Js Split Array Into Chunks
Introduction to Splitting an Array into Chunks:
Splitting an array into smaller, more manageable chunks is a common requirement in various programming scenarios. Whether you are dealing with large datasets, parallel processing, or chunked file uploads, understanding how to split an array into chunks can greatly improve your code’s efficiency and maintainability. This article will provide an overview of the various methods to achieve this task in JavaScript and explore the potential use cases and considerations involved.
Benefits of Splitting an Array into Chunks:
Splitting an array into chunks offers several advantages. Firstly, it allows for better control and manipulation of data by dividing a large dataset into smaller, more manageable portions. This can greatly enhance performance, especially in scenarios where processing large amounts of data can lead to memory issues or performance bottlenecks. Additionally, splitting arrays into chunks facilitates the processing of data in parallel, enabling significant improvements in execution times.
Methods for Splitting an Array into Chunks:
1. Using for loops:
One of the simplest ways to split an array into chunks is by leveraging traditional for loops in JavaScript. By defining the desired chunk size and iterating through the array, chunks can be generated and stored in a separate array.
2. Using the slice method:
The slice method is a built-in function in JavaScript that allows for the extraction of a section of an array. By repeatedly applying this method with appropriate slicing intervals, an array can be partitioned into smaller chunks.
3. Using the reduce method:
The reduce method in JavaScript provides an elegant and concise solution for splitting an array into chunks. By iteratively reducing the array into smaller chunks, the reduce method effectively partitions the array.
4. Using recursion:
Recursive approaches can also be used to split an array into chunks. By repeatedly dividing the array until the desired chunk size is reached, arrays can be recursively subdivided into smaller, more manageable sizes.
Considerations when Splitting an Array into Chunks:
1. Handling uneven chunk sizes:
When splitting an array into chunks, it is crucial to consider scenarios where the array length may not be divisible by the desired chunk size. Implementing logic to handle uneven chunk sizes ensures that no data is lost or misplaced during the splitting process.
2. Performance concerns:
Depending on the size of the array and the chosen method, performance can vary significantly. It is essential to evaluate the efficiency and scalability of the selected approach to avoid potential performance bottlenecks.
Use Cases for Splitting an Array into Chunks:
1. Pagination of large datasets:
When dealing with large datasets, splitting them into smaller chunks can facilitate efficient pagination. By retrieving and displaying only a subset of the data at a time, user experience can be greatly improved.
2. Parallel processing:
Splitting an array into chunks can be advantageous in scenarios where data processing can be distributed across multiple threads or processes, allowing for parallel execution and faster processing times.
3. Chunked file uploads:
When uploading large files, dividing them into smaller chunks enables efficient and reliable transmission. This approach ensures that the upload process is less prone to failures and data loss.
Examples of Splitting an Array into Chunks in JavaScript:
Below are some code snippets showcasing different methods of splitting an array into chunks in JavaScript:
– Split array JavaScript:
“`JavaScript
function chunkArray(array, chunkSize) {
const chunks = [];
for (let i = 0; i < array.length; i += chunkSize) {
chunks.push(array.slice(i, i + chunkSize));
}
return chunks;
}
```
- Java split array into chunks:
```JavaScript
function splitArrayIntoChunks(array, chunkSize) {
const chunks = [];
for (let i = 0; i < array.length; i += chunkSize) {
chunks.push(array.slice(i, i + chunkSize));
}
return chunks;
}
```
- Split array to multiple arrays:
```JavaScript
function splitArrayToMultipleArrays(array, chunkSize) {
return array.reduce((acc, val, i) => {
const chunkIndex = Math.floor(i / chunkSize);
if (!acc[chunkIndex]) {
acc[chunkIndex] = [];
}
acc[chunkIndex].push(val);
return acc;
}, []);
}
“`
– Slice array js:
“`JavaScript
function sliceArray(array, chunkSize) {
const chunks = [];
while (array.length) {
chunks.push(array.splice(0, chunkSize));
}
return chunks;
}
“`
– Lodash chunk:
“`JavaScript
const _ = require(‘lodash’);
const chunkedArray = _.chunk(array, chunkSize);
“`
– TypeScript array slicejs split array into chunks:
“`JavaScript
function splitArrayIntoChunks
// Implementation using any of the above methods
}
“`
FAQs:
Q1: How do you split an array into chunks in JavaScript?
A1: There are several methods to split an array into chunks in JavaScript, including using for loops, the slice method, the reduce method, and recursion. Each method has its own advantages and suitability based on specific use cases and requirements.
Q2: Can you split an array into uneven chunks in JavaScript?
A2: Yes, it is possible to split an array into uneven chunks in JavaScript. However, it is important to handle such scenarios appropriately to ensure data integrity and prevent any loss or misplacement of elements during the splitting process.
Q3: What are the benefits of splitting an array into chunks?
A3: Splitting an array into chunks allows for better control and manipulation of data, enhances performance by improving memory usage and processing times, facilitates parallel processing, and enables the efficient handling of large datasets or chunked file uploads.
Q4: When should I consider splitting an array into chunks?
A4: Splitting an array into chunks is particularly useful in scenarios involving pagination of large datasets, parallel processing, and chunked file uploads. It can significantly improve application performance, scalability, and user experience.
Q5: What are the performance considerations when splitting an array into chunks?
A5: Choosing an efficient method based on the specific requirements is crucial. Performance can vary depending on the size of the array and the chosen splitting method. It is important to evaluate and optimize the selected approach to prevent potential performance bottlenecks.
How To Split Array Into Chunks In Javascript | Split Array In Javascript
Keywords searched by users: js split array into chunks Split array JavaScript, Java split array into chunks, Split array to multiple arrays, Split and add to array in javascript, Slice array js, Slice js, Lodash chunk, Typescript array slice
Categories: Top 37 Js Split Array Into Chunks
See more here: nhanvietluanvan.com
Split Array Javascript
JavaScript is a versatile programming language that empowers web developers with an array of powerful techniques and functions. One such function that often comes in handy is the split() function. In this article, we will explore the split array JavaScript function in depth, discussing its purpose, usage, and potential applications. So let’s dive right in!
Understanding the Split() Function
The split() function is a built-in JavaScript function that allows developers to divide a string into an array of substrings based on a specified separator. By specifying the separator, like a character or regular expression, you can split a string wherever that separator occurs.
Usage and Basic Syntax
The split() function is quite simple to use and follows a basic syntax:
“`
string.split(separator, limit)
“`
The ‘string’ variable represents the initial string that you want to split. The ‘separator’ parameter specifies the character or regular expression pattern at which you want the string to split. Finally, the optional ‘limit’ parameter determines the maximum number of splits to be performed on the given string.
Splitting a String Based on a Character Separator
To split a string based on a specific character separator, you can simply specify that character as the separator. Let’s illustrate this with a practical example:
“`javascript
const fullName = “John Doe”;
const nameArray = fullName.split(” “);
console.log(nameArray);
“`
In this example, the fullName string is split using whitespace as the separator. The resulting array, nameArray, will contain two elements: ‘John’ and ‘Doe’. This functionality is particularly useful when you want to separate first and last names, for example.
Splitting a String Based on a Regular Expression Separator
The split() function also supports the use of regular expressions as separators. Regular expressions allow for more complex matching patterns, enabling a greater level of control over the splitting process. Here’s an example illustrating the use of a regular expression:
“`javascript
const address = “123 Main St, Anytown, USA”;
const addressArray = address.split(/, */);
console.log(addressArray);
“`
In this case, the address string is split using a regular expression that matches a comma followed by zero or more spaces. The resulting addressArray will contain three elements: ‘123 Main St’, ‘Anytown’, and ‘USA’.
Splitting a String with a Specified Limit
By providing a limit parameter, you can control the maximum number of splits performed on a string. Let’s take a look at an example:
“`javascript
const fruits = “apple,banana,grape,orange”;
const fruitArray = fruits.split(“,”, 3);
console.log(fruitArray);
“`
In this example, the fruits string is split at each occurrence of a comma, but the limit is set to 3. As a result, the fruitArray will only include the first three elements: ‘apple’, ‘banana’, and ‘grape’.
Potential Applications
1. Data Parsing: The split array JavaScript function is commonly used in data parsing scenarios. For instance, if you’re working with CSV (comma-separated values) data, you can split each row into an array of values by specifying the comma as the separator.
2. String Manipulation: Splitting a string into an array of substrings enables you to manipulate individual parts of the string easily. You can access and modify separate elements within the array, then join them back together using the join() function.
3. URL Analysis: The split() function can also be used to extract specific information from URLs. By splitting a URL at the forward slashes, you can access parts such as the domain, path, and query parameters.
FAQs
Q1. Can I split a string with multiple separators?
Yes, you can use a regular expression with the `|` (pipe) character to specify multiple separators. For example: `string.split(/,|;/)`.
Q2. What happens if the separator is not found in the string?
In this case, the split() function will return an array with the original string as the only element.
Q3. Can I split an empty string?
Yes, splitting an empty string will result in an array with an empty element.
Q4. What is the difference between split() and join() functions?
The split() function splits a string into an array of substrings, while the join() function combines an array of substrings into a single string.
To conclude, the split array JavaScript function is a valuable tool for manipulating strings and gaining insights from data. Whether you need to parse data, manipulate strings, or analyze URLs, the split() function provides an efficient solution. By understanding its usage and exploring potential applications, you can unlock the true potential of this versatile JavaScript function.
Java Split Array Into Chunks
Introduction to Splitting an Array into Chunks
In Java, there may be times when you need to split an array into smaller, equally-sized chunks. This process is particularly useful when you want to process or manipulate a large array in manageable segments. This article will explore various techniques and approaches that can be used to split an array into chunks in Java, offering in-depth insights to ensure a comprehensive understanding of the topic.
Methods to Split an Array into Chunks
There are several methods that can be employed to accomplish the task of splitting an array into chunks in Java. Let’s explore the most commonly used approaches:
1. Using Loops:
One straightforward technique is to divide the array into chunks using loops. This can be achieved by keeping track of the starting and ending index of each chunk, and then extracting the corresponding elements from the array. Iterate through the array using a loop and increment the index values in each iteration, creating chunks based on specified chunk sizes.
2. Using Arrays.copyOfRange():
The ‘Arrays’ class in Java provides a useful built-in method called ‘copyOfRange()’. This method allows you to create a new array containing a specified range of elements from the original array. By continuously using this method, you can extract chunks of the desired size from the original array.
3. Using Collections.partition():
If your project involves working with collections, another efficient approach is to make use of the ‘partition()’ method from the ‘Collections’ class. This method splits the original array into smaller lists, also known as partitions, by specifying the desired chunk size. It returns a new list containing the partitions, making it easier to work with and manipulate each chunk as needed.
Examples of Splitting an Array into Chunks
Now, let’s delve into some practical examples that demonstrate how to split an array into chunks using the aforementioned methods:
Example 1: Using Loops
“`
int[] originalArray = {1, 2, 3, 4, 5, 6, 7};
int chunkSize = 3;
int startIndex = 0;
int endIndex = chunkSize;
while (startIndex < originalArray.length) {
int[] chunk = Arrays.copyOfRange(originalArray, startIndex, endIndex);
System.out.println(Arrays.toString(chunk));
startIndex += chunkSize;
endIndex = Math.min(endIndex + chunkSize, originalArray.length);
}
```
Example 2: Using Arrays.copyOfRange()
```
int[] originalArray = {1, 2, 3, 4, 5, 6, 7};
int chunkSize = 3;
for (int i = 0; i < originalArray.length; i += chunkSize) {
int[] chunk = Arrays.copyOfRange(originalArray, i, Math.min(i + chunkSize, originalArray.length));
System.out.println(Arrays.toString(chunk));
}
```
Example 3: Using Collections.partition()
```
List
int chunkSize = 3;
List> partitions = new ArrayList<>(Lists.partition(originalList, chunkSize));
for (List
System.out.println(partition);
}
“`
Frequently Asked Questions (FAQs)
Q1: What is the purpose of splitting an array into chunks?
A1: Splitting an array into chunks allows you to process large arrays efficiently, as it becomes easier to work with smaller segments rather than the entire array at once. It facilitates more manageable data manipulation and processing.
Q2: Are there limitations to splitting an array into chunks?
A2: While splitting an array into chunks is a useful technique, it’s important to consider any potential memory limitations, especially when working with very large arrays. Additionally, the chunk size must be carefully selected to ensure optimal performance and to avoid any data loss.
Q3: Are there any other benefits to splitting an array into chunks?
A3: Yes, one notable benefit is the ability to distribute and parallelize the processing of chunks across multiple threads or systems. By dividing the workload, you can leverage the power of concurrent processing to enhance overall performance.
Q4: Are there any performance concerns when splitting an array into chunks?
A4: Splitting an array into chunks can enhance performance by reducing the memory footprint and allowing parallel processing. However, if the chunks are too small, the overhead of splitting and processing them may outweigh the benefits. Careful consideration should be given to finding an appropriate chunk size based on the specific use case.
Conclusion
Splitting an array into chunks is an essential technique in Java that allows for more efficient data manipulation and processing. Through the utilization of various methods such as loops, Arrays.copyOfRange(), and Collections.partition(), developers can split an array into smaller, manageable sections. By applying the techniques presented in this article, you can improve the performance and scalability of your applications when dealing with larger datasets.
Split Array To Multiple Arrays
Introduction
Arrays are one of the fundamental data structures in programming that allow us to store multiple values under a single variable. However, there are situations when we need to split an array into multiple arrays based on specific requirements or conditions. This article aims to delve into the concept of splitting an array, discuss various approaches to achieving this task in different programming languages, and provide answers to frequently asked questions related to array splitting.
Understanding Array Splitting
Array splitting refers to the process of dividing a given array into multiple smaller arrays. There can be various reasons for splitting an array, such as separating data categories, facilitating processing of specific sections, or organizing data for different outputs. Regardless of the reason, the concept remains the same – dividing an array into multiple sub-arrays.
Approaches in Different Programming Languages
1. JavaScript
JavaScript provides a simple and effective mechanism for splitting arrays using the `slice()` method. The `slice()` method returns a new array containing a copy of the elements extracted from the provided starting and ending indices. By manipulating the indices, we can easily split an array into smaller arrays.
“`javascript
const originalArray = [1, 2, 3, 4, 5, 6];
const firstSplit = originalArray.slice(0, 3);
const secondSplit = originalArray.slice(3);
console.log(firstSplit); // Output: [1, 2, 3]
console.log(secondSplit); // Output: [4, 5, 6]
“`
2. Python
Python offers several ways to split arrays, depending on the required granularity and the preferred method. The `numpy.split()` function allows splitting arrays into equally sized sub-arrays along a specified axis. Another approach entails using list comprehensions or loops to iterate over the original array and create new sub-arrays as desired.
“`python
import numpy as np
original_array = np.array([1, 2, 3, 4, 5, 6])
sub_arrays = np.split(original_array, 3)
print(sub_arrays)
# Output: [array([1, 2]), array([3, 4]), array([5, 6])]
“`
Alternatively:
“`python
original_array = [1, 2, 3, 4, 5, 6]
split_size = 3
sub_arrays = [original_array[i:i+split_size] for i in range(0, len(original_array), split_size)]
print(sub_arrays)
# Output: [[1, 2, 3], [4, 5, 6]]
“`
3. Java
Java provides several mechanisms for splitting arrays. One approach involves using the `Arrays.copyOfRange()` method to extract a portion of the original array and create a new one. Another option is to employ the `System.arraycopy()` method to copy specific elements into a different array, effectively splitting the original array.
“`java
int[] originalArray = {1, 2, 3, 4, 5, 6};
int[] firstSplit = Arrays.copyOfRange(originalArray, 0, 3);
int[] secondSplit = Arrays.copyOfRange(originalArray, 3, originalArray.length);
System.out.println(Arrays.toString(firstSplit)); // Output: [1, 2, 3]
System.out.println(Arrays.toString(secondSplit)); // Output: [4, 5, 6]
“`
Frequently Asked Questions
1. What is the benefit of splitting an array into multiple arrays?
Splitting an array offers advantages such as improved code organization, easier data processing, and enhanced modularity. It allows for efficient handling of specific sections and enables customized handling of different data categories.
2. Can the original array be modified after splitting?
By using the mentioned techniques, the original array remains unaltered as the splitting process creates new independent arrays containing desired elements.
3. How can we split an array into unequal sub-arrays?
If we want to divide an array into sub-arrays of different sizes, we can modify the slicing/looping mechanisms described earlier. Rather than using fixed intervals, we can introduce conditional statements or vary the splitting parameters based on specific requirements.
4. Is it possible to split a multi-dimensional array into multiple arrays?
Yes, the principles of array splitting can be applied to multi-dimensional arrays as well. Different programming languages offer various methods to split multi-dimensional arrays based on specific axis-dependent patterns or user-defined criteria.
Conclusion
Splitting arrays into multiple arrays is a common requirement in programming when dealing with extensive datasets or when specific processing needs arise. Understanding the various techniques available in different programming languages, such as JavaScript, Python, and Java, empowers developers to accomplish this task efficiently. By effectively embracing array splitting, programmers can enhance code organization, improve data processing capabilities, and optimize performance in their applications.
Remember to consult language-specific documentation for further details on array splitting capabilities and to adapt the provided examples according to your programming environment.
Images related to the topic js split array into chunks
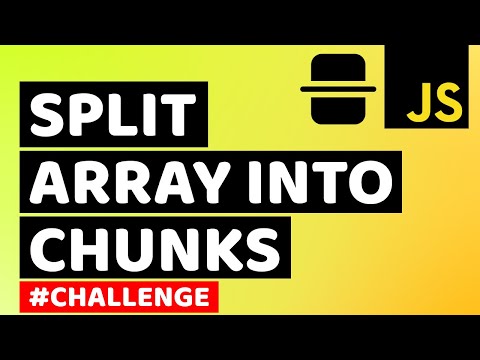
Found 6 images related to js split array into chunks theme
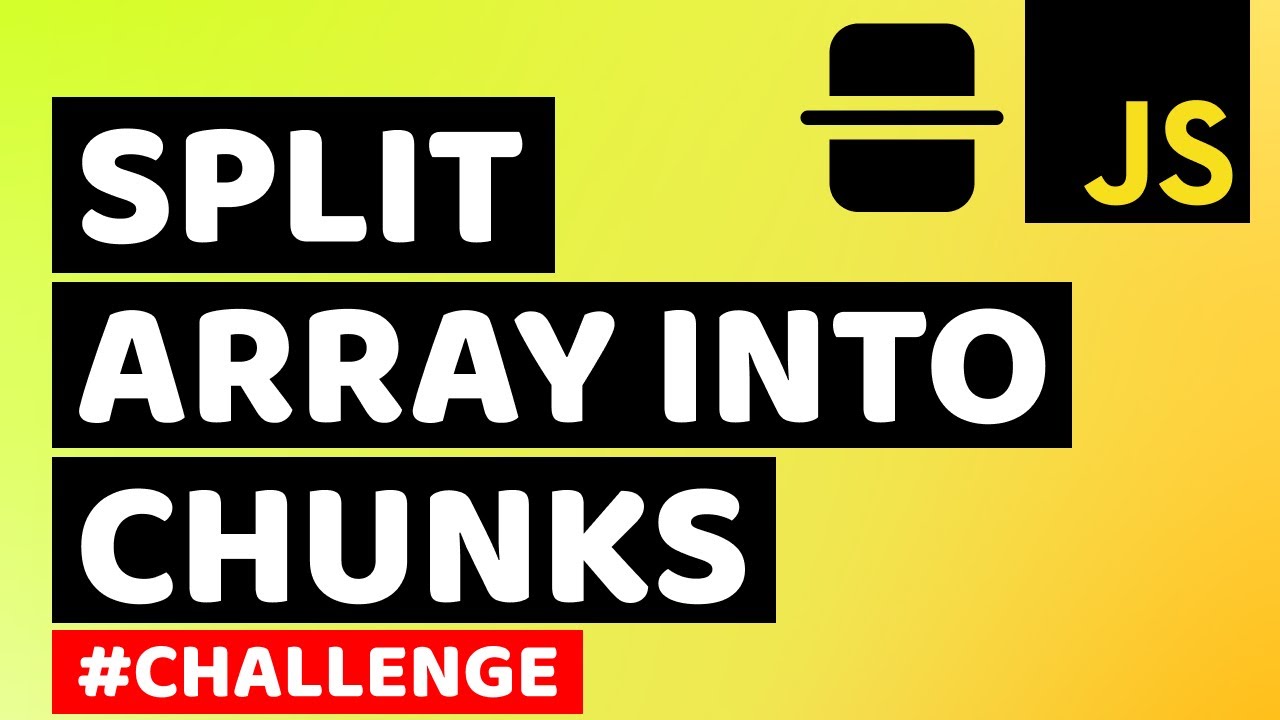
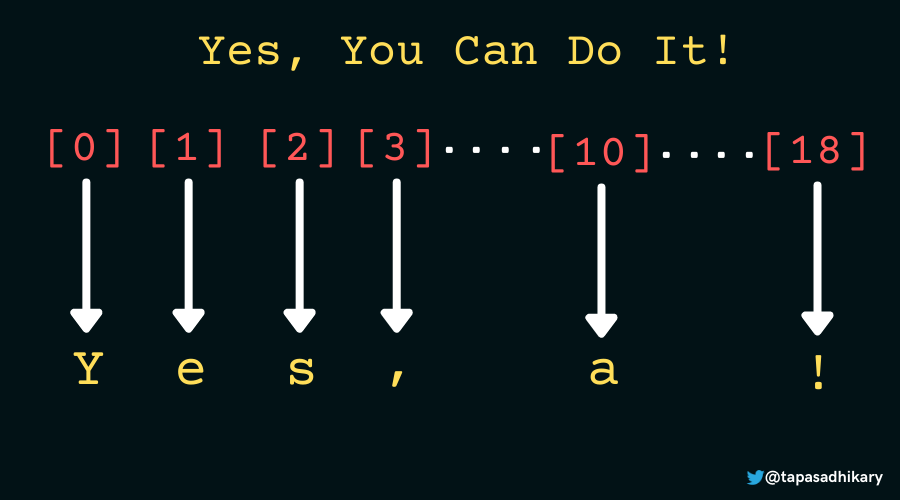

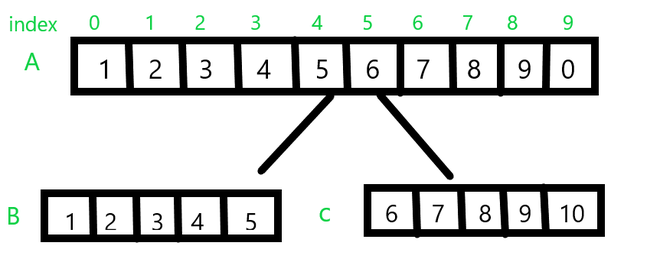
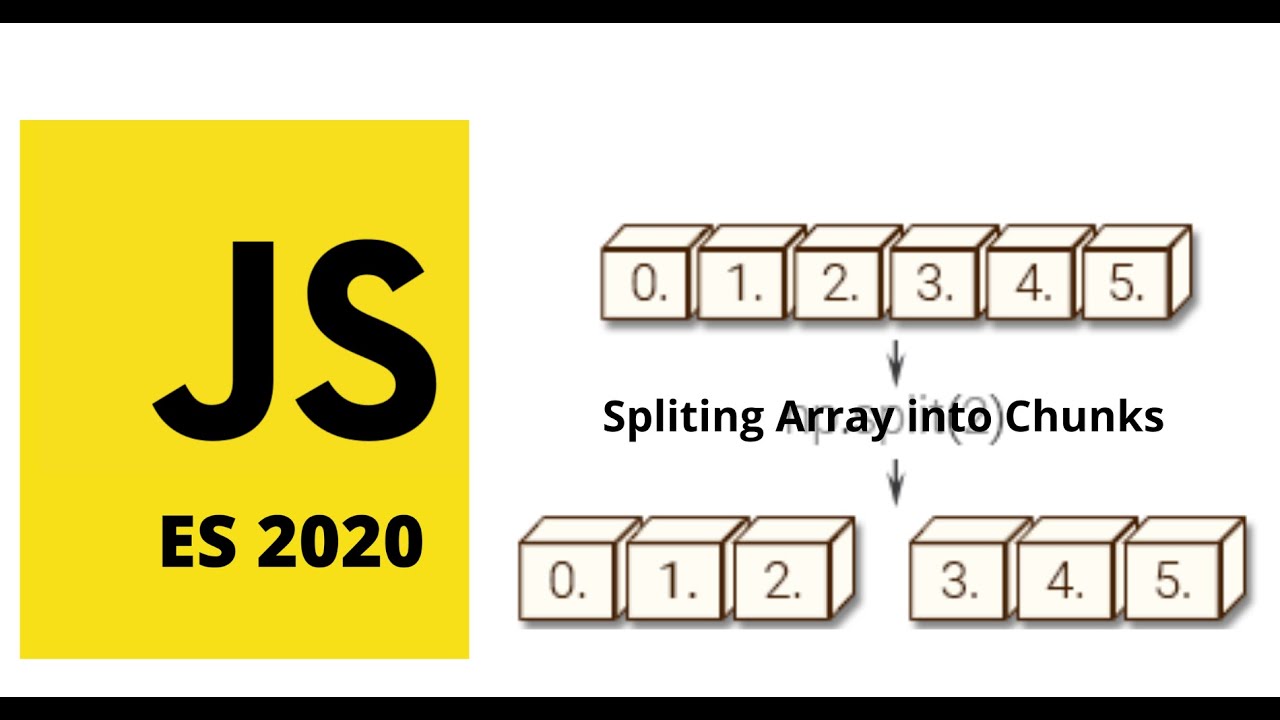

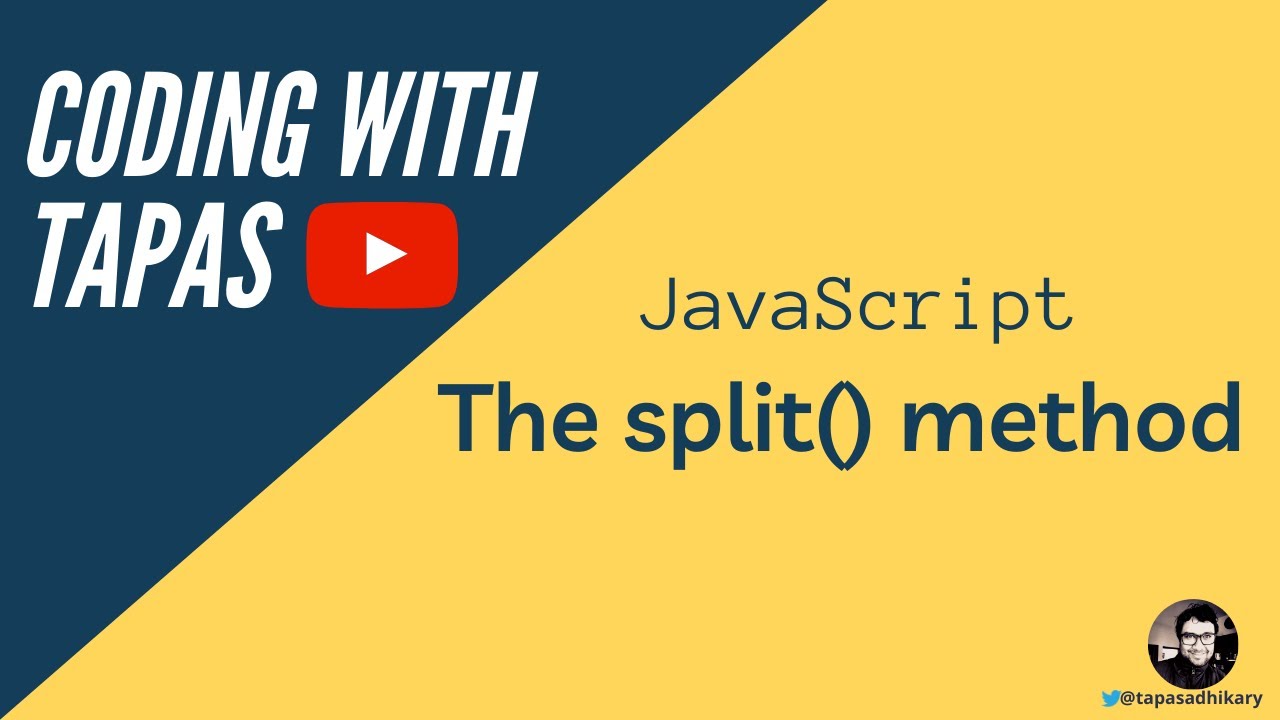


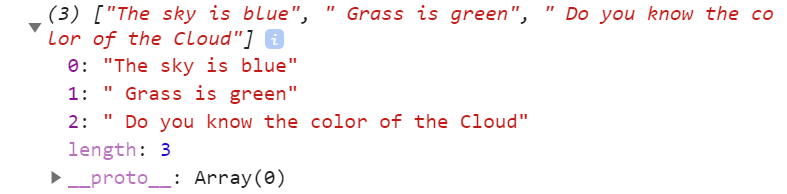
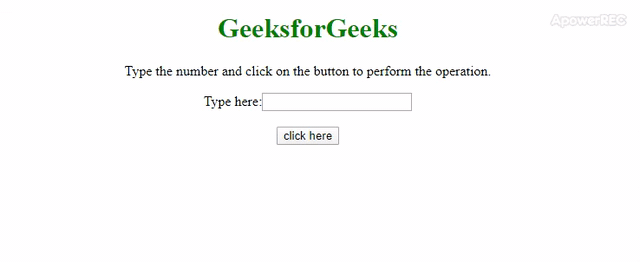


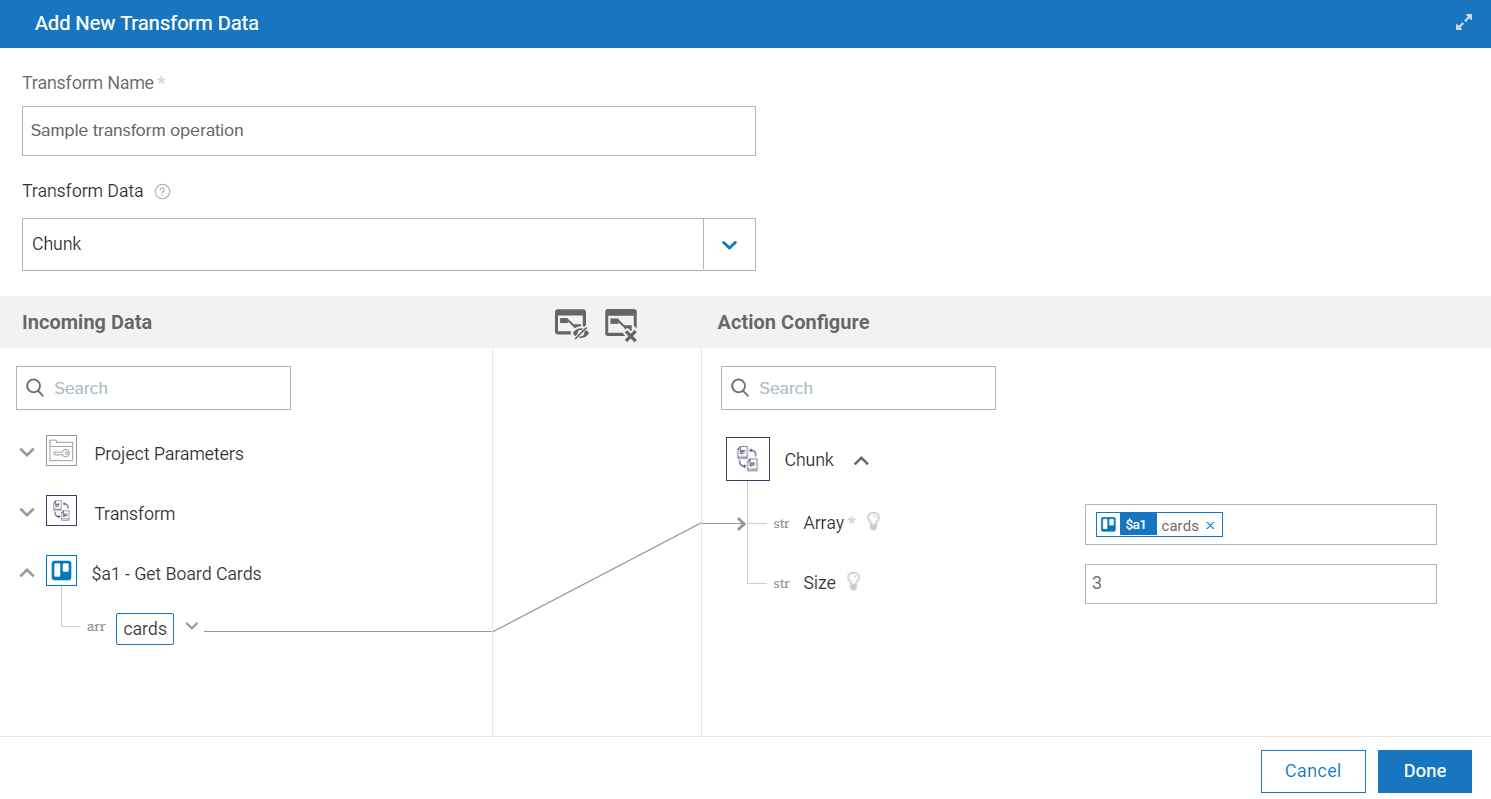
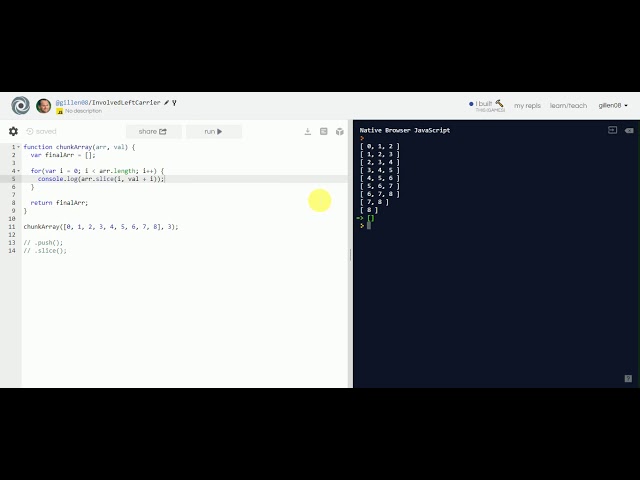

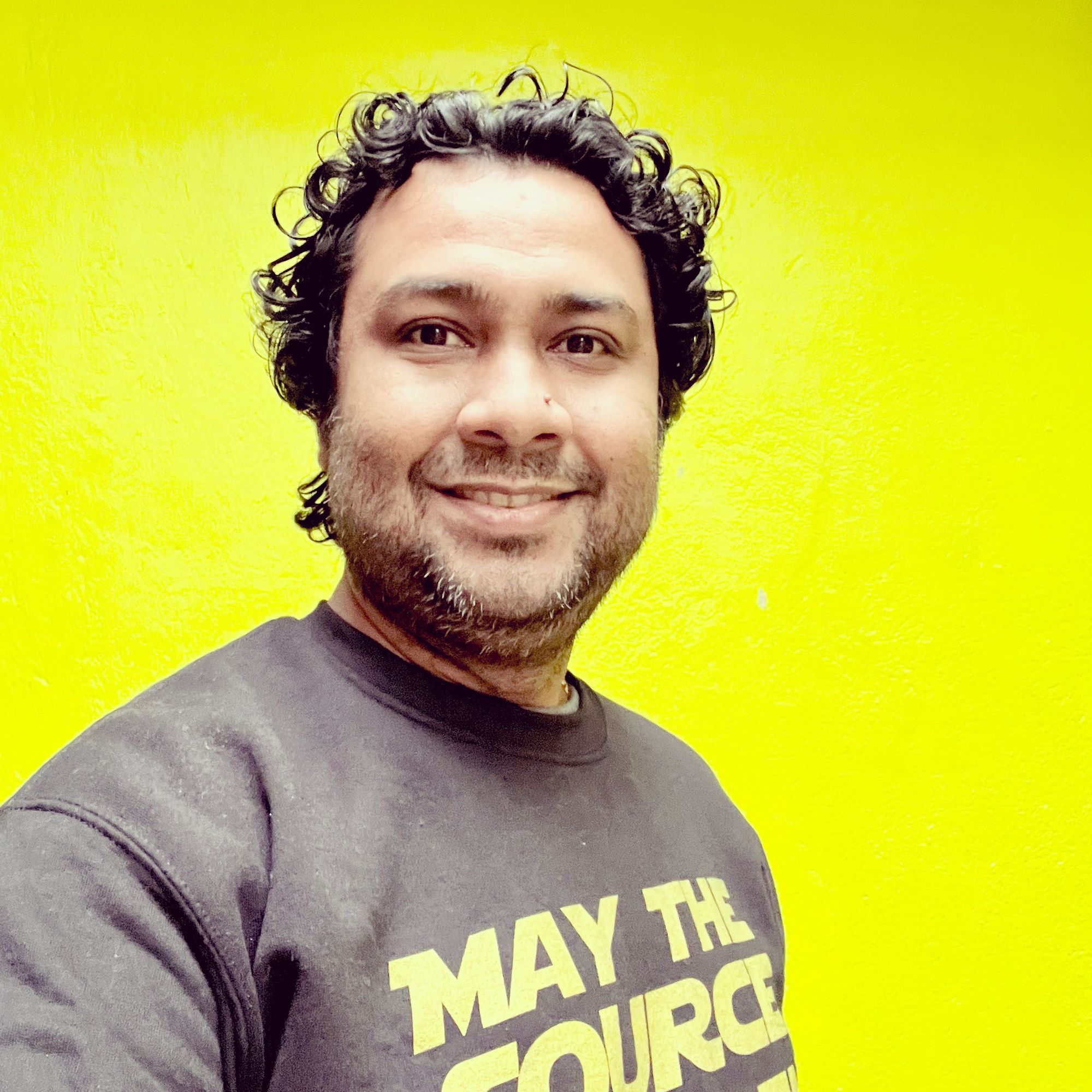

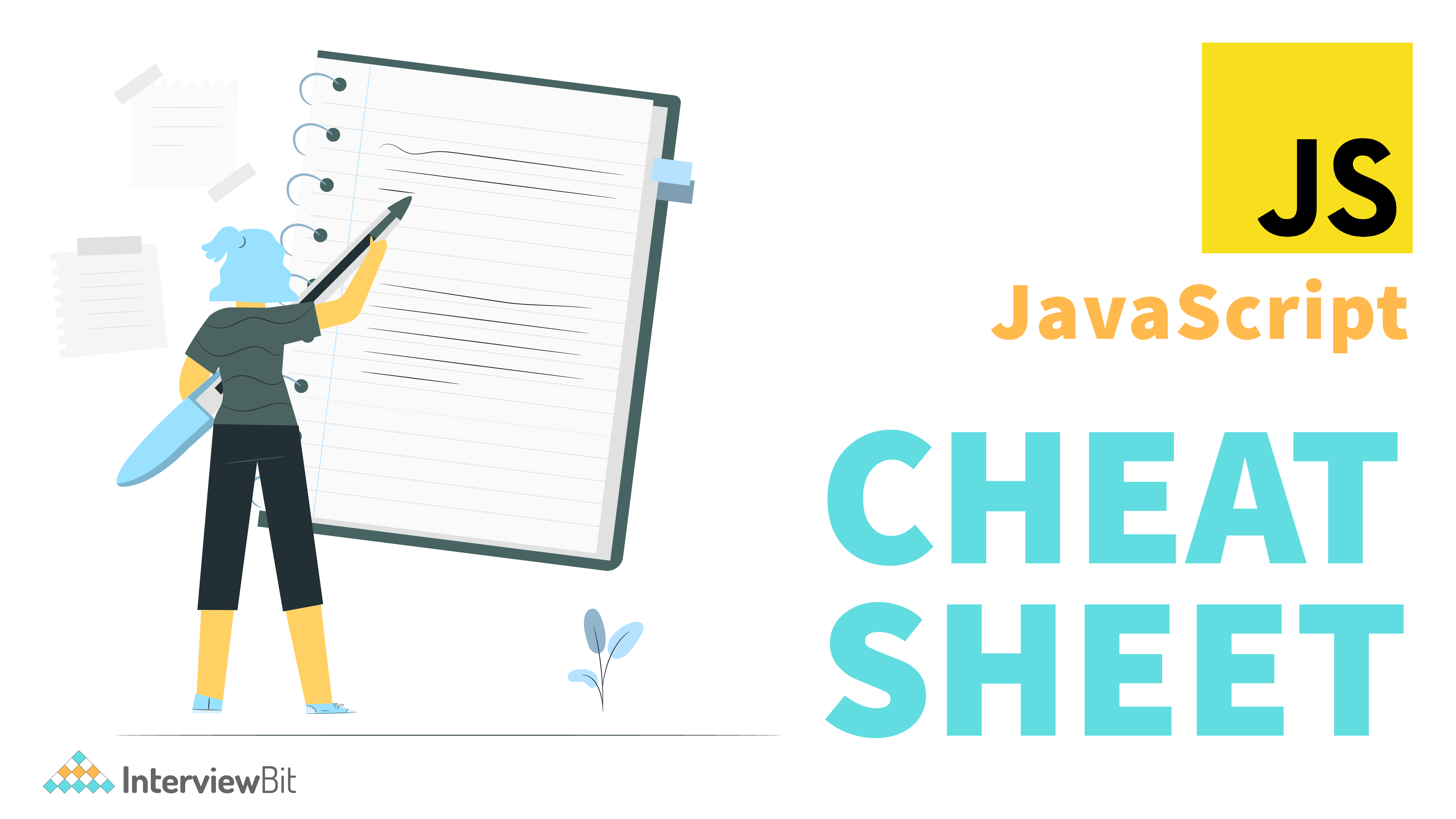
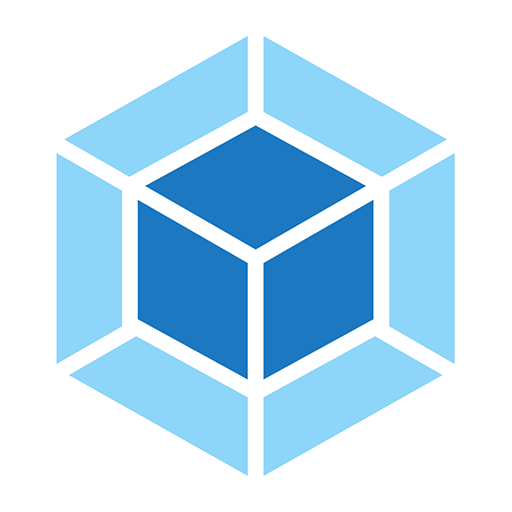
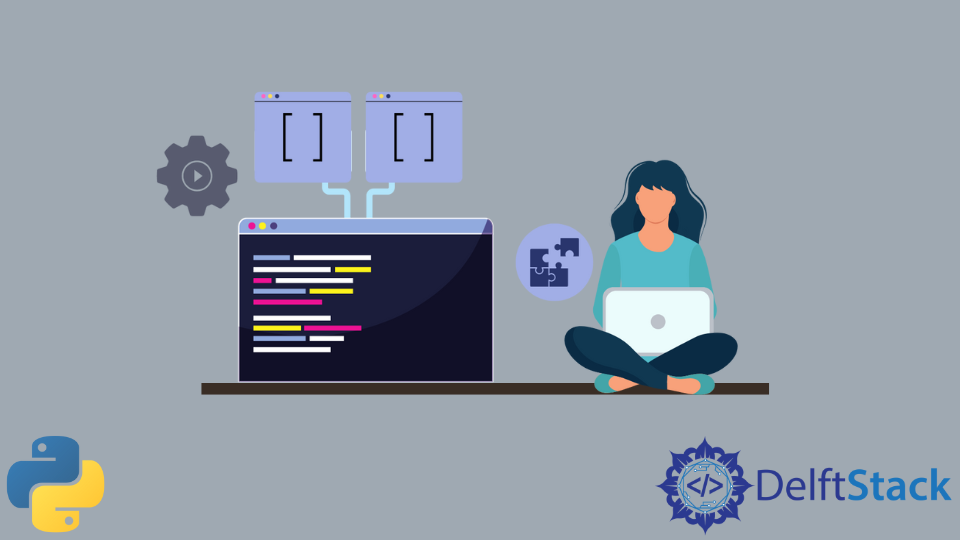
Article link: js split array into chunks.
Learn more about the topic js split array into chunks.
- Split array into chunks – javascript – Stack Overflow
- How to Split an Array into Even Chunks in JavaScript
- JavaScript Program to Split Array into Smaller Chunks
- JavaScript: Split an array into equally-sized chunks (3 …
- How can I split an array into chunks in Javascript? – Gitnux Blog
- Split a Javascript Array into Chunks | by Damion Davy | Medium
- Splitting arrays into chunks – Dom Habersack
- JavaScript: Chunk an array into smaller arrays of a specified size
- Split an array into chunks in JavaScript – GeeksforGeeks