List Of Alphabets Python
Introduction:
Python, a versatile and widely-used programming language, offers a plethora of features and functionalities that make it a popular choice among developers. When it comes to manipulating and processing alphabets, Python provides several techniques and libraries that simplify the task.
This article delves into the world of alphabets in Python, exploring various alphabet-related operations, functions, and techniques. From validating alphabets to sorting and pattern generation, we will cover the essential aspects of working with alphabets in Python. So, let’s dive in!
1. ASCII Alphabets in Python:
ASCII (American Standard Code for Information Interchange) is a character encoding standard used to represent alphanumeric characters in computer systems. In Python, ASCII values can be utilized to represent alphabets and perform various operations. An example of using ASCII values to represent alphabets in Python can be demonstrated by converting characters to their respective ASCII values using the `ord()` function.
2. Unicode Alphabets in Python:
While ASCII is limited to representing a few characters, Unicode offers a vast set of characters from multiple languages and scripts, making it more comprehensive. Python supports Unicode characters, which includes a wide range of alphabets. Unicode values can be used in Python to represent alphabets from different languages and perform operations on them.
3. String Manipulation with Alphabets in Python:
Strings, a fundamental data type in Python, enable developers to store and manipulate sequences of characters, including alphabets. Python provides powerful techniques for manipulating alphabets within a string, such as indexing, slicing, and concatenation. These operations allow easy extraction, modification, and combination of alphabets within a string.
4. Alphabet Sequences in Python:
Python offers built-in functions and libraries to generate sequences of alphabets. Whether you need alphabets in ascending, descending, or random order, Python makes it convenient to create such sequences. These sequences can be utilized for various purposes, such as generating passwords or creating test data.
5. Alphabet Validation in Python:
Validating whether a particular input is an alphabet or not is an essential task in programming. Python provides different techniques to validate alphabets, including built-in functions and user-defined implementations. By exploring these techniques, you can determine whether a given input is an alphabet, facilitating data validation and input handling.
6. Alphabet Counting and Frequency Analysis in Python:
Counting the occurrences of each alphabet in a string or text file is a common requirement. Python enables you to count the frequency of each alphabet and perform advanced analysis using libraries like matplotlib to visualize the results. This information can be utilized for various purposes, such as identifying frequently used letters or checking language patterns.
7. Alphabetic Sorting in Python:
Sorting a list of alphabets is a common task in programming. Python provides various sorting techniques, including built-in functions and sorting algorithms, to sort alphabets in different orders. Whether you want to sort in ascending, descending, or case-insensitive order, Python has the tools to accomplish the task. This section compares different sorting techniques and explores their time complexities.
8. Alphabet Patterns in Python:
The ability to generate various patterns using alphabets is an intriguing aspect of Python programming. By utilizing nested loops and conditional statements, developers can create patterns like letters arranged in a pyramid or diamond shape. This section explores different techniques to generate such patterns and showcases example code.
9. Alphabetic Operations and Functions in Python:
Python provides a range of functions and operations to manipulate alphabets. From converting cases and finding substrings to replacing characters, these functions simplify complex alphabetic operations. Furthermore, advanced techniques like regular expressions can be employed for more intricate tasks. Covering these functions and operations, this section demonstrates their usage with example code snippets.
FAQs:
Q1. How can I print alphabets in Python?
Q2. How do I find an alphabet in a Python list?
Q3. How can I convert an alphabet to its corresponding number in Python?
Q4. How do I generate a list of alphabets in Python?
Q5. How can I get the position of a letter in the alphabet in Python?
Q6. How do I sort a list of alphabets in Python?
Q7. How can I concatenate two lists in Python?
Q8. How can I perform a frequency analysis of alphabets in Python?
Conclusion:
Working with alphabets in Python offers significant advantages for programmers. By understanding Python’s various techniques and operations related to alphabets, you can manipulate, validate, analyze, sort, and generate patterns with ease. This article serves as a comprehensive guide, equipping you with the knowledge and examples necessary to effectively handle alphabets in Python.
6: Python Program To Display All Alphabets From A To Z (Hindi)
What Are Alphabets In Python?
In programming, alphabets play a crucial role when working with strings, which are a sequence of characters. Alphabets refer to the collection of all the English alphabetic characters, from ‘a’ to ‘z’ (lowercase) and from ‘A’ to ‘Z’ (uppercase). Python, being a popular programming language, provides various ways to work with alphabets, allowing programmers to manipulate and perform different operations on them effortlessly.
Understanding Alphabets in Python:
In Python, alphabets are represented by the built-in data type known as strings. A string is a sequence of characters enclosed within quotes, either single (‘ ‘) or double (” “).
To define a string in Python, we can assign a series of alphabets to a variable. For instance:
“`python
alphabet = ‘abcdefghijklmnopqrstuvwxyz’
“`
With this definition, we have assigned all lowercase alphabets to the variable ‘alphabet’. Similarly, we can define a string for uppercase alphabets as well:
“`python
alphabet = ‘ABCDEFGHIJKLMNOPQRSTUVWXYZ’
“`
Python provides several useful methods and operations to manipulate alphabets in strings. For example, one can extract specific characters from a string, search for a particular alphabet, convert alphabets to lowercase or uppercase, and many more.
Common Operations on Alphabets in Python:
1. Accessing Characters:
To access individual characters in a string, we can use indexing. Every character in a string is assigned a unique index, starting from zero. For example:
“`python
alphabet = ‘abcdefghijklmnopqrstuvwxyz’
print(alphabet[0]) # Output: ‘a’
print(alphabet[25]) # Output: ‘z’
“`
2. Slicing Strings:
Slicing allows us to extract a substring from a given string. We can slice a string by specifying the start and end indices separated by a colon (:). For example:
“`python
alphabet = ‘abcdefghijklmnopqrstuvwxyz’
print(alphabet[0:5]) # Output: ‘abcde’
print(alphabet[3:8]) # Output: ‘defgh’
“`
3. Changing Case:
Python provides built-in methods to convert alphabets to lowercase or uppercase. The lower() method converts all characters in a string to lowercase, while the upper() method converts them to uppercase. For example:
“`python
alphabet = ‘abcdefghijklmnopqrstuvwxyz’
print(alphabet.upper()) # Output: ‘ABCDEFGHIJKLMNOPQRSTUVWXYZ’
print(alphabet.lower()) # Output: ‘abcdefghijklmnopqrstuvwxyz’
“`
4. Checking Alphabets:
We can use various methods to check if a character or a string contains alphabets only. The isalpha() method returns True if all characters in a string are alphabets; otherwise, it returns False. For example:
“`python
string = ‘HelloWorld’
print(string.isalpha()) # Output: True
string = ‘Hello World 123’
print(string.isalpha()) # Output: False
“`
5. Joining Alphabets:
The join() method allows us to concatenate alphabets from a sequence into a single string. For example, we can join all lowercase alphabets with a specific delimiter:
“`python
delimiter = ‘-‘
alphabets = ‘abcdefghijklmnopqrstuvwxyz’
joined = delimiter.join(alphabets)
print(joined) # Output: ‘a-b-c-d-e-f-g-h-i-j-k-l-m-n-o-p-q-r-s-t-u-v-w-x-y-z’
“`
FAQs about Alphabets in Python:
Q: Can we include non-English alphabets in Python strings?
A: Yes, Python supports Unicode, which allows representation of characters from various languages.
Q: How can we find the index of an alphabet in a string?
A: We can use the index() method that returns the first occurrence of a specified alphabet in a string.
Q: Can we convert alphabets to ASCII values in Python?
A: Yes, the ord() function can be used to convert a single character to its corresponding ASCII value.
Q: How can we count the occurrences of a specific alphabet in a string?
A: Python provides the count() method that returns the number of occurrences of a specified alphabet or substring in a string.
Q: Is it possible to substitute alphabets in a string with other characters?
A: Yes, Python allows us to replace alphabets using the replace() method which substitutes one or more occurrences of a specified alphabet or substring with another.
In conclusion, alphabets in Python are an essential aspect when working with strings. They can be accessed, sliced, changed in case, checked for validity, joined, and manipulated in various other ways using built-in methods and functions. Understanding alphabets and their operations in Python is crucial for performing string manipulation and processing tasks effectively.
What Is The Range Of Alphabets In Python?
Python, a high-level programming language known for its simplicity and readability, uses Unicode to represent characters, including alphabets. Unicode is a computing industry standard that provides a unique number for every character, regardless of the platform or language. In Python, the range of alphabets extends beyond the basic Latin alphabet to encompass a vast array of international characters, making it a versatile tool for handling text.
The Unicode Standard currently encompasses over 140,000 characters, including alphabets, punctuation marks, symbols, and even emojis. It supports characters from almost all writing systems used around the world. Python, being designed with the principles of inclusivity and flexibility in mind, fully embraces Unicode and offers extensive support for multilingual text handling.
The range of alphabets in Python covers various writing systems such as Latin, Cyrillic, Greek, Arabic, Chinese, Japanese, Korean, and many others. Let’s explore some of the prominent alphabets present within the Unicode range accessible in Python:
1. Basic Latin (ASCII): This is the most common alphabet subset of the Unicode Standard. It encompasses the 26 alphabets used in English and other Western European languages, including both uppercase and lowercase letters.
2. Extended Latin: This subset extends beyond the basic Latin alphabet, incorporating additional diacritical marks and characters used in languages such as French, German, Spanish, and others.
3. Cyrillic: The Cyrillic alphabet is used in various Slavic languages, including Russian, Ukrainian, and Bulgarian. Python supports both uppercase and lowercase Cyrillic letters, allowing for seamless manipulation of text in these languages.
4. Greek: The Greek alphabet, essential for handling texts in Greek, uses a unique set of characters. Python includes the Greek alphabet within its Unicode range, facilitating the programming needs of Greek-speaking developers.
5. Arabic: The Arabic script, which plays a vital role in languages like Arabic, Persian, and Urdu, has a distinct set of characters. Python encompasses the complete Arabic alphabet, enabling software developers to process and manipulate Arabic text effectively.
6. Chinese, Japanese, and Korean (CJK): These East Asian writing systems are a complex set of ideographic characters. While Python can handle CJK characters, understanding the intricacies of these scripts requires specialized knowledge and additional processing techniques due to their unique nature.
7. Other Alphabets: Python also supports various other alphabets such as Hebrew, Devanagari (used for Hindi and other Indian languages), Thai, and others, allowing developers to work with diverse languages and scripts.
FAQs:
Q1. Can Python handle all characters from all languages?
A1. Yes, Python, being based on Unicode, can handle characters from almost all languages and writing systems. It provides the necessary tools and functions to manipulate and process text, regardless of its origin.
Q2. How do I print a specific character in Python?
A2. To print a specific character, you can make use of the `print()` function in Python and refer to the character using its Unicode code point. For example, `print(chr(65))` will print the character ‘A,’ as the Unicode code point for ‘A’ is 65.
Q3. Can Python convert text between different writing systems?
A3. Yes, Python offers powerful encoding and decoding functions that allow for seamless conversion between different character encodings, including those used by various writing systems. The `encode()` and `decode()` methods enable you to convert text from one encoding to another.
Q4. Are there any limitations to handling certain characters in Python?
A4. While Python can handle a vast range of characters, some specialized operations may require additional considerations. For instance, sorting characters from different scripts might need locale-specific collation rules. It’s crucial to be aware of potential challenges and consult Python’s official documentation when working with specific characters or scripts.
Q5. How can I determine the Unicode code point of a particular character?
A5. To find the Unicode code point of a character in Python, you can use the `ord()` function. For example, `print(ord(‘A’))` will display the Unicode code point of the character ‘A,’ which is 65.
In conclusion, the range of alphabets in Python extends far beyond the basic Latin alphabet, covering various writing systems used worldwide. With its strong support for Unicode, Python empowers developers to work with text from diverse languages, enabling the creation of versatile and inclusive software applications.
Keywords searched by users: list of alphabets python Print alphabets in Python, Python list find, Alphabet Python, Convert alphabet to number Python, Generate list Python, Get position of letter in alphabet python, Sort alphabet Python, List plus list Python
Categories: Top 69 List Of Alphabets Python
See more here: nhanvietluanvan.com
Print Alphabets In Python
In python, printing alphabets can be achieved in various ways. This article will cover the different methods to print alphabets in python, giving beginners a thorough understanding of the topic.
Method 1: Using ASCII Values
ASCII (American Standard Code for Information Interchange) is a widely used character encoding standard. Each character is assigned a unique numerical value in ASCII, which can be used to represent alphabets in python.
To print the alphabets using ASCII values, we can utilize a for loop. Declare a variable ‘i’ and initialize it with the ASCII value of ‘a’ (97). In each iteration, increment ‘i’ by 1 and convert the ASCII value back to character using the chr() function. Here’s an example:
“`python
for i in range(97, 123):
print(chr(i), end=’ ‘)
“`
The range() function generates numbers from the starting value (97) to the ending value (122), and chr() converts these numbers back to their corresponding characters. The end=’ ‘ argument ensures the characters are printed on the same line.
Method 2: Utilizing the string module
Python’s string module provides a constant string of all alphabets, both lowercase and uppercase. Let’s take a look at an example:
“`python
import string
print(string.ascii_lowercase)
“`
The string.ascii_lowercase variable contains all lowercase alphabets. Similarly, string.ascii_uppercase contains all uppercase alphabets. You can use these variables to print alphabets in python without the need for any loops. However, keep in mind that you will need to import the string module to use these variables.
Method 3: Using List Comprehensions
Python allows us to use list comprehensions, which provide a concise way to generate a list. We can leverage this feature to print the alphabets.
“`python
alphabets = [chr(i) for i in range(97, 123)]
print(‘ ‘.join(alphabets))
“`
The list comprehension above generates a list of characters using the range() function and chr(), as discussed earlier. We then use the join() method to convert this list into a single string separated by spaces.
FAQs
Q1. Can I print a specific range of alphabets?
Yes, you can print a specific range of alphabets by modifying the start and end values in the range() function. For example, to print the alphabets from ‘c’ to ‘f’, you can use `for i in range(99, 103)`.
Q2. How can I print uppercase alphabets?
You can use the string.ascii_uppercase variable from the string module to print uppercase alphabets. Here’s an example: `print(string.ascii_uppercase)`
Q3. Is it possible to print alphabets in a reverse order?
Yes, you can print alphabets in reverse order by manipulating the range() function. For instance, to print lowercase alphabets in reverse, you can use `for i in range(122, 96, -1)`.
Q4. Can I include both lowercase and uppercase alphabets in the output?
Certainly! You can concatenate the string.ascii_lowercase and string.ascii_uppercase variables to include both lowercase and uppercase alphabets. Here’s an example: `print(string.ascii_lowercase + string.ascii_uppercase)`
Q5. What if I want to print alphabets in a column instead of a row?
To print alphabets in a column, you can modify the print statement within the loop. Simply remove the end=’ ‘ argument to print each character on a new line.
Conclusion
Printing alphabets in Python can be achieved using ASCII values, the string module, or list comprehensions. Beginners can choose the method most suitable to their needs. This article covered the different approaches and also provided FAQs to address common queries. With these techniques, you can easily print alphabets and explore further possibilities in your Python programs.
Python List Find
Python, a widely-used programming language, provides a multitude of built-in functions and methods to handle data efficiently. One such function is the list_find() method, which allows developers to search for a specific value within a Python list. In this article, we will delve into the intricacies of Python list find, explore its syntax and usage, discuss its advantages, and address some frequently asked questions.
Understanding the Basics of Python List Find:
Before we explore the list_find() method, let’s briefly understand what a Python list is. A list is an ordered collection of items, which can be of different data types such as integers, strings, or even other lists. These items are enclosed within square brackets and separated by commas.
Python list find, also known as the index() method, is used to locate the position of the first occurrence of a specified value within a given list. Its syntax is as follows:
“`
list_name.index(value, start_index, end_index)
“`
Here,
– `list_name` refers to the name of the list in which the search operation is to be performed.
– `value` is the desired element that we want to find within the list.
– `start_index` (optional) specifies the index from where the search should start. By default, the search will begin from the beginning of the list.
– `end_index` (optional) defines the index at which the search should end. By default, the search will continue until the end of the list.
It is important to note that if the specified value is not found within the list, Python will raise a `ValueError` exception. Therefore, it is advisable to handle this exception using try…except blocks to ensure smooth execution.
Advantages of Using Python List Find:
1. Efficient Searching: List find allows developers to quickly locate elements within a list, eliminating the need for complex iteration or custom implementations.
2. Versatile Application: Python list find can be used with lists containing diverse data types, enabling efficient searching in different scenarios, ranging from simple lists of strings to complex nested lists.
3. Simple Syntax: The list_find() method comes with a straightforward syntax, making it easy for developers to understand and implement in their codebase without any additional overhead.
4. Minimalistic Approach: By using the built-in list_find() function, developers can avoid cluttering their codebase with custom search algorithms, leading to more readable and concise code.
5. Multiple Matches: In scenarios where there are multiple occurrences of the desired value within the list, list_find() method returns the index of the first occurrence. By iterating over the list until the function returns -1, you can find all the occurrences present in the list.
Frequently Asked Questions (FAQs):
Q1. Can Python list find be used with lists containing nested lists?
Yes, Python list find is versatile and can be used with nested lists as well. It will recursively search through the outer list and its nested lists to find the specified value.
Q2. Is Python list find case-sensitive?
Yes, Python list find is case-sensitive. It distinguishes between lowercase and uppercase letters, so “apple” and “Apple” would be treated as different values.
Q3. How does Python list find handle multiple occurrences of the specified value?
Python list find returns the index of the first occurrence of the specified value within the list. If there are multiple occurrences, you can iterate over the list until the function returns -1 to find all the indices or use a loop to find subsequent occurrences starting from the next index.
Q4. What happens if the desired value is not present in the list?
If the specified value is not found within the list, Python raises a `ValueError` exception. To handle this, it is recommended to enclose the list_find() method within a try…except block to prevent program interruptions.
Q5. Are there any alternatives to Python list find?
Yes, besides Python list find, developers can utilize other methods like using a for loop or list comprehension to achieve similar functionality. However, these alternatives may not be as efficient or concise as the list_find() method.
In conclusion, Python list find is a powerful and versatile function that enables efficient searching within Python lists. Its simplicity, speed, and ability to handle diverse data types make it an invaluable tool for developers. By understanding its syntax and advantages, you can leverage this function to streamline your codebase and improve overall programming efficiency.
Alphabet Python
Python is one of the most popular programming languages in the world, known for its simplicity and versatility. With its wide range of libraries and extensive community support, Python has become a go-to language for tasks ranging from web development to scientific computing. One of the many libraries that have gained significant attention in recent years is Alphabet Python.
Alphabet Python is a library developed by Alphabet Inc., the parent company of Google. Primarily designed for natural language processing (NLP) tasks, this library provides various tools and functionalities to analyze and manipulate textual data. In this article, we will delve into the details of Alphabet Python, exploring its key features, use cases, and how it can benefit developers and researchers alike.
Key Features of Alphabet Python:
1. Text Cleaning and Preprocessing: Alphabet Python offers a set of functions to clean and preprocess textual data. This includes removing unwanted characters, converting text to lowercase, splitting sentences into words, and removing stop words, among others. These preprocessing techniques are crucial for preparing text data before applying advanced NLP techniques.
2. Text Classification and Sentiment Analysis: Alphabet Python provides pre-trained models for text classification and sentiment analysis. Developers can leverage these models to classify texts into categories (e.g., spam or not spam, positive or negative sentiment). The models are trained on large-scale datasets, ensuring accurate predictions.
3. Named Entity Recognition (NER): NER is a core component of many NLP applications that involve identifying and categorizing named entities (such as names, organizations, locations) within text. Alphabet Python incorporates state-of-the-art NER models, allowing users to extract meaningful information from large amounts of text.
4. Language Translation: Alphabet Python integrates translation models that can convert text from one language to another. These models are based on neural networks and have been trained on vast multilingual datasets, resulting in robust and accurate translations.
5. Text Summarization: With the increasing amount of textual data available, automated text summarization has become an essential tool for information extraction. Alphabet Python offers extractive summarization methods that identify key sentences from a given text, providing users with concise summaries.
Use Cases and Applications:
1. Chatbots and Virtual Assistants: Alphabet Python is widely used in developing chatbots and virtual assistants, enabling them to comprehend and respond to user queries more effectively. By applying NLP techniques, chatbots can understand user intent, extract relevant information, and provide appropriate responses.
2. Content Analysis: The library’s text classification and sentiment analysis models have found applications in content analysis. By categorizing and analyzing large volumes of textual data, marketers and researchers can gain valuable insights from customer feedback, social media posts, or product reviews.
3. Information Extraction: Alphabet Python’s NER capabilities play a significant role in information extraction tasks. It can automatically extract relevant entities from news articles, legal documents, or research papers, assisting researchers in extracting valuable insights quickly.
4. Multilingual Websites and Translation Services: With its language translation models, Alphabet Python enables the development of multilingual websites and translation services. This is particularly useful for businesses operating in multiple countries, allowing them to provide content in users’ preferred languages.
5. News Aggregation and Summarization: News aggregators often deal with vast amounts of articles, and manual curation can be time-consuming. By utilizing Alphabet Python’s text summarization capabilities, the relevant content from various sources can be summarized, saving time and effort.
FAQs about Alphabet Python:
Q1. How do I install Alphabet Python?
To install Alphabet Python, you can use pip, the package installer for Python. Simply open your command prompt or terminal and execute the following command:
“`
pip install alphabet-python
“`
Q2. Is Alphabet Python free to use?
Yes, Alphabet Python is an open-source library and is free to use, modify, and distribute under the Apache 2.0 license.
Q3. Can I train my own models using Alphabet Python?
While Alphabet Python provides pre-trained models, you can also fine-tune the existing models or train your own models using your custom datasets. The library provides detailed documentation to guide you through the training process.
Q4. Does Alphabet Python support GPU acceleration?
Yes, Alphabet Python supports GPU acceleration using libraries such as TensorFlow. This allows for faster inference and training times, especially when working with large datasets.
Q5. What are the system requirements for running Alphabet Python?
To run Alphabet Python, you need a system with Python 3.6 or above and sufficient memory to handle the models’ size effectively. Additionally, GPU support requires a compatible GPU and the necessary drivers.
In conclusion, Alphabet Python is a powerful library that brings the natural language processing capabilities developed by Alphabet Inc. to developers and researchers. With its comprehensive set of features for text cleaning, classification, translation, and summarization, developers can build innovative NLP applications with ease. Its extensive applications range from chatbots and virtual assistants to content analysis and information extraction. Whether you are a seasoned developer or a researcher with little experience in NLP, Alphabet Python is a valuable asset in simplifying and enhancing your projects.
Images related to the topic list of alphabets python
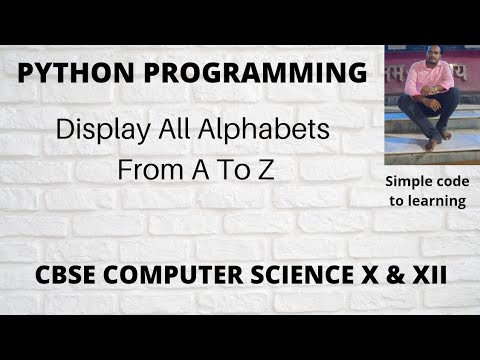
Found 33 images related to list of alphabets python theme
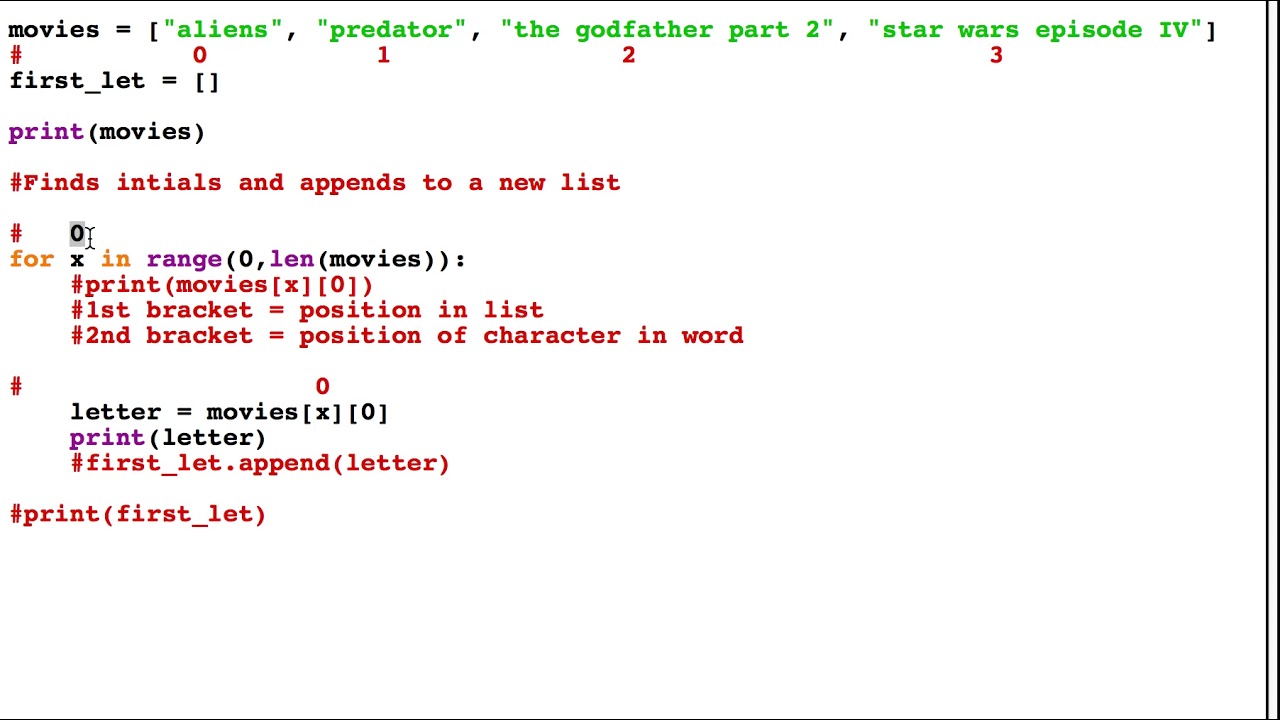
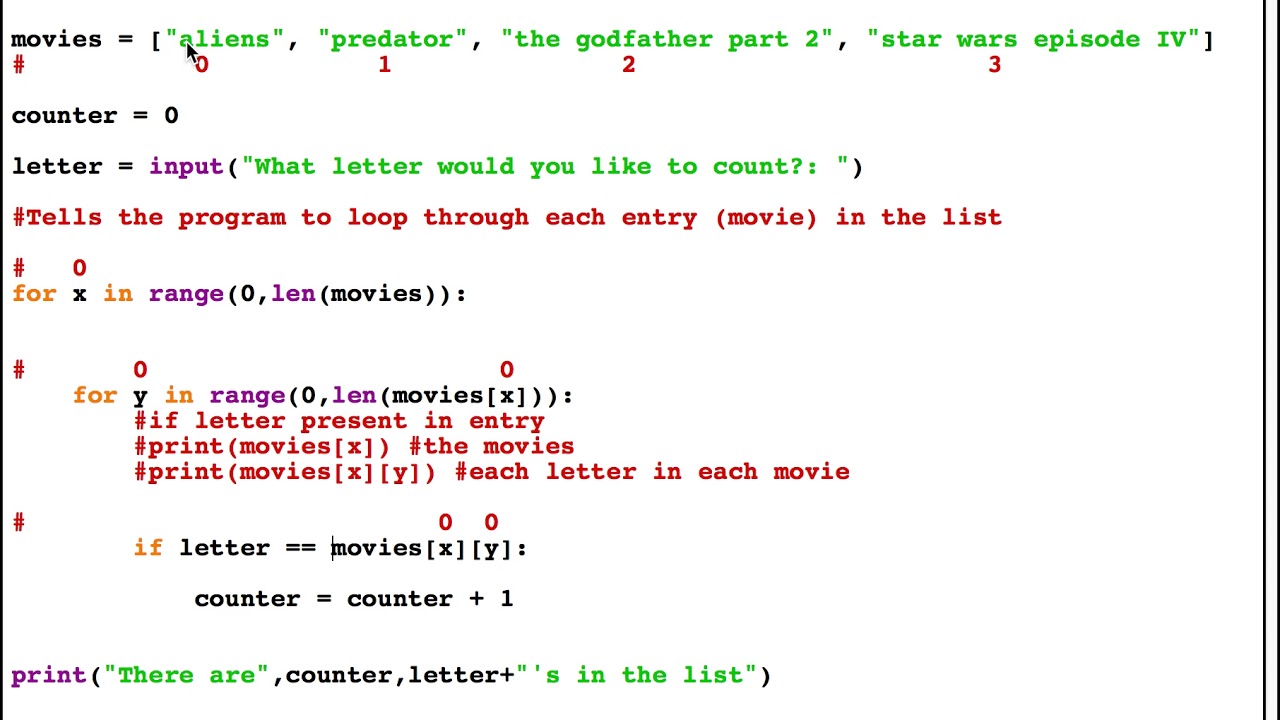
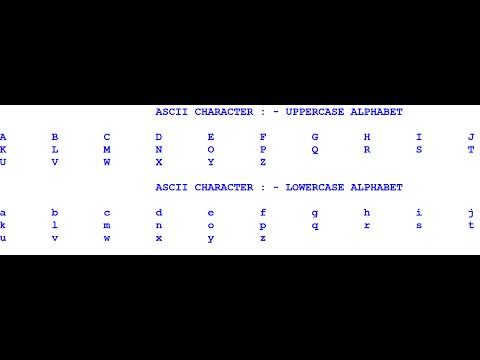


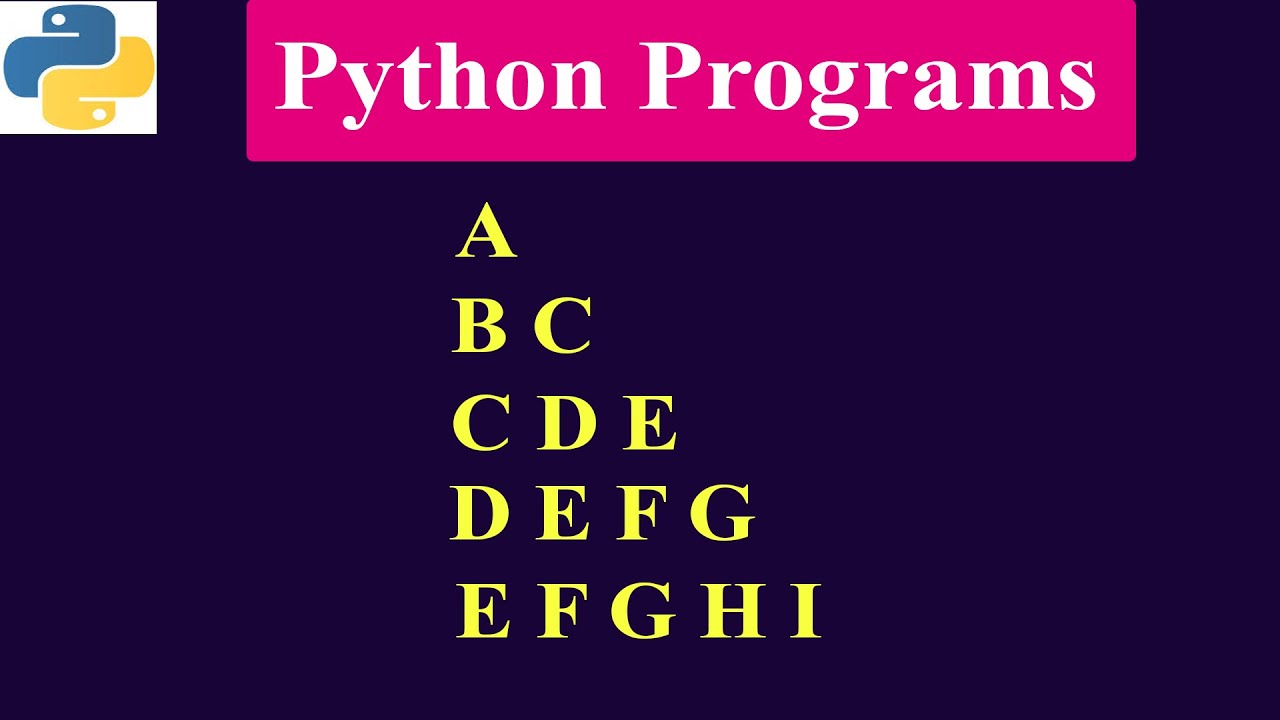


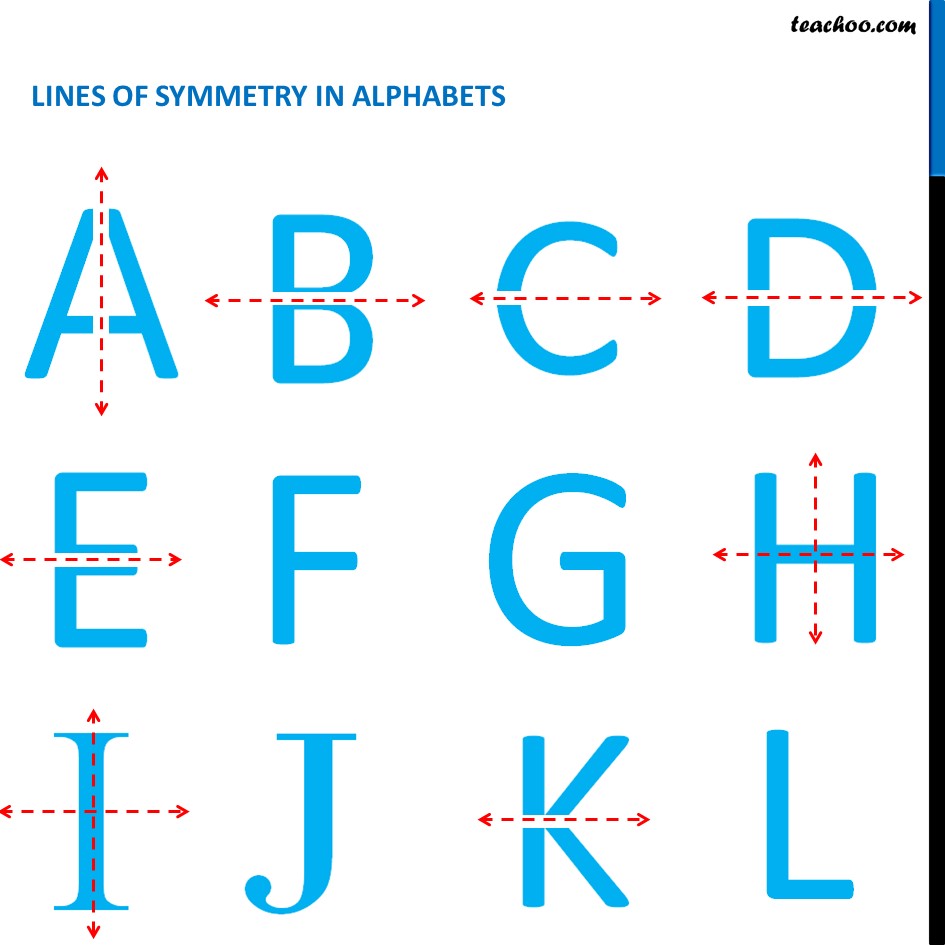
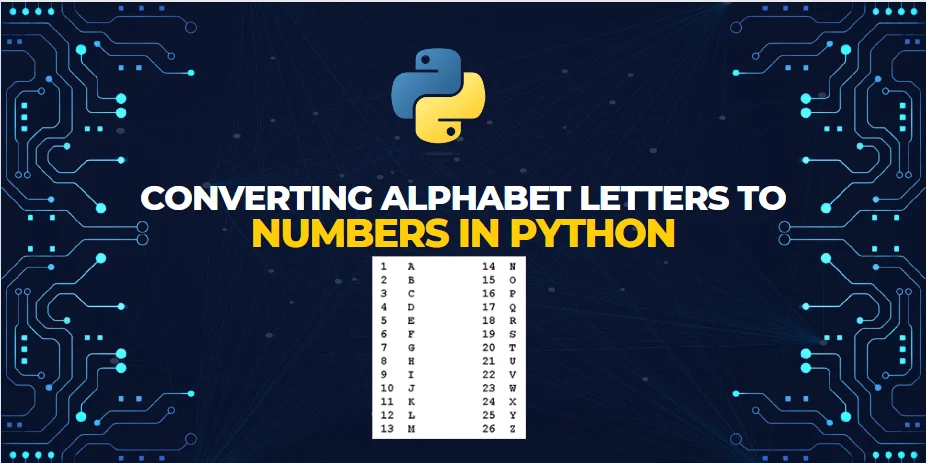
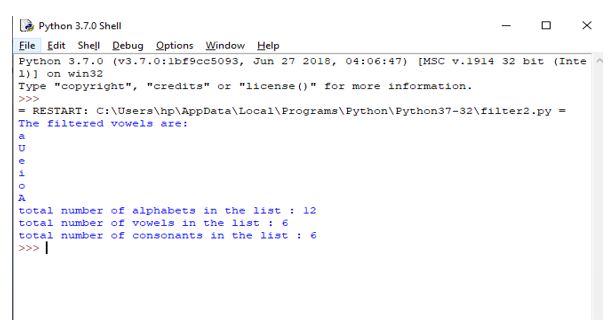
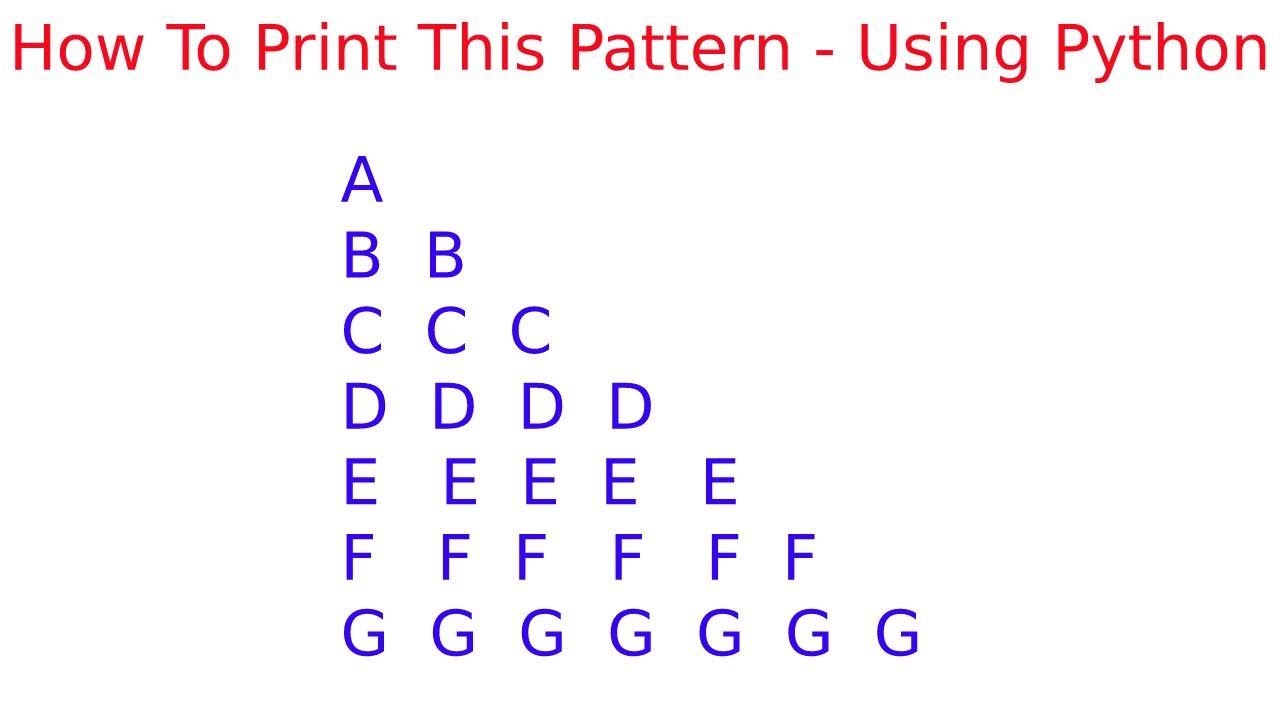
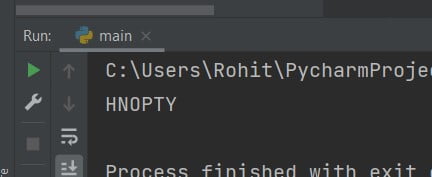
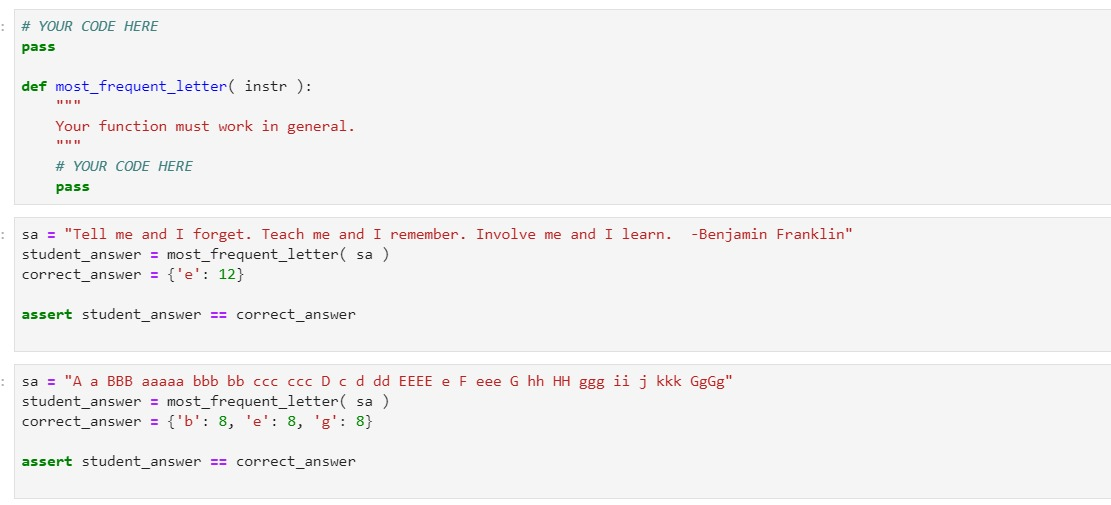
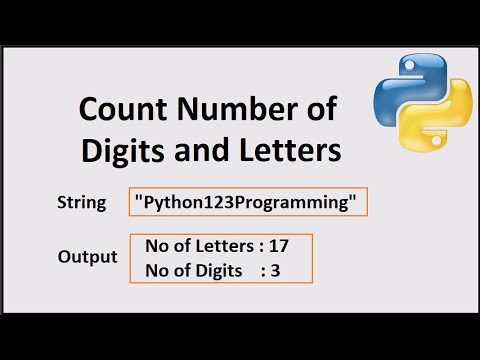
![Lines of symmetry in Alphabets [Full list + How to find] - Teachoo Lines Of Symmetry In Alphabets [Full List + How To Find] - Teachoo](https://d1avenlh0i1xmr.cloudfront.net/53e69ca7-ccb4-48c8-97d7-42dc6b002138/lines-of-symmetric-in-alphabets---teachoo.jpg)
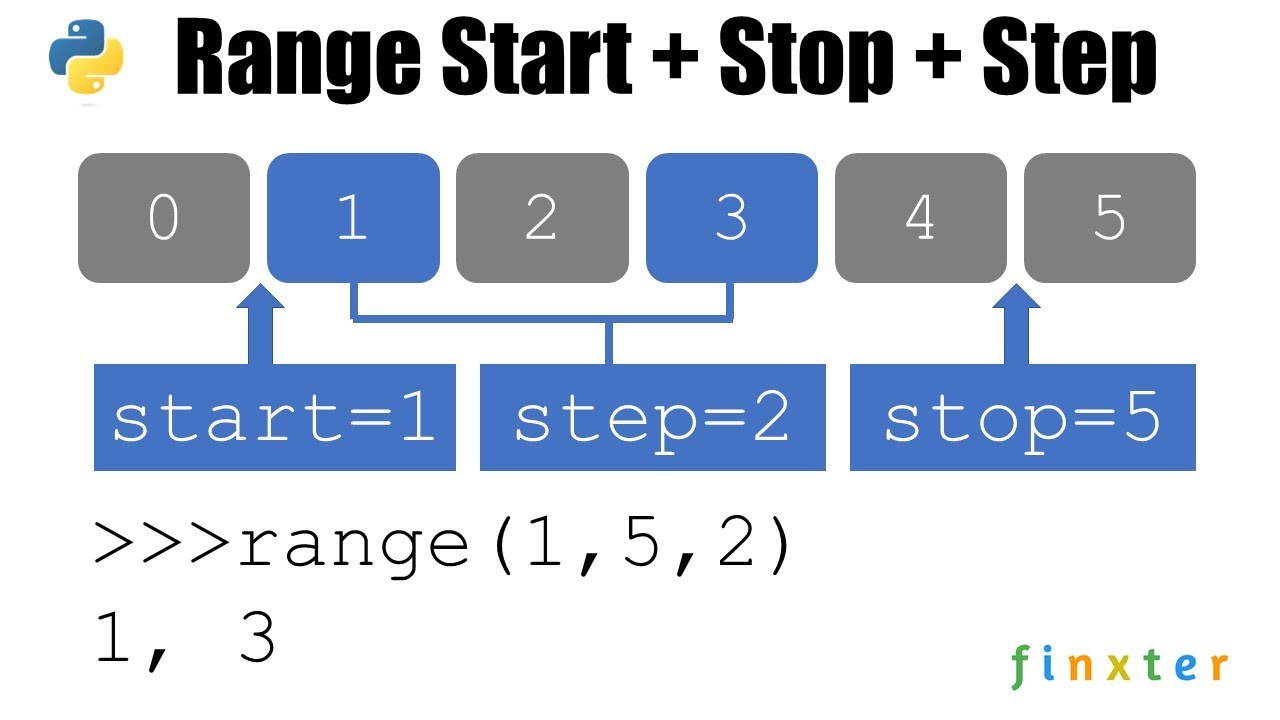

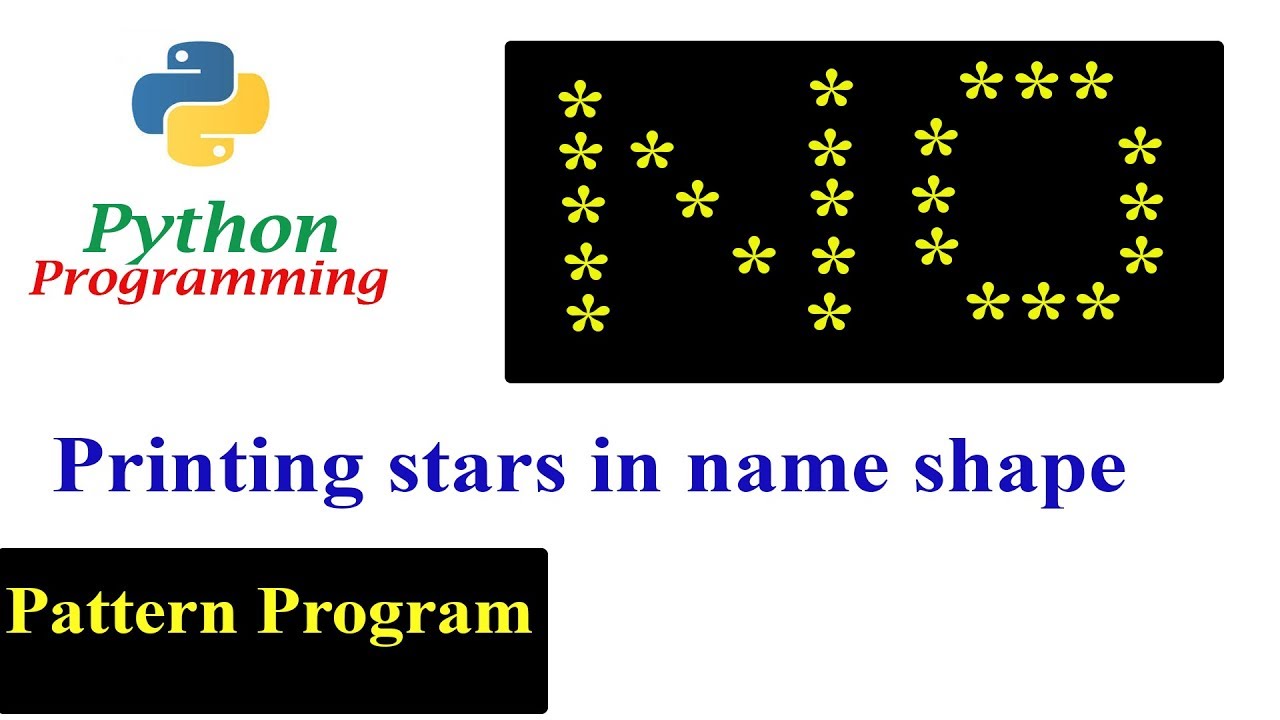
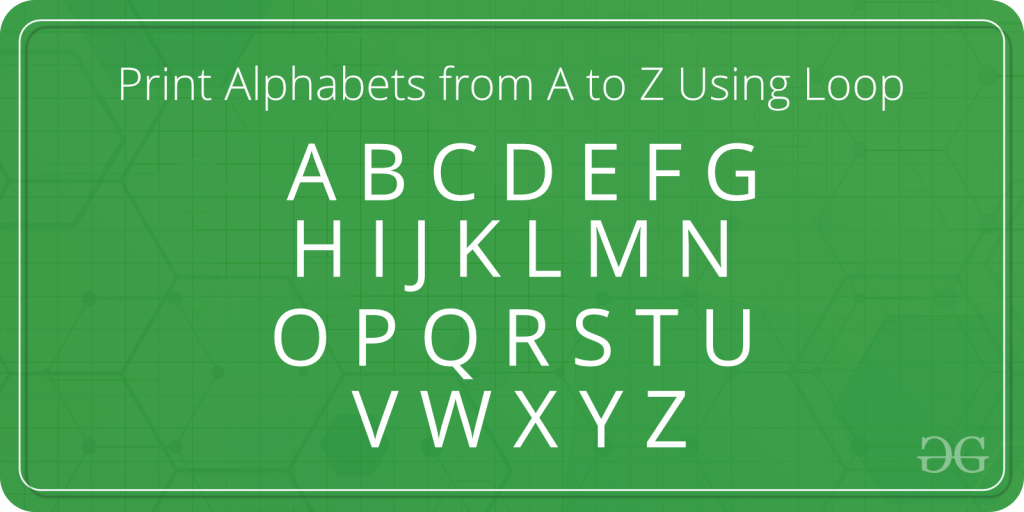

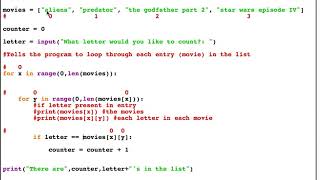

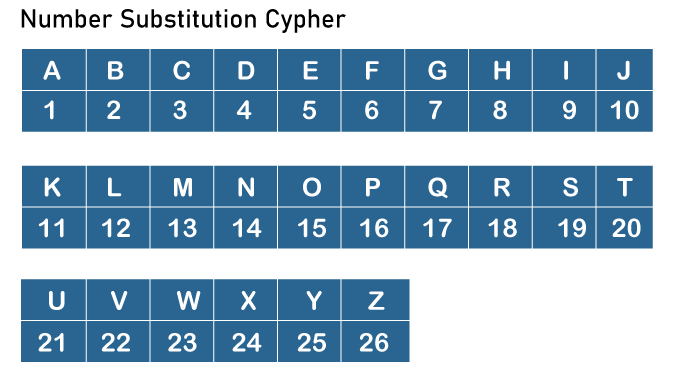
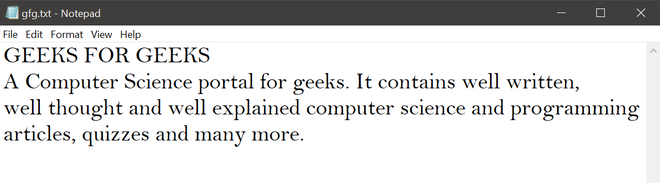
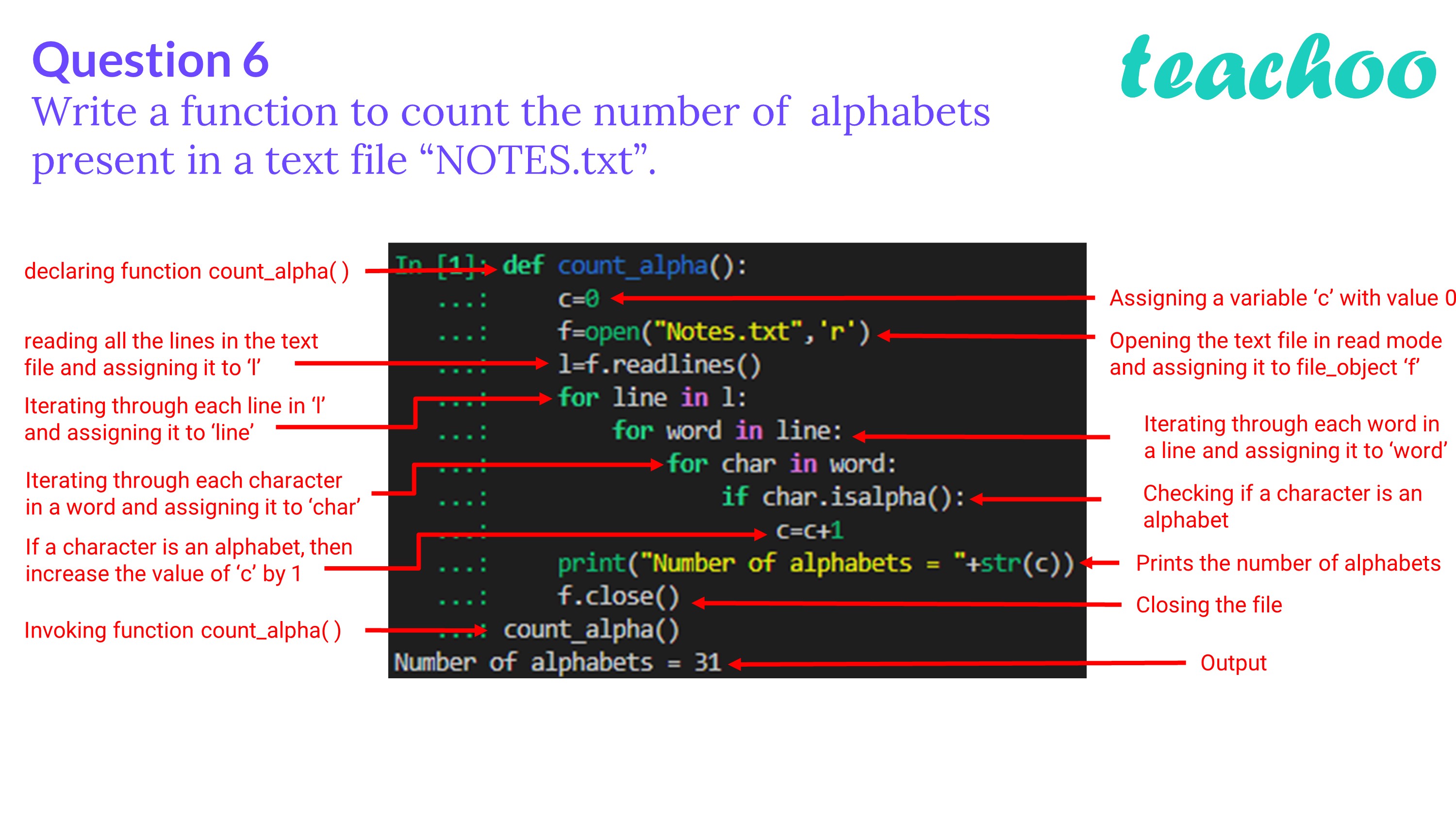

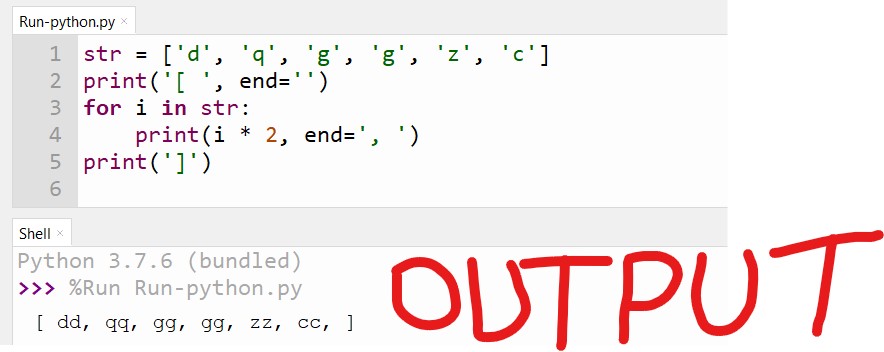
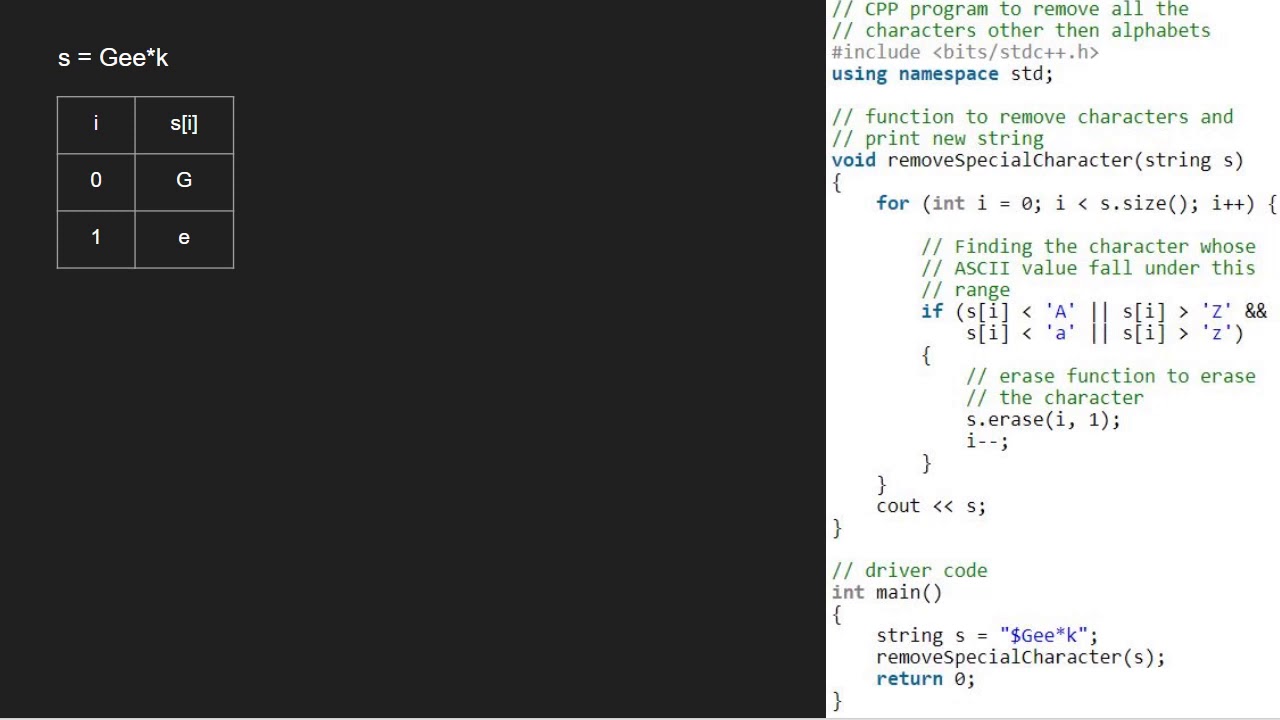
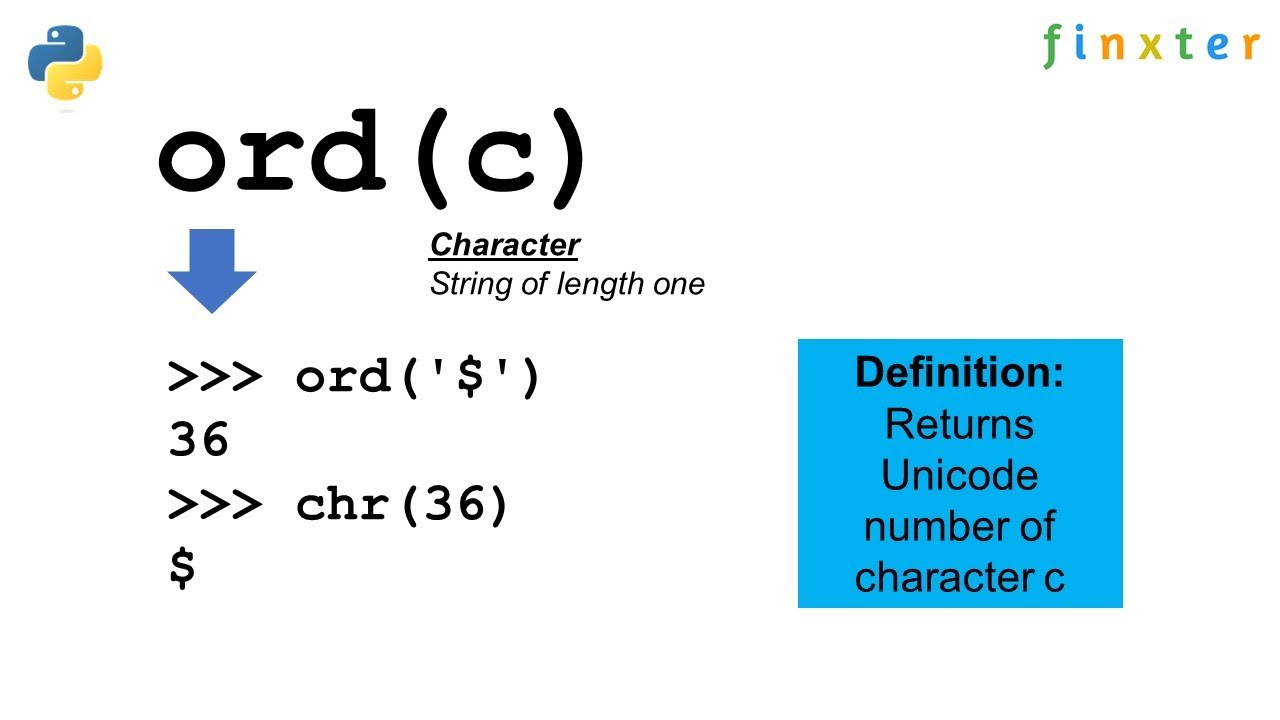

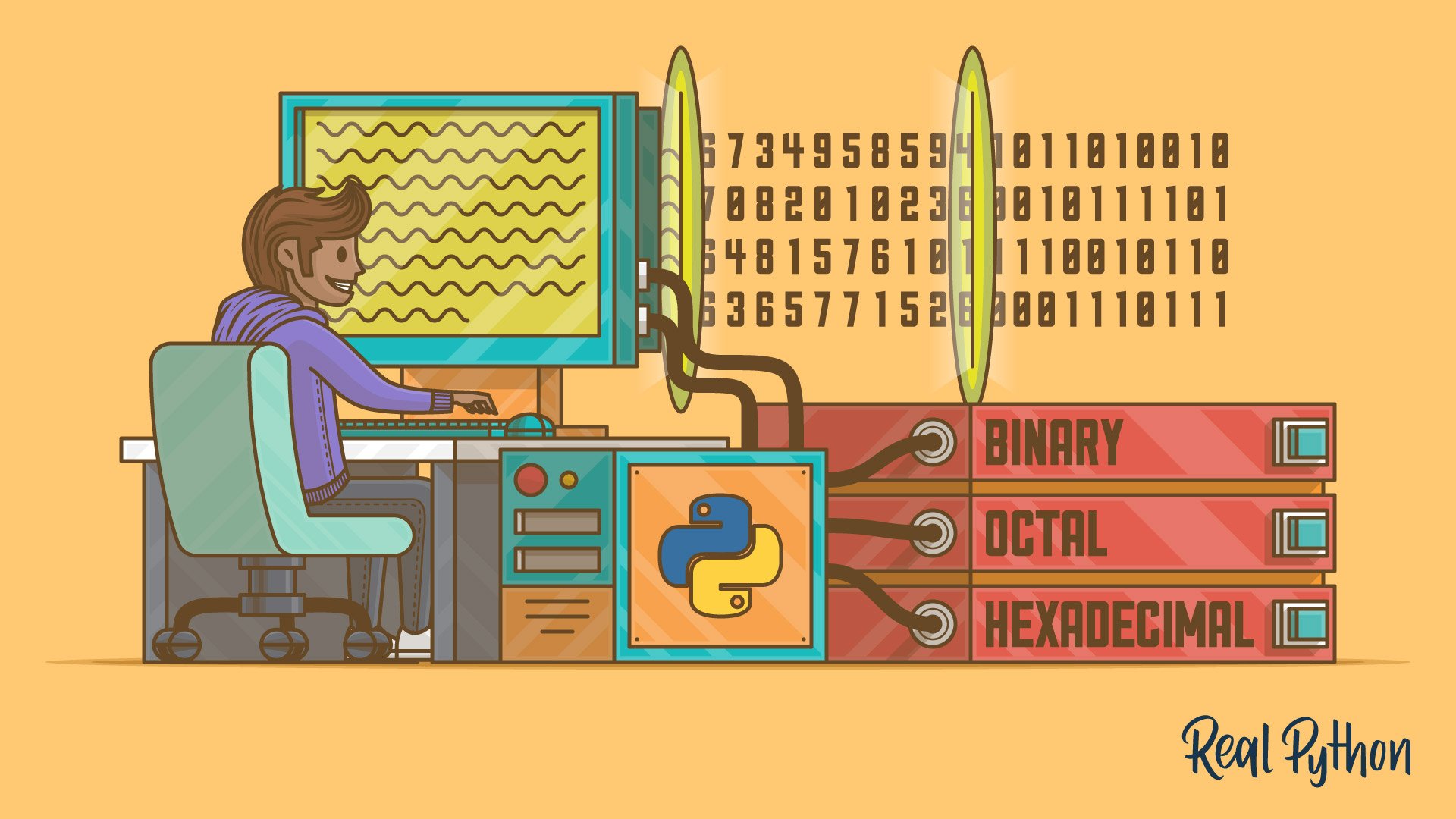
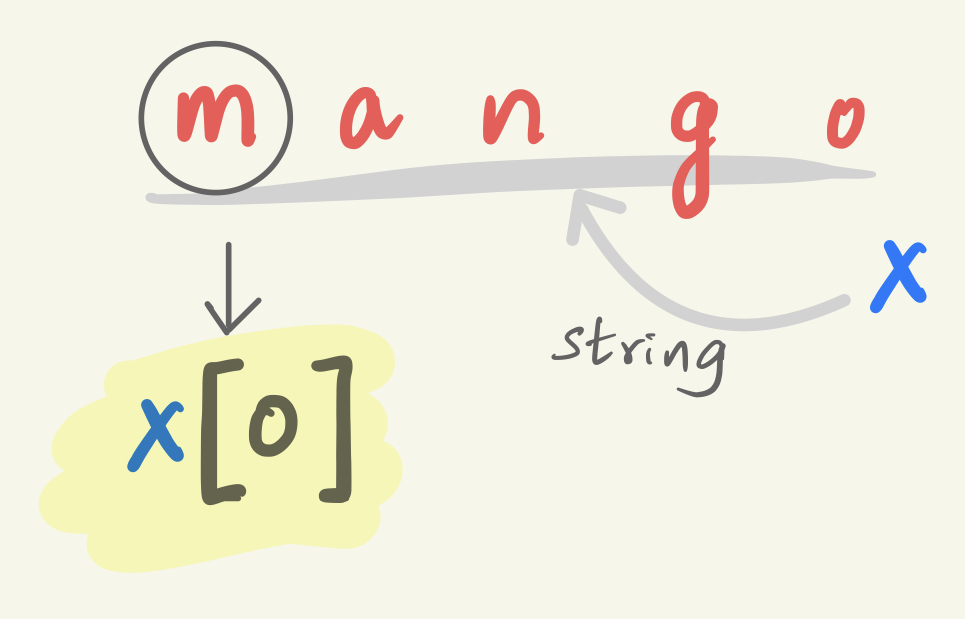
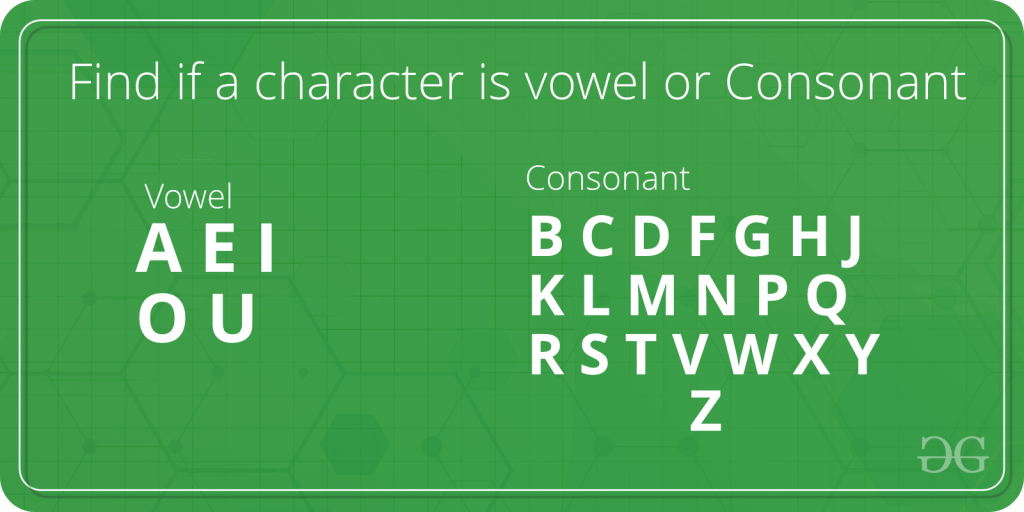

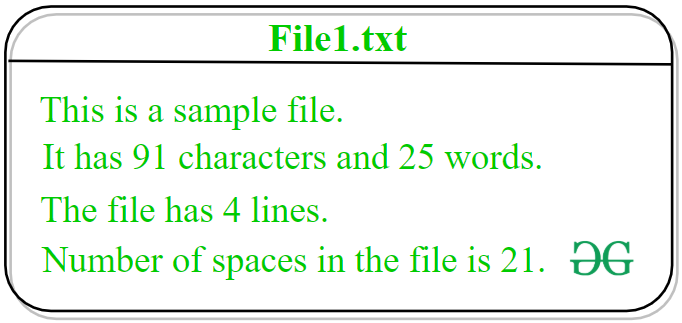
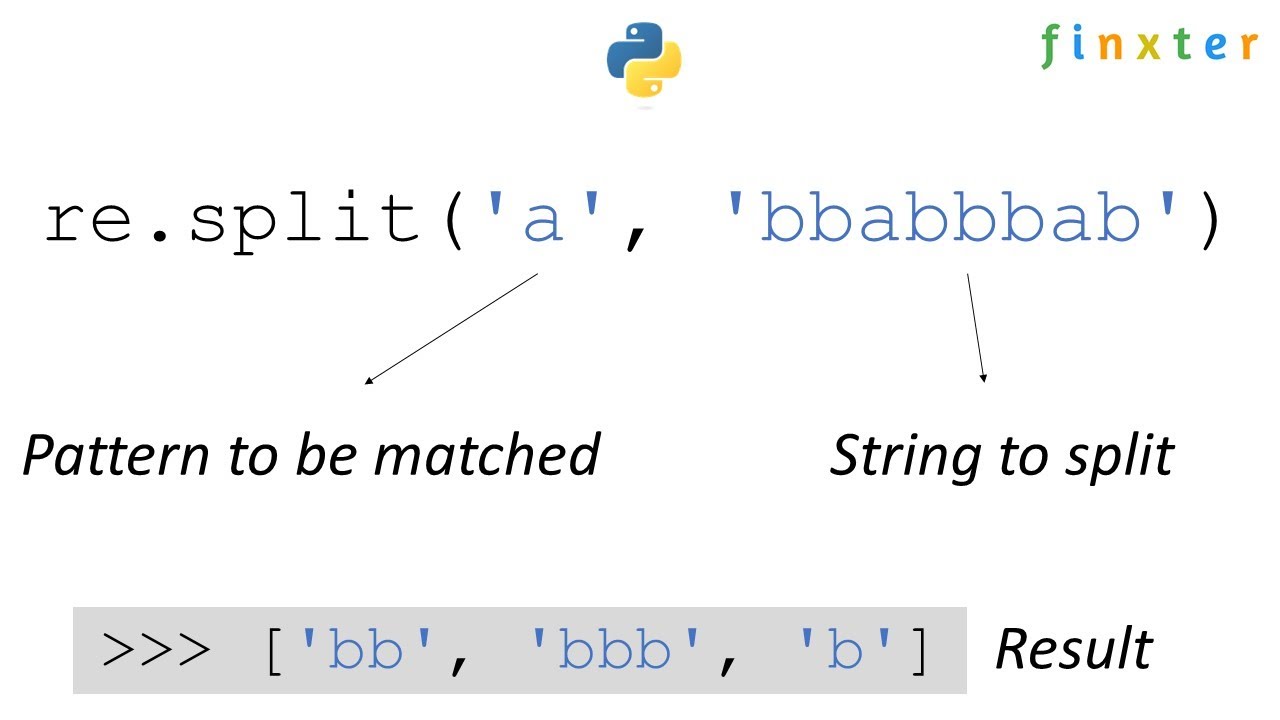

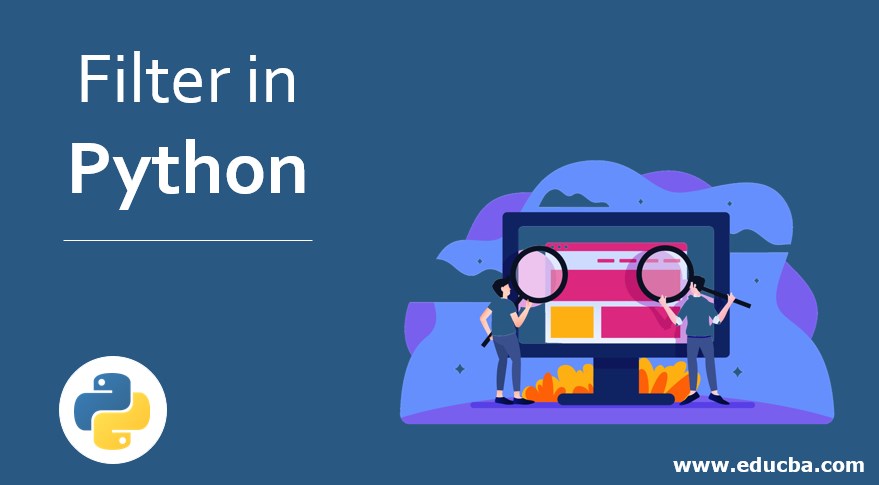
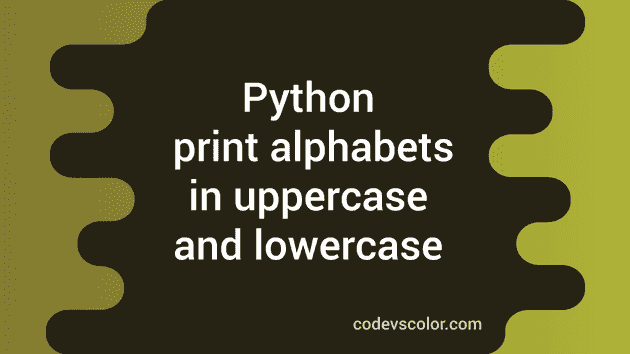
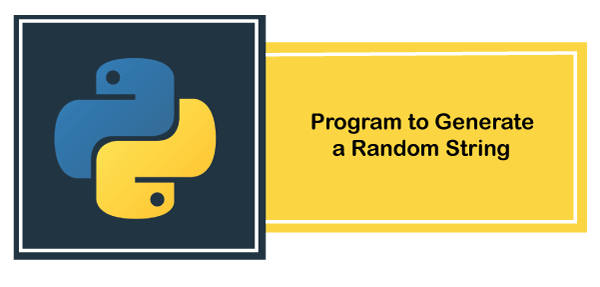


Article link: list of alphabets python.
Learn more about the topic list of alphabets python.
- How to Make a List of the Alphabet in Python – Datagy
- Alphabet range in Python – string – Stack Overflow
- How to make a list of the alphabet in Python – Adam Smith
- isalpha() in Python – Scaler Topics
- String – Alphabet Range in Python – AskPython
- Alphabet in Python – Javatpoint
- Python | Ways to initialize list with alphabets – GeeksforGeeks
- List the Alphabet in Python | Delft Stack
- Different ways to initialize list with alphabets in python
- How to Make a List of the Alphabet in Python – bobbyhadz
- How to Create an Alphabet List in Python? – ItSolutionStuff.com
- How to Easily Create a List of the Alphabet in Python – Oraask
See more: nhanvietluanvan.com/luat-hoc