Js Round To 2 Decimal Places
Understanding Numbers in JavaScript
Before we delve into rounding numbers, it is essential to have a basic understanding of how numbers are represented in JavaScript. JavaScript has two built-in number types: “number” for floating-point numbers and “BigInt” for integers with arbitrary precision. However, for the purpose of this article, we will focus on floating-point numbers.
Floating-point numbers in JavaScript are stored as 64-bit binary format using the IEEE 754 standard. This format allows precise representation of most decimal fractions, but it can sometimes lead to unexpected results due to the inherent limitations of binary representation. When performing operations with floating-point numbers, it is important to be mindful of potential rounding errors.
Rounding Numbers in JavaScript
JavaScript provides several methods for rounding numbers. The most commonly used methods are Math.round(), Math.ceil(), and Math.floor().
1. Math.round(): This method returns the value of a number rounded to the nearest integer. If the fractional part of the number is 0.5 or higher, the number is rounded up; otherwise, it is rounded down. To round a number to 2 decimal places, we can multiply the number by 100, use Math.round(), and then divide the result by 100.
2. Math.ceil(): This method returns the smallest integer greater than or equal to a given number. To round a number up to 2 decimal places, we can multiply the number by 100, use Math.ceil(), and then divide the result by 100.
3. Math.floor(): This method returns the largest integer less than or equal to a given number. To round a number down to 2 decimal places, we can multiply the number by 100, use Math.floor(), and then divide the result by 100.
Rounding to 2 Decimal Places in JavaScript
To round a number to 2 decimal places in JavaScript, we can use the following code snippet:
“`javascript
function roundToTwoDecimalPlaces(number) {
return Math.round(number * 100) / 100;
}
const roundedNumber = roundToTwoDecimalPlaces(3.14159);
console.log(roundedNumber); // Output: 3.14
“`
In this example, the roundToTwoDecimalPlaces() function multiplies the input number by 100, uses the Math.round() method to round it to the nearest integer, and then divides the result by 100 to obtain the rounded number with two decimal places.
Examples of Rounding Numbers in JavaScript
Now, let’s explore some examples of rounding numbers in JavaScript using different methods:
1. Using Math.round():
“`javascript
const number = 3.14159;
const roundedNumber = Math.round(number * 100) / 100;
console.log(roundedNumber); // Output: 3.14
“`
2. Using Math.ceil():
“`javascript
const number = 3.14159;
const roundedNumber = Math.ceil(number * 100) / 100;
console.log(roundedNumber); // Output: 3.15
“`
3. Using Math.floor():
“`javascript
const number = 3.14159;
const roundedNumber = Math.floor(number * 100) / 100;
console.log(roundedNumber); // Output: 3.14
“`
These examples demonstrate how to round numbers to 2 decimal places using the Math.round(), Math.ceil(), and Math.floor() methods.
FAQs
1. What is JavaScript?
JavaScript is a programming language used for developing interactive web elements and applications. It is widely supported by web browsers and enables the creation of dynamic and responsive web pages.
2. How do I round a number to 2 decimal places in JavaScript?
To round a number to 2 decimal places in JavaScript, you can use the Math.round(), Math.ceil(), or Math.floor() methods. Multiply the number by 100, apply the desired rounding method, and then divide the result by 100.
3. Can I round a number to 2 decimal places using jQuery?
Yes, you can use jQuery to round a number to 2 decimal places by utilizing JavaScript’s rounding methods, such as Math.round(), Math.ceil(), or Math.floor(). jQuery itself does not provide specific rounding functions.
4. Can I round a number to 2 decimal places in Java?
Yes, you can round a number to 2 decimal places in Java by using the DecimalFormat class or by manipulating the number using mathematical operations. The specific approach may vary depending on the programming context.
5. How can I round a number to 1 decimal place in JavaScript?
To round a number to 1 decimal place in JavaScript, you can modify the code snippet provided earlier to multiply and divide by 10 instead of 100:
“`javascript
function roundToOneDecimalPlace(number) {
return Math.round(number * 10) / 10;
}
const roundedNumber = roundToOneDecimalPlace(3.14159);
console.log(roundedNumber); // Output: 3.1
“`
6. How can I round a number to 4 decimal places in JavaScript?
To round a number to 4 decimal places in JavaScript, you can modify the code snippet provided earlier to multiply and divide by 10000 instead of 100:
“`javascript
function roundToFourDecimalPlaces(number) {
return Math.round(number * 10000) / 10000;
}
const roundedNumber = roundToFourDecimalPlaces(3.14159);
console.log(roundedNumber); // Output: 3.1416
“`
In conclusion, JavaScript provides several methods for rounding numbers to a specific decimal place. Whether you need to round to 2 decimal places, 1 decimal place, or any other precision, the Math.round(), Math.ceil(), and Math.floor() methods can help you achieve accurate results. Additionally, understanding the inherent limitations of floating-point numbers in JavaScript is crucial to avoid unexpected rounding errors.
How To Round To 2 Decimal Places | Math With Mr. J
Keywords searched by users: js round to 2 decimal places Math round 2 decimal JavaScript, math.round 2 decimal, Math round up JavaScript, Math floor to 2 decimal places, Jquery round to 2 decimal, Java round to 2 decimal places, Js round to 1 decimal, Round number to 4 decimal places javascript
Categories: Top 95 Js Round To 2 Decimal Places
See more here: nhanvietluanvan.com
Math Round 2 Decimal Javascript
Introduction:
In the world of programming, mathematical operations play a crucial role. Whether you are dealing with financial calculations, data analysis, or simple calculations, accuracy is key. JavaScript, being one of the most popular programming languages on the web, provides a powerful built-in function, Math.round(), that allows developers to round numbers to a specific decimal place. In this article, we will explore the Math.round() function in JavaScript and dive into its usage and capabilities. So, let’s begin!
What is the Math.round() function in JavaScript?
The Math.round() function in JavaScript is a built-in mathematical function that is used to round any numeric value to the nearest whole number. This function takes a single parameter, the number that needs to be rounded, and returns the rounded value. However, when it comes to rounding numbers to a specific decimal place, a little more effort is required.
Rounding to 2 Decimal Places:
By default, the Math.round() function does not allow direct rounding to a specific decimal place. But fear not, we can achieve rounding to a specific number of decimal places by combining the Math.round() function with some simple mathematical operations.
Here’s an example to round a number to 2 decimal places using Math.round():
“`javascript
function roundToTwoDecimalPlaces(number) {
return Math.round(number * 100) / 100;
}
// Usage
const roundedNumber = roundToTwoDecimalPlaces(5.6789);
console.log(roundedNumber); // Output: 5.68
“`
In the example above, we multiply the number by 100 to shift the decimal places two positions to the right. After rounding the resulting value to the nearest whole number using the Math.round() function, we divide it by 100 to shift the decimal places back to their original position.
This approach allows us to round numbers to any desired decimal places. For instance, to round a number to 3 decimal places, we would multiply and divide by 1000 instead.
Frequently Asked Questions (FAQs):
Q1. Can Math.round() be used to round negative numbers?
A1. Yes, the Math.round() function is capable of rounding negative numbers. It will round the negative numbers towards zero, so -0.5 will be rounded to -0, while -0.6 will be rounded to -1.
Q2. How does Math.round() handle rounding if the fractional part is exactly 0.5?
A2. When the fractional part of the number is exactly 0.5, Math.round() rounds the number to the nearest even whole number. This is commonly known as “round-half-to-even” or “banker’s rounding” and helps maintain statistical accuracy.
Q3. Can Math.round() be used to round numbers to an arbitrary number of decimal places?
A3. Yes, the approach demonstrated earlier allows us to round numbers to any desired decimal places. By multiplying and dividing by the appropriate power of 10, we can achieve rounding to a specific number of decimal places.
Q4. Are there any other rounding methods available in JavaScript?
A4. Yes, apart from Math.round(), JavaScript also provides Math.ceil() and Math.floor() functions for rounding up and down, respectively, to the nearest whole number. However, these functions do not directly support rounding to a specific number of decimal places like Math.round().
Q5. Is there a performance impact of using Math.round() with arithmetic operations for rounding to decimal places?
A5. While using arithmetic operations causes slight performance overhead, it is generally negligible. Modern JavaScript engines are optimized to handle such mathematical operations efficiently, and the performance impact is rarely noticeable unless executed in extremely large computations.
Conclusion:
Math.round() in JavaScript is a powerful tool when it comes to rounding numbers. While it may not directly provide the ability to round to a specific number of decimal places, we can achieve this by combining the Math.round() function with simple mathematical operations. By understanding how to implement these rounding techniques, developers can accurately handle decimal places in JavaScript. So next time you encounter the need to round numbers to 2 decimal places or any other specific decimal place, you’ll have the knowledge to do so effectively and efficiently.
Math.Round 2 Decimal
Have you ever come across decimal numbers and wished to simplify them for easier understanding or presentation? Perhaps you are a student struggling to round your answers to the required precision, or maybe you are a professional dealing with financial figures that need to be expressed in a more concise format. In either case, the Math.Round function is the perfect tool to help you achieve your goal.
Understanding Math.Round:
Math.Round is a built-in mathematical function available in various programming languages, including but not limited to C#, Java, and JavaScript. Its primary purpose is to round a given value to the nearest specified number of decimal places. When using Math.Round, the number to be rounded is passed as an argument, along with the desired precision.
Syntax: Math.Round(number, precision)
To round a number to two decimal places, the precision argument should be set to 2. Math.Round takes into consideration the decimal places beyond the intended precision and adjusts the number accordingly. When the digit following the precision is 5 or higher, the function rounds the number up. Conversely, if the digit following the precision is 4 or lower, the function rounds the number down.
Example:
Math.Round(3.14159265, 2)
In this case, the number 3.14159265 is rounded to the nearest two decimal places. As the third decimal place is 1 (less than 5), the value is rounded down to 3.14, reflecting the desired precision.
Applying Math.Round:
Math.Round finds application in a variety of fields, particularly where precision is crucial, such as finance, engineering, and scientific research. Here are some common scenarios where Math.Round can be a valuable asset:
1. Financial calculations: When calculating financial figures, such as interest rates or currency conversions, it is often necessary to round to fewer decimal places. Math.Round allows financial professionals to present the numbers in a more concise manner that is easier to comprehend and work with.
2. Grade calculations: For teachers grading assignments or exams, it is prudent to round the scores to a specified number of decimal places. This ensures consistency and fairness among students and simplifies comparisons between different assessments.
3. Statistical analysis: Statistical calculations often generate results with an excess number of decimal places. By rounding to the appropriate precision, researchers can enhance data interpretation without sacrificing accuracy.
4. User interface: In software development, Math.Round is commonly used to manipulate and format numerical inputs for better user experience. For instance, an e-commerce website may display prices rounded to two decimal places to provide customers with clear and concise information.
Frequently Asked Questions (FAQs):
Q1: Can Math.Round be used to round numbers to more than two decimal places?
Yes, Math.Round can round numbers to any specified number of decimal places. To round a number to three decimal places, for instance, the precision argument should be set to 3. Keep in mind that rounding to excessively large decimal places may not be meaningful or necessary in most scenarios.
Q2: How does Math.Round handle negative numbers?
Math.Round handles negative numbers just like positive numbers. It considers the digit following the specified precision and rounds the number based on that digit. For instance, Math.Round(-2.58643, 3) rounds to -2.586, as the fourth decimal place is less than 5.
Q3: Are there any other rounding methods available besides Math.Round?
Yes, there are alternative rounding methods depending on your requirements. Math.Floor always rounds down to the nearest whole number, while Math.Ceiling always rounds up. Additionally, Math.Truncate discards the decimal portion entirely, which differs from rounding where the digit following the precision determines whether to round up or down.
Q4: Can Math.Round introduce errors or inaccuracies?
Math.Round, when used correctly, introduces negligible errors or inaccuracies. However, it is important to be aware of the concept of significant figures. Rounding can impact the precision of calculations, so it is crucial to understand the context in which rounding is being applied and its potential impact on subsequent calculations or data analysis.
In conclusion, the Math.Round function provides a convenient and efficient way to round numbers to a specified number of decimal places. Whether you are a student trying to meet the requirements of an assignment or a professional seeking clearer presentation of figures, Math.Round is a versatile tool that guarantees accuracy and simplicity. By familiarizing yourself with its syntax and applications, you too can harness the power of Math.Round in your mathematical endeavors.
Images related to the topic js round to 2 decimal places
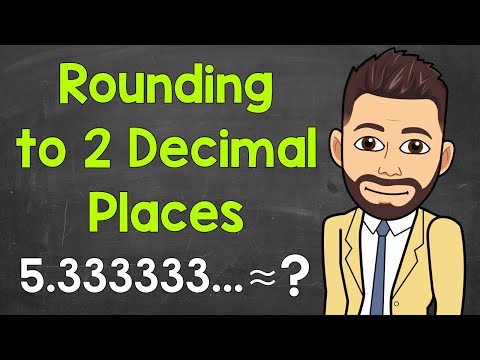
Found 21 images related to js round to 2 decimal places theme
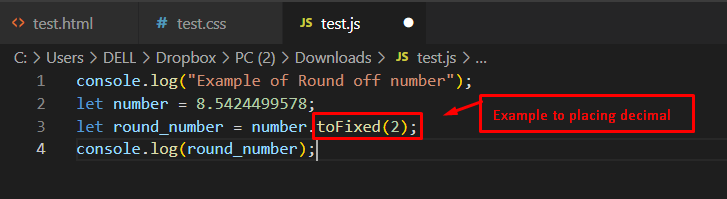
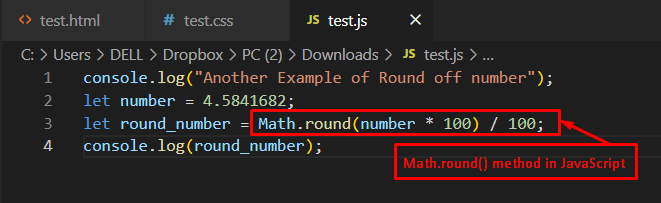
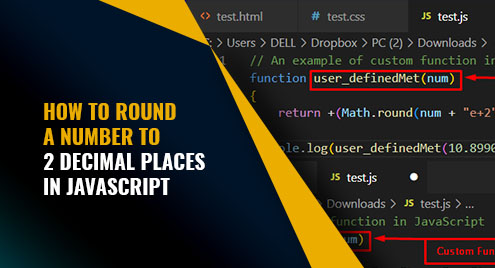
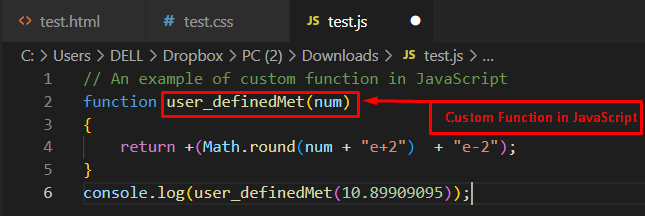
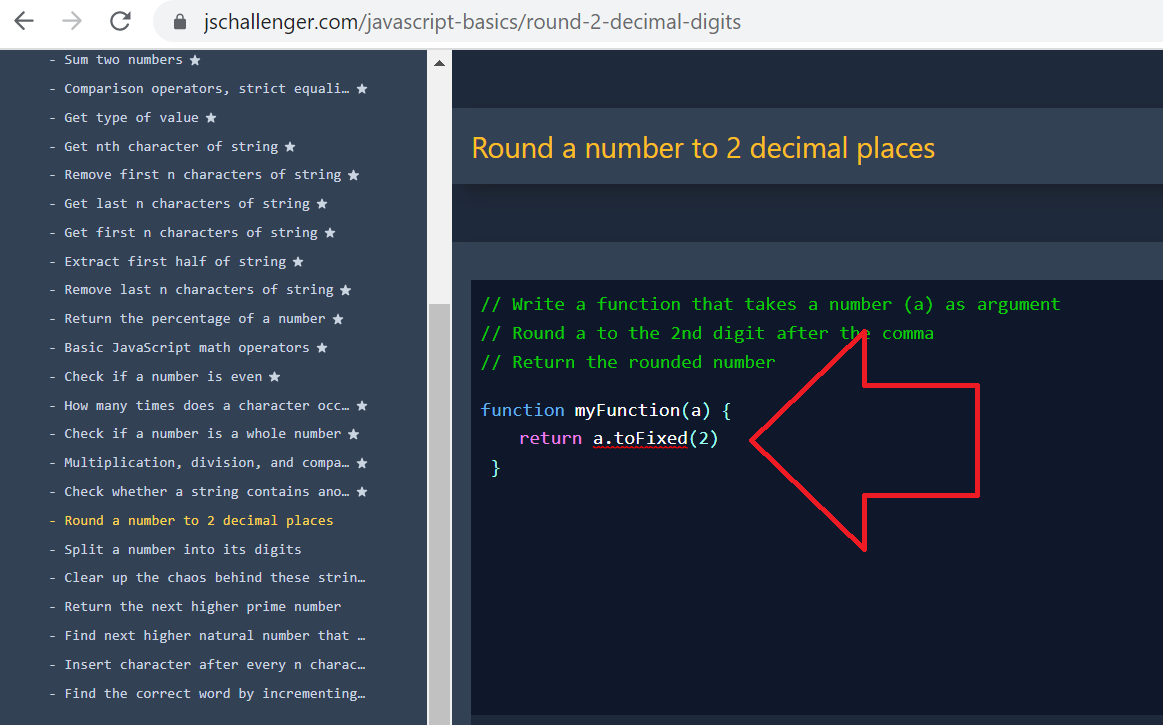
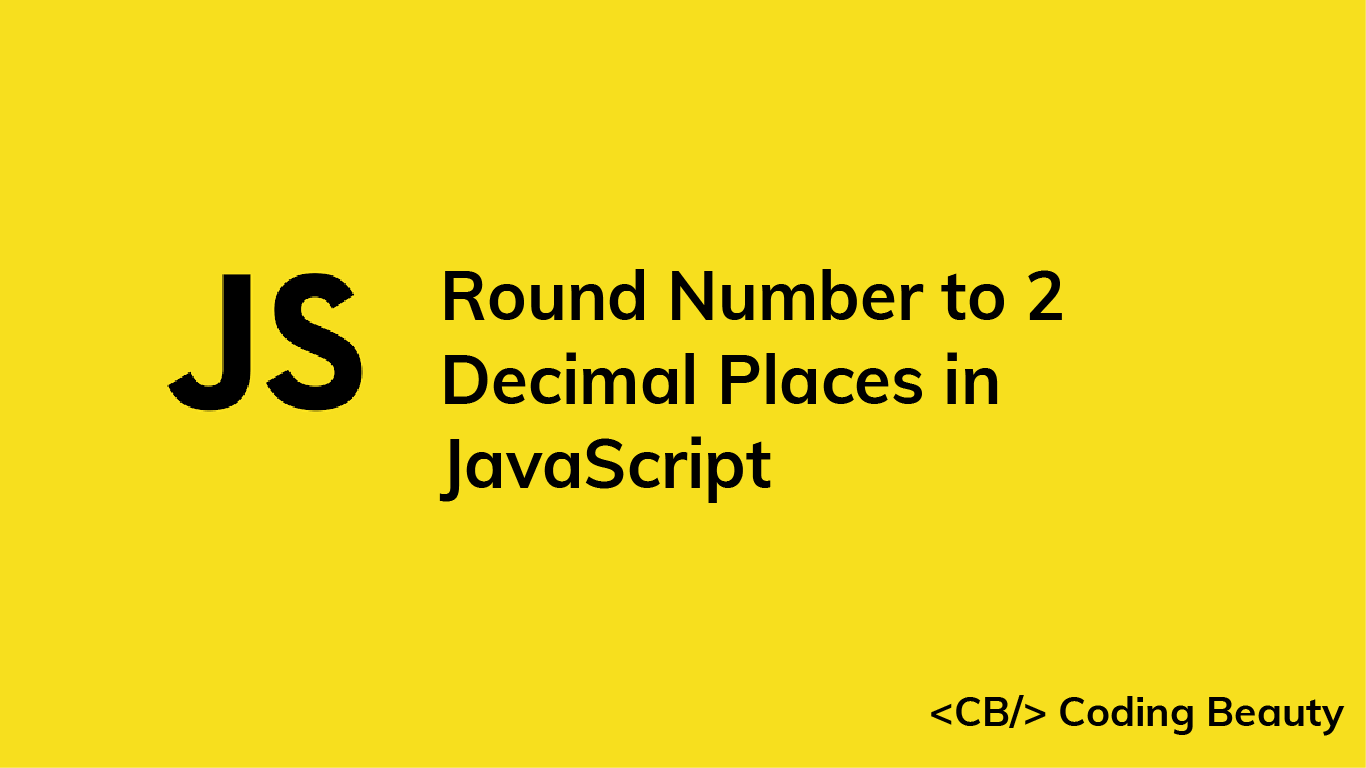
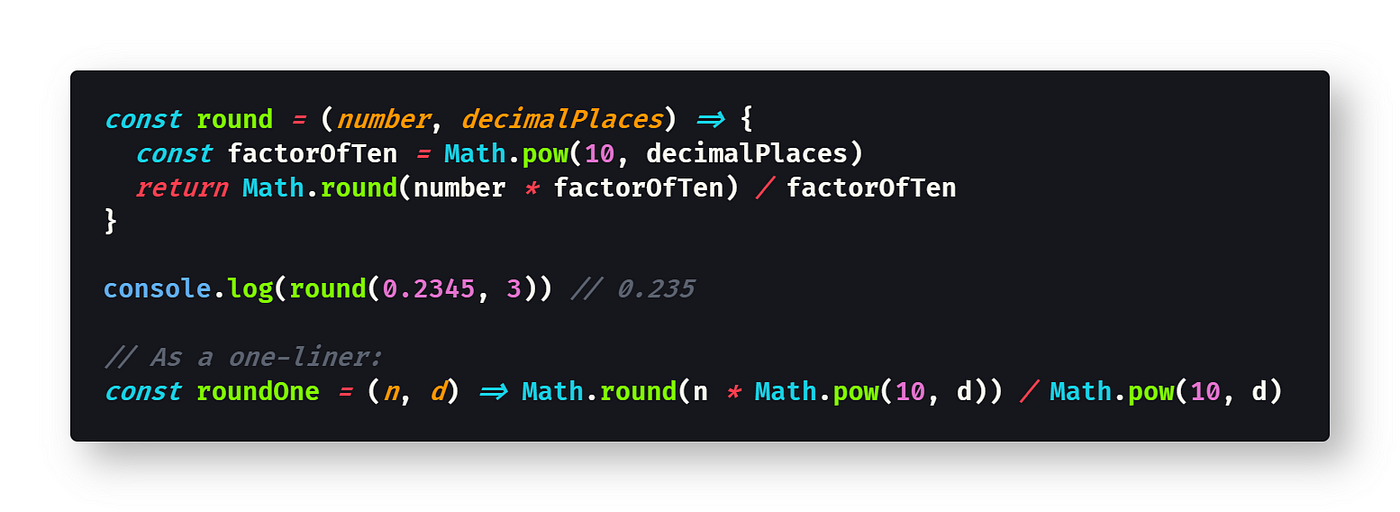
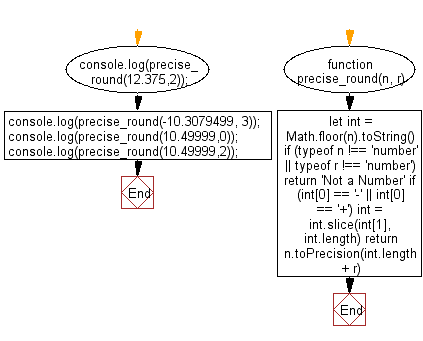
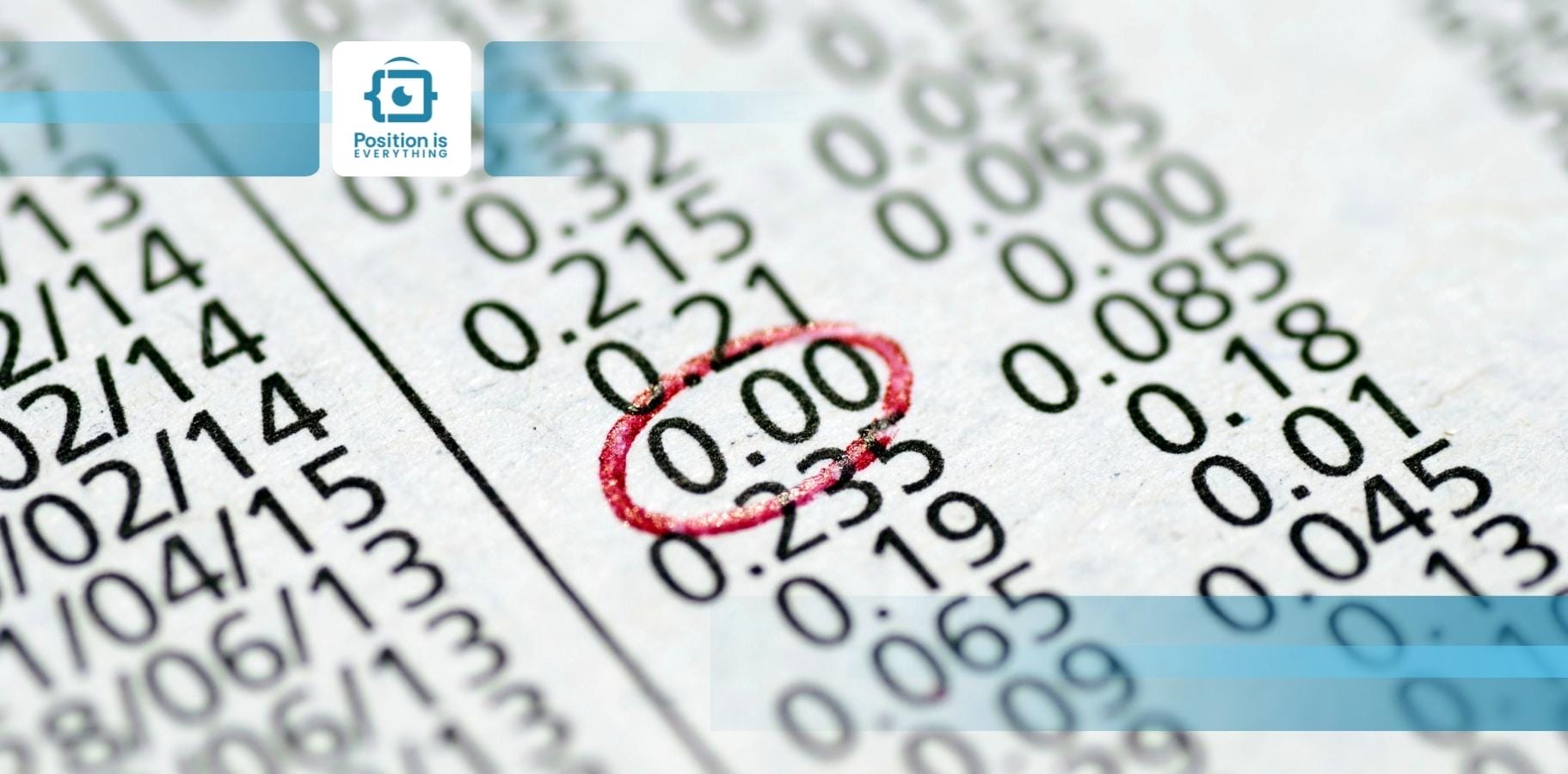
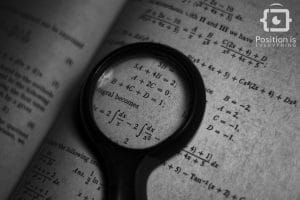

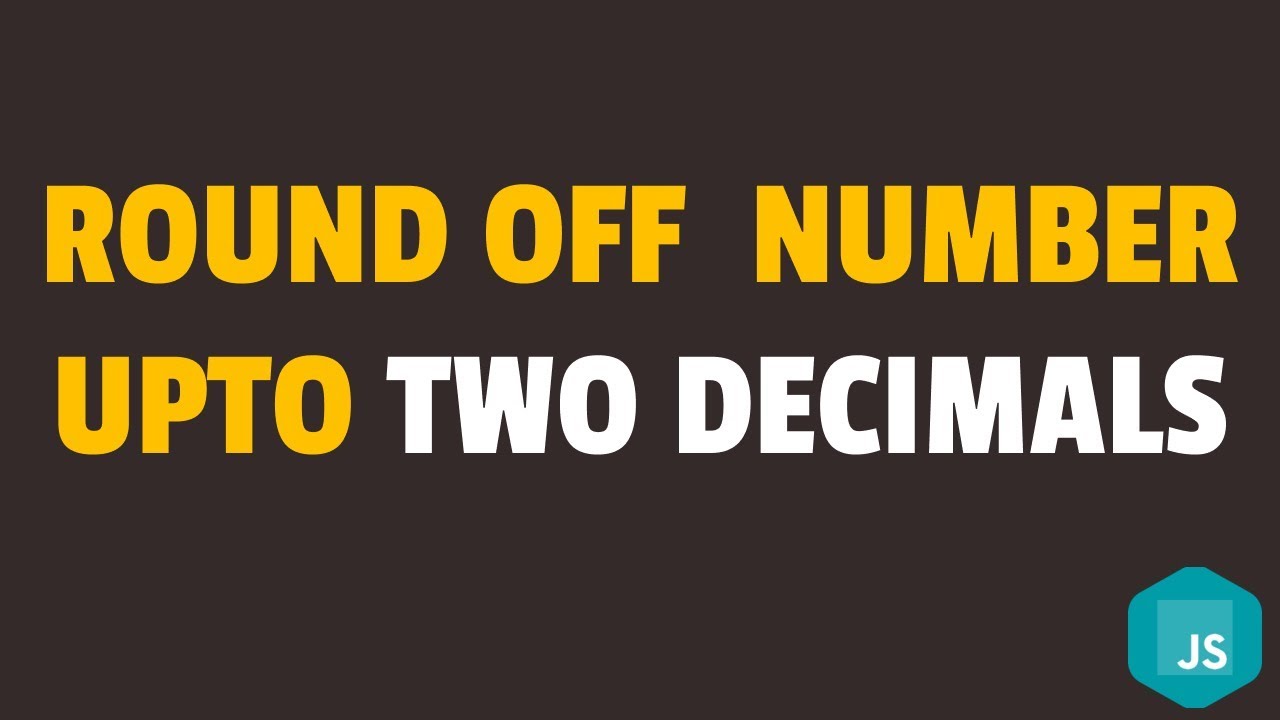

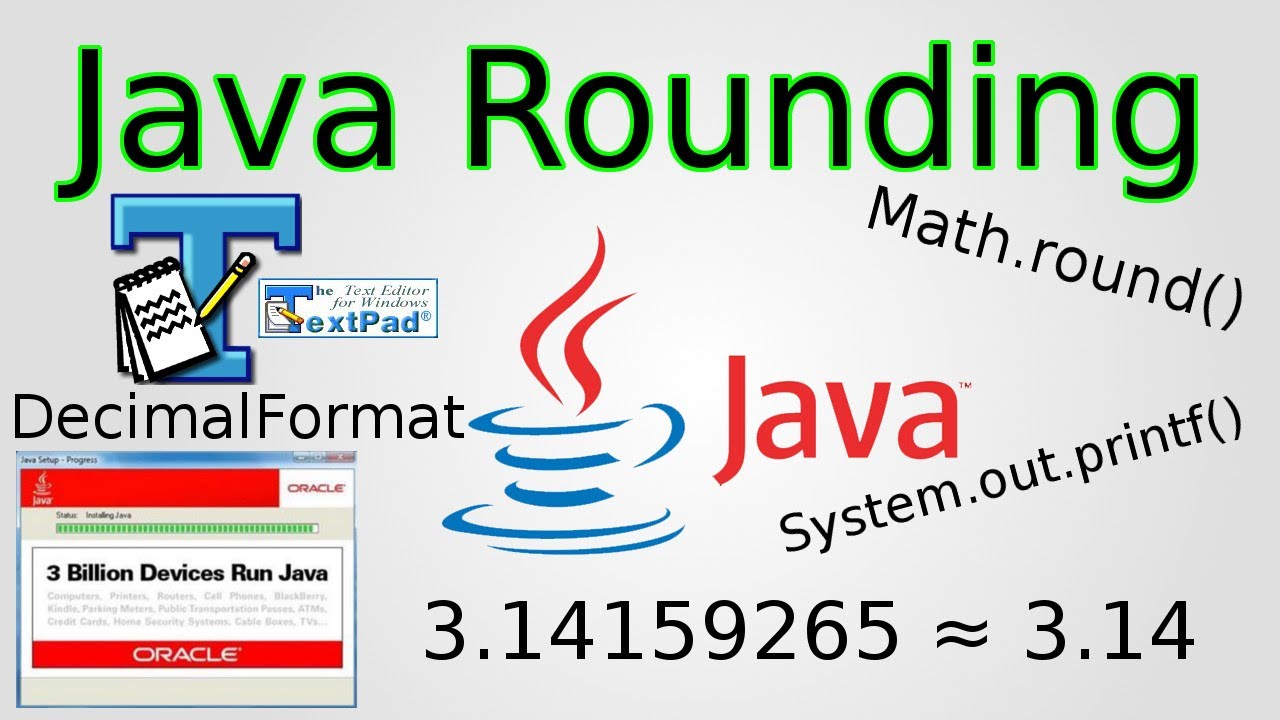
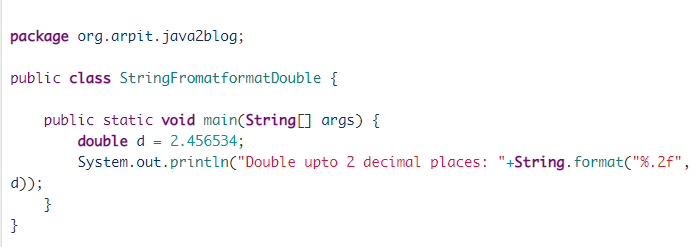

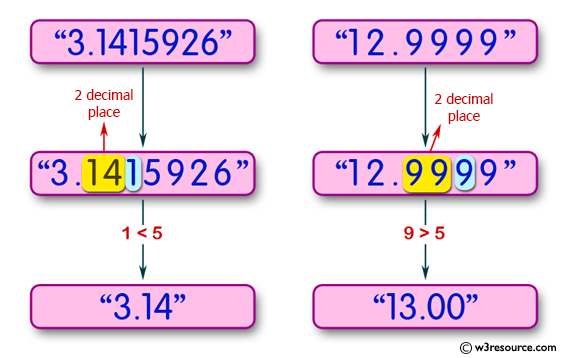
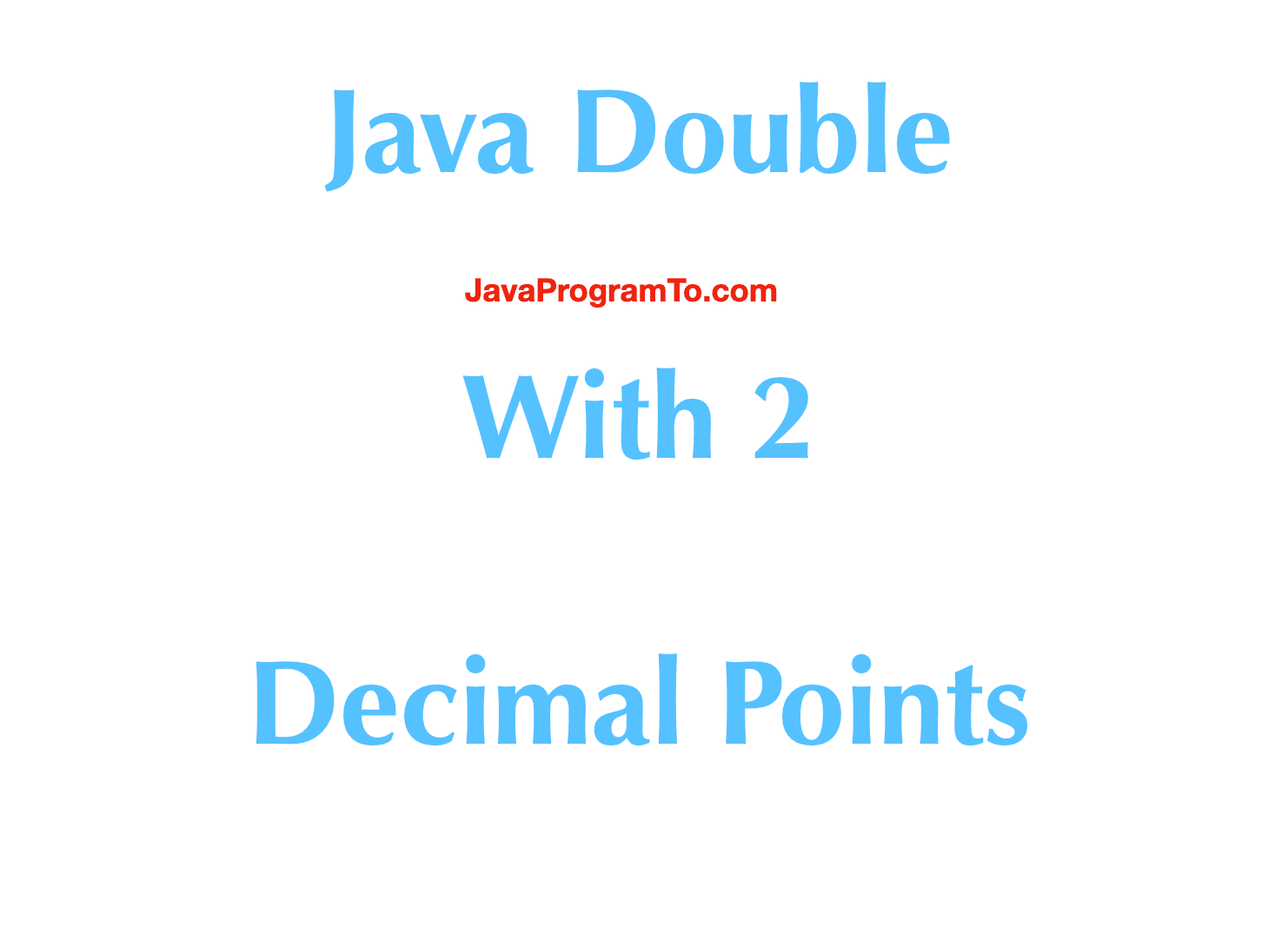
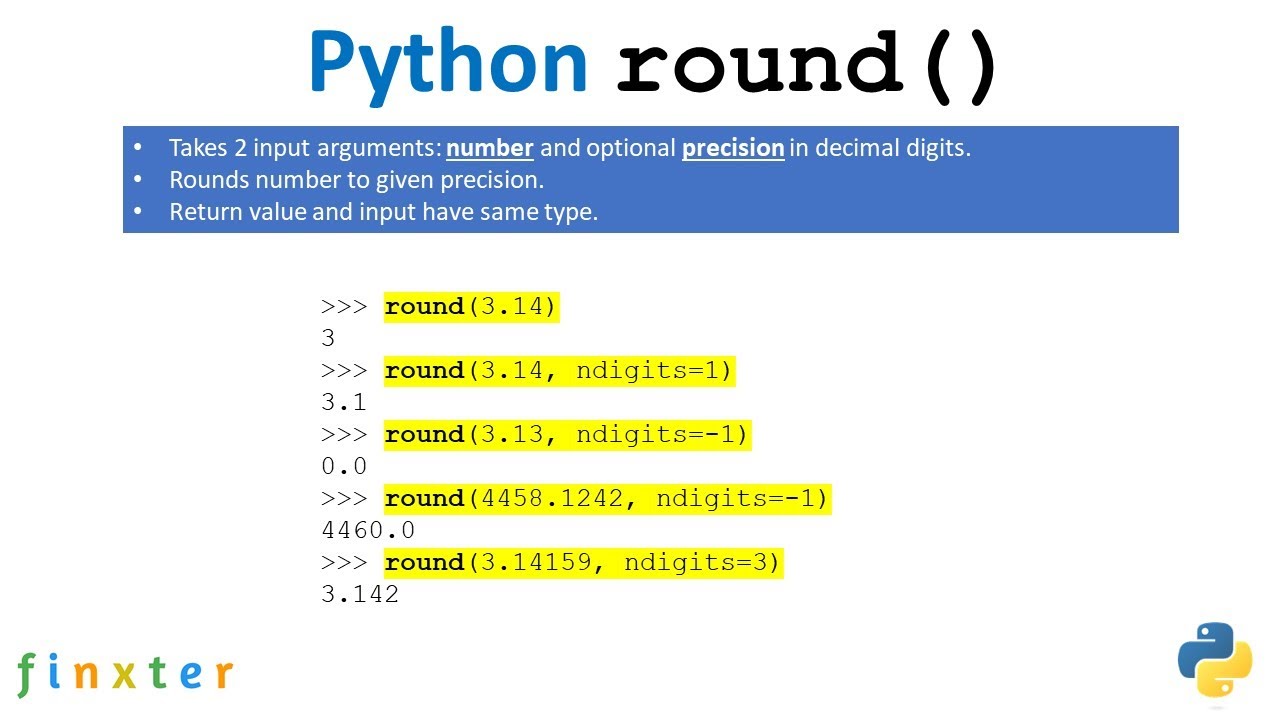

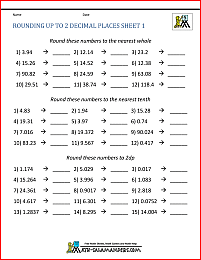

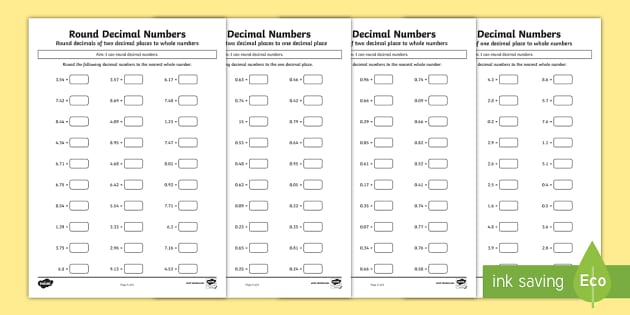
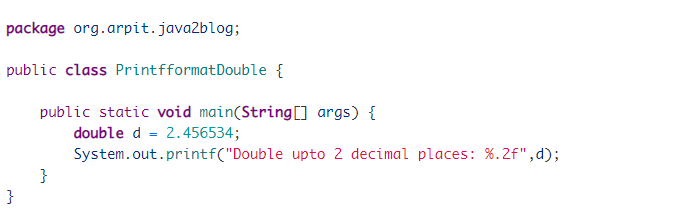
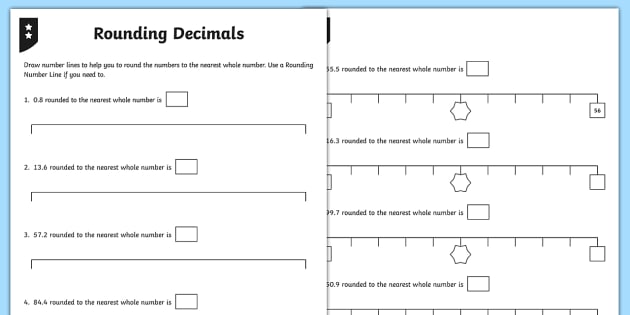







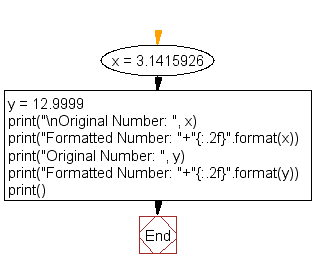
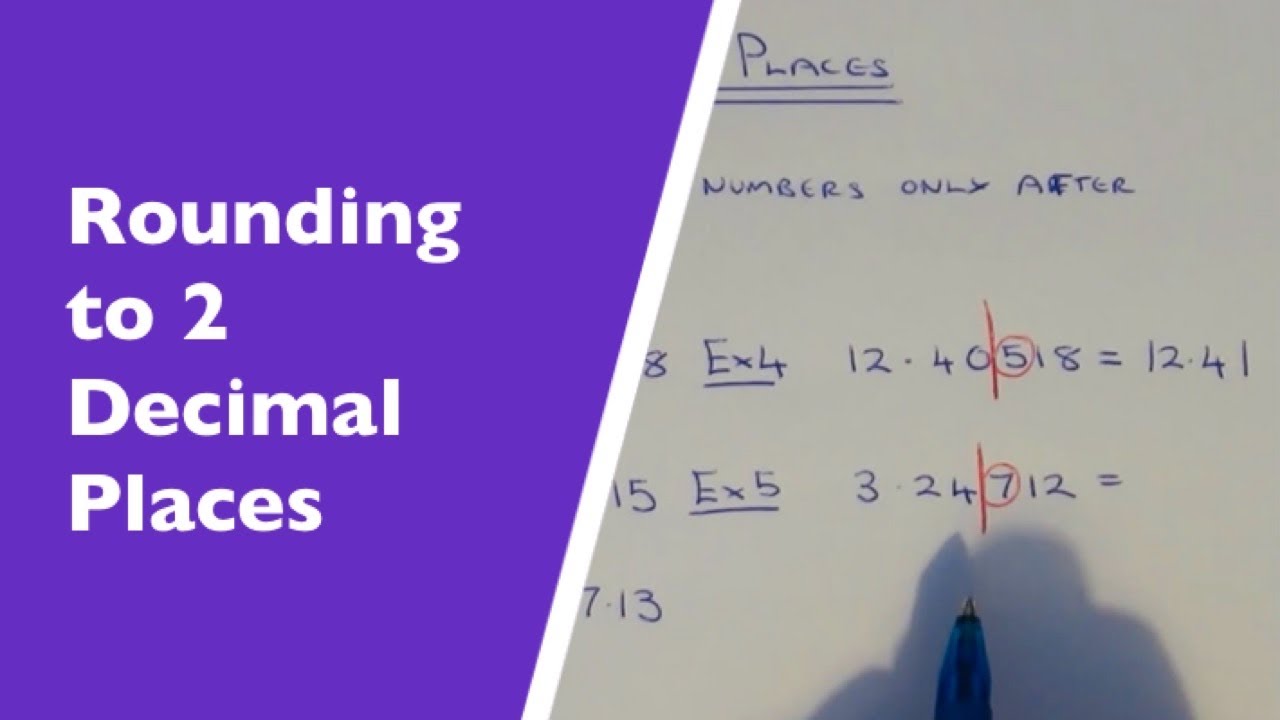

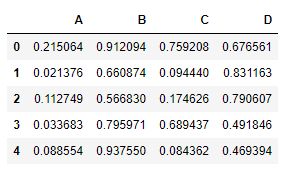

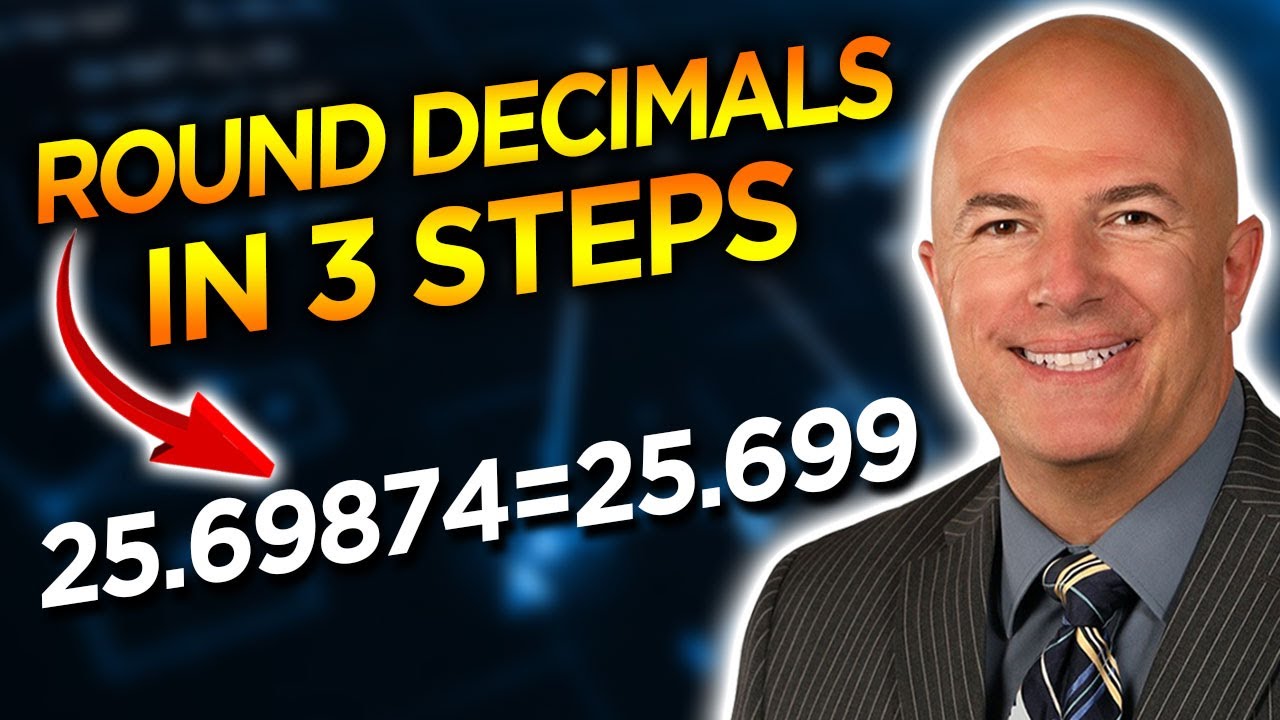

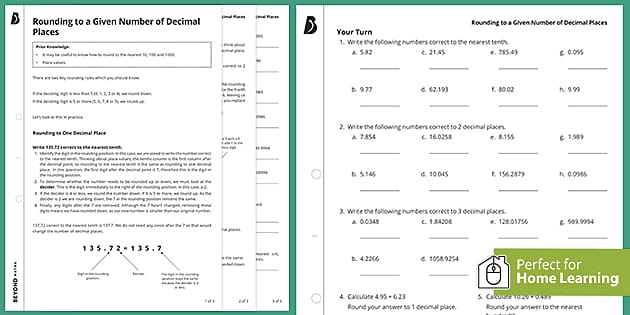
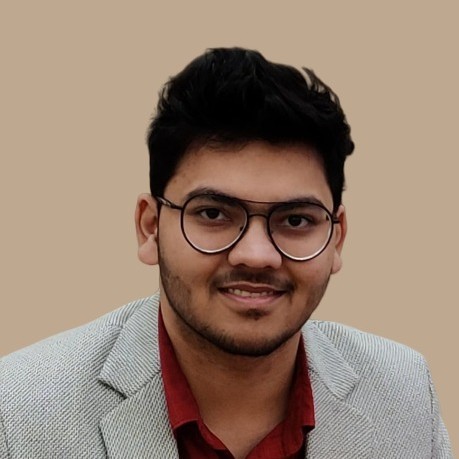


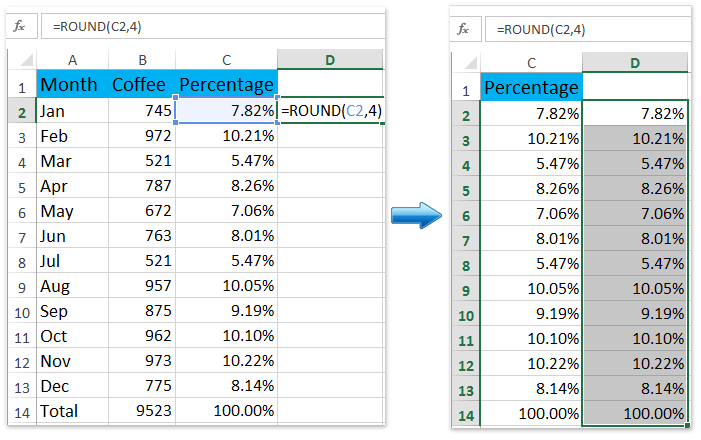
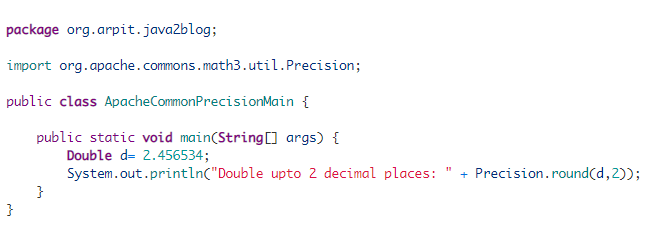
Article link: js round to 2 decimal places.
Learn more about the topic js round to 2 decimal places.
- How to Round a Number to 2 Decimal Places in JavaScript
- How to round to at most 2 decimal places, if necessary
- How to Round a Number to 2 Decimal Places in … – Linux Hint
- How to round to 2 decimal places in JavaScript?
- How to Round to two Decimal Places in JavaScript – STechies
- Round a Number to 2 Decimal Places in JavaScript | Delft Stack
- JavaScript toFixed() Method – W3Schools
- JavaScript round a number to 2 decimal places (with examples)
- Format a number to 2 Decimal places in JavaScript – bobbyhadz
See more: https://nhanvietluanvan.com/luat-hoc/