Matlab Print To Console
Basic Syntax for Printing to the MATLAB Console
The basic syntax for printing to the MATLAB console is simple and intuitive. You can use the `disp` function to display data in the console. Here’s an example:
“`
disp(‘Hello, world!’);
“`
This command will print the string “Hello, world!” to the console. You can also print variables and constants by passing them as arguments to the `disp` function:
“`matlab
x = 42;
disp(x);
“`
In this case, the value of the variable `x` (42) will be printed to the console.
Printing Variables and Constants
To print variables and constants, you can use the `disp` function as shown in the previous example. However, if you want more control over the format of the output, you can use the `fprintf` function. With `fprintf`, you can specify the format string and provide the variables or constants as additional arguments.
Here’s an example that demonstrates how to print variables and constants using `fprintf`:
“`matlab
x = 3.14159;
y = ‘MATLAB’;
fprintf(‘The value of x is %.2f\n’, x); % Prints ‘The value of x is 3.14’
fprintf(‘Welcome to %s!\n’, y); % Prints ‘Welcome to MATLAB!’
“`
In the first `fprintf` call, the format specifier `%.2f` is used to print the value of `x` with two decimal places. In the second `fprintf` call, the variable `y` is printed as a string.
Formatting Output with MATLAB’s fprintf Function
MATLAB’s `fprintf` function provides powerful formatting options for a wide range of data types. Here are a few commonly used format specifiers:
– `%s`: Prints a string.
– `%d` or `%i`: Prints an integer.
– `%f`: Prints a floating-point number.
– `%e` or `%E`: Prints a number in scientific notation.
– `%c`: Prints a character.
You can also specify additional formatting options, such as the field width and precision. For example:
“`matlab
x = 123.456;
fprintf(‘The value of x is %10.2f\n’, x); % Prints ‘The value of x is 123.46’
“`
In this case, the format string `’%10.2f’` specifies that the value of `x` should be printed with a field width of 10 characters and two decimal places.
Printing Arrays and Matrices
Printing arrays and matrices in MATLAB is similar to printing variables. You can use the `disp` or `fprintf` function, depending on your formatting requirements.
Here’s an example that demonstrates how to print an array using `disp`:
“`matlab
A = [1 2 3; 4 5 6; 7 8 9];
disp(A);
“`
This will print the array `A` as follows:
“`
1 2 3
4 5 6
7 8 9
“`
If you want more control over the formatting, you can use `fprintf`:
“`matlab
fprintf(‘%d ‘, A);
fprintf(‘\n’);
“`
This will print the matrix `A` as a single row of space-separated values:
“`
1 2 3 4 5 6 7 8 9
“`
Customizing the Output Display
MATLAB provides various options to customize the output display, including controlling the number of displayed decimal places, aligning output, and adding separators. Here are a few examples:
“`matlab
x = 3.14159;
fprintf(‘The value of x is %.2f\n’, x); % Prints ‘The value of x is 3.14’
A = [1 2 3; 4 5 6; 7 8 9];
fprintf(‘Matrix A:\n’);
fprintf(‘%3d %3d %3d\n’, A); % Prints each element with a field width of 3 characters
“`
Redirecting Printed Output to a File
In MATLAB, you can redirect printed output to a file using the `diary` function. The `diary` function allows you to record all command window output to a file, including both the commands and their outputs.
To redirect output to a file, simply call `diary` and provide the filename as an argument:
“`matlab
diary(‘output.txt’);
disp(‘Hello, world!’);
diary off;
“`
This will create a file named ‘output.txt’ and save the console output to the file.
Controlling the Display Precision for Numerical Values
By default, MATLAB displays numbers with a limited precision. However, you can control the display precision using the `format` command. Here are a few commonly used options:
– `format short`: Displays a shorter, fixed-point format with 4 digits after the decimal point.
– `format long`: Displays a longer, fixed-point format with 15 digits after the decimal point.
– `format short e` or `format long e`: Displays numbers in scientific notation.
For example:
“`matlab
format short;
x = 3.14159;
disp(x); % Prints ‘3.1416’
format long;
disp(x); % Prints ‘3.14159000000000’
format short e;
disp(x); % Prints ‘3.1416e+00’
“`
Printing Messages with Color and Styling
To add color and styling to printed messages, MATLAB provides the `fprintf` function along with escape sequences. Escape sequences are special characters or character combinations that produce non-printable characters, such as colors, underlines, and bold text.
Here’s an example that demonstrates how to print colored text using escape sequences:
“`matlab
fprintf(‘\x1b[31mThis is red text!\x1b[0m\n’);
fprintf(‘\x1b[4mThis is underlined text!\x1b[0m\n’);
“`
In this case, the escape sequence `\x1b[31m` sets the text color to red, `\x1b[0m` resets the color to the default, and `\x1b[4m` underlines the text.
Advanced Techniques for Printing to the MATLAB Console
In addition to the basic printing techniques mentioned above, MATLAB provides several advanced techniques for printing to the console that are beyond the scope of this article. However, it’s worth mentioning a few of them:
– `matlab print string`: This command allows you to print a string variable to the console.
– `matlab print figure`: This command allows you to print a MATLAB figure to the console.
– `matlab print variable`: This command prints the value of a variable to the console.
– `matlab print array`: This command prints an array to the console.
– `simulink print to console`: This command prints Simulink output to the console.
– `matlab disp`: This function displays the value of an expression or variable.
– `matlab print command`: This command prints the syntax of a MATLAB command to the console.
– `matlab print screen`: This command saves the contents of the screen to a file or prints them to a printer.
FAQs
Q: Can I print formatted output using MATLAB?
A: Yes, you can use the `fprintf` function to print formatted output. It allows you to specify the format string and use format specifiers to control the display of variables.
Q: How can I redirect the printed output to a file?
A: You can use the `diary` function to redirect the printed output to a file. Simply call `diary` with the filename as an argument, perform the desired printing, and then call `diary off` to stop recording.
Q: How can I add color and styling to printed messages in MATLAB?
A: You can use escape sequences with the `fprintf` function to add color and styling to printed messages. Escape sequences are special characters or character combinations that produce non-printable characters, such as colors, underlines, and bold text.
Q: Are there any advanced techniques for printing to the MATLAB console?
A: Yes, MATLAB provides several advanced techniques for printing to the console, such as printing strings, figures, variables, arrays, Simulink output, displaying expressions or variables using `disp`, printing command syntax, and saving the screen contents to a file or printer.
In conclusion, printing to the MATLAB console is an essential aspect of programming in MATLAB. Understanding the basic syntax and various techniques for printing different types of data, customizing the output display, redirecting output to a file, controlling display precision, and adding color and styling to printed messages will greatly enhance your MATLAB programming experience. Whether you are a beginner or an experienced MATLAB user, mastering these techniques will make your code more readable and allow you to effectively communicate results.
Elly | Matlab . Print To The Console. Read Excel File To Table.
How To Print Value To Console Matlab?
Printing values to the console is an essential part of any programming language, including MATLAB. It allows you to display important information, debug your code, and communicate with the user. In this article, we will explore various ways to print values to the console in MATLAB.
MATLAB offers several functions and methods to print values, including disp, fprintf, and sprintf. Let’s delve into each method and explore their respective use cases.
1. disp Function:
The disp function is commonly used for displaying a value or a string to the MATLAB command window. It automatically adds a newline character at the end of the output, which separates subsequent outputs.
“`
disp(‘Hello, MATLAB!’)
“`
In the example above, the disp function is used to print the string “Hello, MATLAB!” to the console.
2. fprintf Function:
The fprintf function provides more control over the output format compared to the disp function. It allows you to include variables in the output, specify the number of decimal places, and format the output using specifiers.
“`
x = 3.14159;
fprintf(‘The value of x is %0.2f\n’, x);
“`
In this example, the fprintf function prints the value of the variable x to the console with two decimal places.
3. sprintf Function:
The sprintf function is similar to the fprintf function, but instead of printing the output to the console, it returns a formatted string that can be stored in a variable. This can be useful when you want to manipulate the output or include it in a larger string.
“`
x = 3.14159;
output = sprintf(‘The value of x is %0.2f’, x);
disp(output);
“`
Here, the sprintf function creates a formatted string that is stored in the variable output. The disp function is then used to print the output to the console.
4. Command-Line Variable Output:
Another way to print values to the console is by simply typing the name of the variable and pressing Enter. MATLAB will automatically display the value of that variable.
“`
x = 5;
x
“`
The console will show the value of x as 5.
Now that we have explored different methods to print values to the console, let’s address some frequently asked questions about printing in MATLAB.
FAQs:
Q: How can I print multiple values on the same line?
A: To print multiple values on the same line, you can use multiple fprintf or sprintf statements, or you can concatenate the values using the strcat or sprintf functions.
Q: Can I print complex numbers?
A: Yes, MATLAB supports complex numbers. You can print complex numbers by using the %a or %g format specifier in the fprintf or sprintf functions.
Q: How can I print values with a specific width or alignment?
A: MATLAB provides a variety of format specifiers that allow you to specify the width and alignment of the output. For example, %5d will print an integer with a width of 5 characters, and %-10s will print a string aligned to the left with a width of 10 characters.
Q: Is it possible to redirect the printed output to a file?
A: Yes, MATLAB allows you to redirect the output to a file using the diary function. Simply use the diary(‘filename.txt’) command before printing the values, and all subsequent output will be saved to the specified file.
Q: How can I control the precision of floating-point numbers?
A: You can specify the precision of floating-point numbers by using the %f specifier followed by a dot and the number of decimal places you want to display. For example, %0.3f will display a floating-point number with three decimal places.
In conclusion, printing values to the console in MATLAB is a crucial aspect of programming. Whether you are displaying results, debugging, or communicating with the user, understanding the various methods available will help you effectively present information. By utilizing the disp, fprintf, and sprintf functions, along with command-line variable output, you can print values in different formats and personalize the output in MATLAB according to your needs.
How To Print Matlab Code With Output?
MATLAB is a powerful programming language commonly used in the fields of engineering and science. When working on a project or learning MATLAB, it is often necessary to print the code along with its corresponding output. This allows for better documentation, sharing of results, and a comprehensive overview of the work done. In this article, we will explore different methods to print MATLAB code with output.
Method 1: Command Window Copy-Paste
The most straightforward way to print MATLAB code with output is by manually copying and pasting the code and its output from the Command Window. This method is suitable for small code snippets and works well when you need to print specific sections of code. The steps are as follows:
1. Open MATLAB and run the code in the Command Window.
2. Select the code and output you want to print.
3. Copy the selected section using Ctrl+C (or right-click and select Copy).
4. Open a text editor or a word processing software like Microsoft Word.
5. Paste the copied text using Ctrl+V (or right-click and select Paste).
6. Adjust the formatting as needed (e.g., change font, size, line spacing).
This method provides a quick and easy way to print MATLAB code with output. However, it may become tedious when dealing with large code files or multiple code sections.
Method 2: Save Command Window Session
If you have a lengthy MATLAB code with multiple output sections, saving the Command Window session is a more efficient option. This method allows you to save all the executed code and their outputs in a single file, making it simple to print or share comprehensive details. Follow these steps:
1. Open MATLAB and run the desired code in the Command Window.
2. Go to the “File” menu and select “Save As.”
3. Choose a file name and location for the saved session.
4. Select “Text and Formats” from the “Save as type” drop-down menu.
5. Click “Save.”
This saves the Command Window session with both the executed code and the corresponding outputs. You can now open the file in a text editor, word processor, or any software that supports plain text files to print it. This method ensures you capture all relevant information without the need for manual copy-pasting.
Method 3: Publish MATLAB Code as PDF or HTML
Another useful feature in MATLAB is the ability to publish code as PDF or HTML files. Publishing code automates the process and generates a formatted document with code and output. This can be helpful when sharing code with others or creating a printable version with a professional appearance. Here’s how you can do it:
1. Open MATLAB and navigate to the Script or Function Editor window containing the code.
2. Click on the “Publish” button located in the MATLAB toolbar.
3. Choose either “Publish to PDF” or “Publish to HTML” from the drop-down menu.
4. Specify the desired filename and location for the generated document.
5. Click “Publish.”
MATLAB will process the code and generate a PDF or HTML document, depending on your selection. This document will contain the code, its output, and additional information like comments and section headings. You can print the generated document as you would with any other PDF or HTML file.
FAQs
Q1: Can I customize the appearance of the printed MATLAB code?
A1: Yes, you can customize the appearance of the printed MATLAB code by using different formatting options available in your text editor or word processing software. These options include changing font style, size, color, and line spacing.
Q2: Can I print only specific parts of code and output?
A2: Yes, you can selectively print specific parts of MATLAB code and output using the copy-paste method mentioned in Method 1. Simply select the desired sections before copying and pasting them into a text editor or word processor.
Q3: How can I ensure the printed code remains in sync with the desired output?
A3: To ensure the printed code remains in sync with the desired output, make sure to execute the code and generate the output immediately before copying and printing. This will ensure the printed document accurately represents the latest results.
Q4: Can I print MATLAB code with line numbers?
A4: Yes, you can add line numbers to the MATLAB code before printing by modifying the display preferences. In MATLAB, go to “Preferences” > “Editor/Debugger” > “Language”. Check the “Show line numbers” option to display line numbers in the Editor window, which will then be printed along with the code.
Printing MATLAB code with output is an essential practice for documentation, sharing, and an overall understanding of your work. Whether you choose to manually copy-paste, save the Command Window session, or publish as a PDF/HTML document, MATLAB provides multiple options to accommodate your printing needs. Take advantage of these methods and choose the one that best suits the size, complexity, and purpose of your MATLAB code.
Keywords searched by users: matlab print to console matlab print string, matlab print figure, matlab print variable, matlab print array, simulink print to console, matlab disp, matlab print command, matlab print screen
Categories: Top 25 Matlab Print To Console
See more here: nhanvietluanvan.com
Matlab Print String
Printing strings in English with Matlab is straightforward and can be done using different approaches depending on the desired output format. In this article, we will explore the various methods to print strings in English using Matlab and discuss some frequently asked questions related to this topic.
1. The disp() function:
The disp() function is a versatile tool in Matlab that allows printing strings, variables, and expressions to the command window. To print a string in English with disp(), simply enclose the desired text within single quotes and pass it as an argument to the function. Here’s an example:
“`matlab
disp(‘Hello, world!’);
“`
This will display “Hello, world!” in the command window.
2. fprintf():
Matlab’s fprintf() function enables formatted printing, offering more flexibility when printing strings. It allows developers to include variables or expressions within the printed text. To print strings in English using fprintf(), the format specifier ‘%s’ is used to represent the string. Consider the following example:
“`matlab
name = ‘John Doe’;
age = 25;
fprintf(‘My name is %s, and I am %d years old.\n’, name, age);
“`
This will produce the output: “My name is John Doe, and I am 25 years old.”
3. sprintf():
Similar to fprintf(), sprintf() enables formatted printing but returns the formatted string instead of displaying it directly. The generated string can be assigned to a variable for further processing or manipulation. This can be useful, for instance, when generating dynamic strings for reports or messages. Here’s an example:
“`matlab
name = ‘Jane Smith’;
age = 30;
result = sprintf(‘My name is %s, and I am %d years old.’, name, age);
disp(result);
“`
This will display the same output as in the previous example.
Now, let’s address some frequently asked questions related to printing strings in English with Matlab:
FAQs:
Q1. Can I print multiple strings in one statement?
A1. Yes, you can print multiple strings by separating them using commas in the disp() function or by using multiple format specifiers in fprintf() or sprintf().
Q2. Can I use special characters or escape sequences in printed strings?
A2. Yes, you can use escape sequences such as ‘\n’ for a new line or ‘\t’ for a tab within printed strings.
Q3. How can I concatenate strings with variables?
A3. To concatenate strings with variables, you can use the ‘+’ operator or sprintf() function. Here’s an example using the ‘+’ operator:
“`matlab
name = ‘Alice’;
age = 35;
result = [‘My name is ‘ name ‘, and I am ‘ num2str(age) ‘ years old.’];
disp(result);
“`
This will display the same output as before.
Q4. Can I print strings in other languages besides English?
A4. Yes, Matlab supports printing strings in various languages, not just English. You can print strings in other languages as long as the characters are supported by the character encoding used by Matlab.
Q5. How can I print strings to a file instead of the command window?
A5. To print strings to a file, you can use the fopen(), fprintf(), and fclose() functions. Here’s an example:
“`matlab
fileID = fopen(‘output.txt’, ‘w’);
fprintf(fileID, ‘This is a string that will be written to the file.’);
fclose(fileID);
“`
This will create a file named ‘output.txt’ containing the specified string.
In conclusion, Matlab provides several methods for printing strings in English. From the simple disp() function to the more versatile fprintf() and sprintf(), developers can choose the most suitable approach based on their specific requirements. By understanding the fundamentals of printing strings in English, Matlab users can effectively communicate information, generate reports, and interact with users within their programs.
Matlab Print Figure
When it comes to analyzing and visualizing data, Matlab stands out as a powerful tool in the realm of scientific computing. One crucial aspect of Matlab is its ability to create high-quality figures that effectively communicate complex information. In this article, we will delve into the concept of printing figures in Matlab, discussing the various options, settings, and techniques to optimize your figure output. So, whether you are an experienced Matlab user or a novice seeking guidance, this article has got you covered!
Understanding the Basics
Before we dive into the nitty-gritty details, let’s first understand why printing figures in Matlab is essential. Printing figures allows users to save their plotted data in a format suitable for further analysis, publications, presentations, or even for hard copies. Matlab provides numerous options for printing figures, enabling users to customize the output based on their specific requirements.
Printing Formats
Matlab supports various printing formats to cater to different needs and preferences. The most common formats include JPEG, PNG, PDF, EPS, SVG, and TIFF, among others. Each format has its pros and cons, so it’s important to pick the right one based on what you aim to achieve with your figure. For instance, JPEG is suitable for web-based applications due to its small file size, while PDF is great for document preservation and high-quality printing.
Printing Options and Settings
Matlab offers a range of options and settings to enhance the appearance of the printed figures. By default, Matlab utilizes the screen display size to determine the figure dimensions for printing. However, users can specify the size manually by adjusting the figure’s width and height using the ‘PaperPosition’ property. This allows for precise control over the figure’s appearance.
Moreover, Matlab provides the option to adjust the resolution of the figures to ensure clarity. The ‘dpi’ (dots per inch) parameter allows users to define the pixel density, with higher values yielding more detailed output. It’s worth noting that increasing the resolution may result in larger file sizes, so it’s prudent to strike a balance between quality and file size.
Another valuable tool in Matlab’s printing arsenal is the ‘print’ command. This function enables users to fine-tune additional settings, such as the rendering renderer, font handling, color management, and scaling options. By utilizing the ‘print’ command, users can customize their figures according to specific publication requirements or personal preferences.
FAQs:
Q: How can I save a Matlab figure as an image file?
A: Saving a Matlab figure as an image file is quite easy. Simply use the ‘print’ command followed by the desired format and file name. For instance, to save a figure as a PNG file named ‘my_figure.png’, you can use the following command:
`print(‘my_figure.png’, ‘-dpng’)`
Q: Can I print multiple figures in one file?
A: Certainly! Matlab allows users to save multiple figures in a single file by employing the ‘print’ command with the ‘-append’ flag. This way, figures can be appended one after another, streamlining the output into a unified document.
Q: How can I change the font size of my printed figure?
A: To adjust the font size of your printed figure, you can use the ‘set’ function and modify the ‘FontSize’ property. For instance, the following line of code sets the font size to 12 points:
`set(gca, ‘FontSize’, 12)`
Q: Are there any additional tips for optimizing the printing quality of Matlab figures?
A: Absolutely! One useful tip is to ensure that the figure’s size matches the desired output dimensions. This can be achieved by adjusting the ‘PaperPosition’ property accordingly. Additionally, using vector-based formats like PDF and EPS helps retain the high-quality output and allows for smooth scaling if necessary.
Q: Can I print a Matlab figure without displaying it on the screen?
A: Yes! Matlab allows users to print figures directly without displaying them on the screen. By setting the renderer to ‘zbuffer’, the figure can be created and printed without being shown. Here’s an example:
`set(gcf, ‘Renderer’, ‘zbuffer’);
print -dpng my_figure.png`
In conclusion, printing figures in Matlab is an essential task for sharing and preserving data visualization. By exploring the various options and settings available, users can optimize their figure output for diverse purposes. With this knowledge at your disposal, you are now equipped to create stunning and publication-ready Matlab figures. So, go ahead and leverage the power of Matlab’s printing capabilities to effectively communicate your data!
Images related to the topic matlab print to console
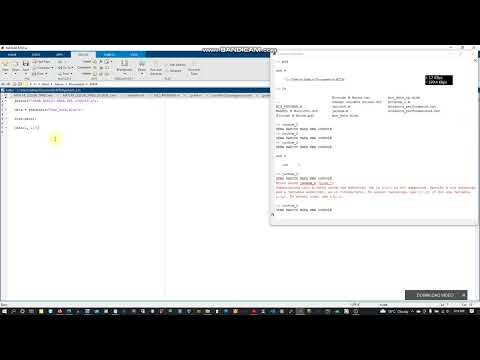
Found 30 images related to matlab print to console theme
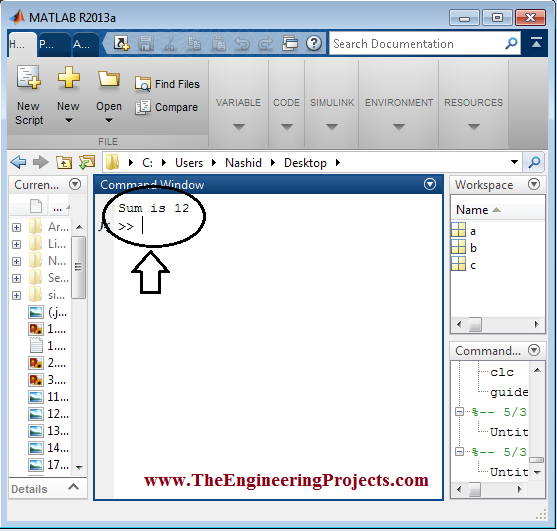
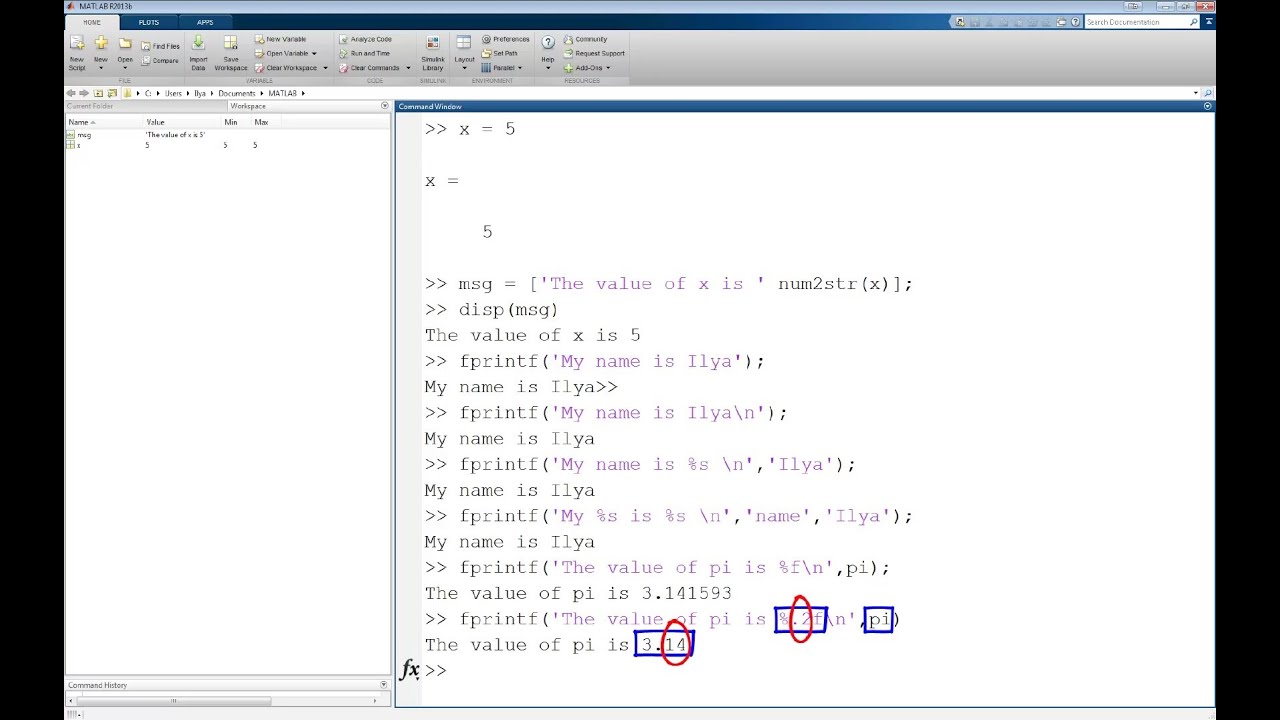
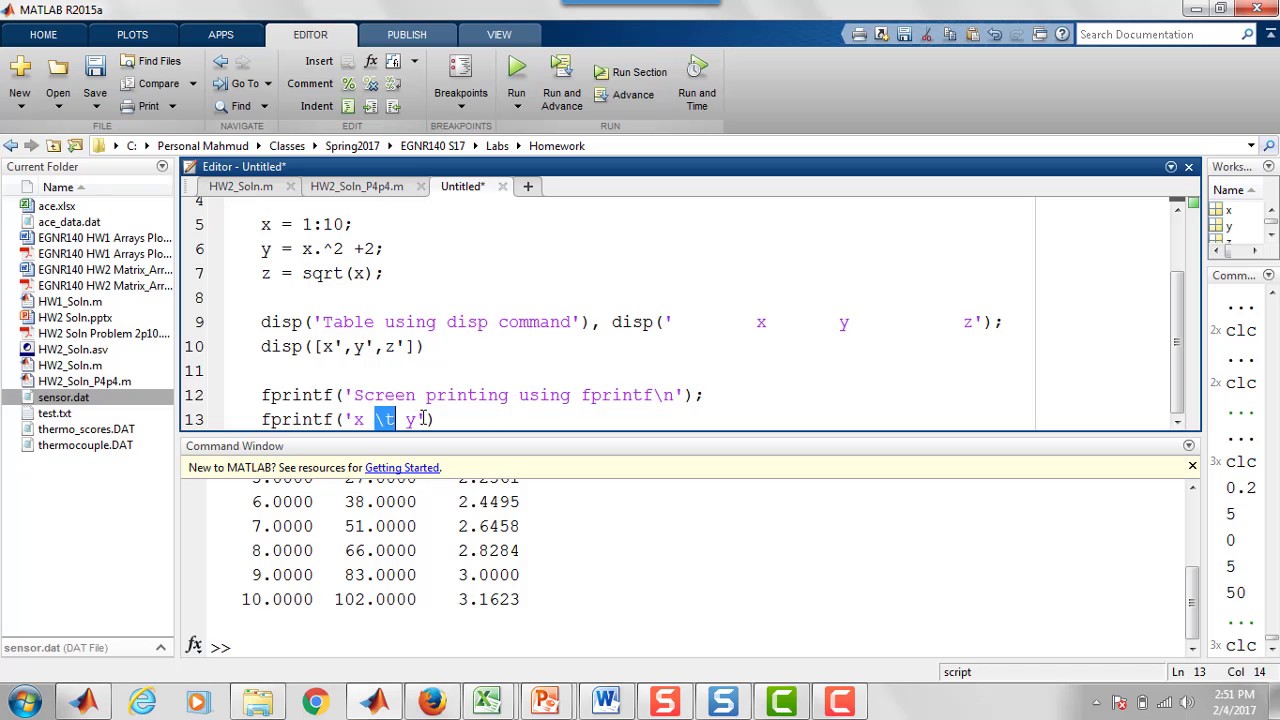

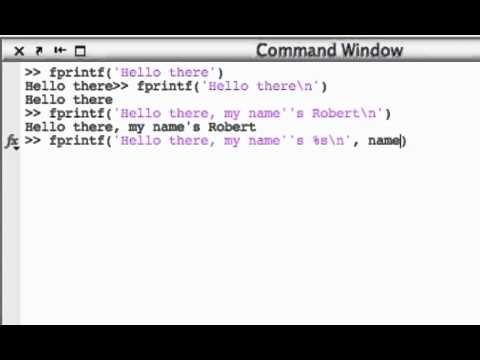
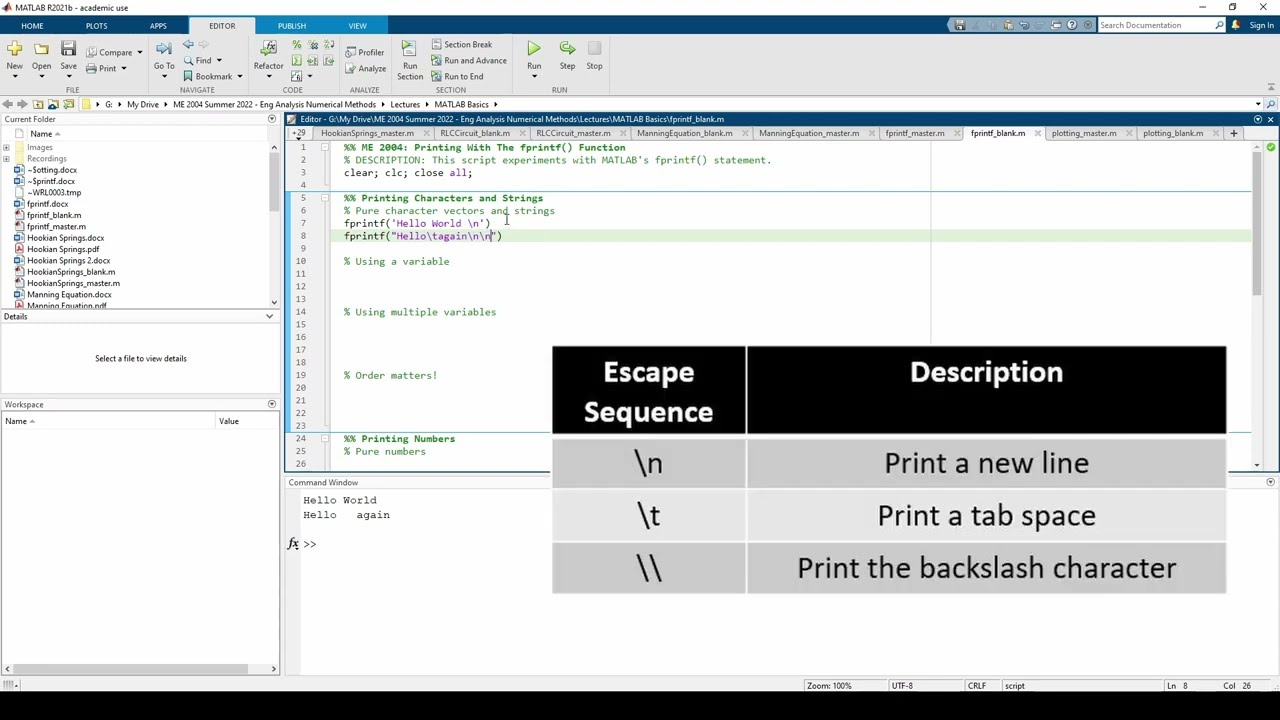

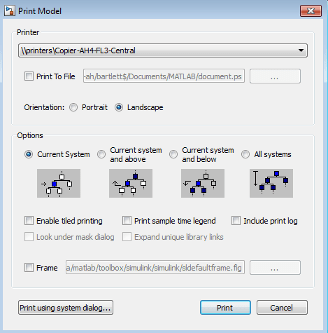
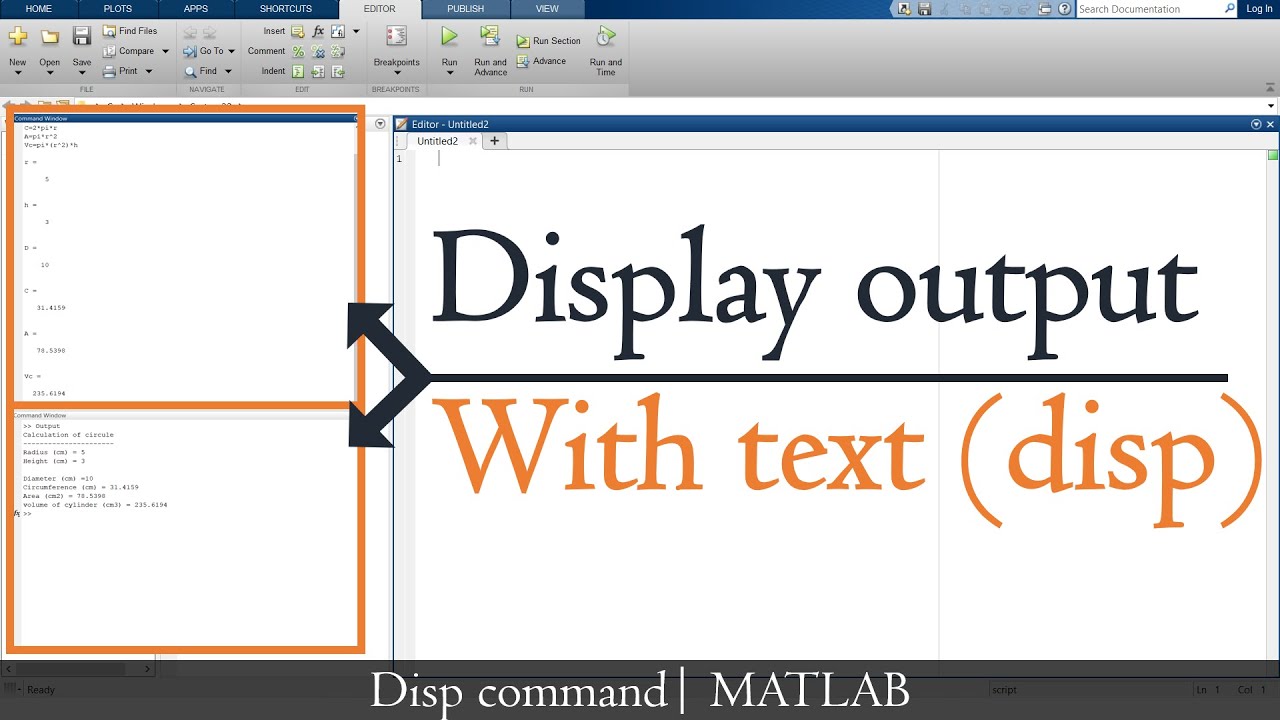
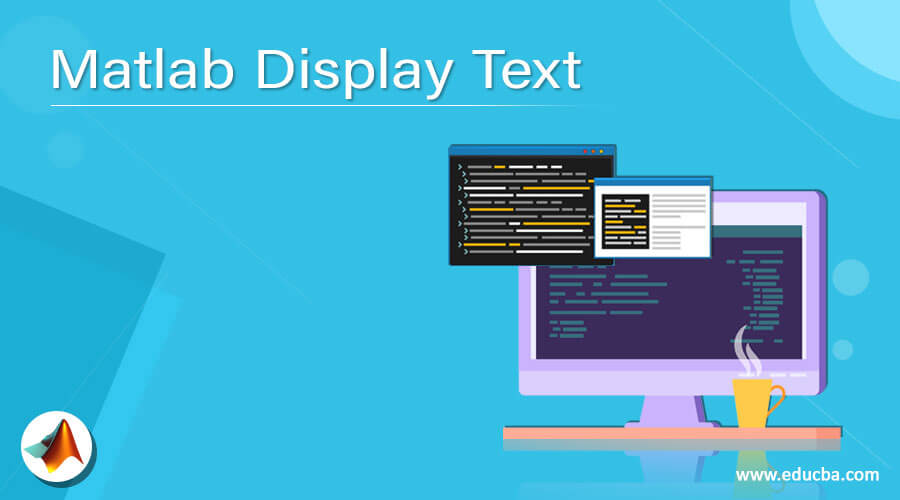

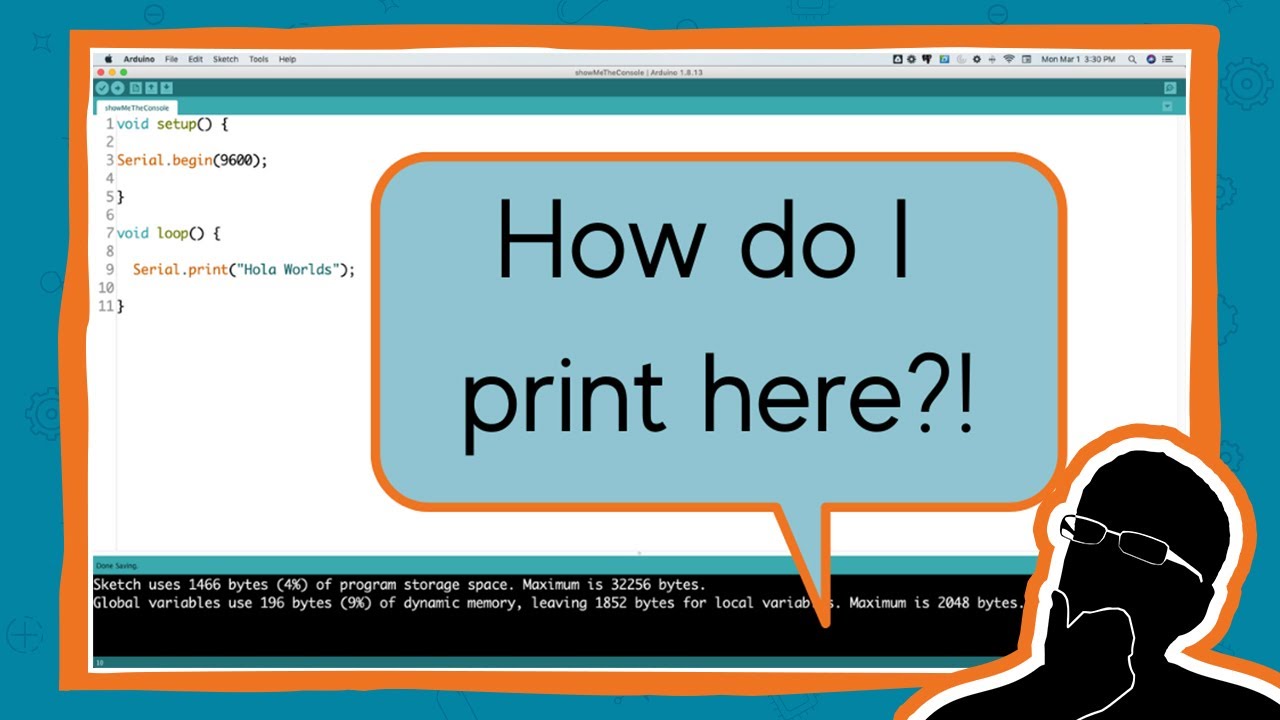

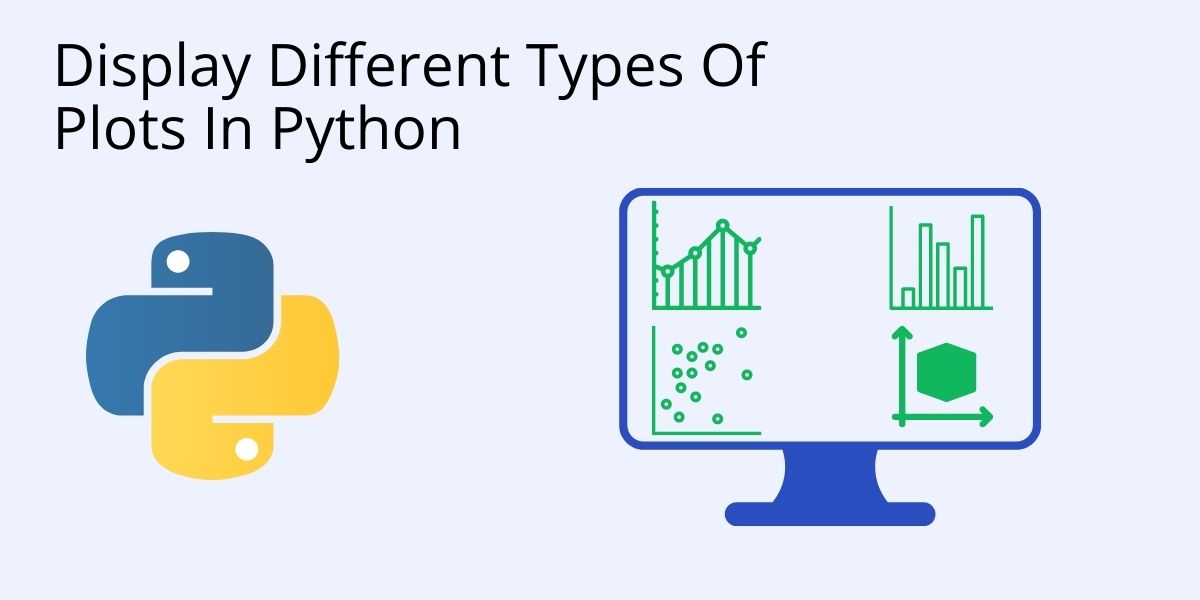

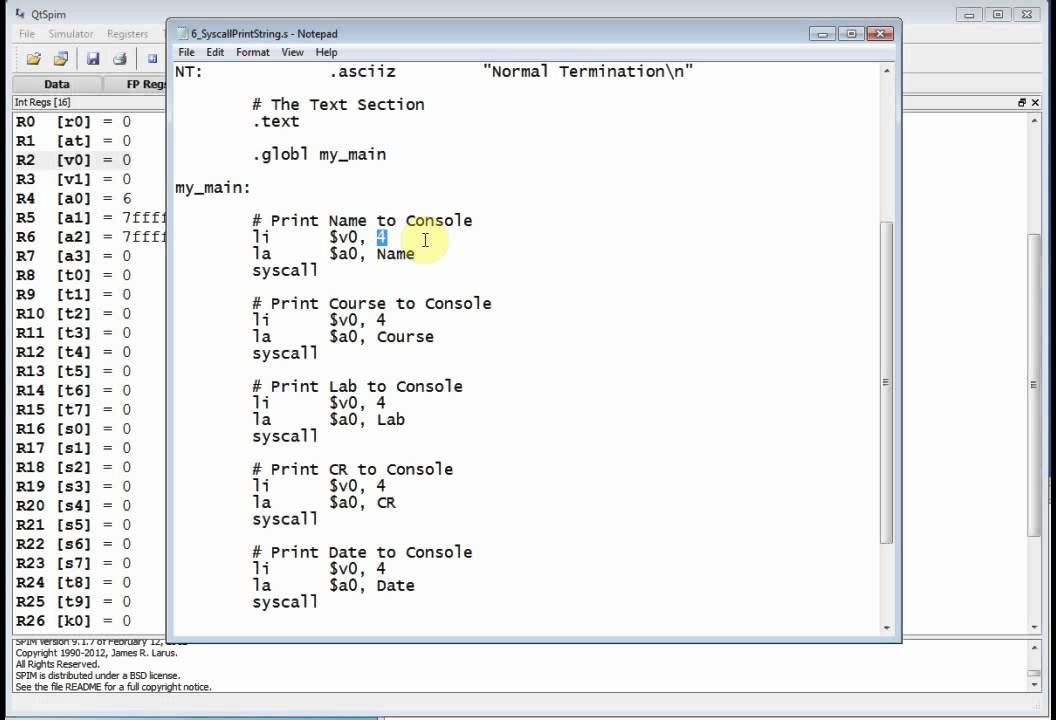


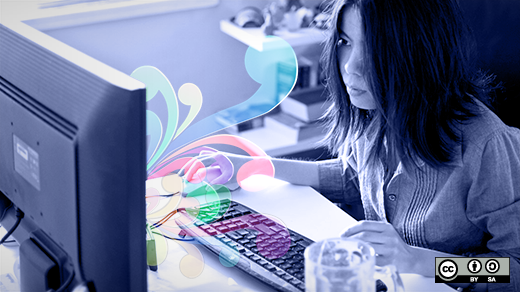

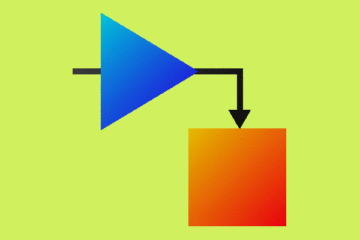

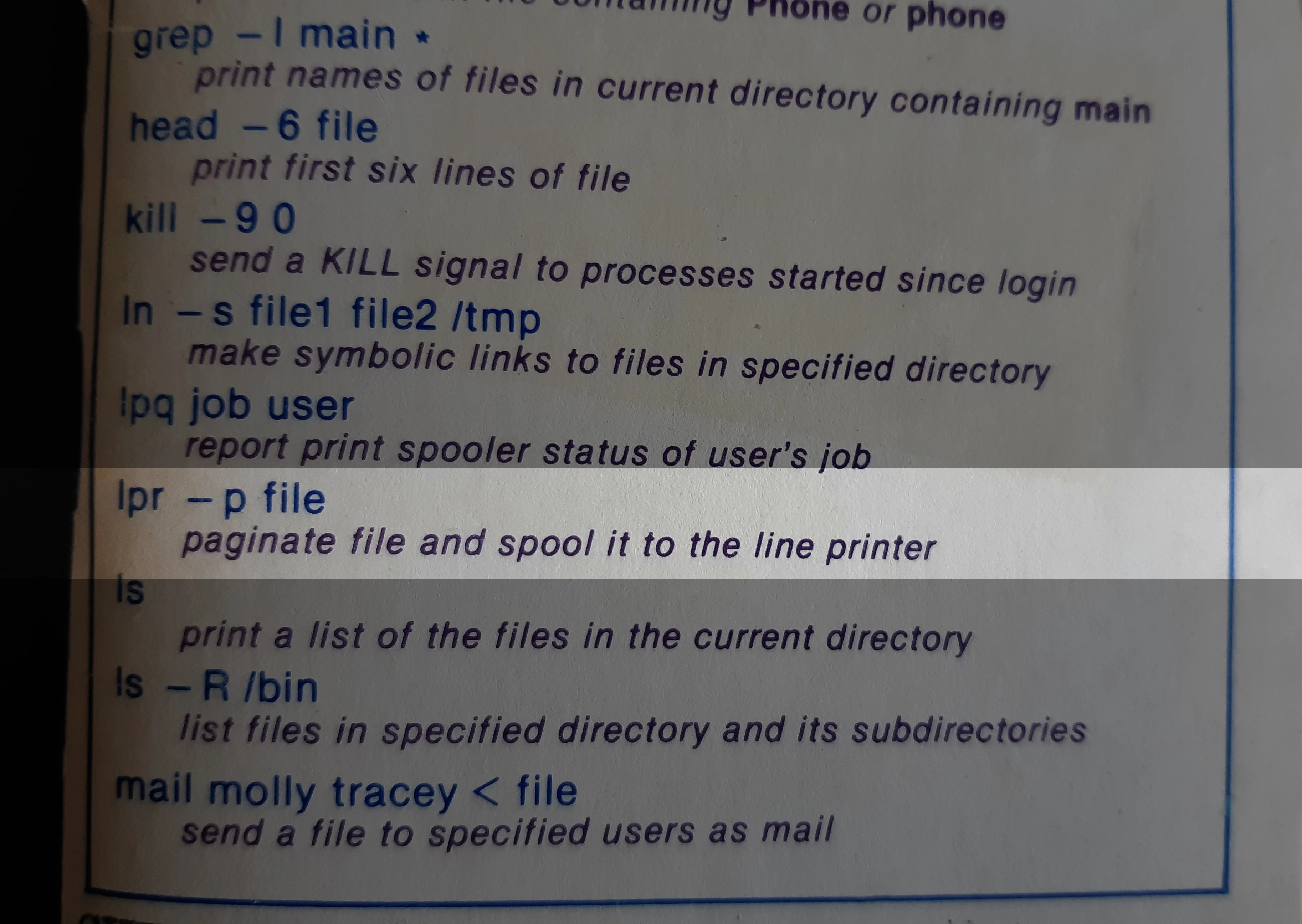
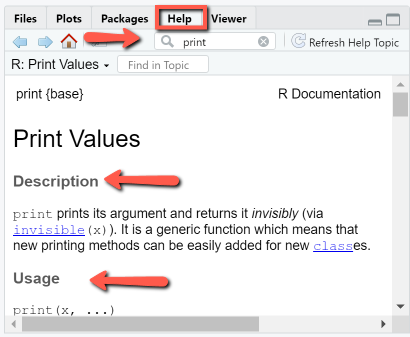
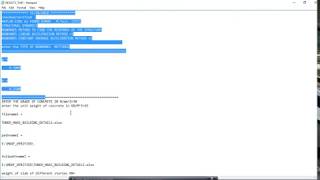

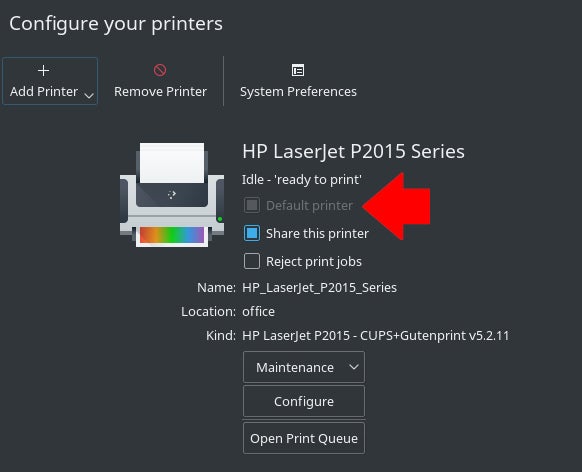

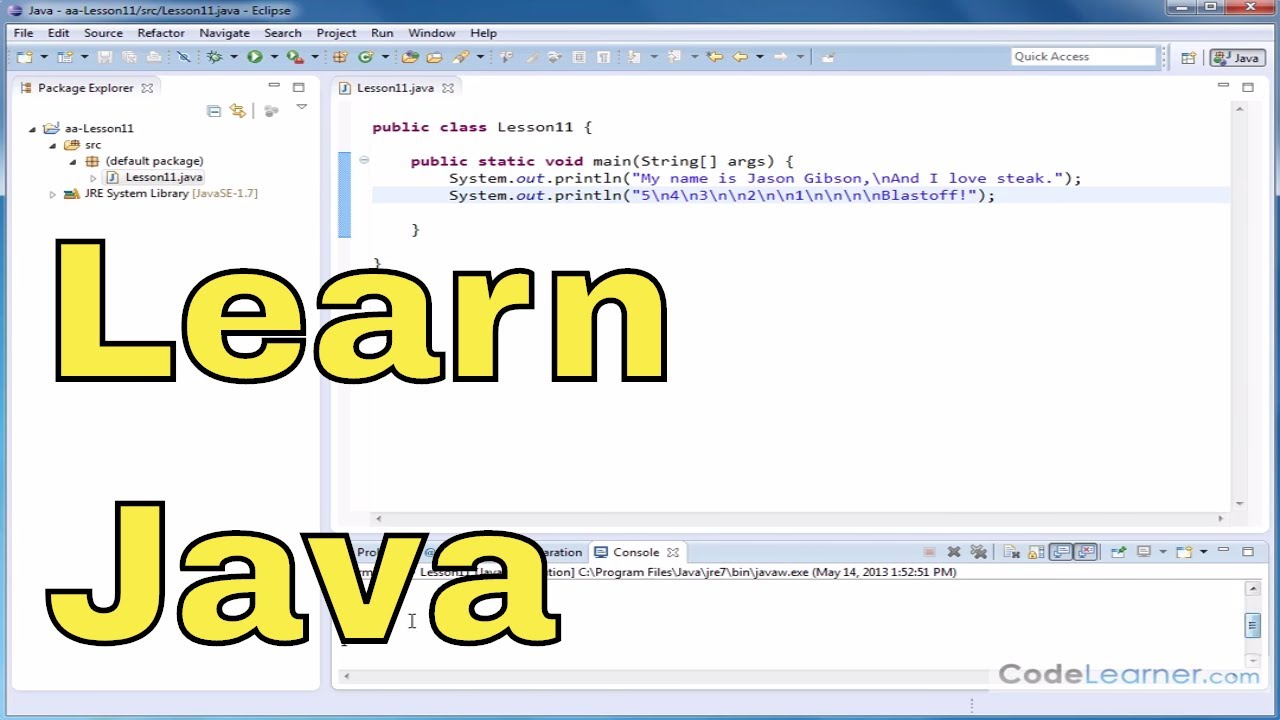
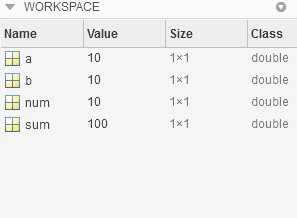
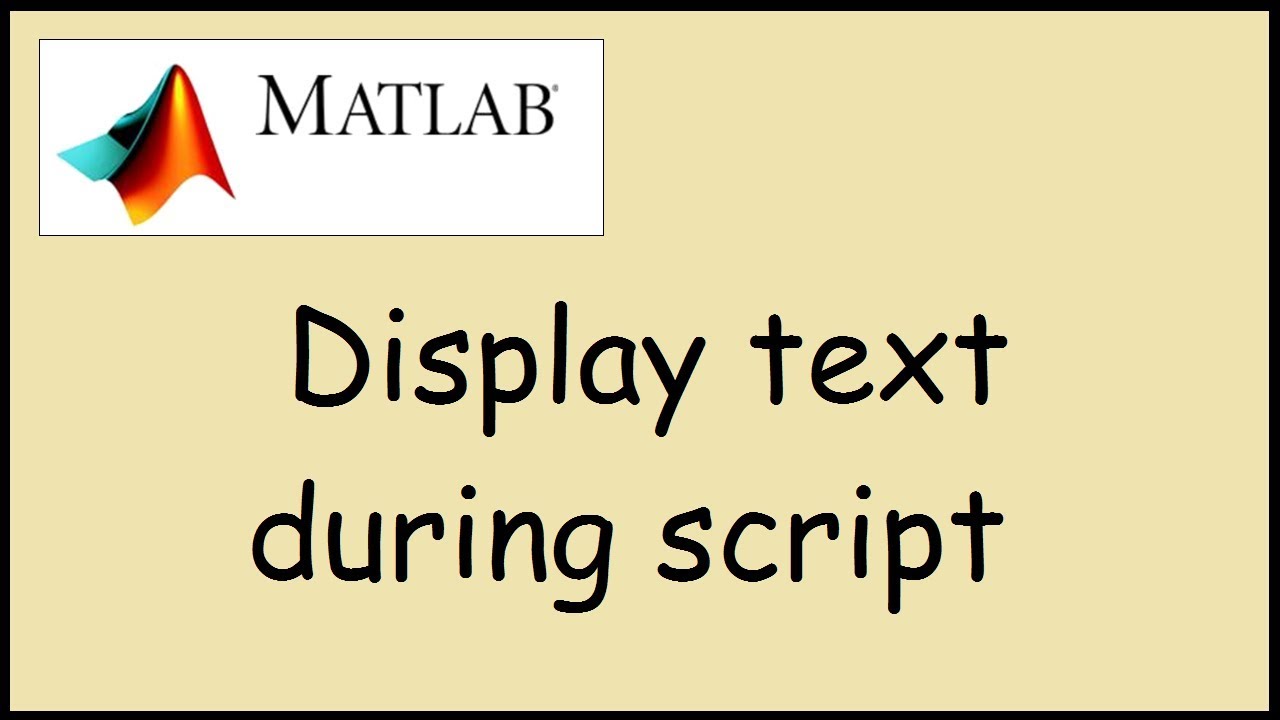
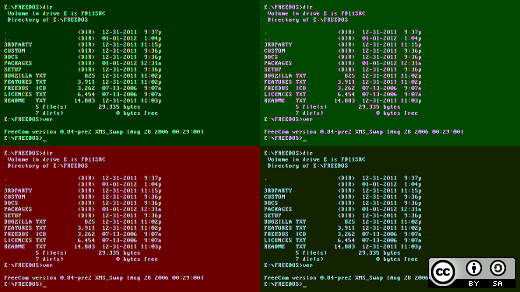
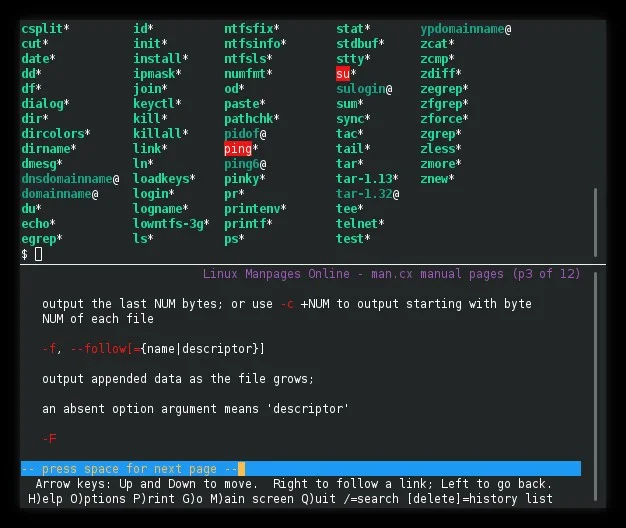
Article link: matlab print to console.
Learn more about the topic matlab print to console.
- How do I print (output) in Matlab? – dspGuru
- Print a statement to the Command Window – MATLAB Answers
- Print a statement to the Command Window – MATLAB Answers
- Print Figure from File Menu – MATLAB & Simulink – MathWorks
- Display value of variable – MATLAB disp – MathWorks
- How to print a string in Matlab? – Stack Overflow
- Print Output in Command Window in Matlab | Delft Stack
- MATLAB console output – Stack Overflow
- Matlab Code – fprintf
- Terminal Output – GNU Octave
- MATLAB Command Window Output – LaTeX Stack Exchange
- Examples of Matlab Display Text – eduCBA
- Disable printing logs in matlab console – CVX Forum
See more: https://nhanvietluanvan.com/luat-hoc