Pretty Print Dictionary Python
In the world of programming, dictionaries are widely used to store and manipulate data in a structured manner. However, when it comes to displaying the contents of a dictionary in a human-readable format, things can get a bit messy. This is where pretty printing comes into play.
Converting a dictionary to a string representation
Sometimes, you may need to convert a dictionary into a string to display it or write it to a file. The most common approach is to use the str() function in Python, which converts any object into its string representation. However, when using str() on a dictionary, the output is not very reader-friendly. It simply displays the dictionary keys and their corresponding values without any formatting.
For example, if we have a dictionary like this:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
“`
And we use str(my_dict), the output would be:
“`
“{‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}”
“`
While this can be useful for certain purposes, it lacks readability and can be challenging to interpret when dealing with larger or nested dictionaries.
The pprint module for pretty printing dictionaries
To overcome the limitations of using str() for dictionary printing, Python provides a built-in module called pprint (pretty print). The pprint module is specifically designed to display complex data structures, including dictionaries, in a more readable format.
To use the pprint module, we first need to import it into our Python script:
“`
import pprint
“`
Once imported, we can utilize the pprint.pprint() function to pretty print dictionaries. Instead of simply displaying the dictionary as a string, pprint arranges the keys and values in a structured manner, making it easier to read and interpret.
For example, if we use pprint.pprint() on the same dictionary as before:
“`
pprint.pprint(my_dict)
“`
The output would be:
“`
{‘name’: ‘John’,
‘age’: 25,
‘city’: ‘New York’}
“`
As we can see, pprint organizes the dictionary elements in a more visually appealing way, with each key-value pair on a separate line and aligned for better readability.
Formatting options with pprint
The pprint module also provides a range of formatting options to further enhance the display of dictionaries. These options allow us to customize the width and indentation of the pprint output.
By default, pprint uses a width value of 80 characters. If the output exceeds this width, it automatically wraps the lines to fit the specified limit. However, we can modify this behavior by specifying a different width value when calling pprint.pprint(). For example:
“`
pprint.pprint(my_dict, width=50)
“`
This would limit the width of the output to 50 characters.
Similarly, we can adjust the indentation level of the pprint output. By default, indentation is set to 1, which means each nested level is indented by four spaces. We can change this value by setting the indent parameter when calling pprint.pprint(). For example:
“`
pprint.pprint(my_dict, indent=2)
“`
This would increase the indentation to two spaces for each nested level.
Handling nested dictionaries with pprint
One of the advantages of using pprint is its ability to handle nested dictionaries gracefully. When a dictionary contains another dictionary as a value, pprint effectively displays the nested structure, making it easier to visualize the data hierarchy.
For example, consider the following nested dictionary:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘address’: {‘street’: ‘123 Main St’, ‘city’: ‘New York’}}
“`
If we use pprint.pprint() on this dictionary, the output would be:
“`
{‘name’: ‘John’,
‘age’: 25,
‘address’: {‘street’: ‘123 Main St’, ‘city’: ‘New York’}}
“`
As we can see, pprint indentations clearly depict the nested structure of the dictionary, allowing us to understand the relationships between the different elements.
Customizing pprint output with pretty printing hooks
While pprint offers a convenient way to display dictionaries, sometimes we may want to customize the output further according to our specific requirements. To achieve this, pprint provides “pretty printing hooks” that allow us to define custom formatting rules for certain dictionary elements.
In pprint, the main class responsible for the printing process is called PrettyPrinter. We can create an instance of this class and then modify its behavior using various methods and attributes.
For example, we can define a custom pretty printing hook for dictionaries with specific structures or values. This hook can be registered with the PrettyPrinter instance to control how those elements are displayed.
Using pprint alternatives for dictionary pretty printing
Although pprint is a powerful and flexible tool for pretty printing dictionaries in Python, there are alternative libraries and methods available for this purpose. Some popular alternatives include json.dumps(), tabulate, and texttable.
json.dumps() from the Python standard library is a convenient option when you want to pretty print a dictionary as a JSON string. It provides similar formatting capabilities to pprint, but with additional support for JSON-specific features.
tabulate and texttable are third-party libraries that offer table-based formatting for dictionaries and other tabular data structures. These libraries are especially useful when you want to display dictionaries in a tabular format with headers and aligned columns.
When choosing between pprint and alternative methods, consider the specific requirements of your project. pprint is a versatile and widely used module that provides a comprehensive solution for pretty printing dictionaries. However, if you require specialized formatting or integration with other tools, one of the alternatives may better suit your needs.
In conclusion, pretty printing dictionaries in Python is essential for enhancing readability and understanding complex data structures. The pprint module offers a straightforward and effective way to achieve this. By utilizing pprint’s formatting options and handling nested dictionaries, we can create clear and visually appealing representations of our data. Additionally, with the ability to customize pprint’s output using pretty printing hooks, we can adapt it to suit our specific requirements.
Pretty Printing A Dictionary
How To Print Dictionary In Pretty Format Python?
When working with Python dictionaries, it is often necessary to display the contents in a more readable and visually appealing format. Fortunately, Python provides several techniques to accomplish this task. In this article, we will explore different methods to print dictionaries in a pretty format using Python.
Before we dive into the different approaches, let’s quickly recap what a dictionary is in Python. A dictionary is an unordered collection of key-value pairs, where each key is unique. It allows us to store and retrieve data efficiently, making it an essential data structure in Python.
Printing Dictionary Items in a Basic Format:
The most straightforward method to print a dictionary is to utilize the built-in print() function. By doing so, the dictionary will be displayed in a basic format, where each key-value pair is separated by a colon. Here’s an example:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
print(my_dict)
“`
Output:
“`
{‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
“`
This approach works fine if you only need a quick glance at the dictionary. However, when dealing with dictionaries containing nested structures or large amounts of data, the output becomes more challenging to interpret.
Using the pprint Module:
To overcome the limitations of the basic print() function, Python offers the pprint module, which provides a more advanced printing mechanism for complex data structures, including dictionaries. The pprint module can be imported using the following statement:
“`
import pprint
“`
Now, let’s see how we can use pprint to print a dictionary in a pretty format:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
pprint.pprint(my_dict)
“`
Output:
“`
{‘age’: 25, ‘city’: ‘New York’, ‘name’: ‘John’}
“`
As you can see, pprint sorts the dictionary keys in alphabetical order and displays the dictionary in a more visually appealing format.
Customizing pprint Output:
The pprint module also allows us to customize the output further through optional parameters. Here are a few useful ones:
– indent: Specifies the indentation level for nested elements. By default, it is set to 1.
– width: Specifies the maximum number of characters per line. By default, it is set to 80.
– depth: Specifies the maximum nested level to print. By default, it is set to None.
Let’s modify our previous example to demonstrate some of these parameters:
“`
import pprint
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘address’: {‘street’: ‘123 Main St’, ‘city’: ‘New York’}}
pprint.pprint(my_dict, indent=4, width=30, depth=1)
“`
Output:
“`
{‘address’: {…},
‘age’: 25,
‘name’: ‘John’}
“`
In this example, the indent parameter is set to 4, resulting in a larger indentation for nested elements. The width parameter is set to 30, limiting the number of characters per line. Finally, the depth parameter is set to 1, causing the nested dictionary to be represented by the “…” ellipsis.
FAQs about Printing Dictionaries in Pretty Format in Python:
Q: Can pprint only be used to print dictionaries?
A: No, pprint is a versatile module that can handle various complex data structures, including lists, tuples, and other nested data types.
Q: How can I sort the dictionary keys in a different order using pprint?
A: By default, pprint sorts the dictionary keys alphabetically. However, you can use the sorted() function before calling pprint to sort the keys in a different order.
Q: Is there any way to convert the pretty-printed dictionary back into a regular dictionary format?
A: No, pprint is designed for readability purposes only. To retrieve the original dictionary, use the standard dictionary operations or data serialization techniques such as JSON encoding/decoding.
Q: Are there any performance concerns when using pprint for large dictionaries?
A: While pprint is convenient for small to medium-sized dictionaries, it may impact performance for extremely large dictionaries due to the additional overhead of creating a pretty-printed output. In such cases, it is advisable to use alternative methods specific to your use case.
In conclusion, printing dictionaries in a pretty format enhances readability and comprehension of complex data structures in Python. We explored the basic print() function, but for more advanced formatting options, the pprint module is highly recommended. By customizing its parameters, we can tailor the output to our specific requirements. Hopefully, this article provides you with a better understanding of how to print dictionaries in a more visually appealing format in Python.
What Is The Best Way To Print Dictionary In Python?
A dictionary is a versatile and widely used data structure in Python that allows you to store and organize data in key-value pairs. When it comes to printing a dictionary, there are several approaches you can take, each with its advantages and disadvantages. In this article, we will explore different methods to print a dictionary in Python and discuss the best ways to do so based on different scenarios.
1. Simple Print Statement:
The most straightforward way to print a dictionary is by using a simple print statement. It allows you to print the entire dictionary, key-value pairs, or specific values based on your needs. For instance, consider the following dictionary:
“`
student = {
“name”: “John Doe”,
“age”: 20,
“grade”: “A”
}
“`
To print the entire dictionary, you can use the following code:
“`python
print(student)
“`
However, this would print the dictionary in a single line, making it hard to read for dictionaries with multiple key-value pairs.
2. Using the pprint Module:
The pprint (pretty print) module in Python provides a way to format and print data structures, including dictionaries, in a more readable and organized manner. By importing the pprint module, you gain access to the `pprint()` function, which handles dictionaries more intelligently.
“`python
import pprint
pprint.pprint(student)
“`
This approach presents the dictionary in a more aesthetically pleasing way, with each key-value pair on a separate line and indentation.
3. Iterating Over the Dictionary:
Another approach to printing a dictionary is by iterating over its key-value pairs using a loop, such as a `for` loop. This method can be particularly useful if you want more control over the printing process or need to apply specific formatting.
“`python
for key, value in student.items():
print(key, “:”, value)
“`
Iterating over the dictionary allows you to access each key-value pair individually and customize the print output based on your requirements. For instance, you can choose to print only the keys, values, or both, separate them with a particular character, or apply specific formatting to the output.
Frequently Asked Questions (FAQs):
Q1: How can I print a nested dictionary?
A nested dictionary is a dictionary where values can also be dictionaries. In Python, you face no restrictions when printing a nested dictionary. You can use any of the above methods to print a nested dictionary as they work perfectly fine with nested data structures. However, keep in mind that the pprint module, in particular, excels at neatly formatting complex and nested structures.
Q2: How can I prevent the pprint module from sorting dictionary keys alphabetically?
By default, the pprint module sorts the keys of a dictionary in alphabetical order before printing. However, if you wish to preserve the original order of the dictionary, you can pass the argument `sort_dicts=False` to the `pprint()` function. This prevents the module from reordering the dictionary and prints it with keys in the original order.
Q3: Can I print only specific keys or values from a dictionary?
Yes, you can selectively print specific keys or values from a dictionary using any of the discussed methods. To print a specific key-value pair, you can directly access it via the key and print it. To print a specific key or value, you can utilize conditional statements to filter the desired pairs or values before printing.
Q4: How can I print a dictionary as a tabular format?
To print a dictionary in a tabular format, you can utilize the `tabulate` module in Python, which helps in generating tables from structured data. This module allows you to specify the headers, alignment, and other parameters for formatting the table.
An example of formatting a dictionary as a table using the tabulate module:
“`python
from tabulate import tabulate
table = tabulate(student.items(), headers=[“Key”, “Value”], tablefmt=”grid”)
print(table)
“`
The `tablefmt` parameter can be adjusted to render the table in various formats, such as “plain,” “fancy_grid,” or “markdown.”
In conclusion, printing a dictionary in Python can be achieved through various methods, such as using a simple print statement, the pprint module, or iterating over the dictionary with a loop. Each method offers its advantages depending on the desired output, level of customization, and complexity of the dictionary. By understanding and utilizing these methods effectively, you can enhance the readability and presentation of dictionaries in your Python programs.
Keywords searched by users: pretty print dictionary python Python pretty print dict, Print key value in dictionary Python, New line in dictionary python, Print element in dictionary Python, Print dictionary Python, Update nested dictionary Python, How to print a value in a dictionary python, Pretty print Python
Categories: Top 76 Pretty Print Dictionary Python
See more here: nhanvietluanvan.com
Python Pretty Print Dict
Python is a dynamically-typed programming language known for its simplicity and readability. Dictionaries are one of the built-in data structures in Python, allowing developers to store data in key-value pairs. However, when dictionaries become large or complex, it can be challenging to comprehend the hierarchical structure and relationships between the keys and values. This is where Python’s pretty print dict comes to the rescue.
The `pprint` module in Python provides the `pprint` function, which enables us to neatly format dictionaries. This module is a part of Python’s standard library, meaning you don’t need to install any additional packages. To utilize the `pprint` function, you must import it into your Python script using the statement `from pprint import pprint`.
Once the module is imported, you can invoke the `pprint` function with a dictionary as the argument. This function automatically prints the dictionary in a visually pleasing manner. The output is organized in a way that each key-value pair is displayed on a new line, making it easier to read and comprehend.
The `pprint` function is not limited to dictionaries; it can also handle parsing of other complex data structures like lists, tuples, and even nested structures such as lists of dictionaries or dictionaries of tuples. This flexibility ensures that complex data can be presented in a well-structured manner.
In addition to formatting dictionaries, the `pprint` function also offers various parameters to customize the output. For instance, you can control the indentation level using the `indent` parameter, allowing you to choose how much space appears before each key-value pair. This flexibility proves useful when differentiating between nested levels of dictionaries.
Moreover, the `width` parameter enables you to set the maximum width of the formatted output. This is particularly helpful when dealing with large dictionaries or when working within limited screen space. You can adjust the value of `width` to suit your specific needs and ensure that the pretty printed dict is easily readable.
Now, let’s move on to address some frequently asked questions about the Python pretty print dict feature:
Q: Can I pretty print a dictionary directly without importing any module?
A: No, the `pprint` module is specifically designed to handle the formatting and printing of dictionaries.
Q: How can I pretty print a dictionary to a file instead of the console?
A: To print the pretty printed dict to a file, you can redirect the standard output to a file by making use of the `sys` module. For example:
“`
import sys
from pprint import pprint
with open(‘output.txt’, ‘w’) as file:
sys.stdout = file
pprint(my_dict)
sys.stdout = sys.__stdout__ # Reset standard output to console
“`
Q: Does pretty printing dictionaries affect their original structure?
A: No, pretty printing does not modify the dictionary in any way. It only affects the formatting of the output, making it easier to understand for humans.
Q: Can I adjust the sorting order of the keys in the pretty printed output?
A: Yes, the `pprint` function provides a parameter called `sort_dicts` that allows you to specify whether the keys should be sorted or not. By default, it sorts the keys in ascending order.
In conclusion, Python’s pretty print dict feature is an invaluable tool for developers working with complex dictionaries. Its ability to neatly format and display dictionaries significantly enhances the readability and comprehension of the data structure. By utilizing the `pprint` function, developers can save time and effort in debugging and understanding their dictionaries effectively.
Print Key Value In Dictionary Python
A dictionary in Python is a data structure that stores data in key-value pairs. Each key in the dictionary must be unique, and it maps to a corresponding value. One common operation when working with dictionaries is to print the key-value pairs. In this article, we will discuss various ways to achieve this in Python.
Printing Key-Value Pairs Using a for Loop
One straightforward approach to print key-value pairs in a dictionary is by using a for loop. In Python, we can iterate over the keys of a dictionary using the `keys()` method. Once we have the key, we can access the corresponding value using the key as an index. Let’s look at an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
for key in my_dict.keys():
print(key, ‘:’, my_dict[key])
“`
Output:
“`
name : John
age : 25
city : New York
“`
In the example above, we first create a dictionary called `my_dict` with three key-value pairs. Then, we iterate over the keys of the dictionary using the `keys()` method. For each key, we print the key and its corresponding value using the key as an index.
Printing Key-Value Pairs Using the items() Method
Another way to print key-value pairs in a dictionary is by using the `items()` method. The `items()` method returns a view object that contains key-value pairs of the dictionary. This view object can then be looped over to print the key-value pairs. Let’s see an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
for key, value in my_dict.items():
print(key, ‘:’, value)
“`
Output:
“`
name : John
age : 25
city : New York
“`
In the example above, we can directly access both the key and value of each key-value pair simultaneously by using the `items()` method. This simplifies the code as we don’t need to use the keys to access the corresponding values.
Printing Key-Value Pairs Using a List Comprehension
Python also allows us to use list comprehensions to print key-value pairs in a dictionary. List comprehensions are concise ways for creating lists based on existing lists or other iterable objects. To print key-value pairs, we can use a list comprehension to create a list of strings containing the key-value pairs, and then join and print them. Let’s examine an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
pairs = [f'{key} : {value}’ for key, value in my_dict.items()]
print(‘\n’.join(pairs))
“`
Output:
“`
name : John
age : 25
city : New York
“`
In the example above, we use a list comprehension to create a list of strings, where each string represents a key-value pair. We join the strings using the `join()` method with a newline character as the separator to print the key-value pairs on separate lines.
FAQs:
Q: Can a dictionary have duplicate keys?
A: No, a dictionary in Python cannot have duplicate keys. Each key must be unique, and if you try to assign a new value to an existing key, it will overwrite the previous value.
Q: How can I print only the keys or values of a dictionary?
A: If you want to print only the keys of a dictionary, you can use the `keys()` method and iterate over it. Similarly, to print only the values, you can use the `values()` method and iterate over it.
Q: Is the order of key-value pairs preserved in a dictionary?
A: Starting from Python 3.7, the order of insertion of key-value pairs is preserved in dictionaries. However, in earlier versions of Python, the order was not guaranteed. If order matters, you can use the `collections.OrderedDict` class from the `collections` module.
Q: How can I check if a key exists in a dictionary before printing its value?
A: To check if a key exists in a dictionary, you can use the `in` keyword. For example, `if key in my_dict:` can be used to check if `key` is present in `my_dict`.
In conclusion, printing key-value pairs in a dictionary is a common task when working with Python dictionaries. Through this article, we have explored several methods, including using a for loop, the `items()` method, and list comprehensions. Choose the approach that suits your requirements and coding style to effectively print key-value pairs in Python dictionaries.
Images related to the topic pretty print dictionary python
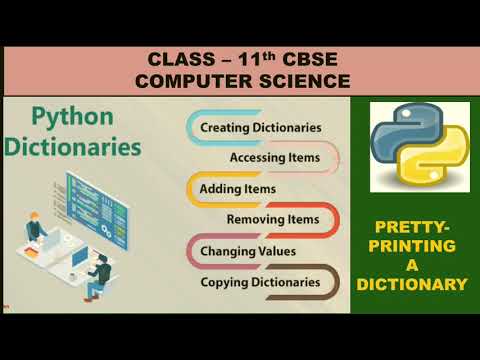
Found 10 images related to pretty print dictionary python theme



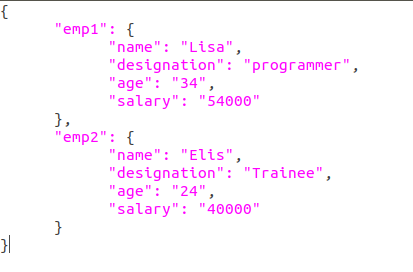

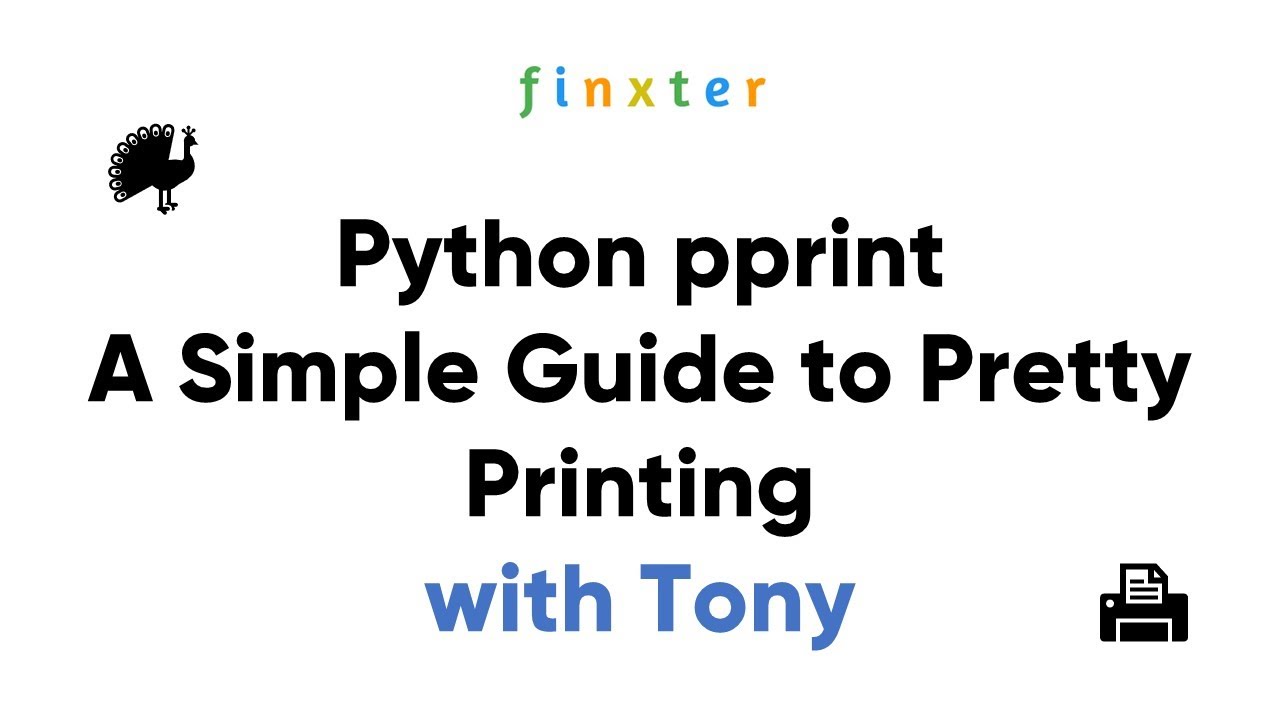
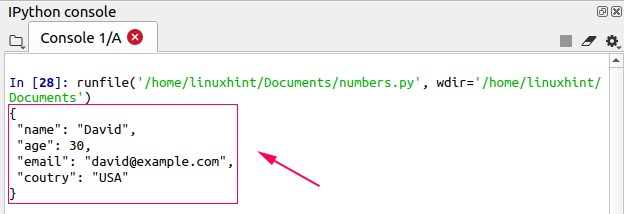
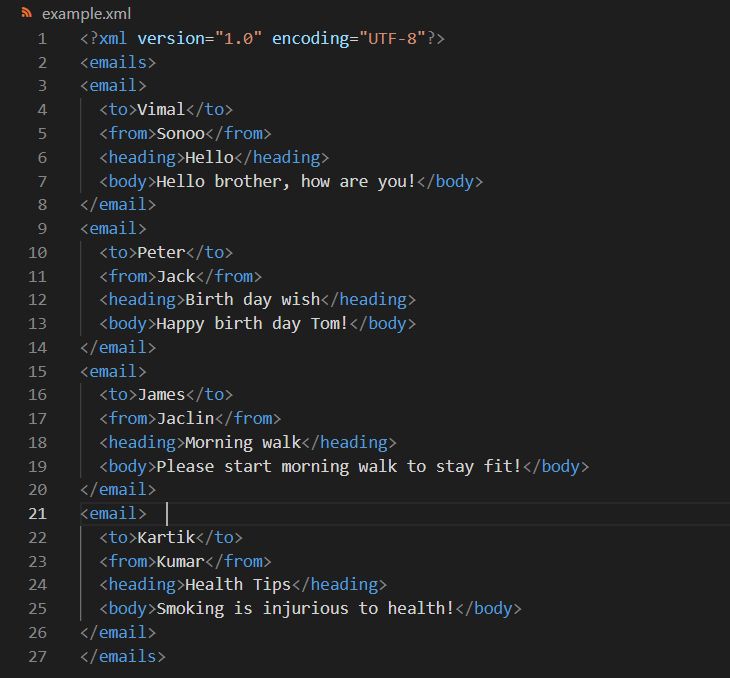


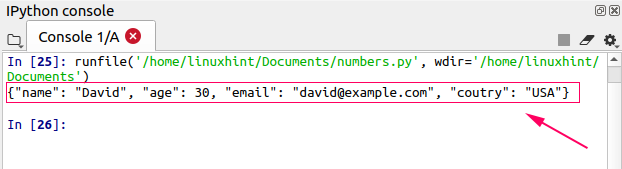

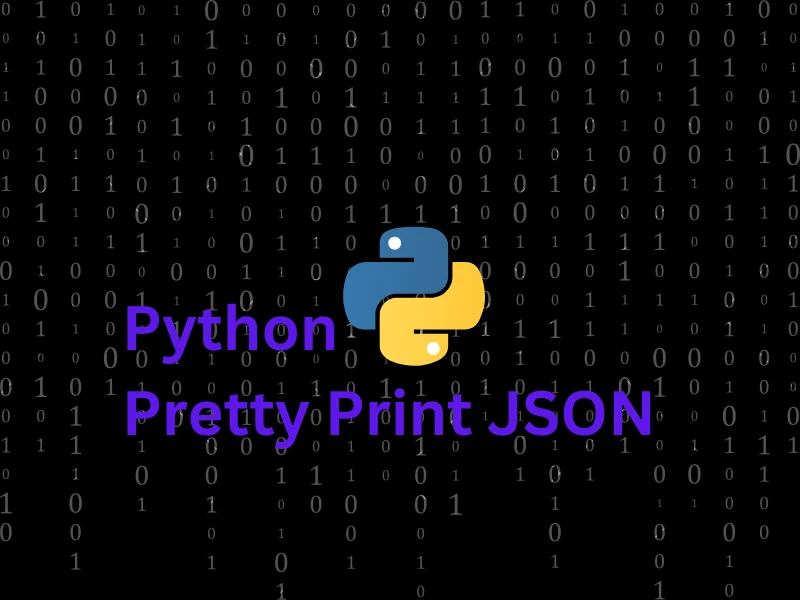
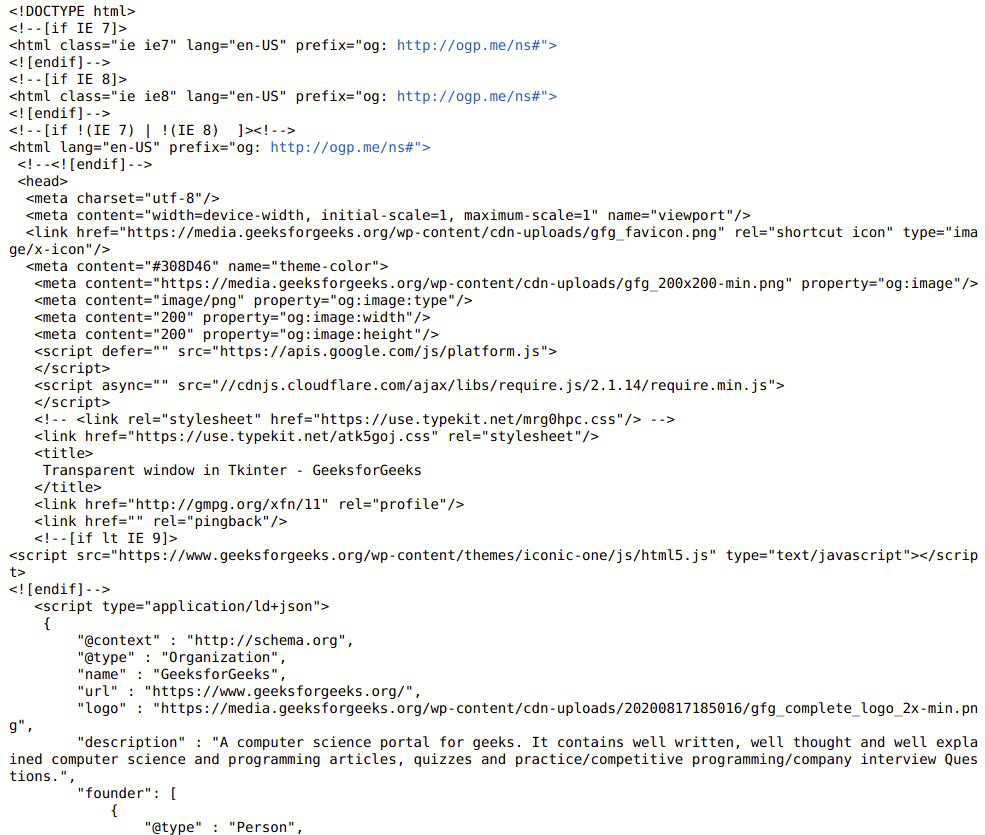


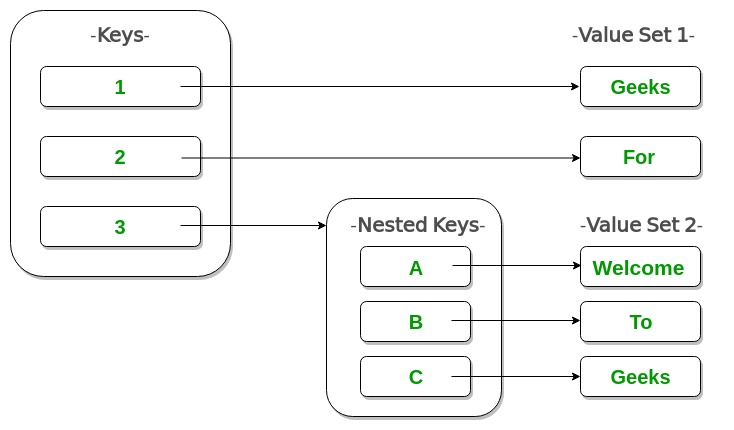
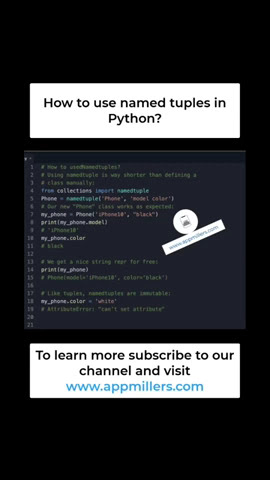

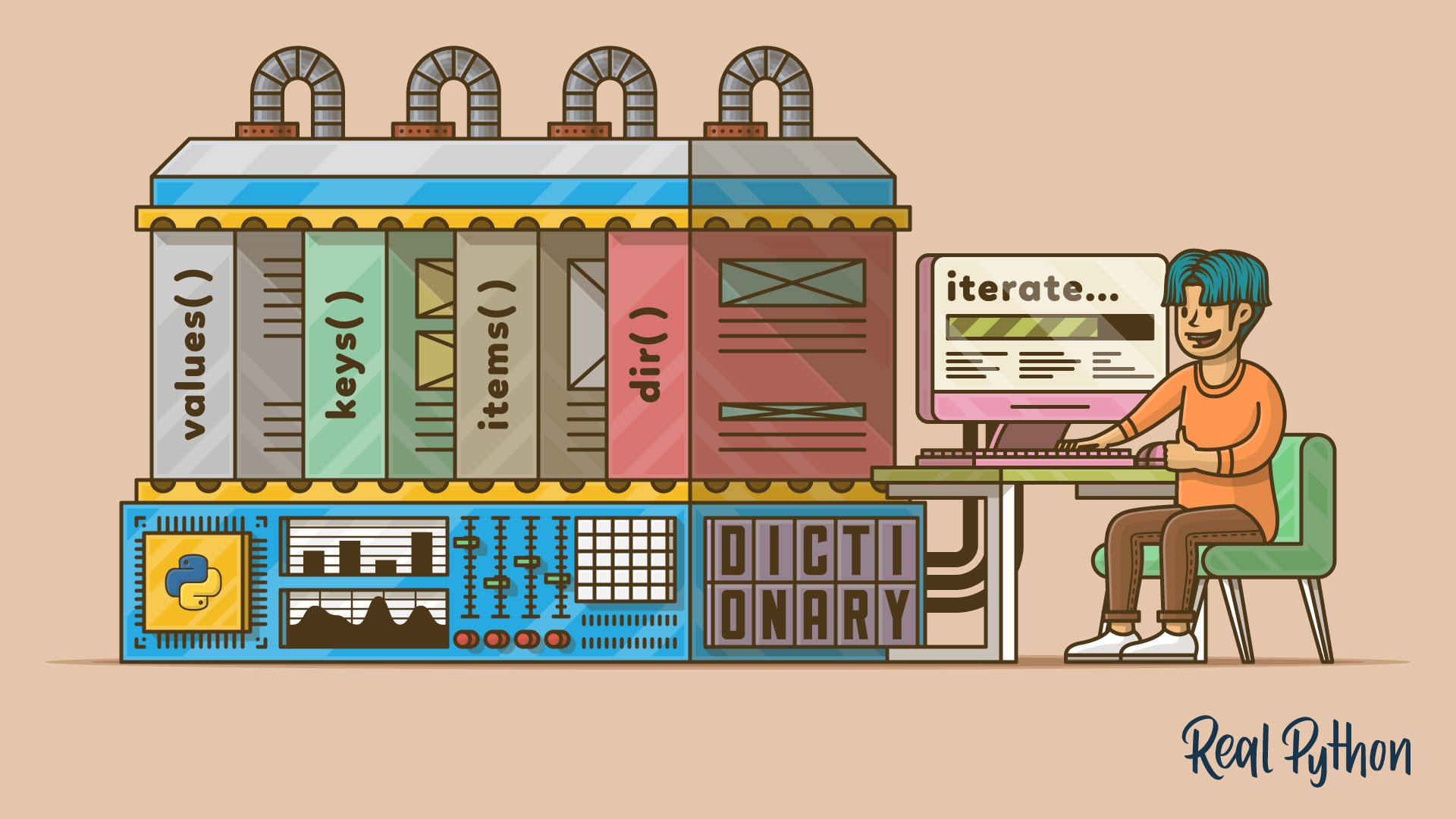
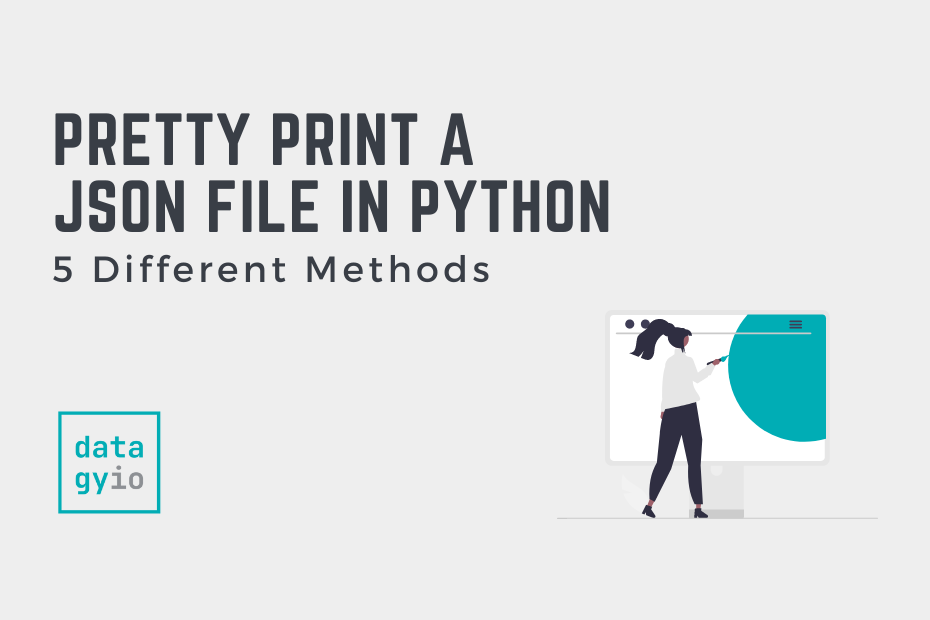
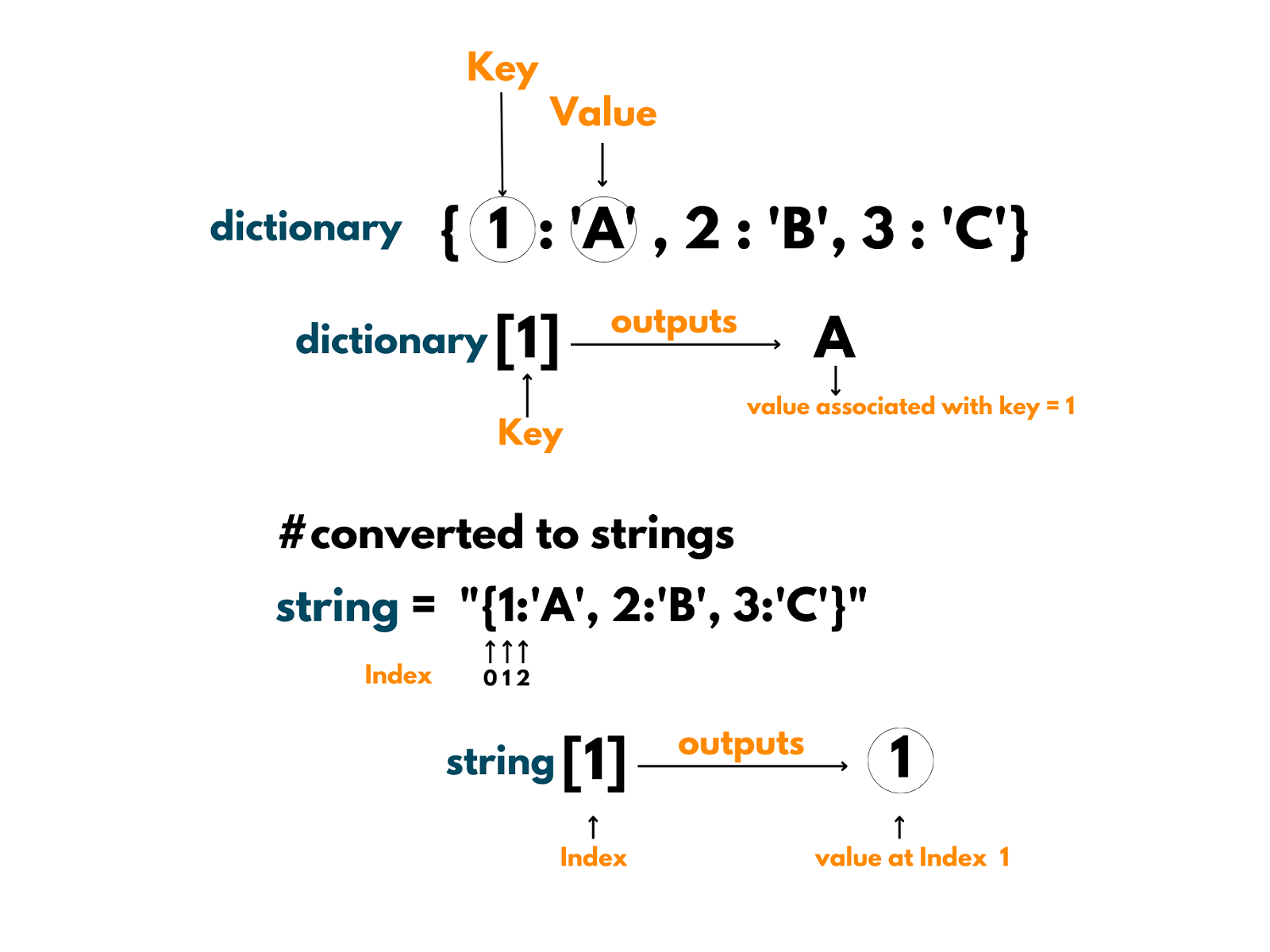
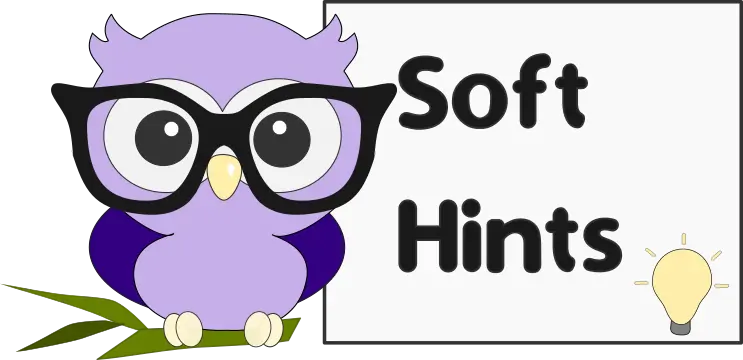
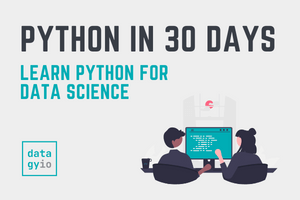

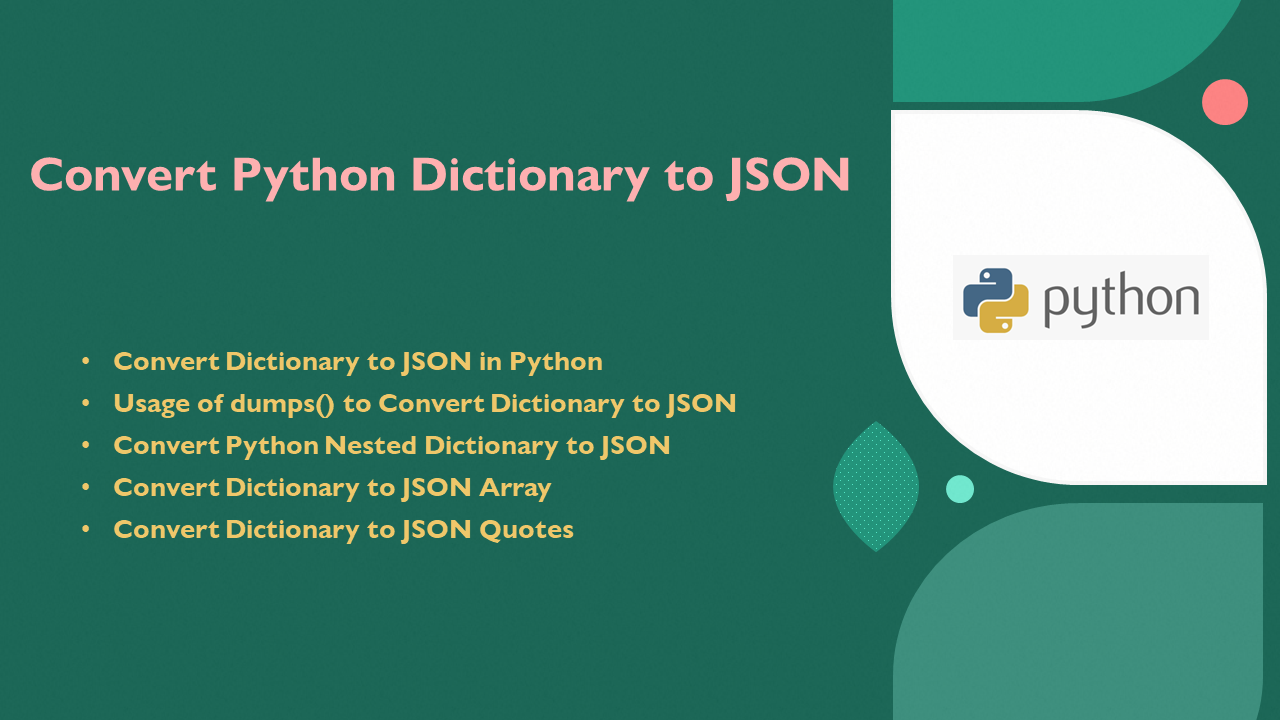
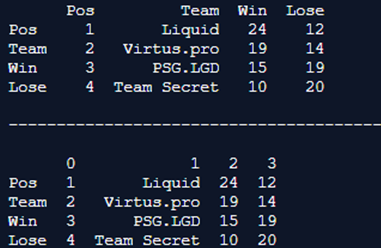


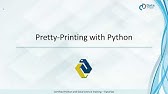
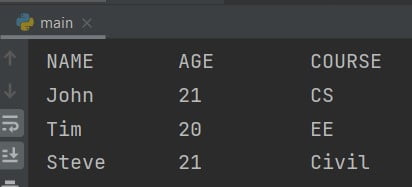
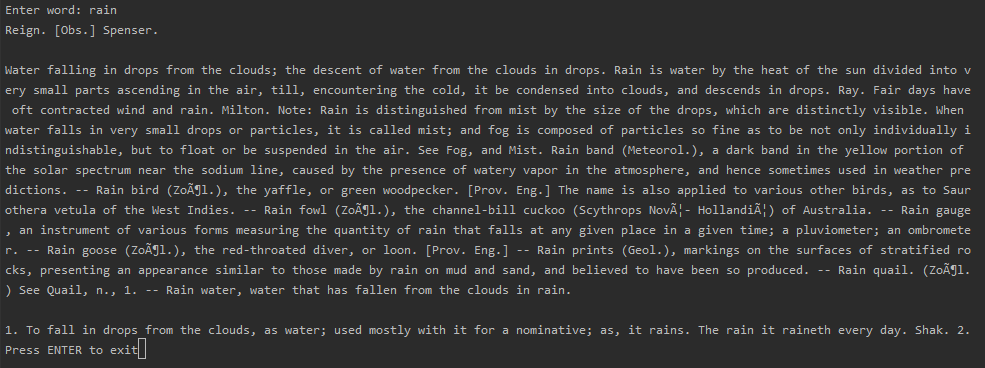
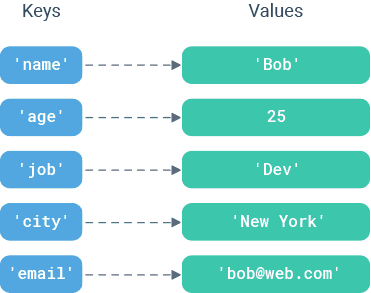
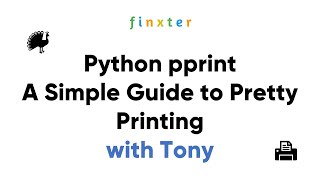
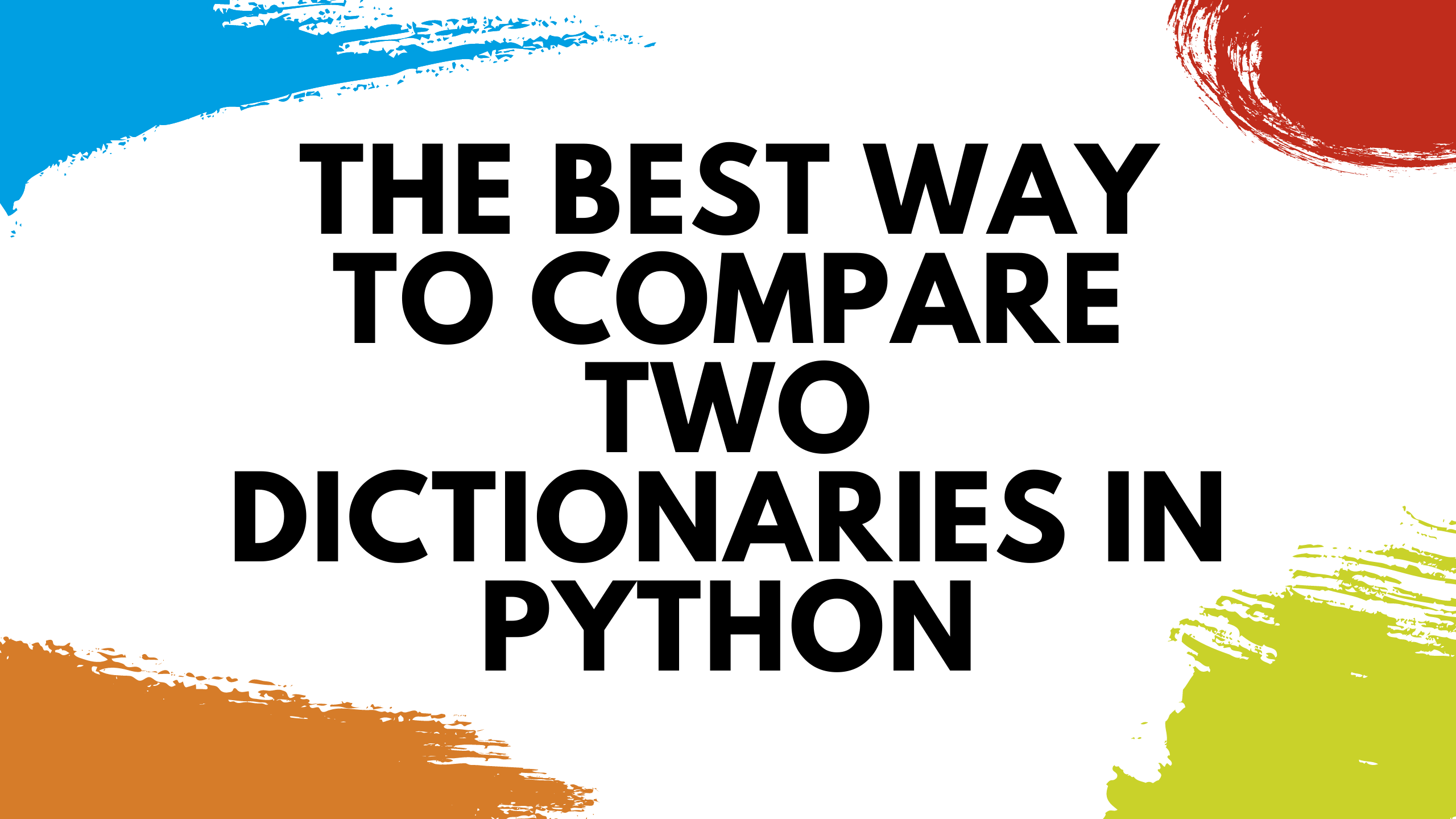


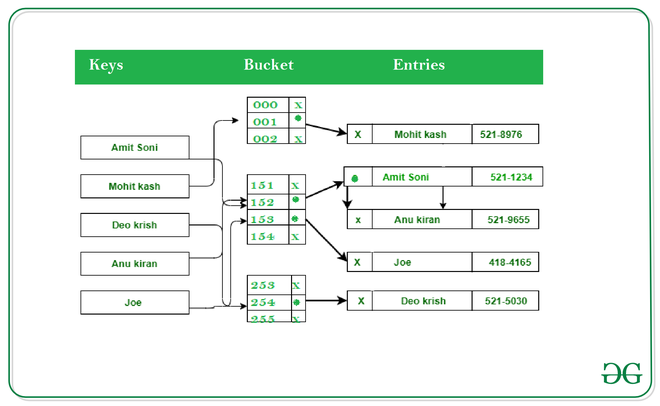
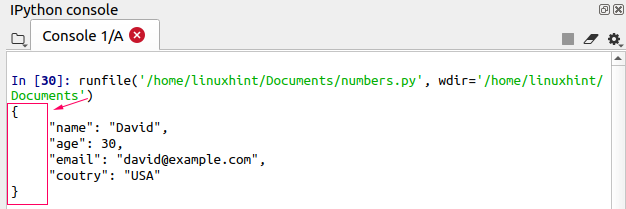
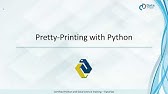
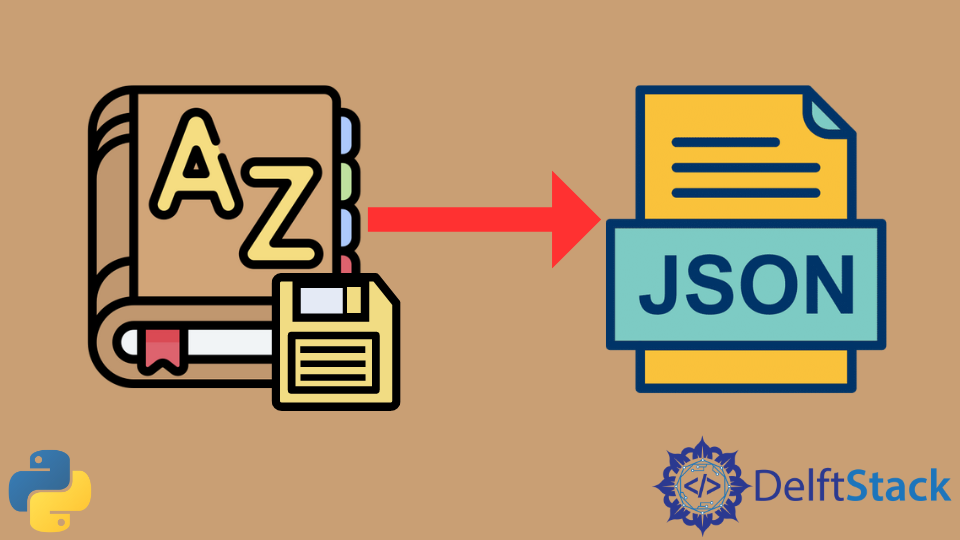

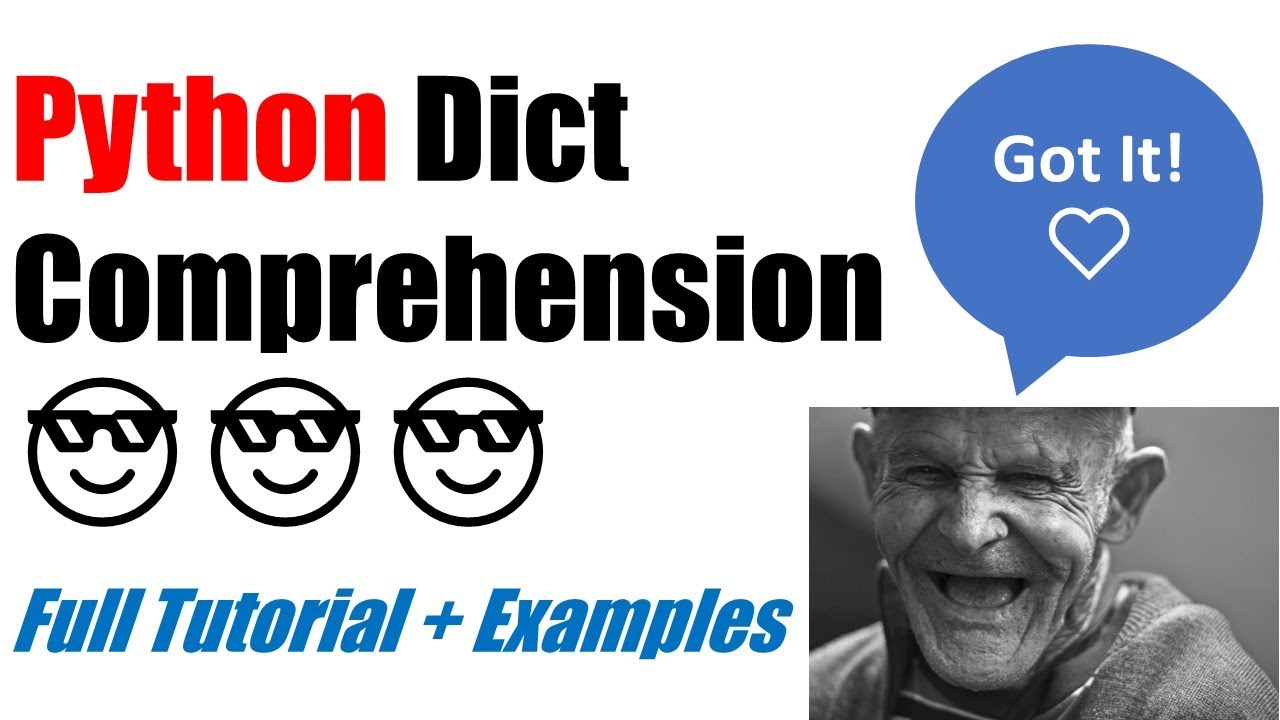


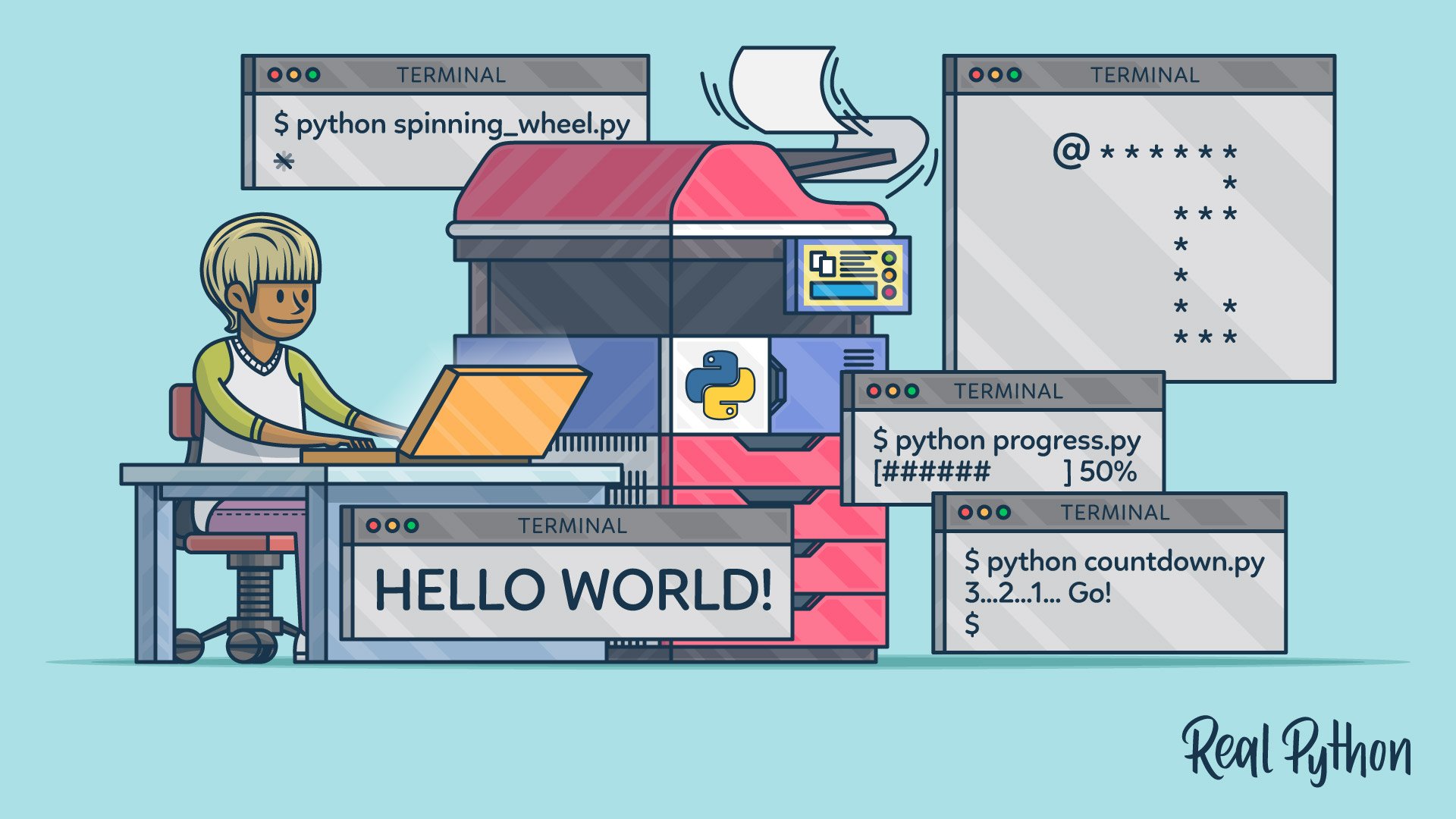
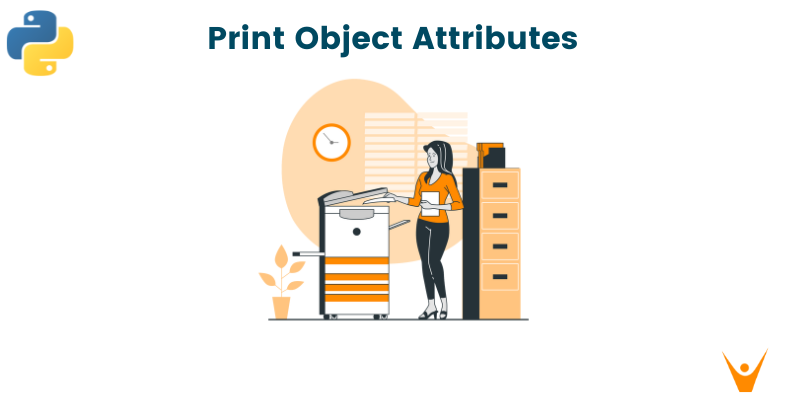
Article link: pretty print dictionary python.
Learn more about the topic pretty print dictionary python.
- Python: Pretty Print a Dict (Dictionary) – 4 Ways – Datagy
- How to pretty print nested dictionaries? – python – Stack Overflow
- Pretty Print Dictionary in Python: pprint & json (with examples)
- Python | Pretty Print a dictionary with dictionary value
- pprint — Data pretty printer — Python 3.11.4 documentation
- Pretty Print Dict Python – Scaler Topics
- How to print all the keys of a dictionary in Python – Tutorialspoint
- Prettify Your Data Structures With Pretty Print in Python
- Python Program to get all unique keys from a List of Dictionaries
- Pretty Print a Dictionary in Python | Delft Stack
- How to Use Pretty Print for Python Dictionaries – Kanaries Docs
- Prettify Your Data Structures With Pretty Print in Python
See more: nhanvietluanvan.com/luat-hoc