Javascript Else If Shorthand
Definition of the JavaScript Else If Statement:
The Else If statement is a conditional statement used in JavaScript to execute a specific block of code if a certain condition evaluates to true. It serves as an extension to the traditional If-Else statement, allowing multiple conditions to be evaluated consecutively. The Else If statement is often used when there are more than two possible outcomes and the code needs to be executed based on different conditions.
Purpose and Use of Else If Shorthand in JavaScript:
The main purpose of using the Else If shorthand in JavaScript is to simplify the code structure and enhance readability. Instead of writing multiple If-Else statements, developers can use the Else If shorthand to handle multiple conditions in a more concise manner. This not only reduces the number of lines of code but also makes it easier to understand the logic behind the conditions.
Syntax of the Else If Shorthand Statement:
The syntax of the Else If shorthand statement in JavaScript is as follows:
“`
if (condition1) {
// code to be executed if condition1 is true
} else if (condition2) {
// code to be executed if condition2 is true
} else if (condition3) {
// code to be executed if condition3 is true
} else {
// code to be executed if none of the conditions are true
}
“`
Example Usage of Else If Shorthand in JavaScript:
Let’s consider an example where we want to determine the grade based on a student’s score. We can use the Else If shorthand statement to handle different score ranges:
“`javascript
let score = 85;
let grade;
if (score >= 90) {
grade = ‘A’;
} else if (score >= 80) {
grade = ‘B’;
} else if (score >= 70) {
grade = ‘C’;
} else if (score >= 60) {
grade = ‘D’;
} else {
grade = ‘F’;
}
console.log(“Grade:”, grade);
“`
In this example, the code checks the score and assigns the corresponding grade. If the score is greater than or equal to 90, it assigns ‘A’. If the score is between 80 and 89, it assigns ‘B’, and so on. Finally, it logs the grade.
Benefits of Using Else If Shorthand in JavaScript:
– Readability: The Else If shorthand improves code readability by clearly indicating the different conditions being evaluated.
– Conciseness: Using the Else If shorthand reduces the number of lines of code required compared to writing multiple If-Else statements.
– Maintainability: The Else If shorthand makes it easier to maintain and modify the code in the future because it follows a logical structure.
Comparison of Else If Shorthand with Traditional If-Else Statements:
In traditional If-Else statements, each condition must be explicitly written using an if keyword, followed by an else keyword. This can result in a long and cluttered code block. On the other hand, the Else If shorthand allows multiple conditions to be evaluated more neatly and concisely.
Common Mistakes to Avoid When Using Else If Shorthand:
While using the Else If shorthand, there are a few common mistakes that developers should be cautious about:
1. Incorrect Ordering: It is crucial to order the conditions correctly. The Else If statement will be evaluated from top to bottom, and the first condition that evaluates to true will execute the corresponding block of code. Improper ordering may lead to unexpected results.
2. Overlapping Conditions: Make sure the conditions do not overlap or conflict with each other. If two conditions are true simultaneously, only the code block associated with the first matching condition will execute.
Tips and Best Practices for Utilizing Else If Shorthand Effectively:
1. Maintain Consistency: Stick to a consistent coding style throughout the project. This will make it easier for other developers to understand and maintain the code.
2. Use Parentheses: Enclosing the conditions in parentheses can help improve code readability and avoid potential errors.
3. Commenting: Consider adding comments to your code to explain the logic behind the conditions and their purpose. It will make it easier for others and your future self to understand the code.
FAQs:
Q: Can I use multiple conditions in a single Else If statement?
A: Yes, the Else If statement supports multiple conditions separated by logical operators, such as `&&` (AND) or `||` (OR).
Q: Can I skip the Else part in the Else If shorthand statement?
A: Yes, the Else part is optional. If none of the conditions evaluate to true, the code will simply move to the next statement or block.
Q: Is the Else If shorthand specific to JavaScript?
A: No, the Else If shorthand is not specific to JavaScript. It is supported by many programming languages, including Python, C++, and PHP.
Conclusion:
The Else If shorthand is a powerful feature in JavaScript that simplifies the handling of multiple conditions. By using this shorthand, developers can improve code readability, reduce the number of lines of code, and maintain a logical structure. It is important to understand the syntax, avoid common mistakes, and follow best practices to utilize the Else If shorthand effectively in JavaScript development.
Javascript: Shorthand For If-Else Statement
What Is The Shorthand For If-Else In Typescript?
TypeScript is a powerful superset of JavaScript that extends the capabilities of the language. It introduces many new features and enhancements to streamline development and improve code readability. One notable feature in TypeScript is the shorthand notation for if-else statements, which allows developers to write more concise and expressive code. In this article, we will explore the shorthand for if-else in TypeScript and discuss its usage.
The shorthand for if-else in TypeScript is called the “conditional operator” or the “ternary operator.” It provides a concise way to write if-else statements in a single line. The syntax of the conditional operator is as follows:
“`
condition ? expressionIfTrue : expressionIfFalse;
“`
Here, the `condition` is an expression that evaluates to either true or false. If the condition is true, the `expressionIfTrue` is executed; otherwise, the `expressionIfFalse` is executed. The conditional operator returns the value of the executed expression.
Let’s consider a simple example to understand the shorthand for if-else in TypeScript better. Suppose we have a variable `age` that holds the age of a person, and we want to determine if the person is an adult or a minor based on their age. We can use the conditional operator to achieve this in a concise manner:
“`typescript
const age: number = 20;
const personType: string = age >= 18 ? “adult” : “minor”;
console.log(personType); // Output: adult
“`
In the example above, the condition `age >= 18` evaluates to true, as the person’s age is 20. Thus, the expression `personType` is assigned the value “adult.” Finally, the result is printed to the console.
The conditional operator is not limited to simple assignments. It can also be used in more complex expressions and statements. For instance, it can be used to conditionally assign a value to a variable or to return a value from a function based on a condition.
Frequently Asked Questions (FAQs):
Q: Can the conditional operator replace all if-else statements?
A: No, the conditional operator has its limitations. It is best suited for simple logic and concise expressions. For more complex logic or multiple conditions, if-else statements are still the preferred choice.
Q: Are there any performance differences between using the conditional operator and if-else statements?
A: The performance differences are negligible in most cases. However, it is worth noting that the conditional operator can result in more readable and concise code, which can be advantageous in terms of code maintainability and developer productivity.
Q: Can I nest conditional operators within each other?
A: Yes, you can nest conditional operators within each other. However, nesting them too deeply can make the code harder to read and understand. It is generally recommended to use if-else statements for complex nesting scenarios.
Q: How does the conditional operator handle truthy and falsy values?
A: Like regular if-else statements, the conditional operator relies on the truthiness or falsiness of the condition. Any value that can be coerced to `true` is considered truthy, while any value that can be coerced to `false` is considered falsy.
Q: Are there any caveats or special considerations when using the conditional operator?
A: While the conditional operator can enhance code readability, it is important to use it judiciously. Overusing it can lead to overly complex code and decrease code clarity. Hence, it is essential to strike the right balance between readability and brevity.
In conclusion, the shorthand for if-else in TypeScript, known as the conditional operator, offers a concise and expressive way to write conditional statements. With its simple yet powerful syntax, developers can streamline their code and enhance code readability. However, it is important to use the conditional operator judiciously and consider the complexity of your logic to maintain a balance between code brevity and readability.
How To Check 3 Conditions In If Statement In Javascript?
The “if” statement is one of the fundamental and commonly used control structures in JavaScript, allowing you to conditionally execute a block of code based on a given condition. However, there may be situations where you need to check multiple conditions simultaneously. In such cases, you can utilize logical operators and proper syntax to efficiently evaluate three or more conditions within an if statement. In this article, we will explore various approaches to accomplishing this task and provide detailed explanations along the way.
Checking multiple conditions enables your code to make decisions based on complex criteria, leading to more flexible and versatile programming solutions. By understanding the different techniques available, you’ll be able to write clearer and more concise code, improving the overall readability and maintainability of your JavaScript projects.
Approach 1: Combining conditions using Logical AND (&&) operator
—————————————————————–
The logical AND (&&) operator can be used to combine two or more conditions within an if statement. It checks whether all the conditions are true, and if so, proceeds to execute the associated block of code. By using this operator, you can check 3 or more conditions in a single if statement. Here’s an example:
“`javascript
if (condition1 && condition2 && condition3) {
// Code to be executed if all conditions are true
}
“`
Approach 2: Nesting if statements
———————————
Another way to check multiple conditions is by nesting if statements. This approach allows you to break down complex conditions into smaller, more manageable parts. Here’s an example:
“`javascript
if (condition1) {
if (condition2) {
if (condition3) {
// Code to be executed if all conditions are true
}
}
}
“`
By nesting the if statements, you can achieve the desired behavior of evaluating three conditions before executing the associated code block.
Approach 3: Utilizing the ternary operator (?)
———————————————–
The ternary operator provides a concise way to evaluate conditions and return a value based on the result. While it may not be as commonly used for checking three conditions, it can still be applicable depending on your specific requirements. Here’s an example:
“`javascript
condition1 && condition2 && condition3 ?
// Code to be executed if all conditions are true
: // Code to be executed if any condition is false
“`
The ternary operator first evaluates all conditions. If all conditions are true, it executes the code before the colon (:). If any of the conditions are false, it executes the code after the colon (:).
These three approaches provide different techniques for checking multiple conditions within an if statement in JavaScript. Choose the one that best fits your code’s logic or readability requirements.
FAQs
—–
Q1. Can I check more than three conditions within an if statement using these approaches?
A1. Absolutely! These approaches can be extended to check any number of conditions. Simply add more conditions using logical operators or nest additional if statements as required.
Q2. Are there any limits on the number of conditions I can check within an if statement?
A2. In theory, there is no limit to the number of conditions you can check. However, keep in mind that excessively complex conditions may decrease the readability of your code and make it harder to maintain. Consider refactoring your code if you find yourself dealing with an overwhelming number of conditions.
Q3. Can I mix different approaches in a single if statement?
A3. While it is technically possible, it is generally recommended to choose one approach and stick with it for consistency and readability purposes. Mixing approaches may lead to confusion and make your code harder to understand for other developers.
Q4. Is there a preferred approach among these options?
A4. The choice of approach depends on your specific use case and coding style. Logical operators offer a concise and straightforward solution, while nesting if statements allows for more complex condition structures. The ternary operator provides a shorter syntax but may not be as readable for complex conditions. Consider the requirements of your project and choose the approach that best suits your needs.
In conclusion, checking three or more conditions within an if statement in JavaScript can be accomplished using various approaches. By understanding and implementing these techniques, you can write cleaner, more efficient, and readable code. Choose the approach that best fits your specific requirements, while keeping in mind the best practices and guidelines for maintaining well-structured code.
Keywords searched by users: javascript else if shorthand If else if shorthand javascript, JS shorthand if without else, If…else one line JavaScript, Shorthand if else Python, Shorten if statement javascript, If…else JS, Javascript else if multiple conditions, If JavaScript
Categories: Top 46 Javascript Else If Shorthand
See more here: nhanvietluanvan.com
If Else If Shorthand Javascript
In JavaScript, conditional statements are essential for controlling the flow of a program. These statements allow developers to execute different blocks of code based on specific conditions. One widely used form of conditional statement is the If Else If construct, which allows for more precise decision-making compared to simple If Else statements. In this article, we will delve into the If Else If shorthand in JavaScript, exploring its syntax, functionality, and some practical examples.
Syntax of If Else If Shorthand:
The If Else If shorthand syntax in JavaScript follows a concise and compact structure. It can be represented as follows:
“`
if (condition1) {
// code block to be executed when condition1 is true
} else if (condition2) {
// code block to be executed when condition2 is true
} else if (condition3) {
// code block to be executed when condition3 is true
} else {
// code block to be executed when none of the conditions are true
}
“`
The first condition (condition1) is checked, and if it evaluates to true, the corresponding code block is executed. If condition1 is false, the script checks the next condition (condition2). If condition2 is true, the associated code block is executed. This process continues until a condition evaluates to true or all conditions have been evaluated and found to be false. If none of the conditions are true, the code inside the else block will be executed.
Functionality and Advantages:
The If Else If shorthand provides a concise and efficient way to handle complex decision-making scenarios. It allows developers to evaluate multiple conditions sequentially, ensuring that only the code block associated with the first true condition is executed. This approach is particularly useful when dealing with a range of possibilities, where different actions must be taken based on varying conditions.
By utilizing If Else If shorthand, developers can avoid the need for nested If Else statements, which can quickly become convoluted and difficult to read. The shorthand notation reduces the amount of code required, making it more concise and easier to maintain.
Practical Examples:
Let’s explore some practical examples to illustrate the application of the If Else If shorthand in JavaScript:
Example 1: Evaluating a Grade
Suppose you are developing a grade calculator program. Let’s assume the grades are between 0 and 100, and we want to output an appropriate message based on the given grade:
“`javascript
const grade = 75;
if (grade >= 90) {
console.log(“Excellent!”);
} else if (grade >= 80) {
console.log(“Good!”);
} else if (grade >= 70) {
console.log(“Average!”);
} else if (grade >= 60) {
console.log(“Pass!”);
} else {
console.log(“Fail!”);
}
“`
In this example, the program evaluates the grade and outputs an appropriate message based on the condition that matches. If the grade is 75, the output will be “Average!” because the condition `grade >= 70` is satisfied.
Example 2: Divisibility Test
Let’s consider a program that determines whether a given number is divisible by 2, 3, or 5:
“`javascript
const number = 12;
if (number % 2 === 0) {
console.log(“Divisible by 2”);
} else if (number % 3 === 0) {
console.log(“Divisible by 3”);
} else if (number % 5 === 0) {
console.log(“Divisible by 5”);
} else {
console.log(“Not divisible by 2, 3, or 5”);
}
“`
In this example, the program checks the number’s divisibility and outputs the corresponding message. Since 12 is divisible by 2, the output will be “Divisible by 2.”
FAQs:
Q: Can we omit the ‘else’ part in the If Else If shorthand?
A: Yes, it is possible to omit the ‘else’ part entirely. If none of the conditions evaluate to true, no code block will be executed.
Q: Can we have multiple conditions inside each ‘if’ block?
A: Yes, you can have multiple conditions inside each ‘if’ block by using logical operators such as ‘&&’ (AND) or ‘||’ (OR) to combine conditions.
Q: Can we use the If Else If shorthand for more than three conditions?
A: Absolutely! If Else If shorthand can be extended to handle any number of conditions by adding more ‘else if’ statements.
Q: What happens if two or more conditions evaluate to true?
A: In the If Else If shorthand, only the code block associated with the first true condition encountered will be executed. The subsequent conditions will not be evaluated.
In conclusion, the If Else If shorthand in JavaScript provides a concise and effective way to handle complex decision-making scenarios. It offers greater precision in evaluating multiple conditions and ensures efficient execution of the corresponding code block. By utilizing this shorthand notation, developers can enhance code readability and maintainability, making it a valuable tool in JavaScript programming.
Js Shorthand If Without Else
In JavaScript (JS) programming, conditionals play a crucial role in controlling the execution flow of our code. The if/else statement is a versatile tool for decision-making in JS, but it can often result in verbose and repetitive code. Thankfully, JS offers a shorthand if/else syntax that allows developers to write more concise and readable conditionals. In this article, we will explore the shorthand if/else in depth, discussing its syntax, use cases, benefits, and common pitfalls. So let’s dive in!
Syntax of Shorthand if/else:
The shorthand if/else statement in JS is commonly known as the ternary operator. It follows a simple syntax that condenses the if/else structure into a single line. Here’s the general structure:
(condition) ? true_expression : false_expression;
The condition is evaluated first. If it is true, the expression before the colon (:) is executed. Otherwise, the expression after the colon is executed. The result of the chosen expression is then assigned to the ternary operator.
Example Usage:
To better understand how to use the shorthand if/else, let’s consider a practical example. Suppose we have a variable called count, and we want to display a message based on its value:
const message = (count > 0) ? “Count is positive” : “Count is zero or negative”;
console.log(message);
In this example, if the value of count is greater than zero, the true_expression “Count is positive” is assigned to the message variable. If the condition is false, the false_expression “Count is zero or negative” is assigned. The message is then displayed on the console.
Benefits of Shorthand if/else:
1. Readability: The shorthand if/else enhances code readability by condensing complex conditionals into a single line. It eliminates the need for opening and closing braces, reducing visual clutter.
2. Conciseness: By using the ternary operator, developers can write conditionals more concisely. This reduces code length, making it easier to understand and maintain.
3. Expressive syntax: The ternary operator provides a more expressive syntax. It clearly shows the intent of the conditional flow, making the code more self-explanatory.
4. Less error-prone: With the ternary operator, the chances of making syntax errors or logical mistakes are minimized due to the simplified structure.
Common Pitfalls:
While the shorthand if/else offers numerous benefits, it’s essential to be aware of potential pitfalls:
1. Complexity: Care should be taken when using nested ternary operators to avoid complexity and confusion. It’s encouraged to keep the expressions simple and use them for small conditional operations.
2. Code obfuscation: Overusing the shorthand if/else can lead to code obfuscation. It’s crucial to strike a balance between conciseness and readability, ensuring that the code remains understandable for other developers.
3. Debugging difficulty: When debugging code that includes the ternary operator, it can be challenging to trace through the nested expressions. It’s advisable to use the shorthand if/else judiciously to maintain code traceability.
FAQs:
Q: Can I use the shorthand if/else without a false_expression?
A: No, the ternary operator requires both a true_expression and a false_expression. If you have a case where you don’t need a false_expression, you can simply use an empty string, null, or any other value that represents the absence of a result.
Q: Is there a limit to the complexity of expressions within the shorthand if/else?
A: While there is no strict limit, it is generally recommended to keep the expressions simple. Avoid excessive nesting or multiple operations within the expressions, as it can impair code comprehension.
Q: Can I use the shorthand if/else with multiple conditions?
A: Yes, you can chain multiple ternary operators by placing one after another. However, keep in mind that maintaining code readability is crucial. If the conditions become too complex, it’s often better to use the traditional if/else structure for clarity.
Q: What happens if I don’t assign the result of the ternary operator to a variable?
A: The result of the ternary operator can be used directly in an expression or function call. If not assigned, it is evaluated but discarded immediately. However, assigning it to a variable enhances code readability and reusability.
In conclusion, the shorthand if/else, or ternary operator, is a powerful syntactic construct in JavaScript. It provides a concise and expressive way to write conditionals, improving code readability and reducing redundancy. While it has its limitations and potential pitfalls, by understanding its proper usage, developers can leverage this feature to write more efficient and elegant JavaScript code. So next time you find yourself writing a simple if/else conditional, consider using the shorthand if/else to streamline your code and enhance its readability.
Images related to the topic javascript else if shorthand
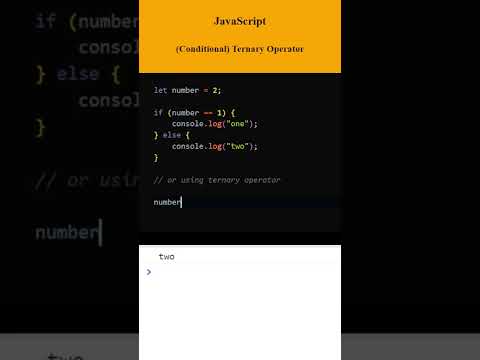
Found 22 images related to javascript else if shorthand theme

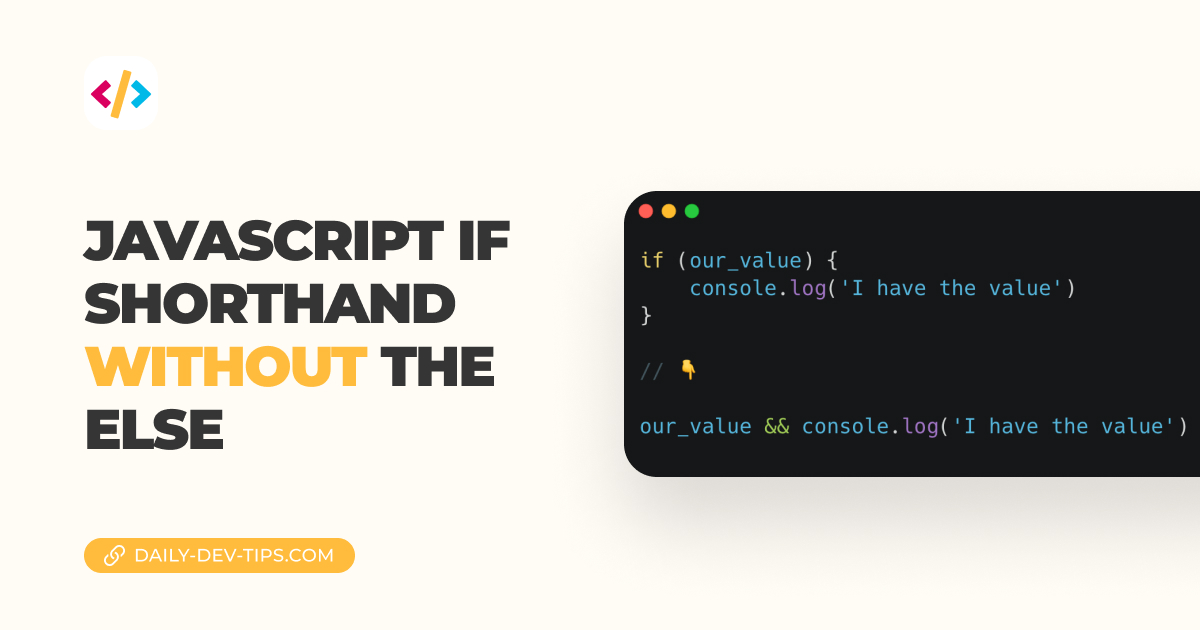

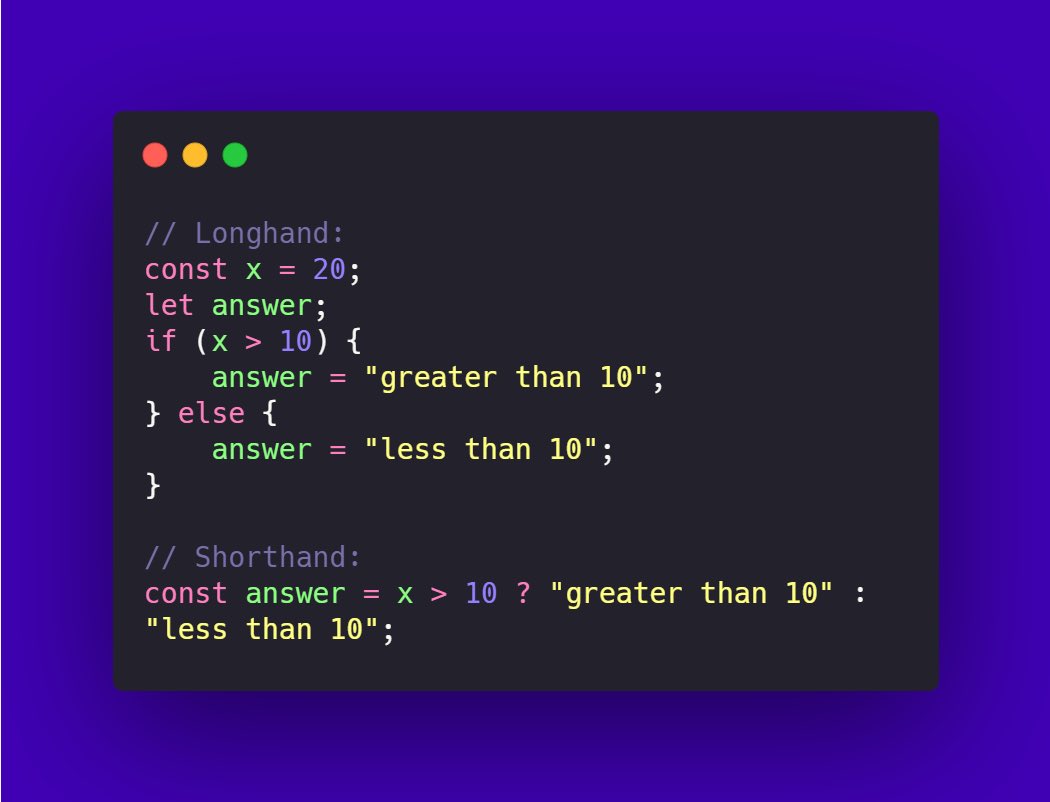
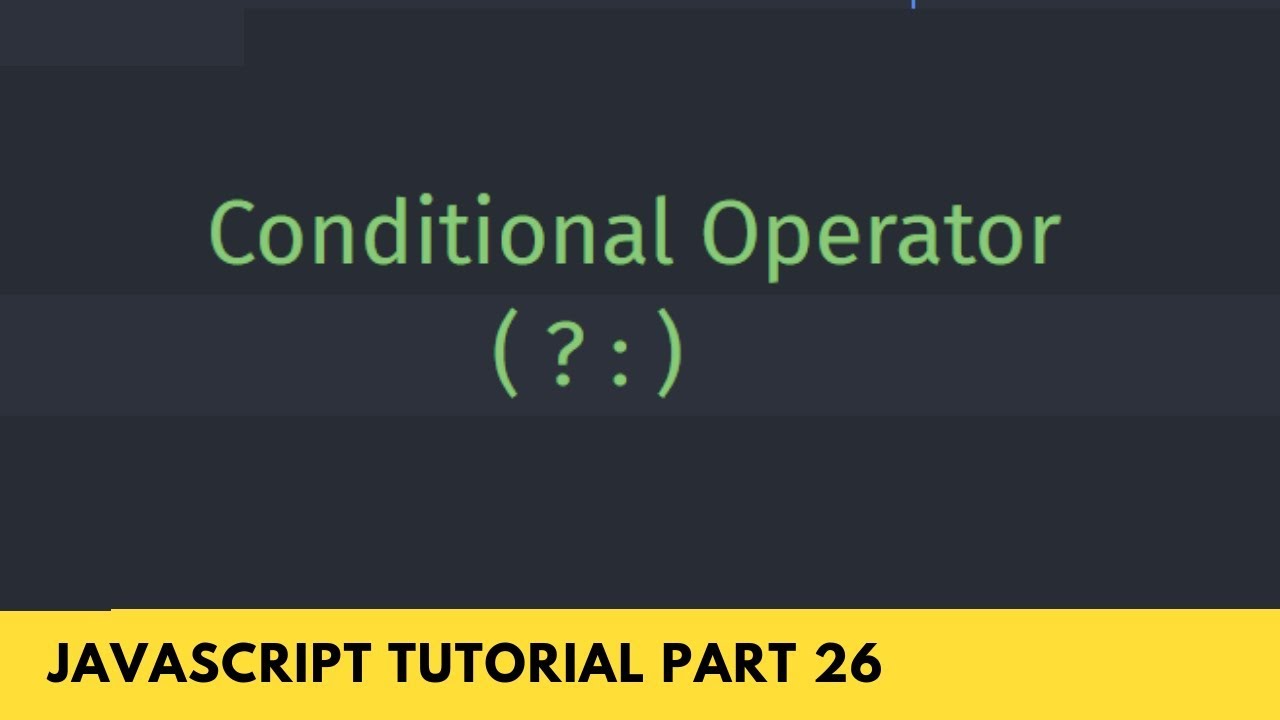
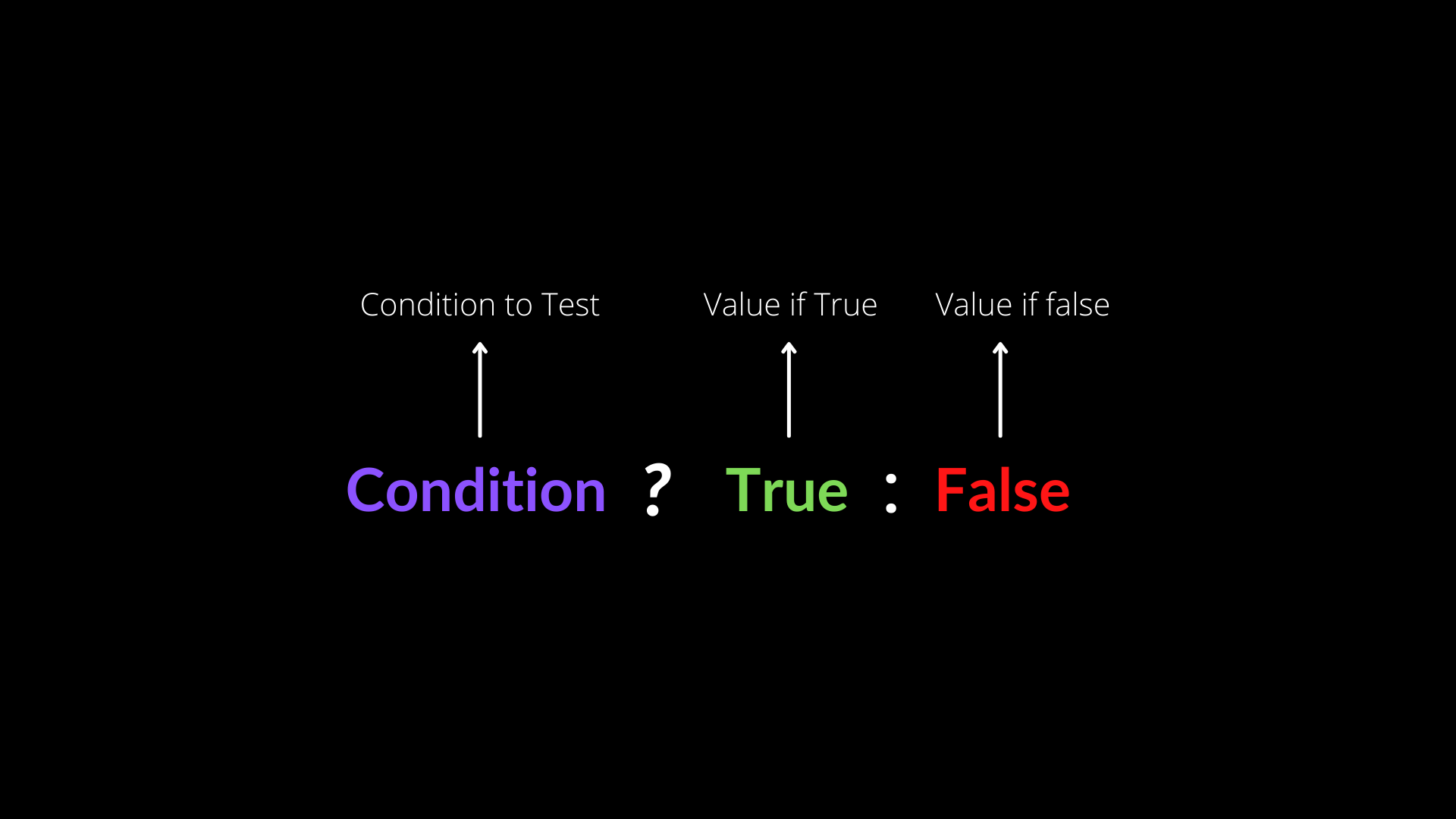
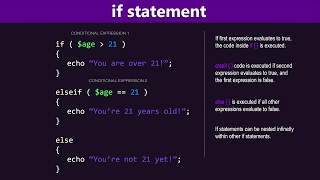
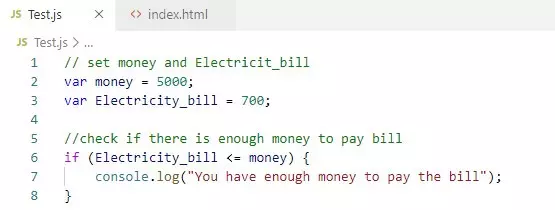
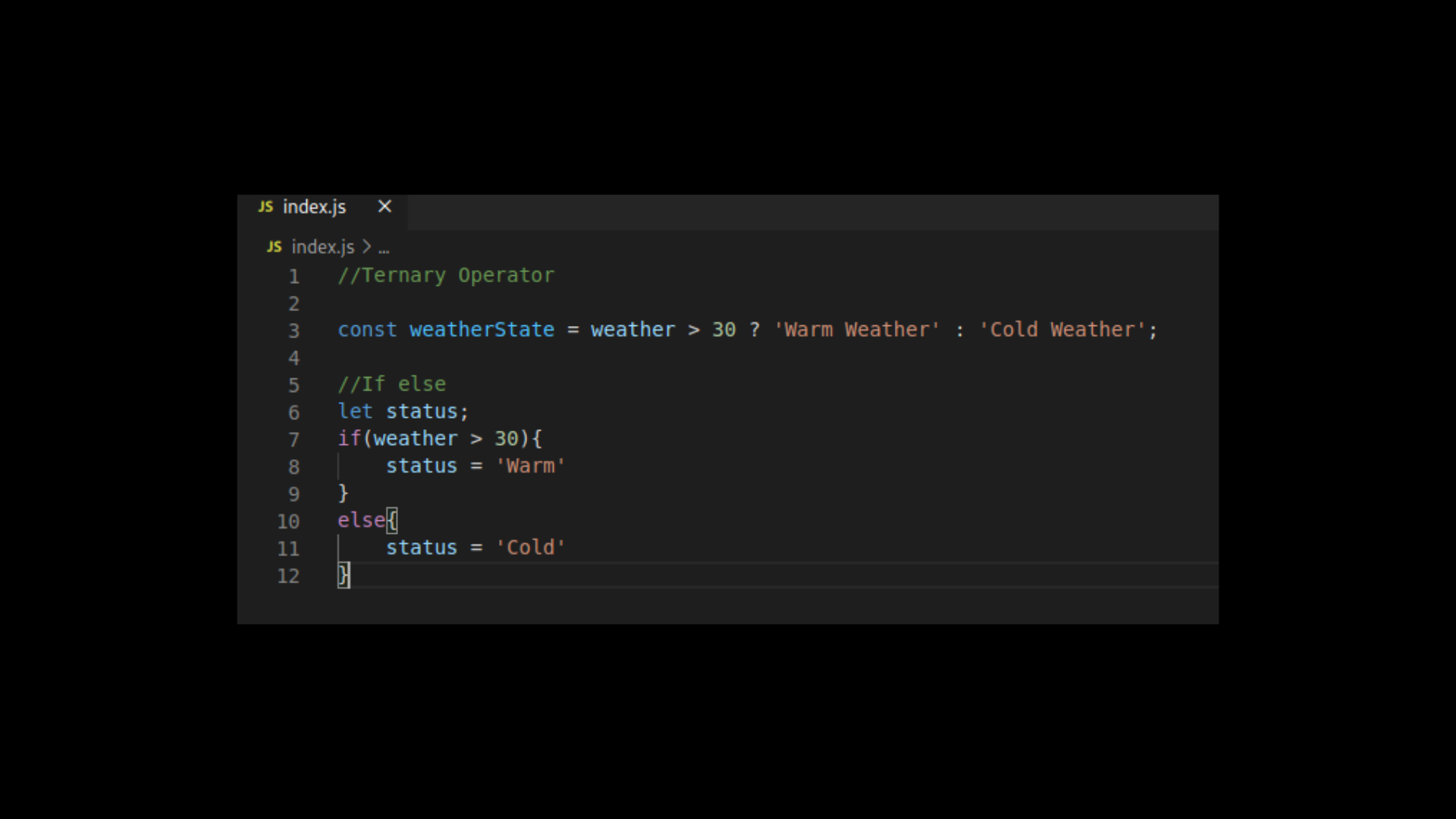
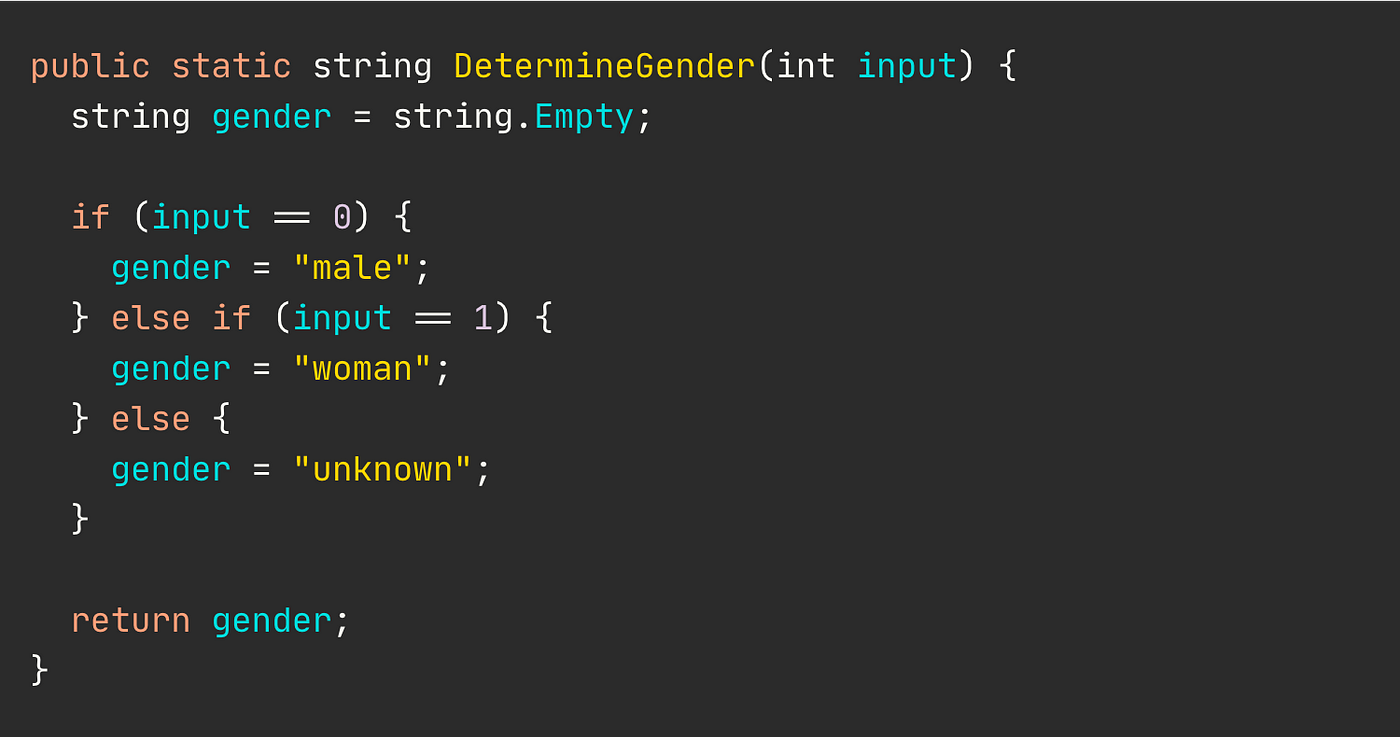
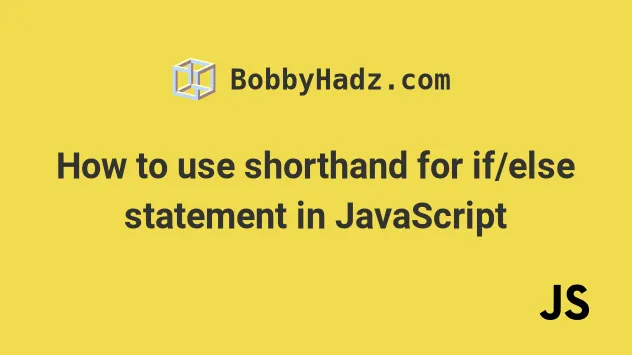
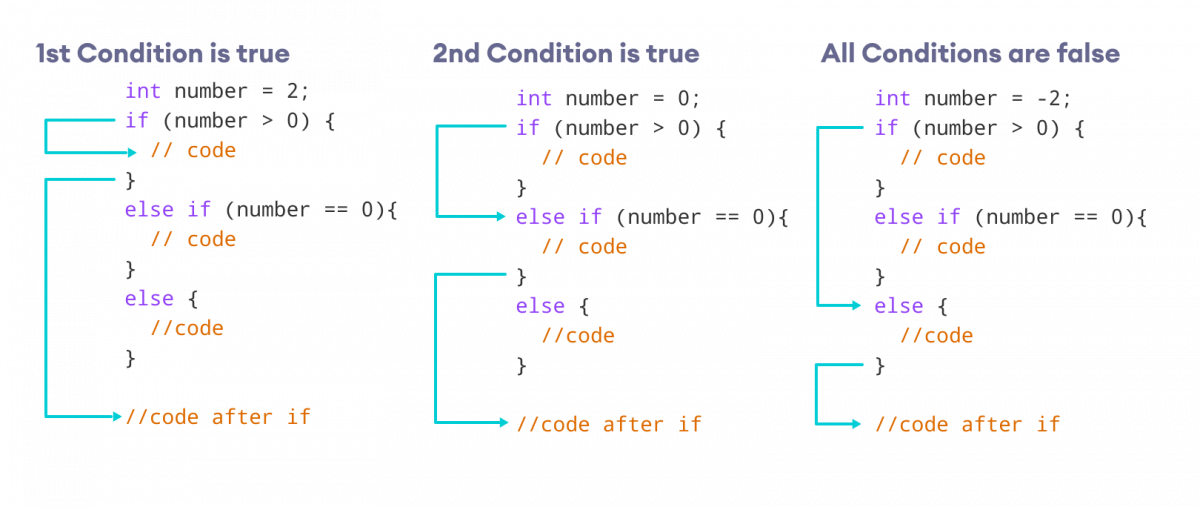
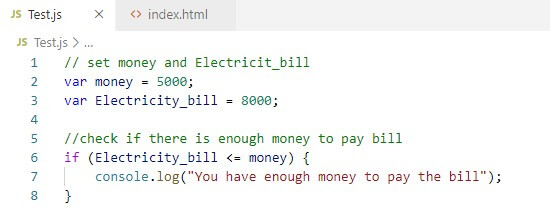



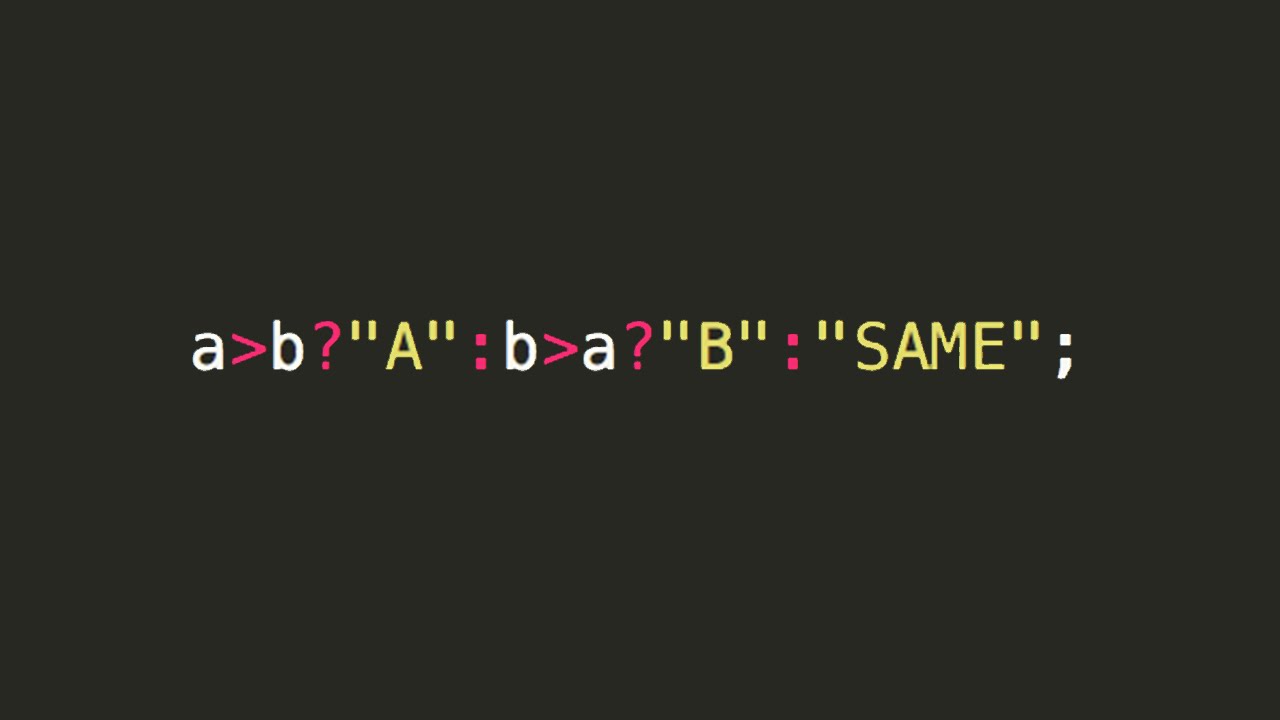
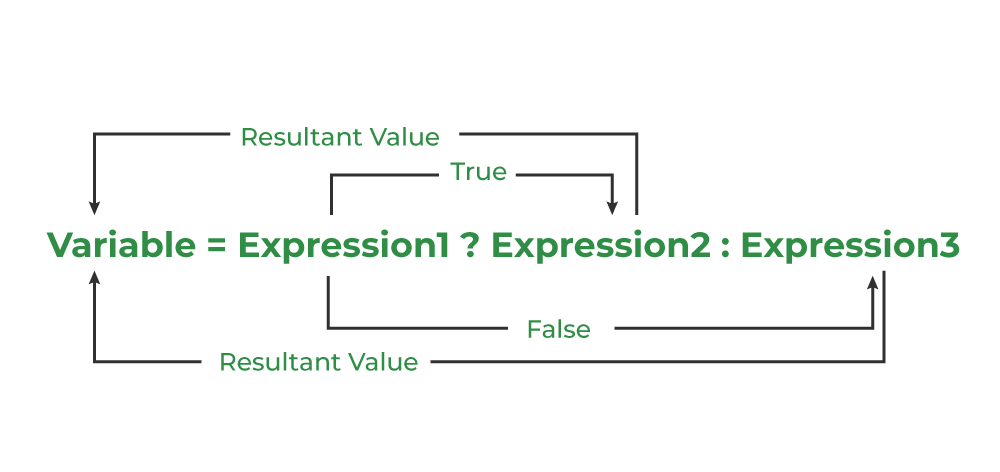
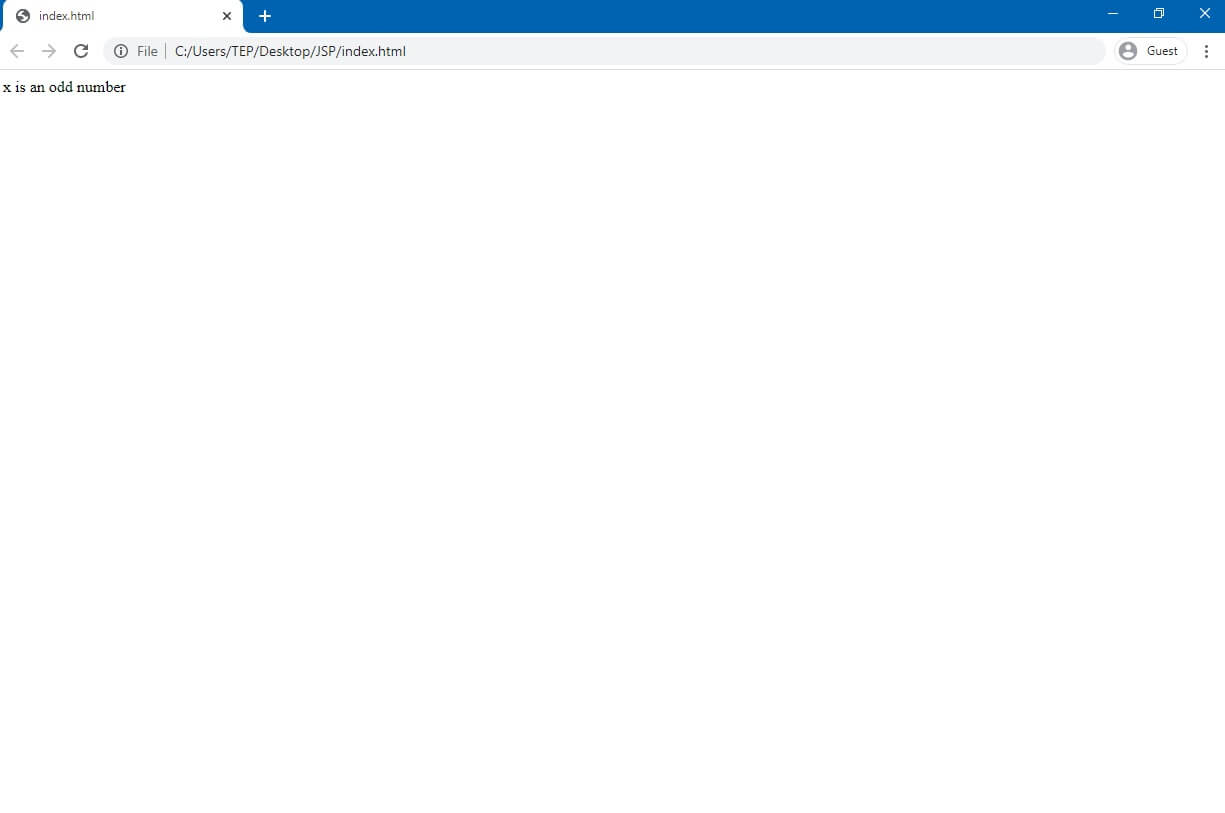



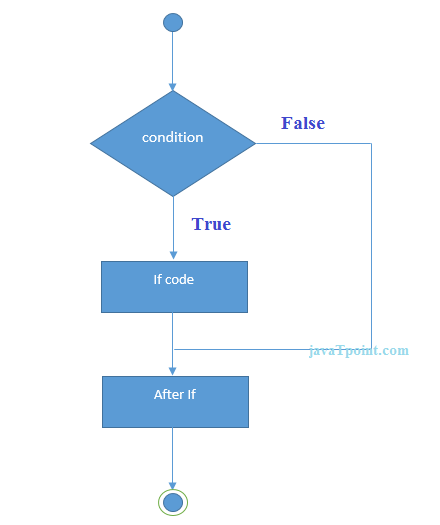
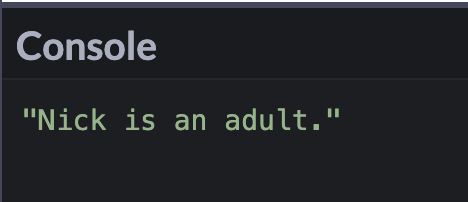

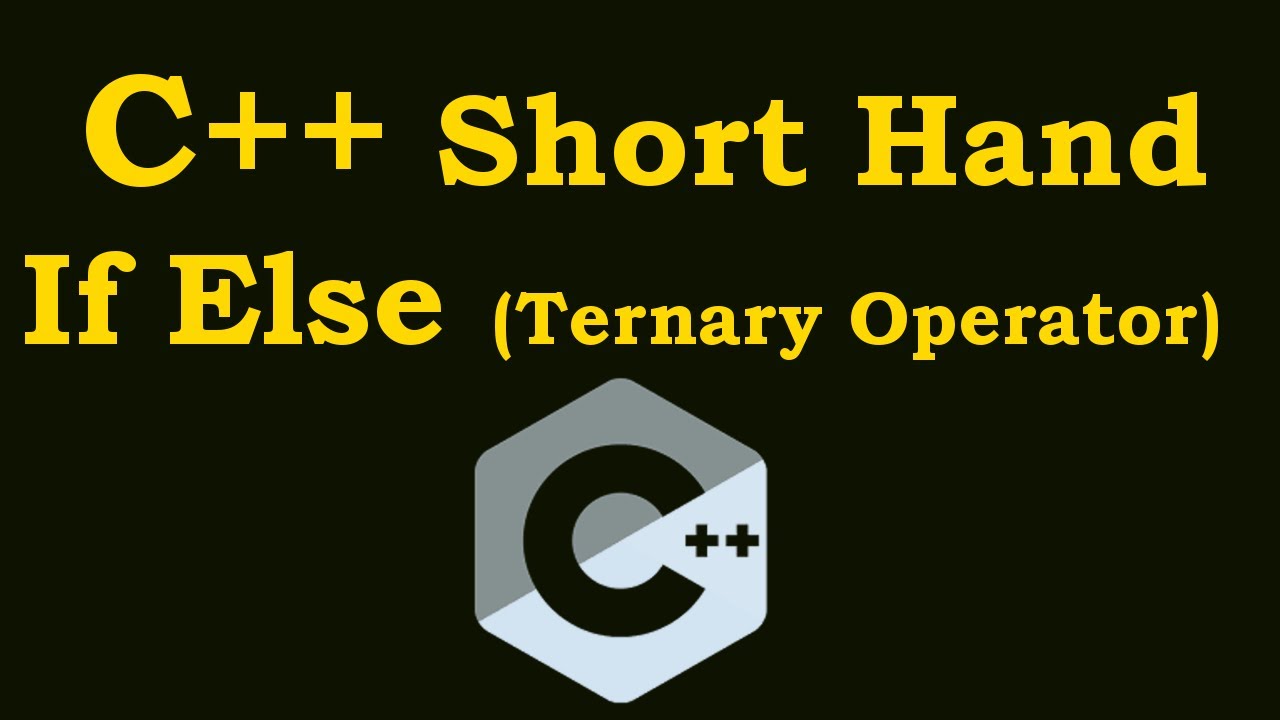
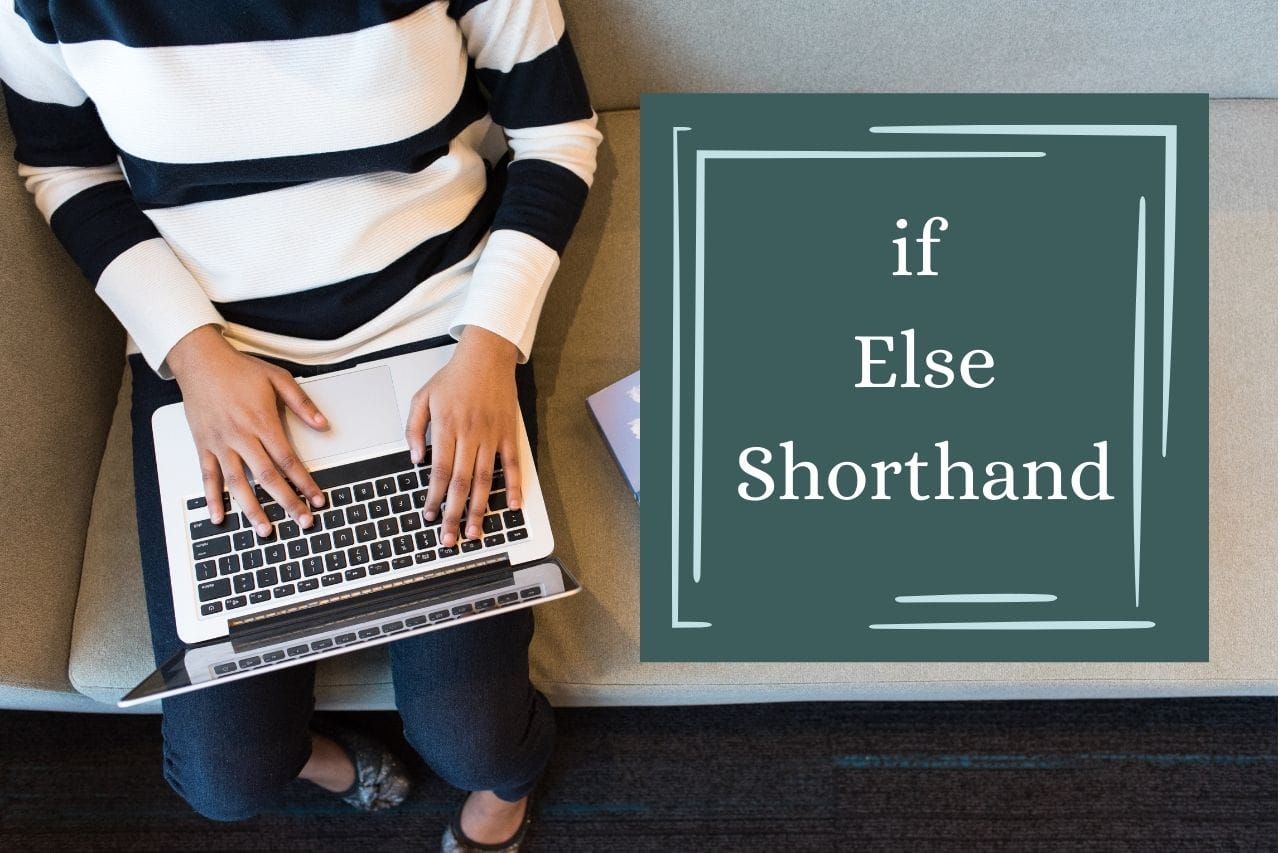
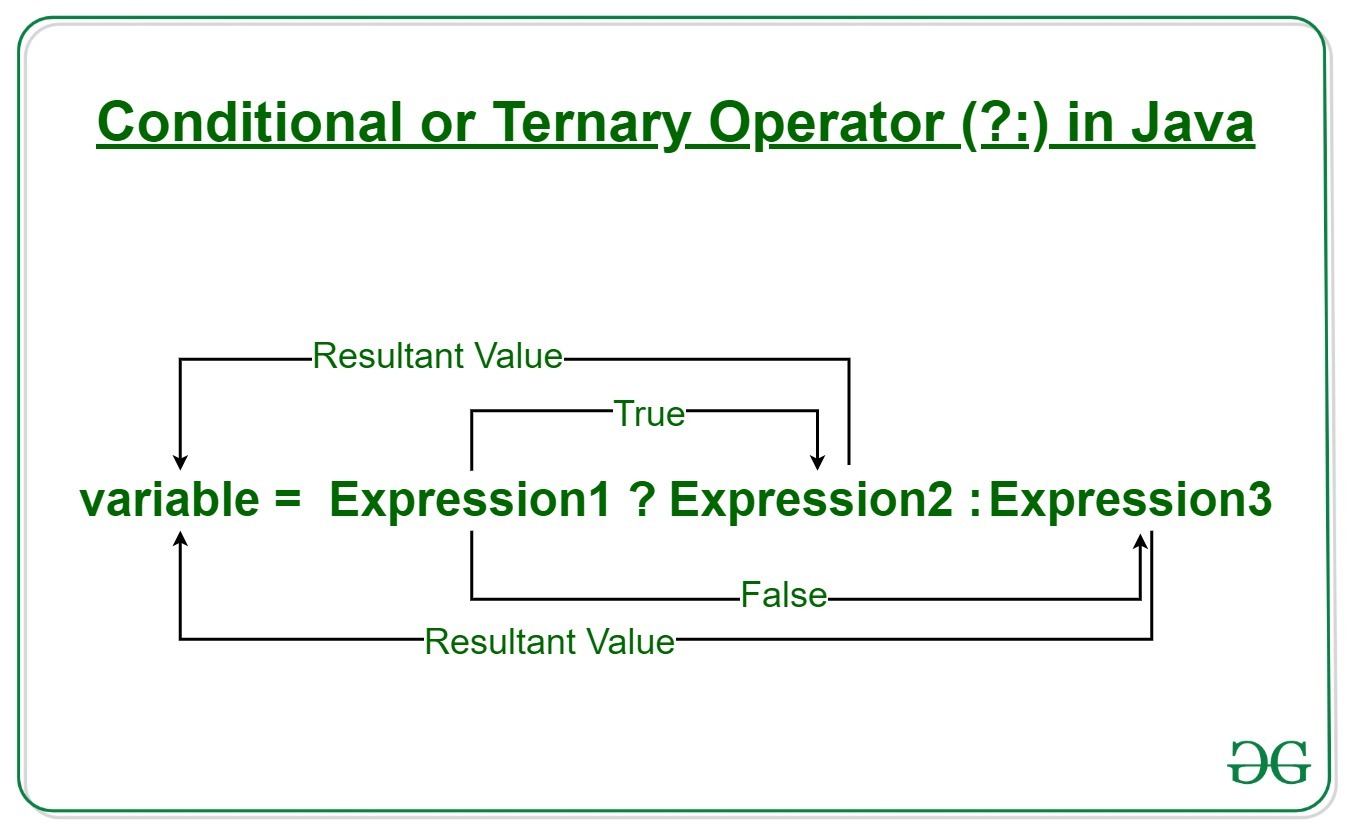
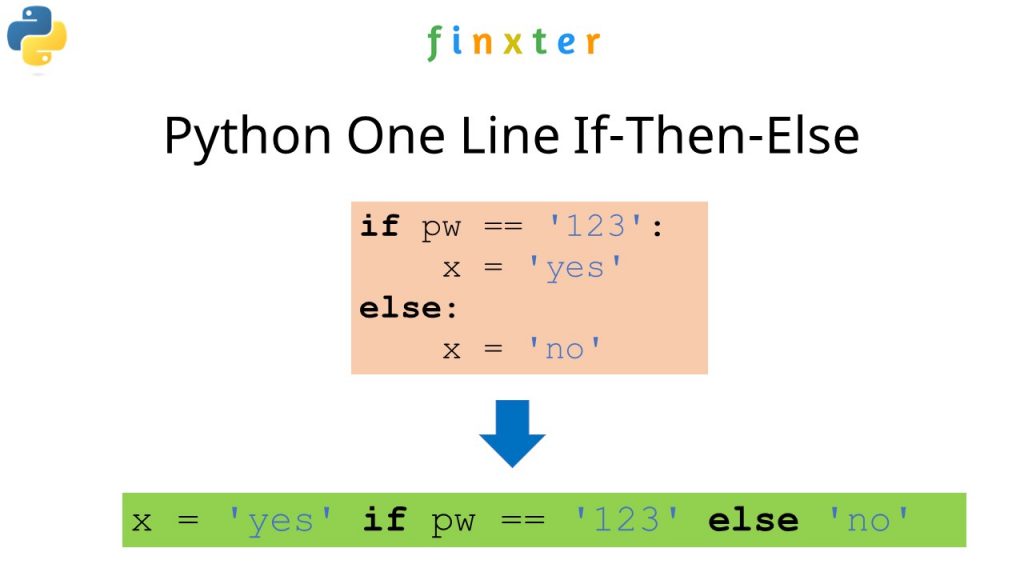
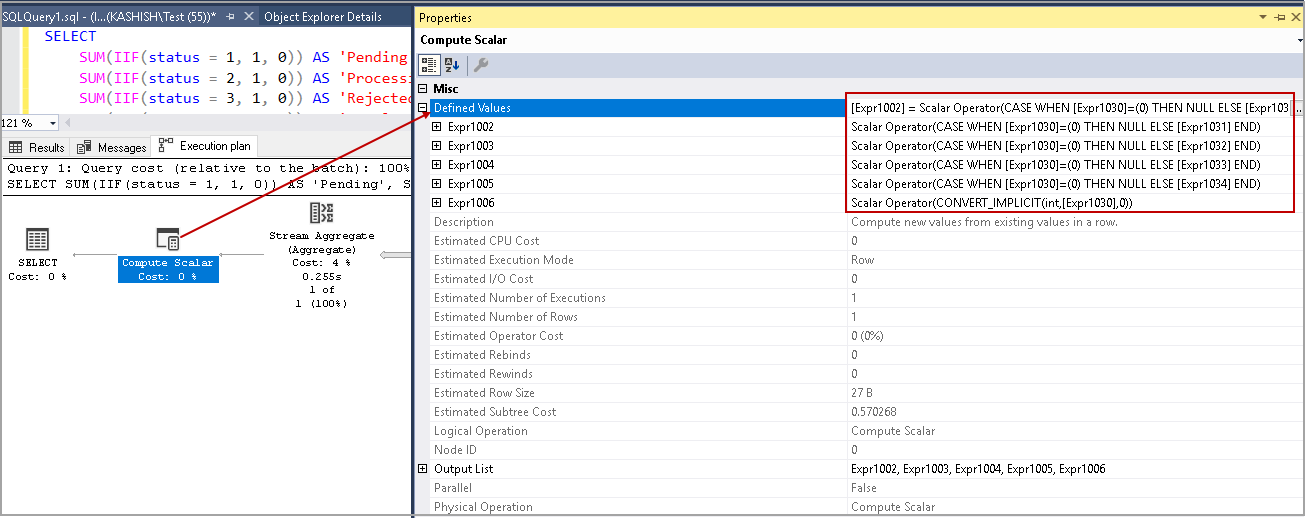


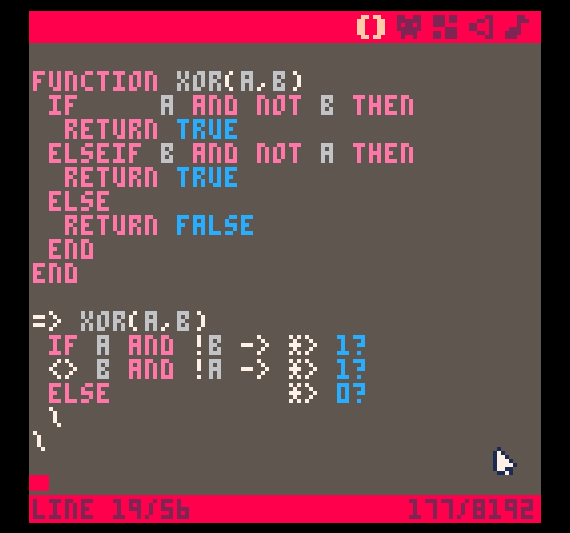
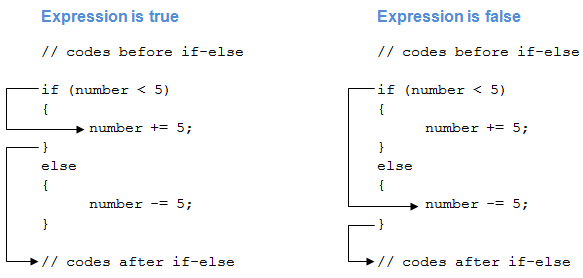
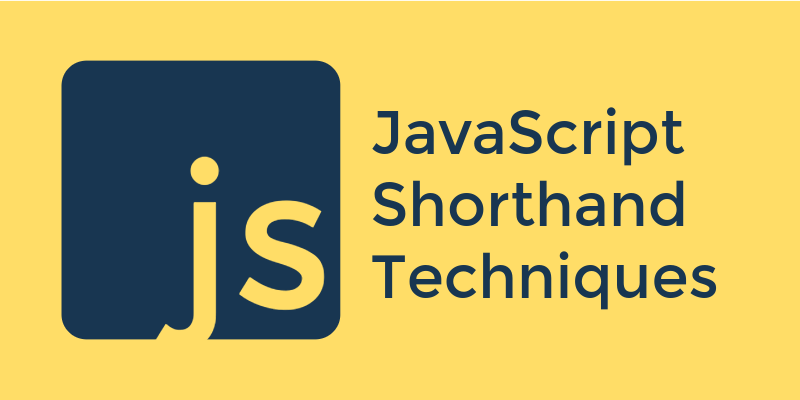
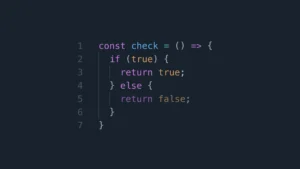
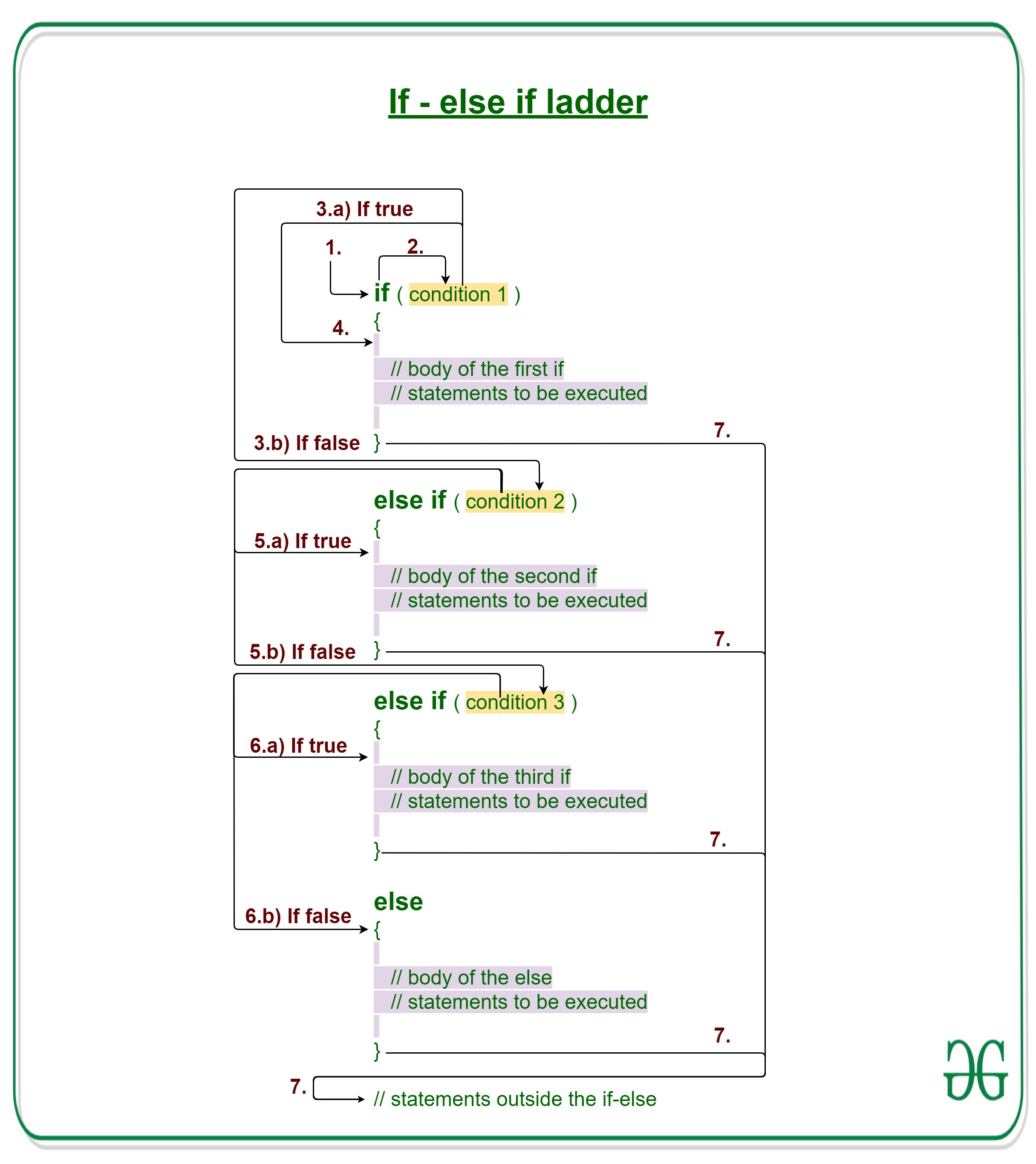
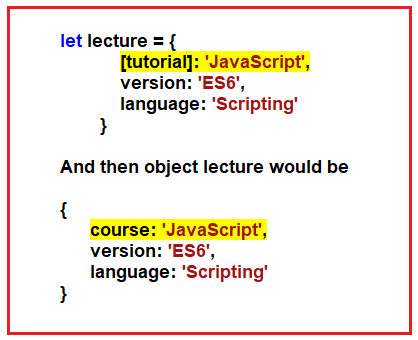

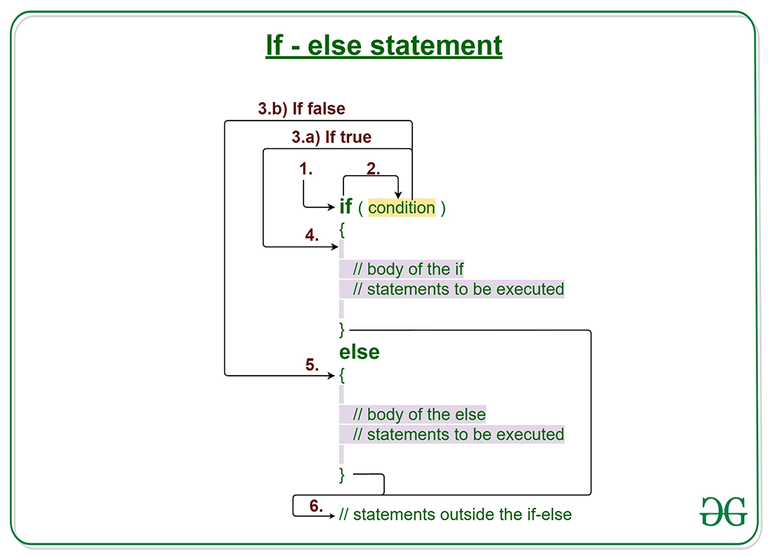
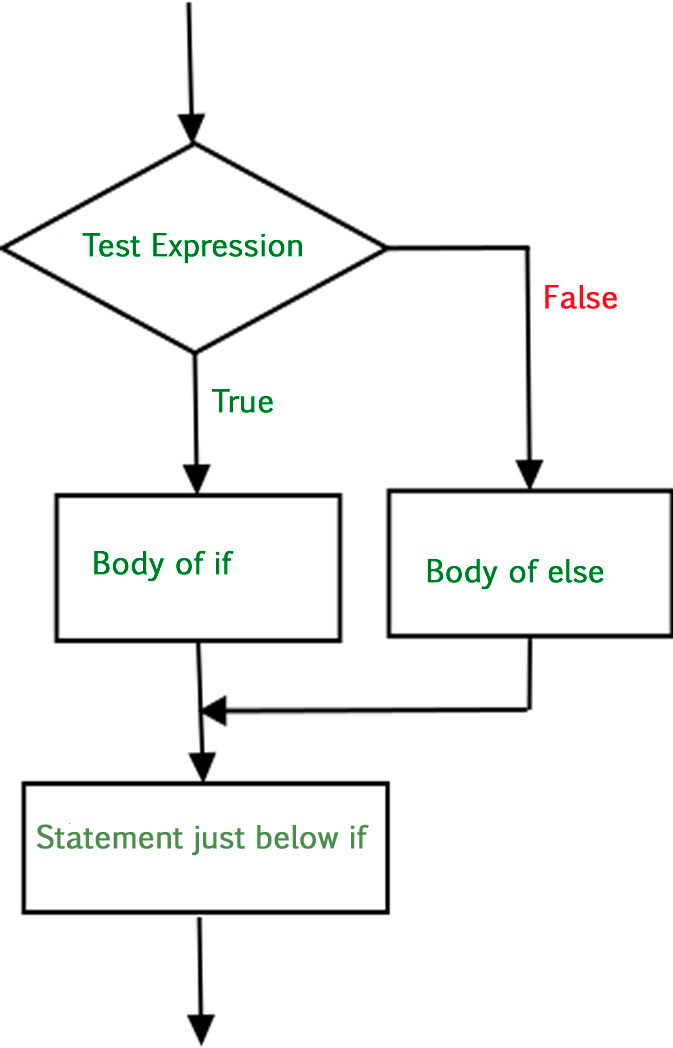

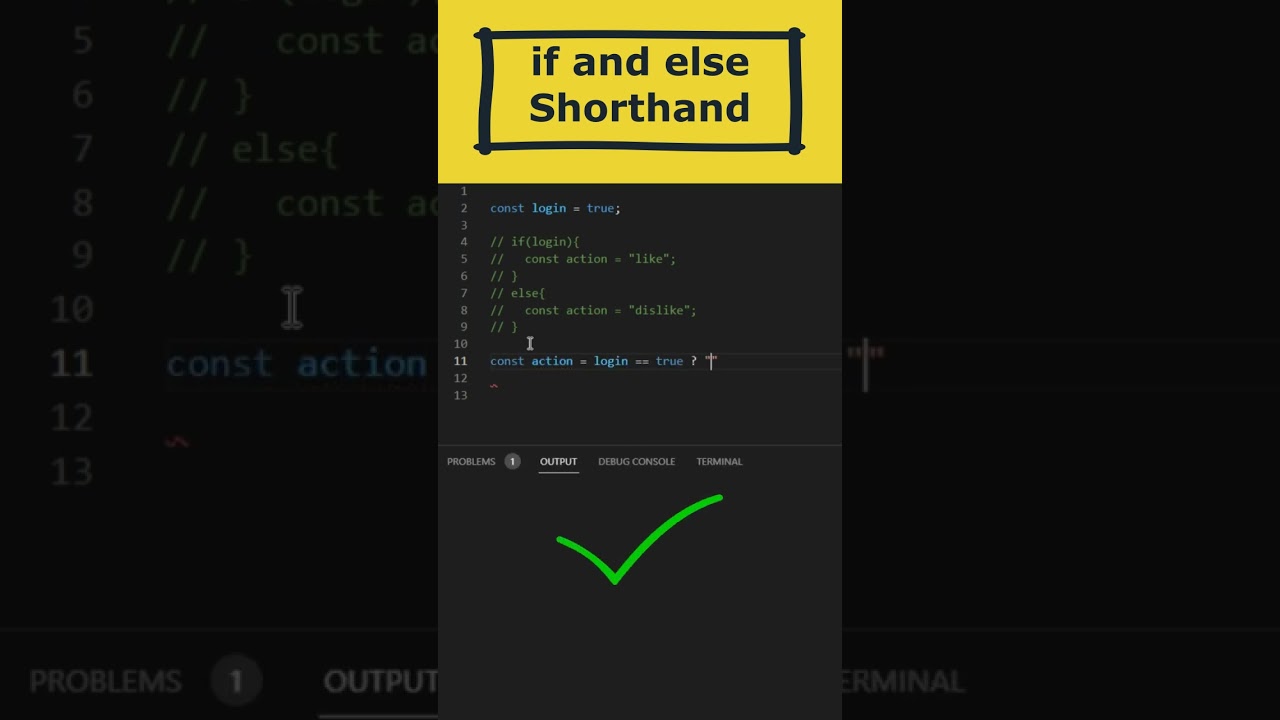

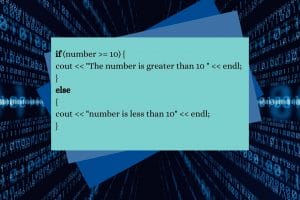
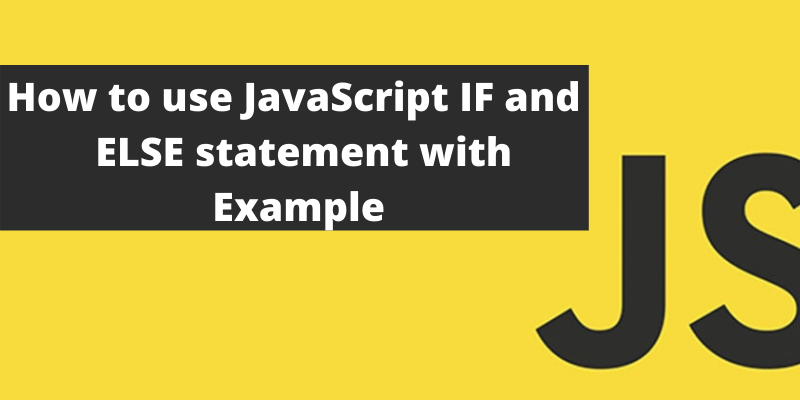
Article link: javascript else if shorthand.
Learn more about the topic javascript else if shorthand.
- How to use shorthand for if/else statement in JavaScript
- shortHand if else-if and else statement – Stack Overflow
- JavaScript if else shorthand | Example code
- TypeScript if else – TutorialsTeacher
- Conditional (ternary) operator – JavaScript – MDN Web Docs
- How can I use a one-line if statement in JavaScript? – Gitnux Blog
- Javascript shorthand for if/else statements (ternary operator …
- Conditional (ternary) operator – JavaScript – MDN Web Docs
- How To Write Conditional Statements in JavaScript
- JavaScript if shorthand without the else – Daily Dev Tips
- JavaScript If-Else and If-Then – JS Conditional Statements
See more: https://nhanvietluanvan.com/luat-hoc/