Max Arg Is An Empty Sequence
Before delving into the concept of “max arg is an empty sequence,” it’s necessary to understand what an empty sequence is. In computer programming, a sequence refers to an ordered collection of elements. This could be in the form of a list, tuple, string, or any other iterable object.
An empty sequence, as the name suggests, is a sequence with no elements. In simpler terms, it is a sequence that is void of any data. It can be visualized as an empty container waiting to be filled. While it may seem trivial, the concept of an empty sequence plays a significant role in programming, particularly when it comes to determining the maximum value within a sequence.
Defining a Sequence
A sequence, as mentioned earlier, is an ordered collection of elements. These elements can be of any data type, such as numbers, strings, or even objects. The order is essential in sequences, as it determines how the elements are arranged and accessed. For instance, in a list, the order is preserved as each element has an index corresponding to its position within the list.
Understanding the Concept of Maximum Value in a Sequence
The maximum value in a sequence refers to the largest element present within the sequence. It is also known as the maximum element. Determining the maximum value is a common task in programming, as it allows us to extract meaningful insights or perform operations based on the largest element.
The Role of the max() Function in Finding the Maximum Value
In many programming languages, including Python, a built-in function named “max()” is available to find the maximum value within a sequence. This function takes an iterable as its argument and returns the maximum element present in that iterable.
Here’s an example of how the max() function can be used in Python:
“`python
numbers = [5, 8, 3, 2, 9, 4]
max_value = max(numbers)
print(max_value) # Output: 9
“`
In this example, the max() function takes the list of numbers as its argument and returns the maximum value, which is 9. The max() function works efficiently with non-empty sequences, but what happens if the sequence is empty?
Exploring Scenarios Where the Sequence is Empty
Now, let’s consider the scenario where the sequence is empty. An empty sequence does not contain any elements, and therefore, there is no maximum value to be found. When attempting to find the maximum value in an empty sequence, an error may occur.
Potential Causes of an Empty Sequence Error
An empty sequence error can occur due to a variety of reasons. Some possible causes include:
1. Incorrect input: It’s possible that the wrong variable or data structure is being used as the sequence, resulting in an empty sequence error.
2. Failure to populate the sequence: If the sequence is expected to be filled with values but is left empty due to a coding mistake or external issues, an empty sequence error can occur.
3. Logic errors: If the logic used to create or populate the sequence is flawed, it can result in an empty sequence error.
Effect of an Empty Sequence on the max() Function
When the max() function is applied to an empty sequence, it raises a ValueError, specifically stating that “max arg is an empty sequence.” This is the default behavior of Python’s max() function when an empty sequence is encountered, indicating that there is no maximum value to return.
Handling an Empty Sequence Error
To handle an empty sequence error, you can incorporate exception handling techniques. In Python, you can use a try-except block to catch the ValueError and define alternative actions to be taken in case of an empty sequence.
Here’s an example of how to handle an empty sequence error using exception handling:
“`python
numbers = []
try:
max_value = max(numbers)
# Perform operations on max_value
print(max_value)
except ValueError:
# Handle the case when the sequence is empty
print(“The sequence is empty.”)
“`
In this example, an empty list is assigned to the variable “numbers.” The try block attempts to find the maximum value using the max() function, but since the sequence is empty, a ValueError is raised. The except block catches the error and executes the alternative code, which prints the message “The sequence is empty.”
Best Practices to Prevent an Empty Sequence Error
To prevent an empty sequence error, it’s crucial to incorporate defensive programming practices. Here are some best practices to consider:
1. Input validation: Ensure that the sequence passed to the max() function is valid and non-empty before using the function. This can be done by explicitly checking the length of the sequence or using conditional statements to verify its contents.
2. Error handling: Implement try-except blocks to catch and handle the ValueError raised by the max() function when an empty sequence is encountered. By defining appropriate actions in case of an empty sequence, you can prevent the error from interrupting the program’s flow.
3. Documentation: Clearly document the expected behavior and requirements of the functions or methods that rely on sequences. Make it explicit whether empty sequences are allowed as inputs and how they should be handled.
4. Test cases: Create comprehensive test cases that cover different scenarios, including both non-empty and empty sequences. This will help identify any issues or potential errors related to empty sequences and ensure the correctness of your code.
In conclusion, an empty sequence refers to a sequence that is void of any elements. When attempting to find the maximum value in an empty sequence using the max() function, a ValueError is raised, signaling that there is no maximum value to return. By implementing proper error handling techniques and following best practices, you can effectively handle empty sequence errors and prevent them from disrupting your program’s execution.
FAQs:
Q: What happens when you try to get the argmax of an empty sequence?
A: When attempting to get the argmax (index of the maximum value) of an empty sequence, an error may occur, similar to the empty sequence error encountered while using the max() function.
Q: How does Python’s “max()” handle an empty list?
A: Python’s “max()” function raises a ValueError with the message “max arg is an empty sequence” when attempting to find the maximum value of an empty sequence.
Q: Can an empty sequence cause issues in other programming languages?
A: Yes, an empty sequence can potentially cause issues in other programming languages as well. However, the specific behavior may vary depending on the language and the functions or methods used to handle sequences. It’s always crucial to understand the behavior of the functions you are using and handle empty sequences accordingly.
Q: Are there any known performance implications of handling empty sequences?
A: Handling empty sequences itself does not have significant performance implications. However, it’s always good practice to validate inputs and perform necessary checks before utilizing functions or methods on sequences to ensure efficient and error-free execution.
Getting Valueerror: Max() Arg Is An Empty Sequence On A Python Script In The Field Calculator In…
What Does Max Arg Is An Empty Sequence Mean?
The “max arg is an empty sequence” statement is a common error message encountered in programming languages such as Python. It typically occurs when we attempt to find the index of the maximum element in an empty sequence (list, tuple, etc.). This article will delve into the details of this error message, explaining its meaning and providing insights into possible causes and solutions.
Understanding the Error Message
When you encounter the error message “max arg is an empty sequence,” it means you are trying to call a max function and provide an empty sequence. The max function is used to find the maximum element within an iterable (such as a sequence). However, when the iterable is empty, there are no elements to compare, resulting in an invalid operation. As a result, the program raises an exception and displays the error message.
Causes of the Error
The primary cause of the “max arg is an empty sequence” error is attempting to find the maximum element in an empty sequence. This situation can occur due to various reasons, including:
1. Incorrect input handling: When a program receives user inputs or operates on data, there might be situations where the data is empty when it shouldn’t be. Neglecting to handle these scenarios properly can lead to this error.
2. Empty containers: If you are using containers like lists or tuples and not properly initializing them with elements, attempting to find the maximum element will result in this error.
3. Faulty loops: Loops play an essential role in working with sequences, and not controlling their termination conditions correctly can lead to empty sequences being passed to the max function.
Solving the Error
To resolve the “max arg is an empty sequence” error, you need to handle empty sequences appropriately and ensure they are not passed to the max function. Here are some strategies to consider:
1. Input validation: When accepting input from users or external sources, incorporate checks to ensure the input is not empty. You could use conditionals like ‘if’ statements to prompt the user to enter valid data before attempting to find the maximum element.
2. Conditionals: Utilize conditionals like ‘if’ statements or try-except blocks to check if the sequence is empty before applying the max function. If the sequence is empty, you can either prompt the user to provide valid data or assign a default value depending on the program’s requirements.
3. Check container status: Before attempting to find the maximum element in a container, verify that it is not empty. If the container might be empty due to certain conditions or operations, add appropriate checks to handle this scenario.
4. Review loop conditions: If you are using loops to process sequences, ensure that the loop termination conditions account for the possibility of an empty sequence. By employing proper conditional checks, you can prevent empty sequences from being passed to the max function.
FAQs
Q: Are there similar error messages for other operations on empty sequences?
A: Yes, depending on the programming language and operation, you might encounter similar error messages, such as “index out of range” when accessing an element in an empty sequence or “division by zero” when performing division on empty sequences.
Q: Is there a way to avoid these error messages altogether?
A: While it is not possible to prevent all runtime errors, following best programming practices, validating input, and applying proper conditional checks can significantly reduce the occurrence of such errors.
Q: Can I use the max function on sequences with non-numeric elements?
A: Yes, the max function is not limited to numeric elements. It can also be used to find the maximum element based on lexicographical or custom comparison rules for different data types.
Q: Does this error message only occur in Python?
A: No, this error message can occur in other programming languages as well. The exact wording may vary, but the concept remains the same.
Q: How can I debug this error message efficiently?
A: Debugging can be done by using print statements or a debugger to examine the state of variables leading to the error. By isolating the problematic code and inspecting the values being passed, you can identify the cause of the error more effectively.
In conclusion, the error message “max arg is an empty sequence” occurs when attempting to find the maximum element in an empty sequence. To prevent this error, proper input validation, handling of empty containers and loops, and applying appropriate conditionals can be implemented. By understanding the causes and solutions outlined in this article, programmers can efficiently troubleshoot and prevent this error from occurring in their code.
What Does Empty Sequence Mean?
In mathematics, sequences are an essential concept that we encounter in various areas of study. A sequence is an ordered list of numbers or terms that follow a specific pattern or rule. However, in some cases, we may come across the term “empty sequence.” This article aims to explore what an empty sequence is, its properties, and its significance in mathematics.
Understanding the Basics of Sequences
Before delving into the concept of an empty sequence, it is crucial to comprehend the fundamentals of sequences. A sequence is a set of numbers arranged in a specific order. For instance, the sequence {1, 3, 5, 7, 9} represents an ordered list of odd numbers progressing by twos. Each number in the sequence is called a term, denoted by the symbol ‘a’ followed by a subscript indicating its position.
Sequences play a significant role in various mathematical fields such as calculus, number theory, and algebra. They aid in analyzing patterns, solving equations, and making predictions. Sequences can be categorized into different types based on their behavior, such as arithmetic, geometric, and Fibonacci sequences, to name a few.
The Concept of an Empty Sequence
An empty sequence, often referred to as a null sequence or a vacuous sequence, is one that contains no elements, meaning it is void or devoid of terms. In other words, it is a sequence with zero terms. Mathematically, it is denoted by the symbol ‘∅’ or ‘()’. Unlike other sequences, it does not possess a defined pattern since it lacks any elements to establish a rule or structure.
Properties of the Empty Sequence
While the empty sequence may seem conceptually obscure, it possesses several unique properties worth noting. Here are a few fundamental characteristics of the empty sequence:
1. Length: As the name suggests, the empty sequence has a length of zero. It does not contain any terms, making it the shortest sequence possible.
2. Subsequences: Since the empty sequence lacks any elements, it cannot have subsequences. Consequently, any sequence can be considered a subsequence of the empty sequence.
3. Sum and Product: The sum of an empty sequence is 0, as there are no terms to add. Similarly, the product of an empty sequence is 1, as multiplying any number by 1 yields the original number.
4. Notation: The empty sequence is denoted by ‘∅’ or ‘()’, differentiating it from other sequences that contain at least one element.
Significance of the Empty Sequence
At first glance, one may question the relevance of an empty sequence in mathematics. However, the concept holds theoretical significance, especially in the field of set theory. In set theory, the empty sequence corresponds to the empty set, which is a fundamental concept in understanding the foundations of mathematical logic and proofs.
In addition, the empty sequence helps establish the identity element in mathematical operations. For example, considering the empty sequence as the base case in an induction proof allows for the establishment of statements that hold true for any sequence.
FAQs
Q: Can a sequence contain no elements and still be considered a sequence?
A: No, a sequence, by its definition, requires at least one element. An empty sequence, however, is a distinct concept in mathematics.
Q: Is the empty sequence unique?
A: Yes, the empty sequence is unique. There is only one empty sequence, denoted by ‘∅’ or ‘()’.
Q: Does the empty sequence have a defined pattern?
A: No, the empty sequence does not possess a defined pattern since it contains no elements. It lacks the necessary information to establish a rule or structure.
Q: Can the empty sequence be a subsequence of any sequence?
A: Yes, any sequence can be considered a subsequence of the empty sequence since it has no terms.
Q: How is the empty sequence used in mathematical proofs?
A: In mathematical proofs, the empty sequence is often used as a base case or as part of induction to establish statements that hold true for any sequence.
Conclusion
In summary, an empty sequence is a sequence without any terms, denoted by ‘∅’ or ‘()’. While it may appear as an abstract or insignificant concept at first, the empty sequence plays a crucial role in set theory and mathematical proofs. Understanding its properties and significance enhances our knowledge of sequences and their applications in various mathematical disciplines.
Keywords searched by users: max arg is an empty sequence Attempt to get argmax of an empty sequence, Python max empty list
Categories: Top 21 Max Arg Is An Empty Sequence
See more here: nhanvietluanvan.com
Attempt To Get Argmax Of An Empty Sequence
Introduction (80 words):
The concept of finding the argmax, which represents an element with the highest value in a collection, plays a crucial role in various fields. However, when dealing with an empty sequence, attempting to determine the argmax can lead to unexpected outcomes. In this article, we will delve deep into this subject, exploring the reasons behind such behavior, possible solutions, and the implications for programming and data analysis.
Understanding argmax (150 words):
Before diving into the issue of finding the argmax of an empty sequence, it is essential to grasp the concept of argmax itself. The argmax function is commonly used in mathematics, computer science, and statistics. It helps identify the element with the highest value within a given collection, often represented in an array, list, or any other sequence.
Generally, finding the argmax involves iterating over the sequence, comparing the values of each element, and returning the index or the element itself associated with the highest value. This fundamental operation provides valuable insights and facilitates decision-making in various contexts, including optimization problems, machine learning algorithms, and data analysis.
The Empty Sequence Dilemma (200 words):
When confronted with an empty sequence, the task of determining the argmax becomes a challenge. Due to the absence of any elements, no values exist to compare, and the argmax calculation may yield unexpected outcomes.
Attempting to obtain the argmax of an empty sequence most commonly results in a “ValueError” or “IndexError” depending on the programming language or software used. These error messages serve as safeguards, preventing any unintended manipulation or faulty calculations based on nonexistent data.
Reasons for Error Messages (250 words):
The occurrence of error messages when trying to compute the argmax of an empty sequence is rooted in the underlying logic of these functions. The implementation of argmax typically relies on an assumption that there exists at least one element in the sequence. This assumption ensures that comparisons can be made, determining which element has the highest value.
However, when faced with an empty sequence, the absence of any values hinders the comparison process, resulting in an inconsistency. The software or programming language detects this discrepancy and raises an error accordingly, indicating that the input does not meet the expected criteria.
Potential Solutions (300 words):
While obtaining the argmax of an empty sequence inherently results in errors, programmers can implement different strategies to handle this scenario. It is crucial to select an approach based on the specific requirements of the task at hand.
1. Raising an Exception: This method entails explicitly raising an exception when an empty sequence is encountered. By doing so, the program indicates that finding the argmax in such a situation is not feasible.
2. Assigning a Default Value: Instead of raising an error, one can select a default value to return when dealing with an empty sequence. This approach allows the program to continue executing without disruptions.
3. Using Conditional Statements: In certain cases, it may be preferable to include conditional statements that check for empty sequences before attempting the argmax calculation. By employing these statements, the program can bypass any problematic situations and proceed accordingly.
4. Modifying the argmax Function: Occasionally, it may be necessary to modify the argmax function itself to include a check for empty sequences. This adaptation ensures that the program can handle these cases appropriately rather than producing errors.
Frequently Asked Questions (200 words):
1. Can the argmax function be used on all types of sequences?
Yes, the argmax function can be applied to various types of sequences, including arrays, lists, tuples, and other collections, as long as they contain comparable elements.
2. Are there any exceptions to the error messages when finding the argmax of an empty sequence?
No, generating error messages is the typical behavior of programming languages and software when attempting to determine the argmax of an empty sequence. This behavior is beneficial to prevent incorrect results based on non-existent data.
3. Can the strategies mentioned for handling empty sequences be combined?
Yes, programmers often combine multiple strategies to address empty sequence scenarios based on the specific requirements of their tasks. This can involve using conditional statements along with raising exceptions or applying default values.
4. How does handling an empty sequence affect overall program performance?
When dealing with empty sequences, it is imperative to consider the impact on program performance. Incorrect handling techniques, such as infinite loops or excessive processing, can result in performance degradation.
Conclusion (50 words):
Successfully finding the argmax of an empty sequence requires careful consideration and cautious handling. Being aware of the inherent limitations and implementing appropriate strategies ensures smoother and error-free execution while dealing with such scenarios.
Python Max Empty List
In Python, the `max()` function is widely used to find the highest value in a list or iterable. However, what happens when we try to find the maximum value in an empty list? This article will delve into the specifics of Python’s behavior when using `max()` on an empty list, explain its implications, and address frequently asked questions regarding this concept.
Understanding `max()` and Empty Lists
Before diving into the specifics of `max()` and empty lists, let’s start with a brief overview of the `max()` function itself. The `max()` function returns the largest item in an iterable or the largest of two or more arguments. It is a built-in Python function that can be used with various data types, including numbers, strings, and even custom objects. When used with a list as an argument, `max()` returns the item with the highest value according to the default or provided comparison criteria.
Now, let’s shift our focus to empty lists. An empty list, as the name suggests, is a list with no elements. It is represented by `[]` in Python and can be created using `empty_list = []`. Empty lists are commonly used in scenarios where data will be populated dynamically or when you want to initialize a list without any values. However, working with empty lists requires special attention, particularly when using functions like `max()`.
Python’s Behavior when using `max()` on an Empty List
When `max()` is used on an empty list, Python raises a `ValueError` exception. The `ValueError` indicates that the list does not contain any elements, and therefore, there is no maximum value to determine. This behavior ensures that you don’t inadvertently get incorrect or unexpected results when using `max()` with an empty list.
Handling `ValueError` Exceptions
To handle a `ValueError` exception raised when calling `max()` on an empty list, you can use a `try-except` block. This allows you to gracefully handle the exception without causing your program to terminate abruptly. Here’s an example:
“`python
try:
maximum_value = max(empty_list)
except ValueError:
print(“The list is empty!”)
“`
In this code snippet, `max()` is called on the `empty_list`, and if a `ValueError` occurs, the message “The list is empty!” will be printed. By utilizing `try-except` blocks, your program will remain stable even when dealing with empty lists.
Common FAQs about `max()` and Empty Lists
1. Q: Why does Python raise a `ValueError` exception when calling `max()` on an empty list?
A: The `max()` function relies on the presence of elements to determine the maximum value. As an empty list has no elements, there is no valid maximum value to return. Python raises a `ValueError` exception to indicate this scenario.
2. Q: Can the behavior of `max()` on an empty list be modified?
A: No, the behavior of `max()` on an empty list cannot be modified. It is defined by Python and cannot be changed. Raising a `ValueError` exception is a deliberate decision to prevent code from producing erroneous results.
3. Q: Is there an alternative to using `try-except` blocks to handle the `ValueError` raised by `max()`?
A: Yes, you can check if a list is empty before calling `max()` to avoid the exception. For example:
“`python
if empty_list:
maximum_value = max(empty_list)
else:
print(“The list is empty!”)
“`
By checking the truthiness of the list, you can safely utilize `max()` without encountering a `ValueError`.
4. Q: Are there any other Python functions that behave similarly to `max()` when used with an empty list?
A: Yes, Python has several built-in functions that raise a `ValueError` when operating on empty lists, such as `min()`, `sum()`, and `sorted()`. The intention is to ensure consistency and prevent inaccurate or invalid results.
In conclusion, using the `max()` function on an empty list in Python results in a `ValueError` exception being raised. This behavior is intentional and ensures that developers are aware of the absence of elements and don’t mistakenly rely on incorrect or misleading results. To handle this exception, `try-except` blocks can be employed, allowing for graceful handling of the error. By understanding Python’s behavior and utilizing proper exception handling, you can effectively work with empty lists and avoid any unexpected outcomes.
Images related to the topic max arg is an empty sequence
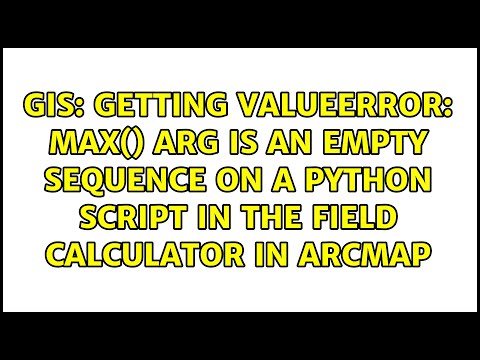
Found 16 images related to max arg is an empty sequence theme
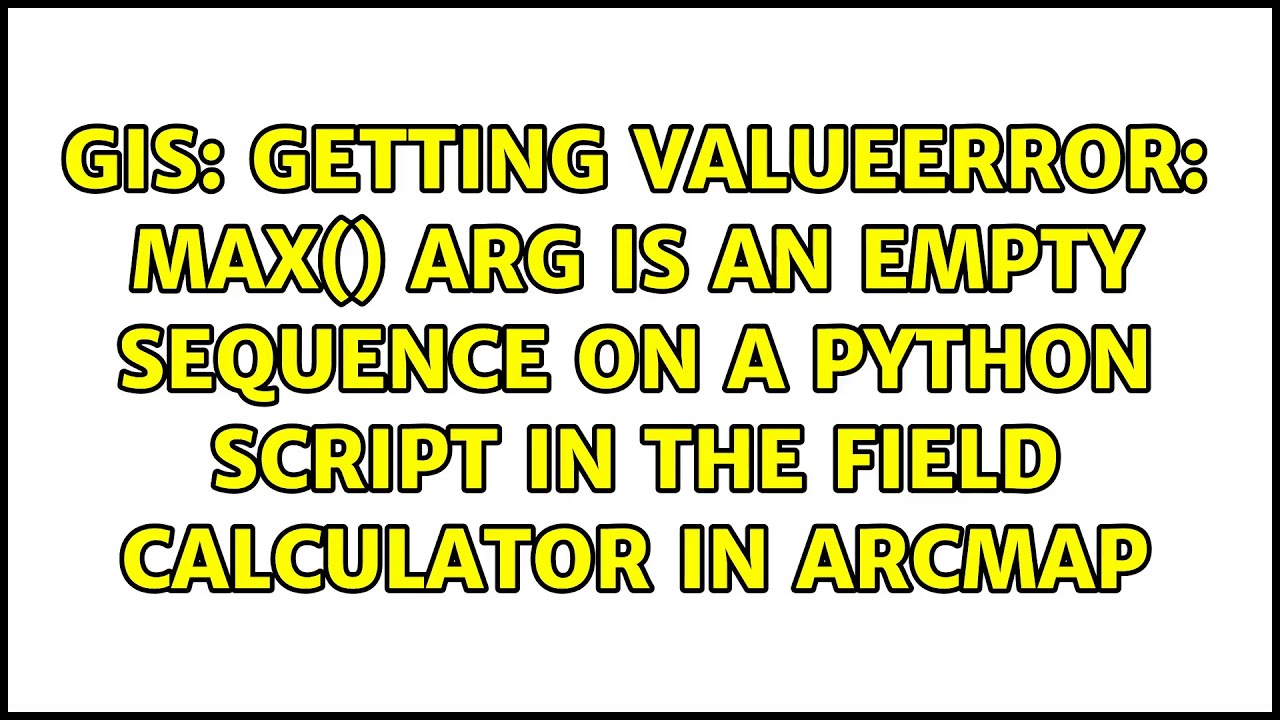

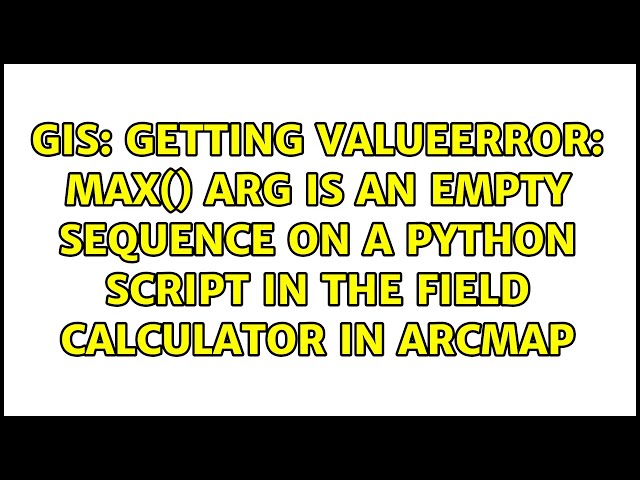

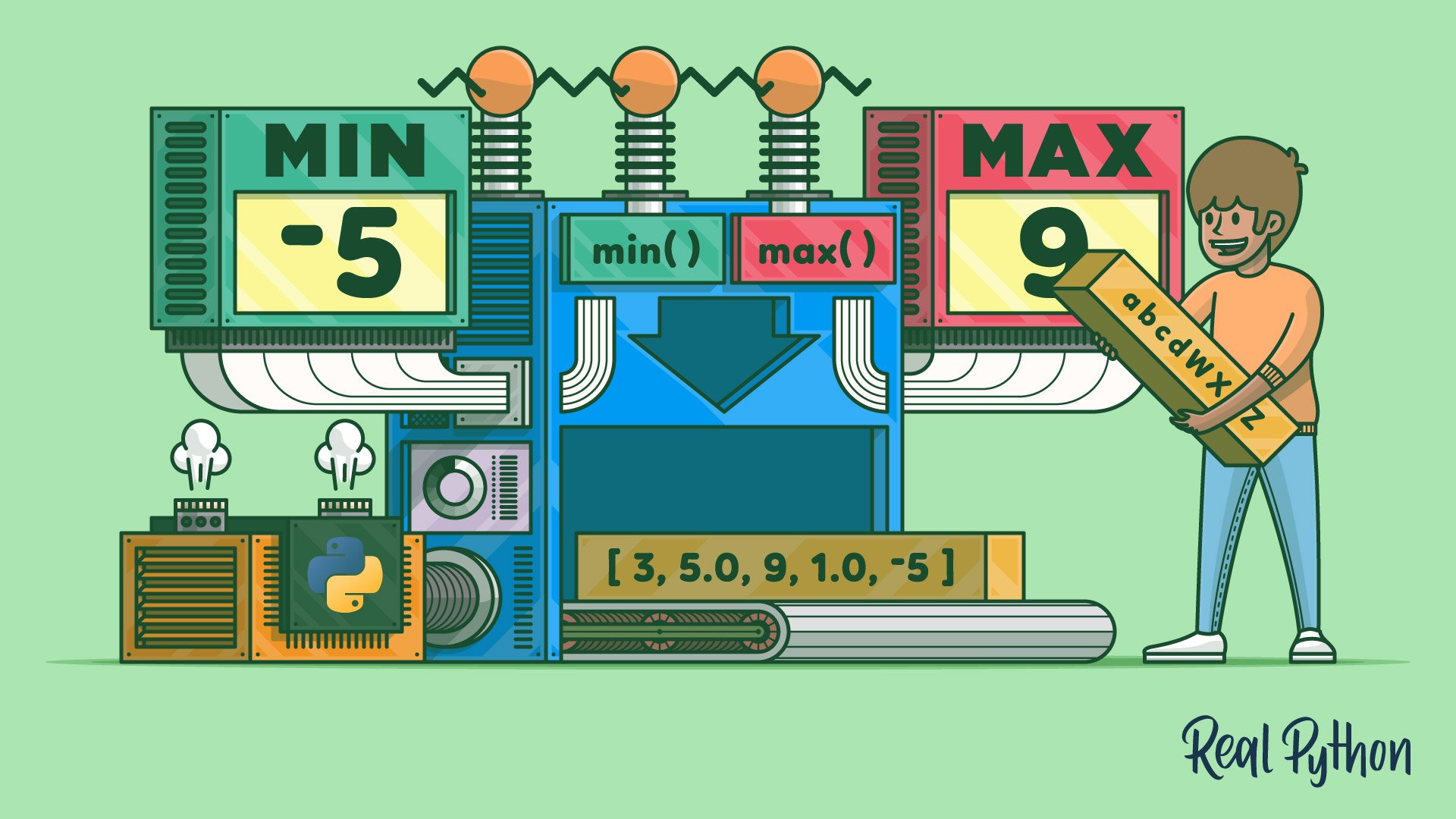

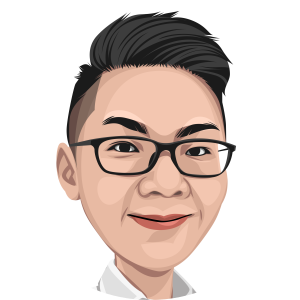
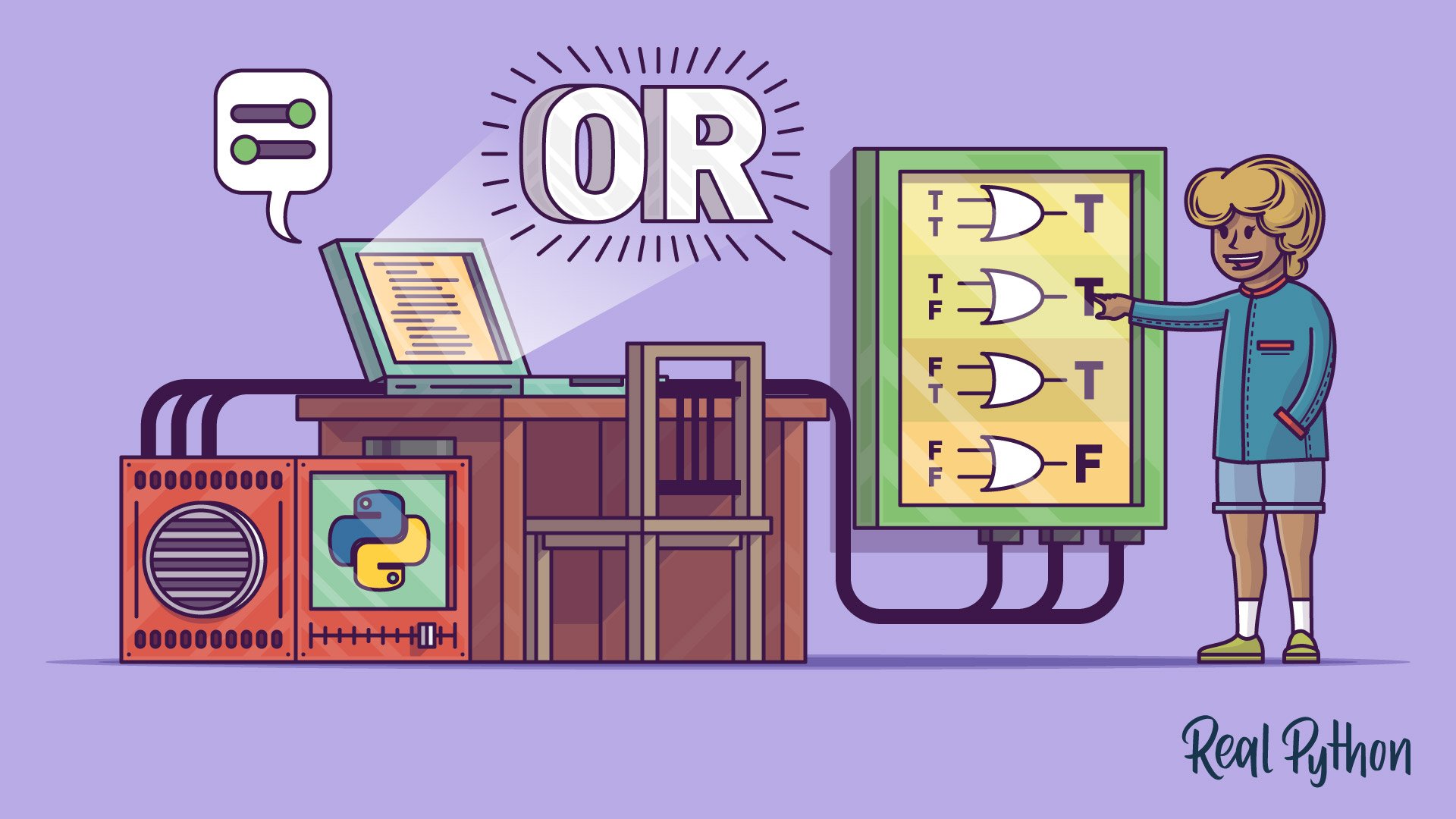
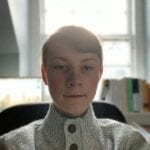


Article link: max arg is an empty sequence.
Learn more about the topic max arg is an empty sequence.
- ValueError: max()/min() arg is an empty sequence in Python
- ValueError: max() arg is an empty sequence – Stack Overflow
- Python ValueError: max() arg is an empty sequence Solution
- Definition:Sequence/Empty Sequence – ProofWiki
- Python max() function – ThePythonGuru.com
- max() Function in Python- Scaler Topics
- Python ValueError: max() arg is an empty sequence Solution
- Solve Python ValueError: max() arg is an empty sequence
- ValueError: max( ) arg is an empty sequence – STechies
- Valueerror max arg is an empty sequence – Itsourcecode.com
- “ValueError: max() arg is an empty sequence” / Using glob …
- why am i getting ValueError: max() arg is an empty sequence
See more: https://nhanvietluanvan.com/luat-hoc