Javascript Change Image Src
When it comes to dynamically changing an image source using JavaScript, the first step is to set up the HTML structure. This usually involves creating an image element with a unique id or class for easy identification. Let’s take a look at an example:
“`html

“`
In this example, we have a basic HTML structure with an image element whose source is set to “original_image.jpg”. We have also given the image element an id of “myImage”, which we will use to access and modify the image source dynamically using JavaScript.
Creating an image element
To change the image source dynamically, we need to first access the image element using JavaScript. This can be done by creating a new Image object and assigning it to a variable. Here’s an example:
“`javascript
var image = new Image();
“`
Accessing the image element
Now that we have created an image object, we can access the image element using the unique id or class we gave it in the HTML structure. Let’s continue with our previous example:
“`javascript
var image = document.getElementById(“myImage”);
“`
In this example, we use the getElementById() function to access the image element with the id “myImage” and assign it to the variable “image”. This allows us to perform actions on the image element in the following steps.
Changing the image source dynamically
Once we have accessed the image element, we can change its source dynamically using JavaScript. This is achieved by modifying the “src” attribute of the image element. Let’s see an example:
“`javascript
image.src = “new_image.jpg”;
“`
In this example, we simply assign the new image source “new_image.jpg” to the “src” attribute of the image element. This change takes effect immediately, and the image on the web page will update to the new source.
Using JavaScript event listeners
In some cases, you might want to change the image source based on user interactions, such as clicking a button. JavaScript event listeners can be used to detect these interactions and trigger the image source change. Here’s an example:
“`javascript
var button = document.getElementById(“myButton”);
button.addEventListener(“click”, function() {
image.src = “new_image.jpg”;
});
“`
In this example, we access the button element with the id “myButton” and add a click event listener to it. When the button is clicked, the anonymous function is executed, and the image source is changed to “new_image.jpg”. This allows for more interactive and dynamic image changes on your web page.
Best practices for optimizing image changes
When changing image sources dynamically using JavaScript, there are a few best practices to keep in mind for optimal performance:
1. Avoid unnecessary image loads: Only change the image source when necessary. If the new image is already loaded on the page, there is no need to initiate another image load.
2. Preload images: If you plan to change an image source frequently, consider preloading the images in advance to reduce latency. This can be achieved by creating new Image objects and assigning the new source URLs to their “src” attributes.
3. Use image placeholders: To prevent broken image links while the new image is loading, consider using placeholder images with a low file size. These placeholders can be replaced with the actual images once they finish loading.
4. Use image compression: Optimize the file size of your images to improve loading times. There are various image compression tools available that can reduce the file size without compromising image quality.
FAQs:
Q: How can I set the image source using jQuery?
A: In jQuery, you can set the image source using the attr() function. Here’s an example:
“`javascript
$(“#myImage”).attr(“src”, “new_image.jpg”);
“`
Q: How can I change the image source using jQuery?
A: Similarly, you can change the image source using the attr() function in jQuery. Here’s an example:
“`javascript
$(“#myImage”).attr(“src”, “new_image.jpg”);
“`
Q: How can I change the image source on click using JavaScript?
A: You can achieve this by adding an onclick event listener to the element that triggers the image change. Here’s an example:
“`javascript
var button = document.getElementById(“myButton”);
button.onclick = function() {
image.src = “new_image.jpg”;
};
“`
Q: How can I set the image source using JavaScript?
A: You can set the image source by directly assigning the new source URL to the “src” attribute of the image element. Here’s an example:
“`javascript
image.src = “new_image.jpg”;
“`
Q: How can I get the current source value of an image tag using JavaScript?
A: You can retrieve the current source value of an image tag using the “src” property of the image element. Here’s an example:
“`javascript
var srcValue = image.src;
“`
Q: How can I change the source of an image using JavaScript?
A: To change the source of an image dynamically using JavaScript, assign the new source URL to the “src” attribute of the image element. Here’s an example:
“`javascript
image.src = “new_image.jpg”;
“`
Q: How can I change the image source on clicking a button using JavaScript?
A: You can add an event listener to the button element and change the image source within the event callback function. Here’s an example:
“`javascript
var button = document.getElementById(“myButton”);
button.addEventListener(“click”, function() {
image.src = “new_image.jpg”;
});
“`
By following these guidelines and utilizing the power of JavaScript, you can easily change image sources dynamically on your web page, providing a more engaging and interactive user experience. Remember to optimize your image changes using best practices to ensure optimal performance. Happy coding!
How To Change Image Src On Click Using Javascript [Howtocodeschool.Com]
How To Change The Src Of An Image Using Javascript?
In today’s digital world, web developers are constantly looking for ways to enhance the interactivity and functionality of their web pages. JavaScript is a powerful programming language that allows developers to achieve this goal by incorporating dynamic elements into their websites. One of the common tasks developers often face is changing the src (source) of an image using JavaScript. In this article, we will explore various methods and techniques to accomplish this task effectively.
The src attribute of an image element specifies the URL of the image to be displayed. Changing the src dynamically enables developers to deliver a dynamic and engaging user experience by altering the image content on their web pages.
Method 1: Using JavaScript’s getElementById() method
One of the simplest ways to change the src of an image is by using JavaScript’s getElementById() method. This method allows us to select an element by its unique id attribute. Here’s how it can be used:
“`javascript
var img = document.getElementById(“myImage”);
img.src = “new-image.jpg”;
“`
In this example, we first select the image element using its id “myImage” and store it in the variable “img”. We then assign the new source URL “new-image.jpg” to the src attribute of the image element.
Method 2: Accessing image elements by their class or tag name
In some cases, it may be more convenient to target multiple image elements at once. This can be achieved by using JavaScript’s getElementsByClassName() or getElementsByTagName() methods. Here’s an example of how to use these methods:
“`javascript
var images = document.getElementsByClassName(“myImages”);
for (var i = 0; i < images.length; i++) {
images[i].src = "new-image.jpg";
}
```
In this example, we first select all image elements with the class "myImages" and store them in the "images" array. We then loop through each image element and assign the new source URL to its src attribute.
Method 3: Using jQuery's attr() method
If you're already using jQuery in your web project, you can take advantage of its convenient attr() method to change the src of an image. Here's an example:
```javascript
$("#myImage").attr("src", "new-image.jpg");
```
In this example, we use jQuery's selector syntax to target the image element with the id "myImage" and change its src attribute to "new-image.jpg".
FAQs:
Q1: Can I change the src of an image without using JavaScript?
A1: No, changing the src of an image dynamically requires the use of JavaScript. It is not possible to accomplish this task using HTML or CSS alone.
Q2: Do I need to load the new image before changing the src?
A2: It is recommended to preload the new image before changing the src attribute. This helps ensure that the new image is fully loaded and ready to be displayed when the src is changed. This can be done by creating a new Image object and setting its src property to the new image URL before assigning it to the image element.
Q3: What happens if the new image source is invalid?
A3: If the new image source is invalid or doesn't exist, the browser will display a broken image icon or a fallback alternative specified by the alt attribute of the image element.
In conclusion, changing the src of an image using JavaScript allows web developers to dynamically alter the images displayed on their web pages. Whether you prefer using pure JavaScript or the convenience of jQuery, there are various methods and techniques available to accomplish this task. By utilizing these techniques, you can enhance the interactivity and visual appeal of your web pages, providing an engaging user experience for your visitors.
How To Dynamically Change The Image Src In Javascript?
JavaScript is a versatile programming language that allows developers to create dynamic and interactive web pages. One of the frequently encountered tasks in web development is changing the source (src) attribute of an image dynamically using JavaScript. In this article, we will explore various methods to accomplish this and delve into examples to illustrate their usage.
Table of Contents:
1. Changing the image src in JavaScript
2. Using click events to change image src dynamically
3. Using input events to change image src dynamically
4. Modifying image src based on user input
5. FAQs
1. Changing the image src in JavaScript:
The most common method of modifying the image src in JavaScript is by accessing the HTML element representing the image and changing its src attribute using JavaScript. This can be achieved by targeting the image element’s id or utilizing a query selector to select the element.
2. Using click events to change image src dynamically:
For many dynamic image changes, one needs to respond to user actions like clicks. To accomplish this, we can attach an event listener to the target element and define a function to handle the event. For example, consider the following code snippet:
“`javascript
document.getElementById(“myButton”).addEventListener(“click”, function(){
document.getElementById(“myImage”).src = “newImageUrl.jpg”;
});
“`
In this example, we attach a click event listener to an HTML button element with the id “myButton”. Upon clicking the button, the image with the id “myImage” will have its src attribute changed to “newImageUrl.jpg”. This allows you to dynamically change the image source based on user interaction.
3. Using input events to change image src dynamically:
Similar to click events, input events can also be utilized to change the image src dynamically when the user interacts with an input element. Consider the following example:
“`javascript
document.getElementById(“myInput”).addEventListener(“input”, function(){
var inputValue = document.getElementById(“myInput”).value;
document.getElementById(“myImage”).src = inputValue;
});
“`
In this example, an input event listener is attached to an input element with the id “myInput”. As the user types in the input field, the image src will be updated with the value entered in the input field. This technique is particularly useful when the user needs to provide a URL or a path to the desired image.
4. Modifying image src based on user input:
Often, dynamic changes to the image src depend on user input or selections. In such cases, we can retrieve the user’s selection or input, and dynamically update the image source accordingly. Consider the following scenario:
“`javascript
function changeImage() {
var selection = document.getElementById(“mySelect”).value;
var image = document.getElementById(“myImage”);
if (selection === “option1”) {
image.src = “image1.jpg”;
} else if (selection === “option2”) {
image.src = “image2.jpg”;
} else {
image.src = “default.jpg”;
}
}
“`
In this example, an onchange event listener is attached to a select element. The JavaScript function retrieves the selected value and accordingly changes the image src. Depending on the user’s selection, a different image will be displayed. This method can be extended for various scenarios, enabling dynamic image changes aligned with user preferences.
FAQs:
Q1. Can I change an image source dynamically without using JavaScript?
While JavaScript is the most common programming language used for dynamic image src changes, you can accomplish basic modifications without JavaScript. For instance, you can use server-side scripting languages like PHP to dynamically generate the HTML code with the desired image src. However, JavaScript offers more flexibility with instant updates based on user actions.
Q2. Why should I change image src dynamically?
Dynamic changes to image src are helpful when dealing with user interactions, such as selecting options from a drop-down menu, clicking buttons, or entering specific inputs. It allows you to enhance the user experience by displaying relevant images based on their actions or choices.
Q3. Can I dynamically change an image src without using element IDs?
While using element IDs is a common approach for targeting specific elements, you can dynamically change image src by using other selectors like classes, attributes, or element relationships. JavaScript provides a wide range of methods to select the desired element.
In conclusion, JavaScript provides several methods to dynamically change the image src in a web page. By attaching event listeners and responding to user inputs, you can create interactive experiences where the image content changes dynamically based on user actions or selections. Understanding these techniques will empower you to create engaging and responsive web applications.
Keywords searched by users: javascript change image src Set src image jQuery, Change src image jQuery, Onclick change image javascript, Set src image JavaScript, Change image js, Get src value img tag javascript, Change source image JavaScript, Click button change image JavaScript
Categories: Top 49 Javascript Change Image Src
See more here: nhanvietluanvan.com
Set Src Image Jquery
jQuery is a popular JavaScript library that simplifies the process of working with HTML documents by providing a wide range of functionalities for web developers. One such functionality is the ability to set the source (src) attribute of an image using jQuery. In this article, we will delve into the details of how to set the src image using jQuery and explore some frequently asked questions on this topic.
Setting the src image using jQuery is a straightforward process that can be accomplished using the `attr()` method. The `attr()` method allows you to get or set the value of an attribute in an HTML element. To set the src image using jQuery, you need to target the specific image element and use the `attr()` method to update its src attribute.
To illustrate this, assume we have an image element with the id “myImage” in our HTML document. We can use the following code to set its src attribute to a new image:
“`
$(‘#myImage’).attr(‘src’, ‘path/to/new/image.jpg’);
“`
In the above code, `$(‘#myImage’)` selects the image element with the id “myImage”, and `.attr(‘src’, ‘path/to/new/image.jpg’)` sets the src attribute of that element to the specified image path.
It’s important to note that the `attr()` method can be used to modify other attributes of an element as well, not just the src attribute. For instance, you can use it to change the alt text, width, height, or any other attribute of an image.
Now let’s dive into some frequently asked questions regarding setting src images using jQuery:
Q1: Can I use a variable to set the src image in jQuery?
A1: Yes, you can use a variable to dynamically set the src image in jQuery. For example, if you have a variable named `newImagePath` storing the path to the new image, you can modify the code mentioned above to:
“`javascript
var newImagePath = ‘path/to/new/image.jpg’;
$(‘#myImage’).attr(‘src’, newImagePath);
“`
Q2: How can I set alternative text (alt) for the image using jQuery?
A2: To set the alternative text for an image using jQuery, you can use the `attr()` method with the alt attribute. Here’s an example:
“`javascript
$(‘#myImage’).attr(‘alt’, ‘Alternative text for the image’);
“`
Replace the text ‘Alternative text for the image’ with your desired alternative text.
Q3: Is it possible to animate the src change of an image using jQuery?
A3: No, you cannot animate the src change of an image directly using jQuery. However, you can achieve a similar effect by using the fadeOut() or slideUp() methods to hide the image, changing the src attribute, and then using the fadeIn() or slideDown() methods to display the new image gradually. Here’s an example:
“`javascript
$(‘#myImage’).fadeOut(function() {
$(this).attr(‘src’, ‘path/to/new/image.jpg’).fadeIn();
});
“`
Q4: Can I set the src image using a URL obtained dynamically?
A4: Certainly! If the image URL is obtained dynamically, you can assign it to a variable and use it to set the src attribute. Here’s an example:
“`javascript
var dynamicURL = getDynamicURL();
$(‘#myImage’).attr(‘src’, dynamicURL);
“`
Replace `getDynamicURL()` with your own method of obtaining the URL.
Q5: What happens if the image specified in the src attribute is not found?
A5: If the image specified in the src attribute is not found, most browsers would display a broken image icon or a placeholder. To handle such cases, you can listen to the ‘error’ event of the image element and take appropriate actions.
“`javascript
$(‘#myImage’).on(‘error’, function() {
$(this).attr(‘src’, ‘path/to/placeholder.jpg’);
});
“`
In the code above, if the image fails to load, it would be replaced with a placeholder image specified by the ‘path/to/placeholder.jpg’.
In conclusion, setting the src image using jQuery is a powerful and versatile feature provided by the library. With the ability to manipulate the src attribute dynamically, you can create engaging and interactive web experiences. Whether it’s changing the image source based on user input, animating the image transition, or handling failed image loading gracefully, jQuery empowers developers to accomplish these tasks with ease.
Change Src Image Jquery
Introduction:
In the ever-evolving world of web development, jQuery has emerged as a powerful tool for altering the behavior of web pages. One common use case of jQuery is to dynamically change the source image of an HTML element. This can be achieved with just a few lines of code, making it a popular choice for many developers. In this article, we will delve deep into the concept of changing the src image using jQuery, covering various techniques and best practices along the way.
Understanding the Basics:
Before diving into the code, it is important to understand the basic structure of an image tag in HTML. The image element is denoted by the ‘‘ tag and has the ‘src’ attribute that specifies the image source. This attribute holds the URL of the image file to be displayed. By manipulating this attribute using jQuery, we can easily change the source image dynamically.
Using jQuery to Change the Src Image:
jQuery provides a simple yet powerful way to alter the src attribute of an image tag. The first step is to select the desired image using a jQuery selector. This can be done by targeting the image element directly, using its class, or even its parent element.
Once the image is selected, the ‘attr’ method can be utilized to modify the source image. The syntax for this method is as follows: $(‘imgSelector’).attr(‘src’, ‘newImageURL’);. In this syntax, ‘imgSelector’ is the selector used to identify the image element by its ID, class, or any other identifying attribute, and ‘newImageURL’ refers to the URL of the new image source.
In addition to the ‘attr’ method, jQuery also provides the ‘prop’ method which can be used in certain cases, such as changing the ‘disabled’ property of an image button. However, for changing the src attribute, the ‘attr’ method is the most commonly used and recommended approach.
Handling Events:
In most scenarios, changing the src image dynamically is triggered by an event, such as a button click or mouse hover. jQuery allows us to easily associate event handlers to elements on the page, facilitating the dynamic manipulation of the src attribute.
To initiate the change in the src image on a button click, for example, we can use the ‘click’ event handler. The code snippet below demonstrates this approach:
$(‘buttonSelector’).on(‘click’, function(){
$(‘imgSelector’).attr(‘src’, ‘newImageURL’);
});
In this code, whenever a button, identified by ‘buttonSelector’, is clicked, the defined function will be executed, changing the src attribute of an image identified by ‘imgSelector’ to the specified ‘newImageURL’. This mechanism can be extended to various other event types, making dynamic image swapping quite versatile.
Frequently Asked Questions:
Q: Can I change the src image multiple times using jQuery?
A: Yes, you can change the src image as many times as needed. jQuery allows you to modify the src attribute multiple times using either the ‘attr’ or ‘prop’ methods.
Q: Can I change the src image to an image file from a different domain?
A: Yes, jQuery permits changing the src image to a file from a different domain. However, it is crucial to be aware of the potential security risks associated with cross-origin resource sharing (CORS) policies.
Q: Can I use jQuery to change the src image of multiple elements at once?
A: Definitely! jQuery allows you to select multiple elements using classes, IDs, or any other attribute selector. By applying the ‘attr’ or ‘prop’ methods to a group of image elements, their src images can be changed simultaneously.
Q: Are there any alternatives to jQuery for changing the src image?
A: Indeed, while jQuery remains a popular choice, there are alternative libraries and frameworks such as React, Angular, or Vanilla JavaScript that offer similar functionalities. However, the choice ultimately depends on your project requirements and personal preferences.
Conclusion:
The ability to dynamically change the src image using jQuery provides endless possibilities for web developers. Whether it is updating product images on an e-commerce website or creating eye-catching image transitions, jQuery allows for fluid and seamless changes. By utilizing the ‘attr’ method and various event handlers, developers can easily manipulate the src attribute of an image tag, adding a layer of interactivity and enhancing the user experience. So, go ahead and explore the power of jQuery to dynamically modify your web page’s image sources!
Onclick Change Image Javascript
Introduction:
In the realm of web development, interactivity plays a significant role in enhancing user experience. One such element is the ability to change an image dynamically using JavaScript. The “onclick” event, widely used in JavaScript, acts as a trigger to execute a specified function when a user clicks on an HTML element. Leveraging this event, developers can easily design an interactive feature that changes images dynamically based on user interaction. In this article, we will explore the ins and outs of onclick change image JavaScript, its implementation, and common FAQs surrounding this topic.
Implementation:
To begin with, let’s delve into the step-by-step process of implementing an onclick change image JavaScript feature.
1. HTML Markup:
Create a simple HTML document with an image element and a clickable HTML element, such as a button or a link. Assign them unique IDs, as these will be used for targeting elements in JavaScript.
“`html

“`
2. JavaScript Function:
In the next step, define a JavaScript function to handle the onclick event. This function will be responsible for swapping the images. To do so, one common approach is to set the “src” attribute of the image element.
“`javascript
“`
3. Associate Function with Element:
To ensure the defined JavaScript function is called when the onclick event occurs, associate the function with the desired HTML element. In this case, when the button is clicked, the “changeImage()” function will be triggered.
“`html
“`
4. Image Replacement:
Upon clicking the button, the image element’s source will be updated, eventually swapping the image displayed on the webpage.
Common FAQs about Onclick Change Image JavaScript:
Q1. Can I change the image multiple times by clicking a single button?
A1. Absolutely! By extending the JavaScript function and image source manipulation, you can change the image any number of times on a single button click event. For instance, you can utilize if-else conditions or switch statements to toggle between different images.
Q2. How can I customize the function to dynamically load images based on user input?
A2. While the above example statically changes images, you can incorporate user input to dynamically swap images. You can add an input field and allow users to provide the image URL, manipulate the JavaScript function to read user input, and update the image source accordingly using the “value” property of the input element.
Q3. Is it possible to change images using elements other than buttons?
A3. Absolutely! Although the example provided uses a button element, the onclick event can be associated with any HTML element that supports user interaction, such as links, images, or even div elements. Simply replace the “button” element with the desired element in both HTML and JavaScript code.
Q4. Can I change images with fading or transition effects using onclick change image JavaScript?
A4. Yes, it is indeed possible to add fading or transition effects while changing images. You can utilize various JavaScript libraries like jQuery or CSS transitions to achieve smooth transitions. By changing the opacity or applying CSS classes with predefined transition effects, you can enhance the visual appeal of image swapping.
Q5. Are there any security implications I should consider when implementing onclick change image JavaScript?
A5. Security should always be a primary concern while handling user input. When incorporating user-submitted URLs for image swapping, ensure you implement proper validation to prevent any potential cross-site scripting (XSS) attacks. Validate and sanitize user input before using it as an image source to prevent any unintended security vulnerabilities.
Conclusion:
Onclick change image JavaScript is a powerful tool for adding interactivity to web pages. By following the step-by-step implementation process and leveraging the onclick event, you can easily create image swapping functionality to enhance user experience. Remember, while the example provided is simplistic, you can expand on this concept to bring more dynamic and creative elements to your website.
Images related to the topic javascript change image src
![How to change image src on click using JavaScript [HowToCodeSchool.com] How to change image src on click using JavaScript [HowToCodeSchool.com]](https://i.ytimg.com/vi/TXcjupbEst4/hqdefault.jpg)
Found 28 images related to javascript change image src theme
![How to change image src on click using JavaScript [HowToCodeSchool.com] - YouTube How To Change Image Src On Click Using Javascript [Howtocodeschool.Com] - Youtube](https://i.ytimg.com/vi/TXcjupbEst4/maxresdefault.jpg)
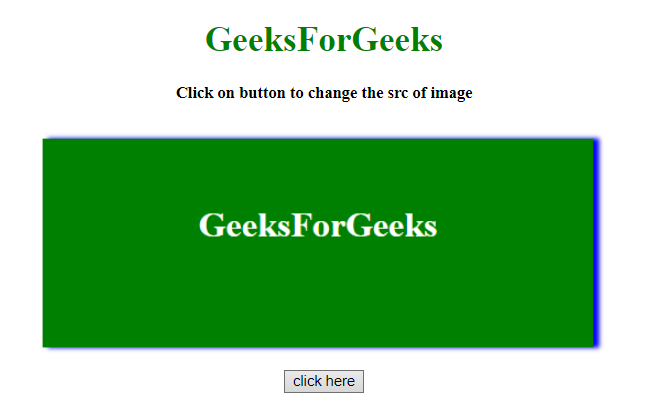
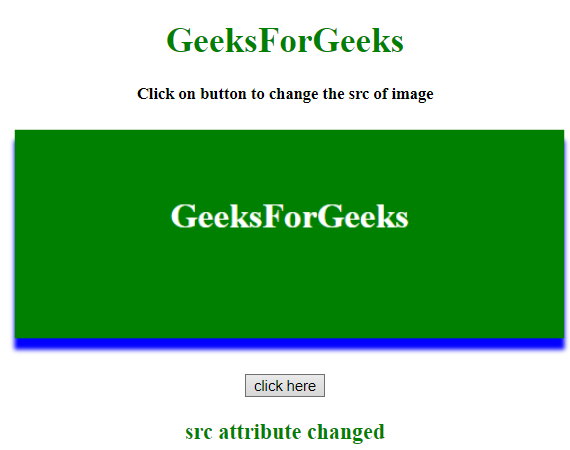


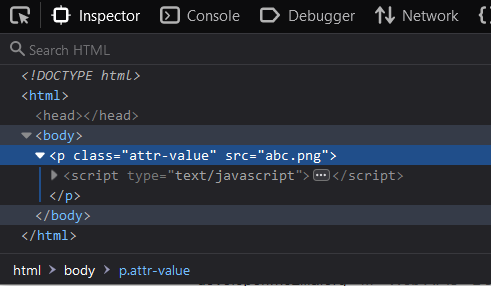


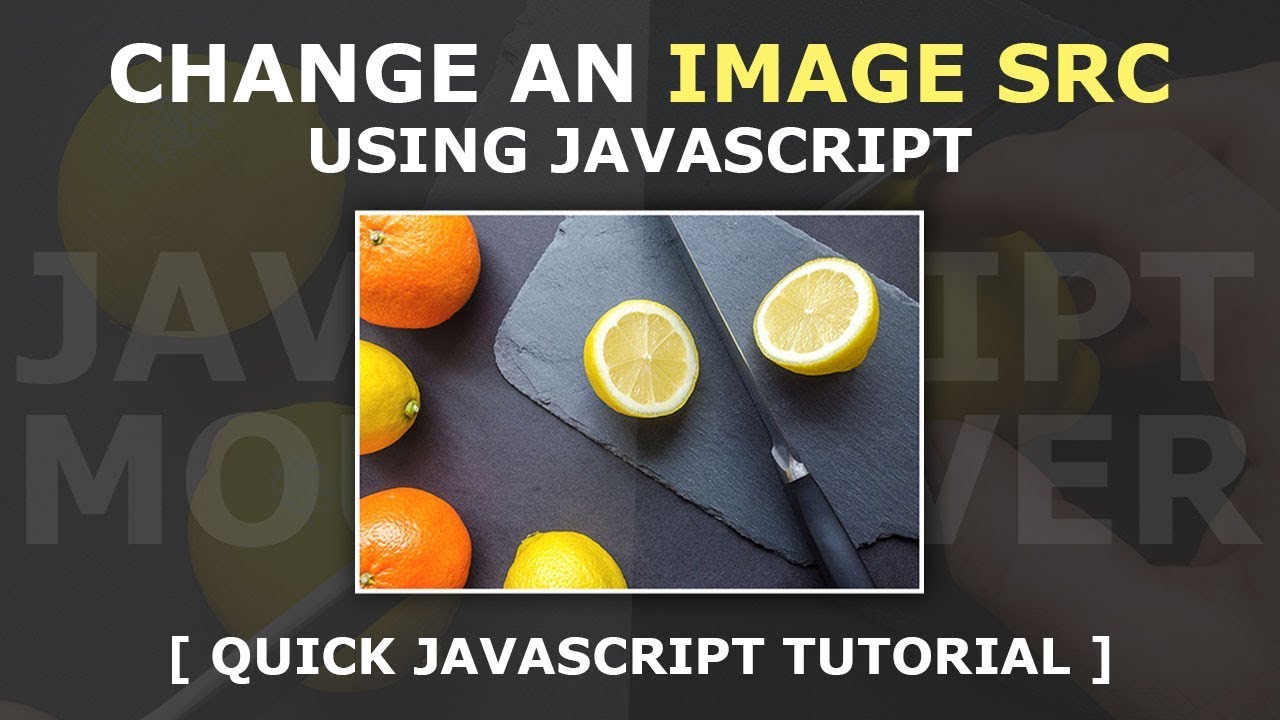
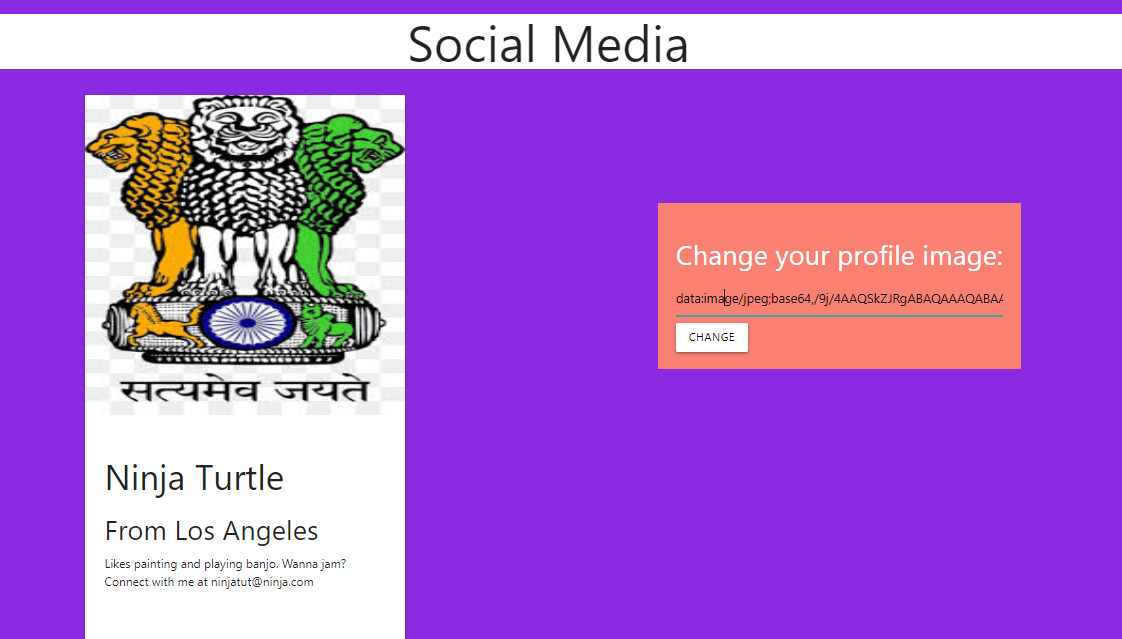
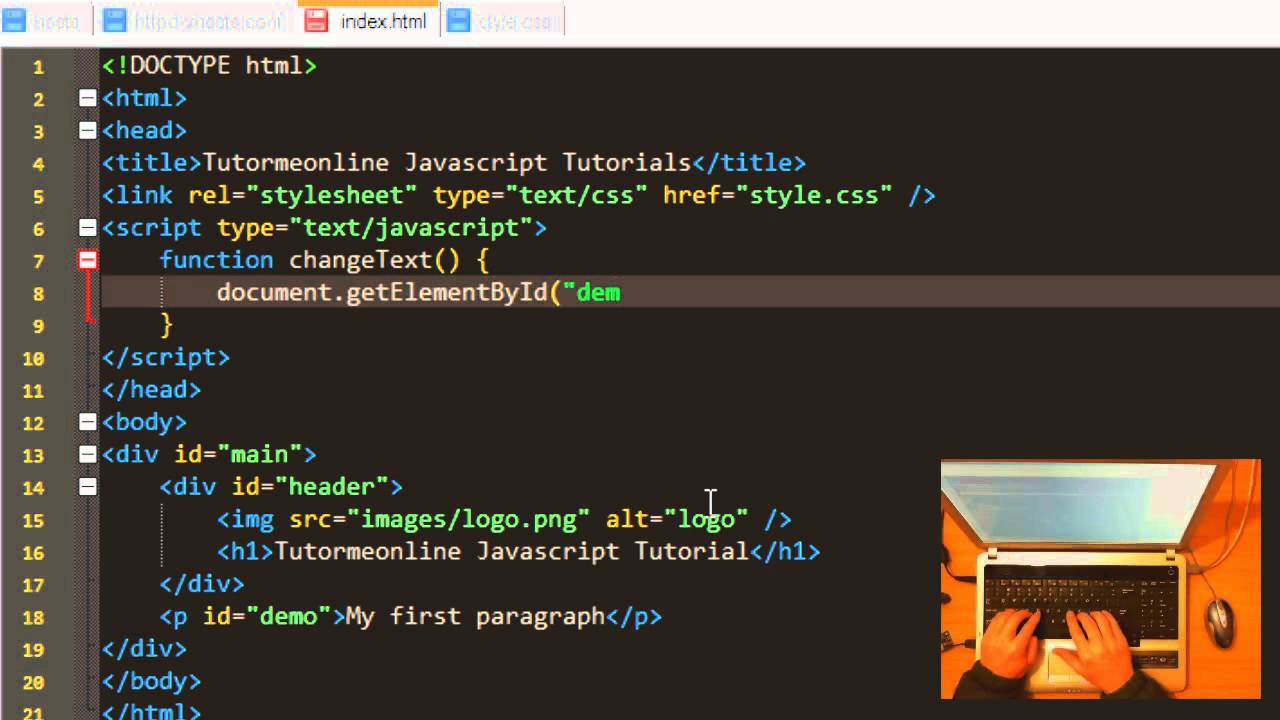
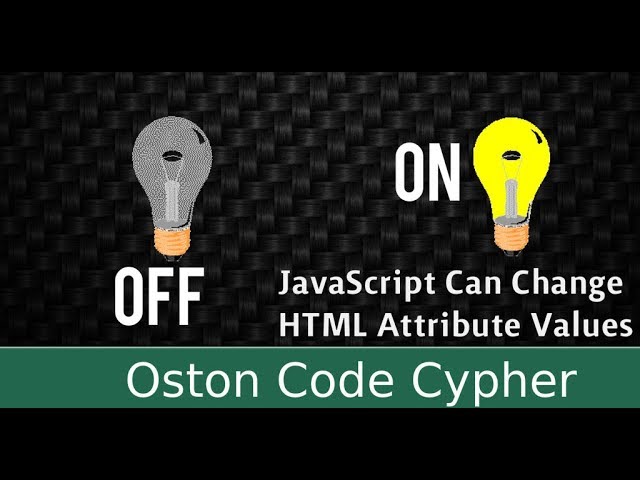
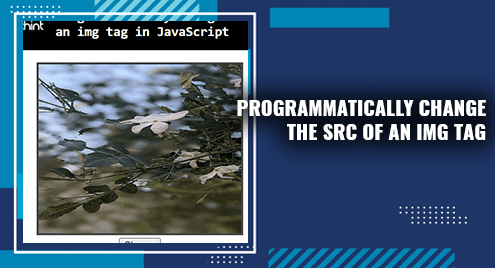
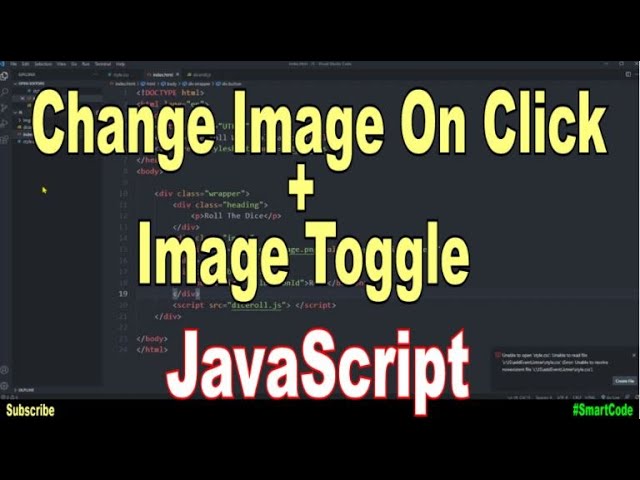

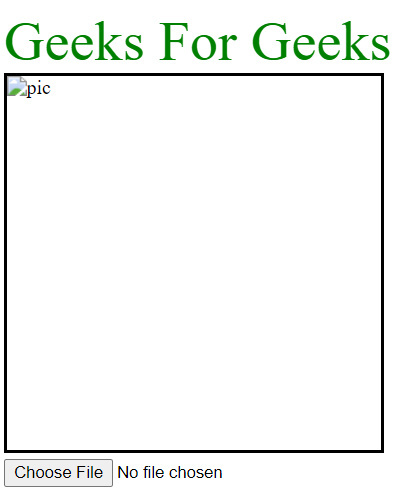
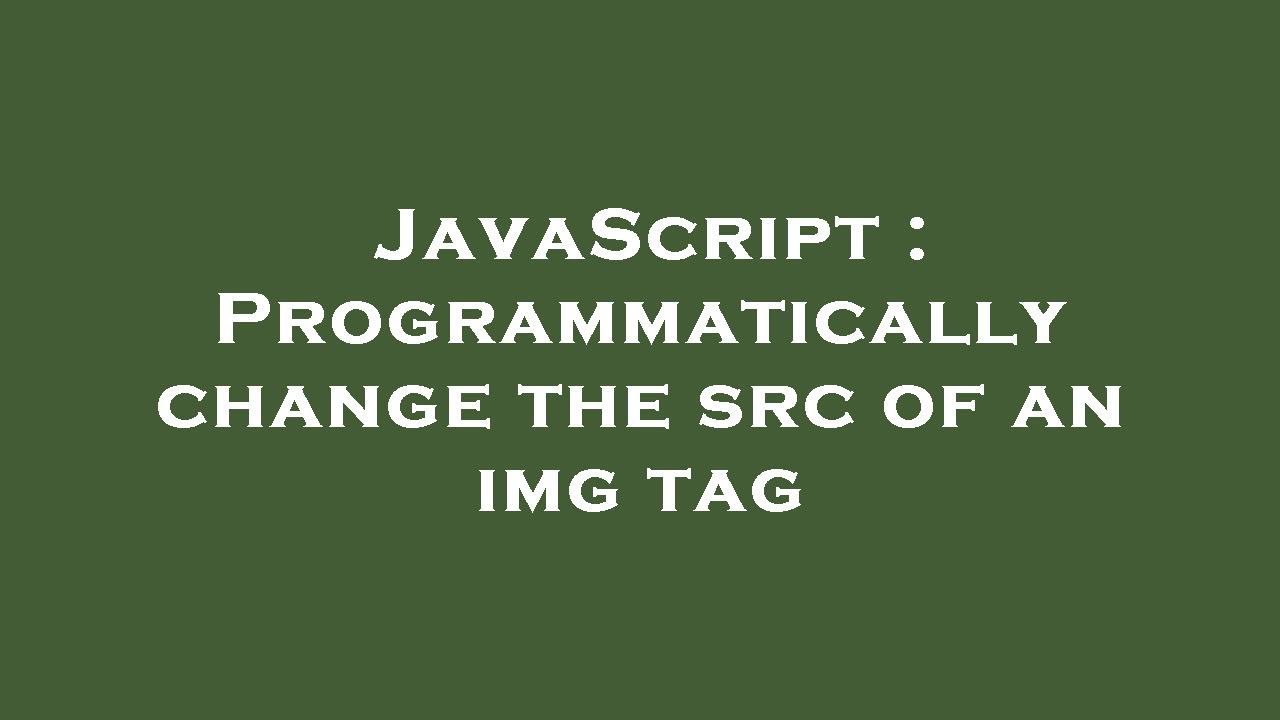
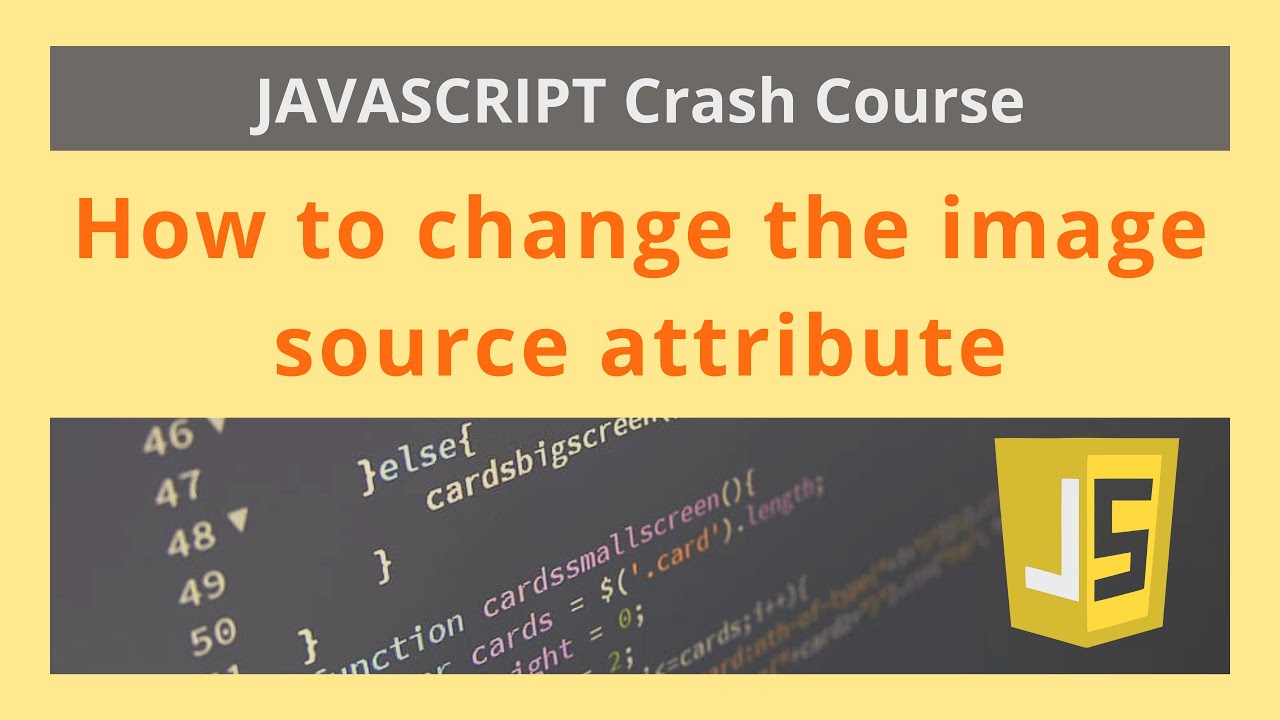
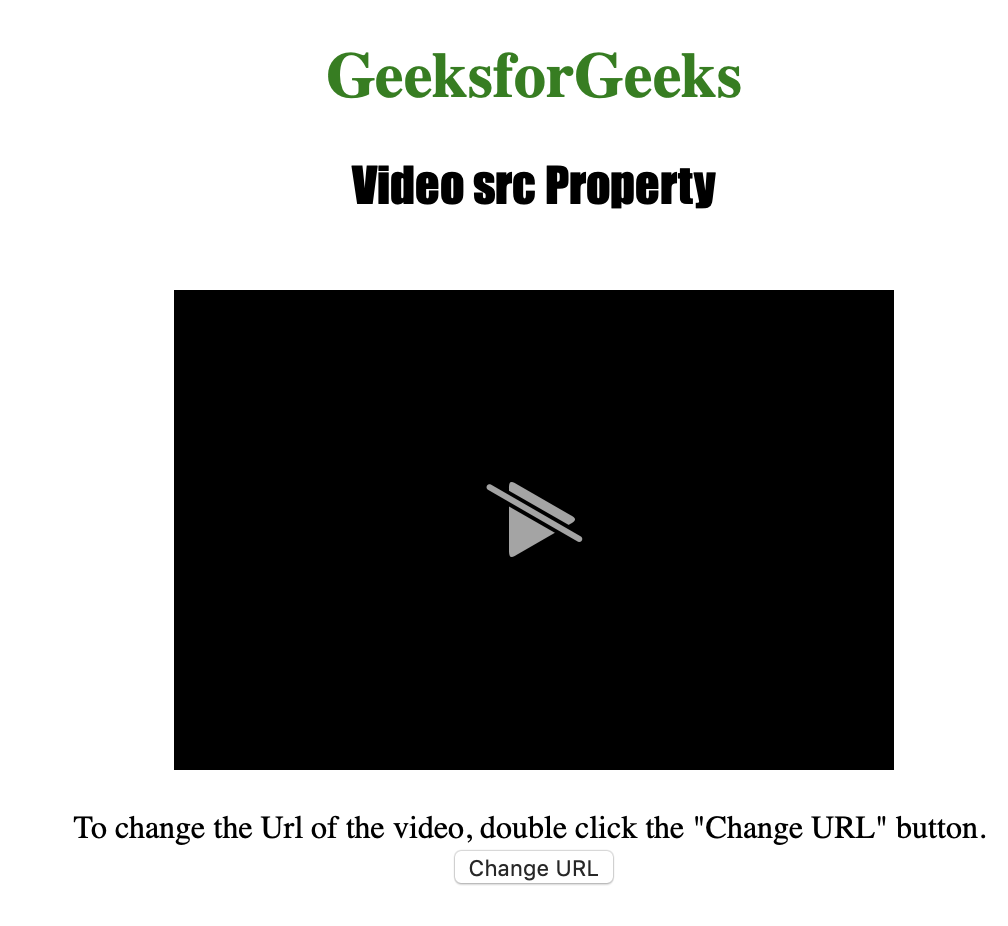

![How to change image src on click using JavaScript [HowToCodeSchool.com] - YouTube How To Change Image Src On Click Using Javascript [Howtocodeschool.Com] - Youtube](https://i.ytimg.com/vi/zXysI9dFCEA/hqdefault.jpg)
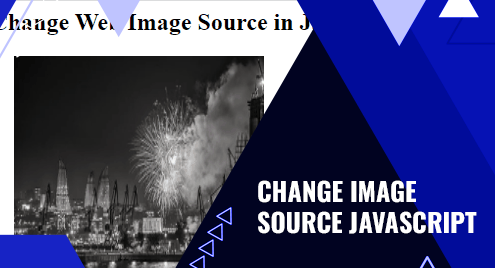
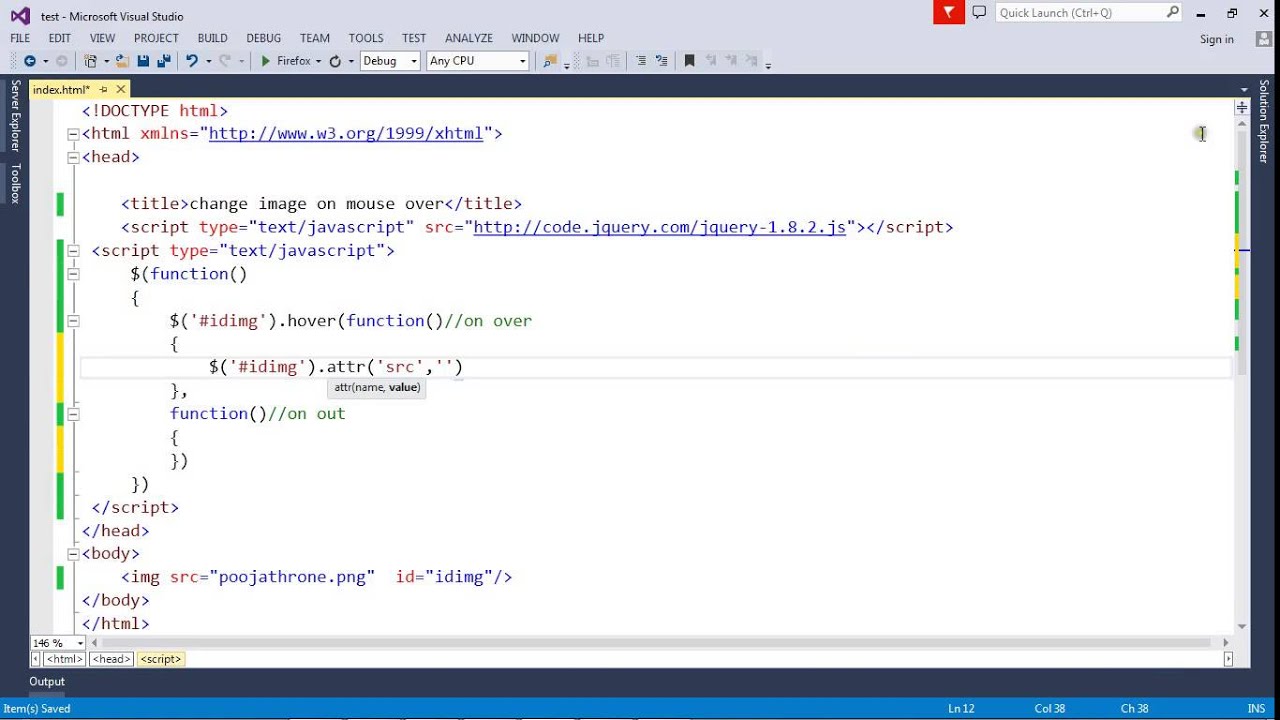

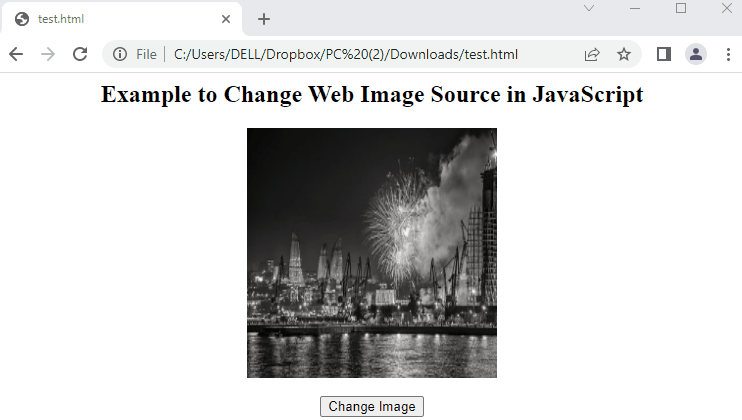
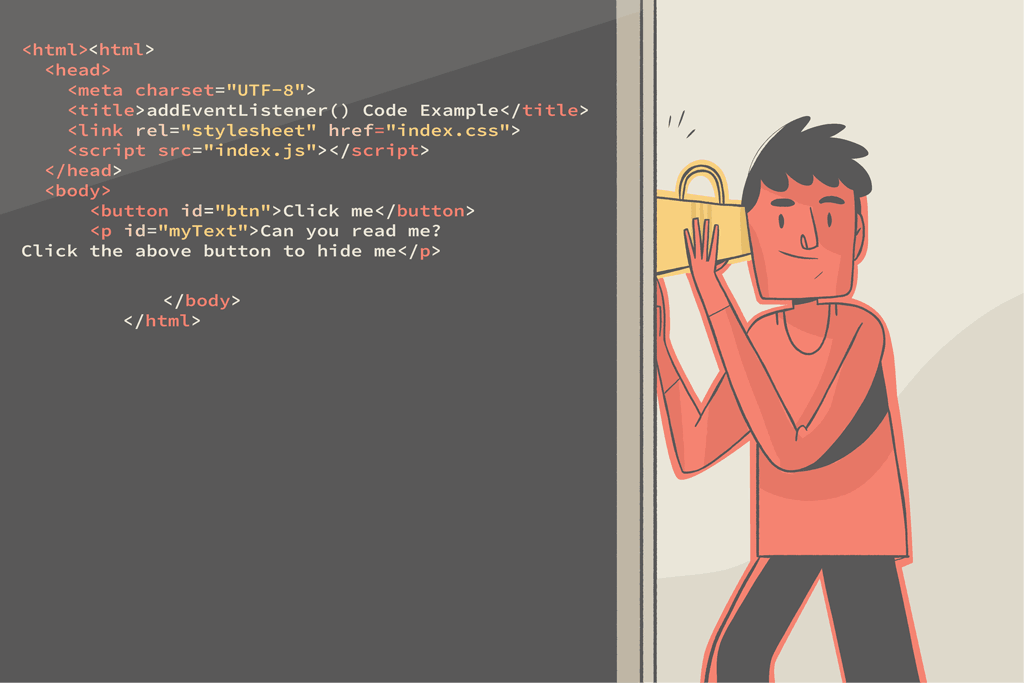
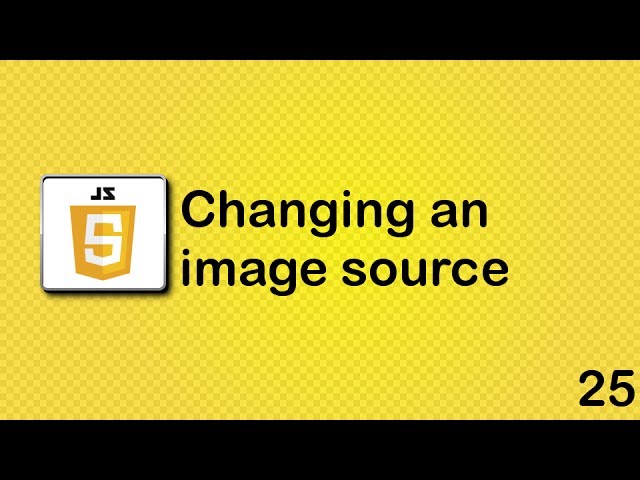

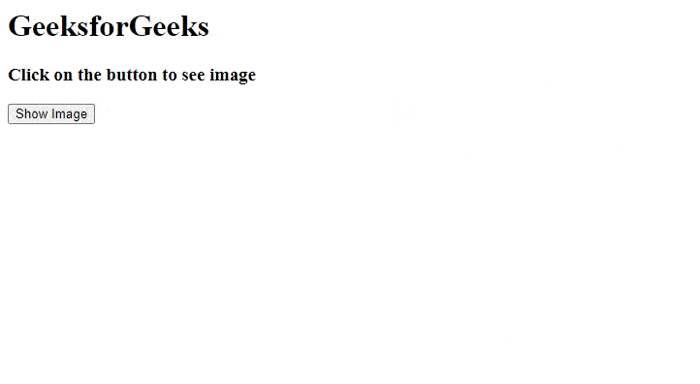
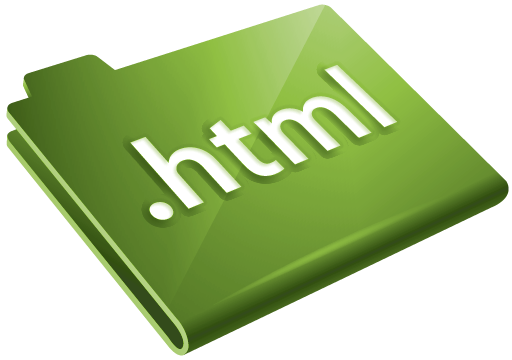
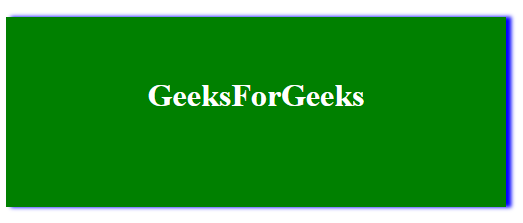
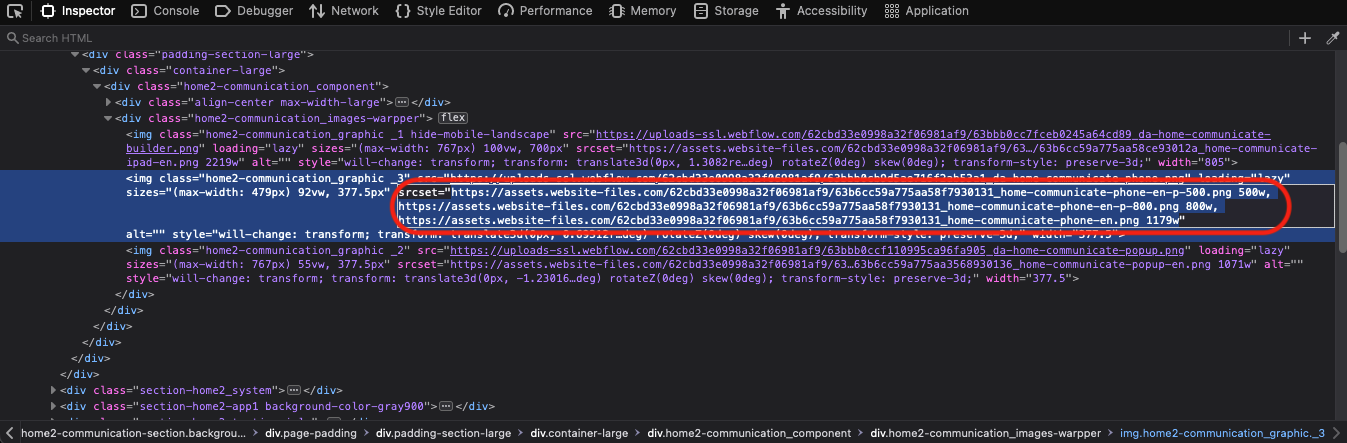
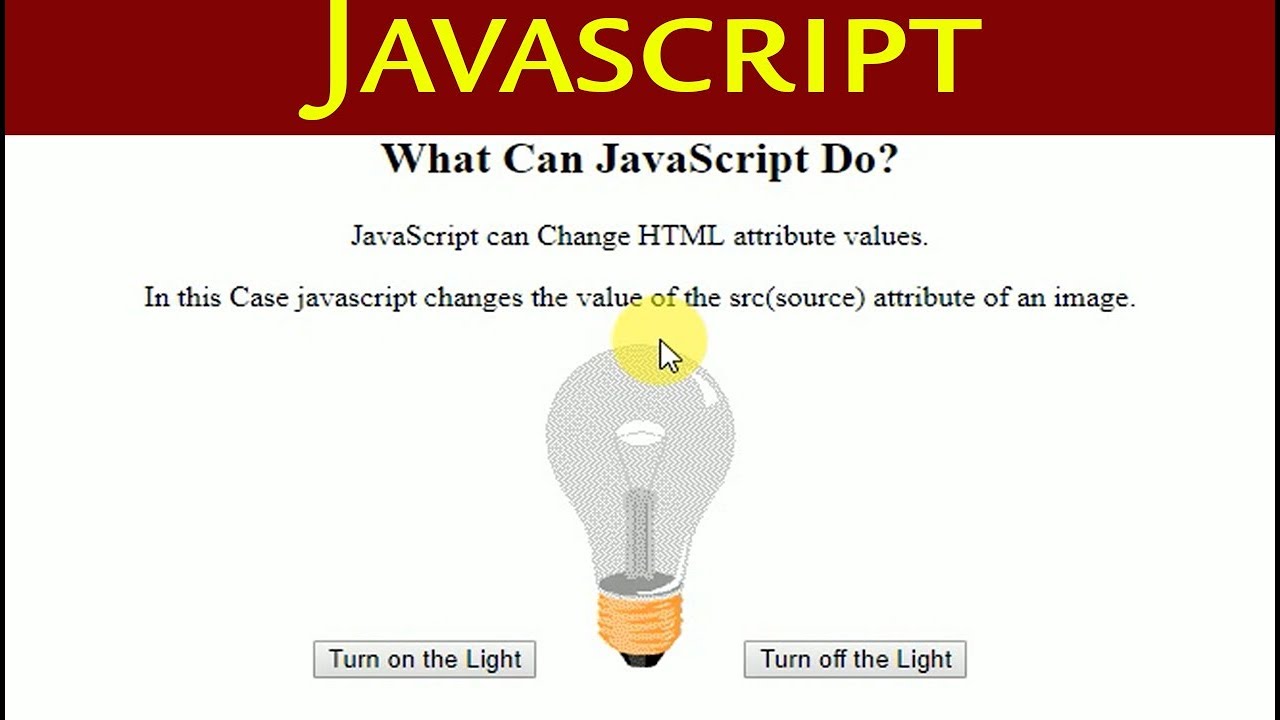
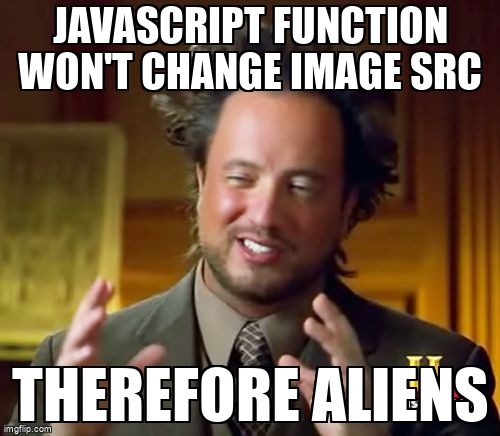
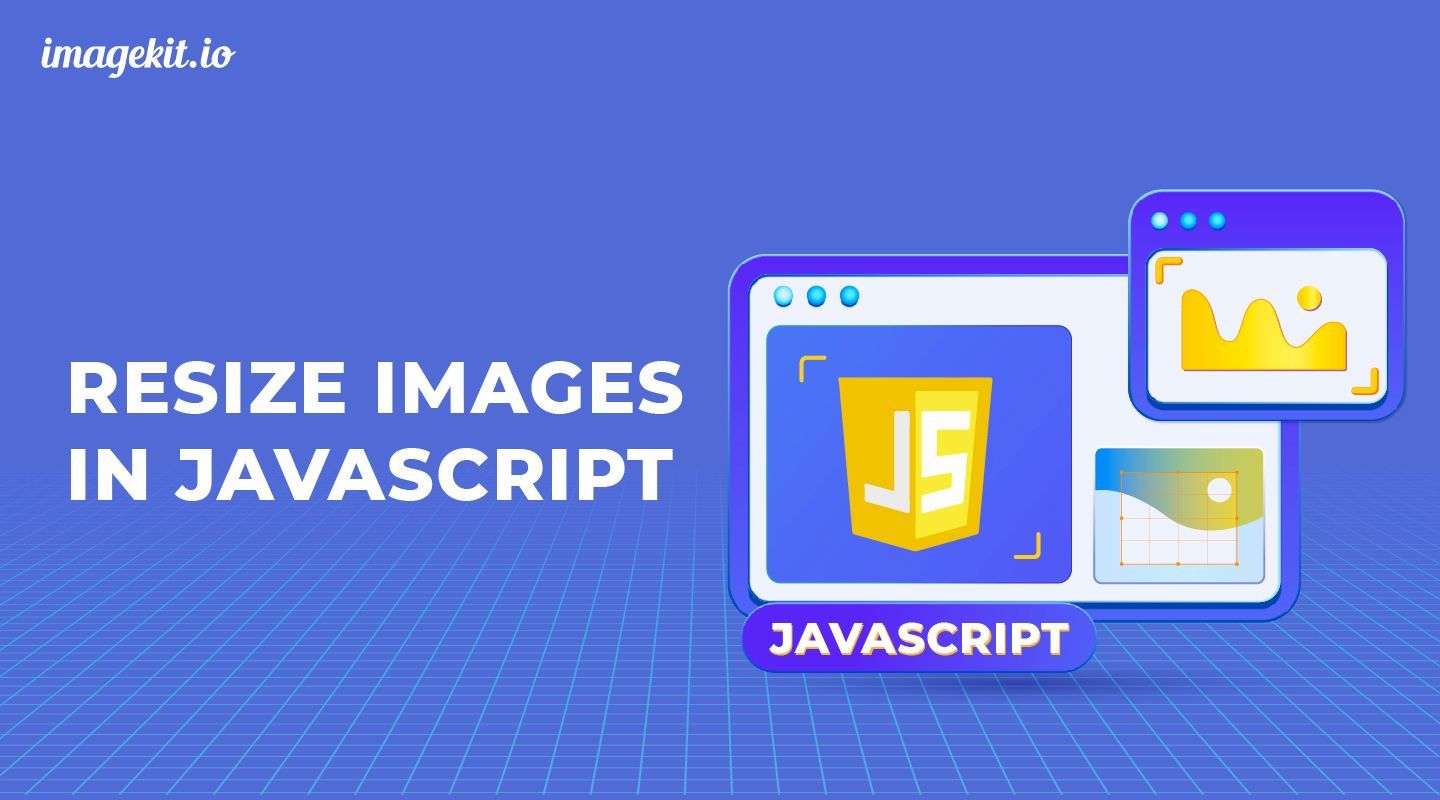

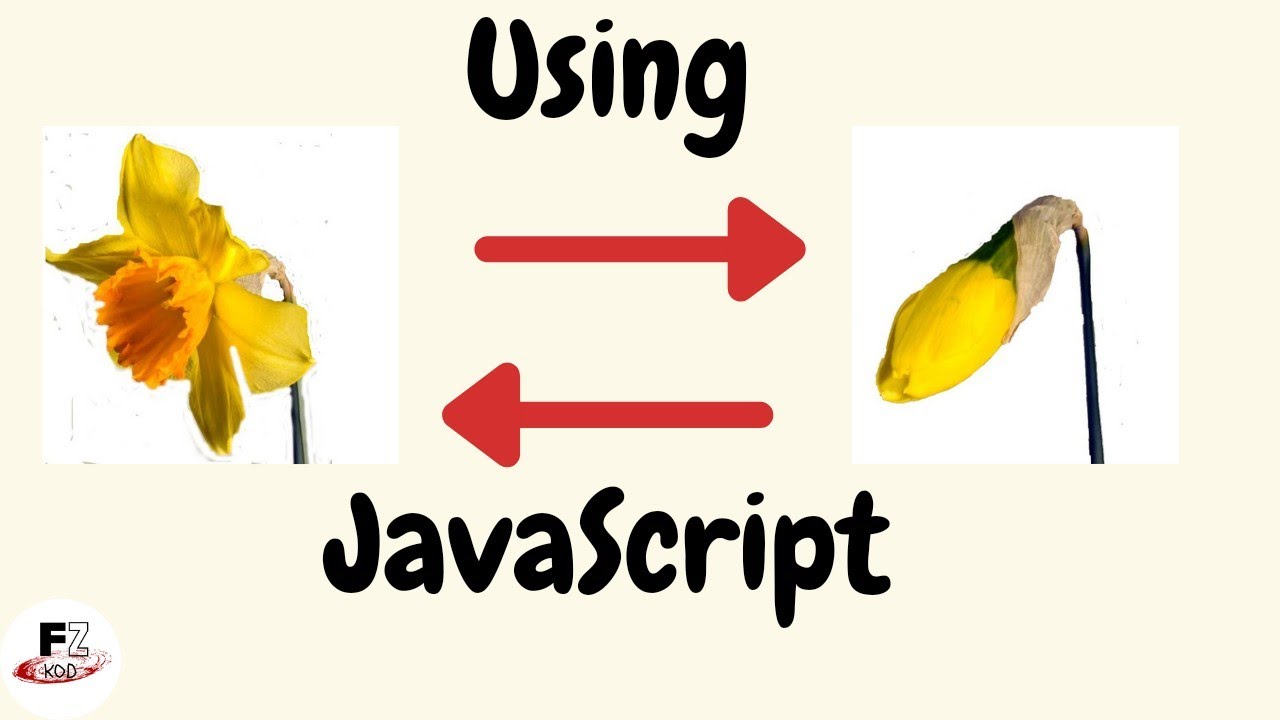




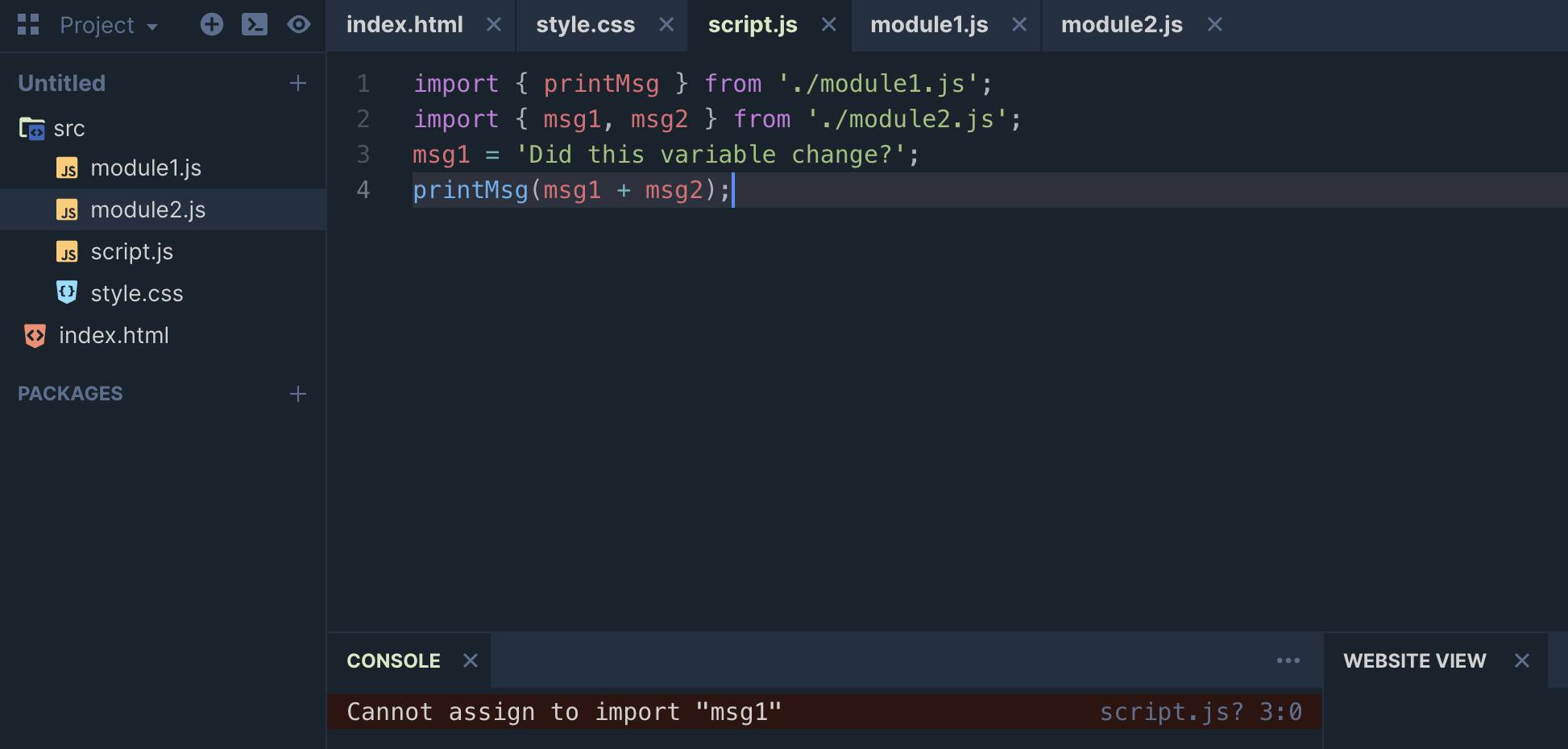
Article link: javascript change image src.
Learn more about the topic javascript change image src.
- How to Change Image Source JavaScript – Linux Hint
- HTML DOM Image src Property – W3Schools
- Programmatically change the src of an img tag – Stack Overflow
- Change Image Source JavaScript | Delft Stack
- How to change the src attribute of an img element in …
- JavaScript Set Image Src Dynamically – TalkersCode.com
- Changing of images in JavaScript – Tech Funda
- How to Change Img Src using JavaScript – Sabe.io
- How to change the src attribute of an img element in …
- How to Change Img Src using JavaScript – Sabe.io
- [JavaScript] – How to change the src in img after clicking
- Clear Img src attribute using JavaScript – bobbyhadz
- How do you change the src value of image using JavaScript?
See more: https://nhanvietluanvan.com/luat-hoc/