Json To One Line
What is JSON?
JSON is a format for representing structured data in a human-readable way. It was derived from JavaScript but is language-independent and can be used with any programming language. JSON is commonly used for transmitting data between a server and a web application, as an alternative to XML.
JSON Syntax:
JSON uses a simple and intuitive syntax for representing data. The data is structured using key-value pairs and can be organized into objects and arrays. Here is an example of JSON syntax:
“`
{
“name”: “John”,
“age”: 30,
“city”: “New York”
}
“`
In the above example, we have an object with three key-value pairs: “name”, “age”, and “city”. The keys are strings, and the values can be strings, numbers, booleans, null, objects, or arrays.
JSON Data Types:
JSON supports several data types:
1. String: A sequence of Unicode characters, enclosed in double quotes.
2. Number: An integer or a floating-point number.
3. Boolean: Either true or false.
4. Null: Represents a null value.
5. Object: An unordered collection of key-value pairs.
6. Array: An ordered list of values.
JSON Objects:
JSON objects are enclosed in curly braces {}. Inside the braces, we define the key-value pairs separated by a colon (:). The keys must be strings, and the values can be any valid JSON data type. Here is an example of a JSON object:
“`
{
“name”: “John”,
“age”: 30,
“city”: “New York”
}
“`
JSON Arrays:
JSON arrays are ordered lists of values, enclosed in square brackets []. The values can be of any JSON data type, including objects and arrays. Here is an example of a JSON array:
“`
[
“Apple”,
“Banana”,
“Orange”
]
“`
JSON Values:
JSON values can be of different types, as mentioned earlier. They can be strings, numbers, booleans, null, objects, or arrays. Here are some examples of JSON values:
“`
“John” (String)
30 (Number)
true (Boolean)
null (Null)
{
“name”: “John”,
“age”: 30
} (Object)
[
“Apple”,
“Banana”,
“Orange”
] (Array)
“`
Accessing JSON Data:
To access data in a JSON object or array, we use dot notation or square brackets. For example, to access the “name” property in the JSON object we mentioned earlier, we can use:
“`
jsonObject.name
“`
or
“`
jsonObject[“name”]
“`
Similarly, to access an element in a JSON array, we use the index inside square brackets. For example:
“`
jsonArray[0]
“`
Creating JSON Data:
To create JSON data in your application, you can use your programming language’s built-in functions or libraries. These functions allow you to define JSON objects and arrays programmatically. For example, in JavaScript, you can create JSON data using the `JSON.stringify()` function.
Parsing JSON Data:
To parse JSON data, i.e., convert a JSON string to an object or array in your programming language, you can use the built-in functions or libraries provided by your programming language. These functions allow you to extract and manipulate JSON data. In JavaScript, you can use the `JSON.parse()` function.
Manipulating JSON Data:
Once you have parsed JSON data into your programming language’s objects or arrays, you can manipulate it using various programming techniques. You can add, delete, or modify elements, perform calculations, filter data, and more.
FAQs:
Q: How can I convert JSON from paragraph to one line?
A: To convert JSON from a multiline format to one line, you can use various online tools or text editors that support find-and-replace operations. Simply remove the line breaks and indentation to convert JSON into a single line.
Q: How can I convert JSON from one line to multiline?
A: To convert JSON from one line to multiline, you can use online tools or text editors that support find-and-replace operations. Insert line breaks and add indentation to make the JSON string more readable.
Q: Is there an online tool to convert JSON to a string?
A: Yes, there are several online tools available that can convert JSON to a string format. You can search for “JSON to string online tool” and choose one that suits your requirements.
Q: How can I format my code to one line?
A: To format your code to one line, you can use code formatter tools or online services that can automatically remove line breaks and indentation.
Q: How can I convert multiline JSON to one line in Notepad++?
A: In Notepad++, you can use the “Replace” function to convert multiline JSON to one line. Use the regular expression mode and replace all line breaks with a space or an empty string.
Q: Are there any JSON viewer plugins for Notepad++?
A: Yes, there are JSON viewer plugins available for Notepad++. These plugins help you visualize and navigate JSON data in a more organized and readable format.
Q: Can Jq output JSON in a single line?
A: Yes, Jq is a command-line JSON processor that can be used to process and manipulate JSON data. It has options to output JSON in a single line or pretty-printed format.
Q: How can I convert JSON to one line using Jq?
A: To convert JSON to one line using Jq, you can use the `-c` or `–compact-output` option. This option ensures that the output is in a compact, single-line format.
In conclusion, JSON is a versatile and widely used format for representing structured data. It offers a simple and intuitive syntax and supports various data types, objects, and arrays. Understanding how to access, create, parse, and manipulate JSON data is essential for any developer working with data interchange. Additionally, knowing techniques for converting JSON between different formats, such as from one line to multiline, can be helpful in various scenarios. With the help of online tools, text editors, or specialized libraries like Jq, developers can easily manipulate and transform JSON data to meet their specific requirements.
How To Parse Json (One Line) (Swift 4 + Xcode 9.0)
How To Convert Json To A Single Line?
JSON (JavaScript Object Notation) is a widely used data interchange format that is known for its simplicity and ease of parsing. It has become the de facto standard for data communication between applications, making it essential to understand how to convert JSON to a single line. By doing so, you can compact complex JSON structures and make them more readable, efficient, and easier to process. In this article, we will explore different methods to achieve this conversion and address common questions surrounding this topic.
Methods to Convert JSON to a Single Line
There are multiple approaches you can take to convert JSON to a single line. Let’s discuss some popular methods:
1. Using Programming Languages:
The most common way to convert JSON to a single line is by using programming languages such as Python, JavaScript, or PHP. These languages provide built-in libraries or functions that allow you to load JSON data, manipulate it, and save it as a single-line string.
For instance, in Python, the `json` library provides a `dumps` function that allows you to serialize JSON data to a string. By passing the `separators` parameter as (`’,’, ‘:’`), you can remove whitespace and newlines, effectively converting the JSON to a single line.
2. Online JSON Tools:
If you prefer a simple and quick way to convert JSON to a single line without writing code, many online JSON tools are available. These tools usually offer features like formatting, validation, and conversion. By selecting the appropriate option, you can easily convert your JSON to a single line.
3. Using Command-Line Tools:
Command-line tools are another popular method to convert JSON to a single line, especially for those comfortable with working in a terminal environment. Tools like `jq` (a command-line JSON processor) provide various options and flags to manipulate and reformat JSON data. By applying specific filters and configurations, you can transform JSON into a single-line output.
FAQs
Q1. Why would I want to convert JSON to a single line?
A1. Converting JSON to a single line offers several benefits. It simplifies the structure, making it easier to read and understand. It also reduces the size of the JSON, resulting in improved efficiency during data transmission and storage. Additionally, a single-line JSON format is often required for compatibility with certain systems or APIs.
Q2. Does converting JSON to a single line affect the data itself?
A2. No, converting JSON to a single line doesn’t modify the data content. It only changes the format by removing whitespace and newlines. All the data elements and their values remain the same.
Q3. What if my JSON contains nested structures?
A3. JSON can have nested structures, including arrays and objects. When converting to a single line, these nested structures are preserved. Each element will be placed on the same line, removing only the whitespace and newlines.
Q4. Can I convert a single-line JSON back to the original format?
A4. Yes, you can convert a single-line JSON back to its original format. For example, in Python, the `json` library’s `loads` function can deserialize the single-line string back into a JSON object or array.
Q5. Are there any implications of converting JSON to a single line in terms of performance?
A5. Converting JSON to a single line does not have significant performance implications. However, processing a single-line JSON may be slightly faster due to the reduced input size and easier parsing.
In conclusion, converting JSON to a single line is a pragmatic approach to simplify data formatting. Whether you prefer using programming languages, online tools, or command-line utilities, making your JSON more compact can enhance readability and efficiency. By adhering to the techniques discussed in this article, you’ll be able to optimize your JSON data for a wide range of applications and systems.
How To Convert Json To Single Line In Java?
JSON (JavaScript Object Notation) is a commonly used data format for storing and exchanging structured data between a server and a client. It is easy to read and write for humans, as well as easy to parse and generate for machines. In certain cases, it becomes necessary to convert JSON to a single line format, which is useful for various purposes like storing JSON in a single line in a text file or transmitting it over a network. This article will explore different approaches to convert JSON to a single line in Java, providing step-by-step explanations and code examples.
Approach 1: Using JSON.simple Library
The JSON.simple library is a lightweight JSON processing library that provides a simple API for manipulating JSON data. To convert JSON to a single line format using this library, follow these steps:
Step 1: Add the JSON.simple dependency to your project. You can download the JAR file from the official website or use a build tool like Maven or Gradle.
Step 2: Import the required classes from the JSON.simple library in your Java code.
“`
import org.json.simple.*;
“`
Step 3: Parse the JSON string using the JSONParser class from the JSON.simple library.
“`
String jsonString = “{\”name\”:\”John\”, \”age\”:30, \”city\”:\”New York\”}”;
JSONParser parser = new JSONParser();
Object jsonObj = parser.parse(jsonString);
“`
Step 4: Convert the parsed JSON object to a single line format using the JSONValue.toJSONString() method.
“`
String singleLineJson = JSONValue.toJSONString(jsonObj);
System.out.println(singleLineJson);
“`
Approach 2: Using Jackson Library
Jackson is a high-performance JSON processor library for Java that provides various features for manipulating JSON data. To convert JSON to a single line format using the Jackson library, follow these steps:
Step 1: Add the Jackson dependency to your project. You can download the JAR files from the official website or use a build tool like Maven or Gradle.
Step 2: Import the required classes from the Jackson library in your Java code.
“`
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
“`
Step 3: Parse the JSON string using the ObjectMapper class from the Jackson library.
“`
String jsonString = “{\”name\”:\”John\”, \”age\”:30, \”city\”:\”New York\”}”;
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
“`
Step 4: Convert the parsed JSON object to a single line format using the toString() method and removing line breaks and whitespaces.
“`
String singleLineJson = jsonNode.toString().replaceAll(“\n”, “”).replaceAll(” “, “”);
System.out.println(singleLineJson);
“`
Approach 3: Using Gson Library
Gson is a powerful JSON library for Java that provides various methods for converting Java objects to JSON and vice versa. To convert JSON to a single line format using the Gson library, follow these steps:
Step 1: Add the Gson dependency to your project. You can download the JAR files from the official website or use a build tool like Maven or Gradle.
Step 2: Import the required classes from the Gson library in your Java code.
“`
import com.google.gson.*;
“`
Step 3: Parse the JSON string using the JsonParser class from the Gson library.
“`
String jsonString = “{\”name\”:\”John\”, \”age\”:30, \”city\”:\”New York\”}”;
JsonParser parser = new JsonParser();
JsonElement jsonElement = parser.parse(jsonString);
“`
Step 4: Convert the parsed JSON object to a single line format using the toString() method and removing line breaks.
“`
String singleLineJson = jsonElement.toString().replaceAll(“\n”, “”);
System.out.println(singleLineJson);
“`
FAQs:
Q1. Can I use any of these approaches to convert a JSON file to a single line format?
Yes, these approaches can be used to convert both JSON strings and JSON files to the single line format. For JSON files, you just need to read the file content and pass it as a string to the respective library’s parser.
Q2. What if my JSON contains nested objects or arrays?
The provided approaches can handle JSON objects and arrays with nested structures. They will recursively convert the entire JSON structure to the single line format.
Q3. How can I pretty print the JSON again?
If you need to revert back to the original JSON format with indentation, you can use the respective library’s methods for pretty printing. For example, in Gson, you can use GsonBuilder with setPrettyPrinting() to generate indented JSON.
Q4. Are there any limitations or performance considerations when converting to a single line format?
Converting JSON to a single line format removes unnecessary line breaks and whitespaces, making the JSON more compact. However, it may make it harder for humans to read and understand, especially for large and complex JSON structures. Performance considerations depend on the size and complexity of the JSON, but in general, these libraries are designed to be efficient.
In conclusion, converting JSON to a single line format can be achieved using different Java libraries, including JSON.simple, Jackson, and Gson. These libraries provide easy-to-use methods for parsing and manipulating JSON data. By following the steps outlined in this article, you can convert JSON strings or JSON files to the desired single line format, and also handle nested objects and arrays.
Keywords searched by users: json to one line Paragraph to one line, JSON one line to Multiline, JSON to string online, Format code to one line, Multiline to one line, Convert text to one line notepad++, JSON Viewer Notepad++, Jq output single line
Categories: Top 31 Json To One Line
See more here: nhanvietluanvan.com
Paragraph To One Line
In the world of written communication, brevity is the golden standard. Whether crafting essays, articles, or even social media posts, conveying ideas succinctly has become a sought-after skill. One way to achieve this is through the transformation of a well-developed paragraph into a single powerful sentence that encapsulates the essence of the message. This innovative technique has gained popularity across the globe for its ability to condense lengthy passages into easily digestible snippets. In this article, we will explore the concept of “Paragraph to One Line,” its evolution, and its impact on the way we communicate.
The idea of transforming an entire paragraph into a solitary sentence might seem daunting at first. However, the aim is not to truncate or dilute the meaning of the original text but to distill its core essence. By focusing on the main points and eliminating unnecessary details, this method enables writers to convey crucial information effectively.
This technique is often employed in headlines, taglines, and social media posts, where space and attention are limited. In these fast-paced mediums, a well-constructed one-liner has the power to capture the reader’s attention instantly. It cuts through the noise and gets straight to the heart of the matter, leaving a lasting impact.
The evolution of the paragraph to one line concept can be traced back to the era of traditional print media. Newspaper headlines, for example, have been using this approach for decades, employing catchy phrases to entice readers in an era of information overload. These concise statements were meant to summarize the story and lure potential readers into delving deeper.
With the rise of online content and social media, the need for an eye-catching one-liner has become even more prevalent. The explosive growth of platforms like Twitter, with its character limitations, has challenged writers to be even more concise in expressing their messages. Consequently, the influence of paragraph to one line has expanded beyond journalism and advertising, finding a place in everyday communication.
So, what makes a powerful one-liner? To begin, clarity is paramount. A sentence that captures the essence of the paragraph while remaining easily understandable is crucial. Moreover, brevity is key; the shorter and more succinct the sentence, the greater its impact. Additionally, incorporating emotion or a compelling hook can engage the reader and make the message more memorable.
FAQs:
Q: Is transforming a paragraph into one line suitable for all types of writing?
A: While the paragraph to one line technique is versatile, it may not be suitable for all types of writing. Lengthier and more in-depth pieces, such as academic essays or scientific research, require a more comprehensive approach in order to convey complex information accurately.
Q: How can one ensure that the original meaning of the paragraph is preserved?
A: When transforming a paragraph into one line, it is crucial to capture the essence and main points, avoiding any potential ambiguity. This can be achieved by focusing on the central idea and eliminating unnecessary supporting details.
Q: Are there any disadvantages to using one-liners?
A: While the efficiency and impact of one-liners are undeniable, there is a risk of oversimplification or loss of context. It is important to strike a balance between brevity and clarity, ensuring that the meaning is not diluted or misconstrued.
Q: Can the paragraph to one line technique be beneficial in personal writing, such as emails or letters?
A: Absolutely. Whether it be for emails, social media posts, or personal correspondence, succinctly presenting your point can enhance clarity and engage the recipient. However, it is vital to maintain a genuine and considerate tone, as overly concise messages may come across as curt or impersonal.
In an era where attention spans are dwindling, the art of transforming a paragraph into one impactful line has become a valuable skill. From newspapers to social media feeds, the ability to convey essential information efficiently has become essential. By embracing this technique, writers can captivate readers, foster engagement, and leave a lasting impression. So, the next time you find yourself crafting a piece of writing, consider the power of Paragraph to One Line and witness the evolution of your communication style.
Json One Line To Multiline
1. Understanding JSON Structures:
Before we explore the conversion process, it is crucial to understand the structure of JSON. JSON consists of key-value pairs, where the key is always a string and the value can be a string, number, boolean, null, array, or another JSON object. The structure is denoted by curly braces {} for objects and square brackets [] for arrays. Additionally, JSON separates keys and values with a colon (:) and multiple key-value pairs with commas (,).
2. The Benefits of Multiline JSON:
Multiline JSON offers several advantages over its single-line counterpart. Firstly, it allows for better readability, making it easier to comprehend the data structure at a glance. When JSON is formatted across multiple lines, individual elements are more clearly separated, enhancing code legibility for developers. Additionally, multiline JSON also aids in error detection and debugging, as syntax or structural issues become more apparent. When working with large or complex JSON files, converting to multiline format can save time and effort, ensuring accurate interpretation and efficient modification of data.
3. Converting JSON from One Line to Multiline:
There are multiple approaches and tools available for converting JSON from a single line to multiline. One common method is to utilize an online JSON formatter or validator, which parses the JSON and formats it in a multiline layout. These tools typically retain the existing structure of the JSON while improving readability. Additionally, most modern integrated development environments (IDEs) provide plugins or built-in functions to convert JSON to multiline format.
4. Manual Conversion:
If you prefer a hands-on approach or have specific formatting requirements, you can convert JSON to multiline manually. Start by opening the JSON file in a text editor that supports search and replace functionality. Regular expressions can be used to aid in this process. For example, find all instances of “{“, “}”, or “,” and replace them with appropriate line breaks and indentation. Ensure that any string values within the JSON, which might contain comma or curly brace characters, are not incorrectly split during the conversion process.
5. Integrated Development Environment (IDE) Assistance:
Most modern IDEs provide features to format JSON automatically. For instance, in Visual Studio Code, you can use the “Format Document” command (usually triggered by Ctrl+Shift+I or selecting the appropriate option in the editor’s context menu) to instantly convert JSON to multiline format. Similarly, other IDEs, such as JetBrains’ IntelliJ IDEA or Eclipse, offer similar functionality. These built-in features make it convenient to convert JSON on the fly during development.
FAQs:
Q1. Will converting JSON to multiline format affect the functionality of the JSON file?
A1. No, the conversion only affects the formatting and does not alter the functionality of the JSON file. It improves readability and ease of use for developers.
Q2. Can I convert multiline JSON back to a single line?
A2. Yes, the conversion can be reversed by using the appropriate tools or IDE functions. This flexibility allows developers to switch between the two formats based on their preferences or project requirements.
Q3. Should I always convert JSON to multiline format?
A3. Converting to multiline format is particularly beneficial when dealing with large or complex JSON files. For smaller or simple JSON structures, the benefits might not be as significant. Consider the size and complexity of the JSON file before deciding whether to convert it.
Q4. Are there any performance implications of multiline JSON?
A4. No, converting JSON to multiline format does not affect its performance. JSON is parsed based on its structure, not the formatting. Once parsed, the execution and performance remain the same, regardless of whether it is presented in a single line or multiline format.
In conclusion, converting JSON from one line to multiline format greatly enhances readability and ease of use. It allows developers to quickly understand the data structure, locate errors and modify the content efficiently. Whether using online tools, manual conversion, or IDE assistance, the process can be tailored according to individual preferences and project requirements. With the ability to switch between these formats effortlessly, developers can adapt to different scenarios and make the most out of this versatile data interchange format.
Json To String Online
One of the key aspects of JSON is its ability to represent complex data structures in a simple, human-readable format. The basic building block of JSON is a key-value pair, where a key is a string and its value can be a string, number, boolean, array, or even another JSON object. This makes JSON ideal for representing structured data, such as the data returned by an API.
In order to use JSON data in a web application, it is often necessary to convert it to a string format. This can be done using a process called JSON serialization. JSON serialization is the process of converting a JSON object into a string representation that can be transmitted over a network or stored in a file. Conversely, when we want to use JSON data in our application, we have to deserialize the string representation back into a JSON object.
Fortunately, there are many online tools and libraries available that make the process of converting JSON to a string and vice versa seamless. These tools allow users to easily visualize JSON data in a readable format and perform conversions quickly and efficiently.
One popular online tool for converting JSON to a string format is JSON to string online. This tool provides a user-friendly interface where you can simply paste your JSON data and instantly get the string representation. It also allows you to customize the output by choosing different settings, such as indentation level and quoting style. JSON to string online is an excellent resource for developers and users who frequently work with JSON data.
To convert JSON to a string using JSON to string online, follow these simple steps:
1. Copy your JSON data to the clipboard.
2. Open the JSON to string online tool in your web browser.
3. Paste the JSON data into the input field.
4. Optionally, customize the output settings.
5. Click the “Convert” button.
6. The tool will instantly generate the string representation of your JSON data.
7. You can then copy the resulting string to use it in your application or further processing.
FAQs:
Q: What is the purpose of converting JSON to a string?
A: Converting JSON data to a string format is necessary when transmitting data over a network or storing it in a file. Many programming languages and APIs require the data to be in string format for proper handling.
Q: Can I convert a JSON string to JSON object using JSON to string online?
A: No, JSON to string online is focused on converting JSON objects to a string format. To convert a JSON string to a JSON object, you can use the built-in JSON.parse() function in JavaScript or similar functions in other programming languages.
Q: Are there any limitations to the size of JSON data that can be converted using JSON to string online?
A: JSON to string online tool may have limitations on the size of JSON data it can handle. Large JSON datasets may take longer to process, and extremely large datasets may not be supported due to memory or processing constraints.
Q: Is it possible to convert a string to JSON using JSON to string online?
A: No, JSON to string online is primarily focused on converting JSON objects to a string format. To convert a string to JSON, you can use the built-in JSON.parse() function in JavaScript or similar functions in other programming languages.
Q: Are there any security concerns when using online JSON conversion tools?
A: It’s important to use reputable and trusted online tools when dealing with sensitive data. Make sure to verify the authenticity and security of the website before pasting any confidential information. Additionally, avoid sharing sensitive data publicly or over untrusted networks.
In conclusion, JSON is a powerful and widely adopted data interchange format. Converting JSON to a string format is a common requirement when working with JSON data in web applications. Online tools like JSON to string online simplify the process and allow users to quickly convert JSON to a string and vice versa. These tools are invaluable for developers and users who frequently work with JSON data, providing convenience and efficiency in their workflows.
Images related to the topic json to one line
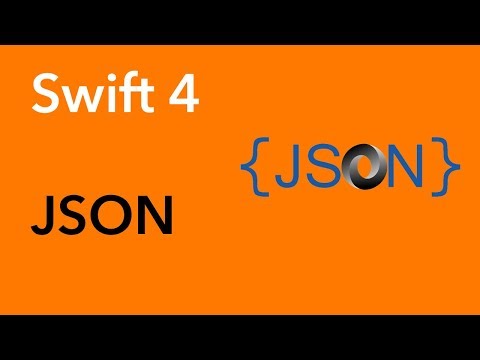
Found 18 images related to json to one line theme
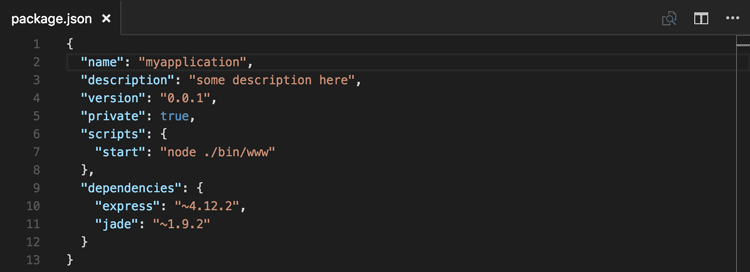

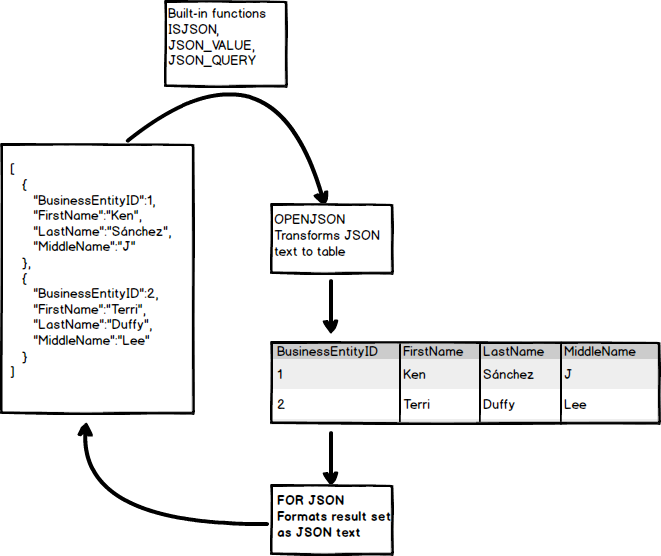
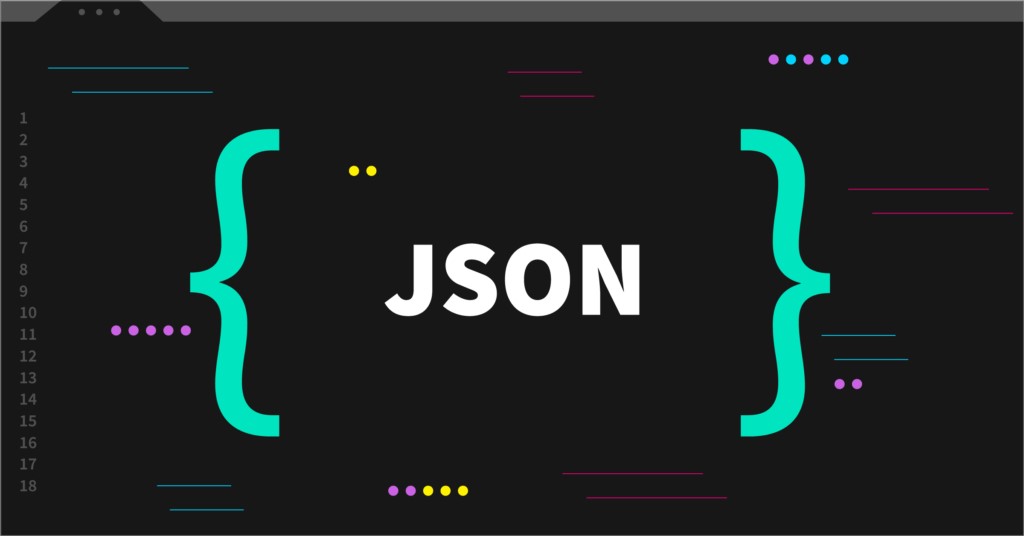
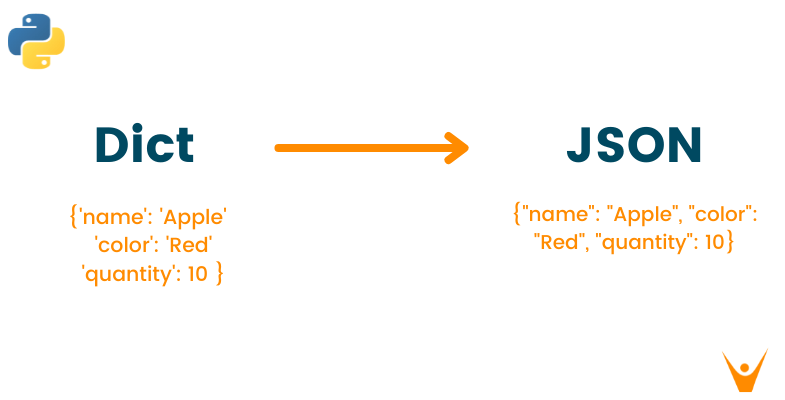
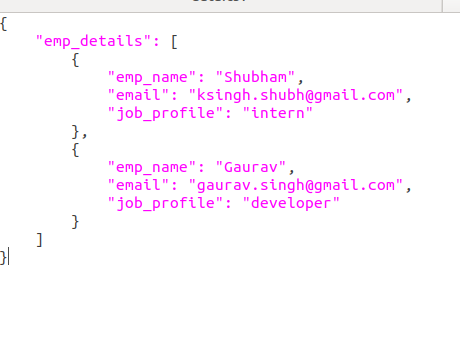


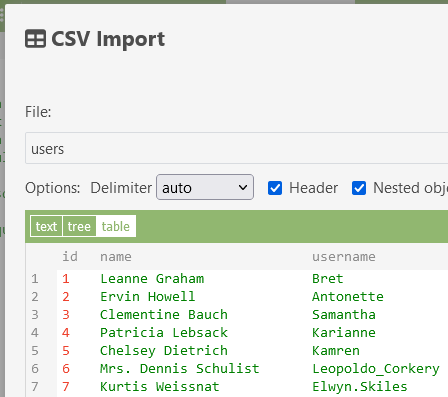
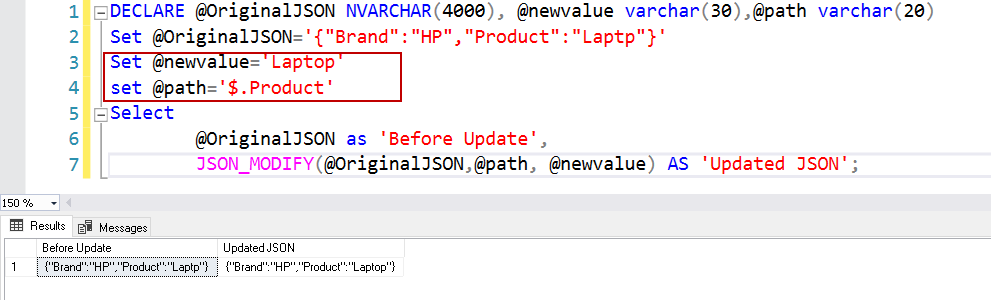

![Solved] How to arrange JSON data in an orderly manner - Questions about Thunkable - Community Solved] How To Arrange Json Data In An Orderly Manner - Questions About Thunkable - Community](https://global.discourse-cdn.com/business4/uploads/thunkable/original/3X/9/a/9ae32857b393a5ac952258d1d32e01a9a3e168c3.png)

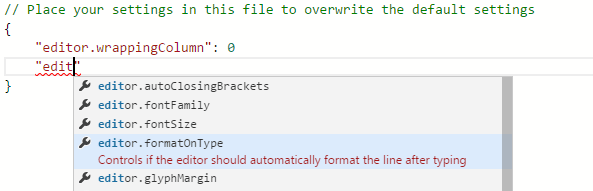
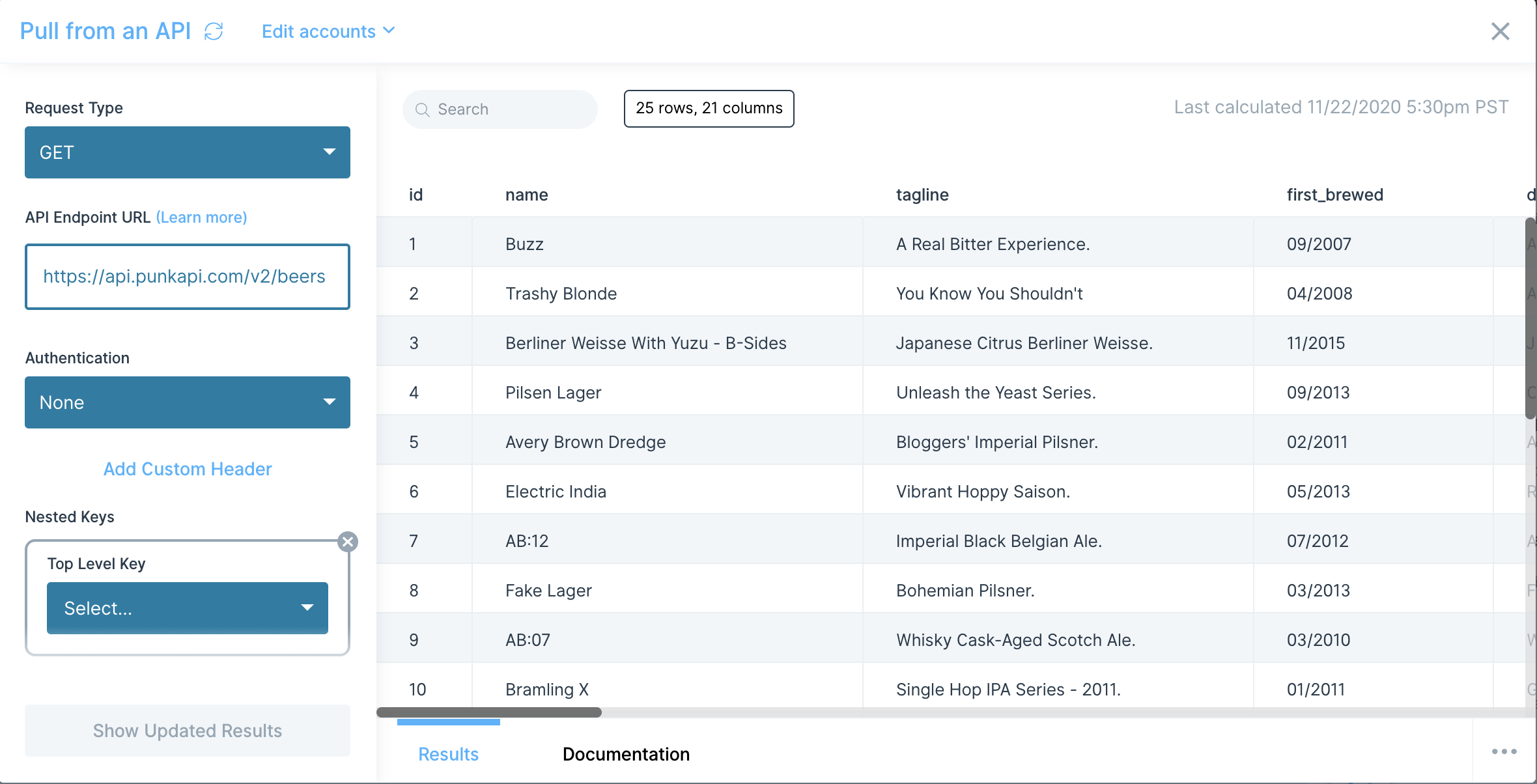

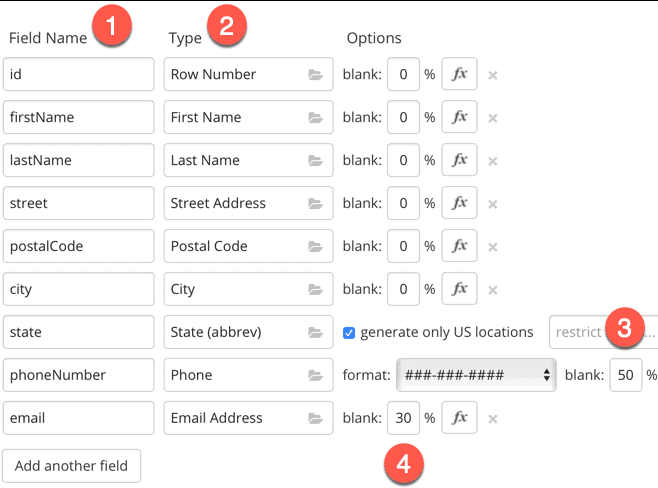



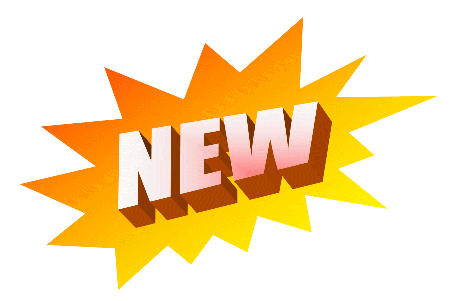
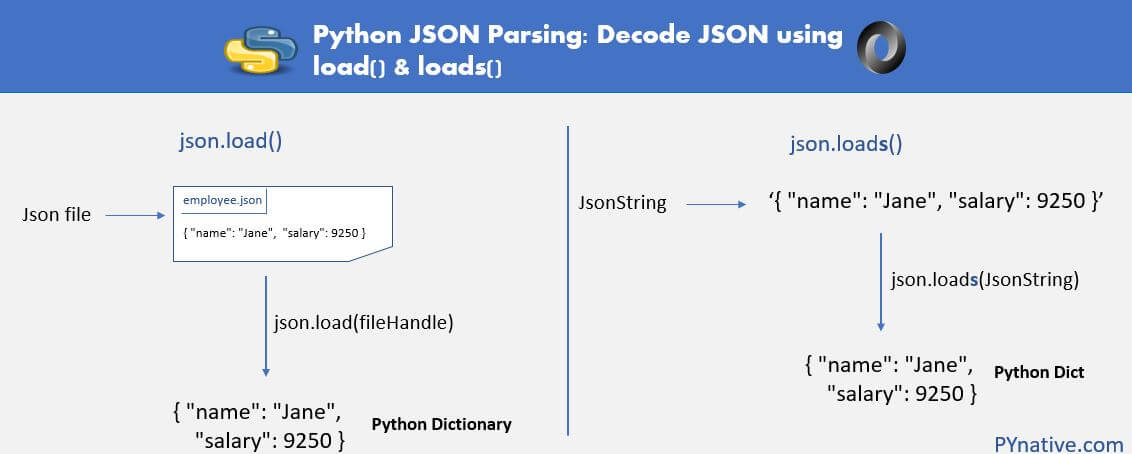

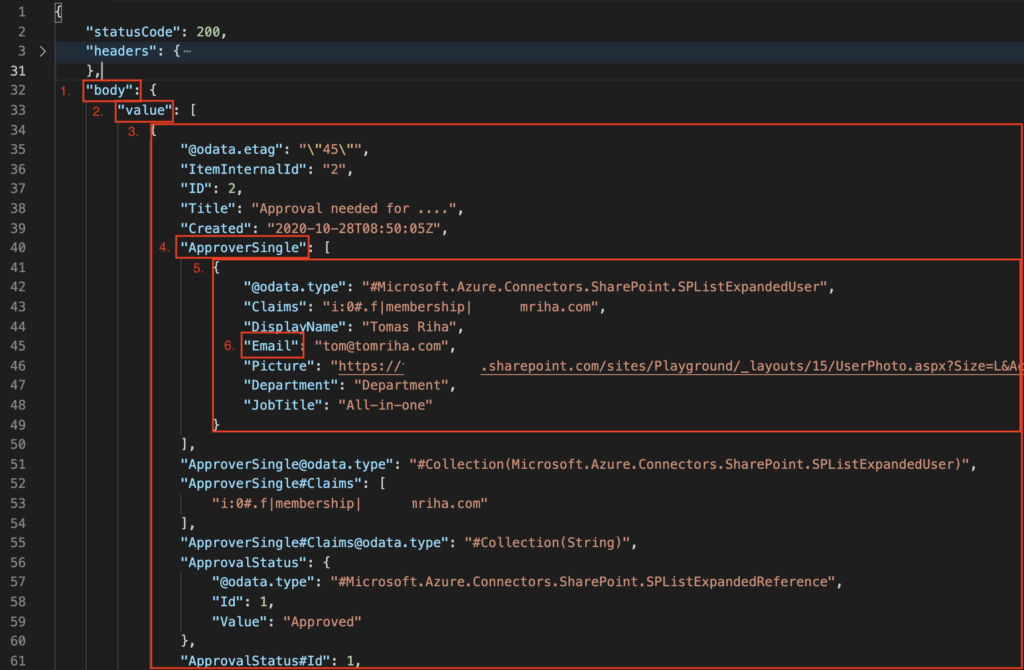


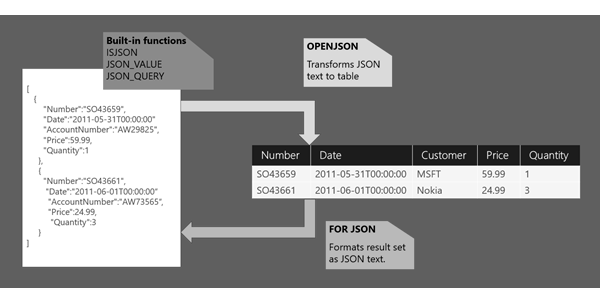
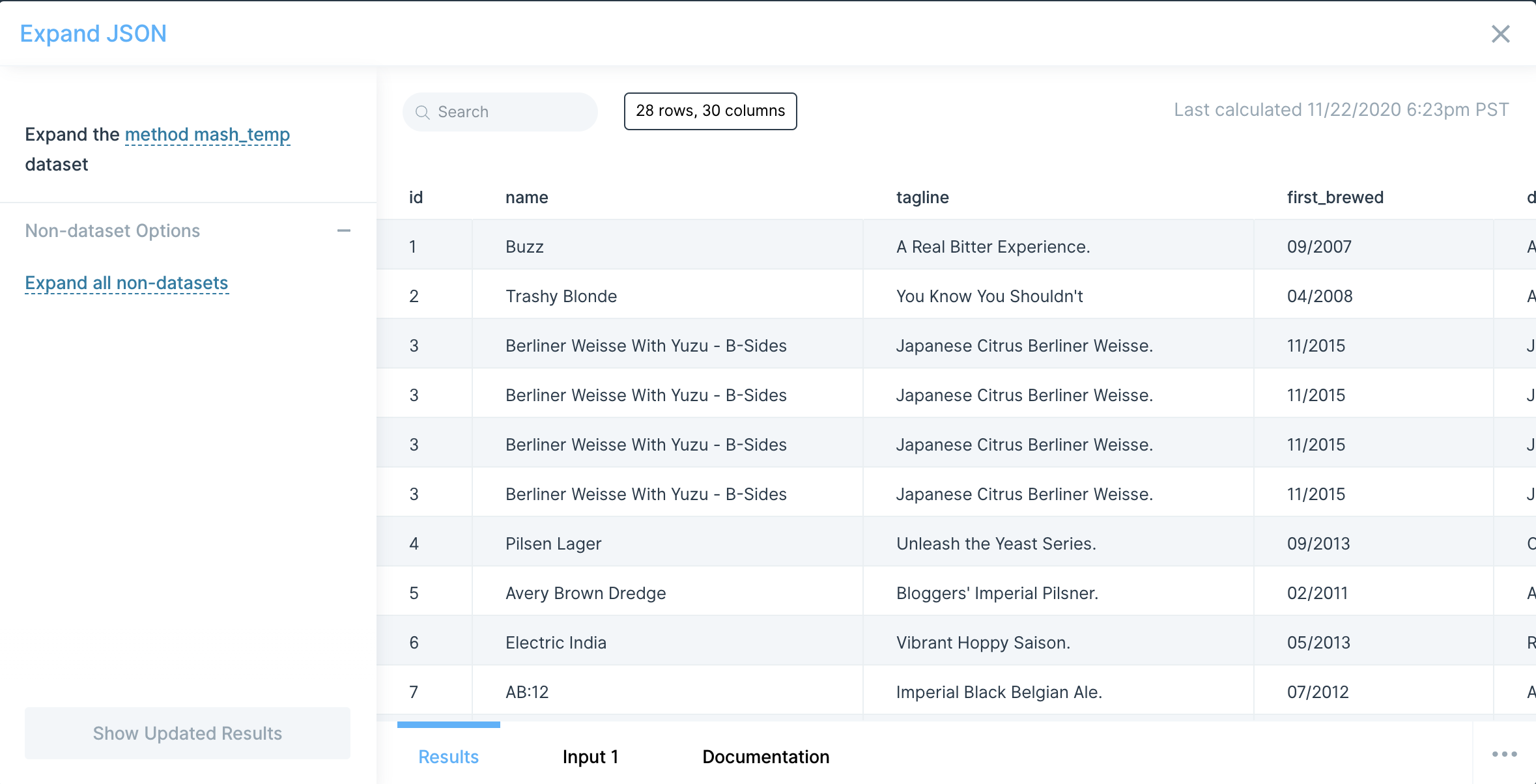



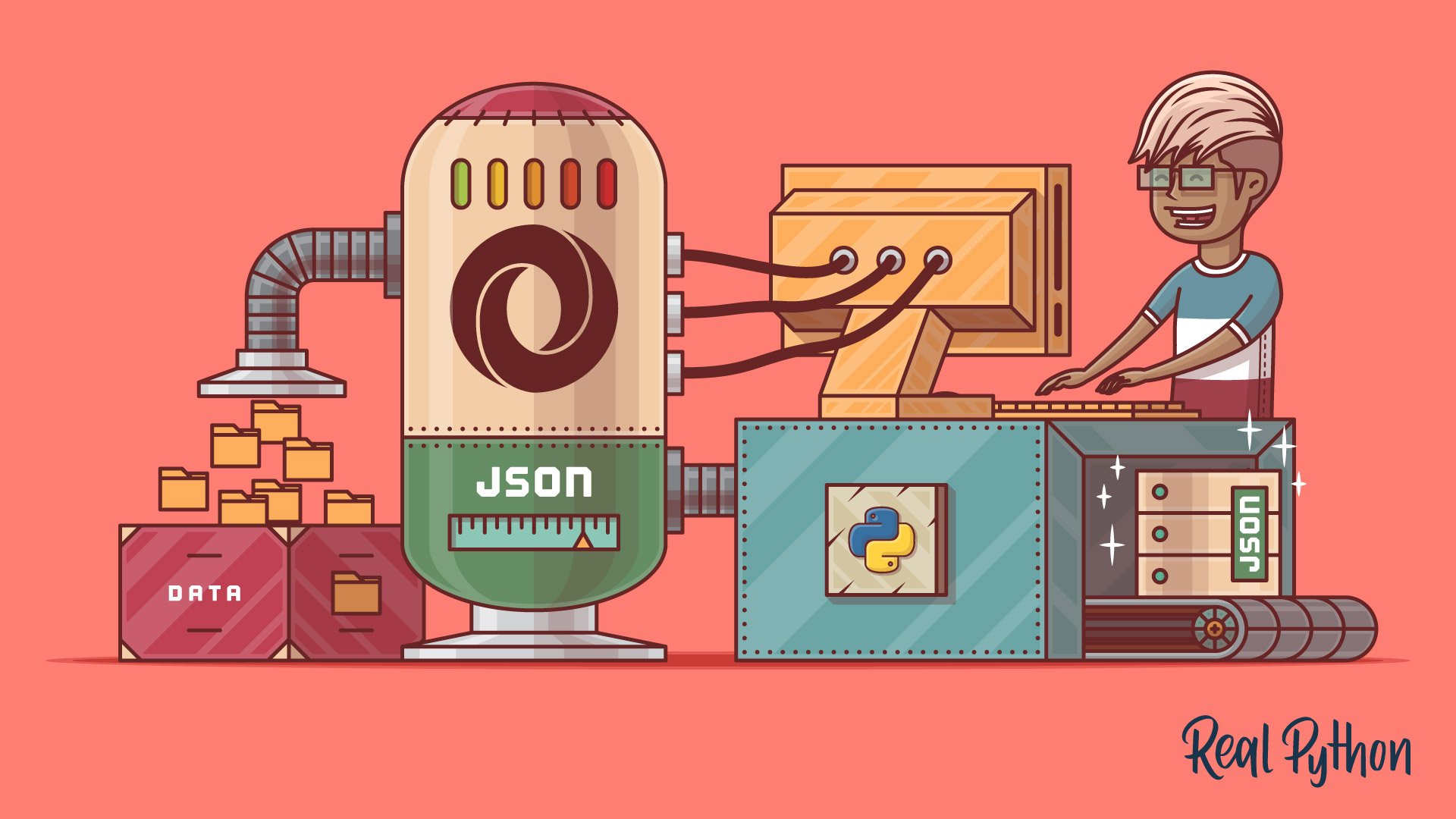
Article link: json to one line.
Learn more about the topic json to one line.
- Json to One Line Converter – Percentage Calculator
- JSON to One Line to convert json to single line
- JSON Formatter & JSON To One Line
- JSON to One Line to convert multiline …
- Online Multiline to Singleline Converter – Text/JSON/XML
- JSON to One Line Converter – JSON Formatter
- JSON Formatter & JSON To One Line – Text-Utils.com
- Do JSON in One line with Jackson + Java | by Mansura H. – Medium
- How to use a line break in JSON? – Hardique Dasore – Medium
- JSON file | Databricks on AWS
- How to convert multiline json to single line? – python
- I need to convert one line of Text to JSON.
- JSON.stringify can create Multi-line, formatted and filtered …
See more: nhanvietluanvan.com/luat-hoc