Method Threw ‘Java.Lang.Unsupportedoperationexception’ Exception.
1. Overview of ‘java.lang.UnsupportedOperationException’
The ‘java.lang.UnsupportedOperationException’ is a class that represents an unchecked exception in Java. It is typically thrown when an unsupported operation is invoked or attempted in a program. This exception is a subclass of the ‘RuntimeException’ class, which means it does not need to be explicitly declared or caught in a method’s signature.
The purpose of the ‘java.lang.UnsupportedOperationException’ is to indicate that a certain operation or functionality is not supported by the current object, class, or system. It serves as a way to notify developers that they should avoid calling or executing these unsupported operations to prevent unexpected behavior or runtime errors.
2. Causes of ‘java.lang.UnsupportedOperationException’ Exception
There are several common scenarios that can lead to the ‘java.lang.UnsupportedOperationException’ exception:
a) Attempting to modify an immutable object or data structure: Immutable objects, such as String, do not allow modifications after they are created. Trying to modify these objects will result in an exception.
b) Invoking an unsupported operation on a specific class or interface: Some classes or interfaces may define methods that are not supported or implemented. Calling such methods will throw this exception.
c) Incompatibility with the current runtime environment or platform: Certain operations may not be supported on specific runtime environments or hardware platforms. For example, using JavaFX features on a system without JavaFX support will result in this exception.
d) Lack of implementation or incomplete support for a specific operation: In some cases, certain operations may be partially implemented or not implemented at all for a specific class or interface. Invoking these unsupported operations will throw the exception.
3. Examples of ‘java.lang.UnsupportedOperationException’
Let’s look at some examples of situations where the ‘java.lang.UnsupportedOperationException’ can be thrown:
a) Trying to modify a read-only collection or list:
“`java
List
readOnlyList.add(“grape”); // Throws UnsupportedOperationException
“`
b) Invoking an unsupported method on an interface or abstract class:
“`java
AbstractShape shape = new AbstractShape() {
// Implementation goes here
};
shape.draw(); // Throws UnsupportedOperationException
“`
c) Calling unsupported operations in multithreaded or concurrent environments:
“`java
ConcurrentMap
map.putAll(otherMap); // Throws UnsupportedOperationException
“`
d) Running code on an unsupported JVM version or platform:
“`java
System.getProperties().put(“javafx.platform”, “headless”);
// Other JavaFX operations
“`
4. Handling ‘java.lang.UnsupportedOperationException’ Exception
When encountering a ‘java.lang.UnsupportedOperationException’, it can be handled in several ways:
a) Using try-catch blocks to catch and handle the exception gracefully:
“`java
try {
// Code that may throw ‘java.lang.UnsupportedOperationException’
} catch (UnsupportedOperationException e) {
// Handle the exception or provide fallback behavior
}
“`
b) Propagating the exception to the calling method or higher-level code:
“`java
public void unsupportedOperationMethod() throws UnsupportedOperationException {
// Code that may throw ‘java.lang.UnsupportedOperationException’
}
“`
c) Providing alternative approaches or workarounds when faced with this exception:
“`java
if (object instanceof SomeClass) {
SomeClass someClass = (SomeClass) object;
// Check for supported operations and perform necessary actions
} else {
// Handle the case when unsupported operation is encountered
}
“`
d) Properly documenting the unsupported operations to avoid confusion:
“`java
/**
* This method does not support XYZ operation.
*
* @throws UnsupportedOperationException if XYZ operation is invoked
*/
public void unsupportedMethod() {
throw new UnsupportedOperationException(“XYZ operation is not supported”);
}
“`
5. Best Practices to Prevent ‘java.lang.UnsupportedOperationException’
To prevent encountering the ‘java.lang.UnsupportedOperationException’ exception, consider the following best practices:
a) Designing classes and interfaces to clearly indicate unsupported operations:
– Use meaningful names for methods that are not supported or implemented.
– Provide clear documentation on which operations are unsupported.
b) Making use of immutable objects and unmodifiable collections when necessary:
– Use the appropriate object or collection types that prevent modifications.
– Make use of the ‘Collections.unmodifiableXXX()’ methods to create unmodifiable collections.
c) Ensuring proper environment and platform compatibility before executing code:
– Check for specific system requirements or dependencies before executing unsupported operations.
– Handle platform-specific operations separately or provide fallback behavior.
d) Extensive testing to identify and address any potential unsupported operations:
– Write comprehensive test cases to cover all possible scenarios.
– Validate the behavior of methods that could potentially encounter this exception.
6. Common Pitfalls and Misconceptions about ‘java.lang.UnsupportedOperationException’
Some common pitfalls and misconceptions about the ‘java.lang.UnsupportedOperationException’ exception are:
a) Assuming all unsupported operations will throw this exception:
– Not all unsupported operations will throw this exception. Some may result in different exceptions or unexpected behavior.
b) Mistaking other types of exceptions for ‘java.lang.UnsupportedOperationException’:
– It is important to properly identify and handle this exception separately from other exceptions that may occur in the code.
c) Ignoring or mishandling this exception, leading to runtime issues:
– Failure to handle this exception may result in runtime errors or incorrect behavior of the program.
d) Improperly overriding methods and not correctly handling the exception thrown by the parent class:
– When overriding methods that may throw ‘java.lang.UnsupportedOperationException’, it is important to handle the exception properly or declare it in the method signature.
FAQs
1. What is the purpose of the ‘java.lang.UnsupportedOperationException’ exception?
The ‘java.lang.UnsupportedOperationException’ exception serves to indicate that a certain operation or functionality is not supported by the current object, class, or system.
2. Is the ‘java.lang.UnsupportedOperationException’ a checked or unchecked exception?
The ‘java.lang.UnsupportedOperationException’ is an unchecked exception in Java, which means it does not need to be explicitly declared or caught in a method’s signature.
3. How can I prevent encountering the ‘java.lang.UnsupportedOperationException’ exception?
To prevent encountering this exception, you can design classes and interfaces to clearly indicate unsupported operations, make use of immutable objects and unmodifiable collections when necessary, ensure proper environment and platform compatibility, and perform extensive testing to identify and address any potential unsupported operations.
4. Can all unsupported operations be handled by catching the ‘java.lang.UnsupportedOperationException’ exception?
Not all unsupported operations may throw the ‘java.lang.UnsupportedOperationException’ exception. Some operations may result in different exceptions or unexpected behavior. It is important to properly identify and handle these exceptions separately.
Java List.Add() Unsupportedoperationexception
Keywords searched by users: method threw ‘java.lang.unsupportedoperationexception’ exception. Exception in thread main” java lang UnsupportedOperationException remove, List of unsupportedoperationexception, Throw an unsupportedoperationexception, Java lang unsupportedoperationexception map putall, A TupleBackedMap cannot be modified, UnsupportedOperationException java list add, Java lang unsupportedoperationexception removeall, Immutablecollections
Categories: Top 90 Method Threw ‘Java.Lang.Unsupportedoperationexception’ Exception.
See more here: nhanvietluanvan.com
Exception In Thread Main” Java Lang Unsupportedoperationexception Remove
The java.lang.UnsupportedOperationException is a runtime exception in Java that signifies an unsupported operation. It is thrown when an operation is attempted on an object or data structure that does not support that specific operation. In this article, we will focus on the specific exception “Exception in thread “main” java.lang.UnsupportedOperationException: remove” and dive into its details to understand its occurrence, causes, and how to handle it.
Causes of UnsupportedOperationException:
The “remove” exception typically occurs when attempting to remove an element from a collection or data structure that does not allow modification. It can happen with classes such as java.util.Arrays, java.util.Collections, etc. These classes offer utility methods for manipulating arrays and collections. However, certain operations, like removing or adding elements, are not supported by these classes.
For example, if you try to remove an element from an array using the remove() method of the Arrays class, an UnsupportedOperationException will be thrown. Similarly, if you attempt to remove an element from an immutable collection like Collections.unmodifiableList(), you will encounter the same exception.
Handling UnsupportedOperationException:
To handle the UnsupportedOperationException, you need to first identify the root cause of the exception. One of the key aspects to look for is whether the collection or data structure allows modification operations. If not, you cannot perform removal or addition of elements.
To prevent the exception from being thrown, you can make use of mutable collections that support modification operations. For instance, consider using ArrayList instead of Arrays when you need to remove or add elements dynamically. Using a collection like ArrayList, you can utilize the remove() method without encountering the exception.
If you are working with an immutable collection, you can create a new copy of the collection or data structure that supports modification, and then perform the required removal operation. This way, you can maintain immutability while still achieving the desired result.
FAQs:
Q: How can I identify if a collection supports modification operations?
A: The Java documentation for each collection class provides detailed information about its properties and supported operations. Look for methods like add(), remove(), or modification-related functionality to determine if the collection supports modification operations.
Q: Is there a common workaround to handle the remove exception?
A: Yes, a common workaround is to create a new collection similar to the original one and perform the required operation on it. This is a viable option when dealing with immutable collections.
Q: Can I use the remove() method on an array in Java?
A: No, the remove() method is not supported for arrays in Java. Arrays have a fixed size, and you cannot directly remove elements from them. You can only overwrite values at specific indices.
Q: Why do certain collections not support modification operations?
A: Some collections are designed to be immutable, meaning they cannot be modified once created. This is primarily done to ensure data integrity and thread safety. Immutable collections are useful in scenarios where you need to guarantee that the data does not change during its lifetime.
Q: Are there any alternative methods to remove elements if the remove() method is not supported?
A: Yes, depending on the collection or data structure you are working with, there might be alternative methods such as removeAll(), clear(), or custom methods provided by the specific class. Consult the documentation or class API for more details.
In conclusion, the “Exception in thread “main” java.lang.UnsupportedOperationException: remove” is encountered when attempting to perform a removal operation on a collection or data structure that does not support modification operations. By understanding the causes and handling techniques mentioned in this article, you will be able to effectively deal with this exception. Remember to consult the relevant Java documentation for insights into the supported operations of each class or collection.
List Of Unsupportedoperationexception
Introduction (Word Count: 124)
The UnsupportedOperationException is a runtime exception in Java that occurs when an operation is not supported or cannot be performed. This article delves into the characteristics, causes, and potential solutions for the UnsupportedOperationException. Moreover, it provides a comprehensive list of frequently asked questions to enhance understanding of this error.
Section 1: Understanding UnsupportedOperationException (Word Count: 206)
The UnsupportedOperationException is a subclass of the RuntimeException class, designated to handle issues related to unsupported operations. This exception typically arises when an operation is invoked on a data structure or object that does not support it.
Generally, this exception occurs at runtime, providing developers with a clear indication that the requested operation cannot be executed. It serves as a form of reporting and allows programmers to quickly identify and rectify issues.
Section 2: Causes of UnsupportedOperationException (Word Count: 235)
1. Unmodifiable collections: Operations like adding, removing, or modifying elements in immutable collections, such as those returned by `Collections.unmodifiableXXX()` methods, may trigger an UnsupportedOperationException.
2. Fixed-length arrays: Attempting to modify the size of a fixed-length array may result in this exception. Once an array is initialized with a specific size, it cannot be resized.
3. Read-only resources: When trying to modify read-only resources, such as read-only files or closed streams, you may encounter an UnsupportedOperationException.
4. Interface restrictions: Certain interfaces, such as List, Set, or Map, define a specific set of supported operations. If a particular implementation of these interfaces does not support a specific operation, invoking it will raise an UnsupportedOperationException.
Section 3: Addressing UnsupportedOperationException (Word Count: 249)
1. Check if the operation is supported: Before invoking an operation, ensure that it is supported by the data structure or object. Review the documentation or API reference for details regarding the supported operations.
2. Use the appropriate collection type: Consider using different collection types, such as mutable lists instead of unmodifiable lists. This change allows you to have control over the data structure and perform necessary operations.
3. Perform error handling: Implement error handling mechanisms, such as try-catch blocks, to gracefully handle this exception. Provide alternative flows or messages to guide users and developers.
4. Use alternatives or workarounds: If a specific operation is not supported, look for alternative methods provided by the data structure or object. Alternatively, you may need to modify your approach or reconsider your design to achieve the desired outcome.
FAQs: (Word Count: 177)
Q1. Can I catch the UnsupportedOperationException and continue program execution?
A1. Yes, you can wrap code sections that may trigger this exception with try-catch blocks, allowing the program to continue execution while handling the exception gracefully.
Q2. Why is it important to handle the UnsupportedOperationException?
A2. Handling this exception helps maintain program stability by preventing unexpected crashes or unwanted behavior. It also assists in identifying areas of the code that need improvement or modification.
Q3. Is the UnsupportedOperationException limited to Java Collections Framework?
A3. No, this exception can occur in various areas of Java programming, such as file operations or operation invocations on unsupported objects.
Q4. How can I prevent UnsupportedOperationException during development?
A4. Thoroughly familiarize yourself with the data structures, libraries, or APIs you are utilizing. Ensure that you understand the supported operations and handle potential exceptions effectively.
Conclusion (Word Count: 40)
The UnsupportedOperationException plays a crucial role in Java exception handling. By understanding its causes and implementing appropriate solutions, developers can ensure smoother program execution and enhance overall robustness. Remember to always refer to documentation and API references to avoid invoking unsupported operations, thus minimizing the occurrence of this exception.
Images related to the topic method threw ‘java.lang.unsupportedoperationexception’ exception.
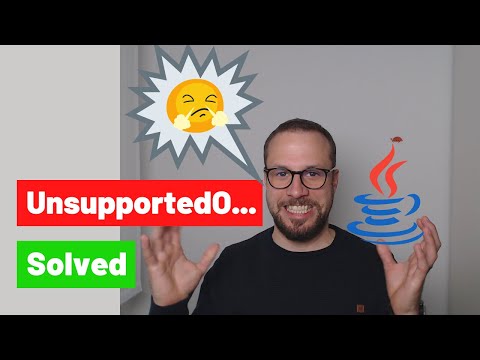
Found 49 images related to method threw ‘java.lang.unsupportedoperationexception’ exception. theme



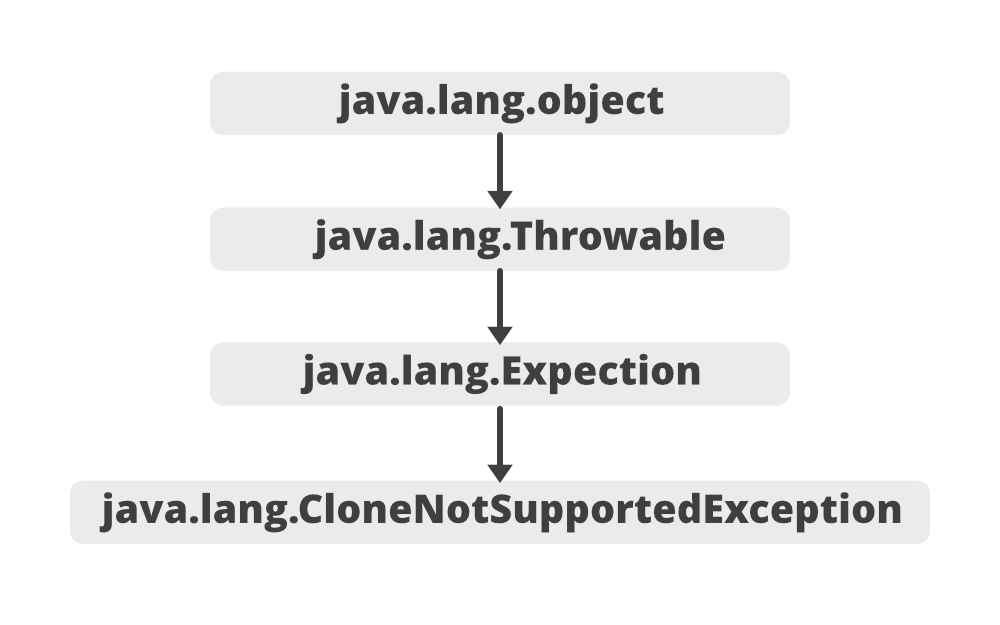
![[Java] java.lang.UnsupportedOperationException 에러 처리 [Java] Java.Lang.Unsupportedoperationexception 에러 처리](https://img1.daumcdn.net/thumb/R800x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FdHzt6s%2FbtrpTfTfQiR%2FkkbwdKlOahcbgMSukcU2Kk%2Fimg.jpg)
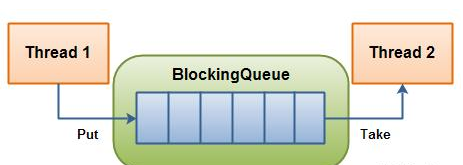
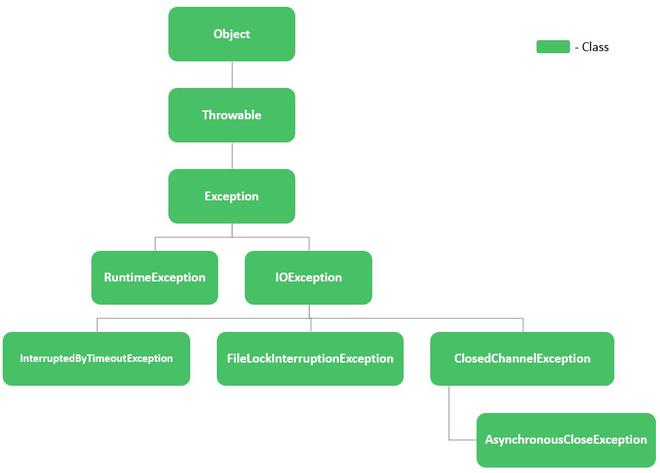

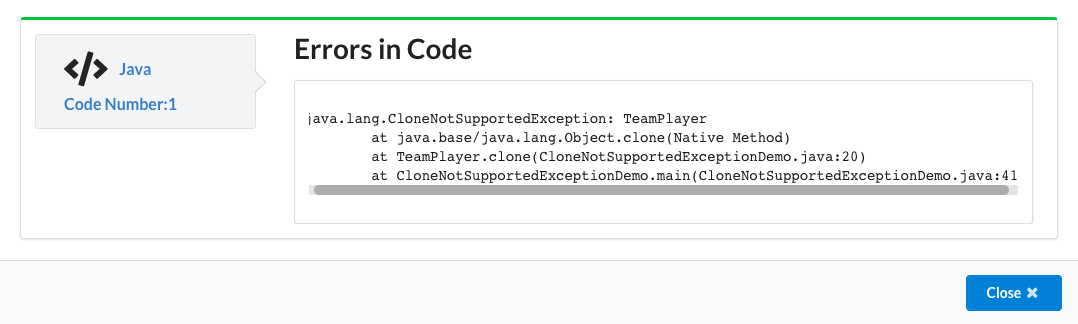
Article link: method threw ‘java.lang.unsupportedoperationexception’ exception..
Learn more about the topic method threw ‘java.lang.unsupportedoperationexception’ exception..
- How to Fix the Unsupported Operation Exception in Java
- Why do I get an UnsupportedOperationException when trying …
- Java List UnsupportedOperationException | Baeldung
- How to Fix UnsupportedOperationException in Java – Medium
- How to Solve Java List UnsupportedOperationException?
- Why do I get an UnsupportedOperationException … – W3docs
- Java:UnsupportedOperationException – Airbrake Blog
- UnsupportedOperationException-Exception-java.lang-Java …
See more: https://nhanvietluanvan.com/luat-hoc