Pandas Vectorized String Operations
In the realm of data manipulation and analysis, efficient processing of string data is crucial. Python’s pandas library provides a wide range of tools for handling and manipulating string operations in a vectorized manner. This article will explore the various advantages and functionalities of pandas vectorized string operations, along with some common use cases and FAQs.
Advantages of Vectorized String Operations:
1. Faster Processing: Vectorized string operations in pandas are optimized for speed. They leverage highly efficient algorithms and underlying C implementations, resulting in significant performance improvements compared to traditional iterative approaches.
2. Ease of Use: Pandas’ vectorized string methods are designed to be user-friendly and intuitive. They allow you to perform various string operations using simple and concise syntax, minimizing the need for complex and error-prone manual coding.
3. Improved Productivity: By leveraging vectorized string operations, you can process large volumes of data quickly and efficiently. This can boost your productivity, allowing you to focus more on analysis and insights rather than spending excessive time on data preparation and cleaning.
Now, let’s dive into some of the key functionalities provided by pandas vectorized string operations:
1. String Accessing and Indexing:
Pandas allows you to access individual characters within a string using the indexing operator `[]`. For example, `df[‘column_name’].str[0]` will return the first character of each element in the specified column.
2. String Slicing and Subsetting:
You can perform string slicing operations using the `.str.slice()` method. It allows you to extract substrings based on specified start and end positions. Additionally, the `.str.contains()` method enables you to subset data based on whether a string contains a particular substring.
3. String Concatenation and Repetition:
Pandas provides the `.str.cat()` method for concatenating strings in a vectorized manner. It allows you to combine strings from multiple columns or series. Similarly, you can repeat a string multiple times using the `.str.repeat()` method.
4. String Searching and Replacing:
Pandas’ vectorized string operations include powerful search and replace methods. The `.str.match()` and `.str.contains()` methods allow you to search for patterns or substrings within strings. Additionally, the `.str.replace()` method enables you to replace substrings with specified values.
5. String Splitting and Joining:
The `.str.split()` method allows you to split strings into substrings based on a specified delimiter. Conversely, you can join multiple strings using the `.str.join()` method, which concatenates the strings using a specified delimiter.
6. String Case Conversion:
Pandas provides various methods to convert the case of strings. You can convert strings to lowercase using `.str.lower()` or to uppercase using `.str.upper()`. There are also methods available for capitalizing the first letter of each word (`str.title()`) and swapping the case of characters (`str.swapcase()`).
7. String Stripping and Padding:
The `.str.strip()` and `.str.rstrip()` methods allow you to remove leading and trailing whitespace from strings. Conversely, the `.str.pad()` method enables you to add padding characters to the left or right of the strings.
8. String Matching and Regular Expressions:
Pandas supports vectorized string matching using regular expressions. The `.str.contains()` method with a regular expression pattern allows you to search for complex patterns within strings. The `.str.extract()` method enables you to extract substrings based on a regular expression pattern.
In addition to these functionalities, pandas offers a range of other vectorized string operations, including string interpolation, string extraction, and more. These tools provide immense flexibility and power for data manipulation with string data.
—
FAQs:
Q1. How does pandas achieve faster processing with vectorized string operations?
Pandas exploits underlying C implementations to optimize string operations. It avoids costly iteration by applying operations on entire arrays of strings at once, resulting in significant performance improvements.
Q2. Can I apply vectorized string operations on entire columns or series in pandas?
Yes, pandas provides methods like `.str` accessor that enables you to apply vectorized string operations on entire columns or series efficiently.
Q3. Can I combine vectorized string operations with other data manipulation functions in pandas?
Absolutely! Pandas allows you to seamlessly integrate string operations with other data manipulation functions like filtering, aggregation, and sorting. This enables you to perform complex data transformations and manipulations easily.
Q4. Are vectorized string operations memory-efficient?
Yes, pandas optimizes memory usage by minimizing intermediate object creation during string operations. This ensures efficient memory utilization, even when dealing with large datasets.
Q5. Can I use regular expressions with pandas vectorized string operations?
Yes, pandas supports vectorized regular expression operations. You can apply regular expressions to search, extract, or manipulate strings using methods like `.str.contains()` and `.str.extract()`.
—
In conclusion, pandas vectorized string operations bring significant advantages in terms of speed, ease of use, and productivity. By leveraging these tools, you can efficiently manipulate and process string data, saving valuable time and effort. Incorporating pandas’ vectorized string operations in your data analysis workflow can greatly enhance your productivity and provide more accurate and meaningful insights.
Introduction – Vectorized String Operations
What Are Vectorized String Operations In Pandas?
Pandas, the popular data manipulation and analysis library in Python, offers a range of powerful tools to handle string operations efficiently. One such feature is vectorized string operations, which provide an efficient way to apply string transformations and manipulations across entire arrays or DataFrames. In this article, we will explore what vectorized string operations are, their benefits, and how to use them effectively in pandas.
Understanding Vectorized String Operations
Traditionally, string operations in Python are performed on individual elements of a string, resulting in slower execution times when working with large datasets. However, pandas addresses this limitation by implementing vectorized string operations, which process entire arrays of strings at once, resulting in faster and more efficient computations.
These operations, built upon the powerful NumPy library, leverage efficient low-level C implementations, enabling large-scale string computations to be performed quickly. They allow us to avoid the need for looping through individual elements and instead process entire arrays in a single step.
Benefits of Vectorized String Operations
The use of vectorized string operations in pandas offers several advantages over traditional element-wise string operations. Let’s explore a few key benefits:
1. Efficiency: Due to the underlying C implementation, vectorized string operations are significantly faster compared to iterative element-wise approaches. This makes them particularly beneficial when working with large datasets or performing computationally intensive tasks.
2. Simplified Syntax: Vectorized operations provide a more concise and readable syntax by eliminating the need for explicit looping constructs or list comprehensions. This simplifies code maintenance, reduces the likelihood of errors, and enhances code clarity.
3. Versatility: Pandas’ vectorized string operations support a wide range of operations, including string extraction, concatenation, substitution, case conversion, pattern matching, and more. These operations enable us to perform complex string manipulations efficiently and in a highly flexible manner.
4. Integration with Pandas Ecosystem: Vectorized string operations seamlessly integrate with other pandas functionalities, such as filtering, grouping, and aggregating, allowing for more efficient and expressive data analysis workflows.
Usage of Vectorized String Operations in Pandas
To leverage the power of vectorized string operations in pandas, we can utilize the `.str` attribute present on string-type columns within a DataFrame or a Series. This attribute provides access to various string methods, allowing us to apply string transformations and manipulations across entire arrays effortlessly.
Let’s take a look at a few examples to illustrate the usage of these operations:
1. Extracting Substrings: We can use methods such as `str.extract()` or `str.split()` to extract substrings from a column of strings based on specified patterns or delimiters.
2. Replacing Substrings: Methods like `str.replace()` or `str.replace()`, facilitate the replacement of specific substrings or patterns with alternate strings.
3. Changing Case: The `.str` attribute provides methods like `str.lower()` and `str.capitalize()` to convert strings to lowercase or capitalize the first letter, respectively.
4. Checking Pattern Match: We can use methods such as `str.contains()` or `str.match()` for pattern matching or checking the presence of a pattern in a string.
FAQs
Q: Are vectorized string operations only applicable to DataFrames?
A: No, vectorized string operations can be applied to both DataFrame columns and individual Series.
Q: Can I apply these operations to non-string columns?
A: No, vectorized string operations are specifically designed for string-type columns and series.
Q: Do vectorized string operations modify the original DataFrame or Series?
A: No, vectorized string operations create new Series or DataFrames, leaving the original unchanged. If needed, we can assign the result back to the desired column.
Q: Are vectorized string operations case-sensitive?
A: By default, most vectorized string operations in pandas are case-sensitive. However, options exist to make them case-insensitive when required.
Q: Can I apply custom string functions using vectorized operations?
A: Yes, pandas allows the application of custom string functions by utilizing the `.apply()` method in combination with vectorized string operations.
In conclusion, vectorized string operations in pandas offer a powerful and efficient way to perform string transformations and manipulations on large datasets. By leveraging these operations, we can greatly enhance the speed and readability of our code while achieving complex string computations with ease. Understanding and utilizing these features enables us to streamline data analysis workflows and extract valuable insights from textual data efficiently.
How To Apply String Operation For A Pandas Column?
Pandas is a powerful data manipulation library in Python that provides numerous features to work with structured data. One of the essential aspects of working with data is dealing with string operations. Pandas offers various functions and methods to perform string operations on columns efficiently. In this article, we will explore different ways to apply string operations for a pandas column and demonstrate how to utilize them effectively.
Understanding Pandas String Operations:
Before diving into the implementation details, it’s essential to comprehend the basics of pandas string operations. Pandas treats string operations as vectorized operations. This means that instead of iterating over each element individually, pandas operates on the entire column as a whole. As a result, string operations in pandas are generally faster compared to traditional iteration-based methods.
Applying String Operations:
Pandas provides several techniques to apply string operations over a column:
1. Accessing String Methods:
Pandas offers an `.str` accessor, which provides access to various string methods. These methods have a similar syntax to regular Python string methods. For instance, to convert all characters to uppercase in a column named `’name’`, we can use `’name’.str.upper()`.
2. Using Regular Expressions (regex):
Pandas allows us to utilize the power of regular expressions to perform complex string operations. The `.str` accessor provides methods like `.contains()`, `.replace()`, `.extract()`, etc., which accept regex patterns as arguments.
3. Splitting or Joining Strings:
Pandas provides convenient methods like `.str.split()` and `.str.join()` to split or join strings in a column. These methods can be beneficial for handling string values that are separated by delimiters or need to be merged together.
Now, let’s delve into some practical examples to see these techniques in action.
Example 1: Convert Names to Uppercase
Suppose we have a pandas DataFrame with a `’Name’` column containing names in lowercase. To convert the names to uppercase, we can apply the `.str.upper()` method:
“`python
import pandas as pd
df = pd.DataFrame({‘Name’: [‘john’, ‘david’, ’emma’]})
df[‘Name’] = df[‘Name’].str.upper()
print(df)
“`
Output:
“`
Name
0 JOHN
1 DAVID
2 EMMA
“`
Example 2: Extracting Portion of a String
Suppose we have a DataFrame with a `’URL’` column containing URLs. We want to extract the domain names from those URLs. We can do this using the `.str.extract()` method with a regex pattern:
“`python
import pandas as pd
df = pd.DataFrame({‘URL’: [‘https://www.example.com’, ‘http://www.test.com’]})
df[‘Domain’] = df[‘URL’].str.extract(r'(https?://(?:www\.)?(.+?)/)?’)
print(df)
“`
Output:
“`
URL Domain
0 https://www.example.com example.com
1 http://www.test.com test.com
“`
FAQs:
Q: Can I apply string operations to multiple columns simultaneously in pandas?
A: Yes, you can apply string operations to multiple columns by specifying them in a list. For example, to convert two columns `’Name’` and `’Address’` to uppercase, you can use:
“`python
df[[‘Name’, ‘Address’]] = df[[‘Name’, ‘Address’]].apply(lambda x: x.str.upper())
“`
Q: Can I use string operations on non-string columns?
A: No, pandas’ string operations work only on columns with string data types. If you try to apply string operations on non-string columns, it will result in an error.
Q: How can I remove leading or trailing whitespaces from a column?
A: You can use the `.str.strip()` method to remove leading or trailing whitespaces from a column. For example, to remove whitespaces from a column `’Text’`, use:
“`python
df[‘Text’] = df[‘Text’].str.strip()
“`
Q: Is it possible to perform case-insensitive comparisons using string operations in pandas?
A: Yes, you can perform case-insensitive comparisons using various string methods like `.contains()`, `.startswith()`, etc., by setting the `case` parameter to `False`. For example:
“`python
df[df[‘Name’].str.contains(‘jO’, case=False)]
“`
Conclusion:
Applying string operations for pandas columns is a fundamental task in data manipulation. Pandas provides a rich set of string methods and regex-based functions to efficiently perform string operations. By utilizing these techniques, you can manipulate and extract valuable information from textual data in pandas, opening the door to a wide range of data analysis possibilities.
Keywords searched by users: pandas vectorized string operations Pandas vectorization, pandas vectorized regex, Np vectorize, pandas fast string processing, Data manipulation with pandas, pandas vectorized string interpolation, Concat string pandas, Pandas string
Categories: Top 52 Pandas Vectorized String Operations
See more here: nhanvietluanvan.com
Pandas Vectorization
Pandas is a popular open-source library in Python that provides high-performance data manipulation and analysis tools. One of the most powerful features of Pandas is its ability to leverage vectorization to efficiently perform operations on large datasets. Vectorization is an essential concept in Pandas, enabling users to apply operations to all elements of an array or DataFrame simultaneously, resulting in faster and more concise code. In this article, we will delve into the world of Pandas vectorization, exploring its benefits, best practices, and addressing some frequently asked questions.
Understanding Vectorization:
Vectorization, in the context of Pandas, involves performing operations on entire arrays or columns of a DataFrame, in a single step, without using explicit loops. Under the hood, Pandas leverages the power of NumPy, a numerical computing library, to implement vectorized operations efficiently using highly optimized and parallelized algorithms. This approach eliminates the need for explicit iteration over each individual element, resulting in significant performance improvements.
Benefits of Vectorization:
1. Improved Performance: By eliminating explicit loops, vectorized operations in Pandas can take advantage of highly optimized and parallelized NumPy routines, resulting in faster execution times when working with large datasets. This performance gain is particularly noticeable compared to traditional iterative operations.
2. Concise and Readable Code: Vectorized operations enable users to express complex operations concisely and in a more readable manner. With just a few lines of code, users can apply a single operation to entire arrays or columns, eliminating the need for verbose and error-prone loops.
3. Exponential Growth with Complexity: As the complexity of operations increases, vectorization becomes increasingly advantageous. With just a few lines of code, users can perform complex operations on multi-dimensional arrays or DataFrames, saving time and effort.
Best Practices for Vectorization:
To harness the full power of vectorization in Pandas, it is essential to follow a few best practices:
1. Utilize Built-in Functions: Pandas provides a wide range of vectorized functions that are specifically optimized for different data types and operations. Leveraging these functions can significantly enhance performance. Instead of writing custom loops, explore the vast array of built-in Pandas functions, such as `apply()`, `map()`, `transform()`, and `groupby()`, and choose the most appropriate one for your task.
2. Avoid Iteration and Conditional Statements: One of the key principles of vectorization is to avoid explicit iteration and conditional statements whenever possible. Instead, try to express operations using vectorized functions or logic. For example, instead of using a loop to iterate over rows of a DataFrame, leverage Pandas methods like `iterrows()` or `apply()` to process data in a vectorized manner.
3. Exploit DataFrame Methods: Pandas provides numerous DataFrame methods that support vectorization. These methods, including `add()`, `mul()`, `div()`, and `sub()`, operate on entire columns or rows of a DataFrame in a single step.
4. Use NumPy Functions: For operations that are not directly available in Pandas, consider using NumPy functions. NumPy seamlessly integrates with Pandas and is optimized for vectorized computation. By applying NumPy functions, such as `np.where()`, `np.vectorize()`, or `np.unique()`, users can efficiently perform complex operations on arrays or DataFrames.
FAQs:
Q1. Can I use vectorization on any type of operation?
Yes, vectorization is applicable to a wide range of operations, including arithmetic operations, conditional operations, string operations, and mathematical functions. However, not all operations can be vectorized. Some complex or custom operations may require iterative or conditional statements.
Q2. Does vectorization improve performance for small datasets?
Vectorized operations in Pandas typically provide the most significant benefits when working with large datasets. For small datasets, the performance gain may not be as pronounced. However, using vectorized operations consistently can still lead to more concise and readable code.
Q3. Are vectorized operations limited to numeric data?
No, Pandas vectorized operations are not limited to numeric data. Pandas provides various string and text manipulation functions that can be applied vectorizedly. These functions are optimized to efficiently process large arrays or columns of strings, enhancing performance.
Q4. Are there any downsides to vectorization?
While vectorization provides numerous advantages, it is important to note that poorly implemented vectorized operations can lead to unexpected results or performance issues. It is crucial to understand the underlying mechanics of vectorization and choose the appropriate functions and methods to avoid any pitfalls.
In conclusion, Pandas vectorization is a powerful tool that significantly improves the performance and readability of data operations in Python. By leveraging vectorized operations, users can process large datasets efficiently, write concise code, and focus on high-level data analysis without getting lost in low-level implementation details. Following best practices and utilizing built-in Pandas functions and NumPy libraries can further enhance the effectiveness of vectorization in Pandas. So, embrace the power of vectorization, and streamline your data manipulation workflow with Pandas!
Pandas Vectorized Regex
Understanding Regular Expressions:
Before diving into vectorized regex in Pandas, it is crucial to understand what regular expressions are. Regular expressions, commonly known as regex, are sequences of characters that define a search pattern. They are widely used for pattern matching and string manipulation in various programming languages. Regex patterns provide a concise and powerful way to identify and extract specific patterns from text data.
Introduction to Vectorized Regex in Pandas:
Pandas provides a vast set of functions that support vectorized regex operations on data columns. This means you can apply complex regex patterns to entire columns of data without having to iterate over individual elements. Not only does this simplify the code, but it also significantly improves performance, especially when dealing with large datasets.
Benefits of Using Vectorized Regex in Pandas:
Using vectorized regex in Pandas brings several advantages, including:
1. Improved Performance: Traditional row-by-row regex operations can be slow, especially on large datasets. Vectorized operations in Pandas leverage underlying optimized C-based implementations, resulting in dramatic speed improvements.
2. Simplified Code: Vectorized regex allows you to write concise code that operates on entire columns, eliminating the need for loops or list comprehensions. This improves code readability and maintainability.
3. Flexibility: With vectorized regex, you can easily define and apply complex patterns to extract, replace, or manipulate data in a flexible manner. This enables efficient data cleaning and transformation.
Usage of Vectorized Regex in Pandas:
Vectorized regex operations in Pandas can be performed using various functions, such as `str.extract()`, `str.contains()`, `str.replace()`, and more. Let’s cover some common use cases:
1. Extraction: The `str.extract()` function allows you to extract specific patterns from text data. For example, you can extract all email addresses from a column containing text using a regex pattern like `(\w+@\w+\.\w+)`. This operation returns a new column with extracted email addresses.
2. Filtering: The `str.contains()` function allows you to check if a particular pattern exists in a column. It returns a boolean mask that can be used to filter rows based on the presence of the pattern.
3. Replacement: The `str.replace()` function enables you to replace specific patterns within a text column. For instance, you can replace all occurrences of a specific word or phrase using a regex pattern such as `sub(r’\bword\b’, ‘new_word’, column_name)`.
4. Splitting: The `str.split()` function splits a text column into multiple columns based on a specified pattern. This is particularly useful when dealing with data that needs to be separated for further analysis.
5. Conditional Operations: You can combine vectorized regex operations with conditional logic for more complex tasks. For example, you can use `np.where()` along with regex to assign specific values to rows based on regex pattern matches.
FAQs:
Q1. Can I use vectorized regex on multiple columns simultaneously?
A1. Yes, Pandas allows you to apply vectorized regex operations on multiple columns simultaneously. You can pass a list of column names to the relevant function to perform regex operations on those columns.
Q2. Are vectorized regex operations case-sensitive?
A2. By default, vectorized regex operations in Pandas are case-sensitive. However, you can make them case-insensitive by using the `case` parameter in relevant functions and setting it to `False`.
Q3. Can I use vectorized regex to manipulate numeric data?
A3. Vectorized regex operations in Pandas are primarily designed for text data manipulation. While some functions can work with numeric data, regex is more commonly used for pattern matching and extraction in text-based columns.
Q4. Are there any limitations to using vectorized regex in Pandas?
A4. While vectorized regex in Pandas offers significant advantages, it is important to note that extremely complex regex patterns might lead to reduced performance. Additionally, regex patterns that require lookaheads or lookbehinds are not fully supported in vectorized regex operations.
Conclusion:
Pandas’ vectorized regex capabilities provide an efficient and flexible way to perform pattern matching and manipulation on data columns. It offers improved performance, simplified code, and greater flexibility compared to traditional row-by-row regex operations. By leveraging Pandas’ vectorized regex functions like `str.extract()`, `str.contains()`, and `str.replace()`, you can easily extract, filter, replace, or split data based on specific patterns. Despite a few limitations, vectorized regex in Pandas is a powerful tool for any data scientist or analyst working with textual data.
Np Vectorize
Introduction:
NumPy (Numerical Python) is a fundamental package for scientific computing in Python. It provides support for large, multi-dimensional arrays and matrices, as well as an extensive collection of mathematical functions to operate on these arrays efficiently. In this article, we will focus on a powerful function in NumPy called np.vectorize.
What is np.vectorize?
The np.vectorize function is a decorator that takes a scalar function as input and returns a new function that can accept arrays or other sequence-like objects as input arguments. This allows you to apply the scalar function to each element of the input arrays, resulting in element-wise operations. The main purpose of np.vectorize is to make it easier to write vectorized functions without having to use explicit loops.
Why use np.vectorize?
When dealing with large arrays or matrices, loops can be a performance bottleneck. Using vectorized operations provided by NumPy can significantly speed up calculations by leveraging highly optimized C or Fortran code implemented under the hood. np.vectorize acts as a convenient bridge between scalar functions and vectorized operations, allowing you to avoid explicit loops and take advantage of the performance benefits from NumPy.
How does np.vectorize work?
When np.vectorize is applied to a scalar function, it internally creates a new function that applies the scalar function to each element of the input arrays sequentially. The generated function uses NumPy broadcasting rules to handle different shaped inputs and ensures that the output matches the largest input size. In this way, vectorized functions can work on arrays of different shapes and sizes without the need for explicit broadcasting operations.
To achieve vectorization, np.vectorize iterates over the inputs, which implies some overhead compared to writing fully vectorized operations from scratch. However, it eliminates the need for explicit loops, leading to more concise and readable code. It is important to note that np.vectorize does not magically optimize your code to match the performance of fully vectorized functions, but it can offer a significant speedup over traditional looping approaches.
Syntax and Usage:
The usage of np.vectorize is straightforward. Let us consider an example of a simple scalar function that doubles a given value, called `double_func`. To vectorize this function, we can simply apply np.vectorize to it:
“`python
import numpy as np
def double_func(x):
return 2 * x
vectorized_func = np.vectorize(double_func)
“`
Now, the `vectorized_func` can be used to apply the `double_func` operation to input arrays:
“`python
array = np.array([1, 2, 3, 4, 5])
result = vectorized_func(array)
print(result)
# Output: [2 4 6 8 10]
“`
In this example, we applied the vectorized function to the input array `array`, which resulted in each element being doubled.
FAQs:
Q1. Can I vectorize any function using np.vectorize?
A1. np.vectorize can vectorize any scalar function; however, it may not always lead to performance improvements. For functions that are already vectorized or can be implemented natively using available NumPy functions, using np.vectorize might introduce unnecessary overhead.
Q2. Are there any limitations to using np.vectorize?
A2. np.vectorize is a convenient tool, but it is not suitable for all use cases. The main drawback is the overhead incurred when iterating over the inputs. For intensive calculations or operations that require more than one input array, it is recommended to write fully vectorized functions.
Q3. Can I use np.vectorize with functions that accept multiple arguments?
A3. Yes, np.vectorize can handle functions with multiple arguments. When calling the vectorized function, you can pass additional inputs as separate arguments:
“`python
import numpy as np
def multiply_func(x, y):
return x * y
vectorized_func = np.vectorize(multiply_func)
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
result = vectorized_func(array1, array2)
print(result)
# Output: [4 10 18]
“`
Q4. How do I check if a function is vectorized or not?
A4. You can use the `np.vectorize.excluded` attribute to determine if a function has been vectorized or not. The attribute will be `True` if the function has been excluded from vectorization due to unsupported features.
Conclusion:
In this article, we explored the np.vectorize function in NumPy, which allows us to easily apply a scalar function to each element of an input array. While it provides a convenient way to write vectorized operations, it does incur some iteration overhead. It is important to consider the complexity of the task at hand and carefully optimize the code using fully vectorized functions when necessary. np.vectorize is a valuable tool in the NumPy library that can help improve code readability and performance when used appropriately.
References:
– NumPy documentation: https://numpy.org/doc/
– NumPy vectorize function: https://numpy.org/doc/stable/reference/generated/numpy.vectorize.html
Images related to the topic pandas vectorized string operations
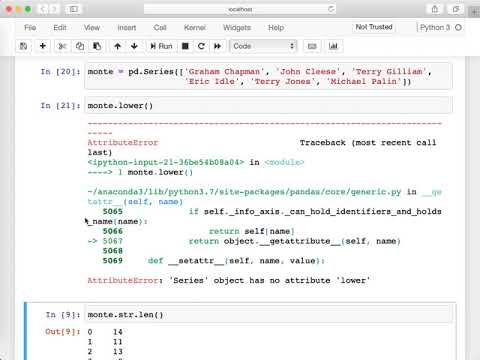
Found 47 images related to pandas vectorized string operations theme
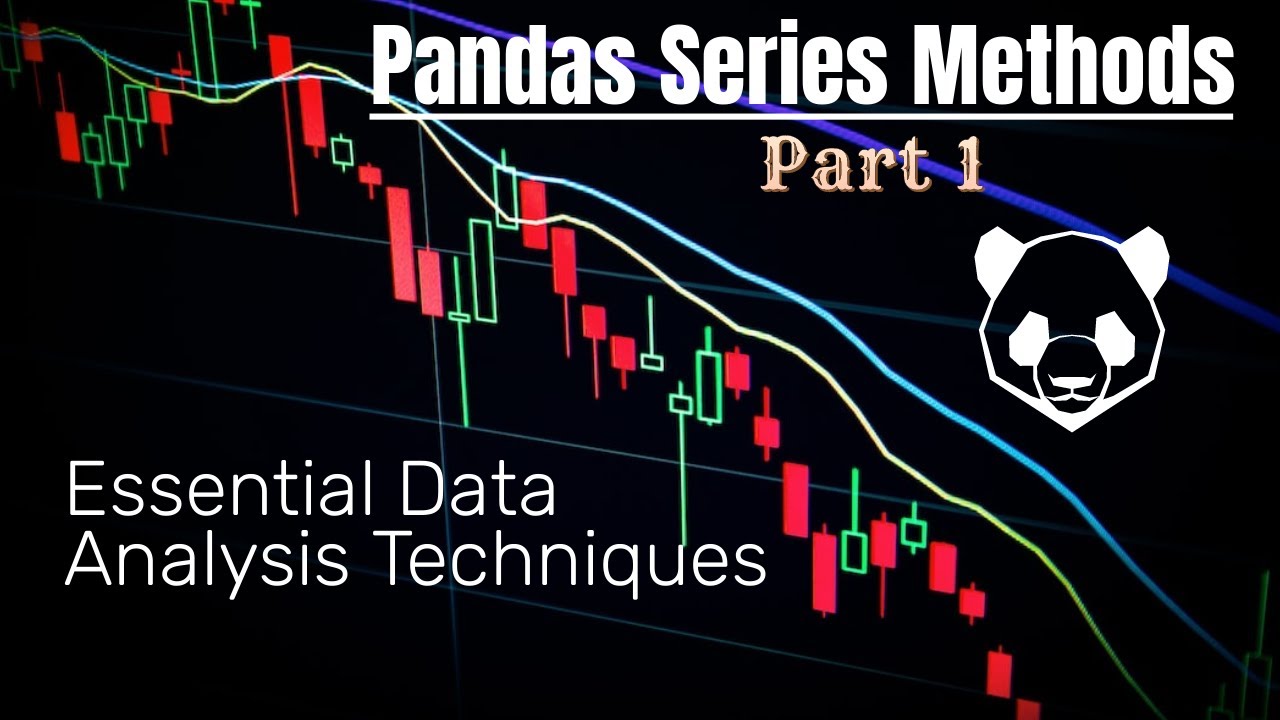
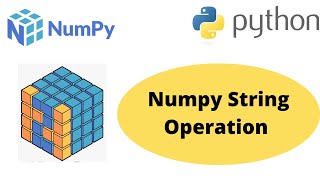
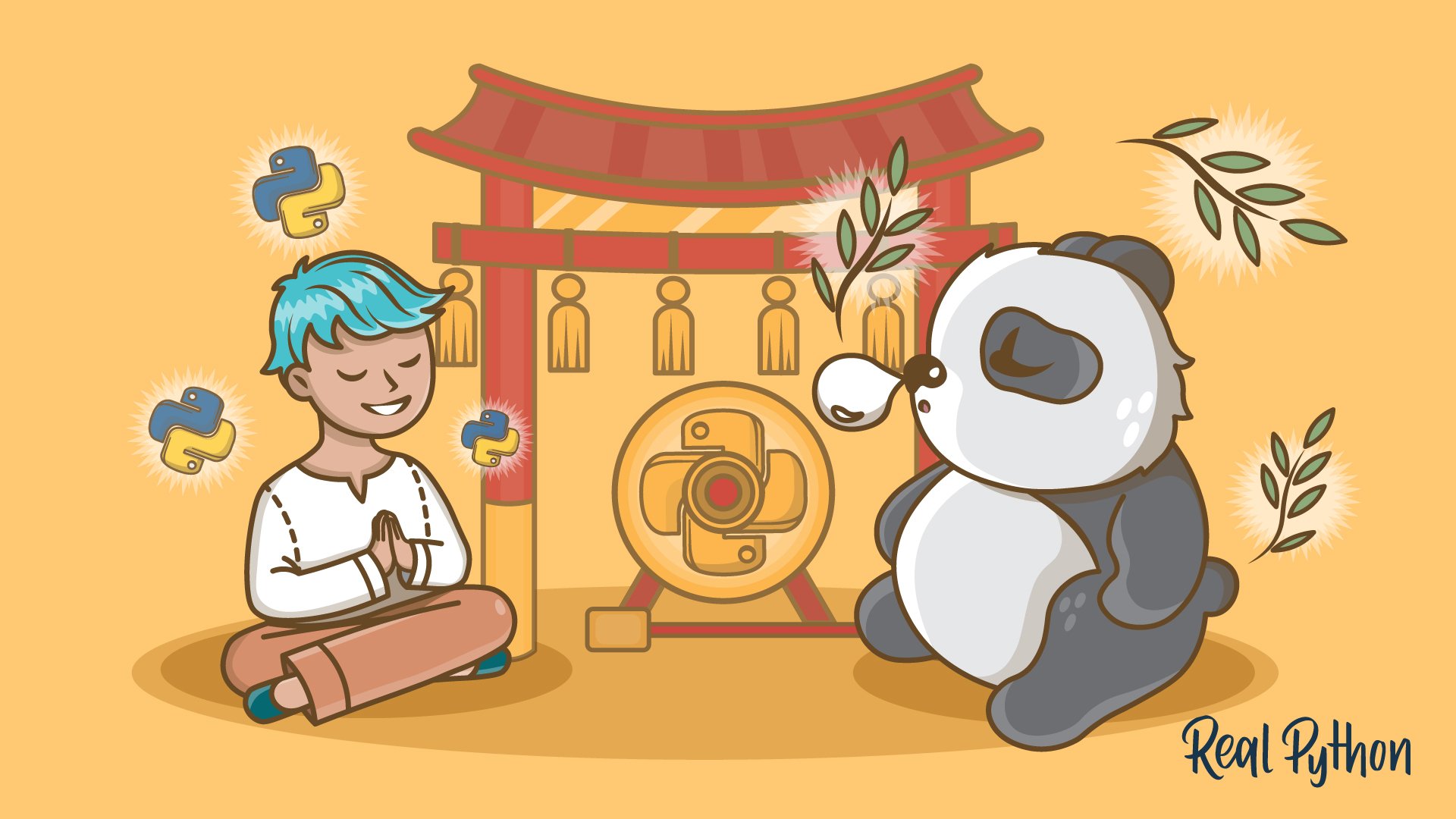
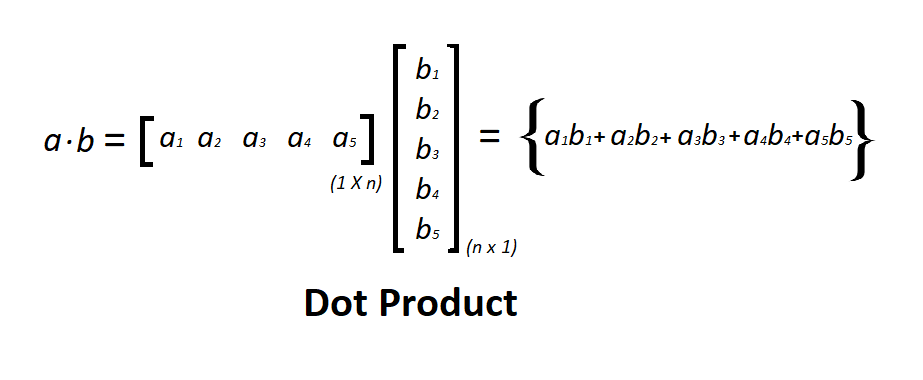
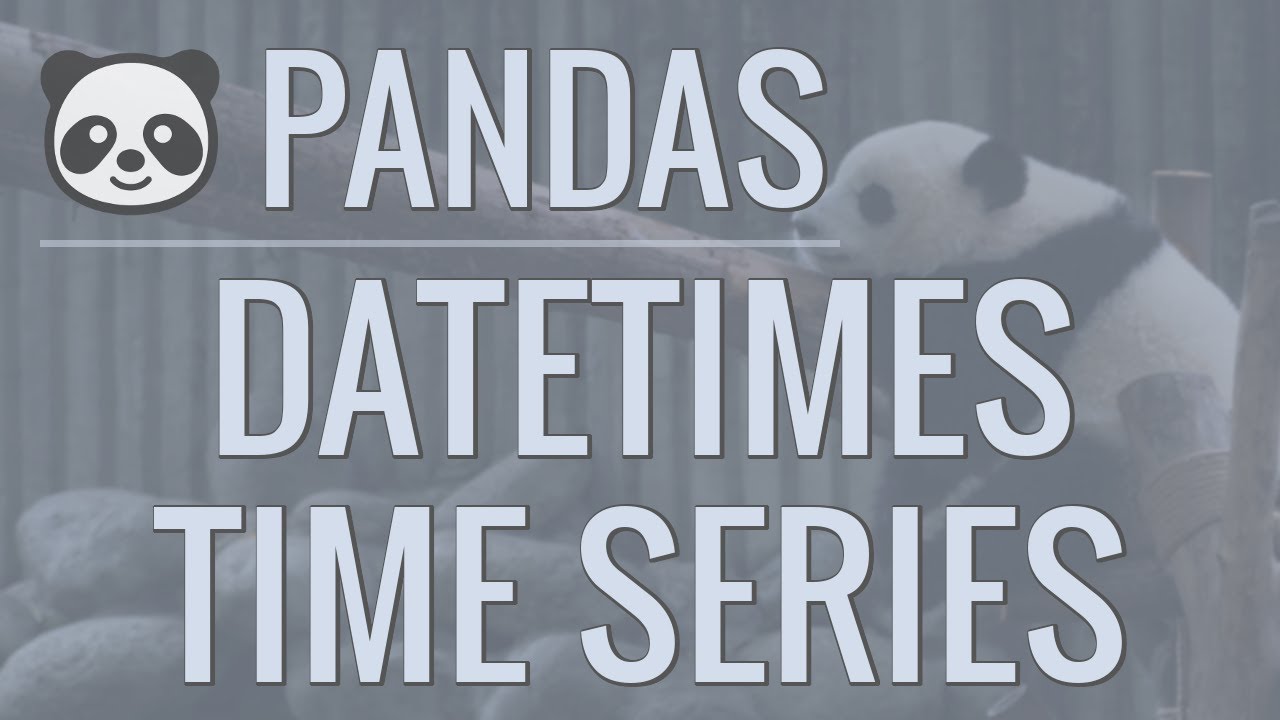
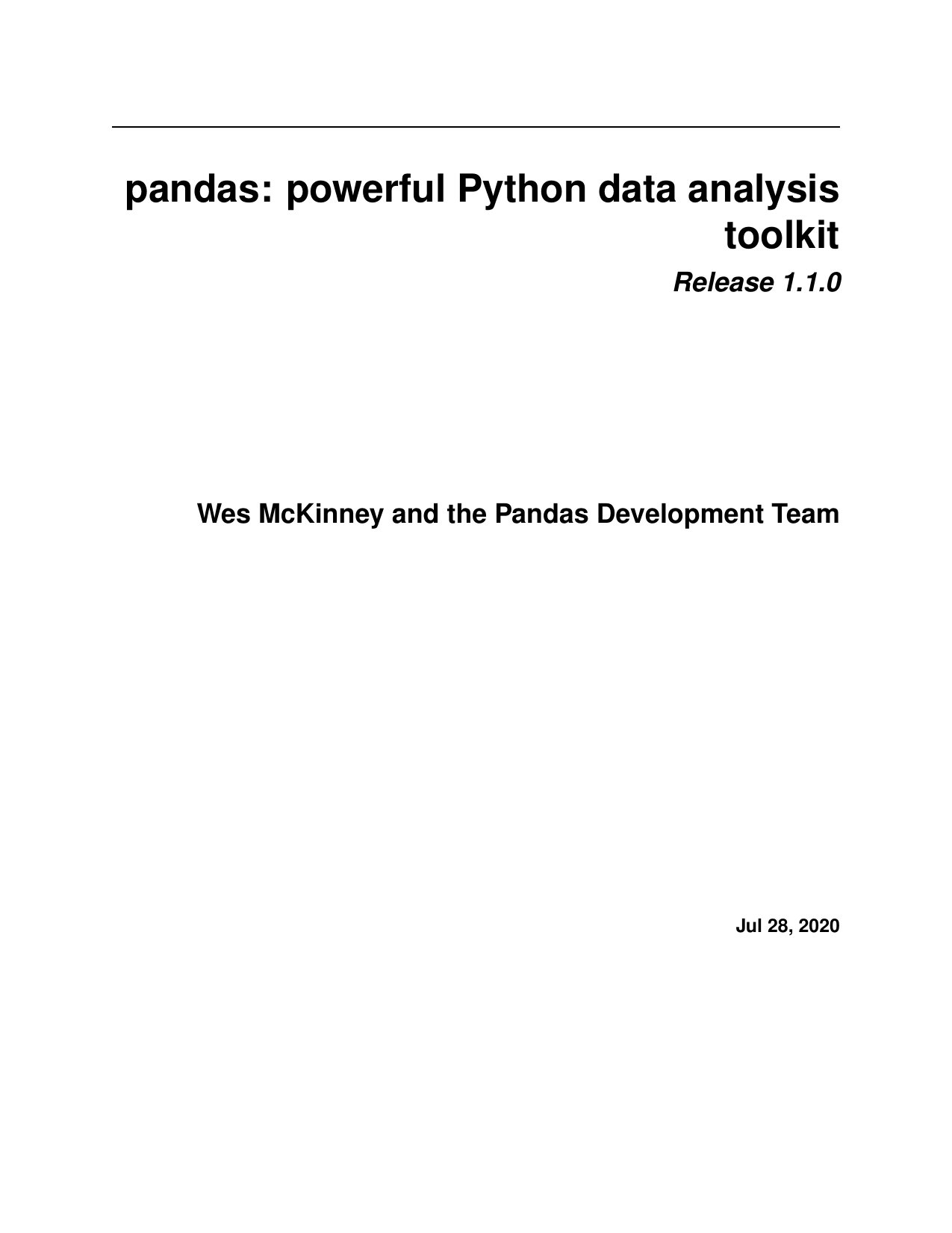
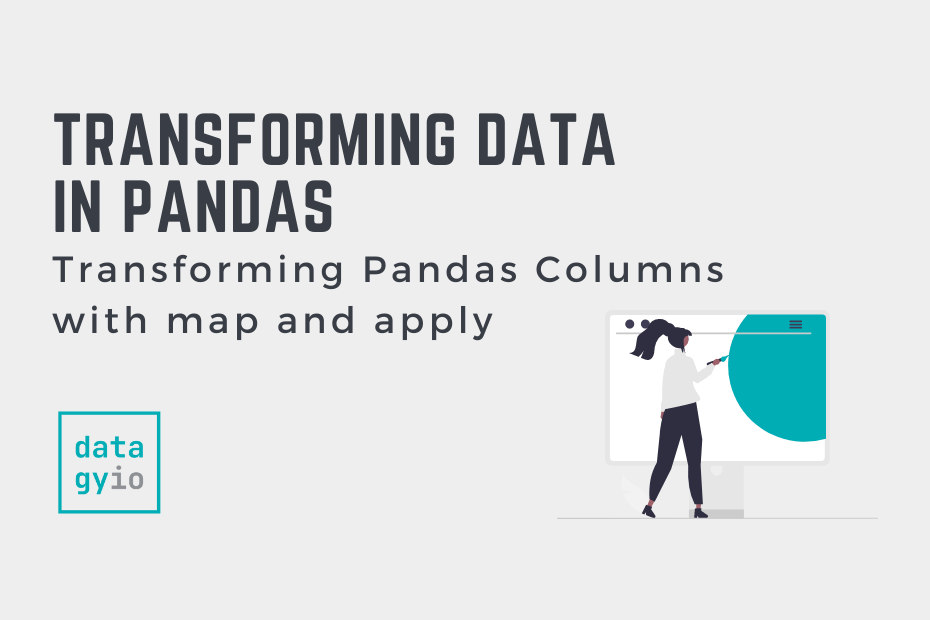
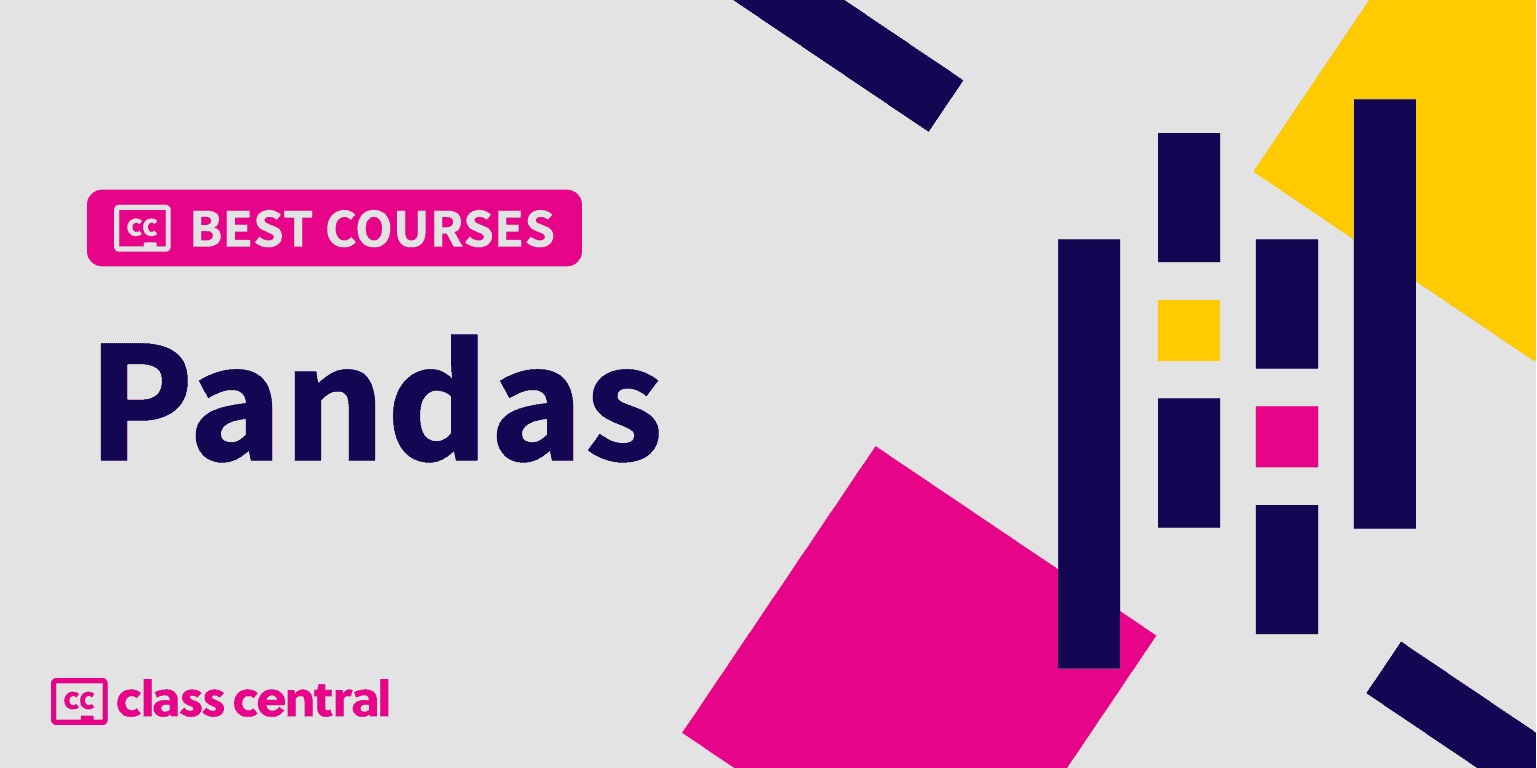
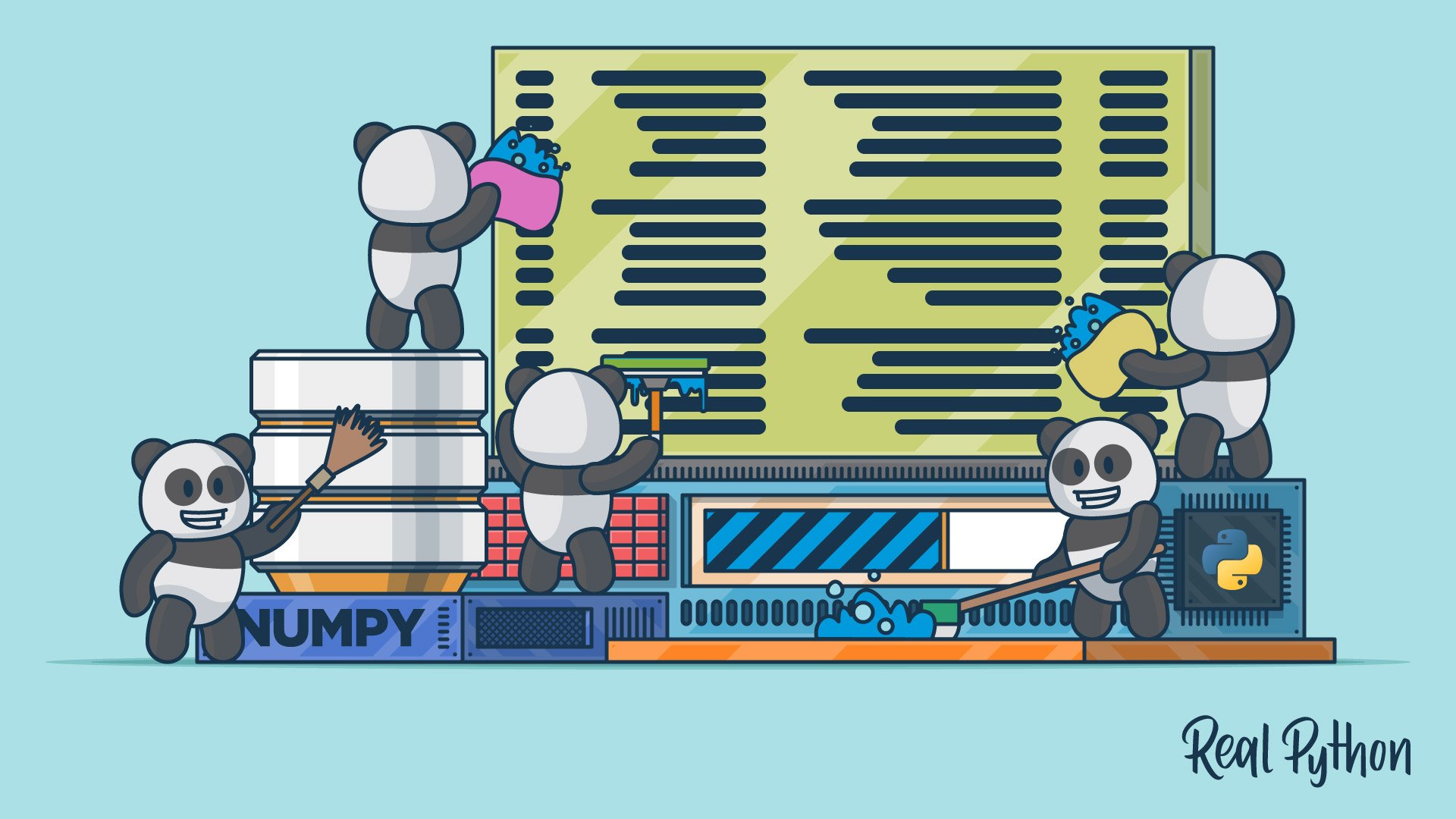
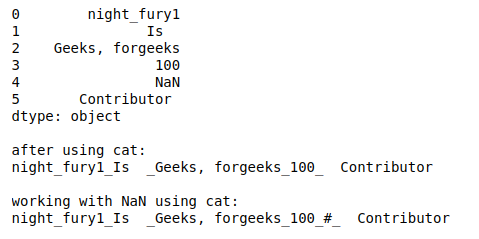
Article link: pandas vectorized string operations.
Learn more about the topic pandas vectorized string operations.
- Vectorized String Operations | Python Data Science Handbook
- Unlocking the Power of Vectorized String Methods in Pandas …
- How to speed up pandas string function? – Stack Overflow
- Vectorized String Operations | Python Data Science Handbook
- String Operations on Pandas DataFrame – Dev Genius
- Advanced String method in Pandas – Scaler Topics
- What is \n in Python? | How to Print New Lines | Meaning & More
- 22. Vectorized String Operations – Python Data Science …
- Working with text data — pandas 2.0.3 documentation
- String manipulations in Pandas DataFrame – GeeksforGeeks
- Using pandas vectorized string functions – Packt Subscription
- Working with string methods in pandas | Drawing from Data
- Faster string processing in Pandas – G Research
- Pandas String Operations
See more: nhanvietluanvan.com/luat-hoc