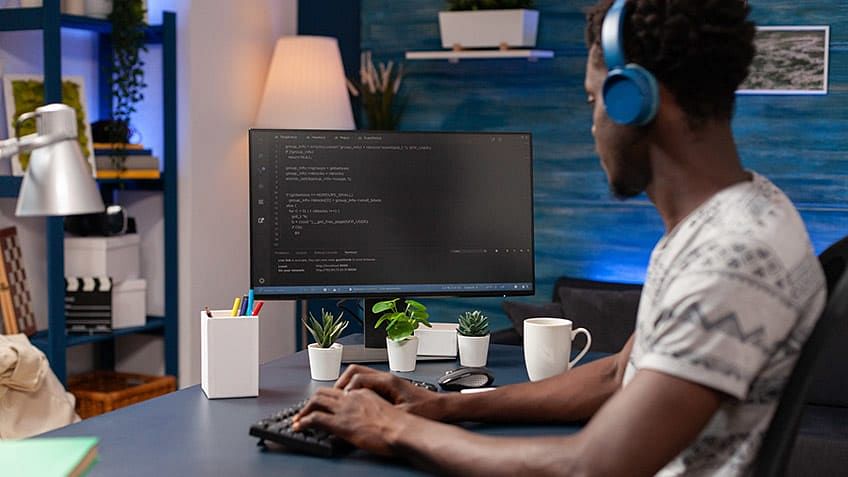
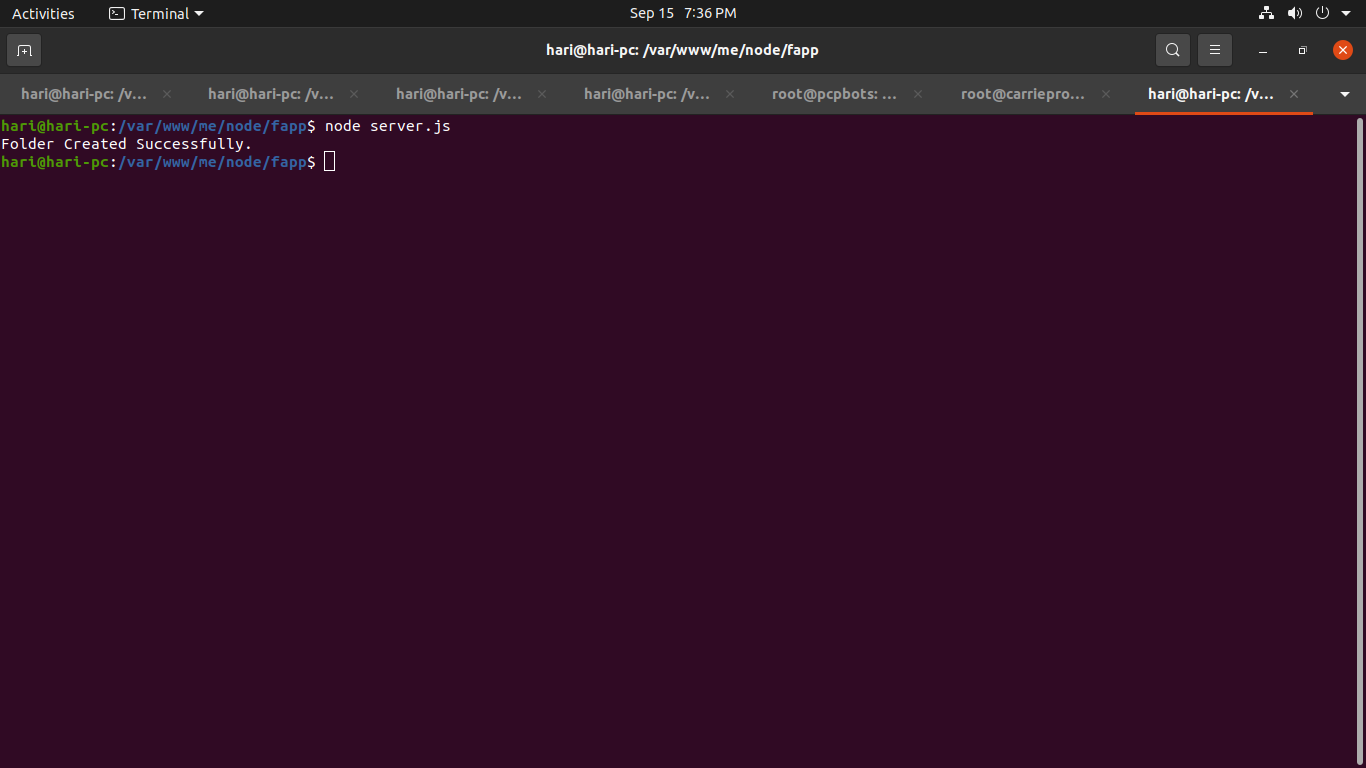
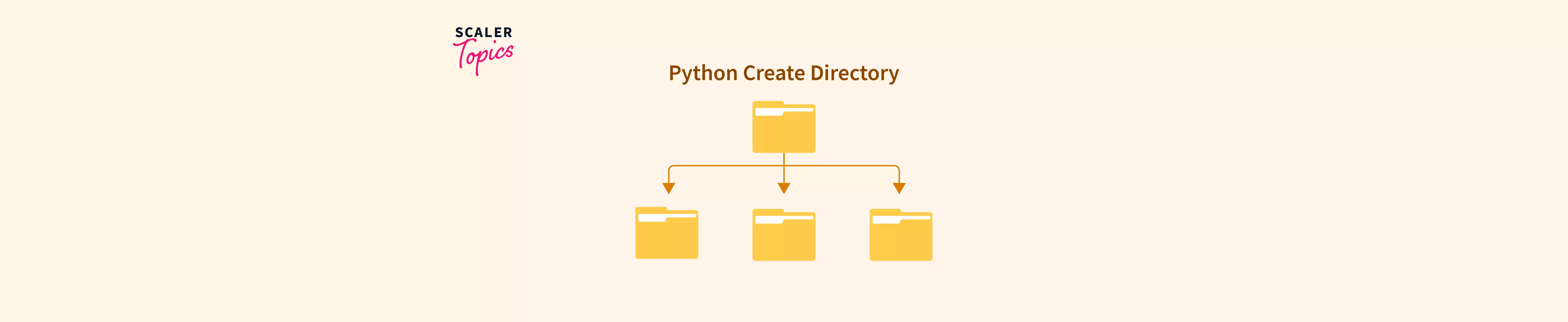
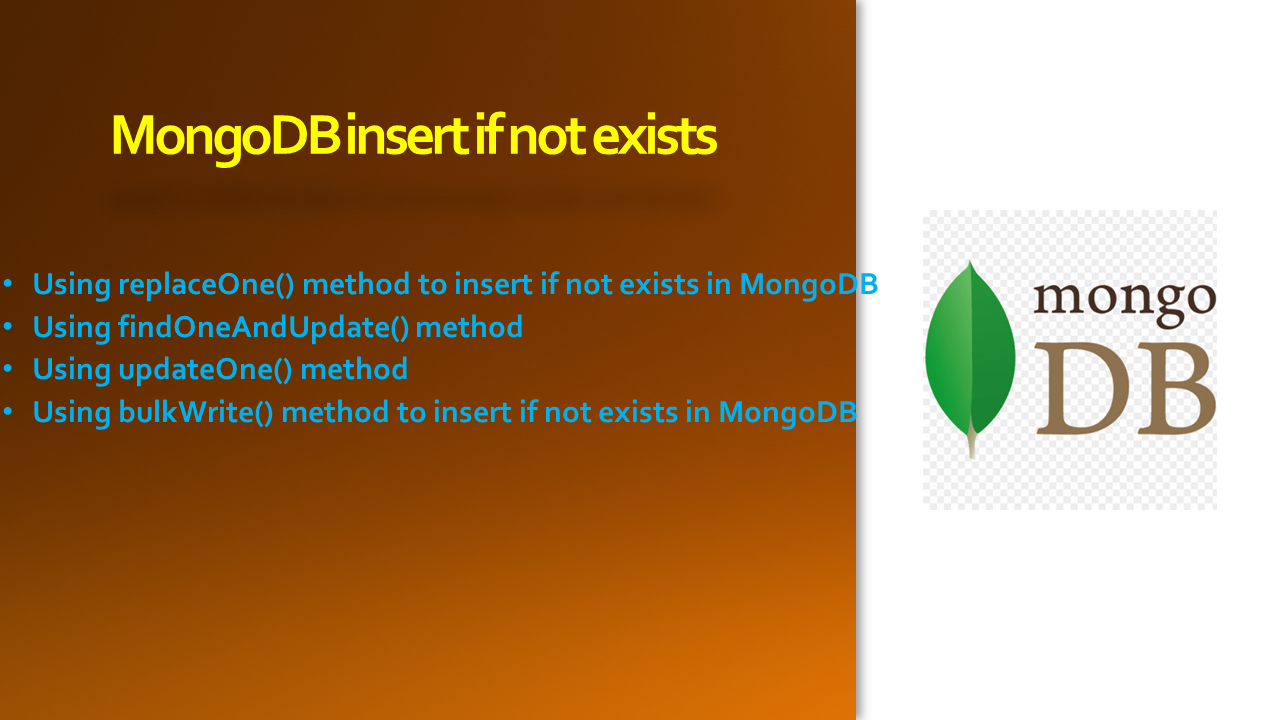
![FileExistsError: [Errno 17] File exists in Python [Solved] | bobbyhadz Fileexistserror: [Errno 17] File Exists In Python [Solved] | Bobbyhadz](https://bobbyhadz.com/images/blog/python-fileexistserror-errno-17-file-exists/directory-already-exists.webp)
Article link: python create directory if not exist.
Learn more about the topic python create directory if not exist.
- How can I create a directory in Python using ‘mkdir if not exists’?
- How can I create a directory if it does not exist using Python
- How to Create Directory If Not Exists in Python – AppDividend
- How do I create a directory, and any missing parent directories?
- How to Create Directory If it Does Not Exist using Python?
- Python: Create a Directory if it Doesn’t Exist – Datagy
- How To Create a Directory If Not Exist In Python – pythonpip.com
- Creating a Directory in Python – How to Create a Folder
- How to check if a file or directory exists in Python
- Create a Directory in Python – Linux Hint
See more: nhanvietluanvan.com/luat-hoc