Jq Select Multiple Fields
Overview of the jq command syntax:
The jq command follows a simple syntax that allows you to specify the JSON data and the operations you want to perform on it. The basic syntax of the jq command is as follows:
“`
jq [options]
“`
– The filter is the most important part of the jq command. It specifies the operations you want to perform on the JSON data. The filter can be a simple field name, a condition, or a combination of both.
– The options allow you to modify the behavior of the jq command. For example, you can use the `-r` option to output the results in raw format instead of JSON format.
The usage of jq to select multiple fields:
One of the common use cases of jq is to select multiple fields from JSON objects. This allows you to extract specific pieces of information from JSON data and perform further processing on them. Here are a few ways you can select multiple fields using jq:
1. Selecting fields with specific names:
You can use the dot notation to specify the field names you want to select. For example, if you have a JSON object with fields like “name”, “age”, and “email”, you can select these fields using the following command:
“`
jq ‘.name, .age, .email’ file.json
“`
This command will output the values of the “name”, “age”, and “email” fields from the JSON data.
2. Using the select() function:
The select() function allows you to filter fields based on specific conditions. You can use this function to select fields that meet certain criteria. For example, if you have a JSON object with a “status” field and you want to select only the fields where the status is “active”, you can use the following command:
“`
jq ‘select(.status == “active”)’ file.json
“`
This command will output only the fields where the “status” field is “active”.
3. Performing complex field selections:
jq provides a lot of flexibility in selecting fields based on multiple criteria. You can use logical operators like AND (&&) and OR (||) to perform complex field selections. For example, if you want to select fields where the “status” is “active” and the “age” is greater than 30, you can use the following command:
“`
jq ‘select(.status == “active” && .age > 30)’ file.json
“`
This command will output the fields that meet both conditions.
4. Selecting and displaying specific fields in JSON arrays:
If your JSON data contains arrays, you can use the map() function to extract multiple fields from the JSON objects within the arrays. For example, if you have a JSON array where each object has fields like “name”, “age”, and “email”, you can select these fields using the following command:
“`
jq ‘.[] | {name: .name, age: .age, email: .email}’ file.json
“`
This command will output the values of the “name”, “age”, and “email” fields for each JSON object within the array.
Applying the jq command to real-world scenarios and examples:
Now let’s see how the jq command can be applied to real-world scenarios and examples.
1. Jq select multiple fields to CSV:
If you want to convert JSON data to CSV format, you can use the jq command to select the fields you want and then use other tools like awk or csvkit to convert the data to CSV. Here’s an example that selects the “name” and “email” fields and converts them to CSV format:
“`
jq -r ‘.name, .email | @csv’ file.json
“`
2. Yq select multiple fields:
Yq is a wrapper for jq that allows you to write YAML documents and select fields from them using the same syntax as jq. To select multiple fields using yq, you can use the following command:
“`
yq eval ‘.name, .age, .email’ file.yaml
“`
This command will select the “name”, “age”, and “email” fields from the YAML document.
3. Jq get field:
If you want to get the value of a specific field from a JSON object, you can use the jq command. For example, if you have a JSON object with a field called “name”, you can get its value using the following command:
“`
jq ‘.name’ file.json
“`
This command will output the value of the “name” field.
4. Select multiple jQuery:
If you’re working with HTML documents and using jQuery, you can use the .map() function to select multiple fields from HTML elements. For example, if you want to select the “href” and “text” fields from all the elements in a webpage, you can use the following jQuery code:
“`javascript
var fields = $(‘a’).map(function() {
return {
href: $(this).attr(‘href’),
text: $(this).text()
};
}).get();
“`
This code will select the “href” and “text” fields from all the elements and store them in the `fields` variable.
5. Cannot index string with string jq:
If you encounter the “Cannot index string with string” error while using the jq command, it means that you’re trying to access a field that doesn’t exist in the JSON data. Make sure that the field name is correct and the JSON structure matches your expectations.
6. Jq get value from JSON:
To get a specific value from a JSON object using jq, you can use the dot notation to access the field. For example, if you have a JSON object with a field called “name”, you can get its value using the following command:
“`
jq ‘.name’ file.json
“`
This command will output the value of the “name” field.
7. Jq export array:
If you want to export an array from JSON data using jq, you can use the @json filter. For example, if you have a JSON array and you want to export it as a string, you can use the following command:
“`
jq ‘.[]’ file.json | jq -s @json
“`
This command will output the JSON array as a string.
8. Jq to CSV:
To convert JSON data to CSV format using jq, you can select the fields you want and then use other tools like awk or csvkit to convert the data to CSV. Here’s an example that selects the “name” and “email” fields and converts them to CSV format:
“`
jq -r ‘.name, .email | @csv’ file.json
“`
FAQs:
Q: Can jq select multiple fields with different criteria?
A: Yes, you can use logical operators like AND (&&) and OR (||) to perform complex field selections with multiple criteria. For example, `jq ‘select(.status == “active” && .age > 30)’` will select fields where the “status” is “active” and the “age” is greater than 30.
Q: Is it possible to convert JSON data to CSV format using jq?
A: Yes, you can select the fields you want using the jq command and then use other tools like awk or csvkit to convert the data to CSV format. For example, `jq -r ‘.name, .email | @csv’` will select the “name” and “email” fields and convert them to CSV format.
Q: Can I use jq to extract values from a specific field in a JSON object?
A: Yes, you can use the dot notation to access a specific field in a JSON object. For example, `jq ‘.name’` will extract the value of the “name” field.
Q: What should I do if I encounter the “Cannot index string with string” error while using jq?
A: This error indicates that you’re trying to access a field that doesn’t exist in the JSON data. Make sure that the field name is correct and the JSON structure matches your expectations.
In conclusion, jq is a versatile tool for selecting multiple fields from JSON data. With its intuitive syntax and powerful functions, you can easily extract specific information from JSON objects, filter data based on specific conditions, and perform complex field selections. Whether you need to convert JSON data to CSV format or extract values from specific fields, jq has got you covered.
Use Jq To Extract And Format Values From A Large Json File | 🖥 Modern Cli
Keywords searched by users: jq select multiple fields Jq select multiple fields to csv, Yq select multiple fields, Jq get field, Select multiple jQuery, Cannot index string with string jq, Jq get value from JSON, Jq export array, Jq to CSV
Categories: Top 68 Jq Select Multiple Fields
See more here: nhanvietluanvan.com
Jq Select Multiple Fields To Csv
Processing and analyzing large datasets can be a daunting task, especially when you are dealing with complex data structures. Fortunately, JQ, a lightweight and versatile command-line tool, comes to the rescue. Specifically, JQ provides a powerful and efficient way to select multiple fields from JSON data and convert them into a comma-separated values (CSV) format. In this article, we will explore the ins and outs of JQ, explain how to use it to select multiple fields, and provide answers to frequently asked questions.
What is JQ?
JQ is a command-line JSON processor that allows you to manipulate and transform JSON data effortlessly. It provides a compact and intuitive syntax, making it an effective tool for filtering, sorting, and transforming JSON objects. With JQ, you can slice, filter, and map data, enabling you to extract the information you need from complex JSON structures.
Why select multiple fields?
In data processing tasks, it is often necessary to extract specific fields of interest from a dataset. Selecting multiple fields allows you to obtain a subset of the data that is relevant to your analysis or processing requirements. By narrowing down your focus to these select fields, you can reduce the amount of data you need to process, leading to improved efficiency and faster execution times.
Selecting multiple fields with JQ
Using JQ, you can effortlessly select multiple fields from JSON data and convert them into CSV format. The syntax for selecting fields with JQ is straightforward:
“`bash
jq -r ‘[“field1”, “field2”, “field3”] | @csv’ input.json > output.csv
“`
In this command, “field1”, “field2”, and “field3” represent the fields you wish to select. They can be any valid JSON field names or dot-separated paths. “input.json” is the JSON file you want to extract the fields from, and “output.csv” is the resulting CSV file.
For instance, let’s say you have the following JSON data:
“`json
[
{
“name”: “John”,
“age”: 30,
“city”: “New York”
},
{
“name”: “Sarah”,
“age”: 25,
“city”: “Los Angeles”
}
]
“`
If you want to select the “name” and “age” fields, you can use the following command:
“`bash
jq -r ‘[“.name”, “.age”] | @csv’ input.json > output.csv
“`
The resulting CSV file will contain the following data:
“`
“John”,30
“Sarah”,25
“`
FAQs
Q: Can I select nested fields using JQ?
A: Yes, JQ supports selecting nested fields by specifying the path using dot notation. For example, if you have a JSON structure like {“person”: {“name”: “John”}}, you can select the “name” field using the expression “.person.name”.
Q: Can I rename the headers in the resulting CSV file?
A: Yes, you can use JQ to rename headers by using the following syntax:
“`bash
jq -r ‘[.field1 as “newName1”, .field2 as “newName2”] | @csv’ input.json > output.csv
“`
Replace “field1” and “field2” with the desired field names, and “newName1” and “newName2” with the new header names.
Q: How can I handle missing fields in the JSON data when selecting with JQ?
A: JQ automatically handles missing fields by inserting a null value in the resulting CSV file. If you want to replace null with a specific value, you can use the “?” operator. For example, “.field1?” will output the field value if it exists or null if it is missing.
Q: Can I filter the JSON data based on specific conditions before selecting fields with JQ?
A: Yes, JQ provides a rich set of filtering and condition-based operations. For example, you can use the `select()` function to filter data based on specific criteria. You can pipe the filtered results to the field selection syntax to obtain the desired output.
In conclusion, JQ is an incredibly useful tool for selecting multiple fields from JSON data and converting them into CSV format. Its simplicity, efficiency, and versatility make it a go-to choice for handling complex JSON structures. By leveraging JQ’s capabilities, you can streamline your data processing workflows and extract the information you need effortlessly. So, next time you find yourself in need of selecting specific fields from JSON data, remember JQ.
Yq Select Multiple Fields
In the world of data analysis and programming, YQ Select Multiple Fields has gained significant recognition for its ability to streamline and optimize data retrieval processes. This powerful tool allows users to extract specific fields or columns from a dataset, providing enhanced efficiency and flexibility for various applications. In this article, we will delve into the details of YQ Select Multiple Fields, exploring its features, benefits, and practical use cases.
What is YQ Select Multiple Fields?
YQ Select Multiple Fields is a query language specifically designed for filtering and selecting specific fields or columns from a dataset. It is particularly prevalent in the field of data analysis and is widely used in industries such as finance, healthcare, and marketing.
How does YQ Select Multiple Fields work?
YQ Select Multiple Fields operates on structured datasets, which are typically organized in the form of tables or spreadsheets. The language provides users with a concise syntax to select specific fields of interest, based on various criteria such as field names, data types, or column positions.
To use YQ Select Multiple Fields effectively, one needs to have a basic understanding of database concepts and the structure of the dataset being queried. Users can specify specific fields they want to extract by simply mentioning their names or by using SQL-like expressions for complex filtering.
Benefits of YQ Select Multiple Fields:
1. Efficient Data Extraction: YQ Select Multiple Fields allows users to extract only the necessary data from a dataset, eliminating the need to retrieve unnecessary columns. This significantly reduces the query execution time and optimizes resource usage.
2. Improved Performance: By selecting only the required fields, YQ Select Multiple Fields minimizes the amount of data that needs to be processed. Hence, it improves the overall performance of data analysis tasks and enhances the efficiency of data-driven applications.
3. Simplified Data Exploration: YQ Select Multiple Fields enables users to focus on specific fields that are of interest and disregard irrelevant data. This simplification and narrowing down of data make it easier to explore and analyze datasets, leading to faster insights and better decision-making.
4. Enhanced Flexibility: YQ Select Multiple Fields offers great flexibility in selecting fields. Users can choose fields based on specific data conditions or business requirements, allowing for a more tailored analysis approach and accommodating varying needs.
Practical Use Cases:
1. Data Analysis: YQ Select Multiple Fields is widely used in data analysis tasks. Analysts can use it to select specific columns for statistical analysis, creating visualizations, or deriving meaningful insights. For example, in marketing, analyzing customer demographics or purchase behavior can be done efficiently by extracting relevant fields using YQ Select Multiple Fields.
2. Data Reporting: Many reporting tools leverage YQ Select Multiple Fields to generate concise and customized reports. Users can select essential fields to be included in the reports, ensuring that only the required information is presented to the stakeholders. This ensures more efficient report generation and improves clarity and relevance.
3. Database Queries: YQ Select Multiple Fields can be used in combination with other query operations to extract valuable information from databases. By specifying the desired fields, users can filter and retrieve specific subsets of data, making database queries more targeted and precise.
4. API Development: YQ Select Multiple Fields can also be integrated into API development, where data needs to be retrieved following specific field requirements. By incorporating YQ Select Multiple Fields in API endpoints, developers can offer clients more granular control over the information they receive.
FAQs
Q: Can YQ Select Multiple Fields handle large datasets efficiently?
A: Yes, YQ Select Multiple Fields is capable of handling large datasets efficiently. By selecting only the necessary fields, the query execution time is greatly reduced, ensuring optimal performance even with substantial volumes of data.
Q: Is YQ Select Multiple Fields compatible with different database systems?
A: Yes, YQ Select Multiple Fields can be used with various database systems. It is supported by many mainstream databases, including MySQL, PostgreSQL, and SQLite, making it highly versatile and adaptable.
Q: Do I need prior programming experience to use YQ Select Multiple Fields?
A: While prior programming experience can be beneficial, it is not mandatory to use YQ Select Multiple Fields. The language has a user-friendly syntax and offers extensive documentation, making it accessible to both programmers and non-programmers.
Q: Can I combine multiple field selection criteria in a single query?
A: Yes, YQ Select Multiple Fields supports combining multiple field selection criteria in a single query. This allows for complex filtering and extraction of specific fields based on various conditions, providing enhanced flexibility and customization.
In conclusion, YQ Select Multiple Fields is a powerful tool that enables efficient and targeted data extraction, offering numerous benefits to data analysts, developers, and other professionals working with structured datasets. Its ability to improve data exploration, performance, and flexibility makes it a valuable asset in diverse data-driven domains. By mastering YQ Select Multiple Fields, users can enhance their data analysis capabilities and unlock new insights from their datasets.
Jq Get Field
jQuery has emerged as one of the most popular JavaScript libraries, revolutionizing the way developers interact with HTML documents, handle events and perform animations. One of the powerful methods jQuery offers is the .get() method. In this article, we will delve into the details of the .get() method, exploring its purpose, syntax, and various applications. So, let’s get started!
Understanding the .get() Method:
The .get() method in jQuery is primarily used to retrieve data from a server using an AJAX HTTP GET request. It allows developers to fetch both HTML and JSON data from a server and use it dynamically within their web pages. The fetched data can be stored in variables, manipulated, and displayed in real-time without the need to refresh the entire page.
Syntax of .get():
The .get() method follows a straightforward syntax:
$.get(URL, data, callback, dataType);
– URL: The URL of the server-side script or resource from which we want to retrieve data.
– Data: Optional parameter to send additional information to the server.
– Callback: A function that executes when the request completes successfully.
– dataType: Optional parameter that specifies the type of data expected from the server, such as “xml”, “json”, or “html”.
Applications of .get():
1. Loading Data:
The most common usage of .get() is to load content from a server and dynamically insert it into specific elements on a web page. For instance, you can use .get() to fetch the contents of a text file, HTML document, or a JSON file, and display it within a designated div or container.
2. Retrieving JSON Data:
The .get() method is particularly helpful when working with APIs that return JSON data. By specifying the “json” dataType in the .get() call, jQuery automatically parses the received JSON data into a JavaScript object. This makes it easier to extract the required information and dynamically update the page without a full page reload.
3. Fetching HTML Fragments:
Another notable application of .get() is loading specific HTML fragments from the server. This allows developers to load parts of a web page dynamically, without reloading the entire page. By targeting specific HTML elements, such as divs or sections, developers can dynamically update the content on their website.
4. Accessing External Websites:
The .get() method also allows developers to fetch HTML content from external websites. This can be useful for scraping data, implementing custom widgets, or creating mashups by combining information from different sources. However, it is important to ensure that accessing external websites complies with ethical guidelines and legal requirements.
FAQs:
Q1. Can I use .get() to send data to the server?
Yes, you can send data to the server using the .get() method. However, .get() is primarily intended for retrieving data from the server. If you need to send data to the server, it is recommended to use the .post() method or the more generic .ajax() method.
Q2. What happens if the request fails?
If the request initiated by .get() fails—for example, due to network issues or an invalid URL—the provided callback function is not executed. Instead, you can use the .fail() method to handle the failed request and display appropriate error messages to the user.
Q3. Is it possible to pass parameters to the server using .get()?
Yes, it is possible to pass additional data to the server using .get(). As shown in the syntax, you can include a data parameter to send additional information to the server. For instance, you can pass parameters as key-value pairs to the server-side script or resource for further processing.
Q4. How can I handle the response of a .get() request?
The response of a .get() request can be handled by utilizing the callback function provided during the .get() call. This function executes when the request has completed successfully. Inside the callback, you can manipulate the received data, update the web page, or perform any necessary operations based on the retrieved response.
Q5. Can I make cross-domain requests using .get()?
Cross-domain requests, also known as cross-origin resource sharing (CORS) requests, are subject to security restrictions imposed by web browsers. By default, .get() does not allow cross-domain requests. However, you can enable CORS on the server-side to allow cross-domain requests or use JSONP (JSON with Padding) as an alternative technique.
In conclusion, the .get() method offered by jQuery allows developers to retrieve data from a server, making it an invaluable tool for dynamic web page manipulation. By fetching HTML or JSON data and using it to update elements on a page without refreshing, developers can enhance user experience and create interactive web applications. Utilizing the comprehensive syntax and understanding its applications will enable you to harness the full potential of .get() in your next web development project.
Images related to the topic jq select multiple fields
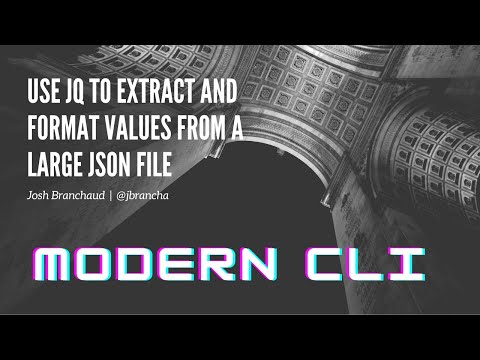
Found 30 images related to jq select multiple fields theme
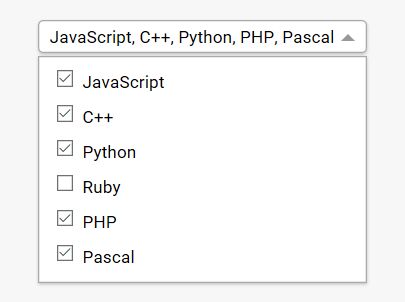





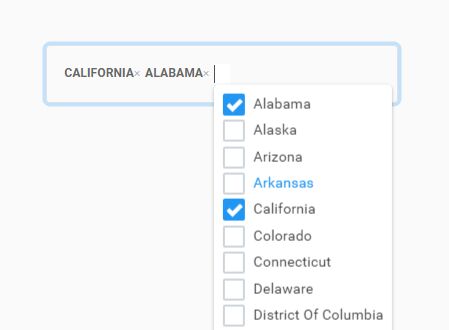


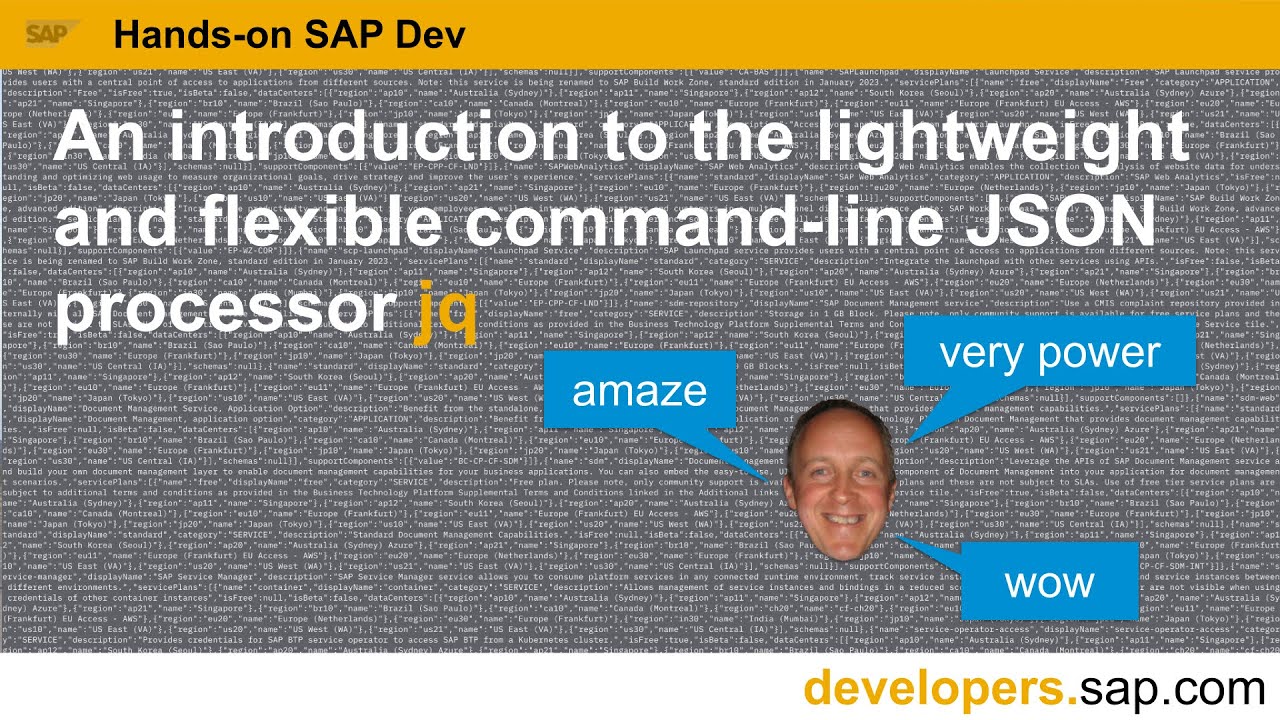

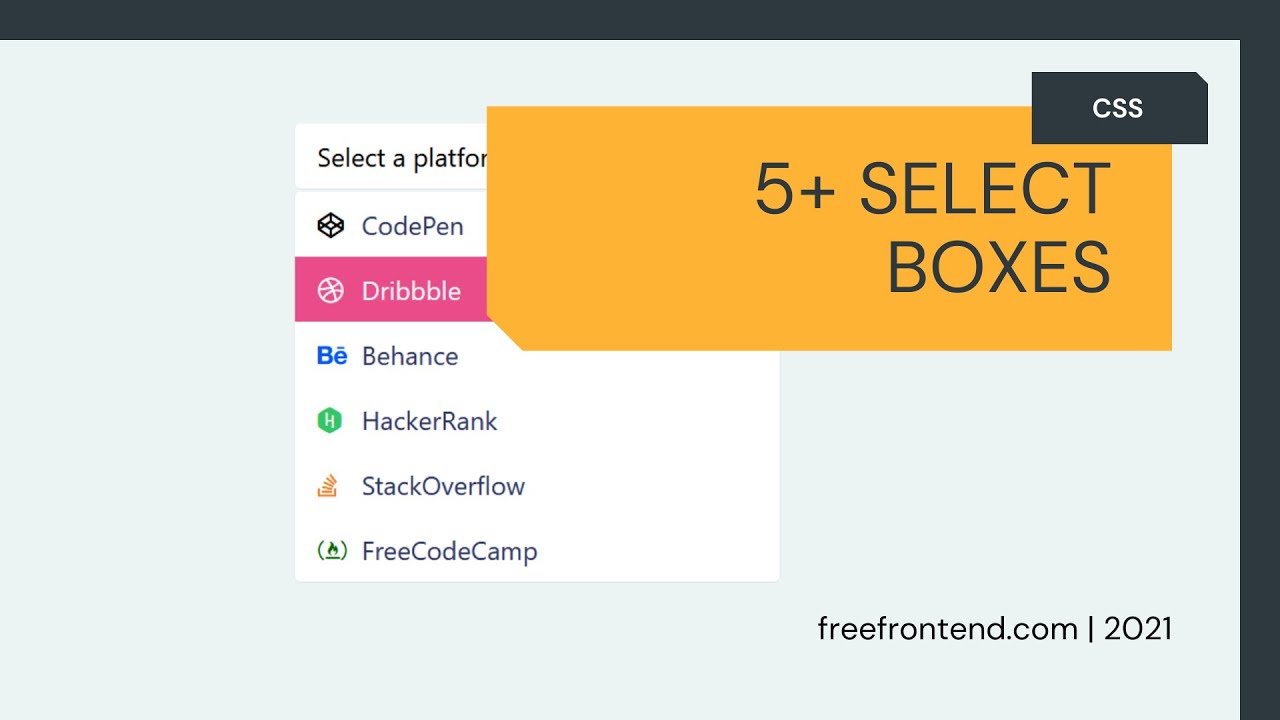
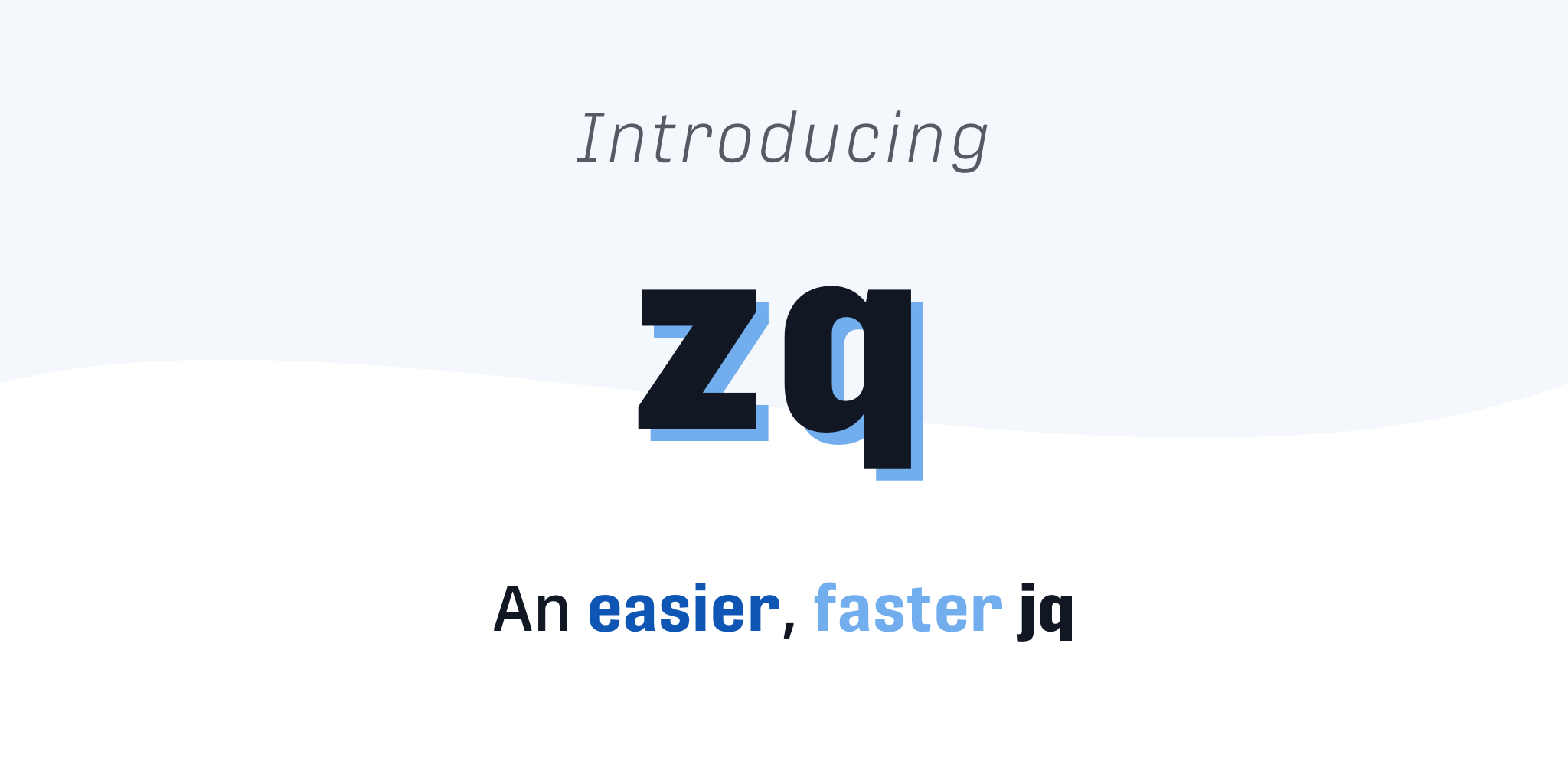
Article link: jq select multiple fields.
Learn more about the topic jq select multiple fields.
- How do I select multiple fields in jq? – Stack Overflow
- An Introduction to JQ – Earthly.dev
- jq: Select multiple keys – Mark Needham
- Get field and nested field at the same time using jq
- Processing JSON using jq – jq Cheet Sheet · GitHub
- How to tell jq to print the content of two keys for each instance …
- Multiple level filters in jq – DJ Adams
- jq and multi-field output to CSV – lekkimworld.com
See more: https://nhanvietluanvan.com/luat-hoc/