Javascript Loop Over Set
Introduction:
In JavaScript, a Set is a data structure that allows you to store unique values of any type. It is similar to an array or an object but with some key differences. This article will explore the Set data structure in JavaScript, explain how it differs from arrays and objects, and provide various methods to loop over Set elements.
What is a Set in JavaScript?
A Set in JavaScript is an unordered collection of unique values. This means that there can be no duplicate values within a Set. Unlike arrays, Sets do not have indexes, and the elements are not stored in any specific order. Additionally, Sets can only store distinct values, ensuring that each value appears only once.
Understanding the Set data structure in JavaScript:
Sets are implemented as ES6 classes in JavaScript. You can create a Set using the `Set` constructor with or without an iterable argument. For example:
“`
const mySet = new Set();
mySet.add(“apple”);
mySet.add(“banana”);
mySet.add(“orange”);
“`
In this example, we create a new Set called `mySet` and add three different fruits to it. Since Sets only store unique values, duplicate items will be automatically ignored.
How Sets differ from arrays and objects in JavaScript:
Sets have some key differences from arrays and objects in JavaScript:
1. Uniqueness: Sets can only store unique values, while arrays and objects can contain duplicate values.
2. Order: Sets do not preserve the order of elements. When you iterate over a Set, the order of elements is not guaranteed. Arrays, on the other hand, maintain the order of elements based on their indexes.
3. Indexing: Sets do not have indexes like arrays. You cannot access a specific element in a Set using indexing. Instead, you have to loop over the Set and find the desired value.
Looping over Sets in JavaScript:
There are several methods available to loop over Set elements in JavaScript. Let’s explore some of the commonly used methods:
1. Using the for…of loop to iterate over a Set:
The `for…of` loop is commonly used to iterate over iterable objects, including Sets. It allows you to loop over each element in a Set without worrying about the order of elements. Here’s an example:
“`
const mySet = new Set([“apple”, “banana”, “orange”]);
for (const element of mySet) {
console.log(element);
}
“`
In this example, we create a Set called `mySet` with three fruits and use the `for…of` loop to iterate over each element and log it to the console. The order of iteration is not guaranteed.
2. Using forEach() with Sets:
The `forEach()` method is another convenient way to loop over Set elements. It allows you to define a callback function that will be executed for each element in the Set. Here’s an example:
“`
const mySet = new Set([“apple”, “banana”, “orange”]);
mySet.forEach((element) => {
console.log(element);
});
“`
In this example, we create a Set called `mySet` and use the `forEach()` method to iterate over each element in the Set. The callback function takes the current element as an argument and logs it to the console.
3. Using the spread operator (…) with Sets:
The spread operator (…) can be used to convert Set elements into an array. This can be helpful when you need to perform array-specific operations or pass the Set elements as arguments to a function. Here’s an example:
“`
const mySet = new Set([“apple”, “banana”, “orange”]);
const myArray = […mySet];
console.log(myArray);
“`
In this example, we create a Set called `mySet` and use the spread operator to convert its elements into an array called `myArray`. The resulting array will contain the same unique elements as the original Set.
Using entries(), values(), and keys() with Sets:
Apart from the methods discussed above, Sets also provide the `entries()`, `values()`, and `keys()` methods for looping over Set elements. These methods return an iterator object that can be used to access the elements within the Set.
Here’s an example of how you can use these methods:
“`
const mySet = new Set([“apple”, “banana”, “orange”]);
// Using entries()
for (const entry of mySet.entries()) {
console.log(entry);
}
// Using values()
for (const value of mySet.values()) {
console.log(value);
}
// Using keys()
for (const key of mySet.keys()) {
console.log(key);
}
“`
In this example, we create a Set called `mySet` and use the `entries()`, `values()`, and `keys()` methods to loop over its elements. The `entries()` method returns an iterator object that contains both entry key-value pairs. The `values()` method returns an iterator object containing only the values, and the `keys()` method returns an iterator object containing the same values as `values()`, for compatibility with Map objects.
Looping over Sets using While loops:
While loops can also be used to iterate over Set elements. However, since Sets do not have indexes, you need to manually maintain a reference to the current element and update it in each iteration. Here’s an example:
“`
const mySet = new Set([“apple”, “banana”, “orange”]);
const iterator = mySet.values();
let current = iterator.next();
while (!current.done) {
console.log(current.value);
current = iterator.next();
}
“`
In this example, we create a Set called `mySet` and obtain an iterator using the `values()` method. We then use a While loop to iterate over the Set by calling `next()` on the iterator object. The `done` property of the iterator object indicates when we have reached the end of the Set.
Using Array.from() with Sets:
The `Array.from()` method can be used to convert Set elements into an array. It takes an iterable object, such as a Set, and returns a new array containing the elements. Here’s an example:
“`
const mySet = new Set([“apple”, “banana”, “orange”]);
const myArray = Array.from(mySet);
console.log(myArray);
“`
In this example, we create a Set called `mySet` and use `Array.from()` to convert its elements into an array called `myArray`. The resulting array will contain the same unique elements as the original Set.
FAQs:
Q: How do I loop through a Set in JavaScript?
A: There are several ways to loop through a Set in JavaScript. You can use a for…of loop, the forEach() method, the spread operator, the entries(), values(), and keys() methods, a While loop, or the Array.from() method.
Q: What is the difference between Set and Array in JavaScript?
A: Sets and arrays in JavaScript have some key differences. Sets can only store unique values, while arrays can contain duplicate values. Sets do not preserve the order of elements, while arrays maintain the order based on their indexes. Additionally, Sets do not have indexes like arrays.
Q: Can I use a Set as a key in a JavaScript object?
A: No, Sets cannot be used as keys in JavaScript objects because Sets are not recognized as primitive values.
Q: Can I add an array to a Set in JavaScript?
A: Yes, you can add an array to a Set in JavaScript. The Set’s add() method accepts an iterable object, so you can pass an array as an argument to add multiple elements at once.
Q: How can I get the value of a Set by its index?
A: Sets do not have indexes, so you cannot access a specific element by index. Instead, you need to loop over the Set and find the desired value.
Conclusion:
In conclusion, Sets in JavaScript provide a powerful data structure for storing unique values. They have unique characteristics compared to arrays and objects, and there are various methods available to loop over Set elements. By leveraging these methods such as the for…of loop, forEach(), spread operator, entries(), values(), keys(), While loop, and Array.from(), you can easily iterate through Sets and perform operations on their elements.
How To Loop Through A Javascript Array
How To Loop Over Set In Javascript?
JavaScript provides various data structures to handle collections of values efficiently. One such data structure is a Set, which stores unique values of any type. Sets offer flexibility, convenience, and performance when managing a collection of unique elements. In this article, we will explore how to loop over a set in JavaScript and demonstrate different looping techniques.
Looping over a set can be necessary in scenarios where you want to perform operations or access each value in the set individually. JavaScript provides multiple ways to achieve this, including using the for…of loop, forEach method, and converting the set to an array and utilizing traditional for loops.
Before diving into the different looping techniques, let’s have a brief overview of sets in JavaScript.
Sets in JavaScript:
A Set is a built-in data structure in JavaScript that allows you to store unique values. It can hold values of any type, whether primitive or object references. Sets automatically remove duplicate values so that only unique elements remain. This unique property makes sets highly useful for creating lists of unique items or checking existence in constant time.
Looping over a Set using for…of loop:
The for…of loop is an efficient and concise way to iterate over elements in a Set. The loop assigns each value directly to a variable, making it convenient to access and utilize the values. Here’s an example:
“`
const set = new Set([1, 2, 3, 4]);
for (const value of set) {
console.log(value);
}
“`
In this code snippet, we create a new Set using the constructor and pass an array of values [1, 2, 3, 4]. The for…of loop is then used to iterate over the set and print each value to the console. The loop runs four times, printing 1, 2, 3, 4 in sequential order.
Looping over a Set using forEach method:
The forEach method is available on Set instances and provides a callback function to execute for each element in the set. This method offers flexibility and allows you to perform actions on each element individually. Here’s an example:
“`
const set = new Set([1, 2, 3, 4]);
set.forEach((value) => {
console.log(value);
});
“`
In this code snippet, a new Set is created with the same values [1, 2, 3, 4]. The forEach method is then called on the set, passing a callback function that prints each value to the console. The forEach method internally iterates over the set and invokes the callback for each element, resulting in the values 1, 2, 3, 4 being printed.
Converting a Set to an array and looping using traditional for loops:
In certain scenarios, you may prefer to convert a Set to an array and utilize traditional for loops for looping purposes. JavaScript provides set conversion to an array using the spread operator (…) or the Array.from method. Once the set is converted, you can loop over the array as usual. Here’s an example:
“`
const set = new Set([1, 2, 3, 4]);
const array = […set];
for (let i = 0; i < array.length; i++) { console.log(array[i]); } ``` In this code snippet, we create a new Set with the values [1, 2, 3, 4]. The spread operator (...) is used to convert the Set to an array, which is stored in the `array` variable. We then utilize a traditional for loop to iterate over the array and access each value individually, printing them to the console. FAQs: Q: Are sets ordered in JavaScript? A: Yes, starting from ECMAScript 2015 (ES6), sets in JavaScript maintain the insertion order of elements, unlike traditional objects. Q: Can a set hold duplicate values? A: No, sets by nature only store unique values. Duplicate values are automatically removed, leaving only distinct elements. Q: Can a set hold values of any type in JavaScript? A: Yes, sets can hold values of any type. They are not limited to specific primitive or object types. Q: Are sets iterable in JavaScript? A: Yes, sets in JavaScript are iterable, meaning you can loop over them using various looping techniques like for...of loop or forEach method. Q: How can I check if a specific value exists in a set? A: You can utilize the `has` method available on Set instances to check if a specific value exists in the set. It returns a boolean indicating whether the value is present or not. In conclusion, JavaScript provides multiple techniques for looping over a Set, allowing you to efficiently iterate and work with each element individually. Whether you choose the for...of loop, forEach method, or convert the Set to an array, consider the specific requirements of your use case and select the most appropriate looping approach. Sets are powerful data structures that bring efficiency and uniqueness to handling collections in JavaScript.
Can We Loop Through A Set Javascript?
Yes, it is possible to loop through a set in JavaScript. Sets were introduced in ECMAScript 6 (ES6) as a new data structure to store unique values of any type. In this article, we will explore how to loop through a set in JavaScript and discuss the various methods and techniques to achieve this.
Looping through a set is similar to looping through an array or other iterable data structures. However, the main difference is that sets do not have a built-in index to access the elements directly. Therefore, we need to utilize built-in methods to iterate over the set’s elements.
Using the forEach() method:
One of the simplest ways to loop through a set is by using the forEach() method. This method executes a provided callback function once for each value in the set. The callback function takes three arguments: the value, the same value again, and the set itself.
Here’s an example of looping through a set using the forEach() method:
“`
const mySet = new Set([1, 2, 3, 4, 5]);
mySet.forEach((value) => {
console.log(value);
});
“`
In this example, we create a new set called `mySet` with some values. We then call the forEach() method on `mySet` and provide a callback function that logs each value to the console.
Using the for…of loop:
Another way to loop through a set is by using the for…of loop. This loop was introduced in ES6 and provides a simpler syntax for iterating over iterable objects. It automatically retrieves the values from the set without the need for an index.
Here’s an example of looping through a set using the for…of loop:
“`
const mySet = new Set([‘a’, ‘b’, ‘c’, ‘d’, ‘e’]);
for (const value of mySet) {
console.log(value);
}
“`
In this example, we create a new set called `mySet` with some string values. We then use the for…of loop to iterate over `mySet` and log each value to the console.
Converting a set into an array:
If you are more comfortable working with arrays, you can convert a set into an array and loop through the array using traditional methods like for loops or array.forEach().
To convert a set into an array, you can use the spread operator (…) or the Array.from() method. Here’s an example:
“`
const mySet = new Set([1, 2, 3, 4, 5]);
// Using spread operator
const myArray = […mySet];
// Using Array.from()
const myArray = Array.from(mySet);
// Looping through the array
for (let i = 0; i < myArray.length; i++) {
console.log(myArray[i]);
}
```
In this example, we create a set called `mySet` and then convert it into an array using both the spread operator (...) and the Array.from() method. We can then loop through the array using a traditional for loop and log each value to the console.
Frequently Asked Questions (FAQs):
Q: Can we loop through a set backward?
A: No, sets do not provide direct methods to iterate backward. However, you can convert the set into an array and use array methods like reverse() or iterate over the array in reverse order.
Q: Can we loop through a set using a for loop?
A: Yes, you can convert the set into an array and then use a for loop to iterate through the array. Alternatively, you can use the for...of loop directly on the set.
Q: Can we modify elements while looping through a set?
A: Modifying elements directly while looping through a set is not recommended, as it can lead to unpredictable results. It is best to create a new set or store the modified values in a separate array.
Q: Can we loop through a set of objects?
A: Yes, sets can contain objects as values. The looping techniques mentioned above can be applied to sets containing objects in the same way as sets with other value types.
Q: Can we loop through a set using forEach() if it contains arrow functions?
A: Yes, the forEach() method can handle arrow functions as callback functions when looping through a set. The arrow function syntax does not affect the iteration process.
In conclusion, looping through a set in JavaScript can be accomplished using methods like forEach() and for...of loop. Additionally, sets can be converted into arrays for more traditional looping techniques. It is important to understand the limitations and caveats of looping through sets, such as the lack of direct backward iteration and potential issues with modifying values while looping. By using the appropriate looping method based on your specific requirements, you can effectively iterate through sets and perform necessary operations on the values they contain.
Keywords searched by users: javascript loop over set Loop through Set JavaScript, Js loop through Map, Loop set typescript, Set entries javascript, Add array to set javascript, Javascript set get value by index, Set forEach JavaScript, Set vs array javascript performance
Categories: Top 17 Javascript Loop Over Set
See more here: nhanvietluanvan.com
Loop Through Set Javascript
Introduction to Sets in JavaScript
Before diving into the looping mechanisms, let’s have a quick overview of Sets in JavaScript. A Set is an unordered collection of unique values, which means that no two elements in a Set can have the same value. This is in contrast to arrays, where duplicate elements can exist. The Set object provides a set of methods to manipulate and retrieve elements from Sets, making them useful for many scenarios.
Looping through a Set Using the for…of Loop
The simplest and most straightforward way to loop through a Set is by using the for…of loop. This loop was introduced in ES6 and provides an easy way to iterate over iterable objects, including Sets. Here’s an example:
“`
const mySet = new Set([1, 2, 3, 4, 5]);
for (const item of mySet) {
console.log(item);
}
“`
In the above code snippet, we create a new Set called `mySet` containing some numbers. We then use the for…of loop to iterate over each element of the Set and log it to the console. The output will be the values 1, 2, 3, 4, and 5 printed in separate lines.
Looping through a Set Using forEach
Another way to loop through a Set is by using the forEach method. The forEach method is available on the Set prototype and is similar to the Array.prototype.forEach method. Here’s an example:
“`
const mySet = new Set([1, 2, 3, 4, 5]);
mySet.forEach((item) => {
console.log(item);
});
“`
In the above code snippet, we create a new Set called `mySet` and use the forEach method to iterate over each item in the Set. The provided callback function is invoked for each item, and the item itself is passed as an argument. Again, the output will be the values 1, 2, 3, 4, and 5 printed in separate lines.
Looping through a Set Using the Array.from Method
If you prefer a more functional programming approach, you can convert the Set into an array using the Array.from method and then loop through the resulting array. Here’s an example:
“`
const mySet = new Set([1, 2, 3, 4, 5]);
const myArray = Array.from(mySet);
for (const item of myArray) {
console.log(item);
}
“`
In the above code snippet, we create a new Set called `mySet` and convert it into an array using Array.from. We then use the for…of loop to iterate over each element in the array and log it to the console. The output will be the same as the previous examples.
FAQs
Q: Can Sets contain objects and other complex data types?
A: Yes, Sets can contain objects and other complex data types. Each item within a Set is considered unique based on its value, even for objects. Two objects with the same contents will still be considered distinct items within a Set.
Q: Does the order of elements matter in a Set?
A: No, Sets are inherently unordered collections. The order in which elements are added to a Set is not preserved, and when looping through a Set, there is no guaranteed order in which the elements will be iterated.
Q: What happens if I try to add a duplicate value to a Set?
A: If you try to add a duplicate value to a Set, it will be ignored, as Sets only store unique values. The duplicate value will not throw an error or affect the existing elements in the Set.
Q: How do I check if a value exists in a Set?
A: You can use the `has` method of a Set to check whether a specific value exists within the Set. The `has` method returns a boolean value indicating whether the value is present or not.
Conclusion
Looping through a Set is an essential skill for JavaScript developers, as Sets are widely used to store unique values. In this article, we explored three different ways to loop through a Set: using the for…of loop, the forEach method, and converting the Set to an array. Each approach has its own advantages, and the choice depends on the specific use case. By leveraging these looping mechanisms, developers can efficiently process and manipulate the contents of Sets in their JavaScript applications.
Js Loop Through Map
JavaScript is one of the most popular programming languages used in web development. It provides many features and functionalities that make it easy to manipulate and process data. One such feature is the Map object, which allows you to store key-value pairs. In this article, we will explore how to loop through a Map in JavaScript, providing you with a comprehensive understanding of this topic.
Understanding the Map Object
The Map object is a built-in JavaScript object that is used to store and manipulate key-value pairs. Unlike the regular JavaScript object, the keys in a Map can be of any data type, including objects and functions. This makes it highly versatile and useful in a variety of scenarios.
Creating a Map
To create a Map object, you can simply use the Map constructor and assign it to a variable. For example:
“`
let map = new Map();
“`
Adding Key-Value Pairs
Once you have created a Map, you can start adding key-value pairs by using the `set()` method. Here’s an example of how you can add key-value pairs to a Map:
“`
map.set(“name”, “John”);
map.set(“age”, 30);
“`
Looping Through a Map Using the .forEach() Method
A simple and straightforward way to loop through a Map is by using the `forEach()` method. This method is available on the Map prototype and takes a callback function as a parameter. The callback function receives three arguments: the value, the key, and the map itself. Here’s an example:
“`
map.forEach((value, key) => {
console.log(key, value);
});
“`
This will print each key-value pair of the Map to the console.
Looping Through a Map Using the for…of Loop
Another way to loop through a Map is by using the `for…of` loop, which is supported by the iterable nature of the Map object. This loop allows you to iterate over the Map, accessing each key-value pair. Here’s an example:
“`
for (let [key, value] of map) {
console.log(key, value);
}
“`
In this example, we use destructuring assignment to access the key and value of each pair in the Map.
Looping Through a Map Using the .keys(), .values(), or .entries() Methods
The Map object also provides three methods: `.keys()`, `.values()`, and `.entries()`, which return an iterable object. You can use these methods in combination with a loop to iterate over the Map. Here’s an example:
“`
for (let key of map.keys()) {
console.log(key);
}
for (let value of map.values()) {
console.log(value);
}
for (let [key, value] of map.entries()) {
console.log(key, value);
}
“`
In these examples, we use the `.keys()`, `.values()`, and `.entries()` methods along with the `for…of` loop to iterate over the Map.
Frequently Asked Questions (FAQs)
Q: Can I change the order of items in a Map while looping through it?
A: No, the order of items in a Map is fixed based on their insertion order. You cannot change the order of items while looping through it.
Q: Can I use a Map without assigning it to a variable?
A: Yes, you can directly use the Map object without assigning it to a variable. However, it is recommended to assign it to a variable for easier access and manipulation.
Q: Can a Map have duplicate keys?
A: No, a Map cannot have duplicate keys. If you try to set a value with an existing key, it will overwrite the existing value.
Q: How can I check if a specific key exists in a Map before looping through it?
A: You can use the `has()` method of the Map object to check if a specific key exists. For example:
“`
if (map.has(“name”)) {
// Perform your operations here
}
“`
Q: Can I use nested Maps and loop through them?
A: Yes, you can have nested Maps and loop through them using any of the looping methods mentioned above. However, nested Maps can become complex, so it is recommended to use them judiciously.
In conclusion, the Map object in JavaScript provides a powerful way to store and manipulate key-value pairs. By understanding how to loop through a Map, you can easily access and process the data stored within it. Whether you choose to use the `forEach()` method, the `for…of` loop, or the `.keys()`, `.values()`, and `.entries()` methods, you now have the knowledge to efficiently iterate over a Map in JavaScript.
Images related to the topic javascript loop over set
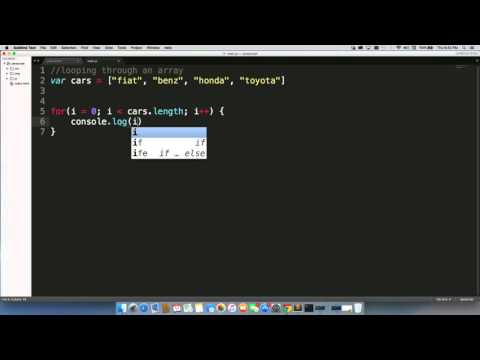
Found 23 images related to javascript loop over set theme

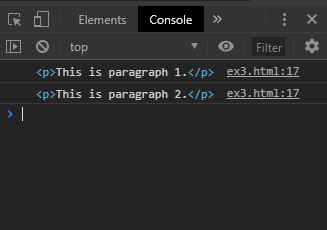
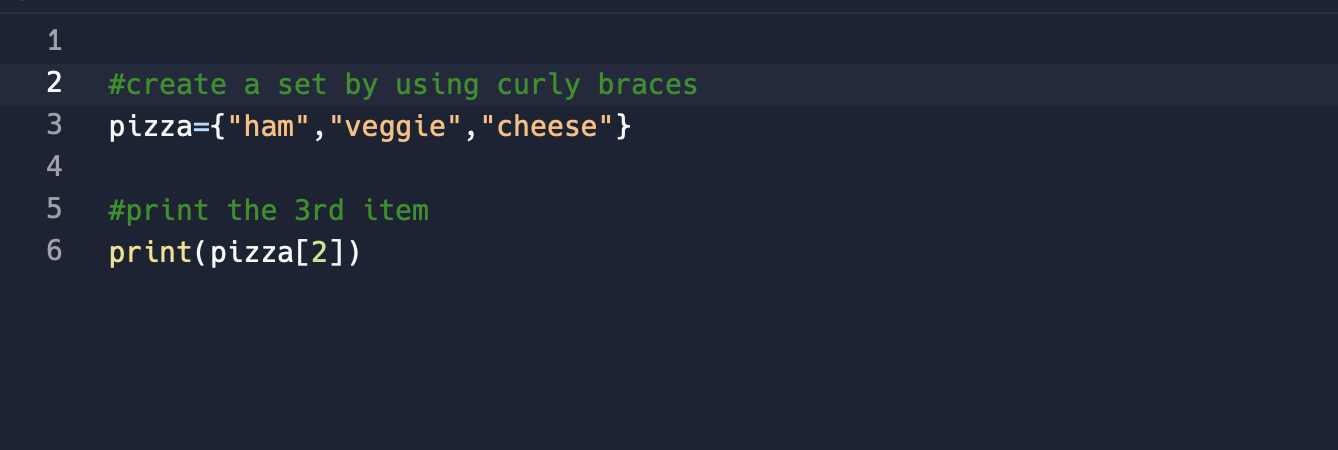
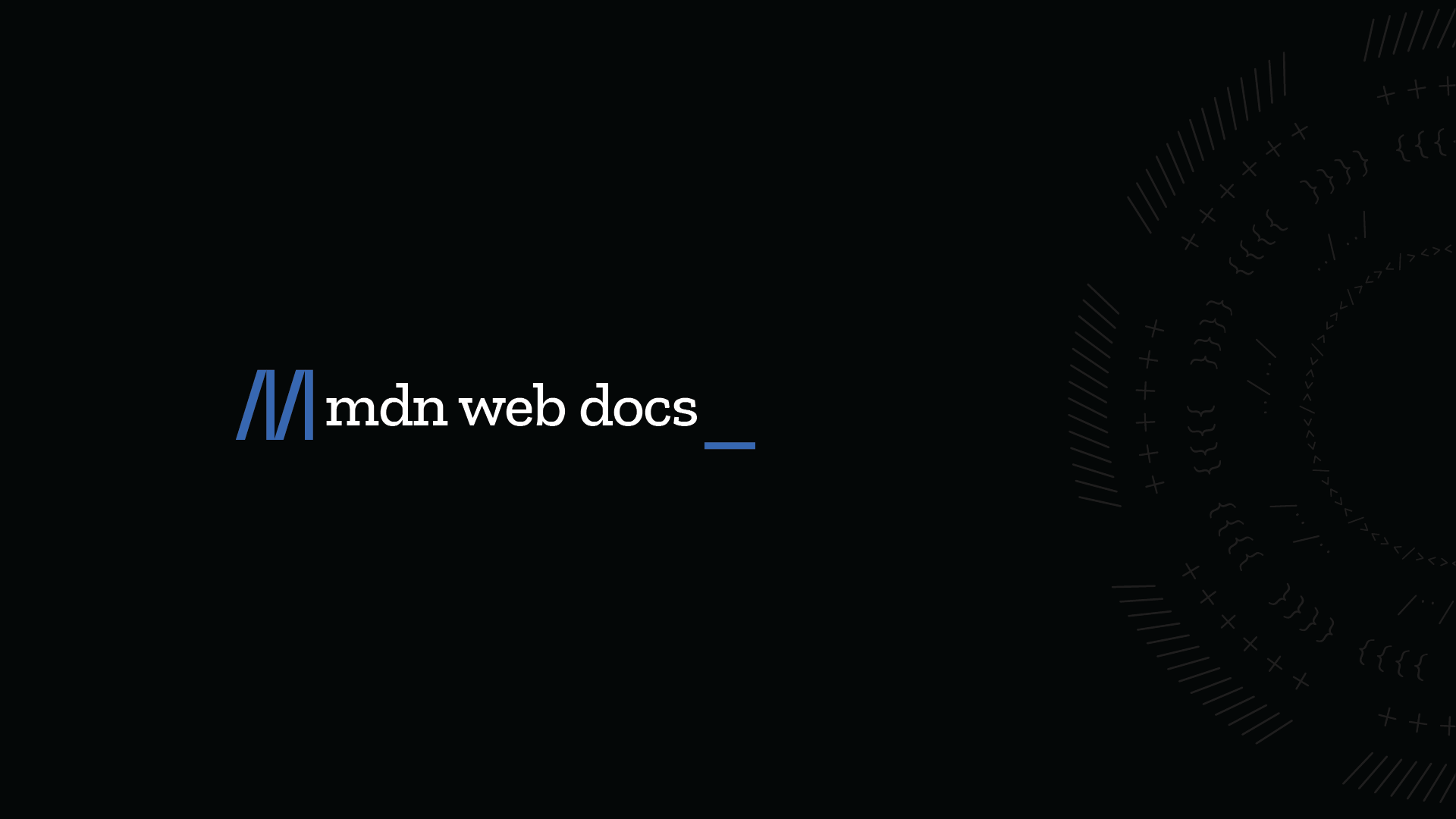
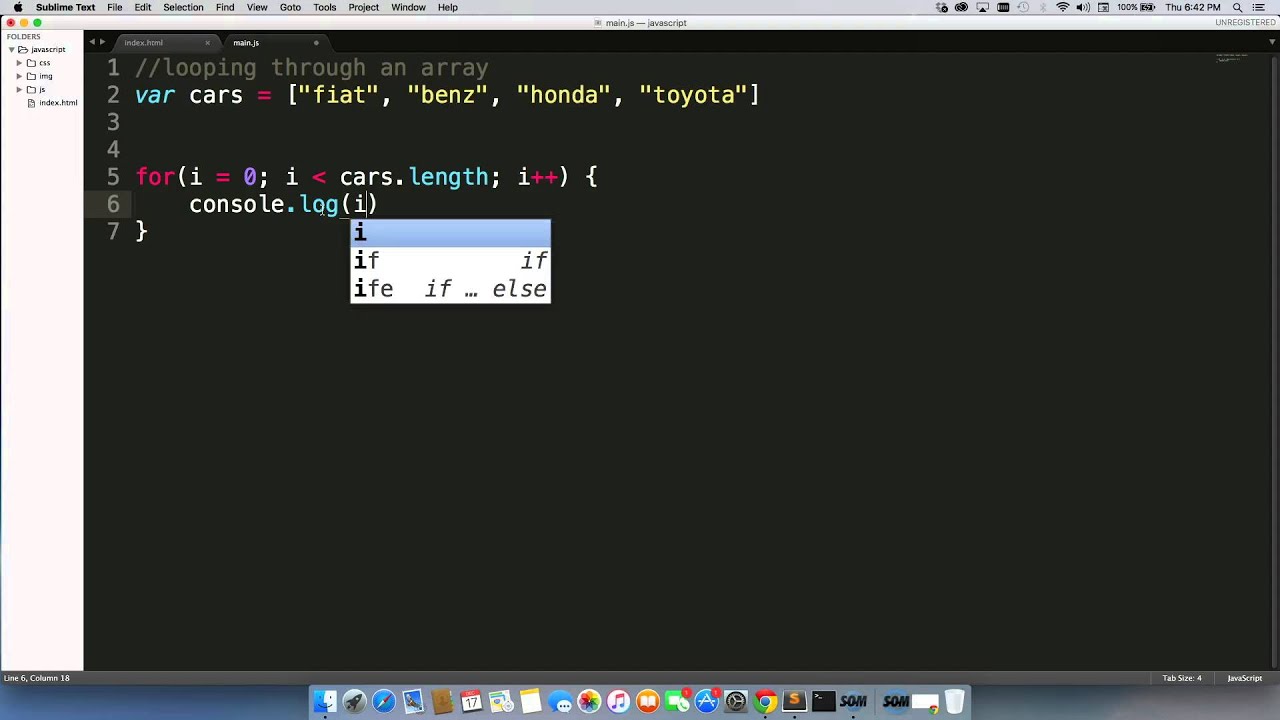
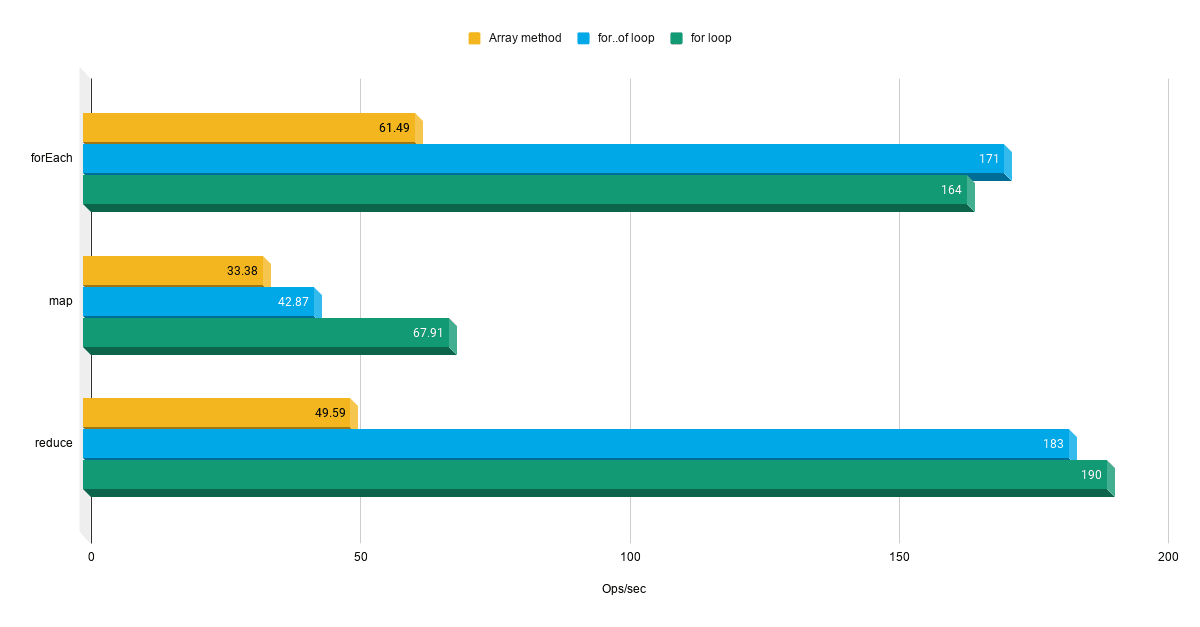
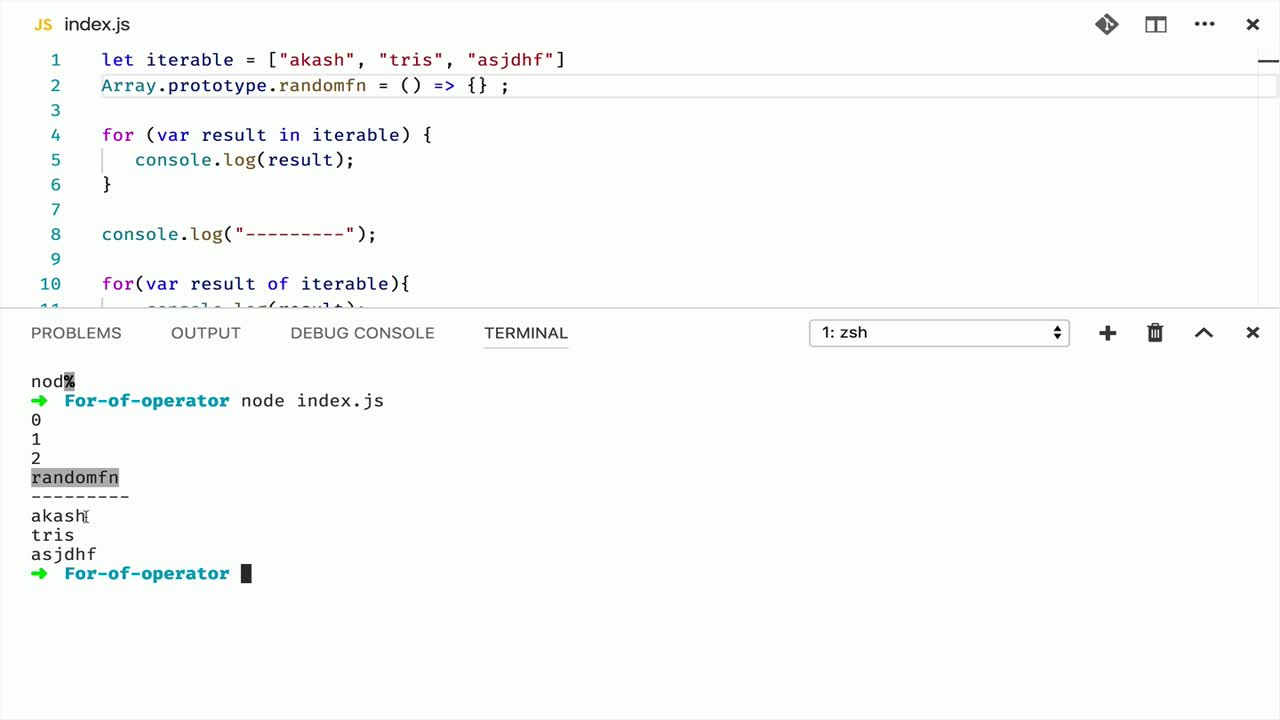
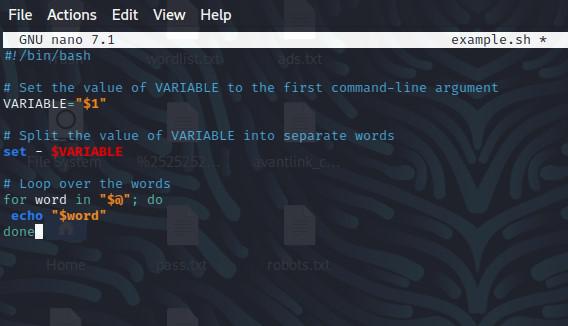
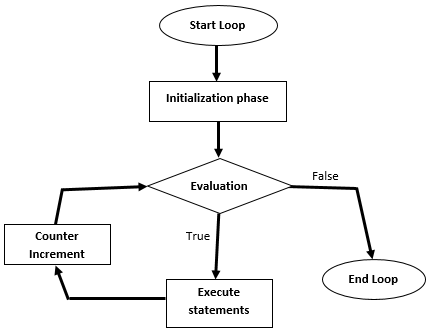
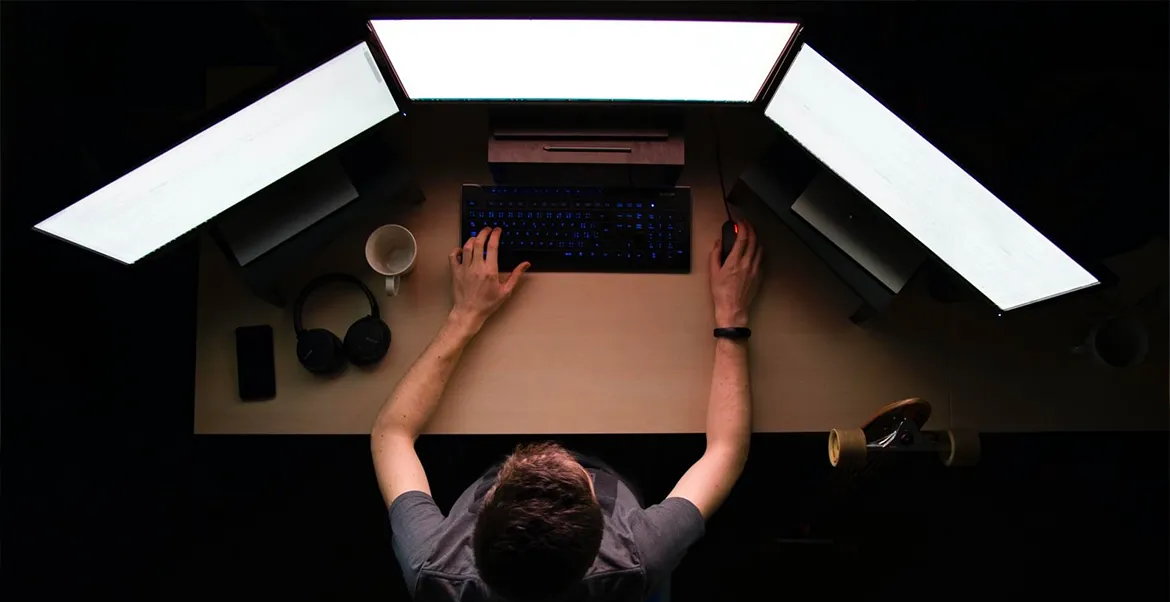

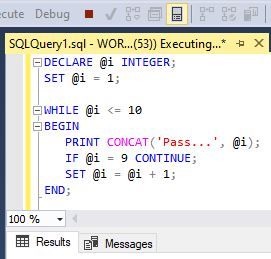
![PHP Loop: For, ForEach, While, Do While [Example] Php Loop: For, Foreach, While, Do While [Example]](https://www.guru99.com/images/2/php_for_loop.png)
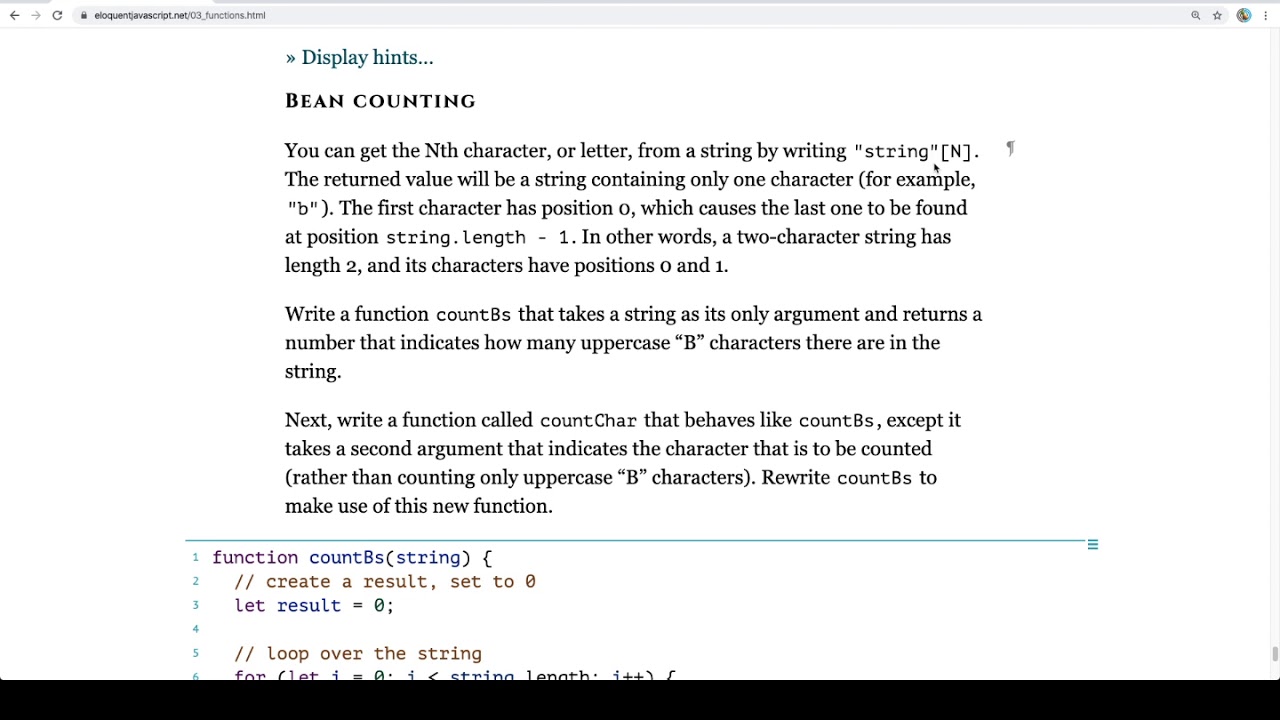
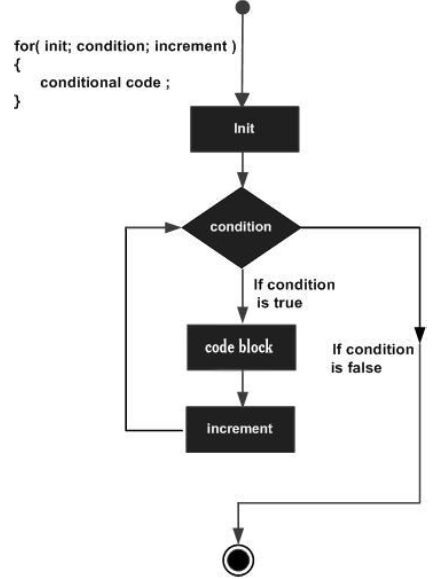

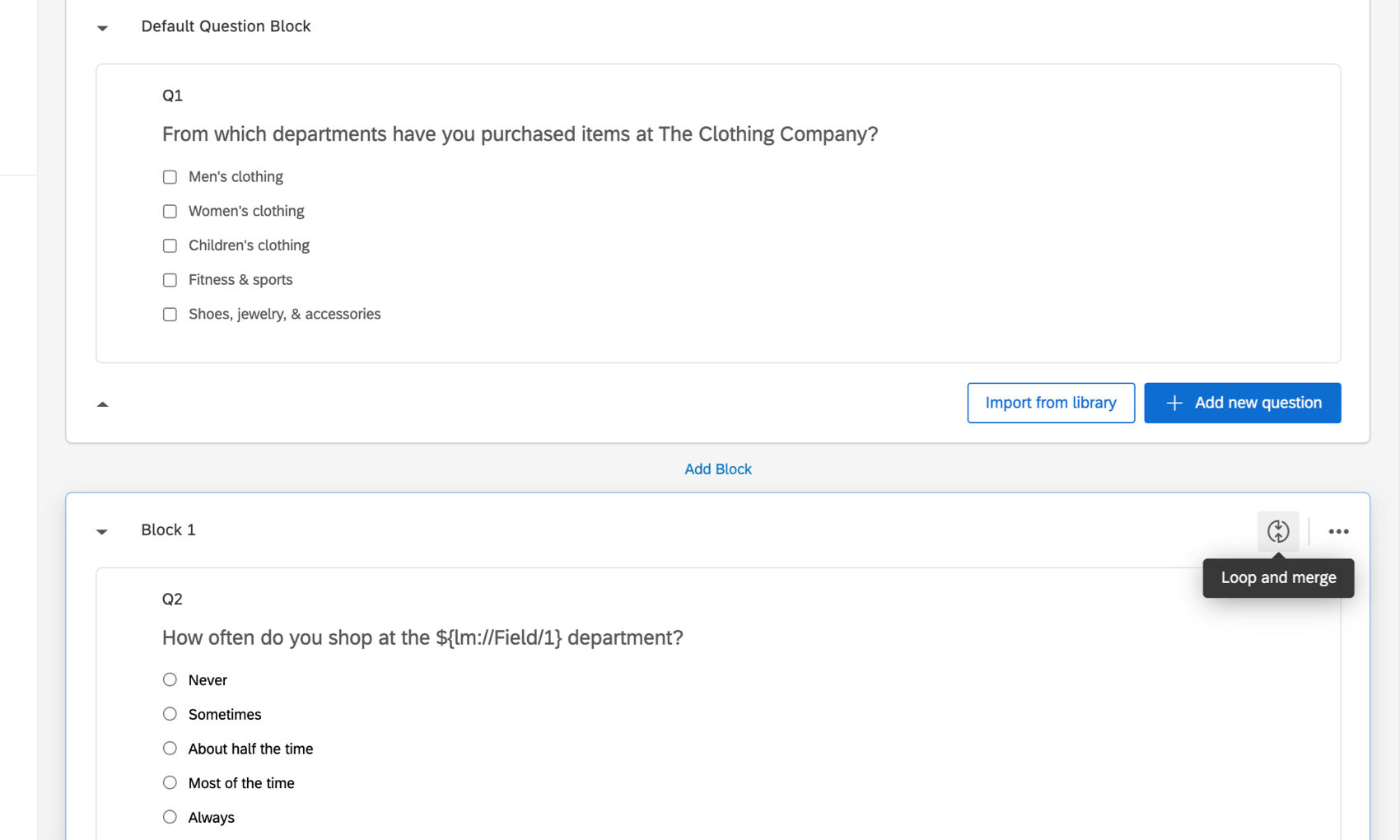
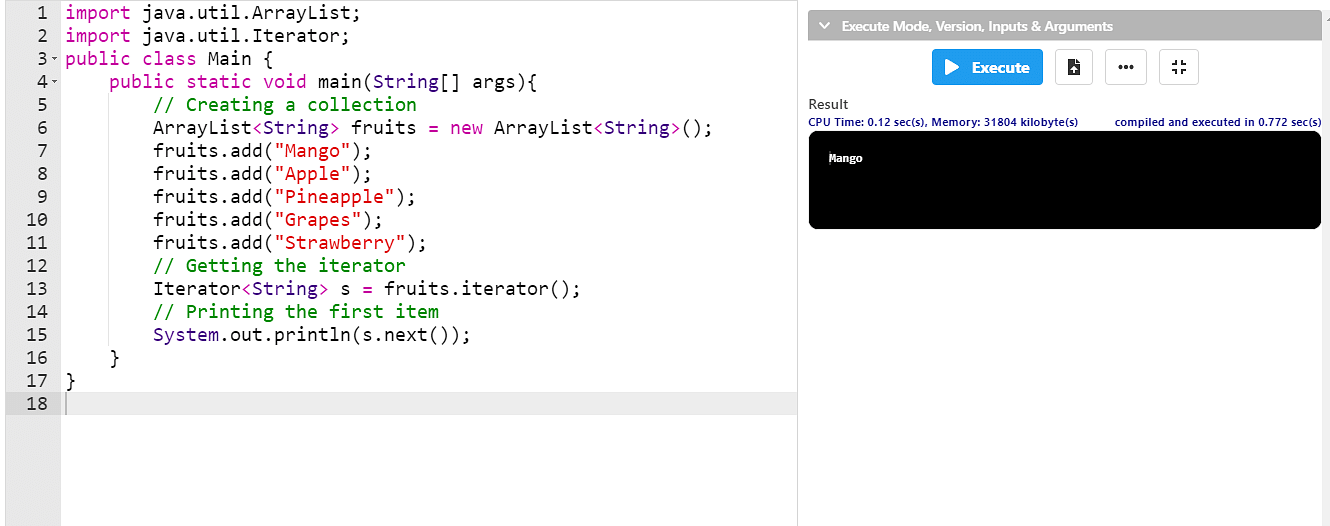
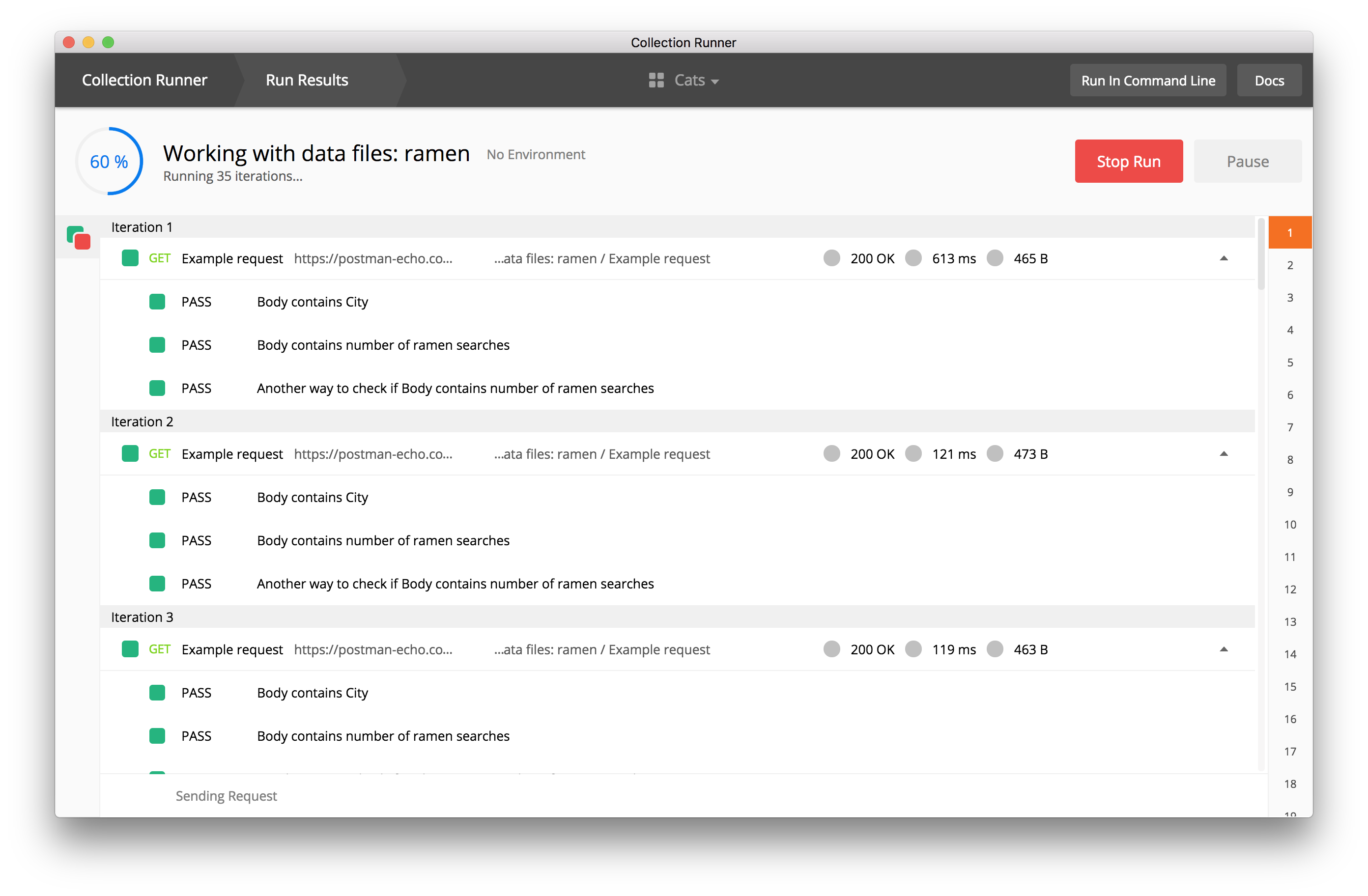
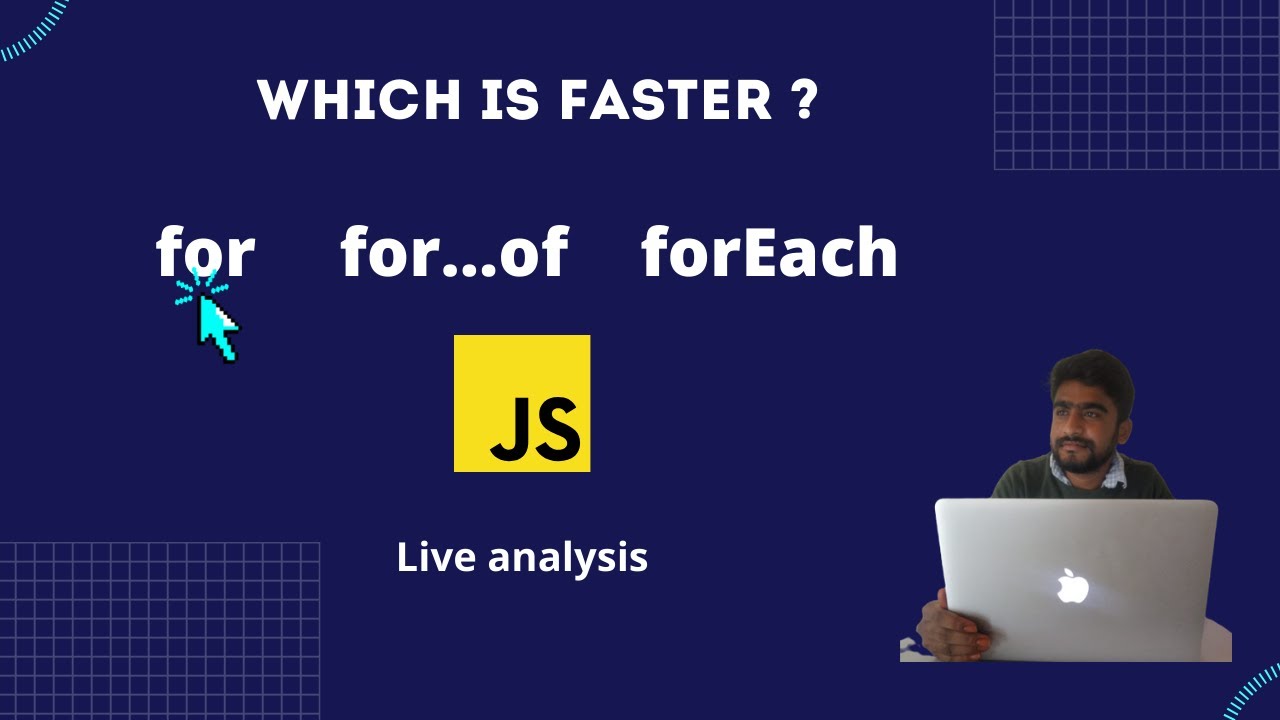
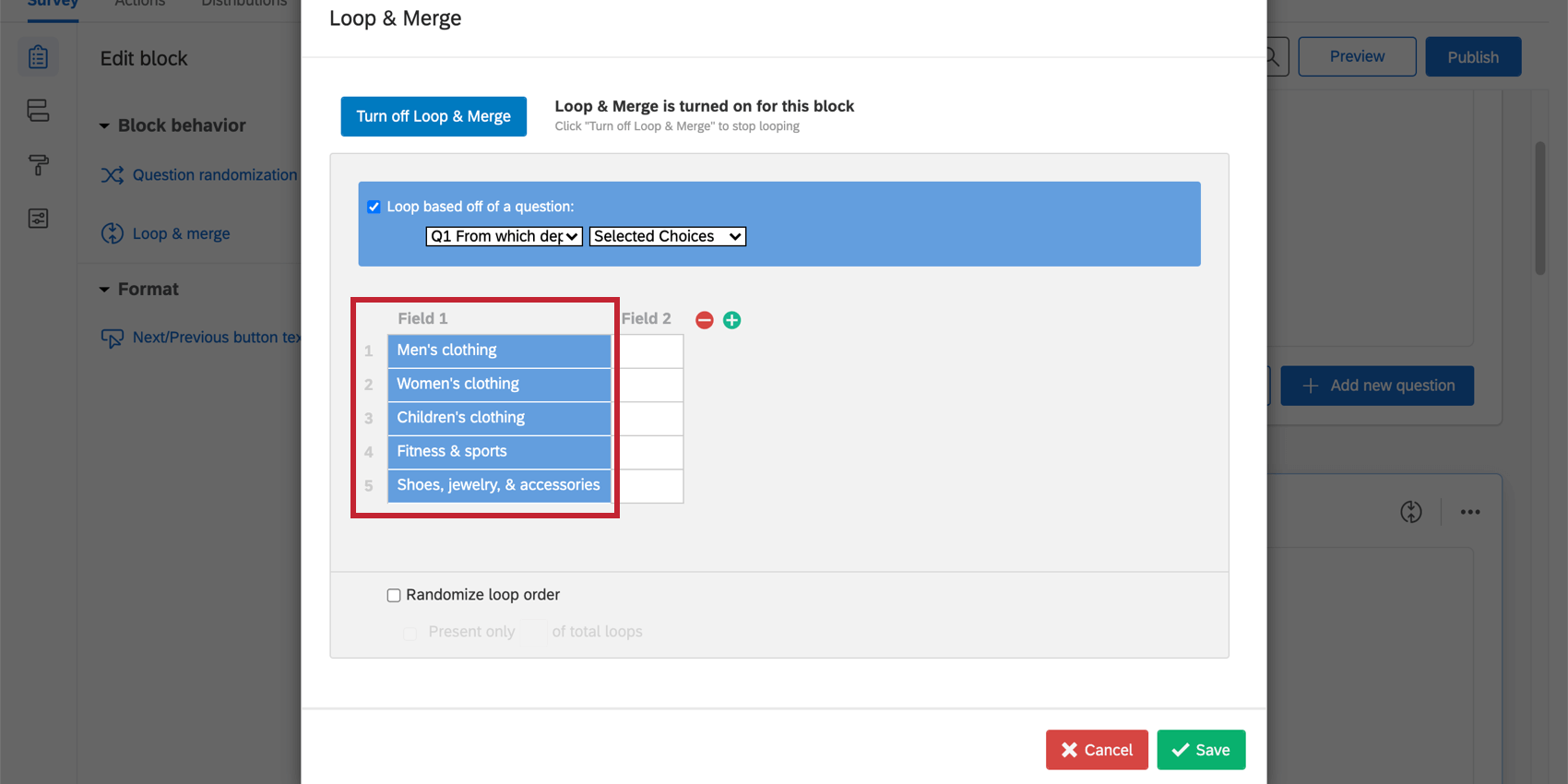
Article link: javascript loop over set.
Learn more about the topic javascript loop over set.
- Iterate over the Elements of a Set using JavaScript – bobbyhadz
- Iterate over set elements – javascript – Stack Overflow
- How to iterate over Set elements in JavaScript – GeeksforGeeks
- How to iterate over Set elements in JavaScript – GeeksforGeeks
- How to iterate over Elements of a Set in JavaScript?
- Python Loop Through Set Items – W3Schools
- Set.prototype.delete() – JavaScript – MDN Web Docs – Mozilla
- Set.prototype.forEach() – JavaScript – MDN Web Docs – Mozilla
- JavaScript Tutorial => Iterating Sets
- How to iterate over Elements of a Set in JavaScript?
- How to Iterate Over Elements of a JavaScript Set? – Designcise
- How to Loop Over a Set in TypeScript? – Linux Hint
- Loop through a Set using Javascript – Tutorialspoint
- Looping through Javascript Set object in React – JS-HowTo
See more: nhanvietluanvan.com/luat-hoc