Max Value Of Long In Java
## 1. Understanding the basics
In Java, long is a primitive data type used to store whole numbers. It is a 64-bit signed two’s complement integer, which means it can represent both positive and negative numbers.
## 2. Signed long and unsigned long
Unlike some other programming languages, Java does not have a built-in unsigned long data type. However, Java does provide support for unsigned operations on long values using some bitwise operators.
## 3. Range of values for long
A signed long in Java has a range of values from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 (inclusive). These values can be represented using 64 bits of memory.
## 4. Calculating the maximum value of long
The maximum value of a signed long can be calculated using the formula (2^63) – 1.
In Java, we can use the constant `Long.MAX_VALUE`to represent the maximum value of a long.
## 5. Explanation of the maximum value
The maximum value of long in Java is 9,223,372,036,854,775,807. This number is the result of using a 64-bit representation and employing two’s complement arithmetic. The leftmost bit is reserved for the sign, leaving 63 bits to represent the actual value.
The sign bit is set to zero for positive numbers, while it is set to one for negative numbers. Hence, the maximum positive value that can be represented is when all 63 bits are set to one.
## 6. Comparing long with other data types
When it comes to representing large integer values, long is the largest standard data type provided by Java. It can hold values significantly larger than other primitive data types like int or byte.
The range of values that can be stored in a long is much bigger than what int can accommodate. An int can store values from -2,147,483,648 to 2,147,483,647, while a long can store values much larger than that.
Comparing long with floating-point data types like Double, the latter can store decimal values with higher precision, but it has a smaller range of values compared to long.
## 7. Potential issues with long and excessive values
While long can store large integer values, care must be taken when handling extremely large numbers. Performing arithmetic operations or storing such values in memory can result in performance issues due to the increased storage requirements and computational complexity.
Additionally, long data type has a fixed size of 64 bits, which means it occupies more memory compared to smaller data types. This can be a concern if memory optimization is necessary.
## 8. Alternatives to long for larger values
If the range of signed long is insufficient for a particular use case, Java provides alternative data types. The BigInteger class is a flexible solution that can handle arbitrarily large integers. It comes with built-in arithmetic and comparison operations suitable for large numbers.
In cases where decimal values need to be represented with high precision, the BigDecimal class is more appropriate. BigDecimal provides arbitrary-precision decimal arithmetic, allowing precise calculations with high accuracy.
## 9. Practical applications and considerations of long in Java
The long data type finds applications in scenarios where a larger range of integer values is required. Some areas where long is commonly used include:
– Storing Unix timestamps, which represent the number of seconds since January 1, 1970. This allows for easy manipulation and conversion of date/time values.
– Calculating durations or time differences that exceed the range of int.
– Handling large numerical calculations, such as computing factors of large numbers or performing cryptographic operations.
### FAQs
Q1: What is the maximum value of int in Java?
A1: The maximum value of int in Java is 2,147,483,647. This can be represented using 32 bits of memory.
Q2: What is the maximum value of BigDecimal in Java?
A2: The maximum value of BigDecimal in Java is implementation-dependent. It can vary based on factors like available memory and processing power.
Q3: Is there a maximum value for BigInteger in Java?
A3: No, there is no theoretical maximum value for BigInteger in Java. It can handle arbitrarily large integers limited only by available memory.
Q4: What is the maximum value of Double in Java?
A4: The maximum finite value of Double in Java can be represented by the constant `Double.MAX_VALUE`. It is approximately 1.8 x 10^308.
Q5: How to initialize a long variable in Java?
A5: A long variable can be initialized using the `L` suffix after the value. For example, `long myVariable = 123456789L;`
In conclusion, understanding the maximum value and practical aspects of long in Java is crucial for writing efficient and reliable code. By considering alternatives and using the appropriate data type for specific use cases, developers can handle large number calculations effectively and avoid potential issues.
Java Tutorial – 03 – Search For The Max And Min Value Of An Array
Keywords searched by users: max value of long in java Integer max value Java, BigDecimal max value, BigInteger max value, Double max value Java, Integer max_value trong Java, Int64 max value, Byte() in Java, Double value in Java
Categories: Top 66 Max Value Of Long In Java
See more here: nhanvietluanvan.com
Integer Max Value Java
In Java, the Integer Max Value refers to the largest integer value that can be represented using the Integer class, which is a wrapper class for the primitive data type `int`. This value is predefined and can be accessed using the constant field `Integer.MAX_VALUE`. Understanding and utilizing the Integer Max Value is essential when dealing with integer data types in Java programming. In this article, we will explore the details of the Integer Max Value, its significance, and how it can be effectively utilized in Java.
Understanding Integer Max Value
The Integer Max Value represents the maximum value that can be stored in a variable of the `int` data type. The `int` data type in Java is a signed 32-bit integer, which means it can hold both positive and negative values. The Integer Max Value is determined by the range of bits that an `int` variable can hold.
The Integer Max Value is defined as 2^31 – 1, which is equivalent to 2147483647. This means that any value greater than this will cause an overflow, resulting in unexpected behavior or errors in the program. It is important to keep this constraint in mind to avoid unexpected results when working with large integer values.
Utilizing Integer Max Value
The Integer Max Value has several practical applications in Java programming. Some common use cases include:
1. Looping: When implementing loops such as `for` or `while`, the Integer Max Value can be used as a limiting condition to execute the loop until a certain limit is reached. For example:
“`java
for (int i = 1; i <= Integer.MAX_VALUE; i++) {
// Execute loop logic
}
```
2. Default values: When initializing variables that hold integer values, assigning them the Integer Max Value can be useful as a placeholder for uninitialized or default values. This ensures that the variable holds a value that is distinguishable from other meaningful values.
3. Array indexing: The Integer Max Value can be used as an index to represent the end of an array when iterating over its elements. This ensures that the loop terminates correctly without going beyond the array's bounds. For example:
```java
int[] array = new int[10];
for (int i = 0; i < Integer.MAX_VALUE; i++) {
// Access array elements
}
```
4. Error handling: The Integer Max Value can be used to compare and validate user inputs that involve integer values. For instance, if a user is asked to provide a positive integer within a certain range, the input can be checked against the Integer Max Value to ensure it falls within the valid range.
Frequently Asked Questions (FAQs)
Q: How do I use the Integer Max Value to check if a value exceeds the maximum range?
A: To check if a value exceeds the maximum range, compare it with the Integer Max Value using the greater than operator (`>`). If the value is greater than `Integer.MAX_VALUE`, it exceeds the range.
Q: Can the Integer Max Value be used for long data types as well?
A: No, the Integer Max Value is specific to the `int` data type in Java. To handle larger integer values, you should use the `long` data type, which has its own maximum value defined by the Long class.
Q: What happens if I assign a value greater than the Integer Max Value to an integer variable?
A: When a value greater than the Integer Max Value is assigned to an integer variable, an overflow occurs. This means that the value wraps around to the negative range and continues from the negative minimum value (Integer.MIN_VALUE).
Q: Can the Integer Max Value be negative?
A: No, the Integer Max Value represents the largest positive value that can be held by an `int` data type. If you need to store negative values, you can use the Integer Min Value, which represents the largest negative value (-2^31).
In conclusion, the Integer Max Value in Java is a predefined constant that represents the largest positive integer value that can be held by the `int` data type. By understanding and utilizing this value effectively, developers can ensure proper handling of integer values and prevent unexpected errors or overflows in their programs. Remember to always validate user inputs and keep the Integer Max Value constraint in mind when dealing with integer data types in Java programming.
Bigdecimal Max Value
BigDecimal is part of the Java.math package and offers a convenient solution for representing and performing arithmetic operations on numbers with arbitrary precision. It provides the ability to handle numbers with a large number of digits and retain accurate results, making it indispensable in financial, scientific, and mathematical applications.
Understanding BigDecimal Max Value:
In Java, BigDecimal is implemented as an immutable object, meaning its value cannot be changed after it is created. This characteristic allows it to hold exceptionally large values without loss of precision. However, every data type, including BigDecimal, has its limits defined by the hardware and programming environment.
The BigDecimal class in Java establishes certain bounds on the values it can hold. For instance, it has a maximum value, which is the largest number that can be stored in the BigDecimal object. The max value of BigDecimal can be accessed through the constant BigDecimal.MAX_VALUE. This constant is defined as a pre-instantiated BigDecimal object with the maximum possible value.
The max value of BigDecimal is theoretically infinite. However, it is practically limited by the memory available, as the size of the value increases with more digits and requires more memory to store. Additionally, the capacity of the hardware and programming environment play a role in determining the maximum value of a BigDecimal that can be reliably handled.
As of Java 8, BigDecimal’s max value is expressed using a scale of 2147483647. The scale of a BigDecimal defines the number of digits to the right of the decimal point. This scale requirement ensures that the value can be accurately represented and manipulated. It is important to note that the max value of BigDecimal is not limited by the scale but by the overall size limitation.
FAQs:
Q: Why would I need to use BigDecimal’s max value in my projects?
A: BigDecimal’s max value is particularly useful when dealing with financial calculations or scenarios where precision is crucial. It allows you to accurately preserve and manipulate large numbers without losing precision. For example, when working with monetary values or scientific computations, using BigDecimal can prevent rounding errors and ensure accurate results.
Q: How do I represent a number larger than the BigDecimal’s max value?
A: BigDecimal’s max value represents a significantly large number already. However, if you require a representation of a number that surpasses BigDecimal’s max value, you might need to consider alternative libraries or custom implementations, such as utilizing arrays or other data structures to represent and manipulate numbers.
Q: How does BigDecimal differ from other numerical types, such as int or double?
A: Unlike int or double, BigDecimal offers arbitrary precision by allowing for an arbitrary number of digits to be stored. Integers and floating-point numbers have fixed sizes and therefore have limited ranges and precision. BigDecimal provides more accurate and reliable results in scenarios that demand high precision, such as financial calculations or scientific modeling.
Q: Can I perform arithmetic operations on BigDecimal values beyond the max value?
A: The arithmetic operations provided by the BigDecimal class support calculations on numbers regardless of their magnitude, up to the memory limitations of the system. You can add, subtract, multiply, divide, and perform other mathematical operations using BigDecimal objects, even if their values exceed the max value. However, it is important to consider the computational resources required when working with extremely large numbers.
Q: Are there any performance considerations when using BigDecimal?
A: BigDecimal operations can be slower compared to primitive numerical types due to the additional memory and processing requirements for arbitrary precision calculations. However, the trade-off is the assurance of accurate and precise results. When working with BigDecimal, it is advisable to optimize your code by minimizing unnecessary operations and being mindful of the potential impact on performance.
In conclusion, BigDecimal’s max value is a crucial aspect in programming when dealing with large and precise numbers. It enables accurate representation, manipulation, and storage of vast numerical values, making it an indispensable component in financial, scientific, and mathematical applications. By understanding its limitations and proper usage, programmers can harness the power of BigDecimal to handle complex calculations with confidence.
Biginteger Max Value
In the realm of computer programming, dealing with large numbers often poses a significant challenge. Most programming languages have specific data types to handle integers within a predefined range. However, when the numbers exceed these bounds, developers must turn to alternative solutions. One such solution is the BigInteger data type, which allows manipulation of arbitrarily large integers. In this article, we will focus on exploring the concept of BigInteger max value, its significance, and its applications.
What is BigInteger?
In most programming languages, integer data types have fixed size limits, such as 32 or 64 bits, which determine the maximum and minimum values they can hold. These limitations confine the range of numbers that can be effortlessly dealt with. BigInteger, on the other hand, is a data type used to represent integers of practically unlimited size. It provides an efficient means to perform arithmetic operations involving enormous numbers.
Java’s BigInteger Class:
Java, being an object-oriented programming language, provides a built-in class called BigInteger to handle arbitrary precision integers. This class encompasses a wide range of methods to perform operations like addition, subtraction, multiplication, division, and more, on exceedingly large numbers.
BigInteger Max Value:
The BigInteger class in Java does not have a maximum predefined value. It can handle numbers of any magnitude that fits into your computer’s memory. In essence, BigInteger empowers programmers to work with integers that would otherwise be impossible to represent using conventional integer data types.
Internally, BigInteger uses an array of integers to store the digits of a number. Since the size of the array is dynamic, it can grow or shrink based on the requirements. Consequently, the maximum value supported by BigInteger is constrained only by the amount of memory available.
Significance of BigInteger Max Value:
The significance of BigInteger max value becomes apparent when dealing with scenarios that involve computations requiring precision and vast numerical scales. Some areas where BigInteger finds applications include:
1. Cryptography: Cryptographic algorithms often involve operations on large prime numbers, which can easily exceed the limits of conventional integer data types. BigInteger enables secure encryption and decryption processes by allowing the manipulation of such large numbers.
2. Financial Calculations: Financial calculations, particularly those dealing with interest rates and large sums of money, may require handling extremely large numbers. BigInteger provides a reliable means of performing precise calculations in such contexts.
3. Scientific Research: Scientific experiments and calculations may involve massive datasets, requiring the usage of numbers beyond the boundaries of regular integer data types. BigInteger facilitates accurate representation and computation of such numbers, leading to precise scientific results.
4. Game Development: Some games involve complex algorithms that handle very large numbers. For instance, randomly generating large prime numbers for cryptographic puzzles in a game may require the usage of BigInteger.
Frequently Asked Questions (FAQs):
Q: Can a BigInteger hold all possible values?
A: No, a BigInteger cannot hold all possible values as it is limited by the amount of memory available on your system. However, it allows for significantly larger numbers than conventional integer data types.
Q: How do I find the maximum BigInteger value?
A: Since the maximum value is determined by the memory available, there is no predefined maximum value for a BigInteger in Java. You can operate with numbers limited only by the memory resources of your system.
Q: Are there any performance implications when using BigInteger?
A: BigInteger operations are more computationally expensive compared to regular integer operations due to their arbitrary precision nature. Therefore, using BigInteger in performance-critical areas should be done judiciously.
Q: Can I convert a BigInteger to a primitive data type?
A: Yes, BigInteger has methods to convert its value to other numerical data types, such as int or long, but be aware that truncation or rounding errors may occur if the BigInteger value is beyond the range of the target type.
Q: Are other programming languages offering the BigInteger data type?
A: Yes, many programming languages, including C#, Python, and JavaScript, offer their own implementations of arbitrary-precision arithmetic, either natively or through libraries.
In conclusion, BigInteger is a valuable tool for programmers grappling with the limitations of conventional integer data types. By enabling the manipulation of arbitrarily large integers, BigInteger expands the boundaries of arithmetic, making it possible to perform precise calculations involving enormous numbers. Its applications in cryptography, finance, science, and gaming underscore its significance in various domains. With no predefined max value, BigInteger allows programmers to push the boundaries of arithmetic operations, limited only by the available memory resources.
Images related to the topic max value of long in java
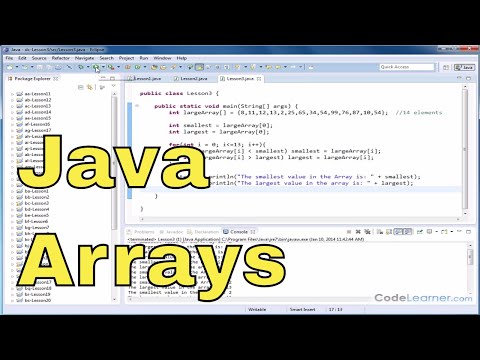
Found 29 images related to max value of long in java theme
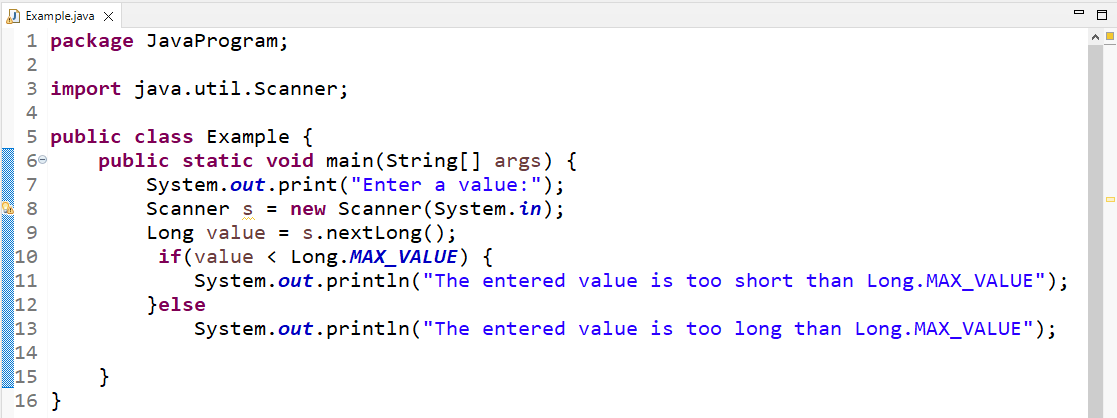


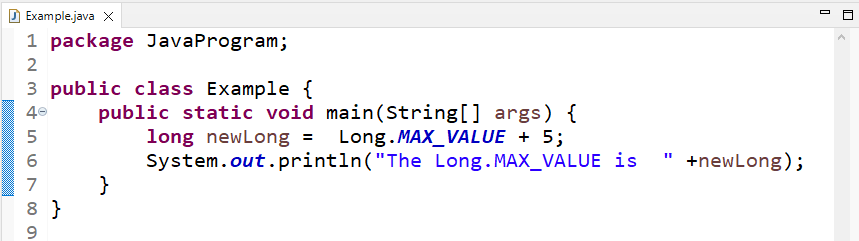
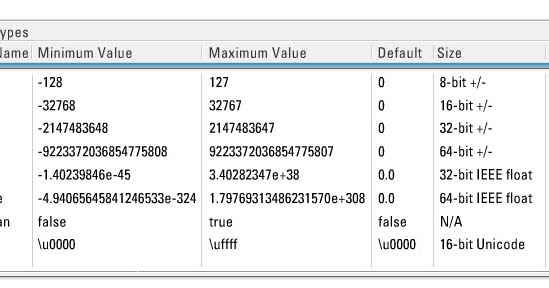
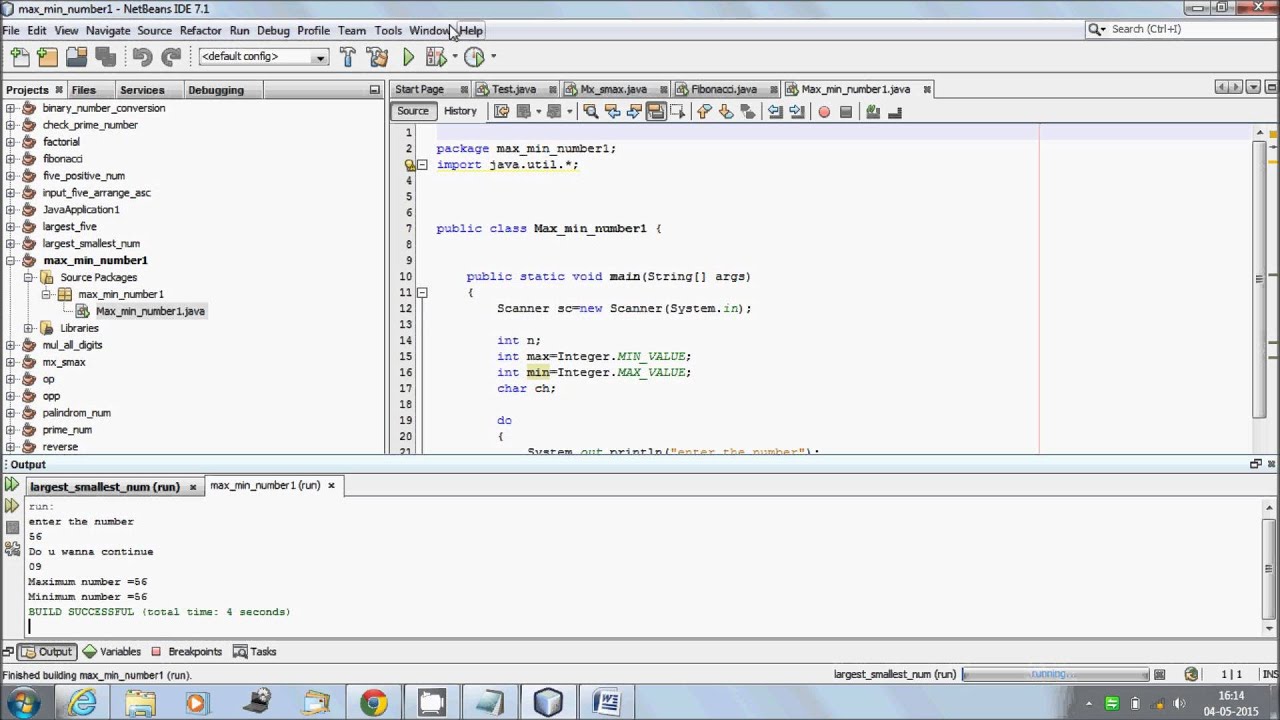
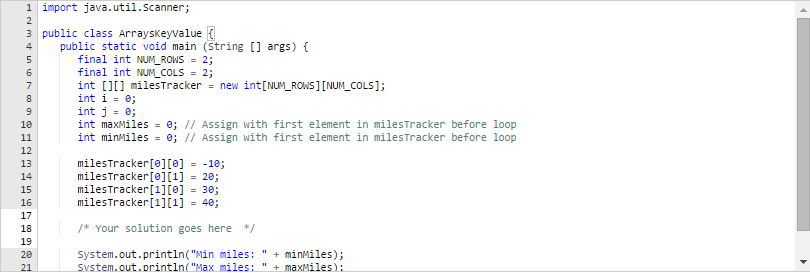
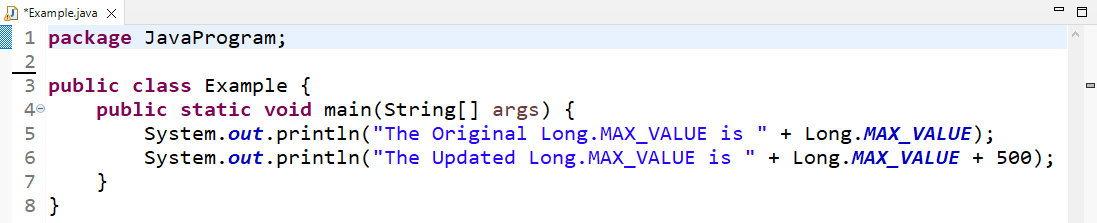

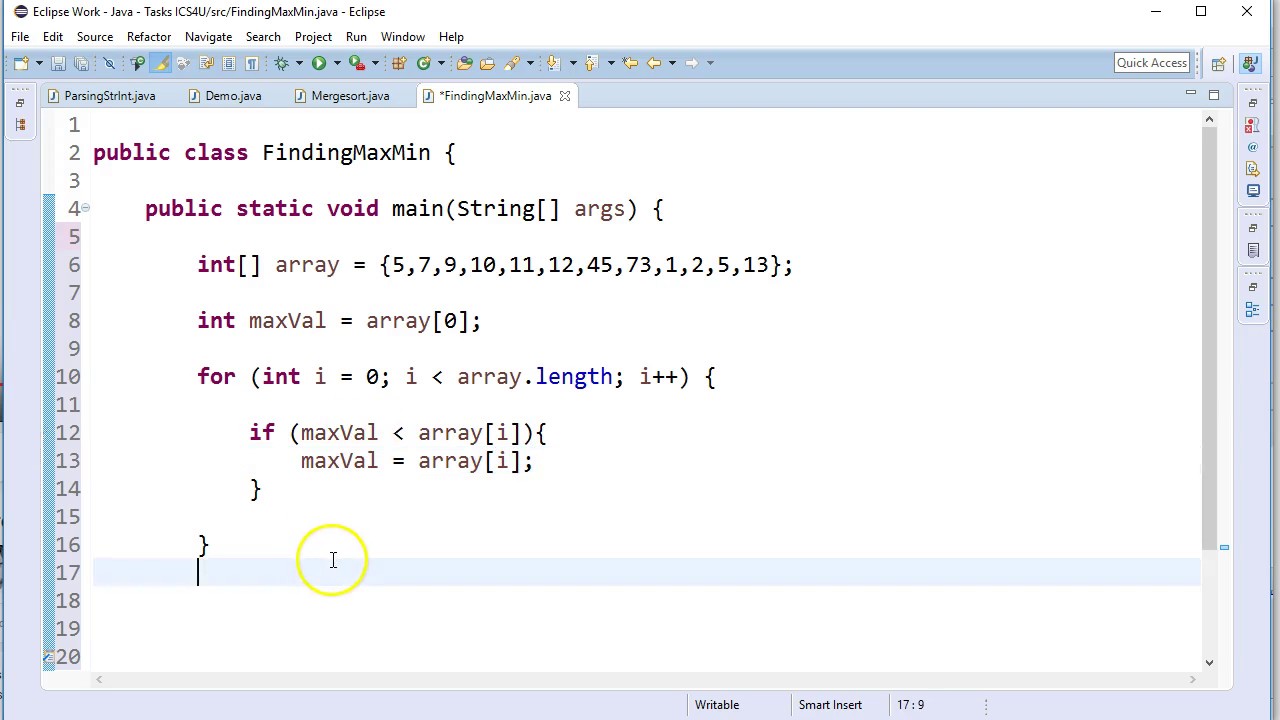



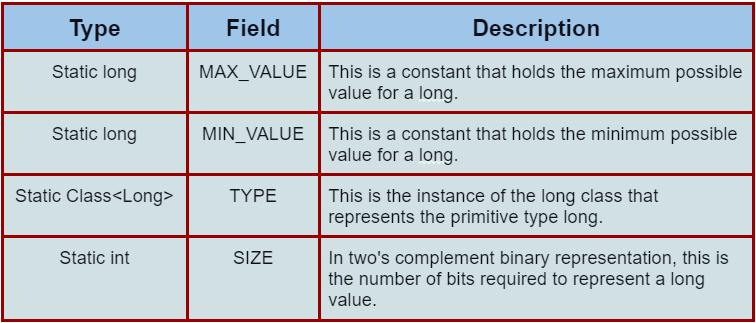


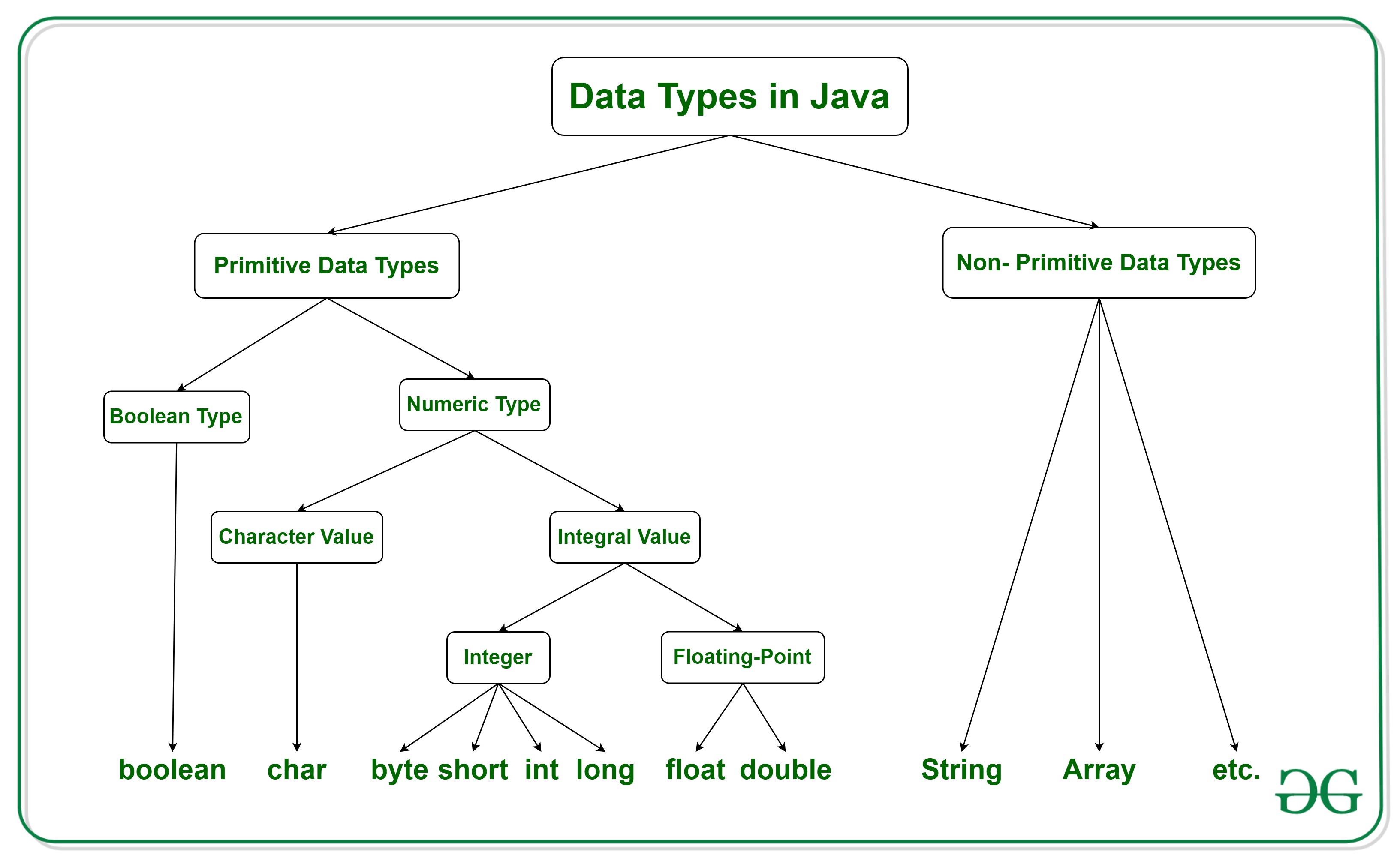
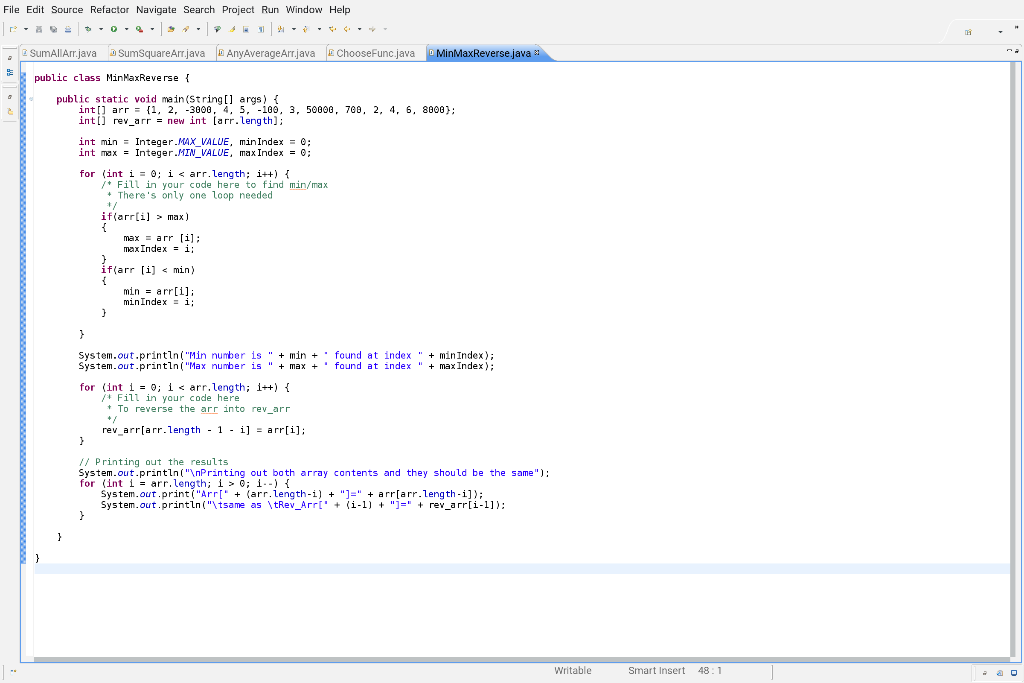
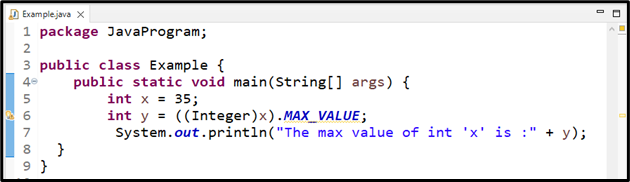

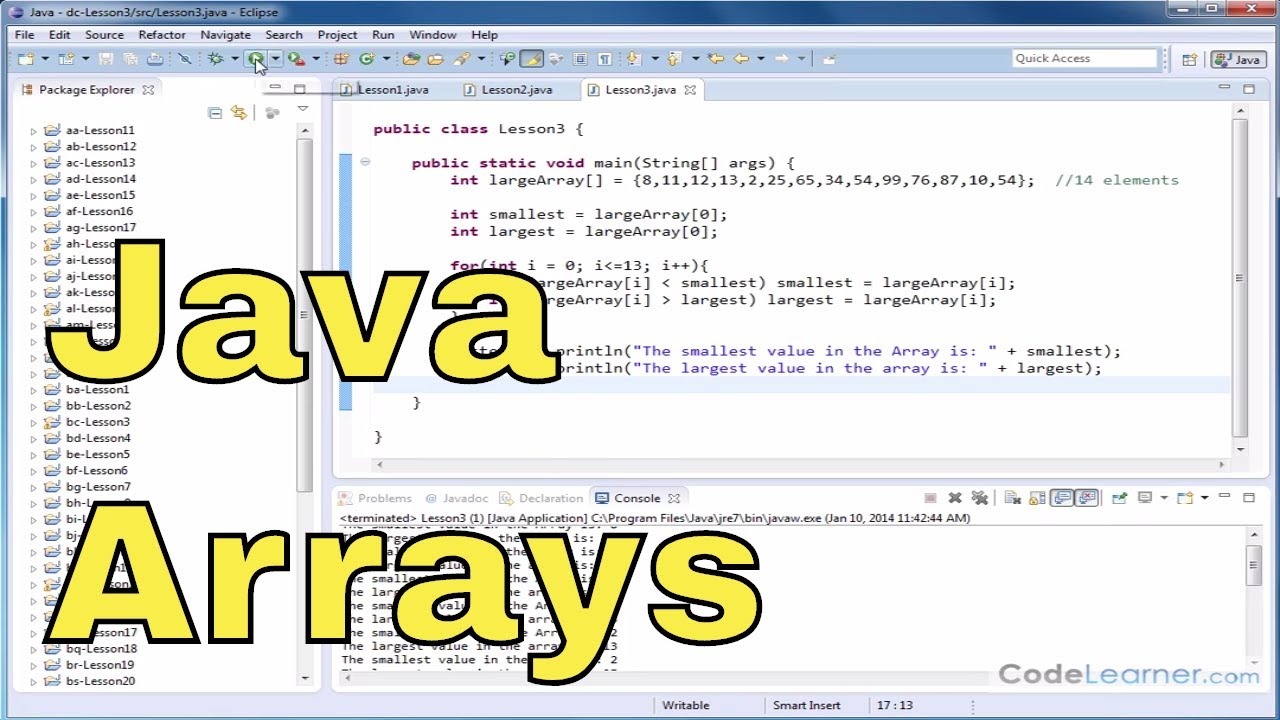

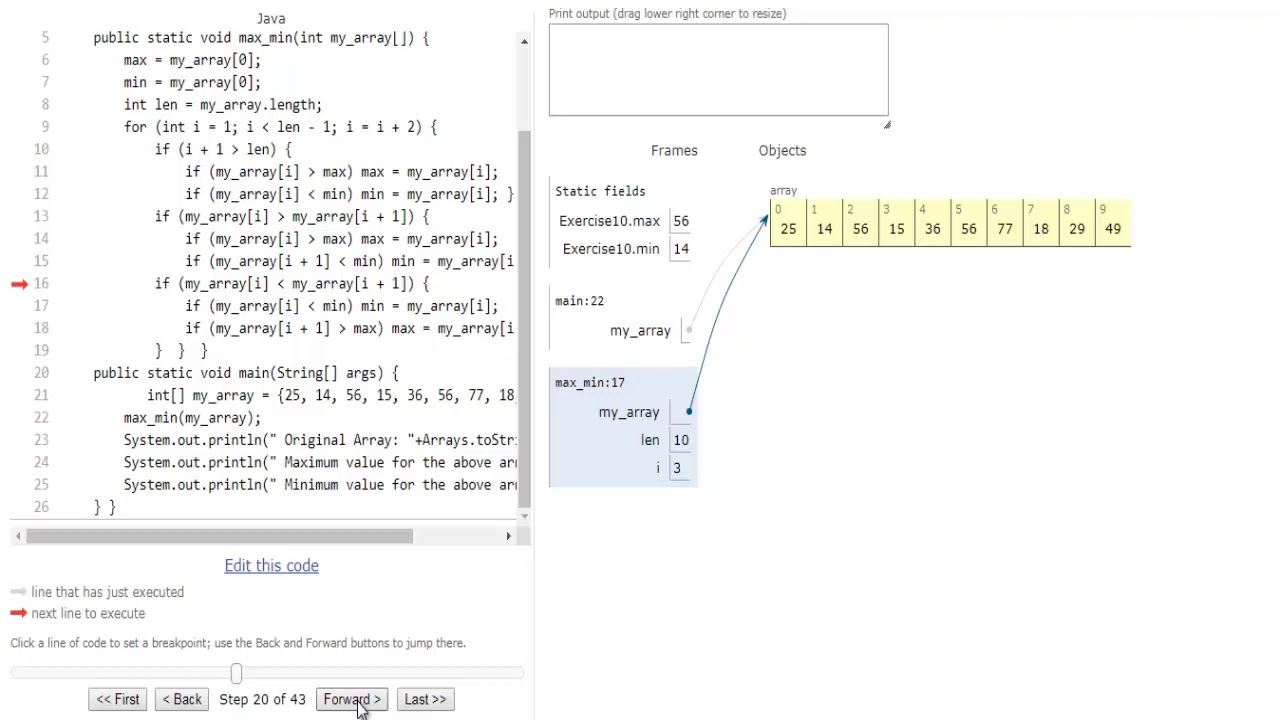
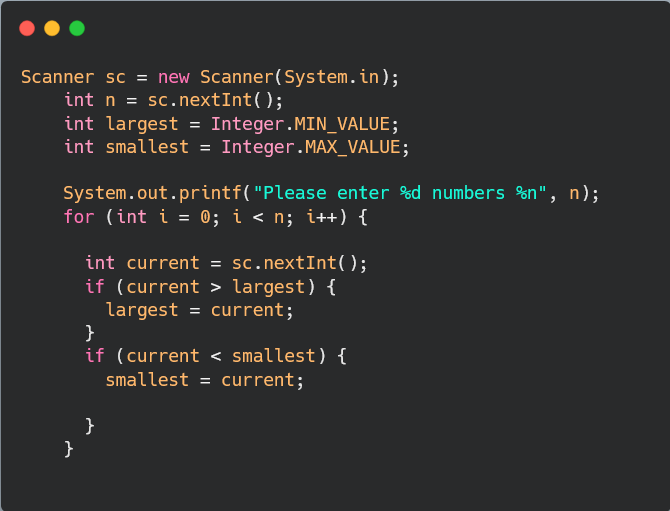

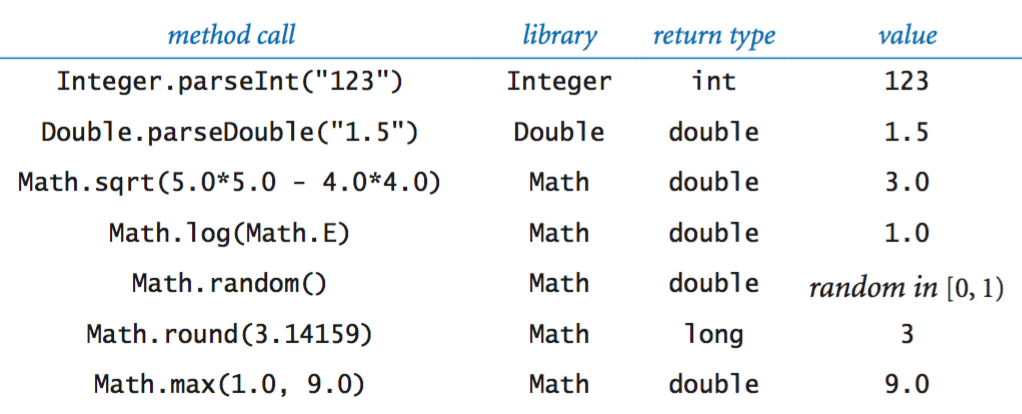

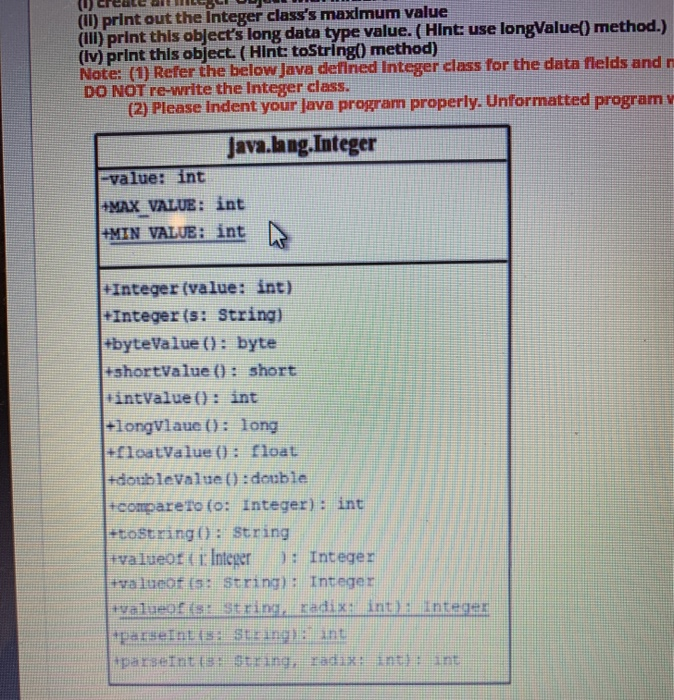
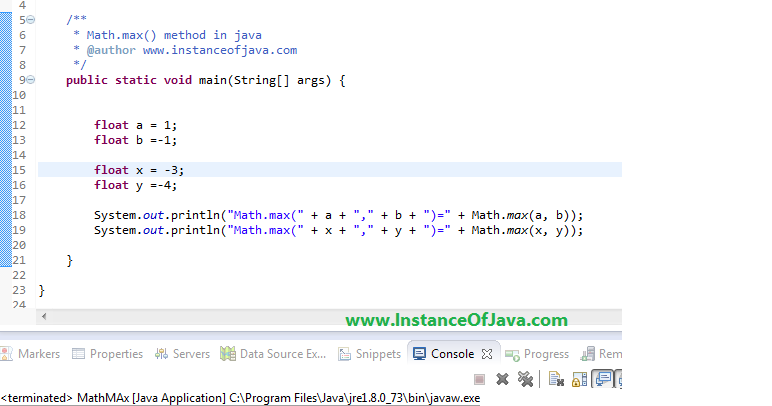

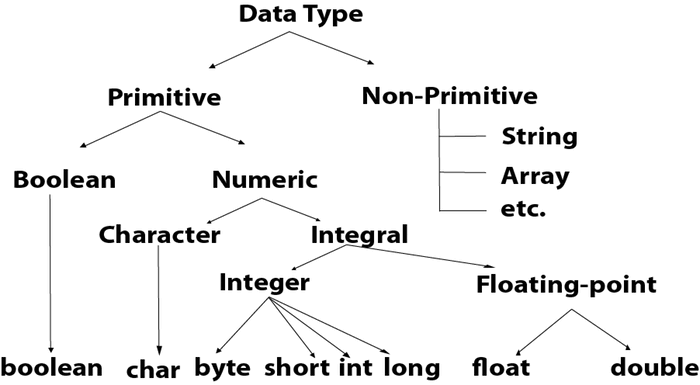

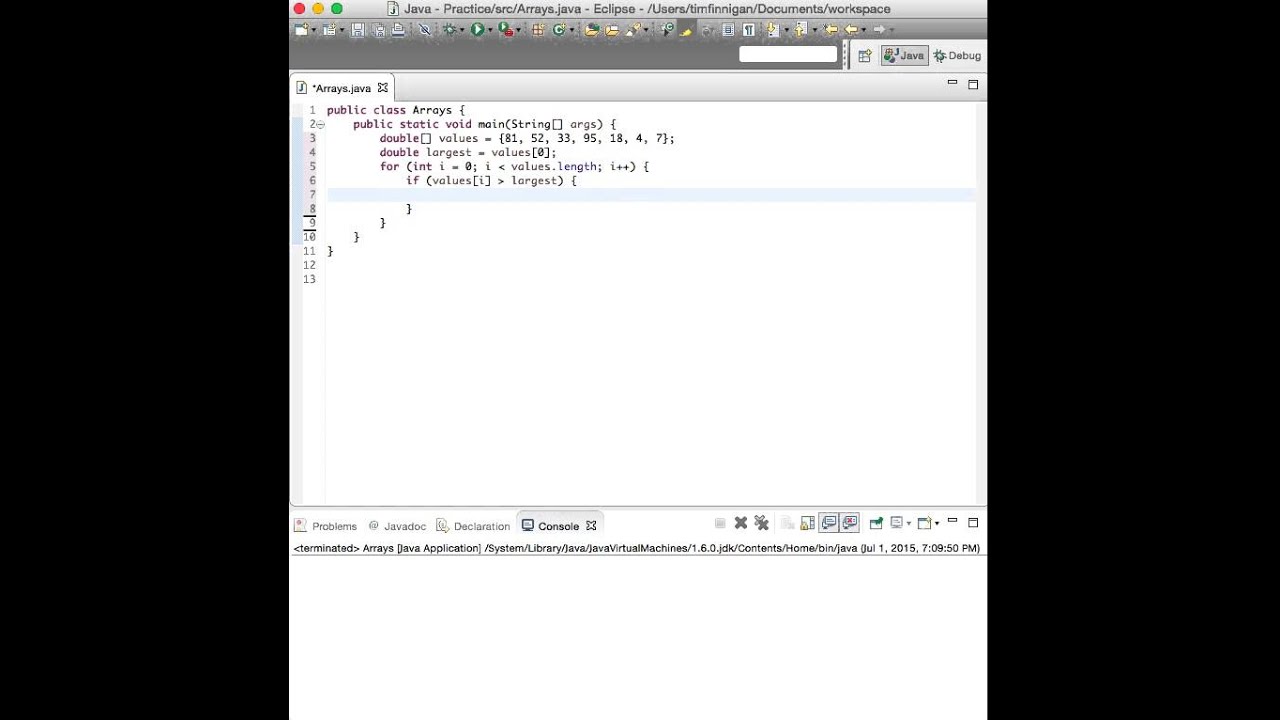
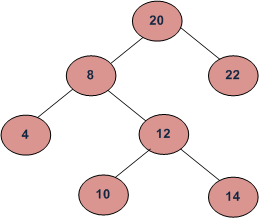
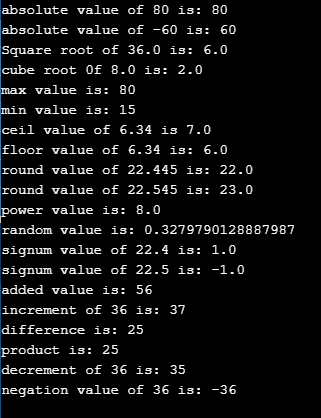
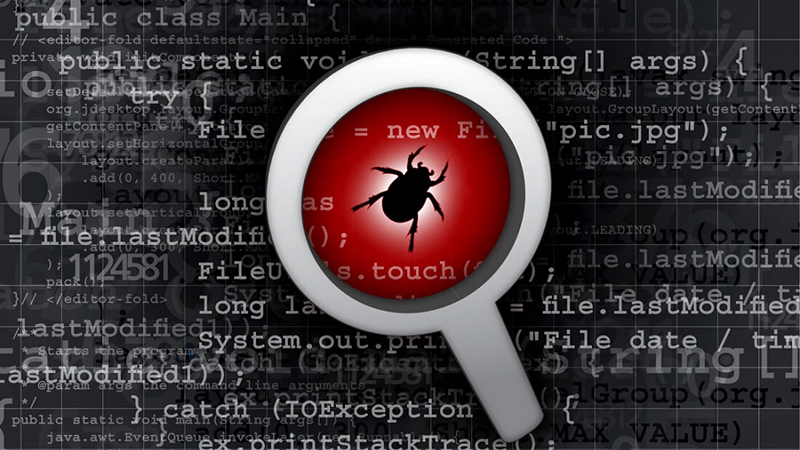
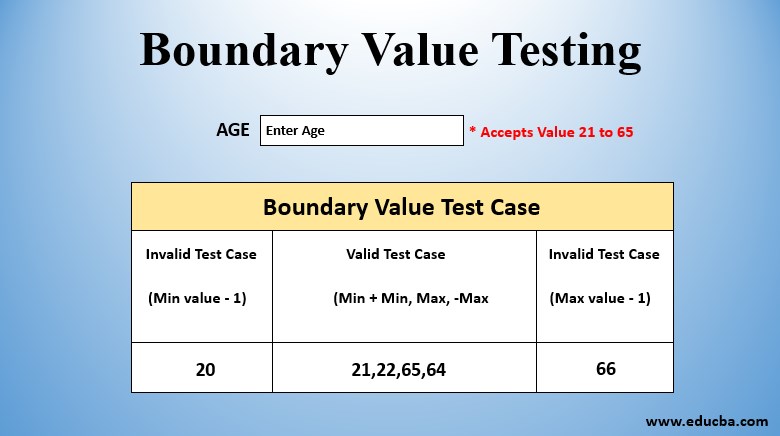
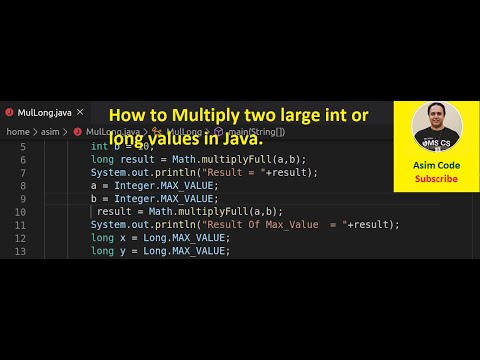
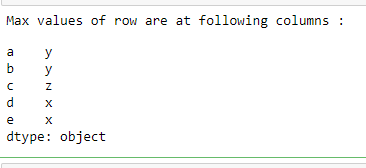


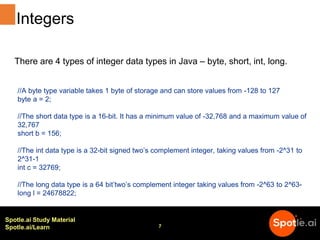
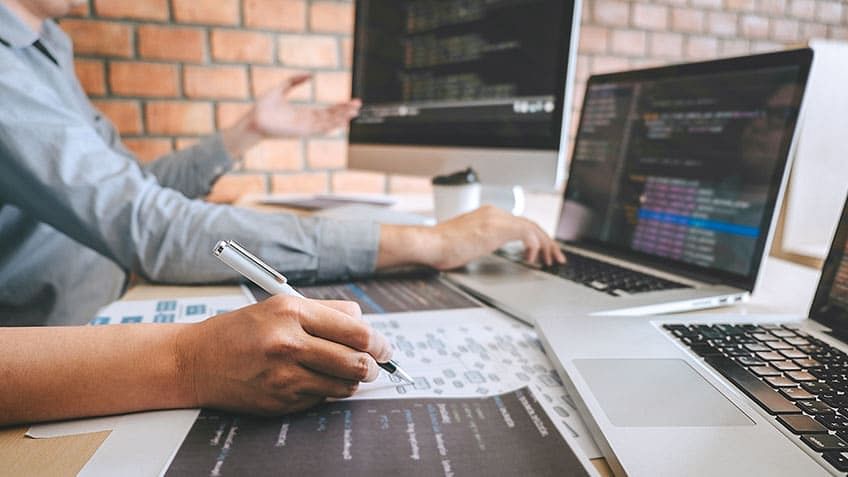

Article link: max value of long in java.
Learn more about the topic max value of long in java.
- How to Use Long.MAX_VALUE in Java | Explained – Linux Hint
- A long bigger than Long.MAX_VALUE – Stack Overflow
- Long.MAX_VALUE in Java | Delft Stack
- Java Long.MAX_VALUE and Long.MIN_VALUE – GoLinuxCloud
- Primitive Data Types – Java Tutorials – Oracle Help Center
- Long.MaxValue Field (Java.Lang) – Microsoft Learn
- Integer.MAX_VALUE in Java with Examples – CodeGym
- long / Reference / Processing.org
- max value of integer – W3docs
- Java – get long max value – Dirask
See more: https://nhanvietluanvan.com/luat-hoc