Nullable Object Must Have A Value
In programming, a nullable object refers to an object that can hold either a valid value or a null value. Unlike non-nullable objects, which must always have a value assigned to them, nullable objects provide flexibility by allowing null as a possible value. This means that the object may or may not have a value assigned to it, depending on the situation.
The Purpose and Use of Nullable Objects in Programming
Nullable objects are utilized in programming to handle scenarios where the absence of a value is a valid state. They are commonly used in situations where a value may not always be available, such as when retrieving data from a database or when dealing with user input. By allowing null as a possible value, nullable objects provide a solution to represent the absence of a value in a program.
Importance of Giving a Nullable Object a Value
Leaving a nullable object without a value can lead to potential issues and pitfalls in programming. When an uninitialized nullable object is accessed without checking for null, it can result in a null reference exception, which is a common error that occurs when an object reference is null and an operation is performed on it. These errors can lead to program crashes, unexpected behavior, and bugs in code.
Handling a Nullable Object Without a Value
To handle a nullable object without a value, it is important to follow certain techniques and best practices. One approach is to use conditional statements, such as if-else or null checks, to verify that the object has a value before performing any operations on it. This ensures that the code does not break or throw null reference exceptions.
Another recommended approach is to use the null coalescing operator, which provides a concise way to assign a default value to a nullable object if it is null. This operator helps avoid null reference exceptions and allows the program to continue execution smoothly.
Preventing Null Reference Exceptions
Null reference exceptions can have a significant impact on software development, causing delays, frustration, and potential security vulnerabilities. To avoid these exceptions, it is essential to ensure that a nullable object always has a value.
One way to prevent null reference exceptions is by using the null conditional operator, also known as the “safe navigation operator” or “?.” This operator allows for safe referencing of an object, as it checks if the object is null before performing any operations on it. This reduces the risk of errors caused by accessing null objects.
Additionally, implementing proper validation and error handling techniques can help catch any instances where a nullable object may not have a value assigned. By validating user input, database queries, or external data sources, developers can ensure that the program handles null values appropriately.
Ensuring the Validity of a Nullable Object
To ensure the integrity of a nullable object’s value throughout the code, a combination of techniques can be employed. One common practice is to validate the value of the object upon assignment or retrieval to ensure it meets the desired criteria. This can include checking for appropriate data types, range validation, or verifying the absence of null values.
Another technique is to document and enforce coding standards that require explicit handling of nullable objects in code reviews. This ensures that all developers are aware of the importance of properly handling nullable objects and helps maintain consistency in codebases.
Benefits of Giving a Nullable Object a Default Value
Assigning a default value to a nullable object can provide several benefits in programming. One advantage is that it ensures the object always has a value, eliminating the need for additional null checks throughout the code. This can simplify the logic and readability of the codebase.
Furthermore, by providing a default value, developers can avoid unexpected behavior or bugs that may occur when operations are performed on a null object. This can prevent errors and make the code more robust and reliable.
Scenarios where assigning a default value to a nullable object can be beneficial include handling optional parameters, initializing variables, or providing fallback values when retrieving data from external sources. By assigning a sensible default value, the code can gracefully handle situations where a value is not explicitly provided.
FAQs:
1. Nullable object must have a value là gì?
“Nullable object must have a value là gì” is the Vietnamese translation for the phrase “nullable object must have a value,” which refers to the requirement of assigning a value to a nullable object in programming.
2. How to handle a nullable object in C#?
In C#, nullable objects can be handled by using conditional statements, null checks, the null coalescing operator, or the null conditional operator. These techniques ensure that the object has a value before performing any operations on it, preventing null reference exceptions.
3. What is Nullable object must have a value in EF Core?
In EF Core (Entity Framework Core), “nullable object must have a value” refers to the requirement of assigning a value to a nullable object when using it in database operations. This ensures the integrity and validity of the data being stored or retrieved.
4. How to set a nullable property in C#?
To set a nullable property in C#, the value can be assigned using the null coalescing operator or by explicitly specifying the nullable value. For example, “int? myNullableProperty = null;” sets a nullable integer property to null.
5. Can a nullable value type be null?
Yes, a nullable value type can be null. Unlike non-nullable value types, nullable value types allow for null as a valid value. This is achieved by adding a question mark “?” after the value type, such as “int? myNullableValue = null;”
6. How to disable nullable in C#?
Nullable reference types were introduced in C# 8.0, allowing developers to enable or disable nullable reference types in their code. To disable nullable reference types, the “nullable” context option can be set to “warnings,” “none,” or “disable” in the project settings or using the “nullable” directive in source files.
7. What is Nullable in C#?
In C#, “Nullable” is a generic structure that allows value types to have null as a valid value. It is represented by the “Nullable
System.Invalidoperationexception: Nullable Object Must Have A Value
What Is A Nullable Object Must Have A Value?
In programming, a nullable object refers to an object that can hold a value of its underlying data type, as well as a null value. This concept is particularly useful in situations where it is necessary to represent missing or undefined data. By allowing objects to be nullable, developers can handle such scenarios more effectively and avoid potential errors. In this article, we will explore the concept of a nullable object in greater detail, discussing its significance, benefits, and how it can be employed in different programming languages.
Understanding Nullable Objects:
Traditionally, non-nullable objects are widely used in programming languages, wherein they are required to have a value at all times. This means that a non-nullable object cannot be left empty or assigned a null value. Consequently, if a non-nullable object does not have an assigned value, it will raise an error or throw an exception when accessed.
On the other hand, nullable objects relax this strictness by allowing a null value in addition to the underlying data type. This essentially means that a nullable object can either have a valid value or be null. By introducing this flexibility, nullable objects address situations where values are optional or unknown.
Benefits of Nullable Objects:
1. Avoiding Null Reference Exceptions: One of the most common errors in programming is the NullReferenceException. In non-nullable objects, if an attempt to access an object that hasn’t been assigned a value is made, it will result in this exception being thrown. However, with nullable objects, developers can proactively check if the object has a value or is null, reducing the occurrence of such exceptions.
2. Clear Representation of Missing Data: In certain scenarios, such as database queries or user input, data may not always be available. Instead of using default or placeholder values, nullable objects provide a more accurate representation of missing data. This ensures that developers can distinguish between intentional absence and a legitimate value.
3. Enhanced Compatibility: Nullable objects enable better compatibility between different programming constructs. For example, when using a collection that can contain null values, such as a List, nullable objects collapse neatly into the collection, harmonizing with the non-nullable aspects of it. This makes it easier to manage data sets that include both nullable and non-nullable elements.
Implementation across Programming Languages:
The implementation of nullable objects may vary across programming languages. Let’s take a look at how some popular languages handle them:
1. C#:
C# introduced native nullable reference types in C# 8.0, allowing developers to define non-nullable types by default while explicitly specifying nullable types using the “?” modifier. This feature encourages safer coding practices and provides compile-time checks to prevent null reference exceptions.
2. Java:
Although Java does not have built-in support for nullable objects, libraries like @Nullable and @NonNullByDefault annotations provided by third-party tools, such as JetBrains’ IntelliJ IDEA, help to achieve similar functionality. These annotations aid in expressing intention and generating warnings or errors during compilation based on nullability annotations.
3. TypeScript:
TypeScript, a superset of JavaScript, natively supports nullable types. By utilizing the “?” operator after a type declaration, variables can be designated as nullable. TypeScript compiler further ensures static analysis, detecting potential null-related errors before runtime.
4. Kotlin:
Kotlin, a language developed by JetBrains, promotes null safety by design. It provides nullable types using the “?” operator while denoting non-nullable types by default. This design philosophy relegates null pointer exceptions to compile-time checks, leading to safer programming.
FAQs:
Q1. Can non-nullable objects be converted to nullable objects and vice versa?
A1. In some programming languages, there are provisions to convert between nullable and non-nullable types. However, when converting from a non-nullable to a nullable object, it is vital to handle potential null values to avoid errors.
Q2. Are nullable objects more memory-intensive?
A2. Nullable objects do not consume additional memory compared to their non-nullable counterparts. Instead, they use the same memory space, with a null value indicating the absence of a assigned value.
Q3. Are nullable objects suitable for all scenarios?
A3. Nullable objects are not suitable for all scenarios. While they help manage optional or unknown data better, their usage should be carefully considered. Overuse of nullable objects can complicate code, reduce clarity, and potentially introduce null reference exceptions if not handled properly.
Q4. Do all programming languages support nullable objects?
A4. No, not all programming languages natively support nullable objects. However, many languages have adopted features or built-in mechanisms to accommodate nullable objects using annotations, third-party libraries, or language-specific constructs.
Conclusion:
Nullable objects play a vital role in programming languages, enabling better handling of optional or undefined data. By being able to represent the absence of a value, nullable objects support more accurate data modeling and help improve code robustness. While their usage must be mindful not to introduce unnecessary complexity, they provide valuable tools for developers to write safer and more effective code. So, embrace the power of nullable objects and unravel the true potential of your programming language.
What Is A Nullable Object?
To understand nullable objects, it is essential to grasp the concept of a null value. In programming, a null value typically indicates the absence of a value or an uninitialized variable. For instance, consider a variable that should represent a person’s age. If that variable is not assigned a specific value, it is considered null until assigned a valid age. In many programming languages, attempting to use a null value without proper handling can lead to errors or unexpected behavior during runtime.
Nullable objects aim to solve this issue by providing a way to declare variables that allow null values. They provide a built-in mechanism to indicate when a variable may not have a valid value. By explicitly allowing null values, developers can differentiate between a variable that hasn’t been assigned a value yet and a variable that represents the absence of a value.
In languages such as C#, Java, and Kotlin, nullable objects are created by appending a question mark (?) after the type declaration. For example, to declare a nullable integer variable in C#, one would write “int? age = null.” Similarly, in Java, it would be “Integer age = null.” The question mark operator signals that the variable can hold a null value.
When working with nullable objects, it is crucial to consider proper null handling to avoid potential runtime exceptions. Developers typically use various techniques to ensure the safe and effective use of nullable objects. For instance, null checking and handling via conditional statements are commonly employed. These checks allow developers to decide how to proceed based on whether a nullable variable is null or contains a valid value.
One popular approach is the use of the null coalescing operator, often denoted by two question marks (??). It provides a concise way to assign a default value when a nullable object is null. For example, given a nullable integer variable “age,” we can assign a default value of 18 to it using the expression “int actualAge = age ?? 18.” If “age” is null, “actualAge” will be assigned 18; otherwise, it will be assigned the value of “age.”
Another useful feature related to nullable objects is the ability to perform null-safe operations via the safe-navigation operator (?.). This operator allows developers to invoke methods, access properties, or retrieve values from a nullable object only if it is not null. If the object is null, the operation is simply skipped, and the result is automatically set to null. This feature helps prevent null-reference errors and improves the robustness of code that deals with nullable objects.
Nullable objects have proven beneficial in many scenarios. They can be helpful when interacting with databases, where certain fields might not have a value in all records. With nullable objects, developers can clearly represent such scenarios and handle them appropriately in their code.
Additionally, nullable objects enable more explicit type declarations, providing clearer intentions in the code. When a variable is declared as nullable, it signals to other developers that the absence of a value is a valid state for that variable.
Frequently Asked Questions:
Q: Can any type be nullable?
A: In most programming languages that support nullable objects, any type can be made nullable, including reference types (i.e., classes) and value types (i.e., structs or primitive types).
Q: Are nullable objects only used when working with databases?
A: No, nullable objects can be used in various scenarios where the absence of a value is a valid state for a variable. Database interactions are one of the common use cases, but they are not limited to that.
Q: Does using nullable objects increase the complexity of code?
A: While nullable objects introduce an additional aspect to consider, proper null handling techniques and understanding can mitigate complexity. By employing null-checking and null-safe operators, the code can remain concise and robust.
Q: Are nullable objects available in all programming languages?
A: No, the presence and implementation of nullable objects vary between programming languages. Languages like C#, Java, Kotlin, and Swift offer built-in support for nullable objects, while others may have alternative approaches or lack direct support.
Q: Are nullable objects equivalent to using null as a default value for variables?
A: No, null as a default value implies that a variable starts as null and can hold null or valid values. On the other hand, nullable objects explicitly indicate that the variable can hold a null value, distinguishing it from variables that should never have null values.
In conclusion, nullable objects tackle the challenge of representing null values in programming languages. They provide a dedicated syntax to differentiate between variables that hold valid values and those that represent the absence of a value. When used properly, nullable objects enhance code clarity and, with null-safe techniques, can significantly reduce errors related to null reference.
Keywords searched by users: nullable object must have a value Nullable object must have a value là gì, Nullable object must have a value C#, Nullable object must have a value EF Core, Set nullable property C#, Nullable value type may be null, Disable nullable c#, Nullable C#, Nullable enable nullable
Categories: Top 27 Nullable Object Must Have A Value
See more here: nhanvietluanvan.com
Nullable Object Must Have A Value Là Gì
Nullable objects exist to address scenarios where the lack of a value can have significance. For example, consider a variable that represents a customer’s age. It is possible that some customers may not have provided their age, or they may have intentionally left it blank. By using a nullable object, developers can handle these situations without resorting to workarounds or assigning default values.
In C#, nullable objects are represented using the “?” symbol after the data type. For instance, “int?” represents a nullable integer. By default, value types in C# cannot be null, but nullable types provide the flexibility to assign null to value types without causing compilation errors.
The importance of nullable objects lies in their ability to prevent null reference exceptions, which occur when a programmer tries to access or operate on an object that is null. By explicitly defining objects as nullable, programmers can avoid runtime errors that may crash an application or yield unexpected behavior.
Nullable objects are particularly useful in database transactions. Whenever a field in a database table allows null values, the corresponding variable in code should also be nullable. This allows developers to accurately handle situations where database fields contain no data, preventing potential errors when trying to access or manipulate such fields.
Several programming languages, such as Java and Swift, also support nullable objects. In Java, developers create nullable objects by appending “?”, while in Swift, the concept of nullable objects is ingrained into the language, and all variables can be nullable. These languages enable programmers to handle nullable objects with ease and ensure a high level of reliability in their code.
To better comprehend the concept of nullable objects, let’s address some frequently asked questions about them:
Q: When should I use nullable objects?
A: Nullable objects should be used when there is a possibility of a variable or field not having a value. If there is a chance that a value may be null, using a nullable object is recommended to avoid null reference exceptions.
Q: How do I assign a value to a nullable object?
A: Nullable objects can be assigned a value using the “=” operator, just like regular objects. However, they can also be assigned a null value explicitly by using the “null” keyword.
Q: Can I perform operations on nullable objects without null reference exceptions?
A: Yes, you can perform operations on nullable objects without encountering null reference exceptions by using conditional statements to check if the object has a value before accessing or manipulating it. This can be done with the help of the null-conditional operator (?.) in C# or similar constructs in other programming languages.
Q: What happens if I try to access a nullable object’s value without checking if it has a value?
A: If you try to access a nullable object’s value without checking its value beforehand and the object is null, a null reference exception will occur, causing your program to crash or exhibit unexpected behavior.
Q: How can I handle a nullable object that has a null value?
A: Several strategies can be employed to handle nullable objects with null values. You can provide a default value, throw an exception, or create conditional logic to handle null values gracefully.
Q: Are nullable objects less efficient than regular objects?
A: Nullable objects can introduce a small performance overhead due to additional checks required to determine if the object has a value. However, the impact is typically negligible, and the benefits of preventing null reference exceptions outweigh the minimal performance impact.
In conclusion, nullable objects provide a convenient and reliable way to handle variables or data types that may or may not have a value. By allowing null values, programmers can more effectively handle scenarios where lack of data is a valid state. Understanding how to work with nullable objects and incorporating them into your programming practice enhances the robustness and reliability of your code, preventing runtime errors and unexpected behavior.
Nullable Object Must Have A Value C#
In C#, a nullable object is represented by adding a question mark ‘?’ after the object’s data type. For example, instead of declaring an integer variable like this: int myNumber = 10, you can declare it as a nullable integer like this: int? myNumber = 10. This syntax allows the variable to contain either a valid value or a null value, meaning it has no value at all.
The main advantage of using nullable objects is that it allows developers to handle scenarios where a value can be absent or unknown. By explicitly marking a variable as nullable, it becomes clear in the code that it can potentially be null, thus reducing the chances of errors caused by incorrect assumptions about its value.
A common use case for nullable objects is when dealing with database records. Databases often contain columns that can be nullable, representing optional fields. In C#, nullable objects can be used to map these nullable database columns to variables in the code. This enables developers to handle null database values gracefully and perform appropriate actions when necessary.
However, it’s important to note that nullable objects must be handled with caution. Since accessing a null value can lead to runtime errors, developers need to ensure that they always check for null before using the value. This can be done using the null-conditional operator ‘?.’ in C#. The null-conditional operator allows you to safely access a property or call a method on a nullable object by automatically checking if the object is null before performing the operation.
For example, consider the following code snippet:
int? myNumber = null;
int squaredNumber = myNumber?.Value * myNumber?.Value;
In this code, we declare a nullable integer variable myNumber and set it to null. We then attempt to square the value of myNumber using the null-conditional operator. Since myNumber is null, the expression myNumber?.Value returns null, and the calculation is not performed. As a result, squaredNumber would also be null.
When working with nullable objects, it’s essential to understand the potential pitfalls. One common mistake is assuming that a nullable object always has a value, leading to null reference exceptions at runtime. It’s crucial to always check for null before accessing the value to avoid such errors.
Developers can check for null using an if statement or the null-coalescing operator ‘??’. The null-coalescing operator provides a concise way to assign a default value if the nullable object is null. For example:
int? myNumber = null;
int actualNumber = myNumber ?? 0;
In this case, if myNumber is null, actualNumber will be assigned the value 0. Otherwise, it will be assigned the actual value of myNumber.
In addition to the null coalescing operator, C# provides several other approaches to handle nullable objects effectively. These include the use of the HasValue property, the Value property, and the GetValueOrDefault() method.
– The HasValue property returns a boolean indicating whether the nullable object has a value or is null.
– The Value property retrieves the underlying value of the nullable object. However, if the nullable object is null, accessing the Value property will result in a null reference exception.
– The GetValueOrDefault() method returns the underlying value if the nullable object has a value, or the default value for that data type if it is null.
Now, let’s address some frequently asked questions about nullable objects in C#:
Q: Can I use nullable objects with any data type in C#?
A: Yes, nullable objects can be used with any value type or reference type in C#. All you need to do is add a question mark ‘?’ after the object’s data type to make it nullable.
Q: What happens if I try to assign a null value to a non-nullable variable?
A: If you try to assign a null value to a non-nullable variable, the compiler will generate a compile-time error. Non-nullable variables cannot have a null value.
Q: Can I use nullable objects in collections such as lists or arrays?
A: Yes, nullable objects can be used in collections. However, it’s important to remember that nullable objects take up more memory than their non-nullable counterparts. So, if you have a large collection, it’s worth considering the memory implications of using nullable objects.
Q: Are there any performance implications of using nullable objects?
A: Using nullable objects does come with a small performance overhead. The null checks and operations performed on nullable objects can add a small amount of computational cost. However, in most cases, the performance impact is negligible unless you’re working with a large number of nullable objects in performance-critical code.
In conclusion, nullable objects in C# provide a powerful mechanism to represent the absence of a value. By using nullable objects, developers can ensure that their code handles scenarios where a value can be unknown or not applicable. However, proper handling of nullable objects is crucial to prevent null reference exceptions and other runtime errors. Always remember to use null checks and the available operators and methods to handle nullable objects effectively and avoid potential issues.
Images related to the topic nullable object must have a value
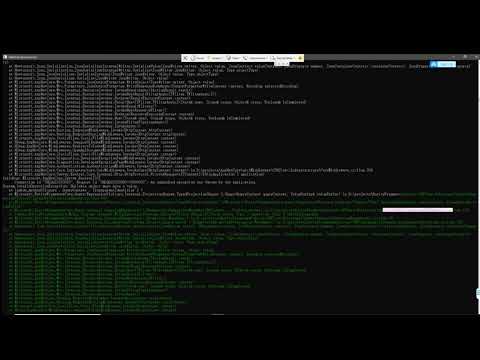
Found 24 images related to nullable object must have a value theme


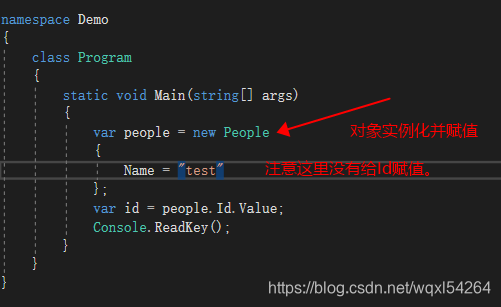

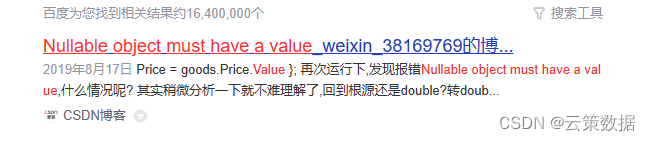
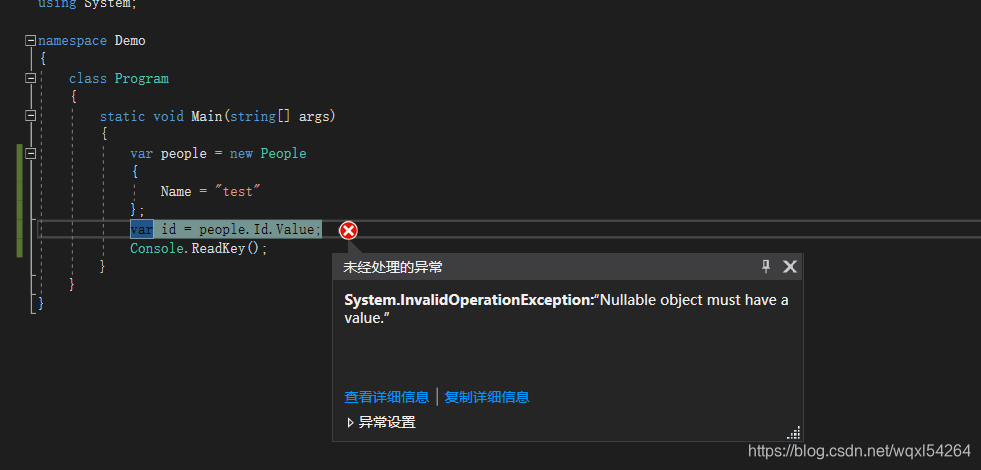
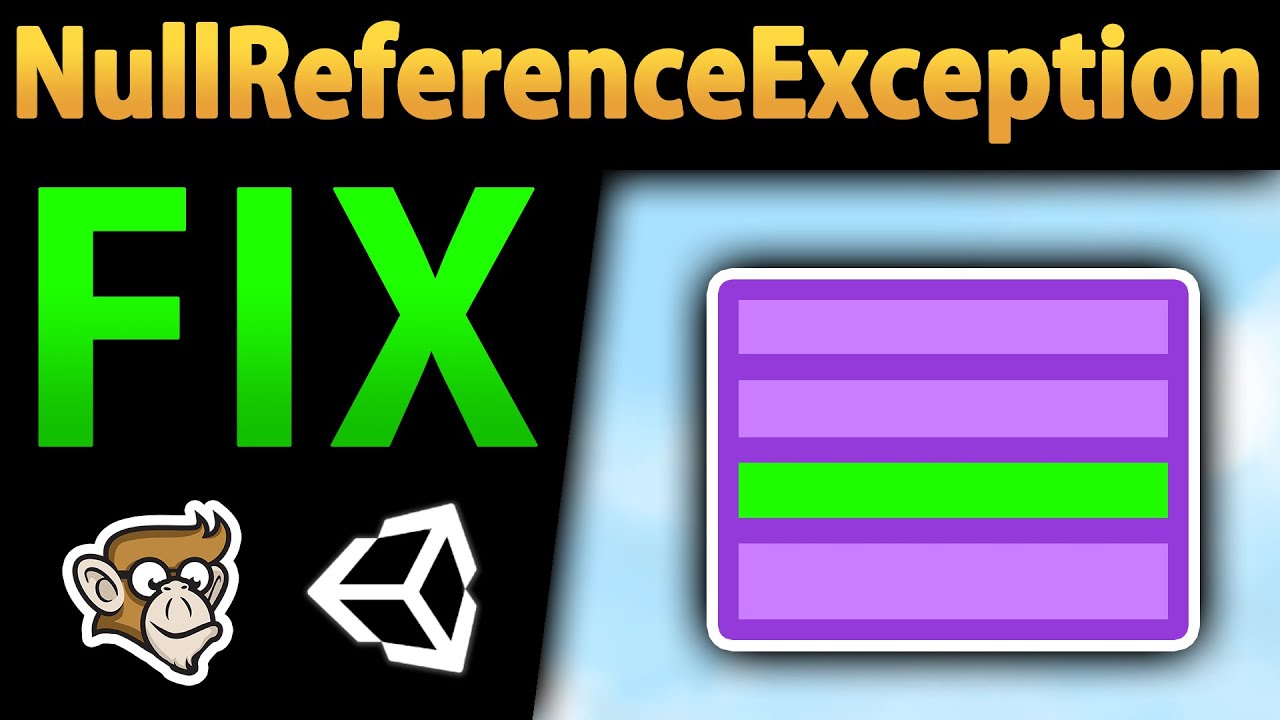
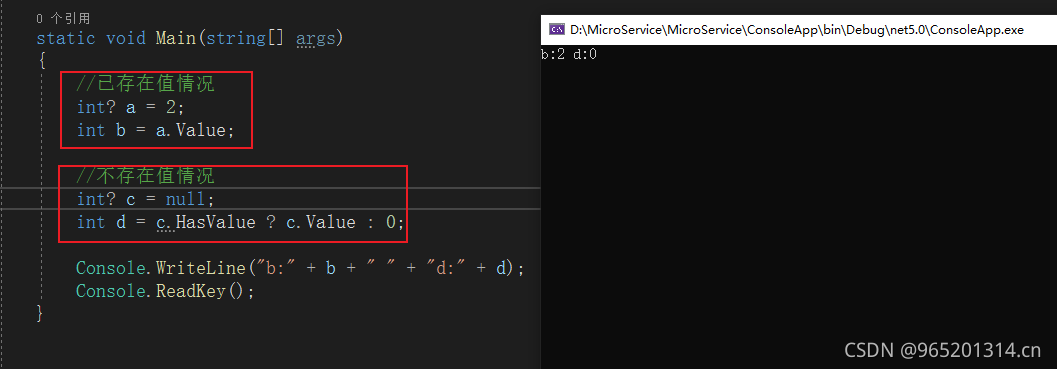






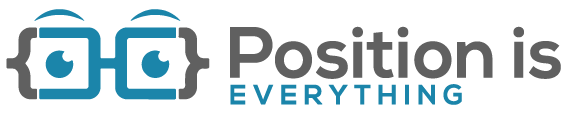
![C#] Null Reference & Reference Types in C# | Plus Nullable Data Types & The NullReferenceException - YouTube C#] Null Reference & Reference Types In C# | Plus Nullable Data Types & The Nullreferenceexception - Youtube](https://i.ytimg.com/vi/Eg0FMAnMiT0/maxresdefault.jpg)


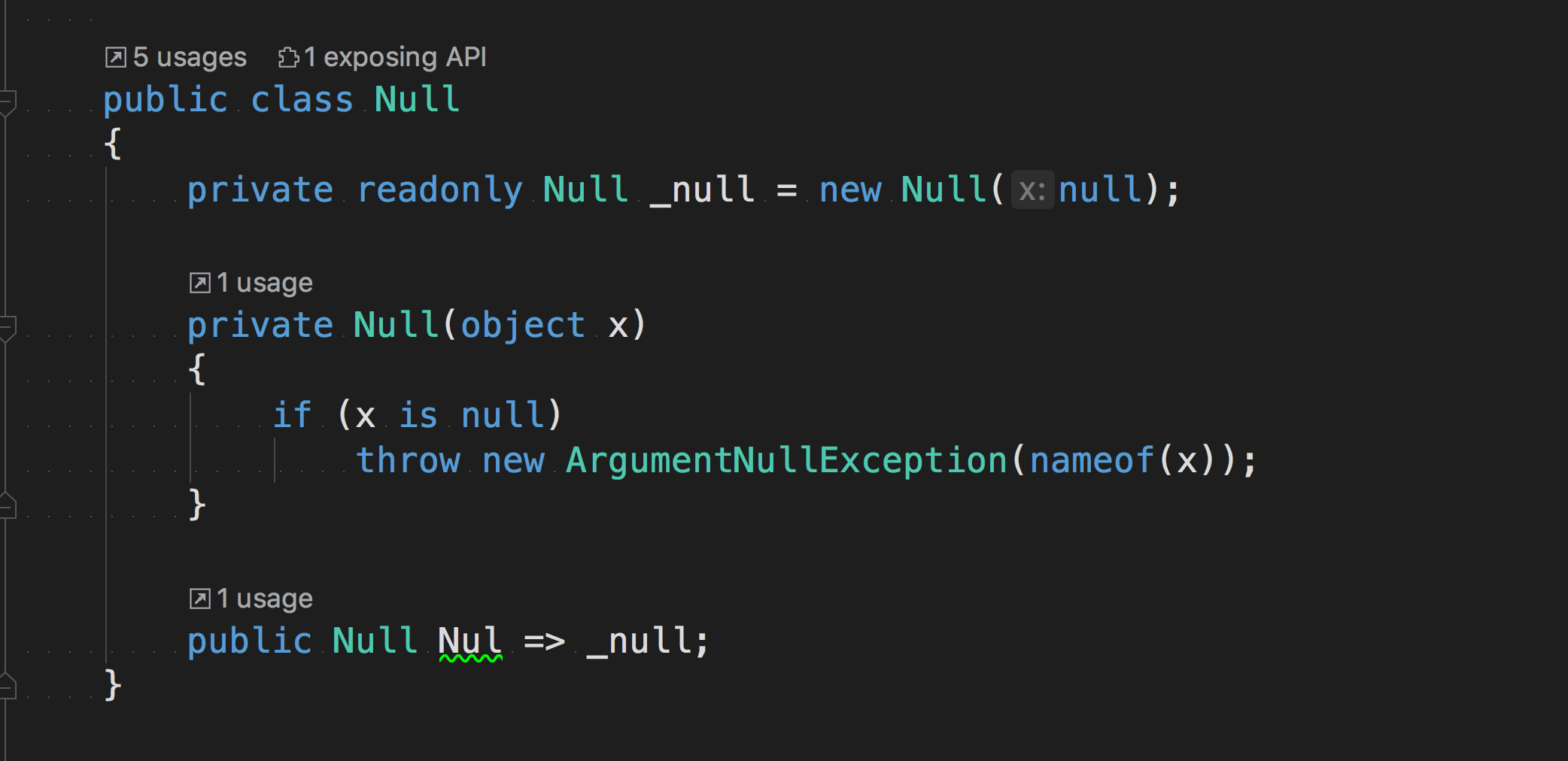
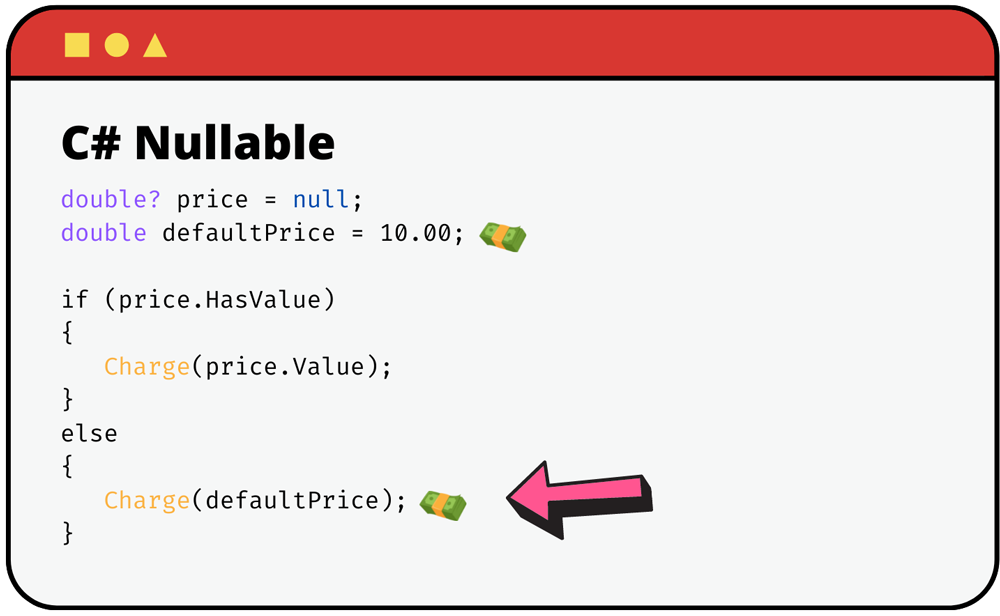


Article link: nullable object must have a value.
Learn more about the topic nullable object must have a value.
- nullable object must have a value – Stack Overflow
- Nullable Object Must Have a Value: Causes and Solutions
- Null object pattern – Wikipedia
- Nullable value types – C# reference – Microsoft Learn
- How to Work with Nullable Types in C# | LoginRadius Blog
- Nullable Object Must Have a Value: Causes and Solutions
- [Solved] Nullable object must have a value – CodeProject
- Nullable object must have a value – Microsoft Learn
- How to troubleshoot ‘Nullable object must have a value’ error?
- Nullable object must have a value in c# – C# Corner
- Getting a “Nullable object must have a value” When Disabling …
- Why I get this “Nullable object must have a value” error in C# …
See more: nhanvietluanvan.com/luat-hoc