Python Check If Dictionary Is Empty
Ways to Check if a Dictionary is Empty:
1. Using the len() function:
The len() function in Python returns the number of items in an object. By applying the len() function to a dictionary, we can determine if it is empty or not. If the length of the dictionary is 0, it means the dictionary is empty.
“`python
my_dict = {}
if len(my_dict) == 0:
print(“The dictionary is empty.”)
“`
2. Using the bool() function:
The bool() function in Python checks whether an object evaluates to True or False. When applied to a dictionary, an empty dictionary will evaluate to False, while a non-empty dictionary will evaluate to True.
“`python
my_dict = {}
if bool(my_dict) == False:
print(“The dictionary is empty.”)
“`
3. Using the not keyword:
We can use the not keyword in Python to check if a dictionary is empty or not. By adding the not keyword before the dictionary name, we can determine if the dictionary is empty. If the dictionary is empty, it will return True, otherwise, it will return False.
“`python
my_dict = {}
if not my_dict:
print(“The dictionary is empty.”)
“`
4. Comparing the dictionary to an empty dictionary:
We can directly compare the dictionary to an empty dictionary using the equality operator (==). If the two dictionaries are equal, it means the dictionary is empty.
“`python
my_dict = {}
if my_dict == {}:
print(“The dictionary is empty.”)
“`
5. Using a for loop to check for key-value pairs:
In Python, dictionaries are iterable, and we can use a for loop to iterate over the dictionary. By checking the number of iterations, we can determine if the dictionary is empty or not.
“`python
my_dict = {}
for key, value in my_dict.items():
break
else:
print(“The dictionary is empty.”)
“`
6. Using the any() and values() functions:
The any() function in Python returns True if at least one element in an iterable is true. By applying the any() function to the values of a dictionary, we can check if any key-value pair exists. If the dictionary is empty, it will return False, otherwise, it will return True.
“`python
my_dict = {}
if not any(my_dict.values()):
print(“The dictionary is empty.”)
“`
7. Using the all() and values() functions:
The all() function in Python returns True if all elements in an iterable are true. By applying the all() function to the values of a dictionary, we can check if all key-value pairs exist. If the dictionary is empty, it will return True, otherwise, it will return False.
“`python
my_dict = {}
if all(not value for value in my_dict.values()):
print(“The dictionary is empty.”)
“`
FAQs:
Q: How do I check if a dictionary is empty in Python?
A: There are several ways to check if a dictionary is empty in Python. You can use the len() function, the bool() function, the not keyword, compare the dictionary to an empty dictionary, use a for loop, or apply the any() and all() functions to the dictionary values.
Q: How do I check if a list is empty in Python?
A: To check if a list is empty in Python, you can use the len() function or compare the list to an empty list.
Q: How do I find an element in a dictionary in Python?
A: To find an element in a dictionary in Python, you can use the key as an index to access the corresponding value.
Q: How do I create an empty dictionary in Python?
A: You can create an empty dictionary in Python by assigning an empty curly braces to a variable.
Q: How do I check if a key exists in a dictionary in Python?
A: To check if a key exists in a dictionary in Python, you can use the ‘in’ keyword followed by the dictionary name and the desired key.
Q: How do I check if a dictionary is empty in JavaScript?
A: In JavaScript, you can use the Object.keys() method to get an array of keys in the dictionary, and then check if the length of that array is 0 to determine if the dictionary is empty.
Q: How do I check if a variable is null in Python?
A: In Python, the equivalent of null is None. To check if a variable is None, you can use the ‘is’ keyword followed by the None keyword.
In conclusion, there are various ways to check if a dictionary is empty in Python. Depending on your specific requirements and coding style, you can choose the method that suits your needs. By using the len() function, the bool() function, the not keyword, comparing to an empty dictionary, using a for loop, or applying the any() and all() functions, you can easily determine if a dictionary is empty or not.
How To Check If A Dictionary Is Empty In Python
Keywords searched by users: python check if dictionary is empty Check dict empty Python, Check if list is empty Python, Find element in dictionary Python, Empty dictionary Python, How to check a key in dictionary Python, Check empty dictionary JavaScript, Python check null, Check if object is null python
Categories: Top 12 Python Check If Dictionary Is Empty
See more here: nhanvietluanvan.com
Check Dict Empty Python
Python, a versatile and powerful programming language, offers various data structures to organize and manipulate data efficiently. One such widely used data structure is a dictionary, also known as a hashmap or associative array. A dictionary allows you to store key-value pairs, where each key is unique and associated with a corresponding value.
While working with dictionaries, it is often necessary to check whether a dictionary is empty or not. In this article, we will dive deeper into the concept of checking if a dictionary is empty in Python. We will explore different approaches, provide code examples, and clarify common doubts through a FAQs section. So let’s get started!
Approaches to Check if a Dictionary is Empty in Python:
1. Using the `len()` function: The simplest way to check if a dictionary is empty is by using the `len()` function. The `len()` function returns the number of key-value pairs present in the dictionary. If the length of the dictionary is zero, it implies that the dictionary is empty.
Consider the following code snippet:
“`python
dictionary = {}
if len(dictionary) == 0:
print(“The dictionary is empty.”)
else:
print(“The dictionary is not empty.”)
“`
Output:
“`
The dictionary is empty.
“`
2. Using the `not` keyword: Another approach is to use the `not` keyword to check the truthiness of a dictionary. In Python, an empty dictionary is considered as “False” in a boolean context, while a non-empty dictionary is considered as “True”.
Here’s an example:
“`python
dictionary = {}
if not dictionary:
print(“The dictionary is empty.”)
else:
print(“The dictionary is not empty.”)
“`
Output:
“`
The dictionary is empty.
“`
Code snippets 1 and 2 are functionally equivalent. However, using `not` in combination with the dictionary makes the code more readable and pythonic.
3. Direct comparison with an empty dictionary: Python allows you to directly compare a dictionary with an empty dictionary using the `==` operator. If the dictionaries match, it means that the dictionary is empty.
Consider the following code snippet:
“`python
dictionary = {}
if dictionary == {}:
print(“The dictionary is empty.”)
else:
print(“The dictionary is not empty.”)
“`
Output:
“`
The dictionary is empty.
“`
This approach is straightforward and readable, but using `==` for comparison may not work with different dictionary objects (e.g., if they have different memory addresses or were created in different ways).
Frequently Asked Questions (FAQs):
Q1. Is there a performance difference between the different approaches?
A1. In most cases, the performance difference is negligible. However, using `len()` or `not` tends to be slightly faster than direct comparison when dealing with larger dictionaries.
Q2. Can I check if a dictionary is empty using an `if-else` statement without any methods?
A2. Yes, you can check if a dictionary is empty by directly using an `if-else` statement with the dictionary as the condition. An empty dictionary will be considered as “False”, while a non-empty dictionary will be considered as “True”. However, using `len()` or `not` is generally preferred for clarity and readability.
Q3. Can I use the `is` operator instead of `==` for direct comparison in Approach 3?
A3. The `is` operator should not be used for comparison against an empty dictionary. The `is` operator checks for object identity, which may not always yield the expected result when comparing dictionaries.
Q4. What if my dictionary contains None as a value, does it still count as empty?
A4. In Python, a non-empty dictionary with values set to None is still considered as non-empty. The dictionary must have no key-value pairs to be considered empty.
Q5. Are there any built-in functions to check if a dictionary is empty in Python?
A5. Python does not provide any specific built-in function for checking if a dictionary is empty. However, the approaches mentioned above using `len()`, `not`, or direct comparison are widely adopted by Python developers.
In conclusion, Python provides several effective methods to check if a dictionary is empty. Whether you choose to use the `len()` function, the `not` keyword, or direct comparison, the choice depends on personal preference and specific use cases. Remember to consider the readability and performance trade-offs while selecting your preferred approach.
Check If List Is Empty Python
In Python, a list is a versatile data structure that allows you to store multiple items of different data types in a single variable. As you work with lists, it is crucial to determine whether a list is empty or not to avoid any errors or unexpected results. In this article, we will discuss various methods to check if a list is empty in Python, along with some common FAQs.
Methods to check if a list is empty in Python:
1. Using the len() function:
The len() function in Python returns the number of elements in a list. By using len() function, we can check if the length of the list is zero, indicating that the list is empty.
“`python
my_list = []
if len(my_list) == 0:
print(“The list is empty.”)
“`
In this example, the len() function is used to determine the length of the ‘my_list’ variable. If the length is 0, it means the list is empty, and the corresponding message will be printed.
2. Using the not operator with len():
Python allows us to use logical operators such as not to perform a logical negation. By combining the not operator with the len() function, we can directly check if a list is empty or not.
“`python
my_list = []
if not len(my_list):
print(“The list is empty.”)
“`
In this example, the not operator is used to negate the value returned by the len() function, resulting in a True or False value. If the list is empty, the condition evaluates to True, and the message will be printed.
3. Using the bool() function:
The bool() function in Python converts a value to its corresponding Boolean value. By using the bool() function with the list as the argument, we can directly check if the list is empty.
“`python
my_list = []
if not bool(my_list):
print(“The list is empty.”)
“`
In this example, the bool() function is used to convert the list into its corresponding Boolean value. If the list is empty, the bool() function returns False, and the message will be printed.
4. Using the if statement with the list:
In Python, we can directly use the list as a condition in the if statement. If the list is empty, it will evaluate to False, and we can execute the desired code accordingly.
“`python
my_list = []
if not my_list:
print(“The list is empty.”)
“`
In this example, the list ‘my_list’ is used directly in the if statement. If the list is empty, the condition evaluates to True, and the corresponding message will be printed.
Frequently Asked Questions:
Q1. Why is it necessary to check if a list is empty?
Checking if a list is empty allows you to handle any potential errors or issues related to empty lists in your Python programs. By verifying if a list is empty, you can avoid trying to perform operations on an empty list which could result in errors and unexpected behavior.
Q2. What will happen if a list contains elements but is considered empty?
If a list contains elements but is identified as empty using any of the above methods, it indicates a flaw in the code logic. It is crucial to review the code and ensure that the appropriate data is stored in the list.
Q3. Are there any performance differences between these methods?
In terms of performance, the differences between these methods are negligible. However, using the `if not my_list:` method is considered the most Pythonic and is widely used in Python programming.
Q4. Can I use these methods to check if other types of objects are empty?
Yes, these methods can be applied to check if objects of other types, such as strings, tuples, dictionaries, or sets, are empty or not. However, the actual implementation might differ depending on the specific data structure or object being checked.
Q5. Is it necessary to check for an empty list before performing operations on it?
Yes, it is good practice to check for an empty list before performing any operations on it. This helps to ensure that the list contains data and prevents any potential errors or exceptions related to empty lists.
In conclusion, checking if a list is empty is a crucial step in Python programming to avoid errors and unexpected behavior. By utilizing methods such as len(), not operator with len(), bool(), or directly using the list in the if statement, you can easily determine if a list is empty or not. Always remember to analyze your code carefully and consider any potential flaws or logic issues when dealing with empty lists.
Images related to the topic python check if dictionary is empty
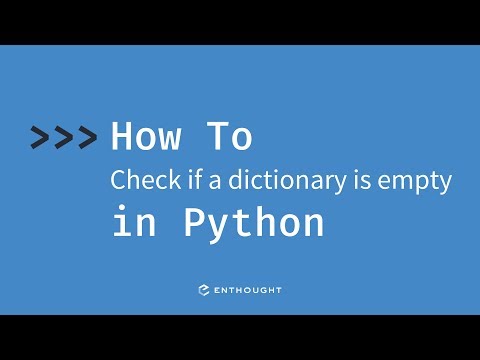
Found 29 images related to python check if dictionary is empty theme
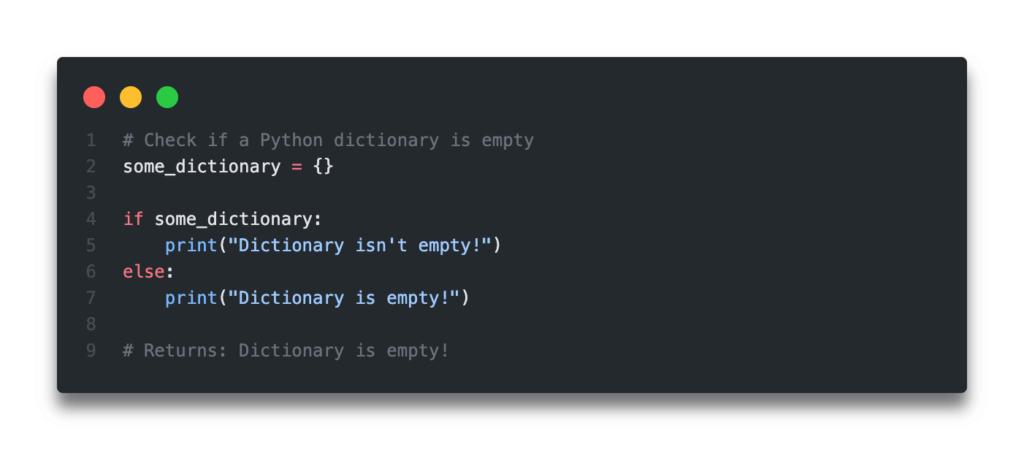
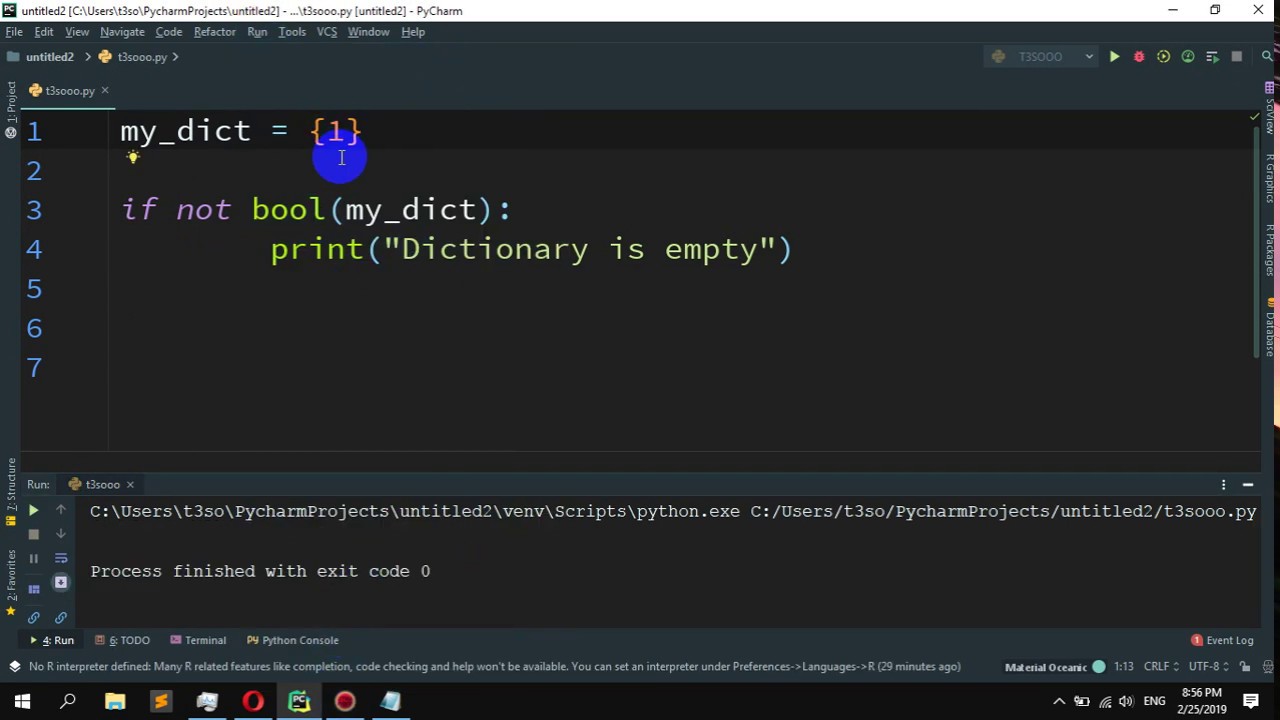
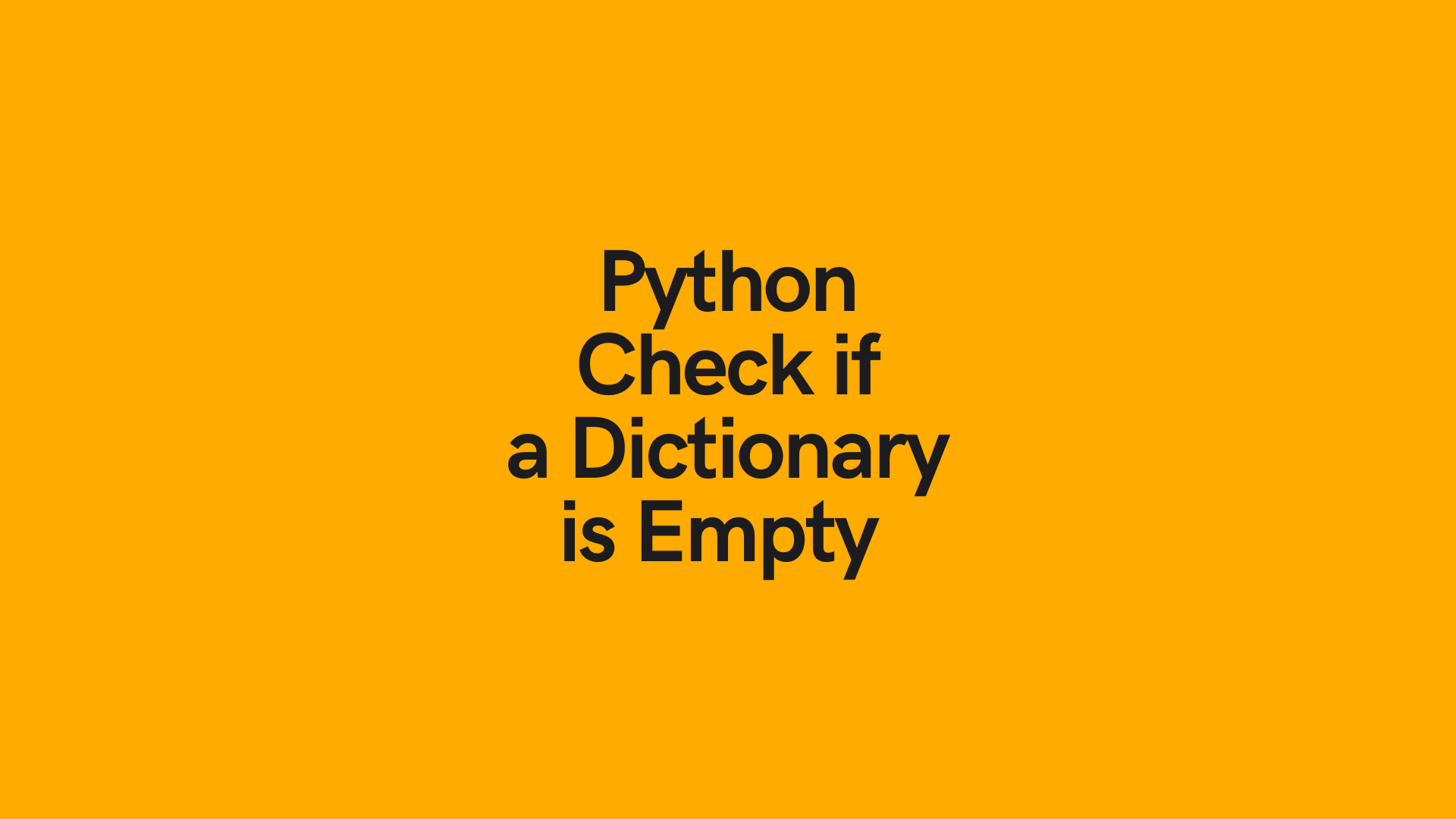
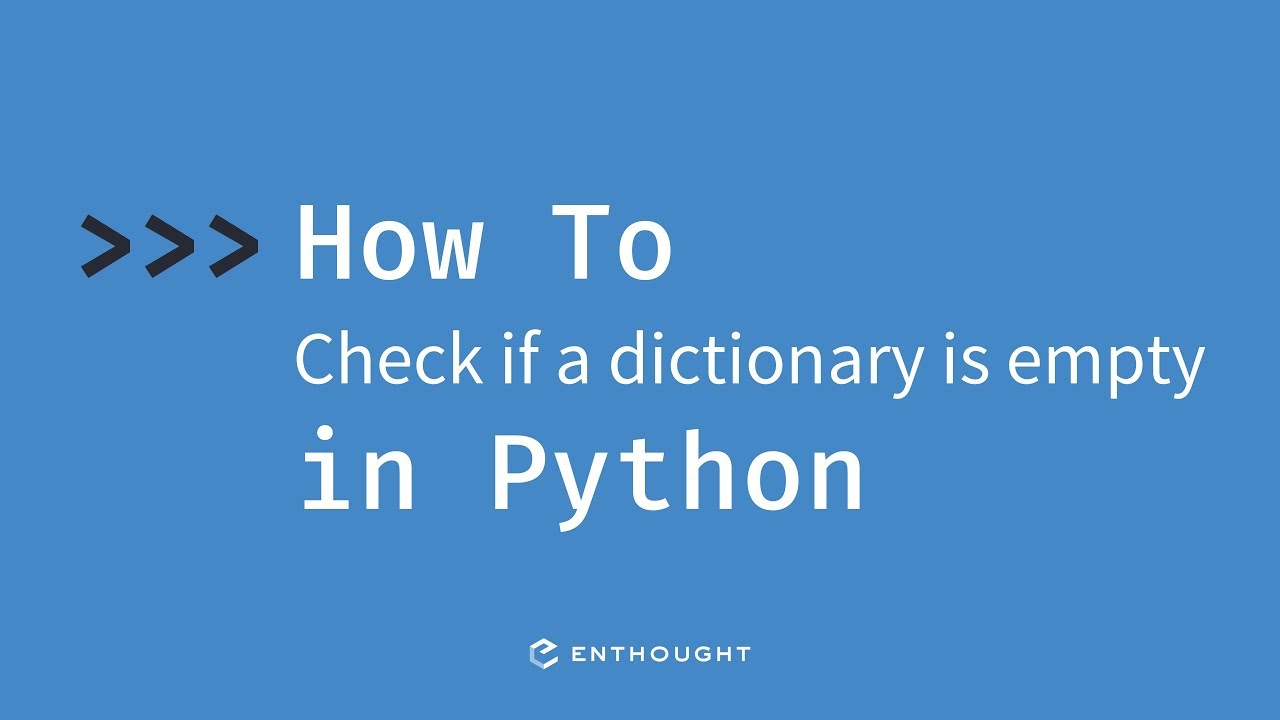

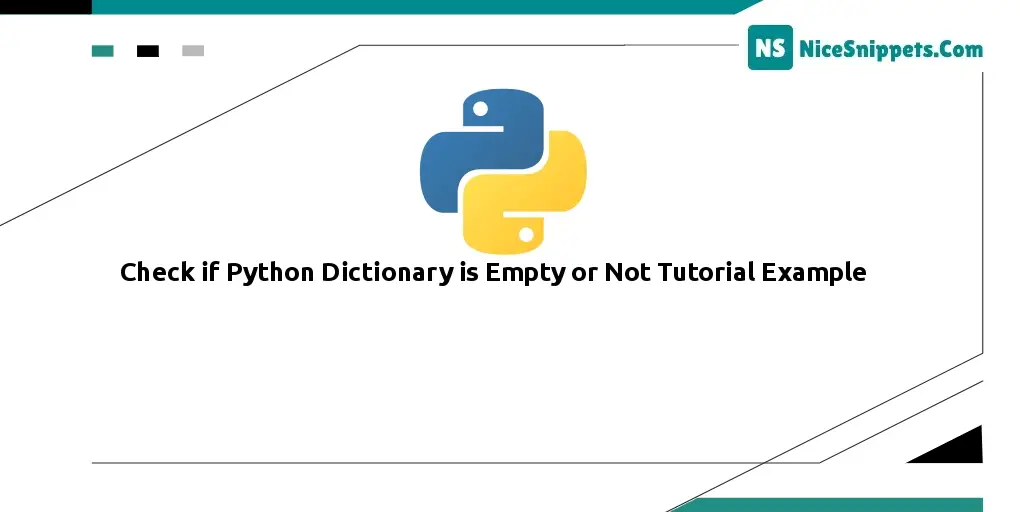
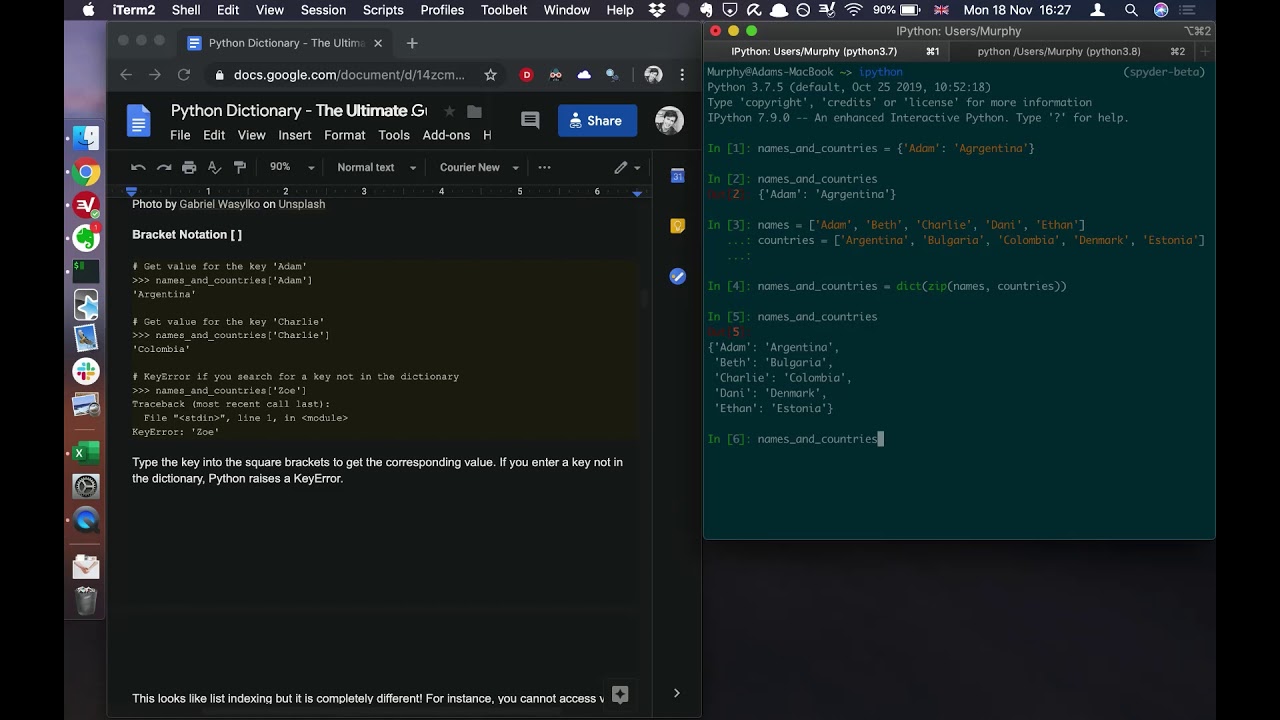

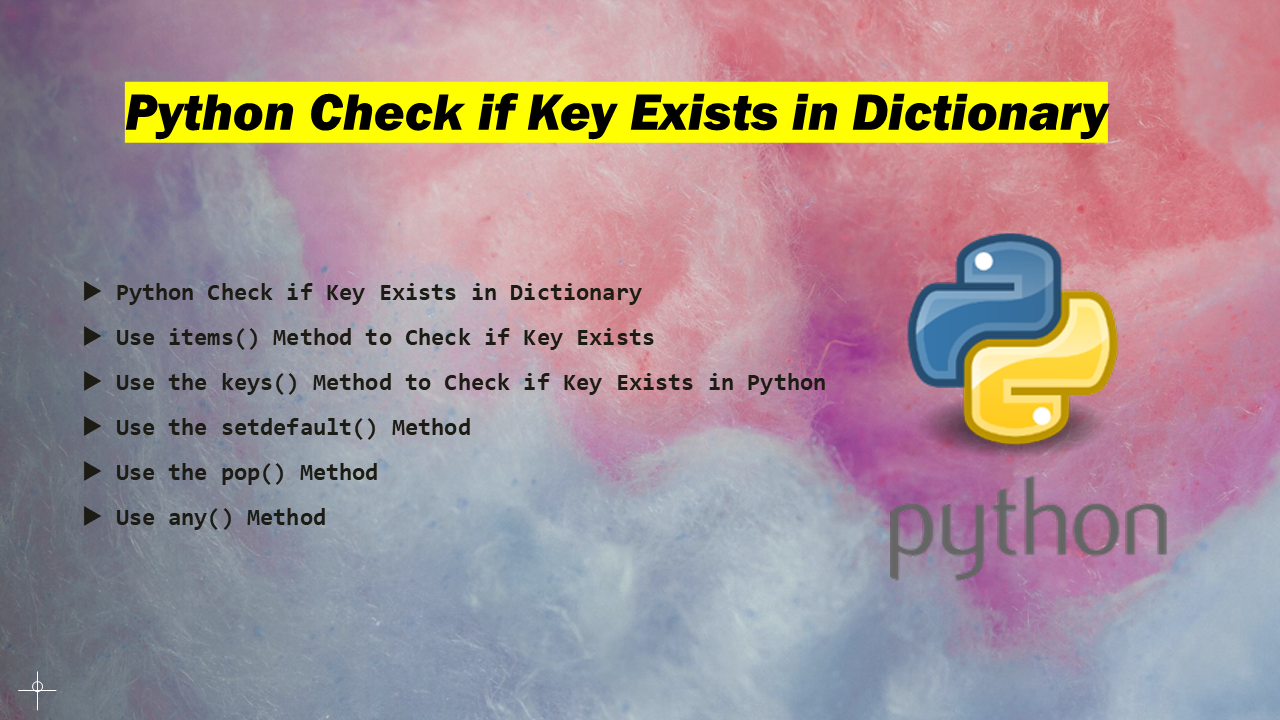


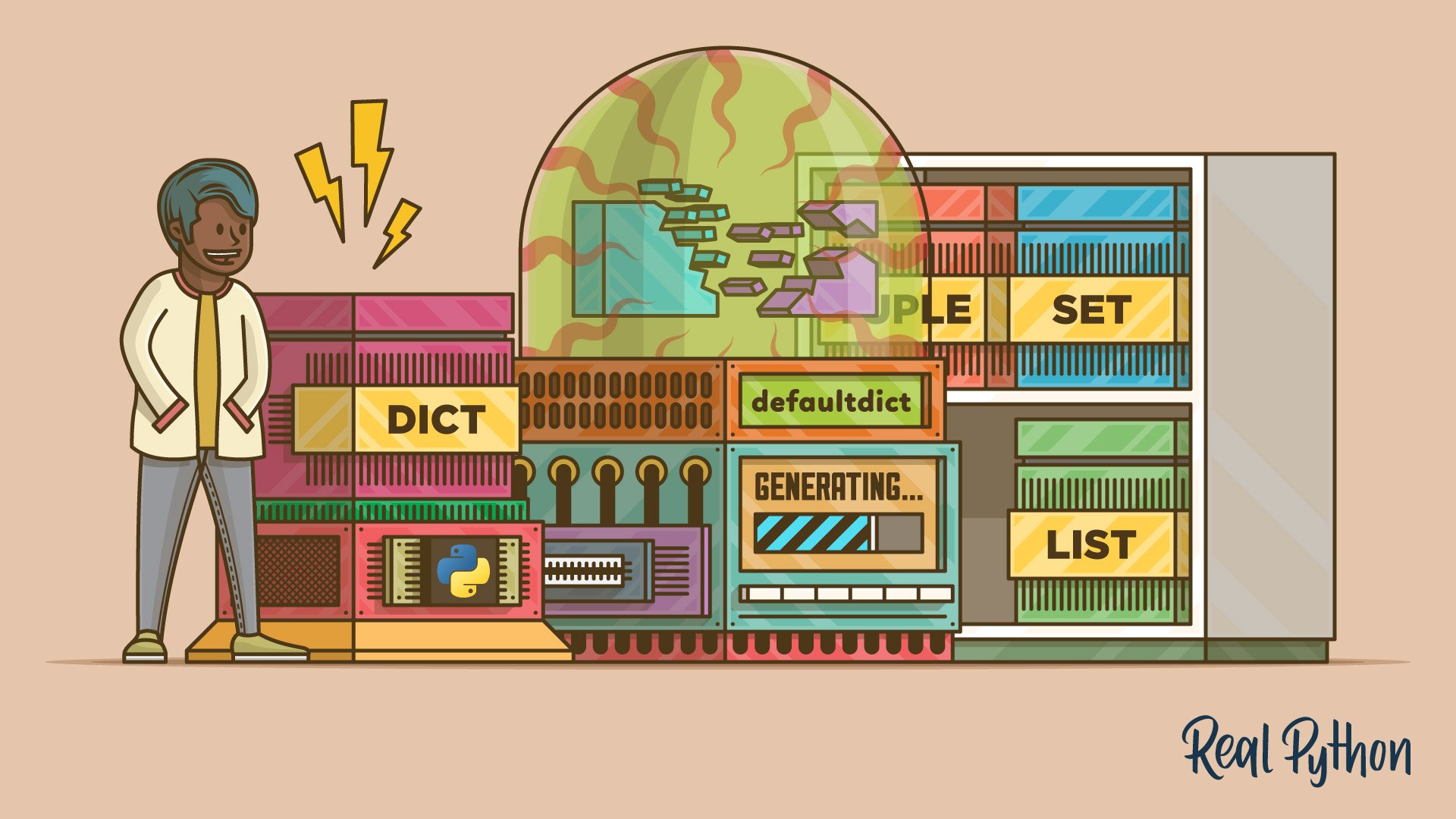
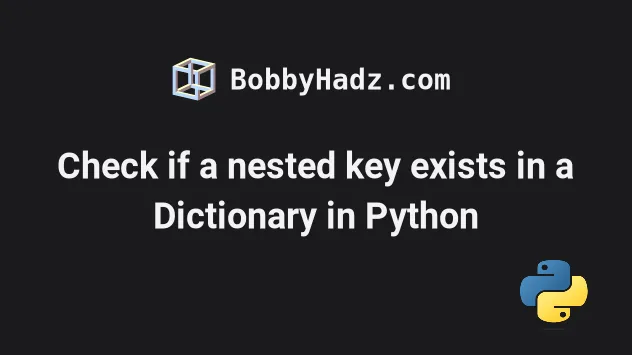
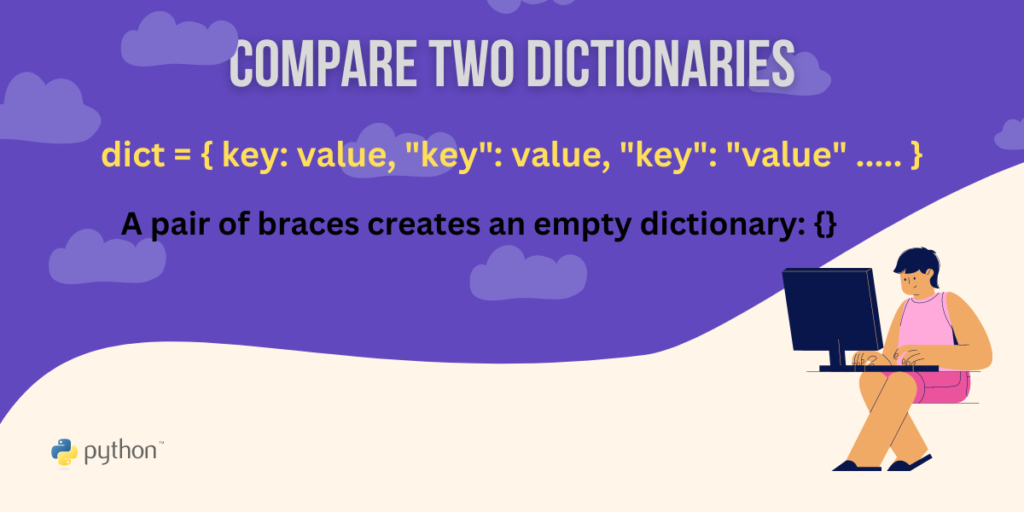
![TIL # 40 : [Python] How to check if a dictionary is empty or not (Falsy Values in Python) Til # 40 : [Python] How To Check If A Dictionary Is Empty Or Not (Falsy Values In Python)](https://images.velog.io/images/mjhuh263/post/5f54c039-8a45-42cd-afc2-4e954f2de287/PYTHON.png)
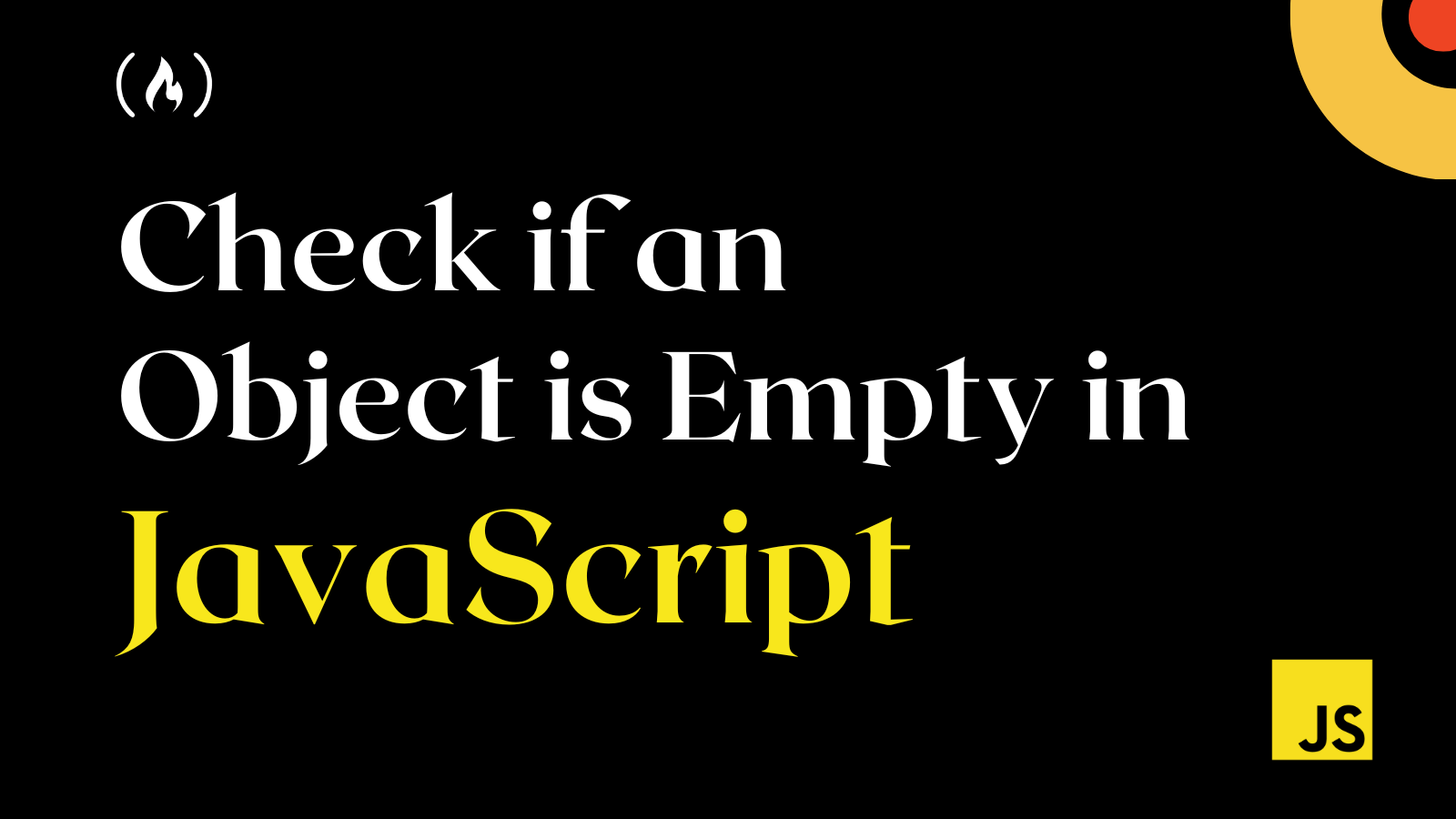
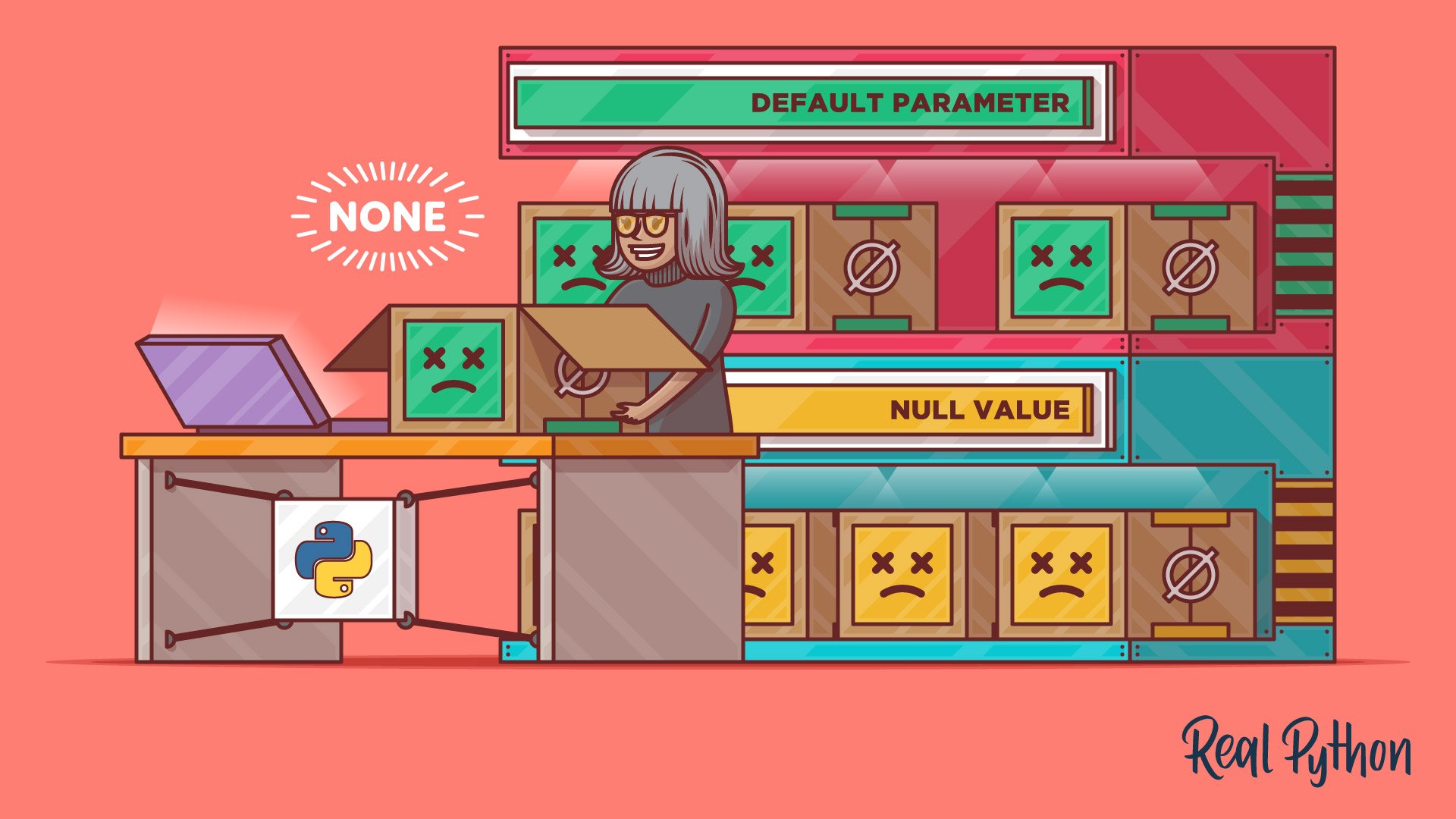

![Class 12] Julie has created a dictionary containing names and marks Class 12] Julie Has Created A Dictionary Containing Names And Marks](https://d1avenlh0i1xmr.cloudfront.net/374bed5d-d4bc-4d9f-b730-4c919131fae6/slide37.jpg)


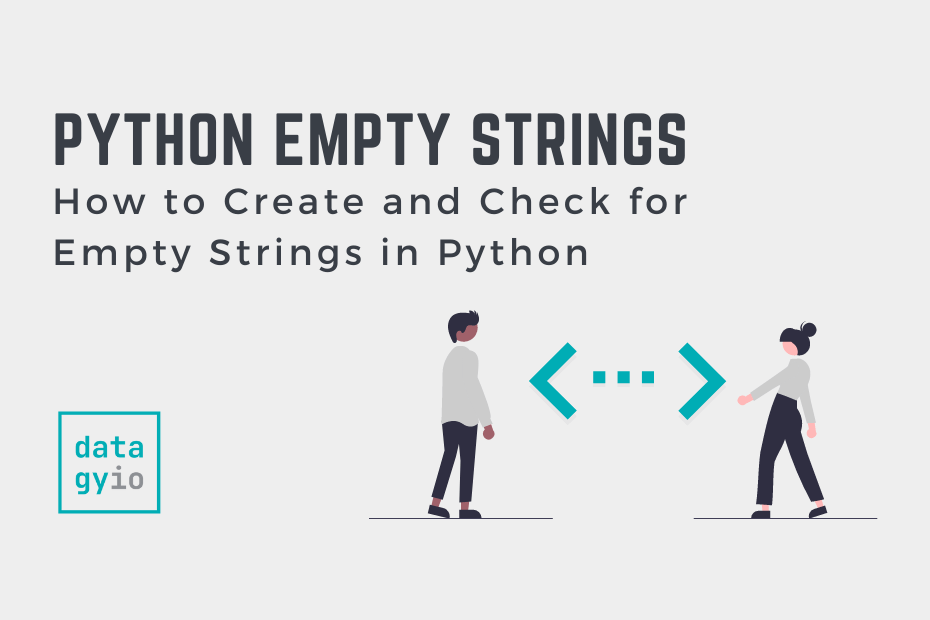
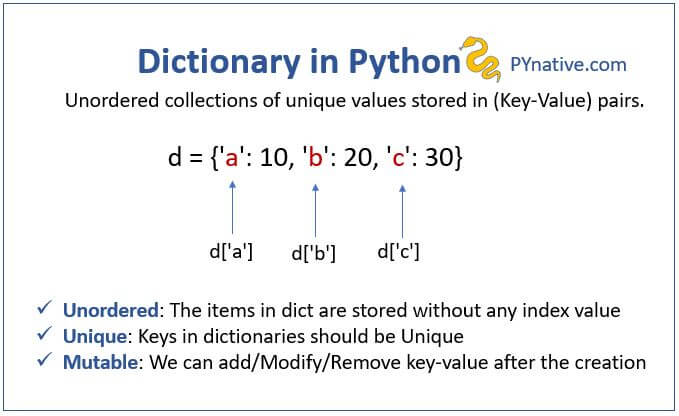

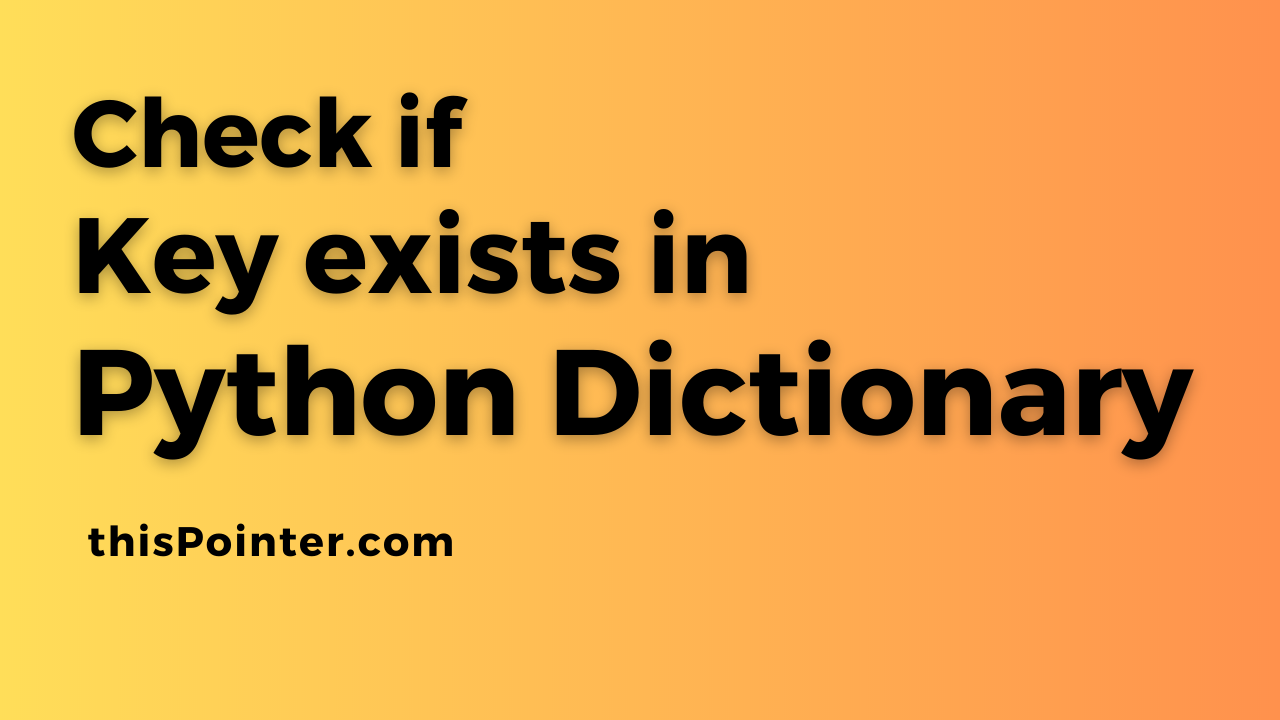
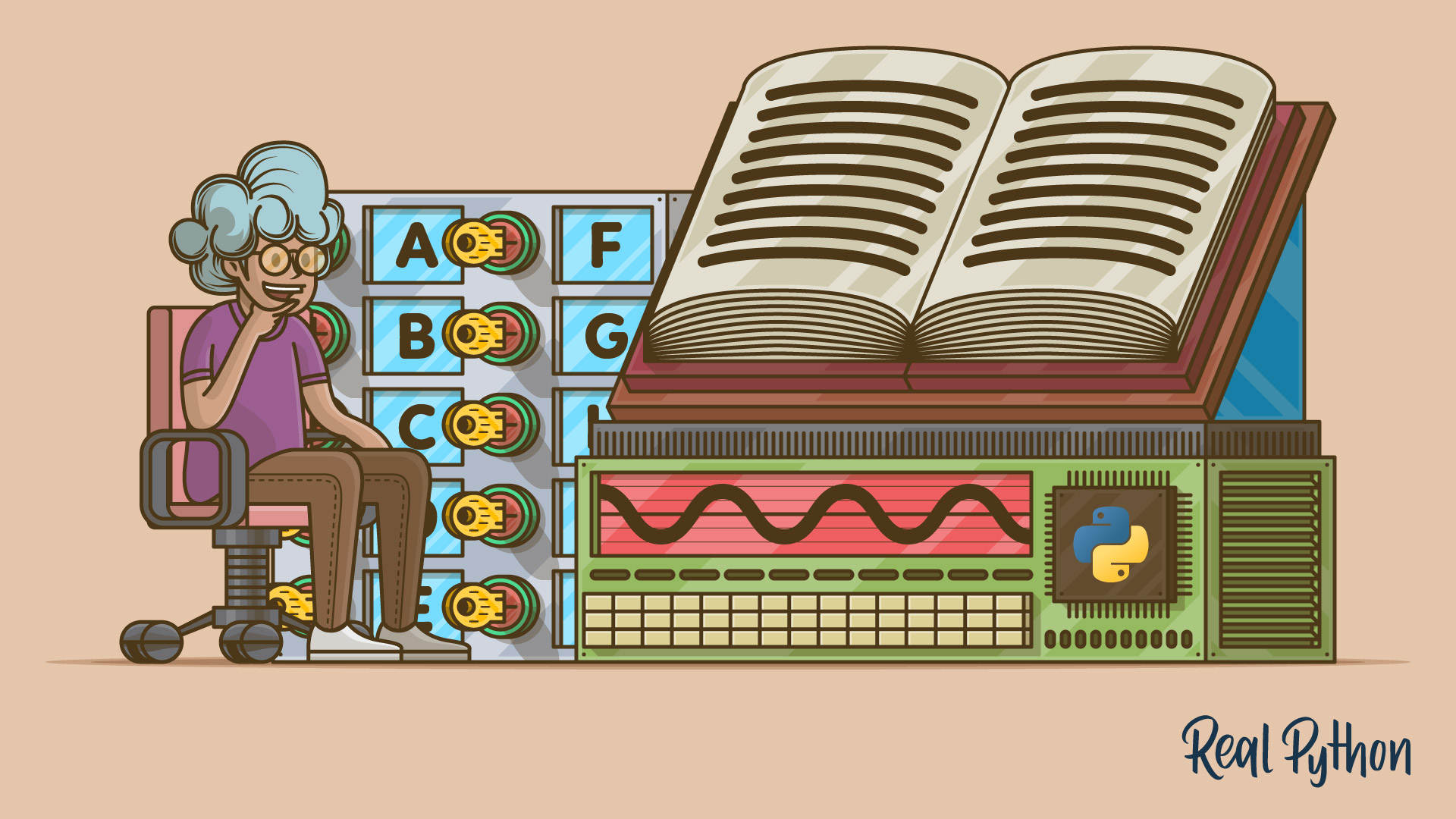

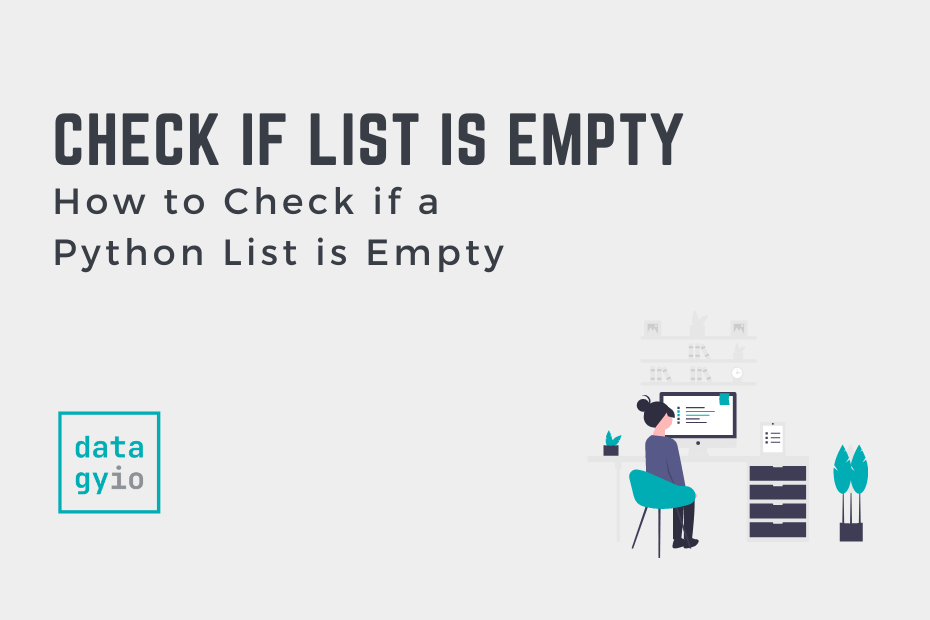

![how to check if a file is empty in python [TXT, CSV,XLSX] - YouTube How To Check If A File Is Empty In Python [Txt, Csv,Xlsx] - Youtube](https://i.ytimg.com/vi/IwPjEiBYvjA/maxresdefault.jpg)
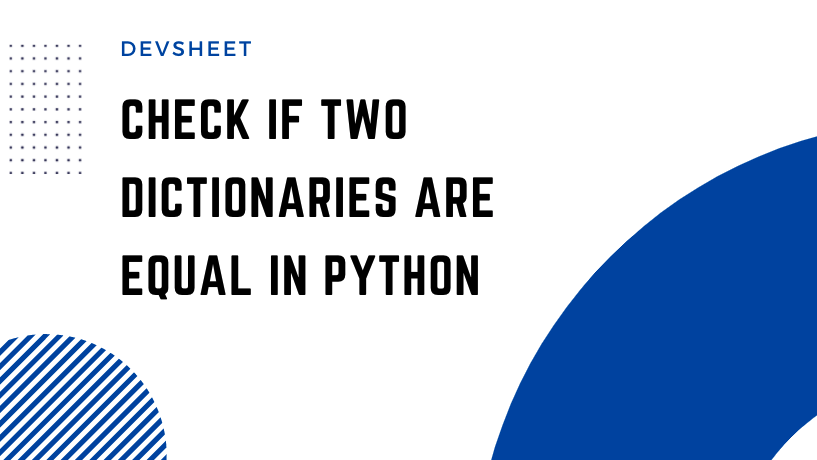
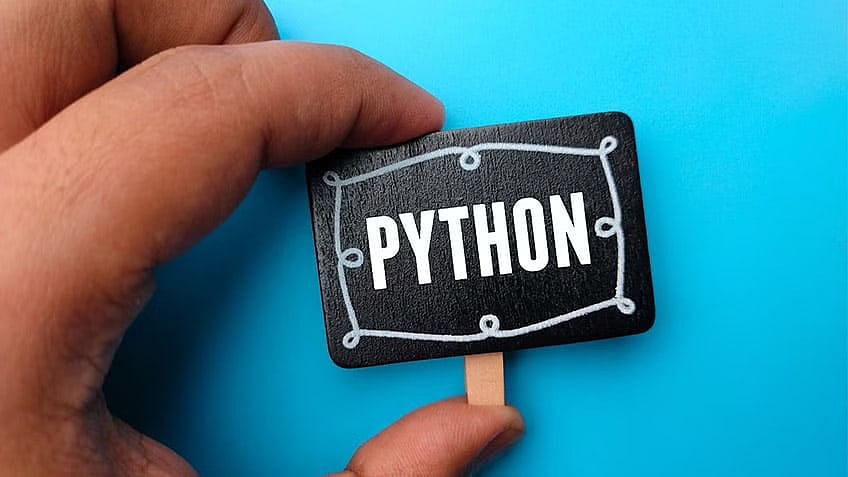
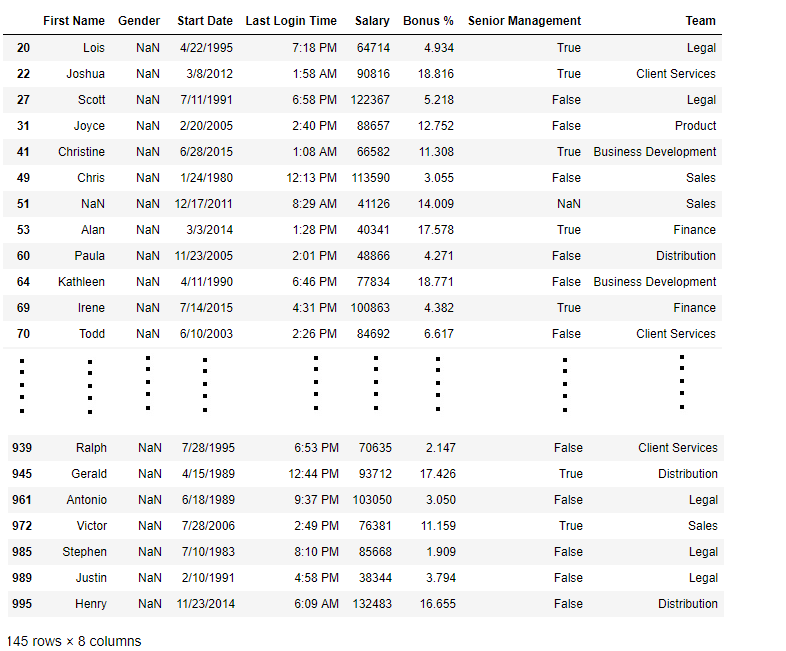
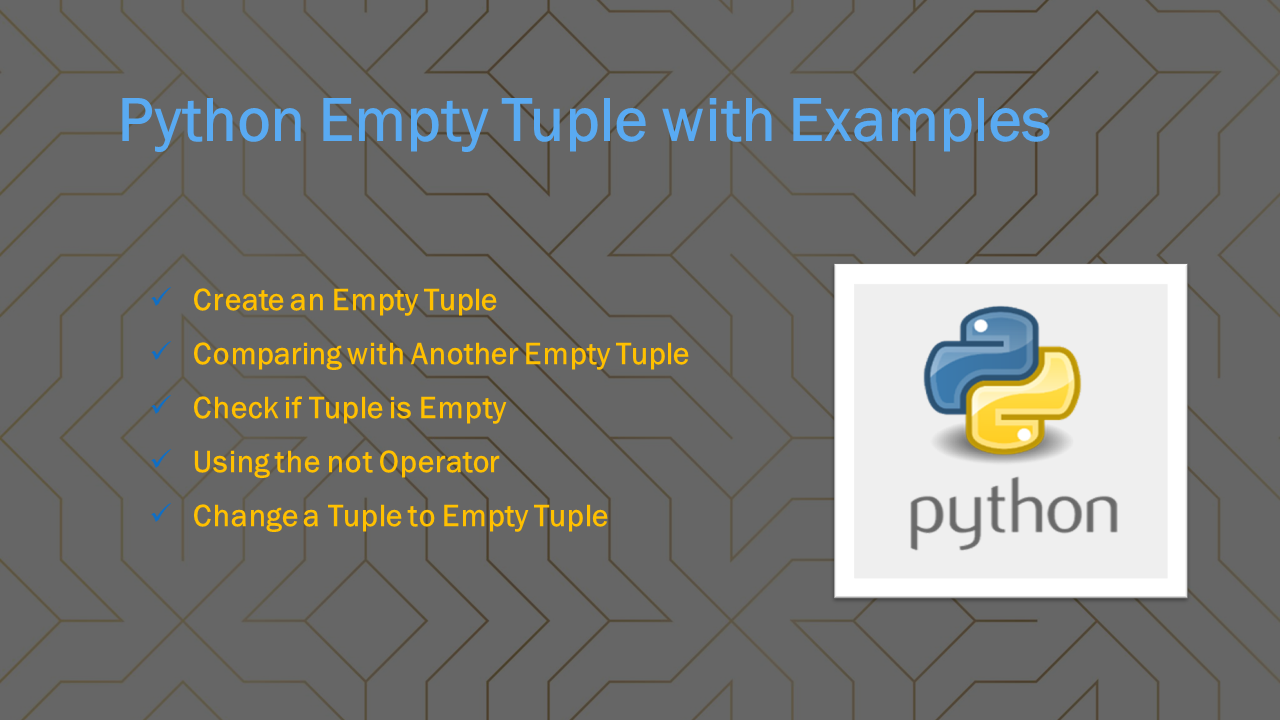

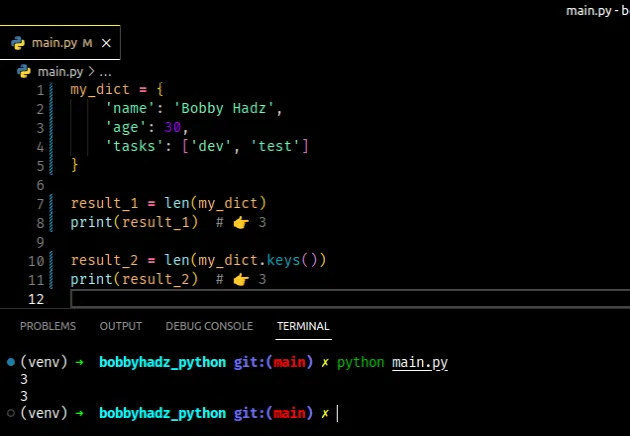
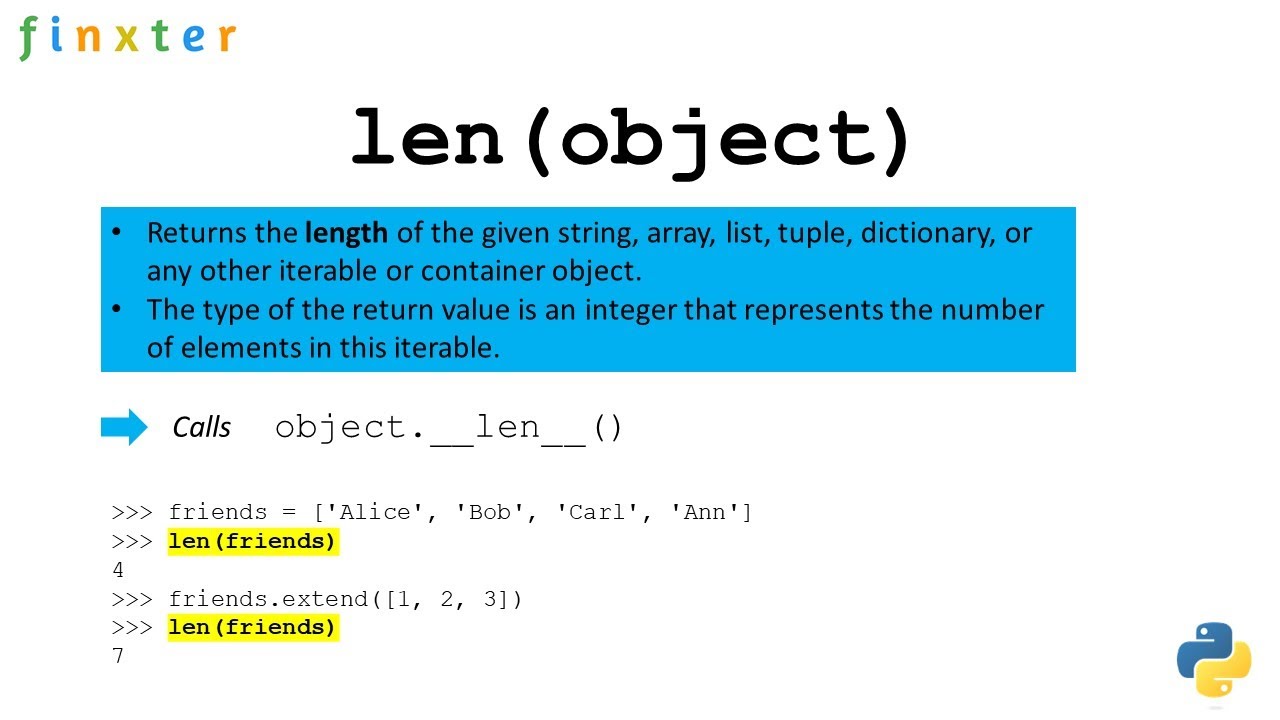
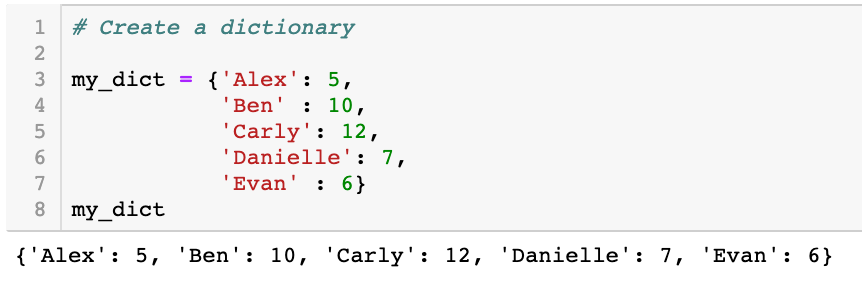
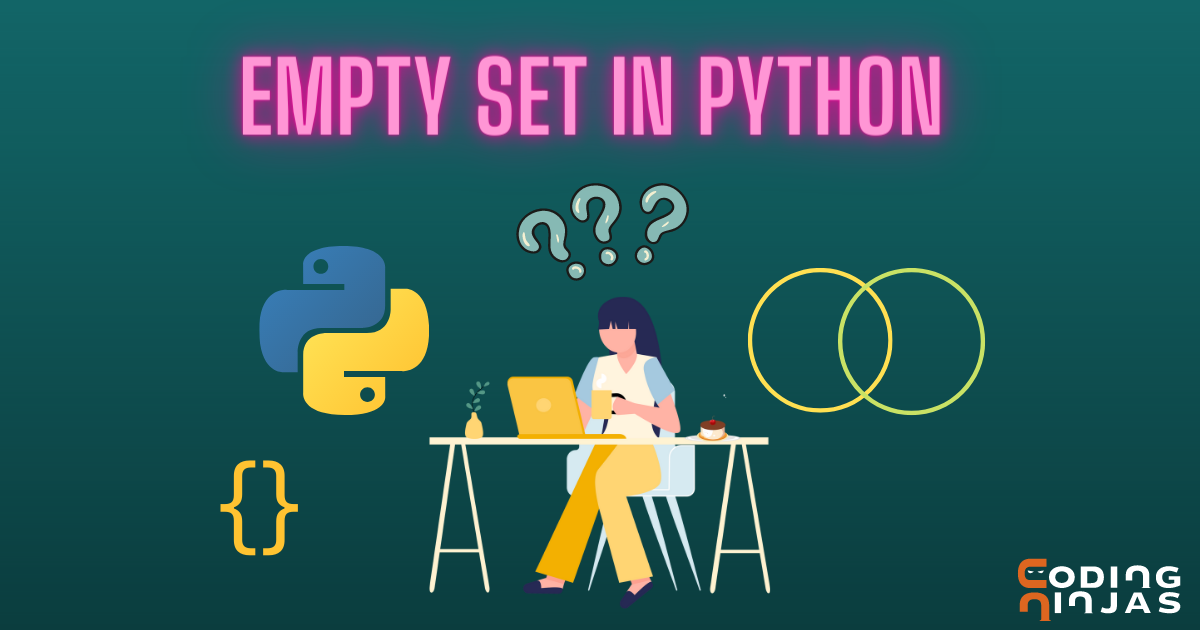
![Class 12] Write a function in Python, Push(SItem) where , SItem Class 12] Write A Function In Python, Push(Sitem) Where , Sitem](https://d1avenlh0i1xmr.cloudfront.net/331f6909-fca7-4599-a579-34f2f298995d/slide5.jpg)

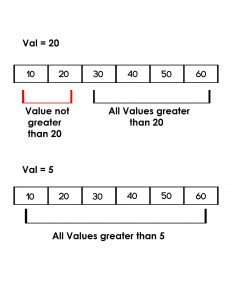
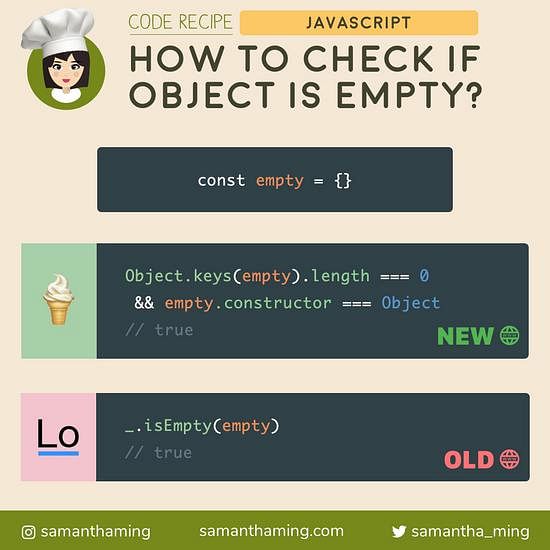
Article link: python check if dictionary is empty.
Learn more about the topic python check if dictionary is empty.
- Python: Check if a Dictionary is Empty (5 Ways!) – Datagy
- How to check if a dictionary is empty? – Stack Overflow
- How can I determine if a dictionary is empty? – Python FAQ
- Python | Check if dictionary is empty – GeeksforGeeks
- How to check if a dictionary is empty in python – Javatpoint
- 5 Ways to Check If a Dictionary is Empty in Python – Finxter
- Python – Check if dictionary is empty – Tutorialspoint
- How to Check if Python Dictionary is Empty?
- Python: Check if a Dictionary is Empty, A Quick Guide
- Python: Check if Dictionary is Empty – STechies
See more: nhanvietluanvan.com/luat-hoc