Python Checking If Key Exists In Dictionary
1. Accessing Dictionary Elements:
To access a specific value in a dictionary, we usually use square brackets and provide the desired key. However, if the key does not exist in the dictionary, this method will raise a KeyError. Therefore, it is important to check if the key exists before accessing it directly.
2. Using the `in` keyword for Checking Key Existence:
The simplest way to check if a key exists in a dictionary is by using the `in` keyword. We can use this keyword followed by the dictionary name and the key we want to check. The `in` keyword returns a boolean value, True if the key is present and False otherwise.
Example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in my_dict:
print(“Key ‘name’ exists in the dictionary”)
else:
print(“Key ‘name’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘name’ exists in the dictionary
“`
3. Using the `get()` Method for Checking Key Existence:
The `get()` method is another way to check if a key exists in a dictionary. It returns either the value associated with the given key or a default value if the key is not present. By passing a default value as the second argument to the `get()` method, we can distinguish between existing and non-existing keys.
Example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if my_dict.get(‘age’) is not None:
print(“Key ‘age’ exists in the dictionary”)
else:
print(“Key ‘age’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘age’ exists in the dictionary
“`
4. Using the `keys()` Method for Key Existence:
The `keys()` method returns a list of all the keys in the dictionary. We can use this list to check if a specific key exists by simply checking if it is present in the list of keys.
Example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘city’ in my_dict.keys():
print(“Key ‘city’ exists in the dictionary”)
else:
print(“Key ‘city’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘city’ exists in the dictionary
“`
5. Using the `has_key()` Method (Python 2.x only):
In older versions of Python (2.x), the `has_key()` method was available to check if a key exists in a dictionary. However, this method has been removed in Python 3.x and is no longer recommended for use.
Example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if my_dict.has_key(‘age’):
print(“Key ‘age’ exists in the dictionary”)
else:
print(“Key ‘age’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘age’ exists in the dictionary
“`
6. Using the try-except Block for Key Existence:
Another approach to check if a key exists in a dictionary is by using a try-except block. We can try to access the key directly and handle the KeyError exception if it occurs. This method allows us to perform specific actions or return a default value in case the key does not exist.
Example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
try:
value = my_dict[‘country’]
print(“Key ‘country’ exists in the dictionary”)
except KeyError:
print(“Key ‘country’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘country’ does not exist in the dictionary
“`
7. Using the `__contains__()` Method for Key Existence:
The `__contains__()` method is an alternative way to check if a key is present in a dictionary. It is automatically called by the `in` keyword when checking for key existence. We can override this method in our custom dictionary classes to define custom behavior for key existence checks.
Example:
“`python
class MyDict(dict):
def __contains__(self, key):
if key.startswith(‘x’):
return True
return super().__contains__(key)
my_dict = MyDict({‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’})
if ‘x_name’ in my_dict:
print(“Key ‘x_name’ exists in the dictionary”)
else:
print(“Key ‘x_name’ does not exist in the dictionary”)
“`
Output:
“`
Key ‘x_name’ exists in the dictionary
“`
FAQs:
Q: How do you check if a key does not exist in a dictionary in Python?
To check if a key does not exist in a dictionary, you can use the `not in` keyword. This keyword returns True if the key is not present in the dictionary and False otherwise.
Example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘country’ not in my_dict:
print(“Key ‘country’ does not exist in the dictionary”)
else:
print(“Key ‘country’ exists in the dictionary”)
“`
Output:
“`
Key ‘country’ does not exist in the dictionary
“`
Q: How do you check if a key exists in an array in Python?
In Python, arrays are called lists. To check if a key exists in a list, you can use the `in` keyword followed by the list name and the key you want to check. The `in` keyword returns True if the key is present and False otherwise.
Example:
“`python
my_list = [‘apple’, ‘banana’, ‘orange’]
if ‘banana’ in my_list:
print(“Key ‘banana’ exists in the list”)
else:
print(“Key ‘banana’ does not exist in the list”)
“`
Output:
“`
Key ‘banana’ exists in the list
“`
Q: How do you check if a value exists in a dictionary in Python?
To check if a value exists in a dictionary, you can use the `in` keyword with the `values()` method. The `values()` method returns a list of all the values in the dictionary, and the `in` keyword checks if the desired value is present in that list.
Example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘John’ in my_dict.values():
print(“Value ‘John’ exists in the dictionary”)
else:
print(“Value ‘John’ does not exist in the dictionary”)
“`
Output:
“`
Value ‘John’ exists in the dictionary
“`
Q: How do you sort a dictionary by value in Python?
To sort a dictionary by value in Python, you can use the `sorted()` function with the `items()` method. The `items()` method returns a list of key-value pairs, and the `sorted()` function sorts this list based on the values.
Example:
“`python
my_dict = {‘apple’: 5, ‘banana’: 3, ‘orange’: 7}
sorted_dict = dict(sorted(my_dict.items(), key=lambda x: x[1]))
print(sorted_dict)
“`
Output:
“`
{‘banana’: 3, ‘apple’: 5, ‘orange’: 7}
“`
Q: How do you add a value to a key in a dictionary in Python?
To add a value to a key in a dictionary in Python, you can simply assign the value to the desired key using the assignment operator `=`. If the key already exists, the value will be updated. If the key does not exist, a new key-value pair will be added to the dictionary.
Example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25}
my_dict[‘city’] = ‘New York’
print(my_dict)
“`
Output:
“`
{‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
“`
Q: How do you add a dictionary to another dictionary in Python?
To add a dictionary to another dictionary in Python, you can use the `update()` method. This method merges the two dictionaries, adding or updating the key-value pairs from the second dictionary into the first one.
Example:
“`python
dict1 = {‘name’: ‘John’, ‘age’: 25}
dict2 = {‘city’: ‘New York’, ‘country’: ‘USA’}
dict1.update(dict2)
print(dict1)
“`
Output:
“`
{‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’, ‘country’: ‘USA’}
“`
In conclusion, Python provides various ways to check if a key exists in a dictionary. The choice of method depends on the specific requirements and the desired behavior when the key does not exist. By using these methods effectively, you can handle key existence checks with ease in your Python programs.
#Python Check Whether A Given Key Is Already Exists In A Dictionary
How To Check If Key Exists In Dict Python?
A dictionary, also called a hash map or associative array, is a fundamental data structure in Python. It consists of a collection of key-value pairs where each key must be unique. When working with dictionaries, it is often necessary to check if a specific key exists within the dictionary. In this article, we will explore various methods to perform this check in Python.
Method 1: Using the ‘in’ keyword
The simplest and most straightforward method to check if a key exists in a dictionary is by using the ‘in’ keyword. This keyword allows us to check for membership within a sequence, such as a dictionary.
Here’s an example:
“`
student = {“name”: “John”, “age”: 21, “grade”: “A”}
if “age” in student:
print(“Key ‘age’ exists in the dictionary”)
“`
In this example, we check if the key “age” exists in the dictionary student. If it does, we print the corresponding message. Otherwise, nothing happens.
Method 2: Using the get() method
The get() method is another useful way to check for the existence of a key in a dictionary. This method returns the value associated with a key if it exists, and a default value otherwise.
Here’s an example:
“`
student = {“name”: “John”, “age”: 21, “grade”: “A”}
age = student.get(“age”)
if age is not None:
print(“Key ‘age’ exists in the dictionary”)
“`
In this example, we use the get() method to retrieve the value associated with the key “age”. If the key exists, the value is assigned to the variable age. We then check if age is not None, indicating that the key exists, and print the message accordingly.
Method 3: Using the keys() method
The keys() method returns a view object that contains all the keys in the dictionary. This allows us to easily iterate over the keys and check for the existence of a specific key.
Here’s an example:
“`
student = {“name”: “John”, “age”: 21, “grade”: “A”}
for key in student.keys():
if key == “age”:
print(“Key ‘age’ exists in the dictionary”)
break
“`
In this example, we iterate over all the keys in the dictionary using the keys() method. Inside the loop, we compare each key with the desired key, “age”, and print the message if a match is found. We also include a break statement to stop the loop once the key is found, as there is no need to continue iterating.
Method 4: Using the has_key() method (deprecated)
In older versions of Python (2.x), the has_key() method was used to check for the existence of a key in a dictionary. However, this method has been deprecated in Python 3.x and no longer recommended for use.
Here’s an example of how it used to work:
“`
student = {“name”: “John”, “age”: 21, “grade”: “A”}
if student.has_key(“age”):
print(“Key ‘age’ exists in the dictionary”)
“`
Although this method may work in older versions of Python, it is not advisable to use it in modern Python code.
FAQs:
Q: What happens if we try to access a non-existent key in a dictionary?
A: If we try to access a non-existent key in a dictionary, it raises a KeyError. To avoid this, it is crucial to check if the key exists before accessing it.
Q: What is the difference between using the ‘in’ keyword and the get() method?
A: The ‘in’ keyword returns a boolean value indicating whether the key exists or not, while the get() method returns the value associated with the key if it exists or a default value if it does not. The choice between the two methods depends on the specific requirement of the program.
Q: Can we use the ‘in’ keyword to check for the existence of nested keys in a dictionary?
A: Yes, the ‘in’ keyword can be used to check for the existence of nested keys in a dictionary. For example, if we have a dictionary called person that contains another dictionary called address, we can check if the key “city” exists in the address dictionary using the statement “city” in person[“address”].
Q: Is it possible to check for the existence of a value in a dictionary?
A: Yes, we can check for the existence of a value in a dictionary by using the ‘in’ keyword in conjunction with the values() method. This method returns a view object containing all the values in the dictionary, allowing us to check for the existence of a specific value.
In conclusion, checking if a key exists in a dictionary is a common task when working with Python dictionaries. We’ve covered several methods to perform this check, including using the ‘in’ keyword, the get() method, the keys() method, and mentioned the deprecated has_key() method. By applying these techniques, you can effectively handle key existence checks to ensure the integrity and functionality of your Python code.
How To Check If Key-Value Pair Exists In Dictionary Python?
Dictionaries in Python are widely used data structures that allow you to store and retrieve data efficiently. They consist of key-value pairs, where each key is associated with a value. It is crucial in many applications to check if a specific key-value pair exists in a dictionary before performing further operations. In this article, we will explore various methods to accomplish this task in Python.
Method 1: Use the ‘in’ Operator
The most common and straightforward method to check if a key-value pair exists in a dictionary is by using the ‘in’ operator. It allows you to check if a key exists in the dictionary, and if so, you can compare the associated value with the desired value.
Consider the following example:
“`
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
if ‘key1’ in my_dict and my_dict[‘key1’] == ‘value1’:
print(“Key-value pair exists!”)
else:
print(“Key-value pair does not exist!”)
“`
In this example, we check if ‘key1’ exists in the dictionary ‘my_dict’ using the ‘in’ operator. Then, we compare the value associated with ‘key1’ with the desired value ‘value1’. If both conditions are satisfied, we print that the key-value pair exists; otherwise, we output that it does not exist.
Method 2: Use the get() Method
Another approach to check if a key-value pair exists is by using the get() method provided by dictionaries. The get() method returns the value associated with a given key if it exists; otherwise, it returns None. By comparing the return value of get() with None, we can determine if a key-value pair exists or not.
Let’s consider an example:
“`
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
if my_dict.get(‘key1’) == ‘value1’:
print(“Key-value pair exists!”)
else:
print(“Key-value pair does not exist!”)
“`
In this example, we use the get() method to retrieve the value associated with ‘key1’ in ‘my_dict’. If the returned value matches the desired value ‘value1’, we conclude that the key-value pair exists; otherwise, we output that it does not exist.
Method 3: Use the items() Method
The items() method returns a view object that contains all the key-value pairs in a dictionary. By iterating over this object, we can check if a specific key-value pair exists.
Consider the following example:
“`
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
for key, value in my_dict.items():
if key == ‘key1’ and value == ‘value1’:
print(“Key-value pair exists!”)
break
else:
print(“Key-value pair does not exist!”)
“`
In this example, we iterate over all the key-value pairs in ‘my_dict’ using the items() method. If we find a key-value pair where the key matches ‘key1’ and the value matches ‘value1’, we print that the key-value pair exists. If no match is found, we output that it does not exist.
FAQs:
Q1. What is the advantage of using the ‘in’ operator to check for key existence in a dictionary?
Using the ‘in’ operator is a commonly used method as it provides a concise and readable way to check for the existence of a key in a dictionary. It is also efficient and has a time complexity of O(1) in average case scenarios.
Q2. Can we use the ‘in’ operator to check for value existence in a dictionary?
No, the ‘in’ operator is used to check for the existence of keys, not values. To check for the existence of a value in a dictionary, we can use methods like values() or manually iterate over the dictionary’s values.
Q3. What happens if we use the get() method with a non-existing key?
If the get() method is used with a non-existing key, it returns None by default. This behavior can be modified by providing a default value as a second argument to the get() method.
Q4. How can we check if any key exists in a dictionary without knowing its value?
To check for the existence of any key without knowing its value, we can use the ‘in’ operator. For example, if ‘key1’ exists in a dictionary, we can simply check if ‘key1’ in my_dict.
In conclusion, checking if a key-value pair exists in a dictionary is essential in various Python applications. This article explored different methods, including using the ‘in’ operator, the get() method, and the items() method. By applying these techniques, you can easily determine the existence of specific key-value pairs in dictionaries, allowing you to perform subsequent operations accordingly.
Keywords searched by users: python checking if key exists in dictionary Check if key not exists in dictionary Python, Check key in dict Python, Python check key in array, Check value in dictionary Python, How to check if value exists in python, Sort dictionary by value Python, Add value to key dictionary Python, Add dictionary Python
Categories: Top 84 Python Checking If Key Exists In Dictionary
See more here: nhanvietluanvan.com
Check If Key Not Exists In Dictionary Python
In Python, a dictionary is a data structure that stores information in key-value pairs. Each key in a dictionary must be unique, making it a convenient way to organize and access data. However, there may be instances when you need to check if a key does not exist in a dictionary. This article will guide you through various ways to accomplish this task and help you understand how to handle such cases effectively.
Methods to Check if a Key Does Not Exist in a Dictionary
There are several ways to determine if a key does not exist in a dictionary in Python. Here, we will discuss the most commonly used methods:
1. Using the ‘in’ operator:
Python allows you to use the ‘in’ operator to check if a given key exists in a dictionary. The ‘in’ operator returns True if the key is present in the dictionary; otherwise, it returns False. By negating the result using the ‘not’ keyword, we can easily determine if a key does not exist. Here’s an example:
“`python
dictionary = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘occupation’ not in dictionary:
print(“The key ‘occupation’ does not exist.”)
“`
In this example, we check if the key ‘occupation’ does not exist in the dictionary. If it doesn’t, the corresponding message is printed.
2. Using the get() method:
The get() method is another useful way to check if a key does not exist in a dictionary. This method allows you to provide a default value that will be returned if the specified key is not found in the dictionary. By comparing this default value with the key, you can determine if the key exists or not. Here’s an example:
“`python
dictionary = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
occupation = dictionary.get(‘occupation’, ‘Not specified’)
if occupation == ‘Not specified’:
print(“The key ‘occupation’ does not exist.”)
“`
In this example, the get() method is used to retrieve the value associated with the key ‘occupation’. Since the key does not exist, the default value ‘Not specified’ is returned. By comparing it with the actual key, we can determine if the key does not exist.
3. Using the keys() method:
The keys() method returns a view object that contains all the keys of a dictionary. You can convert this view object to a list and check if a particular key is present in it or not. If the key is not found in the list, it means that the key does not exist in the dictionary. Here’s an example:
“`python
dictionary = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘occupation’ not in list(dictionary.keys()):
print(“The key ‘occupation’ does not exist.”)
“`
In this example, we use the keys() method to retrieve a view object of all the keys in the dictionary. We then convert this view into a list and check if the key ‘occupation’ is present in it. If it is not, the corresponding message is printed.
FAQs:
Q1. Can I use the ‘in’ operator to check if a key exists and retrieve its value simultaneously?
Yes, the ‘in’ operator can be used along with the dictionary name to check if a key exists. If the key is found, accessing the key will return its corresponding value. Here’s an example:
“`python
dictionary = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in dictionary:
print(dictionary[‘name’])
“`
In this example, we check if the key ‘name’ exists in the dictionary. Since it does, we can retrieve its value (‘John’) and print it.
Q2. What happens if I try to access a key that does not exist in a dictionary without checking its existence?
If you try to access a key that does not exist in a dictionary without checking its existence, a KeyError is raised. This error indicates that the key you are trying to access does not exist in the dictionary. To avoid this error, it is recommended to check if the key exists before accessing it.
Q3. Can I delete a key from a dictionary if it does not exist without raising an error?
Yes, you can use the del keyword to delete a key from a dictionary without raising an error if the key does not exist. Using the ‘in’ operator to check the existence of the key before deleting it ensures that the deletion only occurs if the key is present.
“`python
dictionary = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘occupation’ in dictionary:
del dictionary[‘occupation’]
“`
In this example, the key ‘occupation’ is checked before attempting to delete it from the dictionary. Since it does not exist, the deletion is skipped.
Conclusion:
Checking if a key does not exist in a dictionary is a common operation in Python. By utilizing the ‘in’ operator, the get() method, and the keys() method, you can easily determine if a key is absent from a dictionary. By understanding these methods, you can handle cases where a key is not found efficiently and avoid errors in your code.
Check Key In Dict Python
Introduction
In Python, a dictionary is an essential data structure that allows you to store and retrieve data efficiently. It consists of key-value pairs, where each key must be unique. One common operation when working with dictionaries is checking whether a particular key exists. In this article, we will explore various methods to check if a key is present in a dictionary in Python, along with their pros and cons.
Methods to Check Key in a Dictionary
1. Using the ‘in’ Operator:
The simplest and most straightforward method to check if a key exists in a dictionary is by using the ‘in’ operator. It returns True if the key already exists in the dictionary; otherwise, it returns False. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in my_dict:
print(“Key ‘name’ exists”)
“`
The ‘in’ operator checks for the presence of a key in a dictionary in an efficient manner. It utilizes the hash table-based implementation of dictionaries, which allows for constant-time average case complexity.
2. Using the get() Method:
Another approach to check if a key exists in a dictionary is by using the get() method. This method takes the key as an argument and returns the corresponding value if the key exists; otherwise, it returns a default value, which is None by default. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
value = my_dict.get(‘name’)
if value is not None:
print(“Key ‘name’ exists”)
“`
The get() method allows you to specify a default value to be returned if the key does not exist, which can be useful in certain scenarios.
3. Using the keys() Method:
The keys() method returns a view object that contains all the keys present in a dictionary. It can be used to check if a specific key exists in the dictionary. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in my_dict.keys():
print(“Key ‘name’ exists”)
“`
However, using the keys() method to check for key existence is less efficient compared to the ‘in’ operator or the get() method since it creates a new view object, resulting in additional memory usage.
4. Using the has_key() Method (Python 2 only):
In Python 2, the has_key() method was commonly used to check if a key exists in a dictionary. However, this method has been deprecated in Python 3, and using it in newer versions will lead to a KeyError. Therefore, it is recommended to use the ‘in’ operator or the get() method instead.
FAQs
Q1. What happens if I check for a key that does not exist in a dictionary?
A key that does not exist in a dictionary will return False when using the ‘in’ operator or None when using the get() method. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘address’ in my_dict:
print(“Key ‘address’ exists”) # This won’t be executed
“`
Q2. Which method is the most efficient for checking key existence in a dictionary?
The ‘in’ operator is generally the most efficient method to check key existence in a dictionary. It provides constant-time average case complexity, which means the execution time does not depend on the size of the dictionary.
Q3. Can I check for the existence of a key in nested dictionaries?
Yes, you can check for the existence of a key in nested dictionaries by repeatedly using the ‘in’ operator or the get() method. Here’s an example:
“`
my_dict = {‘person’: {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}}
if ‘name’ in my_dict[‘person’]:
print(“Key ‘name’ exists”)
“`
Q4. What is the difference between the ‘in’ operator and the get() method in terms of key existence checking?
The ‘in’ operator returns a boolean value indicating whether the key exists or not, while the get() method returns the value associated with the key, or a default value if the key does not exist.
Q5. Can I check for the existence of a value rather than a key in a dictionary?
Yes, you can check for the existence of a value in a dictionary by using the ‘in’ operator with the values() method. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘John’ in my_dict.values():
print(“Value ‘John’ exists”)
“`
Conclusion
Checking if a key exists in a dictionary is a common operation when working with Python dictionaries. In this article, we explored various methods to accomplish this task, including the ‘in’ operator, the get() method, and the keys() method. Remember to choose the most appropriate method based on your specific requirements and the efficiency considerations of your program.
Python Check Key In Array
Introduction:
Python is a versatile programming language known for its simplicity and readability. It offers a wide range of built-in functions and libraries that make programming easier and more efficient. One of the common tasks in Python is to check if a key exists in an array. In this article, we will explore various techniques to accomplish this task and provide a detailed explanation of each approach.
Checking Key Existence in an Array:
To check if a key exists in an array, we can use the ‘in’ operator. This operator returns True if the key is present in the array and False otherwise. Let’s consider a simple example where we want to check if the key ‘apple’ exists in an array called ‘fruits’.
“`
fruits = [‘apple’, ‘banana’, ‘cherry’]
if ‘apple’ in fruits:
print(“Key ‘apple’ found in the array”)
else:
print(“Key ‘apple’ not found in the array”)
“`
Output:
“`
Key ‘apple’ found in the array
“`
As seen in the example above, the ‘in’ operator can be used to easily check if a key exists in an array. It is a straightforward, concise, and efficient method for this purpose.
Frequently Asked Questions (FAQs):
Q1. Can an array contain duplicate keys?
Yes, an array can contain duplicate keys. However, when checking for key existence, the ‘in’ operator will only return True if at least one occurrence of the key is present in the array. Let’s consider an example:
“`
fruits = [‘apple’, ‘banana’, ‘apple’, ‘cherry’]
if ‘apple’ in fruits:
print(“Key ‘apple’ found in the array”)
else:
print(“Key ‘apple’ not found in the array”)
“`
Output:
“`
Key ‘apple’ found in the array
“`
In this case, the ‘in’ operator returns True even though ‘apple’ appears twice in the array.
Q2. What if I want to check for key existence in a multi-dimensional array?
To check for key existence in a multi-dimensional array, we can use the ‘in’ operator with nested loops. Let’s consider an example where we have a 2D array called ‘matrix’ and we want to check if a specific key exists:
“`
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
key = 2
key_found = False
for row in matrix:
if key in row:
key_found = True
break
if key_found:
print(“Key”, key, “found in the array”)
else:
print(“Key”, key, “not found in the array”)
“`
Output:
“`
Key 2 found in the array
“`
In this example, we iterate over each row of the matrix and use the ‘in’ operator to check if the key exists. If found, we set the ‘key_found’ variable to True and break the loop. Otherwise, the variable remains False.
Q3. Is there any other method to check for key existence besides the ‘in’ operator?
Yes, apart from the ‘in’ operator, we can also use the ‘count()’ method to count the occurrences of a specific key in an array. The method returns the number of occurrences, which can be used to determine if the key exists. Let’s consider an example where we want to check if the key ‘apple’ exists:
“`
fruits = [‘apple’, ‘banana’, ‘cherry’]
if fruits.count(‘apple’) > 0:
print(“Key ‘apple’ found in the array”)
else:
print(“Key ‘apple’ not found in the array”)
“`
Output:
“`
Key ‘apple’ found in the array
“`
In this case, we use the ‘count()’ method to count the occurrences of ‘apple’ in the array. If the count is greater than 0, we conclude that the key exists.
Conclusion:
In Python, checking if a key exists in an array is a common task. We explored the usage of the ‘in’ operator and the ‘count()’ method to accomplish this. Both methods are effective and efficient, depending on the situation. By using these techniques, you can easily determine if a key is present in an array, enabling you to perform subsequent operations accordingly.
If you have any further questions or doubts regarding this topic, please refer to the FAQs section below.
FAQs:
Q1. Can an array contain duplicate keys?
Yes, an array can contain duplicate keys.
Q2. What if I want to check for key existence in a multi-dimensional array?
To check for key existence in a multi-dimensional array, you can use the ‘in’ operator with nested loops.
Q3. Is there any other method to check for key existence besides the ‘in’ operator?
Yes, apart from the ‘in’ operator, you can use the ‘count()’ method to count the occurrences of a specific key in an array.
Images related to the topic python checking if key exists in dictionary
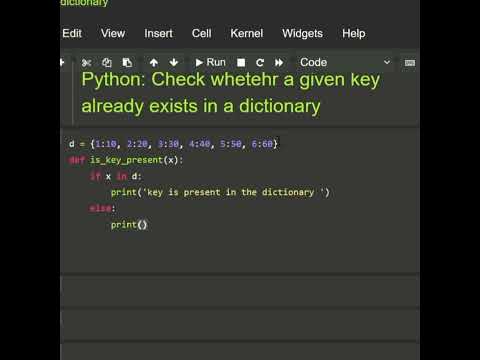
Found 36 images related to python checking if key exists in dictionary theme
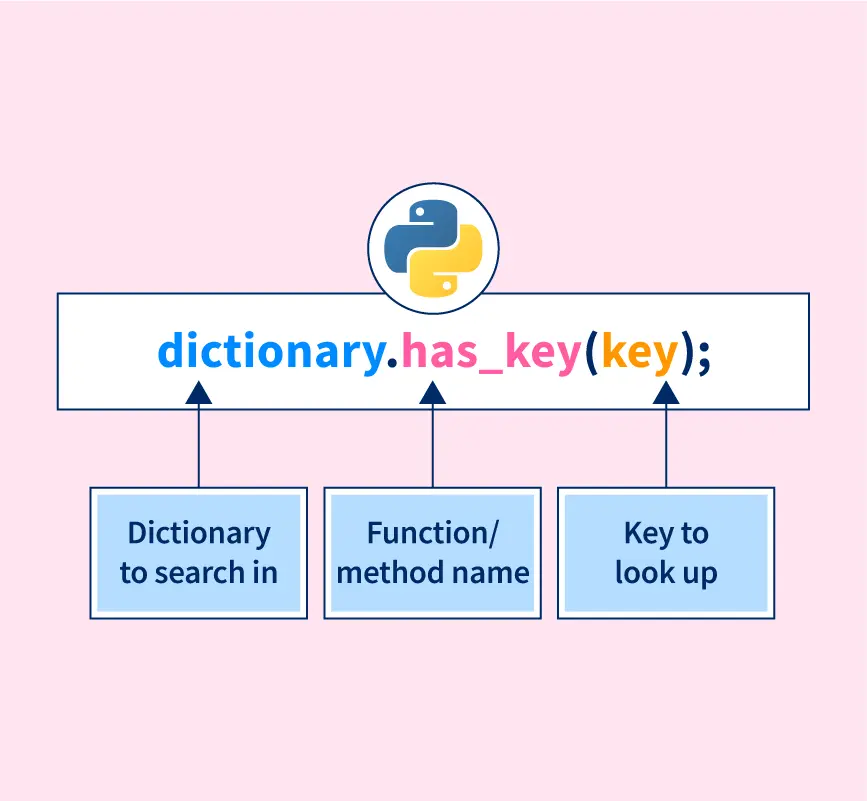
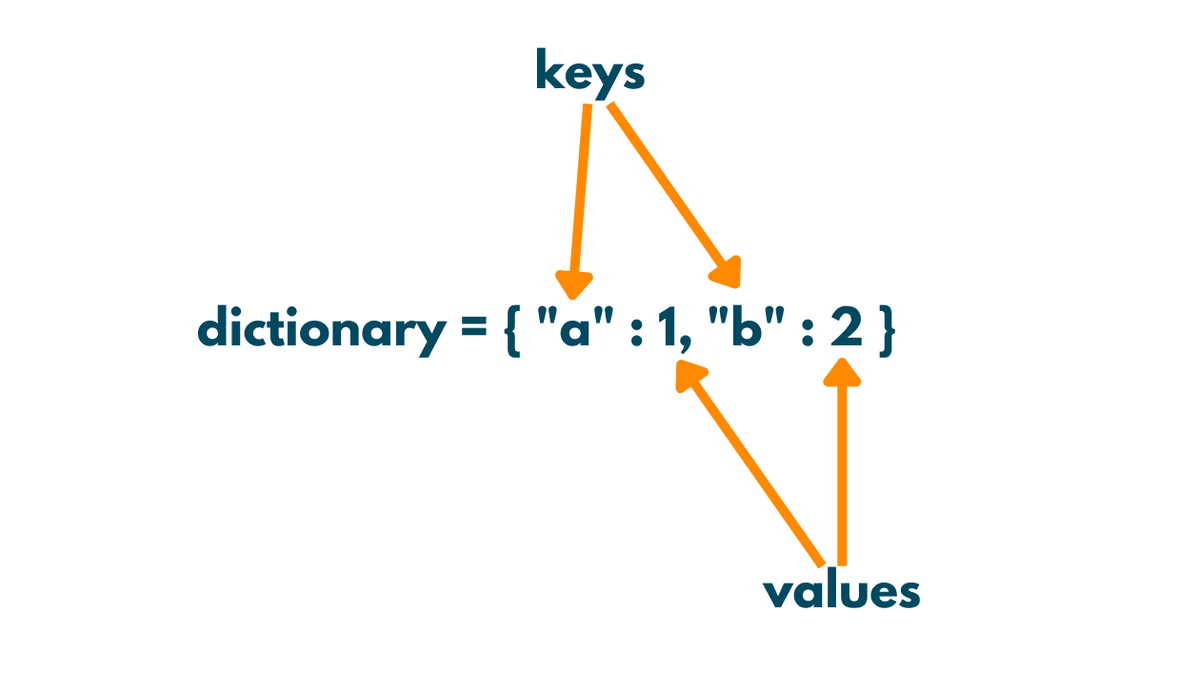
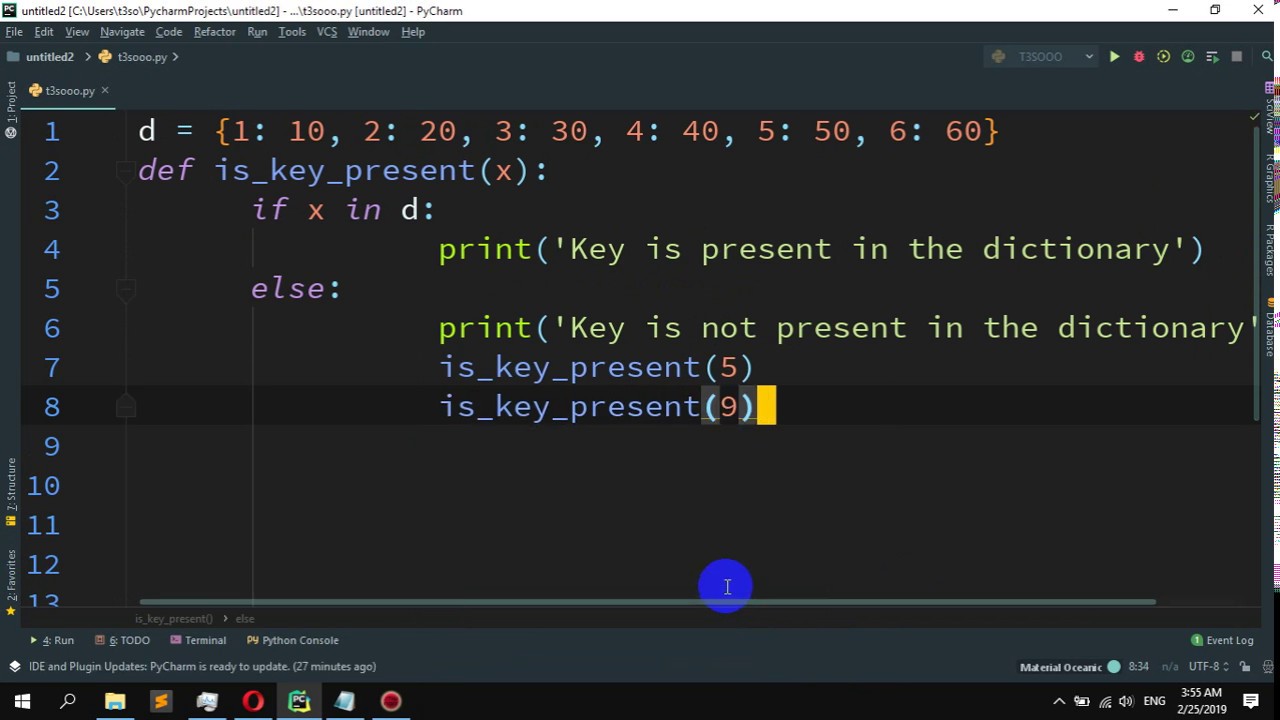
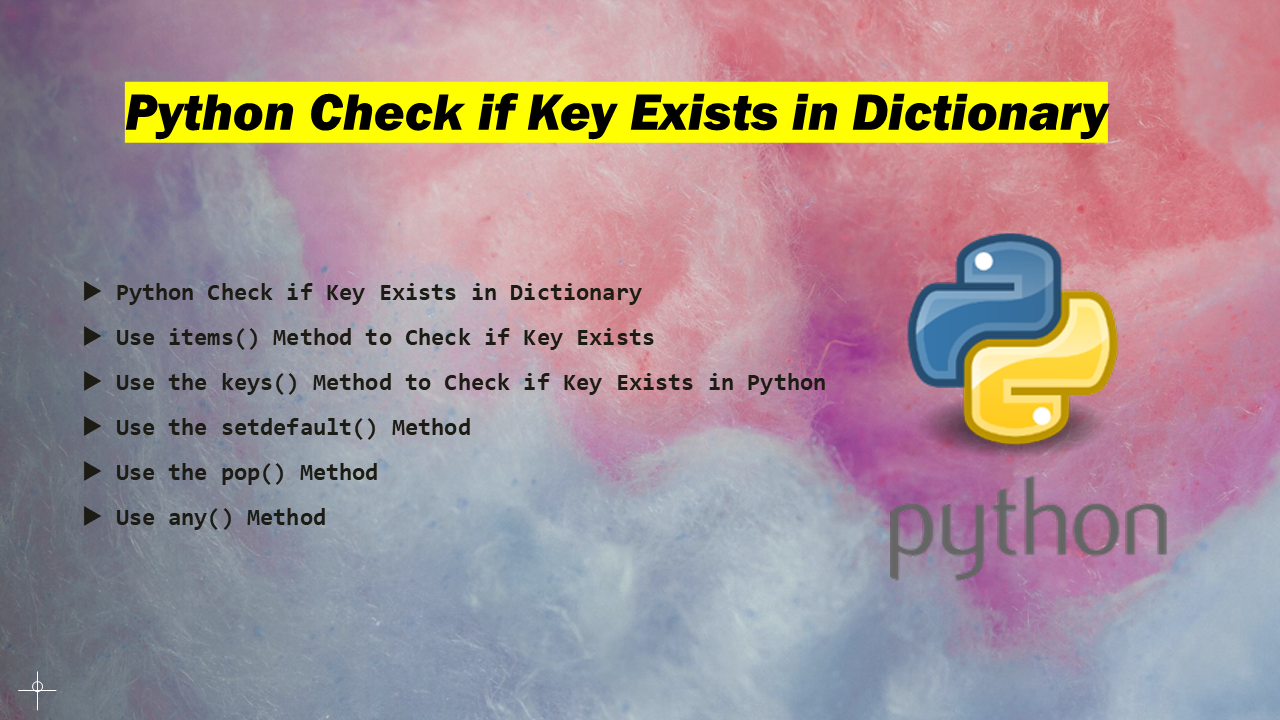

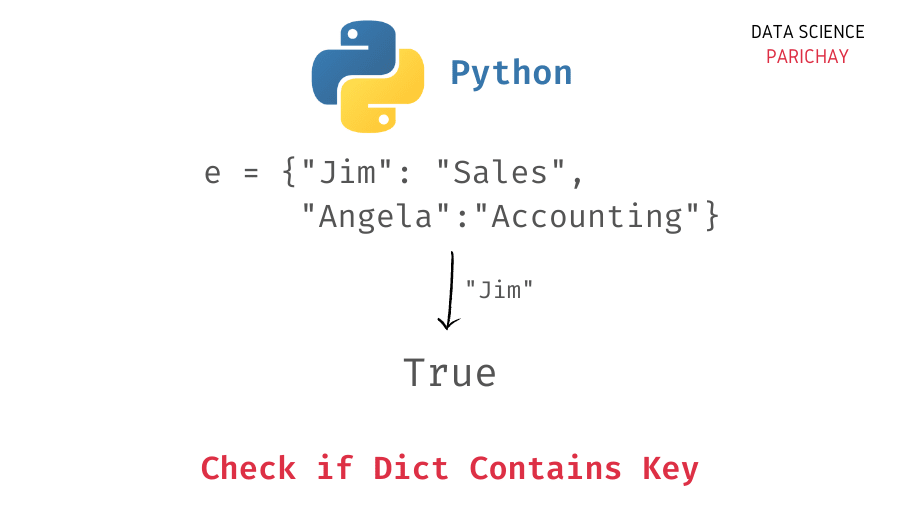
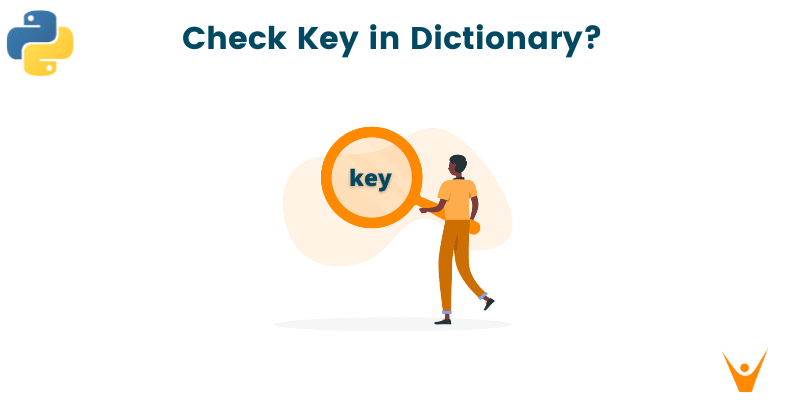
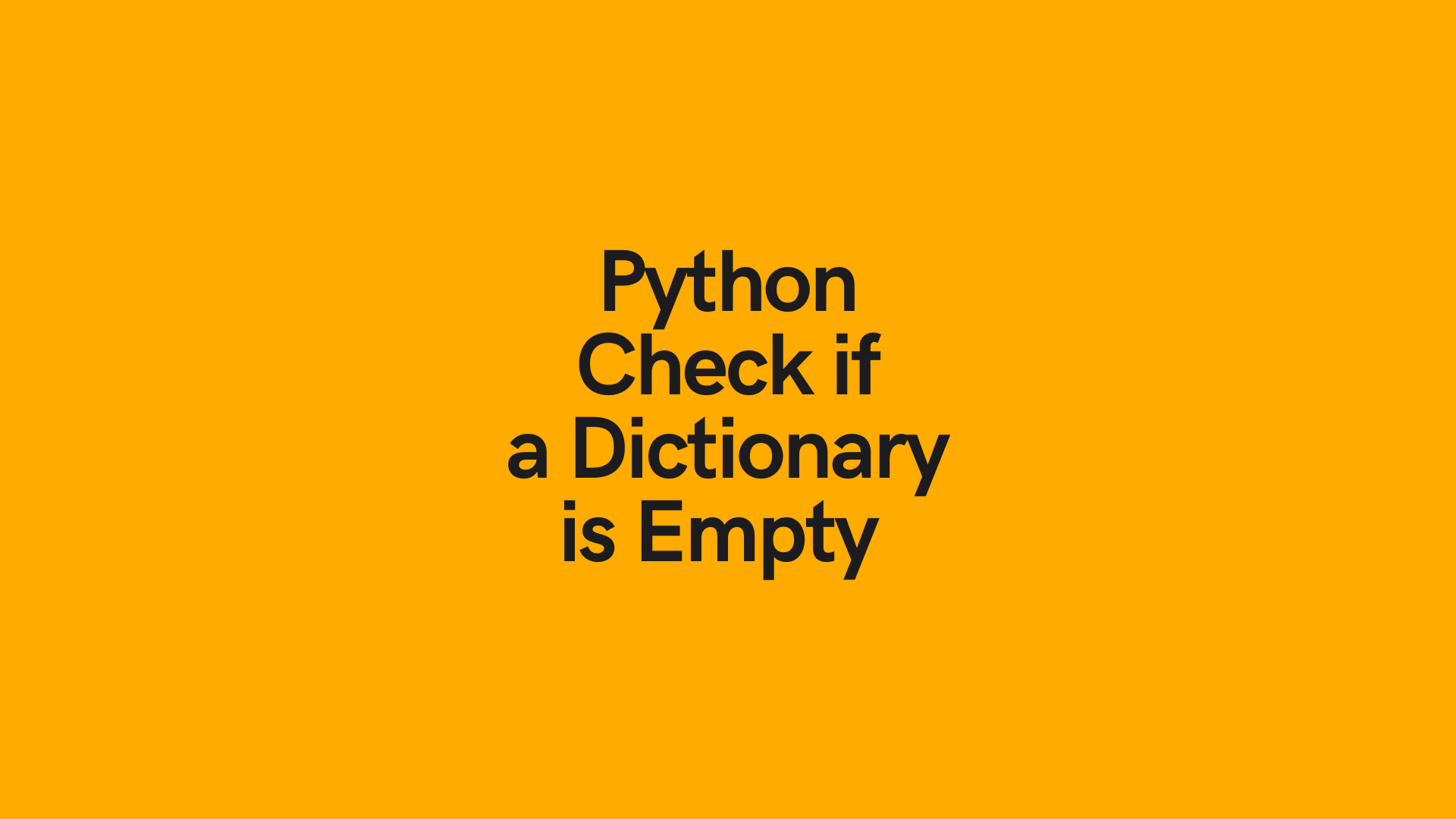

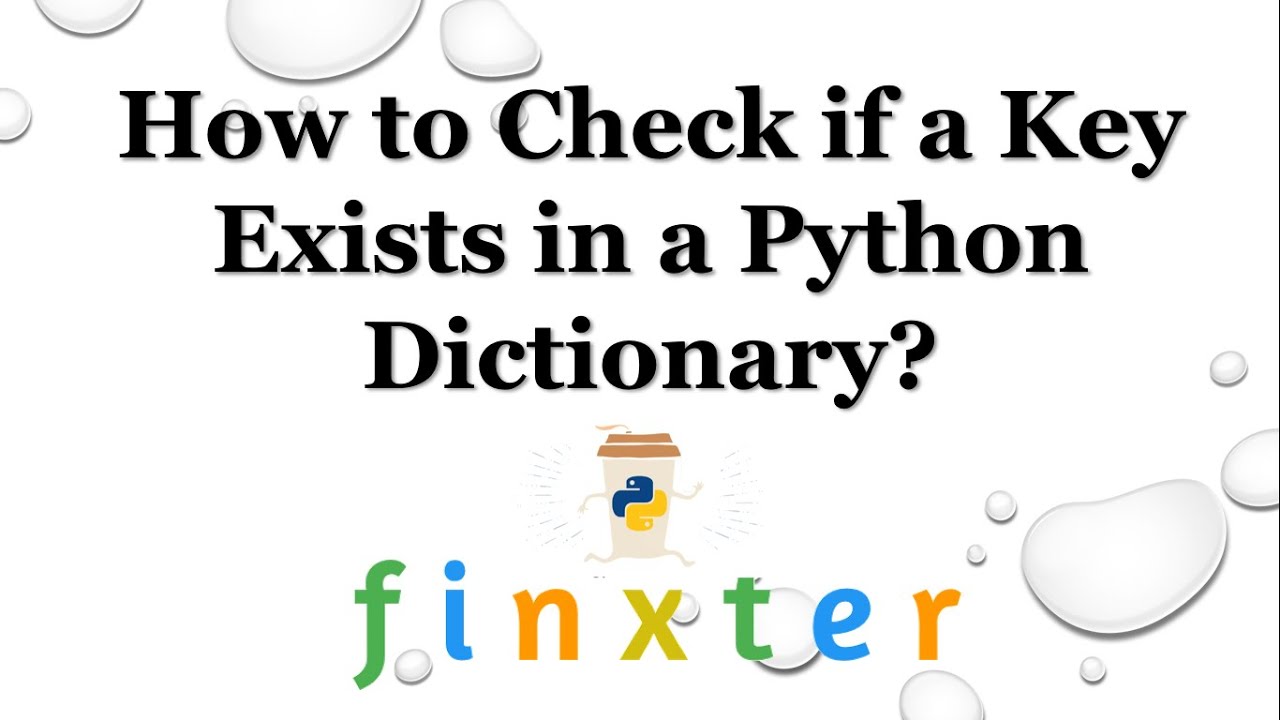
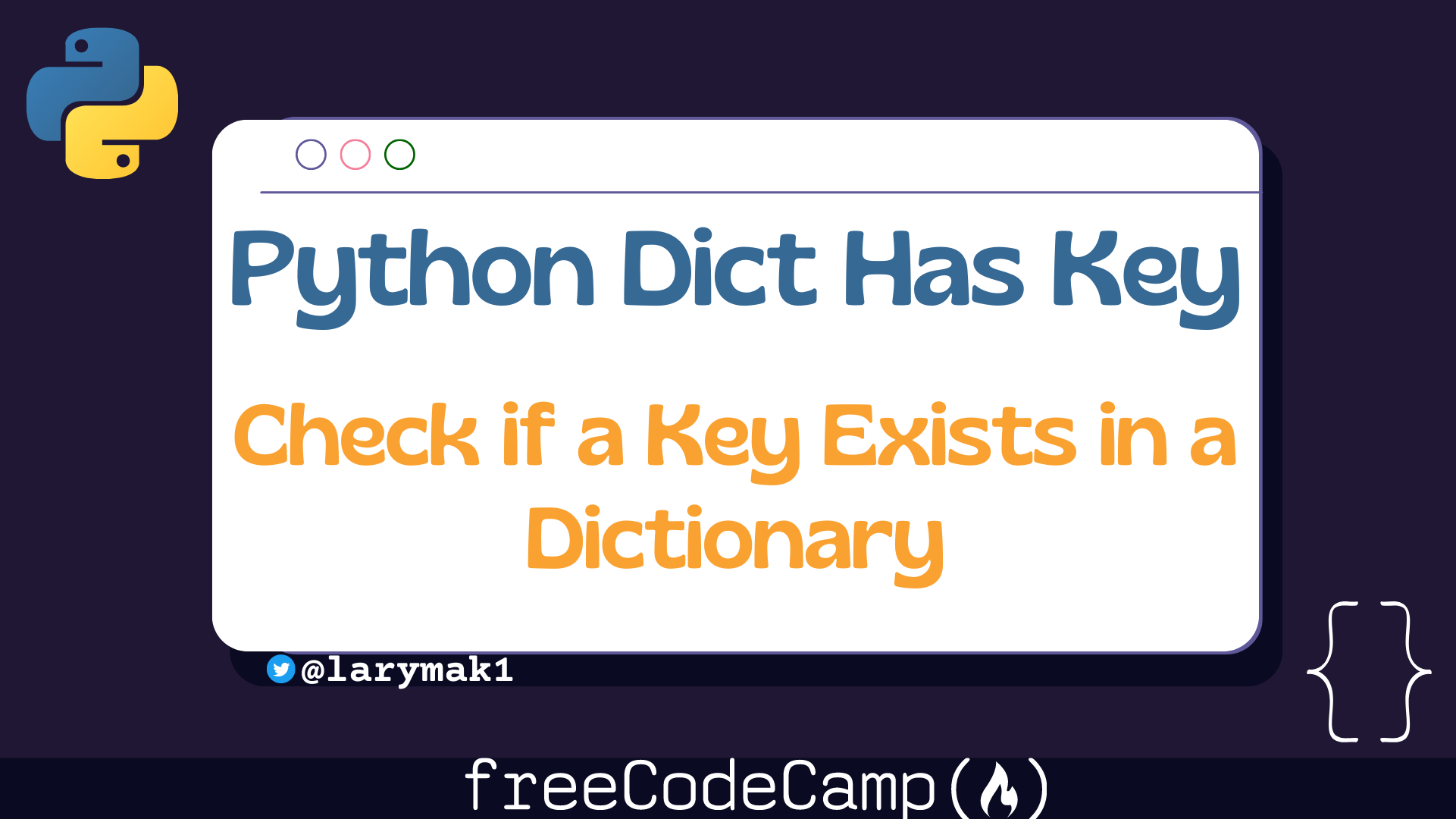
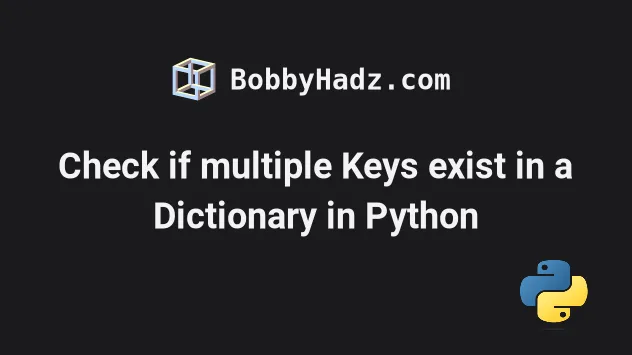


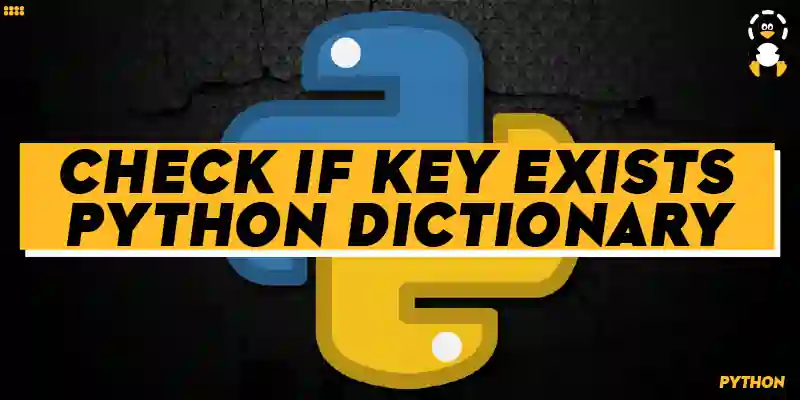


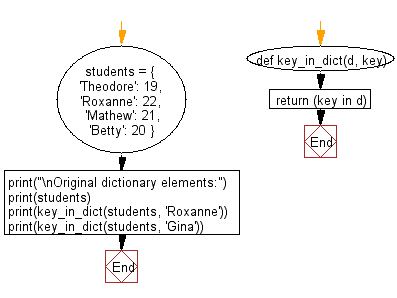

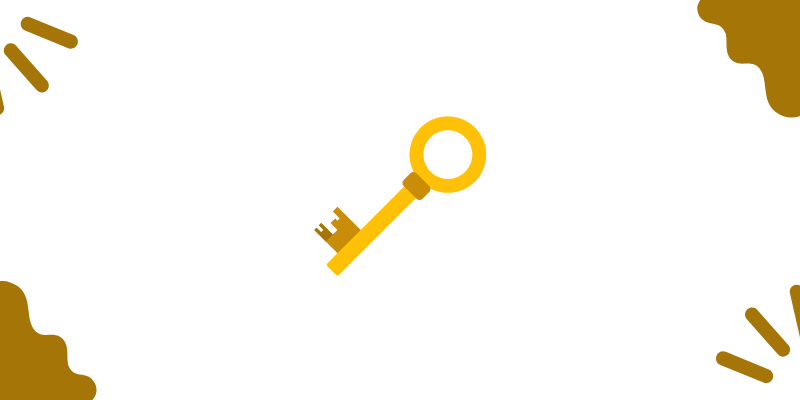
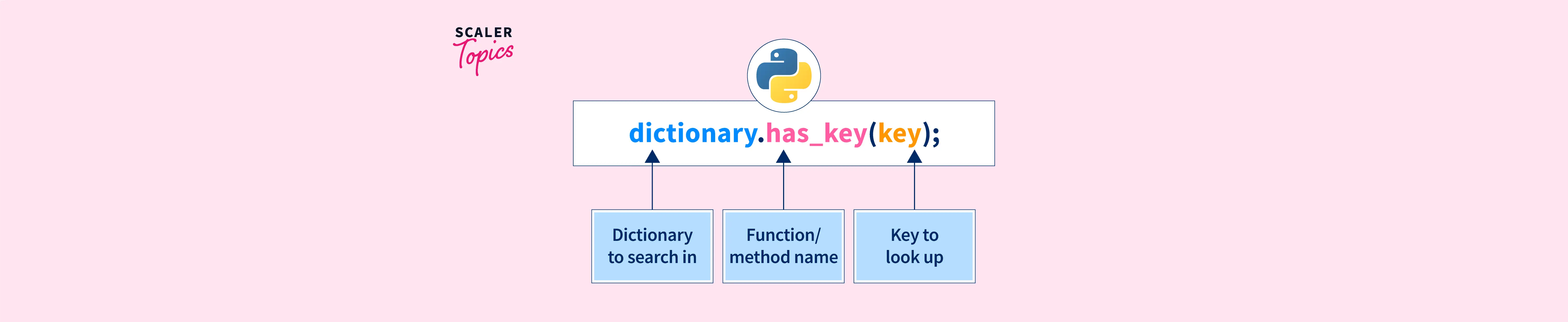
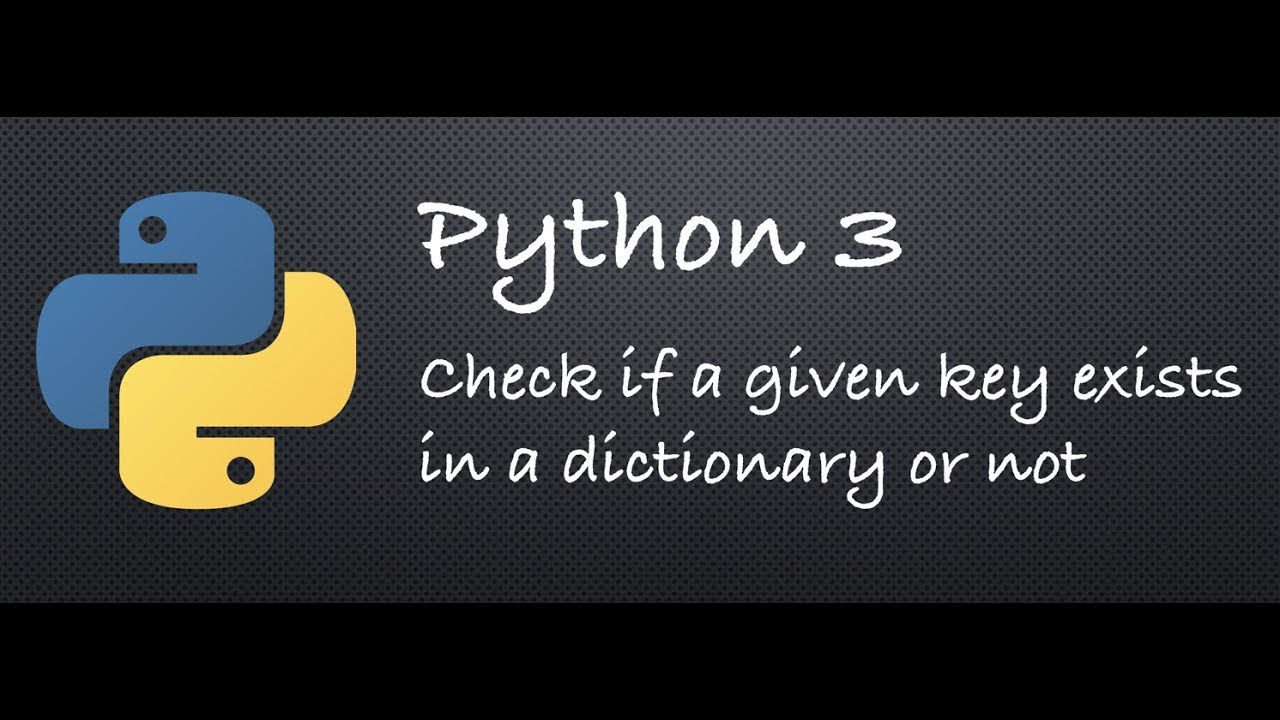
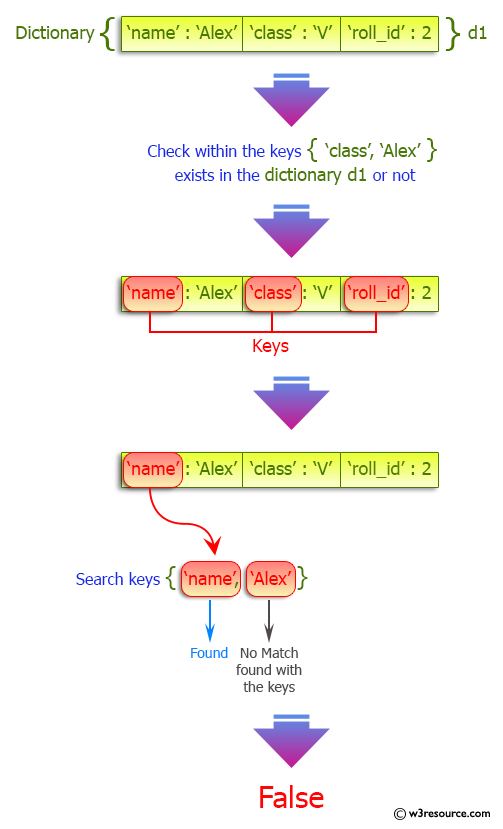

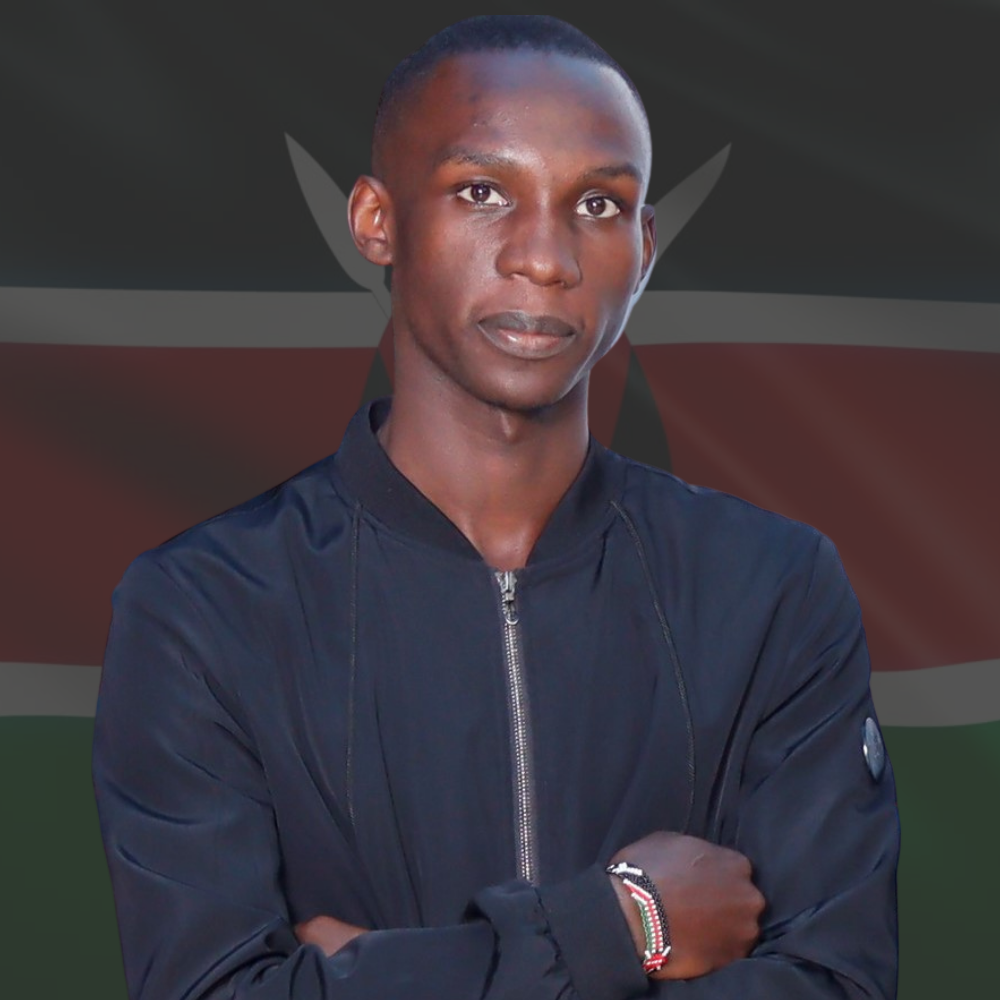
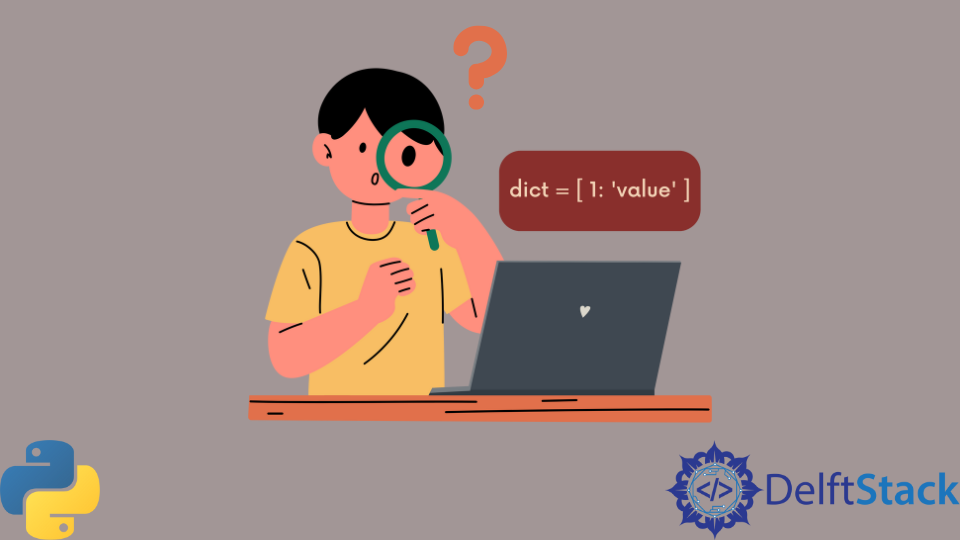
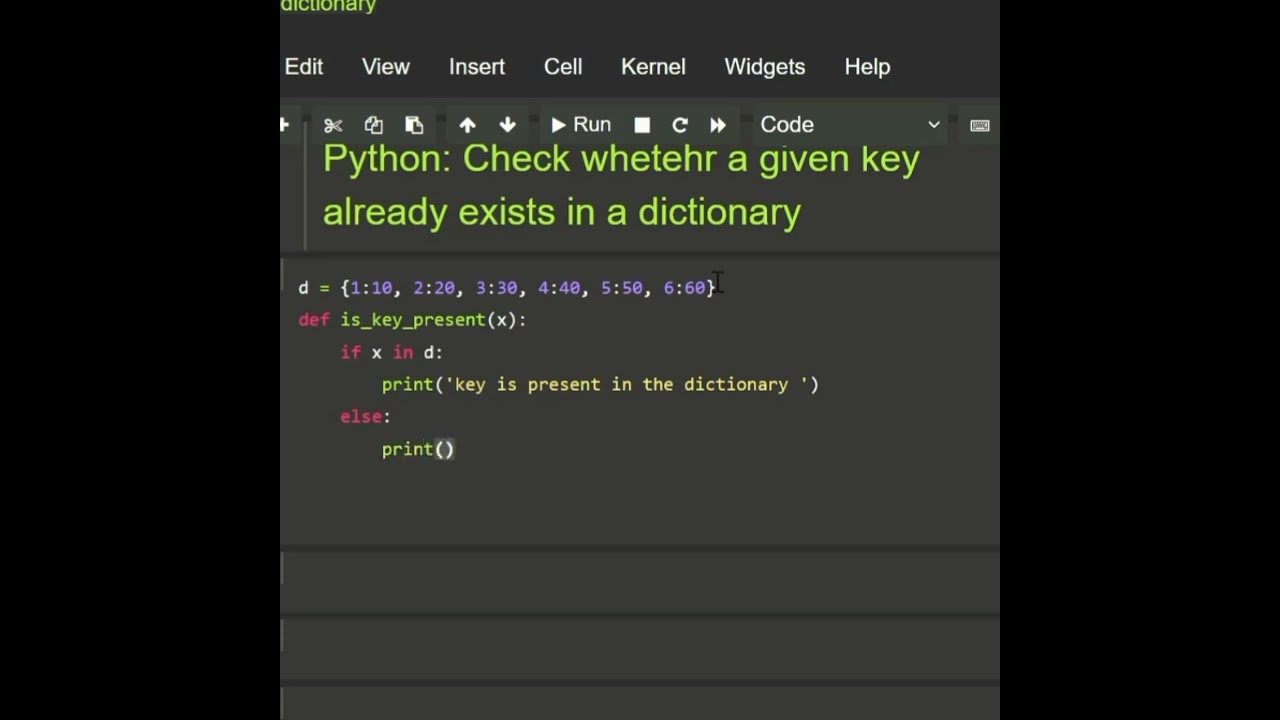

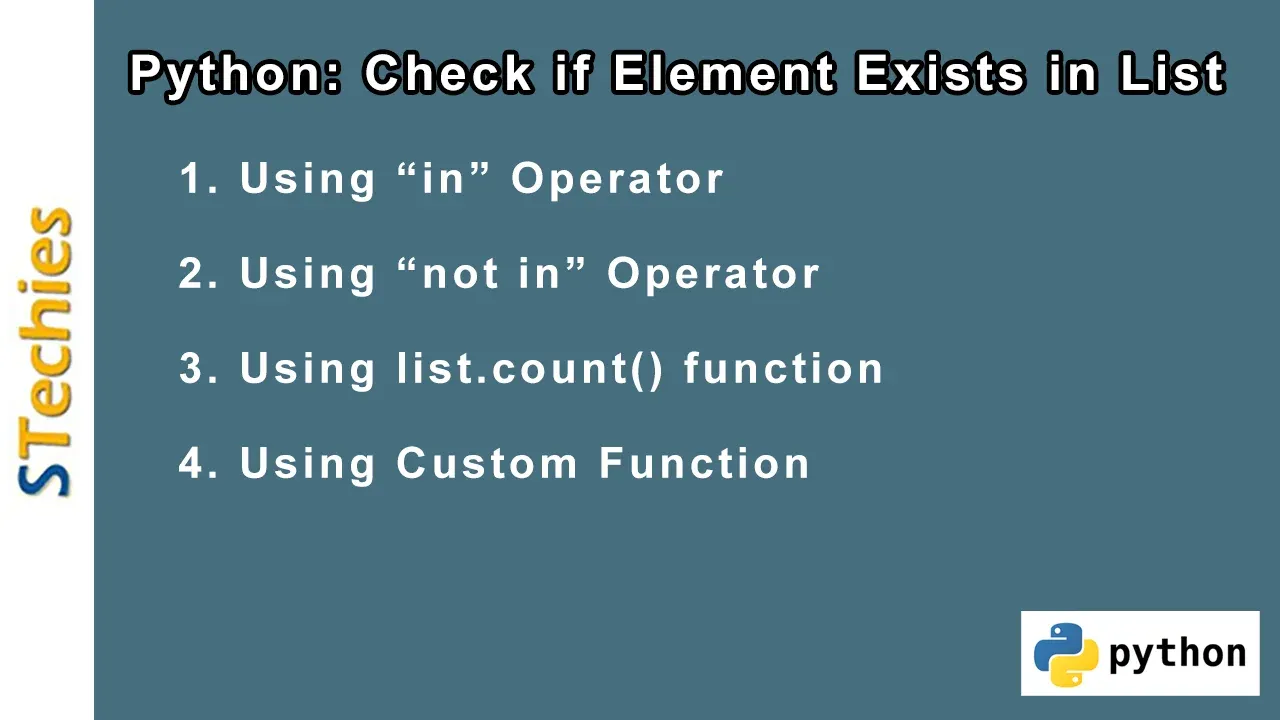
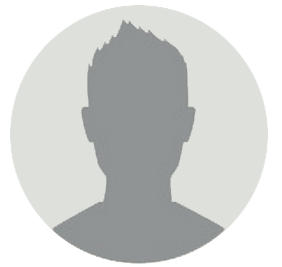
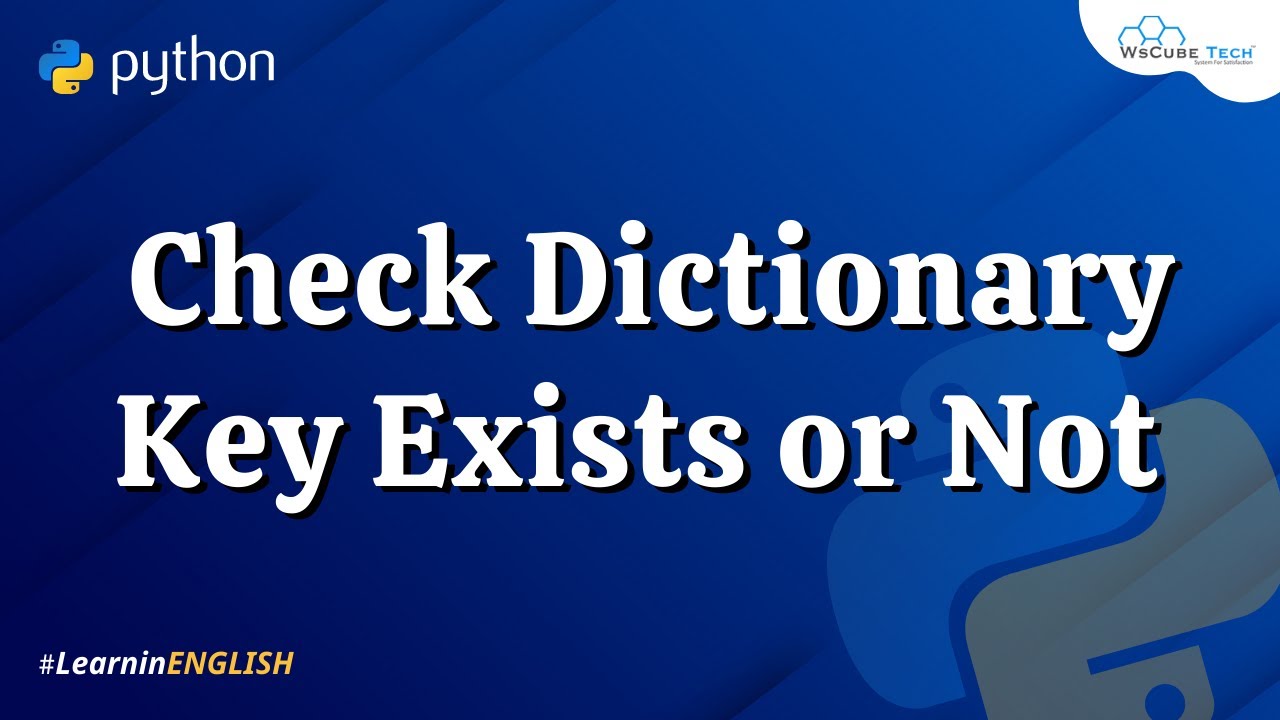

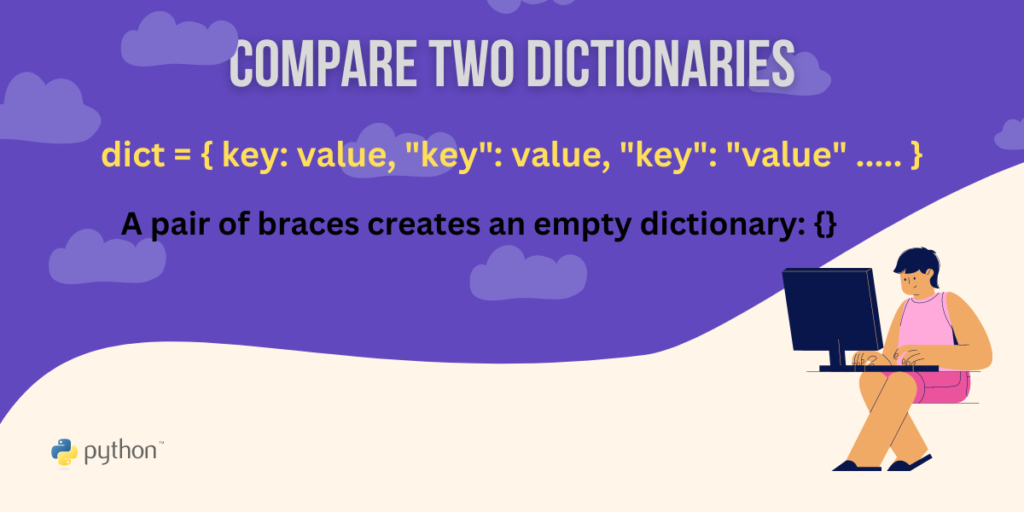


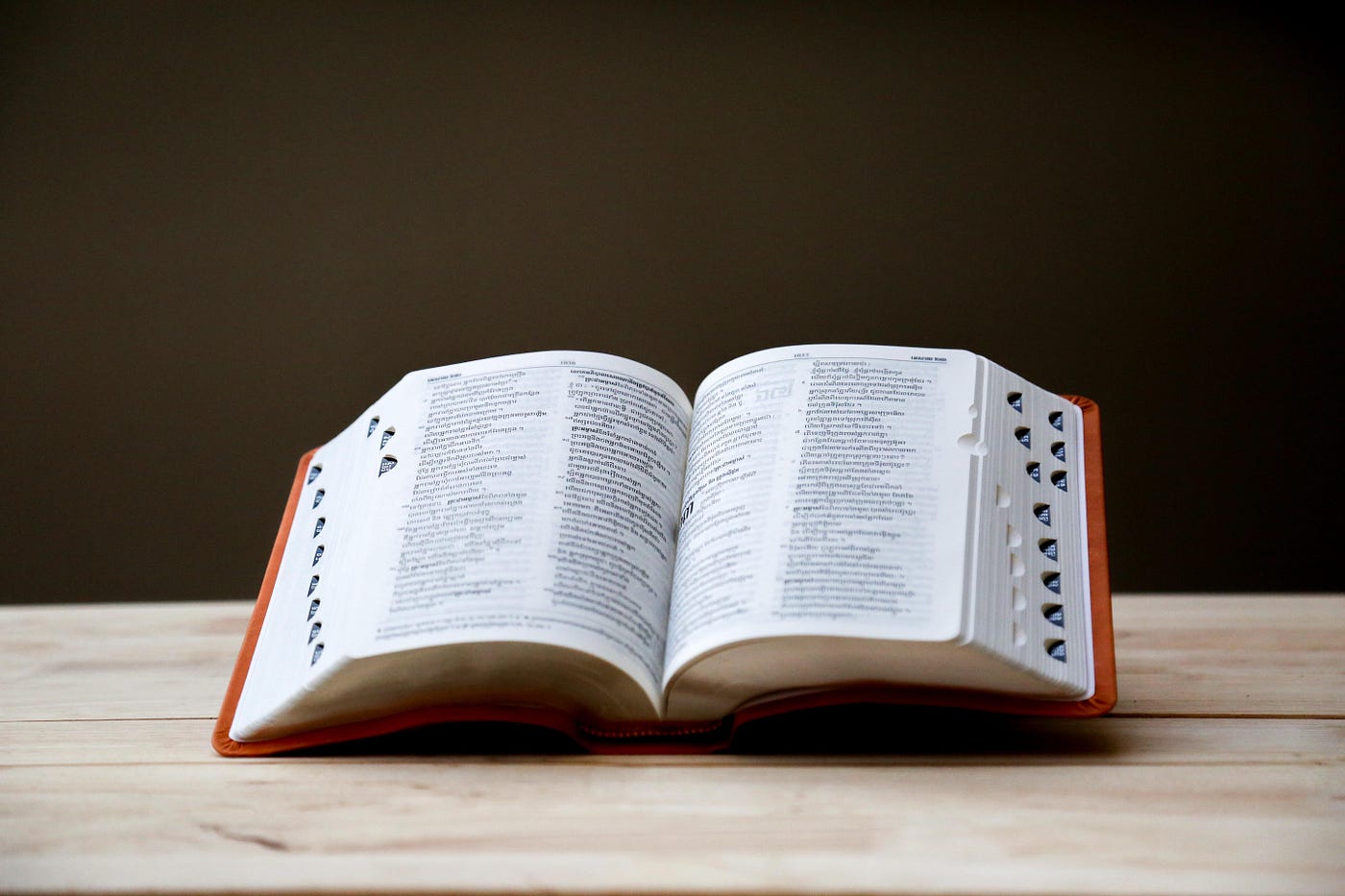
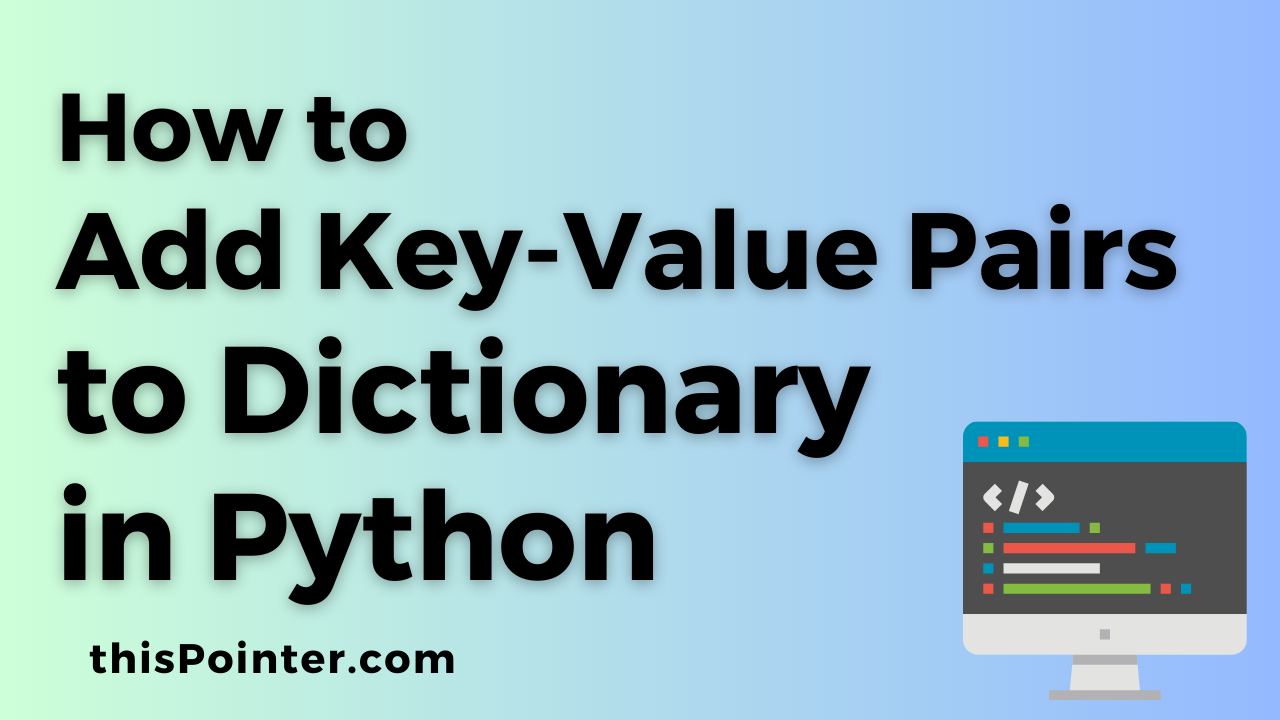
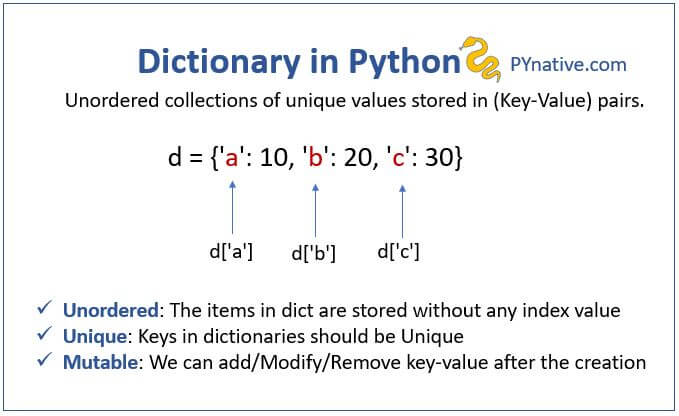
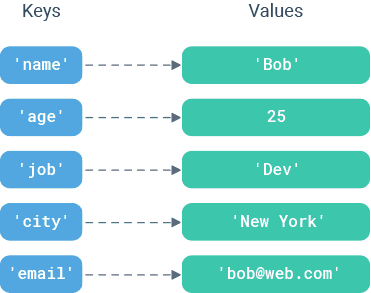
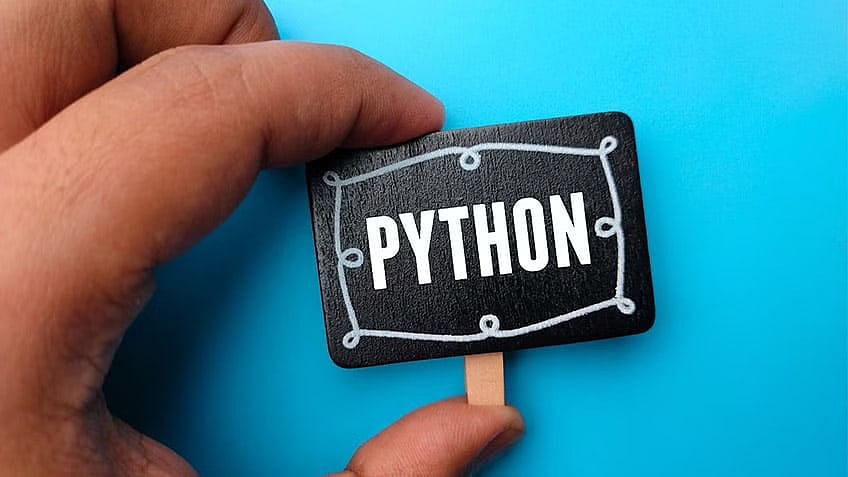

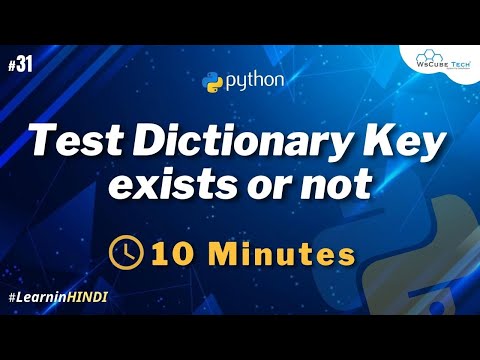
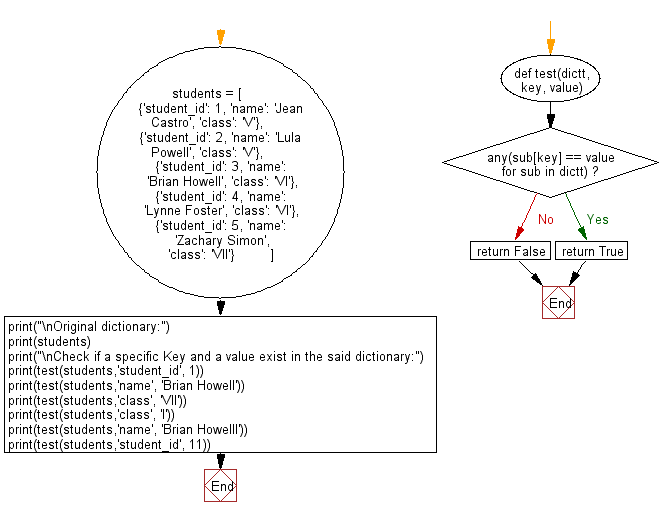
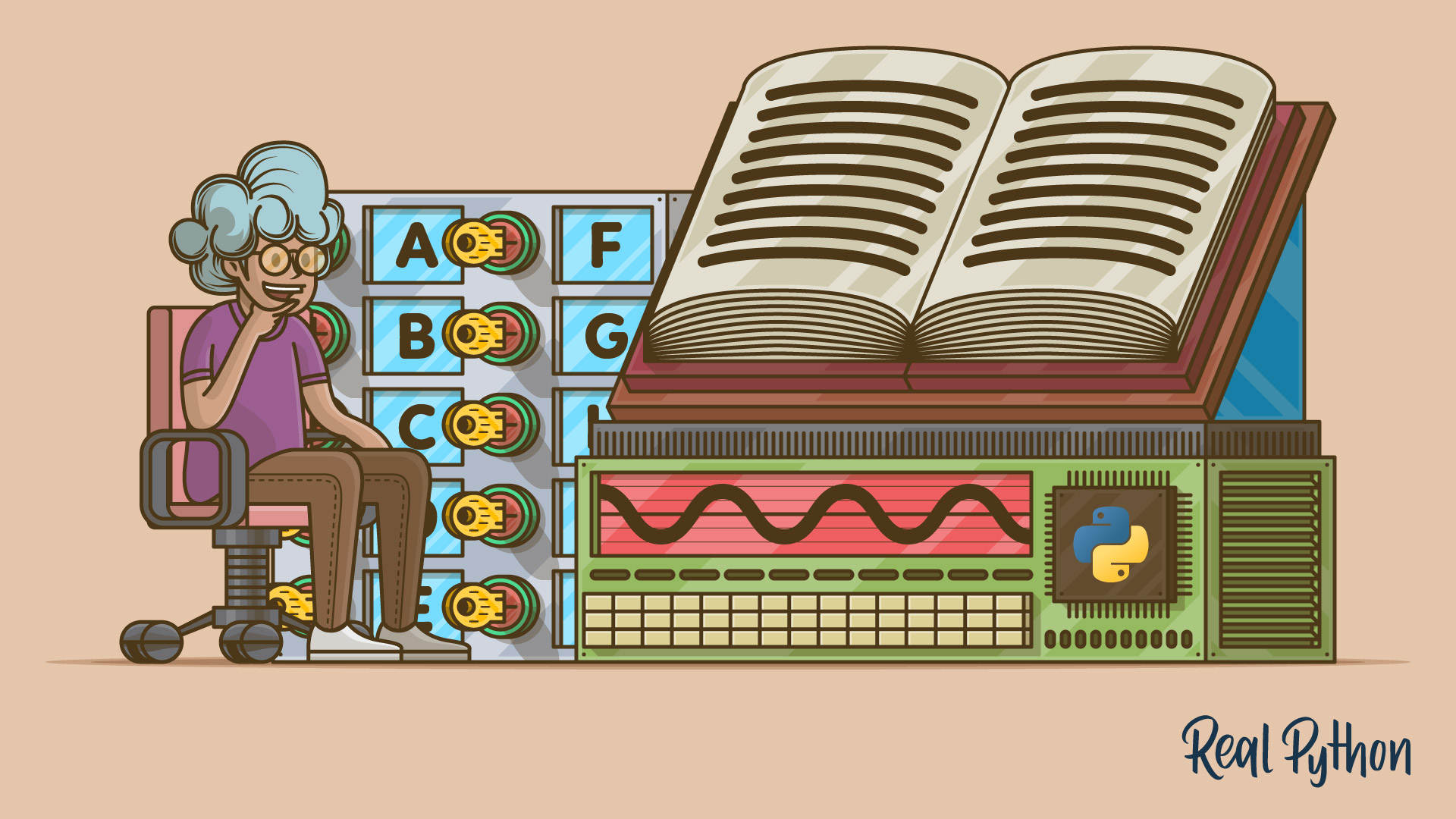
Article link: python checking if key exists in dictionary.
Learn more about the topic python checking if key exists in dictionary.
- Check whether given Key already exists in a Python Dictionary
- Check if a given key already exists in a dictionary
- How to check if key exists in a python dictionary? – Flexiple
- How to check if a key exists in a Python dictionary – Educative.io
- Check if Key Exists in Dictionary Python – Scaler Topics
- Check if Key Exists in Dictionary Python – Scaler Topics
- Check if key/value exists in dictionary in Python – nkmk note
- Python | Check if key has Non-None value in dictionary – GeeksforGeeks
- Python: Check if Key Exists in Dictionary – Stack Abuse
- Check whether given Key already exists in a Python Dictionary
- Check if Key exists in Dictionary (or Value) with Python code
- Python Check if Key Exists in Dictionary – Spark By {Examples}
- How to Check if a Key Exists in a Dictionary in Python
See more: nhanvietluanvan.com/luat-hoc