Java Generate Random String
In Java, there are multiple ways to generate random strings. Whether you need to generate a random alphanumeric string, a secure random string, or a random string based on a specific pattern, Java provides several options to cater to your requirements. In this article, we will explore various ways to generate random strings in Java, covering the java.util.Random class, java.util.UUID class, Apache Commons Lang library, and the SecureRandom class.
The java.util.Random Class:
One of the simplest ways to generate a random string in Java is by using the java.util.Random class. It provides methods to generate random numbers, which can be converted into characters or strings. However, the downside of using this class is that the generated strings will not necessarily contain all the characters of the alphabet, numbers, or special characters.
Using java.util.UUID Class:
The java.util.UUID class provides a convenient method named randomUUID() that generates a random universally unique identifier (UUID). Although the primary purpose of this class is to generate unique identifiers, you can extract a random string from the UUID. However, similar to the java.util.Random class, the generated strings may not have a specific pattern or character requirements.
Apache Commons Lang Library:
Apache Commons Lang is a popular library that provides various utility classes for common programming tasks. One of the classes, RandomStringUtils, offers multiple methods for generating random strings with different specifications. For example, you can generate random strings consisting of only alphabets, numbers, or both. Additionally, you can specify the length of the generated string, whether to include or exclude certain characters, and much more.
SecureRandom Class:
If you need to generate secure random strings for sensitive applications like password generation, you should use the java.security.SecureRandom class. This class provides a more secure way to generate random strings by using cryptographic algorithms. By default, the SecureRandom class uses the SHA1PRNG algorithm on most systems, which is considered secure. You can generate random strings of any desired length by using this class and specifying the set of characters allowed in the generated string.
Generating Random Alphanumeric Strings:
To generate random alphanumeric strings (consisting of both letters and numbers), you can combine letters from the alphabet (both uppercase and lowercase) and numbers. By using the Random class or the RandomStringUtils class from Apache Commons Lang, you can easily generate random alphanumeric strings of any length.
Generating Secure Random Strings:
For generating secure random strings, the SecureRandom class is the recommended approach. By using this class, you can ensure that the generated strings are highly random and suitable for sensitive applications. Secure random strings can be used for password generation, session IDs, encryption keys, and other security-related tasks.
Conclusion:
Generating random strings in Java can be achieved through various methods and libraries. When choosing the appropriate method, consider the requirements of your application and the security level needed for the generated strings. Whether you need a simple random string or a secure one, Java provides the necessary tools and libraries to fulfill your needs.
FAQs:
Q: How can I generate a random string in Java using RandomStringUtils?
A: To generate a random string using RandomStringUtils, you can use the random() method, specifying the length of the desired string and whether to include uppercase, lowercase, or numeric characters.
Q: How do I generate a random string in Java with lowercase letters only?
A: By using RandomStringUtils.random() method along with specifying the desired length and setting the allowed characters to lowercase alphabets only.
Q: Can I generate a random string with both letters and numbers in Java?
A: Yes, you can use either the Random class or the RandomStringUtils class from Apache Commons Lang to generate a random string consisting of both letters and numbers.
Q: How can I generate a secure random string for password generation?
A: To generate a secure random string for password generation in Java, you should use the SecureRandom class and specify the required set of characters allowed in the generated password.
Q: Can I generate a random string based on a specific pattern or regular expression in Java?
A: Yes, you can use the Pattern and Matcher classes from the java.util.regex package to generate a random string based on a specific pattern or regular expression. By combining it with other methods like Random or RandomStringUtils, you can achieve this functionality.
Q: What is the difference between a random string and a secure random string?
A: A random string is generated using basic random number generation techniques and may not fulfill specific requirements such as character patterns or security. On the other hand, a secure random string is generated using cryptographic algorithms and is suitable for security-sensitive tasks like password generation or encryption.
Java Program That Generate Random String
Keywords searched by users: java generate random string RandomStringUtils, java random string a-z, Generate random string number Java, SecureRandom generate random string, Generate random String from Regex Java, Random string, Secure random string, Random password Java
Categories: Top 51 Java Generate Random String
See more here: nhanvietluanvan.com
Randomstringutils
With its extensive feature set and ease of use, RandomStringUtils has become a go-to choice for many developers. In this article, we will dive deep into the features of RandomStringUtils and explore how it can be utilized effectively in various scenarios.
Features of RandomStringUtils:
1. Random String Generation: RandomStringUtils allows developers to generate random strings of any length. This can be accomplished by using the `random(int count)` method, where `count` represents the length of the generated string. For example, to generate a 10-character random string, you can use the following code snippet:
“`
String randomString = RandomStringUtils.random(10);
“`
2. Alphanumeric String Generation: Another common use case is generating random alphanumeric strings, which consist of a combination of uppercase and lowercase letters along with numbers. RandomStringUtils provides the `randomAlphanumeric(int count)` method to generate such strings. For instance:
“`
String alphanumericString = RandomStringUtils.randomAlphanumeric(8);
“`
This will generate an 8-character alphanumeric string like “bF07aR5C”.
3. Random ASCII String Generation: If you require random strings consisting of ASCII characters only, including symbols and special characters, the `randomAscii(int count)` method is your solution. Here’s an example of generating a 6-character random ASCII string:
“`
String asciiString = RandomStringUtils.randomAscii(6);
“`
This may produce a result like “-5J9$@”.
4. Random Numeric String Generation: If you need to generate random strings containing only numeric characters, RandomStringUtils provides the `randomNumeric(int count)` method. Here’s an example:
“`
String numericString = RandomStringUtils.randomNumeric(5);
“`
This will generate a 5-digit numeric string like “78479”.
5. Random String with Specific Characters: RandomStringUtils enables you to generate random strings that include specific characters of your choice. The `random(int count, String chars)` method allows you to pass a set of characters as a String parameter. For instance, to generate a 6-character random string using only the characters “ABC123”, you can use the following code snippet:
“`
String specificCharsString = RandomStringUtils.random(6, “ABC123”);
“`
The result might be “B12A21”.
6. Repeatable Random Strings: RandomStringUtils also supports generating repeatable random strings. By using a seed value in conjunction with the other methods, you can ensure that the generated strings are the same for a specific seed. This feature can be useful when you need consistency in testing or any other repeatable scenario. Consider the following example:
“`
RandomStringUtils.random(10, 0, 0, true, true, null, new Random(12345L));
“`
This will generate the same output (“D16c8pHHaw”) every time, as long as the seed remains the same.
FAQs:
Q: Can RandomStringUtils generate cryptographically secure random strings?
A: No, RandomStringUtils does not provide cryptographic security. If you require secure random strings, it is recommended to use the `SecureRandom` class instead.
Q: How efficient is RandomStringUtils when generating long random strings?
A: RandomStringUtils is highly efficient in generating random strings of any length, including long strings. It utilizes optimized algorithms for memory and processing efficiency.
Q: Can I generate multiple random strings with the same set of characters?
A: Yes, you can generate multiple random strings with the same set of characters by using the same method call multiple times, or by utilizing a loop.
Q: How can I control the distribution of characters in the generated random strings?
A: RandomStringUtils does not provide explicit control over the distribution of characters. It generates random strings based on the provided parameters and their length. If you require a specific distribution, you can manipulate the generated strings programmatically.
Conclusion:
RandomStringUtils is a powerful utility class within the Apache Commons Lang library that simplifies the generation of random strings. Its various methods cater to different requirements, enabling developers to generate alphanumeric, ASCII, numeric, and customizable character strings efficiently. The repeatable feature further enhances its utility in repeatable scenarios. However, for cryptographically secure random strings, developers should resort to SecureRandom. With its ease of use and extensive functionality, RandomStringUtils is an essential tool for any developer needing to generate random strings.
Java Random String A-Z
To generate random strings in Java, we can make use of the `java.util.Random` class, which provides various methods for generating random values. To obtain strings from a-z, we need to generate random numbers between 97 and 122, which correspond to the ASCII values of lowercase ‘a’ and ‘z’, respectively. Then, we can convert these numbers to characters using the `char` data type. Let’s take a look at a simple code snippet to generate a random string of length n:
“`java
import java.util.Random;
public class RandomStringGenerator {
public static String generateRandomString(int length) {
Random random = new Random();
StringBuilder stringBuilder = new StringBuilder(length);
for (int i = 0; i < length; i++) { int randomNumber = random.nextInt(26) + 97; char randomChar = (char) randomNumber; stringBuilder.append(randomChar); } return stringBuilder.toString(); } public static void main(String[] args) { int length = 10; String randomString = generateRandomString(length); System.out.println(randomString); } } ``` In the `generateRandomString` method, we create an instance of `Random` class and a `StringBuilder` to build our random string. Inside the loop, we generate a random number between 97 and 122 using `nextInt` method of `Random` class. We then convert this random number to a character and append it to the `StringBuilder` using the `append` method. Finally, we return the generated random string by converting the `StringBuilder` to a `String` using the `toString` method. By executing the `main` method, we obtain a random string of length 10, consisting solely of alphabetic characters (a-z). But what if we want to include uppercase characters as well? We can achieve this by randomly selecting the case of each character. Here's an enhanced version of our code snippet that generates random strings containing both lowercase and uppercase alphabetic characters: ```java import java.util.Random; public class RandomStringGenerator { public static String generateRandomString(int length) { Random random = new Random(); StringBuilder stringBuilder = new StringBuilder(length); for (int i = 0; i < length; i++) { int randomNumber = random.nextInt(26) + 65; // 65-90 for uppercase int randomCase = random.nextInt(2); // 0 for lowercase, 1 for uppercase if (randomCase == 0) randomNumber += 32; // Add 32 to convert to lowercase char randomChar = (char) randomNumber; stringBuilder.append(randomChar); } return stringBuilder.toString(); } public static void main(String[] args) { int length = 10; String randomString = generateRandomString(length); System.out.println(randomString); } } ``` In this updated version, we generate the random number between 65 and 90 to cover both lowercase and uppercase ASCII values. Additionally, we introduce a random case variable (0 or 1) and if the case value is 0, we convert the random character to lowercase by adding 32 to its ASCII value. Now that we have seen the implementation, let's address some frequently asked questions about generating random strings in Java: FAQs Q1. Can I generate random strings of specific lengths? A. Yes, you can generate random strings of any desired length by modifying the `length` parameter in the `generateRandomString` method. Q2. Can I include numbers or special characters in the generated random string? A. The code snippets provided in this article focus solely on generating random strings containing alphabetic characters (a-z). To include numbers or special characters, you can extend the list of valid ASCII values and expand your character set accordingly. Q3. How can I increase the randomness of generated strings? A. Java's `Random` class uses a seed value to generate random numbers. By default, the seed is based on the current time. To achieve better randomness, you can use a more random seed value, like `System.nanoTime()`, when initializing the `Random` class. Q4. Is it possible to generate secure random strings for cryptographic purposes? A. While the techniques discussed in this article are suitable for most general use cases, if you require randomness for cryptographic purposes, it is recommended to use Java's `SecureRandom` class. This class provides stronger random number generation algorithms to ensure the security of your applications. Q5. Can I generate random strings with a predefined pattern? A. Yes, you can generate random strings with specific patterns by defining custom rules in code. For example, you can create rules to enforce a certain number of uppercase letters, numbers, or special characters. In conclusion, generating random strings in Java is a straightforward process using the `Random` class. By manipulating the ASCII values and employing simple techniques, we can generate random strings consisting of alphabetic characters (a-z) or extend the character set to include uppercase letters, numbers, or special characters. Remember to adjust the code according to your specific requirements. Happy coding!
Generate Random String Number Java
Randomness is a crucial element in a wide range of programming applications. Whether you are working on password generation, token generation, or any other situation that requires uniqueness, generating random string numbers is a common and useful task. In this article, we will delve into the world of generating random string numbers in Java, exploring different techniques and their implementation.
Java provides several methods and libraries to generate random numbers. However, generating random string numbers requires additional steps. The most common approach is to generate random numbers and convert them into strings. Let’s examine an example of generating a random four-digit number.
“`java
import java.util.Random;
public class RandomNumberGenerator {
public static void main(String[] args) {
Random rand = new Random();
int number = rand.nextInt(10000); // Generate random number between 0 and 9999
String randomNumber = String.format(“%04d”, number); // Convert number to four-digit string
System.out.println(“Random Number: ” + randomNumber);
}
}
“`
In this example, we create an instance of the `Random` class from the `java.util` package. We then use the `nextInt` method to generate a random number in the range of 0 to 9999. Next, we format the number using `String.format` to ensure that it is always four digits long, even if leading zeros are required. Finally, we print the generated random number as a string.
Though this method works fine for generating random string numbers, it is not suitable for situations where the length of the string needs to be variable. To address this, we can utilize the `UUID` class in Java to generate universally unique identifiers (UUIDs) as random string numbers. Let’s examine how we can achieve this.
“`java
import java.util.UUID;
public class UUIDGenerator {
public static void main(String[] args) {
String randomString = UUID.randomUUID().toString();
System.out.println(“Random String: ” + randomString);
}
}
“`
In this example, we use the `randomUUID` method from the `UUID` class to generate a random UUID. The generated UUID is then converted to a string using the `toString` method. This method guarantees a unique string value each time it is called. However, the length of the generated string is fixed at 36 characters.
What if you need a random string number with a specific length and a custom character set? Java provides the `SecureRandom` class to generate cryptographically strong random numbers. By combining this with some utility methods, we can generate flexible random string numbers. Let’s take a look at a code snippet.
“`java
import java.security.SecureRandom;
public class RandomStringGenerator {
public static void main(String[] args) {
int length = 8;
String characters = “ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789”;
String randomString = generateRandomString(length, characters);
System.out.println(“Random String: ” + randomString);
}
public static String generateRandomString(int length, String characters) {
SecureRandom rand = new SecureRandom();
StringBuilder sb = new StringBuilder(length);
for (int i = 0; i < length; i++) { int randomIndex = rand.nextInt(characters.length()); char randomChar = characters.charAt(randomIndex); sb.append(randomChar); } return sb.toString(); } } ``` In this example, we define the desired length of the random string and a character set from which the random string will be generated. The `generateRandomString` method takes in these parameters and returns a random string of the specified length. It uses the `SecureRandom` class to generate random indices for selecting characters from the character set. Now let's address some common questions regarding generating random string numbers in Java. **FAQs:** 1. **Q: Can I generate a random string number with a specific pattern?** A: Yes, you can. Java provides the `RandomStringUtils` class in the Apache Commons Lang library, which allows you to generate random strings with customizable patterns. 2. **Q: How can I generate a random string number with a specific length and a mixture of uppercase, lowercase, and numeric characters?** A: You can use the `generateRandomString` method we discussed earlier and provide a character set that includes the desired characters. 3. **Q: Is the `Random` class suitable for generating secure random numbers?** A: No, the `Random` class is not suitable for generating cryptographically strong random numbers. For secure applications, it is recommended to use the `SecureRandom` class as shown in the last example. 4. **Q: Can I generate random alphanumeric strings of varying lengths within a specific range?** A: Yes, you can modify the `generateRandomString` method to accept a minimum and maximum length, and use `rand.nextInt(maxLength - minLength + 1) + minLength` to generate a random length within the desired range. Generating random string numbers in Java is a fundamental task in many applications. By exploring the various techniques and methods available, you can create flexible and secure solutions tailored to your specific needs.
Images related to the topic java generate random string
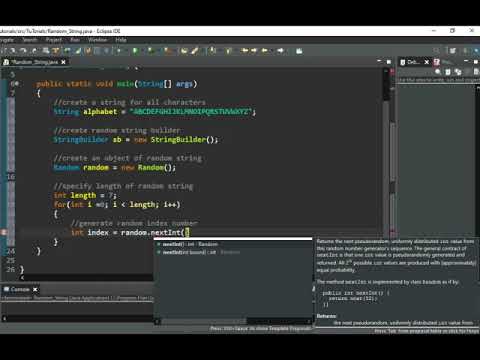
Found 21 images related to java generate random string theme
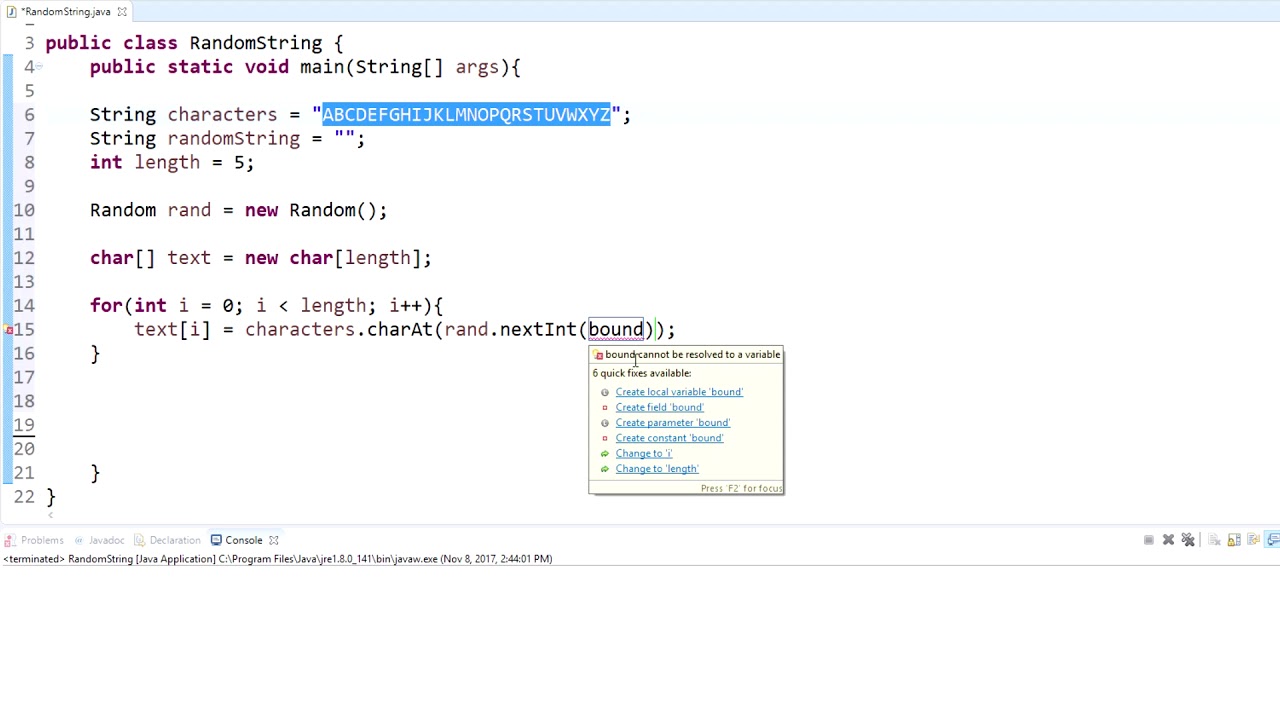
![Speed Programming: Random String Generator [Java] - YouTube Speed Programming: Random String Generator [Java] - Youtube](https://i.ytimg.com/vi/J9uE101hLsg/hqdefault.jpg)
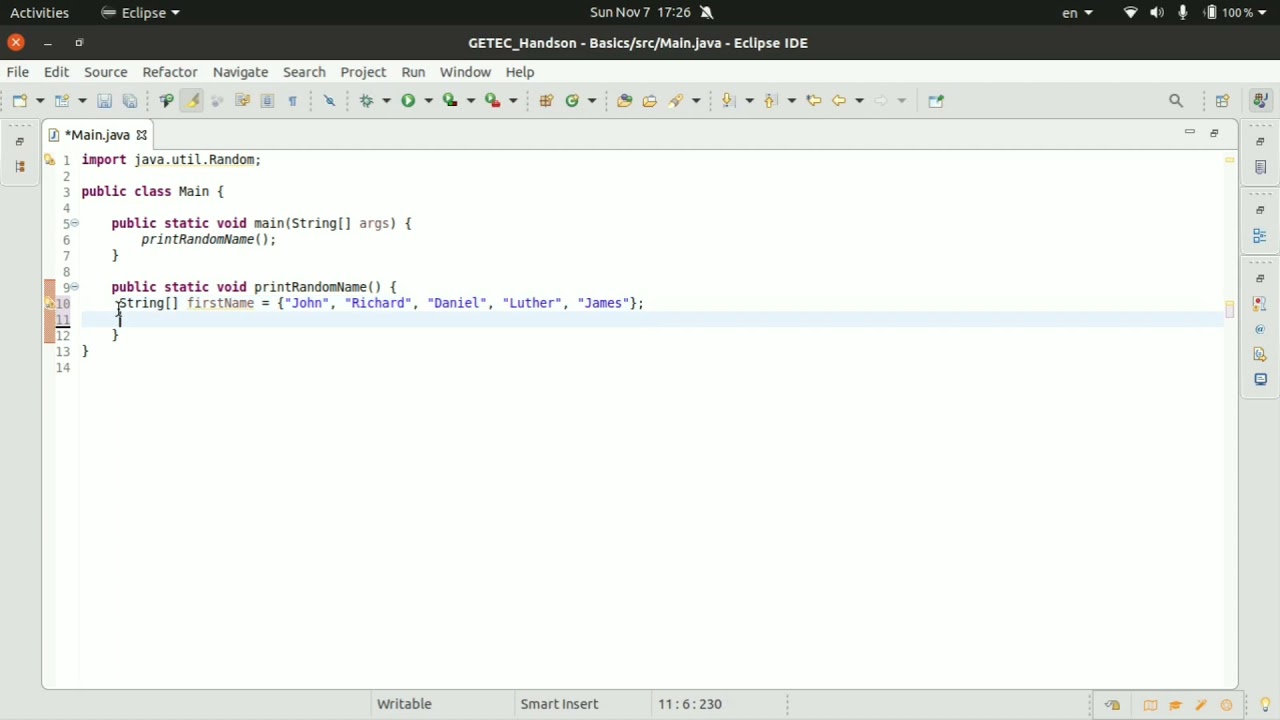
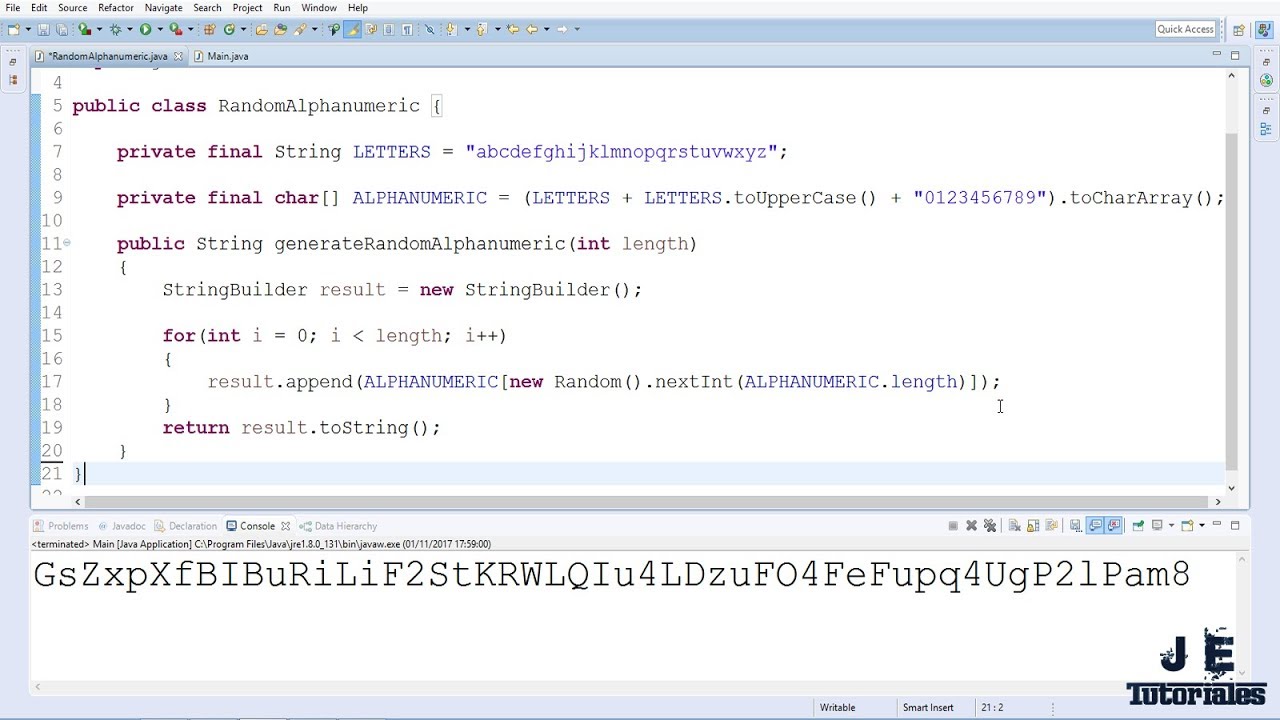
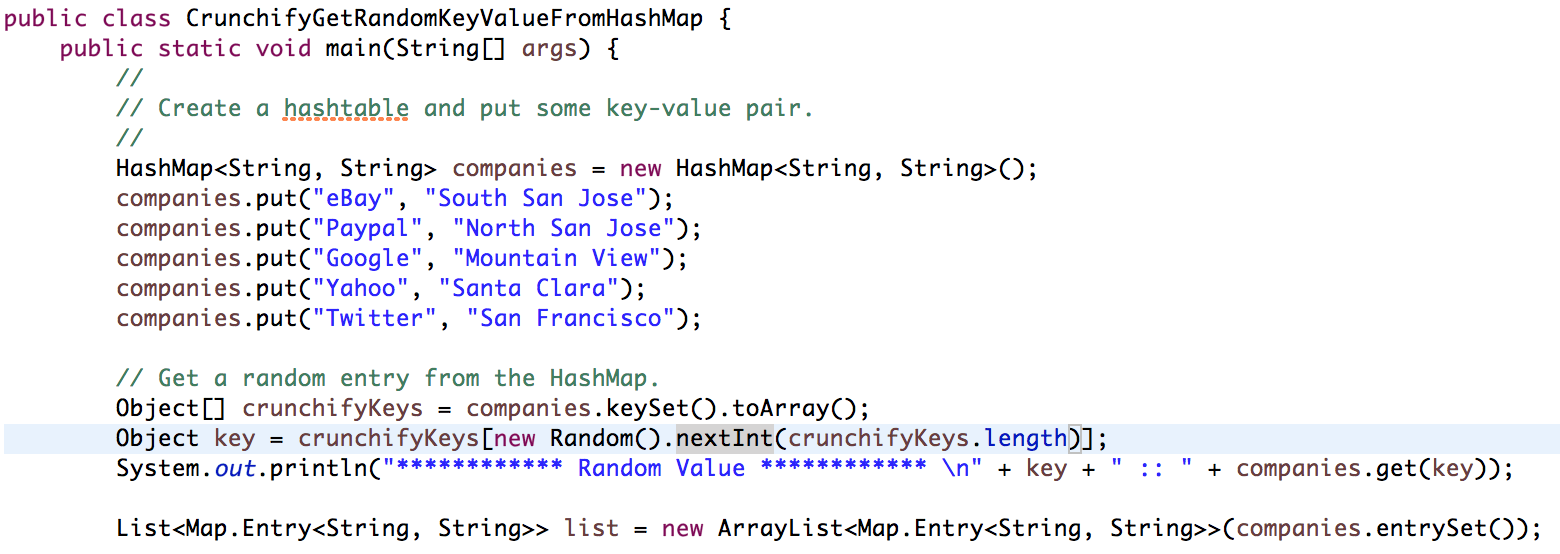
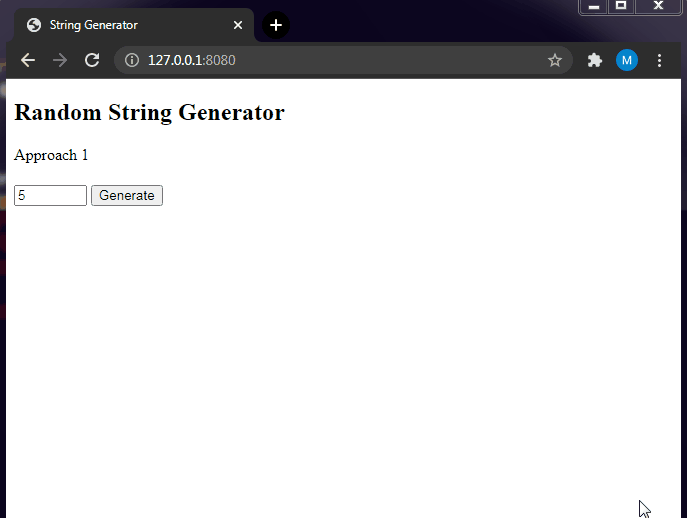
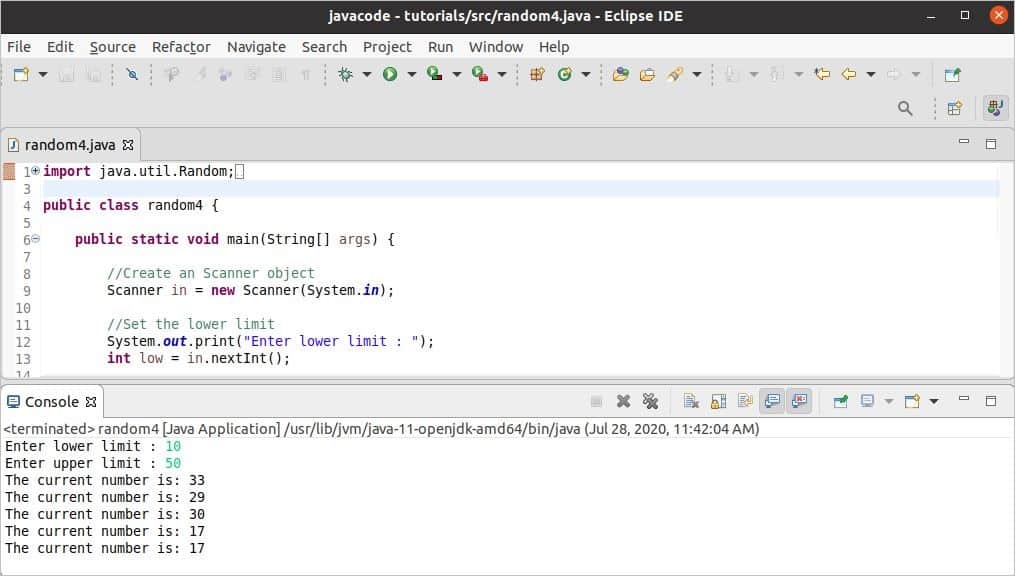
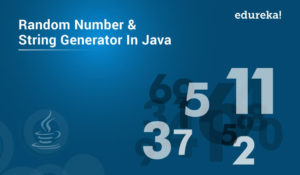
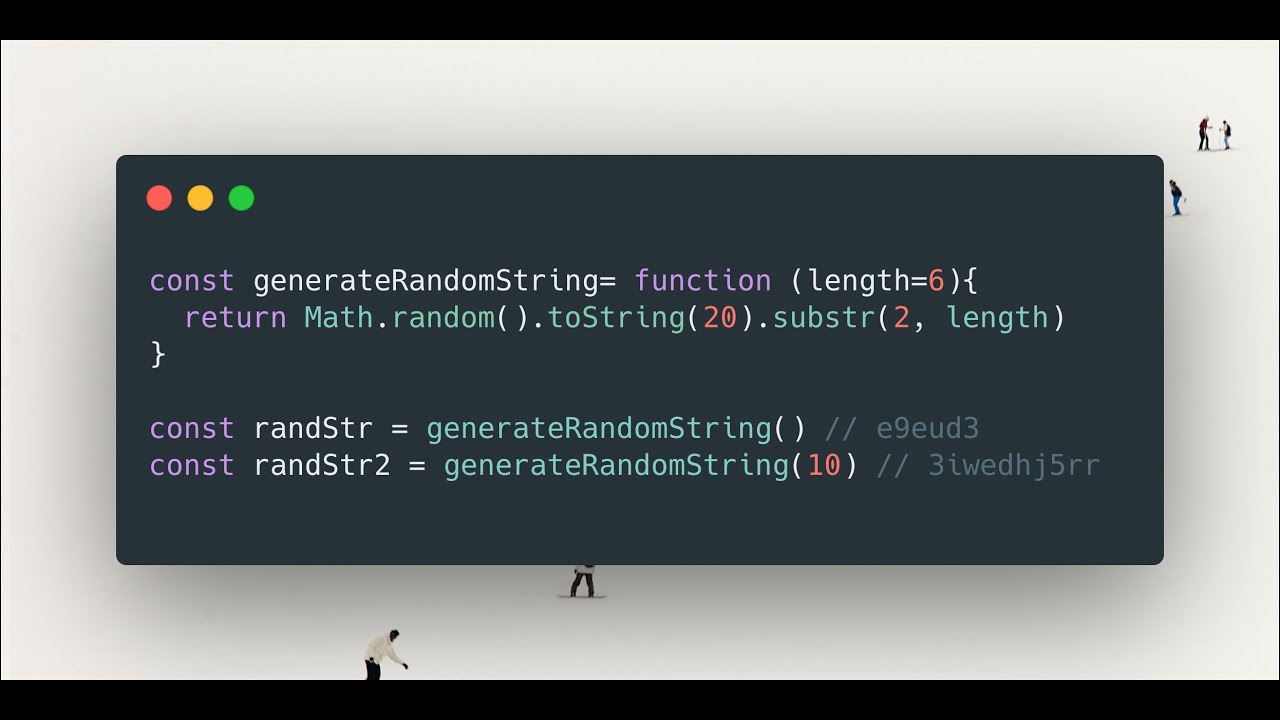
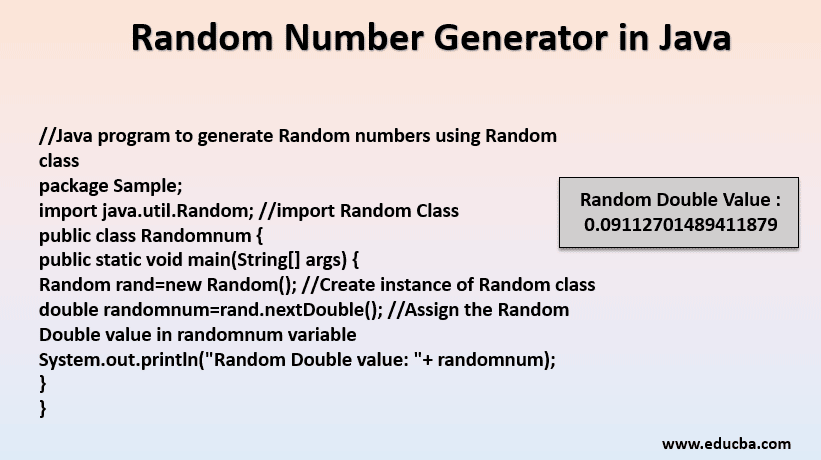


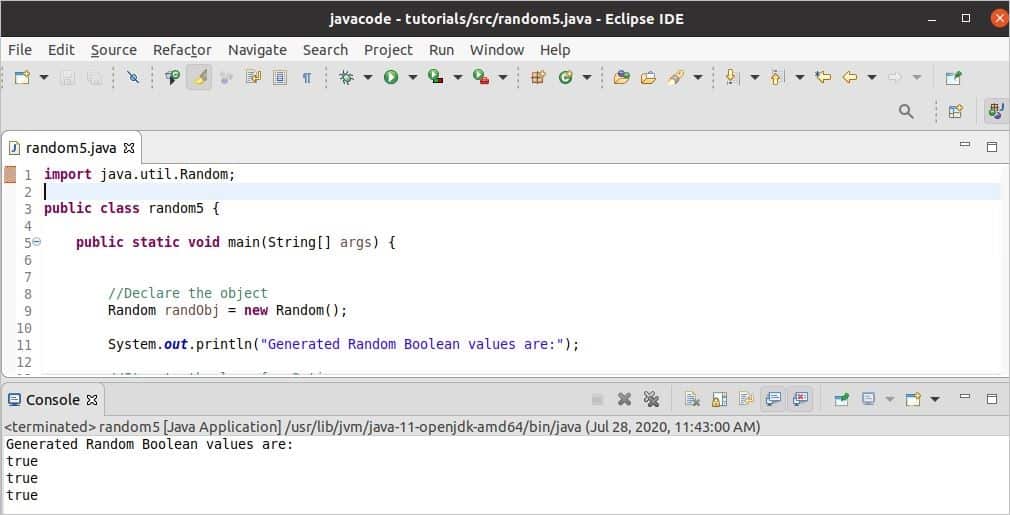


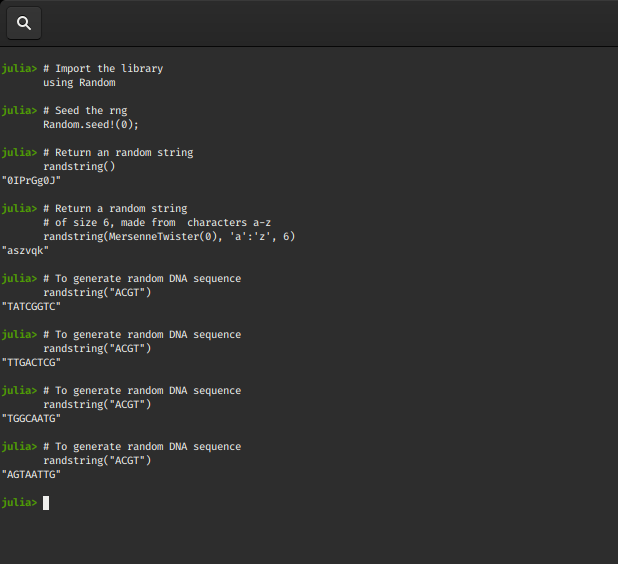
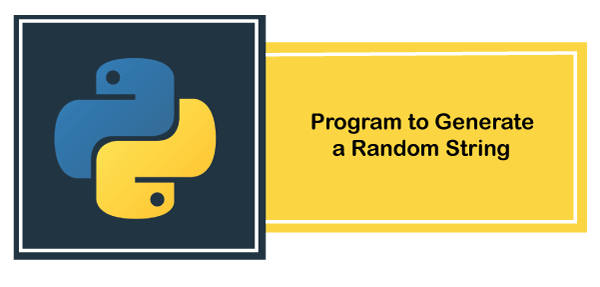
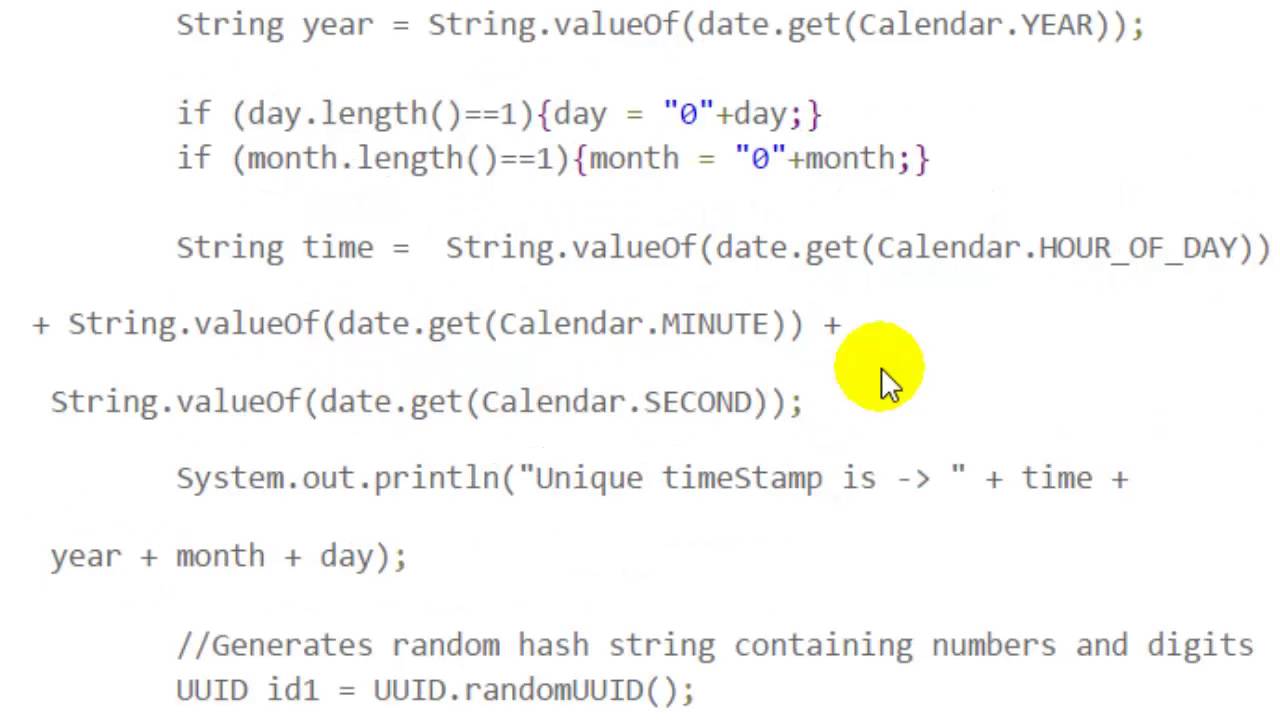

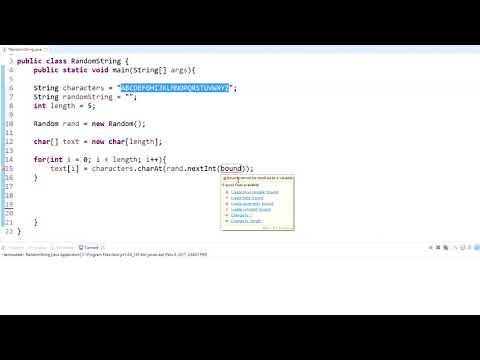
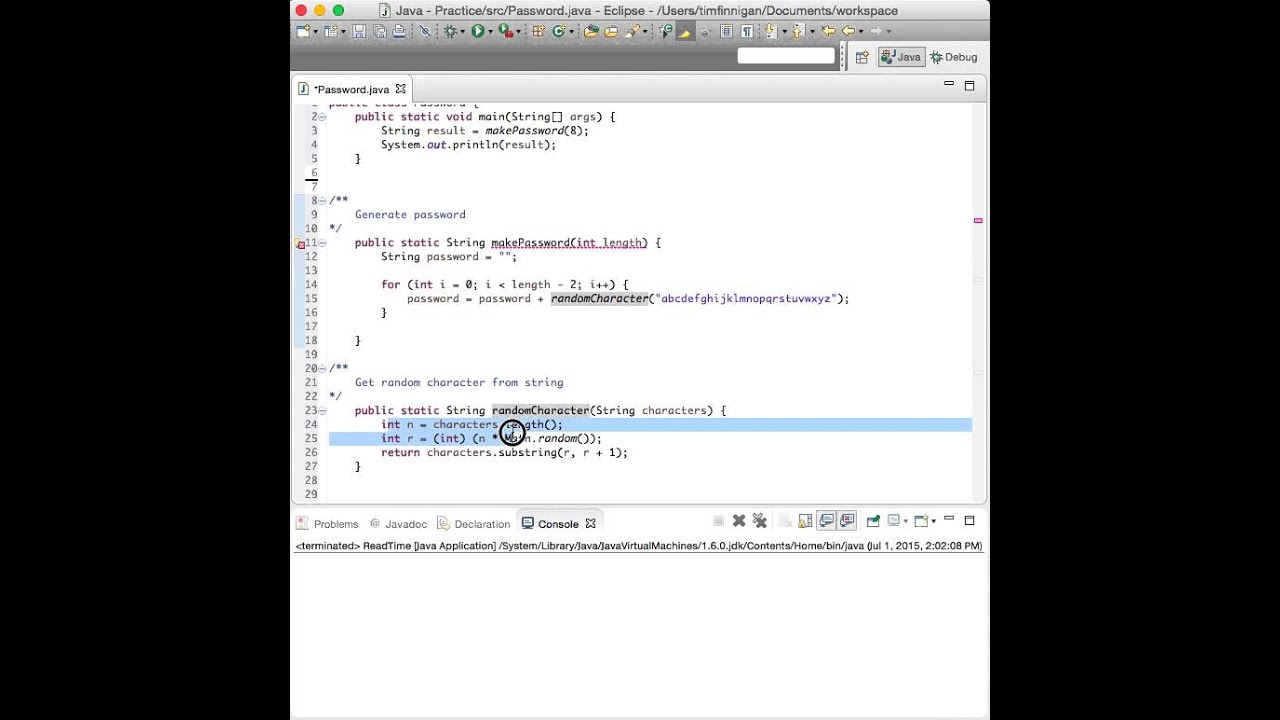
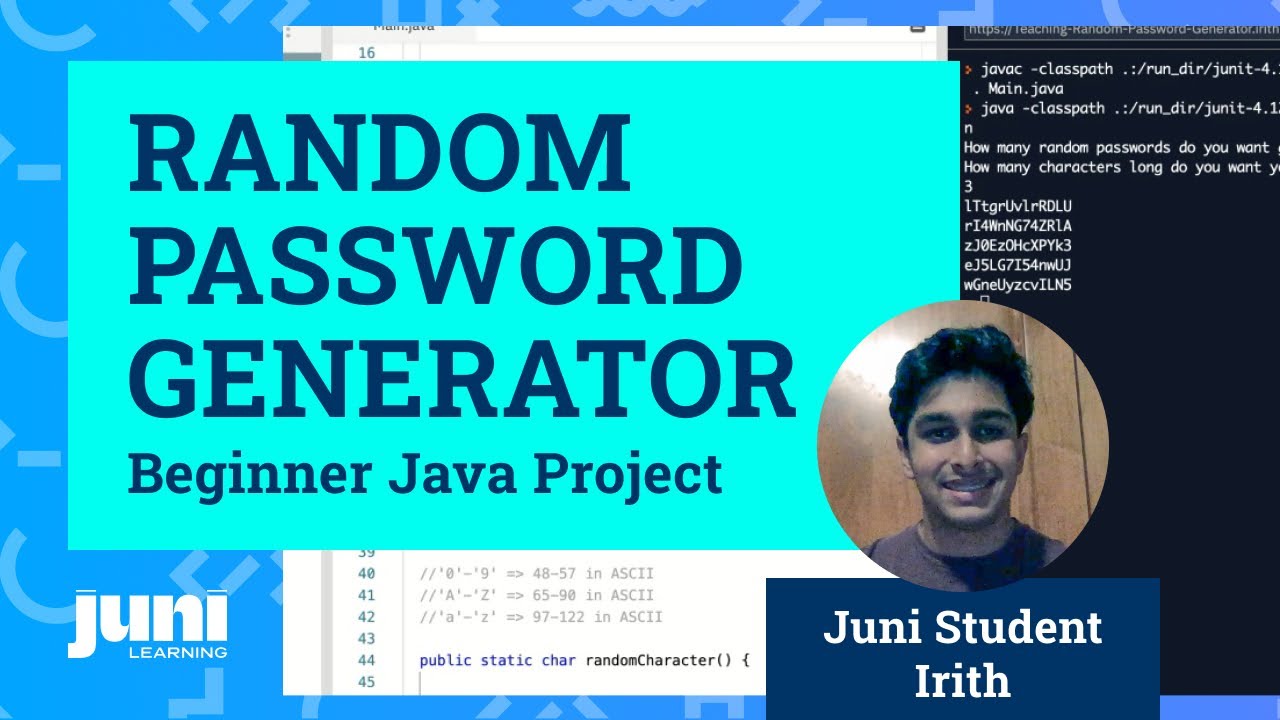



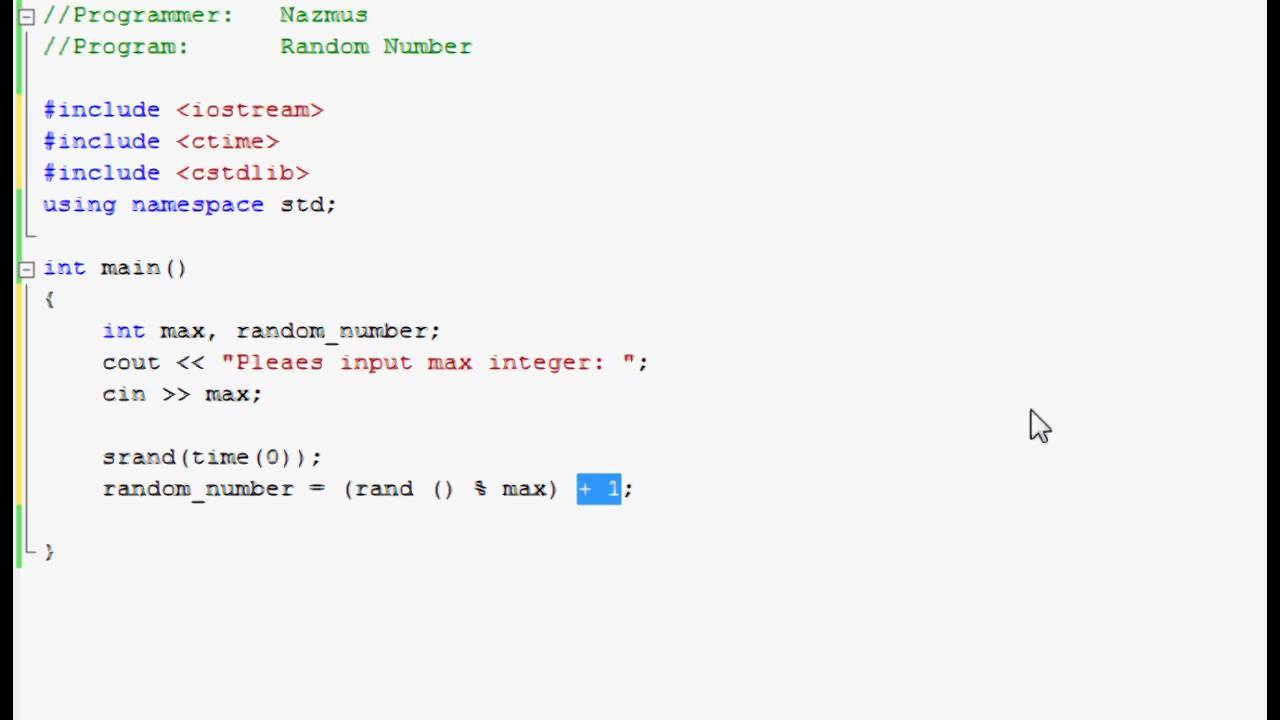
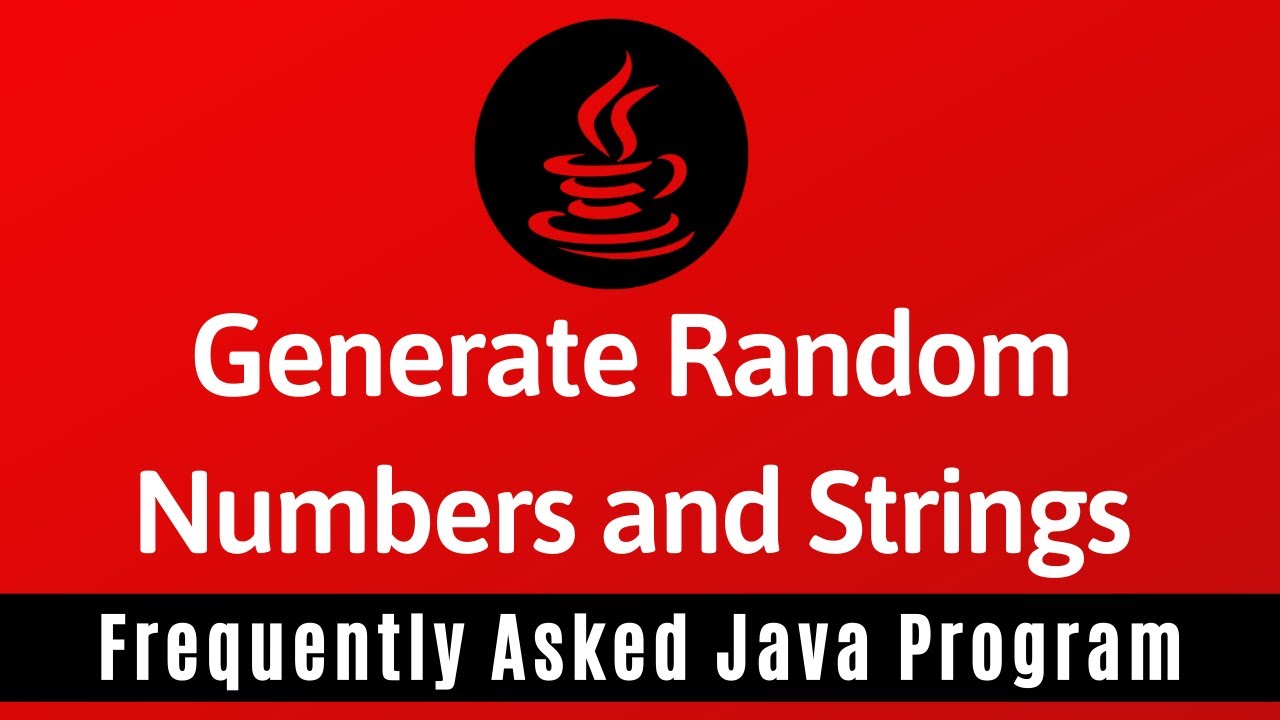
![Golang generate random string Examples [SOLVED] | GoLinuxCloud Golang Generate Random String Examples [Solved] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/golang_gen_random_string.jpg)
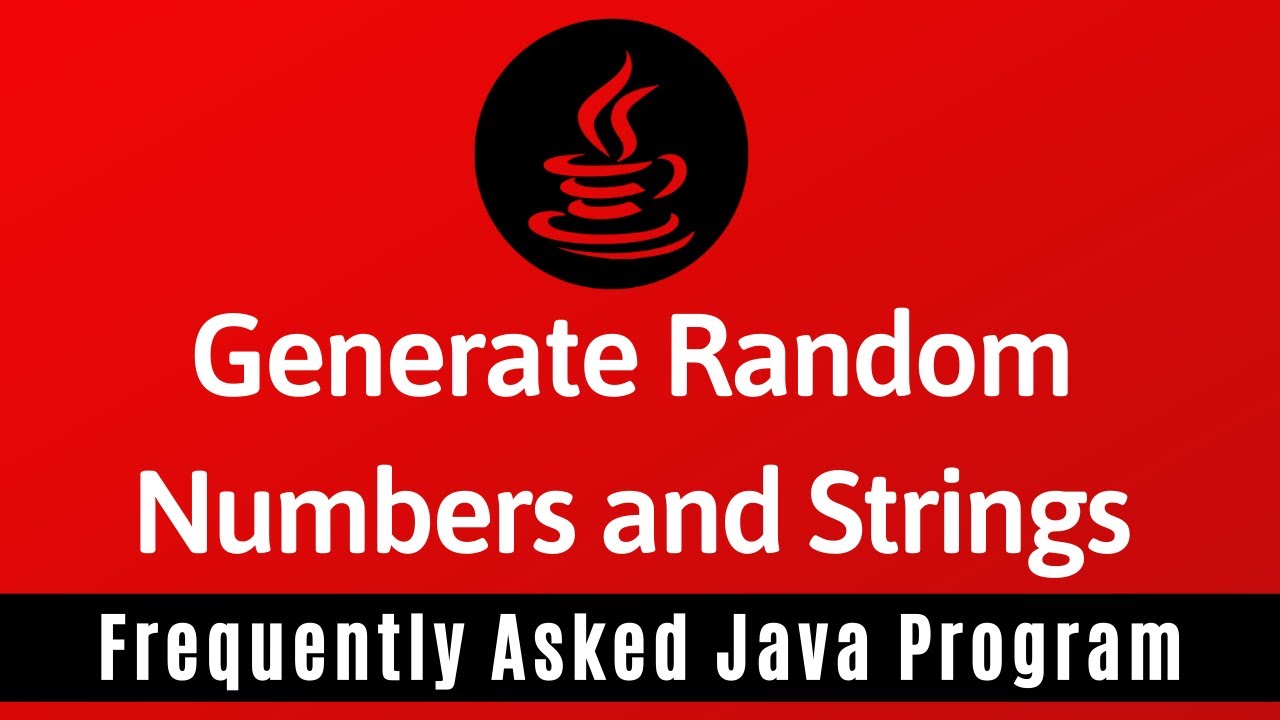
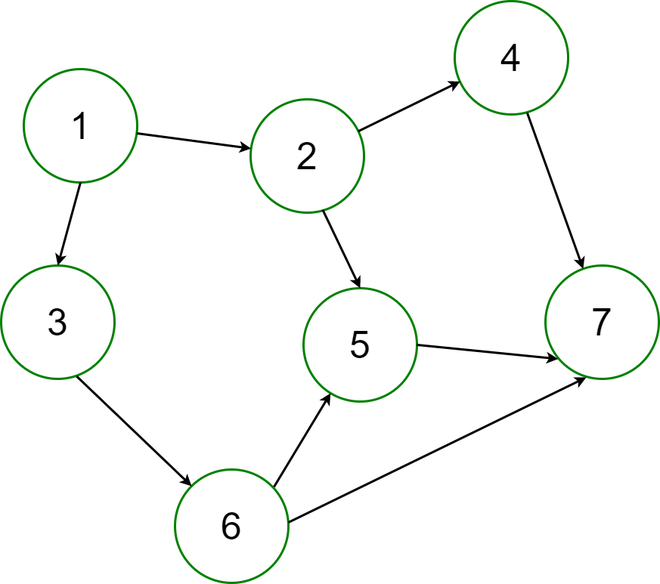
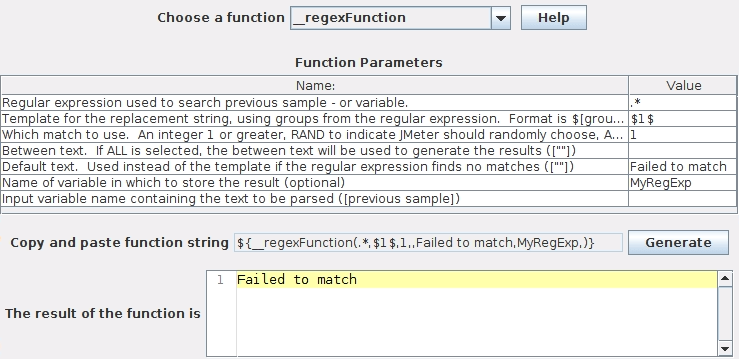
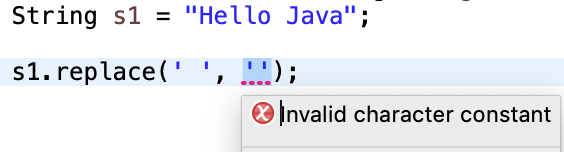
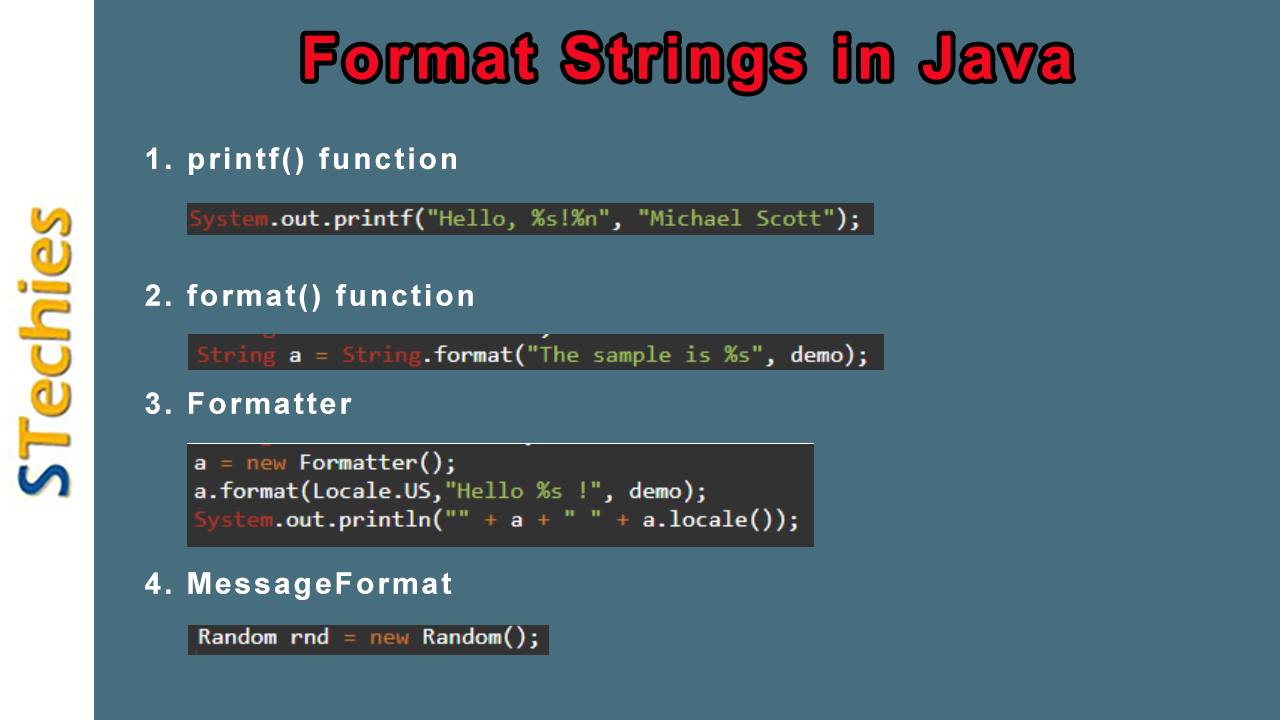
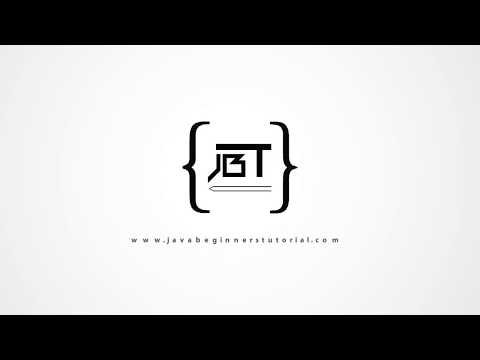
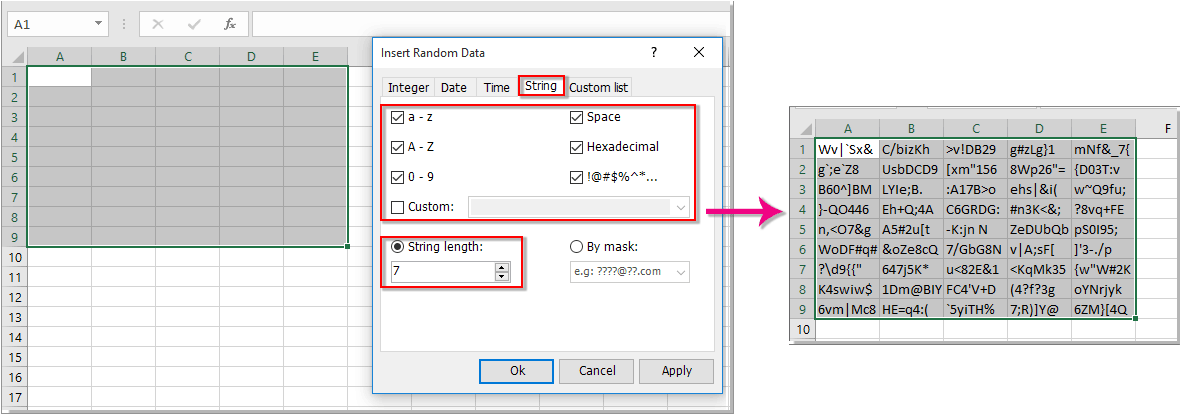

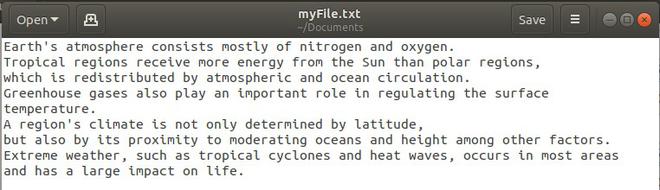




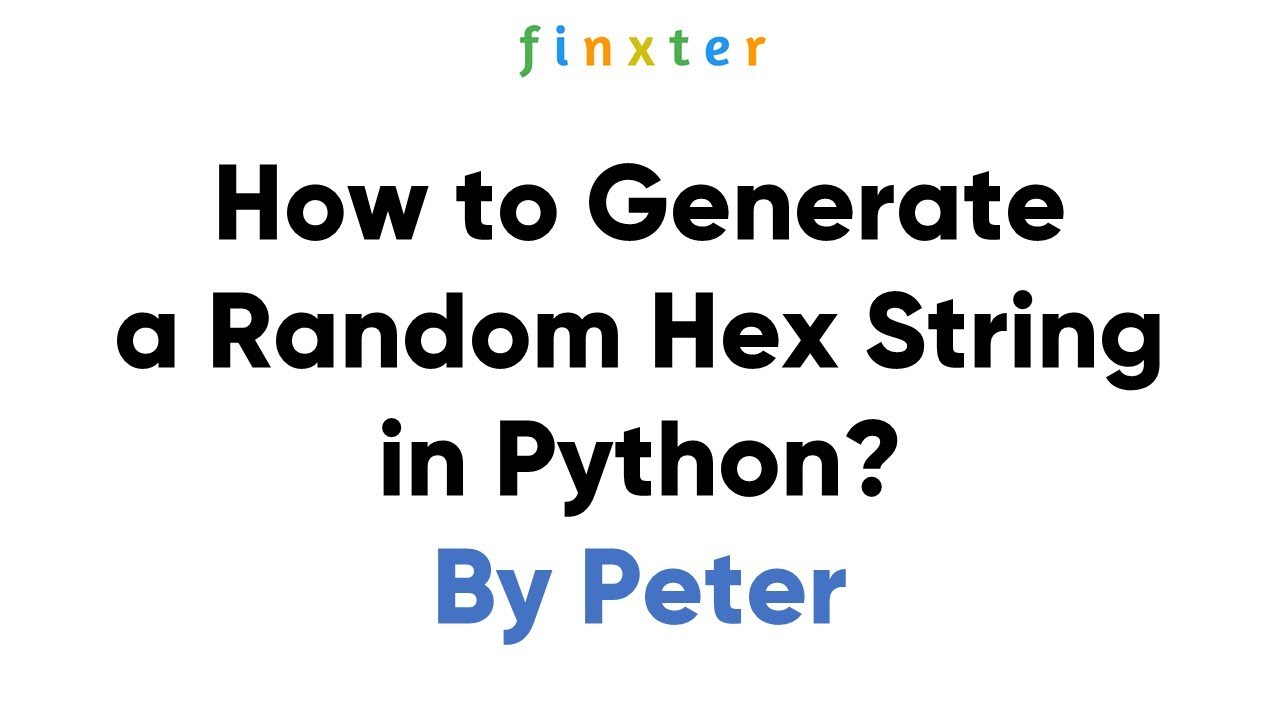
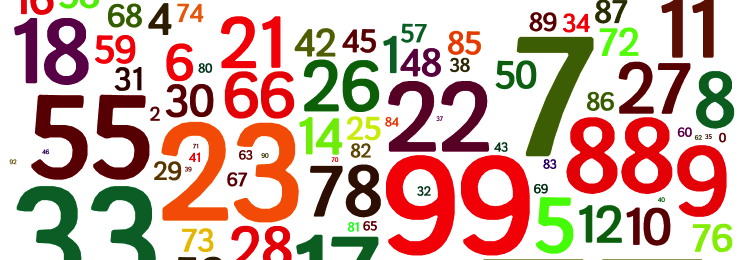
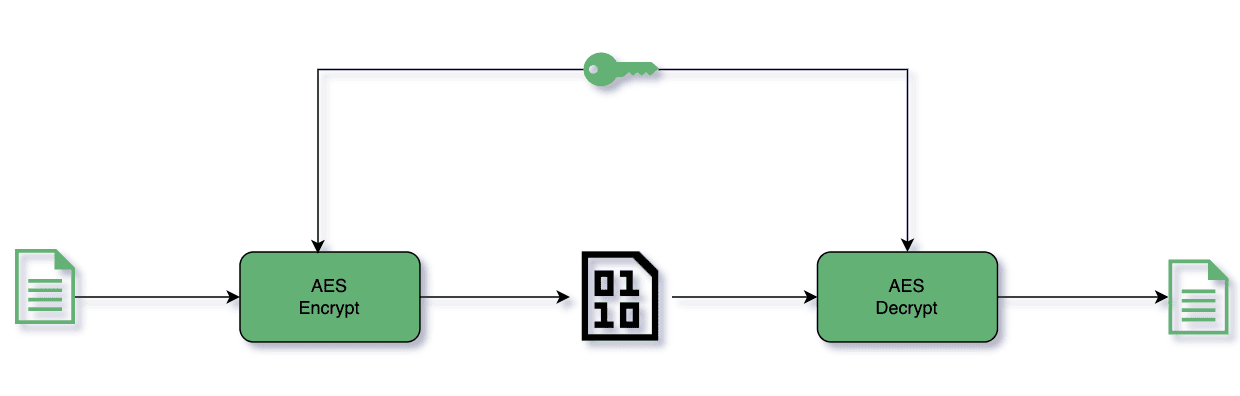
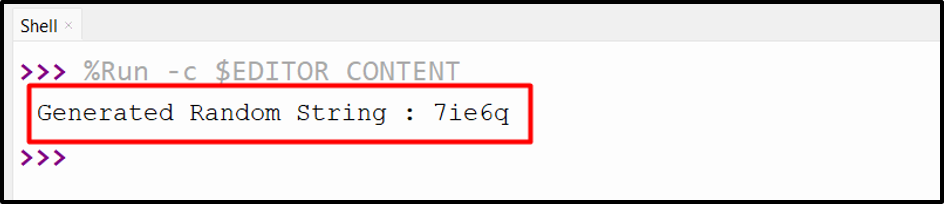
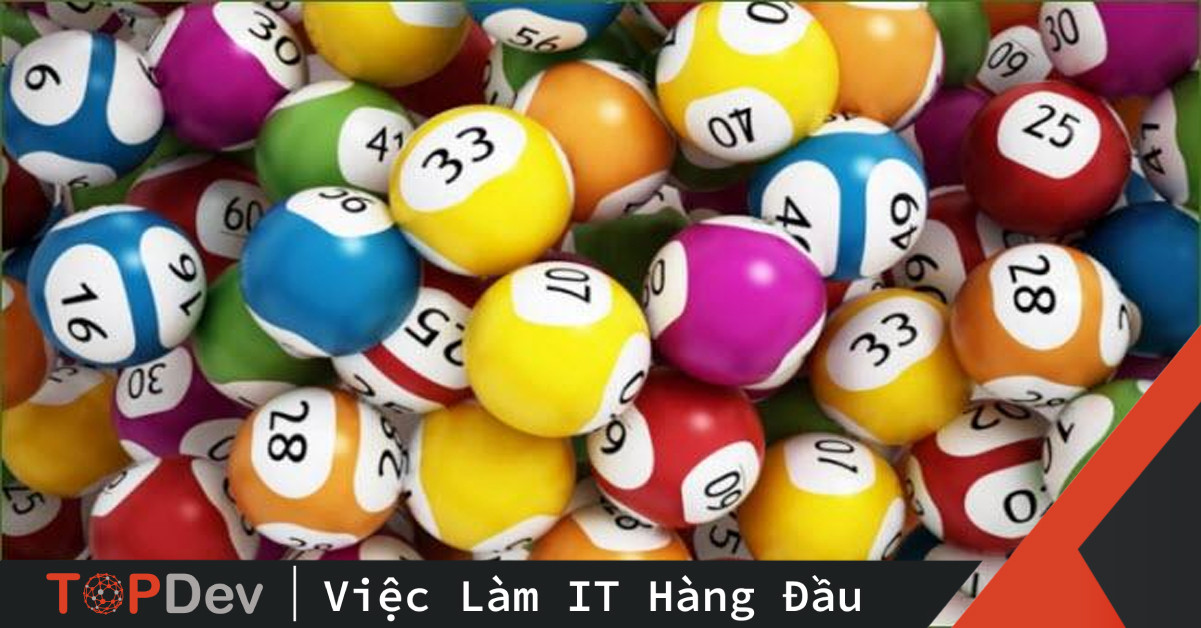


Article link: java generate random string.
Learn more about the topic java generate random string.
- Java – Generate Random String | Baeldung
- Generate random String of given size in Java – GeeksforGeeks
- How to generate a random alpha-numeric string – java
- Java Program to Create random strings – Programiz
- Easiest Ways To Generate A Random String In Java – Xperti
- Generate Random Strings in Java Examples – CodeJava.net
- Tạo số và chuỗi ngẫu nhiên trong Java – GP Coder
- 7 Ways to Create Random String in Java | BeingCoders
- Generate Random String in Java
- Generate Random String in Java – Javacodepoint
See more: https://nhanvietluanvan.com/luat-hoc/