Js Scroll To Bottom
Are you looking to implement an automatic scroll to the bottom feature on your website? Whether you’re using JavaScript, ReactJS, jQuery, or CSS, this article will cover everything you need to know about achieving this functionality. We will explore detecting scroll position, understanding the Window object, utilizing its properties, implementing a scroll event listener, defining a scroll function, and finally, scrolling to the bottom. So let’s dive in!
Detecting Scroll Position:
Before we proceed with the implementation, it’s crucial to understand how to detect the scroll position. JavaScript provides us with the Window object, which includes several properties that can be utilized to determine the current scroll position of the webpage. The key properties to take note of are `window.pageYOffset` and `window.innerHeight`.
– `window.pageYOffset` returns the number of pixels the document has been scrolled vertically.
– `window.innerHeight` represents the height of the browser window’s content area.
Understanding the Window Object:
The Window object represents the browser’s window or frame containing a DOM document. It provides access to various properties and methods to interact with the webpage. By leveraging the Window object, we can easily implement the scroll to bottom feature.
Utilizing the Window Object’s Properties:
To check if the user has scrolled to the bottom, we need to compare the scroll position (`window.pageYOffset`) with the total height of the webpage (`document.documentElement.scrollHeight`). By subtracting the scroll position from the total height and comparing it with the window’s inner height, we can determine if the user has reached the bottom. Here’s an example:
“`javascript
function isScrollAtBottom() {
return window.innerHeight + window.pageYOffset >= document.documentElement.scrollHeight;
}
“`
Implementing Scroll Event Listener:
To continuously monitor the scroll position and trigger the scroll to bottom functionality, we can attach a scroll event listener to the Window object. This event listener will execute a function whenever the user scrolls the webpage.
“`javascript
window.addEventListener(“scroll”, function() {
if (isScrollAtBottom()) {
// User has scrolled to the bottom
// Write your code here to perform desired actions
}
});
“`
Defining the Scroll Function:
Once the user has reached the bottom, we can invoke a scroll function that will automatically scroll the webpage to the desired element. This function can be implemented using various frameworks such as ReactJS, jQuery, or even plain JavaScript with the help of CSS.
Scrolling to the Bottom:
To achieve a smooth scroll effect, we can use JavaScript’s `scrollTo` method. Here’s an example of how you can smoothly scroll to the bottom of the webpage in plain JavaScript:
“`javascript
function scrollToBottom() {
window.scrollTo({
top: document.documentElement.scrollHeight,
behavior: “smooth”
});
}
“`
JavaScript scroll to bottom smooth ensures a seamless scrolling experience for the user.
If you’re using ReactJS, you can utilize the `ref` attribute to select the desired element and then scroll to it. Here’s an example:
“`jsx
import React, { useRef } from “react”;
function ScrollToBottom() {
const bottomRef = useRef();
function scrollToBottom() {
bottomRef.current.scrollIntoView({ behavior: “smooth” });
}
return (
);
}
“`
This example demonstrates how to implement scroll to bottom in ReactJS.
If you prefer using jQuery, you can achieve the scroll to bottom functionality with the help of the `scrollTop` method. Here’s an example:
“`javascript
function scrollToBottom() {
$(“html, body”).animate({
scrollTop: $(document).height()
}, “slow”);
}
“`
jQuery scroll to bottom smoothly moves the webpage to the bottom.
FAQs:
1. Can I detect if the user has scrolled to the bottom without the need for a scroll event listener?
Yes, by comparing the scroll position with the total height of the webpage, you can determine if the user is at the bottom. However, using a scroll event listener provides a more dynamic approach.
2. Is it possible to customize the scroll behavior, such as adjusting the scrolling speed?
Yes, you can control the scroll behavior by adjusting the `behavior` property. Set it to “smooth” for a smooth scrolling effect or “auto” for the default behavior.
3. Can I scroll to a specific div instead of the bottom of the page?
Absolutely! Modify the `top` property in the `scrollTo` method to target a specific element’s top position on the webpage.
4. Is there any alternative to the `scrollTo` method for scrolling to the bottom?
Yes, you can also use the `scrollIntoView` method by passing the desired element as an argument. This will bring the element into view.
In conclusion, implementing a scroll to bottom feature on your website is now within reach. Whether you’re using JavaScript, ReactJS, jQuery, or CSS, the techniques discussed in this article will enable you to achieve a smooth automatic scroll experience. Remember to leverage the Window object’s properties, implement a scroll event listener, define a scroll function, and apply the appropriate scrolling method based on your chosen framework. Happy scrolling!
The \”Scroll\” Event In Javascript | Window.Onscroll
Keywords searched by users: js scroll to bottom JavaScript scroll to bottom smooth, Scroll to bottom ReactJS, Auto scroll to bottom, Scroll to bottom jQuery, Check scroll to bottom javascript, Auto scroll bottom CSS, Js scroll to div, Auto scroll js
Categories: Top 91 Js Scroll To Bottom
See more here: nhanvietluanvan.com
Javascript Scroll To Bottom Smooth
Introduction
In today’s web development landscape, providing a smooth scrolling experience is crucial for enhancing user engagement and improving overall user experience. One popular technique to achieve smooth scrolling is JavaScript scroll to bottom smooth functionality. In this article, we will explore what smooth scrolling is, how to implement it using JavaScript, and answer some frequently asked questions regarding its usage.
Understanding Smooth Scrolling
Smooth scrolling refers to the ability to seamlessly navigate through a web page, eliminating jarring jumps when scrolling, particularly on long or content-heavy pages. Instead of instantly moving from one part of the page to another, smooth scrolling gradually animates the transition, creating a more pleasing and visually appealing effect. This is often accomplished using JavaScript, which allows developers to control the scrolling behavior with various customization options.
Implementing JavaScript Scroll to Bottom Smooth
To implement the JavaScript scroll to bottom smooth functionality, one needs to follow a few simple steps:
Step 1: Attach a scroll event listener to the target element or the window object. This can be done using the `addEventListener` method in JavaScript.
Step 2: Within the event listener, retrieve the current scroll position of the element.
Step 3: Calculate the target scroll position, which in this case would be the bottom of the element or the page.
Step 4: Use a scrolling animation function, such as `requestAnimationFrame`, to gradually scroll from the current position to the target position by incrementally updating the scroll position over small intervals of time.
Step 5: Repeat steps 2 to 4 until the scroll position reaches the target position, thus achieving a smooth scrolling effect.
FAQs about JavaScript Scroll to Bottom Smooth
Q1: Can smooth scrolling be applied to any element on a web page?
A1: Smooth scrolling can be applied to any element that has a scrollable area, such as divs, iframes, or the window itself.
Q2: Are there any JavaScript libraries or plugins available for implementing smooth scrolling?
A2: Yes, there are many popular JavaScript libraries and plugins, such as ScrollMagic, AOS, and Smooth Scroll, that provide ready-to-use smooth scrolling functionality with additional customization options.
Q3: Does smooth scrolling impact performance?
A3: While the animations used for smooth scrolling can introduce some additional rendering overhead, modern browsers are optimized to handle such animations efficiently. However, it is recommended to test the performance on different devices and screen sizes to ensure a smooth experience.
Q4: Can smooth scrolling be customized further, such as changing animation duration or easing functions?
A4: Absolutely! Smooth scrolling can be customized extensively. You can adjust animation duration, easing functions, add delays, or even implement different animations altogether to suit your specific design and user experience requirements.
Q5: Can smooth scrolling be combined with other user interactions, such as mouse or touch events?
A5: Yes, smooth scrolling can be combined with other interactive events, allowing for dynamic scrolling experiences. For example, you can trigger smooth scrolling on button clicks or integrate it with swipe gestures on touch devices.
Concluding Thoughts
Implementing JavaScript scroll to bottom smooth functionality adds a touch of elegance to web pages, enhancing user experience and keeping users engaged. By gradually animating the scrolling transitions, developers can ensure a seamless journey through content-heavy pages, eliminating any abrupt jumps that can disrupt the user’s flow. With the flexibility and customization options available, smooth scrolling can be tailored to match various design styles and preferences. So, if you’re looking to create a visually pleasing, user-friendly website, give JavaScript scroll to bottom smooth a try!
Scroll To Bottom Reactjs
Scroll to bottom functionality is a common requirement in web development, enabling users to effortlessly navigate to the end of a page or a container. With the increasing popularity of ReactJS, a JavaScript library for building user interfaces, developers seek efficient ways to implement this feature. In this article, we will delve into the various approaches and strategies for achieving scroll to bottom functionality in ReactJS, providing you with a comprehensive guide to handle this requirement in your projects.
Understanding ReactJS
ReactJS, developed by Facebook, is a powerful JavaScript library that simplifies the creation of interactive user interfaces. Its component-based architecture enables developers to build reusable UI elements, making the development process more efficient and maintainable.
Implementing Scroll to Bottom in ReactJS
There are several ways to implement scroll to bottom functionality in ReactJS, each with its own advantages and use cases. Let’s explore some of the most popular and effective approaches:
1. Using ref and scrollIntoView()
One way to achieve scroll to bottom functionality is by utilizing the ref attribute and the scrollIntoView() method. Here’s how it works:
– Create a ref using the useRef() hook, which allows us to access and manipulate the underlying DOM element.
– Attach the ref to the container that needs scrolling.
– Whenever the content updates or a specific event occurs, call scrollIntoView() on the ref to scroll to the bottom.
This approach is straightforward, efficient, and suitable for most scenarios where the content is dynamic and constantly changing.
2. Using scrollTop property
Another approach involves using the scrollTop property of a scrollable container. By setting scrollTop to its maximum value, we can achieve the scroll to bottom effect. Here’s the implementation flow:
– Create a ref using useRef(), similar to the previous approach.
– Attach the ref to the container.
– Whenever the content updates or the desired event occurs, set scrollTop to the maximum value, scrolling the container to the bottom.
This method provides more control over the scrolling behavior, making it suitable for cases where precise scrolling is required.
3. Using a third-party library
If you prefer a more specialized and feature-rich solution, you can leverage third-party libraries specifically designed for scroll-related functionalities. Libraries like React-scroll and React-perfect-scrollbar provide additional options and customization for handling scroll to bottom requirements. These libraries offer a wide range of features, including smooth scrolling animations and fine-grained control over scrolling behavior.
Frequently Asked Questions (FAQs):
Q1. Can I use scroll to bottom functionality with a horizontal scroll?
A1. Absolutely! The techniques mentioned above can be applied to both vertical and horizontal scrolling containers, as long as the underlying DOM element supports scrolling.
Q2. Are there any performance considerations when implementing scroll to bottom in ReactJS?
A2. Performance largely depends on the amount of content being scrolled and the underlying hardware. However, using refs and manipulating DOM elements efficiently ensures optimal performance.
Q3. Is it possible to trigger scroll to bottom programmatically?
A3. Yes, you can programmatically trigger scroll to bottom by calling the appropriate method or modifying the scrollTop property based on specific events or conditions.
Q4. Can I apply smooth scrolling animation when scrolling to the bottom?
A4. Yes, you can achieve smooth scrolling animations by using third-party libraries like React-scroll and customizing the animation options according to your preferences.
Q5. Are there any browser compatibility issues with scroll to bottom functionality in ReactJS?
A5. The techniques covered in this article utilize standard JavaScript and ReactJS features, making them compatible with modern browsers. However, older browser versions may have limited support for certain scroll-related properties and methods.
Conclusion
Scroll to bottom functionality is a crucial feature for providing a seamless and effortless browsing experience to users. In this article, we explored several approaches to implement scroll to bottom in ReactJS, including using refs, scrollIntoView(), scrollTop property, and third-party libraries. Each approach offers its own advantages and can be tailored to specific use cases.
By understanding and utilizing these techniques, you’ll be equipped with the necessary knowledge to implement scroll to bottom functionality effectively in your ReactJS projects.
Images related to the topic js scroll to bottom
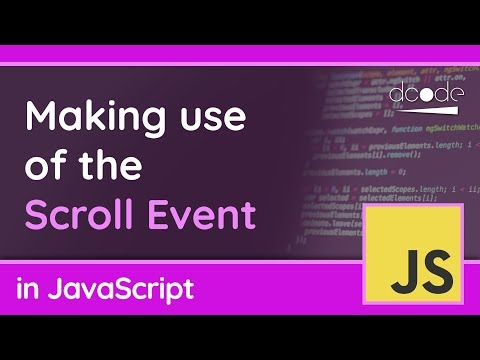
Found 29 images related to js scroll to bottom theme
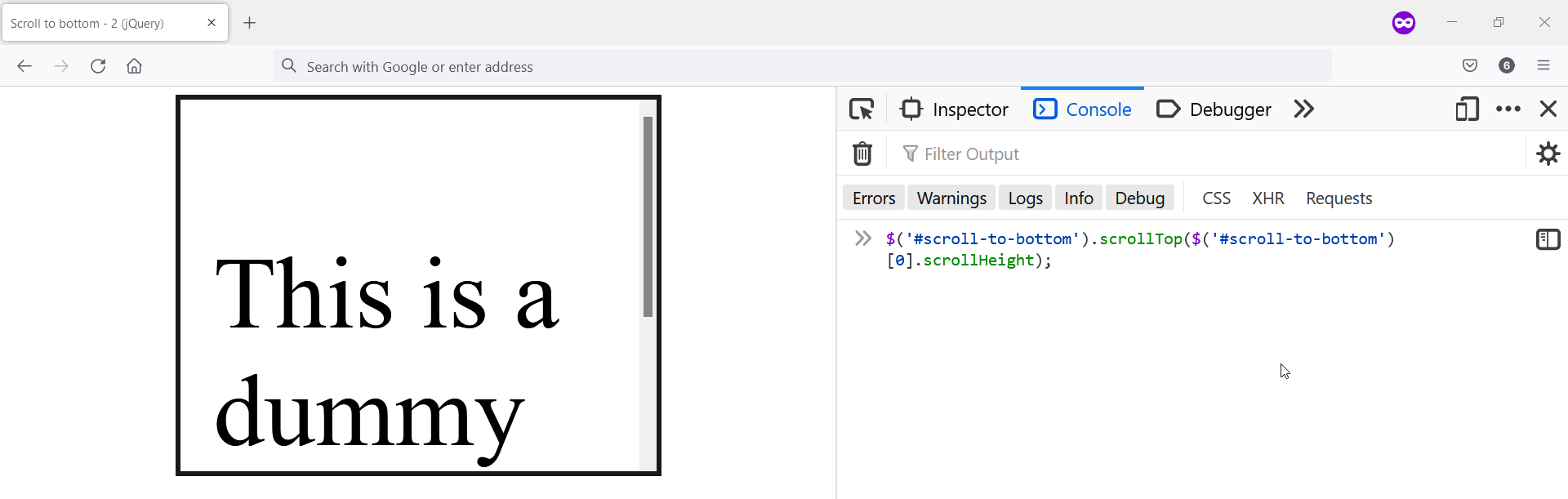

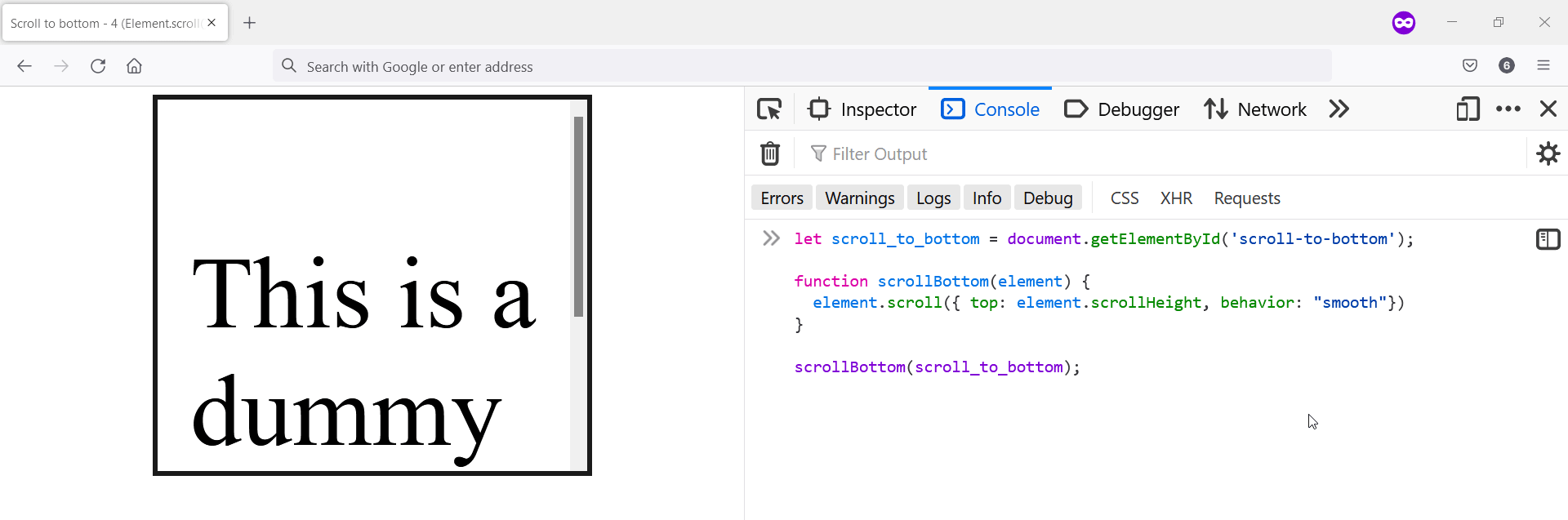
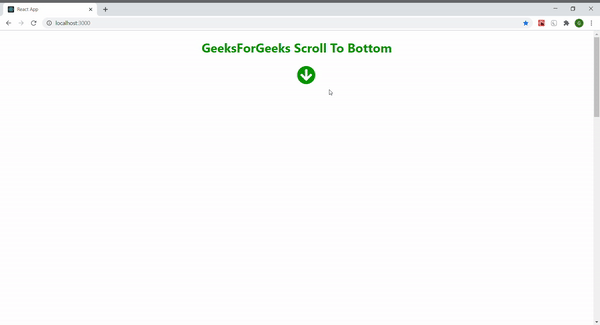
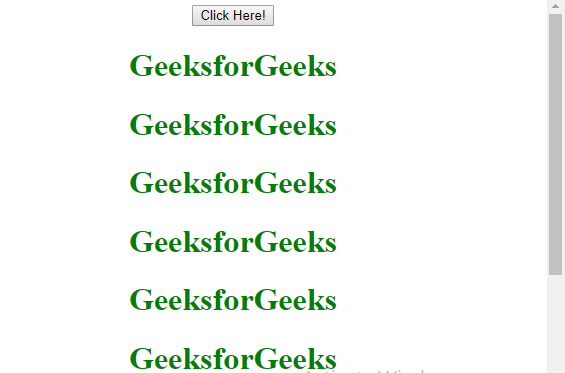
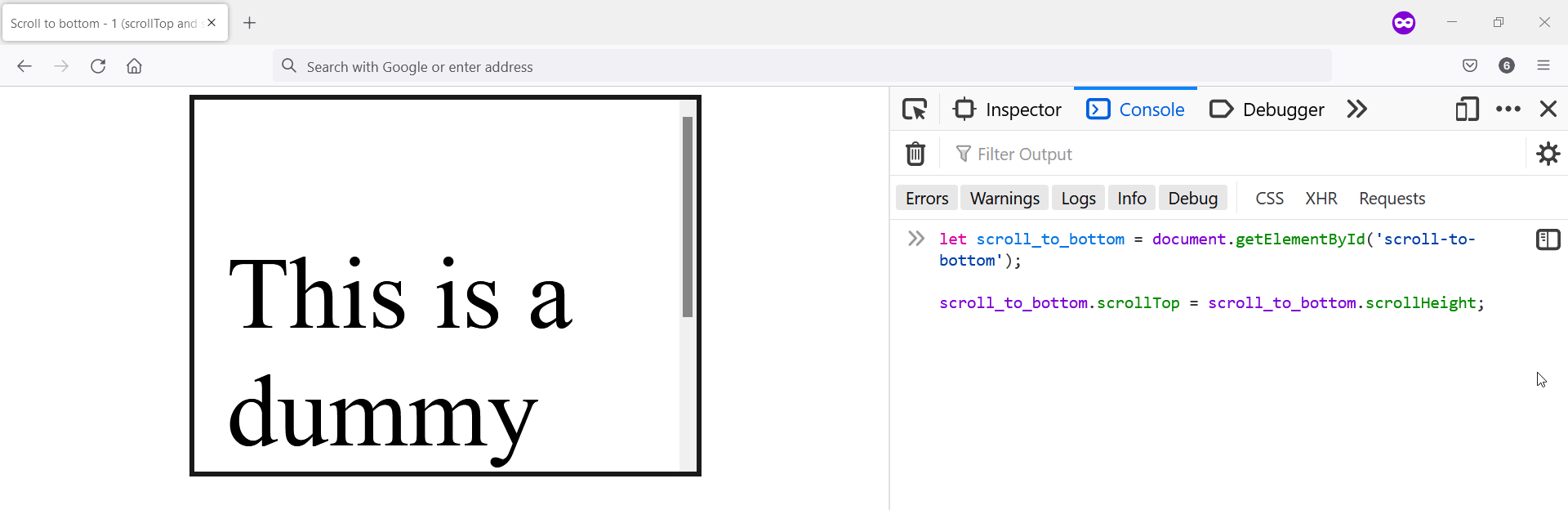
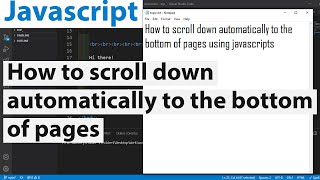
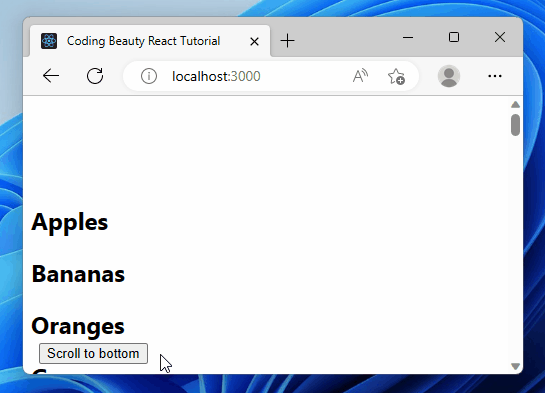
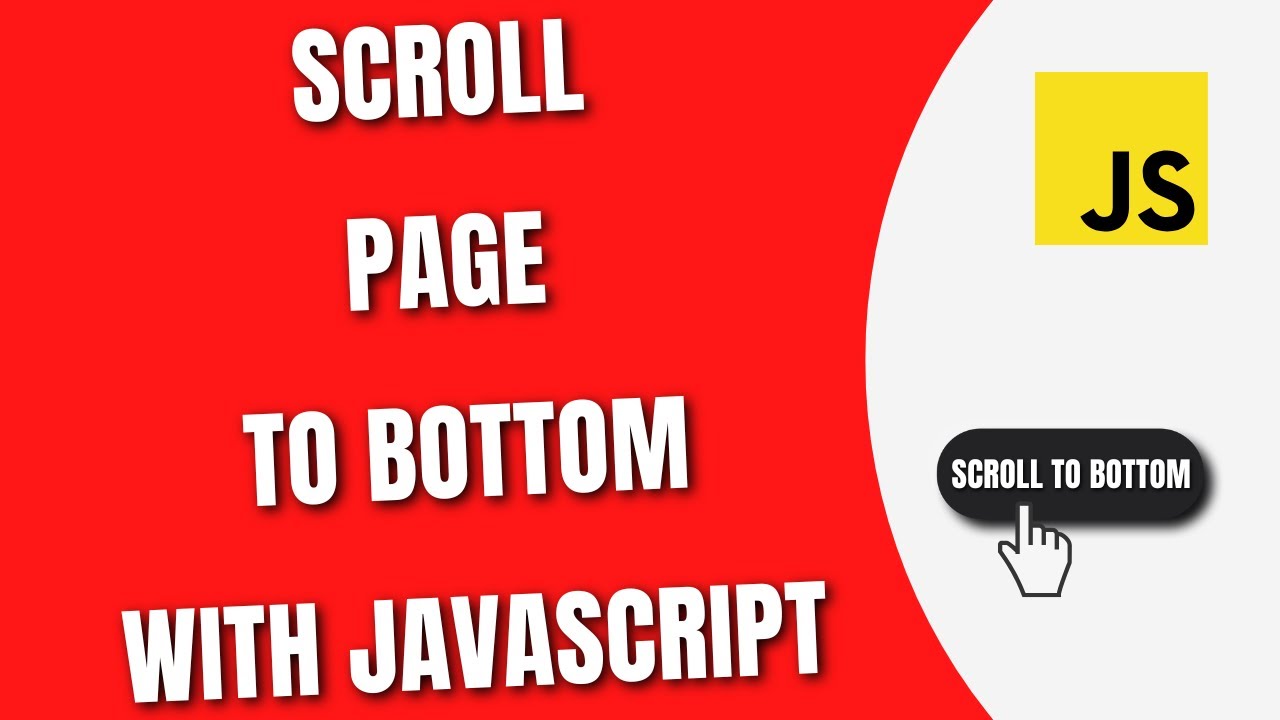
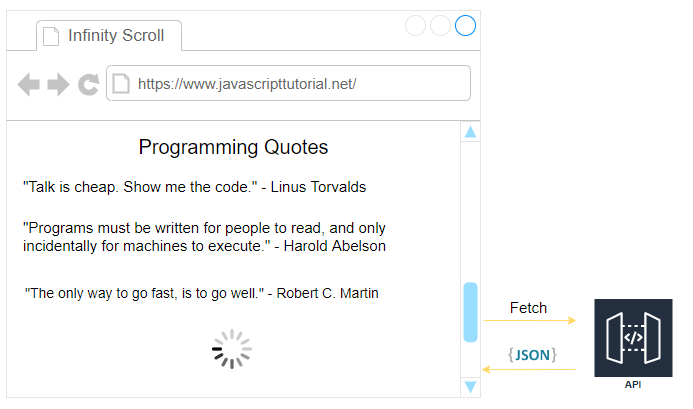
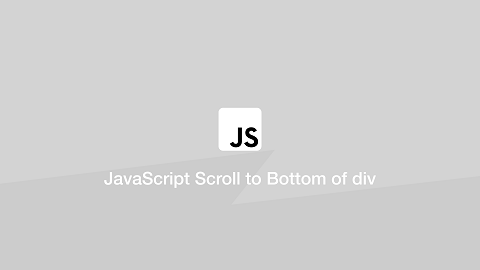

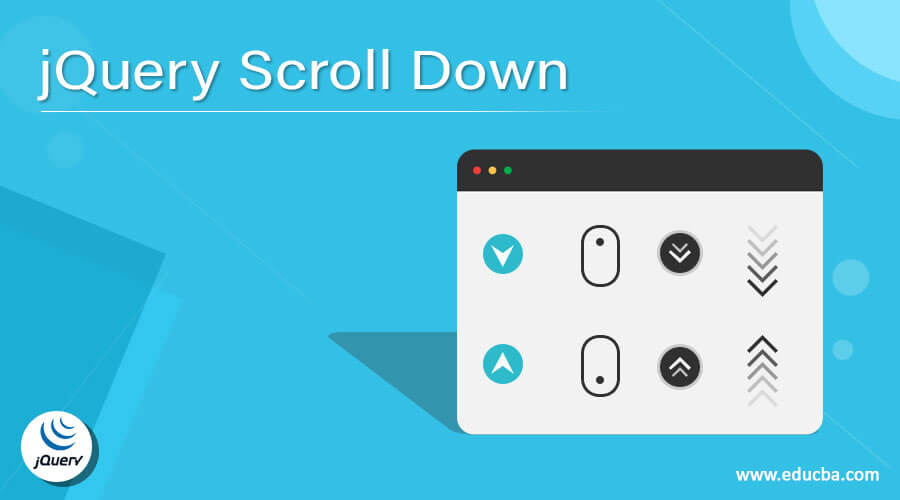

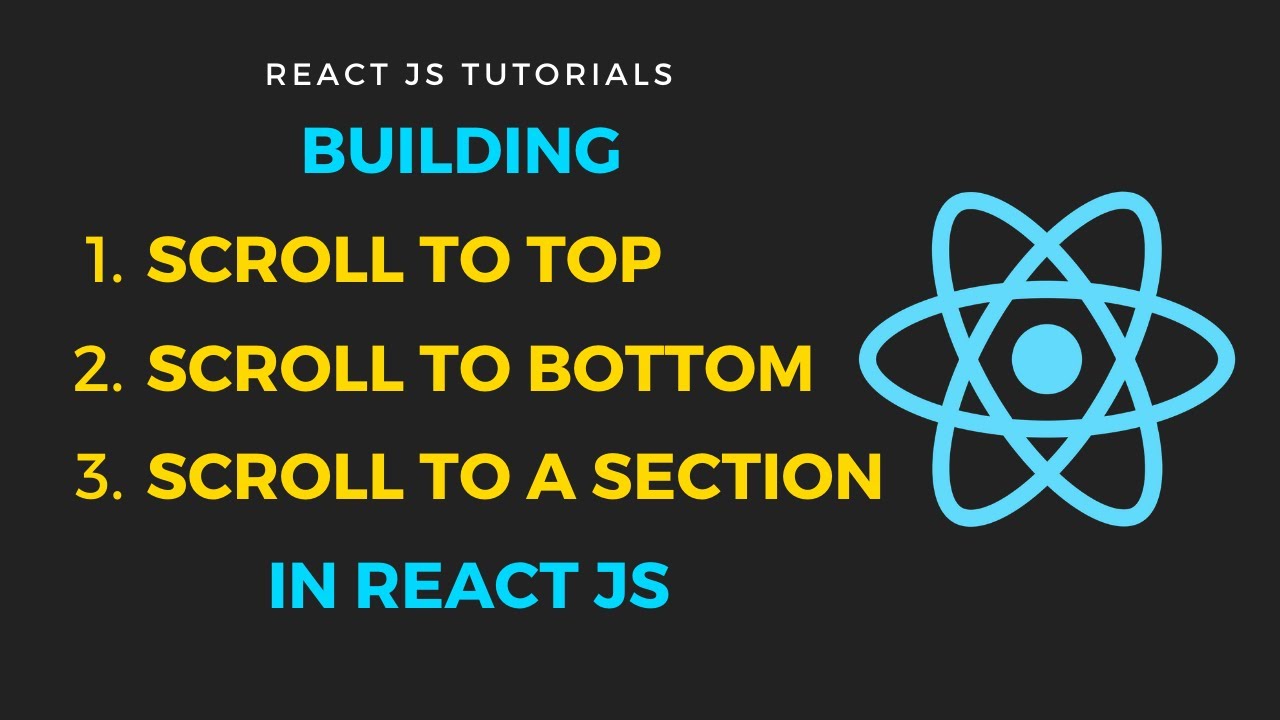
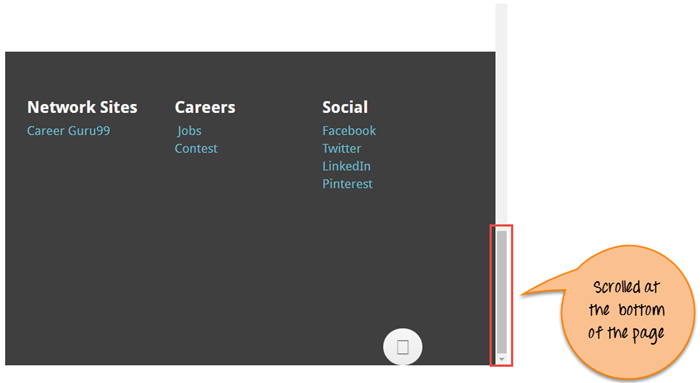
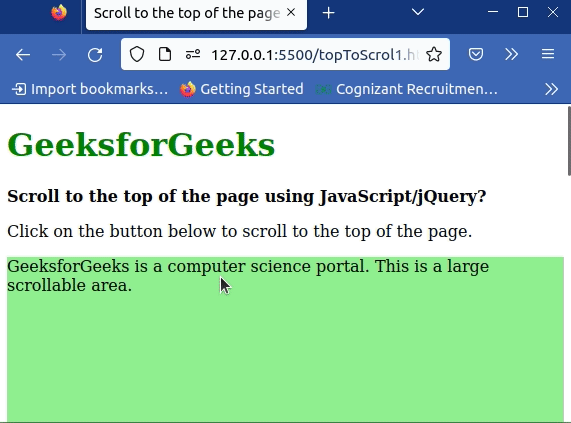

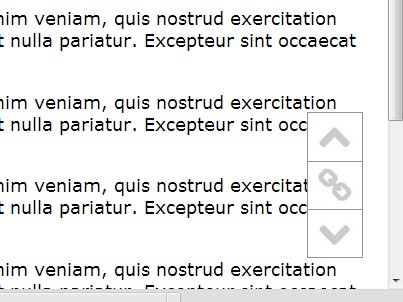
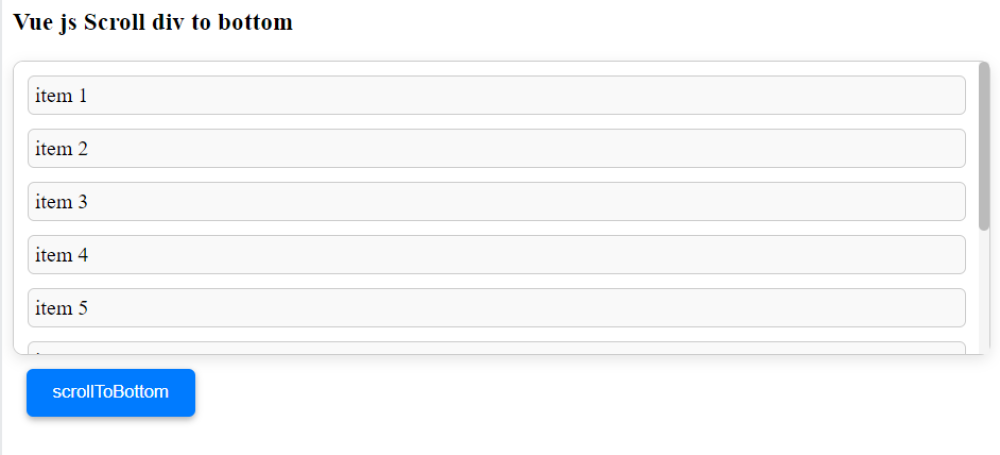


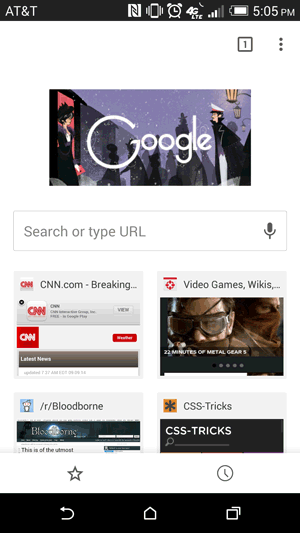

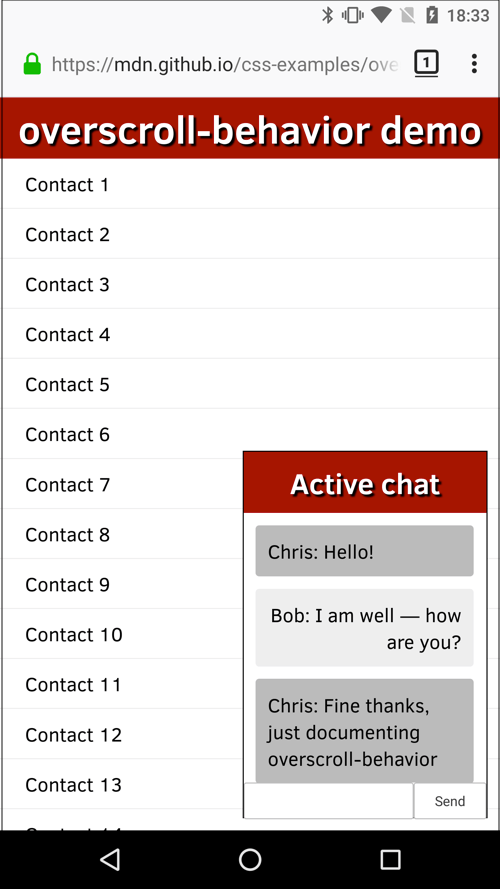
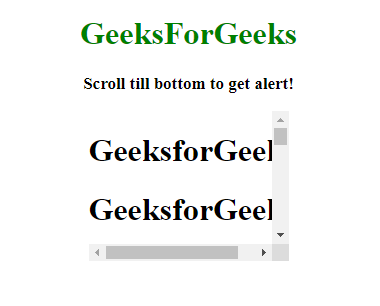

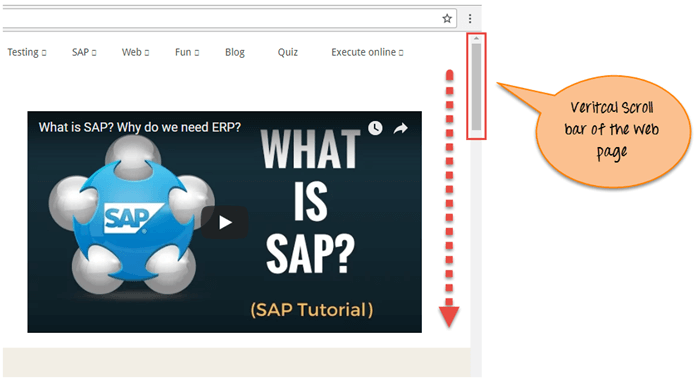

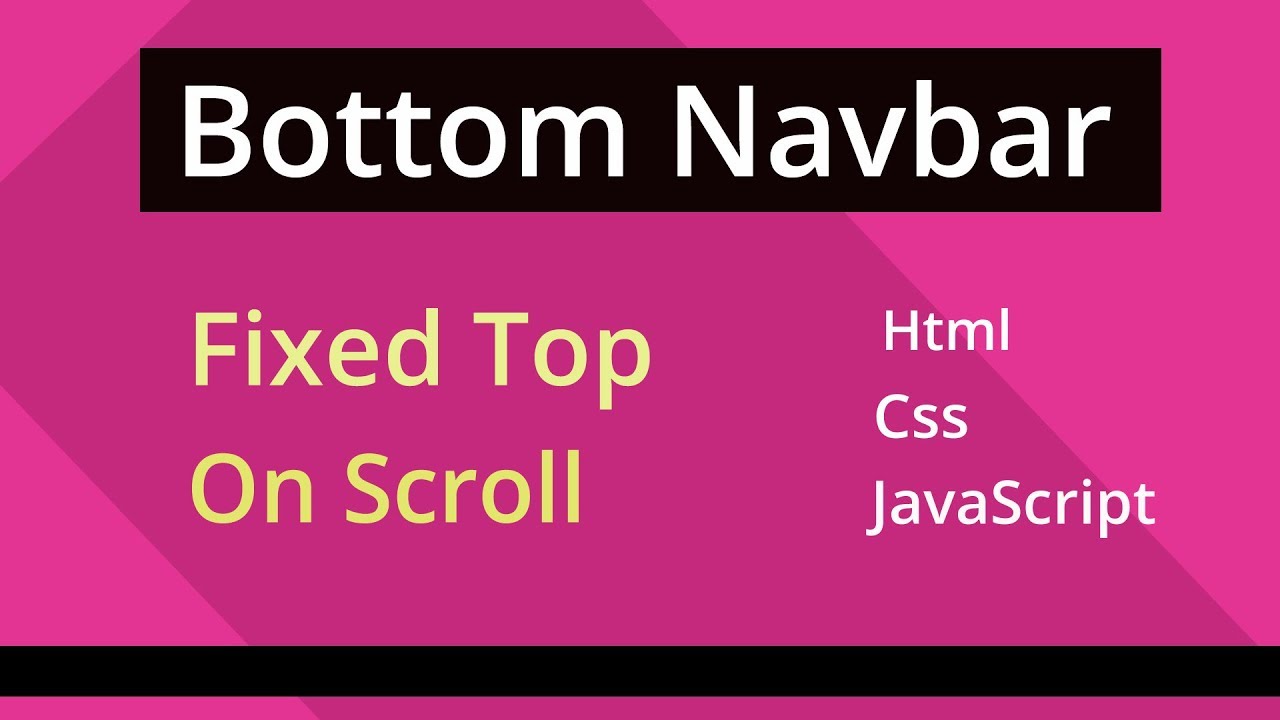



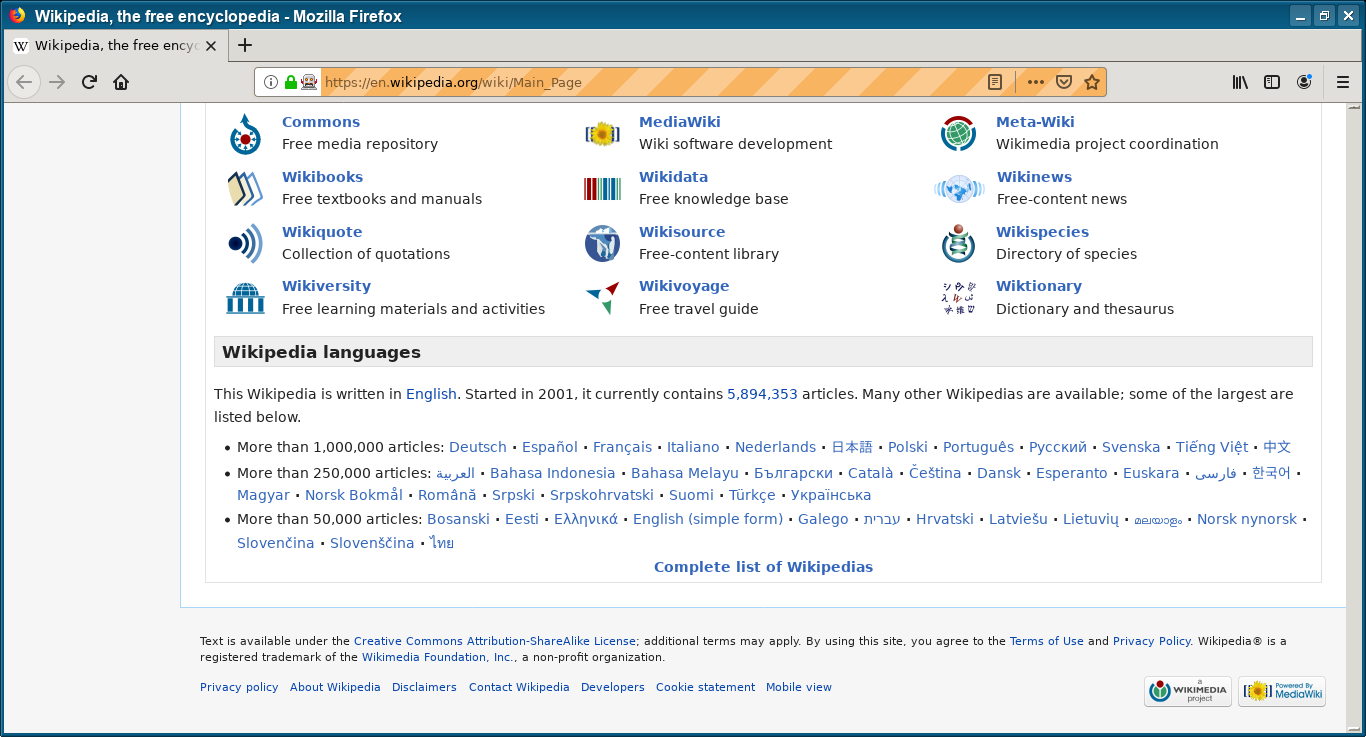

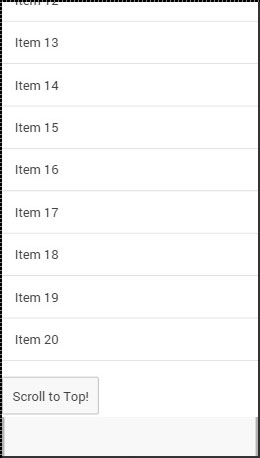
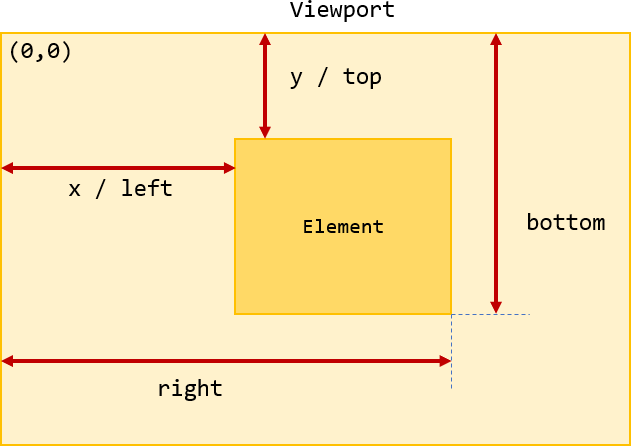
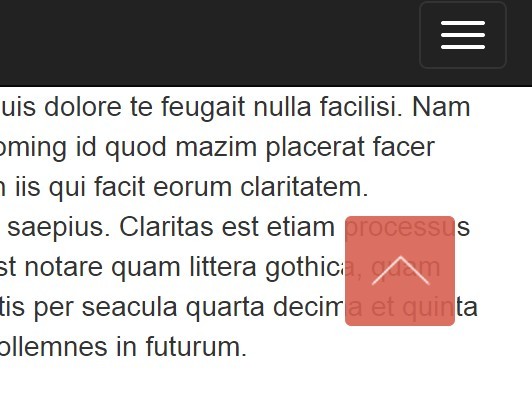

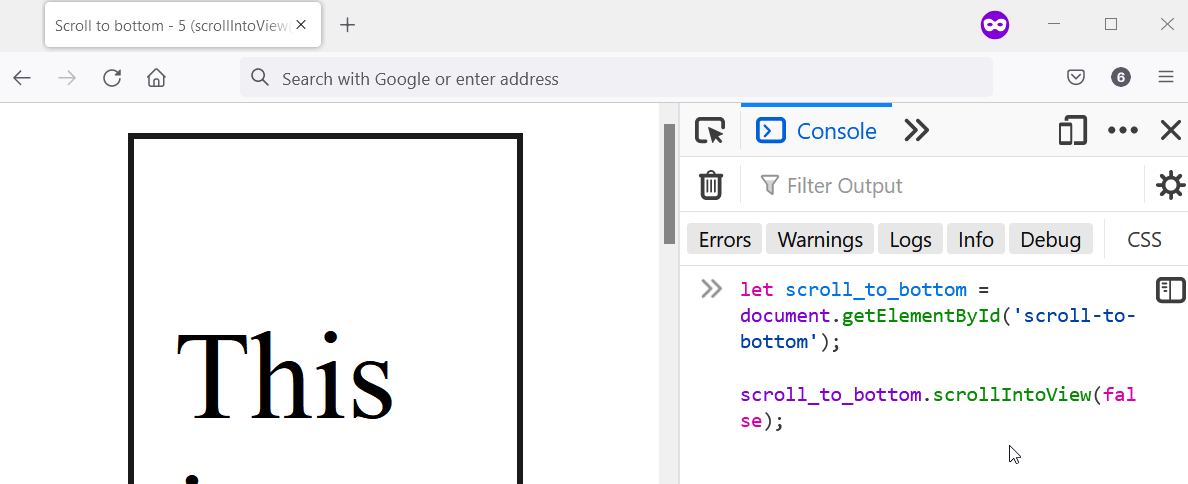
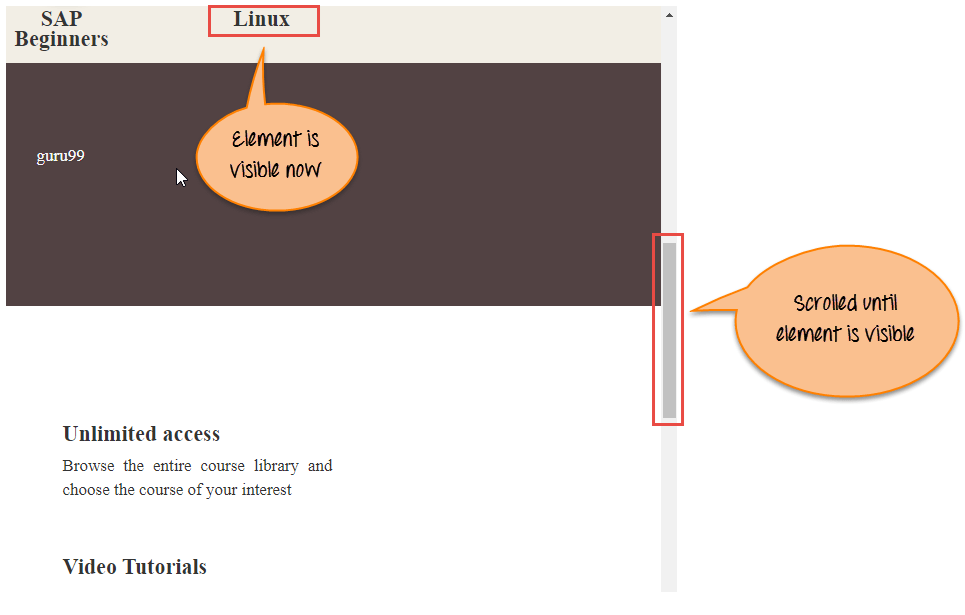
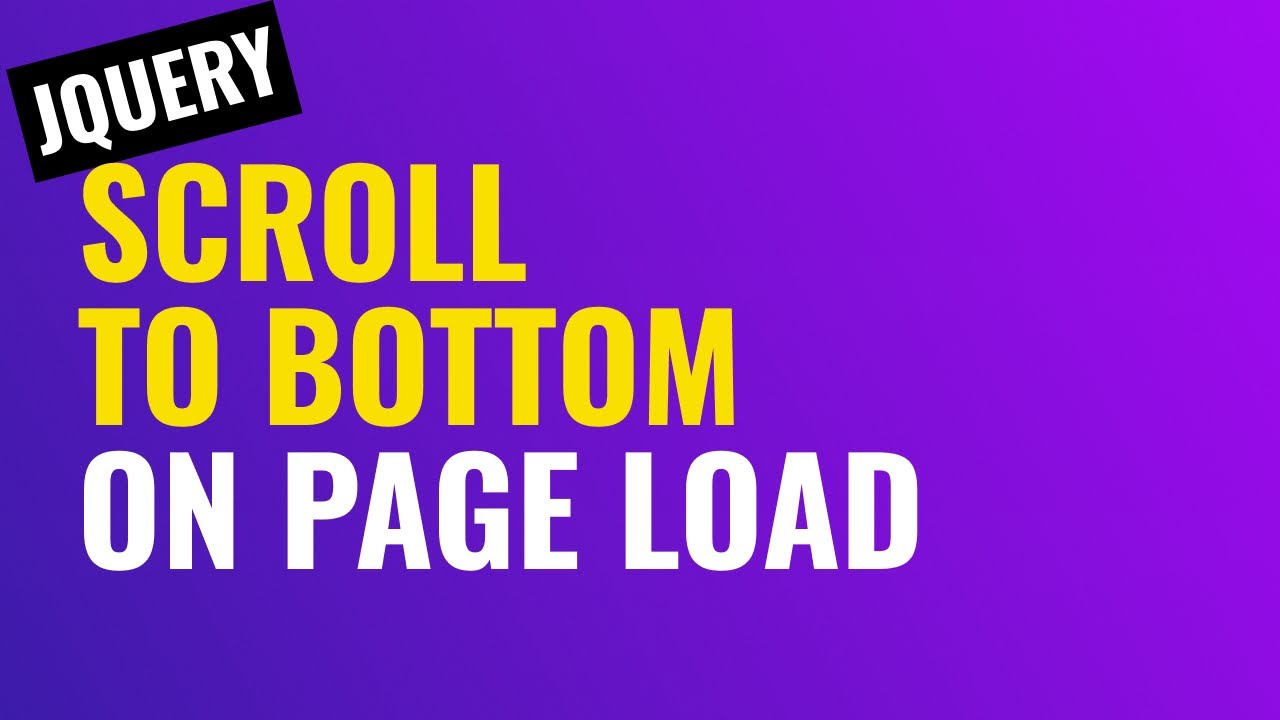
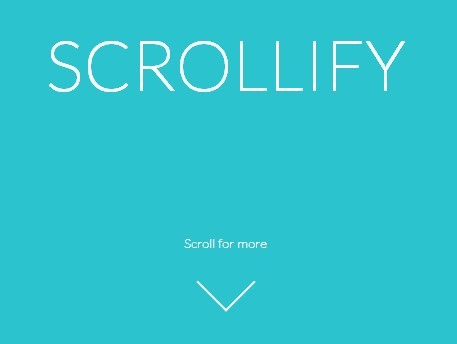

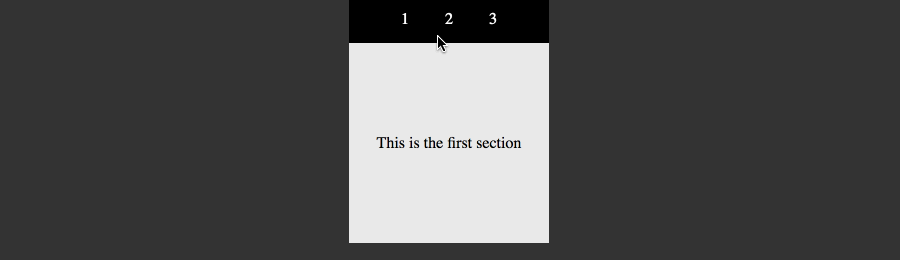
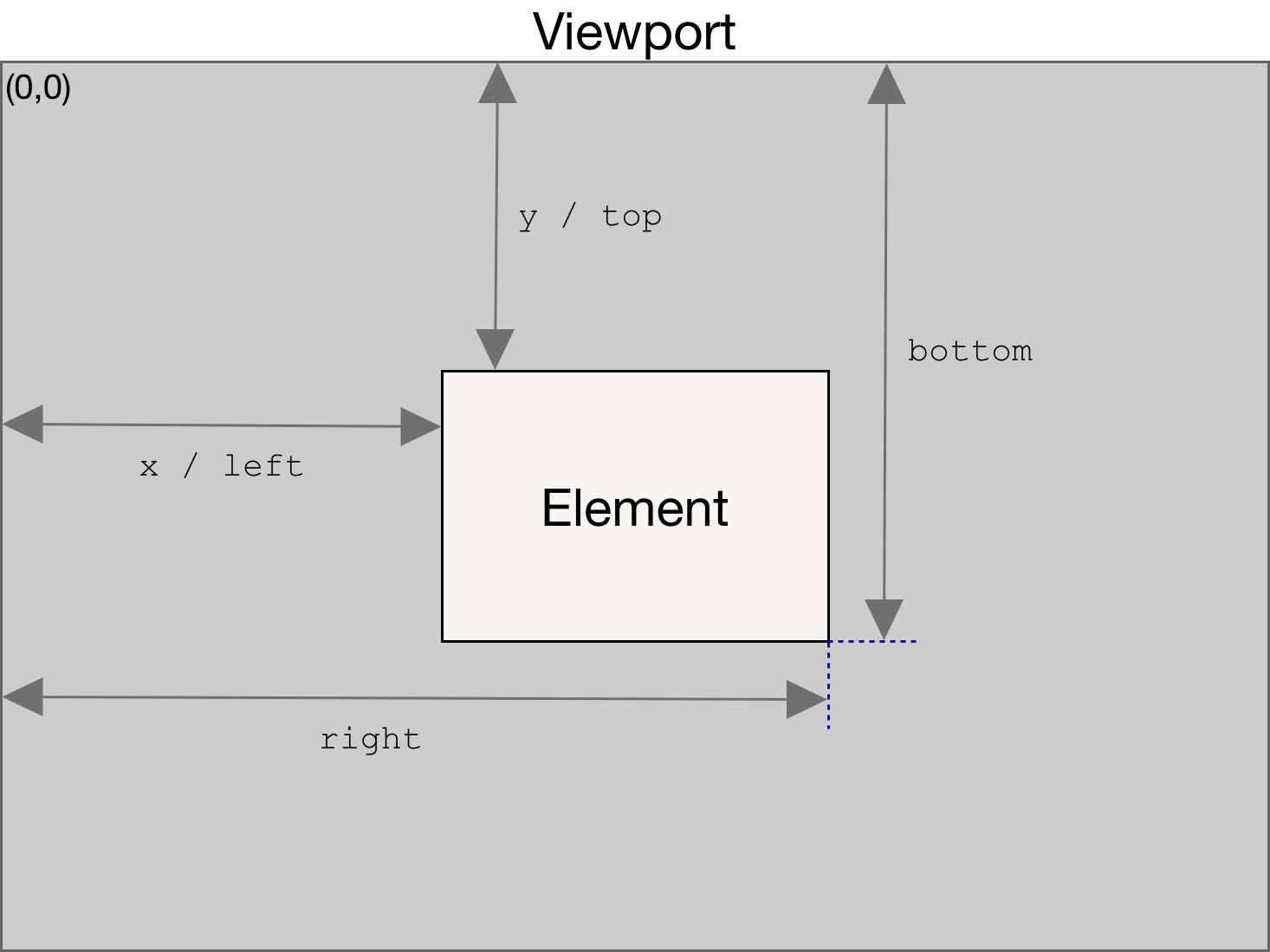
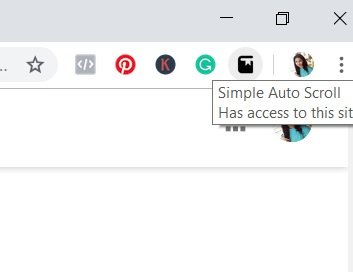

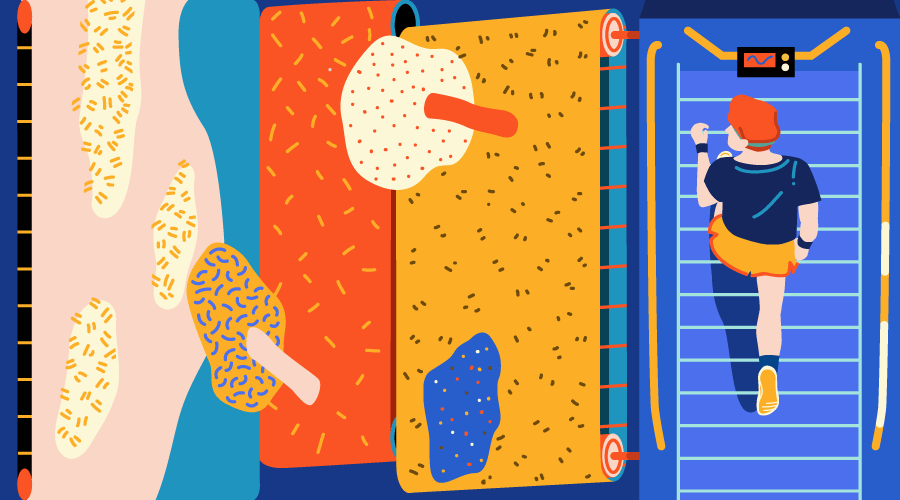
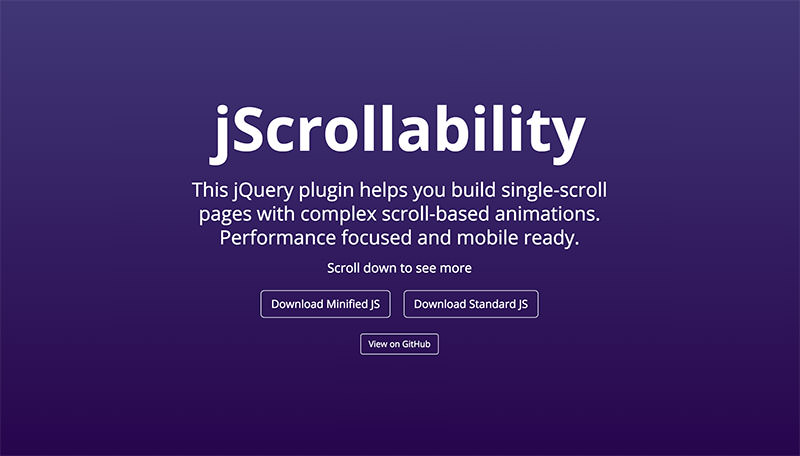
Article link: js scroll to bottom.
Learn more about the topic js scroll to bottom.
- Scroll Automatically to the Bottom of the Page – Stack Overflow
- JavaScript – scroll to bottom of element – Dirask
- How to Automatically Scroll to Bottom of Page in JS – Fedingo
- How to smooth scroll to the bottom of a page with JavaScript …
- JavaScript Scroll Page to Bottom – How To Code School
- How to Scroll to the Bottom of a Div Element in React
- Pin Scrolling to Bottom – CSS-Tricks
- How to Scroll to the bottom of a div in React – bobbyhadz
See more: https://nhanvietluanvan.com/luat-hoc/