Panic: Runtime Error: Invalid Memory Address Or Nil Pointer Dereference
Introduction
Go is a popular programming language known for its simplicity, concurrency, and safety features. However, like any other programming language, it is not immune to errors and bugs. One common error that developers encounter is the panic: runtime error: invalid memory address or nil pointer dereference. This error can be frustrating to deal with, but understanding its causes and finding effective strategies to handle and prevent it can save developers countless hours of debugging.
Understanding Panic in Go
In Go, panic is a built-in function that is used to terminate the normal execution of a program when an unexpected error occurs. When a panic occurs, it triggers a stack unwinding process, which means that the program stops executing its current function and returns to the calling function. If the calling function doesn’t recover from the panic, the program terminates.
Runtime Errors and Their Significance
Runtime errors are errors that occur during the execution of a program. Unlike compile-time errors that are caught during the compilation process, runtime errors are only discovered when the program is running. These errors can be caused by various factors such as invalid input, memory issues, or logic errors in the code. Runtime errors can result in a program crashing or behaving unexpectedly.
Invalid Memory Address Errors
An invalid memory address error occurs when a program attempts to access a memory address that is not valid or has not been allocated. This can happen when the program is trying to read or write data to a pointer that is pointing to an invalid memory address. The error message “runtime error: invalid memory address or nil pointer dereference” indicates that the program has encountered such a situation.
Nil Pointer Dereference Errors
A nil pointer dereference error occurs when a program tries to access or dereference a pointer that has not been initialized or points to nil. In Go, a nil pointer is a pointer that has not been assigned a value or points to nothing. Attempting to access or dereference a nil pointer will result in a panic. The error message “runtime error: invalid memory address or nil pointer dereference” is a common indication of a nil pointer dereference error.
Common Causes of Invalid Memory Address and Nil Pointer Dereference Errors
There are several common causes that can lead to invalid memory address and nil pointer dereference errors in Go:
1. Uninitialized Pointers: If a pointer is not initialized or assigned a valid memory address before it is accessed, it will result in a panic.
2. Dereferencing a Nil Pointer: Accessing or dereferencing a pointer that has not been assigned a value or points to nil will trigger a panic.
3. Null Database Records: When working with databases, it is important to check if a record exists before attempting to access its properties. Trying to access properties of a null database record can lead to a panic.
4. Missing Error Handling: Ignoring or failing to handle errors properly in the code can cause unexpected nil pointer dereference errors.
Strategies to Handle and Prevent Panic: Runtime Error: Invalid Memory Address or Nil Pointer Dereference
Handling and preventing panic errors requires a proactive approach. Here are some strategies to consider:
1. Use Error Handling: Implement proper error handling practices by checking and handling errors throughout your code. This can help prevent nil pointer dereference errors caused by missing error checks.
2. Initialize Pointers: Always initialize pointers before using them to ensure they point to a valid memory address. This can prevent invalid memory address errors.
3. Check for Nil Pointers: Before dereferencing a pointer, check if it is nil. Use conditional statements or Go’s `nil` keyword to handle nil pointer dereference errors gracefully.
4. Validate Database Records: When working with databases, check if a record exists before accessing its properties. Handle null records appropriately to avoid panic errors.
5. Unit Testing: Write comprehensive unit tests for your code. This can help identify and prevent panic errors by catching them before they become a problem in production.
Conclusion
The panic: runtime error: invalid memory address or nil pointer dereference in Go can be a frustrating error to encounter. However, understanding its causes and implementing effective strategies to handle and prevent it can significantly reduce debugging time. By following best practices such as initializing pointers, checking for nil pointers, handling errors properly, and validating database records, developers can write more reliable and robust code in Go.
FAQs (Frequently Asked Questions)
Q: What is “runtime error: invalid memory address or nil pointer dereference” in Go?
A: It is an error that occurs when a program tries to access an invalid memory address or dereference a nil pointer in Go.
Q: How can I prevent panic errors in Go?
A: Some strategies to prevent panic errors include initializing pointers, checking for nil pointers before dereferencing them, handling errors properly, and validating database records.
Q: What causes “invalid memory address or nil pointer dereference” errors in Go SQL?
A: In Go SQL, these errors can be caused by uninitialized pointers, attempting to access properties of null database records, or missing error handling.
Q: What is the significance of runtime errors in Go?
A: Runtime errors occur during the execution of a program and can result in a program crashing or behaving unexpectedly. They are discovered when the program is running, unlike compile-time errors that are caught during compilation.
Q: How can I handle panic errors in Go?
A: Panic errors can be handled by using error handling techniques, such as implementing proper error checks, initializing pointers correctly, and validating database records before accessing their properties. Additionally, writing comprehensive unit tests can help catch panic errors before they reach production.
Nil Pointer Dereference In Golang | Common Mistake In Golang | Dr Vipin Classes
What Is A Nil Pointer In Golang?
In the world of programming, pointers are a fundamental concept that allows us to allocate memory and reference it. They provide a way to efficiently manipulate and access data. Golang, a popular open-source programming language, also supports pointers, including nil pointers.
A nil pointer in Golang is a pointer that does not point to any memory address. It is essentially a placeholder or a special value that indicates the absence of a valid memory address. When a pointer is declared but not assigned any value, it is automatically set to nil.
It is important to note that nil in Golang is not the same as null in other programming languages, as nil is a typed zero value. In Golang, pointers are typed, which means their type must be declared explicitly. Therefore, a nil pointer has a specific type associated with it, indicating the type of data it would normally point to.
When trying to access or dereference a nil pointer, a runtime panic occurs. This panic is a built-in mechanism that Golang employs to handle runtime errors. It prevents the program from continuing to execute, as accessing a nil pointer can lead to unpredictable behavior or crashes. The panic also generates a descriptive error message, indicating that a nil pointer was dereferenced.
To illustrate this concept, consider the following code snippet:
“`go
var ptr *int
fmt.Println(*ptr)
“`
In this example, we declare a variable named `ptr`, which is a pointer to an integer. Since `ptr` has not been assigned any value, it is automatically set to nil. When we try to dereference `ptr` using the `*` operator and print its value, a panic occurs, resulting in an error.
To avoid such panics and safely handle nil pointers, Go allows conditional checks before dereferencing a pointer. This can be achieved using an `if` statement, as shown in the following code:
“`go
if ptr != nil {
fmt.Println(*ptr)
} else {
fmt.Println(“ptr is nil”)
}
“`
By performing the conditional check `ptr != nil`, we ensure that the pointer is not nil before attempting to dereference it. If the check fails, i.e., the pointer is nil, we can handle it appropriately without causing a runtime panic.
Frequently Asked Questions (FAQs):
1. Can I assign a non-nil value to a nil pointer?
No, a nil pointer cannot be assigned a non-nil value directly. However, you can use the reference operator `&` to assign the address of a variable to a nil pointer. For example:
“`go
var ptr *int
var num = 42
ptr = &num
“`
In this case, `ptr` is assigned the address of the `num` variable, making it a valid pointer.
2. How can I check if a pointer is nil?
You can use a simple comparison with the nil value, as shown in the following code snippet:
“`go
if ptr == nil {
fmt.Println(“ptr is nil”)
}
“`
If the pointer is nil, the code within the if block will be executed.
3. What happens if I call a method on a nil pointer?
When a method is called on a nil pointer, Golang’s method set rules treat it as if the method was called on a corresponding value of the same type. However, accessing fields or methods within the method body that require dereferencing the pointer will result in a runtime panic.
4. Can I assign a nil value to a non-pointer variable?
No, you cannot assign a nil value to a non-pointer variable in Golang. Non-pointer variables are always initialized with their zero values according to their type, which represents a sensible default value for that type.
5. Can I compare two nil pointers?
Yes, you can compare two nil pointers using the equality operator, i.e., ==. If both pointers are nil, the comparison will evaluate to true; otherwise, it will evaluate to false.
In conclusion, a nil pointer in Golang represents a pointer that does not point to any valid memory address. It is automatically set to nil when declared without any assignment. When dereferencing a nil pointer, a runtime panic occurs, which can be handled using conditional checks. By understanding and properly handling nil pointers, you can ensure the stability and reliability of your Golang programs.
What Is A Null Pointer Dereference In C++?
In the realm of C++ programming, a null pointer dereference refers to an attempt to access or manipulate the value stored at a memory location using a null pointer. In simpler terms, when a programmer tries to access an object or a variable through a pointer that is assigned NULL (i.e., points to nothing), it leads to a null pointer dereference. This action results in undefined behavior, which can cause the program to crash, produce erroneous outputs, and even introduce security vulnerabilities.
Understanding Pointers in C++
Before delving deeper into null pointer dereferences, it is crucial to have a solid understanding of pointers in the C++ programming language. A pointer is a special type of variable that holds the memory address of another variable. It allows for dynamic memory allocation, efficient data access, and passing variables by reference.
Pointers are incredibly powerful tools in C++, as they enable developers to control memory directly. However, with this power comes added responsibility, as mishandling pointers can lead to various issues, including null pointer dereferences.
The Dangers of Null Pointers
A null pointer is a pointer that does not point to any valid memory location. It is typically represented by the constant value NULL or nullptr in C++. Null pointers occur when a pointer is initialized and assigned no particular memory address or when it is explicitly assigned the value NULL. When a null pointer is dereferenced, attempting to access the object or variable that it points to, the program encounters undefined behavior.
Causes and Implications of Null Pointer Dereferences
Null pointer dereferences can occur for several reasons, including:
1. Failure to initialize a pointer: If a pointer is not initialized, it will have an undefined value, often resulting in a null pointer dereference.
2. Assigning a pointer NULL explicitly: Assigning the value NULL (or nullptr) to a pointer immediately makes it a null pointer, which can lead to dereference issues if not handled properly.
3. Incorrect or forgotten pointer initialization: Pointers can sometimes get assigned NULL during the course of program execution due to programming errors or oversight.
The implications of null pointer dereferences can range from benign to catastrophic, depending on the context and severity of the error. In some cases, the impact may be relatively harmless, resulting in program crashes or exceptions that can be caught and handled. However, null pointer dereferences can also lead to memory corruption, data loss, or even security vulnerabilities if exploited by malicious actors.
Preventing and Detecting Null Pointer Dereferences
To avoid null pointer dereferences, developers should adopt good programming practices and employ various preventive measures, such as:
1. Proper pointer initialization: It is essential to initialize pointers to a valid memory address before usage. This can be achieved by assigning them the address of an existing object or using dynamic memory allocation functions like new to allocate memory.
2. Regularly checking pointers for NULL: Programmers should implement appropriate checks to ensure that pointers are not NULL before attempting to dereference them. By verifying their validity, potential null pointer dereferences can be avoided.
3. Using nullptr instead of NULL: In modern C++, it is recommended to use nullptr instead of NULL to represent null pointers. nullptr provides better type safety and helps avoid certain null pointer-related issues.
Furthermore, static code analysis tools and compilers with advanced error-checking capabilities can aid in identifying potential null pointer dereferences during the development process. Such tools can highlight code segments where null pointer issues may arise, allowing developers to proactively address them.
FAQs:
Q: What happens when a null pointer is dereferenced?
A: When a null pointer is dereferenced, it results in undefined behavior. This can lead to program crashes, erroneous results, memory corruption, or even security vulnerabilities.
Q: How can null pointer dereferences be prevented?
A: Null pointer dereferences can be prevented by initializing pointers to valid memory addresses, checking pointers for NULL before dereferencing, and using modern C++ features like nullptr. Additionally, employing static code analysis tools and error-checking compilers can help identify potential issues.
Q: Are null pointer dereferences specific to C++?
A: No, null pointer dereferences can occur in other programming languages like C and Java, where pointers are also present. However, the impact and handling may vary depending on the language and its specific rules.
Q: Can null pointer dereferences lead to security vulnerabilities?
A: Yes, null pointer dereferences can introduce security vulnerabilities. If exploited, they can allow attackers to control program execution, potentially leading to arbitrary code execution, denial of service attacks, or information disclosure. It is crucial to handle null pointers securely to mitigate such risks.
Q: Are null pointer dereferences always easy to identify?
A: Identifying null pointer dereferences can sometimes be challenging, especially in large codebases. However, with the help of thorough program analysis and diligent programming practices, developers can effectively detect and prevent null pointer dereferences in their code.
Keywords searched by users: panic: runtime error: invalid memory address or nil pointer dereference Invalid memory address or nil pointer dereference, Invalid memory address or nil pointer dereference golang sql, Gorm runtime error invalid memory address or nil pointer dereference, Pointer nil golang, Signal SIGSEGV segmentation violation, Panic in Golang, RowsAffected Golang, Pointer function Golang
Categories: Top 73 Panic: Runtime Error: Invalid Memory Address Or Nil Pointer Dereference
See more here: nhanvietluanvan.com
Invalid Memory Address Or Nil Pointer Dereference
Introduction:
In computer programming, an invalid memory address or nil pointer dereference is a common error that can cause a program to crash or behave unexpectedly. This error occurs when a program attempts to access memory that has not been allocated or points to a null or uninitialized pointer. Understanding this error, its causes, and how to prevent it is crucial for programmers to ensure the stability and reliability of their software.
In this article, we will delve into the details of invalid memory address or nil pointer dereference, exploring its causes, consequences, and potential solutions. Additionally, we will address frequently asked questions (FAQs) related to this error.
Section 1: Causes of Invalid Memory Address or Nil Pointer Dereference
Invalid memory address or nil pointer dereference can result from various programming mistakes or issues. Here are some common causes:
1. Uninitialized Pointers: When a pointer is not assigned a valid memory address before its usage, it will point to nil. Any attempt to access or modify the memory location containing nil will result in an error.
2. Incorrect Memory Allocation: If memory is not allocated or is allocated incorrectly using functions like malloc() or new, attempting to access this unallocated or misallocated memory can lead to the invalid memory address error.
3. Dereferencing a Null Pointer: Dereferencing a pointer that has been explicitly set to null will result in a nil pointer dereference. It is essential to ensure that a pointer has a valid memory address before accessing its value.
4. Inadequate Error Handling: Neglecting to check for potential errors during pointer manipulation or memory allocation can lead to accessing invalid memory addresses or nil pointers. Failing to handle errors properly can aggravate the occurrence of this error.
Section 2: Consequences and Impact
When a program encounters an invalid memory address or nil pointer dereference, severe consequences can arise, affecting both the program and the system it runs on. Here are a few potential consequences:
1. Program Crashes: A common outcome of this error is a program crash, resulting in an abrupt termination of the software. This can lead to data loss or other detrimental effects for users.
2. Unpredictable Behavior: Invalid memory access can cause a program to exhibit unpredictable behavior. It may produce incorrect results, display random values, or invoke undefined behavior, making it challenging to trace the source of the problem.
3. Security Vulnerabilities: In some cases, if an attacker can manipulate a program to trigger invalid memory access, security vulnerabilities may be exploited. This can allow unauthorized access to sensitive data or enable malicious code execution.
Section 3: Preventing Invalid Memory Address or Nil Pointer Dereference
While the consequences of invalid memory address or nil pointer dereference can be severe, there are several preventive measures programmers can take to minimize the occurrence of this error. Here are some best practices to consider:
1. Initializing Pointers: Always initialize pointers before usage to a reasonable default value, such as nil or a valid memory address. This way, you actively avoid accessing uninitialized or null pointers, reducing the risk of encountering this error.
2. Proper Pointer Validation: Before accessing or dereferencing a pointer, ensure it holds a valid memory address. Use conditional checks to verify if a pointer is nil and handle such cases gracefully by avoiding any access or modification.
3. Error Handling: Implement comprehensive error handling mechanisms within your code. Properly check for potential errors, handle them gracefully, and provide informative error messages. This ensures that unexpected pointer-related errors are caught and addressed promptly.
4. Static Analysis and Debugging Tools: Utilize static analysis tools like linters and debuggers to identify potential invalid memory access issues during development. These tools can be invaluable in locating bugs, addressing them early, and preventing crashes in production.
Section 4: FAQs (Frequently Asked Questions):
Q1. Do all programming languages exhibit invalid memory address or nil pointer dereference errors?
A1. Most programming languages that utilize pointers have the potential for this error. However, some modern languages, such as Rust or Go, incorporate features that minimize or eliminate the possibility of encountering invalid memory addresses or nil pointer dereferences.
Q2. Can invalid memory address errors be caused by hardware issues?
A2. Although hardware issues may indirectly lead to memory-related errors, invalid memory address errors primarily occur due to programming mistakes. Modern operating systems and hardware utilize mechanisms to protect against illegal memory access, making it unlikely for hardware to be the root cause.
Q3. How can I locate the source of an invalid memory address or nil pointer dereference error?
A3. Debugging is crucial in identifying the source of this error. Use a debugger to track the flow of your program, analyze error messages, and examine the stack trace. Additionally, static analysis tools and code reviews can help uncover potential issues and minimize the occurrence of these errors.
Conclusion:
Invalid memory address or nil pointer dereference errors can significantly impact program stability, behavior, and security. With a thorough understanding of the causes and preventive measures discussed in this article, programmers can develop more reliable software and minimize the occurrence of this error. Adopting best practices and leveraging debugging tools will be instrumental in detecting and resolving these errors, leading to more robust and predictable software systems.
Invalid Memory Address Or Nil Pointer Dereference Golang Sql
Golang, also known as Go, has gained immense popularity among developers for its simplicity, efficiency, and strong support for concurrency. When it comes to working with databases, many developers turn to Golang’s excellent SQL package, which provides a robust and convenient way to interact with various database systems. However, like any programming language, Golang is not exempt from errors, and one common error that developers often encounter when dealing with Golang SQL is the “Invalid memory address or nil pointer dereference” error. In this article, we will delve into the causes of this error and discuss effective troubleshooting techniques to resolve it.
Understanding the Error:
The “Invalid memory address or nil pointer dereference” error in Golang SQL typically occurs when a developer attempts to access or dereference a nil pointer or invalid memory address. In simpler terms, it means that the variable being referenced is either uninitialized (nil) or pointing to an unknown memory location. This error can manifest itself while performing various database operations such as querying, inserting, updating, or deleting data.
Causes of the Error:
1. Nil Pointer: One common cause of this error is when a variable or pointer in the code is not properly initialized or assigned a value. This can occur if a pointer variable is declared but not explicitly assigned to any valid memory address or if a structure field is not set with a valid value.
2. Database Connection Issues: In some cases, the error results from incorrect or incomplete setup of the database connection. If the connection to the database is not established correctly or if the connection parameters are missing or invalid, the SQL package may encounter a nil pointer or invalid memory address when attempting to perform database operations.
3. Query Execution Problems: The error can also be triggered by executing an incomplete or malformed SQL query. If a query includes incorrect syntax, missing parameters, or attempts to access non-existent database tables or columns, it can result in a nil pointer dereference error.
Troubleshooting Techniques:
1. Check for Nil Pointers: Analyze your code to identify any uninitialized or incorrectly assigned variables. Ensure that all pointers are properly initialized before being utilized. Initializing pointers can be done by assigning them to a new object or using the “make” function for slices or maps.
2. Validate Database Connection: Double-check your database connection parameters such as the host, port, username, and password. Ensure that you correctly establish the database connection before executing any SQL statements. Additionally, confirm that the required database driver is imported and registered in your code.
3. Review and Correct SQL Queries: Inspect your SQL queries for any syntax errors, missing parameters, or incorrect table/column names. Use prepared statements or parameterized queries to avoid injection vulnerabilities and ensure that all required parameters are properly bound before execution.
4. Enable Detailed Logging: Utilize Golang’s built-in logging package to log detailed information about the error. This can help in pinpointing the exact location and cause of the error, making troubleshooting easier. Enable logging for your SQL queries and examine the log output to identify any inconsistencies or abnormalities.
FAQs:
Q1. Can this error occur even if my Go code is correct?
A1. Yes, this error can occur even if your Go code is correct. It often arises from issues related to the database connection or incorrect SQL queries. Double-checking these aspects and following the troubleshooting techniques mentioned above should help resolve the error.
Q2. How can I prevent this error from occurring?
A2. To prevent this error, it is crucial to follow best practices such as properly initializing variables, validating database connections, and using parameterized queries. Additionally, incorporating thorough testing and debugging practices will help catch and resolve such errors early.
Q3. Are there any specific Golang SQL libraries that are less prone to this error?
A3. Golang’s built-in SQL package is widely used and well-regarded. However, there are also third-party libraries available, such as GORM and sqlx, that provide additional features and convenience. While these libraries may have their own error handling mechanisms, understanding the underlying concepts of the “Invalid memory address or nil pointer dereference” error is vital regardless of the library employed.
In conclusion, encountering the “Invalid memory address or nil pointer dereference” error in Golang SQL can be frustrating, but understanding its causes and utilizing effective troubleshooting techniques will help you resolve it. By taking care of variable initialization, validating database connections, and double-checking SQL queries, you can ensure a smoother and error-free experience when working with Golang SQL.
Images related to the topic panic: runtime error: invalid memory address or nil pointer dereference
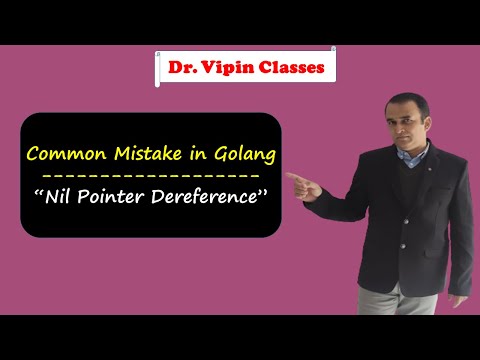
Found 48 images related to panic: runtime error: invalid memory address or nil pointer dereference theme





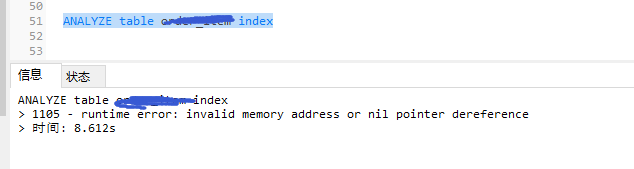
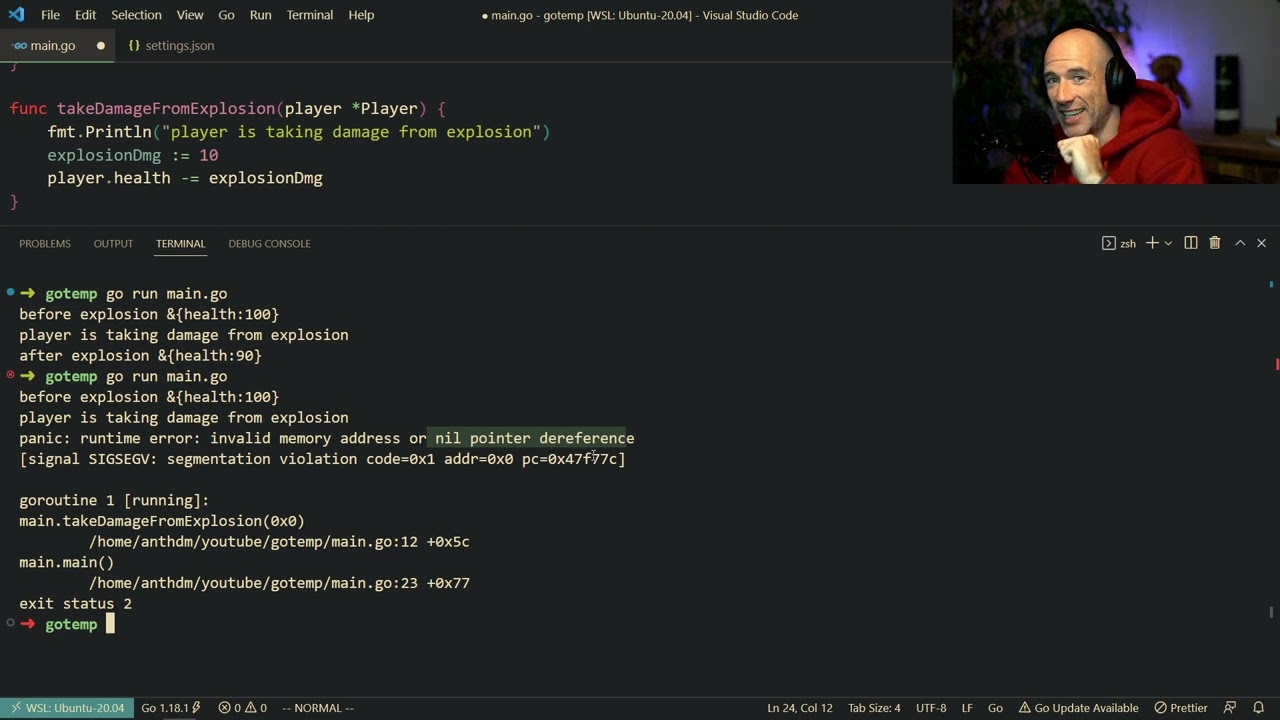


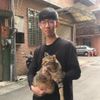
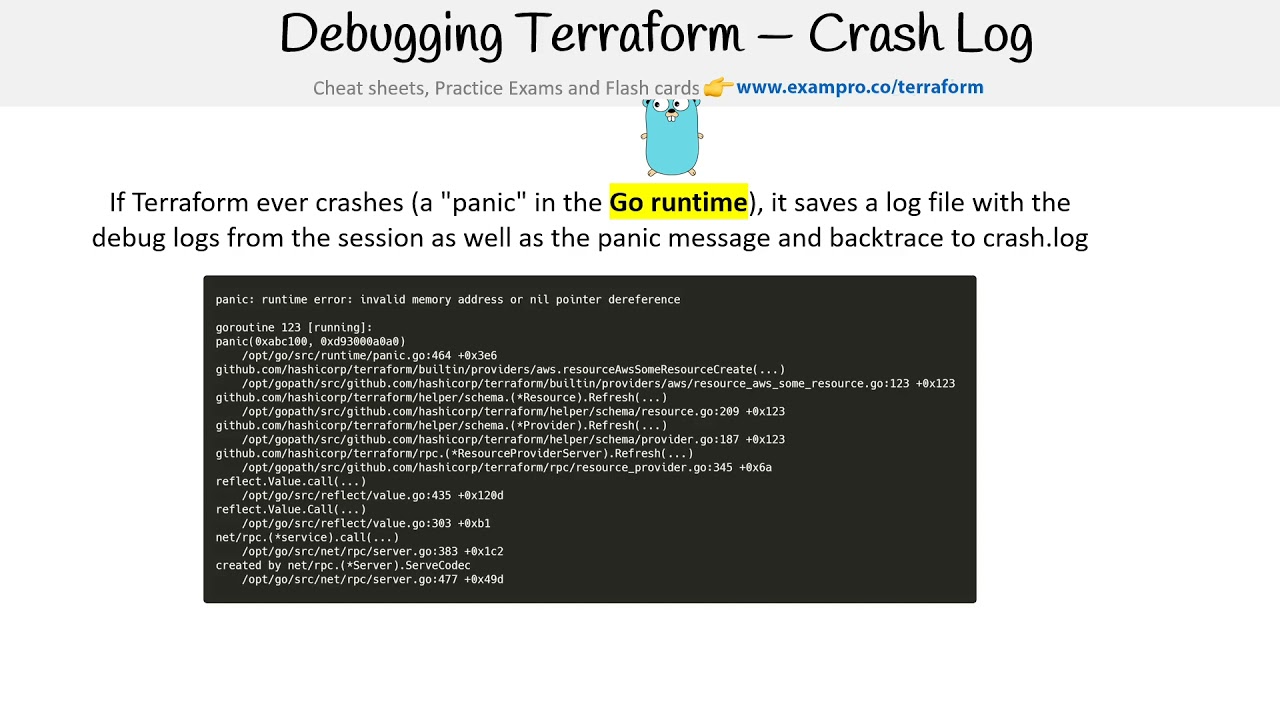


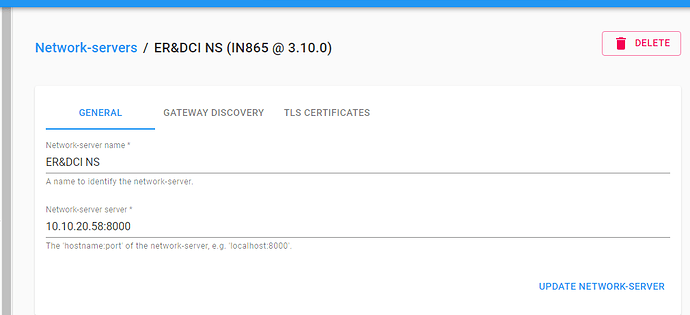
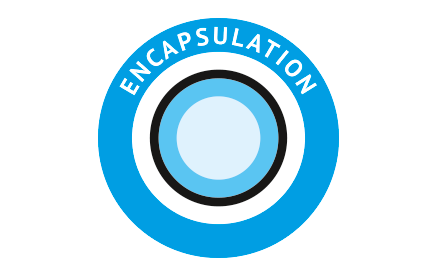
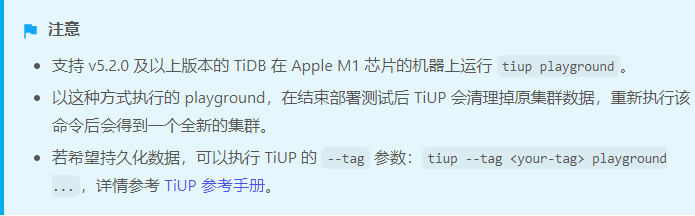

Article link: panic: runtime error: invalid memory address or nil pointer dereference.
Learn more about the topic panic: runtime error: invalid memory address or nil pointer dereference.
- Go: panic: runtime error: invalid memory address or nil pointer …
- Invalid memory address or nil pointer dereference – YourBasic
- Invalid memory address or nil pointer dereference error in …
- How to Fix panic: runtime error: invalid memory address or nil …
- invalid memory address or nil pointer dereference golang …
- 1]:60288: runtime error: invalid memory address or nil pointer …
- Nil Pointer Deference in Golang – Mo Ashouri – Medium
- CWE-476: NULL Pointer Dereference (4.11) – MITRE
- Null reference creation and null pointer dereference
- Null Dereference – OWASP Foundation
- RUNTIME ERROR: INVALID MEMORY ADDRESS OR NIL …
- panic: runtime error: invalid memory address or nil pointer …