Property Vs Field C#
In the world of C# programming, there are two primary ways to store and access data within a class: fields and properties. While they may seem similar at first glance, fields and properties have distinct differences in terms of functionality, accessibility, and performance. Understanding these differences is crucial for writing clean, efficient, and maintainable code in C#. In this article, we will explore the concepts of fields and properties, compare them side by side, and discuss the best practices for choosing between them in different scenarios.
What is a Field in C#
A field in C# is a member variable of a class that stores data. It represents the data associated with an object of a particular class. Fields are typically declared at the top of the class and can be accessed throughout the class. They are defined using specific data types and can be initialized with default values. Here are some key points about fields:
– Definition and purpose of a field: A field is a variable that holds data associated with an object. It represents the state of an object and can be used to store information such as names, ages, or any other relevant data.
– How to declare and initialize a field: Fields are declared within a class using the syntax: access_modifier data_type field_name = initial_value;. For example, to declare an integer field called “age” with an initial value of 0, you would use: private int age = 0;
– Access modifiers for fields: Fields can have different access modifiers like public, private, protected, internal, or protected internal. The access modifier defines the visibility and accessibility of the field within and outside the class.
– Differences between static and instance fields: Static fields are associated with a class rather than an instance of the class. They are shared across multiple instances of the class. Instance fields, on the other hand, belong to a specific instance of the class and have separate values for each instance.
– Advantages and disadvantages of using fields: Fields provide direct access to data and are generally faster to access and modify than properties. However, they lack the validation and encapsulation features provided by properties.
What is a Property in C#
A property in C# is a member of a class that provides controlled access to a private field. It allows reading and/or writing of data associated with an object. Properties are used to encapsulate fields and provide a layer of abstraction for accessing and manipulating data. Here are some key points about properties:
– Definition and purpose of a property: A property is a mechanism that allows controlled access to the private data of a class. It provides a way to expose data while enforcing validation, encapsulation, or computation logic.
– How to declare and use properties: Properties are declared using the syntax: access_modifier data_type PropertyName { get; set; }. For example, to declare a string property called “Name”, you would use: public string Name { get; set; }.
– Access modifiers for properties: Properties can have different access modifiers like public, private, protected, internal, or protected internal. The access modifier defines the visibility and accessibility of the property within and outside the class.
– Differences between read-only and read-write properties: Read-only properties provide only a getter method, allowing the data to be read but not modified. Read-write properties provide both a getter and a setter method, allowing the data to be read and modified.
– Benefits of using properties over fields: Properties offer several advantages over fields. They provide a way to enforce validation and encapsulation, allowing for controlled access to data. They also allow for flexibility in defining custom logic for getting and setting data.
Comparison between Fields and Properties
Now let’s compare fields and properties side by side in terms of various factors:
1. Accessing and modifying values:
– Fields: Values stored in fields can be directly accessed and modified. For example, if the field “age” is public, you can access and modify its value using objectName.age.
– Properties: Values stored in properties can be accessed and modified using getter and setter methods. For example, if the property “Age” is public, you can access and modify its value using objectName.Age.
2. Encapsulation and data validation:
– Fields: Fields lack the ability to enforce validation or provide encapsulation by default. Data stored in fields can be accessed and modified without any restrictions.
– Properties: Properties provide a layer of abstraction over fields, allowing for validation and encapsulation. They allow you to define custom logic for getting and setting data, enabling you to control the access and modification of data.
3. Inheritance and polymorphism:
– Fields: Fields can be hidden or shadowed in derived classes using the “new” keyword. This means that the derived class can declare a field with the same name as a field in the base class, effectively hiding it.
– Properties: Properties can be overridden in derived classes using the “override” keyword. This allows derived classes to provide their own implementation for the getter and/or setter methods of a property defined in the base class.
4. Performance considerations:
– Fields: Accessing and modifying fields is generally faster than using properties because it involves direct memory access. However, fields lack the ability to perform additional logic or validation during access or modification.
– Properties: Accessing and modifying properties involves method calls, which can introduce some performance overhead compared to direct field access. However, properties provide flexibility for implementing validation or computation logic.
Best practices for choosing between fields and properties
Choosing between fields and properties depends on the specific requirements and constraints of your project. Here are some best practices to consider:
– Use fields for simple data storage or internal calculations where direct access and modification are sufficient.
– Use properties when you need to enforce validation, encapsulation, or custom logic during access or modification of data.
– Prefer properties over public fields to provide a layer of abstraction and preserve the flexibility to change the internal implementation without affecting external code.
– Consider the performance impact when using properties, especially in performance-critical scenarios. Direct field access might be preferred for performance-sensitive code.
Examples and Use Cases
Let’s explore a few examples and use cases to illustrate the practical applications of fields and properties in C#:
1. Using fields for simple data storage:
Fields are commonly used to store simple data like names, ages, or any other relevant information. For example, a class representing a person might have fields like “name” and “age” to store the name and age of a person.
2. Leveraging properties for controlled access and manipulation of data:
Properties are useful when you want to enforce validation or encapsulation. For example, a property “Age” might enforce that the age cannot be negative. It can also provide additional logic like converting the age from years to months or vice versa.
3. Implementing computed properties with custom logic:
Properties can be used to implement computed properties, where the value is derived from other fields or properties. For example, a class representing a circle might have properties like “Area” and “Circumference” that calculate and return the corresponding values based on the radius field.
4. Real-world applications of fields and properties in object-oriented programming:
Fields and properties are extensively used in object-oriented programming to model real-world entities and their associated data. For example, in a banking system, a class representing a bank account might have fields for the account number and balance, and properties for the owner’s name and the account type.
Conclusion and Final Thoughts
Understanding the differences between fields and properties is crucial for writing clean, efficient, and maintainable code in C#. Fields provide direct access to data without any additional logic, while properties offer encapsulation, validation, and custom logic. By choosing the appropriate approach based on the specific requirements of your project, you can maximize code readability, maintainability, and performance. Remember to consider factors like direct access, encapsulation, inheritance, performance, and best practices when deciding between fields and properties.
Properties Vs Fields In C#
Keywords searched by users: property vs field c# Field vs property C#, Public field vs property C#, Variable vs property, C# set property value by name, Property và Field, In C# programming language the fields properties, and methods of a class are called the, Field C#, Automatic properties
Categories: Top 47 Property Vs Field C#
See more here: nhanvietluanvan.com
Field Vs Property C#
When programming in C#, developers often come across the decision of choosing between fields and properties. Both fields and properties are used to store data, but they have different characteristics and purposes. In this article, we will explore the differences between fields and properties in C# and discuss when to use each.
Fields in C#
In simple terms, a field is a variable that holds data within a class or struct. It is typically declared at the class or struct level and is accessible throughout the class or struct. Fields are often private and can only be accessed by other members of the class or struct.
For example, consider the following class:
“`
public class Person
{
private string name;
private int age;
// Constructors and other members…
}
“`
In this case, `name` and `age` are fields that store the name and age of a person. These fields can be accessed by other members of the `Person` class, but not from outside the class.
Properties in C#
Properties, on the other hand, provide a level of abstraction over fields. They define a special method called an accessor that allows us to get or set the value of a private field. Properties provide a way to control the access to a field and perform additional logic if needed.
To convert the fields in the previous example to properties, we can use the following syntax:
“`
public class Person
{
private string name;
private int age;
public string Name
{
get { return name; }
set { name = value; }
}
public int Age
{
get { return age; }
set { age = value; }
}
// Constructors and other members…
}
“`
In this updated code, `Name` and `Age` are properties that encapsulate the private fields `name` and `age`. The `get` and `set` blocks define the accessor methods to read and write the values. These properties can be accessed from outside the class, allowing other parts of the program to interact with the data safely.
When to Use Fields and Properties
Choosing between fields and properties depends on the specific scenario and requirements of your code. Here are some guidelines to help you make the right decision:
1. Use fields when:
– You have data that does not require additional logic or validation before being accessed or modified.
– You have internal data that should not be directly accessible from outside the class.
2. Use properties when:
– You want to provide controlled access to the underlying data by adding logic or validation.
– You want to expose data to the outside world while hiding the implementation details.
– You need to raise events or perform additional actions when the data is accessed or modified.
Frequently Asked Questions (FAQs)
Q: Can I use fields and properties together in a class?
A: Yes, it is common to use a combination of fields and properties within a class. Fields are typically used for private data, while properties are used for public or protected data.
Q: Can I change a field to a property or vice versa without breaking the code?
A: Changing a field to a property or vice versa can potentially break the code, especially if the field or property is accessed directly by other parts of the program. It is important to consider the impact of such a change and ensure that it does not introduce any unexpected behavior.
Q: Are properties slower than fields?
A: In most cases, the performance difference between using properties and fields is negligible. The .NET runtime optimizes properties internally, and the overhead they introduce is generally insignificant unless the property includes complex logic or external dependencies.
Q: Are there any naming conventions for fields and properties in C#?
A: Yes, it is common to use camelCase for fields and PascalCase for properties. This convention helps to differentiate between fields and properties and improves code readability.
Q: Can I provide a default value for a property in C#?
A: Yes, you can set a default value for a property by initializing the underlying field in the class constructor. Alternatively, you can use the C# 6.0+ syntax to provide a default value directly in the property definition.
In conclusion, fields and properties are both important components of C# programming. It is essential to understand their differences and use cases to make informed decisions in your code. Fields provide a simple way to store data, while properties add flexibility and control over data access. By choosing the right approach, you can write cleaner and more maintainable code.
Public Field Vs Property C#
When it comes to programming in C#, there are several important concepts to understand. One crucial aspect is the use of public fields and properties. Both serve as a way to access and modify data within a class, but they have distinct differences. In this article, we will delve into the topic of public field vs property in C#, exploring their characteristics, benefits, and best practices.
Understanding Public Fields and Properties
In C#, both public fields and properties are used to expose data from a class. They act as gateways, allowing other code to interact with the data contained within the class. However, it is necessary to differentiate between these two constructs to choose the appropriate one for each situation.
Public fields are simple variables declared within a class. They can be accessed and modified directly by any code that has access to the instance of the class. For example, consider a class called “Person” with a public field called “Name”:
“`
public class Person
{
public string Name;
}
“`
In this case, any code that has an instance of the “Person” class can directly access its “Name” field. However, this direct access can raise concerns because it can bypass any validation or constraints that might be implemented by the class.
On the other hand, properties provide an additional layer of control over the access and modification of data. They are implemented using methods called “accessors.” Properties allow you to define custom logic for reading (get) and writing (set) data. Let’s modify our previous example to use properties instead:
“`
public class Person
{
private string _name;
public string Name
{
get { return _name; }
set { _name = value; }
}
}
“`
In this case, the “Name” property consists of a private backing field called “_name” and two accessors that define how to get or set the value of the property. The private backing field ensures that the data cannot be accessed directly, and the accessors provide the flexibility to enforce any necessary logic.
Benefits of Using Properties
The use of properties instead of public fields brings several benefits to C# programming projects. Here are some of the main advantages:
1. Data Encapsulation: Properties permit better data encapsulation by hiding the internal implementation details of how the data is being stored or constrained. This encapsulation promotes code maintainability and modularity.
2. Validation and Constraints: Since public fields lack control over data assignment, properties provide an opportunity to validate and enforce constraints on the incoming data. By defining custom logic within accessors, one can ensure that data is assigned only if it meets certain criteria.
3. Compatibility with Reflection: Properties play well with C#’s reflection mechanism, allowing developers to introspect and manipulate objects at runtime. Reflection enables powerful features like dynamic object creation and invocation.
4. Code Consistency: The use of properties promotes a consistent style throughout the codebase. It provides a clear indication that accessing and modifying the data should be done through properties rather than bypassing them by directly modifying fields.
Best Practices
While properties offer numerous advantages, it is essential to use them judiciously. Here are some best practices to follow:
1. Consider Using Properties for All Data Access: It is generally recommended to use properties for all data access instead of relying solely on public fields. This allows for future modifications and additions to the class without affecting the external code using the class.
2. Avoid Costly Operations in Properties: Since properties are accessed like fields, it is important to avoid performing expensive or time-consuming operations within property accessors. Accessors should be as lightweight as possible to maintain good performance.
3. Be Mindful of Multi-threading: When using properties in multi-threaded environments, ensure thread safety by considering the usage of locks or thread-safe data structures. This prevents potential race conditions and other synchronization issues.
4. Use Auto-implemented Properties Where Appropriate: C# provides a shorthand syntax called auto-implemented properties, which automatically generate the backing field and accessor code. When no additional logic is required, consider using this concise syntax:
“`
public string Name { get; set; }
“`
Frequently Asked Questions
Q: Can I change a public field to a property without affecting the existing code?
A: Yes, you can change a public field to a property without affecting the external usage of the class. This is due to a concept called binary compatibility in C#. However, directly modifying a field will require code changes if you change it to a property with validation or additional logic.
Q: Are properties slower than public fields?
A: Properties typically have negligible performance impact when compared to public fields. However, it’s still best to avoid expensive operations within property accessors to maintain good performance.
Q: How do I choose between a public field and a property?
A: As a general guideline, it is recommended to use properties for all data access whenever possible. They provide better control and encapsulation of data. Public fields may be appropriate in certain scenarios where simplicity and immediate visibility override these concerns.
In conclusion, understanding the differences between public fields and properties is crucial in C# development. Properties offer many advantages, such as data encapsulation, validation, and compatibility with reflection. By following best practices, developers can make informed decisions on when to use each construct and write cleaner, more maintainable code.
Images related to the topic property vs field c#
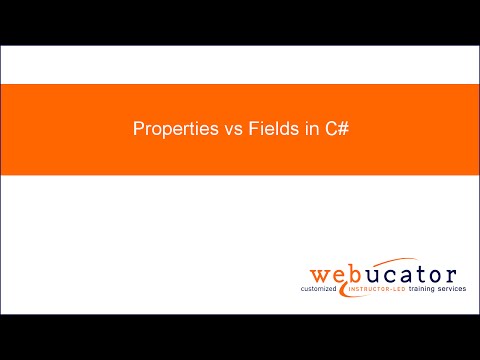
Article link: property vs field c#.
Learn more about the topic property vs field c#.
- What is the difference between a field and a property?
- Difference Between Field and Property in C# | Compare the …
- What is the difference between fields and properties in C#?
- Properties Vs Fields In C#. Fields are normal … – Medium
- Fields and Properties in C#
- Sự khác biệt giữ Filed và Property – How Kteam
- C# Field vs Property (with Examples) – Shekh Ali’s Blog
- Field vs Property in C# | Delft Stack
- Properties – C# Programming Guide | Microsoft Learn
See more: nhanvietluanvan.com/luat-hoc