Max Int In Python
Python is a widely-used high-level programming language known for its simplicity and ease of use. While being beginner-friendly and versatile, Python also has certain limitations when it comes to handling integer values. In this article, we will explore the max int value in Python, discuss the binary representation of integers, declare and initialize maximum integers, compare maximum integer values, explore operations and functions on these values, and address common errors and issues related to maximum integers in Python.
Overflow of Integer in Python:
In Python, an overflow occurs when the result of an arithmetic operation exceeds the maximum representable value of the integer type. Unlike some programming languages, Python does not raise an exception or error when an overflow occurs. Instead, it automatically promotes the integer to a long integer, which can represent much larger values. This feature makes Python ideal for handling large numbers and performing computations involving them.
Binary Representation of Integers in Python:
Under the hood, integers in Python are represented in binary form, consisting of 0s and 1s. The size of an integer depends on the platform or system on which Python is running. In most cases, integers are represented using 32 bits or 64 bits. The actual binary representation of an integer can be obtained using the `bin()` function in Python, which converts the given integer to its binary form.
Declaring and Initializing Maximum Integers in Python:
Python does not have an explicit constant for representing the maximum integer value. Instead, you can use the `sys` module and access the `sys.maxsize` attribute, which returns the largest positive integer that can be used as an index for a Python list or a string. This value represents the maximum integer value for the current platform. Alternatively, you can use the `sys.maxsize` attribute divided by 2 to get the maximum positive integer value.
Comparison of Maximum Integer Values in Python:
When comparing maximum integer values in Python, you need to be cautious of type promotion. As mentioned earlier, when an arithmetic operation involves an overflow, Python automatically promotes the integer to a long integer. Comparing a regular integer with a long integer can lead to unexpected results. Therefore, it is advisable to check for type compatibility before performing any comparisons.
Operations and Functions on Maximum Integers in Python:
Python provides a wide range of operations and functions to perform calculations and manipulations on integer values, including maximum integers. The basic arithmetic operators such as addition, subtraction, multiplication, and division work seamlessly with these values. However, certain operations, such as exponentiation (`**`), bitwise shifting, and modular arithmetic, may produce different results when dealing with maximum integers due to type promotion and overflow.
Common Errors and Issues with Maximum Integers in Python:
1. Python Int Limit: As mentioned earlier, the maximum integer value in Python is platform-dependent. The `sys.maxsize` attribute can be used to determine the maximum integer for a particular platform. However, it is important to note that this value is not fixed and can vary between different systems.
2. Max int Python 3: In Python 3, there is no explicit concept of a maximum integer value. Instead, integers can grow arbitrarily large as long as the system has enough memory to store them. This feature eliminates the need for long integers in Python 3, simplifying the handling of large numbers.
3. Big Integer Python: Python does not have a dedicated “big integer” type. Instead, it automatically promotes regular integers to long integers when they overflow. This allows Python to handle integers of arbitrary size, limited only by the available memory.
4. Max Float Python: Unlike integers, floating-point numbers in Python have a fixed maximum value. The maximum representable floating-point value can be obtained using the `sys.float_info.max` attribute. It is important to note that this value is different from the maximum integer value.
5. Range of Data Types in Python: Python provides several built-in data types, including integers, floating-point numbers, booleans, strings, lists, tuples, and dictionaries. Each data type has its own range and limitations. Understanding these ranges is crucial to avoid common errors and ensure correct program execution.
6. Python Int Type: In Python, the `int` type represents integer values. This type can be used to store both positive and negative integers. The `int` type automatically promotes to a long integer when an overflow occurs.
7. Find Max Python: To find the maximum value among a collection of numbers in Python, you can use the `max()` function. This function takes multiple arguments or an iterable and returns the maximum value. However, if the collection contains a mix of integer and non-integer values, ensure proper type handling to avoid errors.
In conclusion, Python provides a flexible and powerful environment for working with integer values. While there are limitations to the maximum integer value, Python’s automatic promotion to long integers allows for handling of arbitrarily large numbers. By understanding the binary representation of integers, properly declaring and initializing maximum integers, and being mindful of type promotion and compatibility, you can effectively work with maximum integer values in Python.
Python – Max Int
What Is The Max 64-Bit Int In Python?
Python is a popular programming language known for its simplicity and readability. It supports various data types, including integers (int). Integers can be classified into different sizes, ranging from 8 bits to 64 bits, depending on the amount of memory allocated to store them.
In Python, the standard integer data type utilized for most operations is the 32-bit signed integer, also known as int32. However, Python also supports larger integer values through a separate data type called long or int64. This data type is not commonly used in most Python programs, but it is available when a larger range of integers is required.
The int64 data type can represent both positive and negative integers and has a maximum limit that differs from the int32 type. While int32 has a maximum value of 2,147,483,647, the int64 data type can represent much larger numbers.
The maximum value of a 64-bit integer in Python can be computed using the sys module, which provides access to some variables maintained or used by the interpreter and to functions that interact strongly with the interpreter. To determine the maximum value of a 64-bit integer, we can utilize the sys.maxsize attribute.
Here’s an example that demonstrates how to obtain the maximum value of a 64-bit integer in Python:
“`python
import sys
max_int64 = sys.maxsize
print(“The maximum value of a 64-bit integer in Python is:”, max_int64)
“`
When executed, this code will display the maximum value of a 64-bit integer in Python, which is 9,223,372,036,854,775,807. This enormous value indicates the upper limit of int64, thus representing the maximum 64-bit integer in Python.
FAQs
Q: Why do we need a 64-bit integer?
A: In most cases, the standard 32-bit integer data type (int32) suffices for everyday programming needs. However, there are situations that require the representation of extremely large integers, such as cryptography, scientific calculations, or storing large quantities. In these cases, the 64-bit integer data type (int64) becomes necessary to handle such extensive numerical values.
Q: Can Python handle larger integers?
A: Yes, Python provides an additional data type called `bigint`, which stands for big integers. The `bigint` type is not limited by the 64-bit constraint like `int64` and can represent arbitrarily large integers. Python automatically switches to `bigint` when the value exceeds the range of `int64`, making it powerful in dealing with extremely large numerical values.
Q: Can we perform mathematical operations on 64-bit integers?
A: Yes, Python supports standard mathematical operations like addition, subtraction, multiplication, and division on 64-bit integers, just like any other integer data type. However, keep in mind that performing operations on very large integers may consume more system resources and, consequently, take longer to execute.
Q: Are there other data types for handling large numbers?
A: Yes, Python also provides the `float` data type for representing decimal values with a higher precision than integers. Floats can handle very large or very small values. However, they are limited by the underlying hardware and may lose precision in certain calculations, so it is important to consider the appropriate data type depending on the requirements of your program.
In conclusion, the maximum 64-bit integer in Python (int64) is 9,223,372,036,854,775,807. While this data type is not commonly used in everyday programming, it becomes vital when dealing with extremely large numerical values. Python also offers alternative data types like `bigint` and `float` to handle even larger or more complex numerical computations.
What Is The Max 32 Int In Python?
When working with numbers in Python, you might come across the term “int” which stands for integer. Integers are whole numbers, either positive or negative, without any decimal or fractional parts. Python provides various data types for integers, including 32-bit integers. In this article, we will delve into the concept of the max 32 int in Python, exploring its significance, limitations, and potential use cases.
Understanding Data Types in Python
Before diving into the specifics of the max 32 int in Python, it’s important to have a broader understanding of data types in this programming language. Python, unlike some other languages, is dynamically typed, meaning you don’t have to explicitly declare the data type of a variable. The interpreter automatically assigns the data type based on the value assigned to it.
Python supports several numeric data types, such as integer (int), floating-point (float), and complex (complex). In this article, we’ll solely focus on the integer data type, more specifically the 32-bit integer.
What is a 32-bit Integer?
A 32-bit integer, also known as a signed 32-bit integer, is a data type that can hold 32 bits of information. The term “signed” means that it can represent both positive and negative values. The range of values a 32-bit integer can store is from -2,147,483,648 to 2,147,483,647.
The max 32 int refers to the highest positive value that a 32-bit integer can hold, which is 2,147,483,647. Trying to store a value higher than this maximum will result in an overflow, leading to unexpected behavior or errors in your program.
Limitations of the Max 32 Int
While the max 32 int in Python seems quite large, it does have certain limitations. If you need to perform calculations involving larger numbers, you may need to consider using alternative data types or libraries.
1. Limited Range: As mentioned earlier, the range of a 32-bit integer is restricted to -2,147,483,648 to 2,147,483,647. If you attempt to store a value outside this range, you will encounter an overflow error.
2. Precision: Another important aspect to consider is the precision of the calculations. Since the 32-bit integer is limited to holding only whole numbers, any calculations involving fractions or decimals cannot be accurately represented with this data type.
Alternative Data Types
To work with larger numbers or calculations requiring more precision, Python offers alternative data types and libraries. Here are a few options worth considering:
1. Long Integer (long): The long integer data type allows for arbitrarily large numbers, limited only by the available memory. It provides more precision and range compared to the max 32 int. However, it should be noted that starting from Python 3, the distinction between int and long has been removed, and int can handle arbitrarily large numbers.
2. Decimal Module: The decimal module in Python provides a Decimal data type that offers even greater precision for mathematical operations involving decimals. It is particularly useful in scenarios where maintaining precision is crucial, such as financial calculations.
3. NumPy: NumPy is a powerful library in Python for scientific computing that introduces additional data types, including int32, int64, and others. These data types allow for higher precision and larger ranges compared to the default integer data types in Python.
FAQs
Q: What is the purpose of the max 32 int in Python?
A: The max 32 int is the highest positive value that a 32-bit integer in Python can store. It defines the upper limit for whole numbers that can be represented using this specific data type.
Q: Can the max 32 int be exceeded in Python?
A: Yes, attempting to store a value greater than the max 32 int in Python will result in an overflow error. It is important to consider this limitation and use appropriate data types or libraries for calculations involving larger numbers.
Q: How can I handle calculations involving decimals with the max 32 int?
A: Since the max 32 int is limited to whole numbers, it cannot accurately represent calculations involving decimals. To handle decimal calculations, you can use alternative data types such as float or the Decimal module that provides greater precision.
Q: Are there data types in Python that can handle even larger numbers?
A: Python provides options like the long integer and libraries like NumPy, which offer alternative data types with larger ranges and higher precision for handling larger numbers.
In conclusion, the max 32 int in Python represents the highest positive value a 32-bit integer can store. While it has a substantial range for whole numbers, it does have limitations in terms of computing larger numbers and decimals accurately. By understanding these limitations and exploring alternative data types, you can effectively handle a wider range of mathematical calculations in Python.
Keywords searched by users: max int in python Python int limit, Max int Python 3, Big integer Python, Max float Python, Int max, Range of data types in python, Python int type, Find max Python
Categories: Top 20 Max Int In Python
See more here: nhanvietluanvan.com
Python Int Limit
Introduction:
Python is a versatile and popular programming language that excels in numerous domains, including data analysis, web development, and artificial intelligence. When working with numeric data, Python provides a built-in integer data type (int) that allows programmers to perform various mathematical operations. However, like any other data type, Python’s int has its limitations. In this article, we will delve into the Python int limit, exploring the bounds of its numeric representations and addressing common questions associated with this topic.
Understanding the Python int Data Type:
In Python, ints are used to represent whole numbers—positive, negative, and zero—without any fractional or decimal parts. Unlike other programming languages, Python’s int data type has no limit in terms of its size or precision. As long as your computer’s memory allows, Python can handle extremely large integers. This flexibility and infinite range make Python ideal for tasks that require computations involving massive numbers.
Python Int Limit:
Although Python’s int data type theoretically has no limitation on its size or precision, the practical limit is determined by the amount of memory available on your machine. Python’s standard implementation (CPython) stores integers as fixed-size arrays of digits in base 2^30. As a result, the size of an integer in bytes is proportional to its magnitude, with additional overhead for storing metadata.
By default, Python 3 uses a variable-length representation for integers, allowing more efficient memory utilization for small values. This type of representation saves memory space by using a smaller fixed-size array for integers below a certain threshold, typically between -5 and 256. However, for larger integers, Python resorts to a wider array size, proportional to the magnitude of the number.
The maximum size of an int in Python is, therefore, constrained by the amount of available memory. In practical terms, this typically means that the limit is around 2^31 to 2^63-1 on most modern systems. Attempting to create an int beyond this limit leads to a MemoryError, as Python cannot allocate the necessary memory.
Python’s Built-in Overflow Protection:
Python incorporates built-in overflow protection, meaning that calculations resulting in values beyond the int limit do not cause errors or crashes. Instead, they are automatically converted to a different numeric data type called long (or bigint in Python 2). The long data type allows arbitrary precision arithmetic and seamlessly accommodates calculations involving extremely large numbers. This provides programmers with a seamless transition when they surpass the int limit in their computations.
FAQs:
Q1. What happens if I exceed the int limit in Python?
If you attempt to create an int exceeding the memory limit of your system, Python will raise a MemoryError exception, as it cannot allocate the necessary memory. However, Python automatically converts calculations that surpass the int limit to the long (or bigint in Python 2) data type, allowing you to work with larger numbers without the risk of errors or crashes.
Q2. Can I change the int limit in Python?
As the int limit is directly tied to the amount of memory available on your system, it cannot be directly modified within Python. However, you can use third-party libraries, such as NumPy or GMPy, which provide alternative implementations for handling extremely large numbers or arbitrary precision arithmetic.
Q3. Are there any performance implications when working with longs instead of ints?
Yes, there are performance implications when using longs instead of ints. Although Python’s long data type offers the flexibility of arbitrary precision arithmetic, it requires more memory and computational resources compared to regular ints. Therefore, it is crucial to consider these trade-offs and determine if the use of longs is necessary for your particular use case.
Q4. Are there any alternatives to longs for performing calculations with extremely large numbers?
There are several third-party libraries available for performing calculations with extremely large numbers. Some popular ones include NumPy, GMPy, and mpmath. These libraries provide efficient implementations of arbitrary precision arithmetic and offer optimizations for specific use cases.
Q5. Does the int limit vary across Python implementations?
While the theoretical int limit is not defined in the Python language specification, the practical limit is implementation-dependent. Different Python implementations, such as CPython, Jython, and PyPy, may have variations in the maximum size of an int. However, the int limit observed in practice is largely determined by the amount of memory available on the system rather than the specific implementation.
Conclusion:
Python’s int data type offers great flexibility and infinite range, making it suitable for most numerical computations. However, it is essential to be aware of the practical int limit imposed by the amount of available memory. When the int limit is surpassed, Python seamlessly converts calculations to the long data type, enabling the handling of extremely large numbers. By understanding these concepts and considerations, you can confidently work with Python’s int data type and tackle complex mathematical operations effectively.
Max Int Python 3
What is Max Int?
In computing, the maximum integer represents the largest numeric value that can be stored in a given programming language or system. It is a crucial value since it sets the upper limit for numerical computations and for storing whole numbers within a program.
In Python 3, the maximum integer value can be found by using the “sys” module. Specifically, the “sys.maxsize” attribute returns the largest positive integer that can be used as an index for sequences or arrays. It represents the maximum value for the platform’s “native” signed integer type. However, it is important to note that this value may vary across different platforms and operating systems.
How is Max Int Represented in Python?
In Python 3, integers can be of arbitrary size, which means they can grow to practically any size required for a computation. This capability allows Python to handle extremely large numbers without the risk of overflow errors encountered in some other programming languages.
Python represents integers using a variable-length encoding scheme. The memory required to store an integer in Python increases as the integer value grows. This dynamic representation enables Python to utilize only the necessary amount of memory for storing each specific number.
Additionally, Python provides a built-in “int” function that allows conversion from other data types to integers. This function takes into account the maximum integer value in order to correctly convert inputs exceeding the maximum value into a suitable form without resulting in overflow errors.
Implications for Programmers
Understanding the concept of max int is essential for programmers, as it allows them to work within the appropriate numerical limits and avoid potential errors. Here are a few implications for programmers when dealing with max int in Python:
1. Range of Valid Inputs: Programmers need to be aware of the range of integers that their program can handle safely. Inputs exceeding the max int value may result in unexpected behavior or errors. Proper validation and error handling mechanisms should be implemented to handle such scenarios.
2. Performance Considerations: Extremely large integers may require additional computational resources and time to process. Programmers should take this into account when designing algorithms and ensure efficient handling of large numbers.
3. Memory Utilization: As mentioned earlier, Python’s dynamic representation of integers provides flexibility in terms of memory allocation. However, it’s important to be mindful of memory usage, especially when dealing with large data sets or performing intensive numerical computations.
FAQs
Q1. Can I change the max int value in Python?
A: No, the maximum integer value in Python is determined by the platform and system’s native signed integer type. It cannot be modified directly within the Python language.
Q2. What happens if I exceed the max int value when performing calculations?
A: If you exceed the maximum integer value, Python will automatically switch to a different data type called “long,” which can handle arbitrarily large integer values. However, this switch may incur additional memory and computational costs.
Q3. How can I check if a number exceeds the max int value?
A: You can compare a given number with the value of “sys.maxsize” to determine if it exceeds the maximum integer limit.
Q4. Is there a minimum integer value in Python?
A: Yes, Python also has a minimum value for integers, which is represented by the attribute “sys.minsize.” It represents the platform’s minimum value for the native signed integer type.
Q5. Does Python support unsigned integers?
A: No, Python only supports signed integers. However, you can achieve the same effect by using “long” integers, which have no upper limit.
Conclusion
Understanding the concept of max int and its implications is crucial for Python programmers. By being aware of the maximum integer value, programmers can design their programs more effectively, handle large numbers appropriately, and ensure the correctness and optimal performance of numerical computations. Remember to consult the “sys.maxsize” attribute for platform-specific maximum integer information and keep in mind the frequently asked questions discussed in this article. Happy coding!
Big Integer Python
In computer programming, numbers play a fundamental role, with integer values being one of the primary data types used in various applications. However, there are cases where regular integer values fall short because of their limited range. That’s where big integers come into play. In this article, we will explore the concept of big integers in Python and how they can be effectively used to handle large numbers.
Understanding Big Integers:
Regular integer data types in Python have a fixed size that restricts their range. For instance, the ‘int’ data type in Python 3 is limited to values within the range of -2^31 to 2^31-1. However, in certain scenarios, we may need to work with numbers beyond this range, such as when dealing with encryption algorithms, large prime numbers, or scientific computations.
Big integers, also known as arbitrary-precision integers, provide a solution to this problem. Unlike regular integers, big integers have no fixed size and can handle numbers of any size, allowing us to perform calculations accurately without any overflow or loss of precision.
Python’s Big Integer Support:
Python offers built-in support for big integers through its ‘int’ data type, which can automatically switch from regular integers to big integers based on the magnitude of the number. The ‘int’ type in Python can represent arbitrarily long integers and performs operations on them efficiently.
Python internally handles the memory allocation and storage of big integers, allowing programmers to focus solely on their calculations without worrying about the implementation details. This makes Python an excellent choice for working with large numbers, as it provides an intuitive and convenient way to operate on them.
Basic Operations on Big Integers:
Just like regular integers, big integers support various arithmetic operations, including addition, subtraction, multiplication, division, and modulo operations. Python’s ‘int’ class defines these operations, allowing us to perform calculations on big integers seamlessly.
For example, consider the following code snippet:
“`
a = 123456789012345678901234567890
b = 987654321098765432109876543210
c = a + b
print(c) # Output: 1111111111111111111111111111111111
“`
In the code snippet above, we perform addition using big integers ‘a’ and ‘b’, resulting in a sum ‘c’ that is conveniently stored and printed.
Advanced Operations and Utilities:
Python’s ‘int’ class also provides several advanced operations and utilities for big integer manipulation. Some of the notable ones include exponentiation, bitwise operations, comparison operations, and conversions to and from other data types.
Additionally, Python offers the ‘math’ and ‘cmath’ modules for performing advanced mathematical calculations on big integers. These modules provide functions for trigonometric, logarithmic, exponential, and power calculations, among others.
FAQs about Big Integers in Python:
Q1. How large can big integers be in Python?
A. The maximum size of a big integer in Python is determined by the available memory. Python handles the memory allocation dynamically, allowing big integers to scale based on the system’s memory capacity.
Q2. Are there any performance implications when working with big integers?
A. Yes, performing operations on big integers can be slower compared to regular integers due to the additional memory allocation and manipulation required. However, Python’s efficient implementation minimizes these performance impacts, making big integer calculations reasonably fast for most use cases.
Q3. Can big integers be used as keys in dictionaries or indices in lists?
A. Yes, big integers can be used seamlessly as keys in dictionaries or indices in lists, just like regular integers. Python internally handles the comparison and hashing of big integers, ensuring their usability in such data structures.
Q4. Can I convert a big integer to a string or vice versa?
A. Yes, Python provides convenient methods for converting big integers to strings (‘str()’) and vice versa (‘int()’). These conversions allow easy integration with input/output operations or string manipulations.
Q5. Are there any libraries or modules for advanced big integer operations in Python?
A. Along with Python’s built-in functionalities, several third-party libraries like ‘gmpy2’ and ‘sympy’ provide additional support for advanced big integer operations like modular exponentiation, primality testing, and more.
In conclusion, big integers play a vital role in handling large numbers in Python, allowing programmers to perform calculations accurately without any range limitations. Python’s built-in ‘int’ type provides seamless support for big integers, making it an excellent choice for working with large numbers in various domains. By understanding the concept of big integers and utilizing Python’s rich features, programmers can overcome the limitations imposed by regular integer types and embark on new possibilities in their projects.
Images related to the topic max int in python
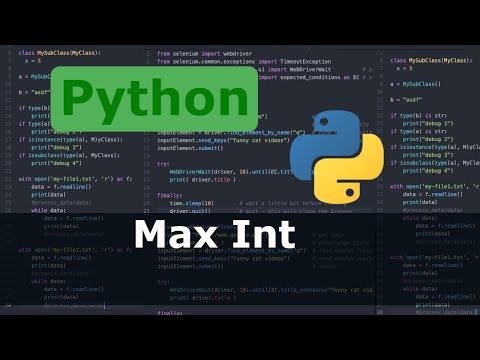
Found 18 images related to max int in python theme


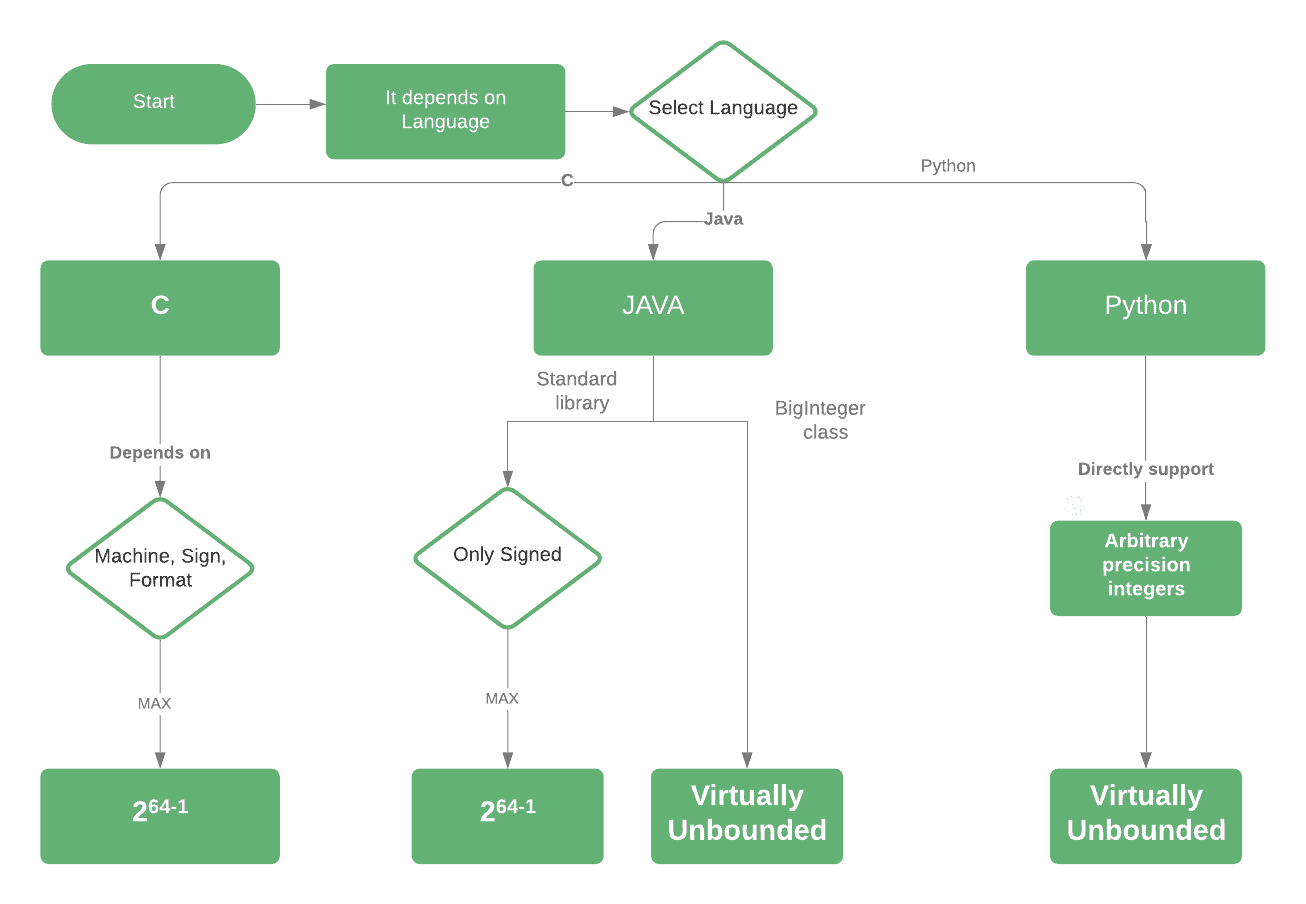


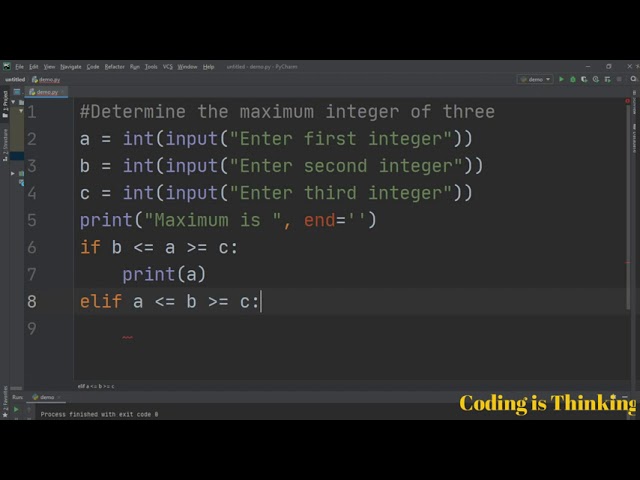



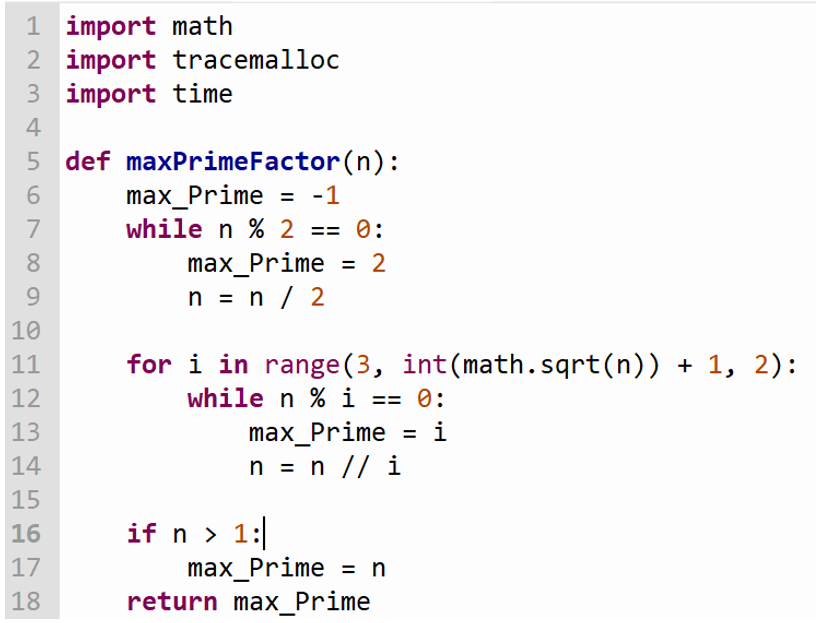
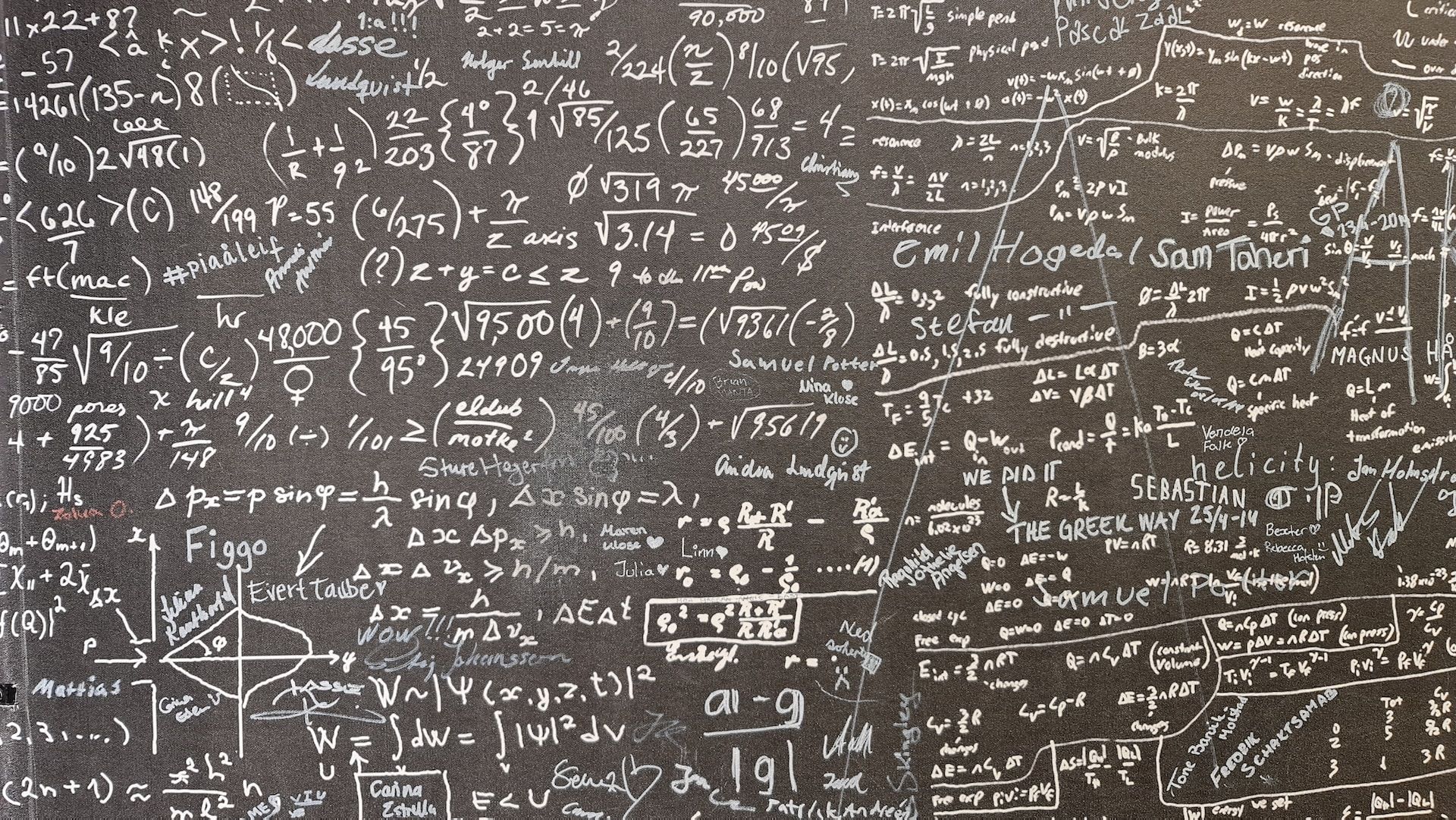
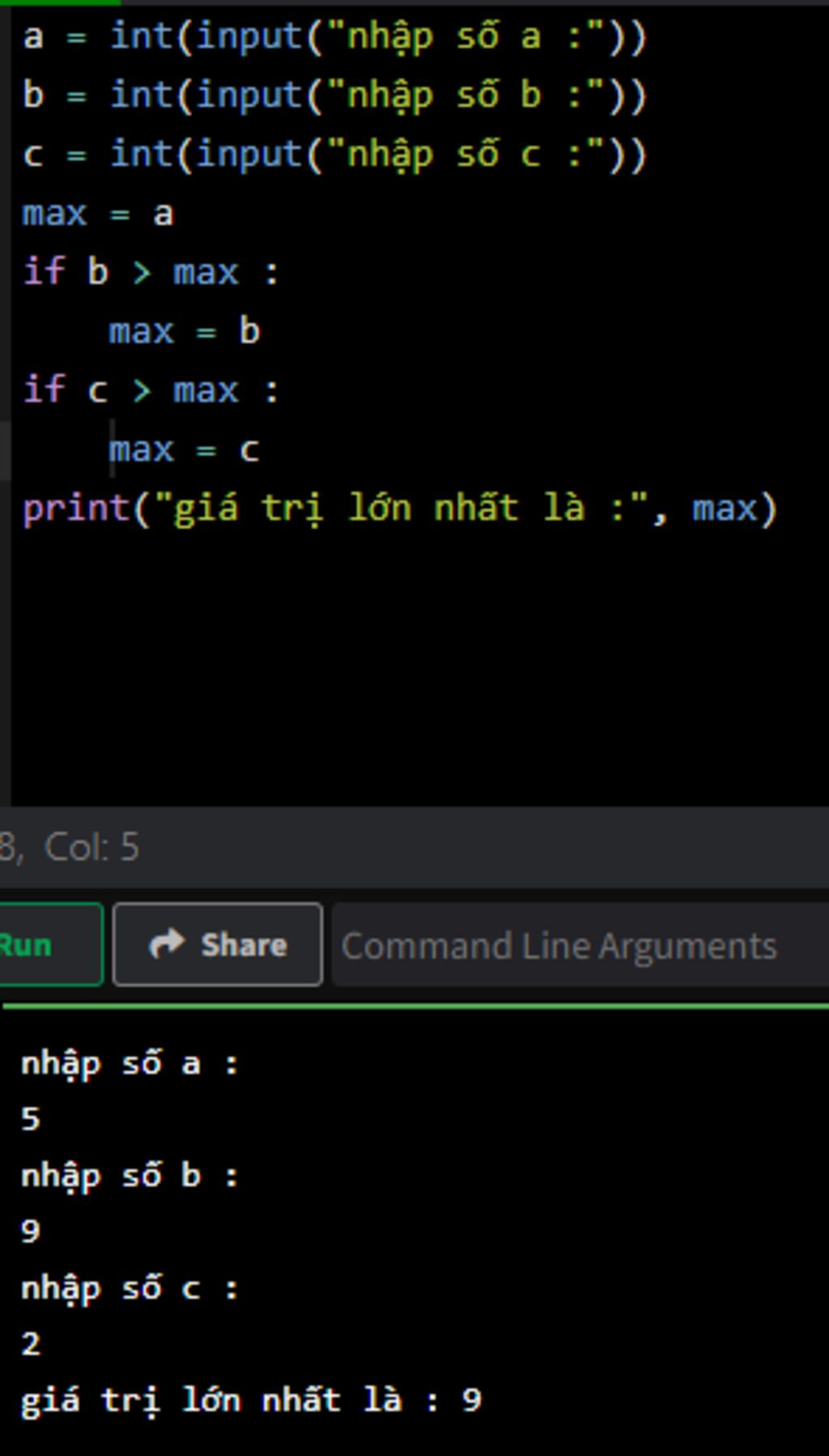



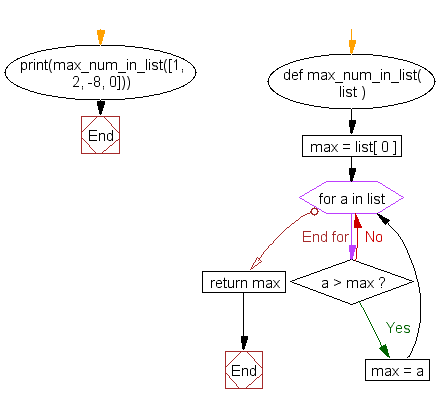
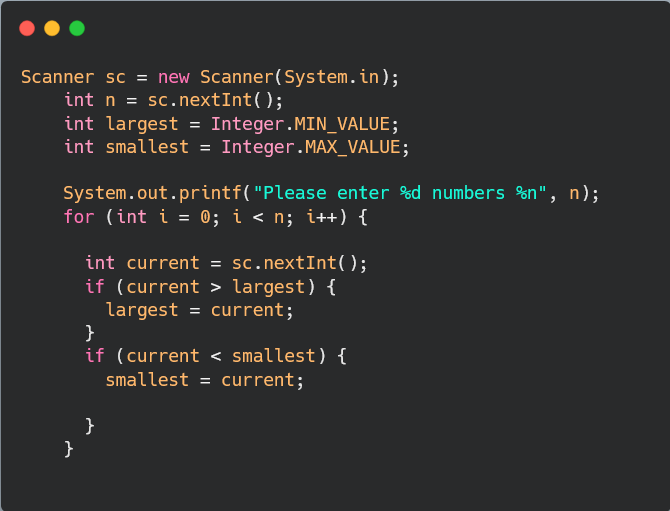

![Understanding Float in Python [with Examples] Understanding Float In Python [With Examples]](https://www.simplilearn.com/ice9/free_resources_article_thumb/FloatInPython_1.png)
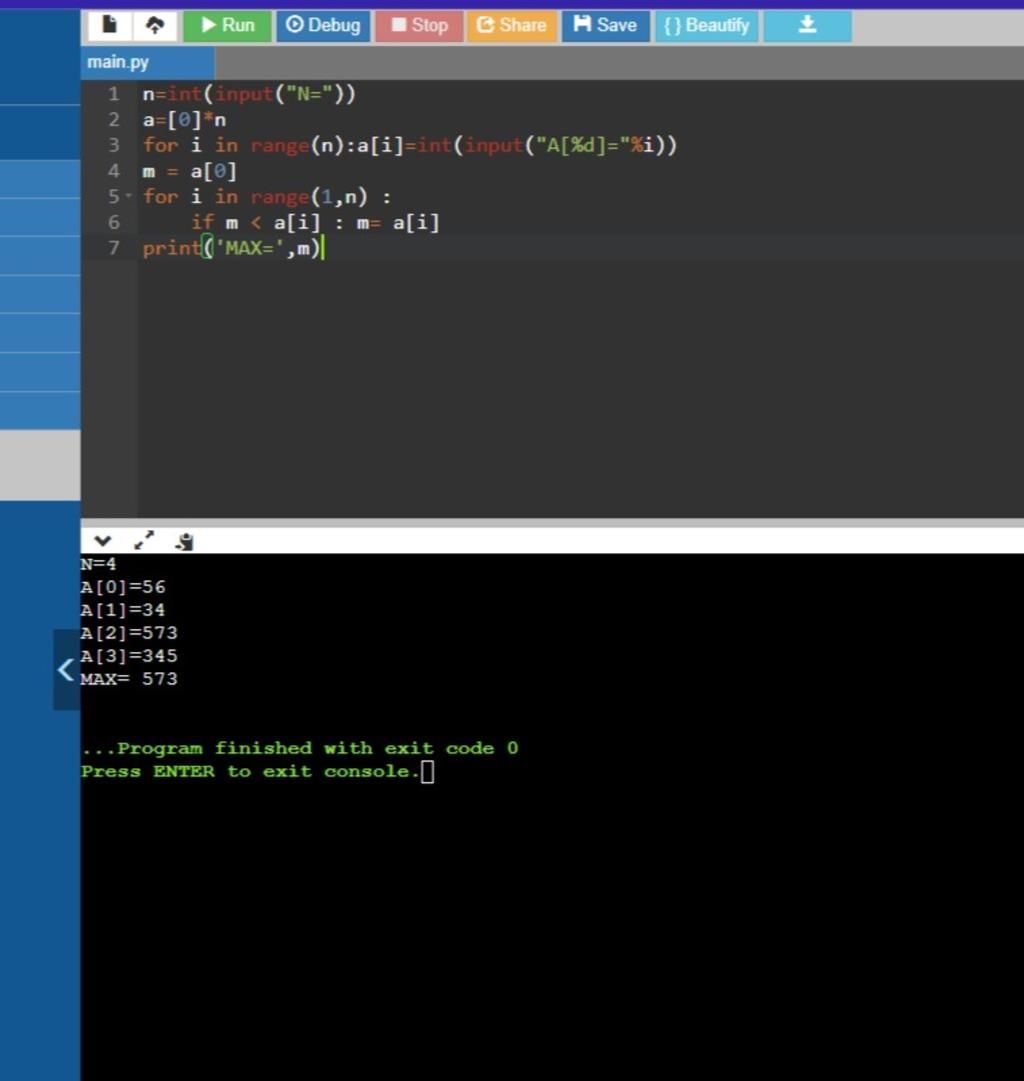
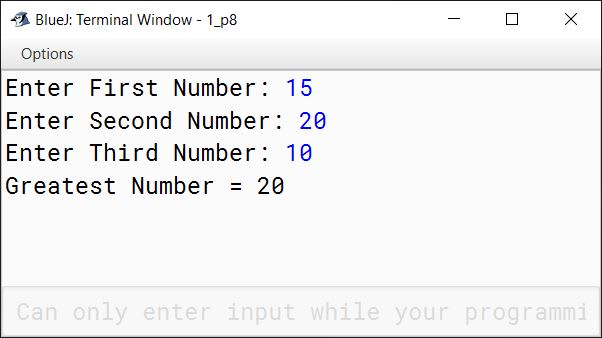
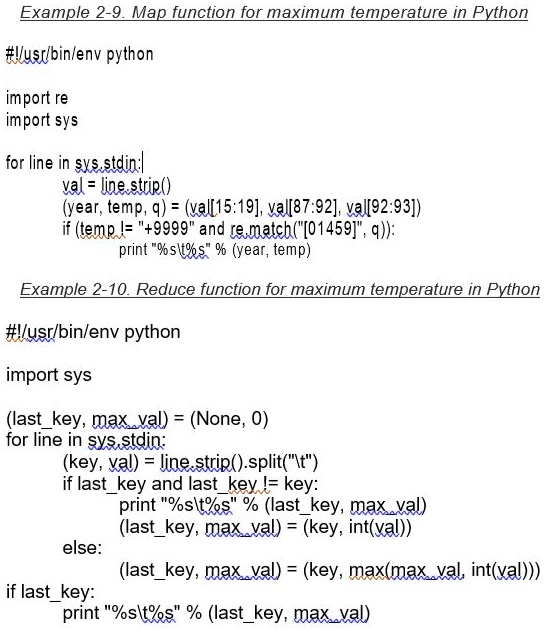
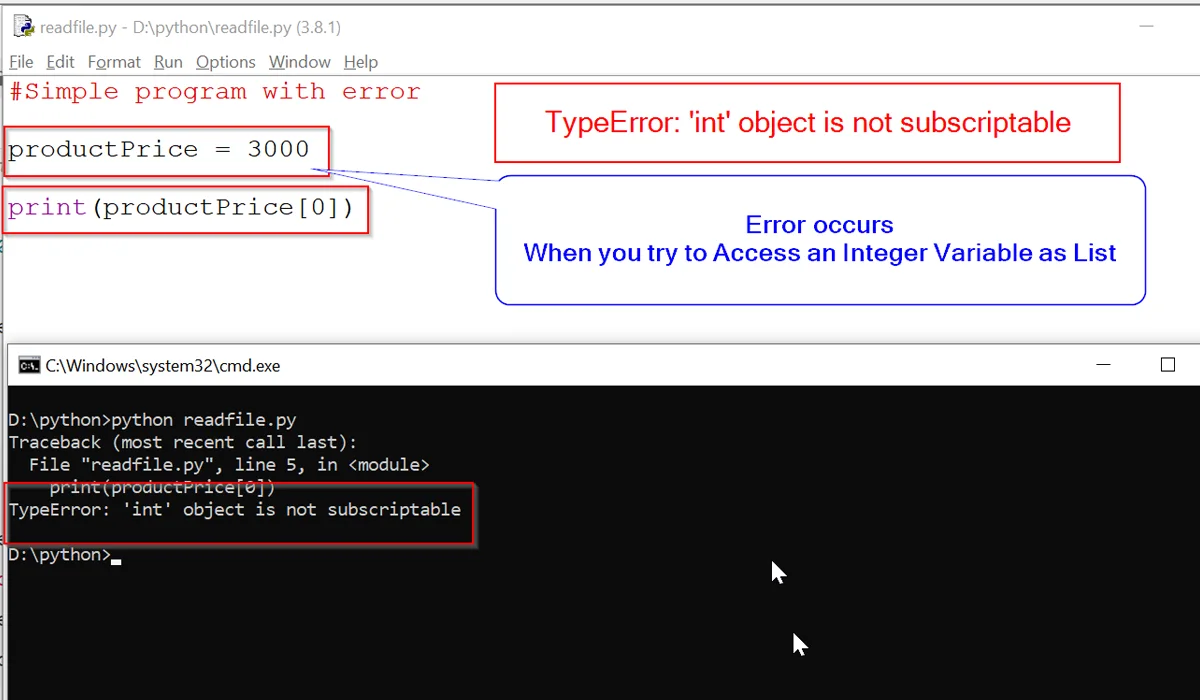
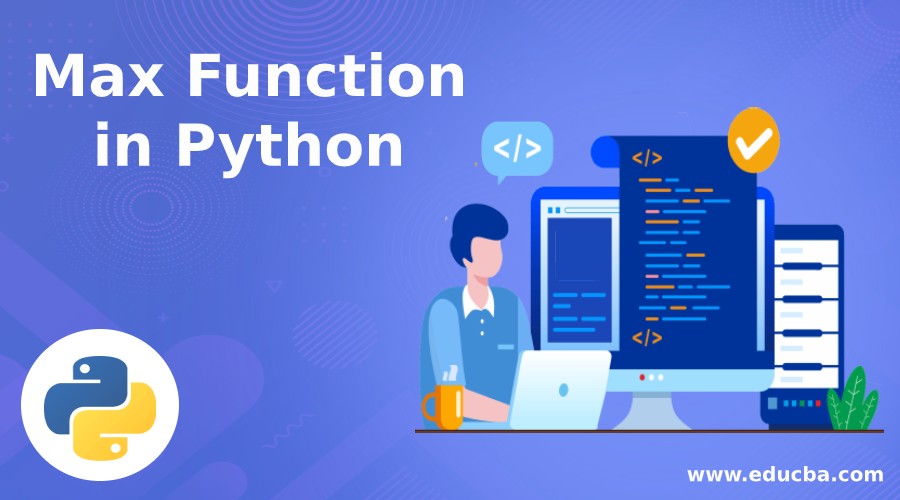
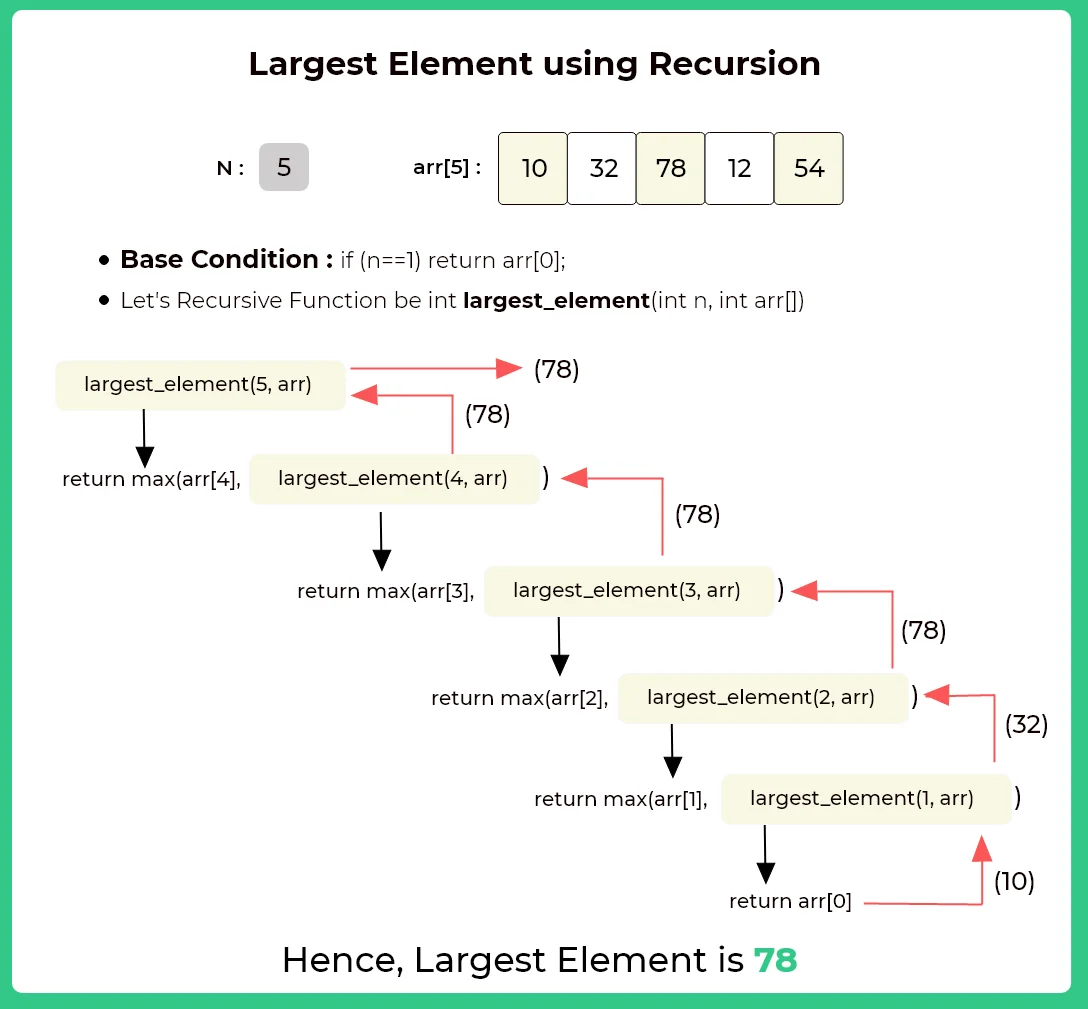
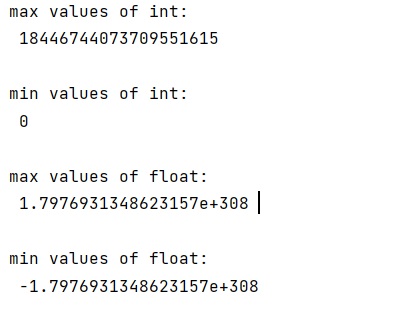
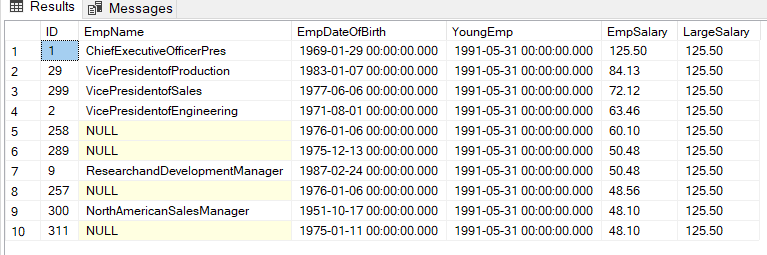
![Understanding Float in Python [with Examples] Understanding Float In Python [With Examples]](https://www.simplilearn.com/ice9/free_resources_article_thumb/FloatInPython_12.png)
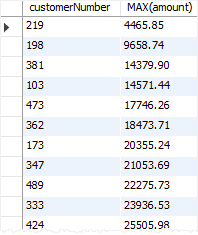
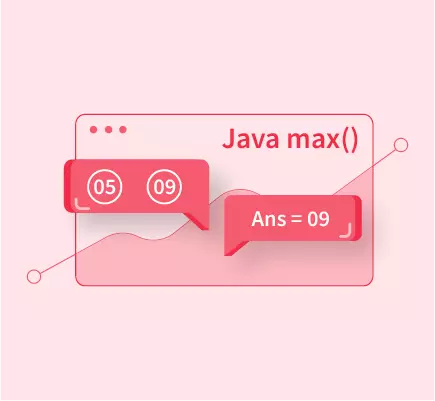


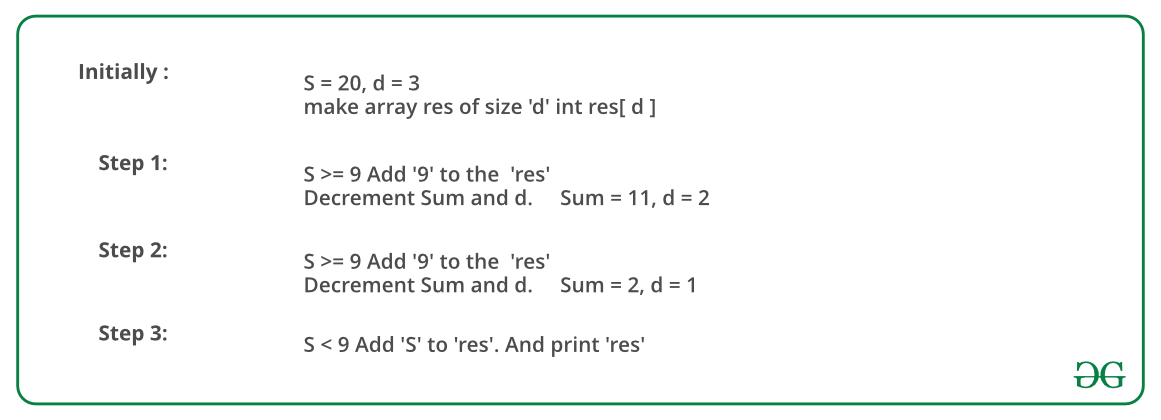




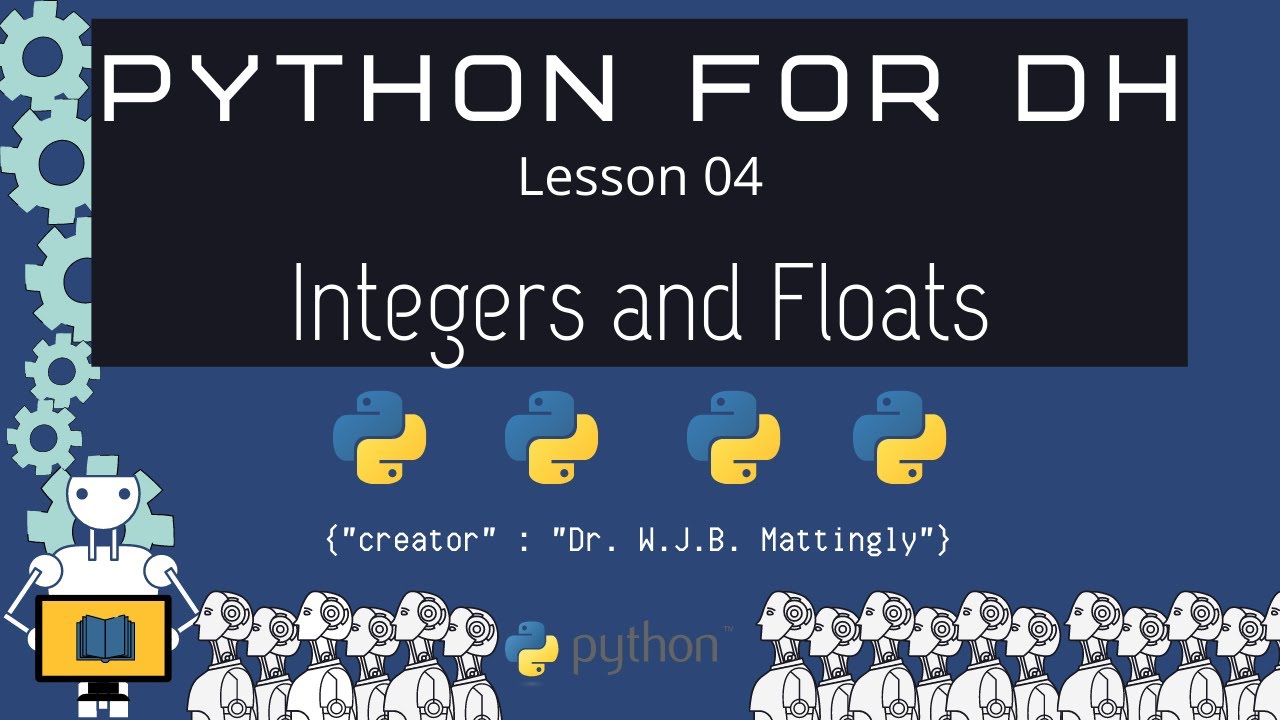
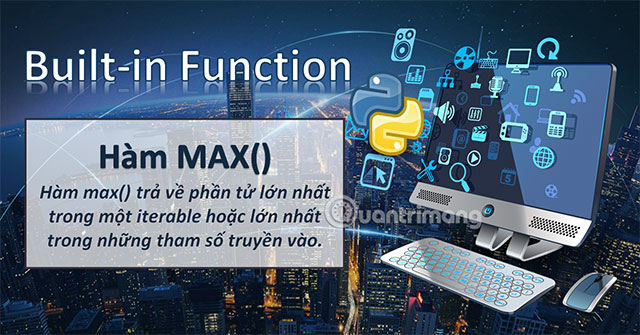
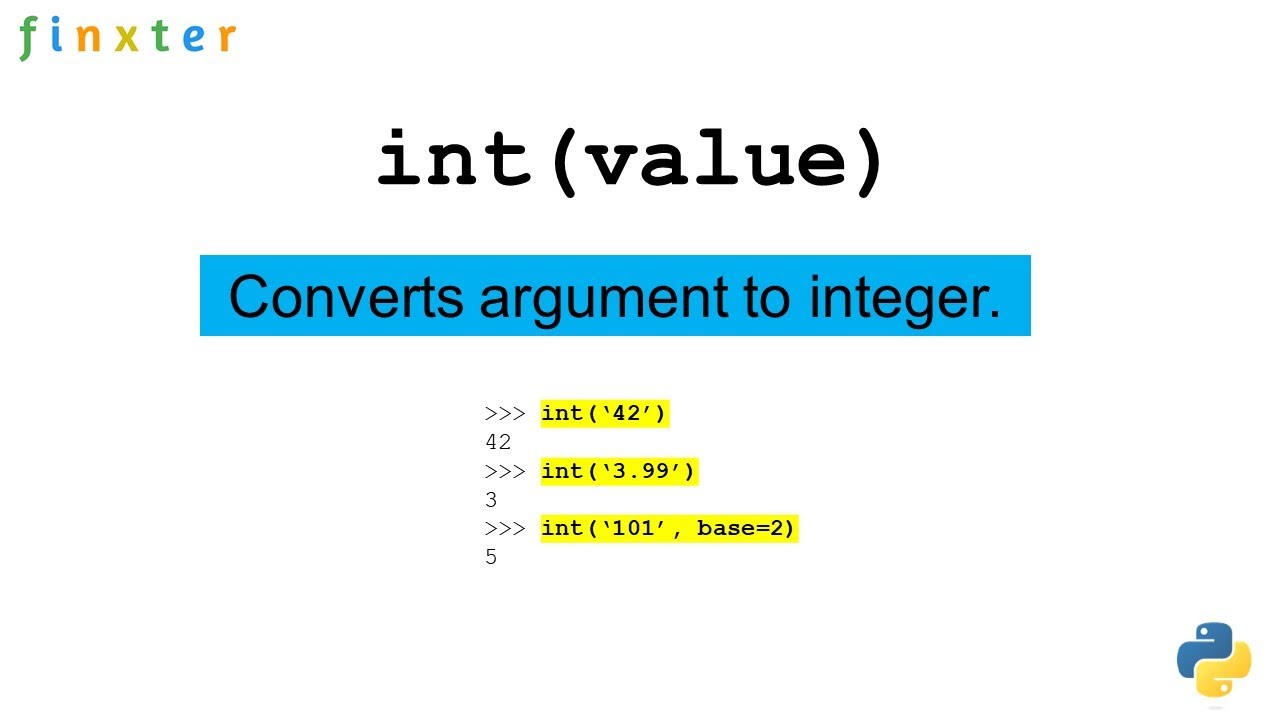

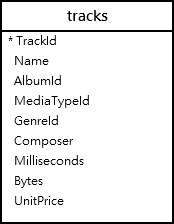
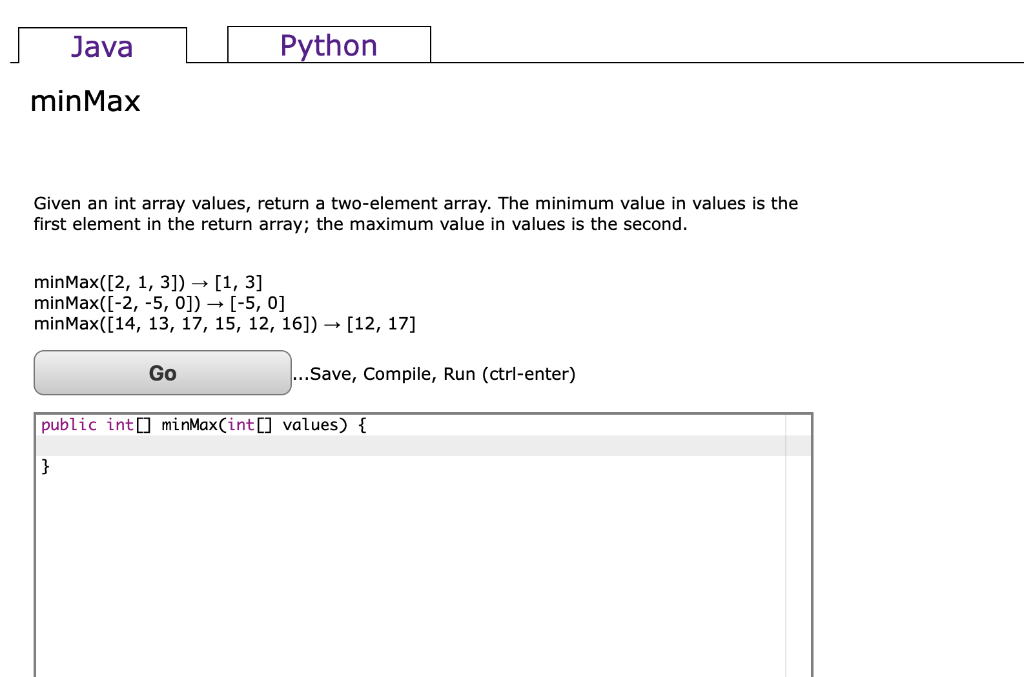
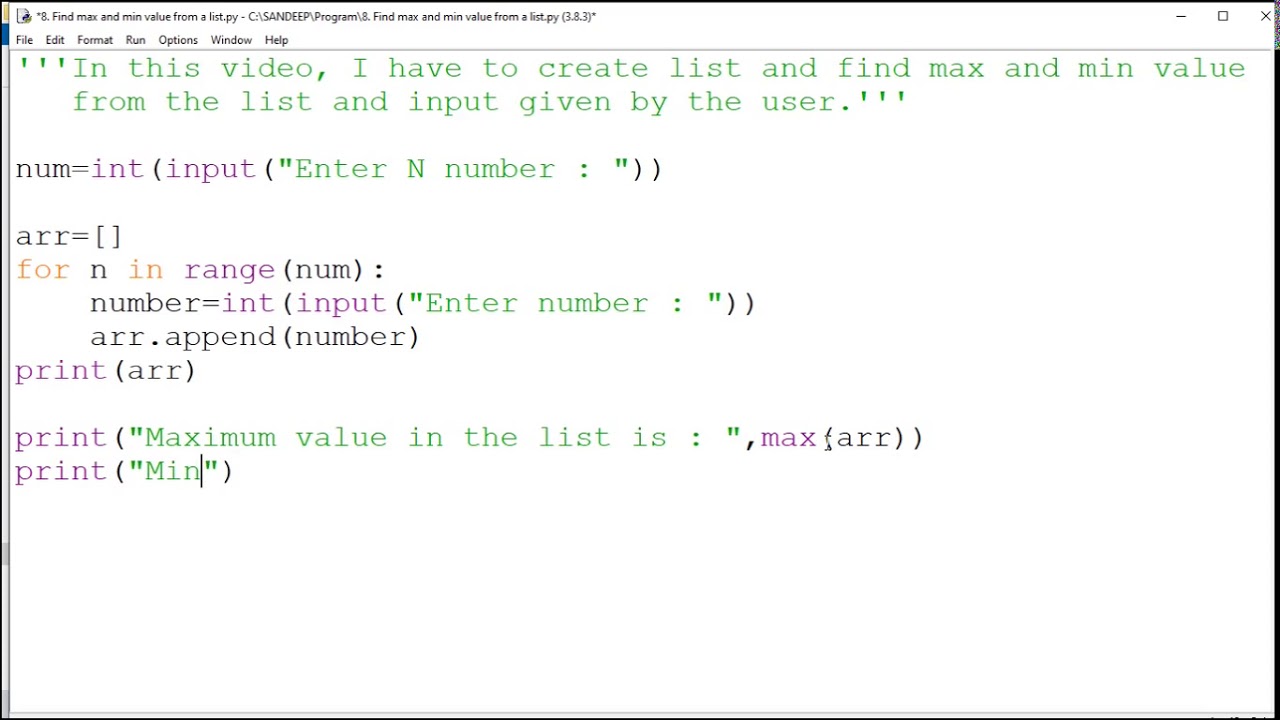
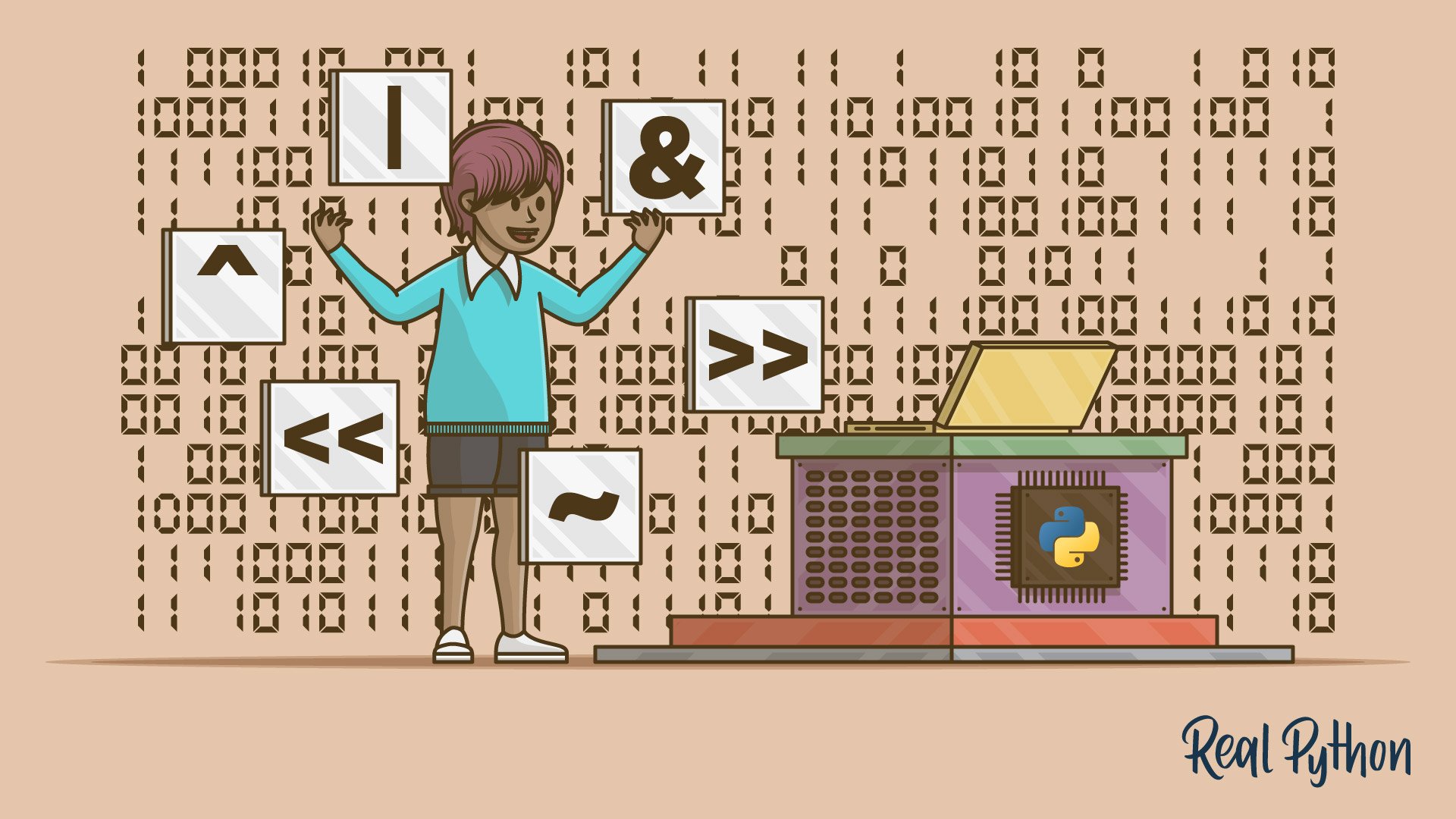
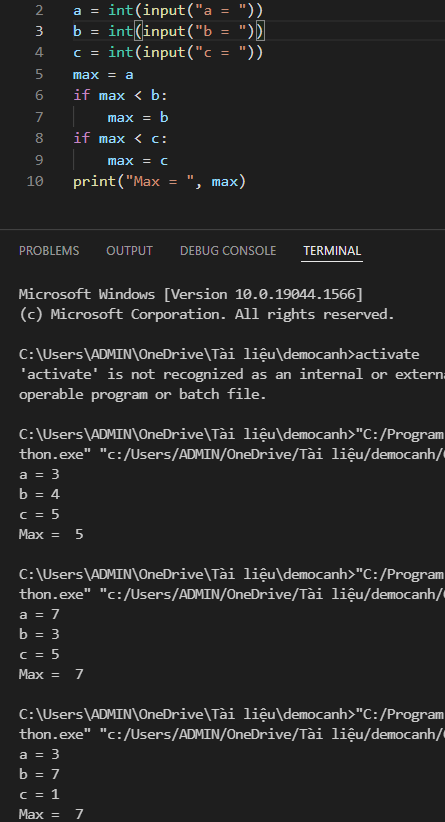
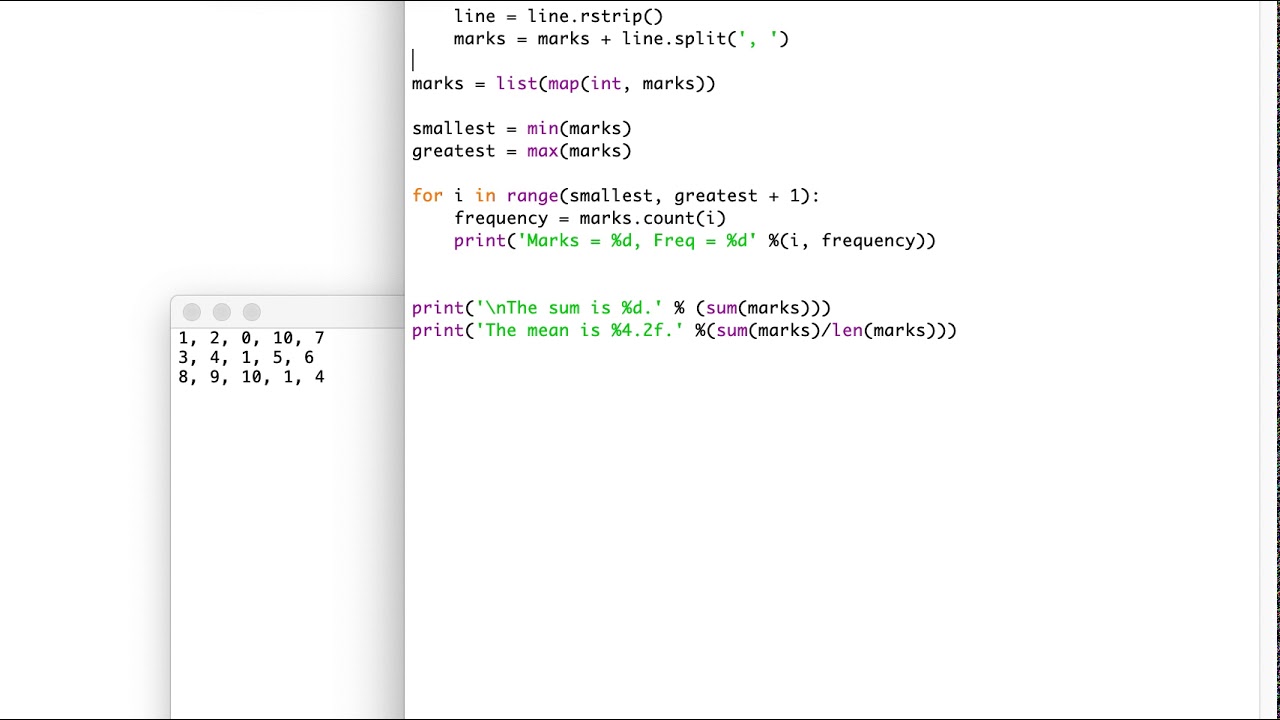
Article link: max int in python.
Learn more about the topic max int in python.
- Integer (int) has no max limit in Python3 – nkmk note
- Max Int in Python: Understanding Maximum Integer Limits
- What is the maximum value for an int32? – Stack Overflow
- Python NumPy: Data Types List – ProgramsBuzz
- INT_MAX and INT_MIN in C/C++ and Applications – GeeksforGeeks
- Int Max in Python – Maximum Integer Size – freeCodeCamp
- Maximum and Minimum values for ints – python – Stack Overflow
- sys.maxint in Python – GeeksforGeeks
- Python Max integer – Linux Hint
- Python Maximum/Minimum Integer Value (with Examples)
- Max Int in Python: Understanding Maximum Integer Limits
- Python max int: Maximum Integer Value in Python – AppDividend
- Max Int in Python | Delft Stack
- Get Maximum & Minimum values for ints in Python – thisPointer
See more: https://nhanvietluanvan.com/luat-hoc