Print A Vector C++
## 1. Introduction to Printing Vectors in C++
Before we dive into the various methods of printing vectors, let’s start with a brief introduction. A vector in C++ is a sequential container that can dynamically grow or shrink its size as elements are added or removed. It is part of the Standard Template Library (STL) and provides powerful features for efficient and flexible storage of elements.
## 2. Initializing and Populating a Vector in C++
Before we can print a vector, we need to initialize and populate it with data. This can be done using multiple approaches, such as initializing with values, filling with values, or even taking input from the user.
To initialize a vector with predefined values, we can use the initializer list syntax:
“`cpp
std::vector
“`
Alternatively, we can initialize an empty vector and fill it with values using the `push_back()` method:
“`cpp
std::vector
numbers.push_back(1);
numbers.push_back(2);
numbers.push_back(3);
“`
Another way to populate a vector is by taking input from the user. We can use a loop, such as a for loop or while loop, to read values from the user and add them to the vector.
## 3. Printing a Vector Using a for Loop in C++
One of the simplest ways to print a vector in C++ is by using a for loop. We iterate over each element of the vector and print it one by one. Here’s an example:
“`cpp
std::vector
for (int i = 0; i < numbers.size(); i++) {
std::cout << numbers[i] << " ";
}
// Output: 1 2 3 4 5
```
In this code snippet, we use the `size()` method of the vector to determine its size. Then, we iterate over the vector using an index-based for loop. Each element is printed using the `cout` stream.
## 4. Printing a Vector Using the range-based for Loop in C++
C++11 introduced a more concise and expressive loop, called the range-based for loop, which simplifies the process of iterating over a container like a vector. Here's how we can use it to print a vector:
```cpp
std::vector
for (int number : numbers) {
std::cout << number << " ";
}
// Output: 1 2 3 4 5
```
In this code snippet, we declare a variable `number` and use it to store each element of the vector `numbers` in each iteration. The range-based for loop automatically iterates over the entire vector, making it easier to read and write.
## 5. Printing a Vector Using the copy() Algorithm in C++
C++ provides a powerful algorithm library that includes various functions for performing operations on collections, such as vectors. One such algorithm is `copy()`, which can be used to copy the elements from a vector to an output iterator.
Here's an example of using `copy()` to print a vector:
```cpp
std::vector
std::copy(numbers.begin(), numbers.end(), std::ostream_iterator
// Output: 1 2 3 4 5
“`
In this code snippet, we pass the `begin()` and `end()` iterators of the `numbers` vector to `copy()`, along with an `ostream_iterator` that represents the output destination. The elements of the vector are then printed to `std::cout` with a space separator.
## 6. Printing a Vector Using the ostream_iterator in C++
Another way to print a vector is by using the `ostream_iterator` directly. This iterator allows us to write data to an output stream, such as `std::cout`, in a concise manner.
“`cpp
std::vector
std::copy(numbers.begin(), numbers.end(), std::ostream_iterator
// Output: 1 2 3 4 5
“`
Here, we again use the `copy()` algorithm, but instead of passing `std::cout` as the output stream, we pass an `ostream_iterator` initialized with `std::cout` and a space separator string.
## 7. Printing a Vector with Custom Formatting in C++
If we want to customize the formatting of the printed vector, such as adding braces or comma separators, we can achieve this using a combination of looping techniques and string manipulations.
Here’s an example of custom formatting using a for loop:
“`cpp
std::vector
std::cout << "[";
for (int i = 0; i < numbers.size(); i++) {
std::cout << numbers[i];
if (i != numbers.size() - 1) {
std::cout << ", ";
}
}
std::cout << "]";
// Output: [1, 2, 3, 4, 5]
```
In this code snippet, we manually add opening and closing square brackets, and comma separators between each element. The if statement helps us avoid printing a comma after the last element.
## 8. Handling Empty Vectors and Edge Cases in C++
It is important to handle edge cases when printing vectors, especially when dealing with empty vectors or vectors with a small number of elements. Failure to handle such cases can result in unexpected behavior or runtime errors.
To handle an empty vector, we can add a simple check before printing. Here's an example:
```cpp
std::vector
if (numbers.empty()) {
std::cout << "The vector is empty.";
} else {
for (int number : numbers) {
std::cout << number << " ";
}
}
```
In this code snippet, we use the `empty()` method to check if the vector is empty. If it is, we print a message indicating the empty state. Otherwise, we iterate and print the elements like before.
Similarly, we can handle other edge cases, such as vectors with only one or two elements, by adding appropriate checks and handling them accordingly.
## FAQs
### Q1. Can I use `printf` to print a vector in C++?
`printf` is a function provided by the C standard library and is not recommended for use in C++. It is preferable to use `std::cout` and the various techniques mentioned above to print vectors in a more C++-style manner.
### Q2. How do I initialize a vector and fill it with values in C++?
As mentioned earlier, you can initialize a vector with predefined values using an initializer list or add elements using the `push_back()` method. Additionally, you can also use the `resize()` method to set the size of the vector and populate it with values using indexing or algorithms like `fill()`.
### Q3. How do I sort a vector in C++ before printing it?
To sort a vector in C++, you can use the `std::sort()` function from the algorithm library. It takes two iterators specifying the range of the vector and sorts the elements in ascending order by default. You can also provide a custom comparison function for sorting in a different order.
### Q4. How do I take input from the user and store it in a vector in C++?
To take input from the user and store it in a vector, you can use a loop, such as a for loop or while loop, along with the `push_back()` method. Inside the loop, you can use `std::cin` to read input from the user and add it to the vector using `push_back()`.
In conclusion, printing vectors in C++ is a fundamental operation when working with collections of data. We explored various techniques like using for loops, range-based for loops, algorithms, and custom formatting to print vectors. Remember to handle edge cases and ensure the vector is properly initialized and populated before printing. Using the methods mentioned, you can easily print vectors in C++ and visualize the data they contain.
Learn C++ With Me #18 – Vectors
Is Vector Available In C?
Vector is a very popular data structure in programming that allows for efficient dynamic allocation and manipulation of elements. It is commonly used in languages like C++, Java, and Python. However, C, being a simpler and older language, does not have a built-in vector data structure. But does that mean programmers in C cannot use vectors? Not necessarily. In this article, we will explore the options available for implementing vectors in C and discuss some frequently asked questions surrounding this topic.
Implementing Vectors in C:
Although C does not have a pre-defined vector data structure, programmers can implement their own version of a vector using arrays and dynamic memory allocation. Here is a step-by-step approach to creating a vector in C:
1. Define a struct: Begin by defining a struct that contains an array to store the elements, an integer to hold the current size of the vector, and an integer to store the capacity (maximum number of elements the vector can hold). This struct will represent the vector data structure.
2. Initialize the vector: Once the struct is defined, initialize the vector by allocating memory dynamically for the array and setting the initial size to 0 and the capacity as desired.
3. Implement basic vector operations: Next, create functions to perform basic vector operations, such as adding elements, accessing elements, resizing, and freeing memory. These functions will manipulate the vector struct and the array within it.
4. Expand the capacity: When adding elements to the vector, if the capacity is reached, reallocate memory for the array, increase the capacity, and copy the existing elements into the new memory space.
5. Shrink the capacity: Conversely, if the number of elements decreases significantly, the capacity can be reduced to save memory. Similar to expanding the capacity, reallocate memory for the array with the new capacity and copy the elements accordingly.
6. Clear and free memory: Implement a function to clear the vector, setting the size to 0. Additionally, free the memory allocated for the array to avoid memory leaks.
Overall, implementing a vector in C can be somewhat complex compared to using the built-in vector in other languages. However, it provides more control and flexibility to the programmer to design the vector data structure according to specific needs.
FAQs:
Q: Why doesn’t C have a built-in vector data structure?
A: C is an older language that was designed to be simpler and more minimalistic. It lacks many of the higher-level data structures and features found in modern languages like C++. The lack of a built-in vector is due to the language’s design philosophy.
Q: Can I use other data structures as an alternative to vectors in C?
A: Yes, C provides other data structures like arrays and linked lists that can be used as alternatives to vectors. However, arrays have a fixed size, while linked lists have their own set of advantages and disadvantages compared to vectors.
Q: Are manually implemented vectors in C as efficient as built-in vectors in other languages?
A: Manually implemented vectors in C might not be as efficient as built-in vectors in languages like C++. The overhead of managing memory and dynamic allocation can introduce slight inefficiencies. However, with careful design and optimization, C programmers can create highly efficient vector implementations.
Q: Are there any third-party libraries available for using vectors in C?
A: Yes, there are third-party libraries such as GLib (part of the GNOME project) and CVector that provide ready-to-use vector implementations in C. These libraries can save time and effort by offering pre-implemented vector functions.
Conclusion:
While the C programming language itself does not provide a built-in vector data structure like C++, implementing vectors in C is certainly possible. By utilizing arrays and dynamic memory allocation, C programmers can design and implement their own vector data structure. Although this approach requires more effort and attention to memory management, creating a vector in C allows for greater control over the data structure. Additionally, third-party libraries are available to simplify the process for those who prefer to use pre-implemented vector functions. By understanding the steps involved and considering the trade-offs, programmers can successfully use vectors in C, opening up new possibilities for data manipulation and dynamic memory allocation.
What Is A Vector In C ++\?
In the world of programming, a vector is a dynamic array that allows the storage and manipulation of data in a sequential manner. In C++, the vector class template is provided by the Standard Template Library (STL) and is one of the most commonly used data structures.
Unlike ordinary arrays, which have a fixed size, a vector in C++ can dynamically grow or shrink in accordance with the number of elements being stored. This dynamic resizing makes vectors particularly useful when the size of the data is unknown or likely to change over the course of a program’s execution.
Vectors in C++ are implemented as a wrapper around a dynamically allocated array, which is automatically managed by the vector class. The elements in a vector are stored in contiguous memory locations, allowing for efficient random access and continuous traversal of the data.
Creating a vector in C++
To use vectors in C++, the
“`cpp
#include
int main() {
std::vector
// Add elements to the vector
numbers.push_back(10);
numbers.push_back(20);
numbers.push_back(30);
// Accessing elements
std::cout << numbers[0] << std::endl; // Output: 10
return 0;
}
```
In the above example, a vector named "numbers" is created to store integers. Elements can be added to the vector using the `push_back()` function, which appends the specified element to the end of the vector. The elements in a vector can be accessed and manipulated using the subscript operator ([]).
Vector operations and functions
Vectors in C++ provide a wide range of operations and functions for efficient data manipulation. Some of the commonly used ones include:
- `push_back()`: Adds an element to the end of the vector.
- `pop_back()`: Removes the last element from the vector.
- `size()`: Returns the number of elements currently stored in the vector.
- `empty()`: Checks if the vector is empty.
- `clear()`: Removes all elements from the vector.
- `front()`: Returns the first element of the vector.
- `back()`: Returns the last element of the vector.
- `insert()`: Inserts an element at a specified position.
- `erase()`: Removes an element from a specified position.
- `resize()`: Resizes the vector to a specified size.
- `sort()`: Sorts the elements in the vector in ascending order.
These functions, among others, make vectors highly versatile and suitable for various programming tasks.
FAQs
Q: Can a vector in C++ store different types of data?
A: Yes, a vector can store different types of data. This is achieved by using templates in C++, allowing vectors to work with any data type.
Q: How does a vector resize itself dynamically?
A: When a vector runs out of space due to the addition of more elements, it automatically allocates a new larger memory block, typically twice the size of the previous block. The elements from the old block are then copied to the new block, and the old block is deallocated.
Q: Can a vector in C++ be resized to a smaller size?
A: Yes, a vector can be resized to a smaller size using the `resize()` function. If the vector is resized to a smaller size, any elements beyond the new size will be removed from the vector.
Q: How is vector different from an array in C++?
A: Vectors provide more flexibility compared to arrays. Arrays have a fixed size and are allocated on the stack, while vectors can dynamically resize themselves and are allocated on the heap. Vectors also provide additional functionality and better memory management.
Q: Are vectors in C++ efficient in terms of memory usage?
A: Vectors do incur some overhead due to the additional functionality they provide, such as managing dynamically allocated memory. However, the STL implementation of vectors in C++ ensures efficient memory usage and performance.
In conclusion, a vector in C++ is a powerful and widely used data structure that provides considerable flexibility and efficiency for storing and manipulating data. Its dynamic resizing capability and extensive range of operations make it a crucial component of many C++ programs.
Keywords searched by users: print a vector c++ Printf vector, Fill vector C++, Out vector c++, Input vector c++, How to print vector in C++, Sort vector C++, Vector C++, Initialize vector C++
Categories: Top 39 Print A Vector C++
See more here: nhanvietluanvan.com
Printf Vector
In the world of C++, one of the most widely used functions is printf(). This function enables the programmer to display text and variable values on the console. It has been a staple of the C programming language for many years and continues to be a fundamental part of C++ development. However, one of the limitations of printf() is that it requires the programmer to manually specify the format of each variable. This is where printf vector comes into play, offering a powerful and efficient alternative for handling multiple variables.
What is Printf vector and how does it work?
A printf vector is an extension of the traditional printf() function that simplifies the process of displaying multiple variables. It allows the programmer to pass an array-like structure, called a vector, to the printf function and have it automatically handle the formatting and display of each element in the vector.
To use printf vector, first, you need to include the
“`cpp
std::vector
“`
In the above syntax, `datatype` specifies the type of data you want to store in the vector, such as int, float, or string, and `variableName` is the name you choose for the vector variable.
After creating the vector, you can populate it with the desired values using various methods provided by the vector class, such as push_back(), which adds elements to the end of the vector. For example:
“`cpp
std::vector
numbers.push_back(3);
numbers.push_back(7);
numbers.push_back(11);
“`
Once the vector is populated, you can pass it to a modified version of the printf function called printf_vector() included in the
“`cpp
printf_vector(numbers);
“`
The output of the printf_vector() function will display each element of the vector on a new line, formatted according to its data type.
Benefits of using Printf vector
Printf vector offers several advantages over traditional printf() when it comes to handling multiple variables:
1. Reduced code complexity – With printf vector, you no longer need to write separate printf statements for each variable. It simplifies code by automatically iterating through the vector and formatting each element for output.
2. Automatic type handling – Printf vector automatically handles the formatting and display of different data types, eliminating the need for manual format specifiers. This saves you from the hassle of remembering the specific format codes for each variable type.
3. Extensibility – Using vectors enables you to easily add or remove elements without modifying the code that handles the display. This allows for greater flexibility and scalability in managing variable outputs.
4. Improved readability – The use of vectors and printf_vector() makes the code more concise and clear, enhancing readability and reducing the chances of errors and bugs.
Frequently Asked Questions
Q1. Can I mix different data types in a single vector?
Yes, you can mix different data types in a vector. However, keep in mind that when using printf_vector(), the function will display each element according to its respective data type. Mixing data types requires careful handling to maintain the desired formatting.
Q2. Can I change the formatting of each element within the vector?
By default, the printf_vector() function will apply the appropriate formatting based on the data type of each element. However, if you want to customize the formatting for specific elements, you may need to create a custom function that overrides the default behavior.
Q3. Are there any performance implications when using printf vector?
The performance impact of using printf vector is negligible in most cases. However, it’s always a good practice to benchmark your code to ensure that it meets your performance requirements.
Q4. Are there any limitations or compatibility issues with using printf vector?
Printf vector is a C++ feature and may not be available in older C or C++ standards. Make sure your compiler supports the required features and headers before using printf_vector().
Q5. Can I use printf vector with other C++ libraries and frameworks?
Yes, you can combine printf vector with other C++ libraries and frameworks. Printf vector is a standard C++ feature and can be used in conjunction with other code without any issues.
In conclusion, printf vector is a powerful tool for C++ developers looking to enhance code readability and maintainability when handling multiple variables. By leveraging the capabilities of vectors and the printf_vector() function, you can streamline the process of displaying variable values, reducing code complexity, and improving overall efficiency. So, next time you find yourself in a situation where you need to display multiple variables, consider using the printf vector approach for an optimized development experience.
Fill Vector C++
The C++ programming language provides a versatile and powerful container called a vector that allows for the efficient storage and manipulation of a sequence of elements. The vector container is part of the Standard Template Library (STL) and offers a wide range of functions to simplify data management. One such function is “fill,” which allows you to assign a specific value to all elements of a vector. In this article, we will explore the fill vector function in C++, its usage, and common questions related to it.
Understanding the Fill Vector Function:
The “fill” function in C++ works by assigning a specific value to all the elements of a vector. It takes two arguments: the iterator range that specifies the portion of the vector to be filled and the value to be assigned. The iterator range is defined by two iterators: the first points to the starting element, and the second points to the element following the last one to be filled. This flexibility allows you to fill a specific portion or the entire vector with the desired value.
The Syntax of Fill Vector:
The syntax of the fill vector function is as follows:
fill(iterator start, iterator end, const Type& value);
Here, “start” refers to the iterator pointing to the first element of the target range. “end” refers to the iterator pointing to the element following the last element of the target range. Finally, “value” represents the value to be assigned to each element in the range.
Example Usage:
Let’s consider an example to illustrate the usage of the fill vector function:
#include
#include
using namespace std;
int main() {
vector
fill(numbers.begin(), numbers.end(), 10); // Fills the entire vector with 10
// Display the vector elements
for (const auto& number : numbers) {
cout << number << " ";
}
return 0;
}
In the above example, we create a vector named "numbers" with a size of 5 elements. We then use the fill function to assign each element the value of 10. Finally, we iterate over the vector and print its elements, resulting in the output: "10 10 10 10 10."
Frequently Asked Questions (FAQs) About Fill Vector in C++:
Q1. Can fill vector be used with user-defined objects?
A1. Yes, the fill vector function can be used with user-defined objects, given that the appropriate assignment operator is defined for the object type.
Q2. Can I fill only a specific portion of a vector with the fill function?
A2. Yes, the fill function allows you to choose a specific portion of a vector to be filled by providing the appropriate iterator range.
Q3. What happens if the iterator range is empty?
A3. If the iterator range is empty (e.g., both iterators are the same), the fill function will simply return without modifying any elements.
Q4. Can I fill a vector with different values for different elements?
A4. No, the fill function assigns the same value to every element in the specified range.
Q5. Is the fill operation efficient for large vectors?
A5. The fill operation has a time complexity of O(n), where n is the number of elements in the specified range. Consequently, the efficiency depends on the size of the range and not the size of the vector itself.
Q6. Can I fill a vector with different values using multiple fill calls?
A6. Yes, if you want to assign different values to different portions of a vector, you can use multiple fill calls with appropriate iterator ranges.
Q7. How does fill differ from assign in vectors?
A7. The fill function assigns a specific value to the elements within a range, while the assign function replaces the entire contents of a vector with copies of a given value or a range of values.
Q8. Can the fill vector function handle multidimensional vectors?
A8. The fill function works with multidimensional vectors as long as you provide the correct iterator range for the desired dimension.
In conclusion, the fill vector function in C++ is a useful tool when you need to assign a specific value to all elements within a vector or a specific range. Its simplicity and flexibility make it an efficient choice for manipulating data stored within vectors. By understanding the syntax and usage of fill, you can effectively manage and modify your vector contents to meet the requirements of your program.
Images related to the topic print a vector c++
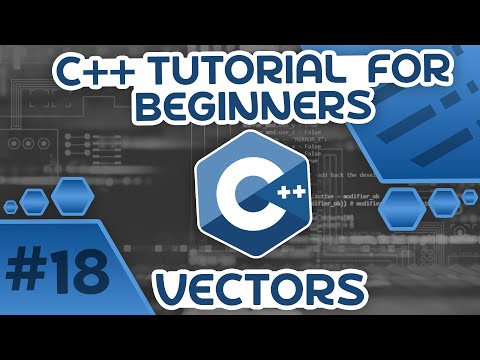
Found 22 images related to print a vector c++ theme
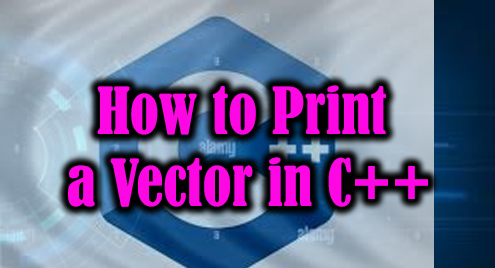

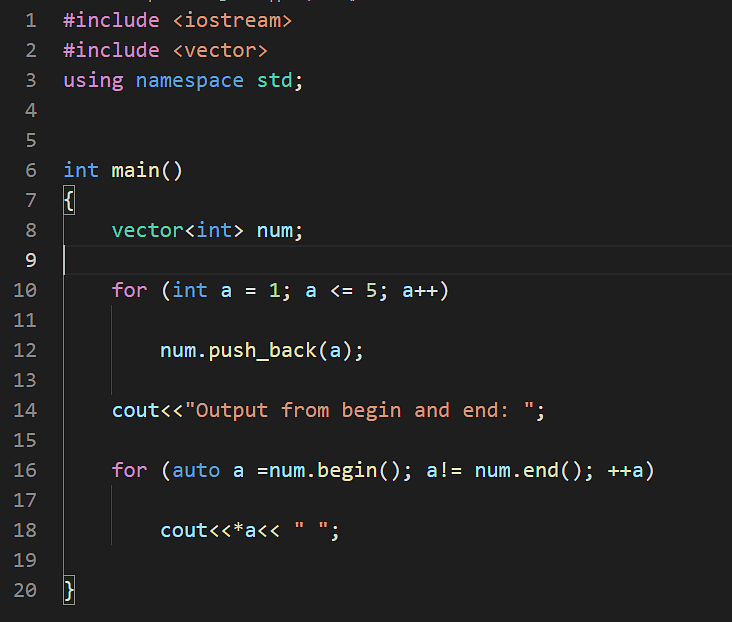
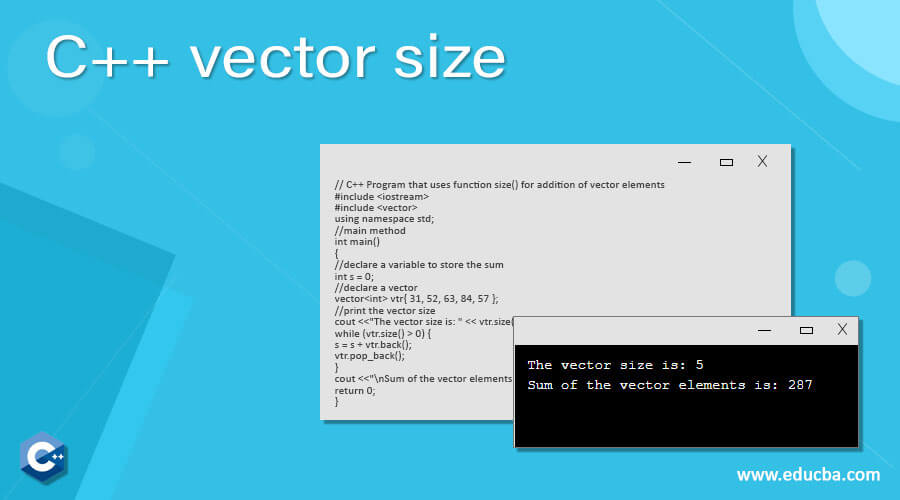
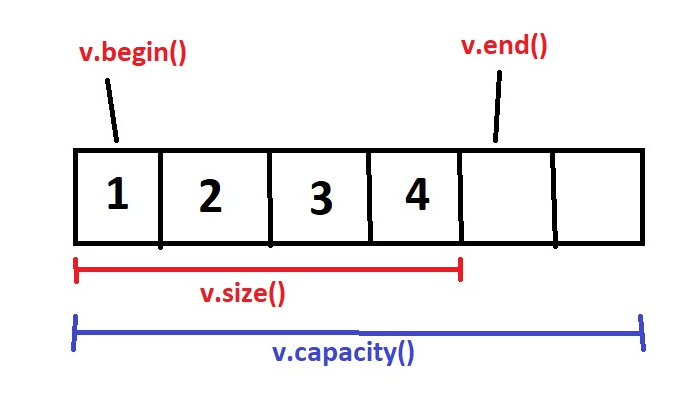

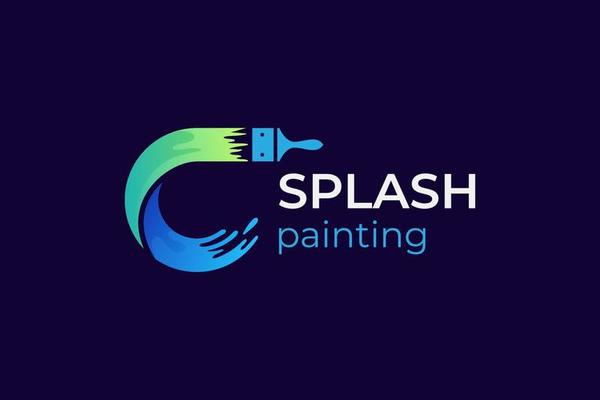
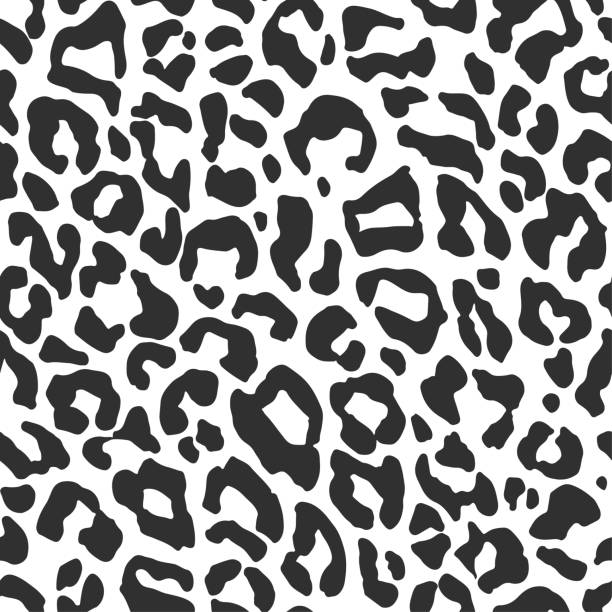
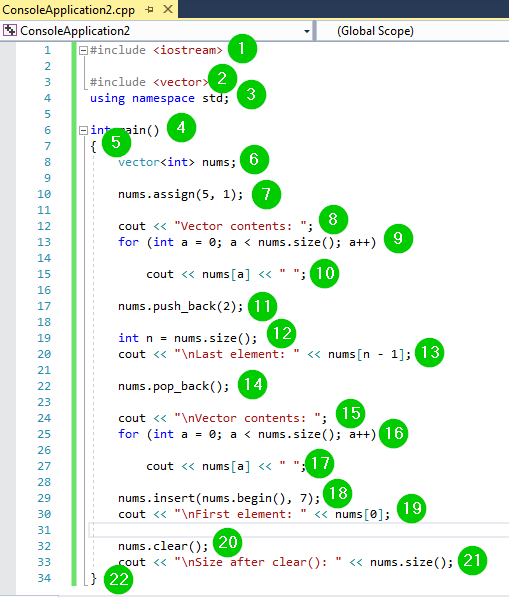
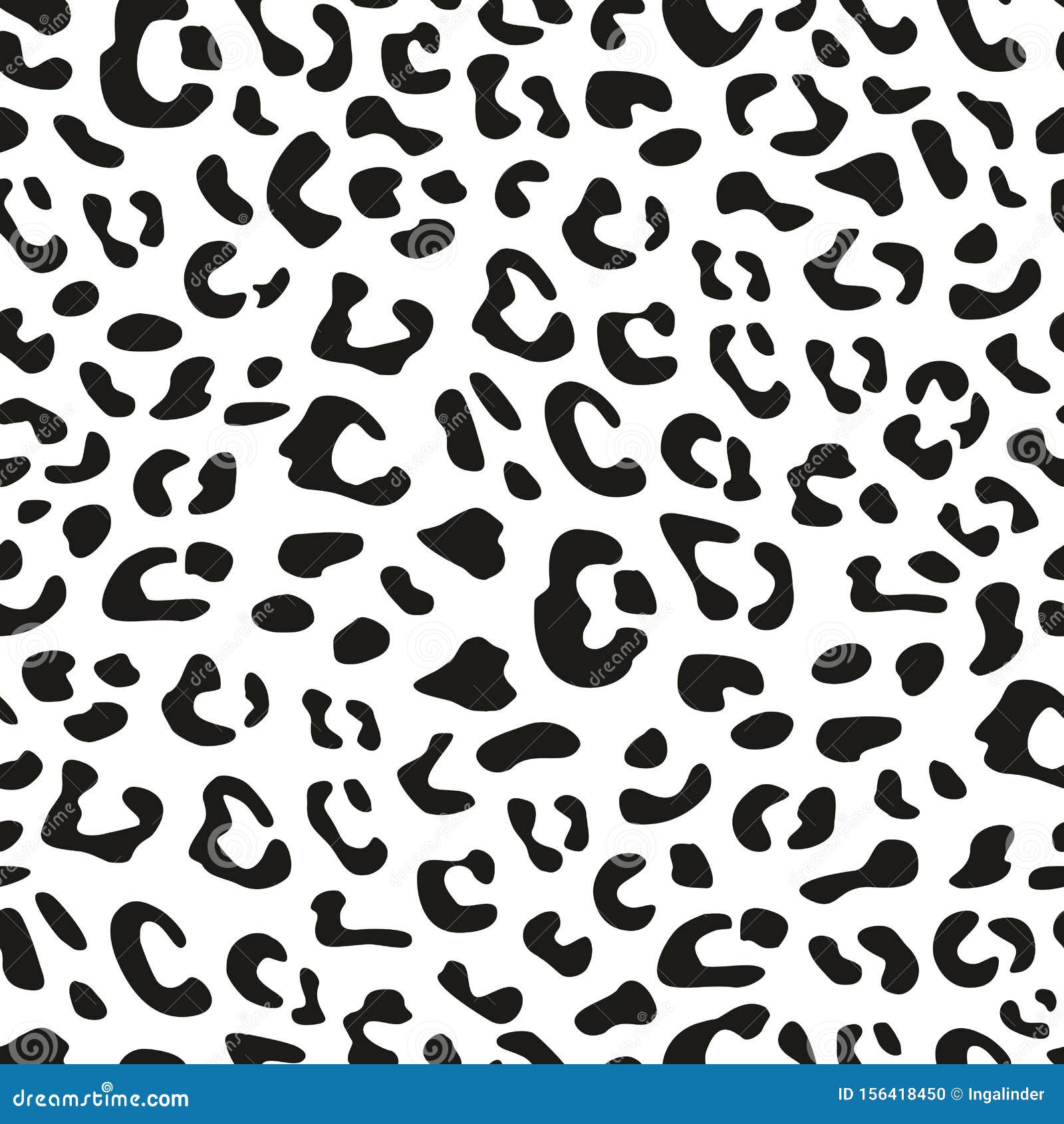


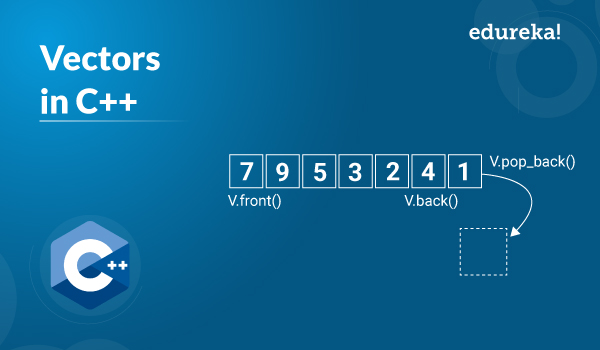

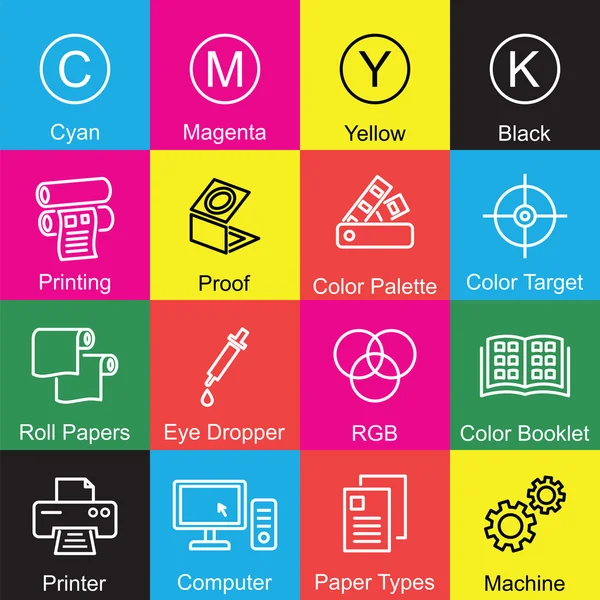


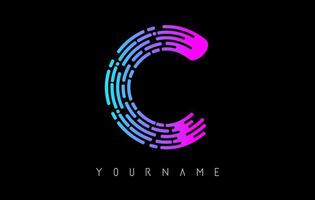
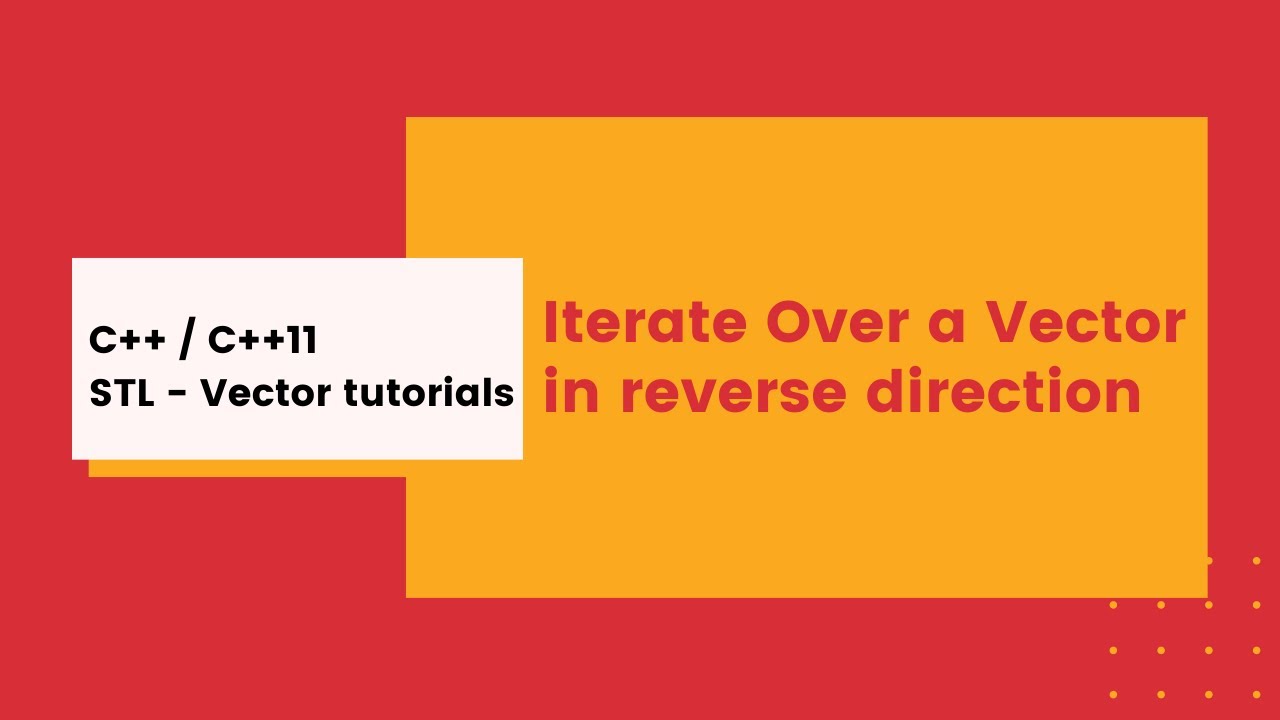


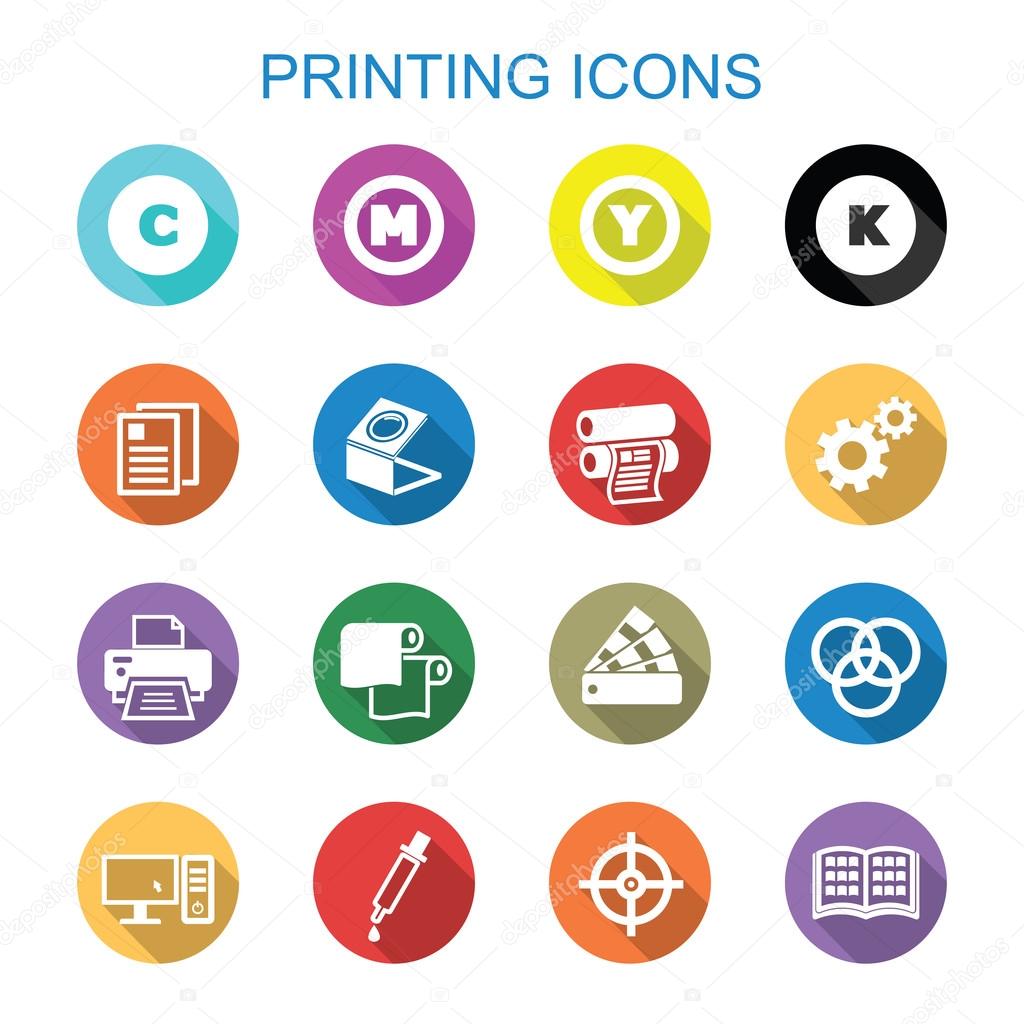
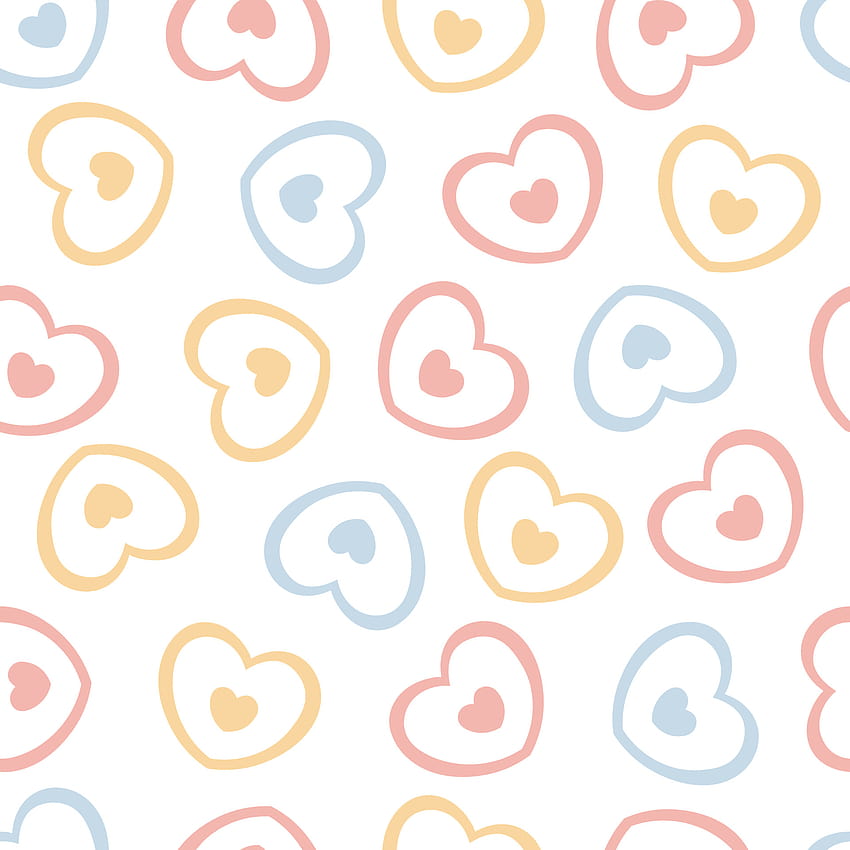



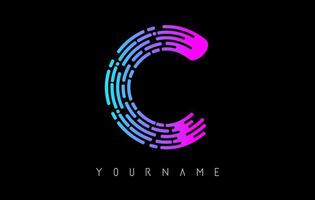
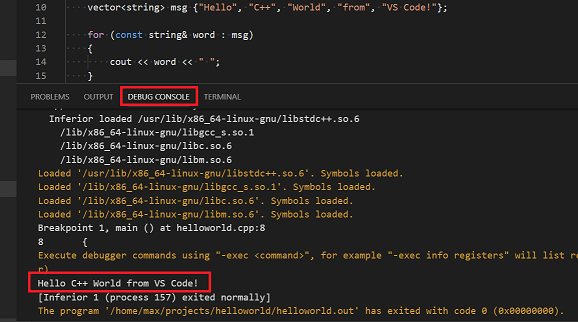
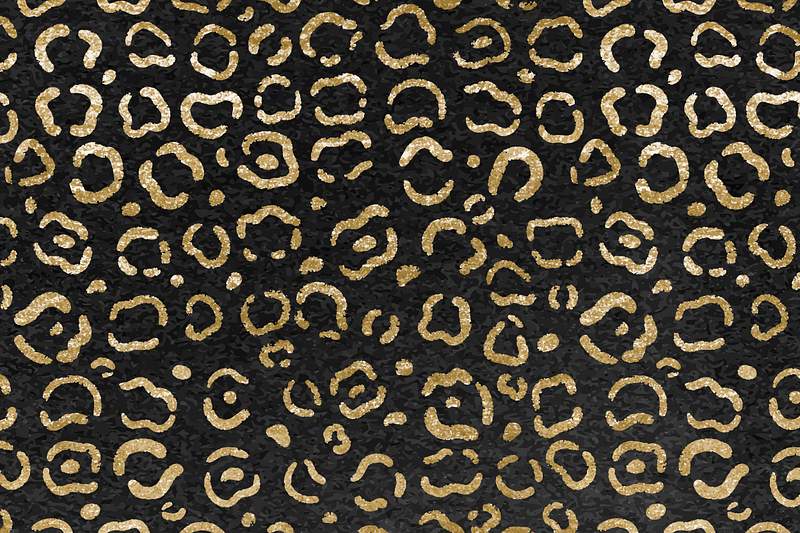
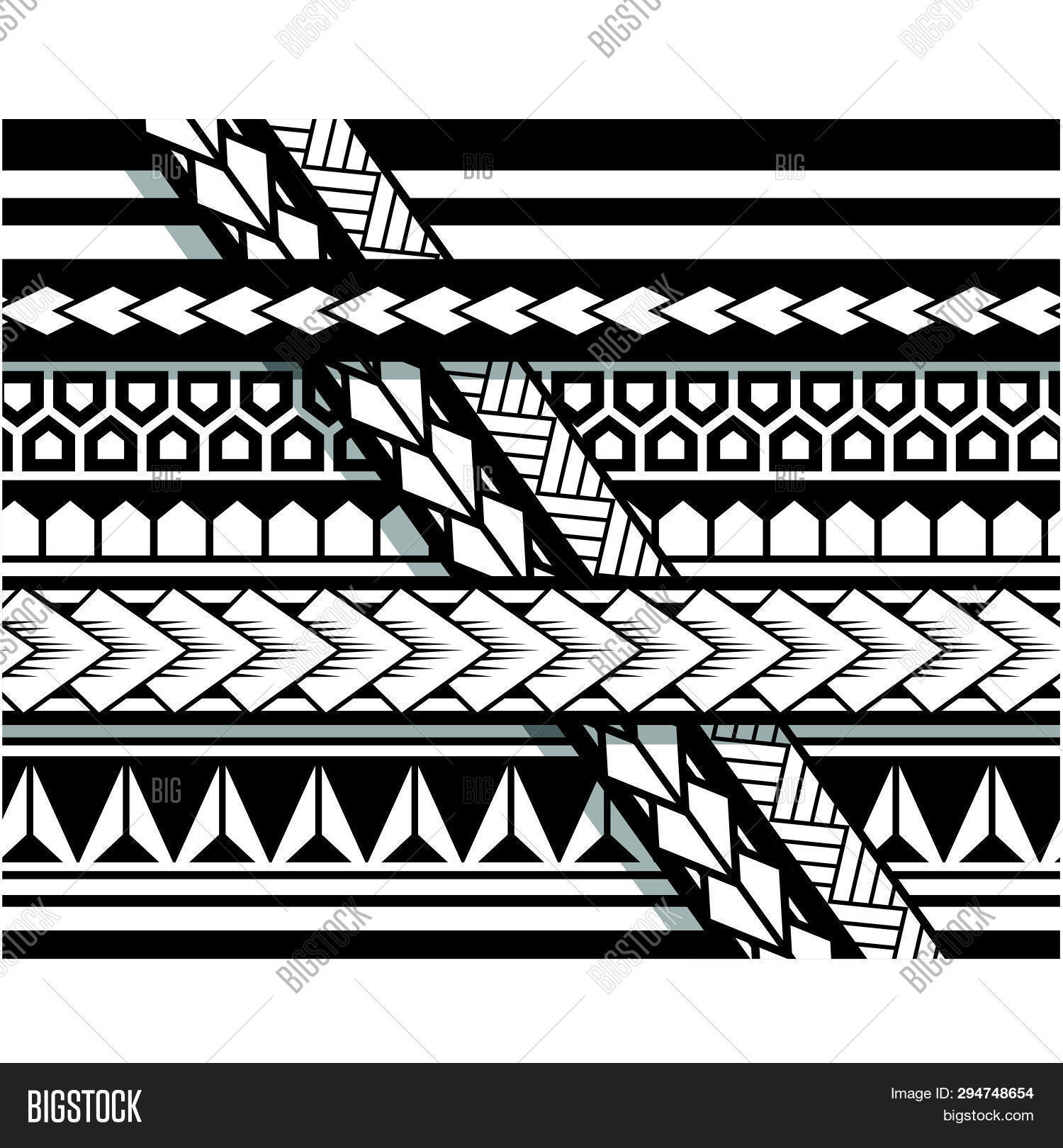



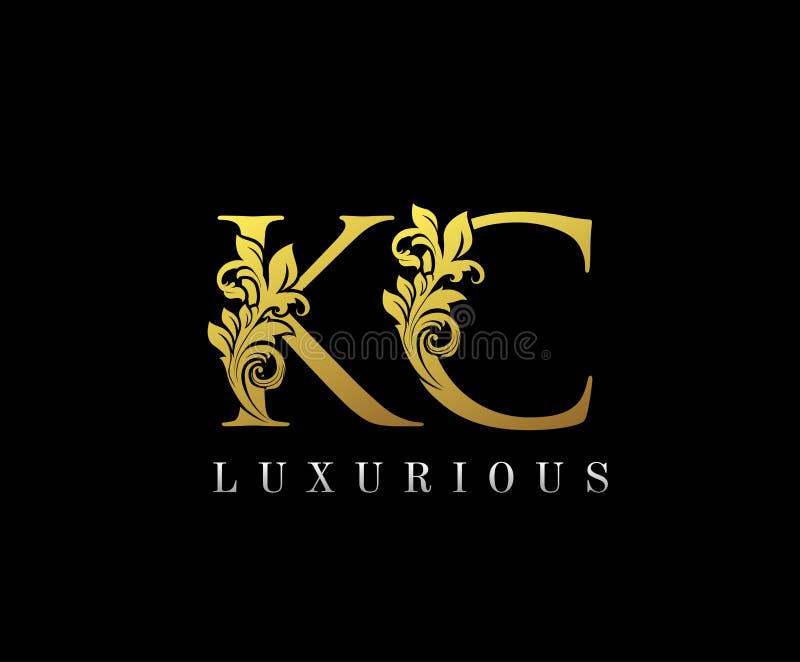

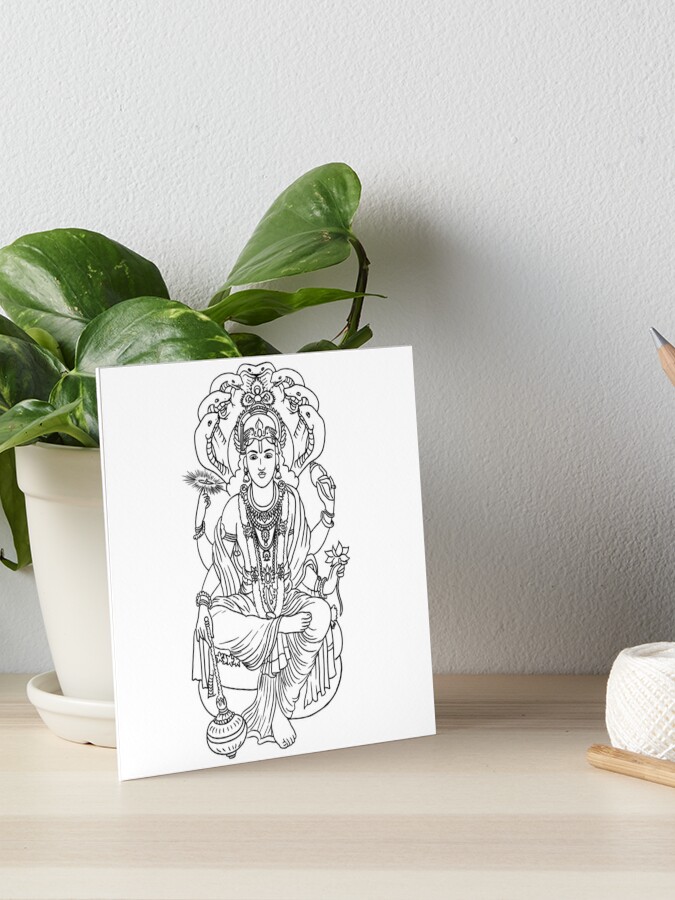
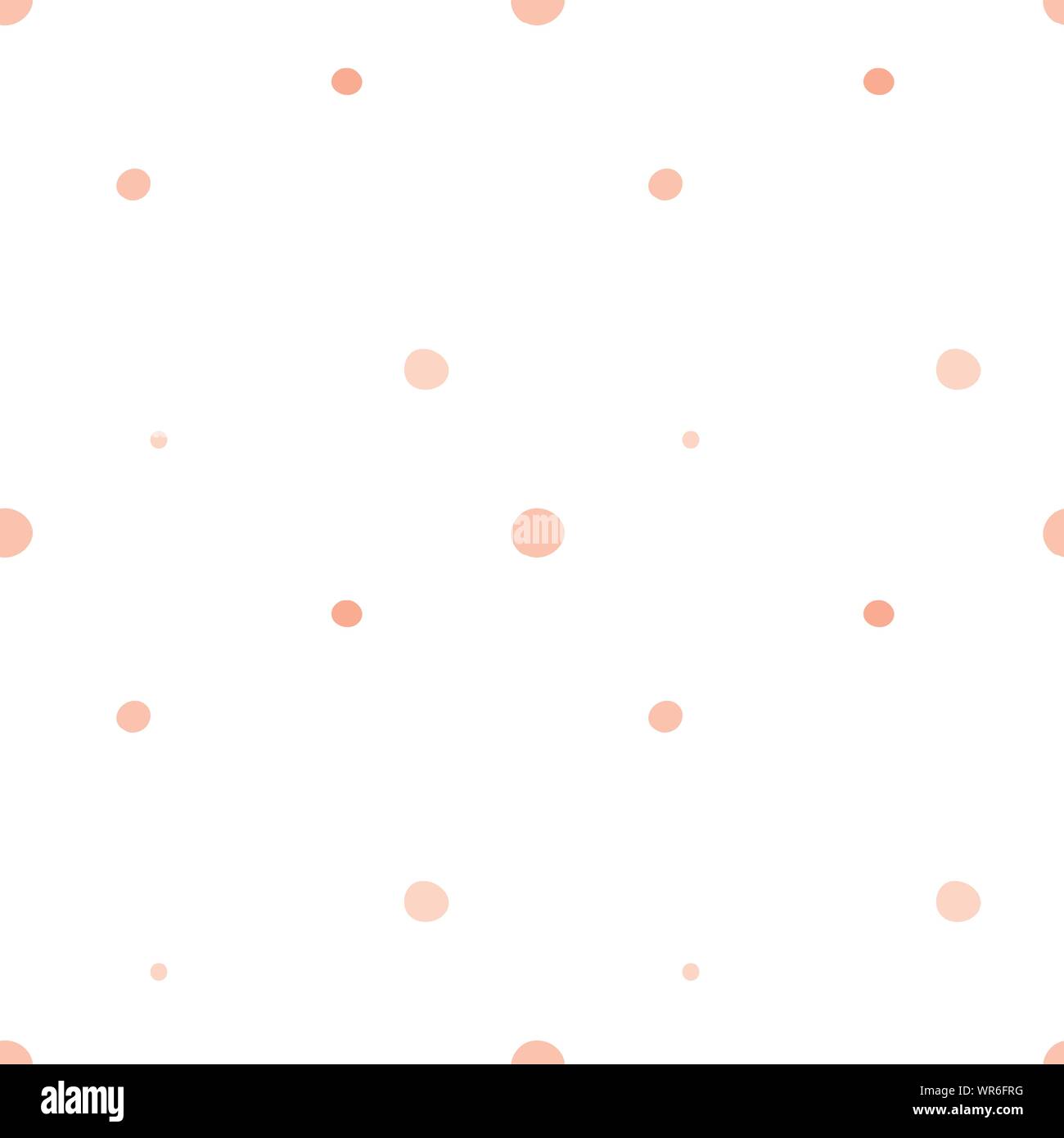

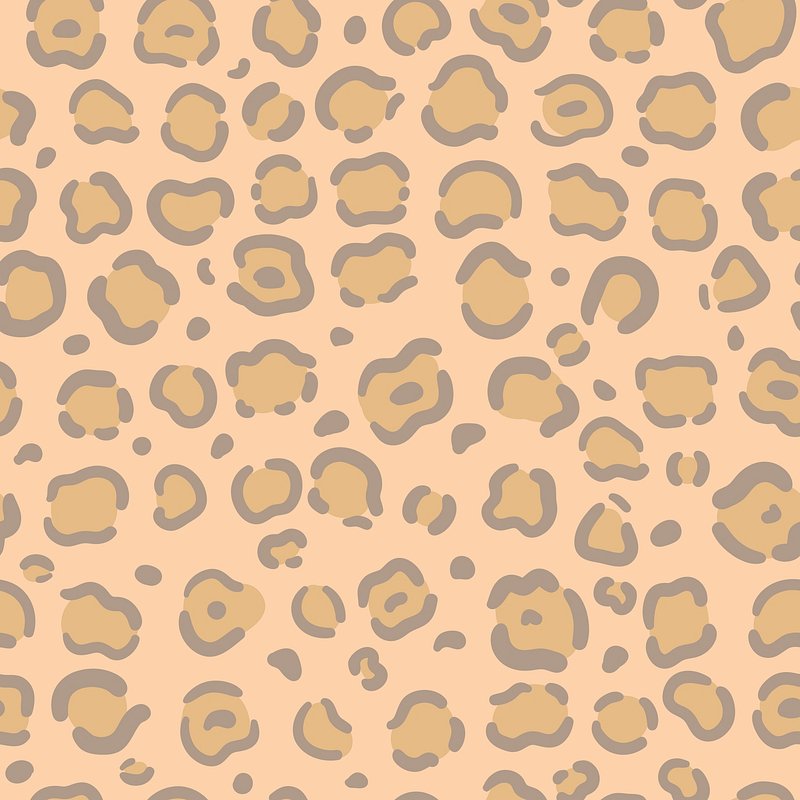



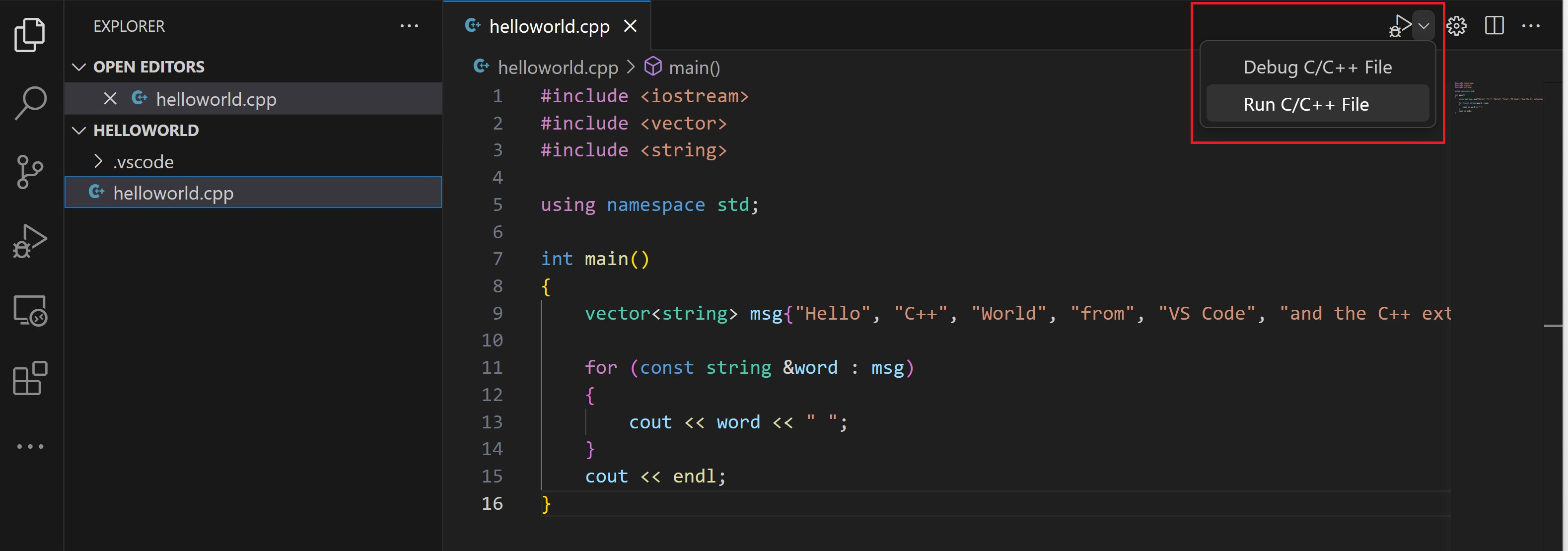
Article link: print a vector c++.
Learn more about the topic print a vector c++.
- Different ways to print elements of vector – GeeksforGeeks
- How do I print out the contents of a vector? – Stack Overflow
- Print a vector in C++ | Techie Delight
- A Quick Guide to Creating Your Own Vectors in C – Udemy Blog
- vector class | Microsoft Learn
- Mashpoe/c-vector: a simple vector library written in C. Can store any type
- C++ vector equivalent in C – Stack Overflow
- How to Print a Vector in C++ – Linux Hint
- C++ Print Vector Function – Position Is Everything
- Print Vector in C++ – Java2Blog
See more: nhanvietluanvan.com/luat-hoc