Is Python Case Sensitive
1. Definition and Explanation of Case Sensitivity in Programming Languages
In programming languages, case sensitivity refers to the distinction between uppercase and lowercase letters when writing code. Case sensitivity determines whether two identical sequences of characters with different letter cases will be treated as the same or different entities by the programming language.
2. Introductory Explanation of Python’s Case Sensitivity Rules
Python is a case-sensitive programming language, meaning that it differentiates between uppercase and lowercase letters. This implies that Python treats variables, functions, keywords, and other identifiers using different letter cases as distinct entities. For example, the variables “myVar” and “myvar” would be treated as separate identifiers and not interchangeable in Python.
3. Variables and Identifiers: Case Sensitivity in Python
When working with variables in Python, it is crucial to understand that the language is case sensitive. Declaring a variable with a specific letter case creates a separate variable from another identifier with a different case. For instance, “myVariable” and “myvariable” will be treated as distinct variables in Python. It is important to use consistent casing when working with variable names to avoid confusion and unintended errors.
4. Python Keywords and Case Sensitivity
Python has a set of reserved keywords that are used to define the language’s syntax and structure. These keywords have predefined meanings and cannot be used as variable names. It is essential to note that Python keywords are case-sensitive, meaning they must be written in lowercase. Using uppercase or mixed-case versions of Python keywords will result in syntax errors.
5. Built-in Functions and Case Sensitivity in Python
Python provides numerous built-in functions that perform specific operations. Similar to Python keywords, these built-in functions are case-sensitive and must be used in lowercase. For example, the function “print()” is a built-in function used for displaying output, and using “Print()” with a capital P will generate an error. Python also offers various string manipulation functions, such as “lower()” and “upper()”, which depend on case sensitivity to transform strings.
6. Modules and Packages: Handling Case Sensitivity in Python
In Python, modules and packages are essential components for organizing code into reusable and manageable units. When working with modules and packages, it is crucial to handle case sensitivity correctly to ensure proper importing and usage. The file names of modules and packages should adhere to the operating system’s case sensitivity rules. For instance, if a module is named “myModule.py”, attempting to import it using “mymodule” or “MyModule” would fail. Therefore, consistency in module and package naming conventions is crucial.
7. File and Directory Names: Case Sensitivity in Python
Python interacts with the file system, and it is important to consider case sensitivity when dealing with file and directory names. The behavior regarding case sensitivity in file systems may vary based on the operating system being used. For example, Windows file systems are case-insensitive, while Unix-based systems (such as Linux) are case-sensitive. Therefore, using consistent casing when referencing file and directory names is vital, especially when migrating code across different platforms.
8. Best Practices for Consistent Case Usage in Python Programming
To promote clean and readable code, it is crucial to follow best practices for consistent case usage in Python programming. Here are some recommendations:
a. Use lowercase letters for variable and function names: Following the Pythonic convention of using lowercase with underscores (snake_case) for variable and function names enhances code readability.
b. Use uppercase letters for constants: Constants, such as global variables that do not change during program execution, are typically written in uppercase with underscores (UPPER_CASE).
c. Be consistent with identifier casing: Avoid mixing uppercase and lowercase letters within the same identifier. Use consistent casing to improve code comprehension and maintainability.
d. Pay attention to Python’s reserved keywords: Be mindful of Python’s reserved keywords and avoid using them as variable names. Always use the appropriate lowercase versions of these keywords.
9. Case Sensitivity in Python: Common Errors and Troubleshooting
When dealing with identifiers, programmers may encounter various case sensitivity-related issues. Here are some frequently asked questions and answers to help troubleshoot common problems:
Q1: Is Python case-sensitive when dealing with identifiers?
A1: Yes, Python is case-sensitive when it comes to identifiers. Using different letter cases in variable names or other identifiers will result in distinct entities.
Q2: What does case-sensitive mean in Python?
A2: Case-sensitive in Python means that uppercase and lowercase letters are treated as different entities. For instance, “myVar” and “myvar” would be considered separate variables.
Q3: How do I find a string in a list case-insensitively in Python?
A3: To perform a case-insensitive search in a list, you can convert both the list elements and the search string to lowercase using the “lower()” method. Then, you can compare the lowercase versions to find a match.
Q4: How do I compare strings in Python while ignoring case?
A4: To compare two strings in Python while ignoring case, you can convert both strings to either lowercase or uppercase using the “lower()” or “upper()” methods. Then, you can compare the transformed strings using logical operators.
Q5: How can I replace a string in Python while ignoring case?
A5: To replace a string while ignoring case, you can use regular expressions with the “re” module in Python. By utilizing the “re.IGNORECASE” flag, you can perform case-insensitive string replacements.
Q6: How do I convert a string to lowercase in Python?
A6: To convert a string to lowercase in Python, you can use the “lower()” method. For example, “myString.lower()” will return the lowercase version of “myString”.
Q7: How do I check if two strings are equal in Python (case-insensitive)?
A7: To check if two strings are equal in Python while ignoring case, you can convert both strings to lowercase or uppercase using the “lower()” or “upper()” methods. Then, you can compare the transformed strings using the equality operator (==).
Q8: How can I convert user input to lowercase in Python?
A8: To convert user input to lowercase in Python, you can use the “lower()” method on the input string. For example, “user_input.lower()” will return the lowercase version of “user_input”.
In conclusion, Python is a case-sensitive programming language that distinguishes between uppercase and lowercase letters. Understanding Python’s case sensitivity rules is crucial for writing clean and error-free code. By following best practices and paying attention to case sensitivity in variables, keywords, and identifiers, programmers can enhance code readability and avoid common errors.
Comparing Case-Sensitive Strings In Python – (Learn String Methods, Ascii, And Encoding) Tutorial
Why Is Python Case-Sensitive?
Python is a popular programming language known for its simplicity, readability, and versatility. As with any programming language, Python has its own set of rules and syntax. One of the important aspects of Python’s syntax is its case-sensitivity, which means that it distinguishes between uppercase and lowercase characters. In this article, we will explore the reasons why Python is case-sensitive and the implications it has on programming.
Case sensitivity refers to the distinction made by a programming language between uppercase and lowercase characters when interpreting text. In case-insensitive languages, such as SQL, uppercase and lowercase letters are considered equivalent. This means that “Hello,” “HELLO,” and “hello” would all be treated as the same word. However, in case-sensitive languages like Python, these three variations would be interpreted as three different words, each with its own meaning and significance.
There are several reasons why Python and many other programming languages embrace case-sensitivity. Let’s delve deeper into some of these reasons:
1. Consistency and Clarity: Case-sensitivity provides consistency and clarity in programming. By requiring the use of both lowercase and uppercase characters, Python ensures that names and identifiers can be easily distinguished. This makes the code more readable, reducing the potential for confusion and misinterpretation.
2. Compatibility with Other Languages: Many programming languages such as C, C++, Java, and JavaScript are also case-sensitive. By adopting case-sensitivity, Python ensures compatibility across different languages and facilitates code sharing and collaborations among developers working with different technologies.
3. Greater Functionality: Case sensitivity allows for the creation of more complex and expressive code. For instance, Python allows you to define different variables or functions with similar names but with different casings. This flexibility can help improve code organization and make it more modular, allowing developers to build sophisticated applications.
4. Respect for Case-Sensitive File Systems: Modern file systems in operating systems like Linux, Unix, and macOS are case-sensitive. By adopting case-sensitivity, Python behaves consistently with these file systems. Developers can now seamlessly work with file names and directories, without having to worry about differences in case leading to errors or unwanted behavior.
While case-sensitivity is a powerful feature in Python, it also presents certain challenges and considerations for developers. Here are a few frequently asked questions regarding Python’s case-sensitivity:
Q: Can I use both upper and lower cases when naming variables and functions?
A: Yes, Python allows you to mix uppercase and lowercase characters when naming variables and functions. However, it is recommended to follow a consistent naming convention to improve code readability and maintainability.
Q: Are there any exceptions to Python’s case-sensitivity?
A: Yes, there are exceptions in the case of reserved words or keywords. Python’s reserved words, such as “if,” “for,” and “while,” are case-sensitive. Therefore, you cannot use variations of these reserved words with different cases.
Q: Can I access a variable with different casing if it has been defined before?
A: No, Python treats variables with different casings as separate entities. If you define a variable as “myVariable” and then try to access it as “myvariable,” Python will consider it as a different variable. It is essential to maintain consistency in casing throughout your code.
Q: How can I deal with case-sensitivity issues?
A: To avoid case-sensitivity issues, it is vital to follow consistent naming conventions. Choose a style (such as camelCase or snake_case) and stick to it throughout your codebase.
Q: Can I change Python’s case-sensitivity behavior?
A: No, Python’s case-sensitivity is an intrinsic part of its design and cannot be changed. It is advised to adapt to Python’s case-sensitivity rules instead of trying to modify them to fit personal preferences.
In conclusion, Python’s case-sensitivity is a fundamental aspect of its syntax that contributes to code consistency, clarity, and compatibility with other programming languages. Understanding and embracing Python’s case-sensitivity rules is essential for writing clean, readable, and bug-free code. While it may present some challenges, following consistent naming conventions and being mindful of case-sensitivity issues will help you navigate this aspect of Python programming.
Is Python Case-Sensitive With Example?
Python, a popular programming language known for its simplicity and readability, has gained incredible popularity among developers. As someone new to programming, you may wonder whether Python is case-sensitive or not. This article will provide a comprehensive answer to this question, as well as include examples to help you understand the concept better.
Python is indeed a case-sensitive language, meaning that the interpreter distinguishes between lowercase and uppercase characters. This sensitivity can be observed in various aspects of Python programming, including variable names, function names, module names, and keywords. Let’s dive deeper into each of these aspects to gain a better understanding.
1. Variable Names:
In Python, the names of variables are case-sensitive. This means that variables named “myVar” and “myvar” would be treated as two separate variables. Consider the following example:
“`
myVar = 10
myvar = 5
print(myVar) # Output: 10
print(myvar) # Output: 5
“`
Even though the variable names are almost identical, Python treats them separately due to its case sensitivity.
2. Function Names:
Similar to variable names, Python distinguishes between lowercase and uppercase characters in function names. For instance:
“`
def myFunction():
print(“Hello from myFunction!”)
def myfunction():
print(“Hello from myfunction!”)
myFunction() # Output: Hello from myFunction!
myfunction() # Output: Hello from myfunction!
“`
In the example above, we define two functions with similar names but differing in casing. When called, Python identifies them as distinct functions and executes them accordingly.
3. Module Names:
Python modules are files containing Python definitions and statements. When importing a module, Python relies on the specified case. If the specified case does not match the exact filename or module name, an error will occur. Here’s an example:
File: myModule.py
“`
def greet():
print(“Hello from myModule!”)
“`
File: mymodule.py
“`
def greet():
print(“Hello from mymodule!”)
“`
main.py
“`
import myModule # Error: ModuleNotFoundError
import mymodule
mymodule.greet() # Output: Hello from mymodule!
“`
In this example, we have two module files with similar names; however, Python does not find `myModule` because it does not match the specified case. On the other hand, `mymodule` works as it matches the case used when importing the module.
4. Keywords:
Python has a set of reserved keywords that are reserved for special purposes and cannot be used as identifiers, such as variables, functions, or class names. Similar to variable and function names, Python is case-sensitive when it comes to keywords. For instance:
“`
def ifExample():
if = 5 # SyntaxError: invalid syntax
“`
In this example, we try to use “if” as a variable name, which results in a SyntaxError. It is because “if” is a reserved keyword in Python and must be used in certain contexts only.
FAQs:
Q1: Are Python string comparisons case-sensitive?
A1: Yes, Python string comparisons are case-sensitive. For example, “hello” and “Hello” would not be considered equal.
Q2: Can I change the case of a Python string?
A2: Yes, Python provides various methods for altering the case of a string. For instance, the `upper()` method converts all characters to uppercase, while `lower()` converts them to lowercase.
Q3: Does Python differentiate between ‘print’ and ‘Print’?
A3: Yes, whereas ‘print’ is a valid keyword used for printing output, ‘Print’ would be considered as a user-defined identifier.
Q4: Is there any way to avoid case-sensitivity in Python?
A4: No, case-sensitivity is an inherent characteristic of the Python language. Developers need to be mindful of the casing they use when writing code.
In conclusion, Python is a case-sensitive programming language. It distinguishes between lowercase and uppercase characters in variable names, function names, module names, and keywords. Understanding this concept is crucial to avoid potential errors and inconsistencies in your Python code.
Keywords searched by users: is python case sensitive Is Python case sensitive when dealing with Identifiers, What is case sensitive does python case sensitive, Find string in list case insensitive python, Python compare string ignore case, Python replace ignore case, Lower case Python, Equal in Python, Lowercase input python
Categories: Top 35 Is Python Case Sensitive
See more here: nhanvietluanvan.com
Is Python Case Sensitive When Dealing With Identifiers
Python is one of the most popular programming languages in the world, known for its simplicity and readability. When writing code in Python, it is essential to understand its syntax and rules. One of the recurring questions that arise is whether Python is case sensitive when dealing with identifiers, specifically in the English language. In this article, we will delve into this topic and provide a comprehensive understanding of Python’s behavior regarding case sensitivity.
Python is indeed case sensitive when it comes to identifiers. An identifier is a name that we give to variables, functions, classes, or any other object in our Python code. Identifiers are essential in referencing and manipulating these objects within the program. Understanding how case sensitivity works with identifiers is crucial in avoiding errors and maintaining consistency in our code.
Every time we define an identifier in Python, we need to follow certain rules. According to the official Python documentation, identifiers are case sensitive, meaning that using different letter cases creates distinct identifiers. For instance, “myVariable” and “myvariable” would be considered two different identifiers in Python, and they represent separate objects.
Let’s consider an example to illustrate this further. Suppose we define a variable called “myVariable” and assign it a value of 10. If we try to print the value of “myvariable” (notice the lowercase ‘v’) using the print() function, we will encounter a NameError. This error occurs because Python treats “myVariable” and “myvariable” as two distinct identifiers, and the latter one has not been defined in our code.
Furthermore, it is worth noting that Python follows the same case sensitivity rules for built-in functions and keywords. Built-in functions are functions that are already defined within Python, such as print(), len(), or str(). If we incorrectly capitalize any part of a built-in function or keyword, we will encounter a SyntaxError. These errors occur because Python relies on specific syntax rules, and any deviation from them can lead to unexpected behavior or errors.
However, it is important to mention that Python is case insensitive when it comes to differentiating between variables, functions, or classes. The only distinction lies in the letter case of the identifier itself. For example, we can define a class called “MyClass” and create an instance of it using “myClass”. Python will treat these as the same entity. It is crucial to precisely match the case whenever using an identifier to access an object within the code.
Frequently Asked Questions:
Q: Does Python distinguish between uppercase and lowercase letters in identifiers?
A: Yes, Python is case sensitive when it comes to identifiers. It treats uppercase and lowercase letters as distinct entities.
Q: Can I define two identifiers with the same name but different letter cases?
A: Yes, Python allows you to define two identifiers with the same name but different letter cases. However, it is considered bad practice as it can lead to confusion and errors.
Q: What happens if I try to access an identifier using a different letter case in Python?
A: If you try to access an identifier using a different letter case than the one defined, Python will consider it a separate identifier, resulting in a NameError.
Q: Are Python keywords and built-in functions case sensitive?
A: Yes, Python keywords and built-in functions are case sensitive. You must use the exact letter case as defined in the Python documentation to avoid SyntaxErrors.
Q: Do I need to be consistent with the letter case when using an identifier in Python?
A: Yes, it is important to be consistent with the letter case when using an identifier in Python. Using different letter cases can lead to errors and make the code difficult to read and maintain.
In conclusion, Python is case sensitive when dealing with identifiers in the English language. It treats uppercase and lowercase letters as distinct entities within the code. Following the rules of case sensitivity is essential to avoid errors and maintain consistency in our Python programs. Being aware of this behavior and adhering to it will ensure that our code runs smoothly and efficiently.
What Is Case Sensitive Does Python Case Sensitive
When it comes to programming languages, one aspect that often confuses beginners is the concept of case sensitivity. Case sensitivity refers to the ability of a programming language to differentiate between uppercase and lowercase letters. In simpler terms, it means that even a small change in the case of a letter can have a significant impact on the execution and outcome of a program.
Python, being a popular and widely-used programming language, is case sensitive. This means that Python distinguishes between lowercase and uppercase letters, and treats them as distinct characters. For example, the variable “count” is different from the variable “Count”, and Python will treat them as two separate entities. This can have implications for variable names, function calls, and other elements of a Python code.
Case Sensitivity in Python – Why is it Important?
Case sensitivity plays a crucial role in programming languages, including Python. It allows programmers to differentiate between different variables, functions, and other elements used in the code. By observing the case of letters, a programmer can quickly identify if two variables are similar or different and if they hold different values or not.
Furthermore, case sensitivity helps maintain consistency and readability in the code. By following a standardized convention, such as using lowercase letters for variable names and uppercase letters for constants, programmers can create more understandable and easily maintainable code.
Consider the example below to understand the significance of case sensitivity in Python:
“`
name = “John”
Name = “Jane”
print(name) # Output: John
print(Name) # Output: Jane
“`
In the above code, even though the variable names are almost identical, Python treats them as two separate variables. This distinction can have a significant impact on the outcome of the program.
FAQs
Q1. Does Python distinguish between lowercase and uppercase strings?
Yes, Python treats lowercase and uppercase strings as distinct characters. For example, “hello” and “Hello” are considered to be two different strings.
Q2. Why is case sensitivity important in programming languages?
Case sensitivity is important in programming languages as it allows programmers to create distinct variables, functions, and other elements. It helps maintain consistency and readability in the code.
Q3. Is it necessary to follow case sensitivity in Python?
Yes, it is necessary to follow case sensitivity in Python. Failing to do so can lead to errors and unexpected outcomes in the program.
Q4. How can I avoid case sensitivity issues in Python?
To avoid case sensitivity issues, it is recommended to follow a consistent naming convention and use lowercase letters for variables and uppercase letters for constants.
Q5. Can I change the case sensitivity behavior in Python?
No, the case sensitivity behavior in Python is fixed and cannot be changed. Python treats lowercase and uppercase letters as distinct entities.
Q6. Are there any programming languages that are not case sensitive?
Yes, some programming languages, such as HTML, SQL, and BASIC, are not case sensitive. This means that they do not distinguish between lowercase and uppercase letters.
Q7. Does case sensitivity affect the performance of a Python program?
No, case sensitivity does not affect the performance of a Python program. Its impact is limited to the readability, maintainability, and correctness of the code.
Q8. Are there any disadvantages of case sensitivity in Python?
While case sensitivity in Python is generally beneficial, it can sometimes lead to confusion and potential errors if not properly managed. Programmers need to be mindful of this and ensure consistent use of case in their code.
In conclusion, Python is a case-sensitive programming language that distinguishes between lowercase and uppercase letters. Programmers must follow a consistent naming convention and be mindful of case sensitivity to avoid errors and ensure code readability.
Find String In List Case Insensitive Python
In Python, searching for a particular string in a list can be done in various ways. However, what if you want to perform a case-insensitive search? This article will guide you through the process of finding a string in a list in a case-insensitive manner using Python.
Case-insensitive search means that the search will treat uppercase and lowercase letters as the same. For example, if you search for “apple” in a list containing “apple”, “Orange”, and “bAnANA”, the search should return the match for “apple” regardless of its case.
Let’s dive into the different techniques you can use to achieve this.
Method 1: Converting the Strings to Lowercase
The simplest way to perform a case-insensitive search in Python is by converting both the search string and the items in the list to lowercase using the `lower()` method. This method returns a new string where all the characters are in lowercase.
Here’s an example:
“`python
my_list = [“apple”, “Orange”, “banana”]
search_string = “APPLE”
for item in my_list:
if item.lower() == search_string.lower():
print(“Found:”, item)
break
else:
print(“String not found in list.”)
“`
This example converts both the search string and each item in the list to lowercase before checking for a match. The `break` statement is used to exit the loop once a match is found. If the loop completes without finding a match, the “String not found in list.” message is displayed.
Method 2: Using Regular Expressions
Another approach to achieve a case-insensitive search is by using regular expressions. Python provides the `re` module, which allows for more advanced pattern matching and string manipulation.
Here’s an example using regular expressions:
“`python
import re
my_list = [“apple”, “Orange”, “banana”]
search_string = “APPLE”
pattern = re.compile(re.escape(search_string), re.IGNORECASE)
for item in my_list:
if re.match(pattern, item):
print(“Found:”, item)
break
else:
print(“String not found in list.”)
“`
In this example, the `re.escape()` function is used to escape any special characters in the search string. The `re.IGNORECASE` flag is used to perform a case-insensitive search. The `re.match()` function is used to check if the pattern matches the item in the list.
FAQs:
Q: Is the case-insensitive search method applicable only to lists?
A: No, the case-insensitive search method can be used with any iterable in Python. This includes lists, tuples, sets, and even strings.
Q: Can I modify the original list while performing a case-insensitive search?
A: Technically, you can modify the original list during the search. However, it is generally not recommended because it may lead to unexpected results or errors. It is better to perform the search on a copy of the list if you need to modify it.
Q: How can I find multiple occurrences of a string in a case-insensitive manner?
A: Both methods mentioned above will find the first occurrence of the string in the list. If you want to find multiple occurrences, you can modify the code to store the matches in a separate list or use a list comprehension.
Q: What is the difference between case-sensitive and case-insensitive search?
A: In a case-sensitive search, strings are compared based on their case. For example, “apple” and “Apple” would not be considered a match. In a case-insensitive search, the comparison is made without considering the case, so “apple” and “Apple” would be considered a match.
Q: Are there any performance differences between the two methods?
A: In general, the first method (converting to lowercase) is simpler and more efficient for most scenarios. However, if you are dealing with large datasets or complex patterns, the regular expression method may be more suitable.
Conclusion:
Performing a case-insensitive search for a string in a list can be accomplished using different techniques in Python. This article has covered two methods: converting the strings to lowercase and using regular expressions. The choice of method depends on the specific requirements of your project. With these techniques, you can efficiently search for strings in a case-insensitive manner and handle various scenarios. Remember to consider the performance implications and choose the method accordingly. Happy coding!
Images related to the topic is python case sensitive
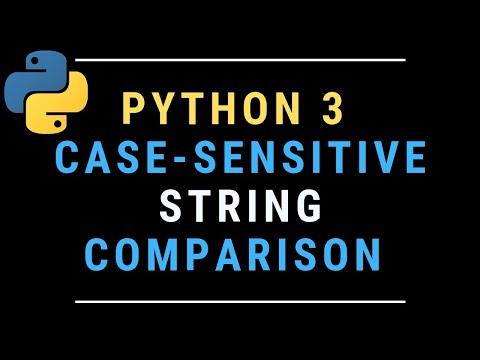
Found 33 images related to is python case sensitive theme
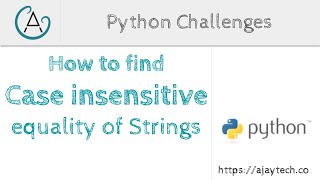

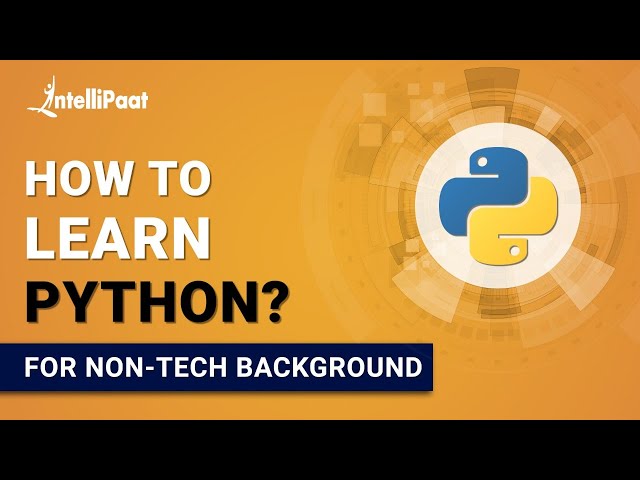
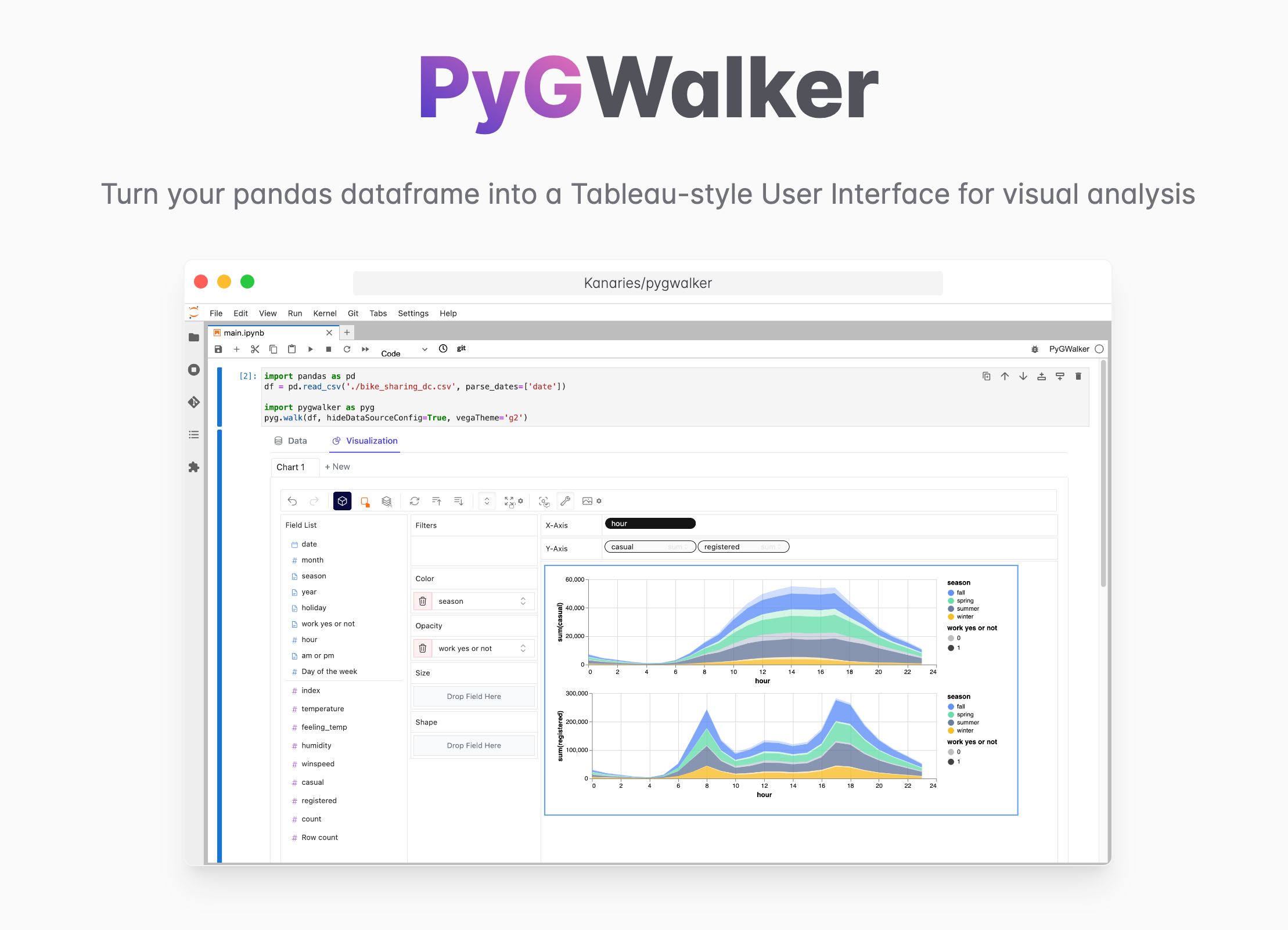
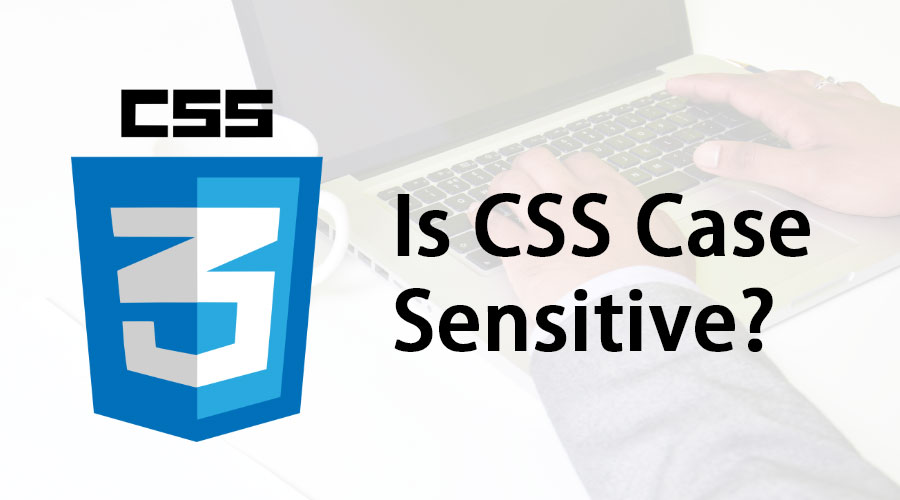



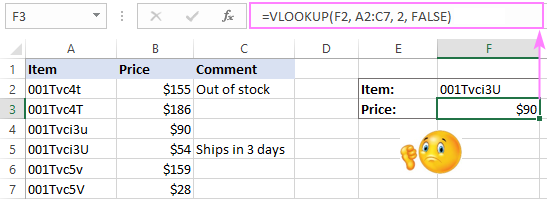

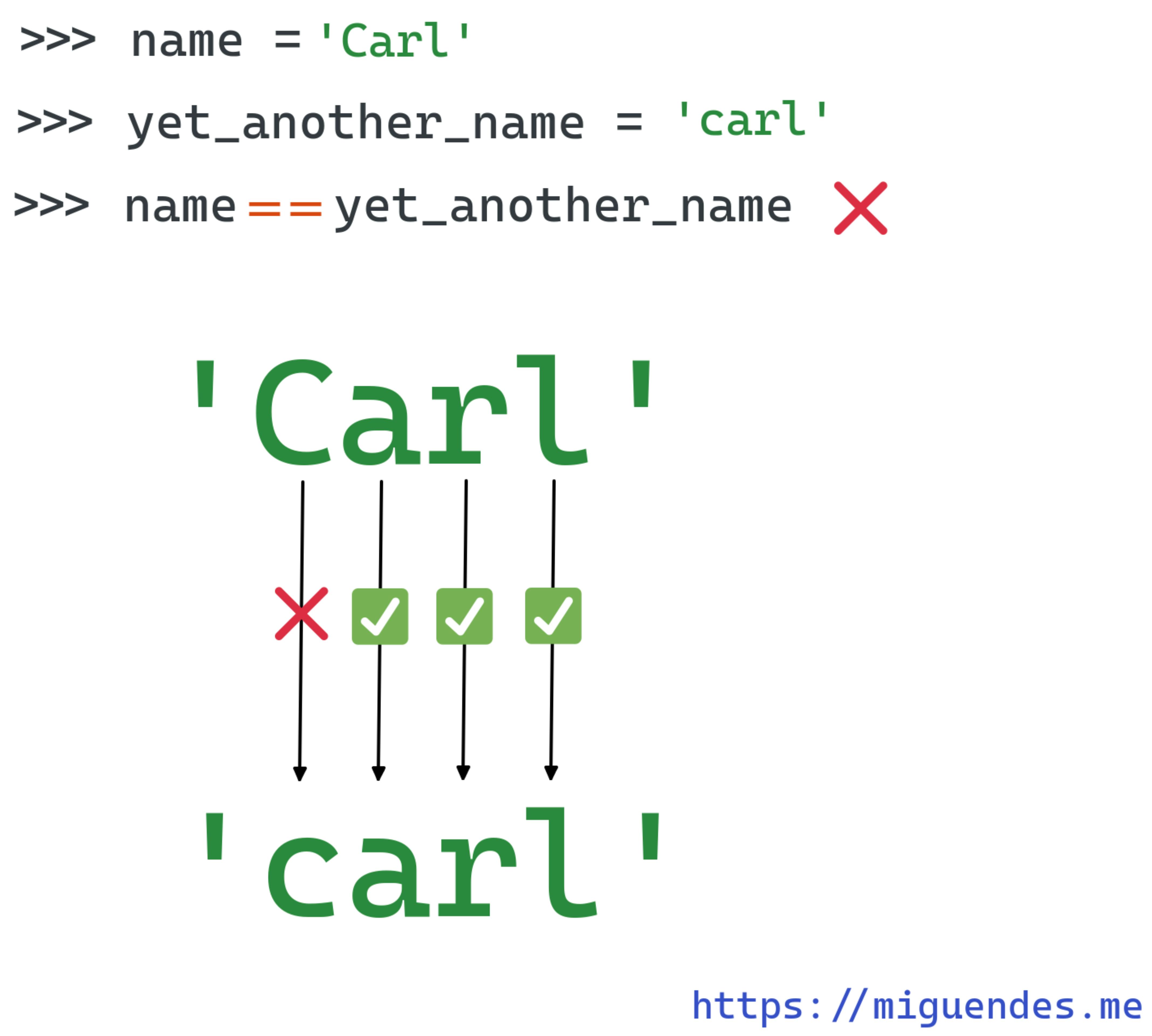
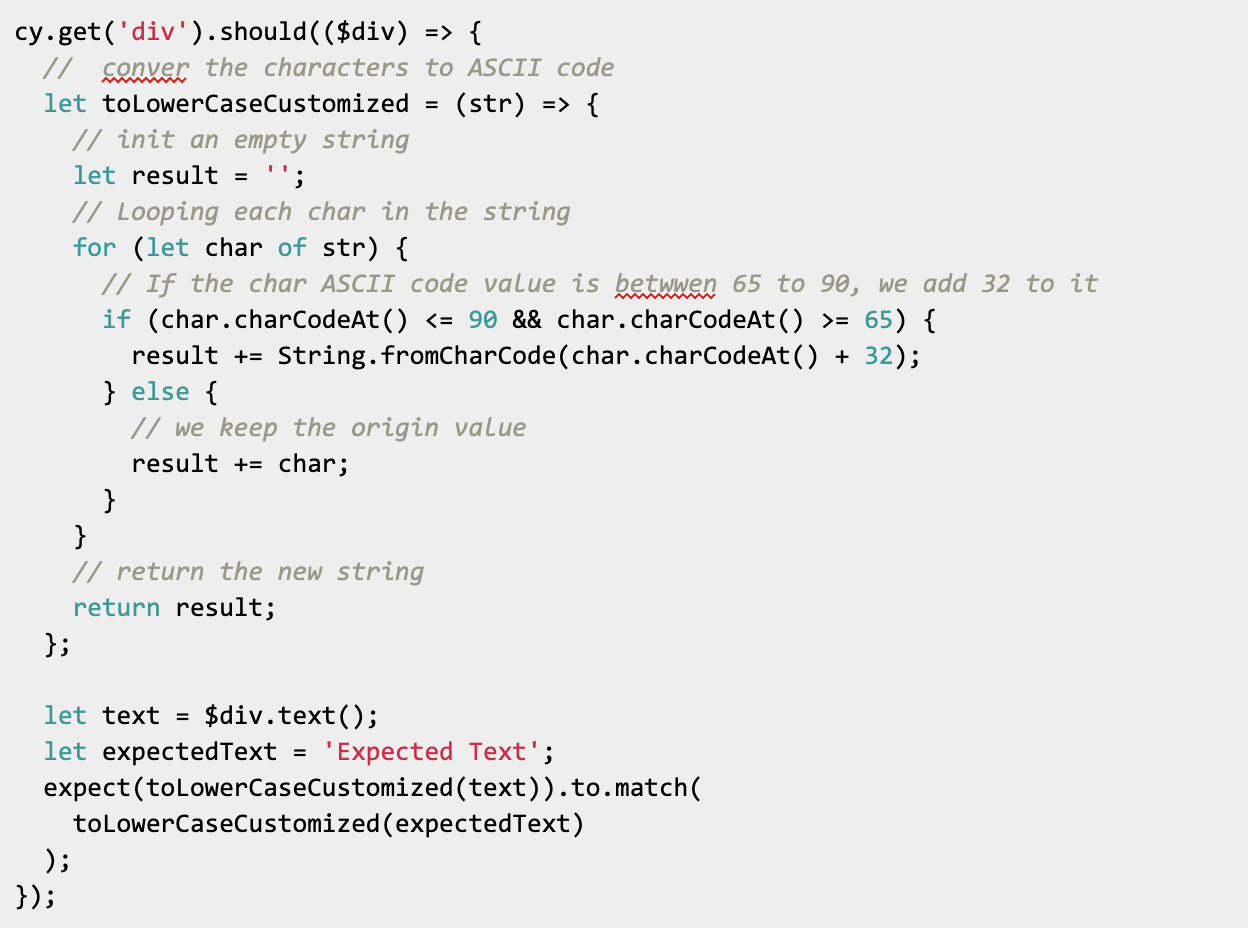
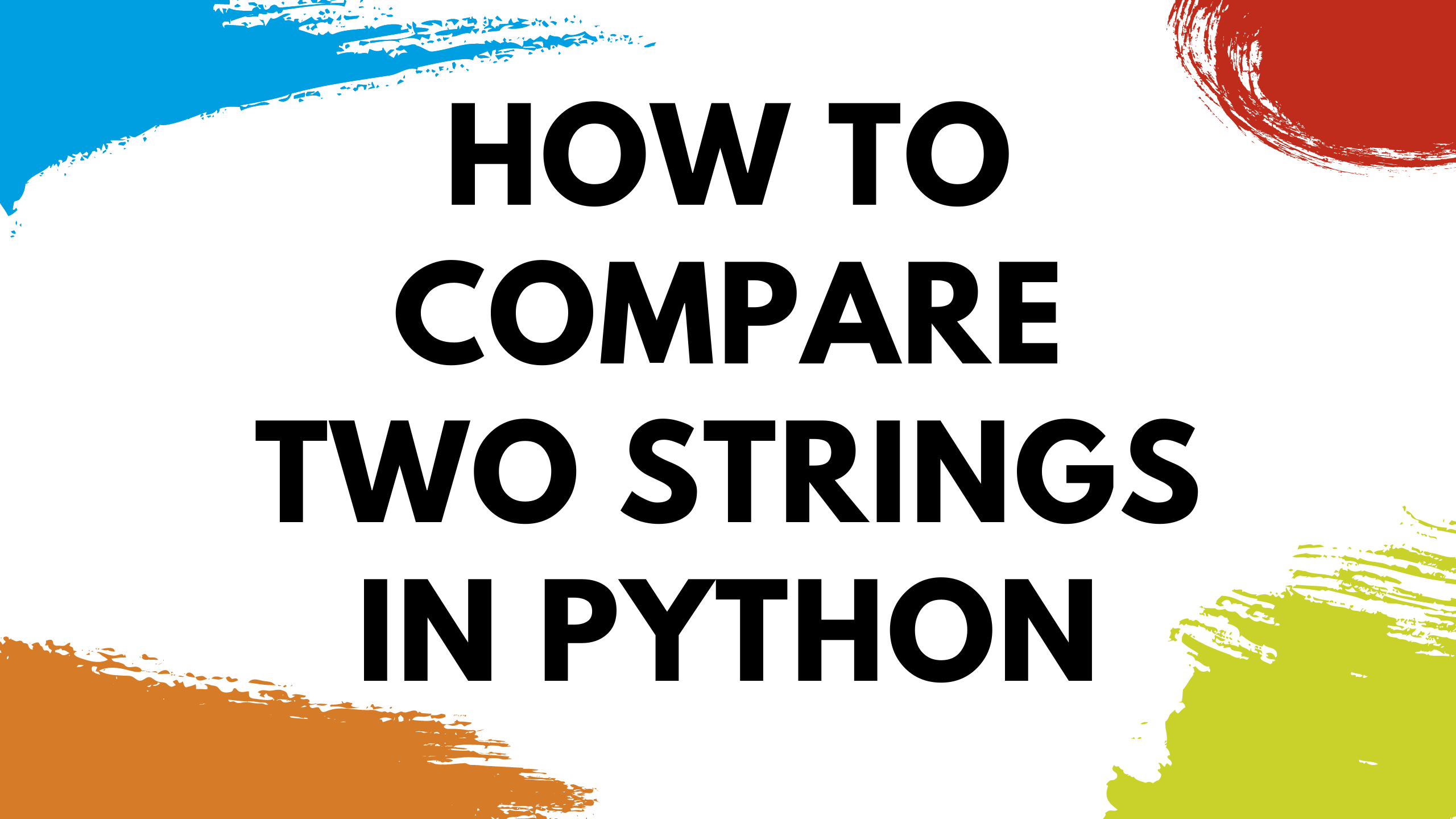
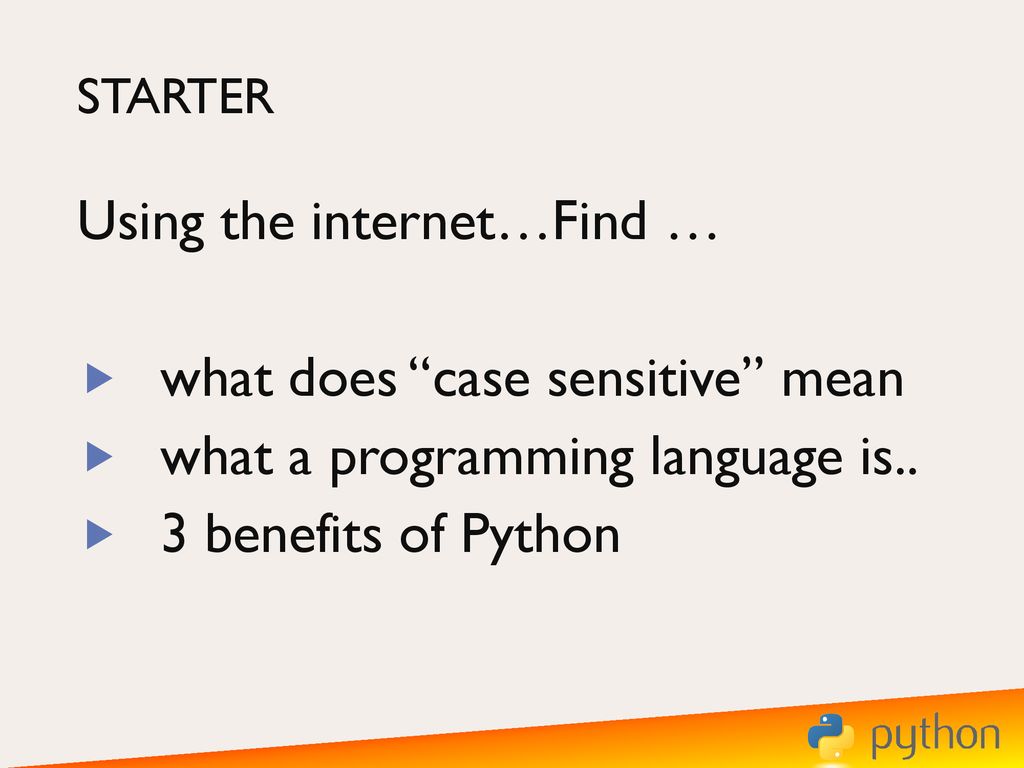
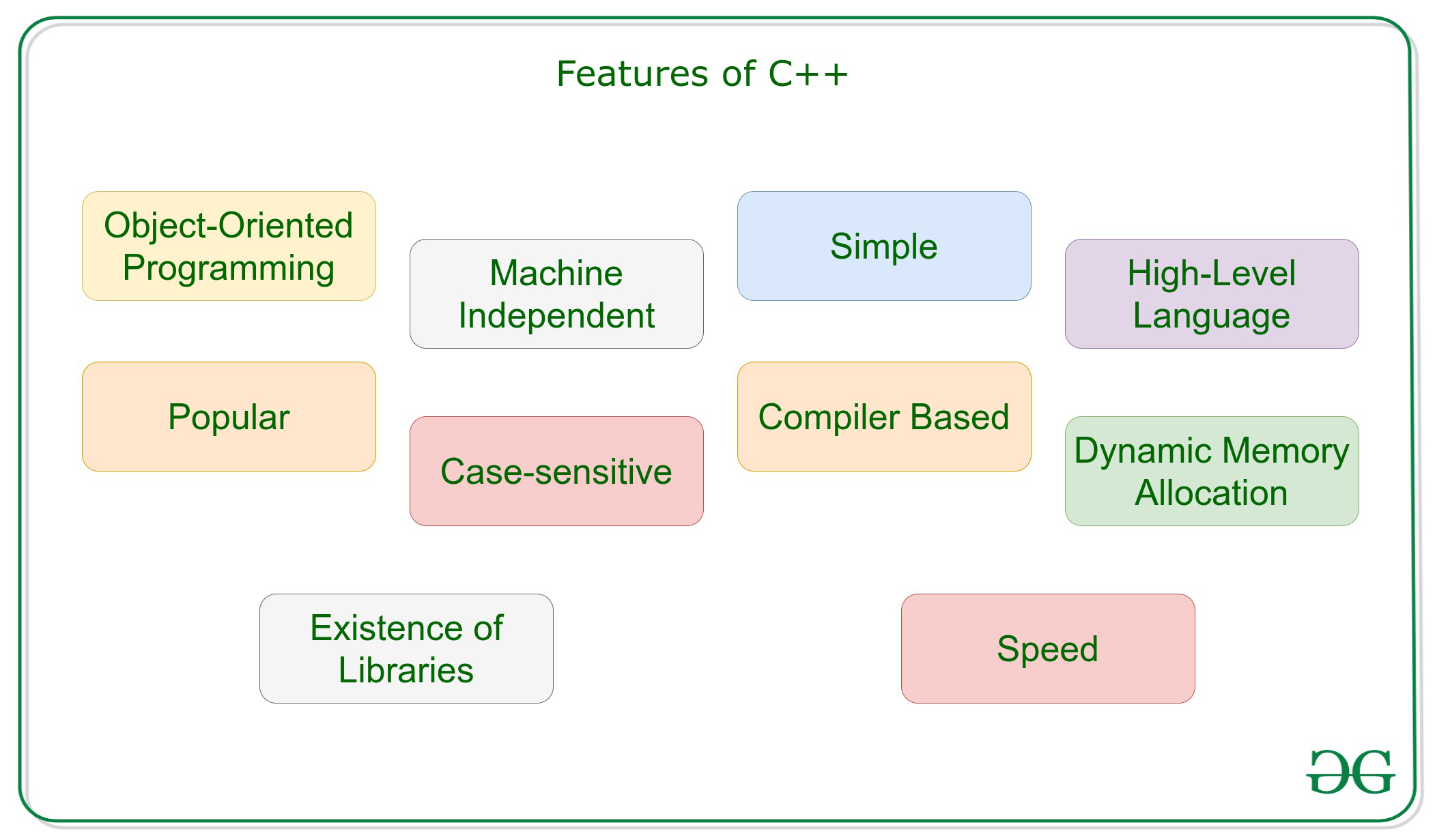

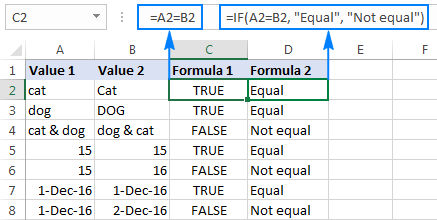
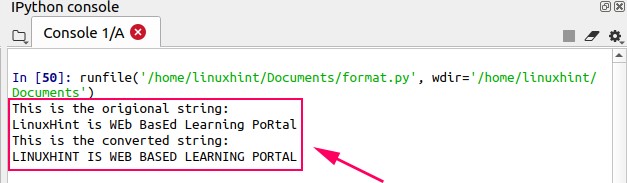
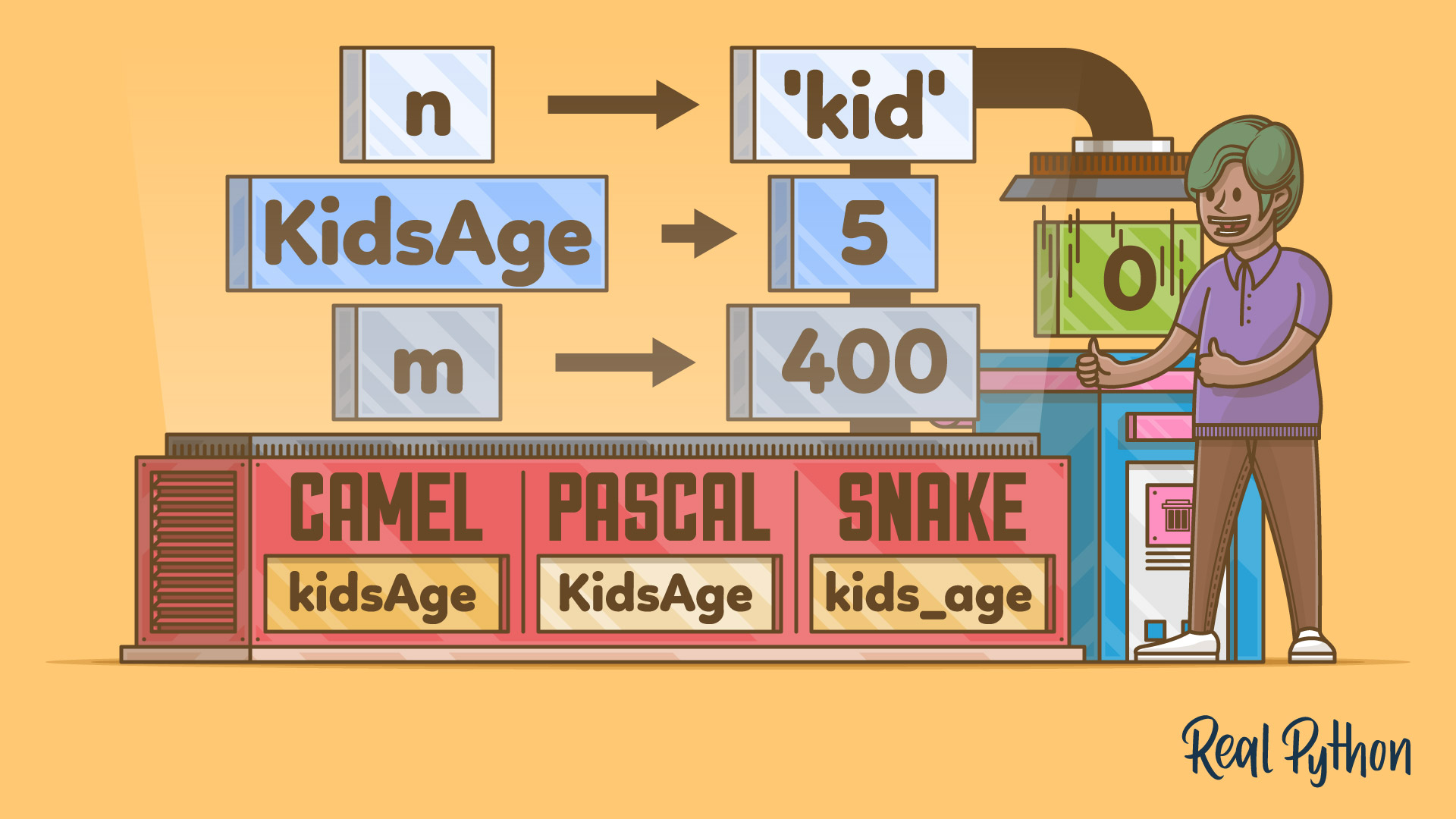

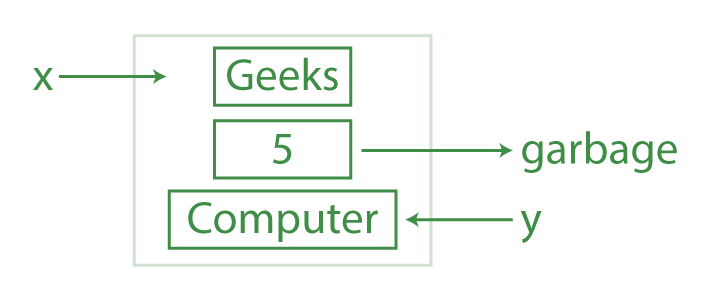

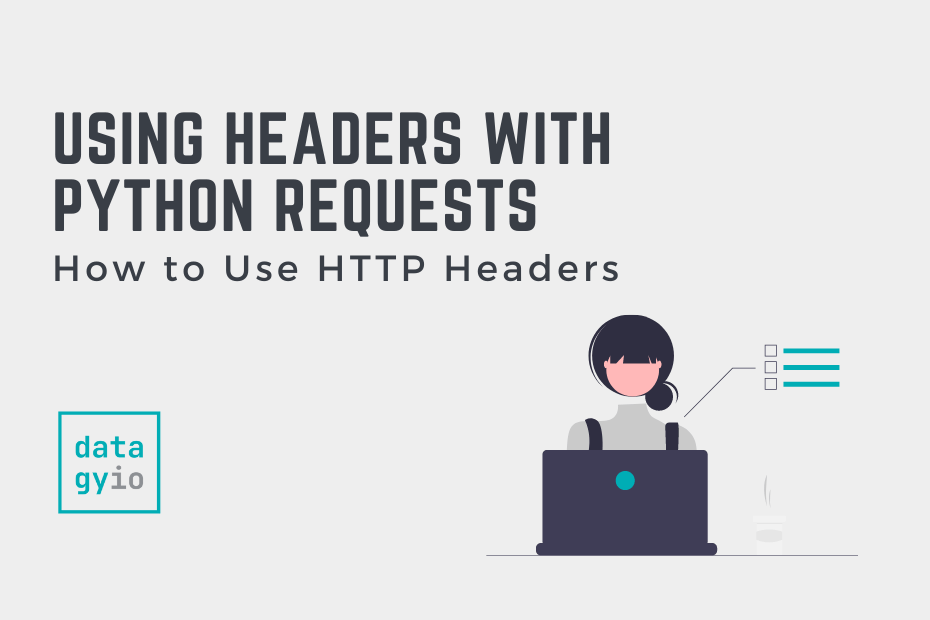
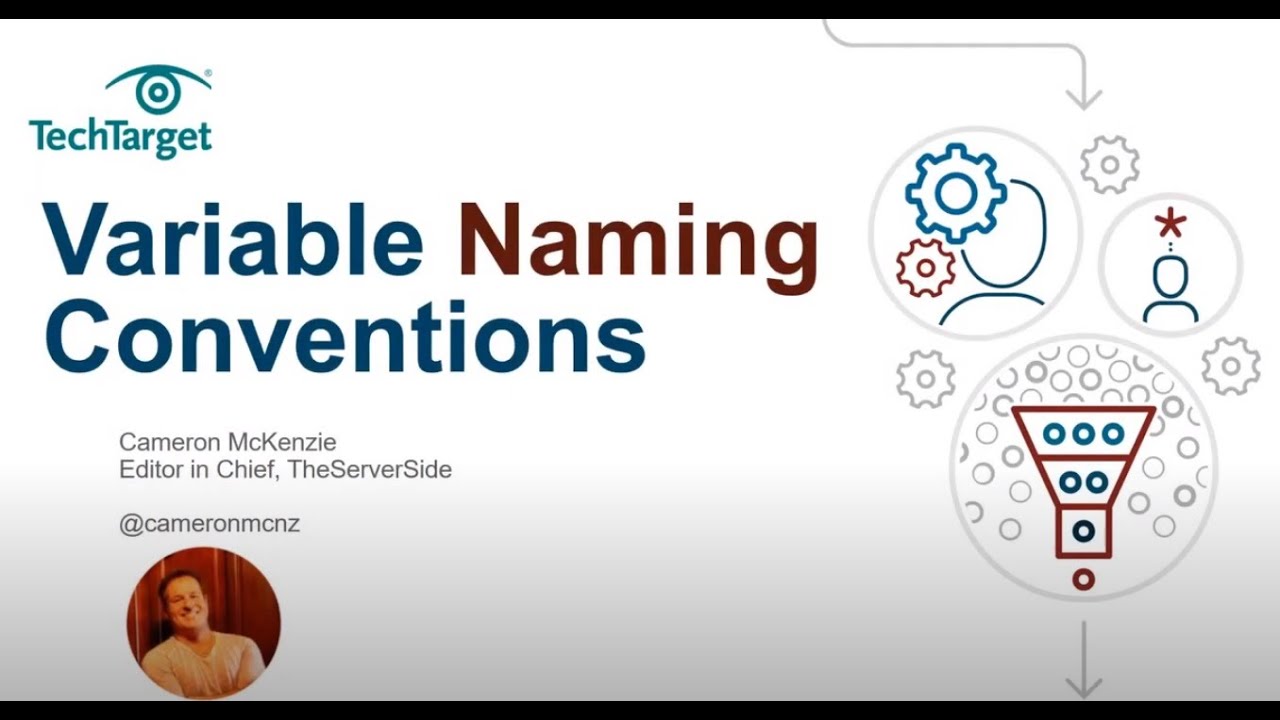
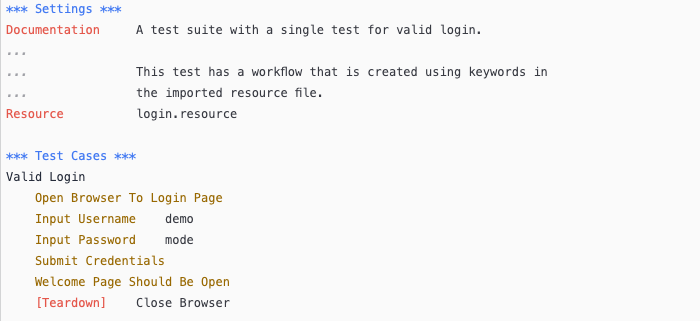
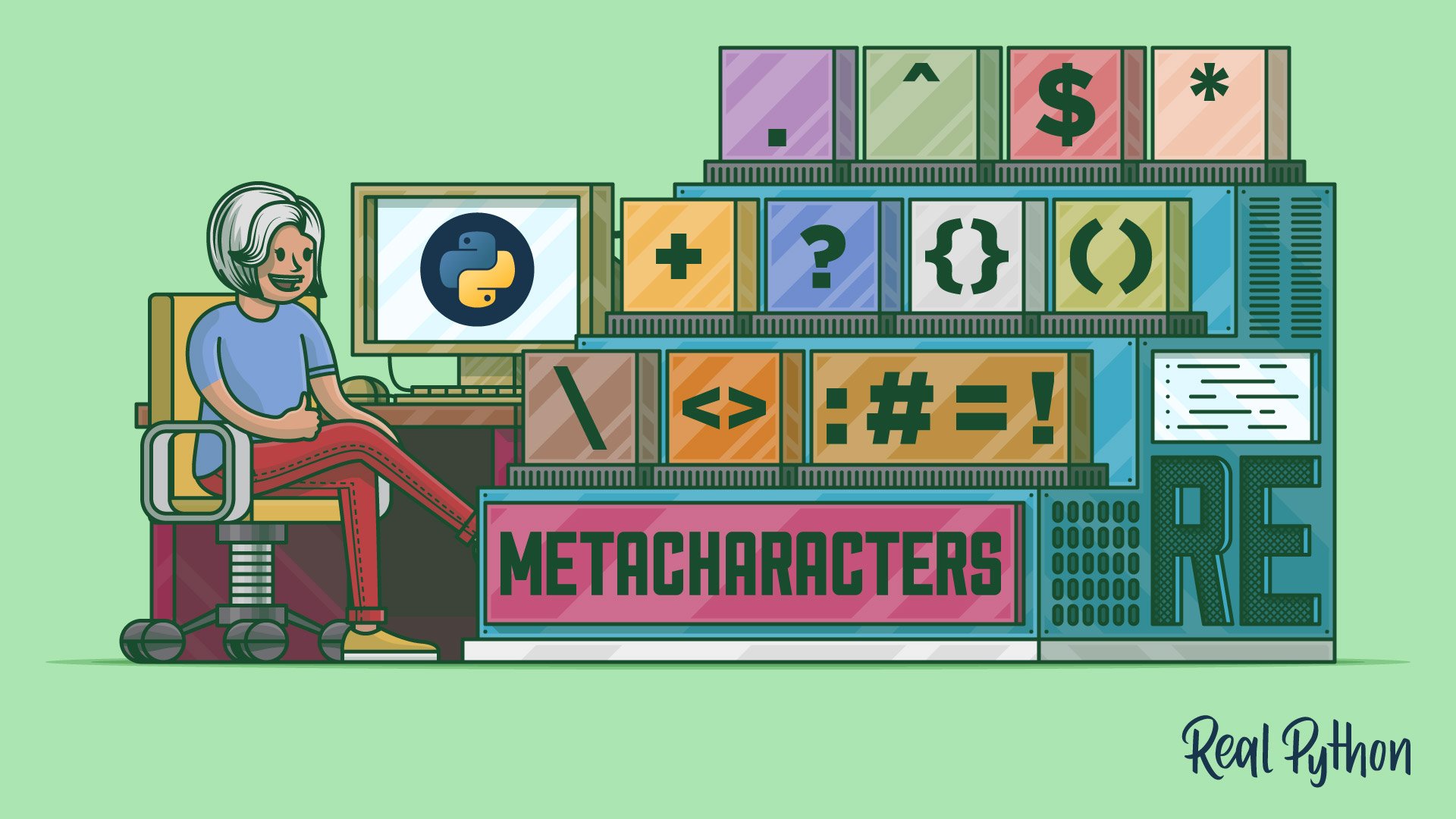

Article link: is python case sensitive.
Learn more about the topic is python case sensitive.
- Is Python case-sensitive or case-insensitive – Tutorialspoint
- Is Python Case Sensitive? – Scaler Topics
- Is Python Case Sensitive – Javatpoint
- Case sensitivity – Wikipedia
- Case Insensitive Programming Languages | Study.com
- Is Python Case-Sensitive? – LearnPython.com
- Is Python Case Sensitive – Javatpoint
- Is Python Case Sensitive? Answer, Example, & Tips
- Is Python Case Sensitive? – PrepBytes
- Is Python Case Sensitive? – Kanaries Docs
- How do I do a case-insensitive string comparison?
- Is python Case sensitive when dealing with identifiers?
See more: https://nhanvietluanvan.com/luat-hoc/