Networkx Iterate Over Nodes
Understanding Nodes in Networkx
In Networkx, a graph is composed of nodes and edges. Nodes represent individual entities in the network, while edges represent the connections or relationships between these entities. Before delving into iterating over nodes in Networkx, it is essential to have a clear understanding of what nodes are.
Nodes are typically represented using hashable objects in Networkx. Hashable objects are those that can be used as keys in Python dictionaries. Nodes can be of any type that is hashable, including numbers, strings, and tuples. The attributes of a node, such as its label or weight, can be stored as a dictionary where the keys correspond to the attribute names.
Methods to Iterate Over Nodes in Networkx
Networkx provides several methods to iterate over nodes, allowing users to perform various operations on the nodes of a graph. Here are some of the common methods to iterate over nodes in Networkx:
1. `nodes()`: This method returns an iterator over all the nodes in the graph.
2. `nodes_iter()`: This method behaves similarly to `nodes()`, but returns a list of node objects.
3. `list(G)`: This method returns a list of all nodes in the graph.
Iterating over Nodes and Utilizing Node Attributes
One of the significant advantages of Networkx is its ability to store and access attributes for nodes and edges. These attributes can provide additional information about the nodes, such as labels, weights, or properties. When iterating over nodes, it becomes essential to understand how to retrieve and utilize these attributes.
Let’s consider a simple example where we have a graph of social network connections. Each node represents a person, and the edges represent friendships. Suppose we want to iterate over all the nodes and print their names:
“`
import networkx as nx
# Creating a graph
G = nx.Graph()
# Adding nodes with attributes
G.add_node(1, name=’Alice’)
G.add_node(2, name=’Bob’)
G.add_node(3, name=’Charlie’)
# Iterating over nodes and accessing attributes
for node in G.nodes():
print(node, G.nodes[node][‘name’])
“`
In this example, we use the `nodes()` method to iterate over all the nodes in the graph `G`. By accessing the node attributes using `G.nodes[node][‘name’]`, we can retrieve the name attribute associated with each node and print it.
Applying Filters while Iterating over Nodes in Networkx
Networkx also allows you to apply filters while iterating over nodes, enabling you to select specific nodes based on criteria such as attribute values or connectivity. By using filters, you can narrow down your iteration to specific subsets of nodes, making your analysis more focused and efficient.
For instance, let’s consider a scenario where we have a graph of email communication, and we want to iterate over only those nodes that have more than 100 outgoing emails. We can achieve this by using a combination of the `nodes()` method and an if statement to apply the filter:
“`
import networkx as nx
# Creating a graph
G = nx.DiGraph()
# Adding nodes with attributes
G.add_node(1, emails_sent=150)
G.add_node(2, emails_sent=50)
G.add_node(3, emails_sent=200)
# Iterating over filtered nodes
for node in G.nodes():
if G.nodes[node][’emails_sent’] > 100:
print(node, G.nodes[node][’emails_sent’])
“`
In this example, we iterate over all the nodes and only consider the nodes where the attribute `emails_sent` is greater than 100. This allows us to focus on the nodes that meet our criteria.
Examples of Iterating Over Nodes in Networkx
To further solidify the understanding of iterating over nodes in Networkx, let’s explore a few more examples that cover different use cases and scenarios.
1. Networkx Iterate Over Edges:
To iterate over edges in Networkx, you can use the method `edges()` or `edges_iter()`. Here’s an example:
“`
for edge in G.edges():
print(edge)
“`
2. Networkx Query Nodes:
If you want to query nodes based on certain conditions, you can use the `nodes()` method along with a conditional statement. Here’s an example where we query nodes with an attribute value of True:
“`
for node in G.nodes():
if G.nodes[node][‘is_admin’]:
print(node)
“`
3. Networkx Select Nodes by Attribute:
To select nodes based on a specific attribute value, you can use a list comprehension. Here’s an example where we select all nodes with an attribute value of ‘male’:
“`
male_nodes = [node for node in G.nodes() if G.nodes[node][‘gender’] == ‘male’]
print(male_nodes)
“`
4. Networkx Label Nodes:
To label nodes in Networkx, you can utilize the `set_node_attributes()` method. Here’s an example where we set labels for all nodes:
“`
nx.set_node_attributes(G, ‘label’, ‘Unknown’)
“`
5. Networkx Get Nodes Connected to Node:
To get all nodes connected to a specific node, you can use the `neighbors()` method. Here’s an example:
“`
neighbors = list(G.neighbors(1))
print(neighbors)
“`
6. Networkx Draw Nodes:
To visualize nodes using Networkx, you can use various drawing functions provided by the package. Here’s an example that uses the `draw_networkx_nodes()` function:
“`
import matplotlib.pyplot as plt
nx.draw_networkx_nodes(G, pos, node_color=’r’, alpha=0.5)
plt.show()
“`
7. Networkx In-Degree:
To iterate over nodes based on their in-degree, you can use the `in_degree()` method. Here’s an example where we print the nodes sorted by their in-degree:
“`
sorted_nodes = sorted(G.nodes(), key=lambda node: G.in_degree(node), reverse=True)
for node in sorted_nodes:
print(node, G.in_degree(node))
“`
Benefits of Iterating Over Nodes in Networkx
Iterating over nodes in Networkx allows users to perform a wide range of operations and analysis on a graph. Here are some of the key benefits:
1. Data manipulation: Iterating over nodes provides a way to access and modify the attributes associated with the nodes. This flexibility allows for data cleaning, enrichment, and transformation.
2. Analysis and visualization: By iterating over nodes, users can perform various analyses, such as calculating centrality measures, identifying communities, or visualizing the network structure. These analyses can provide valuable insights into the network’s properties and characteristics.
3. Selective processing: Using filters while iterating over nodes enables users to focus on specific subsets of nodes, reducing the computational load and improving the efficiency of the analysis.
4. Customization: Networkx provides a wide range of methods and functions to customize the iteration process. Users can define their own criteria, create custom filters, or apply specific algorithms based on their requirements.
FAQs
Q: Can I iterate over nodes in a directed graph?
A: Yes, Networkx supports iterating over nodes in both undirected and directed graphs.
Q: What happens if a node does not have a specific attribute while iterating?
A: If a node does not have a specific attribute that you are trying to access while iterating, an exception will be raised. To avoid this, you can use the `get()` method or an if statement to check for the existence of the attribute before accessing it.
Q: Can I iterate over nodes in a weighted graph?
A: Yes, the weight of the edges does not affect iterating over nodes. You can still iterate over nodes in a weighted graph using the same methods mentioned earlier.
Q: Are there any performance considerations while iterating over large graphs?
A: When dealing with large graphs, iterating over nodes can become time-consuming. It is recommended to utilize Networkx’s efficient algorithms and data structures to optimize performance. Additionally, applying filters and selecting specific subsets of nodes can help improve efficiency.
Q: How can I iterate over nodes in parallel?
A: Networkx does not provide built-in parallelization for iterating over nodes. However, you can use parallel computing frameworks such as `multiprocessing` or `concurrent.futures` to distribute the workload across multiple processes or threads.
Conclusion
Iterating over nodes in Networkx provides a powerful mechanism to analyze, visualize, and manipulate complex networks. By understanding the methods and applying filters, users can explore the attributes, connections, and properties of individual nodes in a graph. Whether it’s calculating centrality measures, querying specific subsets of nodes, or visualizing the graph structure, Networkx’s support for node iteration empowers users to perform in-depth analysis and gain meaningful insights from network data.
Get Node Degree And Node Neighbors In Python | Networkx Tutorial – Part 04
How Many Nodes Can Networkx Handle?
NetworkX is a widely-used Python package for the creation, manipulation, and study of the structure, dynamics, and functions of complex networks. It provides a comprehensive set of tools for network analysis and allows users to develop their own network algorithms. One of the most common questions about NetworkX is, how many nodes can it handle? In this article, we will explore the capabilities of NetworkX and delve into its handling capacity.
NetworkX is designed to handle networks of various sizes, ranging from small to large-scale networks. The maximum number of nodes that can be handled by NetworkX depends on several factors, including the hardware resources available, memory constraints, and the type of operations performed on the network.
For smaller networks with up to thousands of nodes, NetworkX performs efficiently on most modern hardware. It can handle tasks such as network creation, exploration, and basic analysis with ease. Its intuitive and user-friendly interface makes it accessible to researchers and practitioners from various fields.
As the size of the network grows, NetworkX may encounter certain memory constraints, limiting its performance. The exact threshold at which these constraints come into play may vary depending on the available system resources and the specific tasks being performed. However, NetworkX is continuously being improved and optimized to handle larger networks more efficiently.
In the case of networks with millions or even billions of nodes, NetworkX may not be the most suitable tool due to memory limitations. However, it is worth noting that NetworkX can still handle such large networks with certain trade-offs and by leveraging external libraries or distributed computing platforms.
To handle larger networks, NetworkX can utilize sparse data structures and algorithms, reducing memory requirements. It also supports distributed computing frameworks like Apache Spark or Dask, allowing the analysis of large-scale networks across clusters or cloud platforms. By spreading the computational workload across multiple machines, it becomes possible to overcome memory limitations and process massive networks.
Frequently Asked Questions (FAQs):
Q: Is there a specific limit to the number of nodes that NetworkX can handle?
A: NetworkX does not have an exact hard limit on the number of nodes it can handle. The limit depends on various factors such as available memory and the specific operations being performed.
Q: Can NetworkX handle networks with millions or billions of nodes?
A: While NetworkX may encounter memory limitations with extremely large networks, it can still handle them with certain trade-offs and by utilizing sparse data structures and distributed computing platforms.
Q: What are some alternative tools for handling large-scale networks?
A: For extremely large networks, tools like GraphX (part of Apache Spark) and Dask can be utilized alongside NetworkX. These frameworks leverage distributed computing techniques to process big data networks efficiently.
Q: Can NetworkX be used in conjunction with other Python libraries?
A: Yes, NetworkX is compatible with numerous popular Python libraries. For example, it can integrate with Pandas for data manipulation or Matplotlib for visualizations, enhancing the overall network analysis capabilities.
Q: Is NetworkX suitable for real-time or streaming network analysis?
A: NetworkX is primarily designed for static networks. Real-time or streaming network analysis tasks may require specialized tools like Apache Flink or Apache Kafka, which are specifically built to handle streaming data.
In conclusion, NetworkX is a powerful network analysis package that can handle networks of various sizes efficiently. While it performs exceptionally well with smaller networks, it is also capable of processing larger networks by utilizing sparse data structures and distributed computing frameworks. However, for networks with millions or billions of nodes, alternative tools like GraphX and Dask may be more suitable. With its versatility and compatibility with other Python libraries, NetworkX remains a valuable resource for network analysis and research.
What Are Nodes In Networkx?
NetworkX is a powerful Python package widely used for the creation, manipulation, and study of the structure, dynamics, and functions of complex networks. At the core of this library lies the notion of nodes, which are fundamental building blocks of any network. In this article, we will delve into the world of nodes in NetworkX, exploring their characteristics, functionalities, and how they contribute to network analysis.
Nodes, also known as vertices, represent entities or elements within a network. They can be thought of as individual units or points that are interconnected to form a network. In NetworkX, nodes can have attributes associated with them, enabling us to assign additional information or properties to each node. These attributes can range from simple strings or integers to complex data structures, making NetworkX highly flexible and adaptable to various analysis requirements.
Nodes in NetworkX are represented using unique identifiers. These identifiers, commonly strings or integers, serve as labels that differentiate between different nodes within a network. Labels allow us to identify and refer to specific nodes when performing network operations or analyzing data.
The NetworkX library provides many built-in functions and methods to create, manipulate, and retrieve important information about nodes in a network. Let’s explore some of the vital functionalities of nodes in NetworkX:
1. Creation: NetworkX offers numerous ways to create nodes. You can add individual nodes or a collection of nodes to an existing graph using simple syntax, or generate nodes based on specific patterns using functions such as `path_graph()`, `cycle_graph()`, or `complete_graph()`.
2. Attributes: As mentioned earlier, nodes can have attributes associated with them. These attributes can be accessed, modified, or retrieved using NetworkX methods, allowing for efficient manipulation and analysis of node properties.
3. Neighborhood: Each node in a network is connected to other nodes in some way. NetworkX provides several methods to explore the neighborhood of a node, enabling us to find neighboring nodes or obtain detailed information about the connections between nodes.
4. Degree: The degree of a node refers to the number of edges connected to it. In NetworkX, we can easily determine the degree of a node using the `degree()` function, which returns the calculated value.
5. Visualization: NetworkX offers a range of visualization options to represent nodes and their connections. Using functions like `draw()` or `spring_layout()`, we can plot graphs and gain visual insights into the relationships among nodes.
Now that we have covered the basic functionalities of nodes in NetworkX, let us address some frequently asked questions:
Q1. Can nodes be of different types in NetworkX?
Yes, NetworkX allows nodes to be of any valid Python object type. You can use strings, integers, floats, or even custom-defined classes as nodes.
Q2. What if I want to assign multiple attributes to a single node?
You can assign multiple attributes to a node by storing them in a dictionary and associating the dictionary with the node identifier. This allows for efficient retrieval and modification of attribute values associated with a specific node.
Q3. How can I remove a node from a network in NetworkX?
NetworkX provides the `remove_node()` function to remove a single node from a graph. Additionally, the function `remove_nodes_from()` allows for the removal of multiple nodes specified in a list.
Q4. Can I perform mathematical operations on attributes associated with nodes?
Yes, NetworkX supports arithmetic operations on node attributes. This can be useful when performing computations or calculations related to the network analysis.
Q5. Is it possible to assign a weight or importance measure to nodes in NetworkX?
Yes, NetworkX allows for the assignment of weights to nodes. You can add a `weight` attribute to a node, facilitating the incorporation of node importance or centrality measures within a network.
In conclusion, nodes play a vital role in NetworkX, providing the basic units that form networks. They can be assigned attributes, have unique identifiers, and possess numerous functionalities to analyze and manipulate network data. Understanding nodes and their capabilities in NetworkX enables researchers, data scientists, and network analysts to unravel the complexities of intricate networks and extract valuable insights.
Keywords searched by users: networkx iterate over nodes networkx nodes, networkx iterate over edges, networkx query nodes, networkx select nodes by attribute, networkx label nodes, networkx get nodes connected to node, networkx draw nodes, networkx in-degree
Categories: Top 57 Networkx Iterate Over Nodes
See more here: nhanvietluanvan.com
Networkx Nodes
Nodes, also referred to as vertices, are fundamental entities in networkx. They represent the individual entities or elements of a network. Nodes can be any abstract object such as a person, a place, a computer, a biological molecule, or even an idea. They are connected by edges or links, which denote the relationships or interactions between them. Together, nodes and edges form the structure of a network.
In networkx, nodes can be of any hashable data type, such as integers, strings, or even custom objects. The only constraint is that each node must have a unique identifier. This allows us to easily access and manipulate nodes in the network.
The operations that can be performed on nodes in networkx are vast. We can create a new node, delete an existing node, retrieve the neighbors of a node, check if a node is present in the network, and much more. Let’s explore some of these operations in detail:
1. Adding Nodes: To add a node to a network, we simply use the `add_node` function and specify the node identifier as an argument. For example, `G.add_node(1)` adds a node with the identifier 1 to the network `G`.
2. Removing Nodes: In contrast, to remove a node from a network, we use the `remove_node` function. This function also removes all the edges connected to the node. For instance, `G.remove_node(1)` removes the node with the identifier 1 from the network `G`.
3. Getting Neighbors: Neighbors of a node are the other nodes connected to it by an edge. We can obtain a list of neighbors using the `neighbors` function. For instance, `list(G.neighbors(1))` returns a list of neighbors of the node with the identifier 1 in the network `G`.
4. Checking Node Presence: We can easily check if a node is present in a network using the `has_node` function. It returns `True` if the node is present and `False` otherwise. For example, `G.has_node(1)` checks if the node with the identifier 1 exists in the network `G`.
5. Accessing Node Attributes: Nodes can also have attributes associated with them. These attributes can be accessed and modified using the `node` dictionary. For instance, `G.node[1][‘label’]` retrieves the value of the ‘label’ attribute of the node with identifier 1 in the network `G`.
Now, let’s address some frequently asked questions about networkx nodes:
Q1. Can a node have multiple attributes in networkx?
Yes, a node in networkx can have multiple attributes associated with it. These could include properties like weight, color, or any other relevant information.
Q2. Can nodes be added with custom objects as identifiers?
Yes, networkx allows nodes to be of any hashable data type, including custom objects. As long as the object can be uniquely identified, it can serve as a node identifier.
Q3. Are node identifiers unique within a network?
Yes, each node must have a unique identifier within a network. Duplicating node identifiers can lead to unexpected behavior and errors in networkx.
Q4. Can edges be added or removed directly from nodes?
No, edges are independent entities in networkx, and they can only be added or removed using edge-specific functions. However, nodes can be easily added or removed, along with their associated edges. Adding or removing a node automatically updates the edges connected to it.
Q5. Can networkx handle large networks with numerous nodes?
Yes, networkx is designed to handle large networks efficiently. Nevertheless, for extremely large networks, networkx may not be the most performant option due to memory limitations. In such cases, specialized graph libraries like SNAP or Graph-tool might be more suitable.
As we conclude our exploration of networkx nodes, we have discovered the significance of nodes, their operations, and some commonly asked questions. Networkx provides a rich variety of functionalities and tools to work with nodes and networks as a whole, making it a valuable asset for network analysis and visualization. Whether you are studying social networks, biological networks, or any other complex system, networkx offers a robust and intuitive framework to analyze and understand the underlying structures.
Networkx Iterate Over Edges
To begin, let’s start by understanding what an edge is in the context of a graph. In a network, an edge represents a connection or a relationship between two nodes. For example, in a social network, nodes might represent individuals, and edges could represent friendships between them. In a transportation network, nodes could represent cities, and edges could represent the roads or routes connecting them.
NetworkX provides different methods to iterate over edges in a graph. The most straightforward approach is to use the `edges` method. This method returns a list of tuples, where each tuple represents an edge connecting two nodes. Let’s see an example:
“`python
import networkx as nx
# Create a graph
G = nx.Graph()
G.add_edges_from([(1, 2), (2, 3), (3, 4)])
# Iterate over the edges
for edge in G.edges:
print(edge)
“`
Output:
“`
(1, 2)
(2, 3)
(3, 4)
“`
In this example, we create a simple graph with three edges. By using the `edges` method, we retrieve all the edges of the graph, and then iterate over them using a for loop. Each edge is represented as a tuple of two nodes.
Alternatively, you can use the `iter_edges` method, which returns an iterator instead of a list. This can be useful when working with large graphs, as it avoids loading the entire list of edges into memory. Here’s how you can use the `iter_edges` method:
“`python
for edge in G.edges_iter():
print(edge)
“`
Output:
“`
(1, 2)
(2, 3)
(3, 4)
“`
Both `edges` and `iter_edges` methods return each edge as a tuple of source and target nodes. However, if you want to access the nodes individually, you can do so using tuple unpacking:
“`python
for source, target in G.edges:
print(source, target)
“`
Output:
“`
1 2
2 3
3 4
“`
Now let’s move on to the FAQs section to address some common questions about iterating over edges in NetworkX:
#### Q1: Can I iterate over edges with additional edge attributes?
Yes, you can. If your graph contains extra information associated with each edge, such as weight or distance, you can access these attributes while iterating over the edges. For example:
“`python
G.add_edge(1, 2, weight=3)
G.add_edge(2, 3, weight=4)
G.add_edge(3, 4, weight=5)
for source, target, attributes in G.edges(data=True):
weight = attributes[‘weight’]
print(source, target, weight)
“`
Output:
“`
1 2 3
2 3 4
3 4 5
“`
By passing `data=True` to the `edges` method, you can access the attributes of each edge as a dictionary.
#### Q2: How can I iterate over the outgoing and incoming edges of a specific node?
You can use the `out_edges` and `in_edges` methods to iterate over the outgoing and incoming edges of a node, respectively. Here’s an example:
“`python
for edge in G.out_edges(2):
print(edge)
“`
Output:
“`
(2, 1)
(2, 3)
“`
For the incoming edges:
“`python
for edge in G.in_edges(2):
print(edge)
“`
Output:
“`
(1, 2)
(3, 2)
“`
In both cases, the `out_edges` and `in_edges` methods return all the edges where the specified node is the source or target, respectively.
In conclusion, iterating over edges is a common task when working with network analysis and NetworkX provides convenient methods to accomplish this. By using the `edges`, `iter_edges`, or the `out_edges`/`in_edges` methods, you can easily access the edges of a graph in various formats. Additionally, you can take advantage of edge attributes to process extra information associated with each edge.
Images related to the topic networkx iterate over nodes
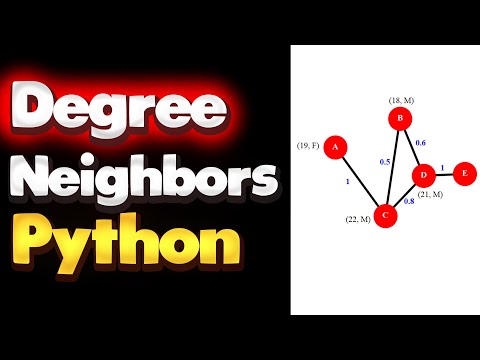
Found 39 images related to networkx iterate over nodes theme







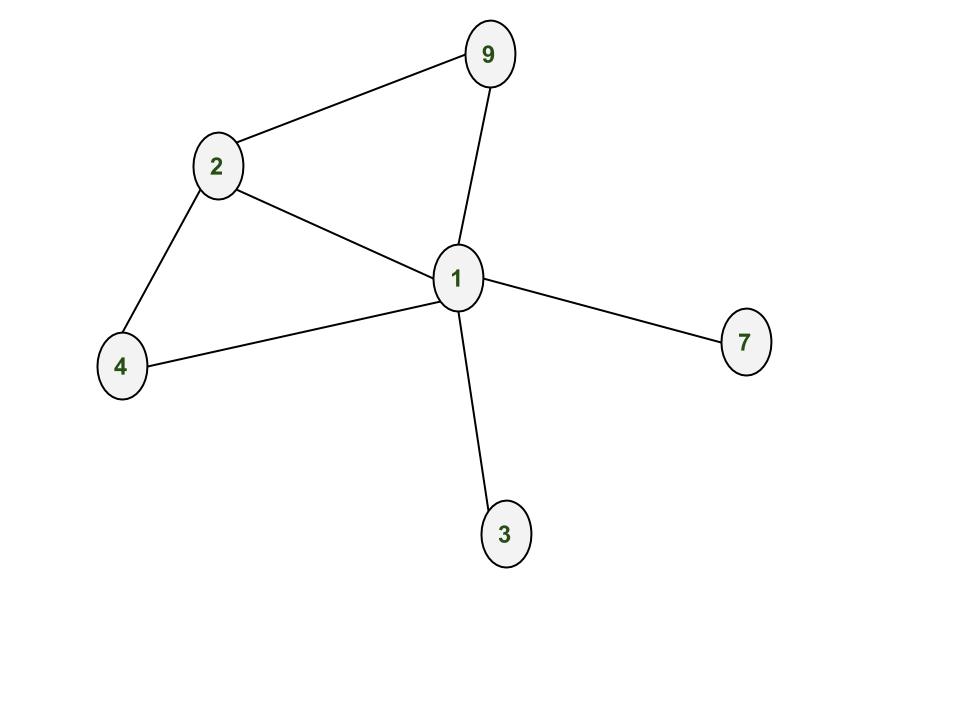

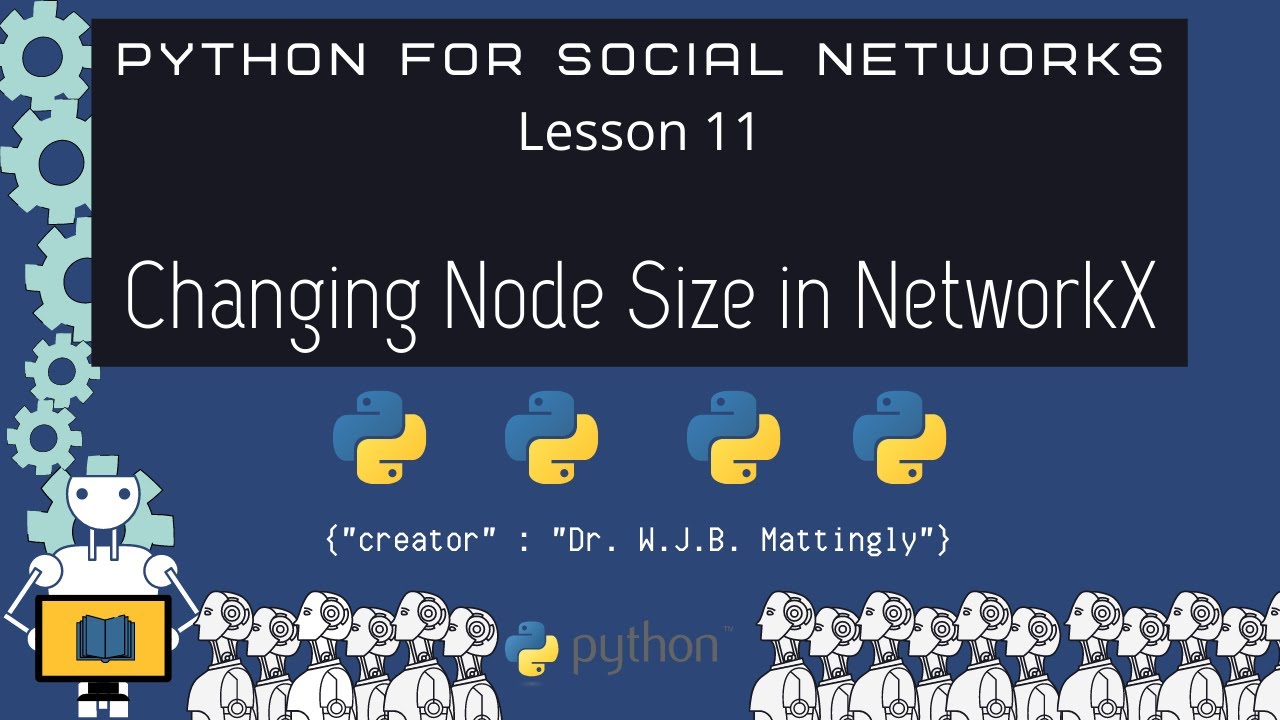
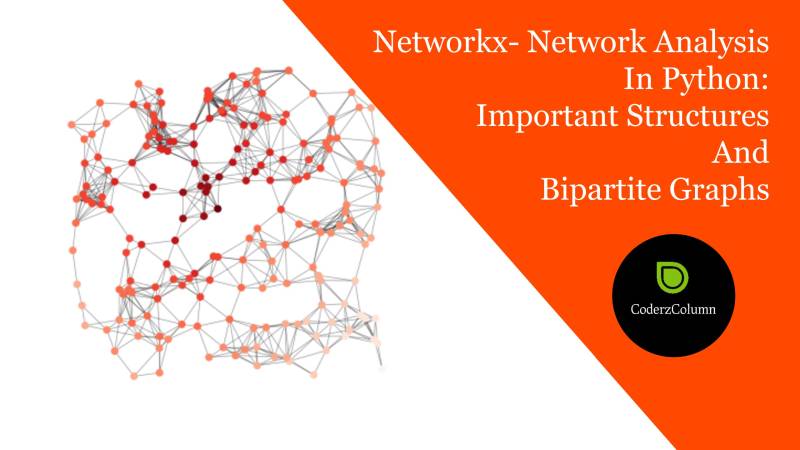

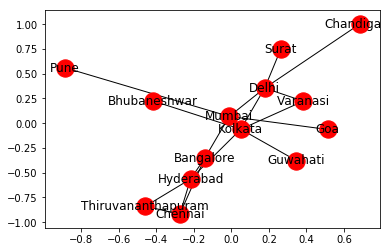

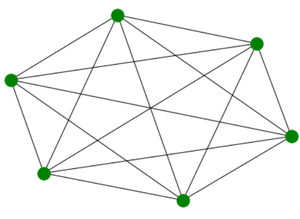






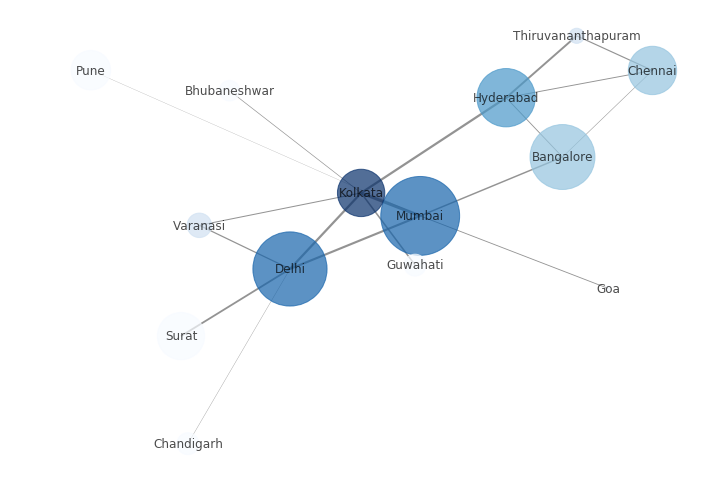

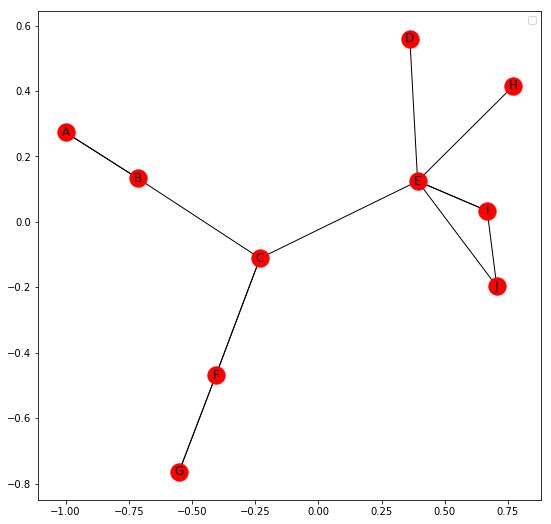

Article link: networkx iterate over nodes.
Learn more about the topic networkx iterate over nodes.
- looping through nodes and extract attributes in Networkx
- Create nodes and edges with attributes and iterate over them
- Chapter 2: The NetworkX API – Network Analysis Made Simple
- Iterating over nodes | oknoname.com
- Working With Large Internal Link Graphs in Python – Briggsby
- Tutorial — NetworkX 3.1 documentation
- NetworkX: Network Analysis with Python – University of Cambridge
- Benchmark of popular graph/network packages – Quasilinear Musings
- Attribute basics | Memgraph’s Guide for NetworkX library
- NetworkX Cheat Sheet by gonz95alo – Cheatography.com
- networkx – Nodes and Edges – | notebook.community
See more: blog https://nhanvietluanvan.com/luat-hoc