Jquery Log To Console
jQuery logging to the console is a powerful tool for web developers that allows them to easily track and troubleshoot code errors, debug complex functions, and monitor the flow of their jQuery scripts. By logging messages, variables, and events to the browser console, developers gain real-time insights into the performance and behavior of their web applications. In this article, we will explore the benefits of using jQuery log to console, how to implement it in your projects, common use cases, tips and best practices, alternatives to consider, and conclude with a summary of the topic.
Introduction to jQuery logging to console:
Logging is an essential part of programming as it helps developers understand the inner workings of their code and identify any issues or bugs that need to be addressed. jQuery, a popular JavaScript library, offers a convenient way to log messages to the browser console. The console is a tool provided by web browsers that allows developers to interact with their running web applications.
Benefits of using jQuery log to console:
1. Enhanced debugging capabilities: jQuery log to console provides real-time logging, enabling developers to easily identify errors, warnings, and messages during the development process. This helps streamline the debugging process and improve code quality.
2. Easy identification of errors: By logging messages, variables, and events with jQuery, developers can quickly pinpoint the source of any issues that arise. This saves time and effort in troubleshooting and resolving bugs.
3. Logging variables and outputs: jQuery log to console allows developers to log the values of variables and outputs of functions. This allows for better understanding of the state of their code at any given point, making it easier to locate and resolve issues.
4. Efficient troubleshooting and error tracking: With jQuery log to console, developers can track the execution of their code step by step, making it easier to identify problem areas. This helps streamline the troubleshooting process and ensures efficient error tracking.
Implementing jQuery log to console:
To start using jQuery log to console, you will first need to include the jQuery library in your web project. This can be done by downloading the jQuery library and linking it to your HTML file using the script tag. Once the library is included, you can use the console.log() method provided by jQuery to log messages, variables, and events to the browser console. The console.log() method takes a parameter, which can be a string, a variable, or an object.
Common use cases for jQuery log to console:
1. Logging user interactions and events: By logging user interactions and events, developers can gather valuable data for analytics purposes, such as tracking button clicks, form submissions, or page scrolls.
2. Debugging and troubleshooting jQuery functions: jQuery log to console is particularly useful for debugging and troubleshooting complex jQuery functions and scripts. By logging the output of functions and their intermediate values, developers can better understand the behavior of their code.
3. Tracking AJAX requests: When working with jQuery AJAX, logging the flow of requests, response data, and error messages can be crucial for troubleshooting and ensuring the smooth functioning of your web application.
4. Monitoring variable values: By logging the values of variables during code execution, developers can easily track the state and values of their variables. This helps in understanding the logic and flow of their code and identifying any issues that may arise.
5. Logging browser or device-related information: In certain scenarios, logging specific browser or device-related information can be helpful for development and debugging purposes. This can include logging the user-agent string, screen size, or viewport dimensions.
Tips and best practices for using jQuery log to console:
1. Use different log levels: jQuery log to console supports different log levels, such as info, warn, and error. Categorizing and prioritizing logged messages based on different levels helps in efficiently identifying and resolving issues.
2. Conditional logging: Using conditional statements allows developers to selectively log messages based on certain conditions. This helps prevent unnecessary clutter in the console and focuses on specific scenarios or events.
3. Grouping related log messages: The console.group() and console.groupEnd() methods can be used to group related log messages together. This helps in organizing and categorizing logged information, especially when dealing with complex codebases.
4. Explore other console methods: Besides console.log(), jQuery log to console supports other useful methods like console.error() and console.trace(). These can be employed when dealing with specific error scenarios or for more detailed tracing and debugging.
5. Regularly review and clean up log statements: It is important to review and clean up log statements regularly, as excessive logging can clutter the console and impact performance. Remove any unnecessary log statements or consider using automated logging solutions for more controlled and efficient logging.
Alternatives to jQuery log to console:
While jQuery log to console offers a robust logging solution, there are alternative approaches and tools available. Some alternatives include:
1. log4javascript: This is a logging framework specifically designed for JavaScript, offering advanced logging features and capabilities.
2. Browser-inspector-based debugging tools: Chrome DevTools and Firebug are popular browser-inspector-based debugging tools that provide comprehensive logging, debugging, and profiling features.
3. Server-side logging tools: In scenarios where more comprehensive logging is required, server-side logging tools and techniques can be employed. This includes logging libraries and frameworks available for server-side languages like Node.js, Java, or PHP.
4. Other JavaScript frameworks and libraries: Different JavaScript frameworks and libraries offer their own logging capabilities. It is worth exploring the documentation and features of frameworks such as React, Angular, or Vue.js to find logging solutions that best fit your needs.
Conclusion:
jQuery log to console is a powerful tool for web developers, providing real-time logging capabilities that greatly enhance debugging and troubleshooting. By easily tracking and monitoring code execution, developers can identify errors, analyze variable values, and gain valuable insights into the behavior of their jQuery scripts. While jQuery log to console is widely used and popular, it is crucial to explore alternative logging solutions and consider their suitability based on specific project requirements. By following best practices and utilizing the capabilities of logging tools, developers can streamline their development process and deliver efficient and error-free web applications.
Jquery Console Log
Can I Use Console Log In Jquery?
jQuery is a popular JavaScript library that simplifies various tasks related to web development. It provides a range of functions and methods for manipulating HTML documents, handling events, and creating animations. However, one common question among jQuery developers is whether or not they can use console.log in jQuery.
The console.log function is a powerful tool in JavaScript that allows developers to log messages, variables, or objects to the browser’s console. It is incredibly useful for debugging purposes, as it provides a way to track code execution and identify errors.
By default, jQuery does not have a built-in console.log function. However, it is important to note that jQuery is built on top of JavaScript, so you can still use the console.log function in jQuery by utilizing the underlying JavaScript functionality.
To access the console.log function in jQuery, simply use the JavaScript code instead. For example, if you want to log a message to the console in jQuery, you can do so by writing:
“`javascript
console.log(“Hello, jQuery!”);
“`
This code will print the message “Hello, jQuery!” to the browser’s console, allowing you to easily track the execution of your code and debug any issues that may arise.
It is worth mentioning that console.log is not limited to jQuery or JavaScript libraries like jQuery. It is a feature provided by most modern browsers and can be used in any environment where JavaScript is supported.
FAQs:
Q: Can I use console.log in all browsers?
A: Console.log is a feature provided by most modern browsers. However, older browsers may not support it or may have limited support. It is always a good practice to include other debugging techniques as well, such as alert statements or writing messages directly to the HTML page.
Q: Are there alternatives to console.log in jQuery?
A: While console.log is a convenient way to debug code, there are alternative methods available in jQuery for debugging purposes. For example, you can use the jQuery .text() method to append messages or variables to an HTML element on the page, allowing you to view the debugging information directly on the web page itself.
Q: Is console.log only used for debugging?
A: Console.log is primarily used for debugging purposes, but it can also be used to monitor the execution of code, track variable values, or log important information during development. It provides developers with a way to inspect and analyze their code during runtime.
Q: Can I use console.log in production code?
A: It is generally discouraged to use console.log statements in production code as they may cause a security risk or impact performance. It is recommended to remove or comment out any console.log statements before deploying your code to production.
Q: How can I use console.log effectively in jQuery?
A: To make the best use of console.log in jQuery, it is important to strategically place the statements throughout your code and provide meaningful messages or variable information. This allows you to identify the execution flow, locate errors, and validate the values of variables or objects.
In conclusion, while jQuery itself does not have a built-in console.log function, you can still use console.log in jQuery by leveraging the underlying JavaScript functionality. Console.log is a powerful tool for debugging and tracking code execution, and it is supported by most modern browsers. However, it is essential to use console.log judiciously and consider alternative debugging techniques when necessary. By using console.log effectively, you can enhance your development process and make debugging tasks more efficient and manageable.
How To Run Jquery Command On Console?
jQuery is a popular JavaScript library that simplifies HTML document traversal, event handling, and adds animation functionalities to web pages. Being a widely used tool, developers often find themselves needing to run jQuery commands directly in the browser console. This article will guide you through the steps of running jQuery commands on the console and provide some FAQs to help you understand the process better.
Running a jQuery command on the console is a straightforward process that requires just a few simple steps. Here’s how you can do it:
Step 1: Open the Console
First, you need to open the console in your browser. There are various methods to open the console, but the most common way is to right-click anywhere on the web page and select “Inspect” or “Inspect Element.” This will open the browser’s Developer Tools, where you’ll find the console tab.
Step 2: Ensure jQuery is Loaded
Before running any jQuery commands, make sure that jQuery is already loaded on the web page. Usually, websites include jQuery by linking to it in the HTML file or by using a Content Delivery Network (CDN) like Google or jQuery’s official CDN. If the website you are working on has properly loaded jQuery, you should see the jQuery library in the network tab of your browser’s developer tools.
Step 3: Invoke the jQuery Object
Once you have access to the console and jQuery is loaded, you can start running commands. To do this, you need to invoke the jQuery object, which is usually denoted by the dollar sign ($) symbol in JavaScript code. In most cases, you can type ‘$’ followed by parentheses ($()) to invoke the jQuery object.
Step 4: Execute jQuery Commands
Once the jQuery object is invoked, you can run any jQuery command or perform various operations. For example, to select an HTML element using jQuery, you can use the dollar sign followed by the CSS selector (e.g., $(‘li’) to select all list items on a page). You can then chain additional jQuery methods to manipulate or gather information about the selected elements.
Additionally, you can utilize jQuery functions such as ‘each’ to loop through selected elements, ‘addClass’ to add a CSS class to the selected elements, or ‘animate’ to create animations. The possibilities are nearly endless, as jQuery offers a wide range of functions to streamline web page development.
FAQs:
Q: Can I use a different symbol instead of the dollar sign to invoke the jQuery object?
A: Yes, jQuery provides a noConflict() method to avoid conflicts with other JavaScript libraries that may also use the dollar sign. By using this method, you can assign jQuery to a different variable, such as ‘j’ or ‘jq’, and then use it instead of the dollar sign.
Q: Can I run multiple jQuery commands in a single line on the console?
A: Yes, you can chain multiple jQuery commands together using dot notation. For example, $(‘li’).addClass(‘highlight’).css(‘color’, ‘red’) would select all list items, add a ‘highlight’ class, and change the text color of those items to red.
Q: Is it possible to pass arguments to jQuery commands when running them on the console?
A: Yes, you can pass arguments to most jQuery functions directly in the console. For example, $(‘li’).css(‘color’, ‘blue’) would change the color of all list items to blue.
Q: Can I test my own jQuery code on the console before implementing it on a web page?
A: Absolutely! The console is an excellent place to test and experiment with jQuery commands before integrating them into your web page or JavaScript files. You can type your own code directly into the console and see the results immediately.
Q: Are there any risks associated with running jQuery commands on the console?
A: Running jQuery commands on the console is generally safe, but it’s essential to exercise caution when interacting with other scripts or making significant modifications on a live website. Make sure you understand what the script does and how it may impact the web page before running it.
In conclusion, running jQuery commands on the console is a powerful way to interact with web pages and test jQuery functionality. By following the steps outlined in this article, you should be able to utilize the console effectively and leverage jQuery’s capabilities to enhance your web development experience.
Keywords searched by users: jquery log to console console.log javascript, Jquery write to console, Console log jQuery, Console log Java, console.log là gì, jQuery console log not working, Print console jQuery, jQuery debug log
Categories: Top 69 Jquery Log To Console
See more here: nhanvietluanvan.com
Console.Log Javascript
At its core, console.log is a method that prints a specific message or variable to the console. This message can consist of plain text, variables, or even complex JavaScript objects. By using console.log, developers can gain visibility into the state and behavior of their code at runtime.
One of the most basic uses of console.log is to output simple messages or log statements. For example, consider the following line of code:
“`
console.log(“Hello, world!”);
“`
When this code is executed, the message “Hello, world!” will be displayed in the console window. This is helpful for verifying that certain parts of your code are being executed correctly or for providing general information during development.
In addition to text, console.log can output the values of variables. This is particularly useful when you want to track the value of a variable as your code executes. For example:
“`
let x = 5;
console.log(“The value of x is: ” + x);
“`
When this code is run, the console will display “The value of x is: 5”. This can be invaluable for understanding how variable values change over time, especially in complex algorithms or functions.
Console.log can also display the contents of JavaScript objects and arrays. This feature allows you to inspect the structure and content of these complex data types. For example:
“`
let person = { name: “John”, age: 30 };
console.log(person);
“`
Running this code will output the entire object to the console, providing a detailed view of its properties and values. This can be extremely helpful for investigating issues related to data manipulation or accessing specific object properties.
Another powerful capability of console.log is the ability to log multiple values or objects at once. This can be achieved by providing multiple arguments to the console.log method. For instance:
“`
let a = 10;
let b = 20;
console.log(“The values of a and b are:”, a, b);
“`
Executing this code will display “The values of a and b are: 10 20” in the console. This feature allows you to easily group related information together, making it easier to understand the relationships between different variables or objects.
Now let’s address some frequently asked questions about console.log:
Q: Can console.log be used on any browser?
A: Yes, console.log is a standard JavaScript method that is supported by all major web browsers.
Q: Does console.log affect the performance of my JavaScript code?
A: While console.log can impact the performance of your code, it is primarily noticeable when logging large amounts of data or within performance-critical sections. It’s generally considered good practice to remove unnecessary console.log statements from production code to optimize performance.
Q: Are there any alternatives to console.log?
A: Yes, there are alternative methods to console.log, such as console.error, console.warn, and console.info, which can be used to output different types of messages. Additionally, some development tools provide more advanced logging capabilities.
Q: Can console.log be used in Node.js?
A: Yes, console.log is also available in Node.js, allowing you to log information to the console when running JavaScript on the server-side.
Q: How can I disable or enable console.log output?
A: In most web browsers, you can disable or enable console.log output by opening the browser’s developer tools and adjusting the settings. However, remember to re-enable console.log before deploying your code to production.
In conclusion, console.log is a fundamental tool in JavaScript programming that allows developers to log messages, variables, and objects to the console. It is an essential technique for debugging, understanding code behavior, and gaining insights during development. With a variety of use cases and the ability to log multiple values or objects at once, console.log empowers developers to effectively analyze and troubleshoot their JavaScript code.
Jquery Write To Console
Console logging refers to the practice of sending messages or data to the browser’s console. This can be useful for various reasons such as debugging code, monitoring variable values, testing functions, or simply providing feedback during development. jQuery provides a few built-in methods that allow developers to write to the console easily.
The most commonly used method is `console.log()`, which allows you to output any values or messages to the console. To use this method, you simply call `console.log()` passing in the value or message you want to log. For example:
“`javascript
console.log(“This is a log message.”);
console.log(variableName);
“`
By executing the above code, the logged message or value will be displayed in the browser’s console. This is particularly useful for printing variable values to ensure they contain the expected data.
Another useful console logging method provided by jQuery is `console.debug()`. This method is generally used for logging debug messages and is often used in combination with breakpoints to analyze the flow of code execution. Similar to `console.log()`, you can pass any values or messages as parameters to `console.debug()`. For instance:
“`javascript
console.debug(“This is a debug message.”);
console.debug(variableName);
“`
`console.debug()` is especially handy for developers who want to trace through their code and see the values of particular variables at specific points.
Additionally, jQuery provides other console logging methods such as `console.warn()` and `console.error()`. These methods allow you to log warning and error messages respectively. They provide visual cues in the console, making it easier to identify potential issues or areas that require attention. Here’s an example of their usage:
“`javascript
console.warn(“This is a warning message.”);
console.error(“This is an error message.”);
“`
Using these methods, you can easily identify warnings and errors in your code and address them promptly.
Now, let’s address some frequently asked questions about jQuery console logging:
Q: Can I use console logging methods in any browser?
A: Yes, you can use jQuery’s console logging methods in modern web browsers, including Chrome, Firefox, Safari, and Edge. However, please note that Internet Explorer does not support the console object unless the developer tools are open.
Q: Why should I use console logging instead of alert()?
A: While `alert()` can be used for displaying messages, it can interrupt the flow of the code and require user interaction. Console logging, on the other hand, provides an unobtrusive way to monitor and test your code without affecting the user experience. It also allows you to log complex objects or data structures easily.
Q: Can I log multiple values or messages at once?
A: Yes, you can log multiple values or messages by passing them as separate parameters to the console logging methods. For example:
“`javascript
console.log(“Message 1”, “Message 2”, variableName);
“`
Q: Can I log objects or arrays?
A: Yes, you can log objects or arrays using the console logging methods. The console will display these complex data structures in an expanded format, making it easier to inspect their contents.
Q: Is it possible to disable console logging in production?
A: Yes, you can disable console logging in production environments by removing or commenting out the console.log statements. It’s generally good practice to remove any unnecessary console logging in production code to minimize performance overhead.
In conclusion, jQuery’s console logging methods provide an efficient way to debug, test, and monitor your code during development. The ability to write to the console allows you to gain insight into the flow of your program, monitor variable values, and identify potential issues. By utilizing console logging, you can streamline your development process and create more robust and error-free web applications.
Images related to the topic jquery log to console
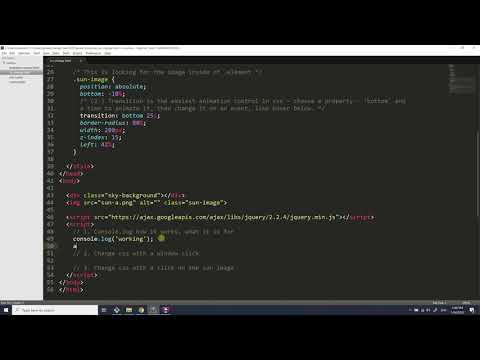
Found 20 images related to jquery log to console theme
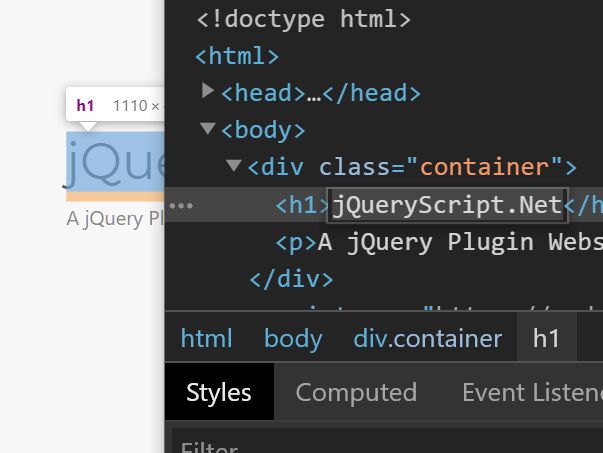
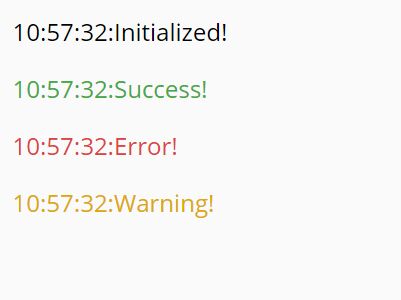
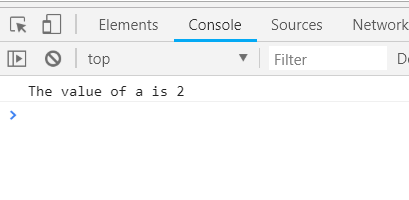
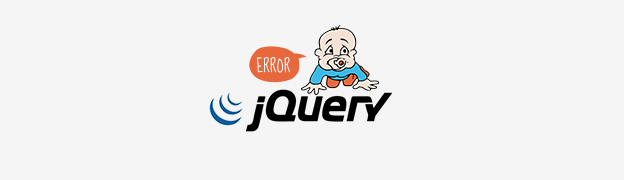



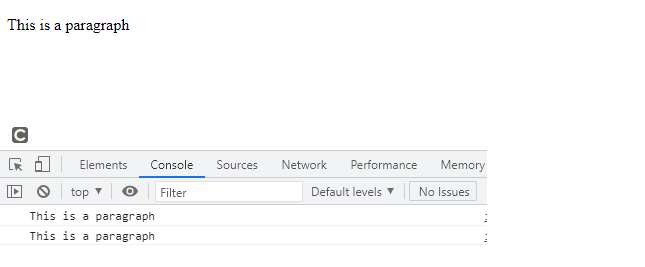

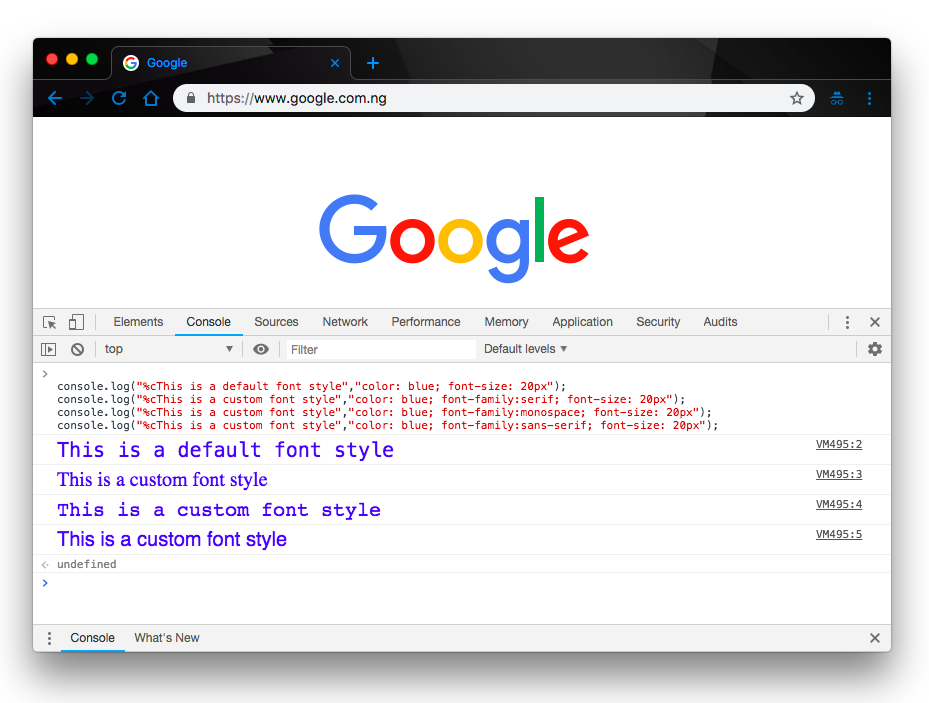
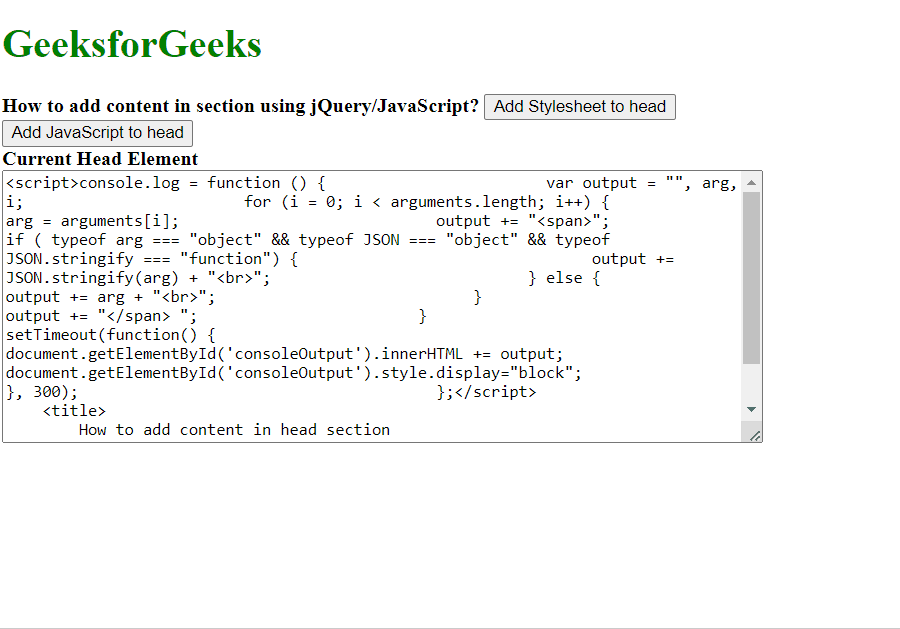


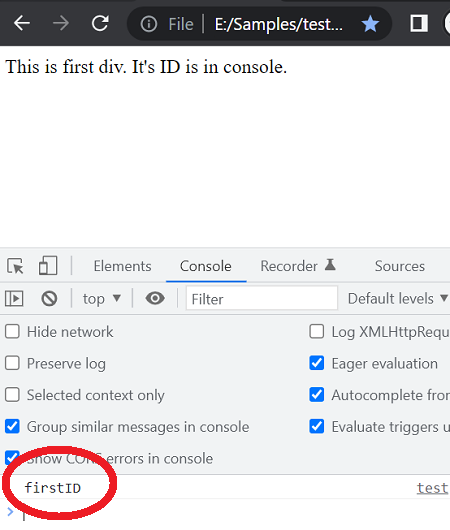



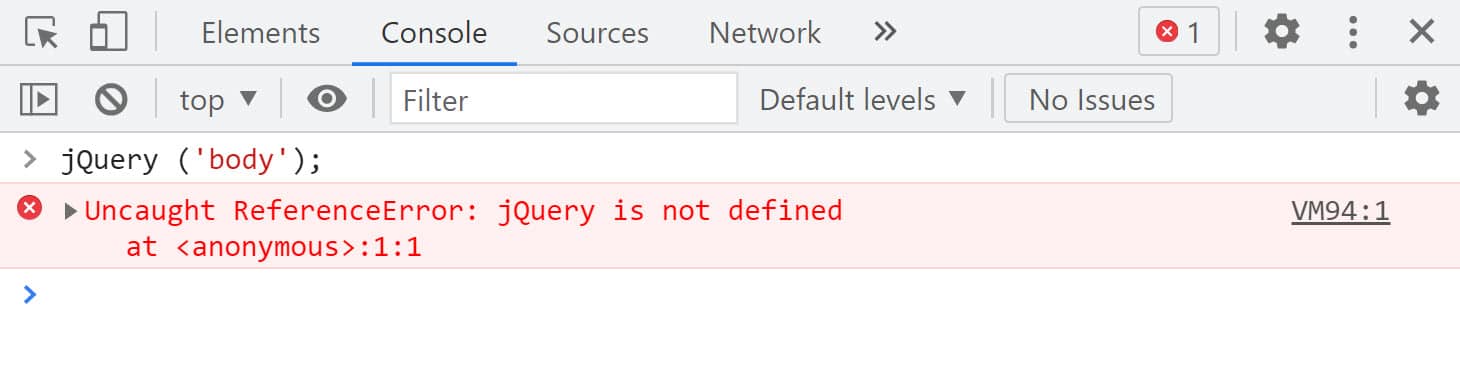
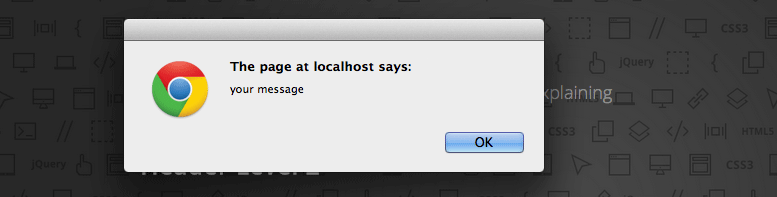
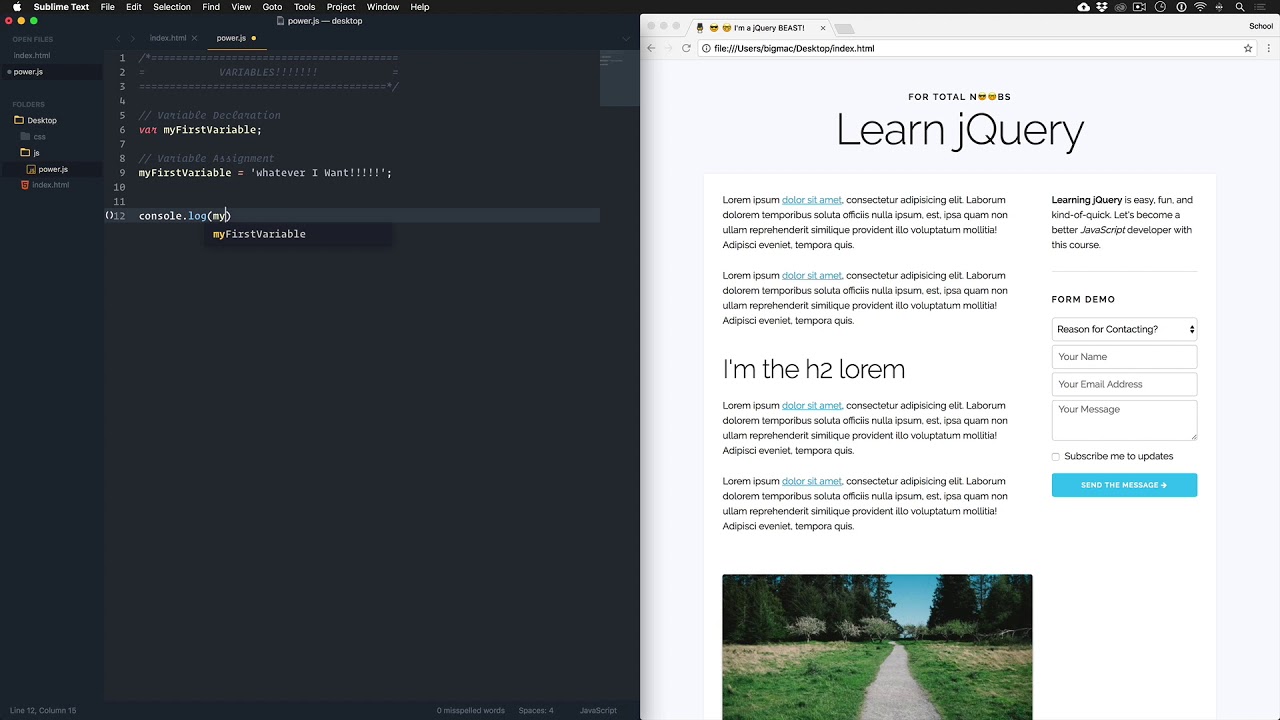




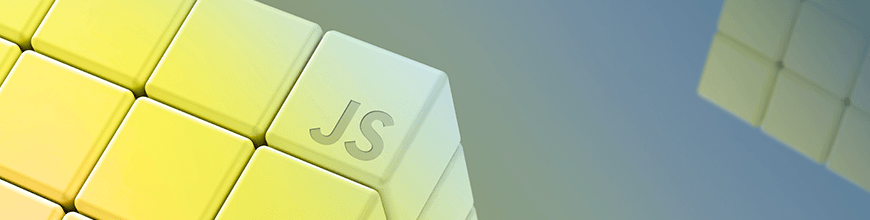
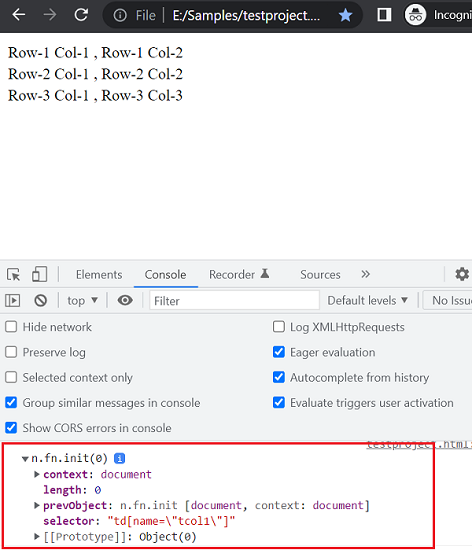
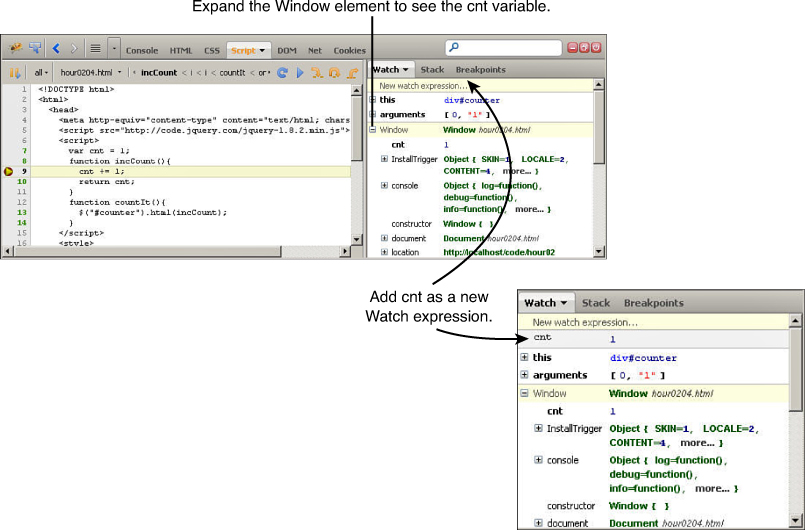
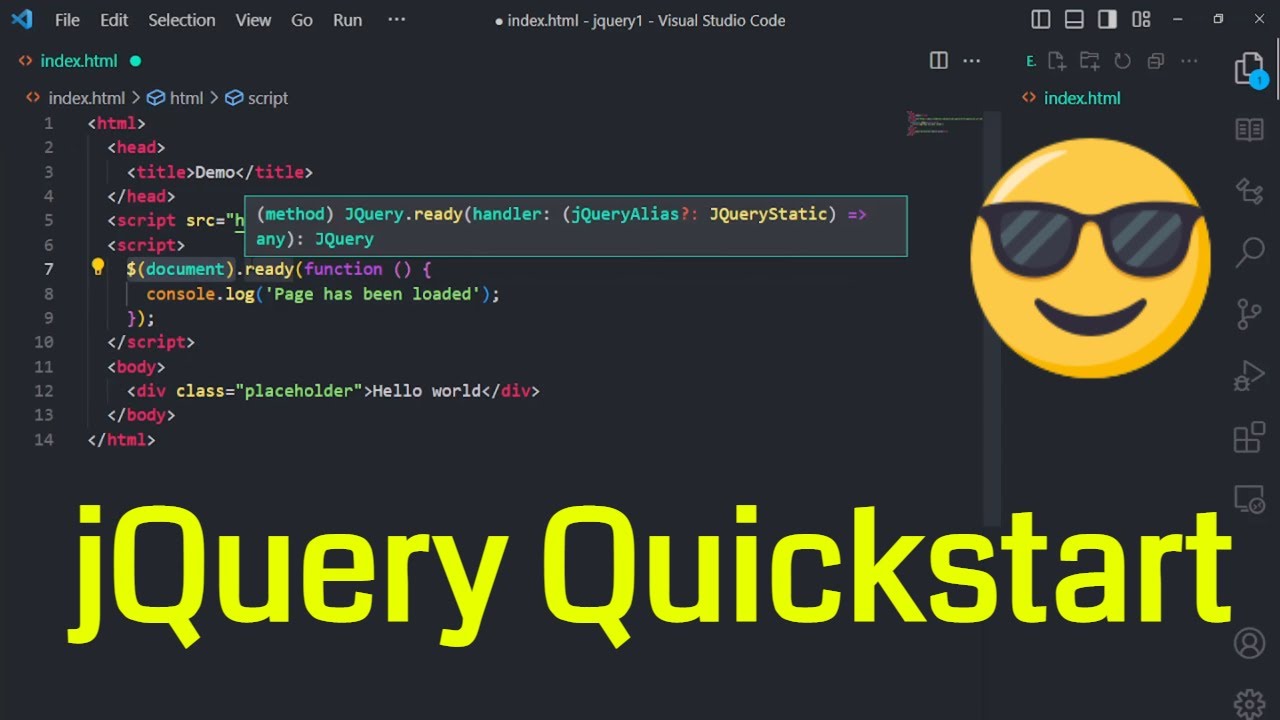

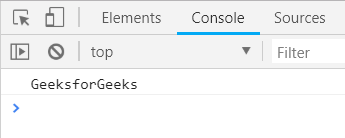
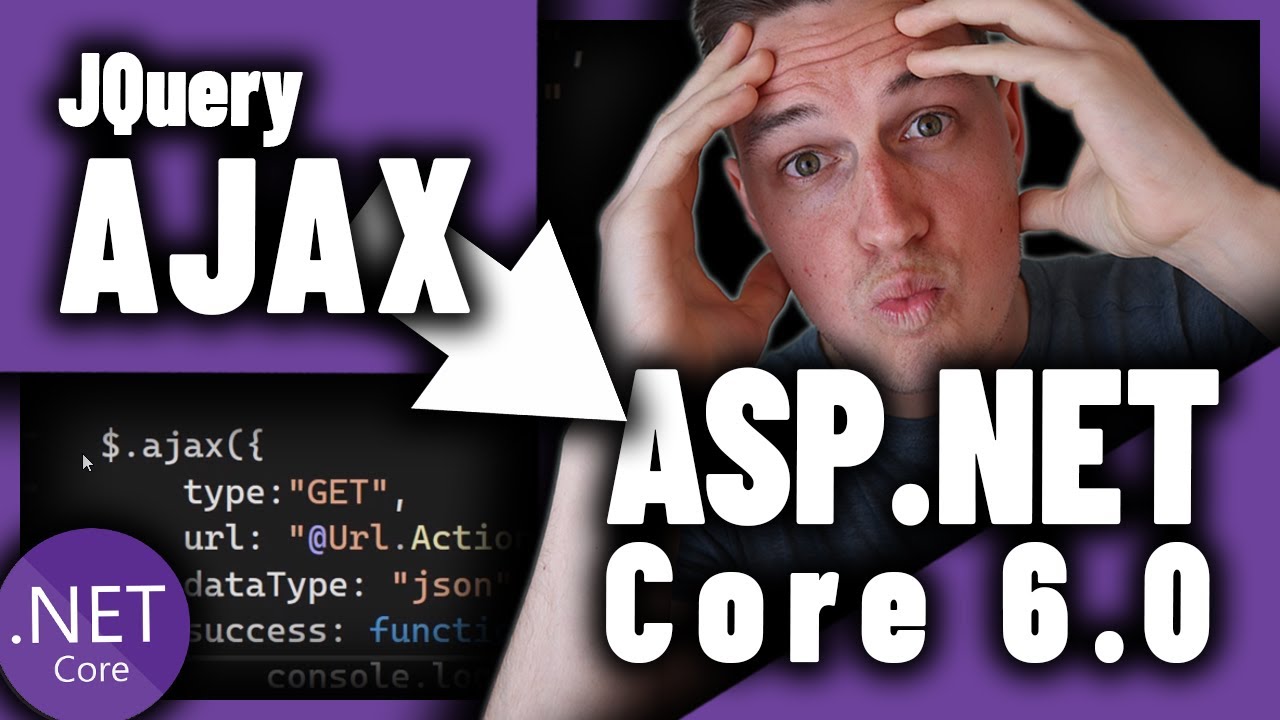
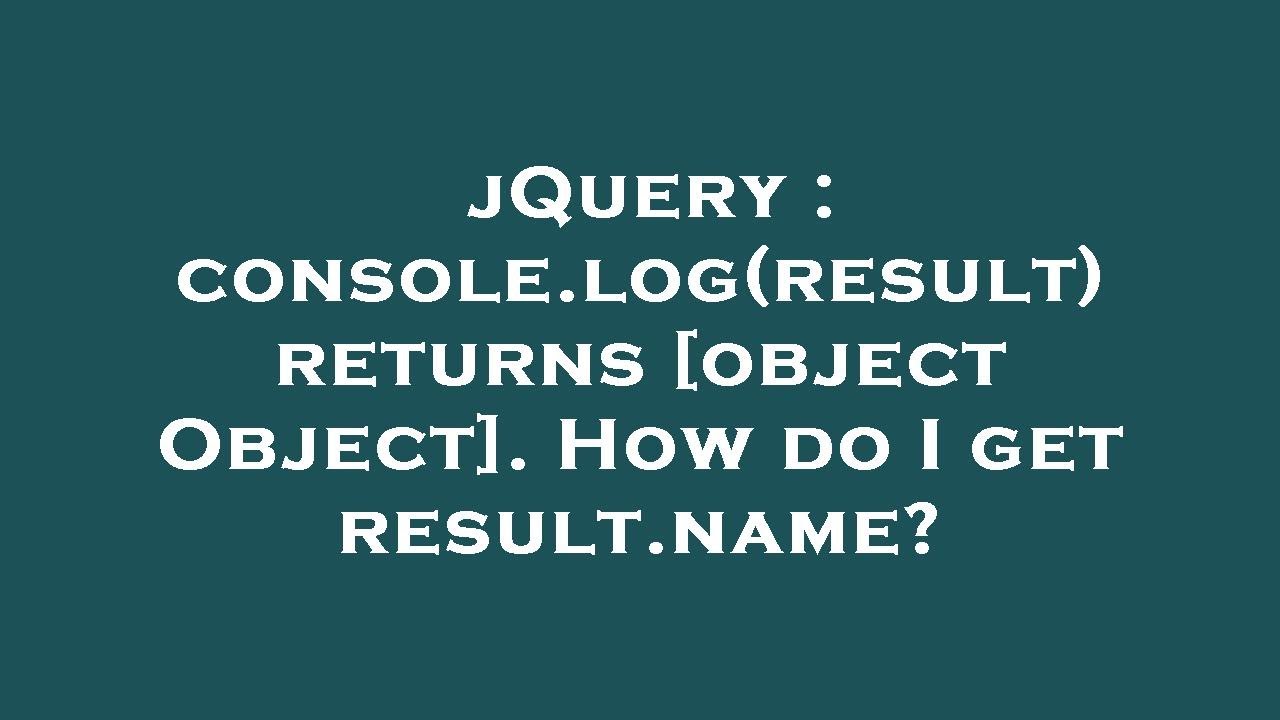
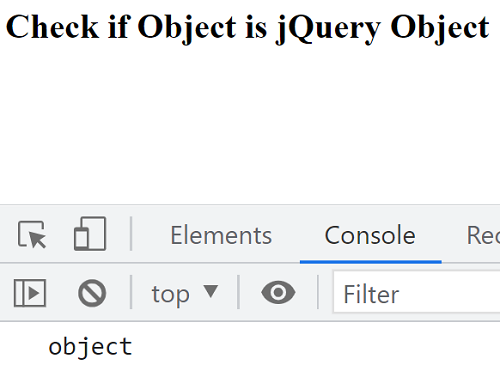


Article link: jquery log to console.
Learn more about the topic jquery log to console.
- jQuery For Complete Beginners – console.log(), scrollTop()
- What is console.log in jQuery? [duplicate] – Stack Overflow
- JavaScript console.log() Method – W3Schools
- Check the jQuery version using Console command (Chrome)
- Run jQuery in Chrome Console – WPLauncher – Courses
- How to get the value in an input text box using jQuery ? – GeeksforGeeks
- console: log() method – Web APIs | MDN
- Debugging jQuery – using the console – Simon Battersby
- console: log() method – Web APIs | MDN
- Read the output of console.log – jQuery Forum
- jQuery object has empty properties, but shows up when used …
- jQuery console log – web-profile
See more: https://nhanvietluanvan.com/luat-hoc/