Loop Through Array Bash
Bash is a powerful scripting language that provides several ways to loop through array elements. In this article, we will explore the different methods to iterate over an array in bash, including using for loops, while loops, and various ways of accessing and manipulating array elements. We will also discuss some common issues and frequently asked questions related to array handling in bash.
Using a for loop to iterate over array elements:
The simplest and most common method to loop through an array in bash is by using a for loop. Here’s an example:
“`bash
array=(“element1” “element2” “element3”)
for element in “${array[@]}”
do
echo “$element”
done
“`
In the above code snippet, the array variable is initialized with some dummy elements. The for loop then iterates over the array elements, and each element is printed using the echo command. By using `${array[@]}`, we ensure that each array element is treated as a separate argument.
Using a while loop to traverse array elements:
Another way to loop through array elements is by using a while loop. Here’s an example:
“`bash
array=(“element1” “element2” “element3”)
index=0
while [ $index -lt ${#array[@]} ]
do
echo “${array[$index]}”
index=$((index + 1))
done
“`
In this approach, we use a while loop that continues until the index variable exceeds the length of the array. We can access individual array elements using the syntax `${array[index]}`.
Using a for loop with indexed array elements:
Bash allows us to loop through indexed array elements using a for loop. Here’s an example:
“`bash
array=(“element1” “element2” “element3”)
for index in “${!array[@]}”
do
echo “${array[$index]}”
done
“`
In this case, the for loop iterates over the indices of the array rather than its elements. By using `${!array[@]}`, we can access all the indices of the array.
Using a for loop with associative array elements:
Bash also supports associative arrays, which allow us to map keys to values. Here’s an example of using a for loop to iterate over associative array elements:
“`bash
declare -A array
array[“key1″]=”value1”
array[“key2″]=”value2”
array[“key3″]=”value3”
for key in “${!array[@]}”
do
echo “Key: $key, Value: ${array[$key]}”
done
“`
In this case, the for loop iterates over the keys of the associative array using `${!array[@]}`, and we can access the corresponding values using `${array[$key]}`.
Using a for loop with a range of array indexes:
Sometimes, we may want to loop through a specific range of indexes in an array. Here’s an example:
“`bash
array=(“element1” “element2” “element3”)
start_index=1
end_index=2
for ((index=start_index; index <= end_index; index++)) do echo "${array[$index]}" done ``` In this approach, we use a traditional for loop construct, where we set the starting and ending index values and iterate within that range. Using a for loop with a combination of array elements and indexes: Bash allows us to combine array elements and indexes into a single loop. Here's an example: ```bash array=("element1" "element2" "element3") for index in "${!array[@]}" do echo "Index: $index, Element: ${array[$index]}" done ``` In this case, the for loop iterates over the indices of the array, and we can access both the index and corresponding element simultaneously. FAQs: Q: How can I loop through an array using Jq? A: Jq is a powerful command-line JSON processor that can be used in conjunction with bash to loop through array elements within JSON data. You can use Jq's `length` function to determine the length of the array, and then iterate over it using a for loop with an index variable. Q: How can I break a loop in Bash? A: To break out of a loop in bash, you can use the `break` keyword. When executed, `break` will immediately terminate the innermost loop and continue executing the next line of code after the loop. Q: I encountered a syntax error: ('' unexpected bash array. What could be causing it? A: This error usually occurs when you have empty parentheses or missing quotes while defining an array in bash. Make sure to use proper syntax when declaring and initializing arrays. For example, use `array=("element1" "element2")` instead of `array=()`. Q: How can I pass an array to a function in bash? A: To pass an array to a function in bash, you can use the special notation `@` or `*` when invoking the function. Inside the function, you can access the entire array by referring to `${array[@]}` or `${array[*]}`. Q: How can I access a specific element in an array in bash? A: You can access a specific element in an array by using its index within square brackets. For example, to access the third element of an array named `array`, you can use `${array[2]}` since array indexes start from zero. Q: How can I remove an element from an array in bash? A: There is no built-in way to remove a specific element from an array in bash. However, you can unset the element using `unset array[index]` and then reassign the array to remove the empty element. Alternatively, you can create a new array excluding the desired element(s). Q: How can I save the output of a command to an array in bash? A: To save the output of a command to an array, you can use command substitution syntax `$(command)`. For example, you can assign the output of a command `command_output` to an array `array` using `array=($(command_output))`. In conclusion, bash provides several methods to loop through array elements, including using for loops, while loops, and various ways of accessing and manipulating array elements. By understanding these techniques, you can effectively work with arrays in bash scripts and handle various array-related tasks efficiently.
Batch Script Loop Through Array | Batch Script Array
Keywords searched by users: loop through array bash Jq loop through array, Break loop in Bash, Syntax error: (” unexpected bash array), Shell script array, Pass array to function bash, Access element in array bash, Remove element in array bash, Bash save output to array
Categories: Top 24 Loop Through Array Bash
See more here: nhanvietluanvan.com
Jq Loop Through Array
### Understanding Jq’s Looping Mechanism
Jq offers a variety of looping methods to help you efficiently iterate through arrays. One of the most commonly used mechanisms is the `foreach` construct. This construct allows you to apply a filter to each element of the array individually.
To start, let’s consider the following array of JavaScript objects as an example:
“`json
[
{
“name”: “John”,
“age”: 25
},
{
“name”: “Jane”,
“age”: 30
},
{
“name”: “Robert”,
“age”: 40
}
]
“`
Now, let’s say we want to extract and print the names of all the individuals in the array using the `foreach` loop construct in Jq. Here’s how we can achieve that:
“`bash
jq ‘.[] | .name’ example.json
“`
The `.` before `[]` refers to the root object, and the `[]` indicates that we are selecting all elements of the array. Then, we use the `foreach` construct (`|`) to apply the `.name` filter to each element individually.
The output would be:
“`
“John”
“Jane”
“Robert”
“`
### Looping with Conditional Statements
In addition to basic looping, Jq also allows you to incorporate conditional statements within the loop construct. This feature becomes particularly useful when you want to filter or manipulate array elements based on certain conditions.
Let’s consider the previous example again. Suppose we only want to print the names of individuals above the age of 30. We can achieve this using the `foreach` construct along with an `if-then` statement:
“`bash
jq ‘.[] | if .age > 30 then .name else empty end’ example.json
“`
The `if-then` statement checks if the `age` field is greater than 30. If true, it applies the `.name` filter to retrieve and display the corresponding name. Otherwise, it returns `empty`.
The output would be:
“`
“Robert”
“`
### Frequently Asked Questions (FAQs)
**Q: Can I modify the array elements while looping through them?**
Yes, you can modify array elements within the loop using Jq’s powerful update-assignment operator (`|=`). For example, if you want to increment the age of all individuals in our previous example by 5, you can do so as follows:
“`bash
jq ‘.[] |= .age += 5’ example.json
“`
This would update the array to:
“`json
[
{
“name”: “John”,
“age”: 30
},
{
“name”: “Jane”,
“age”: 35
},
{
“name”: “Robert”,
“age”: 45
}
]
“`
**Q: How can I combine looping with other Jq functionalities?**
Jq offers an extensive range of functions, filters, and operators that can be combined and nested with looping constructs to achieve complex data processing tasks. You can use functions like `map`, `select`, `reduce`, and many others to manipulate and transform array elements during looping.
**Q: Can I loop through nested arrays or objects?**
Yes, Jq provides seamless support for looping through nested arrays or objects. You can use multiple levels of looping constructs and access specific elements using dot notation, as demonstrated in previous examples. This allows you to extract, filter, or modify data at any desired depth in the JSON structure.
**Q: Are there any limitations to Jq’s looping capabilities?**
While Jq’s looping mechanism is powerful and versatile, it is important to keep in mind that complex iterations or transforms might require more advanced programming techniques. Jq is primarily designed for concise data manipulation, and in certain scenarios, writing custom scripts or programs may be more appropriate.
In conclusion, Jq’s loop through array feature provides an intuitive and efficient way of processing JSON data. Whether you need to extract specific elements, apply conditional logic, or even modify array values, Jq’s looping capabilities offer a flexible solution. By understanding the syntax and mastering the available constructs, you can leverage this feature to accomplish complex data processing tasks effortlessly.
Break Loop In Bash
Introduction:
In the world of programming, loops play a vital role in iteratively executing a set of instructions until a specific condition is met. Bash, the command language interpreter used in Unix-based systems, offers a variety of loop structures. These loops, such as the ‘for’ and ‘while’ loops, provide the flexibility to control the flow of execution in scripts. However, there may arise situations where it becomes necessary to alter the normal course of a loop’s execution. In such cases, the ‘break’ statement in Bash comes to the rescue. In this article, we will dive deep into the concept of breaking a loop in Bash, exploring its syntax and providing practical examples.
Break Statement Syntax:
The ‘break’ statement in Bash is used to prematurely exit from a loop, terminating its execution. Its syntax is simple yet powerful:
“`bash
break
“`
When this statement is encountered within a loop, the program immediately exits the loop block and continues with the next instruction following the loop.
Breaking ‘for’ Loops:
The ‘for’ loop is a popular looping construct in Bash, used to iterate over a sequence of elements or values. To break a ‘for’ loop, simply include the ‘break’ statement within the loop’s body. Let’s consider an example:
“`bash
for fruit in apple banana cherry
do
if [[ $fruit == “banana” ]]; then
break
fi
echo “Current fruit: $fruit”
done
“`
In the above code snippet, the loop iterates over three fruits. Once the condition `$fruit == “banana”` is met, the ‘break’ statement is executed, terminating the loop. As a result, the output will only display “Current fruit: apple”.
Breaking ‘while’ Loops:
The ‘while’ loop in Bash is used to repeatedly execute a set of instructions while a certain condition evaluates to true. To break a ‘while’ loop, include the ‘break’ statement within the loop’s body. Here’s an example:
“`bash
counter=0
while [[ $counter -lt 5 ]]
do
if [[ $counter -eq 3 ]]; then
break
fi
echo “Counter: $counter”
((counter++))
done
“`
In the above code snippet, the ‘while’ loop continues until the value of ‘counter’ reaches 3. At that point, the ‘break’ statement is encountered, causing the loop to terminate prematurely. Consequently, the output will display the numbers 0, 1, and 2.
Breaking Nested Loops:
Sometimes, it becomes necessary to break out of multiple nested loops simultaneously. To achieve this, you can use labels and the ‘break’ statement together. Consider the following example:
“`bash
outerloop:
for ((i=0; i<3; i++))
do
innerloop:
for ((j=0; j<3; j++))
do
if [[ $i -eq 1 && $j -eq 1 ]]; then
break outerloop
fi
echo "i: $i, j: $j"
done
done
```
In this example, we have an outer 'for' loop and an inner 'for' loop. The 'break' statement, labeled as 'outerloop', is encountered when the condition $i -eq 1 and $j -eq 1 is met. The execution then exits both loops simultaneously. The output will display the combinations (0, 0), (0, 1), (0, 2), (1, 0), and (2, 0).
FAQs:
Q1. Can 'break' be used outside of loops?
A1. No, the 'break' statement can only be used within loop constructs in Bash, such as 'for', 'while', 'until', or nested loops.
Q2. What happens if 'break' is not used in a loop?
A2. Without the 'break' statement, a loop will continue executing until its condition evaluates to false or the loop is manually interrupted.
Q3. Can we use labels in all types of loops?
A3. Labels, along with the 'break' statement, can be used in any loop construct in Bash, including 'for', 'while', and 'until' loops.
Q4. How does 'break' differ from 'continue'?
A4. The 'break' statement terminates the entire loop, while the 'continue' statement skips the current iteration and proceeds to the next one.
Q5. Can 'break' be used in switch-case statements?
A5. No, 'break' cannot be used to exit a switch-case construct in Bash. Instead, the ';;' syntax is used to move to the next case.
Conclusion:
In this article, we explored the power of the 'break' statement in Bash, which allows us to control the execution flow within loop constructs. We covered its syntax and provided practical examples of breaking 'for', 'while', and nested loops. Understanding how to break a loop effectively enhances the flexibility and efficiency of scripting in Bash, enabling us to handle diverse scenarios.
Syntax Error: (” Unexpected Bash Array)
Bash, short for “Bourne Again Shell,” is a widely used command-line interpreter in Unix-like operating systems. It allows users to interact with the operating system by entering and executing commands. While Bash is user-friendly and powerful, it is not immune to errors. One such error is the “Syntax Error: (” Unexpected Bash Array).” In this article, we will delve into the causes of this error, explain its implications, and provide potential solutions. Let’s dive in!
What is the “Syntax Error: (” Unexpected Bash Array)”?
The “Syntax Error: (” Unexpected Bash Array)” is a runtime error in Bash scripting. It occurs when an unexpected ” (empty string) is encountered while working with an array in the script. This error halts the execution of the script, preventing it from proceeding further.
Causes of the “Syntax Error: (” Unexpected Bash Array)”
This error typically arises due to incorrectly defining or manipulating arrays within a bash script. Some common causes include:
1. Missing or mistyped syntax: For arrays, a common mistake is leaving out essential syntax elements. For example, forgetting to include the “=” sign when assigning a value to an array variable can lead to this error.
2. Incorrect quoting: Quoting plays a crucial role in Bash scripting. Failing to use proper quotes, such as using double quotes (“”) instead of single quotes (”), or vice versa, can result in syntax errors, including the “Syntax Error: (” Unexpected Bash Array)”.
3. Nested arrays: Arrays in Bash can contain other arrays. However, nesting arrays incorrectly or not handling them properly within the script can trigger this error.
4. Incompatible Bash versions: Rarely, the error may be caused by running a script written for a newer version of Bash on an older version, leading to syntax incompatibilities.
Implications of the “Syntax Error: (” Unexpected Bash Array)”
Encountering the “Syntax Error: (” Unexpected Bash Array)” will disrupt the execution of your script. The script will exit at the point where the error is encountered, preventing any further instructions from being executed. This can hinder the functionality of the script and cause unexpected behavior or undesired results.
Resolving the “Syntax Error: (” Unexpected Bash Array)”
To resolve the “Syntax Error: (” Unexpected Bash Array),” you must carefully examine your script to identify the cause of the error. Here are some steps to help you troubleshoot and fix the issue:
1. Check syntax and quotes: Verify that you have correctly defined array variables, using proper syntax and quotation marks as required. Ensure you employ consistent quoting throughout the script.
2. Debugging with echo: Append “echo” statements to print relevant parts of your script, especially around the array operations. This helps identify any potential issues and displays the values being assigned or accessed within the arrays.
3. Handling nested arrays: If your script involves nested arrays, ensure you handle them correctly. Verify that you access the elements through the appropriate indices without any missing or extra brackets.
4. Check Bash version compatibility: If you suspect a compatibility issue, make sure your Bash script is compatible with the version you are using. Consider updating the script or altering the syntax to match your Bash version.
5. Test small sections: If your script is substantial, isolate smaller sections and test them individually. This approach helps determine the exact point where the error occurs, making it easier to identify and fix the problem.
Frequently Asked Questions (FAQs)
Q: Can this error occur if I am not using arrays in my script?
A: No, the “Syntax Error: (” Unexpected Bash Array)” specifically pertains to errors related to arrays in Bash scripting. If you are not using arrays in your script, you won’t encounter this particular error.
Q: Are there any tools or utilities specifically designed to identify syntax errors in Bash scripts?
A: Yes, there are numerous tools available for Bash script analysis and debugging, such as shellcheck and Bash Debugger. These tools can help you identify and rectify syntax errors efficiently.
Q: Is this error exclusive to Bash scripting on Unix-like systems?
A: Yes, the “Syntax Error: (” Unexpected Bash Array)” is specific to Bash scripting and occurs within Unix-like operating systems. Other shells, such as csh or zsh, have their own sets of syntax and error messages.
Q: Can this error occur due to a typographical mistake?
A: Yes, typographical errors, such as forgetting to include a character or using an incorrect one, can cause the “Syntax Error: (” Unexpected Bash Array).” It is crucial to review your script carefully for such mistakes.
Q: How can I prevent this error from occurring in the first place?
A: To avoid encountering the “Syntax Error: (” Unexpected Bash Array),” it is recommended to develop a strong understanding of Bash syntax and quotation rules. Additionally, testing your script regularly and using proper debugging techniques can help catch and address any errors early on.
In conclusion, the “Syntax Error: (” Unexpected Bash Array)” is an error specific to working with arrays in Bash scripting. It can be caused by various factors, such as incorrect quoting, missing syntax elements, or incompatible Bash versions. Identifying and resolving the error requires careful scrutiny of the script, debugging techniques, and ensuring correct syntax and quotation rules are followed. By understanding this error and implementing proper troubleshooting measures, you can effectively enhance the reliability and functionality of your Bash scripts.
Images related to the topic loop through array bash
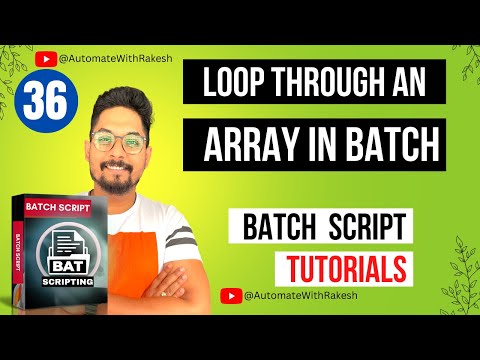
Found 28 images related to loop through array bash theme

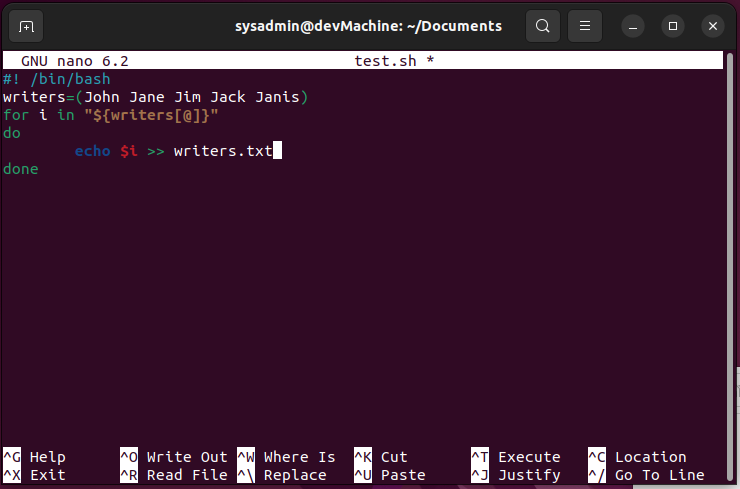
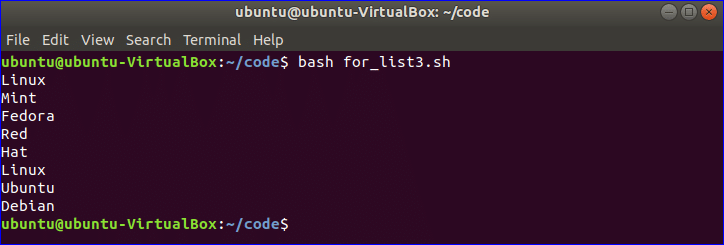

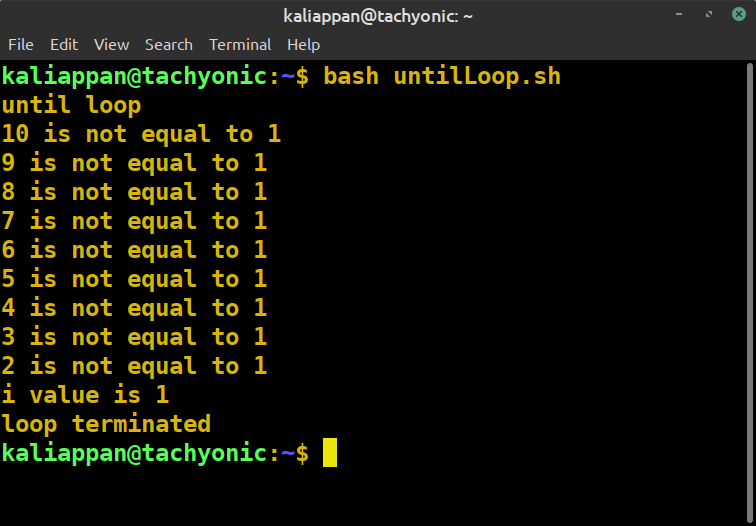
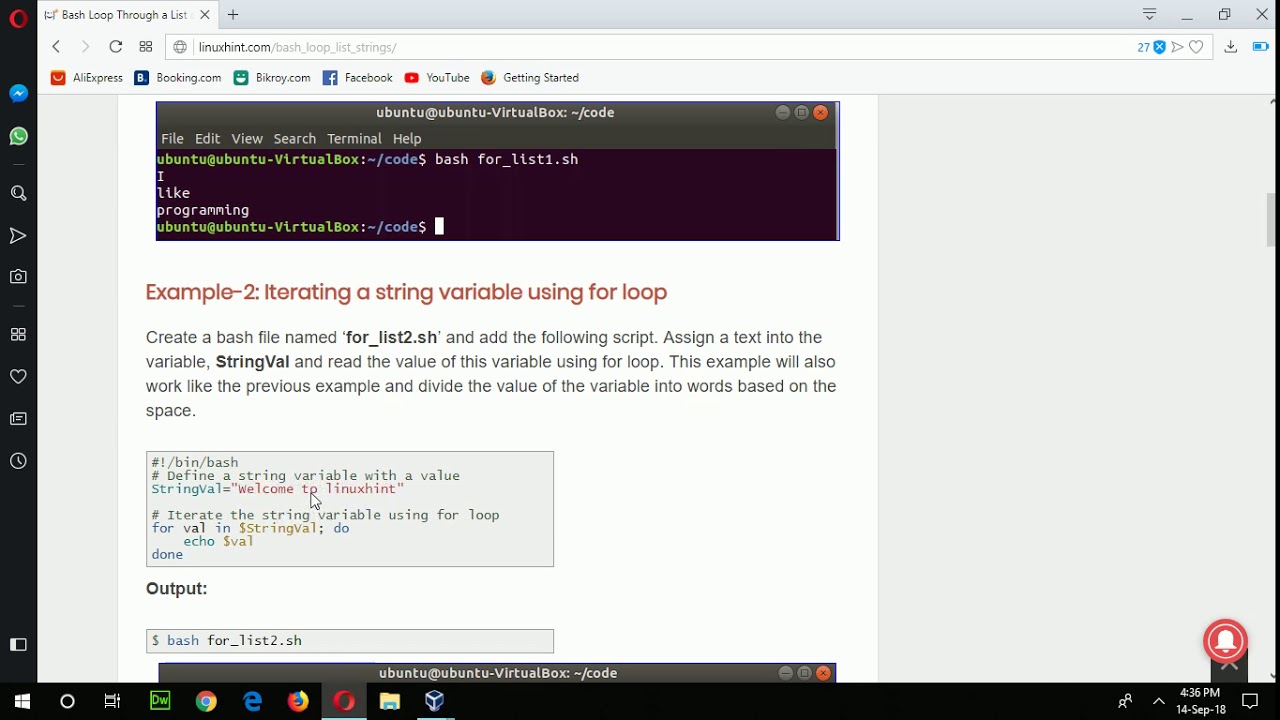

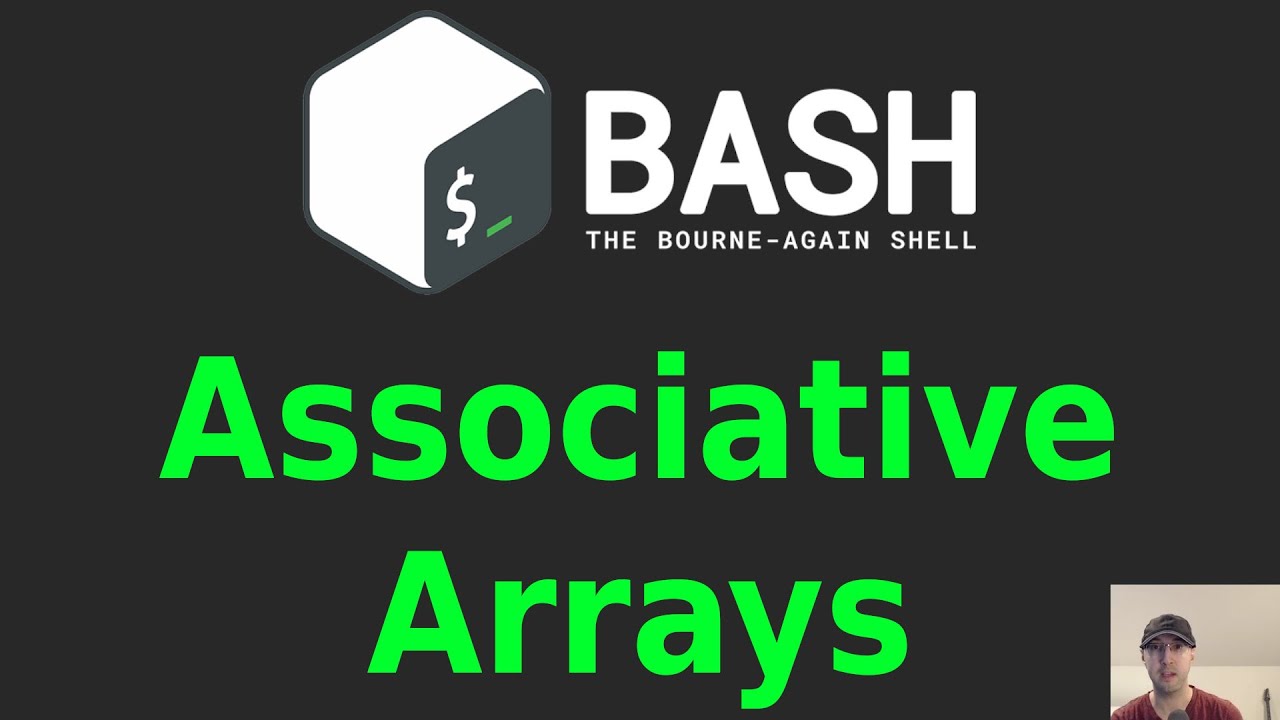
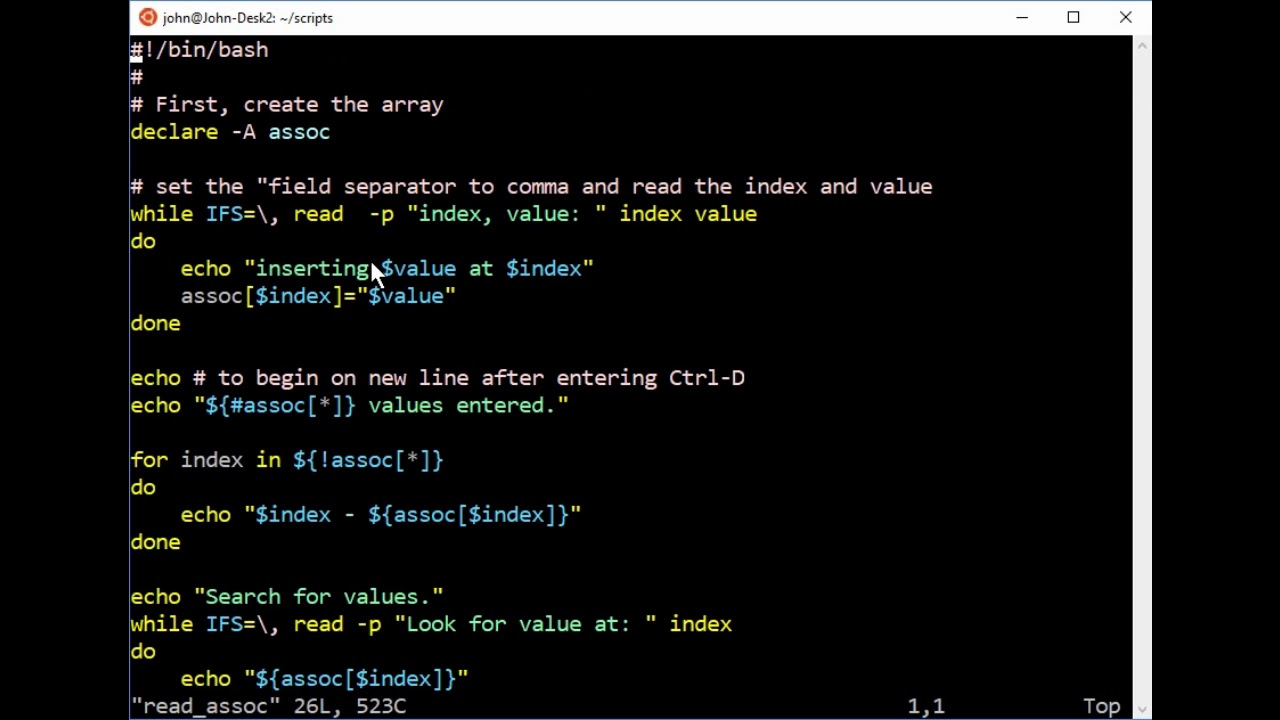

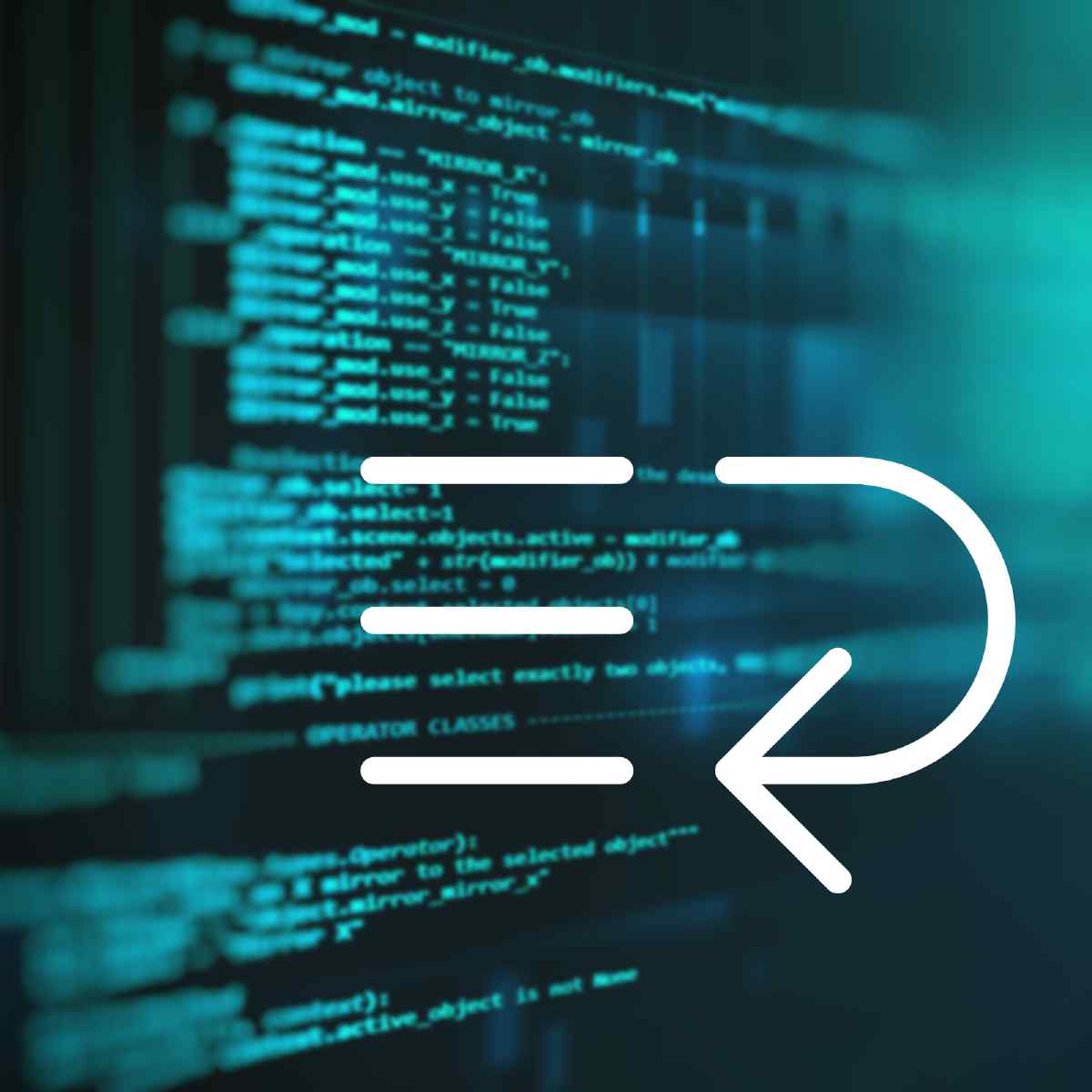

![How to loop through array in Node.js [6 Methods] | GoLinuxCloud How To Loop Through Array In Node.Js [6 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/nodejs_loop_array.jpg)

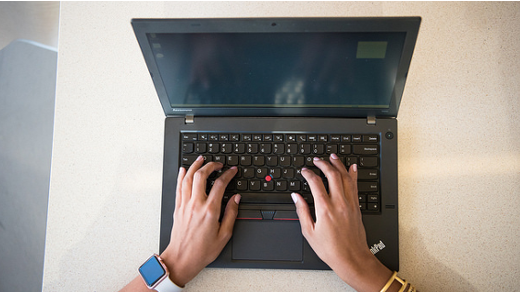




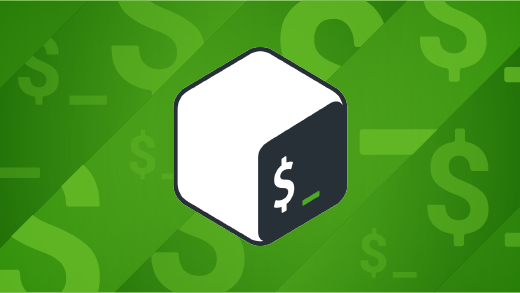
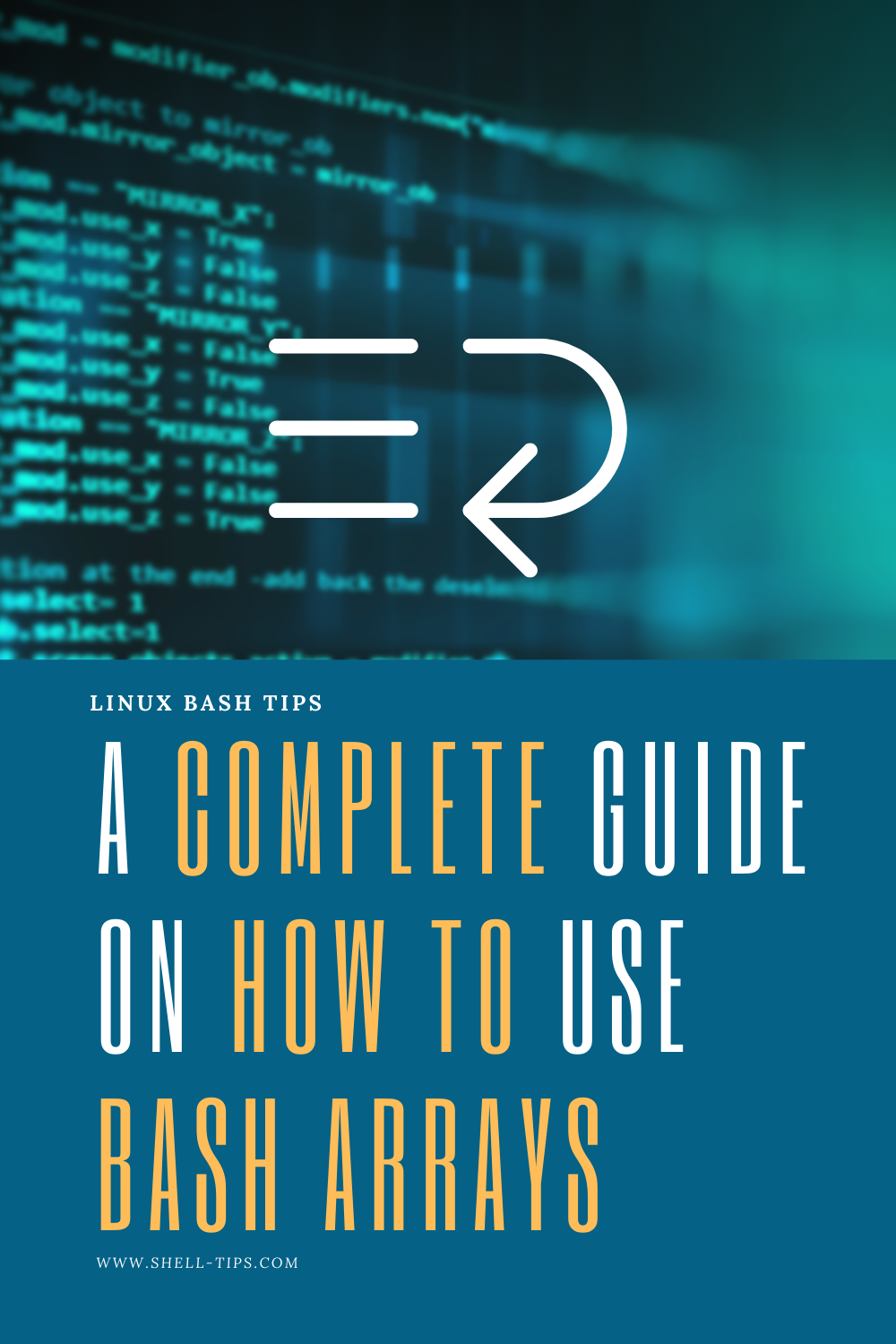


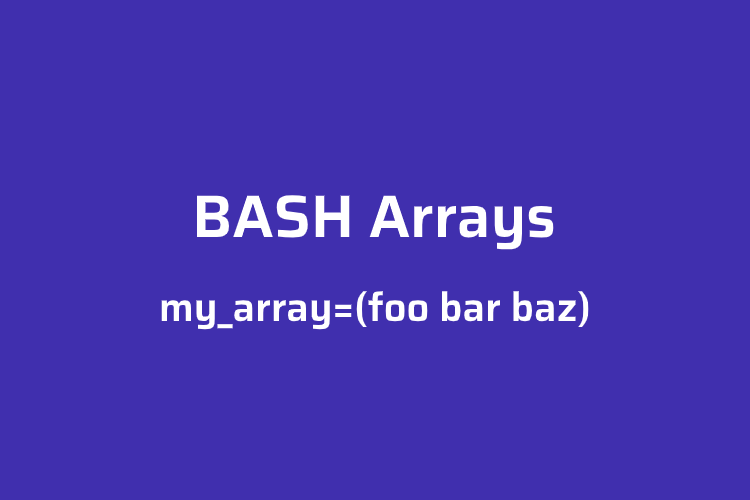
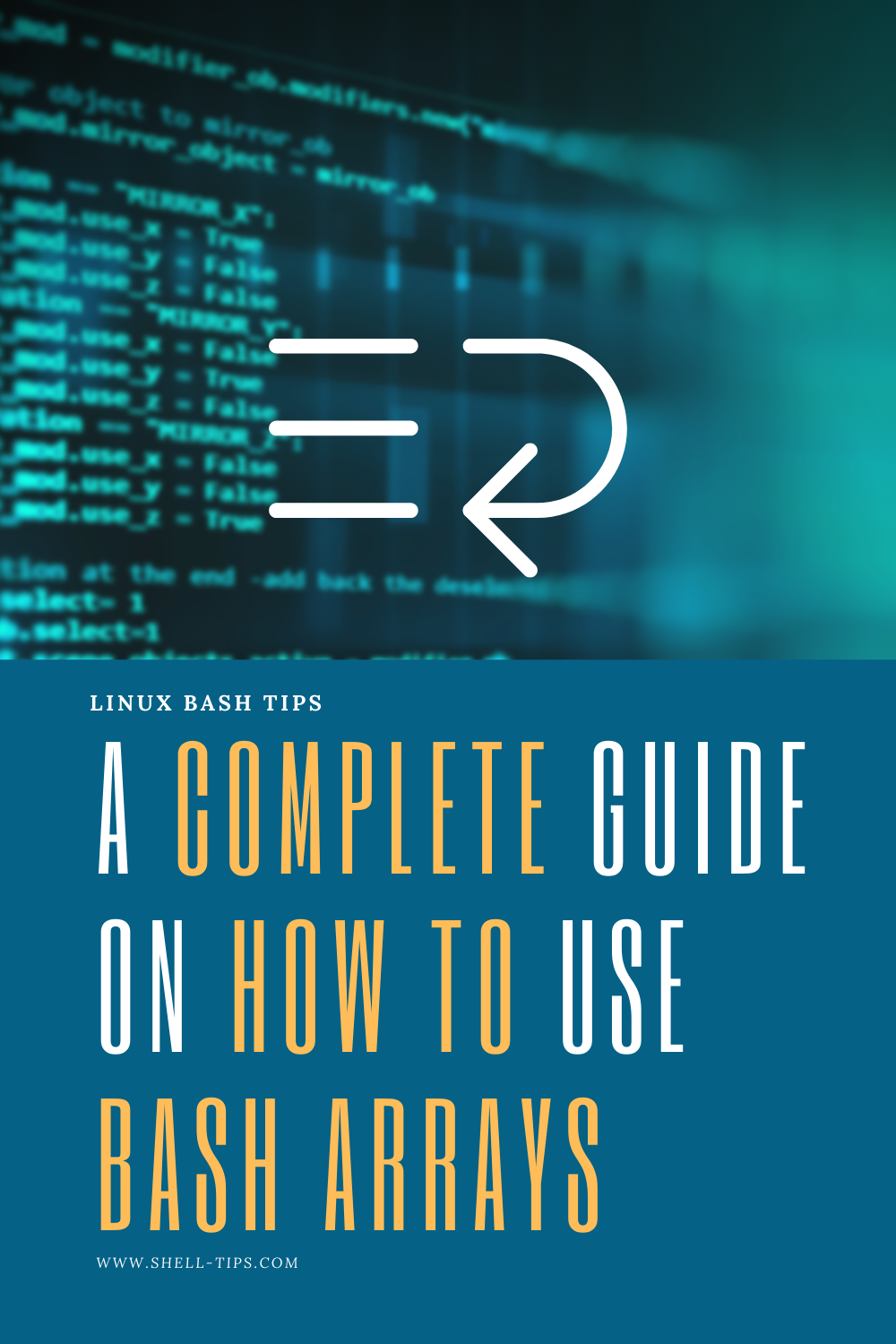


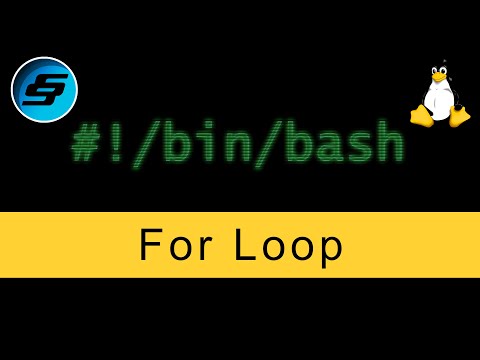
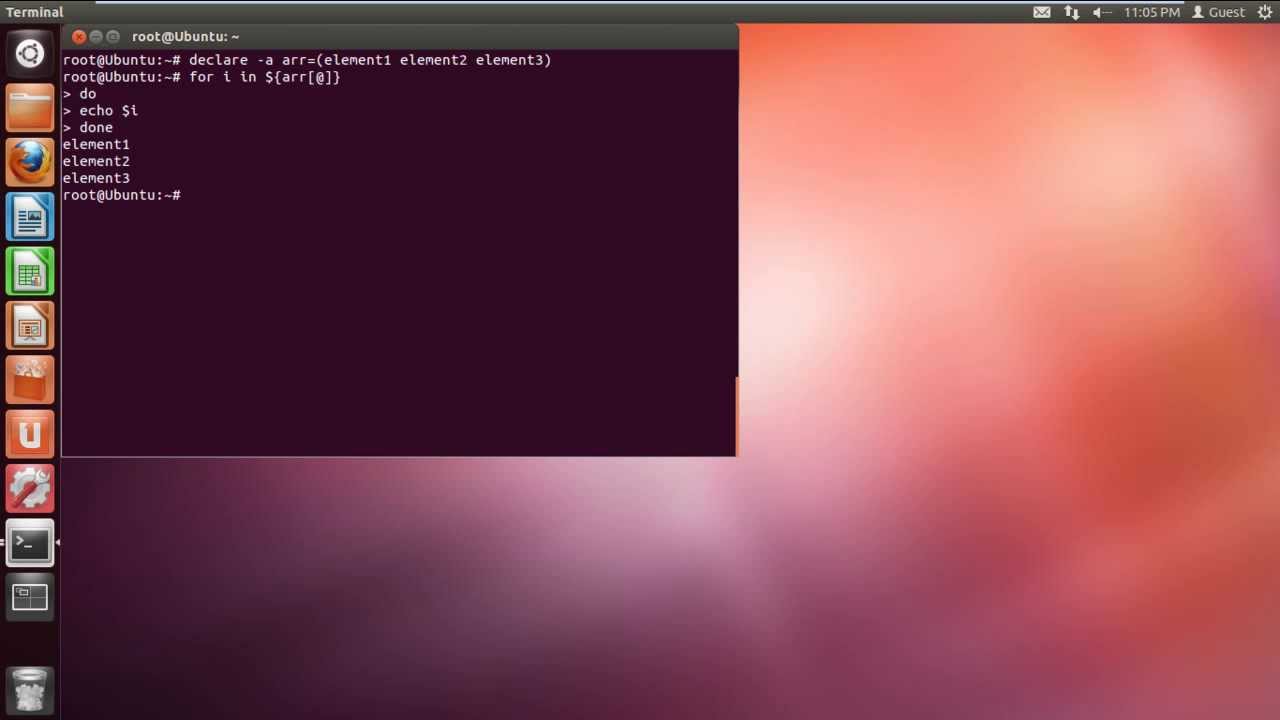
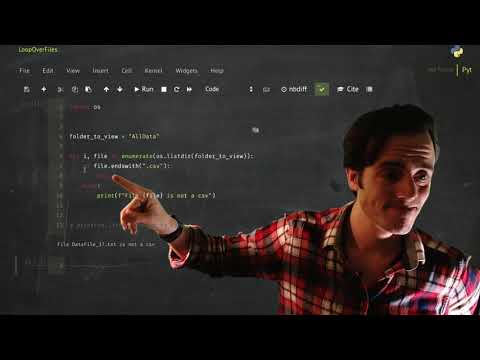

![How to loop through array in Node.js [6 Methods] | GoLinuxCloud How To Loop Through Array In Node.Js [6 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/use-while-loop.jpg)

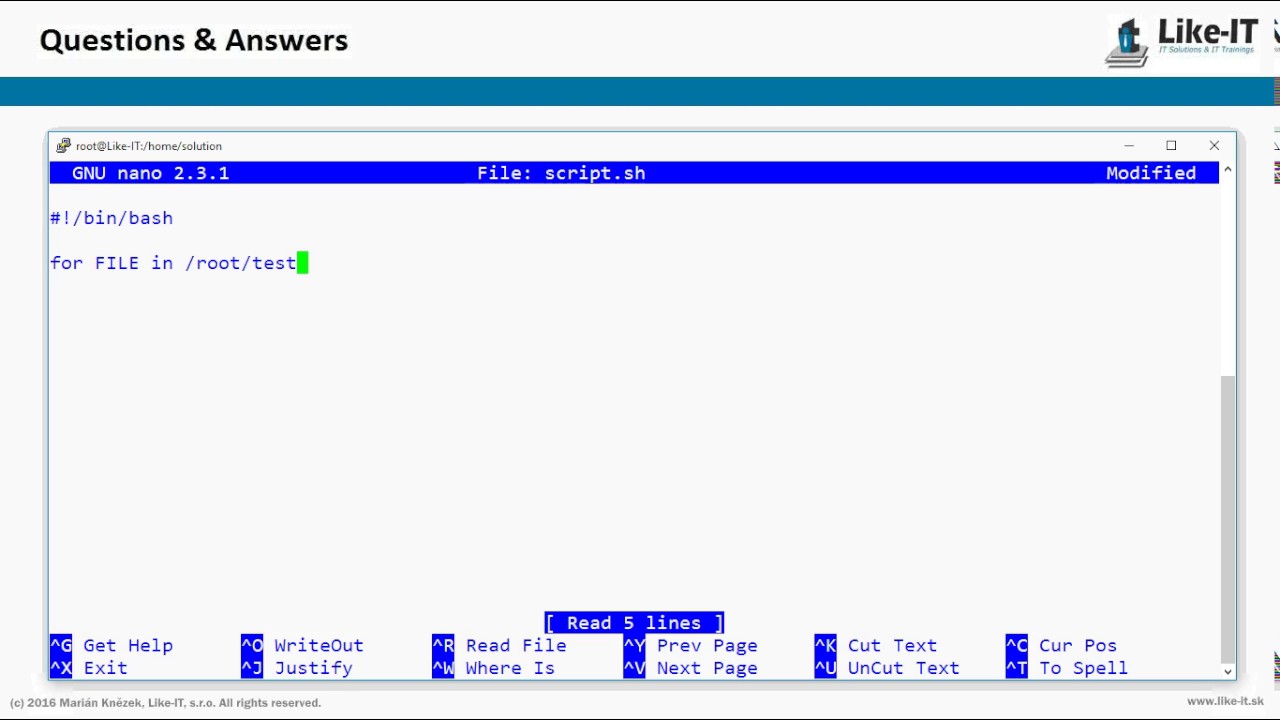
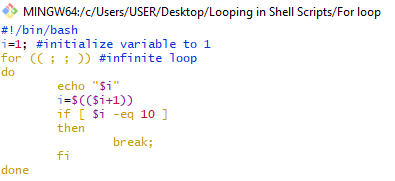
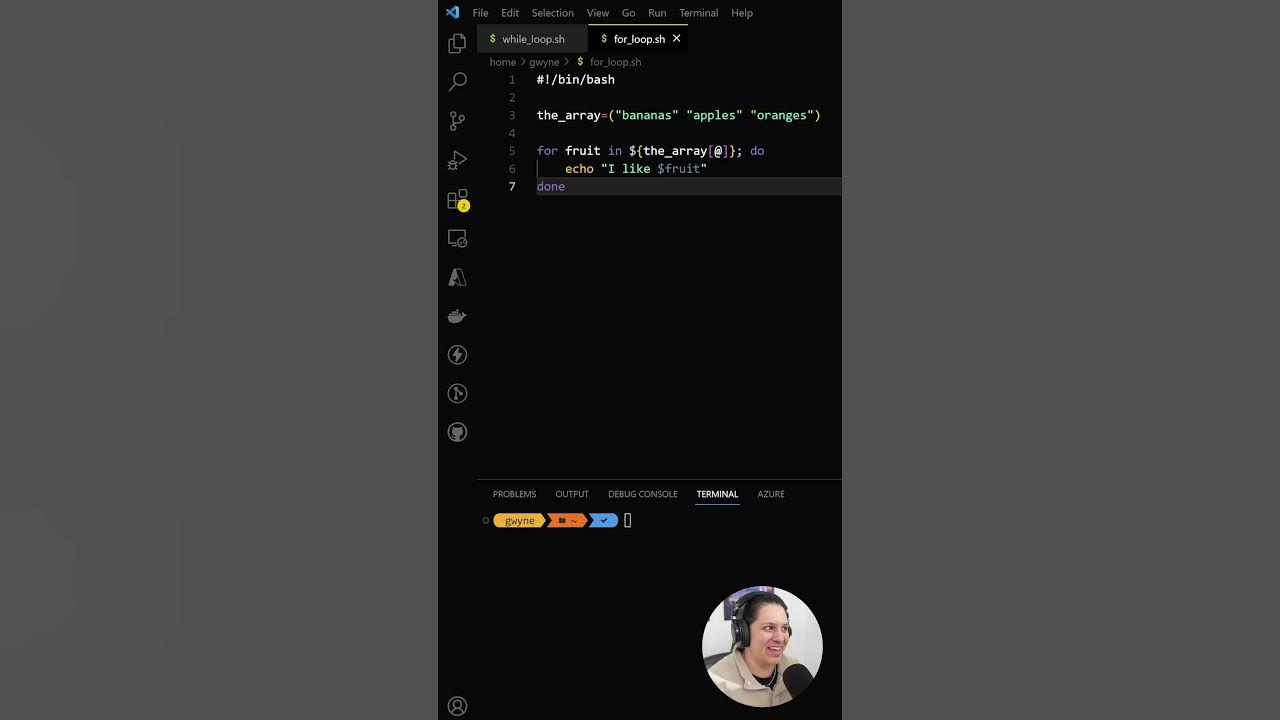
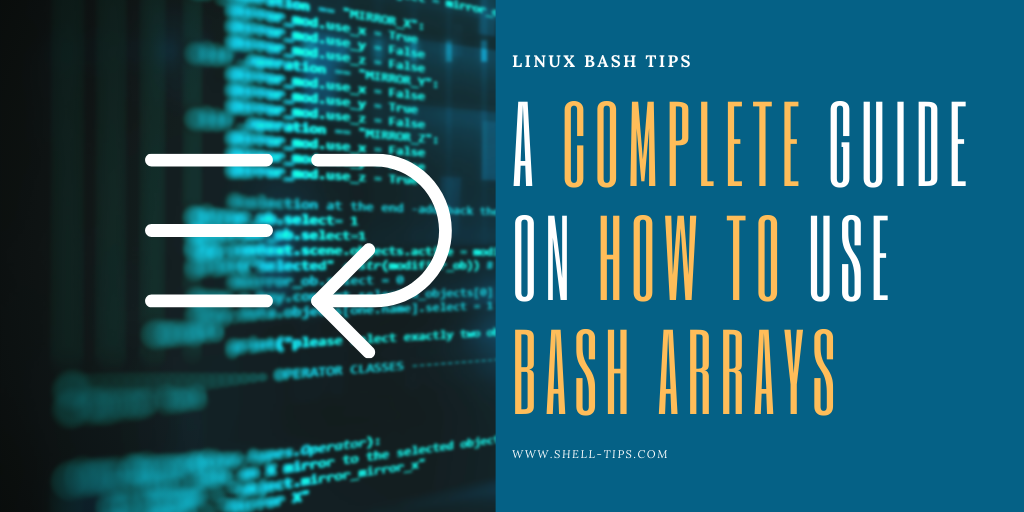


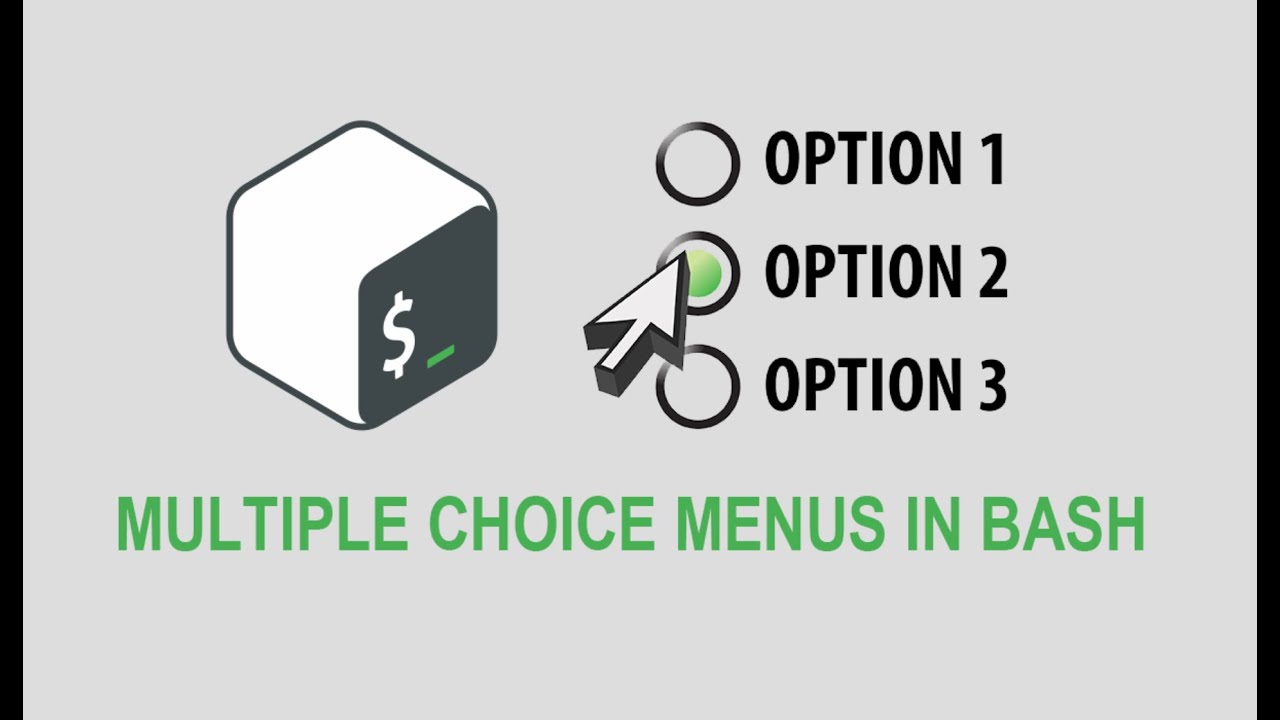

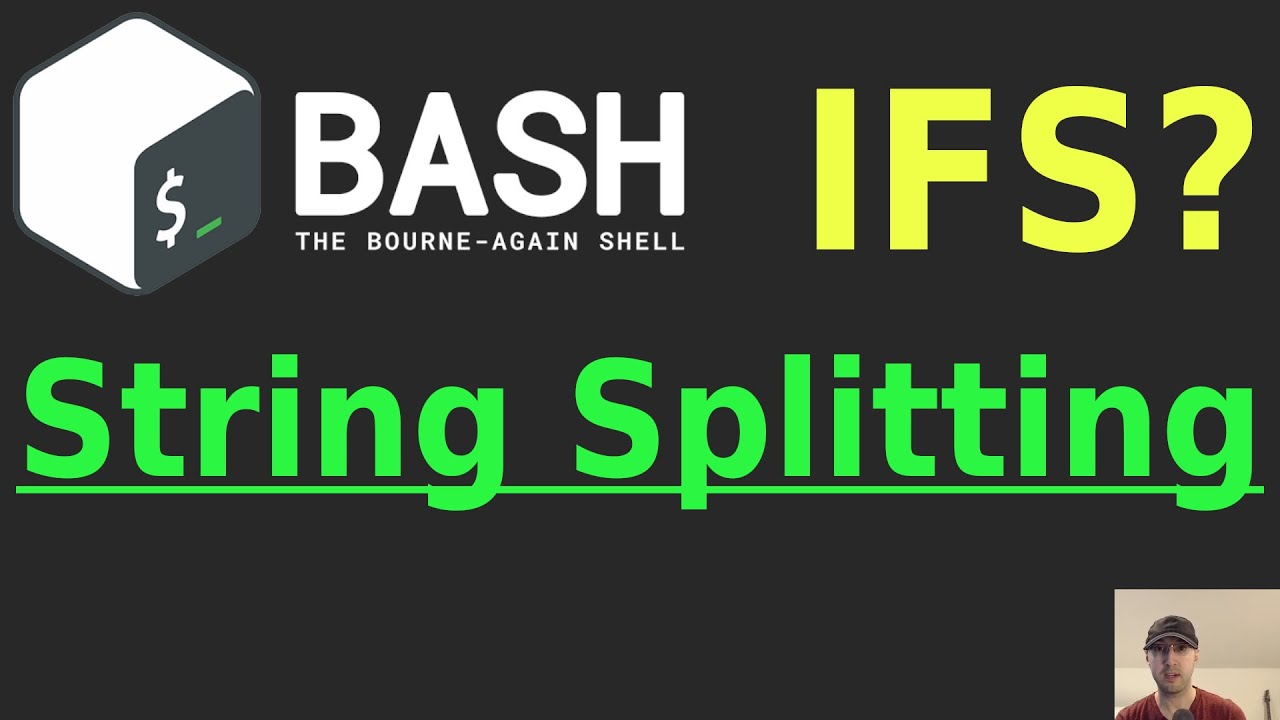
Article link: loop through array bash.
Learn more about the topic loop through array bash.
- Bash For Loop Array: Iterate Through Array Values – nixCraft
- Loop through an array of strings in Bash? – Stack Overflow
- Array Loops in Bash – Stack Abuse
- 10 Bash For Loop Examples with Explanations – Geekflare
- Bash “For” Loop To Iterate Through an Array – Linux Hint
- Looping through arrays in bash with a thorough syntax …
- Bash Tutorial => Looping through an array
- Iterating in Reverse Using for Loops and Other Constructs
See more: https://nhanvietluanvan.com/luat-hoc