Io.Unsupportedoperation Not Readable Python
The `io.UnsupportedOperation` error is a common exception in Python that occurs when attempting to perform an unsupported I/O operation on a file or stream. This error is raised by the `io` module, which provides a consistent interface for working with different types of I/O in Python.
Explanation of the `io` Module in Python
The `io` module in Python is part of the standard library and provides the classes and functions necessary for performing input and output operations. It includes classes such as `TextIOWrapper` for working with text files, `BufferedReader` for reading binary data, and `BytesIO` for working with in-memory byte streams.
Understanding the `UnsupportedOperation` Class
The `UnsupportedOperation` class is a subclass of the built-in `io.IOError` exception. It is raised when an unsupported I/O operation is performed, such as trying to read from a file opened in write-only mode or trying to write to a closed file.
Causes of the `io.UnsupportedOperation` Error
There are several causes that can lead to the `io.UnsupportedOperation` error:
1. Attempting to read from a file that was opened in write-only mode.
2. Trying to write to a file that was opened in read-only mode.
3. Performing a seek operation on a file that does not support random access, such as a network socket.
4. Calling `flush()` on a non-writable stream.
5. Trying to close a file that has already been closed.
Common Scenarios leading to the `io.UnsupportedOperation` Error
1. Opening a file in write-only mode and then attempting to read from it without closing and reopening it in read mode.
2. Writing to a file that was opened in read-only mode.
3. Performing a seek operation on a network socket or a pipe.
4. Calling `flush()` on a file that was opened with the `read` mode.
5. Closing a file multiple times or trying to perform any operation on a closed file.
Handling the `io.UnsupportedOperation` Error
To handle the `io.UnsupportedOperation` error, you can use a try-except block to catch the exception and handle it appropriately. For example:
“`python
try:
# Perform the unsupported operation
except io.UnsupportedOperation as e:
# Handle the exception
“`
In the except block, you can log the error, display a user-friendly error message, or take any other necessary action based on your specific needs.
Tips for Debugging the `io.UnsupportedOperation` Error
1. Check the mode in which the file was opened. Ensure that you are performing the correct operation based on the file mode.
2. Verify that the file or stream is in the expected state. For example, check if the file has been closed before attempting to perform any operations on it.
3. Review the code leading up to the error and check for any potential mistakes or incorrect assumptions about the file or stream.
4. Use print statements or a debugger to inspect the values of variables and objects involved in the I/O operation.
Best Practices for Avoiding the `io.UnsupportedOperation` Error
1. Always ensure that you open a file in the appropriate mode for the operations you intend to perform.
2. Double-check the file state before performing any I/O operations on it. For example, verify if the file is closed or if it supports seek operations.
3. Consider using context managers (`with` statement) when working with files to ensure proper handling of file resources and avoid potential issues with closing files.
4. Handle any exceptions raised during I/O operations gracefully to prevent the program from terminating unexpectedly.
5. Follow best practices for file handling, such as closing files when they are no longer needed and using appropriate error handling techniques.
In conclusion, the `io.UnsupportedOperation` error is a common exception in Python that occurs when performing unsupported I/O operations on files or streams. Understanding the causes of this error and following best practices for file handling can help avoid and handle the exception effectively.
Io Unsupportedoperation Not Writable- Solved
Why Is My File In Python Not Readable?
Python is a versatile programming language widely used for various purposes, including file input and output operations. However, sometimes you may encounter issues with reading a file in Python. This can be frustrating, especially when you have invested time and effort into creating or obtaining the file. In this article, we will explore some common reasons why your file in Python may not be readable, as well as discuss possible solutions to resolve these issues.
1. Incorrect File Path:
One of the most common reasons why a file may not be readable in Python is due to an incorrect file path. When providing the file path to Python, it is crucial to ensure that you have specified the correct directory location and file name. Even a minor mistake such as a misspelled file name or missing directory can lead to a read error. Therefore, double-checking the file path by examining the directory structure and file name can help rectify this issue.
2. File Permissions:
Another reason why a file may not be readable is due to inadequate file permissions. In some cases, the file you are trying to read might have restricted access rights set up by the operating system or the file owner. If the file’s permissions are set to deny read access to certain users or groups, Python would not be able to read the file. To address this, you will need to ensure that you have the necessary read permissions for the file. On Unix-like systems, you can use the “chmod” command to modify the file permissions and grant yourself the required access.
3. File Format:
File formats play a crucial role in determining if a file can be read by Python. Although Python supports a wide variety of file formats, it might encounter difficulties reading certain file types that are not supported or require specific libraries. For instance, if you are trying to read an Excel spreadsheet file (.xlsx), you will need to install the “pandas” library to handle this format. Similarly, handling PDF files requires the installation of the “PyPDF2” or “pdfminer” libraries. Checking if the file format you are attempting to read is supported by Python or installing the appropriate libraries can resolve such issues.
4. Corrupted File:
A corrupted file may also prevent Python from successfully reading it. Corruption can occur due to various reasons, such as incomplete downloads, hardware failures, or software issues. If you suspect that your file may be corrupted, you can try opening it with other software applications to determine if the issue persists. In case the file is indeed corrupted, you might need to consider obtaining a new, uncorrupted version of the file.
5. Outdated Python or Libraries:
Compatibility issues may arise if you are using an outdated version of Python or the required libraries. Python and its libraries are regularly updated to fix bugs and introduce new features, and using an outdated version might prevent you from reading certain files. To ensure that you can read files seamlessly, it is recommended to update both Python and the relevant libraries to their latest versions. This can be done by using the package manager (e.g., pip) to install the latest updates.
6. Encoding Issues:
Python assumes a default encoding when reading a file, usually ASCII or UTF-8. However, if the file you are attempting to read has a different encoding, it may result in unreadable data or even raise encoding-related errors. In such cases, explicitly specifying the file encoding when opening the file can resolve this issue. For example, to read a file in UTF-16 encoding, you can use the following code: “open(‘file.txt’, encoding=’utf-16′)”.
Now that we have explored some common reasons why a file may not be readable in Python, let’s address a few frequently asked questions (FAQs):
FAQs:
Q: Why do I receive a “FileNotFoundError” even though I’m certain the file exists?
A: Double-check the file path, making sure it contains the correct directory and file name. Remember that Python is case-sensitive, so ensure the case matches the file exactly.
Q: How do I change file permissions in Python?
A: Python does not provide direct methods to change file permissions. You can use platform-specific commands, such as “chmod” on Unix-like systems, or execute terminal commands from within Python using the “subprocess” module.
Q: I have installed the required libraries, but still cannot read the file. What should I do?
A: Ensure that you have imported the required libraries in your Python script using the “import” statement. Also, confirm that the libraries are correctly installed by checking their version and verifying if any errors occur during the import process.
Q: How can I determine the encoding of a file?
A: You can use the “chardet” library in Python to automatically detect the encoding of a file. By analyzing the file contents, “chardet” can provide an estimation of the most likely encoding used.
In conclusion, there are various factors that may prevent Python from reading a file. By thoroughly examining the file path, permissions, file format, and encoding, you can troubleshoot and resolve most reading issues. Regularly updating Python and relevant libraries, as well as verifying file integrity, can also contribute to a successful file reading experience in Python.
What Is Unsupported Operation Unreadable?
In the world of software development, encountering error messages is not an uncommon occurrence. These error messages can vary in complexity, but one that often leaves users perplexed is the “Unsupported Operation: Unreadable” error. What does it mean? Why does it appear? And most importantly, how can it be resolved? In this article, we will delve into the depths of this error message, providing a comprehensive understanding to bring clarity to this technical conundrum.
Understanding Unsupported Operation: Unreadable
The “Unsupported Operation: Unreadable” error is an error message that frequently appears when attempting to perform a certain action on a file or object. It indicates that the operation being executed is not supported, and as a result, the file or object being accessed cannot be read. This error message is often encountered in programming languages, such as Java or Python, where it is thrown as an exception when an inappropriate operation is attempted on an object.
Causes of the Unsupported Operation: Unreadable Error
There are several reasons why this error message may appear. Let’s explore some of the common causes:
1. Incompatible File Formats: One possible cause of this error is attempting to read a file in a format that is not compatible with the current system or application. For example, opening a Microsoft Word document (.docx) using a text editor may trigger this error due to incompatibility issues.
2. Insufficient Permissions: Another common cause is inadequate user permissions. If the user does not have the necessary rights to read a particular file or access an object, the “Unsupported Operation: Unreadable” error may arise.
3. Corrupted Files: Corrupted files can lead to various errors, including the “Unsupported Operation: Unreadable” error. If the file being accessed is damaged or incomplete, it becomes unreadable and triggers this error message.
4. Software Bugs: Like any piece of software, bugs can creep into applications or programming languages. These bugs can manifest as the “Unsupported Operation: Unreadable” error when executing specific operations.
Resolving the Unsupported Operation: Unreadable Error
Now that we have gained an understanding of what triggers this error message, let’s explore some potential solutions for resolving it:
1. Check File Compatibility: Ensure that the file being accessed is in a supported format and compatible with the application or system you are using. If necessary, use appropriate file conversion tools to convert the file into an acceptable format.
2. Grant Sufficient Permissions: Make sure that you have the necessary permissions to access and read the file or object. Contact the system administrator or file owner to grant appropriate permissions if needed.
3. Repair or Replace Corrupted Files: If the error is being caused by a corrupted file, attempt to repair it using file recovery tools or restore it from a backup. If the file cannot be repaired, you may need to obtain a new copy.
4. Update Software or Programming Language: If the error is occurring due to a bug in the software or programming language, check for updates or patches. Developers often release fixes to address such issues. Applying the latest updates may resolve the error message.
5. Seek Technical Support: If all else fails, it is advisable to seek technical assistance from the software provider, application developer, or programming language community. They might have specific insights or solutions for the issue you are encountering.
Frequently Asked Questions (FAQs):
Q1. Can I fix the “Unsupported Operation: Unreadable” error myself?
A1. Yes, in many cases, you can resolve the error by following the steps mentioned above. However, complex scenarios may require professional assistance, so do not hesitate to seek technical support if needed.
Q2. Why am I receiving this error when I try to open some files but not others?
A2. This error occurs because certain files have incompatible formats or are corrupted, making them unreadable. Other files with compatible formats or no corruption will not trigger this error.
Q3. Are there any preventive measures to avoid encountering this error?
A3. To minimize the chances of encountering this error, ensure that your software, applications, and programming languages are up to date. Additionally, regularly back up your files to mitigate the risk of corruption.
Q4. Does the “Unsupported Operation: Unreadable” error only occur in programming languages?
A4. No, while this error is often encountered in programming languages, it can also appear in various software applications when attempting to perform unsupported operations on certain files or objects.
Q5. Can this error be caused by hardware issues?
A5. Although this error is primarily related to software or file compatibility issues, hardware problems, such as faulty storage devices or connectivity issues, may indirectly contribute to the error, leading to reading difficulties.
In conclusion, the “Unsupported Operation: Unreadable” error is a message that indicates an unsupported operation or an inability to read a file or object due to various causes. By understanding the potential triggers and employing the suggested solutions, you can effectively troubleshoot and resolve this error message, bringing your operations back on track. Remember to seek professional assistance if needed, as some cases may require specialized knowledge or expertise to achieve a resolution.
Keywords searched by users: io.unsupportedoperation not readable python Python not readable, Io unsupportedoperation not writable json python, Io UnsupportedOperation not writable, I/O operation on closed file, File io python, Overwrite in python, Python io, A bytes-like object is required, not ‘str
Categories: Top 30 Io.Unsupportedoperation Not Readable Python
See more here: nhanvietluanvan.com
Python Not Readable
However, in order to truly appreciate the power and flexibility of Python, one must venture beyond its straightforward English-readable syntax. Python also supports a range of non-English characters, making it capable of handling languages from around the world. In this article, we will explore the concept of Python not being readable in English and delve into how it can be used effectively with non-English languages.
Python’s Extensive Unicode Support:
Python is built on the Unicode standard, which allows programmers to work with a vast array of characters from different languages and scripts. Unicode support means that Python can handle various languages, including but not limited to Chinese, Arabic, Hindi, Russian, and many more.
In Python, strings are represented using the Unicode character encoding, which assigns unique code points to each character. This ensures that characters from any language can be stored and processed correctly. Unlike some other programming languages, Python doesn’t require any additional libraries or modules to handle Unicode characters, as it is built-in.
Non-English Variable Names:
One of the most interesting aspects of Python’s support for non-English languages is the ability to use non-English variable names. In Python, variable names can consist of any character from the Unicode character set, including those from non-English languages. This allows developers to choose variable names that are relevant and meaningful in their native language, making the code more expressive and easier to understand.
For example, a developer who speaks Spanish might use variable names like “nombre” (name), “edad” (age), or “ciudad” (city) in their code. Similarly, a programmer familiar with Hindi could use variable names like “नाम” (name), “आयु” (age), or “शहर” (city). These non-English variable names can enhance the readability and maintainability of code for developers who are more comfortable with their native language than English.
Writing Non-English Comments:
Python also allows programmers to write comments in non-English languages. Comments are informative lines of text that are inserted within the code but are not executed or interpreted by the compiler or interpreter. They serve as explanatory notes to help developers understand the code’s functionality.
By allowing comments in non-English languages, Python fosters inclusivity and enables developers from different linguistic backgrounds to collaborate effectively. Non-English comments can be particularly useful when working on international projects or collaborating with teams from non-English-speaking countries.
Non-English Documentation:
Python has an extensive ecosystem of libraries and modules, many of which are developed and maintained by open-source communities worldwide. These communities often create documentation to help users understand and utilize their libraries effectively.
Just as Python supports non-English variable names and comments, it also encourages non-English documentation. Developers can write documentation in their native language to facilitate the adoption and understanding of their libraries, especially in regions and communities where English is not widely spoken or understood.
FAQs:
Q: Is it recommended to use non-English in Python code?
A: While Python supports non-English characters in variable names, comments, and documentation, it is generally recommended to use English for consistency and collaboration reasons. English is considered the lingua franca of programming, and using it as a common language makes it easier for developers from different backgrounds to understand and work on the codebase. Moreover, using English also minimizes the risk of encountering compatibility issues with certain tools or libraries that might not fully support non-English characters.
Q: Are there any disadvantages to using non-English in Python code?
A: Using non-English in Python code can lead to several challenges. First, it may make the code less accessible to developers who are not fluent in the specific non-English language used. This can hinder collaboration and limit the pool of potential contributors or maintainers for a project. Additionally, non-English code may require special settings or configurations to properly handle and display non-English characters, which can add complexity and potential compatibility issues.
Q: Can Python handle non-English user input?
A: Absolutely! Python can handle and process non-English user input with ease. Since Python uses Unicode for string handling, it can handle input in any language, provided the necessary encodings or decoding mechanisms are correctly implemented. This makes Python an excellent choice for developing multilingual applications or those that require support for specific languages.
In conclusion, Python’s support for non-English languages is a testament to its versatility and flexibility as a programming language. Through its extensive Unicode support, the ability to use non-English variable names, comments, and documentation, Python enables developers worldwide to express their ideas and concepts effectively. While caution should be exercised in using non-English in code to ensure collaboration and accessibility, Python’s inclusivity sets it apart from many other programming languages.
Io Unsupportedoperation Not Writable Json Python
JSON (JavaScript Object Notation) is a widely used data interchange format that provides a lightweight and readable way to store and transmit data between different systems. In Python, the JSON module provides functions to encode and decode JSON data by parsing it into Python objects and converting Python objects into JSON format.
However, while working with JSON in Python, you may encounter the `IOError: UnsupportedOperation: not writable` error. This error typically occurs when you try to write JSON data to a file or stream that is opened in read-only mode or is not writable. In this article, we will delve deep into the causes behind this error and explore possible solutions to fix it.
Causes of `IOError: UnsupportedOperation: not writable`
1. Read-Only File: One possible cause of this error is when you attempt to write JSON data to a file that is opened in read-only mode. When opening a file in Python, you can specify the mode as `’r’` for read-only, `’w’` for write-only (which overwrites the existing file content), `’a’` for append (which appends data at the end of the existing file), or `’x’` for exclusive creation (which creates a new file if it doesn’t exist). If you try to write to a file that is opened in read-only mode, the `IOError` is raised.
2. File Permissions: Another possibility is that the file you are attempting to write to does not have the required write permissions. In certain cases, files may be set to read-only mode due to system configurations or permissions imposed by the file owner. If you are working with a file that is read-only, you will not be able to write JSON data to it, resulting in the `IOError` being raised.
3. Invalid File Object: If you are using a file object that does not support writing operations, such as a file object that is opened with `’r’` mode or a stream that is not writable, the `IOError: UnsupportedOperation: not writable` error may occur when trying to write JSON data.
Solutions and Workarounds
1. File Mode: Make sure that the file you are attempting to write to has been opened in a write mode (e.g., `’w’`, `’a’`, or `’x’`). You can use the `open()` function in Python to open a file and specify the appropriate mode. For example, if you want to open a file named “data.json” in write mode, use `open(“data.json”, “w”)`. If the file already exists, this will overwrite its contents, and if it doesn’t exist, a new file will be created.
2. File Permissions: Check the file permissions to ensure that the file is not set to read-only mode. On most operating systems, you can modify file permissions using the `chmod` command. For example, in Unix-based systems, you can run `chmod +w filename` to grant write permissions to the file. If you do not have the necessary permissions to modify the file, consider working with a different file or requesting the required permissions.
3. Check File Object: If you are encountering this error while using a file object, ensure that you have opened the file with write mode or an append mode. Check if the file object is writable using the `writable()` method before attempting to write JSON data to it. If the file object does not support writing, consider creating a new file object with the appropriate mode.
Frequently Asked Questions (FAQs)
Q1. Can I write JSON data to a file opened in read mode?
No, you cannot write JSON data to a file that is opened in read mode. The file must be opened in a write mode (e.g., `’w’`, `’a’`, or `’x’`) to enable writing operations.
Q2. How can I change the file mode to make it writable?
You can change the file mode to make it writable by modifying its permissions. On Unix-based systems, you can use the `chmod` command and grant write permissions to the file by running `chmod +w filename`. However, note that you need appropriate permissions to modify the file.
Q3. What should I do if I cannot change the file permissions?
If you do not have the necessary permissions to modify the file permissions, consider working with a different file or requesting the required permissions. Alternatively, you can write JSON data to a different file that you have proper access to.
Q4. How can I check if a file object is writable?
You can check if a file object is writable by using the `writable()` method. For example, if you have a file object named `file_obj`, you can check its writability using `file_obj.writable()`. This method returns `True` if the file object is writable and `False` otherwise.
Conclusion
The `IOError: UnsupportedOperation: not writable` error in Python occurs when you try to write JSON data to a file or stream that is opened in read-only mode or doesn’t have required write permissions. This article covered the possible causes of this error and provided solutions, including opening the file in write mode, modifying file permissions, or checking the file object’s writability. By applying these solutions, you can successfully write JSON data to files or streams.
Io Unsupportedoperation Not Writable
Introduction:
The Io programming language is known for its simplicity and conciseness. It provides powerful features that allow developers to write code in a highly flexible and dynamic manner. However, like any programming language, Io has its limitations. One such limitation is the Io.UnsupportedOperation: not writable error. In this article, we will explore the reasons behind this error, its implications for developers, and provide solutions to common issues related to it.
Understanding Io.UnsupportedOperation: not writable error:
The Io.UnsupportedOperation: not writable error occurs when attempting to perform an operation that modifies an object that is not writable. In Io, objects can have different levels of mutability, ranging from completely mutable to completely immutable. When an object is not writable, it means that any attempt to modify its state will result in this error.
Potential causes of Io.UnsupportedOperation: not writable error:
1. Immutable Object: The most common cause of this error is attempting to modify an immutable object. Immutable objects are those whose state cannot be changed once they are created. In Io, objects can be made immutable using the “immutable” method. Trying to modify such objects will trigger the unsupported operation error.
2. Predefined Objects: Certain Io objects, such as numbers and strings, are predefined and hard-coded to be immutable. While they can be used as values, any attempt to modify them directly will result in the unsupported operation error. To modify these objects, you must create a new object with the desired changes.
Handling Io.UnsupportedOperation: not writable error:
1. Check Object Mutability: Before modifying an object, check its mutability using the “isWritable” method. If the object is not writable, consider creating a new instance with the desired changes instead of modifying the existing object.
2. Immutable Objects: If an object is intentionally made immutable, refrain from modifying it directly. Instead, create a new object with the desired changes based on the immutable object.
3. Object Cloning: In case you need to modify an object, but its mutability is restricted, you can create a clone of the original object using the “clone” method. Make your modifications on the clone and use it accordingly.
Frequently Asked Questions:
Q1. Why does Io have immutable objects?
A1. Immutable objects guarantee that their state will not change during program execution, providing benefits such as improved concurrency, simpler debugging, and better code optimization.
Q2. Can I make an object writable after it has been created as immutable?
A2. No, once an object is created as immutable, its state cannot be modified. To make changes, create a new object with the updated properties.
Q3. How can I identify if an object is writable or not?
A3. You can use the “isWritable” method to check if an object is writable.
Q4. Can I modify predefined objects, such as numbers and strings?
A4. No, predefined objects in Io are immutable. Attempting to modify them directly will result in the unsupported operation error.
Q5. Are there any performance advantages to using immutable objects in Io?
A5. Yes, since immutable objects cannot change their state, they can be safely shared across multiple threads, making concurrency management easier and potentially leading to better performance.
Q6. What is the difference between cloning an object and creating a new one?
A6. Cloning creates a new object with the same state as the original, allowing you to modify the clone without affecting the original. Creating a new object refers to constructing an entirely new object with desired initial values.
Conclusion:
The Io.UnsupportedOperation: not writable error occurs when attempting to modify an object that is not writable. Understanding the causes and solutions to this error is crucial for Io developers. By following best practices, checking object mutability, and utilizing appropriate methods like object cloning, developers can avoid this error and write efficient code in Io. Embracing immutability can also lead to improved performance and simplify concurrent programming in Io.
Images related to the topic io.unsupportedoperation not readable python
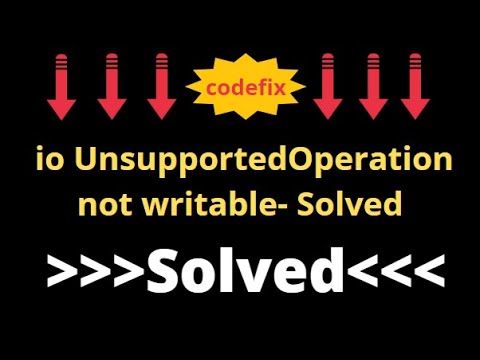
Found 48 images related to io.unsupportedoperation not readable python theme
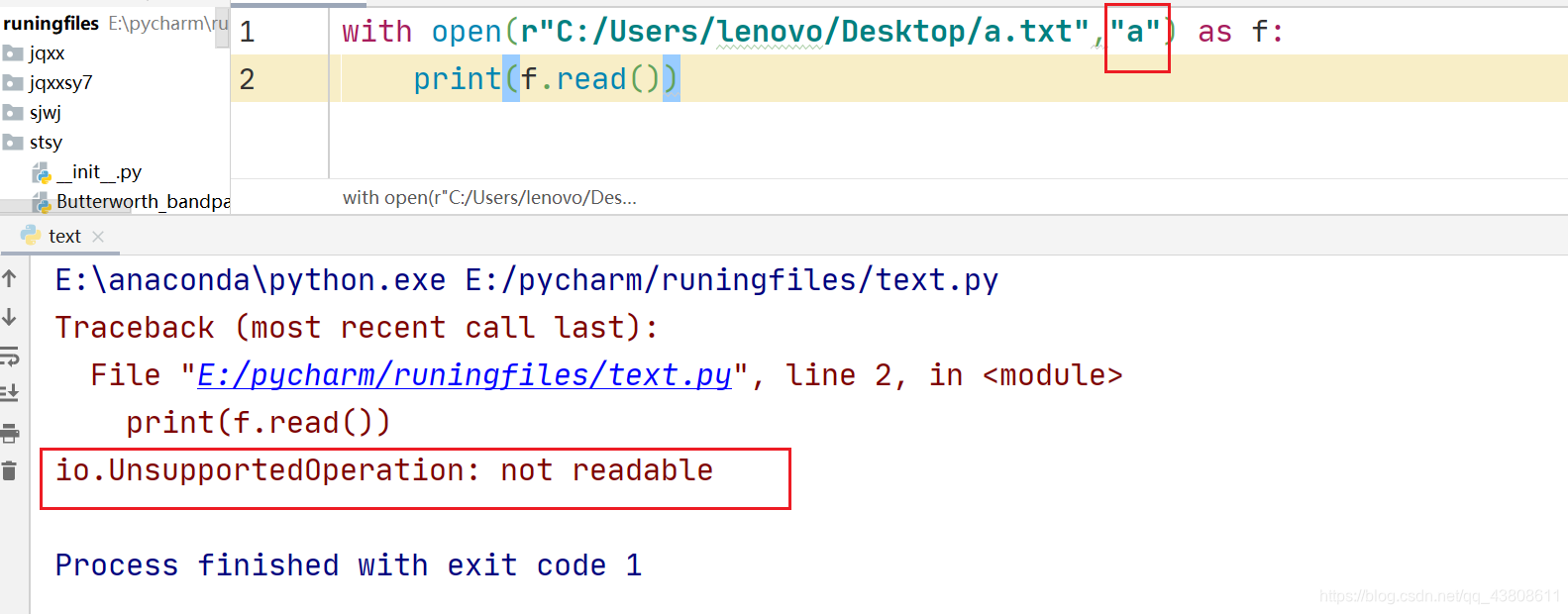
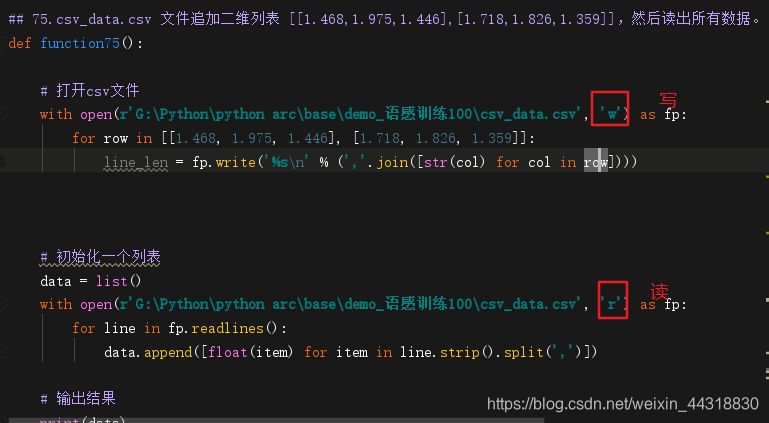
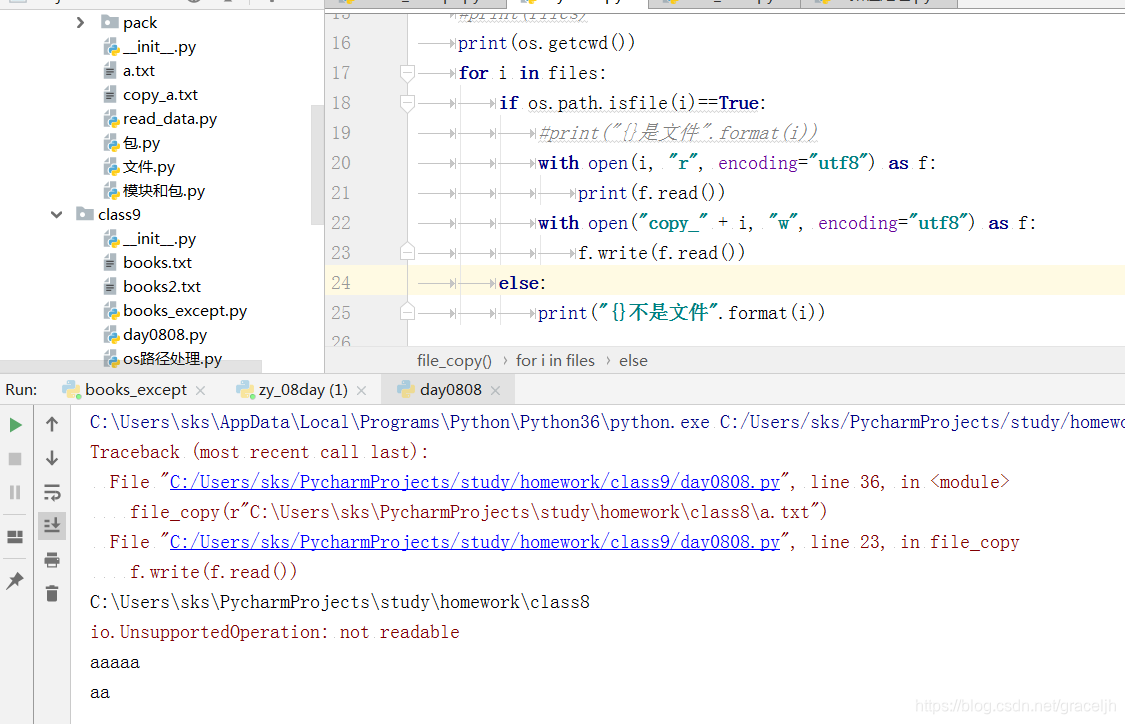
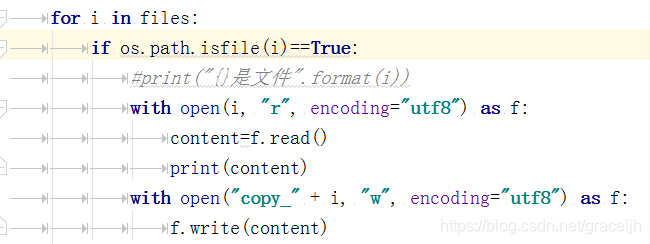




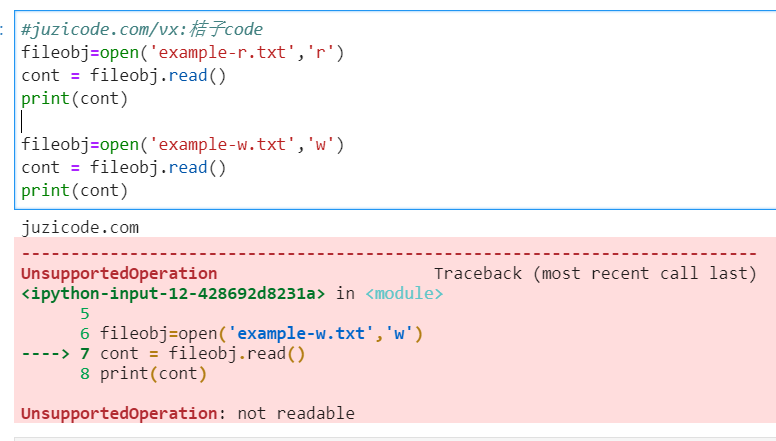
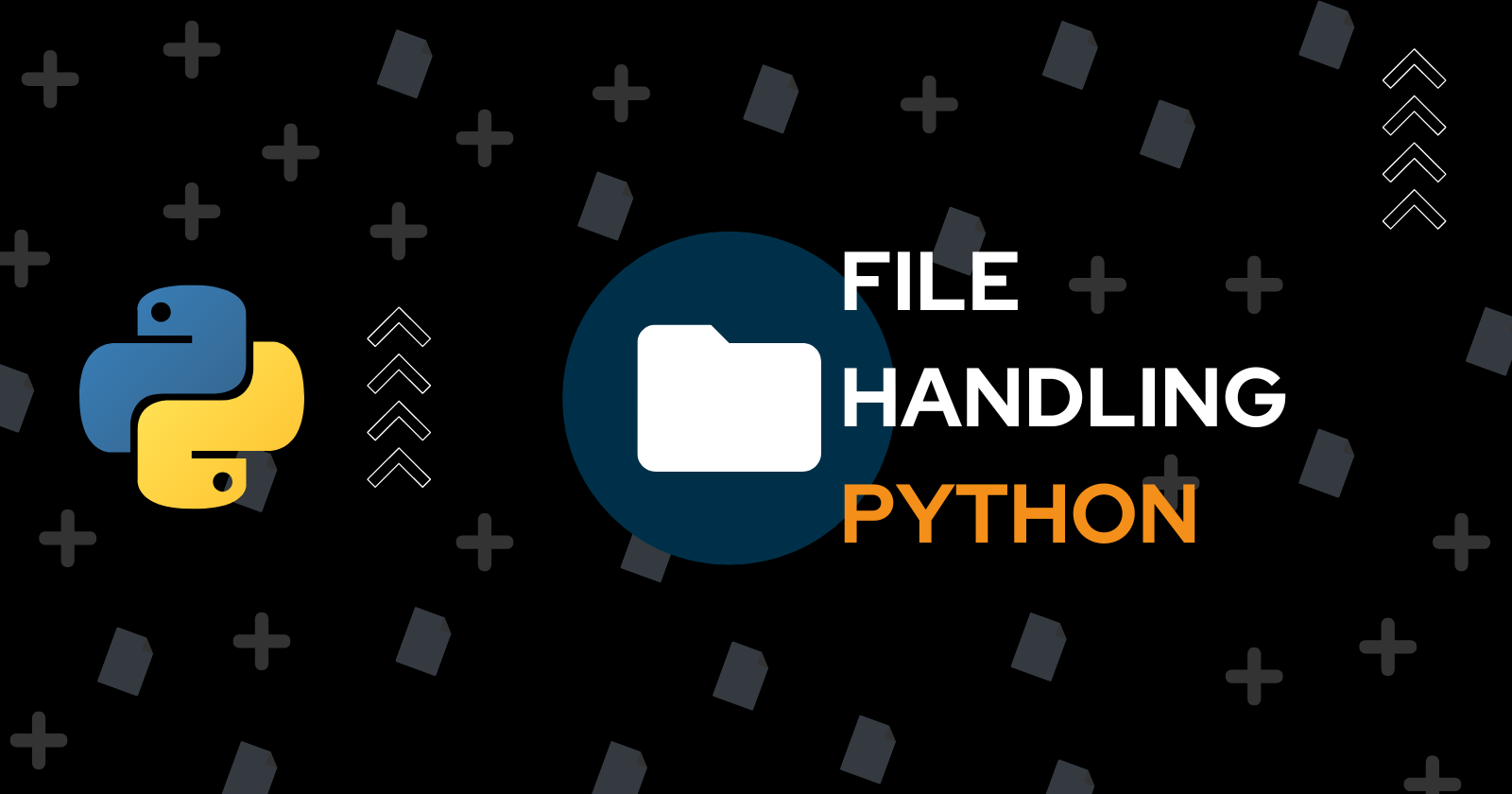
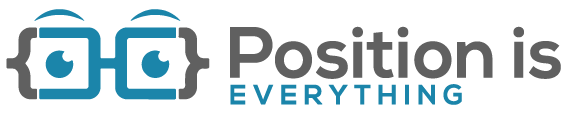
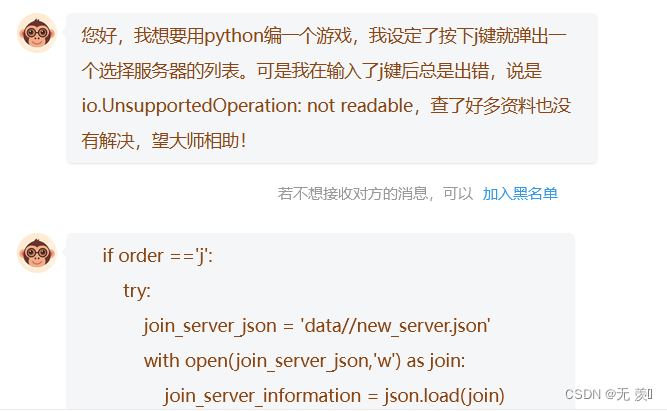
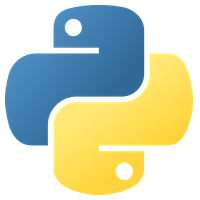

Article link: io.unsupportedoperation not readable python.
Learn more about the topic io.unsupportedoperation not readable python.
- file – Python error message io.UnsupportedOperation: not …
- [Fixed] “io.unsupportedoperation not readable” Error
- io.UnsupportedOperation: not readable/writable Python Error
- io.UnsupportedOperation: not readable/writable Python Error
- [Fixed] “io.unsupportedoperation not readable” Error
- io.UnsupportedOperation: not readable
- IO.UnsupportedOperation: Not Writable Error in Python
- Python error message io.UnsupportedOperation: not …
- Help me understand error unsupported operation
- Python Examples of io.UnsupportedOperation
- Message 148928 – Python tracker
- [python-3.x] Python error message io.UnsupportedOperation
See more: nhanvietluanvan.com/luat-hoc