Javascript Function Returns Undefined Instead Of Value
Proper Declaration and Definition of Functions
In JavaScript, functions are declared using the `function` keyword followed by the name of the function. The function name should be unique and descriptive. It is important to include parentheses after the function name, as they are used to pass arguments to the function. Additionally, curly braces `{}` should be used to enclose the function body.
Example:
“`javascript
function myFunction(arg1, arg2) {
// function body
}
“`
Misplaced or Missing Return Statement
One common mistake that can lead to a function returning undefined is forgetting to include a return statement. When a function is called, it executes the code within its body and should return a value using the `return` keyword. If a return statement is missing or misplaced, the function will not return the desired value.
Example of missing return statement:
“`javascript
function add(a, b) {
// forgot to include a return statement
console.log(a + b);
}
console.log(add(2, 4)); // Output: undefined
“`
The above example will log the result of the addition, but since there is no return statement, the function will return undefined.
To fix this, make sure to include the return statement at the appropriate location within the function body.
Handling of Asynchronous Operations
JavaScript functions that involve asynchronous operations, such as API calls or setTimeout, can also return undefined if not handled properly. Asynchronous operations do not block the execution of the code, and the function may return before the asynchronous operation is complete.
To handle asynchronous operations, JavaScript provides mechanisms like callbacks, promises, and async/await. The callback approach involves passing a function as an argument to the asynchronous function, which will be executed once the operation is complete. Promises and async/await provide a more structured and readable way to handle asynchronous operations.
Example using callback:
“`javascript
function fetchData(callback) {
setTimeout(() => {
const data = “Some data”;
callback(data);
}, 2000);
}
fetchData((data) => {
console.log(data); // Output: Some data
});
“`
In this example, the `fetchData` function takes a callback as an argument and invokes it after a simulated 2-second delay. The callback receives the data as a parameter.
Errors in Variable Declarations or Assignments
Errors in variable declarations or assignments within a function can also lead to undefined return values. It is important to declare and assign variables correctly, with proper syntax and scope.
Example of incorrect variable declaration:
“`javascript
function multiply(a, b) {
const result = a * b;
console.log(result);
// incorrect variable declaration
result = result * 2;
return result;
}
console.log(multiply(2, 3)); // Output: Uncaught TypeError: Assignment to constant variable.
“`
The `result` variable is declared using the `const` keyword, which makes it immutable. However, a subsequent assignment attempt results in an error, preventing the function from returning the intended value.
To fix this, ensure that variables are declared correctly and any assignments follow the appropriate syntax.
Incorrect Usage of Function Arguments
Passing incorrect arguments to a function can also lead to undefined return values. Functions rely on the correct types and number of arguments to execute properly.
Example of incorrect argument usage:
“`javascript
function greet(name) {
return “Welcome, ” + name;
}
console.log(greet()); // Output: Welcome, undefined
“`
In this case, the `greet` function expects a `name` argument, but it is not provided. As a result, the function returns “Welcome, undefined”.
To avoid this issue, make sure to provide all required arguments when calling a function and ensure their types match the function’s expectations.
Scoping Issues
Variable scope in JavaScript can cause undefined return values if not handled correctly. JavaScript has both global and local scope, and variables defined within a function have a local scope by default.
Example of scoping issue:
“`javascript
function calculateArea(radius) {
const pi = 3.14;
area = pi * radius * radius; // missing ‘var’, ‘let’, or ‘const’
return area;
}
console.log(calculateArea(5)); // Output: 78.5
console.log(area); // Output: 78.5
“`
In this example, the variable `area` is not declared with the `var`, `let`, or `const` keyword, making it a global variable by mistake. This can result in unexpected behavior and undefined return values.
To prevent scoping issues, always use var, let, or const to declare variables and define them within the appropriate scope.
Undefined Behavior in Conditional Statements
Conditional statements, such as if-else statements, can also lead to undefined behavior if not written correctly. Common mistakes include incorrect conditions or misplaced return statements within conditionals.
Example with misplaced return statement:
“`javascript
function isPositive(number) {
if (number > 0) {
return true;
}
// misplaced return statement
return false;
}
console.log(isPositive(-2)); // Output: undefined
“`
In this example, if the number is not greater than 0, the function should return false. However, the return statement is mistakenly placed outside the if-block, causing the function to return undefined.
To avoid undefined behavior in conditional statements, ensure that return statements are correctly placed within the desired condition.
Debugging Techniques
To identify the cause of a function returning undefined, effective debugging techniques can be used. The following strategies can help to pinpoint the issue:
1. Use console.log() statements: Place console.log() statements at various points within the function to inspect the values of variables and trace function execution.
2. Utilize debugging tools: Use browser developer tools, such as the Chrome DevTools, or debugging features of IDEs to set breakpoints, inspect variables, and step through the code.
3. Read error messages and stack traces: Carefully analyze error messages and stack traces to identify the source of the issue. Look for any error codes or line numbers that can guide you towards the problem area.
Common Mistakes and Tips
In addition to the issues discussed above, there are other common mistakes and tips to keep in mind when dealing with functions that return undefined:
– Always check for typographical errors: Double-check the spelling and case of function names, variable names, and keywords to avoid syntax errors.
– Ensure proper function invocation: Make sure to use the correct syntax when calling a function, including the use of parentheses and passing the correct arguments.
– Be aware of implicit returns: In certain cases, JavaScript functions may implicitly return undefined if no explicit return statement is provided. Make sure to provide a return statement when necessary.
– Test and validate inputs and outputs: Validate inputs to functions and test their outputs with different scenarios to ensure the desired behavior.
– Follow best coding practices: Keep your code clean, modular, and well-organized. Use meaningful variable and function names, and consider breaking down complex functions into smaller, reusable ones.
FAQs:
Q: Why does my JavaScript function not return a value?
A: There could be various reasons, such as missing return statements, scoping issues, incorrect usage of arguments, or errors in variable declarations. Review the suggestions above to troubleshoot and fix the problem.
Q: How can I handle asynchronous operations in JavaScript functions?
A: JavaScript provides mechanisms like callbacks, promises, and async/await to handle asynchronous operations. These mechanisms allow you to control the flow of execution and ensure proper return values.
Q: What is the best way to debug a function that returns undefined?
A: Using console.log() statements, debugging tools like browser developer tools, and carefully reading error messages and stack traces can help identify the cause of undefined returns. Review the debugging techniques section for more details.
Q: Are there any best practices I should follow when writing JavaScript functions?
A: Yes, some best practices include proper function declaration and definition, using return statements where required, validating inputs, following naming conventions, and organizing code in a clear and modular manner. Refer to the common mistakes and tips section for more guidance.
In conclusion, when a JavaScript function returns undefined instead of the expected value, it is essential to review the proper declaration and definition, check for misplaced or missing return statements, handle asynchronous operations correctly, validate variable declarations and assignments, use function arguments properly, address scoping issues, write conditional statements correctly, apply effective debugging techniques, and follow best practices. By applying these guidelines and troubleshooting methods, you can mitigate the issue of undefined return values and ensure the desired behavior of your JavaScript functions.
Understanding Undefined Value Returned From A Function (Basic Javascript) Freecodecamp Tutorial
Why Is My Function Returning Undefined Javascript?
JavaScript is a powerful and dynamic programming language that allows developers to create interactive websites and web applications. One common issue that developers often encounter is the “undefined” value being returned from a function. This can be frustrating and may lead to unexpected results in the code. In this article, we will explore the various reasons why a function may return undefined and discuss potential solutions to address this issue.
Understanding undefined in JavaScript
To better understand why a function may return undefined, it is important to first understand what undefined means in JavaScript. Undefined is a primitive value that is automatically assigned to variables that are declared but not assigned a value. It is also the default return value of a function if no explicit return statement is provided.
Reasons why a function may return undefined
1. Missing return statement:
One of the most common reasons for a function returning undefined is the absence of a return statement. If a function does not have a return statement, or if it is unreachable due to conditional statements, the function will return undefined by default.
For example:
“`
function add(a, b) {
if (a && b) {
return a + b;
}
}
console.log(add(2, 3)); // Output: undefined
“`
In the above example, if the condition in the if statement evaluates to false, the function does not have a return statement, resulting in undefined being returned.
2. Implicit return:
Another scenario where a function may return undefined is when an implicit return is encountered. An implicit return occurs when a function is written as an arrow function without the use of curly braces. In these cases, if a return statement is not provided, the function will default to returning undefined.
For example:
“`
const multiply = (a, b) => a * b;
console.log(multiply(2, 3)); // Output: undefined
“`
In the above example, the multiply function is written as an arrow function without curly braces. As no explicit return statement is provided, the function returns undefined.
3. Variable hoisting and order of execution:
JavaScript has a concept called variable hoisting, where variable declarations are moved to the top of their scope during the compilation phase. This can sometimes lead to a function returning undefined if it relies on variables that have not been declared yet.
For example:
“`
function doSomething() {
return x;
var x = 5;
}
console.log(doSomething()); // Output: undefined
“`
In this example, the variable x is hoisted to the top of the function scope, but it is not assigned a value until after the return statement. Therefore, when the function tries to return x, it is undefined.
4. Asynchronous operations:
Functions that involve asynchronous operations, such as fetching data from an API or reading from a file, often use callbacks, promises, or async/await syntax. These operations are non-blocking, meaning that the function does not wait for the result before moving on to the next line of code. As a result, the function may return undefined before the asynchronous operation has completed.
For example:
“`
function fetchData() {
let data;
fetch(‘https://api.example.com’)
.then(response => response.json())
.then(result => {
data = result;
});
return data;
}
console.log(fetchData()); // Output: undefined
“`
In this example, the fetchData function makes an asynchronous fetch request to an API. However, since the fetch request is non-blocking, the function continues executing and returns the data variable before it has been assigned a value.
Solutions to address functions returning undefined
1. Ensure a return statement is present:
To avoid a function returning undefined, you should ensure that a return statement is present in all code paths.
For example:
“`
function add(a, b) {
if (a && b) {
return a + b;
}
return 0; // Default case
}
console.log(add(2, 3)); // Output: 5
“`
In this updated example, a return statement is added at the end of the function to handle the case when the condition in the if statement evaluates to false. This ensures that the function always returns a value.
2. Review variable scoping:
If your function relies on variables, make sure they are declared and initialized before being used to avoid unexpected undefined values.
For example:
“`
function doSomething() {
var x = 5;
return x;
}
console.log(doSomething()); // Output: 5
“`
In this modified example, the variable x is declared and assigned a value before being used in the return statement, preventing it from returning undefined.
3. Use asynchronous programming techniques:
When dealing with functions that involve asynchronous operations, use appropriate techniques such as callbacks, promises, or async/await to handle the flow of execution and ensure that the function waits for the operation to complete before returning a value.
For example:
“`
function fetchData() {
return fetch(‘https://api.example.com’)
.then(response => response.json());
}
fetchData().then(data => {
console.log(data);
});
“`
In this example, the fetchData function returns a promise that resolves to the fetched data. By using the then method, the data can be accessed once the promise is fulfilled.
FAQs:
Q: Why am I getting undefined in my console log?
A: If you are seeing undefined in your console log, it means that the function you are calling is returning undefined. Check the function and ensure that a return statement is present or review the other possible reasons mentioned in this article.
Q: How can I debug functions returning undefined?
A: To debug functions returning undefined, you can use console.log statements at different points in the function to track the flow of execution and inspect variables. This can help identify where the undefined value is originating from.
Q: Can I assign a default value to prevent undefined returns?
A: Yes, you can assign a default value to a function’s return statement using the logical OR operator. If the function’s return value is undefined, it will be replaced by the default value.
Q: Why does JavaScript have an undefined value?
A: JavaScript has the undefined value as a way to represent the absence of an assigned value. It helps indicate when a variable has been declared but not assigned a value, or when a function does not explicitly return a value.
Conclusion
The “undefined” value being returned from a function in JavaScript can occur due to various reasons, such as the absence of a return statement, variable hoisting, asynchronous operations, or other programming errors. It is essential to understand the underlying causes and employ appropriate solutions to ensure correct function behavior. By implementing the suggested solutions and understanding the FAQs provided, developers can effectively troubleshoot and resolve the issue of functions returning undefined in their JavaScript code.
How To Stop A Function From Returning An Undefined In Javascript?
JavaScript is a powerful and versatile programming language used extensively in web development. While it offers various functionalities, you may encounter situations where your function returns an undefined value. This can lead to unexpected behavior and errors in your code. In this article, we will delve into the reasons why functions return undefined, explore different techniques to overcome this issue, and provide some valuable tips to prevent it from happening in the future.
Understanding Why Functions Return Undefined
To effectively stop a function from returning undefined, it is crucial to comprehend the underlying reasons for this behavior. There are several common causes:
1. No Return Statement: When a function lacks a return statement or an explicit value to return, it automatically returns an undefined value.
2. Return Without a Value: Similar to the previous scenario, if a function has a return statement without any value, it will return undefined.
3. Calling a Function Without Parentheses: In JavaScript, if you call a function without including parentheses, it is treated as a reference to the function itself rather than the function call. Thus, it returns the function object, leading to undefined.
4. Missing Arguments: Functions can have parameters that are not assigned any value when called. In such cases, those parameters become undefined.
5. Asynchronous Operations: Functions that perform asynchronous operations, such as AJAX requests or timers, often return undefined until the operation is completed. This behavior is due to the async nature of these functions.
Techniques to Prevent Functions from Returning Undefined
Now that we have identified some common causes, let’s explore various techniques to avoid functions returning undefined in JavaScript:
1. Ensure a Return Statement: Always include a return statement in your function, even if it is not expected to return a value. This avoids any unexpected results.
2. Return an Appropriate Value: If your function is intended to return a value, ensure you explicitly define the value that needs to be returned. This can be done by specifying the return statement followed by the desired value.
3. Use Parentheses while Calling Functions: To avoid referencing the function object, ensure you call functions with parentheses, even if they have no arguments. This will invoke the function and provide the expected return value.
4. Check and Handle Undefined Parameters: When defining functions that depend on parameters, add checks within the function to handle any undefined values. You can do this using if statements, default values, or the logical OR operator (||).
5. Utilize Promises or Callbacks: If you are dealing with asynchronous operations, consider using Promises or callbacks to handle the asynchronous nature of the function rather than expecting an immediate return value.
Frequently Asked Questions (FAQs):
Q1. What is the significance of returning a value from a function in JavaScript?
A1. Returning a value from a function allows you to pass data to the caller, enabling them to use the result in further computations or actions. It provides a way to communicate the outcome of the function to the rest of the program.
Q2. Can a function return multiple values in JavaScript?
A2. No, JavaScript functions can only return a single value. However, you can overcome this limitation by returning an object or an array containing multiple values.
Q3. Why is it important to handle undefined parameters in a function?
A3. Handling undefined parameters is crucial for maintaining the reliability and stability of your code. By checking and handling undefined values, you can prevent unexpected errors and ensure your function performs as expected in different scenarios.
Q4. How can I debug a function that returns undefined?
A4. Debugging a function that returns undefined can be achieved by utilizing various techniques, such as console.log statements, step-by-step debugging tools, or using browser developer tools to inspect variables and execution flow.
Q5. Are there any recommended coding practices to prevent undefined returns?
A5. Yes, keeping your code clean and well-structured can greatly reduce the occurrence of undefined returns. Be consistent in your coding style, assign default values to function parameters when appropriate, and ensure you explicitly return values whenever needed.
In conclusion, understanding why functions return undefined is crucial in preventing unexpected behavior and errors in your JavaScript code. By following the techniques mentioned in this article, such as including a return statement, handling undefined parameters, and properly dealing with asynchronous operations, you can ensure your functions return the expected values. Additionally, it is essential to adopt recommended coding practices to prevent undefined returns and maintain the overall reliability of your code.
Keywords searched by users: javascript function returns undefined instead of value javascript function not returning value, javascript function is undefined, function returning undefined react, node js function return undefined, Async function return undefined, Check object undefined JavaScript, this returns undefined, javascript object undefined
Categories: Top 73 Javascript Function Returns Undefined Instead Of Value
See more here: nhanvietluanvan.com
Javascript Function Not Returning Value
Why does a JavaScript function sometimes not return a value?
JavaScript functions are designed to perform a certain task and optionally return a value. However, there are several reasons why a function might not return the expected result.
1. Missing return statement: The most common reason for a function not returning a value is the absence of a return statement. If a function is executed without explicitly stating what value to return, it will default to returning “undefined”. To ensure the desired value is returned, it is crucial to include a return statement within the function’s body.
2. Incorrect placement of return statement: Another common mistake is placing the return statement in an unreachable part of the code. For example, placing a return statement after an if statement that evaluates to false means the return statement will never be executed, causing the function to not return a value. Careful attention must be paid to the flow of execution to ensure that the return statement is reached and executed.
3. Asynchronous nature of JavaScript: JavaScript is a single-threaded language, but it supports asynchronous programming through mechanisms like callbacks, promises, and async/await. When dealing with asynchronous operations, it is important to understand that functions may not return immediately. Instead, they may rely on callbacks or other asynchronous constructs to handle the returned value. Failure to account for this can result in unexpected behavior and functions appearing to not return a value.
4. Logic errors and conditional branches: A function might not return a value if it encounters a logic error or conditional branch that prevents it from reaching the return statement. These errors could be caused by incorrect conditional statements or improper variable manipulation. Thoroughly testing and debugging the function can help identify and rectify such issues.
How to ensure a JavaScript function returns a value
To ensure a JavaScript function returns a value as expected, follow these best practices:
1. Explicitly specify a return statement: Declare the return statement within the function and ensure it returns the expected value. This will prevent the function from defaulting to “undefined” or any other unintended value.
2. Test conditional and logical branches: Verify that the function correctly handles different paths depending on conditional statements and logical branches. This involves testing all possible scenarios and ensuring that the function reaches the expected return statement.
3. Understand asynchronous operations: If a function relies on asynchronous operations, such as API calls or database queries, ensure that you handle the returned values correctly when they become available. Familiarize yourself with promises, async/await, or other relevant constructs to properly handle asynchronous behavior.
4. Review code for logic errors: Analyze the function’s code for any logic errors that might impede the function from reaching the return statement. Double-check conditional statements, variable assignments, and calculations. Debugging tools, such as browser developer tools or dedicated debugging environments, can be immensely useful in identifying and resolving such errors.
FAQs
Q1. Can a JavaScript function return multiple values?
A1. No, JavaScript functions can only return a single value. However, you can use an object or an array to return multiple values by encapsulating them within the container and returning that container.
Q2. What is the difference between returning a value and using console.log()?
A2. Returning a value allows you to use that value for further computation or manipulate it within the program. On the other hand, console.log() simply prints the value to the browser’s console for debugging or informational purposes.
Q3. Can a JavaScript function modify variables outside its scope?
A3. Yes, JavaScript functions can modify variables outside their scope if those variables are within the function’s closure scope or are declared as global variables.
In conclusion, understanding why JavaScript functions sometimes fail to return a value is essential for efficient and error-free coding. By employing proper coding techniques and debugging strategies, developers can overcome this issue and ensure that functions consistently return the desired values. Stay vigilant, test thoroughly, and utilize the rich debugging tools available to effectively troubleshoot and resolve any encountered problems.
Javascript Function Is Undefined
## Understanding the “Function is Undefined” Error
The “function is undefined” error occurs when you try to call a function that does not exist or has not been declared before calling it. This error message is fairly straightforward and usually indicates a syntax or logic mistake in your code.
A function is a block of code that performs a specific task and can be reused throughout the program. Before calling a function, it needs to be defined or declared in your JavaScript code. If you try to call a function that hasn’t been defined yet, you will encounter this error.
## Common Causes of the Error
1. **Typo or Case Sensitivity**: JavaScript is a case-sensitive language. So, if you misspell the function name, use incorrect capitalization, or make a mistake in the function’s name or assignment, it may result in the “function is undefined” error. Double-check your function names and ensure they are spelled and capitalized correctly.
2. **Script Loading Order**: If your JavaScript file is not loaded before the code that calls the function, the function will appear as undefined. Ensure that your JavaScript files are properly included and loaded in the correct order.
3. **Scope Issues**: JavaScript has its own rules for variable scopes. If a function is defined within a different scope than where it is being called, it may lead to the “function is undefined” error. Make sure the function is accessible and within reach of the code that calls it.
4. **Timing Problems**: JavaScript code executes sequentially. If you attempt to call a function before it has been defined or loaded, it will result in the “function is undefined” error. Ensure your function is declared or loaded before calling it.
## Troubleshooting the Error
When encountering the “function is undefined” error, follow these steps to troubleshoot and resolve the issue:
1. **Check Function Name**: Double-check the spelling and capitalization of the function name to ensure it matches the function declaration and function call.
2. **Script Loading Order**: Confirm that the JavaScript file containing the function is loaded before code attempting to call the function.
3. **Scope Issues**: Review the scope of the function declaration and the calling code to ensure they are in the same scope or accessible to each other.
4. **Timing Problems**: Ensure the function is defined or loaded before calling it. Consider using the `window.onload` event or defer attribute to ensure the entire page is loaded before executing JavaScript code.
5. **Generate Error Message**: Add a console log or an alert box just before the function call to verify if it is executed. This can help identify any missing code or other issues that may result in the error.
6. **Code Review**: Carefully analyze your code for any logical mistakes, missing parentheses, or incorrect syntax. Review the entire project and look for any missing or duplicate function declarations.
If you have followed these troubleshooting steps and the error persists, reviewing your code with a peer or seeking help on a developer community or forum can be beneficial.
## FAQs
Q: Can multiple functions with the same name cause the “function is undefined” error?
A: Yes, if multiple functions with the same name exist, JavaScript will use the last defined function. Ensure that there are no duplicate function declarations.
Q: I have a function defined, but I am still getting the error. Why?
A: Make sure the function is in the correct scope and is accessible from where it is being called. If the function is defined within a conditional statement or loop, it may not be available outside of that block.
Q: Do I need to define a function before calling it?
A: Yes, functions must be defined or declared before calling them. Placing the function declaration above the code that calls it will prevent the “function is undefined” error.
Q: Does this error only occur in JavaScript?
A: Yes, this error is specific to JavaScript since other programming languages may have different error messages for similar situations.
Q: Can a syntax error cause the “function is undefined” error?
A: Yes, a syntax error within the function definition or any other code preceding the function could potentially cause the “function is undefined” error. Ensure your code is syntactically correct.
In conclusion, the “function is undefined” error is a common issue in JavaScript that occurs when you call a function that has not been defined or declared before usage. Understanding the possible causes and following the troubleshooting steps can help you resolve this error more efficiently.
Images related to the topic javascript function returns undefined instead of value
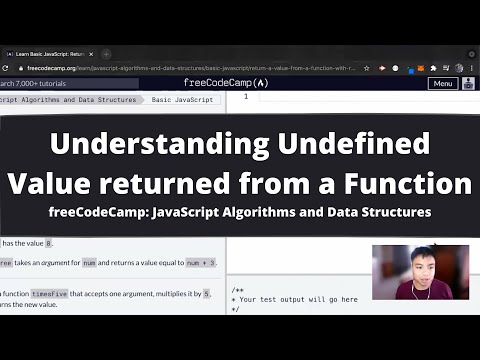
Found 38 images related to javascript function returns undefined instead of value theme

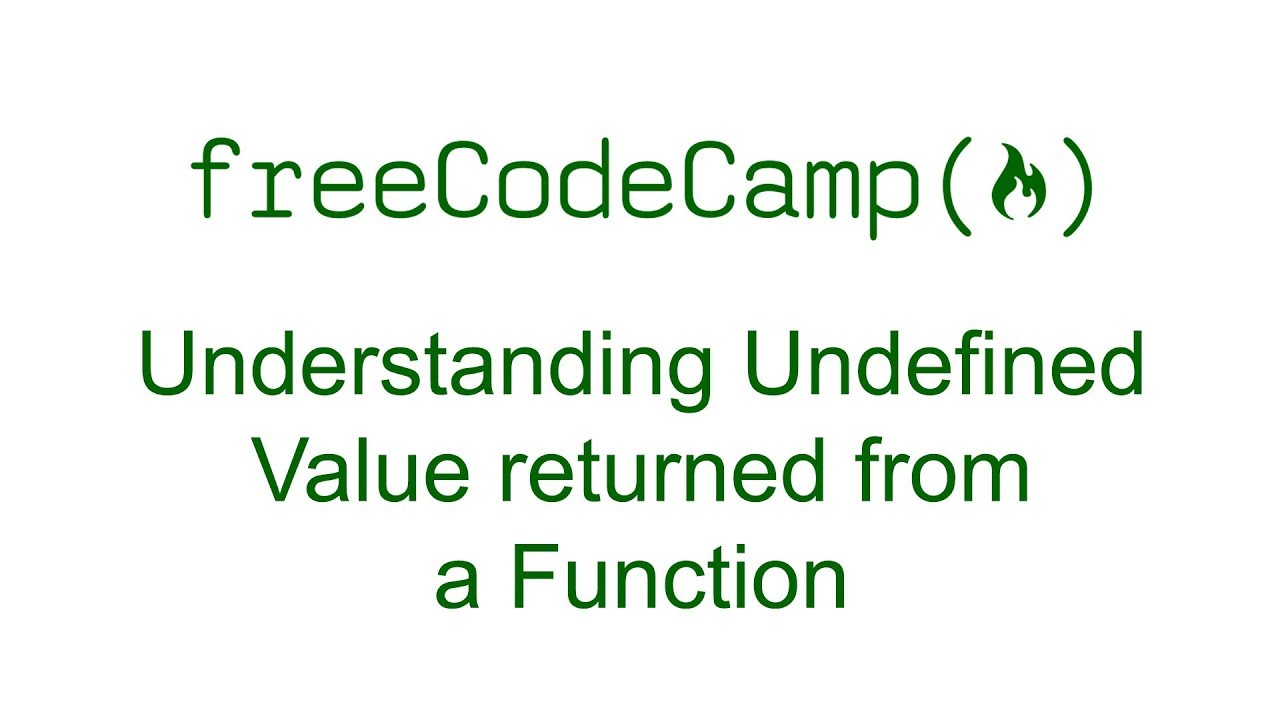
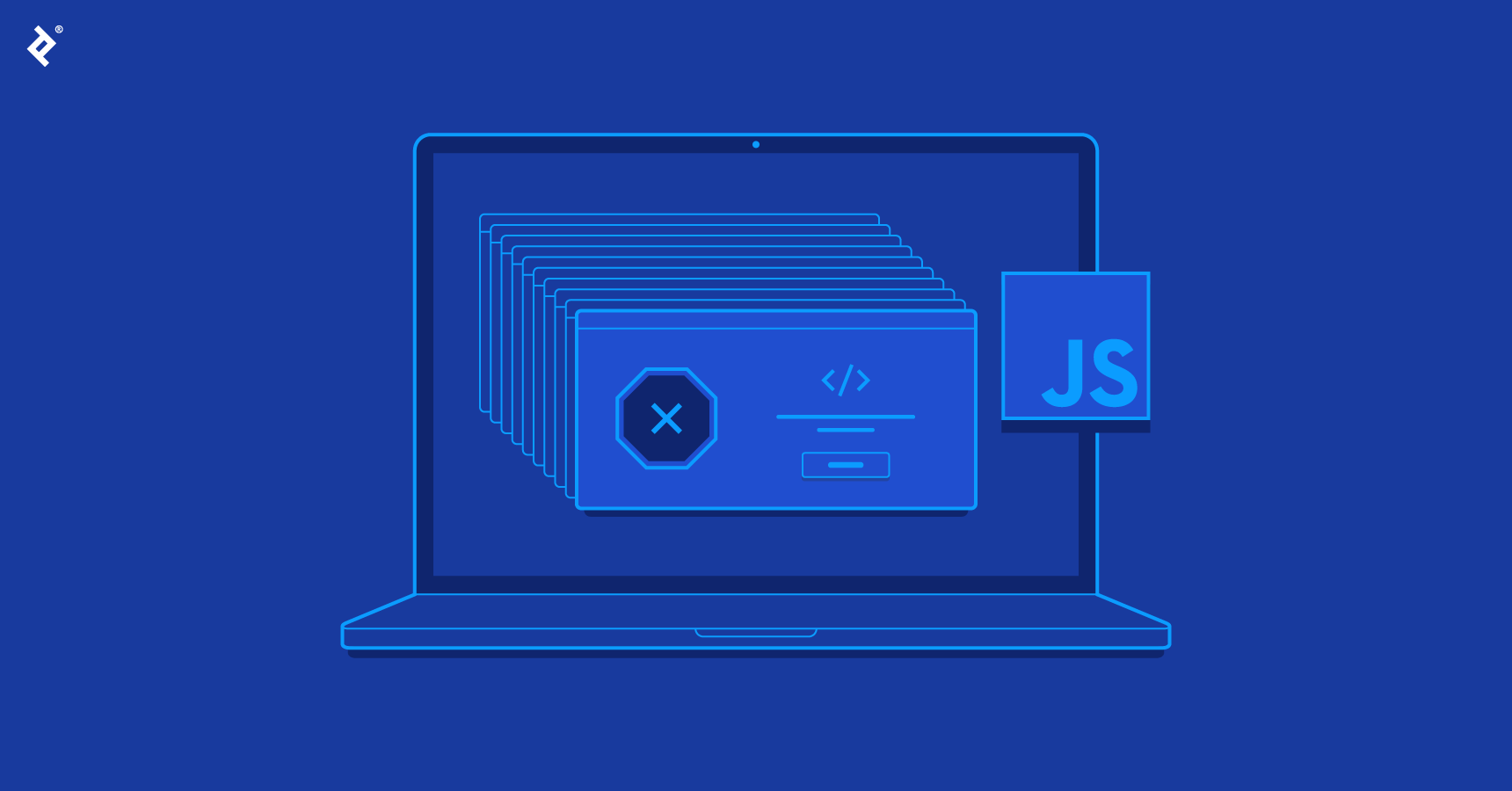
Article link: javascript function returns undefined instead of value.
Learn more about the topic javascript function returns undefined instead of value.
- Fix JavaScript Function Returns Undefined Instead of Value
- Why is Javascript Function Return Undefined – Dev Genius
- javascript function returns undefined instead of object literal
- undefined – JavaScript – MDN Web Docs – Mozilla
- How do I avoid my return function from returning “undefined” when no …
- JavaScript Check if Undefined – How to Test for Undefined in JS
- Why is my function returning “undefined” instead of boolean
- undefined – JavaScript – MDN Web Docs – Mozilla
- Function returns undefined in js – Fork My Brain
- why does this return undefined ????? | Codecademy
- Returning undefined – JavaScript – The freeCodeCamp Forum
- 7 Tips to Handle undefined in JavaScript – Dmitri Pavlutin
- map() method returns undefined in JavaScript [Solved]
- How to return undefined from a function in JavaScript – Quora
See more: https://nhanvietluanvan.com/luat-hoc/