Python Check If Key Exists In Dictionary
Python dictionaries are versatile and useful data structures that store key-value pairs. One common requirement when working with dictionaries is to check if a specific key exists within the dictionary. In this article, we will explore several methods that Python provides to accomplish this task.
Using the ‘in’ Operator
The simplest way to check if a key exists in a Python dictionary is by using the ‘in’ operator. This operator returns a boolean value, True if the key is present in the dictionary, and False otherwise. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
if ‘age’ in my_dict:
print(“Key ‘age’ exists in the dictionary”)
“`
Using the ‘not in’ Operator
Similarly, the ‘not in’ operator can be used to check if a key does not exist in a dictionary. This operator also returns a boolean value. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
if ‘city’ not in my_dict:
print(“Key ‘city’ does not exist in the dictionary”)
“`
Using the get() Method
The get() method in Python dictionaries provides a safe and elegant way to check if a key exists. It returns the value associated with the key if it exists, and a default value (None by default) if the key is not found. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
name = my_dict.get(‘name’)
if name is not None:
print(f”Key ‘name’ exists in the dictionary with value: {name}”)
“`
Using the keys() Method
The keys() method in Python dictionaries returns a view object that contains all the keys in the dictionary. It provides an easy way to check if a specific key exists by converting the view object into a list and using the ‘in’ operator. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
if ‘name’ in my_dict.keys():
print(“Key ‘name’ exists in the dictionary”)
“`
Using the has_key() Method (Python 2)
In Python 2, dictionaries have a method called has_key() that can be used to check if a key exists. However, this method has been removed in Python 3 due to its redundancy with the ‘in’ operator. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
if my_dict.has_key(‘country’):
print(“Key ‘country’ exists in the dictionary”)
“`
Using a Try-Except Block
Another approach to determining if a key exists in a Python dictionary is by using a try-except block. You can attempt to access the key within a try block and handle the KeyError exception if the key does not exist. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
try:
value = my_dict[‘city’]
print(“Key ‘city’ exists in the dictionary”)
except KeyError:
print(“Key ‘city’ does not exist in the dictionary”)
“`
Using the items() Method
The items() method in Python dictionaries returns a view object that contains all the key-value pairs as tuples. We can leverage this method to check if a key is present in the dictionary along with its associated value. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
for key, value in my_dict.items():
if key == ‘age’:
print(f”Key ‘age’ exists in the dictionary with value: {value}”)
“`
Using the list() Function
Python provides the list() function that can be used to convert the keys of a dictionary into a list. This list can then be used to check if a key exists by using the ‘in’ operator. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
keys_list = list(my_dict)
if ‘country’ in keys_list:
print(“Key ‘country’ exists in the dictionary”)
“`
Check if key not exists in dictionary Python
To check if a key does not exist in a Python dictionary, you can use the ‘not in’ operator. This operator returns True if the key is absent and False if it is present.
Find element in dictionary Python
Finding an element in a Python dictionary refers to checking if a key exists and, if found, accessing its associated value. This can be done using any of the methods mentioned above, such as using the ‘in’ operator or the get() method.
Python check key in array
The concept of an array does not directly apply to dictionaries since they are not ordered collections of elements. However, you can check if a key exists in a dictionary by using any of the methods described earlier, such as using the ‘in’ operator.
Check value in dictionary Python
To check if a value exists in a Python dictionary, you can use the ‘in’ operator along with the values() method. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
if 25 in my_dict.values():
print(“Value 25 exists in the dictionary”)
“`
Add value to key dictionary Python
To add a value to a specific key in a Python dictionary, you can simply assign a value to that key. If the key does not exist, it will be created. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
my_dict[‘city’] = ‘New York’
print(my_dict)
“`
Output:
{‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’, ‘city’: ‘New York’}
Python list key-value
To list the key-value pairs of a Python dictionary, you can use the items() method. It returns a view object containing tuples of the key-value pairs. If needed, you can convert this view object to a list. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
key_value_list = list(my_dict.items())
print(key_value_list)
“`
Output:
[(‘name’, ‘John’), (‘age’, 25), (‘country’, ‘USA’)]
Python check if object has key
In Python, dictionaries are the primary data structure to store key-value pairs. Therefore, if you have an object and you want to check if it has a specific key, you can treat the object as a dictionary and use any of the methods mentioned earlier to check for the key’s existence.
For key-value in dict Pythonpython check if key exists in dictionary
To iterate over the key-value pairs of a Python dictionary and check if a specific key exists, you can use a for loop along with the items() method. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
for key, value in my_dict.items():
if key == ‘age’:
print(f”Key ‘age’ exists in the dictionary with value: {value}”)
“`
In conclusion, Python provides several methods to check if a key exists in a dictionary. Choose the method that suits your specific use case and take advantage of the flexibility and power that Python dictionaries offer.
FAQs:
Q: Can I use multiple methods to check if a key exists in a dictionary?
A: Yes, you can combine different methods based on your requirements. For example, you can use the ‘in’ operator and the get() method together to first check if a key exists and then retrieve its value.
Q: What happens if I try to access a key that does not exist in a dictionary?
A: If you try to access a key that does not exist in a dictionary using the square bracket notation (e.g., my_dict[‘key’]), a KeyError exception will be raised. To handle this situation, you can use a try-except block or the get() method.
Q: Which method is the most recommended for checking if a key exists in a dictionary?
A: The ‘in’ operator is the most commonly used method for checking key existence as it is concise and efficient. However, the choice of method depends on the specific use case and your coding style.
Q: Are dictionaries the only Python data structure that supports key-value pairs?
A: No, besides dictionaries, Python also provides the OrderedDict class in the collections module, which is similar to a dictionary but maintains the order of insertion.
Q: Can I use these methods to check if a value exists in a dictionary?
A: Yes, you can use some of these methods, such as the ‘in’ operator with the values() method, to check if a value exists in a dictionary.
Python: Check If Value Exists In Dictionary
Keywords searched by users: python check if key exists in dictionary Check if key not exists in dictionary Python, Find element in dictionary Python, Python check key in array, Check value in dictionary Python, Add value to key dictionary Python, Python list key-value, Python check if object has key, For key-value in dict Python
Categories: Top 49 Python Check If Key Exists In Dictionary
See more here: nhanvietluanvan.com
Check If Key Not Exists In Dictionary Python
In Python, a dictionary is an unordered collection of key-value pairs. Each key is linked to a value, allowing for efficient searching and retrieval of data. However, there may be instances where you need to check if a key does not exist in a dictionary. In this article, we will explore different methods to accomplish this task.
Method 1: Using the ‘in’ keyword
Python provides the ‘in’ keyword, which allows us to check if a key is present in a dictionary. By using the ‘not’ keyword in conjunction with ‘in’, we can negate the condition and check if a key does not exist. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
if ‘address’ not in my_dict:
print(“Key does not exist in the dictionary”)
“`
In this example, we are checking if the key ‘address’ does not exist in the ‘my_dict’ dictionary. If the condition evaluates to True, we print a message indicating that the key does not exist.
Method 2: Using the dictionary’s ‘get()’ method
Python dictionaries provide a ‘get()’ method, which allows us to retrieve the value associated with a specified key. If the key does not exist, the ‘get()’ method returns a default value, which can be specified as an argument. By checking if the returned value is None, we can determine if the key is not present in the dictionary. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
if my_dict.get(‘address’) is None:
print(“Key does not exist in the dictionary”)
“`
In this example, we are using the ‘get()’ method to retrieve the value associated with the key ‘address’. If the returned value is None, we conclude that the key does not exist in the dictionary.
Method 3: Using a try-except block
Python allows us to handle exceptions using the try-except block. We can use this approach to check if a KeyError is raised when trying to access a non-existent key in a dictionary. By catching the exception, we can determine that the key does not exist. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘country’: ‘USA’}
try:
value = my_dict[‘address’]
except KeyError:
print(“Key does not exist in the dictionary”)
“`
In this example, we are trying to access the value associated with the key ‘address’ in the dictionary. If the key does not exist, a KeyError is raised, and we handle it by printing a message indicating that the key does not exist.
Frequently Asked Questions:
Q1. Why should I check if a key does not exist in a dictionary?
A1. Checking if a key does not exist in a dictionary allows you to handle specific cases where certain keys are crucial for your algorithm or program. By ensuring that a key is present in a dictionary, you can avoid potential errors or inconsistencies in your code.
Q2. Can I use the ‘not in’ keyword to check if a key does not exist in a dictionary?
A2. Yes, you can use the ‘not in’ keyword to achieve the same result as the ‘not’ keyword in conjunction with ‘in’. Both approaches are valid and provide the same outcome.
Q3. What is the difference between using the ‘get()’ method and the try-except block to check if a key does not exist?
A3. Using the ‘get()’ method allows you to provide a default value if the key does not exist, whereas the try-except block does not provide this functionality. The choice between these two methods depends on your specific needs and requirements.
Q4. How can I check if multiple keys do not exist in a dictionary?
A4. You can use any of the methods mentioned above in combination with logical operators, such as ‘and’ and ‘or’, to check for multiple keys simultaneously. For example, you can use the ‘in’ keyword multiple times or use multiple try-except blocks to check for the absence of multiple keys.
In conclusion, checking if a key does not exist in a dictionary is an essential skill when working with Python dictionaries. By using the ‘in’ keyword, the ‘get()’ method, or a try-except block, you can efficiently handle cases where specific keys are crucial for your code. Understanding these methods will enable you to write more robust and error-free programs.
Find Element In Dictionary Python
Python is a versatile and powerful programming language that offers a wide range of functionalities. One of its major strengths lies in its built-in data structures, including dictionaries. In Python, a dictionary is an unordered collection of key-value pairs, where each key is unique. The dictionary allows us to access, update, and manipulate the elements efficiently. In this article, we will explore how to find an element in a dictionary using different approaches and techniques.
Method 1: Using the in Operator
Python provides the in operator, which allows us to check if a key exists in a dictionary. Here’s an example demonstrating its usage:
“`python
# Create a sample dictionary
sample_dict = {‘apple’: 15, ‘banana’: 10, ‘cherry’: 5}
# Find an element in the dictionary
if ‘apple’ in sample_dict:
print(“The element is present in the dictionary.”)
else:
print(“The element is not present in the dictionary.”)
“`
In this example, we created a dictionary called `sample_dict` with three key-value pairs. Using the in operator, we checked if the key `’apple’` is present in the dictionary. If the key is found, it will print “The element is present in the dictionary.” Otherwise, it will print “The element is not present in the dictionary.” This method is simple and efficient while working with dictionaries.
Method 2: Using the get() Method
The get() method in Python allows us to access the value associated with a key in a dictionary. If the key is not found, it returns a default value instead of raising an error. Here’s an example:
“`python
# Create a sample dictionary
sample_dict = {‘apple’: 15, ‘banana’: 10, ‘cherry’: 5}
# Find an element in the dictionary
element = sample_dict.get(‘apple’, ‘Element not found.’)
# Print the result
print(element)
“`
In this example, we used the get() method to find the value associated with the key `’apple’`. Since the key exists in the dictionary, it will return the value `15`. If the key is not found, it will return a default value `’Element not found.’`. The get() method is useful when we want to avoid raising an error when a key is not present in a dictionary.
Method 3: Using a for Loop and Keys
We can also find an element in a dictionary by iterating over its keys using a for loop. Here’s an example:
“`python
# Create a sample dictionary
sample_dict = {‘apple’: 15, ‘banana’: 10, ‘cherry’: 5}
# Find an element in the dictionary
element = ‘apple’
element_found = False
for key in sample_dict.keys():
if key == element:
element_found = True
break
# Print the result
if element_found:
print(“The element is present in the dictionary.”)
else:
print(“The element is not present in the dictionary.”)
“`
In this example, we used a for loop to iterate over the keys in the dictionary. Inside the loop, we checked if a key matches the desired element. If a match is found, we set `element_found` to True and break out of the loop. Finally, we print the result based on the value of `element_found`. Although this method works, it is less efficient compared to the previous approaches.
Method 4: Using the keys() Method and the in Operator
Another way to find an element in a dictionary is by using the keys() method in conjunction with the in operator. Here’s an example:
“`python
# Create a sample dictionary
sample_dict = {‘apple’: 15, ‘banana’: 10, ‘cherry’: 5}
# Find an element in the dictionary
element = ‘banana’
# Check if the element is present
if element in sample_dict.keys():
print(“The element is present in the dictionary.”)
else:
print(“The element is not present in the dictionary.”)
“`
In this example, we first retrieved all the keys in the dictionary using the keys() method. Then, we used the in operator to check if the desired element exists in the retrieved keys. If it’s found, we print “The element is present in the dictionary.” Otherwise, we print “The element is not present in the dictionary.” This method provides a concise way to find an element in a dictionary.
FAQs:
Q1: Can we use the find() method to find elements in a dictionary in Python?
No, the find() method is not applicable for dictionaries in Python. It is primarily used for string manipulation and searching within strings.
Q2: Are dictionaries in Python ordered collections?
No, dictionaries are unordered collections in Python. The elements are stored in no specific order. If order is important, one can use the OrderedDict class from the collections module.
Q3: What happens if we try to access a non-existing key in a dictionary?
If a non-existing key is accessed in a dictionary directly using indexing (e.g., `sample_dict[‘non_existing_key’]`), it will raise a KeyError. However, using the get() method with a non-existing key as the argument will return a default value or None, as specified in the method.
Q4: How can we find the value associated with a key if we know the key’s index in the dictionary?
Since dictionaries are not ordered in Python, we cannot access elements using an index like lists or arrays. To find the value associated with a key, we need to specify the key itself.
Q5: Can we use the values() method to find elements in a dictionary?
The values() method retrieves all the values from a dictionary and not the keys. Therefore, it cannot be directly used to find elements in a dictionary. It is mainly used when we are interested in only the values and not the keys.
In conclusion, Python provides several methods to find elements in a dictionary. We can use the in operator, the get() method, a for loop with keys, or a combination of the keys() method and the in operator. Each method has its own advantages and suitability, depending on the specific requirements of the task at hand. By leveraging these techniques, we can effectively retrieve and manipulate data in dictionaries, making Python a robust choice for handling complex data structures.
Python Check Key In Array
Introduction
Python is a versatile and powerful programming language that offers a wide range of built-in functionalities. One common task in programming is to check whether a specific key or element exists in an array (also known as a list) in Python. In this article, we will delve deep into various methods to accomplish this task, along with detailed explanations and examples.
Table of Contents
1. What is an Array in Python?
2. Checking for a Key in an Array
3. Methods to Check for a Key in an Array
a. Linear Search
b. The “in” Operator
c. List Comprehension
d. The Numpy Library
4. Frequently Asked Questions (FAQs)
5. Conclusion
1. What is an Array in Python?
An array, also called a list, is a collection of items that are stored in a particular order. It allows us to store multiple values of any data type, such as integers, strings, or even other arrays/lists. In Python, arrays can be easily created using square brackets [ ].
Example:
my_array = [1, 2, 3, 4, 5]
print(my_array)
Output: [1, 2, 3, 4, 5]
2. Checking for a Key in an Array
When dealing with arrays, it’s quite common to check if a specific element or key exists in the array before performing any further operations. This ensures that your code doesn’t encounter any unexpected errors due to missing elements. Python provides several approaches to achieve this.
3. Methods to Check for a Key in an Array
a. Linear Search:
The simplest approach to check for a key in an array is to perform a linear search. This involves iterating through each element in the array and comparing it with the desired key. If a match is found, we know that the key exists in the array; otherwise, it does not.
Example:
def linear_search(arr, key):
for element in arr:
if element == key:
return True
return False
my_array = [1, 2, 3, 4, 5]
print(linear_search(my_array, 3))
Output: True
b. The “in” Operator:
Python provides a more elegant and straightforward way to check for a key in an array using the “in” operator. This operator returns True if the key is present in the array; otherwise, it returns False.
Example:
my_array = [1, 2, 3, 4, 5]
print(3 in my_array)
Output: True
c. List Comprehension:
List comprehension is a powerful feature in Python that allows us to create a new list based on another list or iterable. We can utilize this feature to check for a key in an array and return a filtered list containing only the matching elements.
Example:
my_array = [1, 2, 3, 4, 5]
filtered_list = [element for element in my_array if element == 3]
print(len(filtered_list) > 0)
Output: True
d. The Numpy Library:
Numpy is a popular Python library for performing mathematical operations on arrays. Although it is mainly focused on numerical computing, it provides functions that can help check for keys in arrays efficiently. The “in1d” function in the numpy library checks if each element of one array is present in another array and returns a Boolean array.
Example:
import numpy as np
my_array = np.array([1, 2, 3, 4, 5])
print(np.in1d(3, my_array))
Output: True
4. Frequently Asked Questions (FAQs)
Q1. Can we check for a key in a multidimensional array?
Yes, the methods mentioned above can be applied to both one-dimensional and multidimensional arrays. Simply use nested loops to iterate through the elements of each dimension and check for the desired key.
Q2. What happens if we try to check for a key in an empty array?
If you try to check for a key in an empty array, the “in” operator and list comprehension method will return False, while the linear search and numpy library methods will return False or an empty list.
Q3. Are there any time or space complexities associated with these methods?
Yes, each method has its own time and space complexity. The linear search method has a time complexity of O(n), where n is the length of the array. The “in” operator, list comprehension, and numpy library methods also have a time complexity of O(n). However, the numpy library method may have a slightly higher space complexity due to the need for creating a numpy array.
5. Conclusion
Checking for a key in an array is a fundamental task in programming, and Python provides various methods to accomplish it efficiently. In this article, we explored different approaches, such as linear search, the “in” operator, list comprehension, and the numpy library. Each method has its own advantages and limitations, and their appropriateness will depend on the specific requirements of your code. By selecting the most suitable method, you can ensure your code operates smoothly without encountering unexpected errors.
Images related to the topic python check if key exists in dictionary
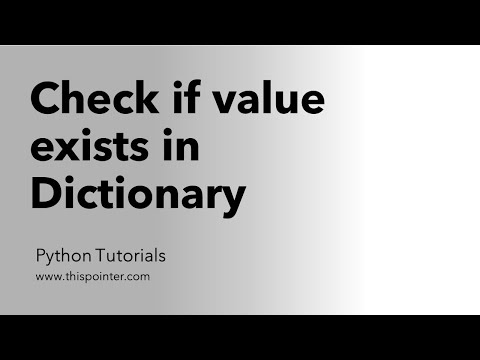
Found 49 images related to python check if key exists in dictionary theme
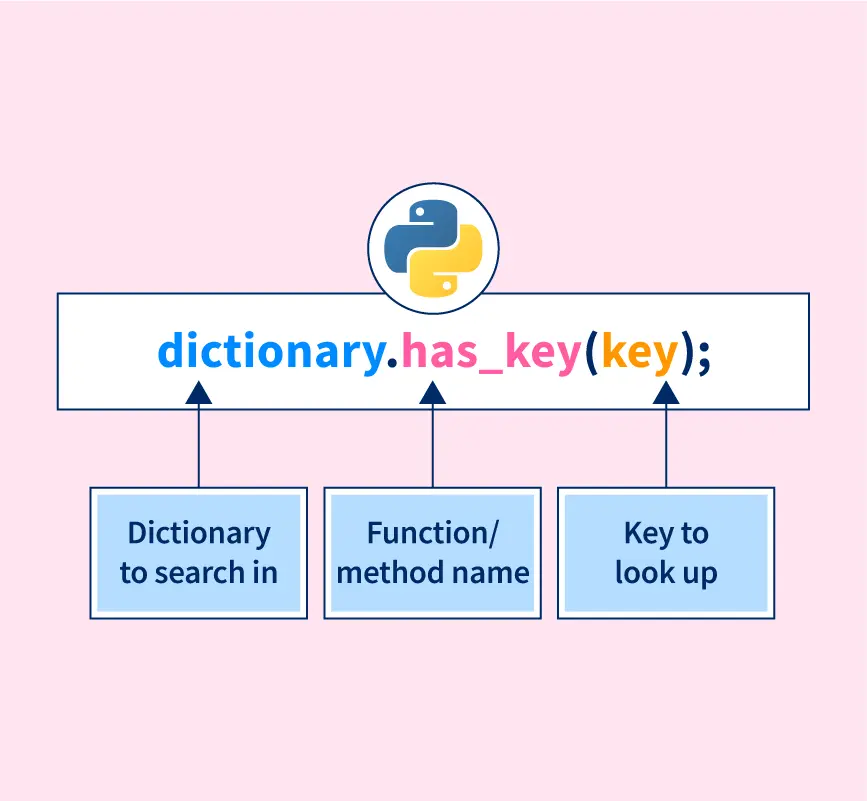
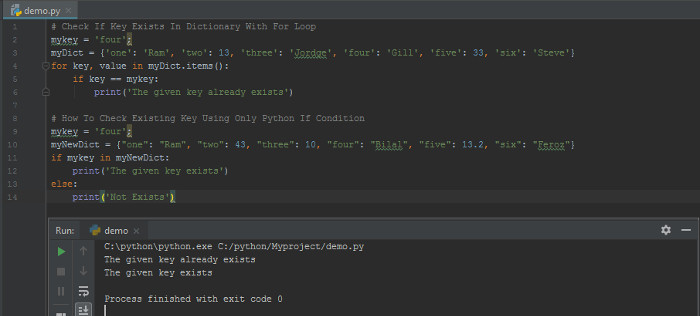
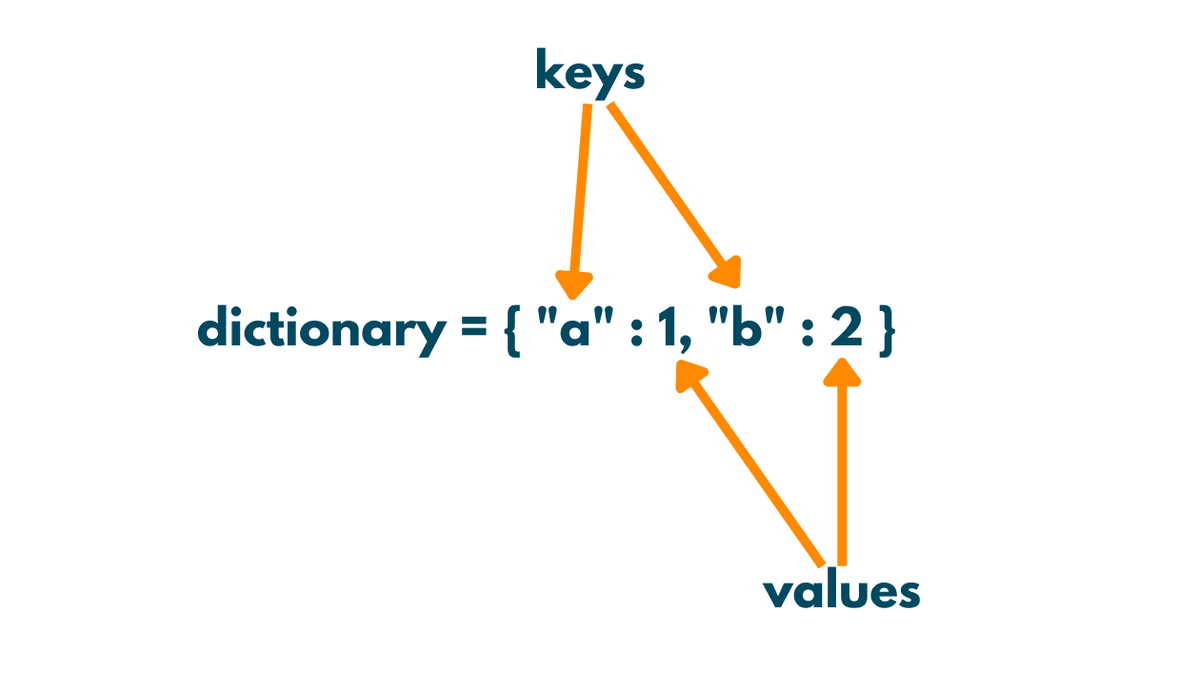
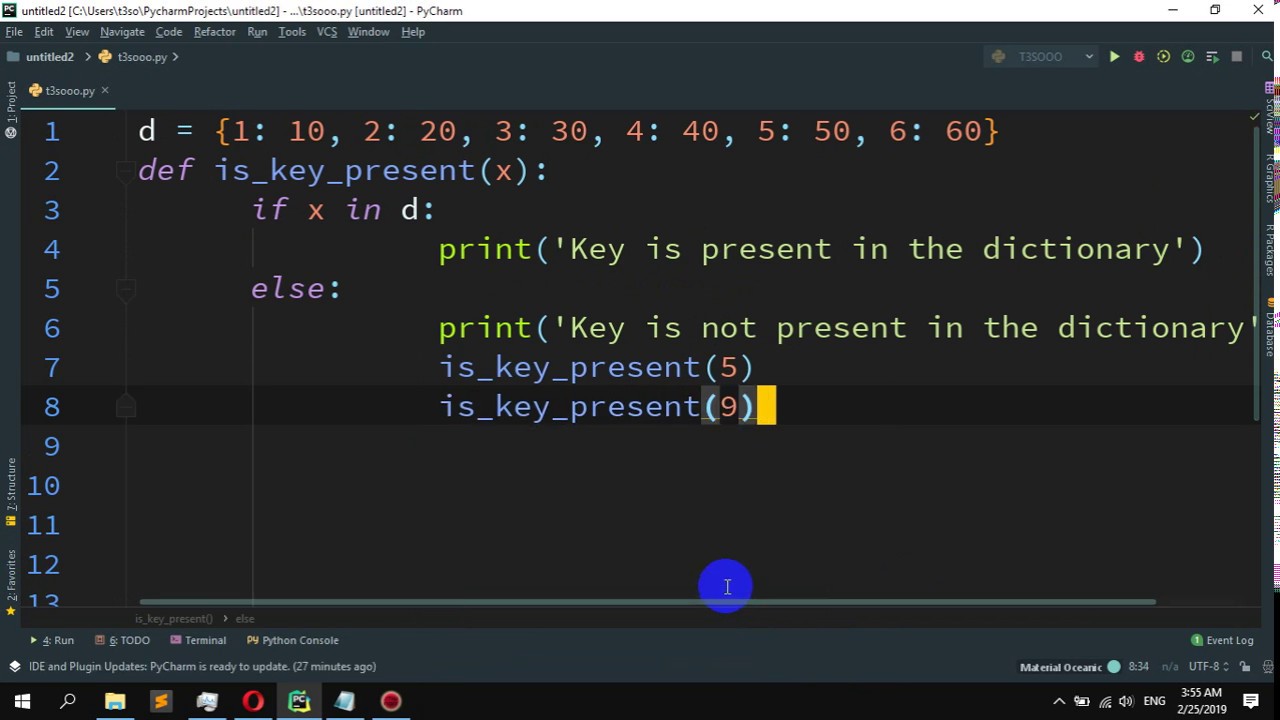

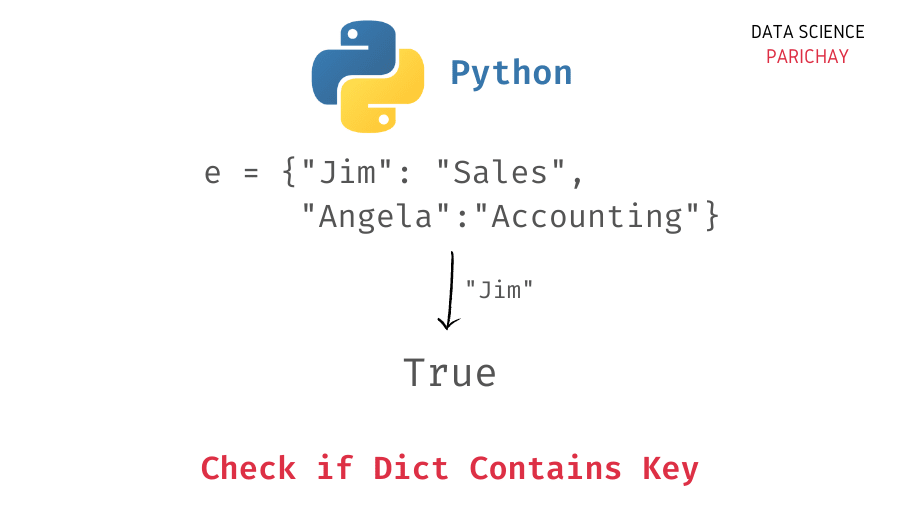
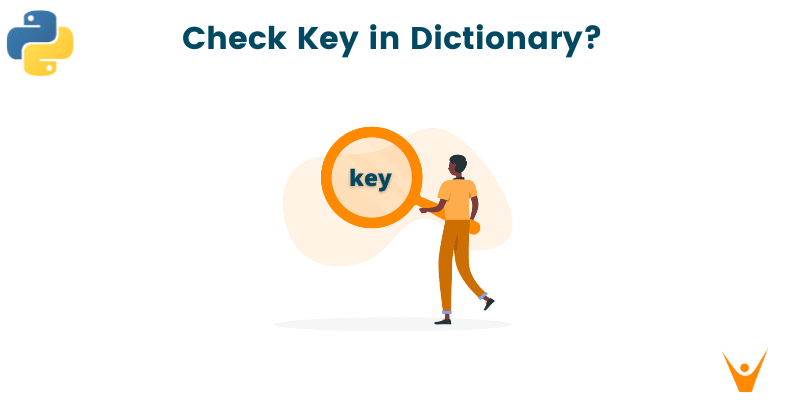
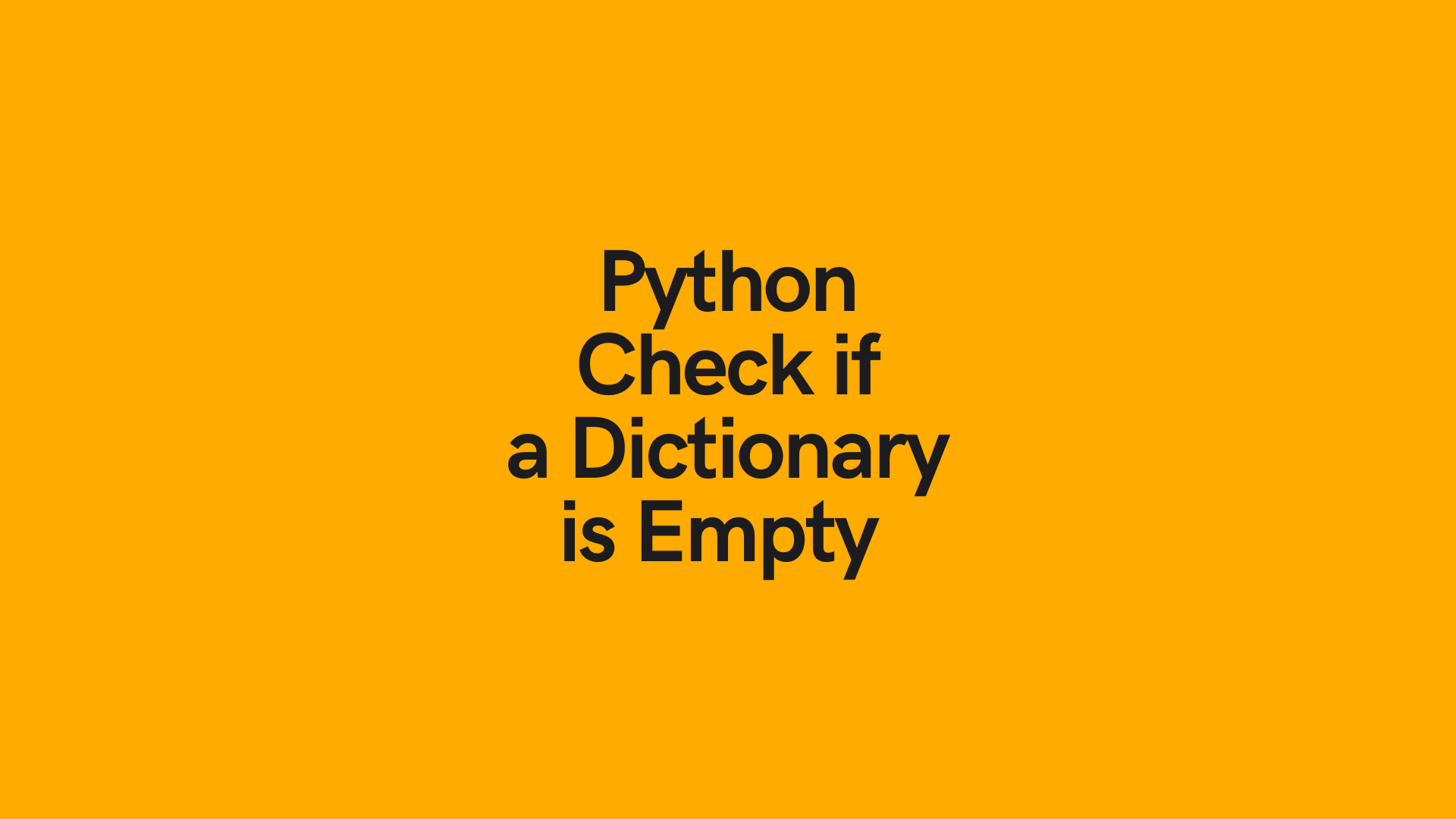

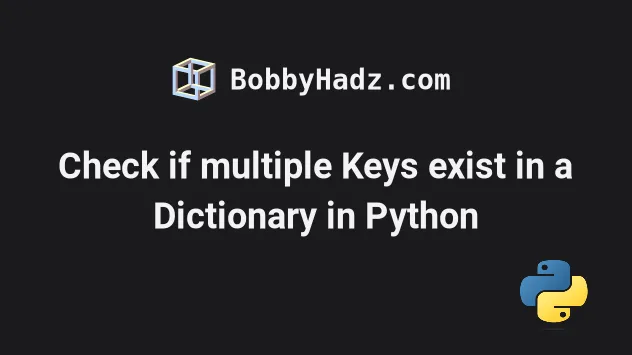
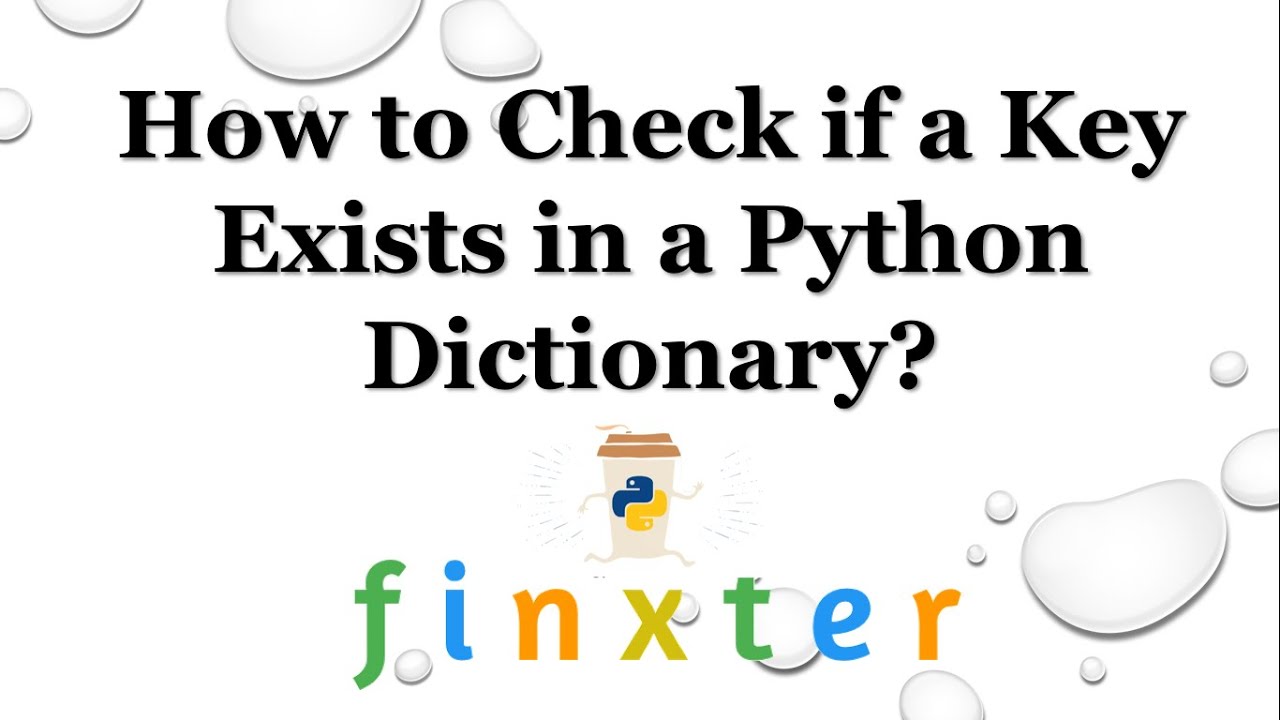


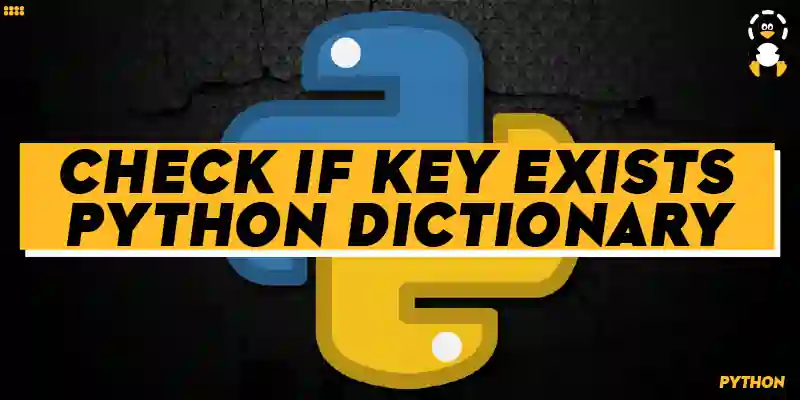
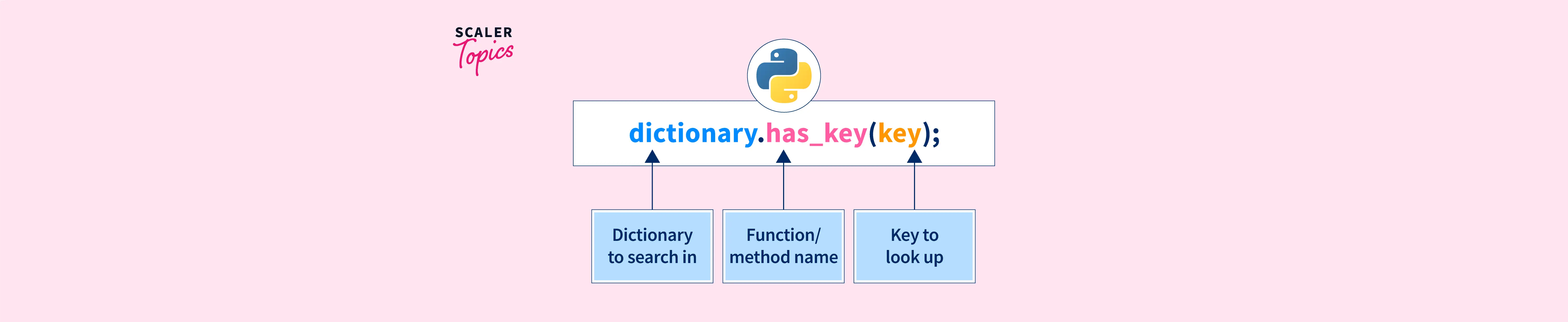



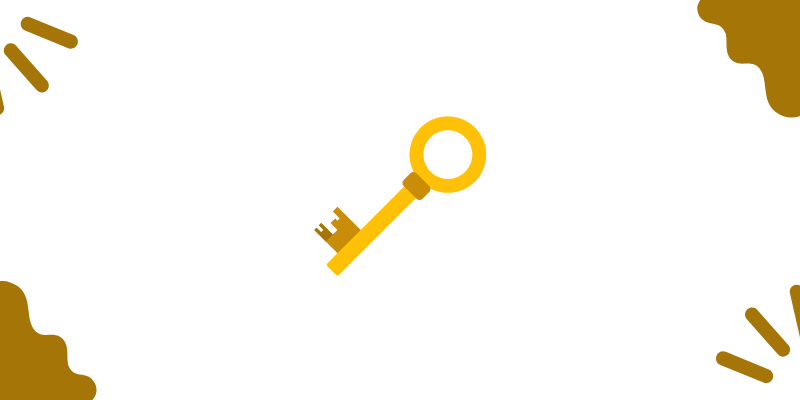
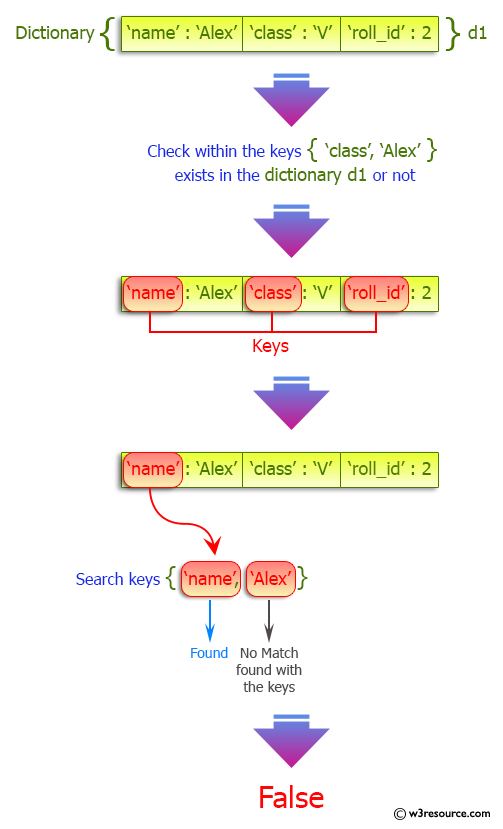
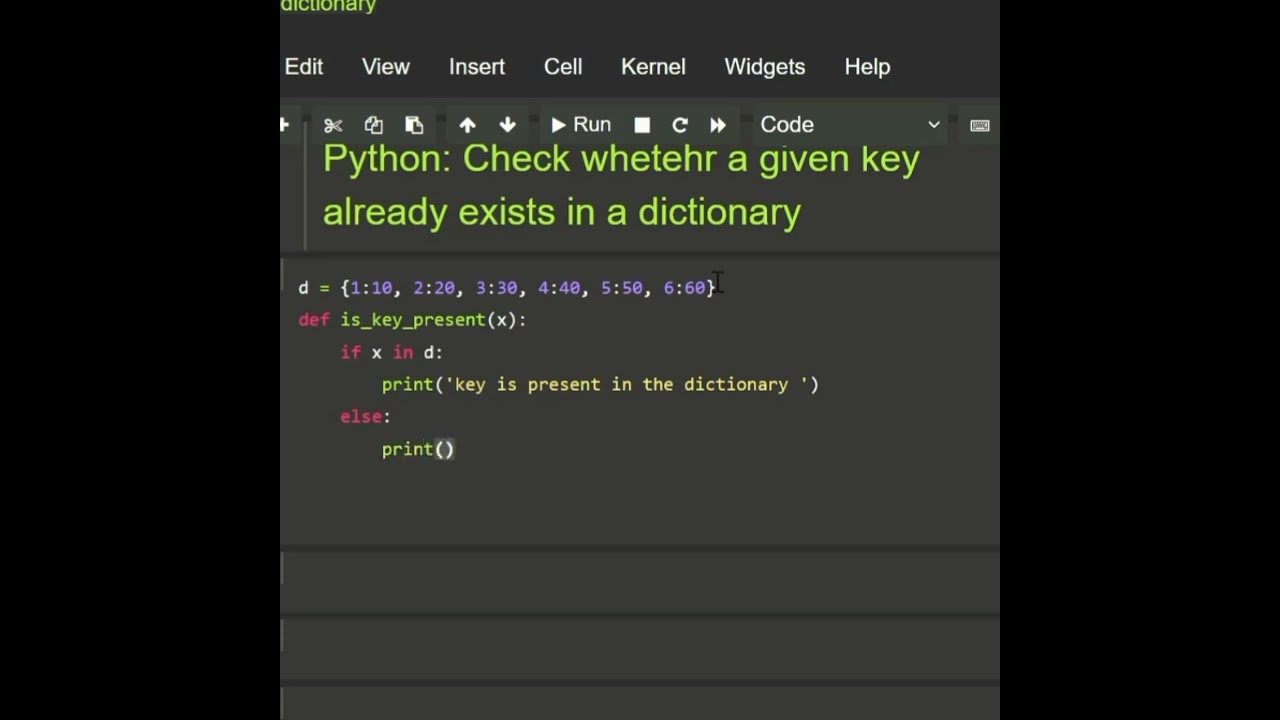

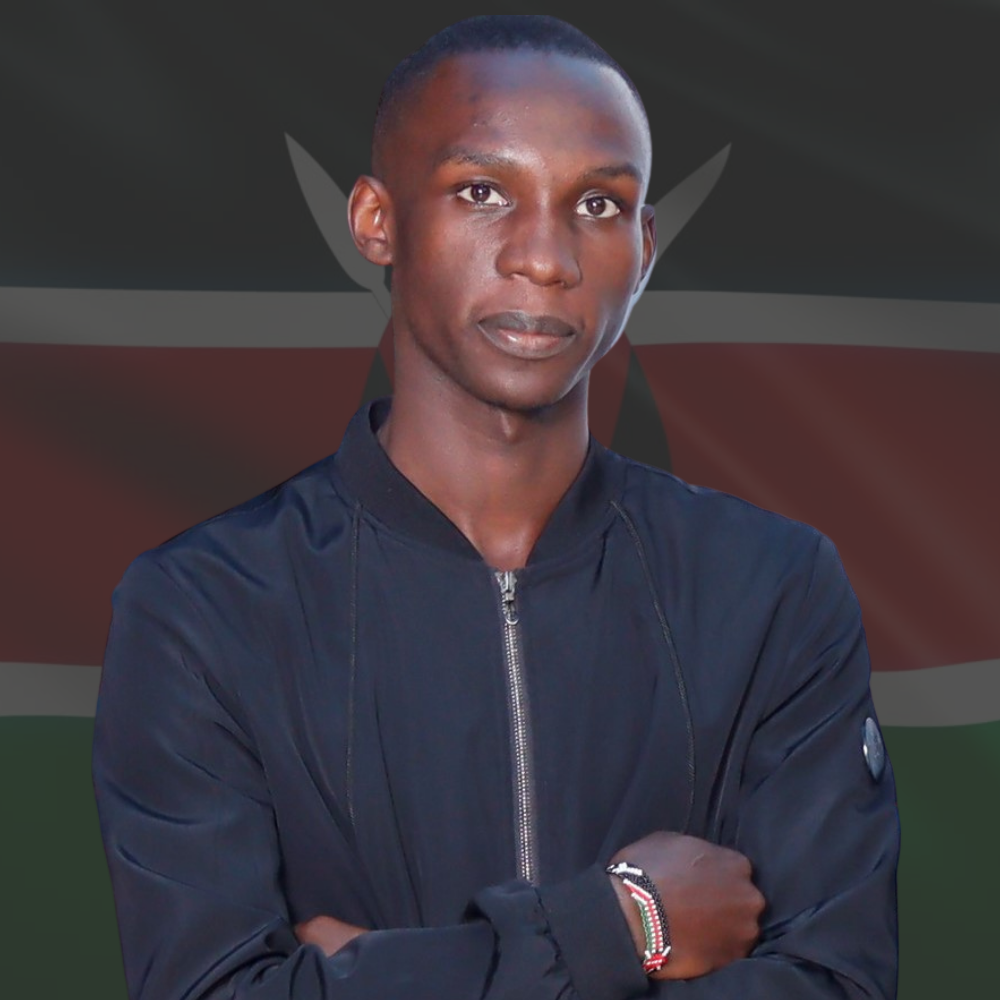
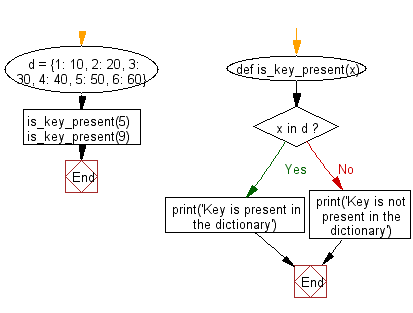
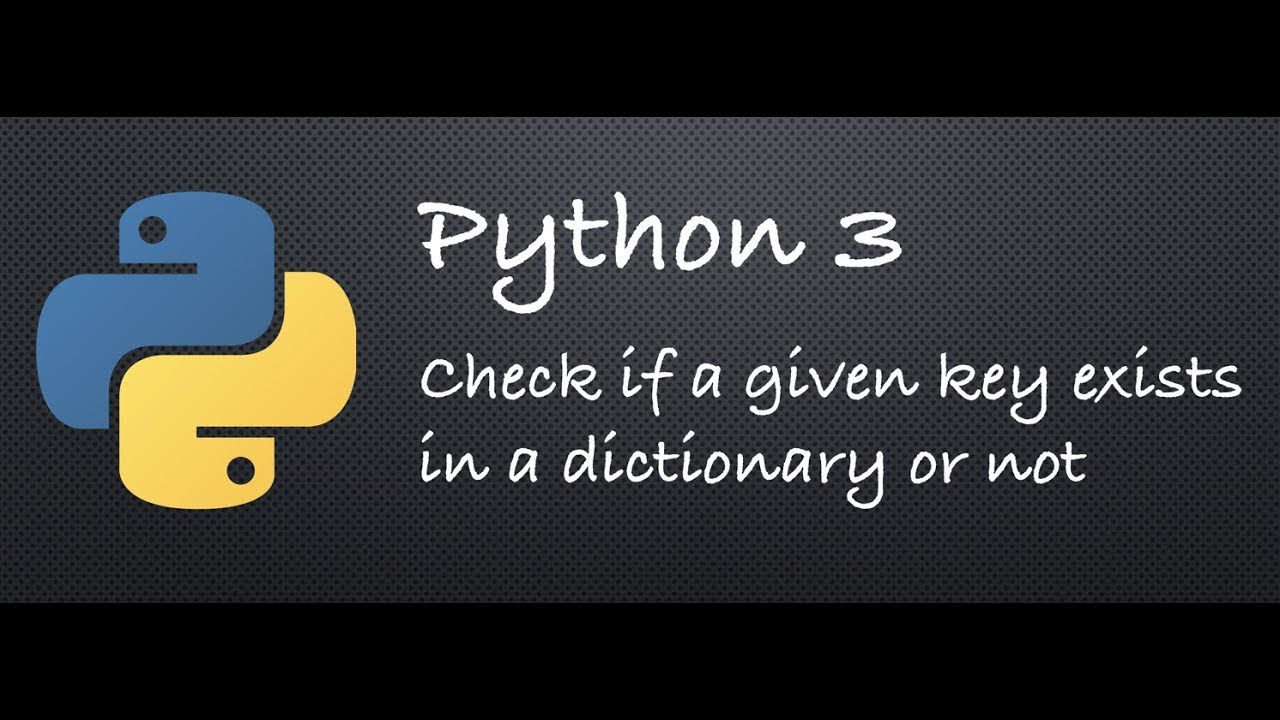

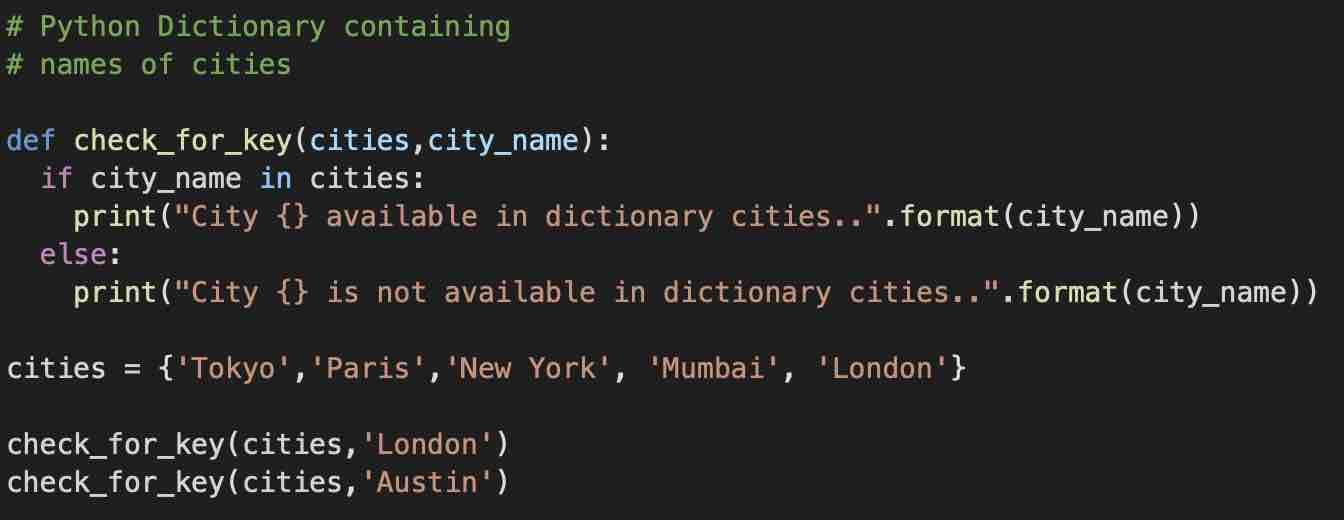

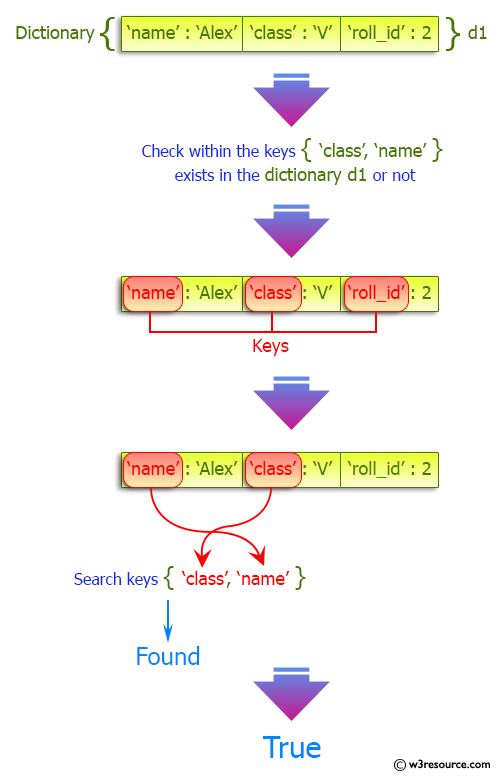
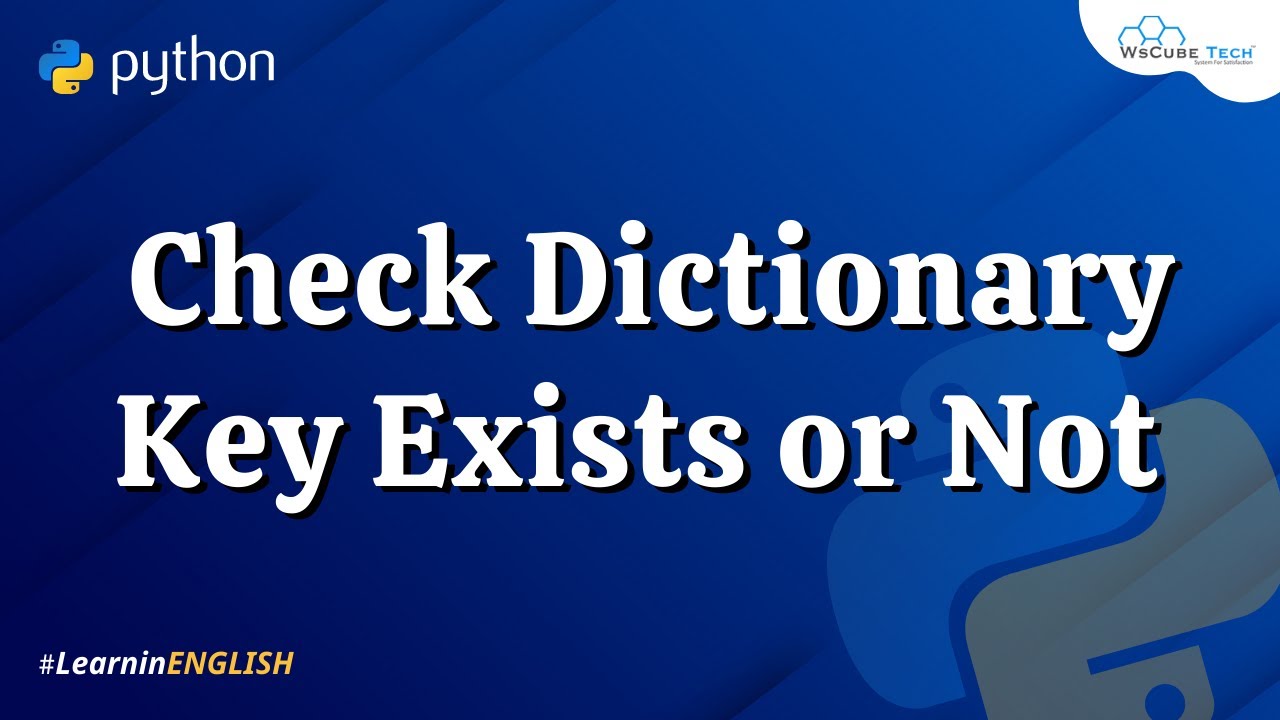


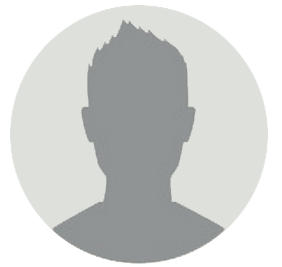
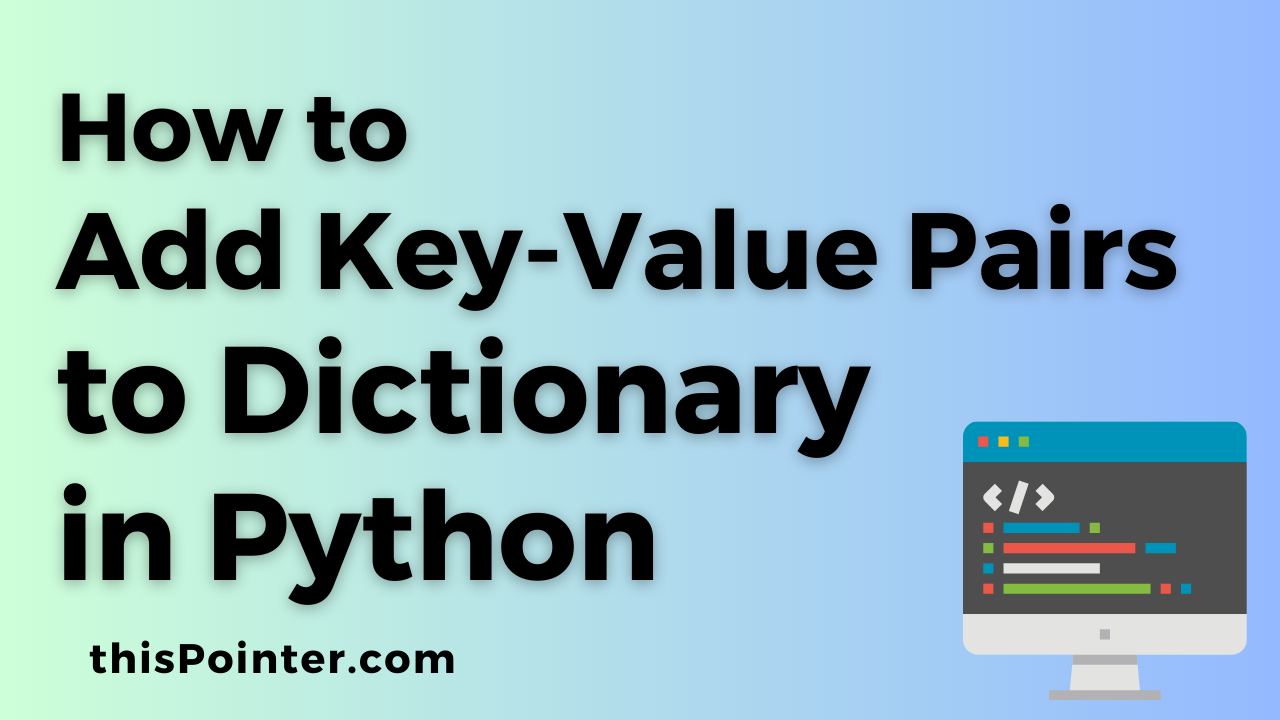

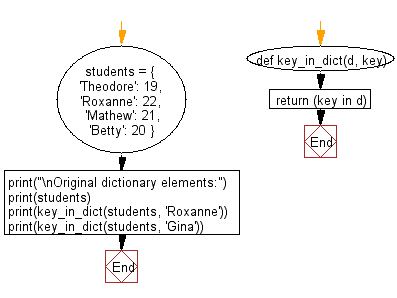
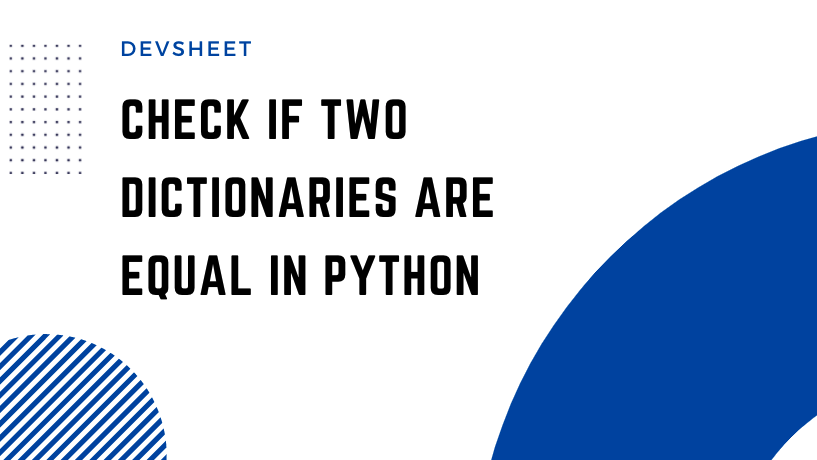

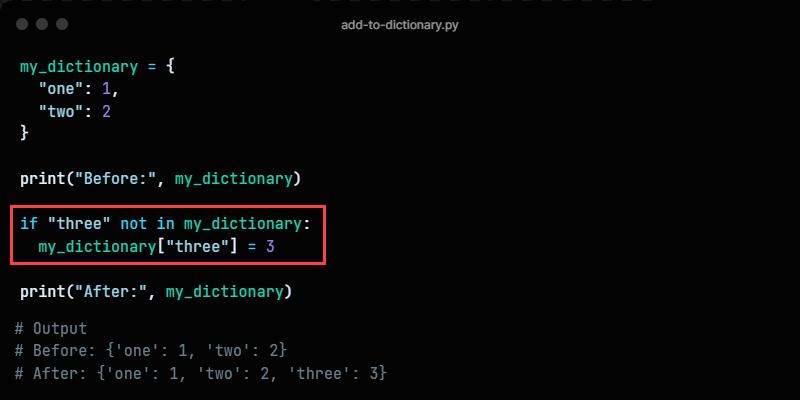
![SOLVED] Check if key exists in object in JS [3 Methods] | GoLinuxCloud Solved] Check If Key Exists In Object In Js [3 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/example3.jpg)
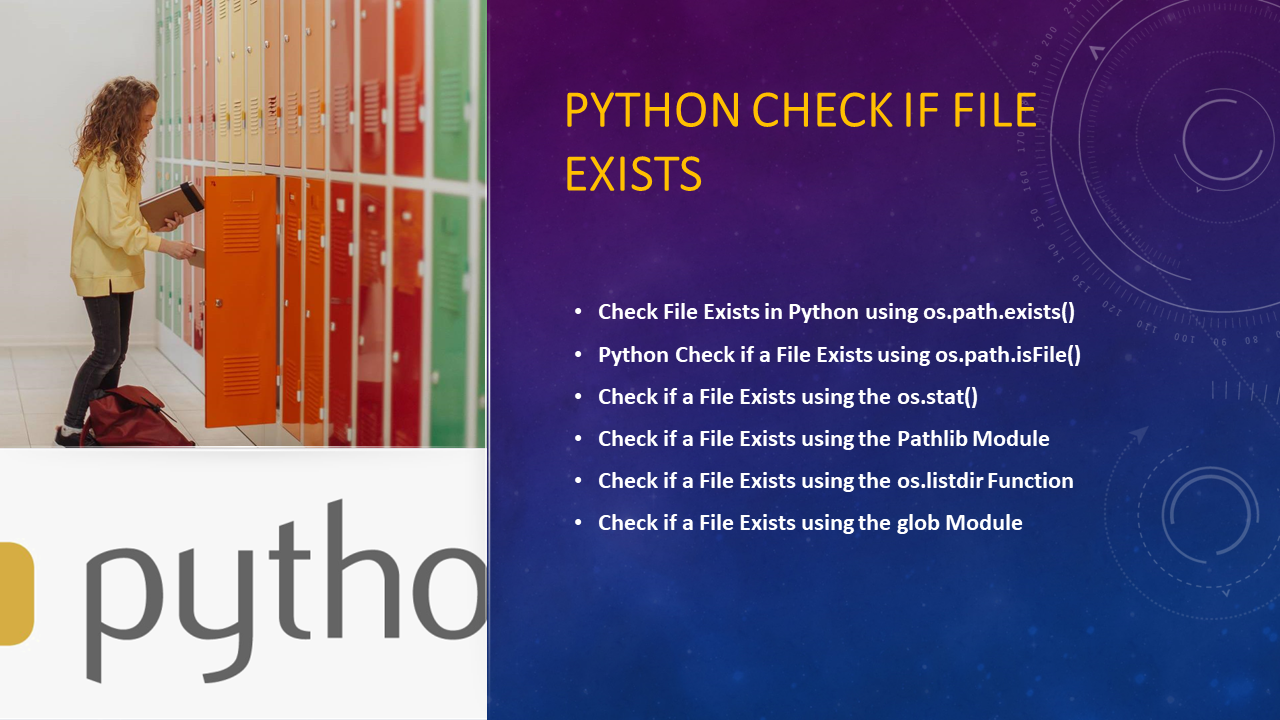
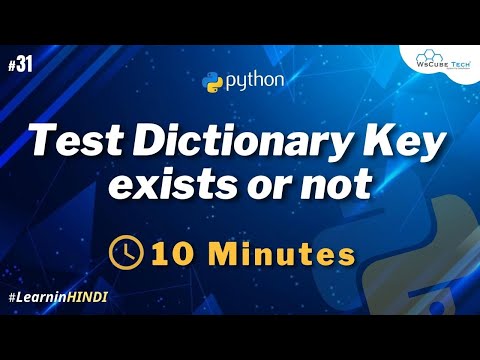
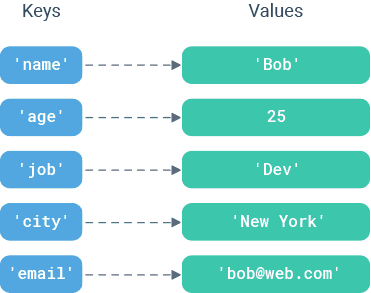
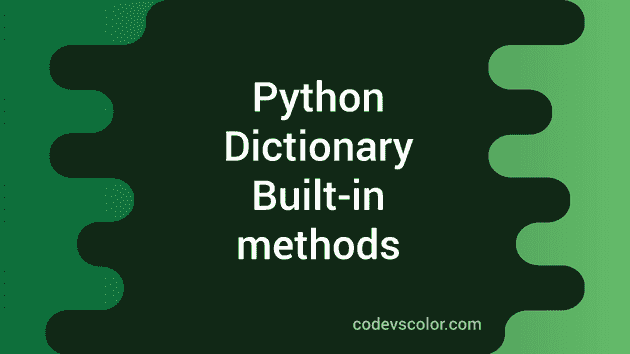
Article link: python check if key exists in dictionary.
Learn more about the topic python check if key exists in dictionary.
- How to check if key exists in a python dictionary? – Flexiple
- Check if a given key already exists in a dictionary
- Check whether given Key already exists in a Python Dictionary
- Check if Key Exists in Dictionary Python – Scaler Topics
- How to check if a key exists in a Python dictionary – Educative.io
- Check whether given Key already exists in a Python Dictionary
- Check if Key exists in Dictionary (or Value) with Python code
- How To Check If Key Exists In Dictionary In Python?
- Python Check if Key Exists in Dictionary – Spark By {Examples}
- How to Check if a Key Exists in a Dictionary in Python
See more: nhanvietluanvan.com/luat-hoc