Prisma Many To Many
## The Concept of Many-to-Many Relationships in Prisma
In a relational database, a many-to-many relationship occurs when multiple records in one table are associated with multiple records in another table. For instance, consider the scenario of a library management system, where multiple books can be borrowed by multiple users. This scenario reflects a many-to-many relationship as each book can be borrowed by multiple users and each user can borrow multiple books.
Traditionally, managing many-to-many relationships required the use of intermediate tables commonly known as junction tables or join tables. These tables act as connectors between the two tables involved in the relationship. However, Prisma simplifies this process by abstracting away the need for explicit junction tables and providing a more intuitive and declarative way to model and work with many-to-many relationships.
## Understanding Prisma’s Data Modeling for Many-to-Many Relationships
Prisma’s data modeling approach for many-to-many relationships involves defining a relation between two tables using the `@relation` directive in the schema definition. The `@relation` directive allows you to specify the type of relationship (e.g., one-to-one, one-to-many, many-to-many), as well as additional configuration options.
To model a many-to-many relationship between two tables, you need to define a relation field in both tables, specifying the `@relation` directive with the appropriate configuration. For instance, in our library management system example, we would define a `User` model and a `Book` model, and establish a many-to-many relationship between them by adding a `books` field in the `User` model and a `borrowers` field in the `Book` model, both decorated with the `@relation` directive.
## Implementing Many-to-Many Relationships in Prisma’s Schema Definition
To implement a many-to-many relationship in Prisma’s schema definition, you need to define the relation fields in both models, specifying the appropriate `@relation` directive configuration. Let’s continue with our library management system example and create the schema definition for the `User` and `Book` models:
“`prisma
model User {
id Int @id @default(autoincrement())
name String
books Book[]
}
model Book {
id Int @id @default(autoincrement())
title String
borrowers User[]
}
“`
In this example, we have defined a many-to-many relationship between the `User` and `Book` models. The `books` field in the `User` model represents the books borrowed by the user, whereas the `borrowers` field in the `Book` model represents the users who have borrowed the book.
Note that Prisma automatically creates the necessary database tables and foreign key relationships based on the defined models and their relations.
## Creating Many-to-Many Relationships Between Two Tables in Prisma
Now that we have defined our schema, we can create many-to-many relationships between the `User` and `Book` tables using Prisma’s CRUD operations. Prisma provides a convenient API for creating relationships between tables.
To create a relationship between a user and a book, we can use the `connect` operation. For example, to associate a user with a book, we can execute the following code:
“`javascript
const book = await prisma.book.create({
data: {
title: “The Pragmatic Programmer”,
borrowers: {
connect: [{ id: 1 }, { id: 2 }, { id: 3 }] // Connect the book to users with IDs 1, 2, and 3
}
}
});
“`
In this example, the book with the title “The Pragmatic Programmer” is being associated with the users with IDs 1, 2, and 3.
## Retrieving and Querying Many-to-Many Relationships in Prisma
Retrieving and querying data from many-to-many relationships in Prisma is straightforward using Prisma’s powerful query capabilities. Prisma provides a simple and expressive query API that allows you to fetch data based on various conditions and filters.
To retrieve all the books borrowed by a specific user, we can use the following code:
“`javascript
const userBooks = await prisma.user
.findUnique({
where: { id: 1 } // Fetch the user with ID 1
})
.books(); // Fetch all the books associated with the user
“`
This code fetches the user with ID 1 and retrieves all the books associated with that user.
Similarly, we can query for all the books borrowed by multiple users by using the `findMany` operation with appropriate conditions and filters.
## Modifying Many-to-Many Relationships in Prisma
Modifying many-to-many relationships in Prisma involves updating the relationship between entities by creating, connecting, disconnecting, or deleting records.
For instance, if we want to add a book to a user’s borrowed books, we can use the `connect` operation as follows:
“`javascript
const updatedUser = await prisma.user.update({
where: { id: 1 },
data: {
books: {
connect: [{ id: 4 }] // Connect the book with ID 4 to the user’s borrowed books
}
}
});
“`
In this example, the book with ID 4 is being connected to the user’s borrowed books.
To remove a book from a user’s borrowed books, we can use the `disconnect` operation:
“`javascript
const updatedUser = await prisma.user.update({
where: { id: 1 },
data: {
books: {
disconnect: [{ id: 4 }] // Disconnect the book with ID 4 from the user’s borrowed books
}
}
});
“`
This code disconnects the book with ID 4 from the user’s borrowed books.
## Handling Updates and Deletions in Many-to-Many Relationships in Prisma
When updating or deleting entities with many-to-many relationships in Prisma, it’s crucial to consider how the relationships will be affected. By default, if you update an entity, Prisma will update the associated relationships based on your changes. Similarly, if you delete an entity, Prisma will take care of deleting the relationships as well.
For example, if we update a book’s information, the associated relationships will be preserved:
“`javascript
const updatedBook = await prisma.book.update({
where: { id: 1 },
data: {
title: “New Title” // Update the book’s title
}
});
“`
This update will only affect the book’s title, leaving the many-to-many relationships intact.
On the other hand, if we delete a user, the associated relationships will be updated accordingly:
“`javascript
const deletedUser = await prisma.user.delete({
where: { id: 1 } // Delete the user with ID 1
});
“`
Prisma will automatically remove any associations between the deleted user and the associated books.
## Best Practices and Considerations for Many-to-Many Relationships in Prisma
When working with many-to-many relationships in Prisma, it’s essential to keep a few best practices and considerations in mind:
1. **Data Consistency**: Ensure that your data remains consistent by properly managing relationships and performing the necessary validations to maintain data integrity.
2. **Performance**: Consider the performance implications of querying and modifying many-to-many relationships, especially when dealing with large datasets. Proper indexing and query optimization techniques can improve performance significantly.
3. **Error Handling**: Handle errors and exceptions that may occur during relationship management, such as unique constraint violations or missing records.
4. **Scalability**: Design your data model and relationships with scalability in mind. As your application grows, you may need to consider sharding or partitioning strategies to handle increased load and data volumes.
FAQs:
**1. What is a one-to-many relationship in Prisma?**
A one-to-many relationship in Prisma occurs when a record in one table can be associated with multiple records in another table. For example, a `User` can have multiple `Posts`, representing a one-to-many relationship between the two tables.
**2. How can I find multiple related records in Prisma?**
You can find multiple related records in Prisma by using the `findMany` operation with appropriate conditions and filters. For example, to find all the posts written by a specific user, you can use `prisma.post.findMany({ where: { author: { id: 1 } } })`.
**3. How can I update many-to-many relationships in Prisma?**
Updating many-to-many relationships in Prisma involves using the `connect`, `disconnect`, or `set` operations. The `connect` operation connects related records, `disconnect` operation disconnects related records, and `set` operation replaces the entire set of related records.
**4. How can I query with multiple conditions in Prisma?**
You can query with multiple conditions in Prisma by using the `where` parameter of the respective Prisma query operation. For example, to find users with a specific name and age, you can use `prisma.user.findMany({ where: { name: “John”, age: 30 } })`.
**5. How can I perform transactions in Prisma?**
Prisma supports transactions through the use of the `prisma.$transaction` method. Transactions allow you to execute multiple operations as a single unit of work, ensuring data consistency and integrity.
**6. What does “Unique constraint failed on the constraint” error mean in Prisma?**
The “Unique constraint failed on the constraint” error in Prisma is thrown when a unique constraint defined in the database schema is violated. This typically occurs when you try to insert or update a record with a value that already exists in a column with a unique constraint.
**7. How can I handle dates in Prisma?**
You can handle dates in Prisma by using the `DateTime` scalar type provided by Prisma. This scalar type represents a date and time value and can be used to define date-related fields in your Prisma models.
**8. How can I check if a relation exists in Prisma?**
You can check if a relation exists in Prisma by using the `include` parameter with the respective Prisma query operation. Including the related field in the query result will indicate that a relation exists.
In conclusion, Prisma provides a robust set of tools and features to effectively handle many-to-many relationships in your application’s data model. By understanding the concept of many-to-many relationships in Prisma, modeling them in the schema definition, and utilizing Prisma’s query and mutation capabilities, you can efficiently work with these complex relationships in your database management system. Remember to follow best practices and consider performance, scalability, and data consistency while working with many-to-many relationships in Prisma.
How To Model Relationships (1-1, 1-M, M-M)
What Is A Prisma Many-To-Many Connection?
Prisma is an open-source database toolkit that simplifies database access for developers. It offers an ORM-like interface to interact with databases and provides powerful features like automatic schema generation, data modeling, and a type-safe query builder. One of Prisma’s key features is its ability to handle many-to-many relationships between tables efficiently.
In traditional database systems, a many-to-many relationship between two tables is typically implemented using an intermediate table referred to as a junction or associative table. This table helps establish the relationship by storing the foreign keys of both tables. For example, let’s consider a scenario where we have two tables: “Users” and “Projects.” A user can be associated with multiple projects, and a project can have multiple users. To represent this relationship accurately, we create an “User_Projects” table that holds the user_id and project_id as foreign keys.
However, managing this intermediate table manually might become cumbersome as the complexity of the system grows. This is where Prisma’s many-to-many connection feature proves to be incredibly beneficial. Prisma automates the process of creating and managing the intermediate table, allowing developers to focus on the application’s logic rather than database intricacies.
Setting up a many-to-many connection in Prisma involves a few straightforward steps. First, we define our data model using Prisma’s declarative language, Prisma Schema. Let’s take our previous example and model it using Prisma:
“`prisma
model User {
id Int @id @default(autoincrement())
name String
projects Project[]
}
model Project {
id Int @id @default(autoincrement())
name String
users User[]
}
“`
In this example, we have two models: “User” and “Project.” The `User` model has a one-to-many relationship with the `Project` model, and vice versa. This relation is defined by the `projects` field in the `User` model and the `users` field in the `Project` model.
Once the schema is defined, Prisma generates the necessary database tables and SQL queries at compile-time. Prisma automatically creates the intermediate table and handles the insertion, retrieval, and deletion of related records smoothly. It provides convenient methods and functions to interact with the many-to-many relationship effortlessly.
Some of the common operations you can perform with Prisma’s many-to-many connection include:
1. Adding a relation: To link a user to a project, you can simply use the generated method `addToProjects` on the `User` model:
“`javascript
const user = await prisma.user.findUnique({ where: { id: 1 } });
const project = await prisma.project.findUnique({ where: { id: 1 } });
await prisma.user.update({
where: { id: user.id },
data: {
projects: {
connect: { id: project.id },
},
},
});
“`
2. Retrieving all related records: To fetch all the projects associated with a user, Prisma offers the `include` directive to eager-load the related records:
“`javascript
const user = await prisma.user.findUnique({
where: { id: 1 },
include: { projects: true },
});
console.log(user.projects); // Array of projects
“`
3. Removing a relation: If you wish to remove a user’s association with a project, Prisma provides the `disconnect` directive:
“`javascript
await prisma.user.update({
where: { id: user.id },
data: {
projects: {
disconnect: { id: project.id },
},
},
});
“`
These are just a few examples of how Prisma’s many-to-many connection simplifies the management of complex relationships in databases. It greatly reduces the amount of boilerplate code, minimizes errors, and improves overall developer productivity.
FAQs:
Q: Can Prisma handle additional fields in the intermediate table?
A: Yes, Prisma allows you to add additional fields to the intermediate table, enabling you to store related information specific to the relationship.
Q: Does Prisma support many-to-many connections with more than two tables?
A: Yes, Prisma supports many-to-many connections involving multiple tables. You simply need to define the relationships between the models and let Prisma handle the rest.
Q: Can Prisma work with different databases?
A: Yes, Prisma supports various databases like PostgreSQL, MySQL, SQLite, and SQL Server. You can switch between different databases easily without needing to modify your Prisma schema.
Q: Can I customize the naming conventions used by Prisma?
A: Yes, Prisma allows you to configure the naming conventions for tables, columns, and other database-related elements, providing flexibility based on your preferences or existing conventions.
Q: Is Prisma suitable for large-scale applications?
A: Absolutely! Prisma has proven to be performant even in large-scale applications with complex data models and high query volumes. It offers features like query batching and caching to optimize query execution time.
In conclusion, Prisma’s many-to-many connection feature simplifies the management of complex relationships in databases by automating the creation and maintenance of intermediate tables. It frees developers from handling tedious database-related tasks, enabling them to focus on building robust applications efficiently. With Prisma, many-to-many relationships become a breeze to work with.
What Is An Example Of A Many-To-Many Relationship?
In the world of databases and data management, relationships between entities play a crucial role in organizing and representing information. When it comes to structuring relationships between entities, one common scenario is the many-to-many relationship. This type of relationship occurs when multiple records on one side of the relationship can be associated with multiple records on the other side.
To understand the concept of a many-to-many relationship, let’s delve into an example that is quite familiar to most of us – the relationship between students and courses in an educational institution.
Imagine you are responsible for designing a database for a university. In this scenario, multiple students can enroll in multiple courses, and a single course can have multiple students. Here, we have a classic many-to-many relationship between students and courses.
To represent this relationship accurately in a database, we would need three tables:
1. Student table: This table would contain information about each student, such as their ID, name, contact details, etc.
2. Course table: This table would store details about each course offered by the university, including the course name, course code, duration, etc.
3. Enrollment table: This table acts as a bridge between the student and course tables, capturing the associations between students and the courses they are enrolled in. It would typically include the student ID and the course ID as foreign keys.
By establishing this many-to-many relationship, we can ensure that each student can be associated with multiple courses, and each course can have enrollment from multiple students. This flexible and efficient representation allows for easier querying and management of data.
Another example of a many-to-many relationship can be seen in the relationship between employees and projects. In a company, multiple employees can work on multiple projects, and each project can involve multiple employees. By defining an appropriate database structure with separate tables for employees, projects, and a mapping table to establish the relationship, we can effectively manage these complex associations.
It’s worth noting that many-to-many relationships are not limited to just two entities. They can involve any number of entities where multiple occurrences on one side can be associated with multiple occurrences on the other side.
FAQs about many-to-many relationships:
Q: Are many-to-many relationships common in database design?
A: Yes, many-to-many relationships are quite common in database design as they accurately represent real-world scenarios where multiple entities can be associated with multiple others.
Q: Can you provide more examples of many-to-many relationships?
A: Apart from the student-course and employee-project examples, there are multiple other instances of many-to-many relationships. Some examples include authors and books, actors and movies, tags and blog posts, and users and groups in a social media platform.
Q: How is a many-to-many relationship represented in an entity-relationship diagram (ERD)?
A: In an ERD, a many-to-many relationship is depicted by connecting the two entities with a double line. It also shows the cardinality of the relationship on each side (e.g., “0 to many,” “1 to many”).
Q: Are there any challenges in managing many-to-many relationships?
A: While many-to-many relationships provide a flexible way to model complex associations, they can pose challenges when it comes to querying and maintaining data integrity. However, these challenges can be overcome with proper database design and the use of appropriate techniques such as indexing and referential integrity constraints.
Q: Can a many-to-many relationship be converted into a one-to-many relationship?
A: In certain scenarios, a many-to-many relationship can be converted into one or more one-to-many relationships by introducing an intermediate table or entity. This helps break down the complex relationship into simpler ones, improving manageability.
In conclusion, many-to-many relationships are a vital tool in database design for representing complex associations between entities. From students and courses to employees and projects, these relationships allow multiple occurrences on one side to be associated with multiple occurrences on the other. By establishing appropriate tables and mapping entities, we can accurately organize and retrieve information, ultimately aiding in efficient data management.
Keywords searched by users: prisma many to many Prisma one-to-many, Findmany relation prisma, Update many-to-many prisma, Prisma where multiple conditions, Prisma transaction, Unique constraint failed on the constraint prisma, Date prisma, Prisma where relation exists
Categories: Top 22 Prisma Many To Many
See more here: nhanvietluanvan.com
Prisma One-To-Many
In the realm of data management, efficient and flexible relationships between different tables are crucial for optimizing the performance and functionality of applications. Prisma, a modern database toolkit, offers a powerful feature known as “Prisma One-to-Many” that simplifies the management and querying of one-to-many relationships in databases. In this article, we will explore the basics of Prisma, delve into the concept of one-to-many relationships, and discuss how Prisma’s One-to-Many functionality can enhance the development process.
Understanding Prisma and Database Relationships
Prisma is an open-source database toolkit that acts as an intermediary layer between your application and your database. It provides an amazing set of tools and building blocks that simplify complex database queries, schema management, and overall data access. Prisma allows developers to work with databases using the Prisma Client, an auto-generated query builder that eliminates the need for writing raw SQL queries.
Database relationships, particularly one-to-many relationships, play a fundamental role in organizing and structuring data. In a one-to-many relationship, a record in one table can be associated with multiple records in another table. For example, consider a scenario where we have two tables: “customers” and “orders.” Each customer can have multiple orders associated with them. The relationship between the “customers” and “orders” table is a typical one-to-many relationship, as one customer can have several orders.
One-to-many relationships are prevalent in all kinds of applications, ranging from e-commerce platforms to social media. Efficiently managing these relationships and retrieving data can become complicated, especially as databases grow in size and complexity. This is where Prisma’s One-to-Many functionality comes into play, providing an elegant solution for developers.
Introducing Prisma One-to-Many
Prisma’s One-to-Many functionality simplifies the process of working with one-to-many relationships in databases. Using Prisma, developers can effortlessly define and query one-to-many relationships without dealing with complex SQL joins or writing repetitive code. With Prisma One-to-Many, developers can focus more on building features and less on implementing intricate database relationships.
To understand Prisma One-to-Many, let’s continue with the earlier example. Imagine we have a Prisma schema that outlines the structure of our “customers” and “orders” tables. With Prisma, we can easily define the relationship between these two tables using a simple syntax. By adding a “relationship” field in our schema, Prisma automatically generates the necessary database queries and relationships.
Once the relationship is defined, Prisma enables powerful querying capabilities. Developers can effortlessly navigate the one-to-many relationship and retrieve associated data using intuitive query builders provided by Prisma. The resulting code is concise, readable, and optimized, ensuring efficient retrieval of data from the database.
Benefits of Prisma One-to-Many
Prisma One-to-Many offers numerous benefits to both developers and applications that rely on complex database relationships. Some notable advantages include:
1. Simplified Codebase: Prisma One-to-Many greatly reduces the amount of code required to handle one-to-many relationships. By eliminating the need for raw SQL joins and repetitive code, developers can write cleaner code, leading to improved code maintainability and reduced bugs.
2. Faster Development: With Prisma abstracting away complex database interactions, developers can focus on building application features and logic rather than spending time tweaking queries and relationships. This results in faster development cycles and increased productivity.
3. Performance Optimization: Prisma’s query builders generate highly optimized SQL queries, avoiding costly joins and optimizing the retrieval process. This improves the performance of applications, enabling faster response times, and more efficient data retrieval.
4. Scalability and Flexibility: As applications grow and evolve, so do their associated databases. Prisma’s One-to-Many functionality ensures that as data structures change or expand, the relationships remain accurate and easily manageable. This provides developers with the flexibility to scale their applications without worrying about complex database restructuring.
5. Data Consistency: Prisma’s One-to-Many relationships guarantee data consistency by automatically managing relationships, ensuring that all records are properly linked within the database. This eliminates the risk of orphaned or inconsistent data, resulting in a reliable and well-structured database.
FAQs
Q: Can Prisma be used with any type of database?
A: Prisma currently supports PostgreSQL, MySQL, and SQLite. However, the Prisma team is actively working on expanding support for additional database systems.
Q: Is Prisma One-to-Many suitable for large-scale databases?
A: Absolutely! Prisma’s One-to-Many functionality is designed to handle databases of all sizes. Its optimized query generation and efficient data retrieval make it highly performant even with substantial amounts of data.
Q: Can I use Prisma One-to-Many with an existing database?
A: Yes, Prisma can be used with existing databases. By defining relationships within the Prisma schema, you can leverage Prisma’s One-to-Many functionality to simplify and enhance your existing database relationships.
Q: Is Prisma One-to-Many compatible with popular programming languages?
A: Prisma is compatible with various programming languages, including JavaScript/TypeScript, Python, and Go. It provides client libraries for these languages, allowing seamless integration with your preferred development stack.
In conclusion, Prisma’s One-to-Many functionality revolutionizes the way we manage one-to-many relationships in databases. By abstracting complex SQL joins and providing powerful query builders, Prisma simplifies the development process, enhances performance, and ensures consistent and scalable database relationships. With Prisma, developers can focus more on what matters most – building exceptional applications.
Findmany Relation Prisma
Introduction to FindMany Relation in Prisma
Prisma is a powerful and efficient Object-Relational Mapping (ORM) tool that facilitates database operations in various programming languages. One of the key features offered by Prisma is its built-in support for various types of relationships between database tables. In this article, we will explore the FindMany relation, one of the most commonly used relations in Prisma, and understand how it can be leveraged to build complex and efficient applications.
What is a FindMany Relation?
A FindMany relation is a type of relationship between two tables in a database. It represents a one-to-many relationship, where a single record in one table is associated with multiple records in another table. This type of relation is established by defining a foreign key in the child table that references the primary key of the parent table. With the help of Prisma’s ORM capabilities, developers can easily navigate and query data across these related tables.
Defining a FindMany Relation in Prisma
To establish a FindMany relation in Prisma, you need to define the relationship between two database models in your schema. Let’s consider a hypothetical example where we have two tables: “Author” and “Book”. An author can have multiple books, so the “Author” table will be the parent table while the “Book” table will be the child table.
In Prisma’s schema definition, you can define the FindMany relation as follows:
model Author {
id Int @id @default(autoincrement())
name String
books Book[]
}
model Book {
id Int @id @default(autoincrement())
title String
author Author @relation(fields: [authorId], references: [id])
authorId Int
}
In the above schema, the FindMany relation is established by defining an array of “Book” objects in the “Author” model and a foreign key “authorId” in the “Book” model that references the “id” field in the “Author” model.
Querying Data with FindMany Relation
Prisma’s FindMany relation enables intuitive querying of data across related tables, making it easy to fetch data from the child table based on the parent table. Let’s consider a scenario where we want to retrieve all the books written by a specific author.
With Prisma, performing such queries is straightforward. Here’s an example of how you can retrieve all books written by an author with a specific ID:
const books = await prisma.author.findUnique({
where: { id: authorId },
}).books();
The above code snippet uses Prisma’s findUnique method to retrieve the unique author based on the given ID and then uses the books() method to fetch all the books associated with that author. This simplifies the process of querying related data and allows for efficient retrieval of relevant information.
FAQs about FindMany Relation in Prisma
Q: Can I establish multiple FindMany relations between two tables?
A: Yes, you can establish multiple FindMany relations by defining multiple arrays of objects in the parent table, each representing a specific relationship with the child table.
Q: Can I apply filters while querying data using FindMany relation?
A: Yes, Prisma allows you to apply various filters, such as sorting, pagination, and conditional constraints, while querying data across related tables.
Q: Can I use FindMany relation in combination with other types of relations?
A: Yes, Prisma supports a wide range of relations, including One-to-One, One-to-Many, and Many-to-Many. You can combine FindMany relation with other relation types to build complex and comprehensive database models.
Q: Can I update data in both tables simultaneously using FindMany relation?
A: Yes, Prisma provides a convenient way to create, update, or delete data across related tables using its intuitive API. You can update data in both tables simultaneously with ease.
Q: Is the FindMany relation limited to specific databases?
A: No, Prisma supports various popular databases, such as PostgreSQL, MySQL, SQLite, and SQL Server. The FindMany relation can be used with any of these databases.
Conclusion
In this article, we explored the FindMany relation in Prisma, a powerful ORM tool used for efficient database operations in different programming languages. We learned how to define a FindMany relation in Prisma, query data across related tables, and discussed its various features and capabilities. With Prisma’s FindMany relation, developers can easily set up and manage one-to-many relationships in their applications, enhancing the efficiency and flexibility of their database operations.
Update Many-To-Many Prisma
In the realm of data modeling and database management, handling complex relationships between entities is a crucial aspect. One such relationship type, the many-to-many relationship, often poses challenges when it comes to efficiently managing the data. However, with the recent update to Prisma, a popular database toolkit, managing many-to-many relationships has become simpler and more intuitive. In this article, we will explore the update to many-to-many Prisma relationships, its significance, and how it enhances data modeling capabilities.
Understanding Many-to-Many Relationships
A many-to-many relationship exists between two entities when multiple instances of one entity can be associated with multiple instances of another entity. For example, consider a scenario in which a product can be sold by multiple salespersons, and each salesperson can sell multiple products. This creates a many-to-many relationship between the “Product” and “Salesperson” entities.
Traditionally, implementing many-to-many relationships in a database required an intermediary table, known as a junction or join table. This table would store the foreign keys of both entities, effectively establishing the relationship. However, managing the data and querying it efficiently could become cumbersome, especially as the complexity of relationships increased.
The Power of Prisma
Prisma, a modern database toolkit, simplifies the process of working with databases, making data modeling and querying more streamlined. It offers an easy-to-use Object-Relational Mapping (ORM) layer and provides a comprehensive set of APIs for performing database operations.
In the latest update, Prisma has introduced significant enhancements to its many-to-many relationship handling capabilities. It now offers a more intuitive and efficient way to manage data relationships, eliminating the need for the intermediary join table.
Utilizing the @relation Attribute
The update to many-to-many relationships in Prisma revolves around the usage of the @relation attribute. This attribute allows developers to define the relationship between entities directly in the Prisma schema.
By using the @relation attribute, developers can define the relationship type, its cardinality, and the fields through which the entities are related. Additionally, they can specify the `references` directive to determine the foreign keys used for relating the entities.
With these enhancements, developers can now establish many-to-many relationships by referencing the entities directly, eliminating the need for an intermediary table and reducing the complexity associated with managing such relationships.
Efficient Querying with Prisma Client
Apart from simplifying the modeling process, Prisma’s update enhances the querying capabilities for many-to-many relationships. Prisma automatically generates a specialized Prisma Client for each defined Prisma model, enabling efficient CRUD operations and sophisticated querying.
Developers can utilize the Prisma Client to query many-to-many relationships without worrying about constructing complex join queries manually. Prisma handles the querying logic internally, providing a seamless experience for developers.
FAQs
Q: How does the update to many-to-many Prisma relationships simplify data modeling?
A: The update allows developers to define many-to-many relationships directly in the Prisma schema, eliminating the need for an intermediary join table.
Q: What are the benefits of using Prisma for managing many-to-many relationships?
A: Prisma simplifies the process of working with databases, offers an intuitive way to define relationships, and provides efficient querying capabilities.
Q: Does Prisma generate code to handle many-to-many relationships automatically?
A: Yes, Prisma automatically generates a specialized Prisma Client for each model, enabling efficient CRUD operations and simplified querying on many-to-many relationships.
Q: Can existing many-to-many relationships be updated to utilize the new Prisma capabilities?
A: Yes, existing many-to-many relationships can be updated by modifying the Prisma schema to utilize the @relation attribute and the new relationship syntax.
Q: Are there any performance improvements associated with the update?
A: While the update primarily focuses on simplifying data modeling and querying, Prisma’s internal optimizations may result in improved performance for many-to-many relationships.
Conclusion
The recent update to Prisma’s many-to-many relationship handling capabilities has brought significant improvements to data modeling and querying. By simplifying the way relationships are defined and managed, Prisma has made it easier for developers to handle complex data structures efficiently. With its enhanced querying capabilities, Prisma provides a seamless experience for developers working with many-to-many relationships. As a result, Prisma continues to solidify its position as a top choice for modern data management and ORM solutions.
Images related to the topic prisma many to many
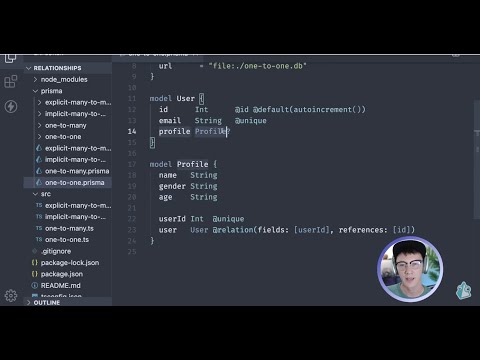
Found 31 images related to prisma many to many theme
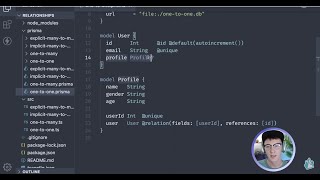
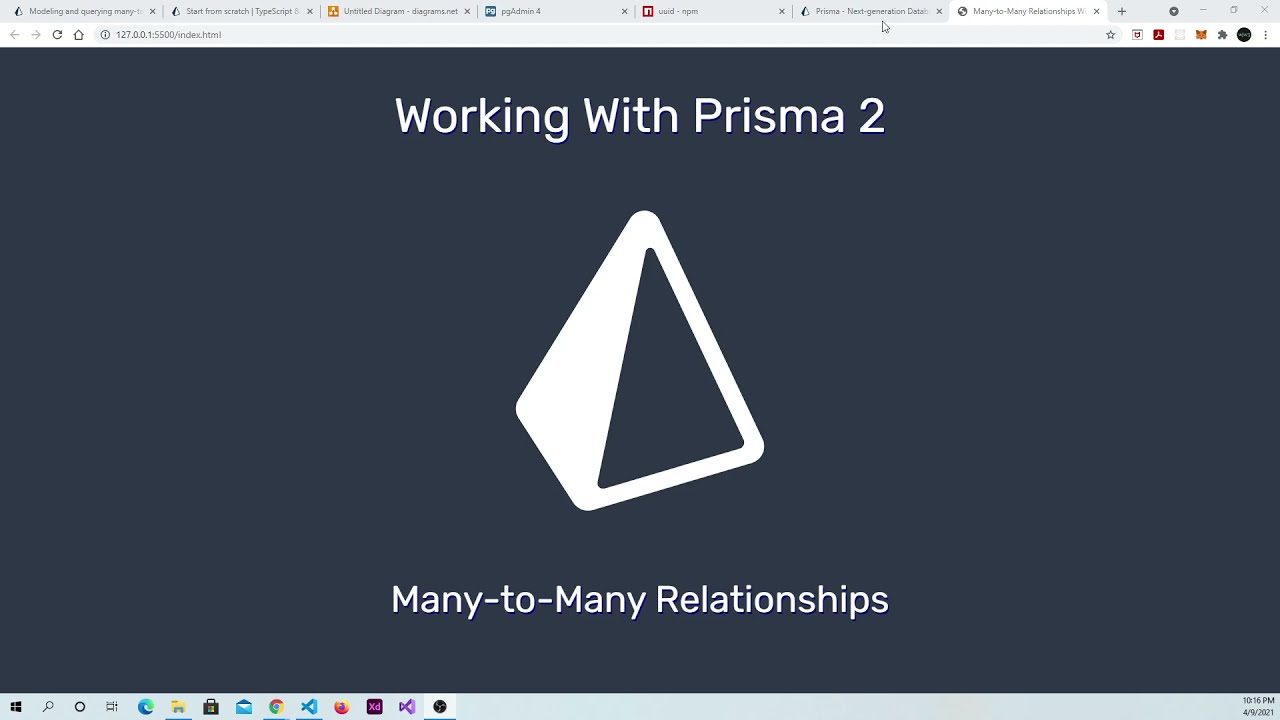

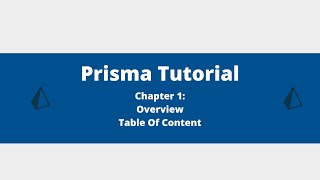



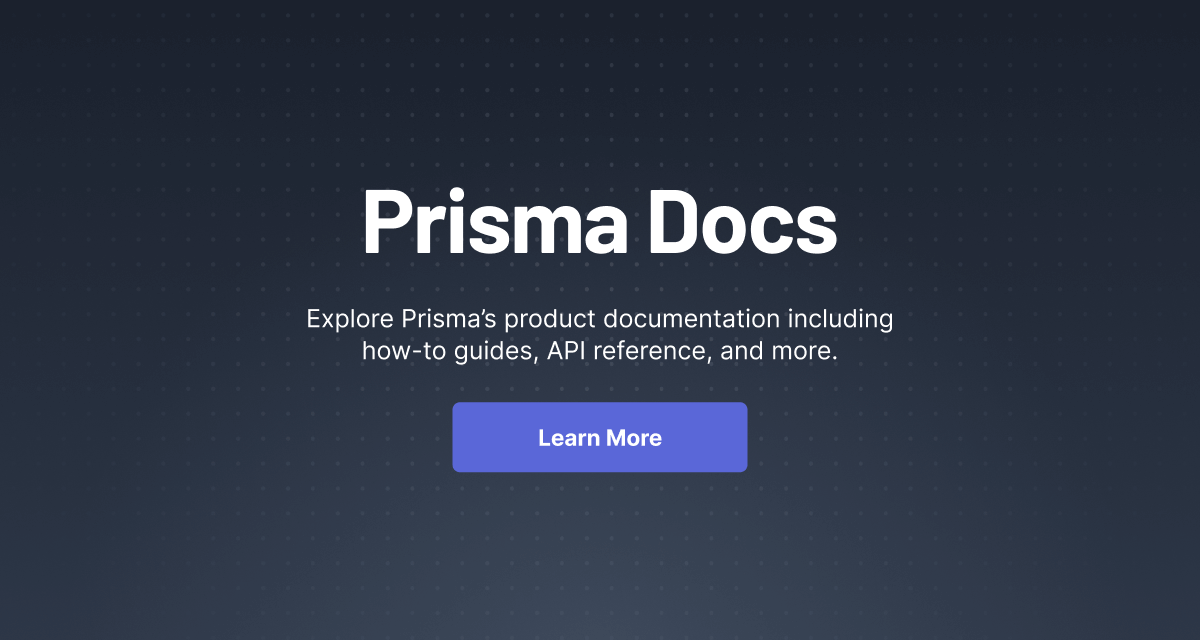



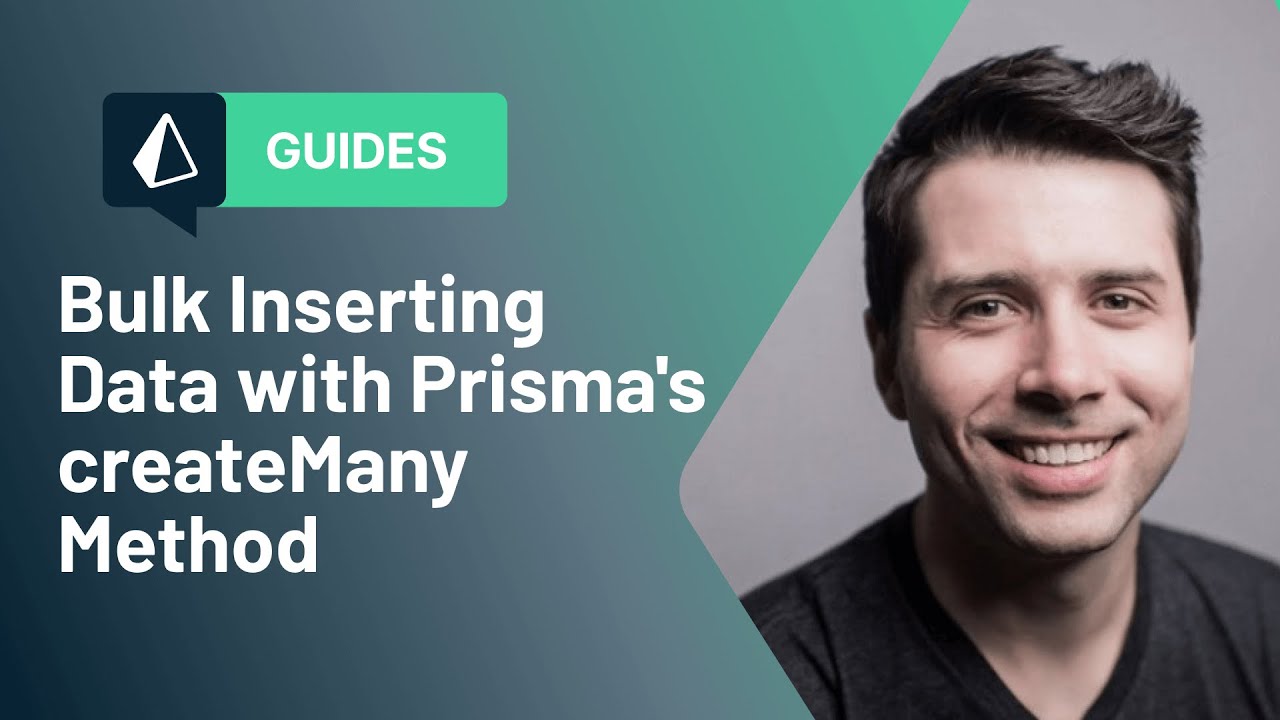
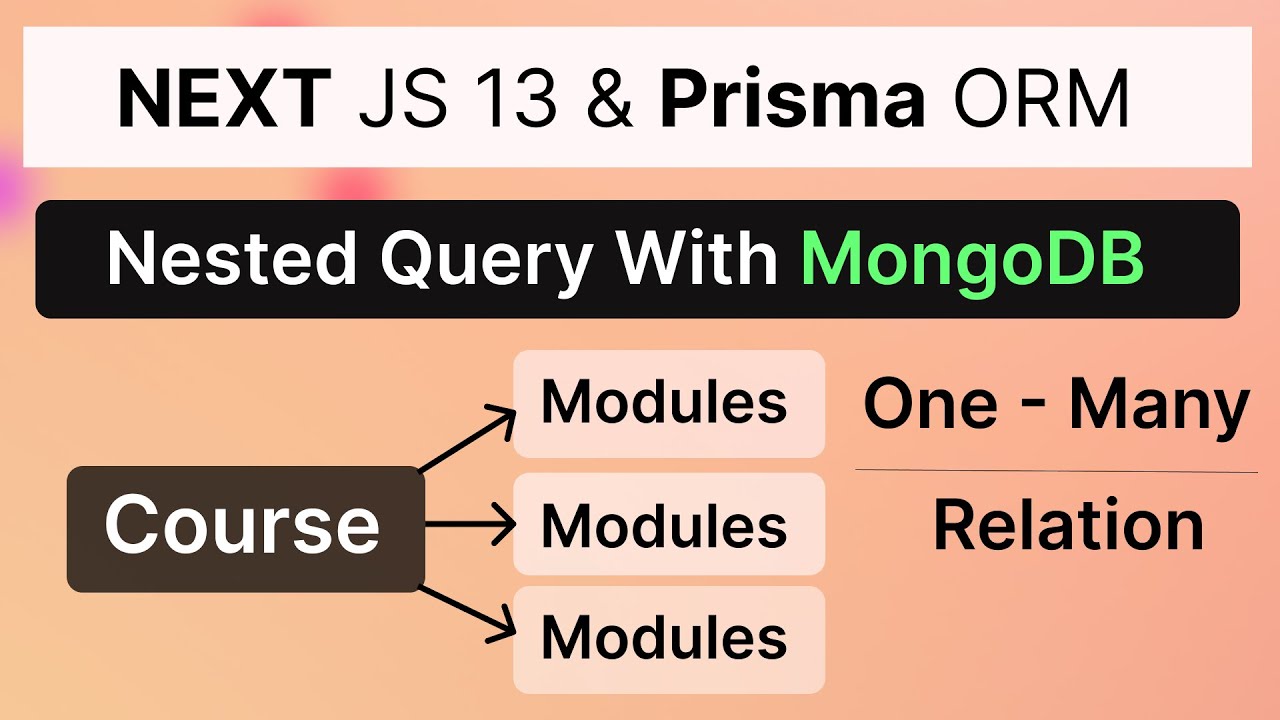
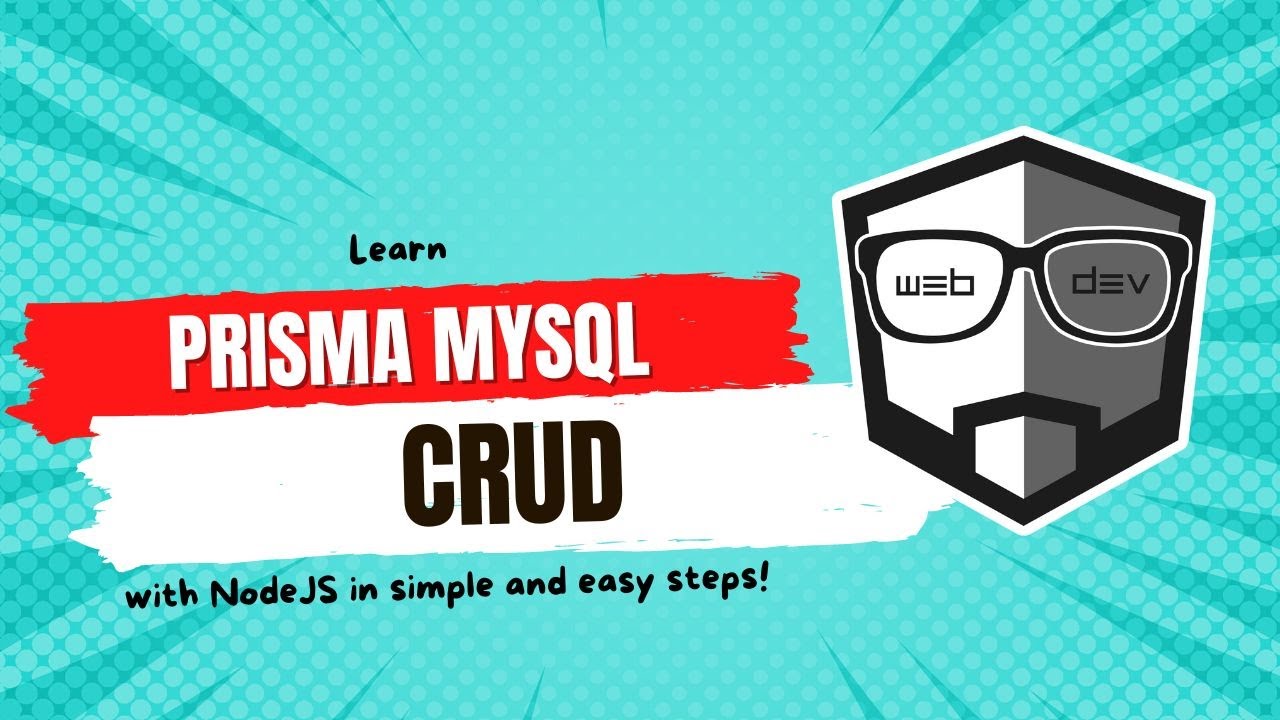
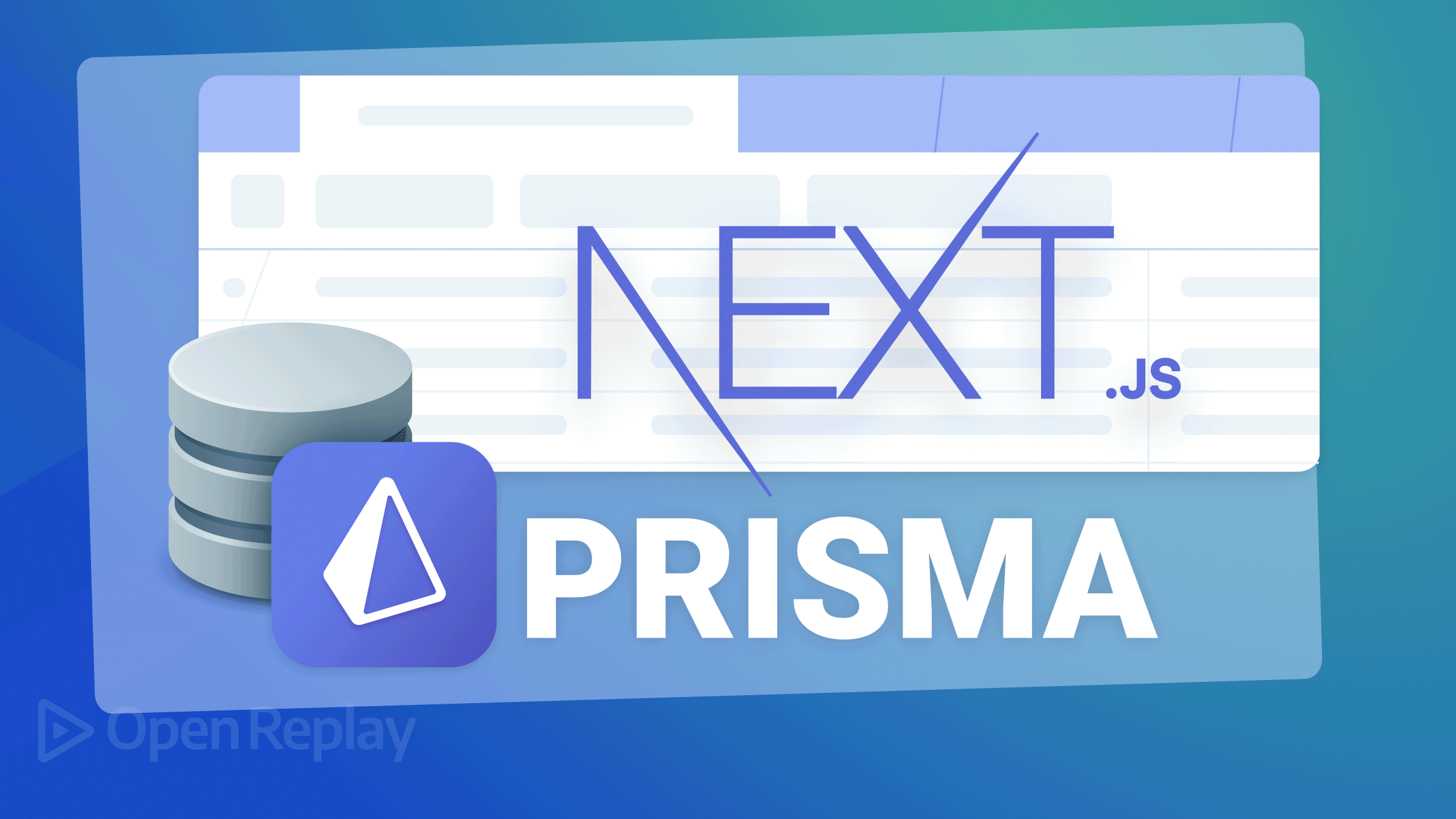
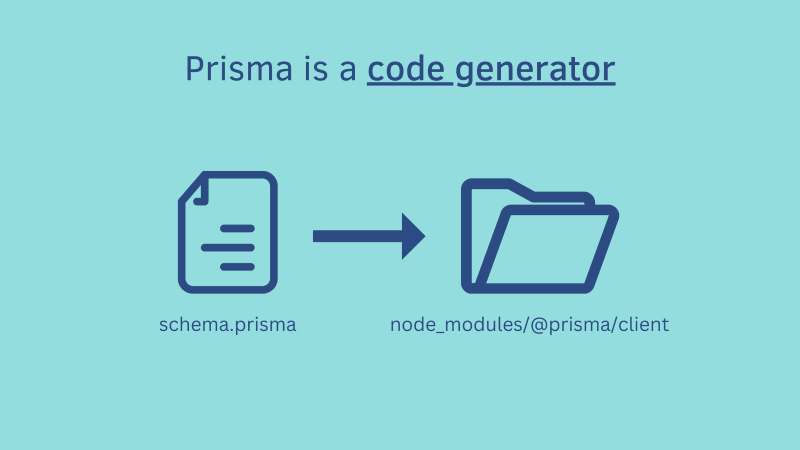
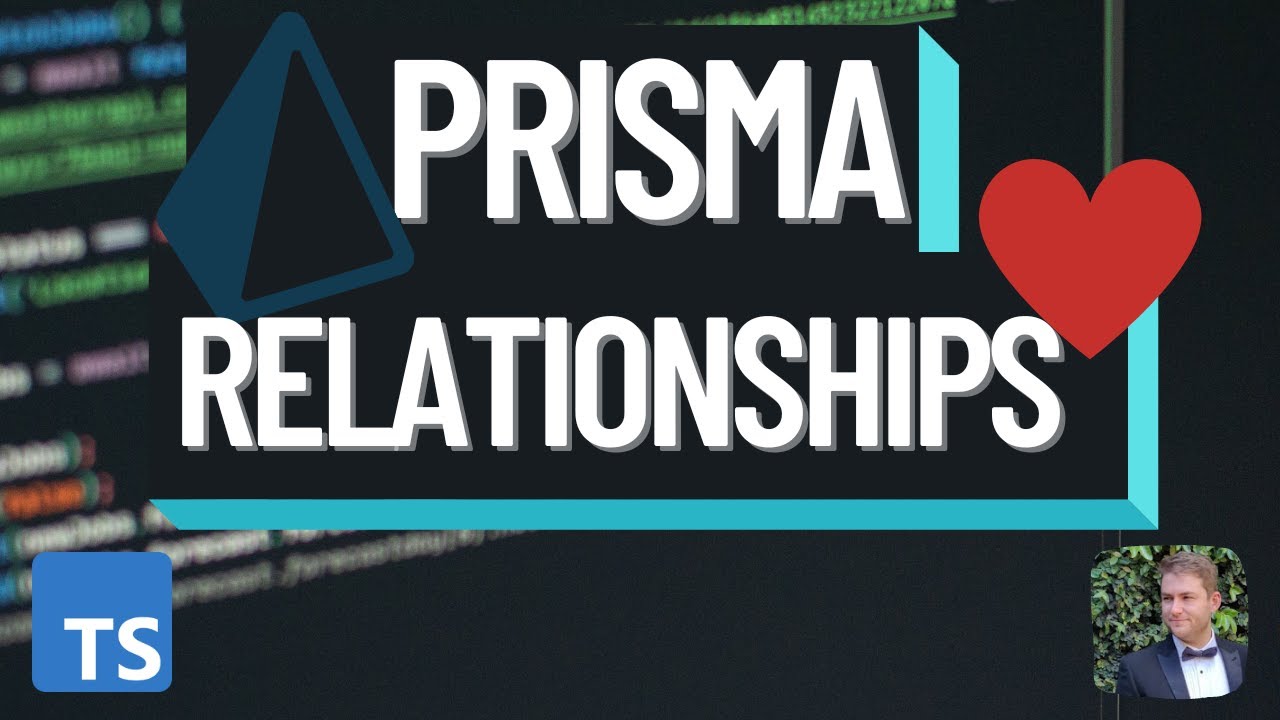
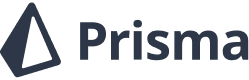
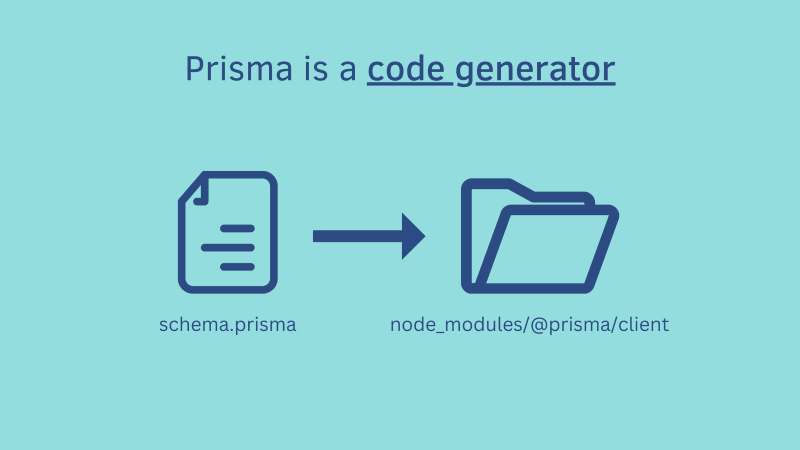

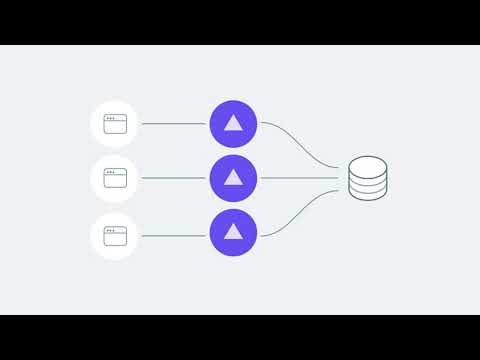
Article link: prisma many to many.
Learn more about the topic prisma many to many.
- Many-to-many relations – Prisma
- Video: Create many-to-many relationships – Microsoft Support
- Many-to-many relationships
- Tables Relations in SQL Server: One-to-One, One-to-Many, Many-to …
- Handle a Many-to-Many relationship with Prisma and Node.js
- Implicit or Explicit Many to Many relationship in prisma