Nodejs Create Csv File
Benefits of Creating CSV Files with Node.js
CSV (Comma-Separated Values) is a common file format used to store tabular data. It is widely supported by various applications such as spreadsheets, databases, and data analysis tools. By using Node.js to create CSV files, you can leverage the following benefits:
1. Simplified Development: Node.js offers a vast array of modules and libraries to simplify CSV file creation. These modules provide functionalities for reading, writing, parsing, and manipulating CSV data.
2. Scalability: Node.js is known for its ability to handle large numbers of concurrent requests efficiently. This makes it ideal for applications that require processing and generating large CSV files quickly.
3. Cross-platform Compatibility: Node.js is platform-independent, enabling you to create CSV files that can be easily accessed and used across different operating systems.
4. Flexibility in Data Manipulation: Node.js allows you to easily manipulate and format data before writing it to a CSV file. This flexibility is essential when dealing with complex data structures or when performing data transformations.
5. Integration with Other Technologies: Node.js seamlessly integrates with other technologies, such as databases and web frameworks, enabling you to fetch data from various sources and export it to CSV files effortlessly.
Common Use Cases for Creating CSV Files with Node.js
Creating CSV files with Node.js can be useful in a variety of scenarios. Some common use cases include:
1. Data Export: Exporting data from databases, data warehouses, or other data sources to CSV files for further analysis or sharing with external stakeholders.
2. Data Visualization: Generating CSV files for visualization tools, like spreadsheets or charting libraries, to create reports, graphs, or dashboards.
3. Data Transformation: Formatting and transforming data before exporting it to CSV files. This can involve data cleansing, merging, filtering, or converting data types to meet specific requirements.
4. Data Migration: Converting data from one format to another, such as exporting data from a database into CSV files for migration purposes.
5. Data Integration: Combining data from multiple sources into a single CSV file for easy integration with other systems or data analysis tools.
Installing the Required Modules for Creating CSV Files with Node.js
To create CSV files with Node.js, you’ll need to install a suitable module. Some popular modules for CSV file creation in Node.js include:
1. node-csv: A lightweight module that provides functionalities to read, write, and parse CSV files. It supports various customization options and is easy to use.
2. csv-parse: A powerful module for parsing CSV data. It can handle complex CSV structures and provides options for flexible data manipulation.
3. csv-stringify: A module that allows you to convert JavaScript objects or arrays into CSV-formatted strings. It supports customizable separators, quote characters, and other formatting options.
4. fast-csv: A high-performance CSV parser and formatter that offers features like data streaming, automatic type conversion, and error handling.
You can install these modules using npm, the package manager for Node.js. Open your command-line interface and run the following command to install a module:
“`shell
npm install module_name
“`
Replace “module_name” with the name of the desired module (e.g., “node-csv”, “csv-parse”, “csv-stringify”, or “fast-csv”).
Reading and Writing CSV Files with Node.js
Once you have installed the required module, you can start reading and writing CSV files with Node.js. Here’s a basic example using the `node-csv` module:
“`javascript
const csv = require(‘csv’);
// Create new CSV file
const writer = csv.writer();
writer.pipe(fs.createWriteStream(‘data.csv’)); // Specify the file path
// Write data rows
writer.write([‘Name’, ‘Age’]);
writer.write([‘John Doe’, 30]);
writer.write([‘Jane Smith’, 25]);
writer.end();
console.log(‘CSV file created successfully!’);
“`
In the above example, we first import the `csv` module and create a writer object using `csv.writer()`. We then pipe the writer to a writable stream, which in this case is a file stream created using `fs.createWriteStream()`. By calling the `write()` method on the writer object, we can add rows of data to the CSV file. Finally, we call `end()` to signal the end of writing and close the file stream.
To read data from an existing CSV file, you can use the following example:
“`javascript
const csv = require(‘csv’);
// Read CSV file
csv.parse(fs.readFileSync(‘data.csv’), (error, data) => {
if (error) {
console.error(‘Failed to read the CSV file:’, error);
return;
}
console.log(‘CSV data:’, data);
});
“`
In this example, we use the `csv.parse()` function provided by the module to parse the CSV data in the file. We provide the file content using `fs.readFileSync()` and handle the parsed data within the callback function.
Validating and Formatting CSV Data in Node.js
Before writing data to a CSV file, it’s essential to validate and format it correctly. Node.js provides various methods and libraries to help with this task.
To validate CSV data, you can check the values against specific rules, such as data types or length constraints. For more complex validations, you can use libraries like `csv-parse` or `fast-csv`, which provide options for custom validation logic and error handling.
For formatting CSV data, you can use the `csv-stringify` module or manually manipulate the data before writing. Formatting options include custom separators, quoting characters, or escaping rules. You can also modify specific values or convert data types, ensuring the CSV file adheres to your desired format.
Handling Large Data Sets in CSV Files with Node.js
When working with large data sets, it’s crucial to consider performance and memory usage. Node.js provides several techniques to handle large CSV files efficiently:
1. Streaming: Rather than loading the entire CSV file into memory, streaming allows you to process the data in smaller chunks. Modules like `fast-csv` provide streaming capabilities, allowing you to read and write data from and to CSV files without loading the entire file at once.
2. Database Integration: If the data is stored in a database, you can fetch and write data in batches, using pagination or limit-offset techniques. This process reduces memory consumption and enhances overall performance.
3. Data Processing in Chunks: Processing large CSV files in smaller chunks can prevent memory overflow. You can read and process a specific number of rows, write them to the output file, and repeat until all data has been processed.
Implementing Advanced Features for CSV Files in Node.js
Node.js offers advanced features to enhance your CSV file creation processes. Some of these features include:
1. Asynchronous Processing: By utilizing the asynchronous nature of Node.js, you can perform multiple data operations concurrently, improving overall processing speed.
2. Error Handling: Error handling is critical when dealing with data operations, especially on large datasets. Ensure your code includes proper error-checking and logging mechanisms to handle unexpected situations gracefully.
3. Data Encoding: Node.js supports various character encodings for reading and writing CSV files. It allows you to specify the desired encoding when creating or reading a file, ensuring proper data representation.
4. Data Compression: For storage or transmission purposes, you can compress CSV files using compression algorithms like gzip. Node.js provides libraries to compress and decompress data efficiently.
Best Practices for Creating and Managing CSV Files with Node.js
To ensure efficient and reliable CSV file creation, consider the following best practices:
1. Handle Errors: Implement proper error handling and logging mechanisms to provide clear and meaningful error messages, enabling easier debugging and issue resolution.
2. Validate Input Data: Validate input data to ensure it adheres to the CSV format and any specific requirements. This step helps prevent data corruption and formatting issues.
3. Optimize Performance: Consider performance optimizations, such as using streaming techniques, processing data in chunks, or using parallel processing paradigms, when dealing with large datasets.
4. Test and Debug: Thoroughly test your CSV file creation code and debug any potential issues. Use unit tests, integration tests, and mocking frameworks to verify your code’s behavior under different scenarios.
5. Employ Code Reusability: Utilize reusable functions, modules, or libraries to promote code reusability and maintainability. This practice reduces redundancy and allows for easier maintenance and updates.
FAQs
Q: How do I write a CSV file in Node.js?
A: You can write a CSV file in Node.js using modules like `node-csv`, `csv-stringify`, or `fast-csv`. Refer to the article for a detailed example.
Q: How do I read a CSV file in Node.js?
A: Reading a CSV file in Node.js is straightforward. You can use modules like `node-csv` or `csv-parse` to parse the CSV file content. Refer to the article for a detailed example.
Q: What are the benefits of creating CSV files with Node.js?
A: Node.js offers simplified development, scalability, cross-platform compatibility, flexibility in data manipulation, and seamless integration with other technologies when creating CSV files.
Q: What are some common use cases for creating CSV files with Node.js?
A: Common use cases include data export, data visualization, data transformation, data migration, and data integration with other systems or tools.
Q: How can I handle large data sets when creating CSV files with Node.js?
A: Techniques like streaming, database integration, and processing data in smaller chunks can help efficiently handle large data sets in CSV files.
How To Write Csv File In Nodejs
How To Create A Csv File In Nodejs?
CSV (Comma Separated Values) files are commonly used for data storage and exchange, especially in spreadsheet applications. If you are working with Node.js and need to generate CSV files dynamically, this article will guide you through the process step by step. We will cover everything from installing the required packages to writing data to the CSV file.
Prerequisites:
Before we start, make sure you have Node.js installed on your machine. You can download it from the official website and follow the installation instructions.
Step 1: Setting up the project
Create a new directory for your project and navigate into it using the command line or terminal. To initialize a new Node.js project, run the following command:
“`
npm init -y
“`
This command initializes a new project with default settings and creates a package.json file.
Step 2: Installing required packages
To generate CSV files in Node.js, we need a couple of packages. We will use the `csv-writer` package to handle the creation and writing of CSV files. Install it by running the following command:
“`
npm install csv-writer
“`
This installs the `csv-writer` package as a dependency into your project.
Step 3: Writing data to a CSV file
Let’s write a simple script that will create a CSV file and write some data to it. Create a new file with a `.js` extension, for example `csvCreator.js`, and open it in your preferred text editor. Add the following code to the file:
“`javascript
const createCsvWriter = require(‘csv-writer’).createObjectCsvWriter;
const csvWriter = createCsvWriter({
path: ‘output.csv’,
header: [
{id: ‘name’, title: ‘Name’},
{id: ‘age’, title: ‘Age’},
{id: ’email’, title: ‘Email’}
]
});
const records = [
{name: ‘John Doe’, age: 25, email: ‘[email protected]’},
{name: ‘Jane Smith’, age: 30, email: ‘[email protected]’},
{name: ‘Mark Johnson’, age: 28, email: ‘[email protected]’}
];
csvWriter.writeRecords(records)
.then(() => console.log(‘CSV file created successfully’))
.catch((error) => console.error(error));
“`
In the above code, we import the `createObjectCsvWriter` function from the `csv-writer` package and create a new instance of it by providing the path and the header for our CSV file. The header defines the column names and consists of an array of objects, where each object has an `id` and a `title`. We then define our records as an array of objects.
Next, we use the `writeRecords` method of the `csvWriter` object to write the records to the CSV file. The method returns a promise, so we can use `.then()` to handle success and `.catch()` to handle any errors.
Save the file and run it using the command `node csvCreator.js`. This will execute the script, and you should see a message “CSV file created successfully” if everything goes well. You can check the project directory, and you should find the file `output.csv` containing the data you specified.
FAQs
Q: How can I append data to an existing CSV file?
To append data to an existing CSV file, you need to set the `append` option to `true` when creating the `csvWriter` object. Modify the code snippet from Step 3 as follows:
“`javascript
const csvWriter = createCsvWriter({
path: ‘output.csv’,
header: [
{id: ‘name’, title: ‘Name’},
{id: ‘age’, title: ‘Age’},
{id: ’email’, title: ‘Email’}
],
append: true
});
“`
Now, when you run the script again, the new records will be appended to the existing `output.csv` file.
Q: How can I format data in a specific way before writing it to the CSV file?
The `csv-writer` package provides a `formatters` option in the `createObjectCsvWriter` function to format data. You can define formatters for individual fields or the entire CSV writer. Here’s an example of formatting the `age` field as a string in the `csvCreator.js` file:
“`javascript
const csvWriter = createCsvWriter({
path: ‘output.csv’,
header: [
{id: ‘name’, title: ‘Name’},
{id: ‘age’, title: ‘Age’, formatter: (value) => String(value)},
{id: ’email’, title: ‘Email’}
]
});
“`
In the above code, we added a `formatter` function to the `age` field definition that converts the value to a string before writing it to the CSV file.
Conclusion:
Generating CSV files dynamically in Node.js is straightforward. By using the `csv-writer` package, you can easily create CSV files, define headers, and write data to them. Utilize the FAQs section to find answers to common questions you may encounter while working with CSV files in Node.js. Happy coding!
Can I Create My Own Csv File?
A Comma Separated Values (CSV) file is a popular data format used for storing and exchanging large amounts of structured data in a simple and easily readable format. It is commonly used in various applications, databases, and spreadsheets. Many people wonder if they can create their own CSV files, and the answer is a resounding yes! In this article, we will explore the process of creating a CSV file, provide step-by-step instructions, and answer some frequently asked questions about CSV files.
Creating your own CSV file is a straightforward task that requires minimal technical knowledge. All you need is a text editor or a spreadsheet program like Microsoft Excel or Google Sheets. Follow these simple steps to create your own CSV file:
Step 1: Determine the structure of your data
Before creating a CSV file, it is essential to determine the structure of your data. Decide what information you want to include in your file and how you want to organize it. For example, if you are creating a CSV file for a customer database, you may include columns such as name, email, age, and address.
Step 2: Open a text editor or a spreadsheet program
Once you have decided on the structure of your data, open a text editor like Notepad (Windows) or TextEdit (Mac), or a spreadsheet program such as Microsoft Excel or Google Sheets.
Step 3: Start entering your data
In your chosen application, start entering your data into columns. Each column should represent a different data point, and each row should contain the corresponding values. Make sure to separate each value with a comma. For example:
Name,Email,Age,Address
John Doe,[email protected],30,123 Main Street
Jane Smith,[email protected],25,456 Elm Street
Step 4: Save the file as a CSV
After entering your data, save the file with a .csv extension. This will signify that it is a CSV file. Ensure that you save it in a location where you can easily access it later.
Congratulations! You have successfully created your own CSV file. You can now use this file to import data into various applications, databases, or even share it with others.
Frequently Asked Questions (FAQs)
Q1: Can I create a CSV file with any type of data?
A: Yes, you can create a CSV file using any type of structured data. Whether you have a list of contacts, financial information, or inventory details, CSV files can handle a wide range of data types.
Q2: Can I create a CSV file using special characters and symbols?
A: While CSV files can support special characters and symbols, it is essential to ensure that you correctly handle them. Special characters like commas, quotes, and line breaks need to be appropriately formatted so that they do not interfere with the structure of the CSV file.
Q3: Can I edit a CSV file after creating it?
A: Yes, you can easily edit a CSV file using a text editor or a spreadsheet program. Simply open the file, make the desired changes, and save it again. However, it is important to maintain the structure and formatting of the CSV file while editing to avoid any issues.
Q4: Can I convert an existing file into a CSV file?
A: Yes, you can convert an existing file into a CSV file. For example, if you have a spreadsheet in Microsoft Excel, you can save it as a CSV file by selecting the “Save As” option and choosing the CSV format. This will create a new file in CSV format while preserving the data from the original file.
Q5: Can I import a CSV file into a database or a spreadsheet?
A: Absolutely! CSV files are commonly used for importing data into databases or spreadsheets. Many applications and platforms provide functionalities to import CSV files, allowing you to populate your databases or spreadsheets easily.
In conclusion, creating your own CSV file is a simple and practical way to organize and exchange structured data efficiently. With minimal technical knowledge required, anyone can create their own CSV file using a text editor or a spreadsheet program. By following the step-by-step instructions provided in this article, you can easily create and utilize CSV files for various purposes. Happy file creating!
Keywords searched by users: nodejs create csv file Nodejs write CSV file, node-csv, NodeJS export csv file, csv-parse, csv-stringify, csv-parser npm, fast-csv, Reading CSV file nodejs
Categories: Top 40 Nodejs Create Csv File
See more here: nhanvietluanvan.com
Nodejs Write Csv File
To begin with, let’s understand what a CSV file is and why it is widely used. A CSV file is a simple text file that stores tabular data in plain text, with each line representing a row and each value separated by commas. CSV files are widely supported by various applications and databases, making them an excellent choice for data exchange between different systems.
To write a CSV file in Node.js, we can make use of the built-in `fs` module. The `fs` module provides a set of functions that allow us to interact with the file system. To get started, we first need to include the `fs` module with the `require` keyword:
“`javascript
const fs = require(‘fs’);
“`
Next, we need to define the data that we want to write to the CSV file. For the sake of simplicity, let’s consider an array of objects representing employees:
“`javascript
const employees = [
{ name: ‘John Doe’, age: 30, designation: ‘Software Engineer’ },
{ name: ‘Jane Smith’, age: 25, designation: ‘UI/UX Designer’ },
{ name: ‘Robert Johnson’, age: 35, designation: ‘Project Manager’ }
];
“`
Now, we can proceed to write the CSV file. We start by creating a writable stream using the `fs.createWriteStream` function, which takes the file path as an argument:
“`javascript
const filePath = ’employees.csv’;
const writeStream = fs.createWriteStream(filePath);
“`
After creating the write stream, we need to write the CSV header row to define the column names. In our case, the column names would be “name”, “age”, and “designation”. To write the header row, we can use the `write` method of the write stream:
“`javascript
writeStream.write(‘name,age,designation\n’);
“`
Next, we can loop through the employees array and write each employee’s data as a new row in the CSV file. We can achieve this using the `forEach` method:
“`javascript
employees.forEach(employee => {
const row = `${employee.name},${employee.age},${employee.designation}\n`;
writeStream.write(row);
});
“`
Finally, we need to close the write stream to ensure that all the data is written to the file:
“`javascript
writeStream.end();
“`
That’s it! The above code will create a new file named “employees.csv” in the same directory as your Node.js script and write the employee data as a CSV file.
Now, let’s address some common questions that might arise while writing CSV files in Node.js.
—
### FAQs
**Q: Can I append data to an existing CSV file?**
Yes, you can append data to an existing CSV file by using the `fs.createWriteStream` function with the `{ flags: ‘a’ }` option. This option tells Node.js to open the file in append mode, allowing you to add new records without overwriting the existing ones.
**Q: How can I handle errors while writing a CSV file?**
You can handle errors by listening to the `’error’` event on the write stream. The `’error’` event is emitted when a write operation fails. To handle errors, you can attach a listener to the write stream and log or display the error message accordingly.
“`javascript
writeStream.on(‘error’, error => {
console.error(‘An error occurred:’, error.message);
});
“`
**Q: Are there any libraries available for writing CSV files in Node.js?**
Yes, there are several third-party libraries available that provide additional features and utilities for working with CSV files in Node.js. Some popular libraries include `csv-writer`, `fast-csv`, and `papaparse`. These libraries offer a high-level API and simplify the process of reading, writing, and manipulating CSV files.
—
In conclusion, writing CSV files in Node.js is a straightforward task using the built-in `fs` module. By leveraging the `fs` module, you can create, write, and modify CSV files to store and exchange tabular data efficiently. Handle errors effectively, consider using third-party libraries for more advanced functionality, and make the most out of Node.js to empower your web development projects.
Node-Csv
Node.js is a popular runtime environment that allows developers to build scalable and efficient web applications. One of the essential components of web applications is the ability to interact with and manipulate CSV (comma-separated values) files. Node-csv is a powerful library that provides an easy-to-use interface for parsing, transforming, and writing CSV files in Node.js. In this article, we will explore the features and capabilities of node-csv and learn how to use it effectively in our Node.js projects.
Features and Capabilities of Node-csv:
1. Parsing CSV Files:
Node-csv simplifies the process of parsing CSV files by providing a straightforward API. You can easily read a CSV file using the `csv.parse()` method, which emits events for each row and field in the file. This allows for efficient processing of large CSV files without loading the entire content into memory. Additionally, you can configure various options, such as delimiter, escape character, and row limit, to accommodate different file formats and requirements.
2. Writing CSV Files:
Node-csv also provides a seamless way to create and write CSV files. You can use the `csv.stringify()` method to convert an array of objects or an array of arrays into a CSV string. This string can then be written to a file using Node.js’ built-in file system module. The library supports various configuration options, including delimiter, quote character, and header row, to ensure compatibility with different systems and applications.
3. Transformed Output:
In many scenarios, it is necessary to transform the data before writing it to a CSV file or after parsing it from a CSV file. Node-csv allows you to easily manipulate the data using a transform function. This function can be applied to each row or field, giving you the flexibility to modify, filter, or enrich the data as needed. The transformed output can then be written to a new CSV file or processed further in your Node.js application.
4. Stream Integration:
One of the key advantages of using node-csv is its seamless integration with Node.js streams. Streams provide an efficient way to process data in small chunks rather than loading it entirely into memory. Node-csv leverages streams throughout its API, allowing you to parse, transform, and write CSV files in a memory-efficient manner. Whether you are working with large files or real-time data streams, node-csv has you covered.
FAQs:
Q1. Can node-csv handle CSV files with different delimiters?
Yes, node-csv supports various delimiters, including the default comma delimiter. You can specify the delimiter of your choice using the `csv.parse()` or `csv.stringify()` methods.
Q2. How can I handle CSV files with headers?
Node-csv provides an option to include a header row in the output CSV file. By setting the `columns` option to `true` in the `csv.stringify()` method, the library automatically uses the object keys or array indices as headers.
Q3. Is node-csv only for reading and writing CSV files?
No, node-csv is not limited to reading and writing CSV files. It also offers powerful data manipulation capabilities through its transform function. You can use this function to modify, filter, or enrich the data during parsing or before writing it to a file.
Q4. Can node-csv handle large CSV files efficiently?
Yes, node-csv is designed to handle large CSV files efficiently. It uses streams internally, allowing you to process data in chunks rather than loading the entire file into memory. This makes it suitable for working with CSV files of any size.
Q5. Are there any performance considerations when using node-csv?
Node-csv is optimized for performance and provides a high level of efficiency. However, keep in mind that complex transformation or processing logic may impact performance, especially when dealing with large files. It is recommended to benchmark and optimize your code if performance is a concern.
Conclusion:
Node-csv is a versatile and powerful library for handling CSV files in Node.js. Its straightforward API, support for data transformation, stream integration, and efficient performance make it an excellent choice for developers working with CSV data. By leveraging the features and capabilities of node-csv, you can easily parse, manipulate, and write CSV files in your Node.js applications, enabling seamless integration with various systems and data sources.
Nodejs Export Csv File
Introduction:
Node.js is a popular runtime environment for executing JavaScript outside of a web browser. It provides various modules and libraries that enable developers to build scalable and high-performing applications. One such functionality is the ability to export data to a CSV file seamlessly. In this article, we will explore the process of exporting CSV files using Node.js and delve into the various techniques and libraries available for this purpose.
Exporting CSV Files with Node.js:
CSV (Comma-Separated Values) files are widely used for data exchange between different platforms and systems. Exporting data to a CSV file involves writing data in a tabular format with each record represented as a line and individual field values separated by commas. Node.js offers multiple ways to achieve this. Let’s explore a few popular options:
1. Using Built-in File System Module:
The built-in fs module in Node.js allows interaction with the file system, including reading and writing files. To export data to a CSV file using this module, we need to follow a series of steps:
i. Create a writable stream using the fs.createWriteStream() function.
ii. Write the CSV headers to the stream.
iii. Loop over the data and write each record to the stream.
iv. Close the stream and save the file.
While this approach provides control and flexibility, it requires manual handling of headers and stringifying data. Fortunately, there are libraries that simplify this process.
2. Using CSV Writer Libraries:
a. Objects-to-CSV: This library allows exporting JavaScript objects directly to CSV format. It automatically converts object properties into CSV headers and values. Objects-to-CSV supports a wide range of data types and offers additional features like custom delimiter selection and appending data to an existing file.
b. Csv-writer: Csv-writer is another popular library that provides an intuitive API for generating CSV files. It allows customization of headers, delimiter selection, and options like writing headers only once or appending data to an existing file. Csv-writer also offers extensive cell formatting options, including date and number formats.
These libraries considerably simplify the CSV export process, eliminating the need for manual formatting and writing records line by line. Developers can focus on generating the required data and let the libraries handle the rest.
3. Using Database Query Results:
Many applications leverage databases to store and manage data. Node.js frameworks like Express.js facilitate interaction with databases through various ORMs (Object-Relational Mappers) and query builders. Exporting query results directly to a CSV file without manually handling the data transformation can be achieved using libraries like Knex.js and pg-promise.
a. Knex.js: Knex.js is a query builder library compatible with multiple relational databases. It allows developers to write SQL queries using JavaScript and provides a convenient method, .stream(), to export query results directly to a writable stream. This stream can then be piped to a CSV file.
b. pg-promise: pg-promise is a PostgreSQL library that enables efficient communication with PostgreSQL databases. Along with its querying capabilities, it offers a convenient CSV output mode to export query results directly to a CSV file. This simplifies the process of exporting large datasets as CSV files.
Frequently Asked Questions (FAQs):
Q1. Can I use Node.js to export large datasets as CSV files?
A1. Yes, Node.js is well-suited for exporting large datasets as CSV files. Using libraries like Knex.js and pg-promise, you can directly export query results to CSV files without loading the entire dataset into memory.
Q2. How can I specify the CSV delimiter used while exporting data?
A2. Libraries like Objects-to-CSV and Csv-writer allow customization of delimiter options. You can specify a delimiter of your choice when exporting data to CSV files.
Q3. Can I append data to an existing CSV file using Node.js?
A3. Yes, both Objects-to-CSV and Csv-writer libraries allow you to append data to an existing CSV file. Simply instantiate the library with the existing file path, and any new records will be appended to it.
Q4. What are the advantages of using libraries over manual CSV file generation?
A4. Libraries simplify the CSV export process by automatically handling headers, data conversion, and stringifying. They also provide additional features like delimiter customization, cell formatting, and appending data to existing files. This saves development time and reduces the chance of errors.
Q5. Are there any security considerations when exporting CSV files with Node.js?
A5. When exporting CSV files, ensure that the input data is properly validated and sanitized to prevent any possible injection attacks. Additionally, restrict access permissions to prevent unauthorized read/write operations on the generated CSV files.
Conclusion:
Node.js, with its rich ecosystem of modules and libraries, provides several effective methods to export data as CSV files. Developers can choose between manual file system operations, specialized CSV writer libraries, or leverage ORM functionalities to seamlessly export data from databases. With the information provided in this article, you should be well-equipped to export CSV files using Node.js and handle various scenarios with ease.
Images related to the topic nodejs create csv file
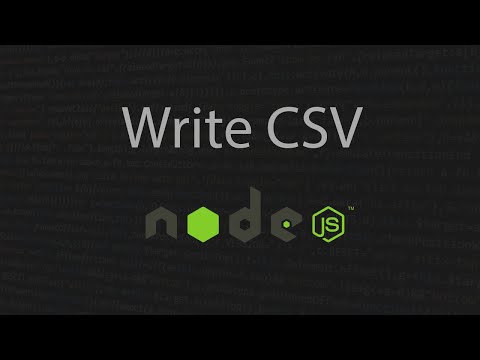
Found 47 images related to nodejs create csv file theme

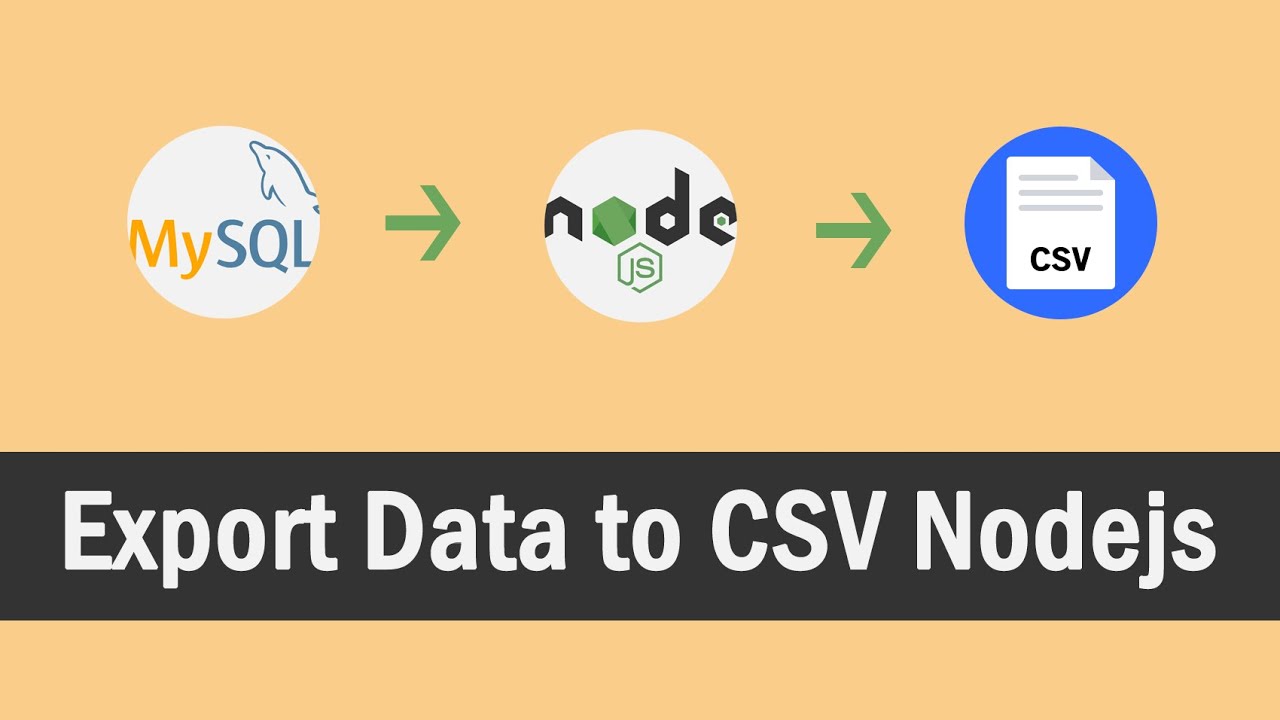
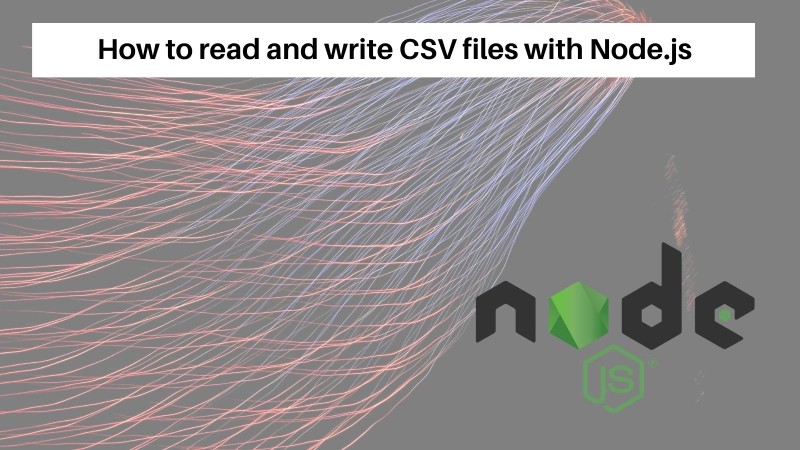
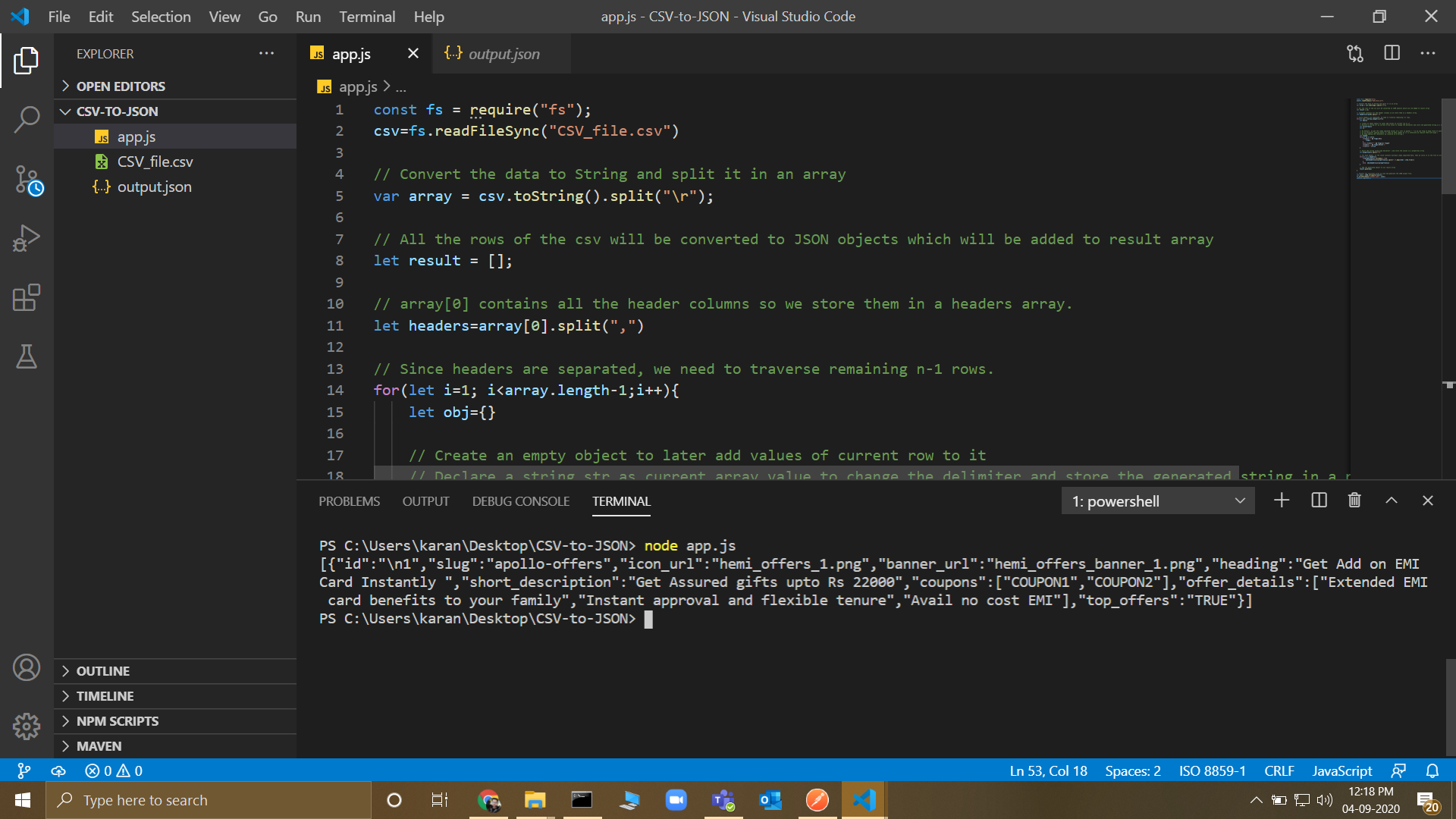
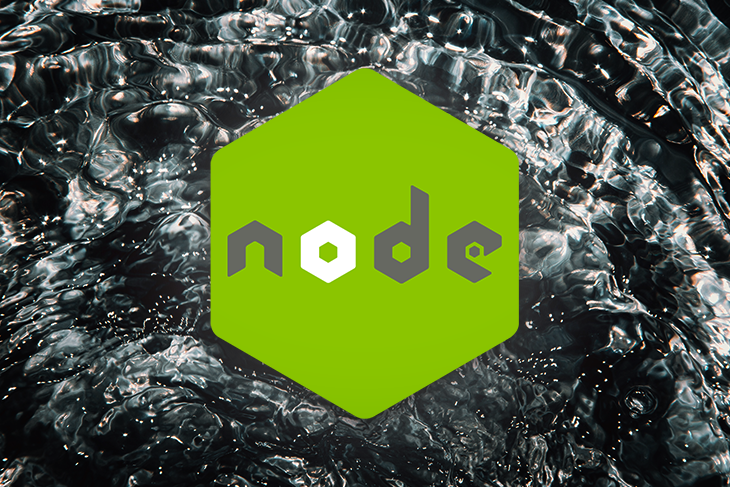

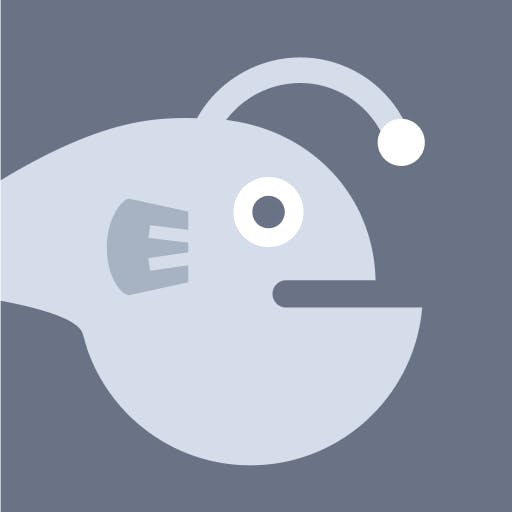
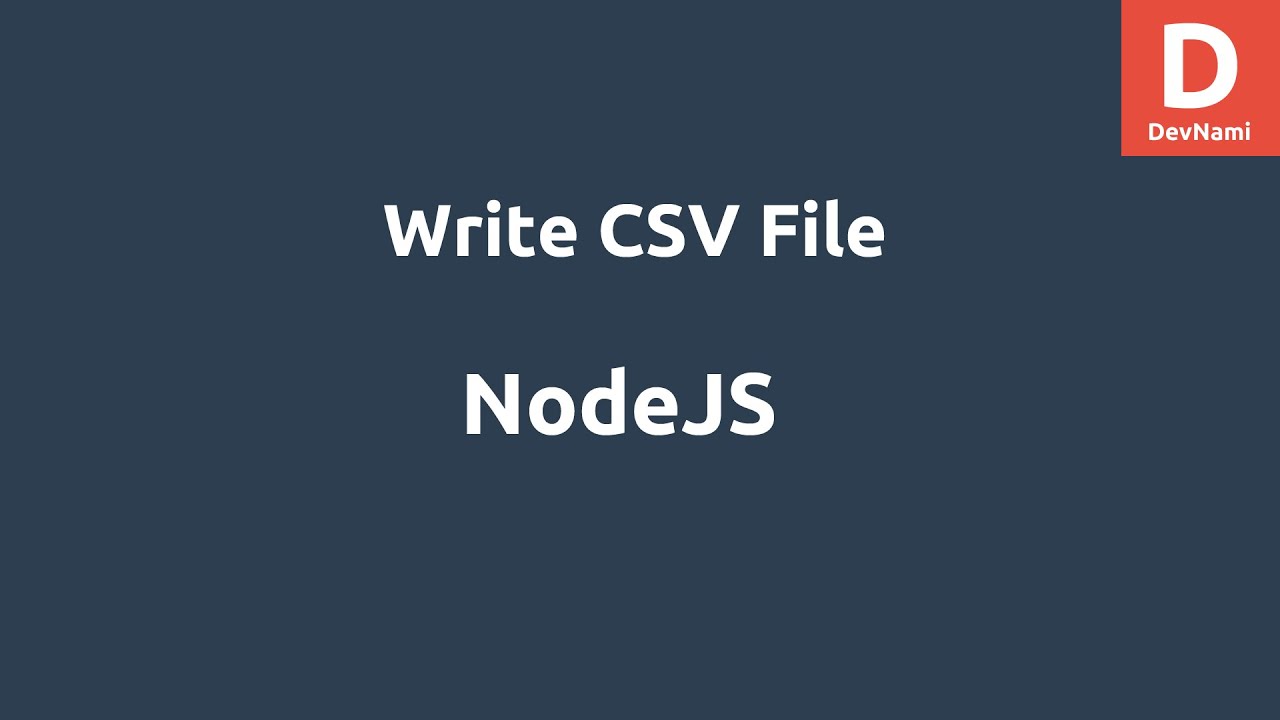
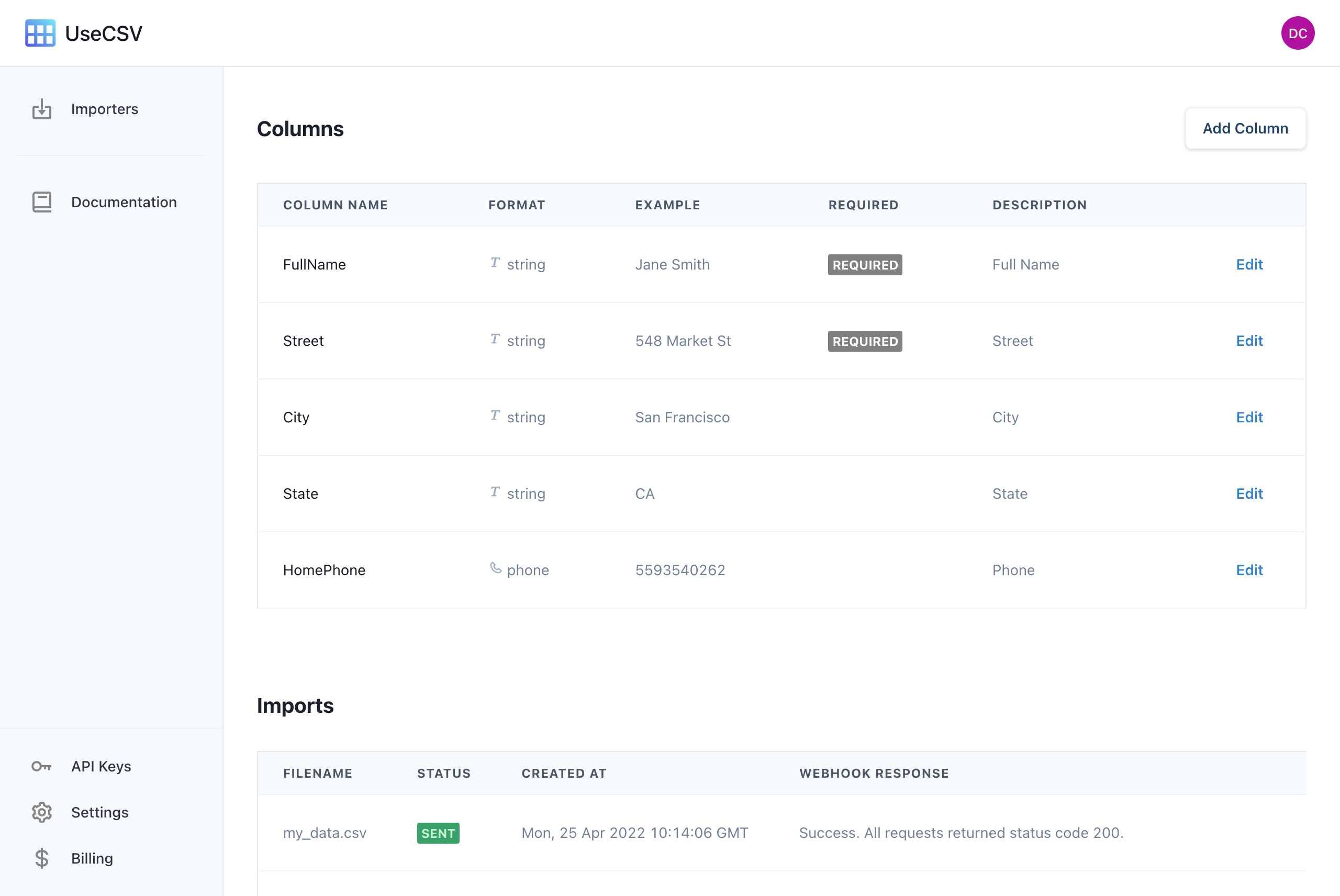
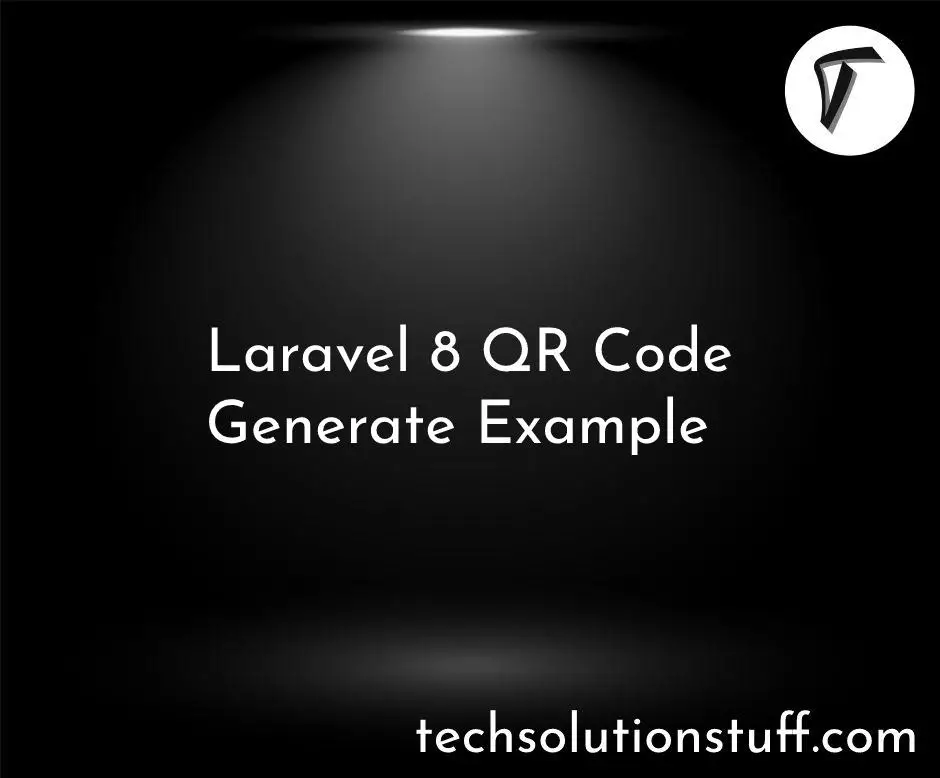


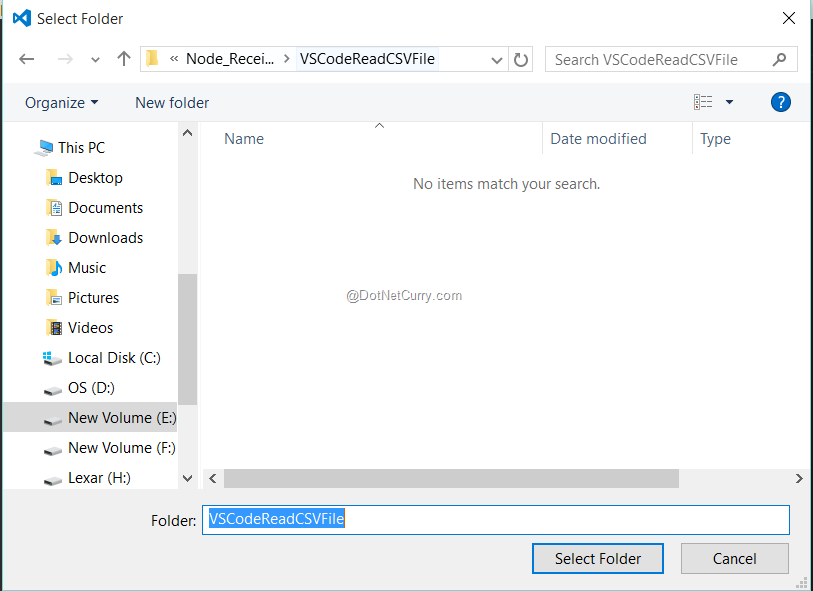
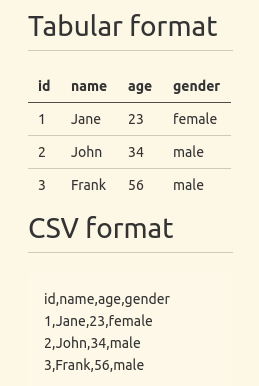
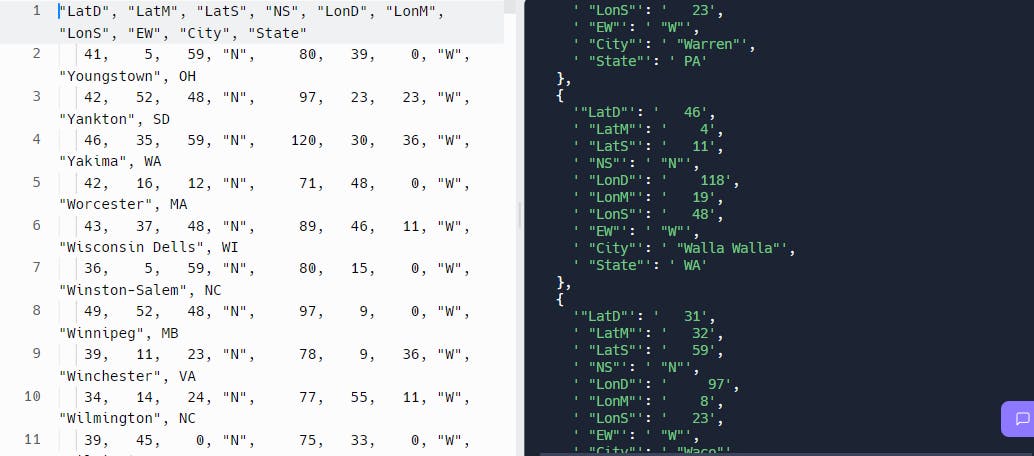
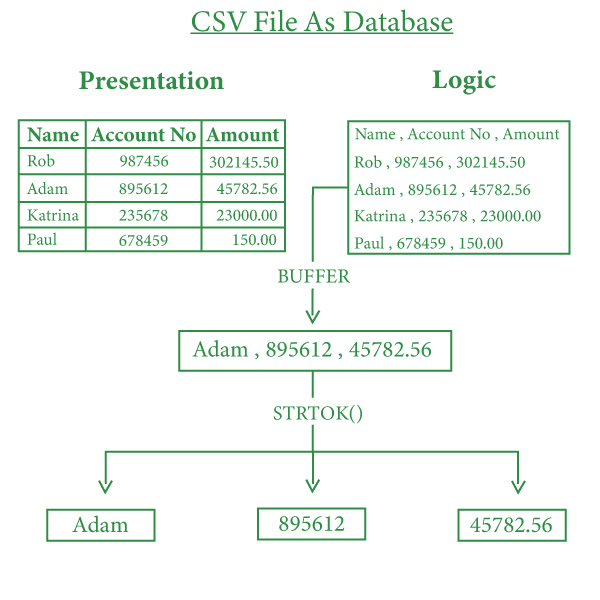
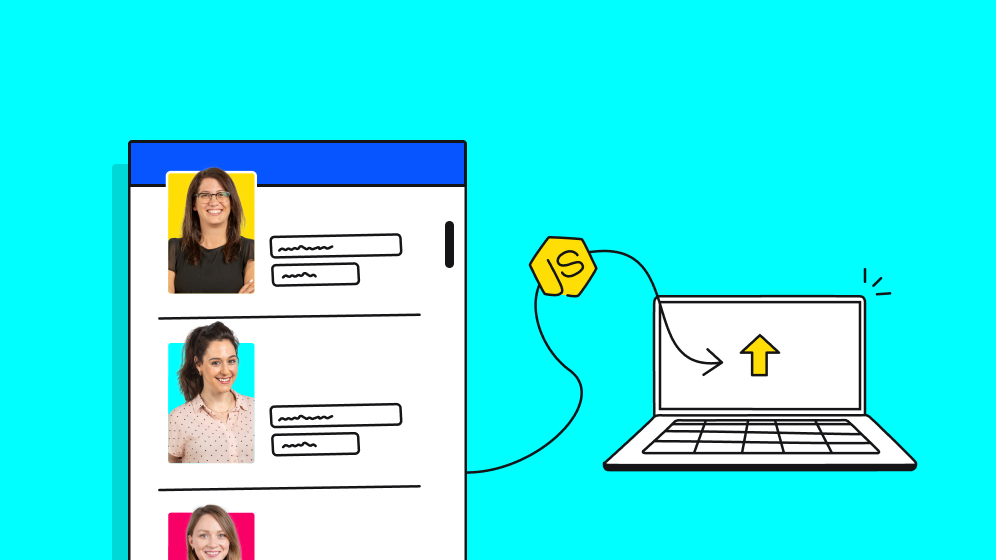
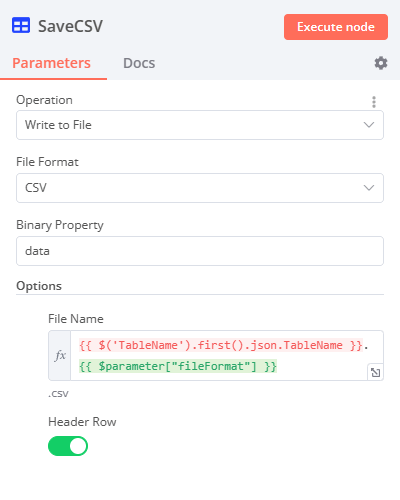

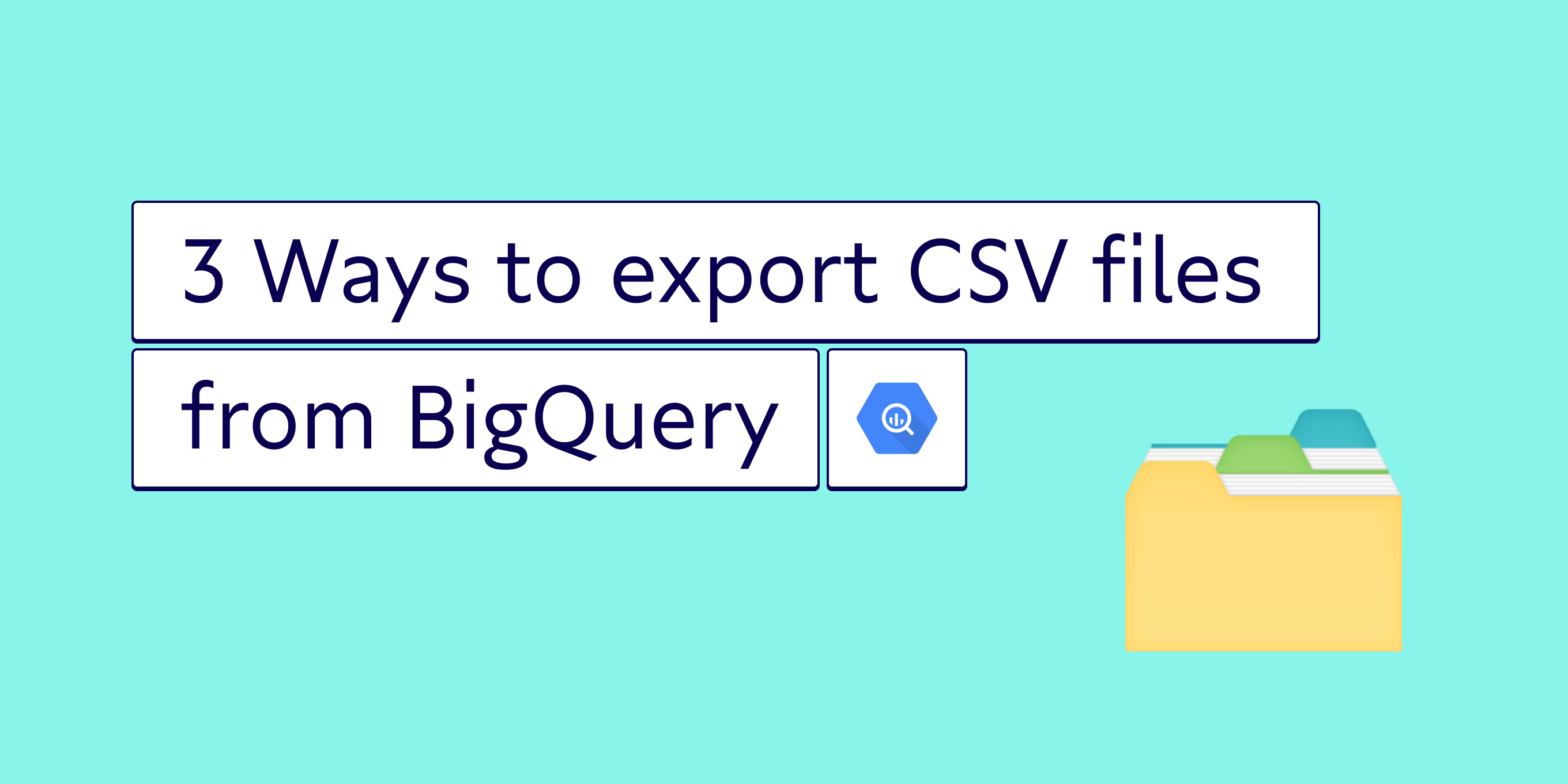
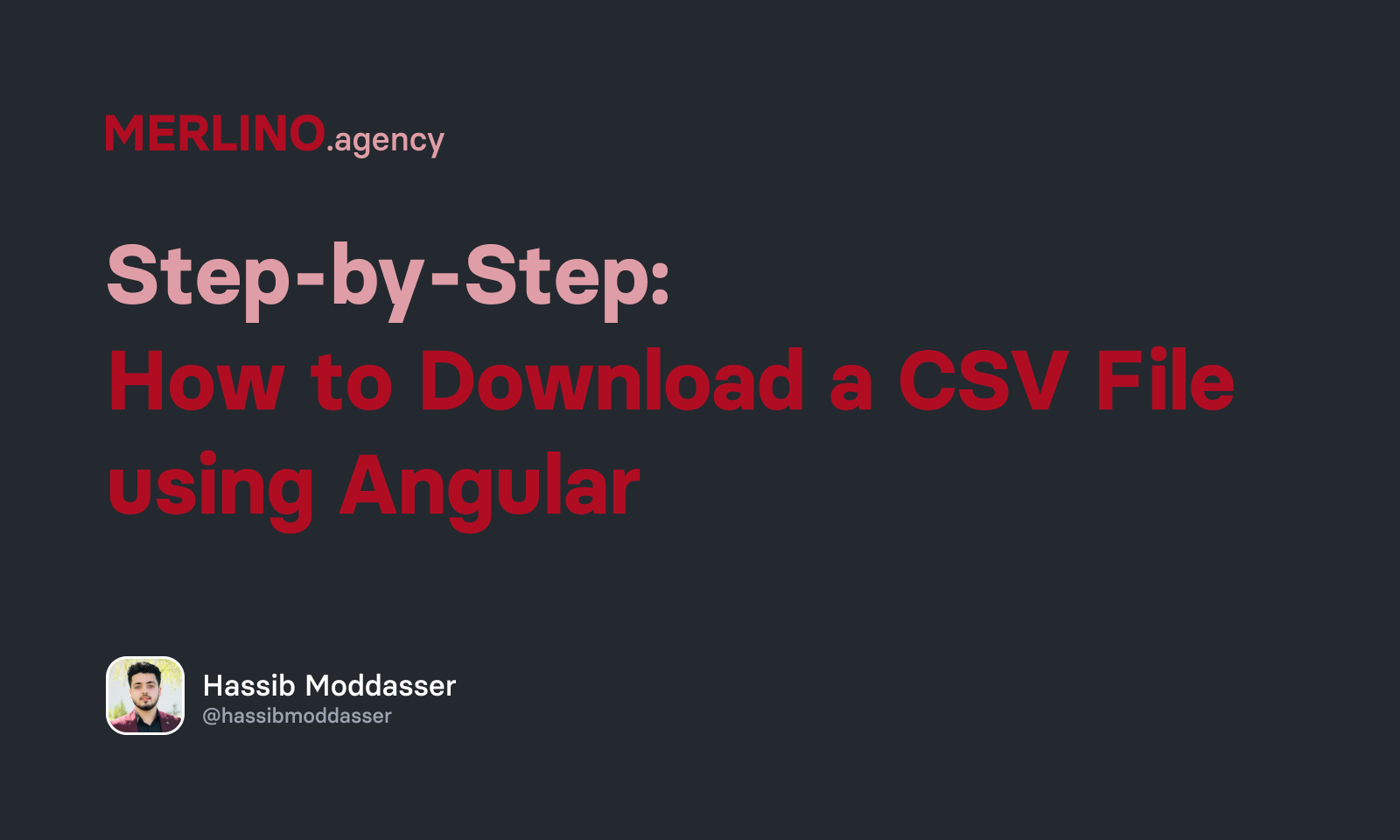
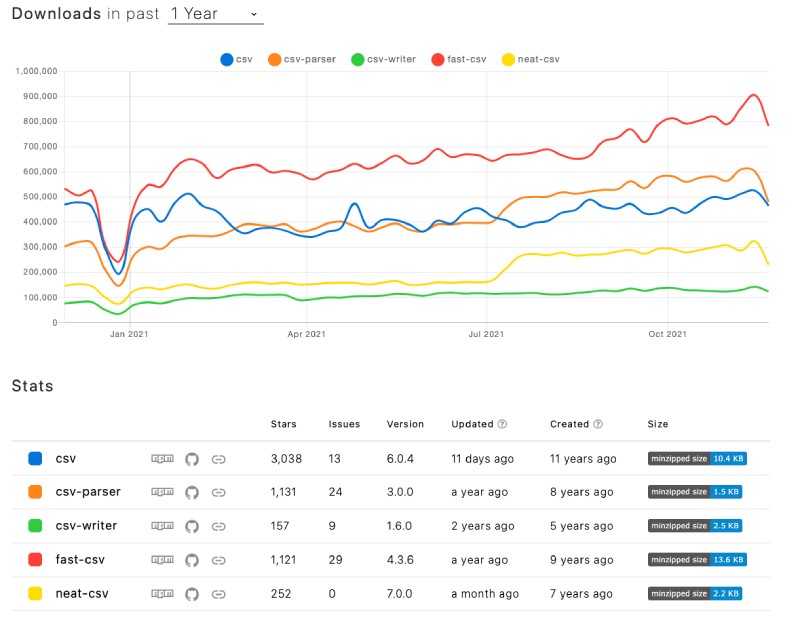
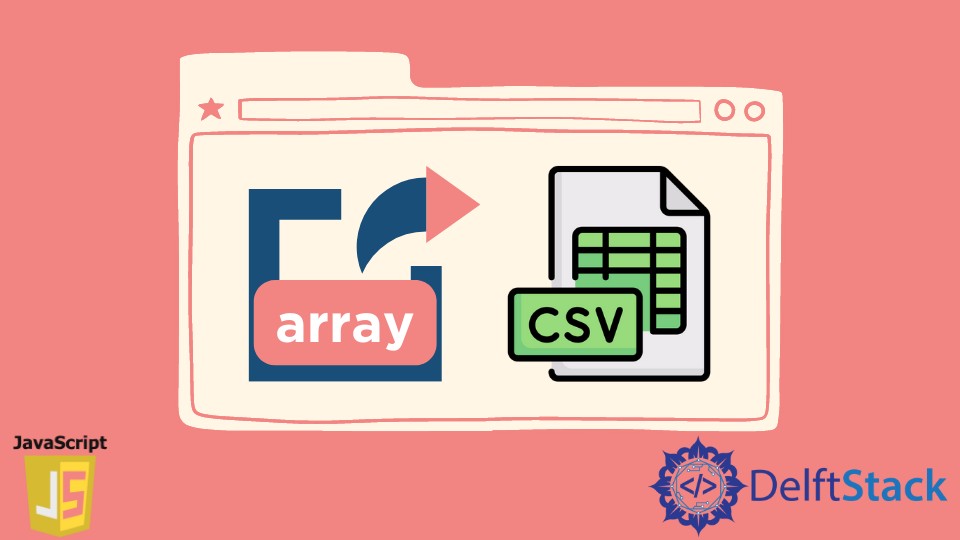
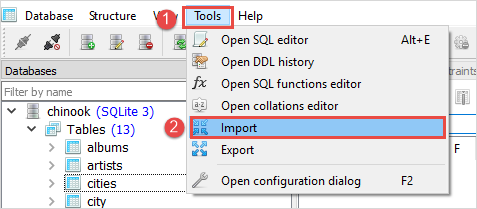
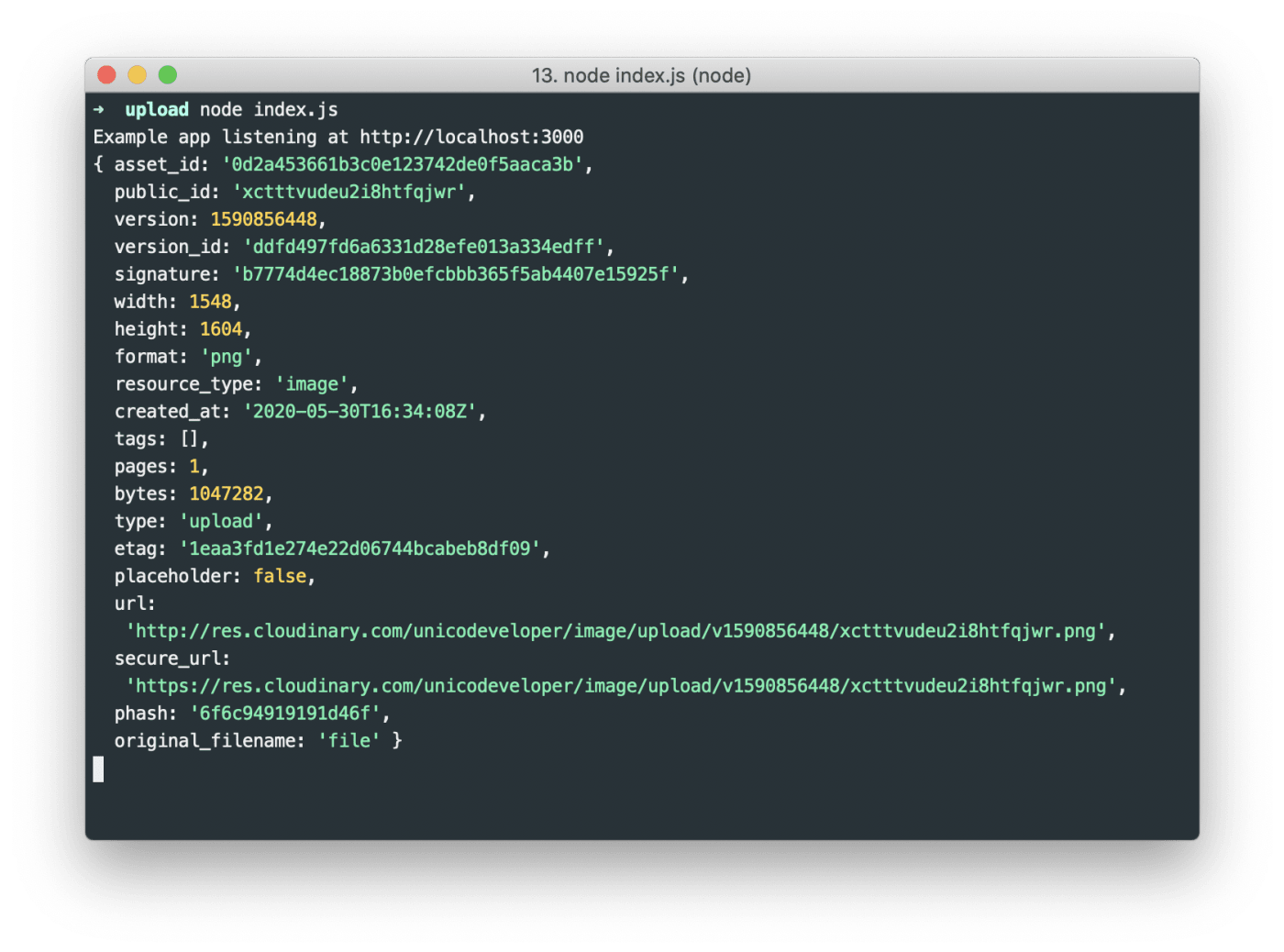
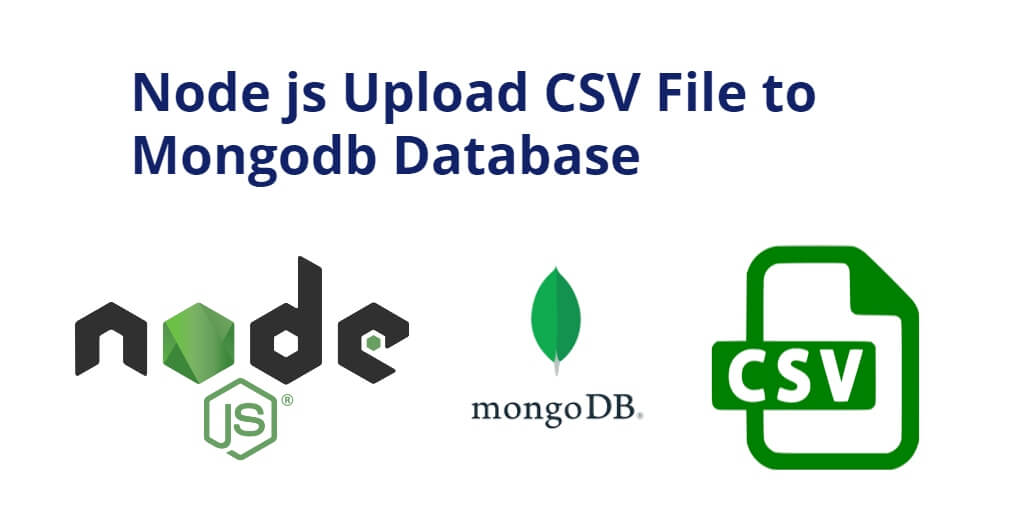
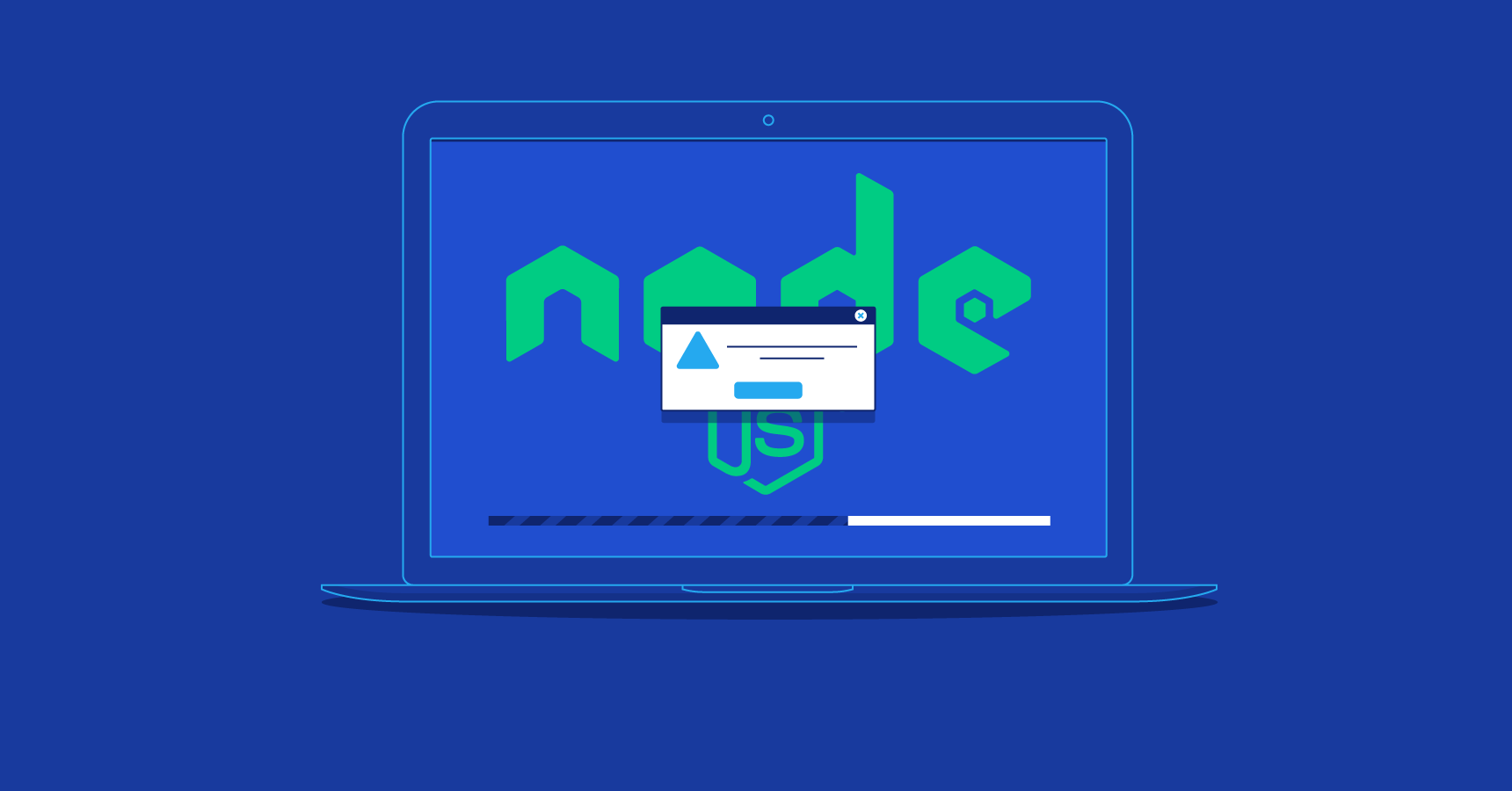
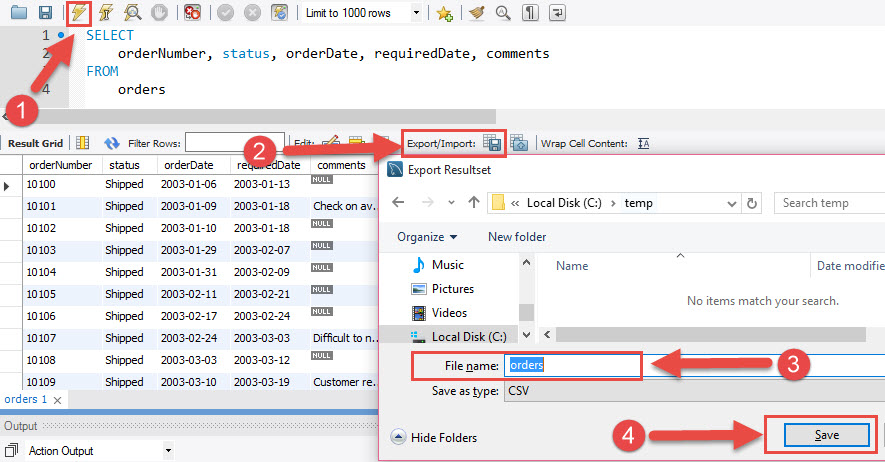
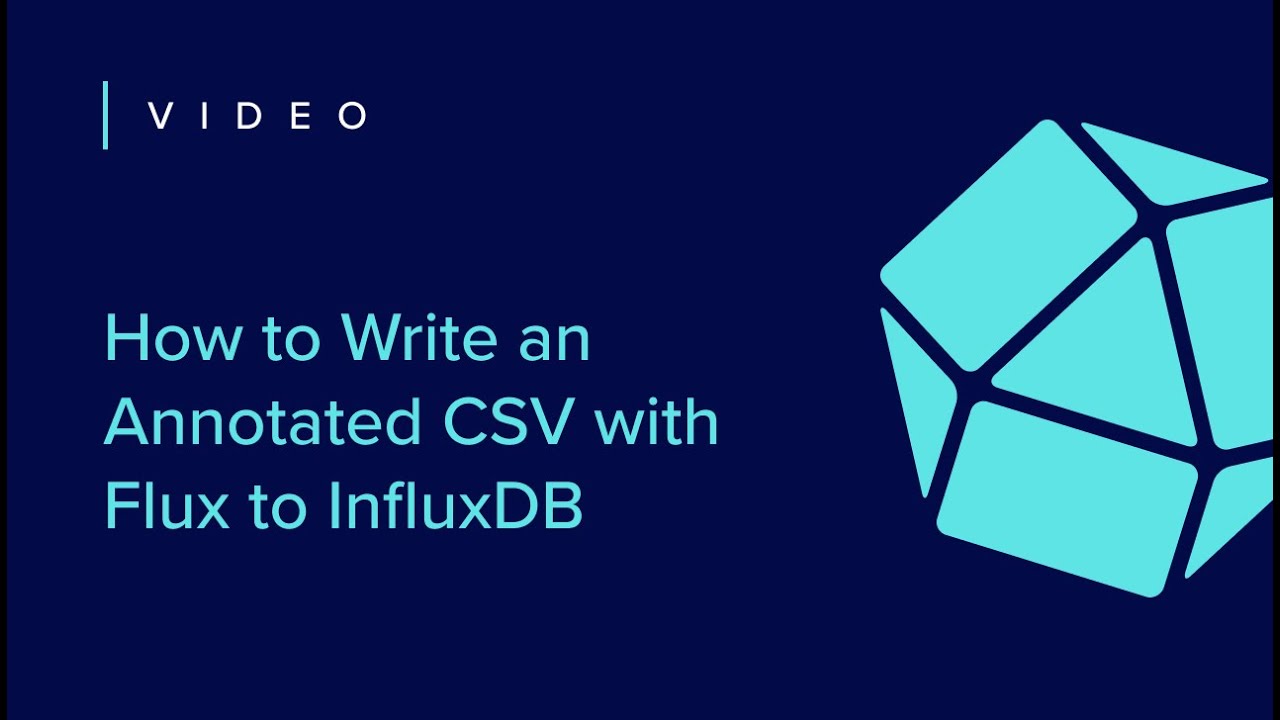
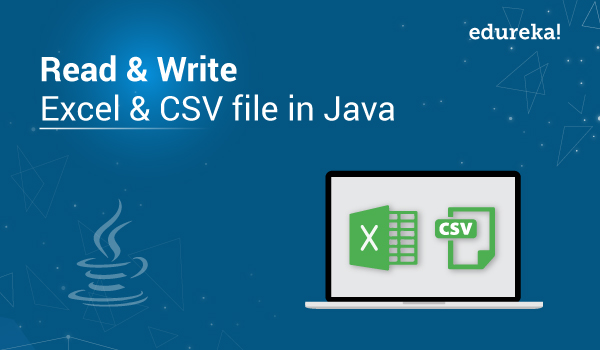

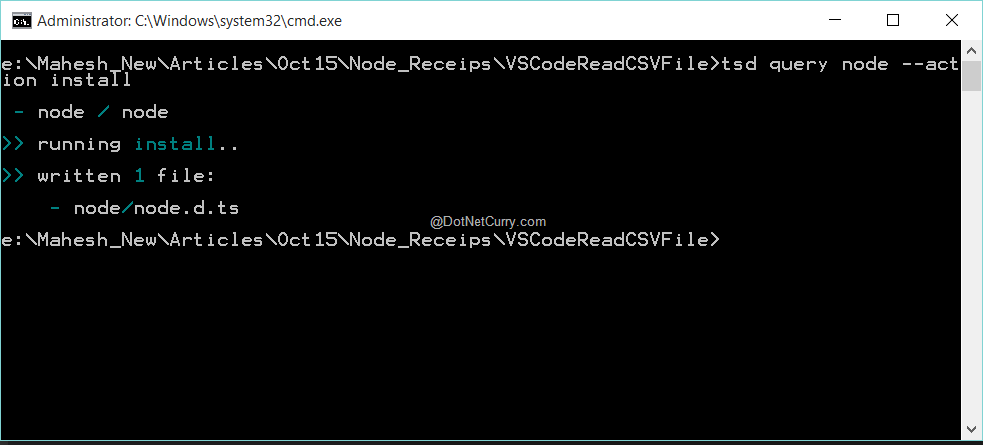
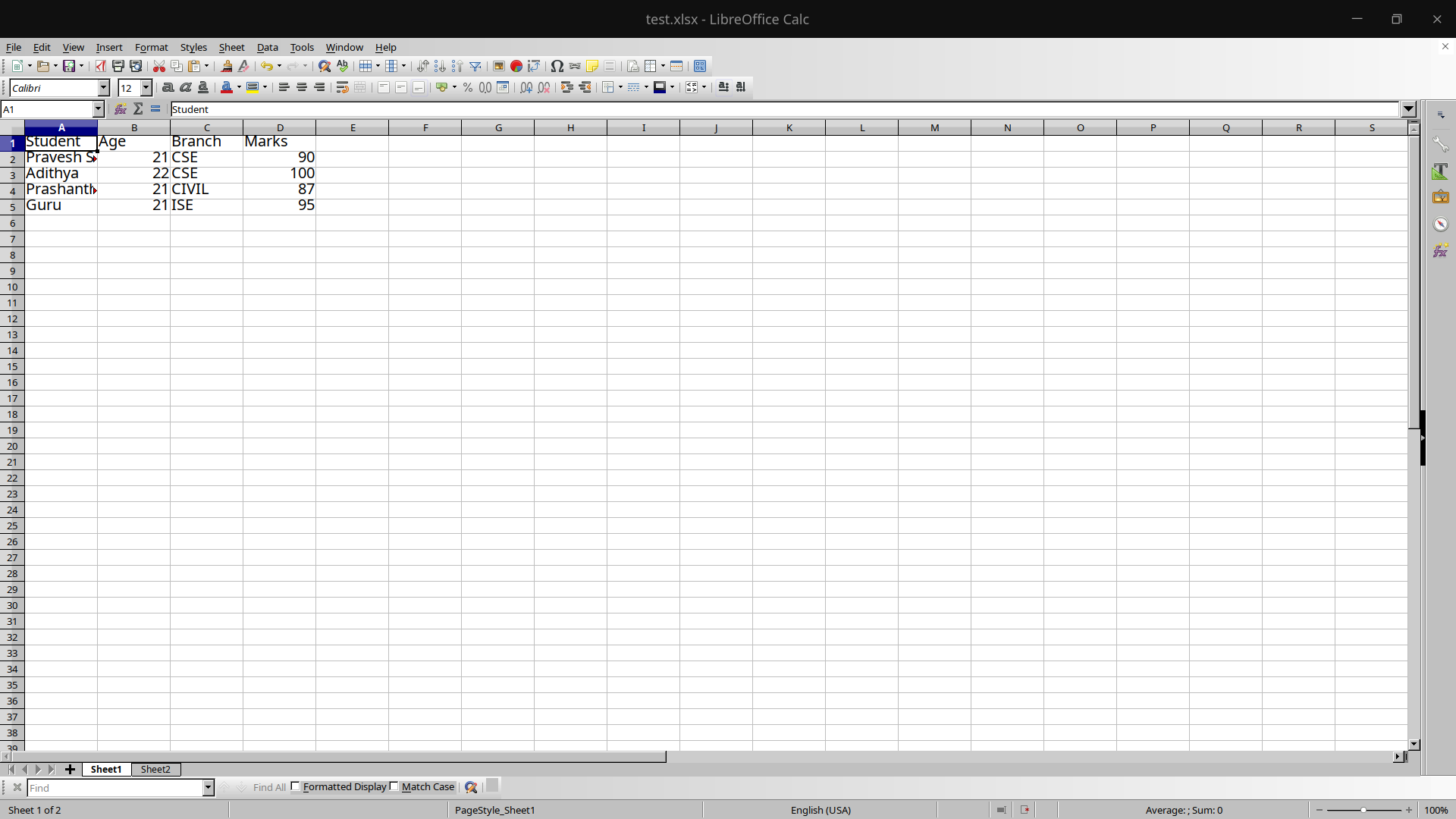

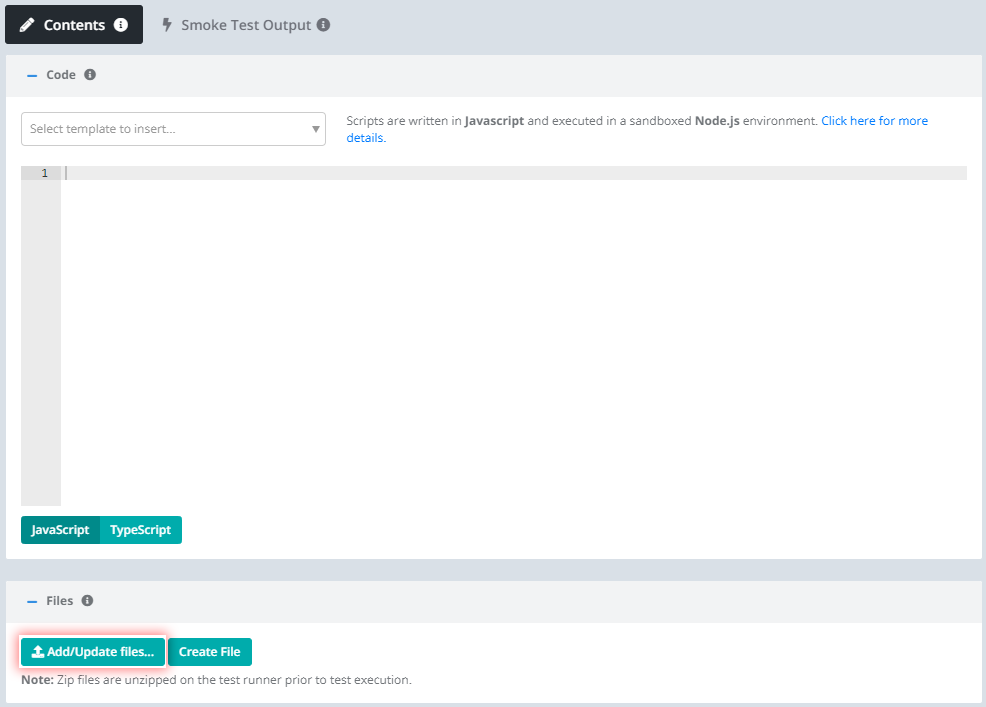
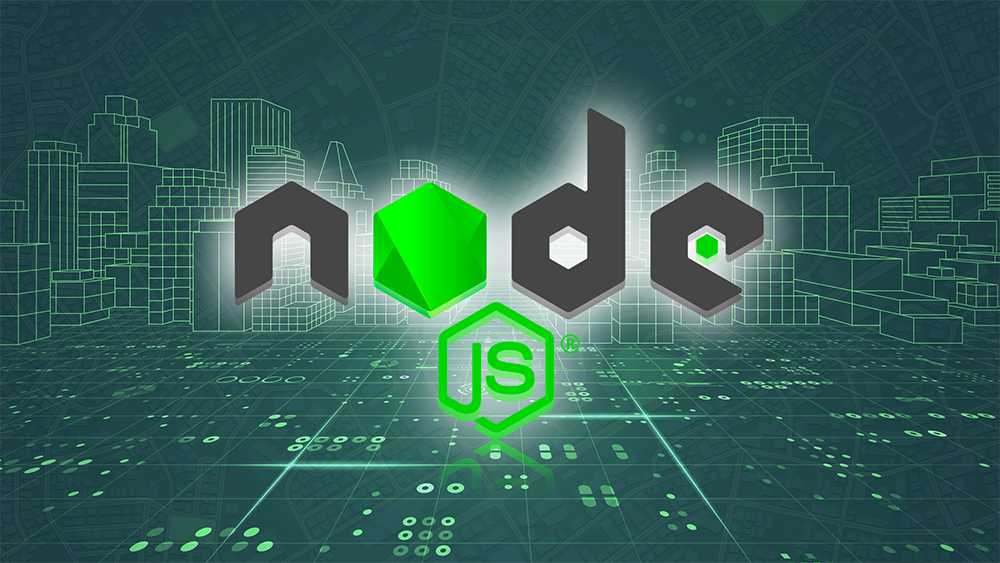
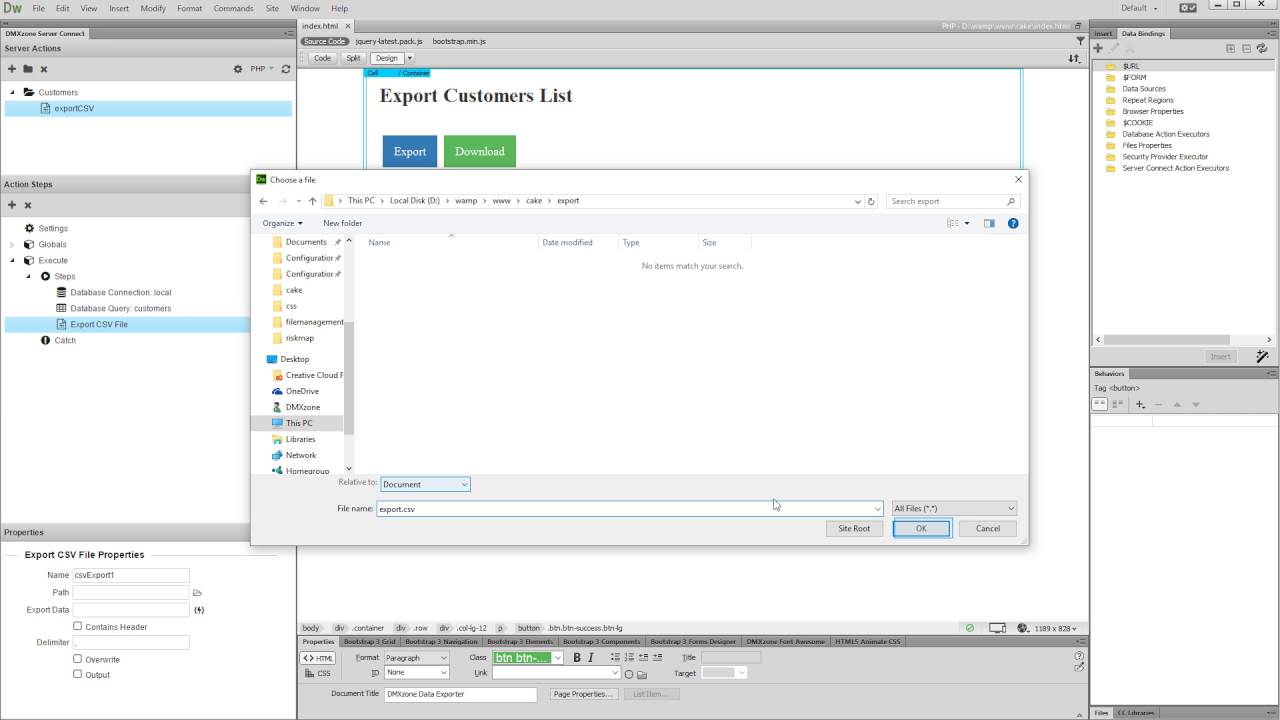

Article link: nodejs create csv file.
Learn more about the topic nodejs create csv file.
- How To Read and Write CSV Files in Node.js Using Node-CSV
- Write CSV Files In NodeJS (Very Simple Examples) – Code Boxx
- How To Write CSV Files With Node.js – CodingTheSmartWay
- Write to a CSV in Node.js – javascript – Stack Overflow
- How to Write to CSV File | JavaScript and Node.js – YouTube
- How to Create a CSV File Using Microsoft Excel – Mass.gov
- How to Read and Write to CSV file in NodeJS – Ashish Maurya’s Blog
- Node.js File System Module – W3Schools
- Generate a CSV file from data using Node.js – Teco Tutorials
- How to Write CSV File Data in Node JS? – NiceSnippets
- A complete guide to CSV files in Node.js – LogRocket Blog
See more: https://nhanvietluanvan.com/luat-hoc/