Laravel Query Builder Exists
The Laravel query builder exists method is a powerful tool in the Laravel framework that allows developers to verify the presence of records in a database table. This method simplifies complex SQL queries by providing a more expressive and fluent interface for interacting with the database. In this article, we will explore the purpose of the Laravel query builder exists method, understand its syntax, and discover various ways of utilizing it to enhance your Laravel applications.
The Purpose of the Laravel Query Builder Exists Method
The primary purpose of the Laravel query builder exists method is to check the existence of records in a database table. It returns a boolean value indicating whether there are any matching records or not. This method is particularly useful when you need to conditionally perform certain actions based on the presence or absence of data in the database.
Understanding the Syntax of the Exists Method
The syntax of the Laravel query builder exists method is straightforward and easy to understand. It takes a Closure as an argument, which defines the query to be executed. Here’s an example of the basic syntax:
“`php
DB::table(‘users’)->whereExists(function ($query) {
$query->select(DB::raw(1))
->from(‘orders’)
->whereRaw(‘orders.user_id = users.id’);
});
“`
In this example, we are checking if there are any orders associated with a user. If there exists at least one order for a user, the query will return true, indicating that the user has orders. Otherwise, it will return false.
Using the Exists Method with Simple Queries
The Laravel query builder exists method can be used with simple queries to check for the existence of records matching certain criteria. Consider the following example:
“`php
$hasAdminRole = DB::table(‘users’)
->where(‘role’, ‘=’, ‘admin’)
->exists();
if ($hasAdminRole) {
// Perform admin-specific actions
} else {
// Perform user-specific actions
}
“`
In this case, we are checking if there is at least one user with the role ‘admin’. If such a user exists, we can perform actions specific to the admin role; otherwise, we can perform actions specific to regular users.
Applying the Exists Method with Subqueries
The Laravel query builder exists method can also be used with subqueries, allowing developers to perform more complex queries. Subqueries are queries nested within other queries. Let’s consider an example:
“`php
$orders = DB::table(‘orders’)
->whereExists(function ($query) {
$query->select(DB::raw(1))
->from(‘users’)
->whereRaw(‘users.id = orders.user_id’);
})
->get();
“`
In this example, we are retrieving all orders that are associated with existing users. By using the exists method with a subquery, we can easily filter out orders that belong to non-existing users.
Utilizing the Exists Method in Join Queries
The Laravel query builder exists method can also be used in join queries to check if a record exists in a related table. Let’s look at an example:
“`php
$orders = DB::table(‘orders’)
->join(‘users’, function ($join) {
$join->on(‘users.id’, ‘=’, ‘orders.user_id’)
->whereExists(function ($query) {
$query->select(DB::raw(1))
->from(‘products’)
->whereRaw(‘products.id = orders.product_id’);
});
})
->get();
“`
In this example, we are retrieving orders and joining them with users only if the corresponding product exists in the products table. This allows us to filter out irrelevant data and retrieve only valid records.
Performing Complex Queries with Exists Method in Conditional Statements
The Laravel query builder exists method can be used in conditional statements to perform complex queries efficiently. Let’s consider an example:
“`php
$query = DB::table(‘users’);
if ($isAdmin) {
$query->whereExists(function ($query) {
$query->select(DB::raw(1))
->from(‘roles’)
->whereRaw(‘roles.user_id = users.id’)
->where(‘roles.role’, ‘=’, ‘admin’);
});
}
$users = $query->get();
“`
In this example, we are retrieving users with specific roles based on a condition. If the user is an admin, we add an exists clause to check if they have the admin role. This conditional usage of the exists method allows for dynamic and flexible queries.
Optimizing Performance with the Laravel Query Builder Exists Method
To optimize the performance of your Laravel applications when using the exists method, you can follow a few best practices:
1. Use indexes on the columns involved in the exists condition. Indexing can significantly improve the performance of the query by speeding up the lookup process.
2. Avoid using complex or nested exists conditions, as they may impact performance. Instead, try to simplify your query and use indexes effectively.
3. Use eager loading to fetch related data in bulk, instead of relying on multiple exists queries. This can reduce the number of database hits and improve performance.
Best Practices for Using the Laravel Query Builder Exists Method
Here are some best practices to keep in mind when working with the Laravel query builder exists method:
1. Use the exists method only when necessary. In some cases, it might be more efficient to use other query builder methods like whereNotNull or whereExists.
2. Be mindful of the database structure and relationships when using subqueries with the exists method. Make sure the query reflects the desired logic accurately.
3. Don’t forget to sanitize user input before using it in the exists method to prevent potential security vulnerabilities such as SQL injection.
4. Familiarize yourself with other useful query builder methods like orWhereIn, db::select, and andWhere, as they can complement the exists method in various scenarios.
Conclusion
The Laravel query builder exists method is a valuable tool that simplifies database interactions and enhances the readability of your code. By understanding its purpose, syntax, and various usage scenarios, you can leverage the power of this method to optimize performance and create more efficient queries in your Laravel applications.
Part 15 Exist And Notexist In Laravel | Laravel Query Builder | How To Check Data Exist In Laravel
How To Check If Data Exists In Laravel?
When working with data in Laravel, it is crucial to check if the required data exists before performing any actions on it. By verifying the existence of data, you can ensure that your code functions smoothly and avoids any potential errors. In this article, we will explore various methods to check if data exists in Laravel and provide a comprehensive guide to help you utilize them effectively.
1. Checking if a Model Exists
One common scenario is to check if a record exists in the database based on your model. Laravel provides a convenient method called `exists()` that you can use to determine if a particular record exists. This method returns a boolean value, indicating whether the record is found or not. Here’s an example:
“`php
if (User::where(’email’, $email)->exists()) {
// The user with the given email exists
} else {
// The user does not exist
}
“`
In the above code snippet, we are checking if a user with a specific email address exists in the `users` table. Utilize the `where()` method to specify the condition for the existence check, and then call `exists()` to determine the result.
2. Checking if a Record Exists Based on Multiple Conditions
Sometimes, you may need to check if a record exists based on multiple conditions. Laravel provides the `count()` method to retrieve the number of matching records. By checking if this count is greater than zero, you can ascertain if any records exist. Here’s an example:
“`php
if (User::where(’email’, $email)->where(‘status’, ‘active’)->count() > 0) {
// At least one user with the given email and active status exists
} else {
// No users with the given email and active status found
}
“`
In the code above, we are verifying the existence of a user with a specific email and active status. The `count()` method counts the number of matching records and we perform a simple greater than zero check to determine existence.
3. Checking if a Data Collection Exists
If you are working with collections of data, Laravel provides several methods to check their existence. One such method is `isNotEmpty()`, which returns `true` if the collection is not empty, indicating that data exists within it. Here’s an example:
“`php
$users = User::where(‘status’, ‘active’)->get();
if ($users->isNotEmpty()) {
// The collection of active users is not empty
} else {
// No active users found
}
“`
In the code snippet above, we retrieve all active users and then use the `isNotEmpty()` method to check if any users are present. If the collection contains data, the code inside the `if` statement will execute.
4. Checking if a Variable or Array is Not Empty
Laravel also provides a helpful method called `filled()` to determine if a variable or an array is not empty. You can use this method to ensure that the required data is present before proceeding with any operations. Let’s consider an example:
“`php
$name = $request->input(‘name’);
if ($name->filled()) {
// The name input is not empty
} else {
// The name input is empty
}
“`
In the code above, we are checking if the `name` input field is not empty. By calling the `filled()` method on the `$name` variable, we can determine whether data is present to proceed with subsequent actions.
In addition to the above methods, Laravel provides many other ways to check for data existence based on different scenarios and requirements. Here are some frequently asked questions to further enhance your understanding:
FAQs:
Q1. Can I use the `exists()` method on custom-made models?
Yes, you can use the `exists()` method on any Eloquent model that extends the base `Illuminate\Database\Eloquent\Model` class. Ensure that your model class is correctly defined and mapped to the corresponding database table.
Q2. What’s the difference between `exists()` and `count()` methods?
The `exists()` method returns a boolean value indicating if a single record exists, while the `count()` method retrieves the number of matching records. Use `exists()` if you only need to check for the presence of a single record and `count()` if you require the number of matching records.
Q3. Can I combine multiple conditions while checking data existence?
Yes, you can easily combine multiple conditions using Laravel’s query builder methods. Simply chain the `where()` method to add additional conditions, allowing you to query based on multiple column values simultaneously.
In conclusion, it is essential to incorporate data existence checks into your Laravel projects to ensure accurate and error-free code execution. Laravel provides various methods to achieve this, including `exists()`, `count()`, `isNotEmpty()`, and `filled()`. By utilizing these methods effectively, you can ensure your application responds gracefully even when dealing with potential data issues.
Does Laravel Have Query Builder?
Yes, Laravel does have a powerful query builder that allows developers to build database queries using a convenient and expressive syntax. This query builder provides an ORM (Object-Relational Mapping) layer and a fluent interface to interact with the database.
The query builder in Laravel supports a variety of database systems, including MySQL, PostgreSQL, SQLite, and SQL Server. It provides a convenient way to write raw SQL statements, but also offers a higher-level abstraction for creating queries using PHP methods.
With the query builder, developers can easily perform common database operations such as selecting data, inserting records, updating existing entries, and deleting records. It also supports advanced features like joining tables, ordering results, grouping data, and limiting the number of returned records.
One of the main advantages of using Laravel’s query builder is its ease of use. With a simple and intuitive syntax, developers can write complex database queries without dealing with the cumbersome and error-prone process of writing raw SQL statements.
Setting up a basic query with Laravel’s query builder is as simple as calling the `table` method and passing the table name as a parameter. For instance, to select all records from a table called “users”, one could write:
“`php
$users = DB::table(‘users’)->get();
“`
This will return a collection of user records fetched from the database. The query builder also allows developers to specify the columns they want to select, apply conditions, and order the results:
“`php
$users = DB::table(‘users’)
->select(‘id’, ‘name’)
->where(‘age’, ‘>’, 18)
->orderBy(‘name’, ‘asc’)
->get();
“`
In this example, only the “id” and “name” columns of the “users” table will be fetched for users above the age of 18, sorted by name in ascending order.
Besides the basic CRUD operations, Laravel’s query builder supports various other functionalities. For example, developers can easily perform joins between tables using the `join` method, apply aggregate functions like `count` or `sum`, or even execute subqueries.
Another notable feature of Laravel’s query builder is the ability to write parameterized queries, which helps prevent SQL injection attacks. Developers can pass parameters to the query builder using the `where` method, providing an extra layer of security.
Moreover, Laravel offers an eloquent ORM built on top of the query builder, allowing developers to work with models and relationships instead of directly interacting with the database. Eloquent provides a higher level of abstraction, making it easier to manage complex database operations and maintain a clean and organized codebase.
FAQs
1. Can I use Laravel’s query builder with other PHP frameworks?
Yes, the Laravel query builder can be used independently of the Laravel framework. It is a standalone package that can be installed via Composer and used in any PHP project.
2. Is the query builder suitable for large-scale applications?
Yes, Laravel’s query builder is designed to handle large-scale applications efficiently. It provides various optimization techniques such as eager loading, query caching, and lazy loading, which help improve performance when dealing with large amounts of data.
3. What database systems does Laravel’s query builder support?
Laravel supports a wide range of database systems, including MySQL, PostgreSQL, SQLite, and SQL Server. The query builder utilizes the PDO library to establish connections and perform database operations, ensuring compatibility across different database platforms.
4. Can I still use raw SQL statements with Laravel’s query builder?
Yes, Laravel’s query builder allows developers to write raw SQL statements when needed. The `selectRaw`, `whereRaw`, and `orderByRaw` methods can be used to include raw SQL expressions in queries.
5. Does Laravel’s query builder support transactions?
Yes, Laravel’s query builder provides a simple and elegant way to handle database transactions. Developers can use the `transaction` method to start a new transaction, and the `commit` and `rollback` methods to commit or rollback the changes made within the transaction.
In conclusion, Laravel’s query builder is a powerful and versatile tool for interacting with databases. With its intuitive syntax, support for various database systems, and advanced features, it simplifies the process of writing database queries while promoting clean and maintainable code. Whether used independently or in conjunction with Laravel’s ORM, the query builder is an essential component for building efficient and scalable applications.
Keywords searched by users: laravel query builder exists Query builder Laravel, whereNotNull laravel, Where exists Laravel, Laravel query, db::raw laravel, orWhereIn laravel, db::select laravel, Andwhere Laravel
Categories: Top 88 Laravel Query Builder Exists
See more here: nhanvietluanvan.com
Query Builder Laravel
Laravel Query Builder offers a convenient way to interact with the database without directly writing SQL queries. It provides a consistent method syntax that makes it easy to read and understand the code. Additionally, Query Builder handles various edge cases and automatically escapes values, preventing SQL injection vulnerabilities.
Some of the key features of the Laravel Query Builder include:
1. Select Queries: With Query Builder, you can easily perform select queries to fetch records from the database. The `select` method allows you to specify the columns you want to retrieve. You can also apply various conditions using the `where` clause to filter the results.
2. Insert and Update Queries: Query Builder simplifies the process of inserting and updating records in the database. The `insert` method allows you to insert multiple rows at once, while the `update` method lets you update records based on specific criteria.
3. Join Queries: One of the powerful features of Query Builder is the ability to perform join queries. You can use the `join` method to join multiple tables based on common columns. Query Builder supports different types of joins, such as inner join, left join, and right join.
4. Sorting and Limiting: Query Builder provides methods like `orderBy` and `limit` to sort and limit the result set. You can easily specify the column and direction for sorting, as well as the number of records to retrieve.
5. Aggregates and Grouping: Query Builder allows you to perform aggregate functions like `count`, `sum`, `avg`, etc. You can also group the results based on a specific column using the `groupBy` method.
Now, let’s see how to use Query Builder in Laravel:
To start using Query Builder, you need to import the `DB` facade at the top of your file. The `DB` facade provides access to the Query Builder methods. Here’s an example of a simple select query using Query Builder:
“`
use Illuminate\Support\Facades\DB;
$users = DB::table(‘users’)
->select(‘name’, ’email’)
->where(‘age’, ‘>’, 18)
->get();
“`
In the above example, we select the ‘name’ and ’email’ columns from the ‘users’ table where the age is greater than 18. The `get` method retrieves the records that match the query.
Besides the basic usage, you can also chain multiple methods together to build more complex queries. For instance, you can perform joins, apply sorting and limiting, or even execute raw SQL queries if required.
FAQs:
Q: Can I use Query Builder with different database systems?
A: Yes, Laravel Query Builder is designed to work with various database systems, including MySQL, PostgreSQL, SQLite, and SQL Server. You just need to configure the database connection in the Laravel configuration file.
Q: Is Query Builder slower than writing raw SQL queries?
A: In most cases, the performance difference between Query Builder and raw SQL queries is negligible. Laravel’s Query Builder is optimized and generates efficient SQL queries. However, for complex queries, writing raw SQL might be more efficient.
Q: How can I debug and see the actual SQL queries generated by Query Builder?
A: Laravel provides a helpful `toSql` method that you can call on a Query Builder instance to see the generated SQL query without executing it. You can also enable the query log in Laravel’s configuration to log all executed queries.
Q: Can I use Query Builder in Laravel’s Eloquent ORM?
A: Laravel’s Eloquent ORM already utilizes Query Builder under the hood. So, if you are using Eloquent models to interact with the database, you can directly use Query Builder methods on your models.
In conclusion, Laravel Query Builder is a powerful tool that simplifies database interactions in PHP projects. It offers a fluent API, making it easy to build and execute SQL queries programmatically. Query Builder provides various features like select queries, insert and update queries, join queries, sorting, and limiting. It also handles edge cases and prevents SQL injection vulnerabilities. By leveraging Laravel Query Builder, developers can write cleaner and more maintainable code while interacting with the database efficiently.
Wherenotnull Laravel
Introduction
In Laravel, one of the most popular PHP frameworks, data validation plays a crucial role in ensuring the accuracy and integrity of your application’s data. Laravel provides developers with various methods to perform data validation, and among them, the “whereNotNull” method stands out as a powerful tool to validate and filter data. In this article, we will delve into the concept of whereNotNull Laravel and explore its benefits, usage, and best practices.
Understanding whereNotNull Laravel
Laravel’s whereNotNull method is an elegant solution for validating the presence of a specific column in a database table. It allows developers to filter query results based on the existence of a non-null value in the specified column. Essentially, this method acts as a conditional filter, letting you retrieve only the records that have a non-null value in a specific column.
Usage and Syntax
The whereNotNull method follows the convention of Laravel’s eloquent queries, making it intuitive and easy to use. Let’s take a look at the basic syntax:
“`php
$filteredData = Model::whereNotNull(‘column’)->get();
“`
In the example above, replace “Model” with the name of the Laravel Eloquent model you are querying, and “column” with the name of the column you want to validate. By executing this code, you will retrieve all records from the model’s table where the specified column is not null.
Benefits and Advantages
whereNotNull Laravel offers various benefits that enhance the data validation process in Laravel applications. Let’s explore some of its advantages:
1. Data Integrity: By utilizing whereNotNull, you can ensure that the retrieved data does not contain any null values in the specified column. This is especially useful when working with data that must have specific fields filled out.
2. Improved Performance: Filtering query results using whereNotNull helps optimize the performance of your application. By only retrieving records with non-null values, unnecessary data retrieval and processing can be avoided, resulting in faster response times.
3. Cleaner and More Maintainable Code: The whereNotNull method provides a clean and concise way to handle data validation in Laravel. It simplifies the codebase by encapsulating the validation logic in a single method, promoting code reusability and maintainability.
Best Practices
To make the most of whereNotNull Laravel, it is essential to follow some best practices when using this method:
1. Combine with Other Validation Methods: whereNotNull works effectively with other validation rules provided by Laravel. You can chain multiple validation methods to create more specific and comprehensive data validation scenarios.
2. Leverage Error Handling: When validating and filtering data, it is crucial to handle potential error scenarios gracefully. Laravel offers convenient error handling mechanisms through exceptions, which can be caught and displayed to the user or logged for debugging purposes.
3. Optimize Query Performance: Although whereNotNull helps optimize data retrieval, it is still important to optimize the underlying database queries for maximum performance. Utilize appropriate indexing, fine-tune query execution plans, and analyze query performance to minimize any bottlenecks in your application.
FAQs
Q1. Can I use whereNotNull with multiple columns simultaneously?
A1. Yes, you can use whereNotNull with multiple columns by chaining multiple whereNotNull methods together or using the where(function ($query){…}) syntax.
Q2. What happens if I use whereNotNull on a column that contains null values?
A2. If you use whereNotNull on a column that contains null values, those records will be excluded from the query result.
Q3. Is whereNotNull case-sensitive?
A3. No, whereNotNull is not case-sensitive and can be used on any column regardless of its case sensitivity settings.
Q4. Can I perform whereNotNull validation on nested relationships?
A4. Yes, whereNotNull can be used on nested relationships by specifying the relationship path in the query.
Conclusion
whereNotNull Laravel empowers developers to effectively validate and filter data in their applications. By leveraging this method, you can ensure data integrity, improve application performance, and maintain cleaner code. Remember to follow best practices, combine with other validation methods, and optimize query performance to maximize the benefits of whereNotNull. With its simplicity and effectiveness, whereNotNull Laravel is an invaluable tool that contributes to the development of robust and reliable Laravel applications.
Where Exists Laravel
Laravel, an open-source PHP framework, has gained immense popularity among developers due to its simplicity, scalability, and robust features. It offers an elegant syntax that allows developers to create web applications effortlessly. Laravel works on the Model-View-Controller (MVC) architectural pattern, making it easier to separate the logic and presentation layers of an application.
Laravel finds its existence in various aspects of web development, including:
1. Web Applications:
Laravel is primarily used for building web applications. Its powerful routing system enables developers to define the URLs and handle HTTP requests with ease. Laravel’s Blade templating engine simplifies the process of creating dynamic views, while its ORM (Object-Relational Mapping) called Eloquent facilitates database interaction in an intuitive way.
Moreover, Laravel’s authentication and authorization mechanisms provide developers with ready-to-use features for user management, login, and access control. Its support for user-friendly URLs, form handling, and file uploading further enhances its capabilities for building web applications.
2. RESTful APIs:
Laravel is a great choice for building robust RESTful APIs. It provides features like middleware, request handling, and JSON responses, making it simple to develop APIs that can be consumed by both web and mobile applications. Laravel’s authentication system can be easily integrated with APIs to ensure secure access.
3. Content Management Systems (CMS):
Laravel has become a popular choice for developing content management systems. With Laravel as the underlying framework, developers can create custom CMS solutions tailored to the unique needs of their clients. Laravel’s modular structure, combined with its extensive ecosystem of packages, allows developers to add features and functionality to their CMS projects effortlessly.
4. E-commerce Platforms:
Laravel’s capabilities extend to the creation of sophisticated e-commerce platforms. Its elegant syntax, coupled with its powerful database manipulation features, enables developers to build robust shopping cart systems. Laravel’s integration with popular payment gateways simplifies the process of handling transactions securely, ensuring a seamless shopping experience for customers.
5. Enterprise Applications:
Laravel has found its way into the development of enterprise-level applications. Its scalability and modular structure make it suitable for handling large and complex projects. Laravel’s built-in support for caching, queueing, and task scheduling enables developers to build high-performance applications that can handle heavy traffic.
6. Prototyping and MVPs:
Laravel proves to be an excellent choice for prototyping and developing Minimum Viable Products (MVPs). Its ease of use, combined with its extensive feature set, allows developers to rapidly build functional prototypes with minimal effort. Laravel’s community-driven ecosystem also provides access to various packages that can expedite the development process.
FAQs:
Q: Is Laravel suitable for beginners in web development?
A: Yes, Laravel is beginner-friendly and offers extensive documentation and resources to support newcomers. Its clean syntax and intuitive features make it easy to grasp and understand.
Q: Can Laravel handle large-scale applications?
A: Yes, Laravel is well-suited for large-scale applications. Its modular structure, support for caching, and ability to integrate with other tools make it suitable for applications handling high traffic and complex workflows.
Q: Is Laravel a secure framework?
A: Laravel takes security seriously and provides measures to protect against common web threats, including cross-site scripting (XSS) and SQL injection attacks. However, as with any framework, developers need to follow best practices to ensure the security of their applications.
Q: Does Laravel have an active community?
A: Yes, Laravel has a vibrant and active community of developers. The Laravel community provides support through forums, official documentation, packages, and numerous online resources.
Q: What is the future of Laravel?
A: Laravel continues to evolve with each update, introducing new features and improvements. With its growing popularity and strong community support, Laravel is expected to maintain its position as one of the leading PHP frameworks in the future.
In conclusion, Laravel exists in various domains of web development, making it a versatile and powerful framework. Its simplicity, scalability, and extensive feature set make it a preferred choice for developers building web applications, APIs, content management systems, e-commerce platforms, enterprise applications, and prototypes. With a vibrant community and a promising future, Laravel remains at the forefront of modern PHP frameworks.
Images related to the topic laravel query builder exists
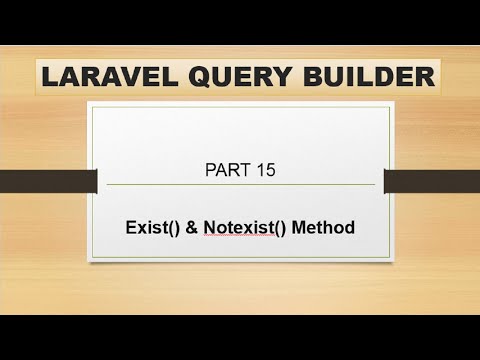
Found 25 images related to laravel query builder exists theme
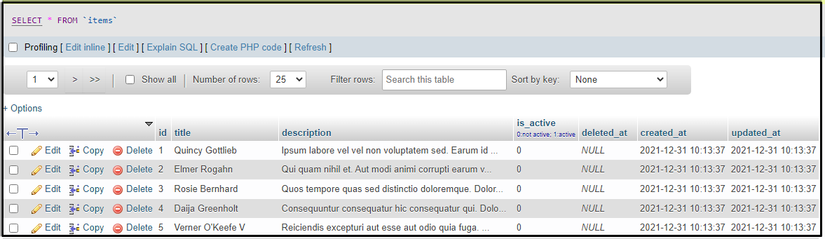
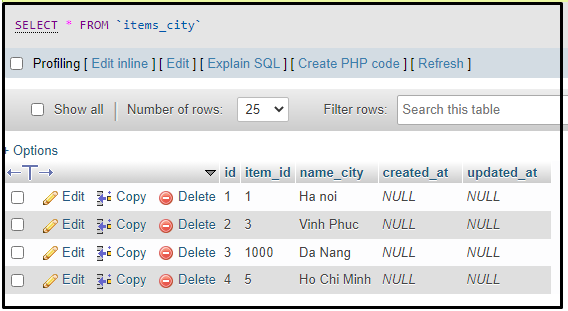
![php - Property [X] does not exist on the Eloquent builder instance in laravel 9 - Stack Overflow Php - Property [X] Does Not Exist On The Eloquent Builder Instance In Laravel 9 - Stack Overflow](https://i.stack.imgur.com/JzaXh.png)
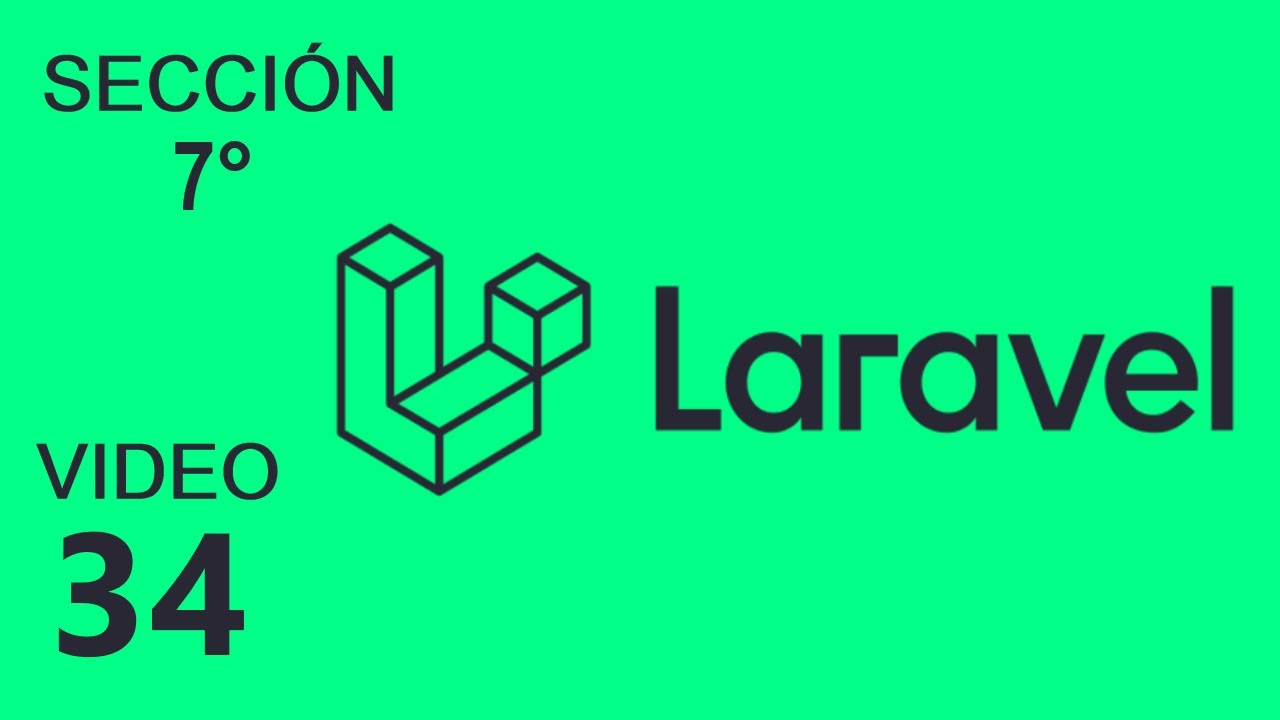
![php - Property [X] does not exist on the Eloquent builder instance in laravel 9 - Stack Overflow Php - Property [X] Does Not Exist On The Eloquent Builder Instance In Laravel 9 - Stack Overflow](https://i.stack.imgur.com/9SkQS.png)
![Laravel 10 Fundamental [Part 86] - Query Builder - Using exists() method - YouTube Laravel 10 Fundamental [Part 86] - Query Builder - Using Exists() Method - Youtube](https://i.ytimg.com/vi/T79USFMPbwg/maxresdefault.jpg)

![SQLSTATE[42S02]: Base table or view not found: 1146 Table 'wkaranjarealtorsdb.categories' doesn't exist on php artisan migrate Sqlstate[42S02]: Base Table Or View Not Found: 1146 Table 'Wkaranjarealtorsdb.Categories' Doesn'T Exist On Php Artisan Migrate](https://i.stack.imgur.com/XNOzk.png)
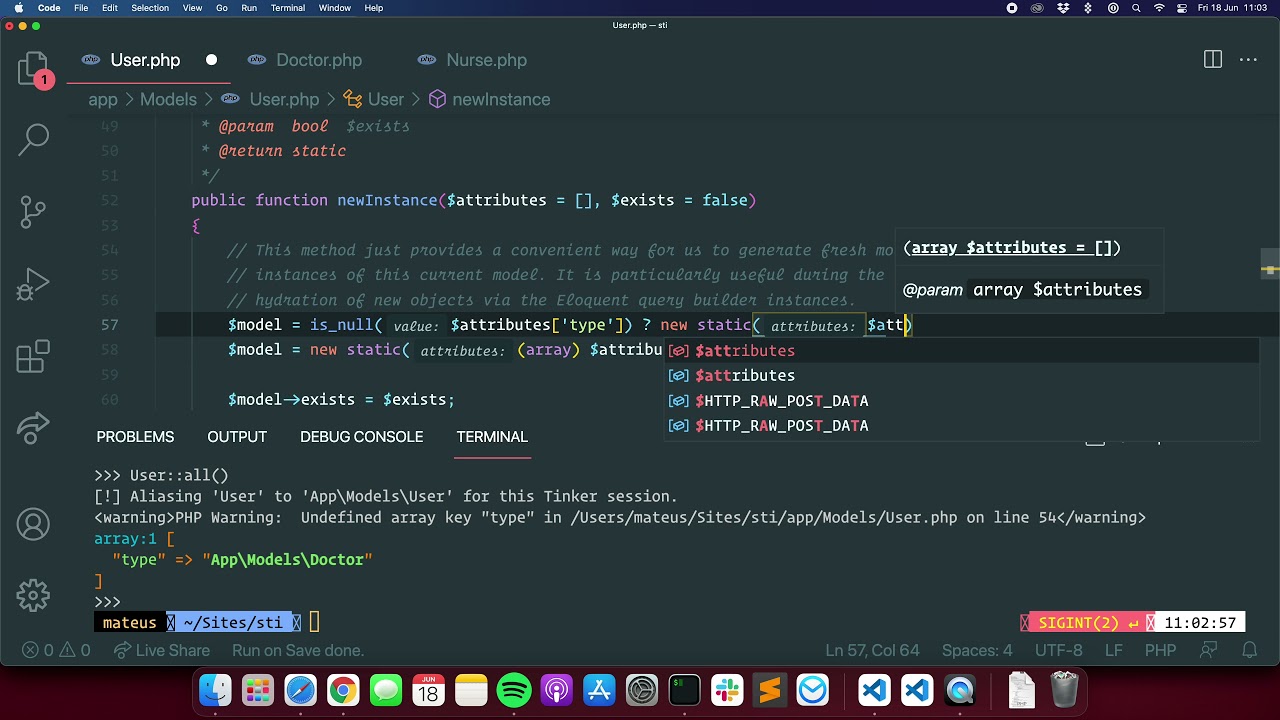
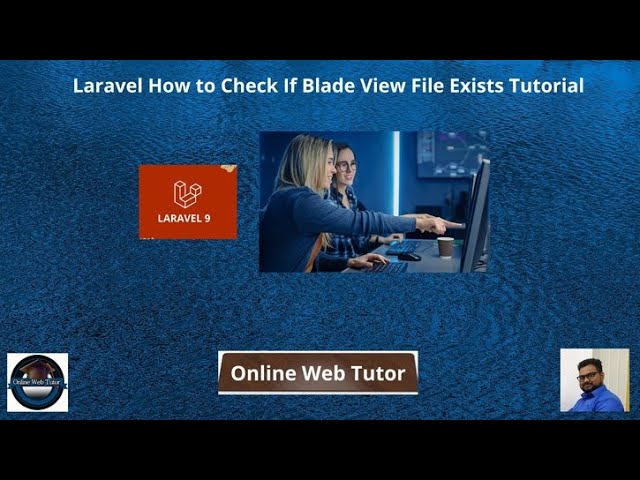
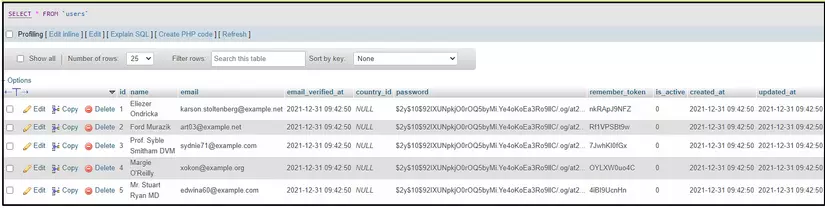
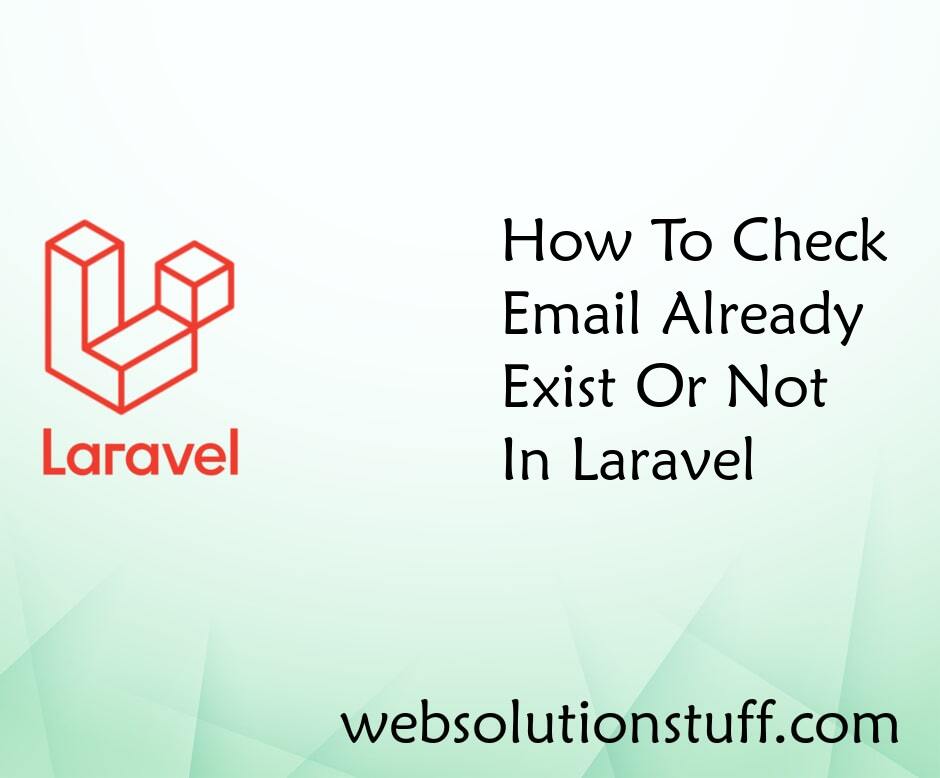
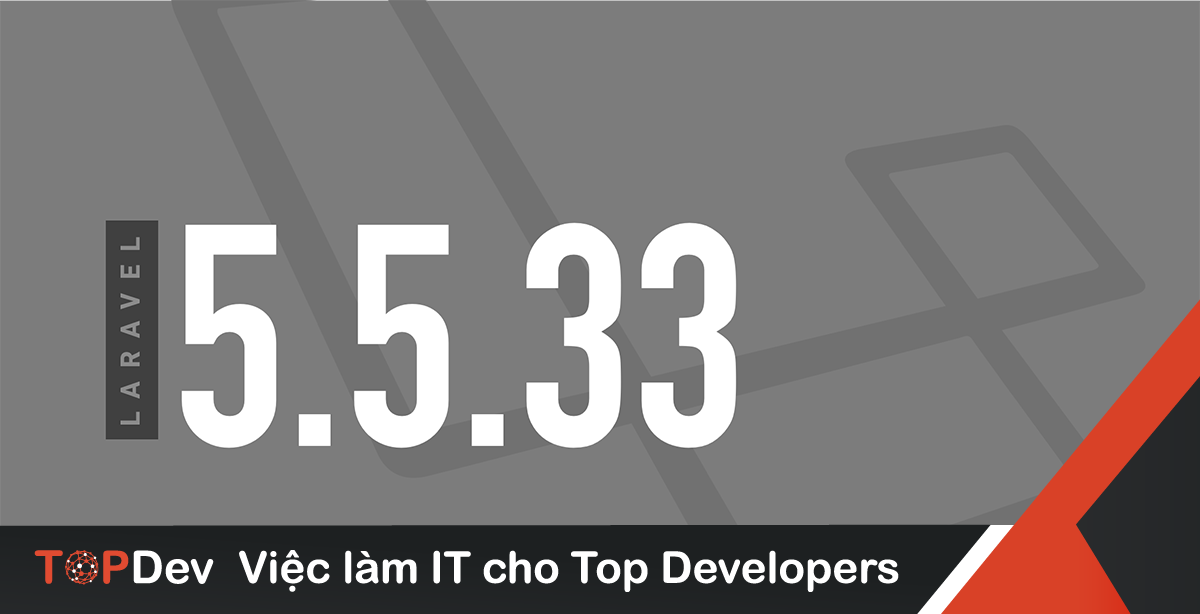
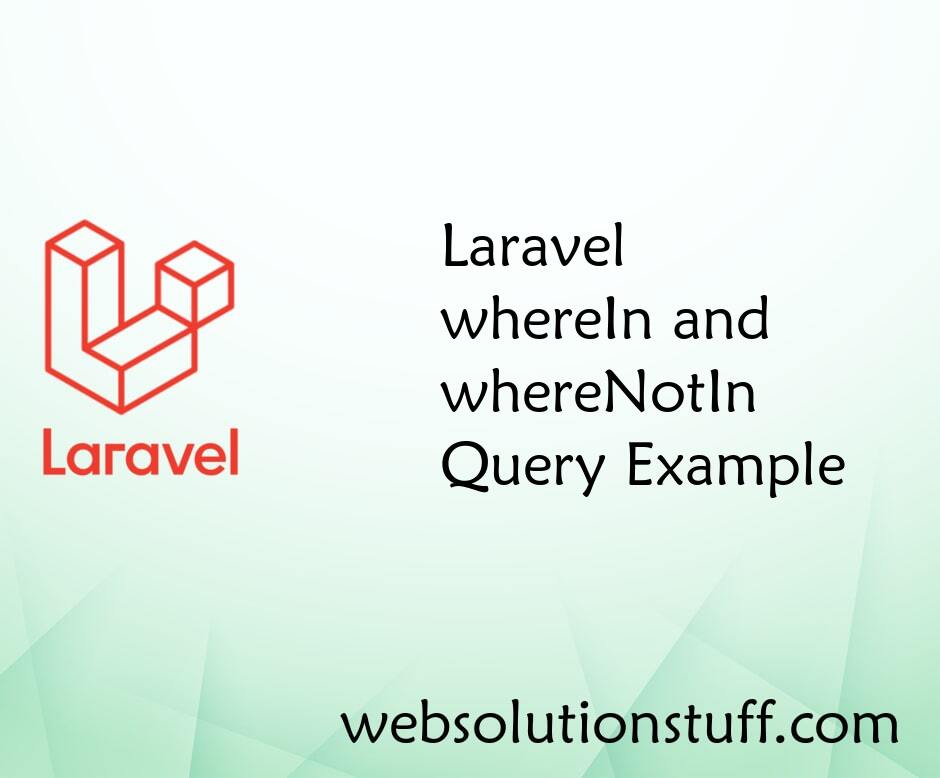
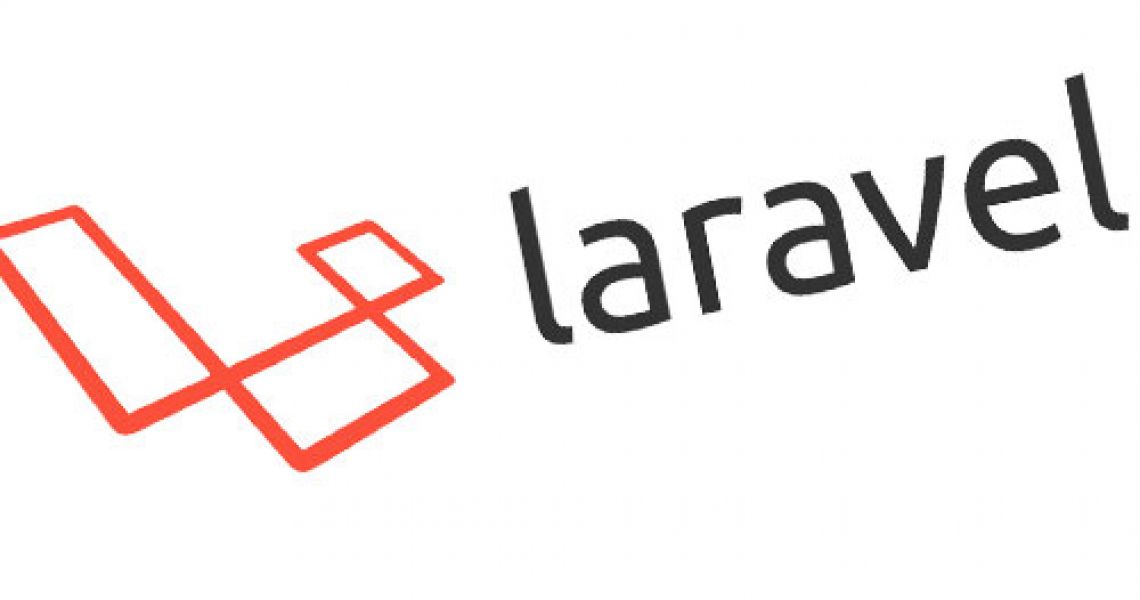
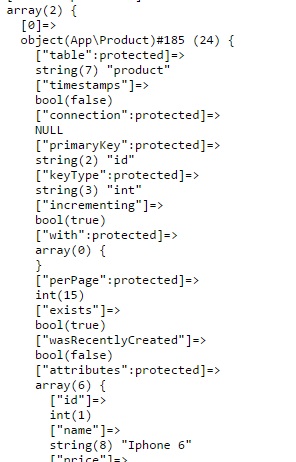
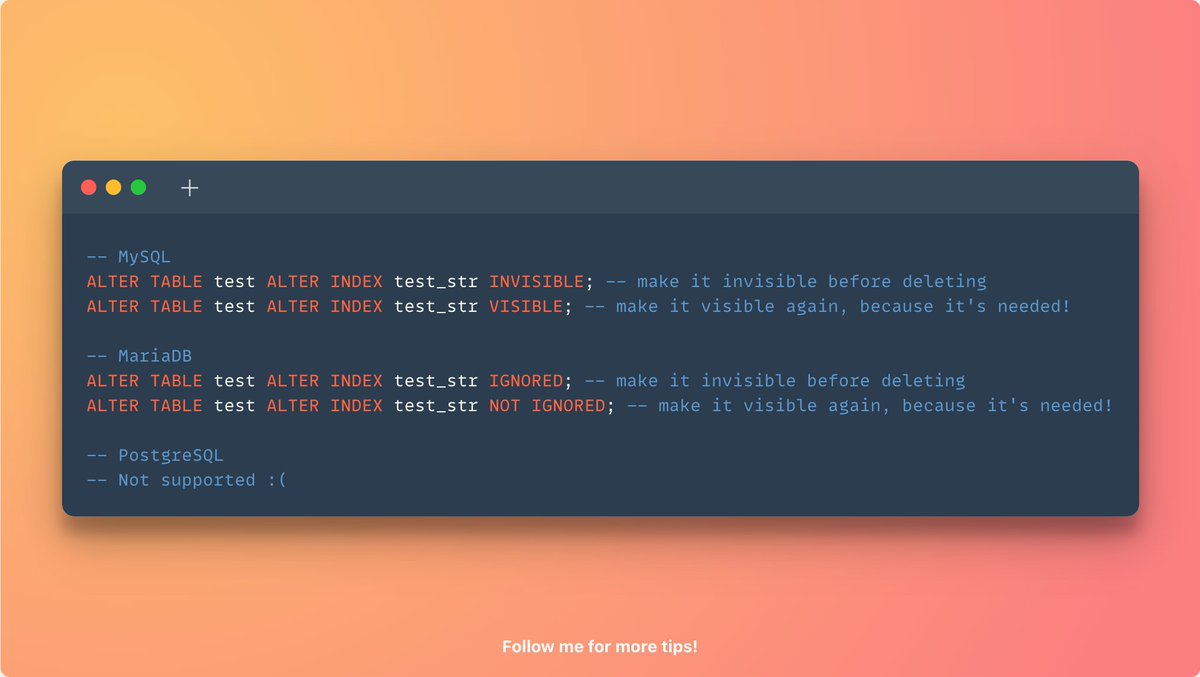
;-(4)-front_list.jpg)

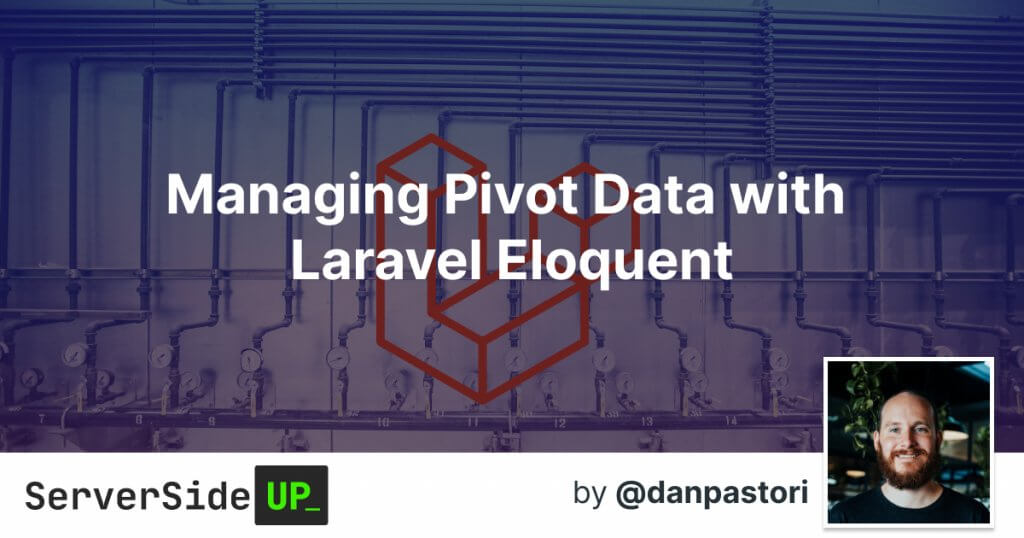
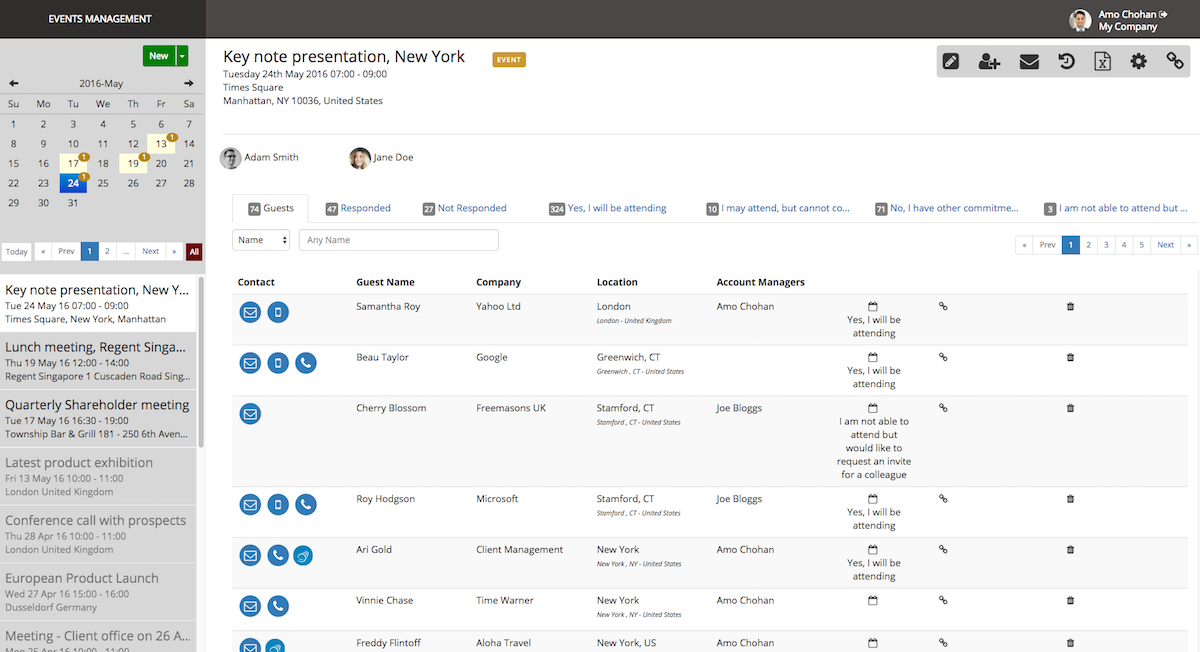
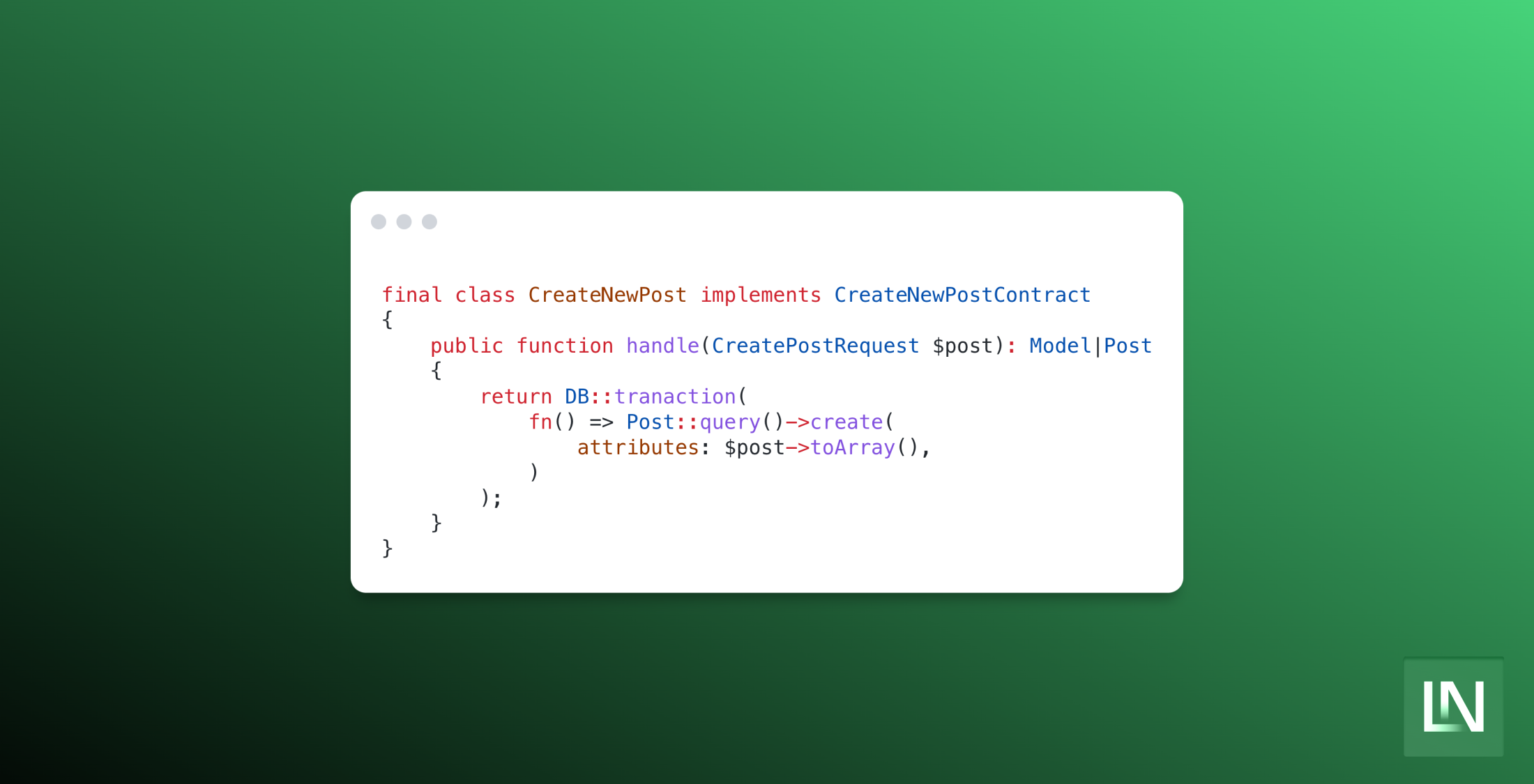
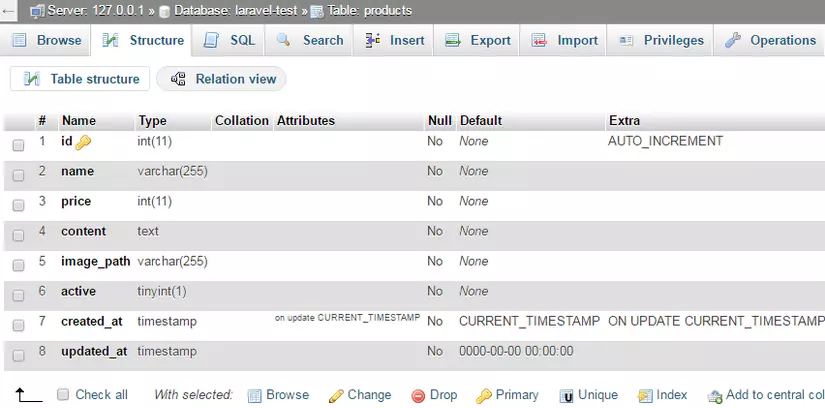
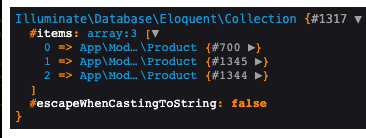
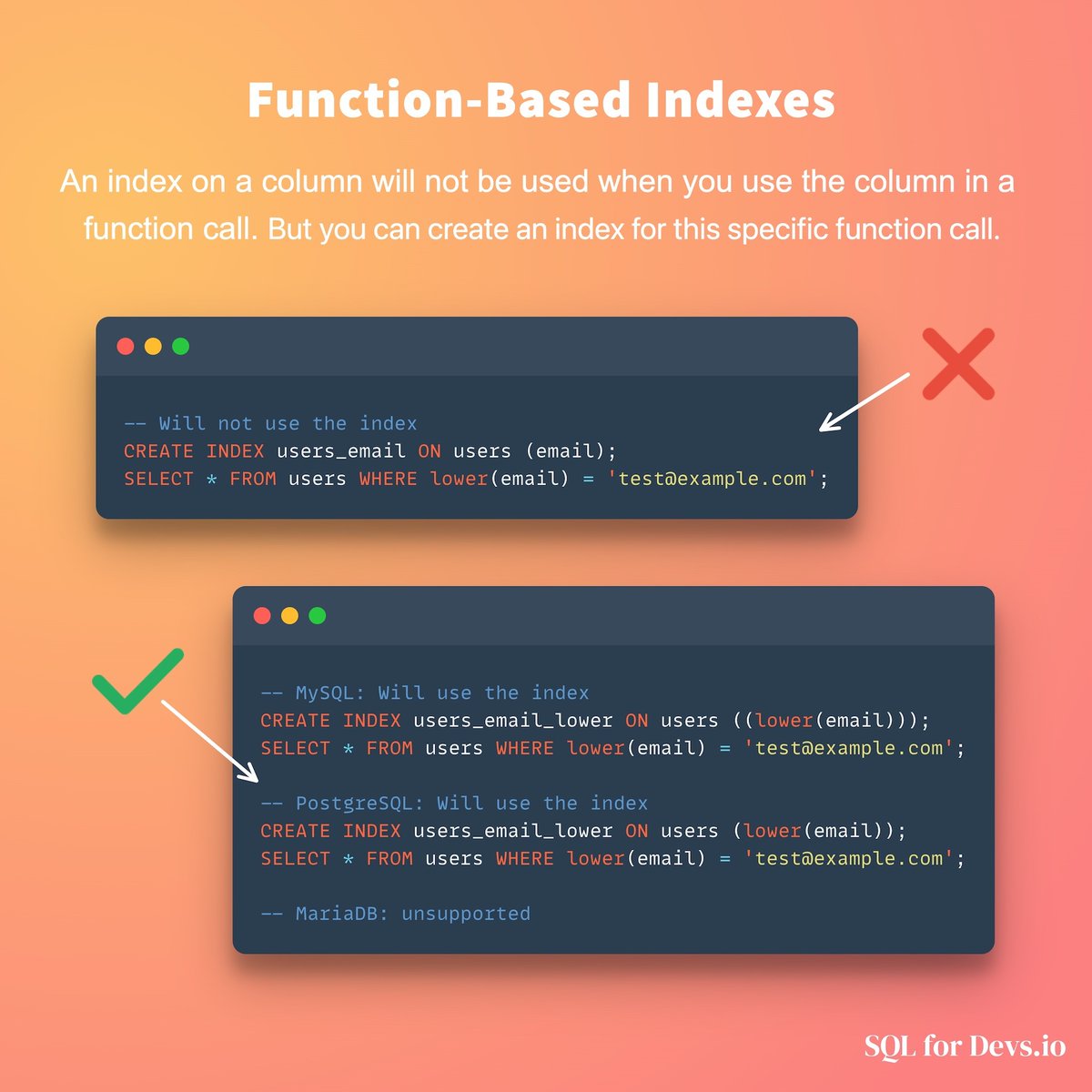
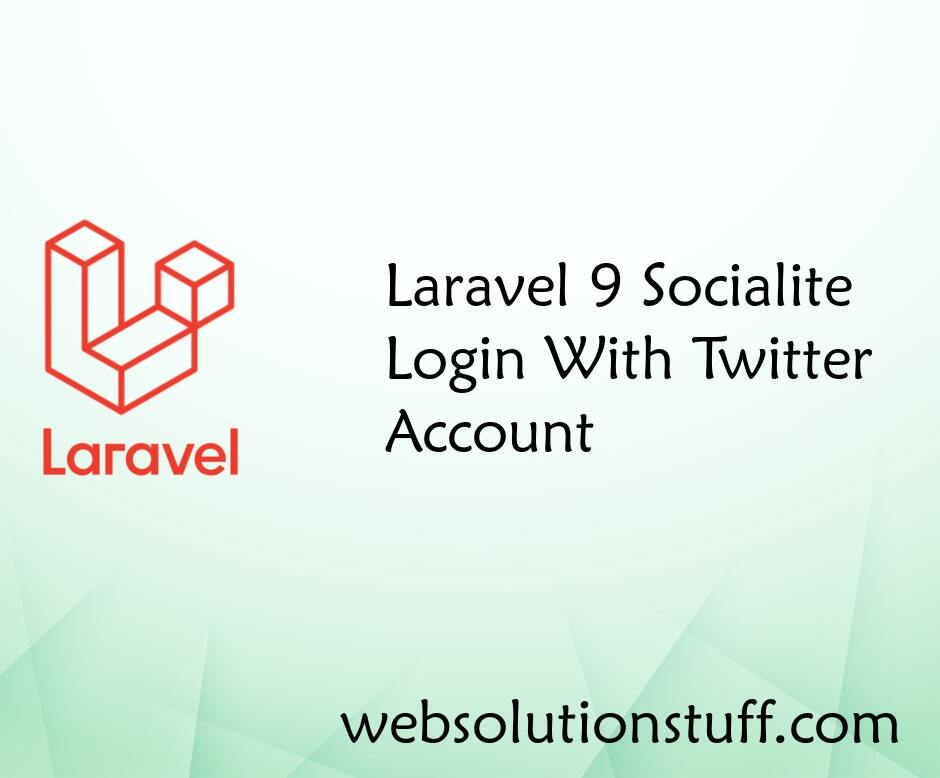
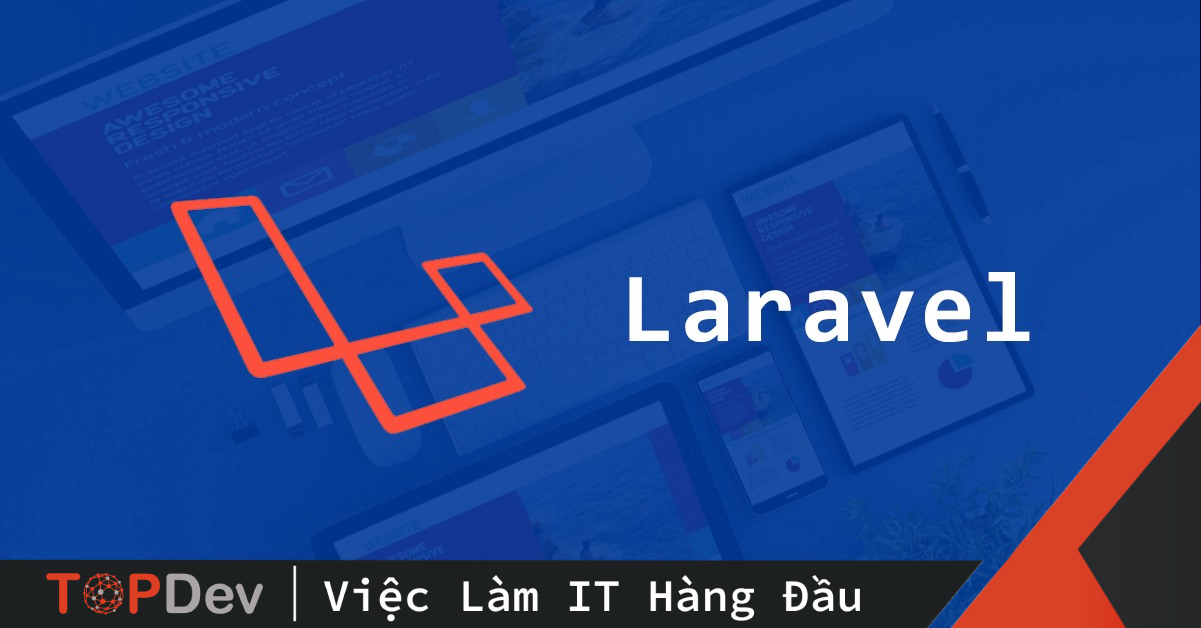
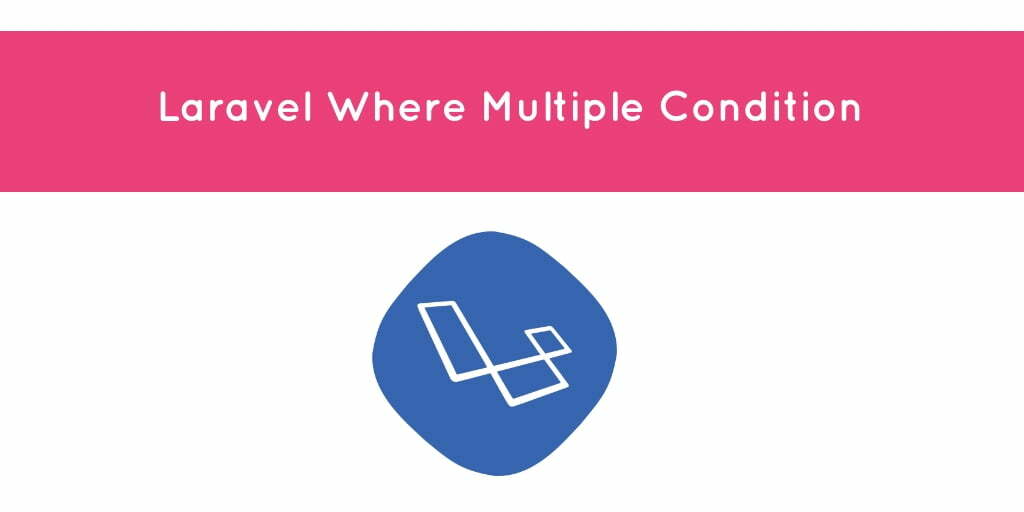
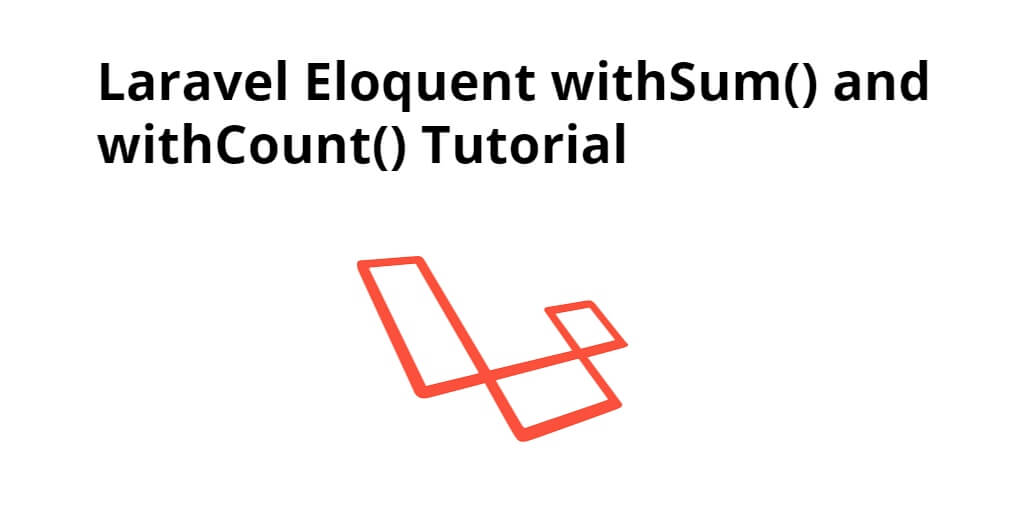
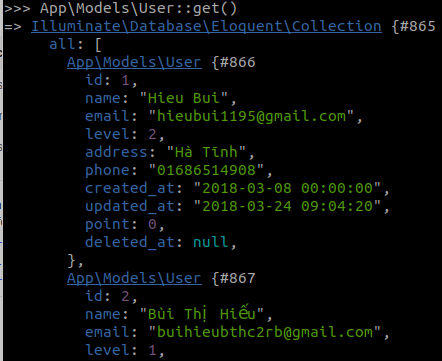
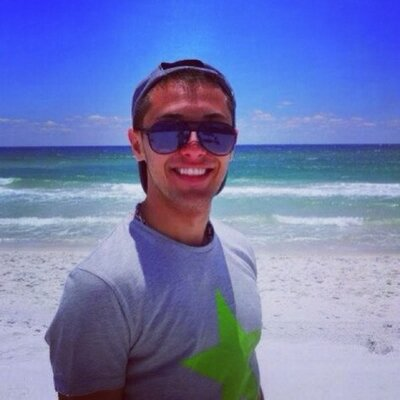

Article link: laravel query builder exists.
Learn more about the topic laravel query builder exists.
- Query Builder – Laravel – The PHP Framework For Web Artisans
- Running “exists” queries in Laravel query builder
- Sử dụng Query Builder Where Exists trong Laravel – Viblo
- Laravel Query Builder Where Exists Example
- How to determine if a record exists in the database in Laravel
- Laravel Query Builder Where Exists Example
- Laravel Query Builder Where Exists Example – Raviya Technical
- How to determine if a record exists in the database in Laravel
- Query Builder – Laravel – The PHP Framework For Web Artisans
- How to check if a record already exists in a laravel | Edureka Community
- How To Use The Laravel Query Builder – vegibit
See more: nhanvietluanvan.com/luat-hoc