Isnull Or Empty C#
In C#, it is common to come across situations where you need to check whether a value is null or empty. Dealing with null or empty values requires careful handling to avoid potential exceptions or unexpected behavior. This article will guide you through different methods and best practices for checking null or empty values in C#, providing you with the knowledge to effectively handle these scenarios in your code.
Creating a Method to Check for Null or Empty Values
Defining the method signature:
To start, let’s define a method that can be used to check if a value is null or empty. The method signature could be something like:
“`C#
public static bool IsNullOrEmpty(string value)
{
// Check for null or empty values here
}
“`
Implementing the logic to check for null or empty values:
Inside the `IsNullOrEmpty` method, you can implement the logic to check for null or empty values. One way to do this is by utilizing the `string.IsNullOrEmpty` method, which checks if a string is null or empty:
“`C#
public static bool IsNullOrEmpty(string value)
{
return string.IsNullOrEmpty(value);
}
“`
Returning a boolean value indicating whether the value is null or empty:
Finally, the method should return a boolean value indicating whether the value is null or empty. In this case, the `IsNullOrEmpty` method simply returns the result of `string.IsNullOrEmpty`:
“`C#
public static bool IsNullOrEmpty(string value)
{
return string.IsNullOrEmpty(value);
}
“`
Handling Null Values
Understanding the concept of null values:
In C#, null is a special value that indicates the absence of a reference to an object. It is different from an empty string or an empty collection. Understanding the concept of null values is crucial to correctly handle them in your code.
Using the null-coalescing operator (??) to handle null values:
To handle null values, you can use the null-coalescing operator (??). This operator allows you to provide a default value if a given value is null. Here’s an example:
“`C#
string name = null;
string displayName = name ?? “Anonymous”;
Console.WriteLine(displayName); // Output: “Anonymous”
“`
In this example, the value of `name` is null, so the null-coalescing operator assigns the default value of “Anonymous” to `displayName`.
Implementing conditional statements to handle null values:
Another approach to handle null values is by using conditional statements. You can check if a value is null using the `==` operator and perform a specific action accordingly. For example:
“`C#
string name = null;
if (name == null)
{
Console.WriteLine(“Name is null”);
}
else
{
Console.WriteLine(“Name is not null”);
}
“`
In this case, since the value of `name` is null, the condition `name == null` evaluates to true, and the corresponding message is displayed.
Treating Empty Strings
Understanding the concept of empty strings:
Empty strings are different from null values. An empty string represents a zero-length string, containing no characters. It’s important to differentiate between null values and empty strings when working with string inputs.
Using the `string.IsNullOrEmpty` method to check for empty strings:
To check for empty strings, you can utilize the `string.IsNullOrEmpty` method. This method returns true if a given string is null or empty. Here’s an example:
“`C#
string input = “”;
if (string.IsNullOrEmpty(input))
{
Console.WriteLine(“Input is null or empty”);
}
else
{
Console.WriteLine(“Input is not null or empty”);
}
“`
In this example, the string `input` is empty, so the condition `string.IsNullOrEmpty(input)` evaluates to true.
Implementing string manipulation methods to handle empty strings:
Sometimes, you may need to prevent or handle empty strings. In such cases, you can use various string manipulation methods, such as `Trim`, `Replace`, or `Substring`, to modify the string and make it non-empty. Here’s an example that trims leading and trailing whitespace from a string:
“`C#
string input = ” Example “;
string trimmedInput = input.Trim();
Console.WriteLine(trimmedInput); // Output: “Example”
“`
In this example, the `Trim` method removes the extra whitespace from the input string.
Validating User Input
Using input validation to prevent null or empty values:
When accepting user input, it’s important to validate and ensure that the input is not null or empty. This can be done using input validation techniques, such as checking for null values or empty strings before further processing. Here’s an example:
“`C#
string userInput = GetUserInput();
if (string.IsNullOrEmpty(userInput))
{
Console.WriteLine(“Invalid input. Please provide a valid value.”);
}
else
{
// Process user input
}
“`
Implementing error handling mechanisms to prompt users for valid input:
In addition to input validation, implementing error handling mechanisms can prompt users for valid input when null or empty values are encountered. You can display appropriate error messages and request the user to provide valid input. Here’s an example:
“`C#
string userInput = GetUserInput();
while (string.IsNullOrEmpty(userInput))
{
Console.WriteLine(“Invalid input. Please provide a valid value.”);
userInput = GetUserInput();
}
// Process user input
“`
In this example, the user is repeatedly prompted for valid input until a non-null and non-empty value is provided.
Displaying appropriate error messages when null or empty values are encountered:
When null or empty values are encountered, it’s important to display meaningful error messages to guide users. This helps them understand the issue and provide the necessary valid input. For example:
“`C#
string userInput = GetUserInput();
while (string.IsNullOrEmpty(userInput))
{
Console.WriteLine(“Invalid input. The input cannot be null or empty.”);
userInput = GetUserInput();
}
// Process user input
“`
In this example, the error message clearly states that null or empty input is not allowed.
Working with Database Fields
Retrieving values from database fields and checking for null or empty values:
When working with database fields, it’s crucial to handle null or empty values appropriately. When retrieving values from database fields, you can check for null or empty values before further processing or displaying the data. Here’s an example:
“`C#
string firstName = ReadFirstNameFromDatabase();
if (!string.IsNullOrEmpty(firstName))
{
Console.WriteLine(“First Name: ” + firstName);
}
“`
In this example, the value of `firstName` is checked for null or emptiness before being displayed.
Handling null or empty values in database queries:
Database queries may also involve checking for null or empty values. When constructing database queries, you can utilize SQL functions to handle null or empty values appropriately. Here’s an example:
“`C#
SELECT * FROM Customers WHERE Email IS NULL OR Email = ”
“`
In this example, the `IS NULL` condition checks for null values, while the `= ”` condition checks for empty values in the `Email` field.
Using SQL functions to handle null or empty values in database operations:
In addition to database queries, you can also use SQL functions to handle null or empty values in other database operations, such as inserts or updates. For example:
“`C#
INSERT INTO Customers (Email) VALUES (NULLIF(@Email, ”))
“`
In this example, the NULLIF function eliminates the empty string if the `@Email` parameter holds an empty value.
Best Practices for Dealing with Null or Empty Values
Using data annotations to enforce non-null or non-empty values:
To enforce non-null or non-empty values, you can utilize data annotations in C#. Data annotations provide a convenient way to validate and specify constraints on properties or fields. Here’s an example using the `Required` attribute:
“`C#
public class Person
{
[Required]
public string Name { get; set; }
[Required]
[EmailAddress]
public string Email { get; set; }
}
“`
In this example, the `Required` attribute enforces that the `Name` and `Email` properties should not be null or empty.
Following a consistent coding style to avoid null or empty value issues:
Following a consistent coding style and adhering to established conventions can help avoid null or empty value issues. This includes properly initializing variables, handling potential null values, and providing default values where appropriate.
Writing unit tests to validate the behavior of methods handling null or empty values:
Unit testing is essential to ensure that the methods handling null or empty values behave as expected in various scenarios. By writing unit tests, you can validate the behavior of these methods and catch any potential issues early on.
Using Conditional Operators to Simplify Null or Empty Checks
Utilizing conditional operators to simplify null or empty checks:
In C#, you can utilize conditional operators, such as the ternary operator (`?:`) or the null conditional operator (`?.`), to simplify null or empty checks. These operators provide concise ways to write conditional statements. Here’s an example using the ternary operator:
“`C#
string name = GetName();
Console.WriteLine(name != null ? name : “Unknown”);
“`
In this example, the ternary operator checks if `name` is not null. If it’s not null, the value of `name` is printed; otherwise, “Unknown” is printed.
Demonstrating examples of using conditional operators for more concise code:
Using conditional operators can lead to more concise code. Here’s an example comparing a traditional if-else statement and the null conditional operator:
“`C#
string address = GetAddress();
if (address != null)
{
Console.WriteLine(address.City);
}
“`
“`C#
string address = GetAddress();
Console.WriteLine(address?.City);
“`
In the first example, a traditional if-else statement is used to check if `address` is not null before accessing the `City` property. In the second example, the null conditional operator (`?.`) achieves the same result in a more concise manner.
Exploring potential drawbacks and limitations of using conditional operators:
While conditional operators can simplify code, it’s important to be aware of their limitations. For example, the null conditional operator (`?.`) can only be used with reference types and may not work as expected with value types or custom classes. Additionally, excessive usage of conditional operators can lead to less readable code, so it’s important to strike a balance.
Summary of Null or Empty Checking in C#
In this article, we discussed various methods and best practices for checking null or empty values in C#. We learned about creating a method to check for null or empty values, handling null values using the null-coalescing operator or conditional statements, treating empty strings using string manipulation methods, validating user input, working with database fields, and using conditional operators to simplify null or empty checks. Along with this, we covered best practices such as using data annotations, following coding styles, and writing unit tests. By implementing these techniques and practices, you can effectively handle null or empty values in your C# codebase and ensure the robustness of your applications.
FAQs
Q: What is the difference between null and empty values?
A: Null represents the absence of a reference to an object, while an empty value indicates a zero-length string or collection.
Q: How can I check if a string is null or empty in C#?
A: You can use the `string.IsNullOrEmpty` method to check if a string is null or empty. For example: `string.IsNullOrEmpty(value)`.
Q: How can I handle null values in my code?
A: You can handle null values by using the null-coalescing operator (??) or conditional statements to provide default values or perform specific actions when a value is null.
Q: Can I use conditional operators with value types or custom classes?
A: Conditional operators, such as the null conditional operator (?.), can only be used with reference types and may not work as expected with value types or custom classes.
Q: Why is it important to handle null or empty values in my code?
A: Handling null or empty values helps prevent potential exceptions, unexpected behavior, or data corruption in your applications. It improves the reliability and robustness of your code.
Q: Should I use data annotations to enforce non-null or non-empty values?
A: Data annotations provide a convenient way to enforce non-null or non-empty values. They help ensure data integrity and can be useful for validation purposes.
Q: What should I do if I encounter null or empty values in database fields or queries?
A: When working with database fields or queries, you can use SQL functions to handle null or empty values appropriately. Additionally, validating user input and displaying appropriate error messages are recommended practices.
How To Check String Is Null Or Empty Or Whitespace In C#
How To Check If Null Or Empty In C?
When writing code in C, it’s essential to ensure proper handling of null or empty values to avoid unexpected behavior or program crashes. In this article, we will discuss various techniques and best practices to check if a variable is null or empty in the C programming language. We will cover different scenarios, explain the differences between null and empty, and provide examples and code snippets to clarify the concepts.
Understanding Null and Empty
Before diving into the checking techniques, it’s important to understand the distinction between null and empty values. In C, null is a special value that represents the absence of a meaningful object or value. On the other hand, an empty value refers to a variable that has been declared but is not holding any valid data at a particular point in time.
Checking for Null
To determine if a variable is null, we can use the equality (==) operator. However, it’s crucial to note that the equality operator only checks for strict equality and cannot differentiate between null and other types of values like zero or an empty string. Hence, it’s recommended to compare the variable with the null pointer constant, which is represented by ‘NULL’. Here’s an example:
“`
int* ptr = NULL;
if (ptr == NULL) {
// The variable is null
}
“`
Checking for Empty
To check if a variable is empty, we need to consider its data type. For primitive types like integers, floats, or characters, there is no concept of emptiness. These types always hold a value. However, for arrays or strings, we can examine their length or check if they are null.
For arrays, it’s preferable to determine their length explicitly or use a sentinel value to indicate emptiness. For instance, if we have an integer array ‘numbers,’ we can define a constant ‘EMPTY’ with a value that indicates an empty array:
“`
#define EMPTY -1
int numbers[10] = {1, 2, 3};
int length = sizeof(numbers) / sizeof(numbers[0]);
if (length == 0 || numbers[0] == EMPTY) {
// The array is empty
}
“`
For strings, we can use the standard library function ‘strlen()’ to check their length. If the length is zero, we conclude that the string is empty. Consider the following example:
“`
#include
char name[20] = “John”;
if (strlen(name) == 0) {
// The string is empty
}
“`
Frequently Asked Questions
Q1: What is the difference between null and empty?
A1: Null refers to the absence of a meaningful object or value, whereas empty refers to a variable that is declared but is not holding any valid data.
Q2: Are null and zero the same in C?
A2: No, null and zero are not the same in C. Null is a special value representing the absence of a meaningful object, whereas zero is a valid data value.
Q3: How do I check if a variable is null or empty in C?
A3: To check if a variable is null, compare it with the null pointer constant (‘NULL’). To check if it’s empty, consider the data type. For arrays, check their length or sentinel value. For strings, use the ‘strlen()’ function.
Q4: Can I use the equality operator (==) to check for null or emptiness in C?
A4: While the equality operator can be used to check for null, it cannot differentiate between null and other types of values. Hence, it’s recommended to compare with the null pointer constant or use appropriate techniques based on the data type.
Q5: What are the best practices for checking null or empty values in C?
A5: It’s considered good practice to explicitly handle null or empty values, especially for pointers and dynamically allocated memory. Performing proper checks reduces the likelihood of unexpected crashes or undefined behavior.
In conclusion, ensuring proper null or empty checks is crucial when programming in C. By understanding the differences between null and empty values, and utilizing appropriate techniques for different data types, you can write robust and error-free code. Remember to follow best practices, handle null or empty values explicitly, and choose the appropriate approach based on the data being evaluated.
What Is Empty Vs Null In C?
When working with the C programming language, it is important to understand the difference between “empty” and “null.” These terms might seem similar at first, but they have distinct meanings and usage in the context of C programming. In this article, we will explore what empty and null mean in C, how they are used, and clarify any confusion surrounding these concepts.
Understanding empty in C:
In C, the term “empty” refers to the absence of any value in a particular data type or variable. This means that the variable has not been initialized or assigned a value. When a variable is empty, its content is undefined, and attempting to access its value may lead to unpredictable results. It is essential to assign a value to a variable before using it to avoid such issues.
For example, consider the following code snippet:
“`
int x; // declaring an integer variable
printf(“%d”, x); // accessing the variable without initializing it
“`
Here, the variable “x” is empty as it has not been assigned any value. Accessing it using the printf function will result in undefined behavior. The value printed on the screen could be any garbage value or may even cause a program crash.
Understanding null in C:
On the other hand, “null” in C refers to a special value that is used to indicate the absence of meaningful data or an invalid or uninitialized pointer. Pointers are variables that store memory addresses as their values. By assigning a null value to a pointer, it indicates that the pointer is not currently pointing to any valid memory location.
Consider the following example:
“`
int *ptr = NULL; // assigning a null value to a pointer
“`
In this case, the pointer “ptr” is assigned a null value. This means that it does not point to any valid memory address. It is crucial to initialize pointers with a valid memory address to avoid issues like segmentation faults or runtime errors.
Distinguishing between empty and null:
While both empty and null represent the absence of a value, they differ in their contexts and usage. Empty is used for uninitialized variables, whereas null is specifically used for pointer variables. It is important to keep these distinctions in mind to write reliable and bug-free C programs.
Frequently Asked Questions:
Q: Can I assign an empty value to a pointer variable?
A: No, the concept of empty value does not apply to pointers. Pointers should either hold the address of a valid memory location or be assigned a null value.
Q: Is it necessary to initialize variables to empty or null explicitly?
A: It is a good programming practice to initialize variables explicitly before using them. Initializing variables prevents accessing garbage values, reduces the risk of unexpected behavior, and improves the overall reliability of the program. While some programming languages automatically initialize variables to default values, C does not do so.
Q: How can I check if a variable is empty or null?
A: In C, there is no direct way to check if a variable is empty. However, you can use conditional statements or comparison operators to check for specific values. For pointers, you can check if they are null by comparing them to the null macro or using the logical operator `== NULL`.
Q: What happens when an empty or null variable is used in an expression?
A: Using an empty or null variable in an expression can lead to undefined behavior, unexpected results, or even program crashes. Before using any variable, it is crucial to assign a meaningful value to it.
Q: Can I assign a value to an empty variable?
A: Yes, you can assign a value to an empty variable after declaring it. However, it is recommended to initialize variables at the time of declaration itself to avoid any confusion or potential issues.
In conclusion, the terms “empty” and “null” have different meanings and usage in C programming. Empty refers to the absence of a value in a variable, while null is specifically used for indicating an invalid or uninitialized pointer. Understanding these concepts is crucial to ensure reliable and bug-free C programs. Always remember to initialize variables and assign valid memory addresses to pointers before using them to avoid any unexpected behavior or runtime errors.
Keywords searched by users: isnull or empty c# Is null or empty C#, Check string is empty C#, string.empty trong c#, IsNullOrWhiteSpace, String null to empty c#, Check list == null or empty C#, C# check array is null or empty, Check string is null
Categories: Top 89 Isnull Or Empty C#
See more here: nhanvietluanvan.com
Is Null Or Empty C#
Introduction:
In C#, developers often come across situations where they need to determine whether a string or collection is empty or null. To address these scenarios, the C# programming language provides a convenient method called IsNullOrEmpty. This method allows developers to check whether a given string or collection is null or empty. In this article, we will explore the IsNullOrEmpty method in detail, discussing its purpose, usage, and potential benefits. Additionally, we will provide answers to some commonly asked questions related to this topic.
What is IsNullOrEmpty?
IsNullOrEmpty is a static method provided by the string class in C#. It is used to determine whether a string or collection is either null or empty. The method evaluates the given string or collection and returns a boolean value indicating whether it is null or contains no elements. When the target is neither null nor empty, the method returns false; otherwise, it returns true.
Usage of IsNullOrEmpty:
The IsNullOrEmpty method is primarily used in scenarios where developers need to handle inputs appropriately, ensuring they are not null or empty before further processing. This is particularly useful in cases involving user input, data validation, and handling external data sources. By using IsNullOrEmpty, developers can easily validate strings or collections, preventing unexpected errors and improving the overall reliability of their code.
To utilize the IsNullOrEmpty method, simply pass the string or collection object as an argument. For example:
string name = “John Doe”;
bool isNameNullOrEmpty = string.IsNullOrEmpty(name);
Console.WriteLine(isNameNullOrEmpty); // Output: False
In the above example, the variable name contains a non-null and non-empty string. Hence, the method returns false, indicating that the string is neither null nor empty.
Benefits of IsNullOrEmpty:
The IsNullOrEmpty method offers several benefits for developers, including:
1. Improved Code Readability: Utilizing IsNullOrEmpty enhances code readability by explicitly stating the intention of checking for null or empty values. This makes the code easier to understand and maintain for both the original developer and future collaborators.
2. Simplified Conditional Statements: By using IsNullOrEmpty, developers can simplify their conditional statements, leading to cleaner and more concise code. Conditional checks become more straightforward when using this method, improving code readability and reducing the potential for logic errors.
3. Efficient Null and Empty Value Handling: IsNullOrEmpty provides a straightforward way to handle null or empty values. Instead of manually checking for both conditions separately, developers can rely on a single method call, saving time and effort.
Frequently Asked Questions (FAQs):
Q1: How is IsNullOrEmpty different from checking for null and empty separately?
A1: While it is possible to manually check for both null and empty conditions separately, using IsNullOrEmpty simplifies the process by combining both checks into a single statement. This improves code readability and reduces the potential for logical errors.
Q2: Can IsNullOrEmpty be used with collections other than strings?
A2: Yes, the IsNullOrEmpty method can be used with any type of collection, not just strings. Its usage is not limited to a specific type; any collection object can be passed as an argument to determine whether it is null or empty.
Q3: Does IsNullOrEmpty throw an exception when applied to a collection?
A3: No, the IsNullOrEmpty method does not throw an exception when applied to a collection. If a collection is null or empty, it simply returns true; otherwise, it returns false. This behavior allows developers to handle empty or null collections gracefully in their code.
Q4: Are there any performance implications when using IsNullOrEmpty?
A4: The IsNullOrEmpty method itself is highly optimized and has negligible performance implications. However, excessive usage of this method in large-scale applications may impact performance due to the frequent string or collection evaluations. As with any coding practice, it is important to consider performance optimization guidelines while incorporating IsNullOrEmpty into your code.
Q5: Can IsNullOrEmpty be used with other programming languages apart from C#?
A5: IsNullOrEmpty is a specific method in the C# programming language and is not available in other languages. However, most programming languages provide similar functionalities to check for null or empty values, although the method or syntax may differ.
Conclusion:
In C#, the IsNullOrEmpty method plays a crucial role in ensuring null or empty string or collection values are handled properly. By using this method, developers can enhance code readability, simplify conditional statements, and efficiently handle null and empty values. Understanding the purpose and usage of IsNullOrEmpty is essential for writing robust, reliable, and maintainable code.
Check String Is Empty C#
In C#, checking whether a string is empty or not is a common requirement in programming. An empty string can be defined as a string that has no characters or consists only of whitespace. In this article, we will explore various methods to check if a string is empty in C# and discuss some commonly asked questions related to this topic.
Methods to Check if a String is Empty in C#:
1. String.IsNullOrEmpty Method:
The `String.IsNullOrEmpty` method is a built-in method in C# that checks if a string is null or an empty string. It returns true if the string is null or empty; otherwise, it returns false. This method is a simple and convenient way to check for an empty string.
“`csharp
string myString = “”;
if (String.IsNullOrEmpty(myString))
{
Console.WriteLine(“The string is empty.”);
}
“`
2. String.IsNullOrWhiteSpace Method:
In some cases, we may need to consider a string as empty even if it contains only whitespace characters. The `String.IsNullOrWhiteSpace` method checks if a string is null, empty, or contains only whitespace characters. It returns true if the string meets these conditions; otherwise, it returns false.
“`csharp
string myString = ” “;
if (String.IsNullOrWhiteSpace(myString))
{
Console.WriteLine(“The string is empty or contains only whitespace characters.”);
}
“`
3. String.Length Property:
Another way to check if a string is empty is by using the `Length` property of the string. If the length of the string is zero, it signifies that the string is empty. However, it is important to note that using the `Length` property on a null string will result in a `NullReferenceException`. Hence, it is advisable to use this method only when you are sure that the string is not null.
“`csharp
string myString = “”;
if (myString.Length == 0)
{
Console.WriteLine(“The string is empty.”);
}
“`
4. String.IsNullOrWhitespace Extension Method:
We can create an extension method to simplify the code for checking whether a string is empty or contains only whitespace characters. This method checks if a string is null, empty, or contains only whitespace characters and returns true if it does; otherwise, it returns false.
“`csharp
public static class StringExtensions
{
public static bool IsEmptyOrWhitespace(this string value)
{
return value == null || String.IsNullOrWhiteSpace(value);
}
}
“`
Now, we can use this extension method as follows:
“`csharp
string myString = ” “;
if (myString.IsEmptyOrWhitespace())
{
Console.WriteLine(“The string is empty or contains only whitespace characters.”);
}
“`
FAQs:
1. What is the difference between `String.IsNullOrEmpty` and `String.IsNullOrWhiteSpace`?
The `String.IsNullOrEmpty` method checks if a string is either null or an empty string, while the `String.IsNullOrWhiteSpace` method checks if a string is either null, empty, or contains only whitespace characters.
2. Why use the `String.IsNullOrWhitespace` extension method instead of the `String.IsNullOrWhiteSpace` method?
The `String.IsNullOrWhitespace` extension method simplifies the code by providing a single method to check if a string is empty or contains only whitespace characters. This extension method can be reused throughout the application, promoting code reusability.
3. What happens if we try to use the `Length` property on a null string?
Using the `Length` property on a null string will result in a `NullReferenceException`. Therefore, it is important to ensure that the string is not null before accessing its `Length` property.
In conclusion, checking whether a string is empty or not is a common requirement in C# programming. In this article, we discussed various methods to check if a string is empty in C#, including the built-in methods `String.IsNullOrEmpty` and `String.IsNullOrWhiteSpace`, as well as using the `Length` property and creating a custom extension method. By understanding these methods, you can effectively handle empty string scenarios in your C# projects.
String.Empty Trong C#
Introduction:
In C#, handling string variables is a common task for developers. When working with strings, it is crucial to have a clear understanding of the various methods and properties available. One such property is String.Empty. In this comprehensive article, we will delve deep into String.Empty in C#, exploring its definition, usage, and significance. Additionally, we will address some frequently asked questions to help clarify any potential queries.
What is String.Empty?
String.Empty is a static property of the String class in C#. It represents an empty string literal, containing no characters. Programmatically, it is denoted as “” (two double quotes). Unlike a null value, which denotes the absence of a string object, String.Empty signifies a string object with no content.
Understanding the Usage of String.Empty:
1. String Initialization:
One of the primary purposes of String.Empty is to initialize an empty string. Instead of using two double quotes directly, you can leverage String.Empty to improve readability and ensure consistency in your code.
For instance:
string name = String.Empty;
OR
string name = “”;
2. String Comparison:
String.Empty can be useful when comparing strings for equality. While it may seem trivial, comparing to an empty string allows for a specific check and ensures the code remains self-explanatory.
For example:
string userInput = Console.ReadLine();
if (userInput == String.Empty)
{
// Perform an action if the user input is empty
}
3. Concatenation and Building Strings:
String.Empty can also be employed while concatenating strings or building new strings. By using an empty string as a starting point, you can create a flexible and efficient approach to formulating a desired output.
Consider the following example:
string firstName = “John”;
string lastName = String.Empty;
string fullName = firstName + ” ” + lastName;
In this case, the string “John ” would be assigned to the fullName variable.
4. Trimming Strings:
String.Empty can also be utilized while trimming excess whitespace from strings. The Trim() method removes leading and trailing whitespace. However, for removing whitespace from the middle of a string, String.Empty can be used along with the Replace() method.
For instance:
string someString = ” this is a test”;
someString = someString.Replace(” “, String.Empty);
Significance of String.Empty:
The use of String.Empty plays a key role in improving code readability, performance, and consistency. By employing String.Empty, the purpose or intention of the code becomes clearer, reducing potential confusion and increasing maintainability. Additionally, String.Empty is more efficient than “” (two double quotes) as it avoids the creation of a new string instance.
Frequently Asked Questions (FAQs):
Q1. How does String.Empty differ from null?
A1. String.Empty represents an empty string literal, while null signifies the absence of a string object. In other words, String.Empty is a string object with no content, whereas null does not point to a string object at all.
Q2. Is String.Empty equivalent to “” (two double quotes)?
A2. Yes, String.Empty and “” are functionally equivalent, both denoting an empty string. However, String.Empty is preferred for better code readability and to maintain consistency.
Q3. Can we modify the value of String.Empty?
A3. String.Empty is a read-only property and cannot be modified. It always represents an empty string literal.
Q4. Is there any performance gain by using String.Empty instead of “”?
A4. Yes, using String.Empty instead of “” can result in minor performance gains. When using String.Empty, no new string instance needs to be created, reducing memory allocation and improving overall performance.
Q5. When should I use String.Empty instead of “”?
A5. While the usage of String.Empty instead of “” is mainly a matter of personal preference, it is recommended to use String.Empty for consistent code readability throughout the project.
Conclusion:
In the world of C# development, a clear understanding of String.Empty is crucial to handle string variables effectively. We have explored the definition, usage, and significance of String.Empty in this comprehensive article. By utilizing String.Empty, you can improve the readability, maintainability, and performance of your code when working with strings. Remember, String.Empty represents an empty string object with no content and is a valuable tool at a developer’s disposal.
Images related to the topic isnull or empty c#
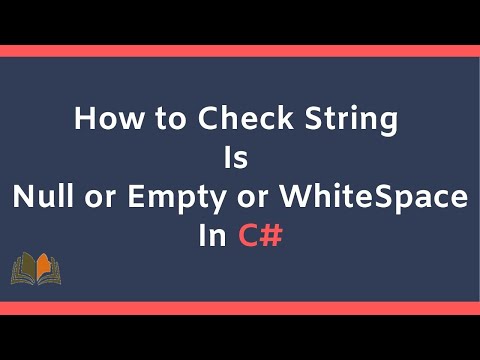
Article link: isnull or empty c#.
Learn more about the topic isnull or empty c#.
- C# | IsNullOrEmpty() Method – GeeksforGeeks
- String.IsNullOrEmpty(String) Method (System) – Microsoft Learn
- How to Check if a String is Empty in C – Sabe.io
- Null String vs Empty String – Coding Blocks Discussion Forum
- How to check if C string is empty – Stack Overflow
- Understanding Null Versus the Empty String – Oracle Help Center
- How can I check whether a string variable is empty or null in C …
- IsNullOrEmpty() Method in C – Tutorialspoint
- How to check if a string is empty in C# | Reactgo
- C# String IsNullOrEmpty() method – Javatpoint
- Checking For Empty or Null String in C# – C# Corner
- Check if a String Is Null or Empty in C# | Delft Stack
See more: https://nhanvietluanvan.com/luat-hoc/