Javascript If Else Shorthand
JavaScript, alongside HTML and CSS, is one of the core technologies employed in web development. When it comes to writing concise and efficient code, JavaScript offers various shorthand techniques, making programming tasks easier and more readable. Among these techniques, the JavaScript if else shorthand, also known as the Ternary Operator, is widely used in coding. In this article, we will explore the syntax, use cases, and differences between the Ternary Operator and the Nullish Coalescing Operator.
The Syntax of the Ternary Operator
The Ternary Operator allows you to express conditional statements in a simplified manner. Its syntax is as follows:
condition ? expression1 : expression2;
In this syntax, the “condition” is evaluated, and if it is true, the value of “expression1” is returned. If the condition is false, the value of “expression2” is returned instead. This shorthand reduces the need for the if-else statement, resulting in cleaner and more concise code.
Using the Ternary Operator for Simple Conditions
Let’s consider a simple example where we want to check if a number is even or odd and display a corresponding message. Traditionally, we would use an if-else statement. However, with the Ternary Operator, we can achieve the same result in a single line of code:
const number = 7;
const message = number % 2 === 0 ? “Even” : “Odd”;
console.log(message); // Output: Odd
In the example above, the condition “number % 2 === 0” checks if the number is divisible by 2 without any remainder. If true, the value of “message” becomes “Even”; otherwise, it becomes “Odd”. As we can see, the Ternary Operator provides a concise way to handle such simple conditions.
Using the Ternary Operator for Nesting Conditions
The Ternary Operator also allows us to handle nested conditions efficiently. Consider a scenario where we want to categorize a person’s age into three groups: “Child,” “Teenager,” and “Adult.” We can accomplish this using nested Ternary Operators:
const age = 20;
const category = age <= 12 ? "Child" : age <= 19 ? "Teenager" : "Adult";
console.log(category); // Output: Adult
In the above example, the first Ternary Operator checks if the age is less than or equal to 12. If true, the value of "category" becomes "Child." If false, another Ternary Operator is executed, checking if the age is less than or equal to 19. If true, the value becomes "Teenager." Otherwise, it becomes "Adult." This nesting capability of the Ternary Operator is particularly useful when multiple conditions need to be evaluated.
The Nullish Coalescing Operator
In addition to the Ternary Operator, JavaScript introduced the Nullish Coalescing Operator (??) in ES2020. While it shares some similarities with the Ternary Operator, they have distinct use cases.
The Difference Between Ternary Operator and Nullish Coalescing Operator
The Ternary Operator acts based on the truthiness or falsiness of a condition, while the Nullish Coalescing Operator focuses on the presence of a value being null or undefined.
Consider the following code snippet using the Ternary Operator:
const name = user.name ? user.name : "Unknown";
In this example, if the user's name exists, the value of "name" will be assigned to user.name. Otherwise, it will default to "Unknown" when the condition is false.
On the other hand, if we utilize the Nullish Coalescing Operator:
const name = user.name ?? "Unknown";
The value of "name" will only be assigned to user.name if it is not null or undefined. Otherwise, it defaults to "Unknown." This operator provides a cleaner approach when dealing with null-like values.
Combining Ternary Operator and Nullish Coalescing Operator
In some cases, you may find it beneficial to combine both the Ternary Operator and the Nullish Coalescing Operator. Consider the following example:
const message = number > 0 ? “Positive” : number < 0 ? "Negative" : "Zero";
In this case, we use the Ternary Operator to check if the number is positive, negative, or zero. Within each condition, we can further use the Nullish Coalescing Operator to handle more specific scenarios.
FAQs
Q: Is it possible to use the Ternary Operator without an 'else' statement?
A: Yes, it is possible to use the Ternary Operator without providing an 'else' statement. In this scenario, if the condition evaluates to false, the expression following the question mark will not be executed. You can simply end the line with the Ternary Operator, like "condition ? expression1;"
Q: Can an if...else statement be written on a single line in JavaScript?
A: Yes, with the use of the Ternary Operator, it is possible to write an if-else statement on a single line in JavaScript. This shorthand technique improves code readability and efficiency.
Q: Are there any best practices when using if...else statements in JavaScript?
A: Yes, it is recommended to use the Ternary Operator or the Nullish Coalescing Operator when possible, as they provide concise and readable code. However, for complex conditions or multiple statements, the traditional if...else statement may be more appropriate.
In conclusion, the JavaScript if else shorthand, also known as the Ternary Operator, offers a concise and efficient way to handle conditional statements. Whether for simple conditions or nested scenarios, the Ternary Operator simplifies code and improves readability. Additionally, the Nullish Coalescing Operator further enhances JavaScript's shorthand capabilities. By understanding and employing these shorthand techniques, you can write cleaner and more efficient code in JavaScript.
Keywords: JS shorthand if without else, If...else JS, If...else one line JavaScript, Shorthand if else Python, Shorten if statement javascript, If else best practices javascript, PHP if/else shorthand, Shorthand if elsejavascript if else shorthand.
Javascript: Shorthand For If-Else Statement
What Is The Shorthand Of If-Else In Javascript?
JavaScript is a versatile programming language that offers several shorthand techniques to simplify code and improve readability. Among these techniques, the shorthand of if-else statements is a powerful tool that reduces the amount of code required to achieve conditional logic. In this article, we will explore the different shorthand methods available in JavaScript to express if-else statements and delve into their advantages and limitations.
Understanding if-else statements
Before exploring the shorthand techniques, it is crucial to have a clear understanding of if-else statements. In JavaScript, if-else statements are used to execute certain code blocks based on specified conditions. The basic syntax of an if-else statement is as follows:
“`javascript
if (condition) {
// code to be executed if the condition is true
} else {
// code to be executed if the condition is false
}
“`
The above code structure checks a condition, and if it evaluates to true, the code within the if block is executed. If the condition evaluates to false, the code within the else block is executed instead.
Ternary operator ( ? : )
The ternary operator, denoted by `?` and `:`, is a concise shorthand method to express if-else statements. It provides a more compact syntax to evaluate a condition and return a value based on the result. The ternary operator syntax is as follows:
“`javascript
condition ? valueIfTrue : valueIfFalse;
“`
Let’s consider an example to illustrate the usage of the ternary operator:
“`javascript
const age = 18;
const isAdult = age >= 18 ? ‘Yes’ : ‘No’;
console.log(isAdult); // Output: Yes
“`
In the example above, we have assigned the value `’Yes’` to the variable `isAdult` if `age` is greater than or equal to 18. If the condition is false, the value `’No’` is assigned instead. This shorthand method provides a more concise and readable approach to express the logic in a single line.
Short-circuit evaluation ( && and || )
Another shorthand technique to achieve if-else behavior is through the use of logical operators like `&&` (AND) and `||` (OR). These operators can be leveraged to evaluate multiple conditions and return values accordingly.
The `&&` operator evaluates the conditions from left to right and returns the first falsy value encountered, or the last truthy value if all conditions are true. The `||` operator, on the other hand, returns the first truthy value encountered, or the last value if all conditions are falsy.
Let’s take an example to further understand short-circuit evaluation:
“`javascript
const isWeatherGood = true;
const outdoorActivity = isWeatherGood && ‘Go for a hike’;
console.log(outdoorActivity); // Output: Go for a hike
“`
In the example above, the code assigns the value `’Go for a hike’` to `outdoorActivity` only if `isWeatherGood` evaluates to true. If `isWeatherGood` is false, the expression short-circuits, and `outdoorActivity` will not be assigned any value.
FAQs
Q: Are there any limitations to using shorthand if-else statements?
A: While shorthand if-else statements offer compact syntax and can enhance code readability, they might not always be the best choice. If the code within the if or else block is complex, it is advised to use the traditional if-else structure for better maintainability and readability.
Q: Can I nest shorthand if-else statements?
A: Yes, you can nest shorthand if-else statements within one another. However, nesting them extensively can lead to less readable code. In such cases, it is recommended to use traditional if-else statements for better clarity.
Q: Are there any performance differences between shorthand and traditional if-else statements?
A: Generally, there are no significant performance differences between shorthand and traditional if-else statements. However, it is vital to write code that is easily understandable and maintainable, rather than optimizing solely for performance.
Q: Can I use shorthand if-else statements in other programming languages?
A: While the specific syntax may vary between programming languages, most languages provide similar if-else shorthand techniques. It is advised to consult the official documentation of the respective language to understand the shorthand if-else syntax.
In conclusion, JavaScript offers multiple shorthand methods to express if-else statements, including the ternary operator and short-circuit evaluation. These techniques enable developers to write more concise and readable code. However, it is essential to consider the complexity of the code and maintainability before deciding to use shorthand if-else statements extensively. By understanding these shorthand techniques, developers can improve their coding efficiency and write more elegant JavaScript code.
What Is The Shorthand For If-Else In Typescript?
TypeScript is a superset of JavaScript that introduces static typing to the JavaScript ecosystem. Being a statically typed language, TypeScript provides numerous features to enhance code readability and maintainability. One such feature is the shorthand for if-else statements. In TypeScript, this shorthand is commonly referred to as the conditional (ternary) operator.
The conditional operator is used to express if-else logic in a concise and elegant manner. It takes three operands: a condition, a value to be returned if the condition evaluates to true, and a value to be returned if the condition evaluates to false. The general syntax for the conditional operator is as follows:
condition ? valueIfTrue : valueIfFalse
Let’s dive into some examples to further understand how this shorthand for if-else statements works in TypeScript.
Example 1:
“`typescript
const age: number = 18;
const isAdult: string = age >= 18 ? ‘Yes’ : ‘No’;
console.log(isAdult); // Output: Yes
“`
In this example, we have a variable `age` that holds the value 18. Using the conditional operator, we check if the `age` is greater than or equal to 18. If the condition evaluates to true, the value `’Yes’` is assigned to `isAdult`, otherwise, the value `’No’` is assigned. As the `age` is exactly 18, the condition evaluates to true, which results in the value `’Yes’` being assigned to `isAdult`. Finally, we log the value of `isAdult`, which outputs `’Yes’` to the console.
Example 2:
“`typescript
const points: number = 70;
const grade: string = points >= 90 ? ‘A’ : points >= 80 ? ‘B’ : points >= 70 ? ‘C’ : points >= 60 ? ‘D’ : ‘F’;
console.log(grade); // Output: C
“`
In this example, we have a variable `points` that holds the value 70. The conditional operator is used multiple times to determine the corresponding grade based on the number of points. If `points` is greater than or equal to 90, the grade is assigned `’A’`. If not, the conditional operator is used again to check if `points` is greater than or equal to 80. This process continues for each grade until it reaches the final conditional expression `’F’`. As `points` is 70, the condition for `’C’` is satisfied, and the value `’C’` is assigned to `grade`. The result is logged to the console, outputting `’C’`.
Using the shorthand for if-else statements can greatly simplify and enhance the readability of your code. However, it is important to note that complex logic or multiple conditions might adversely impact code comprehension. Therefore, it is crucial to strike a balance between code brevity and clarity.
Frequently Asked Questions (FAQs):
1. Can the shorthand for if-else be nested?
Yes, the shorthand for if-else can be nested. You can use multiple conditional operators to express complex if-else logic within a single statement. However, it is advisable to ensure that the code remains easily understandable.
Example:
“`typescript
const value: number = 10;
const result: string = value > 0 ? ‘Positive’ : value < 0 ? 'Negative' : 'Zero';
console.log(result); // Output: Positive
```
In this example, the result is determined based on whether the `value` is positive, negative, or zero. If `value` is greater than zero, the first condition is satisfied, and `'Positive'` is assigned to `result`. Otherwise, the condition for negative numbers is checked. If `value` is less than zero, `'Negative'` is assigned; otherwise, if none of the conditions are met, `'Zero'` is assigned.
2. Can the shorthand for if-else be used with different data types?
Yes, the shorthand for if-else can be used with different data types in TypeScript. The condition can be any expression that evaluates to a boolean, and the values returned can be of any compatible types. TypeScript's type inference system ensures type safety while using the conditional operator.
Example:
```typescript
const isLoggedIn: boolean = true;
const greeting: string = isLoggedIn ? 'Welcome back!' : 'Please log in.';
console.log(greeting); // Output: Welcome back!
```
In this example, the value of `isLoggedIn` is used as a condition. If it is true, `'Welcome back!'` is assigned to `greeting`; otherwise, `'Please log in.'` is assigned. The type of `greeting` is inferred as a string, ensuring type safety.
In conclusion, the shorthand for if-else statements, known as the conditional (ternary) operator, offers a concise and expressive approach to handle simple if-else logic in TypeScript. Its usage can enhance code readability and maintainability. However, it is important to use it judiciously and avoid excessive nesting to ensure code comprehension.
Keywords searched by users: javascript if else shorthand JS shorthand if without else, If…else JS, If…else one line JavaScript, Shorthand if else Python, Shorten if statement javascript, If else best practices javascript, PHP if/else shorthand, Short hand if else
Categories: Top 41 Javascript If Else Shorthand
See more here: nhanvietluanvan.com
Js Shorthand If Without Else
In JavaScript (JS), conditional statements are an essential part of programming logic. They allow us to control the flow of our code based on certain conditions. One commonly used conditional statement is the “if” statement, which executes a block of code if a specified condition evaluates to true. Typically, this is followed by an “else” statement to handle the code execution when the condition is false. However, there exists a shorthand if statement without the else clause, which provides a more compact and concise way to handle single condition scenarios. In this article, we will explore the JS shorthand if statement without else and explain how it works.
How does the Shorthand If Without Else work?
The shorthand if statement without else is also known as the ternary operator, represented by the “?” symbol in JS. It offers a shorter syntax to evaluate a condition and execute one of two expressions based on the condition’s outcome. Its general structure is as follows:
condition ? expression1 : expression2
Here, “condition” is an expression that evaluates to either true or false. If the condition is true, the expression1 gets executed; otherwise, expression2 gets executed. This format allows for compact representation of simple conditional tasks in a single line of code.
Example Usage:
Let’s consider an example scenario where we want to check if a user is logged in and display a different message accordingly. Using the shorthand if without else, we can write the code like this:
const isLoggedIn = true;
const message = isLoggedIn ? “Welcome back!” : “Please log in.”;
In this example, if the variable isLoggedIn is true, the value assigned to the message variable will be “Welcome back!”. Conversely, if isLoggedIn is false, the value assigned will be “Please log in.” This compact syntax eliminates the need for traditional if-else statements, making the code more concise and readable.
Benefits of Using Shorthand If Without Else:
1. Readability: The ternary operator condenses the code into a single line, making it easier to read and understand. It eliminates unnecessary braces and excessive indentation, especially when dealing with simple conditional statements.
2. Conciseness: By omitting the else clause, the shorthand if statement reduces the number of lines required for conditional tasks, resulting in more compact and efficient code.
3. Ease of Maintenance: With a shorter syntax, code modifications and updates are simpler to implement, reducing the chances of introducing errors.
4. Improved Performance: Although the performance difference between traditional if-else statements and the ternary operator is minimal, the compact nature of the shorthand if can enhance execution speed in some scenarios.
FAQs:
Q: Is the shorthand if statement without else limited to simple conditions only?
A: No, the shorthand if statement can handle more complex conditions by nesting it within other ternary operators. For example:
const age = 20;
const message = age >= 18 ? “You are an adult” : (age >= 13 ? “You are a teenager” : “You are a child”);
Q: Can multiple expressions be executed within the shorthand if statement without else?
A: No, the ternary operator can only execute one expression for each outcome. If multiple expressions are required, it is recommended to use the traditional if-else statement.
Q: Are there any drawbacks to using the shorthand if statement without else?
A: The compact nature of the shorthand if can sometimes sacrifice readability, especially when the expressions become lengthy or complex. It is crucial to strike a balance between brevity and code clarity.
Q: Is it possible to use the shorthand if statement without else within a larger block of code?
A: Yes, the ternary operator can be embedded within code blocks and used in conjunction with other JavaScript syntax, such as variable assignments or function calls.
In conclusion, the shorthand if statement without else, also known as the ternary operator, provides a concise and readable way to handle simple conditional statements in JavaScript. It offers improved code efficiency, maintenance, and performance, making it a valuable addition to any developer’s toolkit. However, a careful balance between brevity and readability should always be maintained.
If…Else Js
Understanding If…else Statements in JavaScript
Conditional statements are essential in programming as they enable developers to control the flow of their code based on certain conditions. The If…else statement is one such conditional construct that allows for the execution of different blocks of code depending on whether a specified condition evaluates to true or false.
The basic syntax for an If…else statement in JavaScript is as follows:
“`
if (condition) {
// Block of code to be executed if the condition is true
} else {
// Block of code to be executed if the condition is false
}
“`
Here, the condition can be any expression that evaluates to either true or false. If the condition evaluates to true, the code block within the if statement will be executed. Conversely, if the condition evaluates to false, the code block within the else statement will be executed.
Multiple If…else statements can also be nested within one another, allowing for more complex conditional behavior. This can be achieved using the if…else if…else syntax:
“`
if (condition1) {
// Block of code to be executed if condition1 is true
} else if (condition2) {
// Block of code to be executed if condition2 is true
} else {
// Block of code to be executed if all conditions are false
}
“`
In this case, if condition1 is true, the code block within the first if statement will be executed. If condition1 is false and condition2 is true, the code block within the else if statement will be executed. If both condition1 and condition2 are false, the code block within the else statement will be executed.
Common Use Cases for If…else Statements
If…else statements are extensively used in JS programming, and they offer remarkable flexibility in controlling program flow. Here are a few common use cases:
1. Validating User Input: If…else statements are often used to validate user input. For example, when a user submits a form, an If…else statement can be employed to check if they have entered valid data. If the input is valid, the code within the if block can be executed, and if it is invalid, the code within the else block can display an error message.
2. Implementing Navigation: If…else statements play a crucial role in website navigation. Using the current page URL or other variables, developers can determine the active page and highlight the corresponding link.
3. Handling Conditions: If…else statements are ideal for handling various conditions and performing different calculations based on those conditions. For instance, based on the value of a variable, calculations can be performed differently.
4. Controlling Loop Execution: If…else statements are often used within loops to control their execution. By incorporating an If…else condition within a loop, developers can choose to continue or break the loop based on specific criteria.
Frequently Asked Questions (FAQs)
Q1. Can an If statement be used without an else statement?
Yes, an If statement can be used without an else statement. In such cases, if the condition evaluates to true, the code within the If block will be executed, and if the condition evaluates to false, nothing will happen.
Q2. How many else if statements can be used in a single If…else statement?
There is no specific limit to the number of else if statements that can be used in a single If…else statement. Developers can add as many else if statements as necessary, depending on the complexity of the conditions they need to evaluate.
Q3. Can there be multiple if blocks within an If…else statement?
No, an If…else statement can only have one if block. However, multiple If…else statements can be nested within each other if multiple conditions need to be evaluated.
Conclusion
If…else statements are an indispensable part of JavaScript programming, allowing developers to create dynamic and personalized websites and applications. With the ability to control program flow based on specified conditions, these conditional statements empower programmers to implement various functionalities with ease. By understanding the syntax and applications of If…else statements, developers can enhance the interactivity and efficiency of their JS code.
Images related to the topic javascript if else shorthand
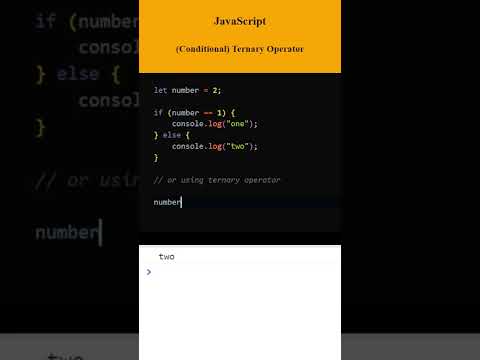
Found 21 images related to javascript if else shorthand theme
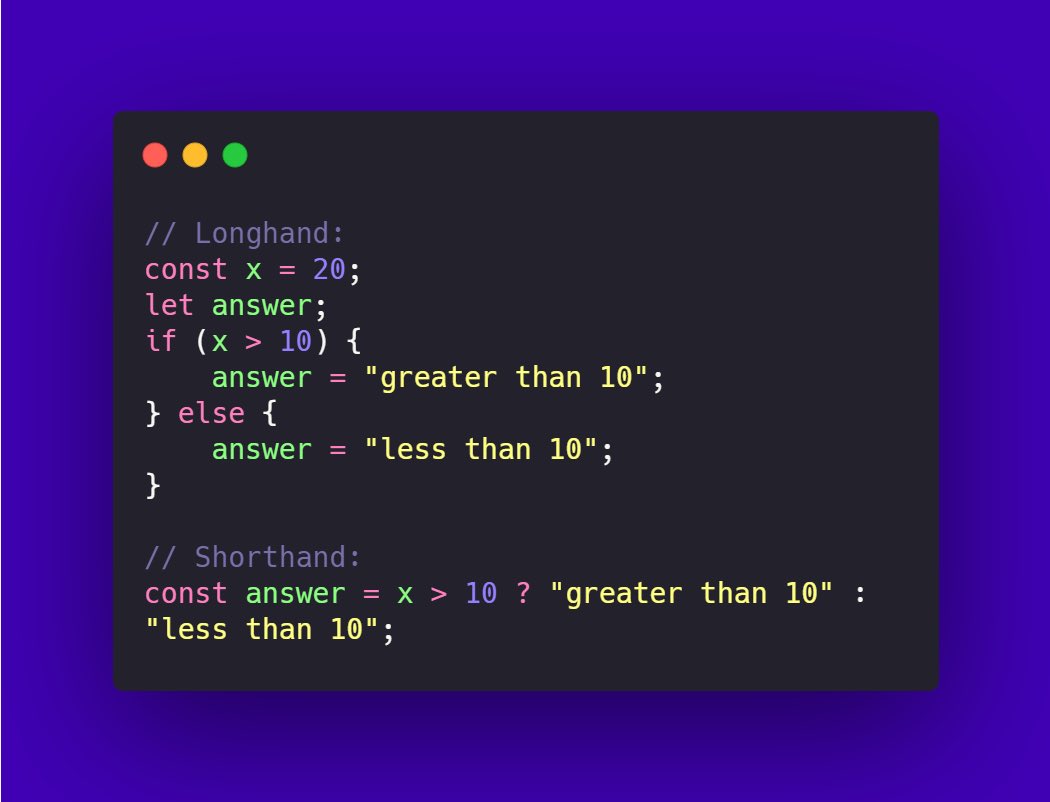
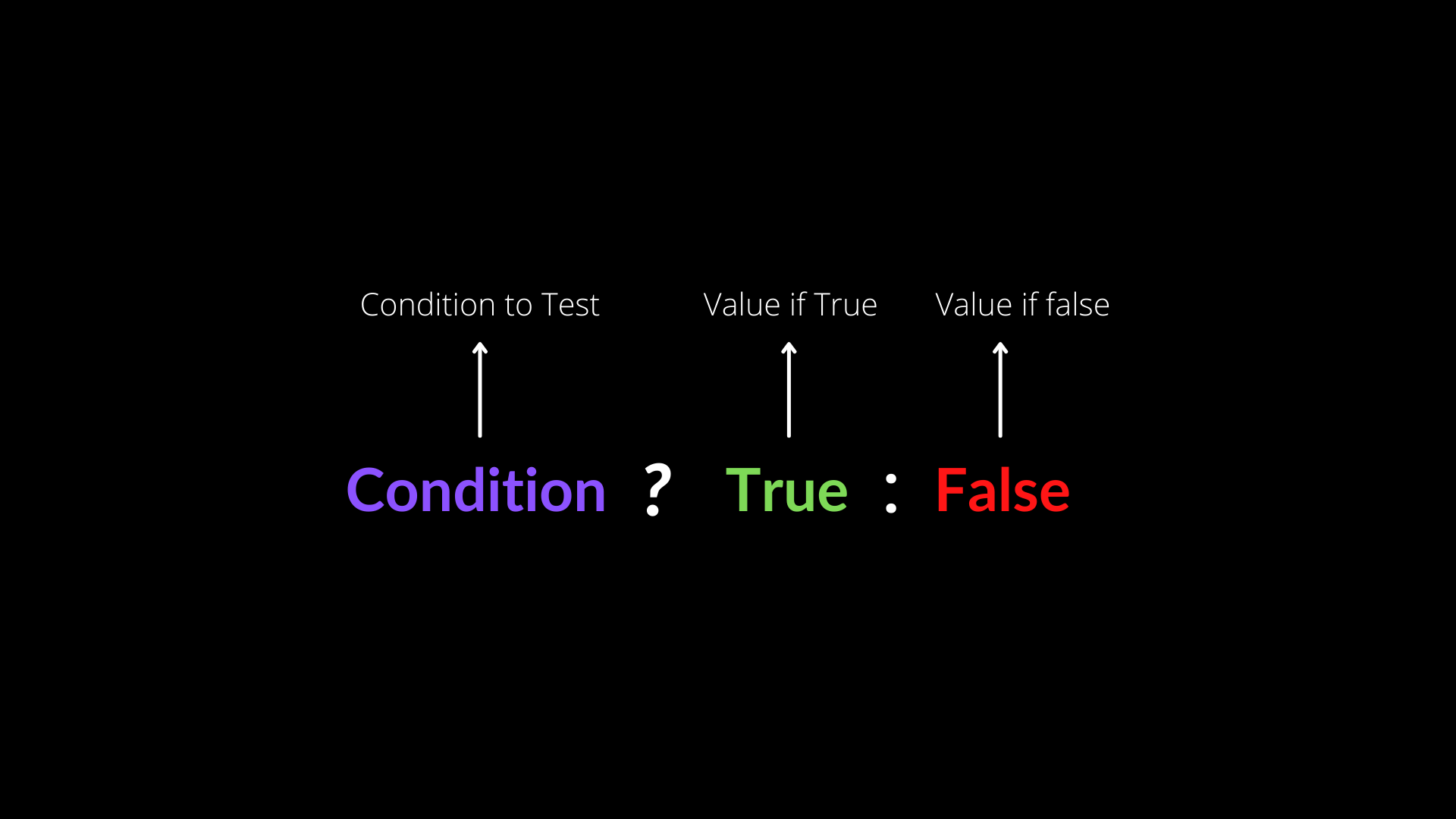
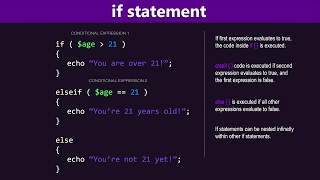
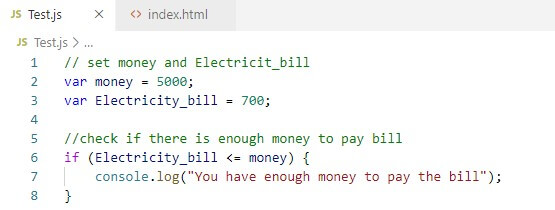
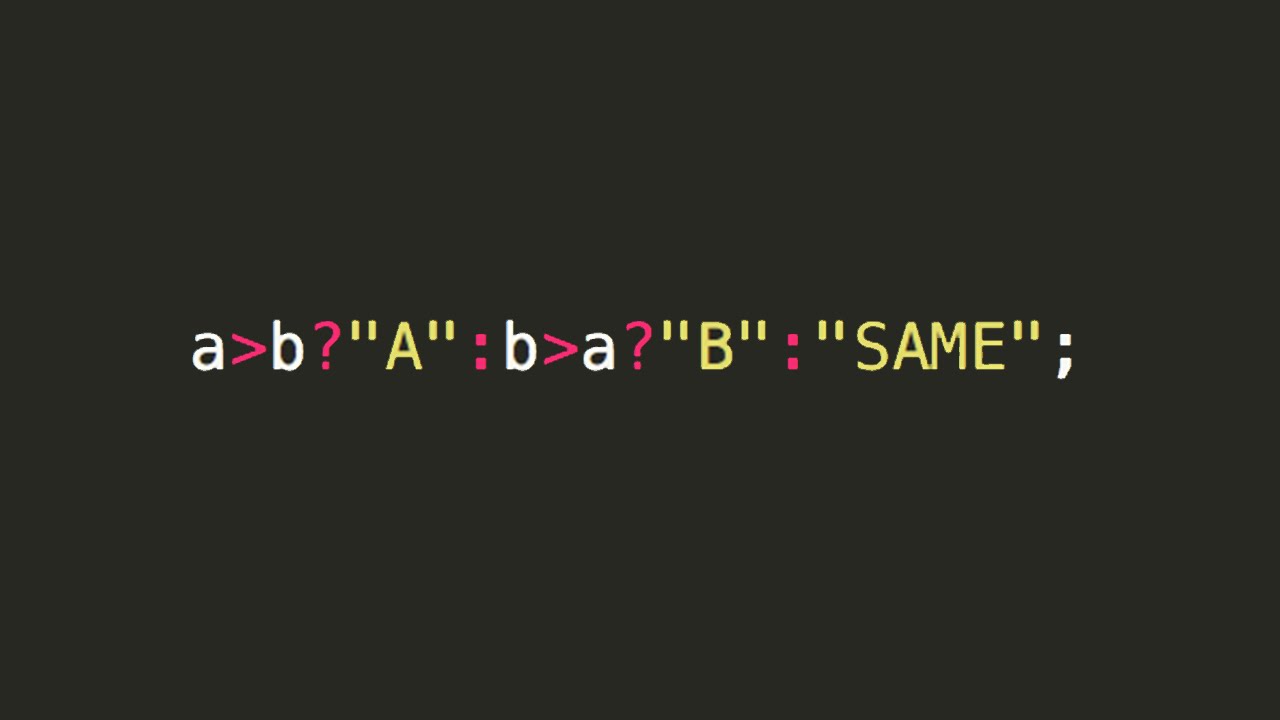
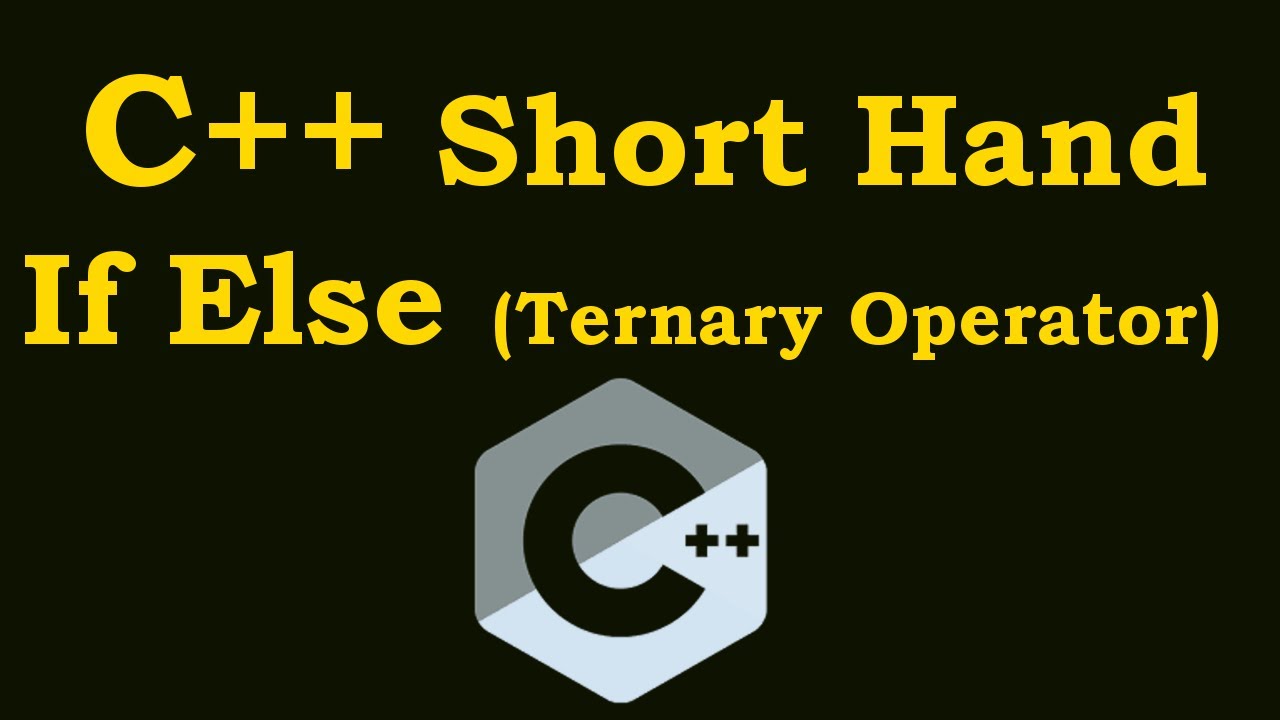



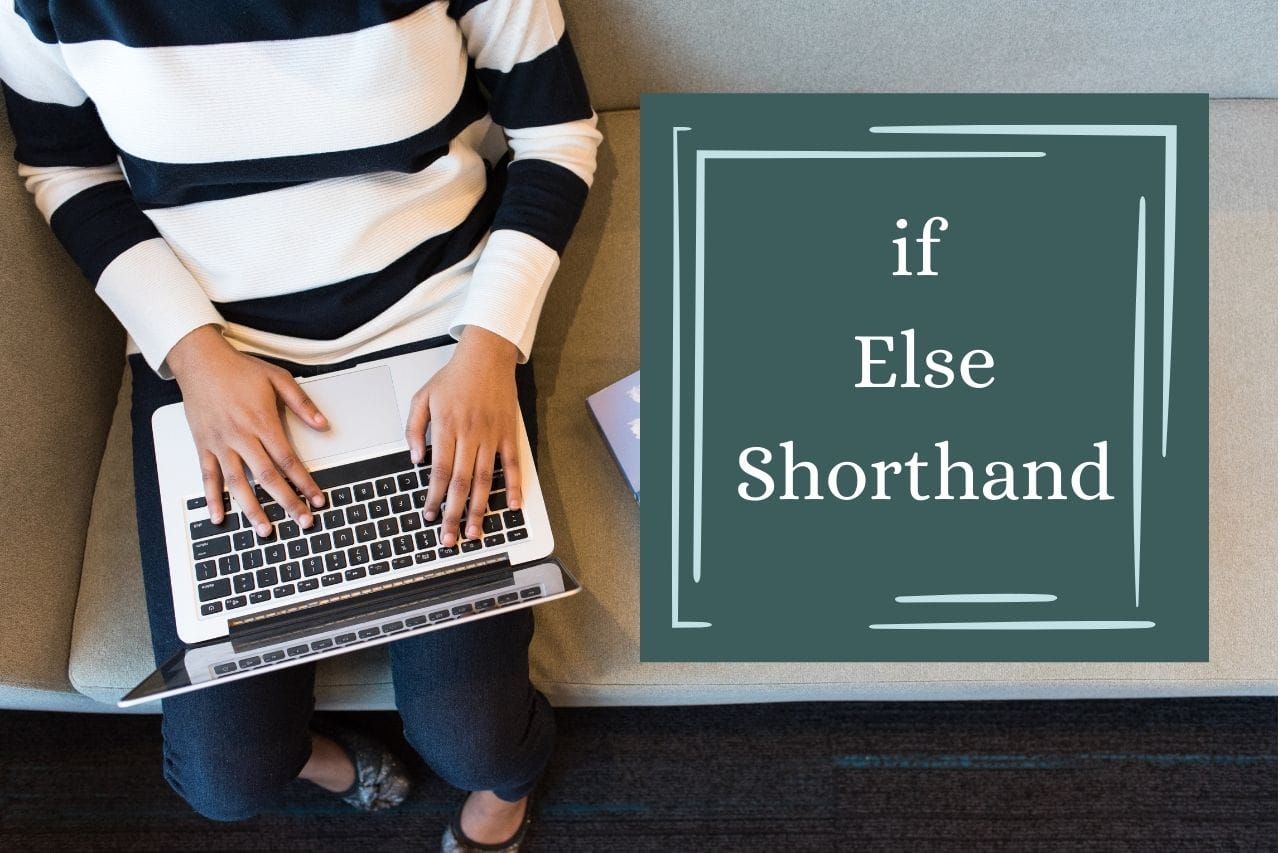
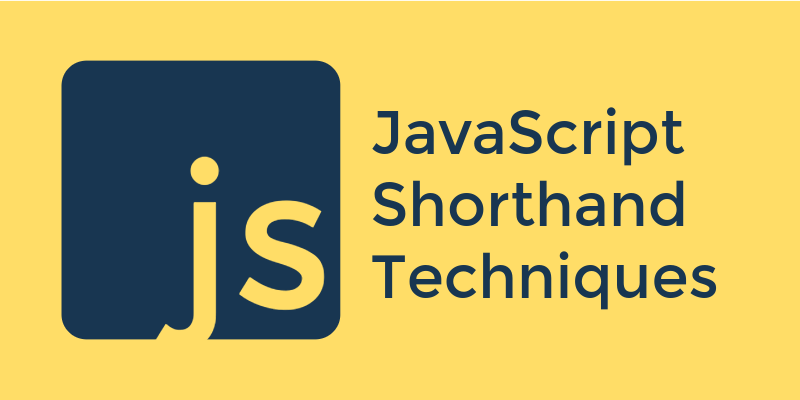
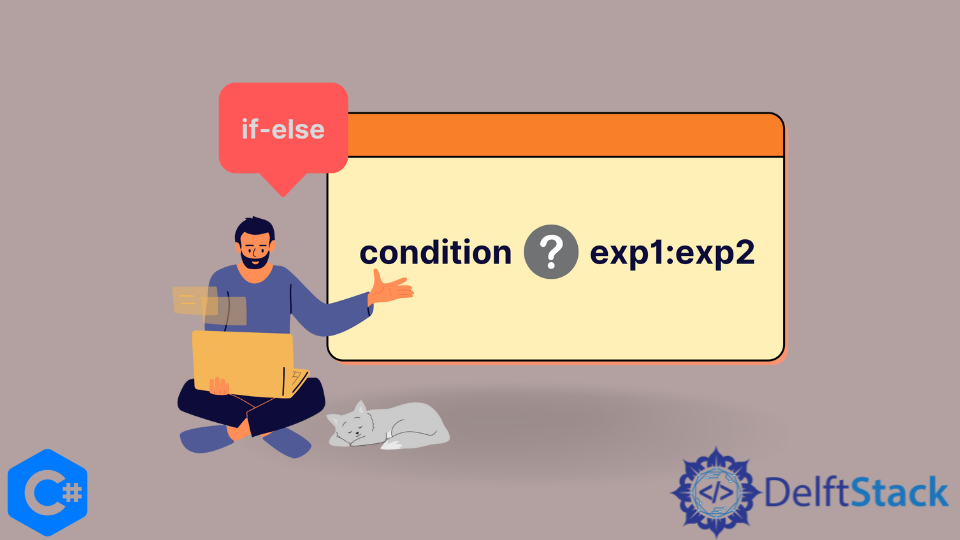
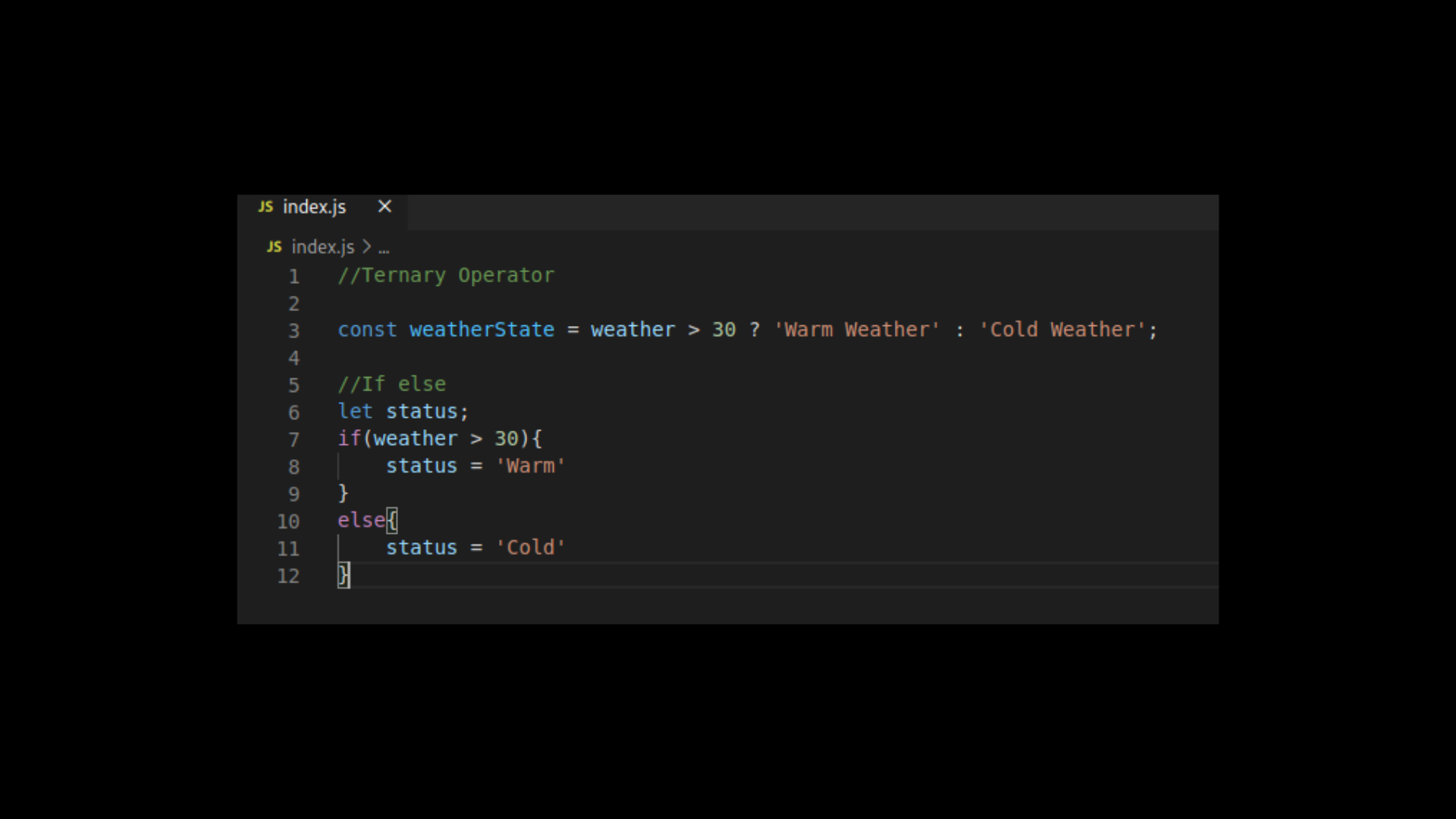
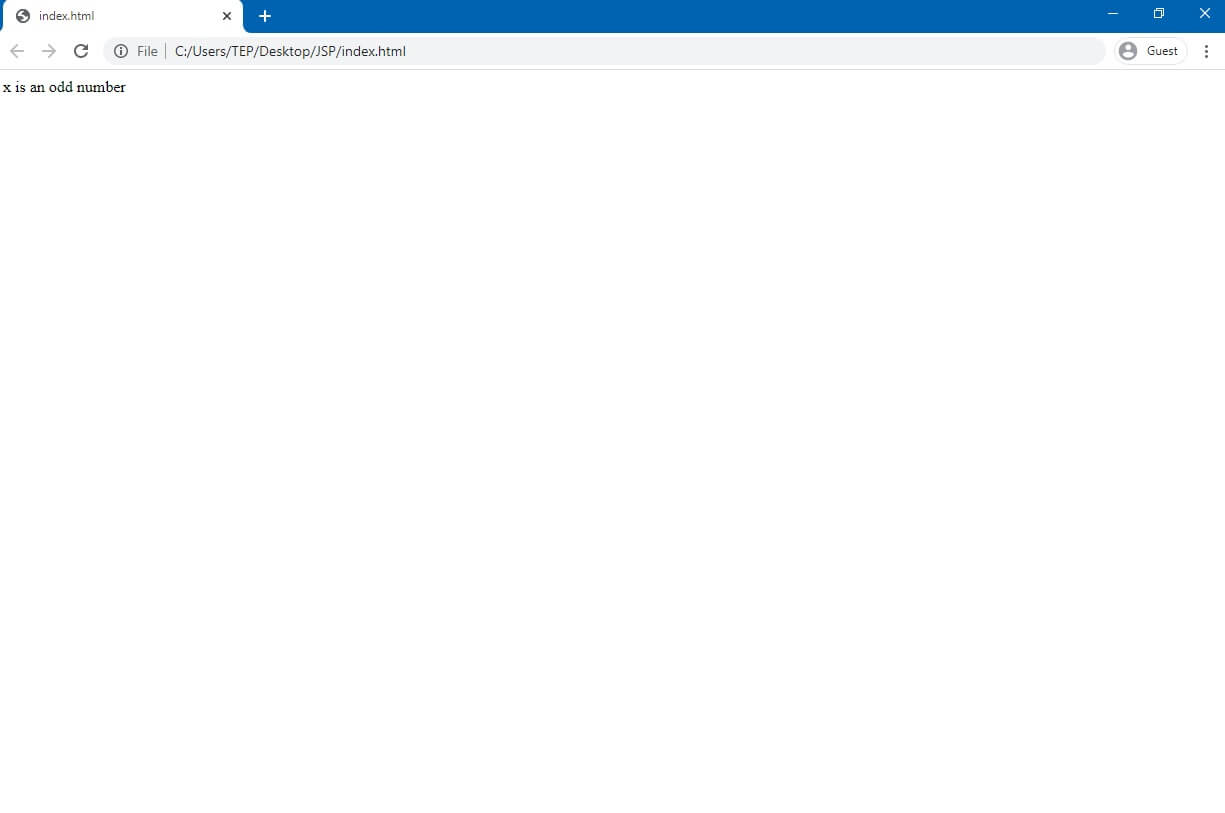


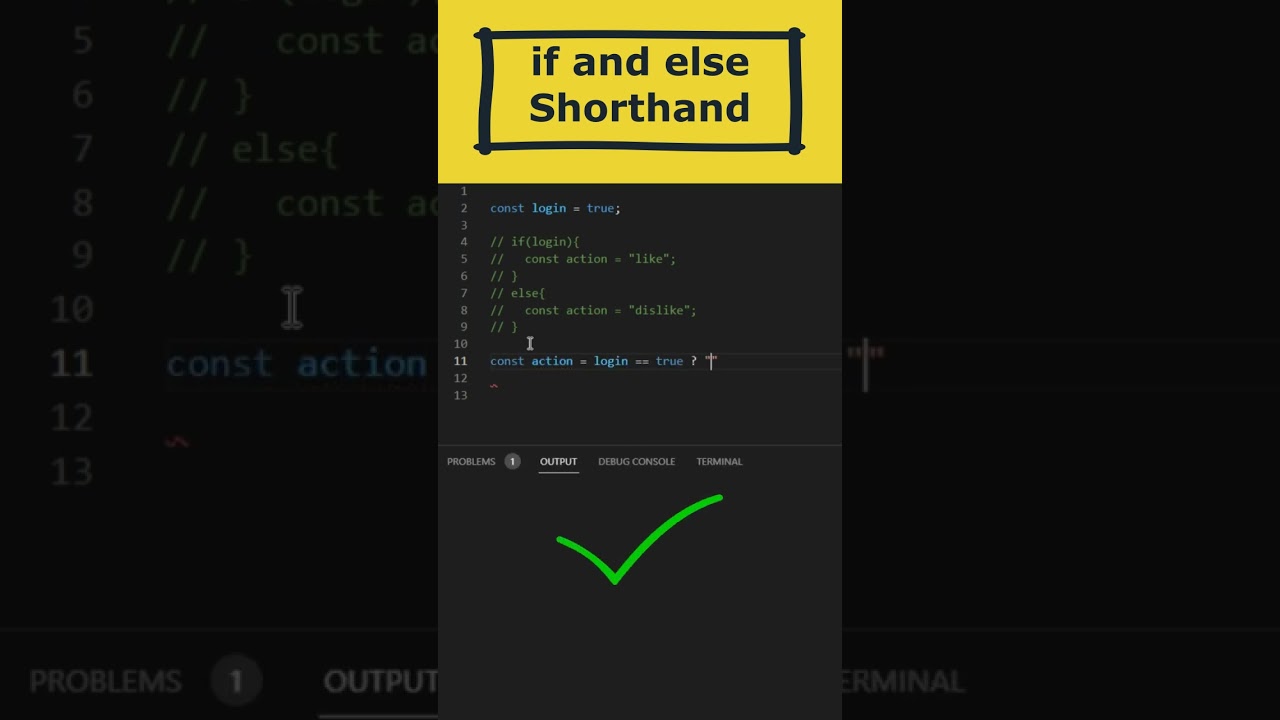
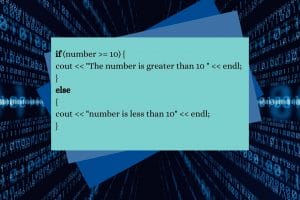


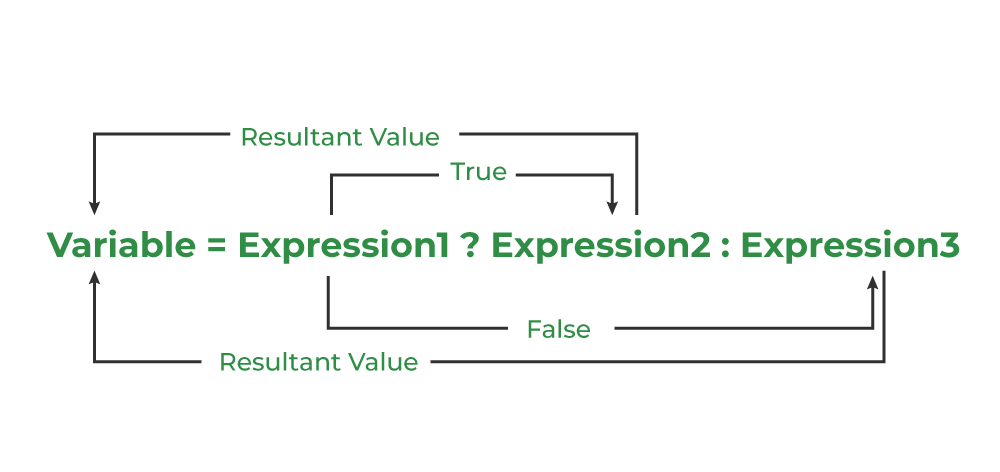
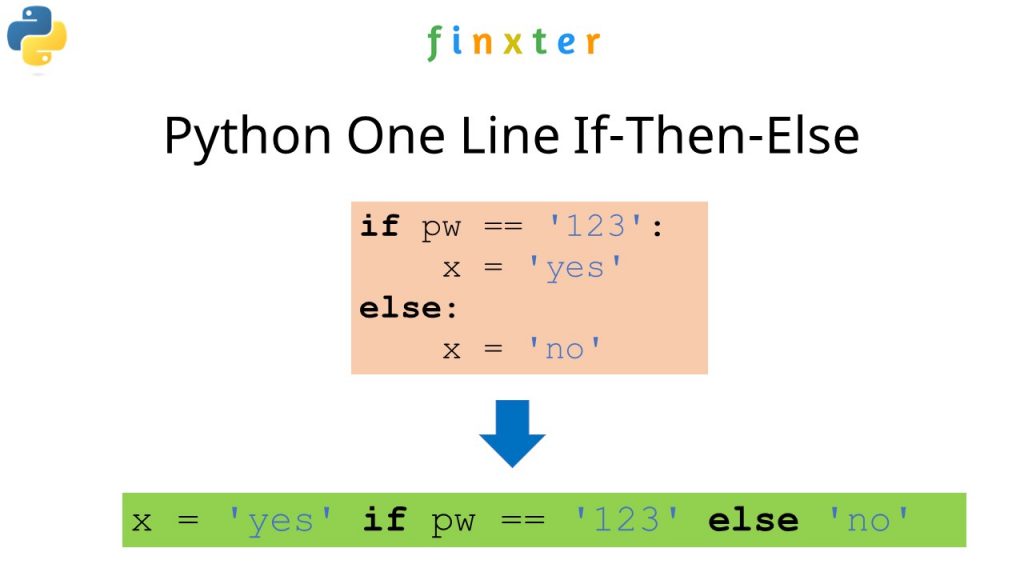


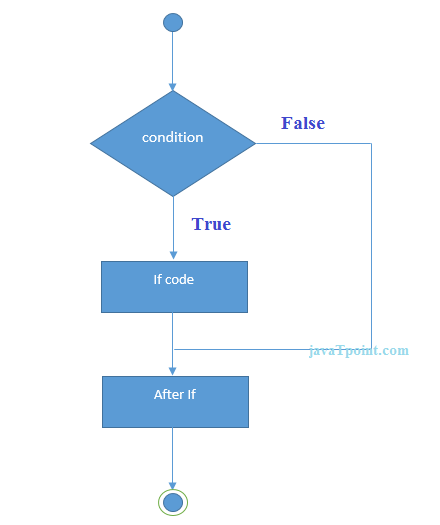




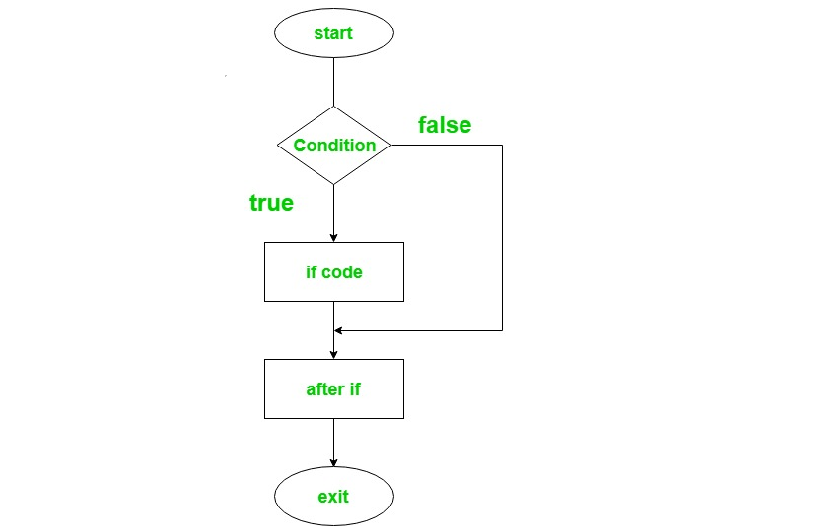
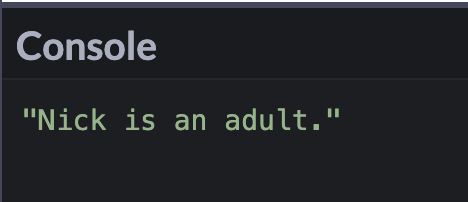
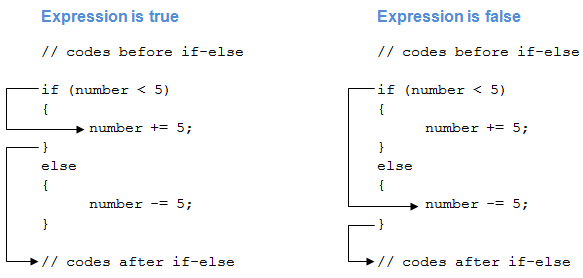
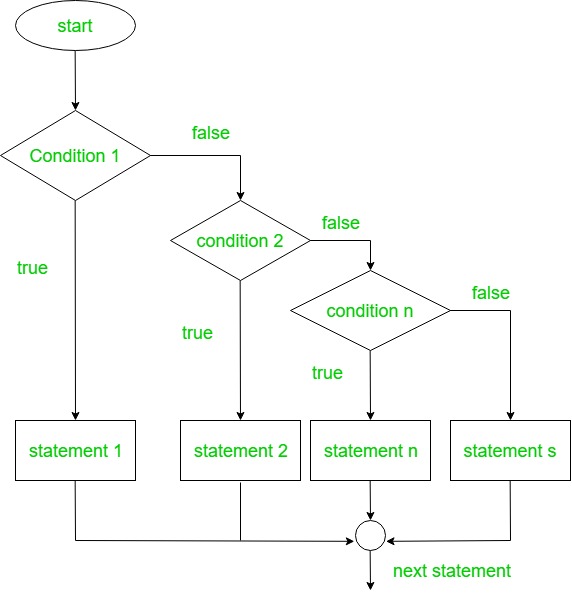


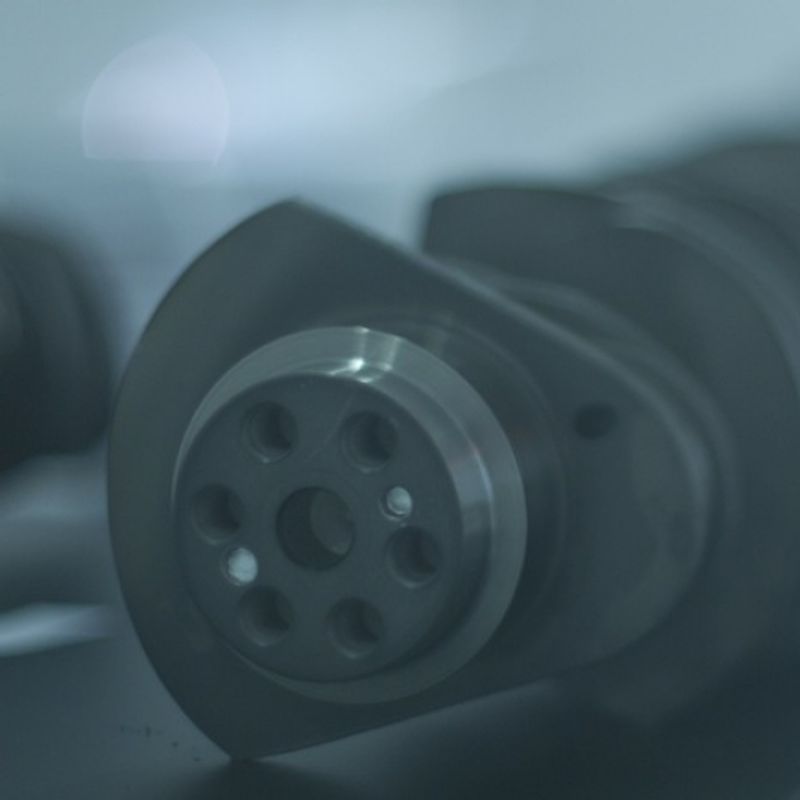
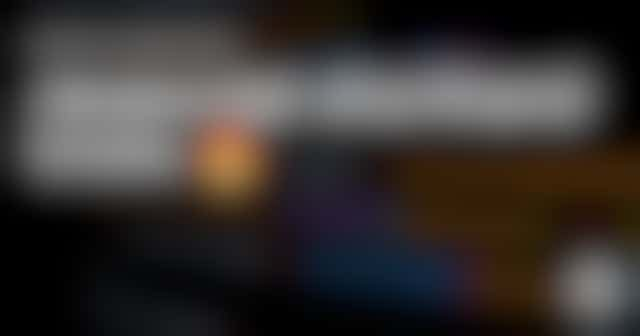
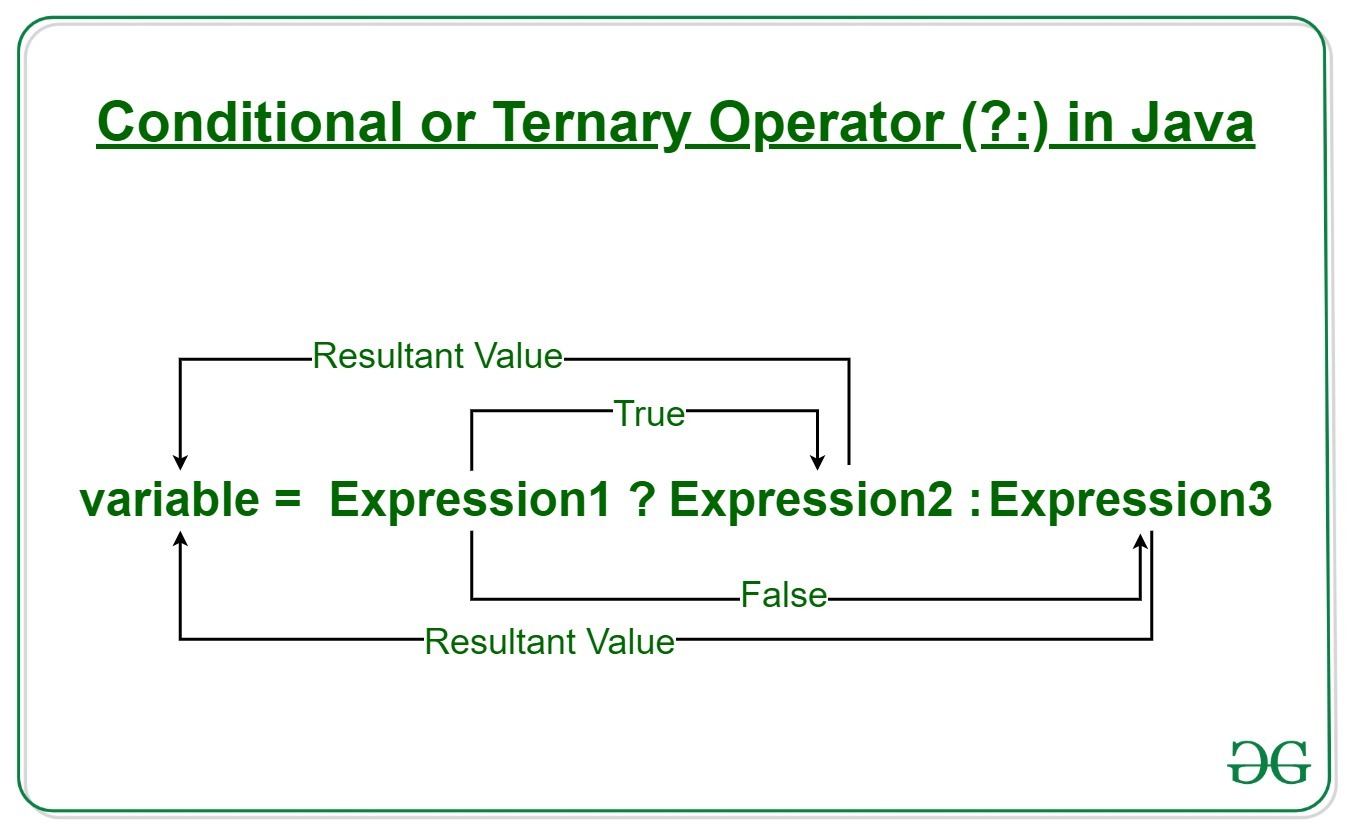


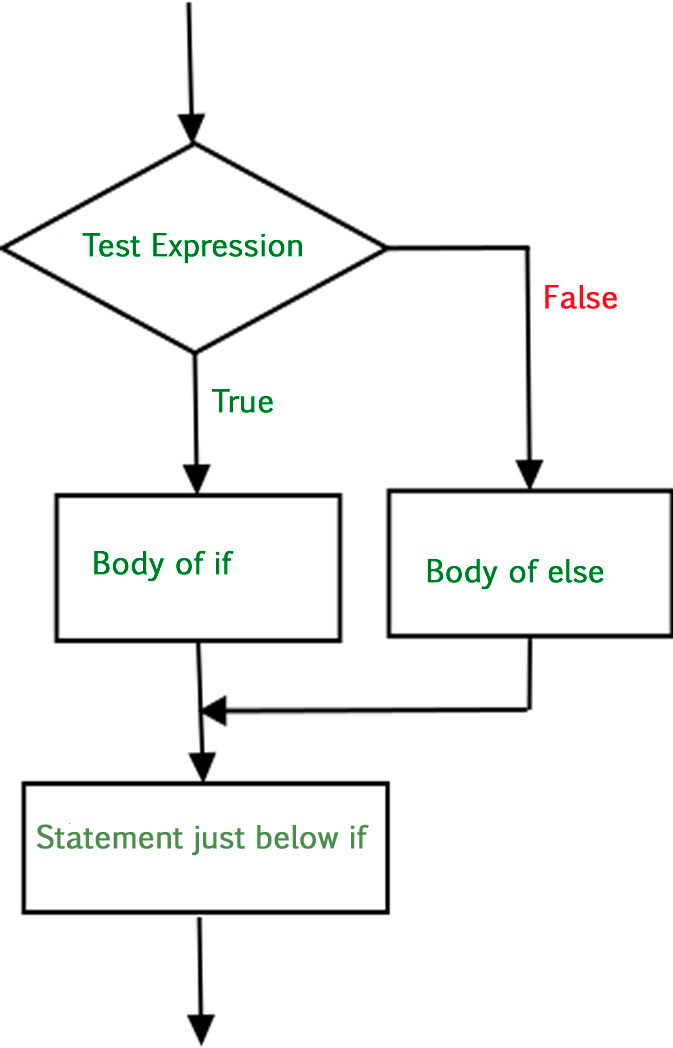
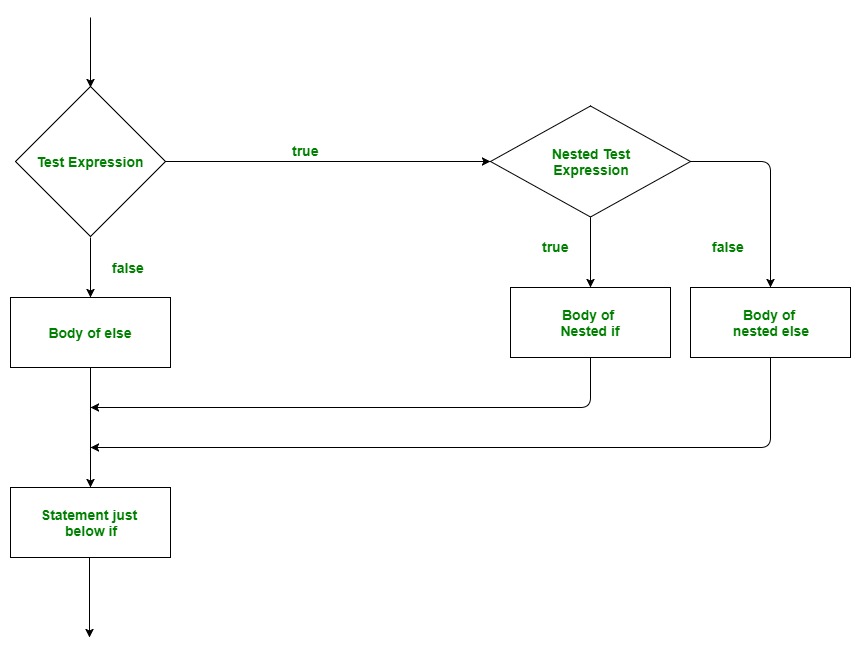
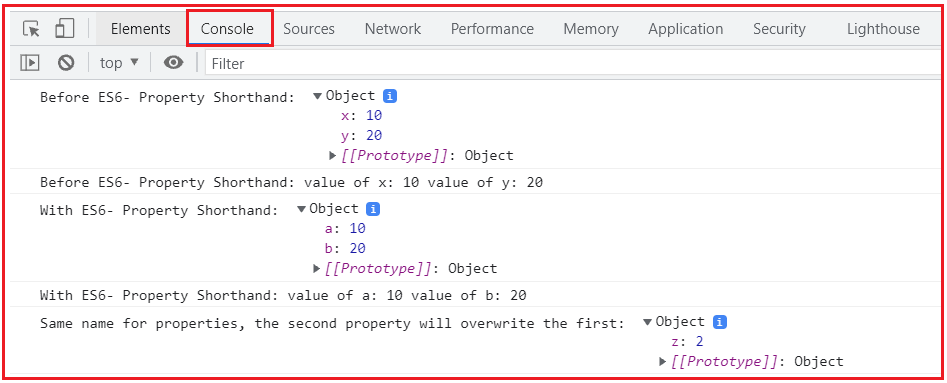
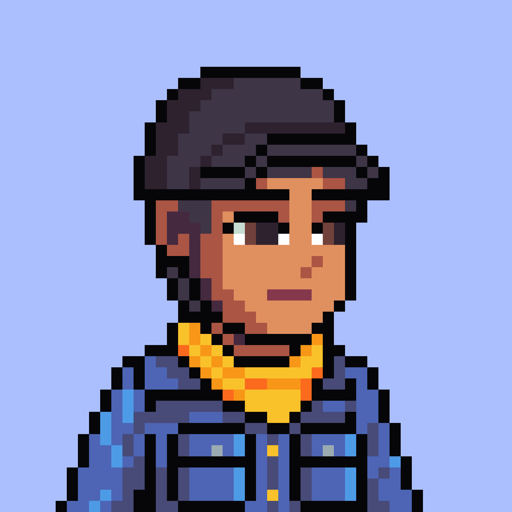

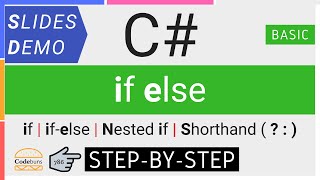
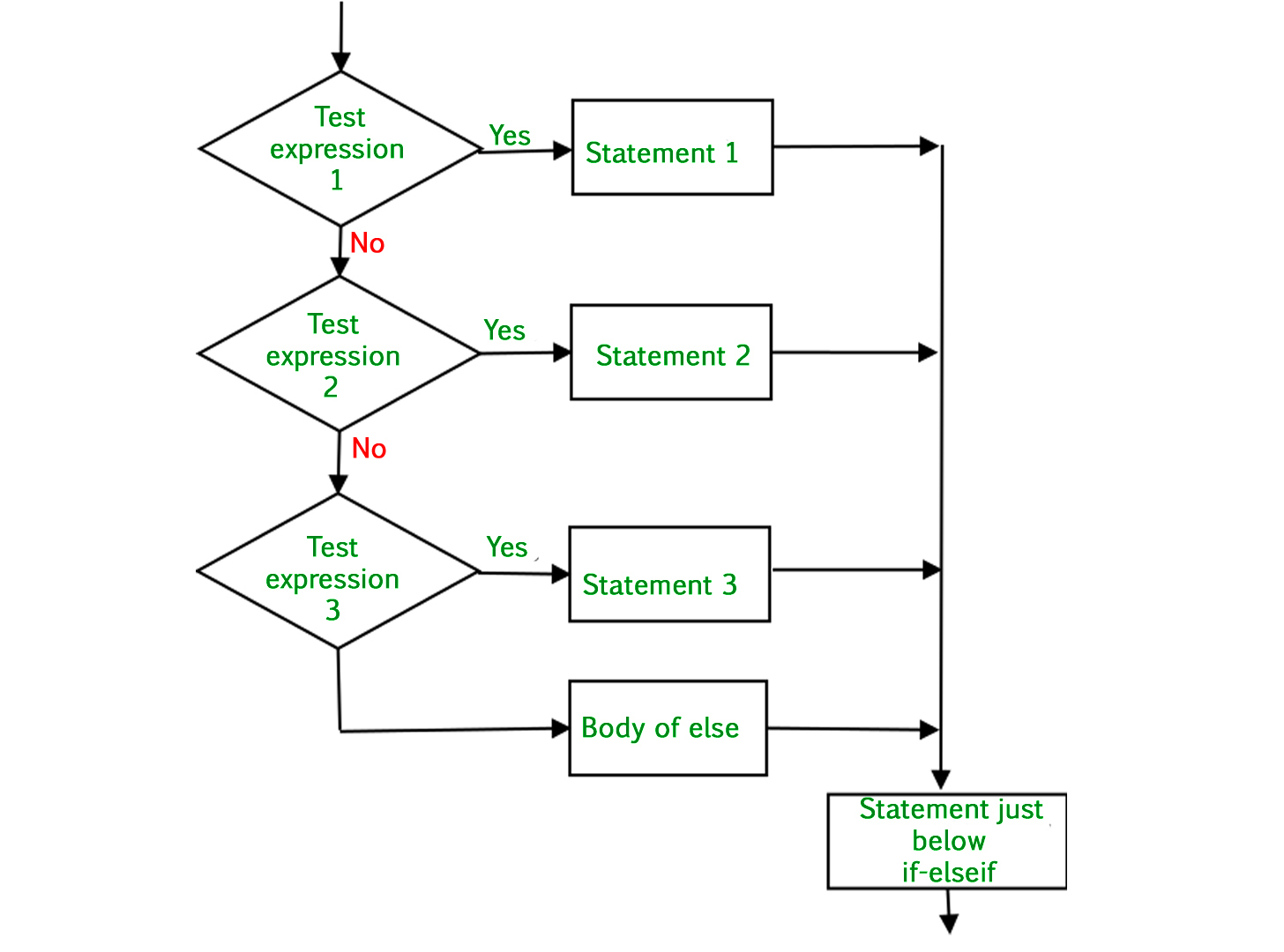
Article link: javascript if else shorthand.
Learn more about the topic javascript if else shorthand.
- Conditional (ternary) operator – JavaScript – MDN Web Docs
- Shorthand for if-else statement – javascript – Stack Overflow
- How to use shorthand for if/else statement in JavaScript
- How To Write Conditional Statements in JavaScript
- TypeScript if else – TutorialsTeacher
- Conditional or Ternary Operator (?:) in C – GeeksforGeeks
- Conditional (ternary) operator – JavaScript – MDN Web Docs
- Javascript shorthand for if/else statements (ternary operator …
- How To Write Conditional Statements in JavaScript
- JavaScript if else shorthand | Example code
- JavaScript if shorthand without the else – Daily Dev Tips
- Short Hand If…Else (Ternary Operator) – W3Schools
See more: https://nhanvietluanvan.com/luat-hoc/