Importing From Parent Directory Python
Importing and accessing files from the parent directory in Python can be a useful technique when working on complex projects or organizing your codebase efficiently. In this article, we will explore various methods and best practices for importing modules, packages, and files from the parent directory in Python programming language.
Understanding the Directory Structure:
In Python projects, it is essential to understand the directory structure as it forms the foundation for importing files from the parent directory. Organizing your code and files efficiently can greatly enhance readability and maintainability. It is also crucial to differentiate between the current directory (where the script or module is executed) and the parent directory (the directory containing the current directory).
Importing Modules from the Parent Directory:
Modules in Python are reusable blocks of code that can be imported and utilized in different parts of your project. Importing modules allows you to access functions, classes, or variables defined in those modules. When importing standard library modules, absolute imports are commonly used. However, importing modules from the parent directory can be challenging due to the differences in the directory structure.
To import modules from the parent directory, the sys module can be used. By manipulating the sys.path list, which contains the directories Python searches for modules, you can include the parent directory and import modules successfully.
Importing Packages from the Parent Directory:
Packages in Python are hierarchical structures that organize modules into directories and subdirectories. Importing packages is similar to importing modules but requires additional steps. When importing packages, absolute imports are typically used. However, importing packages from the parent directory can also pose challenges.
To import packages from the parent directory, you can use the sys module in conjunction with the site module. The site module helps modify the sys.path list, making it easier to import packages from the parent directory.
Importing Specific Files from the Parent Directory:
In some cases, you may need to import specific files from the parent directory rather than entire modules or packages. This can be achieved using different approaches, such as manipulating the sys.path, using relative imports, or providing the full path to the file. However, it is important to consider the limitations and potential issues when importing specific files.
Using relative imports is a common approach for importing specific files from the parent directory. It allows you to specify the relative path to the file, making it easier to import files across modules.
Best Practices and Techniques:
To optimize your codebase, adopting best practices and techniques in importing from the parent directory is crucial. Organizing files and directories in your Python projects helps maintain a clear structure and makes it easier to import files. The use of the __init__.py file in packages and directories enables proper initialization and importation.
Avoiding name clashes when importing from parent directories is important. By using unique names or aliasing imported objects, you can prevent conflicts and improve code readability. Additionally, optimizing code readability and maintainability by following consistent import styles and avoiding deep directory nesting is recommended.
Common Errors and Troubleshooting:
Importing files from the parent directory can lead to common errors and issues. Identifying these errors is essential for effective troubleshooting. Some common errors include attempting relative imports beyond top-level packages, circular imports, or problems with the import path.
To troubleshoot these issues, it is helpful to understand the specific error messages and debug the import statements. Techniques such as checking the Python version, examining the sys.path list, or utilizing debugging tools can aid in resolving import-related problems.
Conclusion:
Importing and accessing files from the parent directory in Python is a valuable technique for working on complex projects and organizing code more effectively. By understanding the directory structure, leveraging the capabilities of the sys and site modules, and following best practices, you can import modules, packages, and files from the parent directory efficiently. Continuously exploring advanced import methods will further enhance your Python skills and flexibility in handling various project scenarios.
Python – Importing Your Modules (Part 2: Import From A Different Folder ~7 Mins! No Ads)
How To Import A Python File From A Parent Directory In Python?
Python is a versatile programming language that allows developers to organize their code into reusable modules and packages. However, when working on a complex project with multiple subdirectories, you may encounter situations where you need to import a Python file from a parent directory. In this article, we will explore various methods to achieve this task effectively.
Method 1: Modifying sys.path
One way to import a Python file from a parent directory is by modifying the sys.path variable. The sys module provides access to various parameters and functions that interact with the Python interpreter. By appending the parent directory path to sys.path, you can import files from that location. Here is an example:
“`python
import sys
sys.path.append(“..”)
from parent_folder import file
“`
In the above code snippet, we add “..” to sys.path to indicate the parent directory. Then we can import the desired file from the parent_folder.
Method 2: Using the absolute path
Another approach to importing a Python file from a parent directory is by using an absolute path. This method provides a more explicit way of locating the parent directory and importing the file. Here’s an example:
“`python
import os
import sys
parent_dir = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
sys.path.append(parent_dir)
from parent_folder import file
“`
In this method, we use the os module to obtain the absolute path of the current script (__file__), then retrieve the parent directory path using os.path.dirname multiple times. Finally, we append the parent directory path to sys.path and import the desired file.
Method 3: Modifying PYTHONPATH
Python provides an environment variable called PYTHONPATH, which allows you to specify additional directories where Python searches for modules. By modifying this variable, you can import files from a parent directory. Here’s how to do it:
“`bash
$ export PYTHONPATH=”$PYTHONPATH:../”
“`
After setting the PYTHONPATH correctly, you can import the desired file like any other module in Python.
FAQs
Q1: Can I import a file from a grandparent directory?
Yes, you can import a file from a grandparent directory by modifying sys.path or PYTHONPATH in a similar manner. Simply adjust the path to include the desired number of parent directories.
Q2: Why should I be careful when modifying sys.path?
Modifying sys.path is a powerful technique, but it can lead to potential issues. If you’re not careful, you may accidentally import the wrong file or create conflicts with other modules. It’s important to ensure the path modifications are specific and do not disrupt the overall structure of your project.
Q3: Is it recommended to use absolute paths?
Using absolute paths may seem more reliable; however, it can make your code less portable. Absolute paths may not work on different operating systems or when the project is moved to a different location. Therefore, it is advised to use relative paths wherever possible.
Q4: How can I organize my project to avoid complicated import statements?
To avoid relying on complex import statements, consider using Python’s packaging system. By organizing your code into packages and modules, you can import files using relative imports within the package structure. This approach not only simplifies import statements but also improves code organization.
In conclusion, importing a Python file from a parent directory can be achieved through various methods, such as modifying sys.path, using absolute paths, or modifying PYTHONPATH. Each method has its advantages and considerations, so choose the one that best fits your project’s requirements. Remember to be cautious when modifying system variables and consider using Python’s packaging system to keep your project organized.
How To Import Parent Package In Python?
Python, being a versatile and widely used programming language, offers numerous features and functionalities to simplify developers’ tasks. One such feature is the ability to organize code into packages and modules, providing a structured and manageable approach to coding. In this article, we will explore the concept of importing parent packages in Python and discuss various techniques to accomplish this.
Understanding Packages and Modules in Python:
Before delving into the specifics of importing parent packages, let’s briefly understand the basics of packages and modules in Python. Packages are namespaces that contain multiple modules, which in turn are files containing Python code. Packages provide a way to organize related modules and offer a hierarchical structure to manage code effectively.
Importing Packages and Modules at the Same Level:
When importing modules or packages located at the same level, Python utilizes the principle of relative imports. For example, consider a directory structure with the following modules:
“`
my_package/
__init__.py
module1.py
module2.py
“`
To import `module1.py` from `module2.py`, you can use the following code snippet:
“`python
from . import module1
“`
The `.` (dot) represents the current package, and using it allows you to import modules present at the same level. However, this method cannot be used to import parent packages. Let’s explore various techniques to import parent packages in Python.
Importing Parent Packages:
Python offers several ways to import parent packages. Let’s examine different scenarios where you might need to import parent packages and explore suitable solutions for each case.
1. Importing Sibling Packages:
In situations where you have sibling packages at the same level, you can use the `..` notation to import the parent package. Here’s an example:
“`python
from ..parent_package import module
“`
The `..` represents the parent package, enabling you to import sibling packages residing at the same level.
2. Importing Subpackages:
To import a subpackage from within a package, you can utilize the `.` notation followed by the subpackage name. For instance, consider the following directory structure:
“`
my_package/
__init__.py
subpackage/
__init__.py
module3.py
“`
To import `module3.py` from `__init__.py` in the parent package, you can use the following code:
“`python
from .subpackage import module3
“`
The `.` indicates that you want to import the subpackage named `subpackage` from the parent package.
3. Importing Parent Packages from Outside the Package:
Sometimes, you may need to import a parent package from a file that resides outside the package. In such cases, you can use the `sys.path` approach.
First, you need to obtain the absolute path of the parent package, and then update the `sys.path` to include the path. Here’s an example:
“`python
import sys
sys.path.append(‘/path/to/parent_package’)
from parent_package import module
“`
Make sure to replace `’/path/to/parent_package’` with the actual absolute path of the parent package on your system.
Using this technique, you can import parent packages from anywhere within your project, even if the files are located outside the package structure.
FAQs:
Q: Why do I need to import parent packages in Python?
A: Importing parent packages allows you to access modules and subpackages residing at higher levels in the package hierarchy.
Q: Can I use relative imports to import parent packages?
A: No, relative imports are limited to importing modules from the same level or below in the package hierarchy.
Q: Are there any potential pitfalls when importing parent packages?
A: Yes, one common issue is the usage of duplicate package names in different locations within your project. This can lead to ambiguous import paths and cause import errors. Be cautious while organizing and naming your packages to avoid such conflicts.
Q: Is it recommended to import parent packages from outside the package itself?
A: Importing parent packages from outside the package should be used sparingly and only when necessary. It can make your code more complex and less portable. It’s generally advised to structure your code in a way that avoids the need for importing parent packages from external files.
In conclusion, importing parent packages in Python is crucial for accessing modules and subpackages residing above the current level in the package hierarchy. By using relative imports, accessing sibling packages, subpackages, and even parent packages from outside the package becomes easier. It’s essential to understand these techniques and utilize them appropriately to maintain a well-organized and manageable codebase.
Keywords searched by users: importing from parent directory python Python import module from parent directory, Python import module from another directory, Python3 import from parent directory, Get parent directory Python, Import function from parent folder python, attempted relative import beyond top-level package, Python import from sibling directory, Python __all__
Categories: Top 32 Importing From Parent Directory Python
See more here: nhanvietluanvan.com
Python Import Module From Parent Directory
Python is a versatile programming language that offers a wide range of tools and libraries to build and develop applications. One of the most powerful features of Python is its ability to import modules, which allows developers to reuse code and extend functionality. While importing modules from the same directory or from installed packages is straightforward, importing modules from a parent directory can be a bit more challenging. In this article, we will explore various techniques to import modules from a parent directory in Python and provide answers to frequently asked questions.
1. Overview of the module import system in Python
Python’s import system enables developers to access code and resources from external files. When a module is imported, Python searches for it in a predefined set of directories, including the current directory (or script’s directory) where the code is executed, and the directories specified in the PYTHONPATH environment variable. Once the module is located, Python compiles it into bytecode and executes it.
2. Importing modules from the same directory
Before diving into importing modules from a parent directory, let’s quickly review how to import modules from the same directory. Assuming we have a module called “example_module.py” in the same directory as our script, we can import it using the following syntax:
“`python
import example_module
“`
or
“`python
from example_module import some_function
“`
3. Importing modules from a parent directory
When you need to import a module from a parent directory, the directory structure becomes important. Assuming the following structure:
“`
parent_directory
├── subdirectory
│ └── module.py
└── script.py
“`
To import “module.py” from “script.py” in the parent directory, you can follow one of the following approaches:
3.1 Relative import
You can use a relative import to access the required module. In our case, the import statement would look like this:
“`python
from subdirectory import module
“`
This imports the “module.py” from the “subdirectory” folder, assuming both “script.py” and “subdirectory” are in the parent_directory.
3.2 Dynamic modification of system path
If you prefer to use an absolute import instead of a relative import, you can modify the system path dynamically to include the parent directory. Here’s an example of how you can achieve this:
“`python
import sys
sys.path.insert(0, ‘../’)
from subdirectory import module
“`
Adding the parent directory to the system path allows Python to locate the required module, even if it is not in the same directory as the script.
It’s worth noting that modifying the system path dynamically can have consequences in larger projects and should be used with caution.
4. Frequently Asked Questions (FAQs)
Q1. Why should I import a module from a parent directory?
Importing a module from a parent directory is useful when you want to reuse existing code or access resources located in a different directory. It provides flexibility in organizing your project structure and encourages code reusability.
Q2. Can I directly import a module from an arbitrary directory?
Python’s import system does not support importing modules from arbitrary directories by default. It is recommended to follow best practices and structure your project in a way that allows for seamless import of modules.
Q3. Is it possible to import multiple modules from different parent directories?
Yes, it is possible to import multiple modules from different parent directories using either relative import or dynamically modifying the system path.
Q4. What is the difference between a relative import and modifying the system path?
A relative import imports a module from a specific directory relative to the current script, whereas modifying the system path allows importing modules from any directory on the system. Relative imports are generally preferred over modifying the system path to ensure portability and maintainability of the code.
Q5. How can I import a module located in a grandparent directory?
To import a module located in a grandparent directory, you can use either relative imports with multiple dots or dynamically modify the system path accordingly.
In conclusion, Python’s import system provides various techniques to import modules from different directories. While importing modules from the same directory is straightforward, importing modules from a parent directory requires a slightly different approach. By using relative imports or modifying the system path, developers can effectively access modules from parent directories and promote code reusability. Remember to structure your project in a logical manner to ensure smooth importing of modules and maintainability of your codebase.
Python Import Module From Another Directory
When working on complex projects, it is common for developers to separate their code into multiple directories or packages based on different functionality or modules. This organization makes code easier to understand, test, and collaborate on. However, when modules are in separate directories, it may initially seem challenging to import and use them in other parts of the code. Fortunately, Python provides several ways to overcome this hurdle.
The most straightforward way to import a module from another directory is by adding the directory to the Python path. By adding a directory to the path, Python will search for modules in that directory when importing a module. The sys module in Python provides a simple way to modify the Python path dynamically. Here’s an example of how it can be done:
“`python
import sys
sys.path.append(‘/path/to/directory’)
import module_name
“`
In the above code snippet, the directory ‘/path/to/directory’ is added to the Python path using sys.path.append(). Once the directory is added, the module_name can be imported and used in the code without any issues.
Another method to import a module from another directory is by using the imp module. The imp module provides a set of functions that allow importing modules from files, directories, or even from within zip archives. Here’s an example of how it can be done:
“`python
import imp
module = imp.load_source(‘module_name’, ‘/path/to/directory/module_name.py’)
“`
In this code snippet, the imp.load_source() function is used to load the module from the specified path. The module can then be used like any other module imported in Python.
Additionally, if you have a large project with multiple directories and packages, you can use the setuptools package to define and manage dependencies. Setuptools allow you to install packages and automatically manage their dependencies. This simplifies the process of importing modules from different directories, as setuptools handles the package management for you.
Now, let’s address some frequently asked questions related to importing modules from another directory in Python:
1. Can I import a module from a parent directory?
Yes, you can import a module from a parent directory by appending the relative path to the parent directory using the sys.path.append() method.
2. How can I import a module from a subdirectory?
To import a module from a subdirectory, you can either add the subdirectory to the Python path or use the appropriate relative import statement.
3. What if the module I want to import has the same name as a built-in module?
If the module you want to import has the same name as a built-in module, you can use the “as” keyword to provide an alias for the module. For example, `import os as my_os` will import the os module but alias it as my_os to avoid conflicts.
4. Can I import modules from a zip archive?
Yes, Python allows you to import modules from zip archives using the imp module. By using imp.load_module(), you can load modules directly from a zip archive.
In conclusion, Python’s ability to import modules from different directories is a powerful feature that allows developers to organize their code in a modular and manageable way. Whether by adding directories to the Python path, using the imp module, or utilizing setuptools for package management, Python provides multiple options to seamlessly import modules from other directories. Understanding these techniques will enable developers to efficiently build complex applications in Python.
Images related to the topic importing from parent directory python
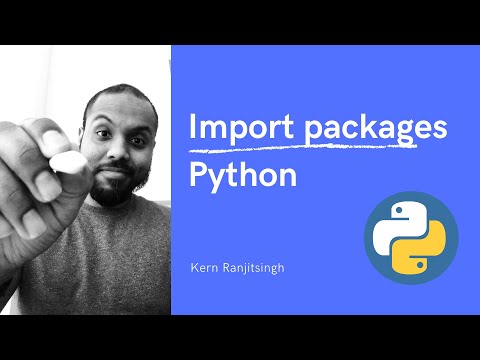
Found 21 images related to importing from parent directory python theme
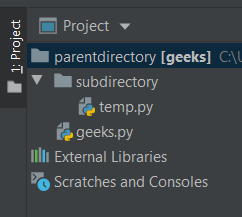
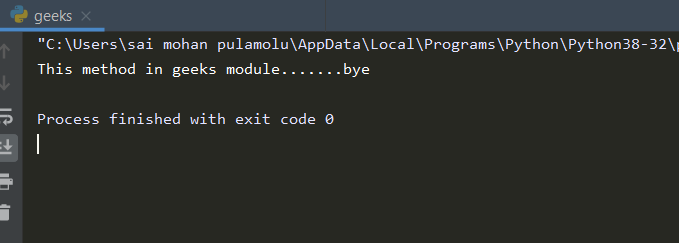
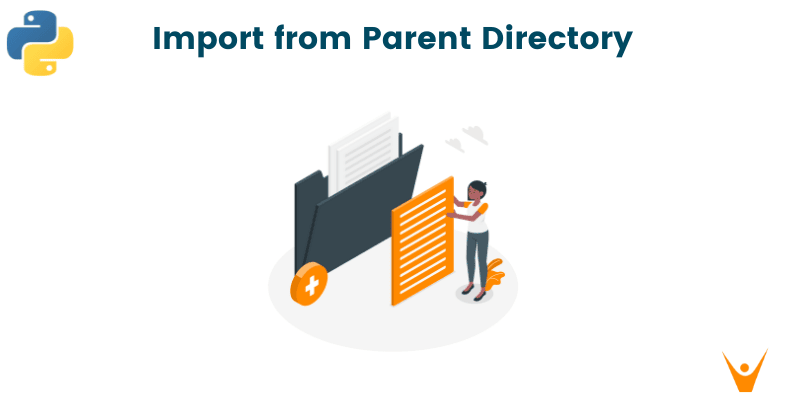
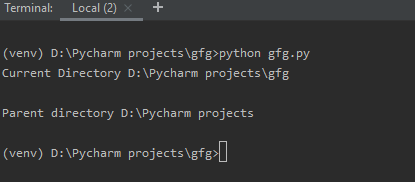
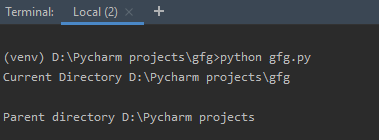
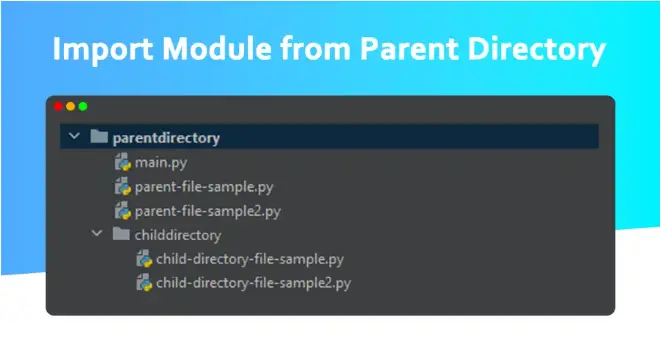

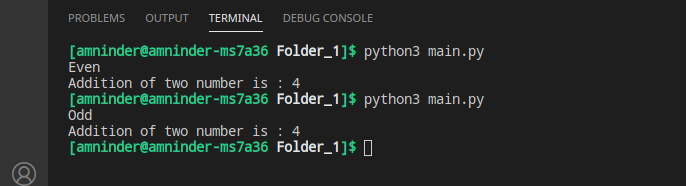
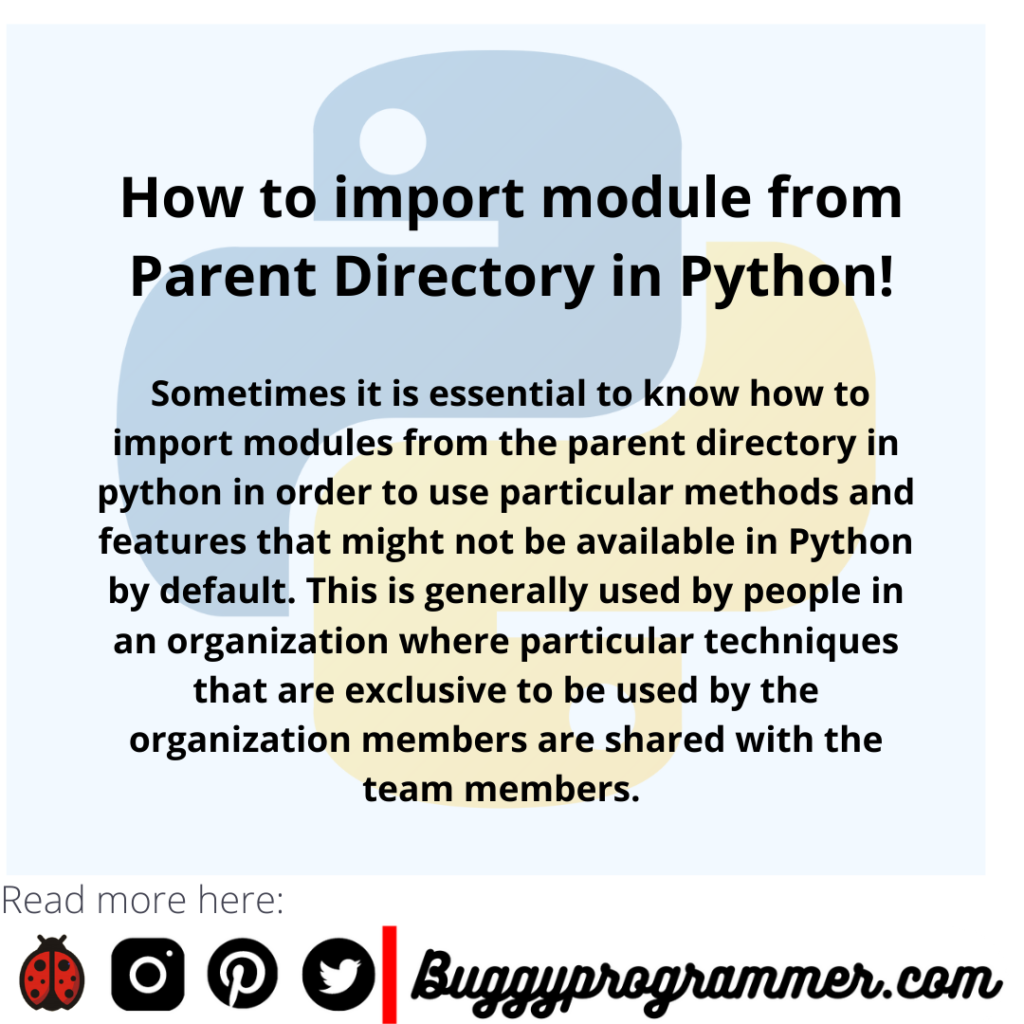

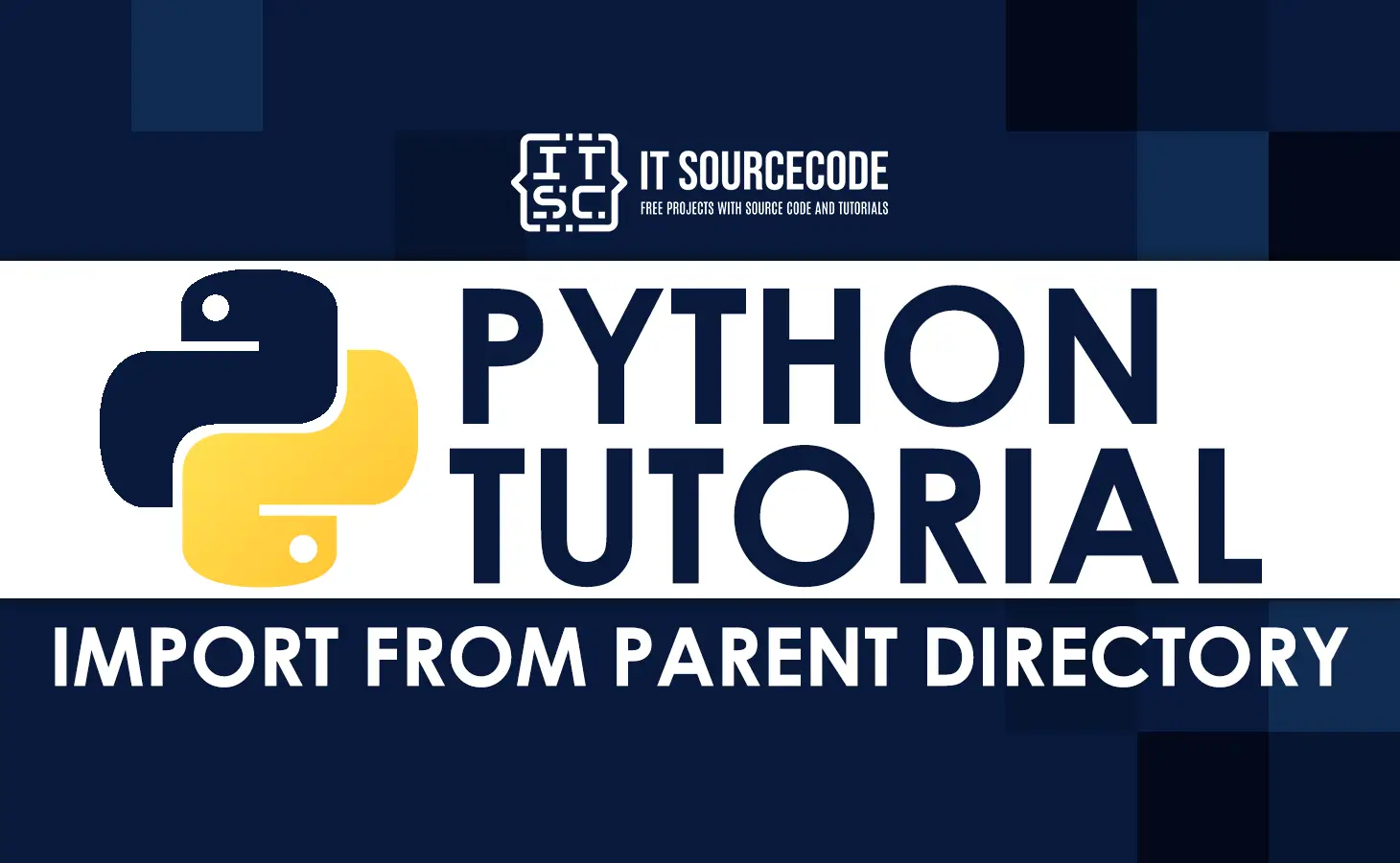
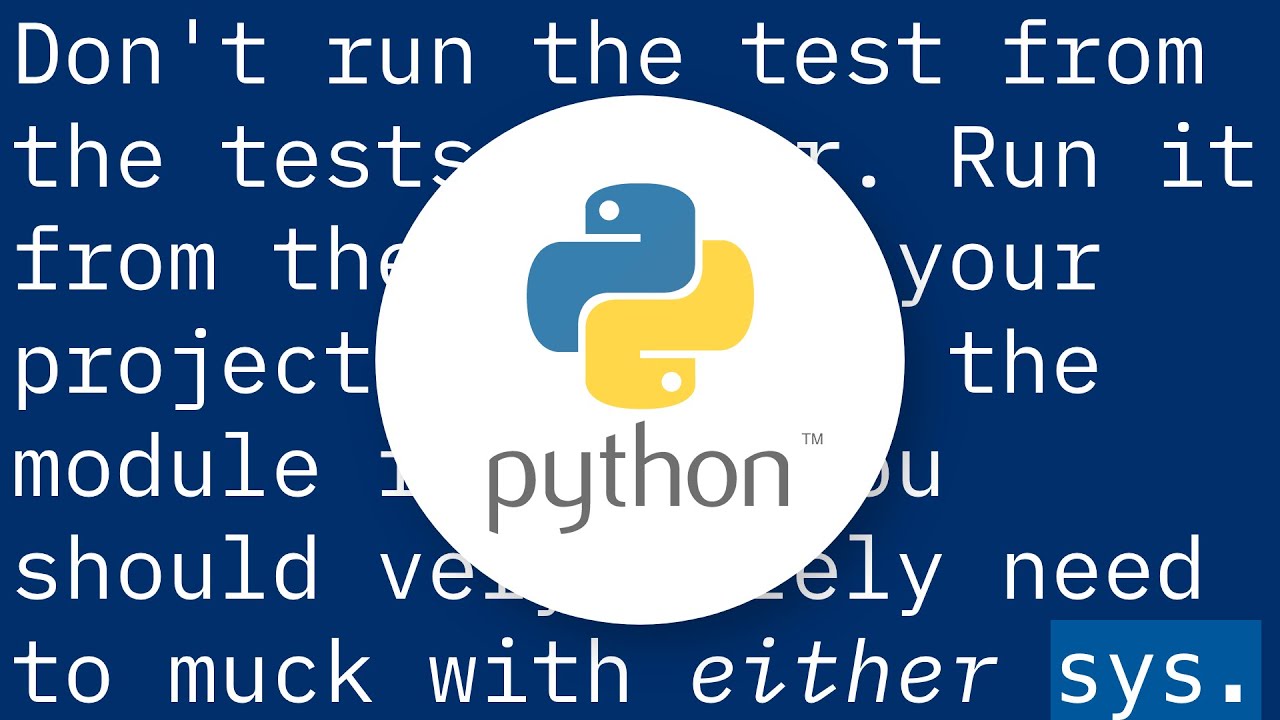


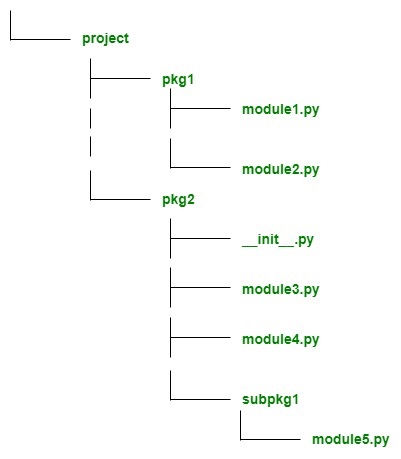
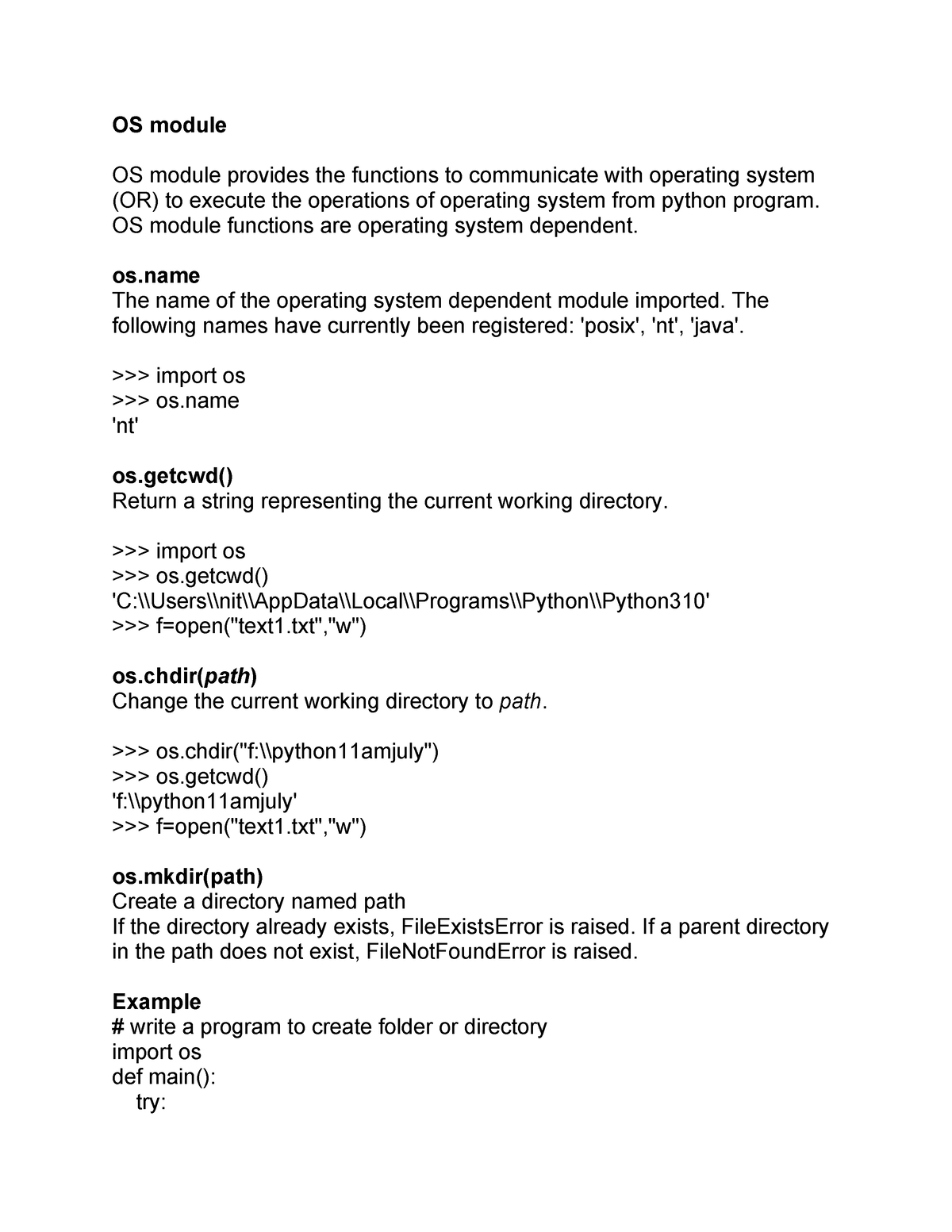
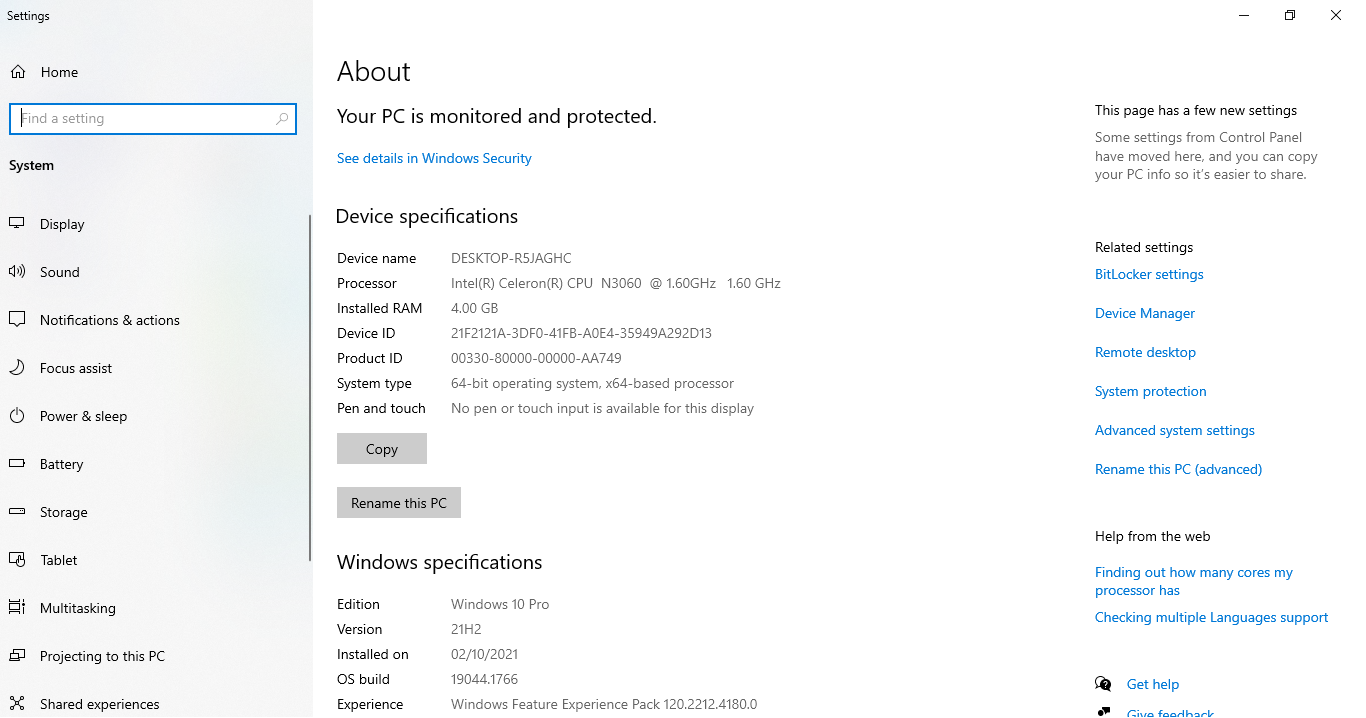
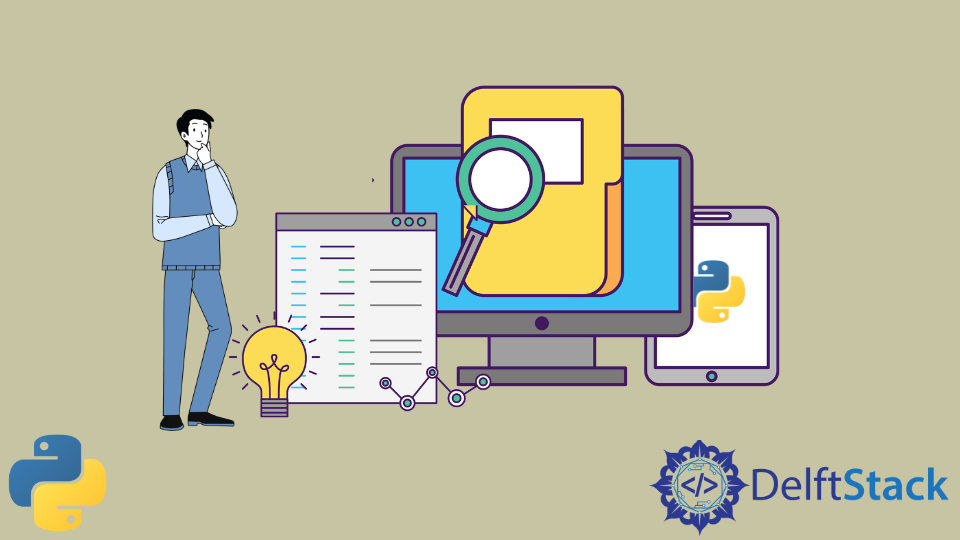


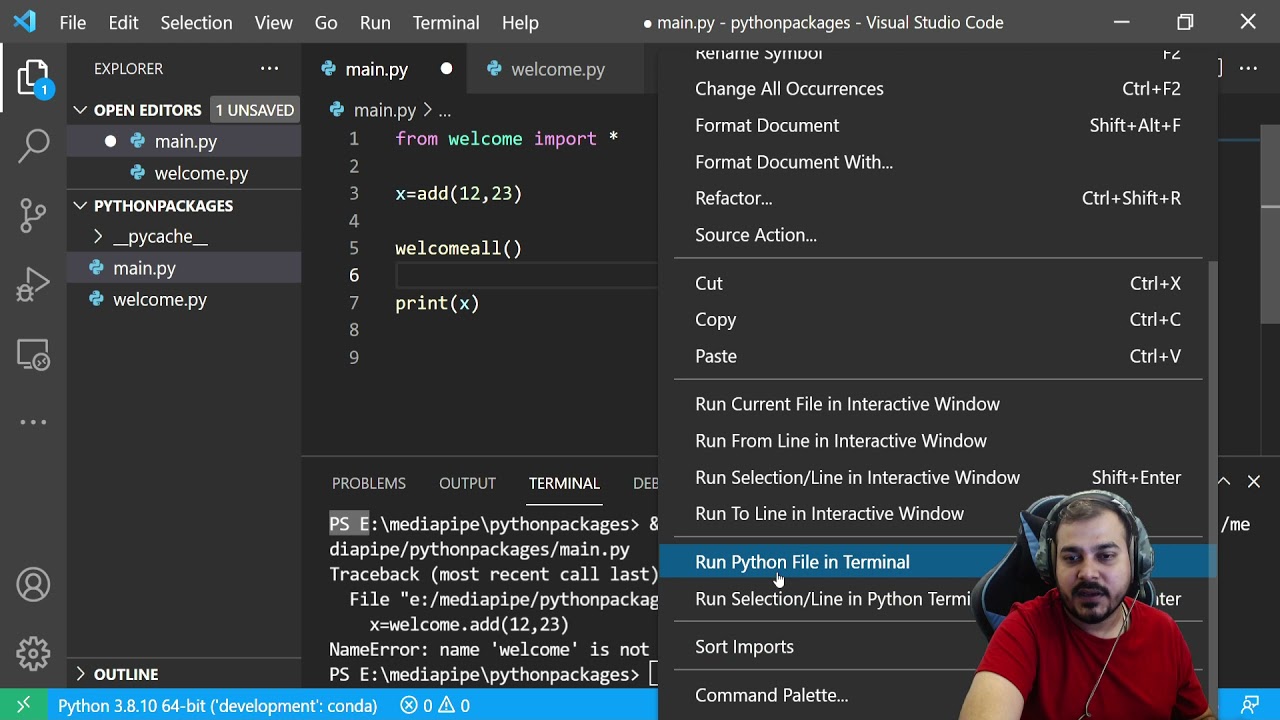
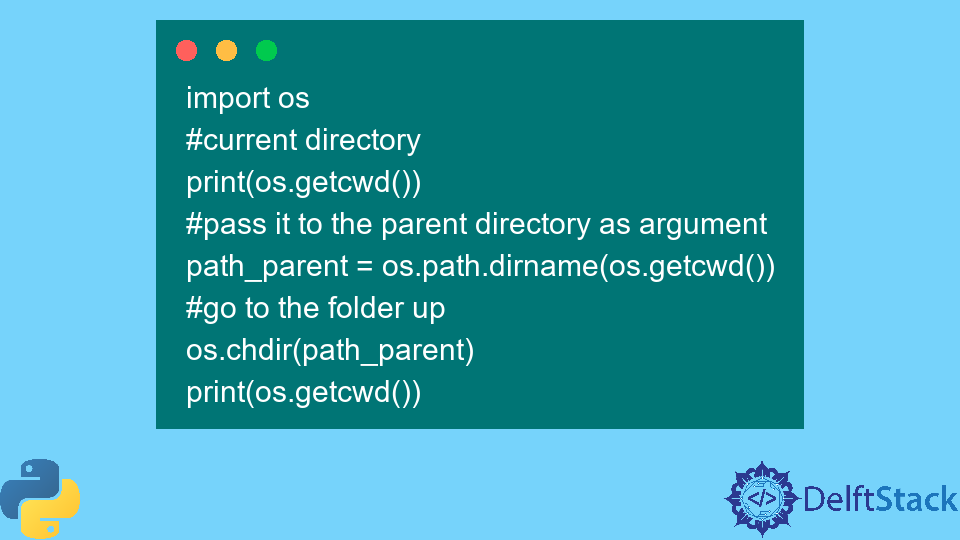
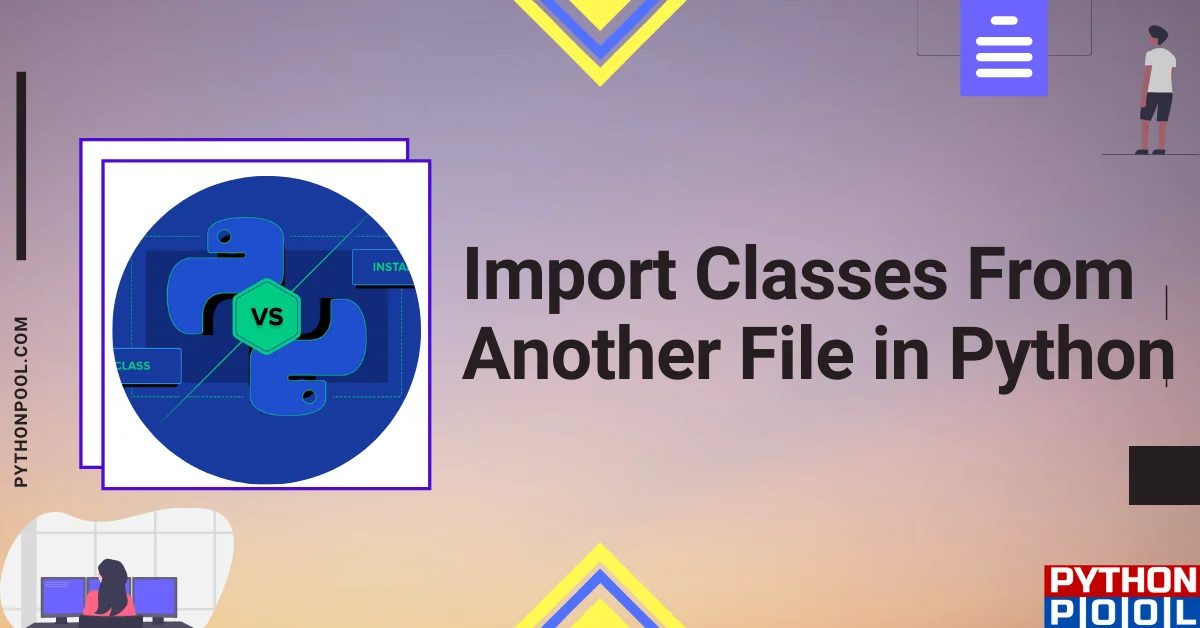

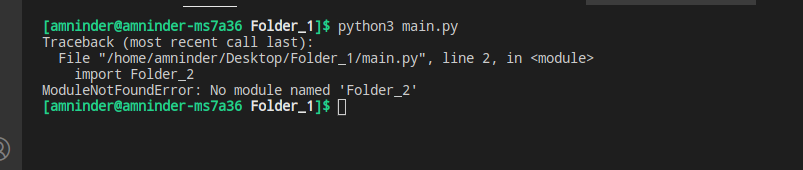
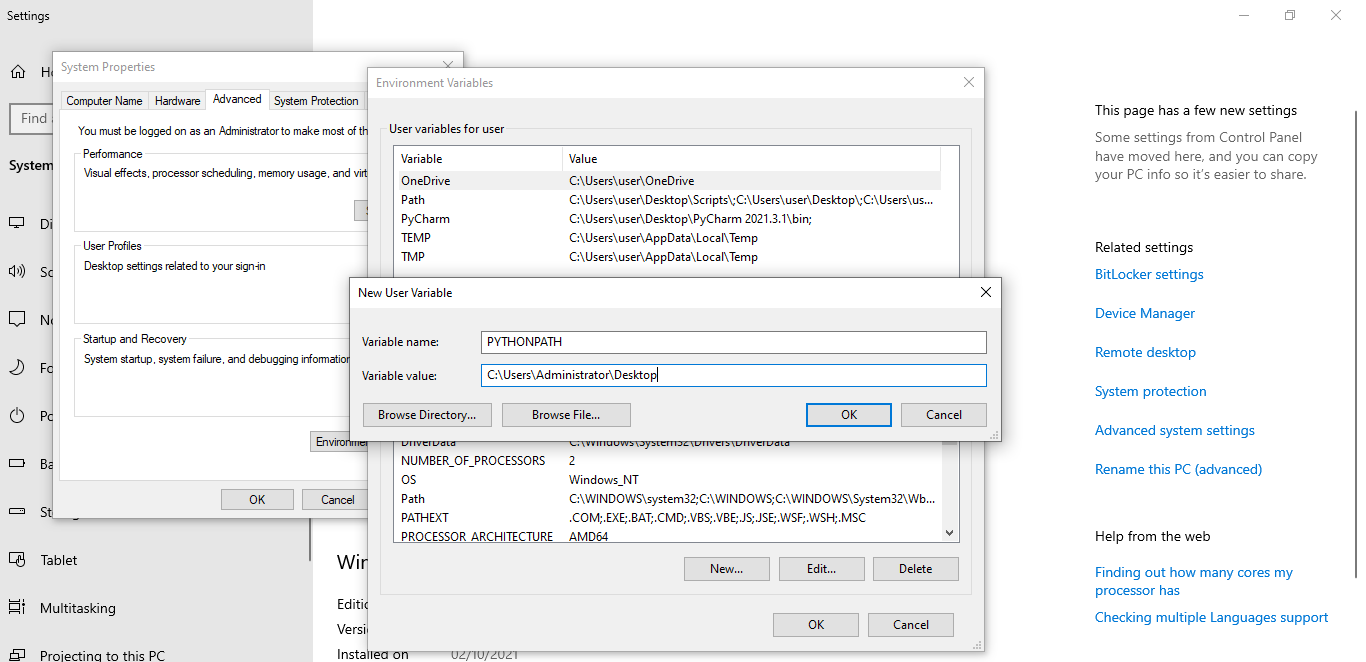

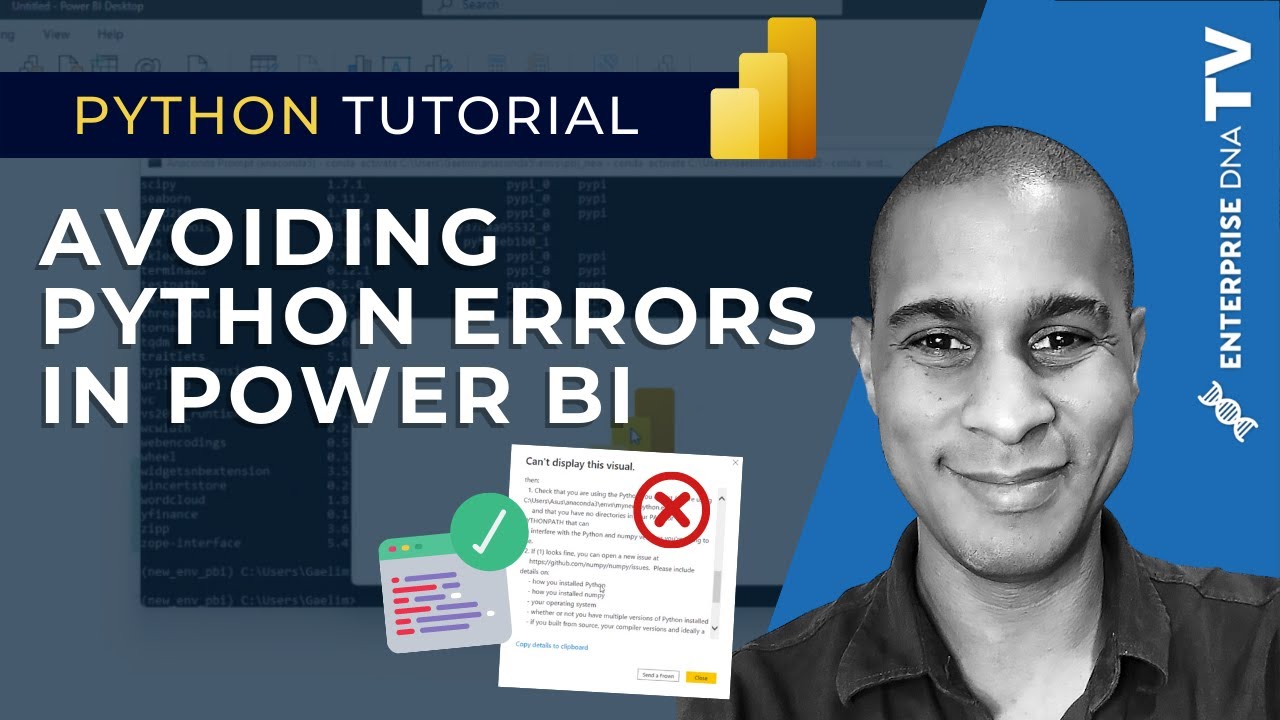

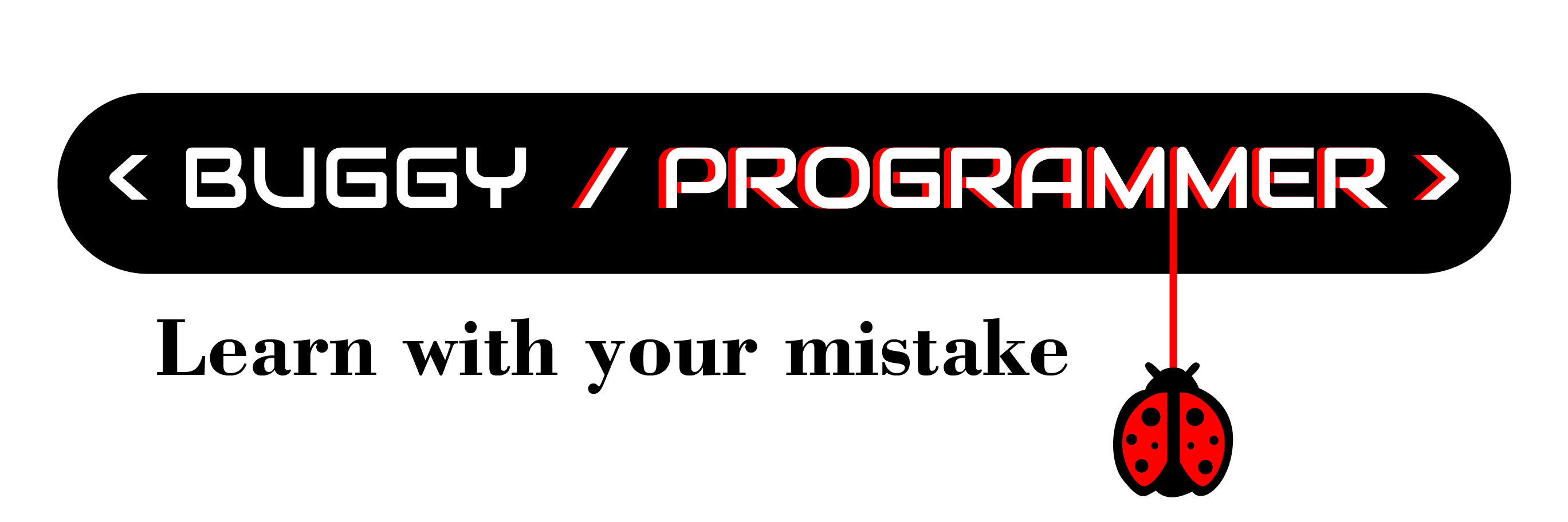

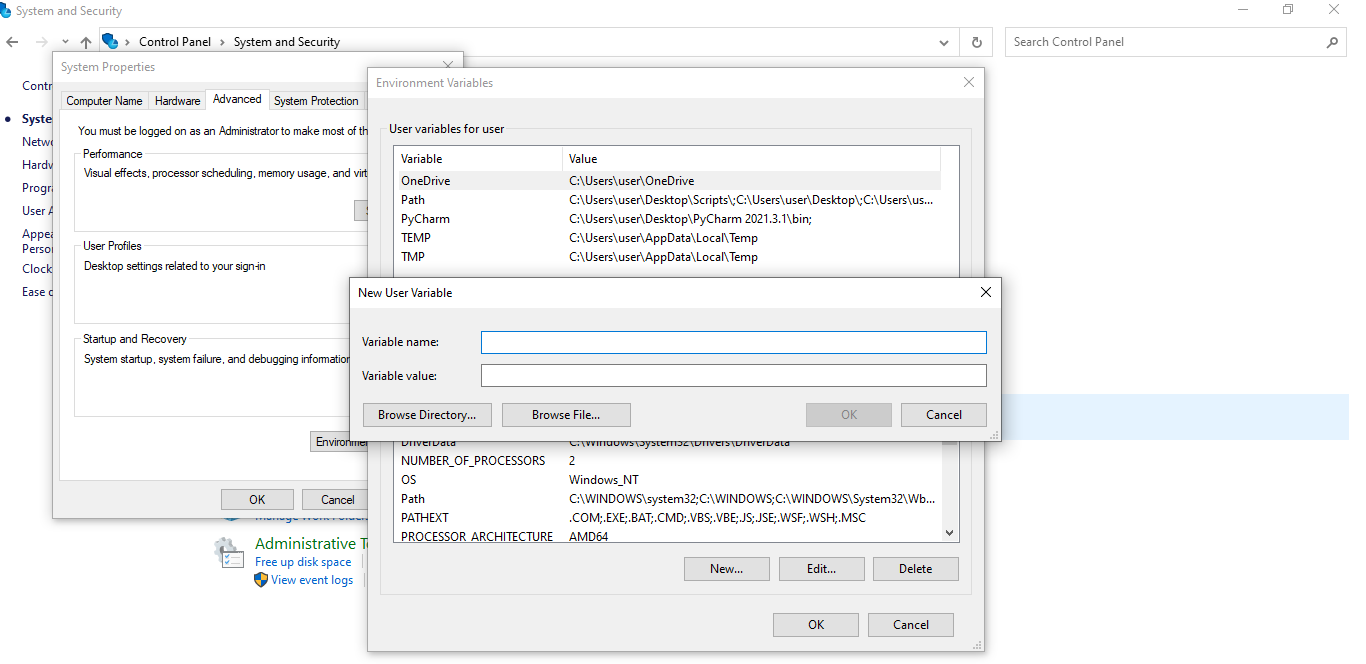
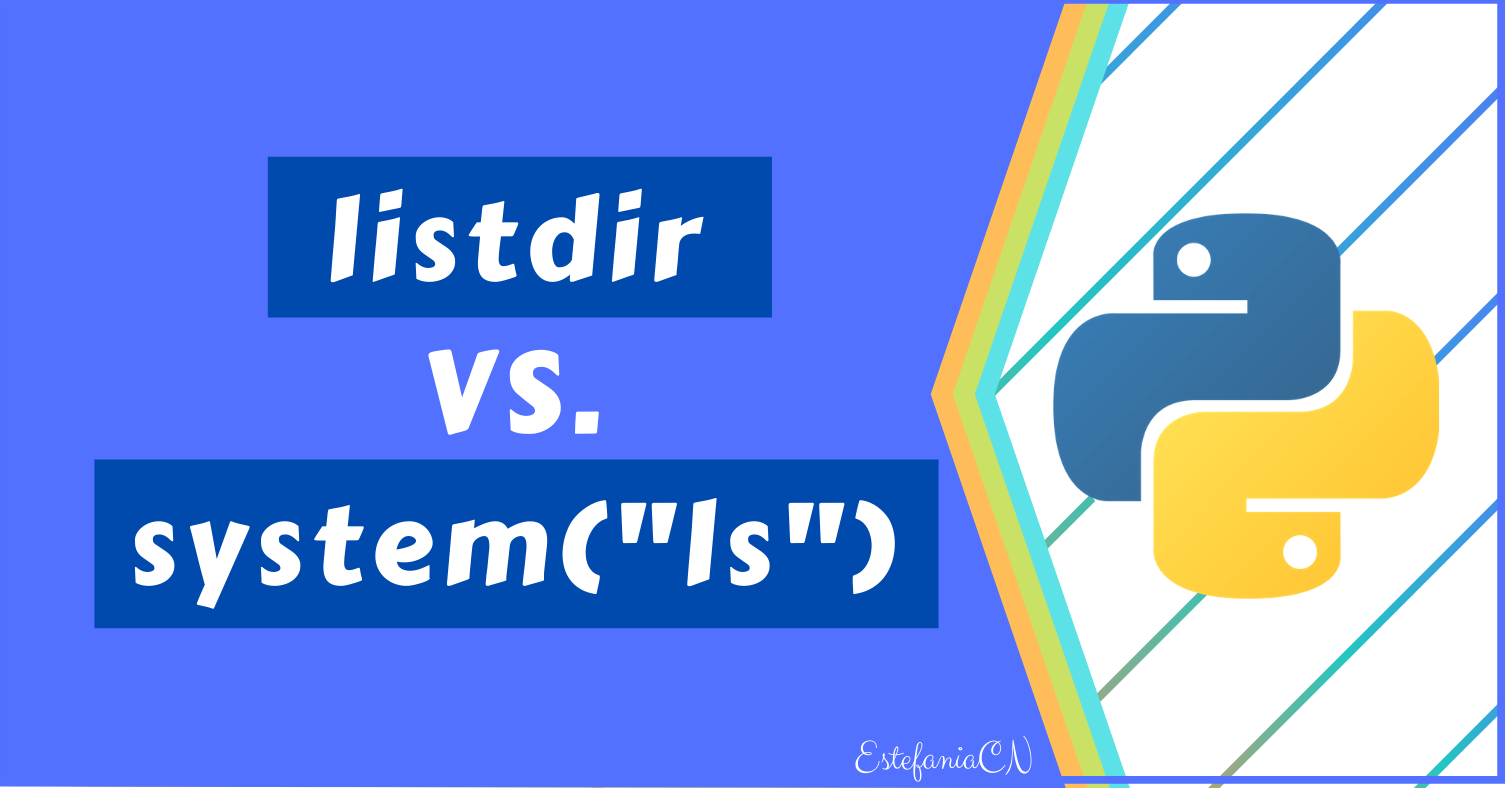
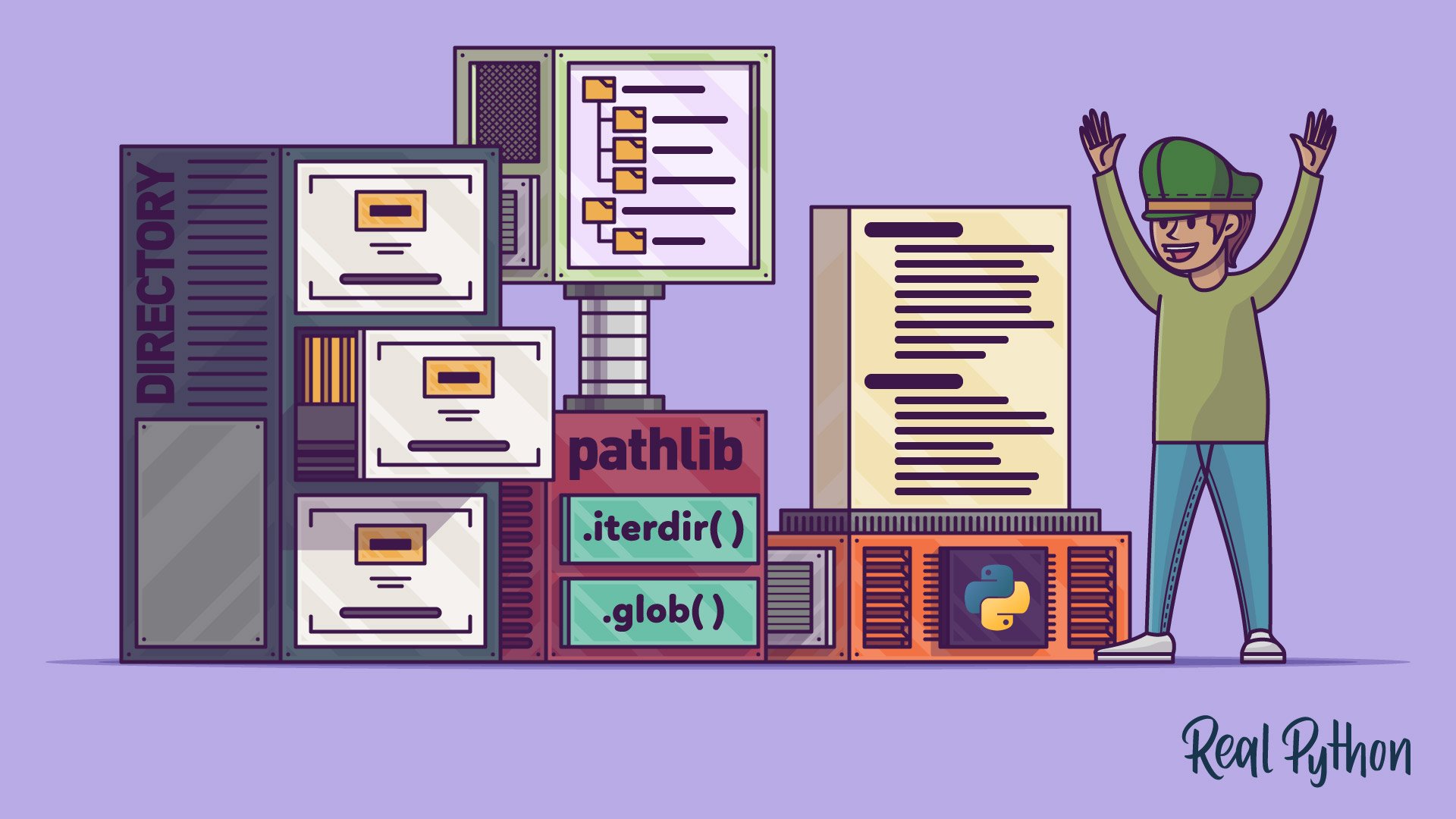
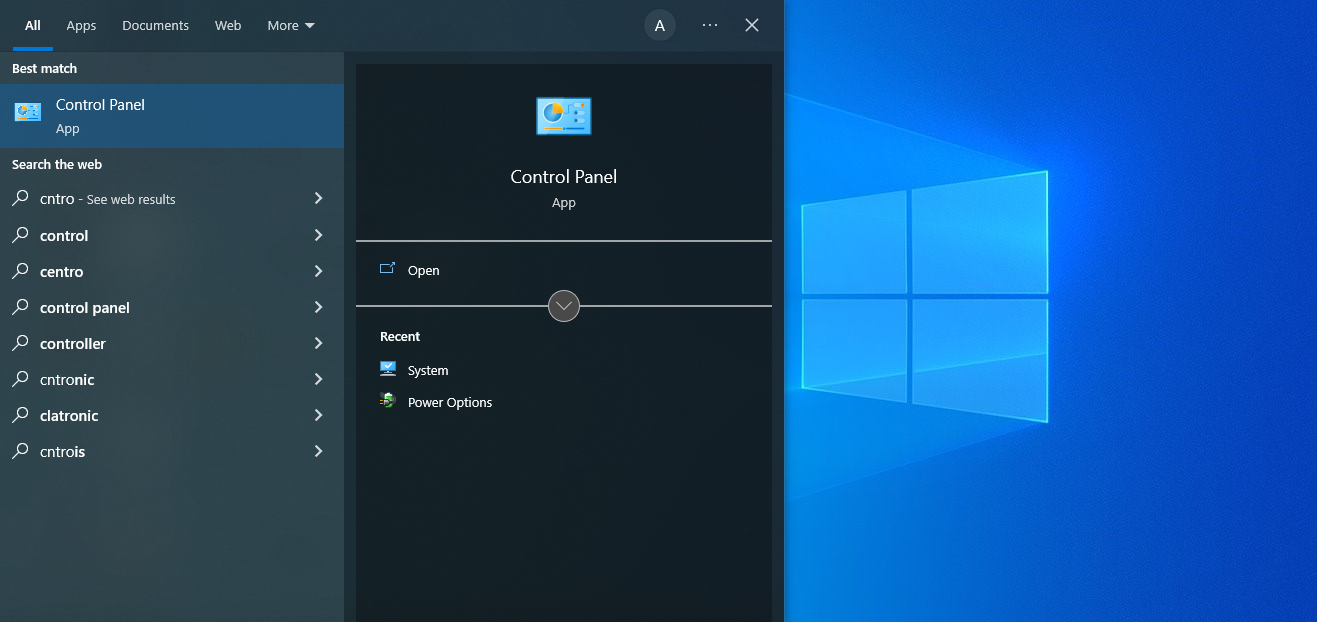
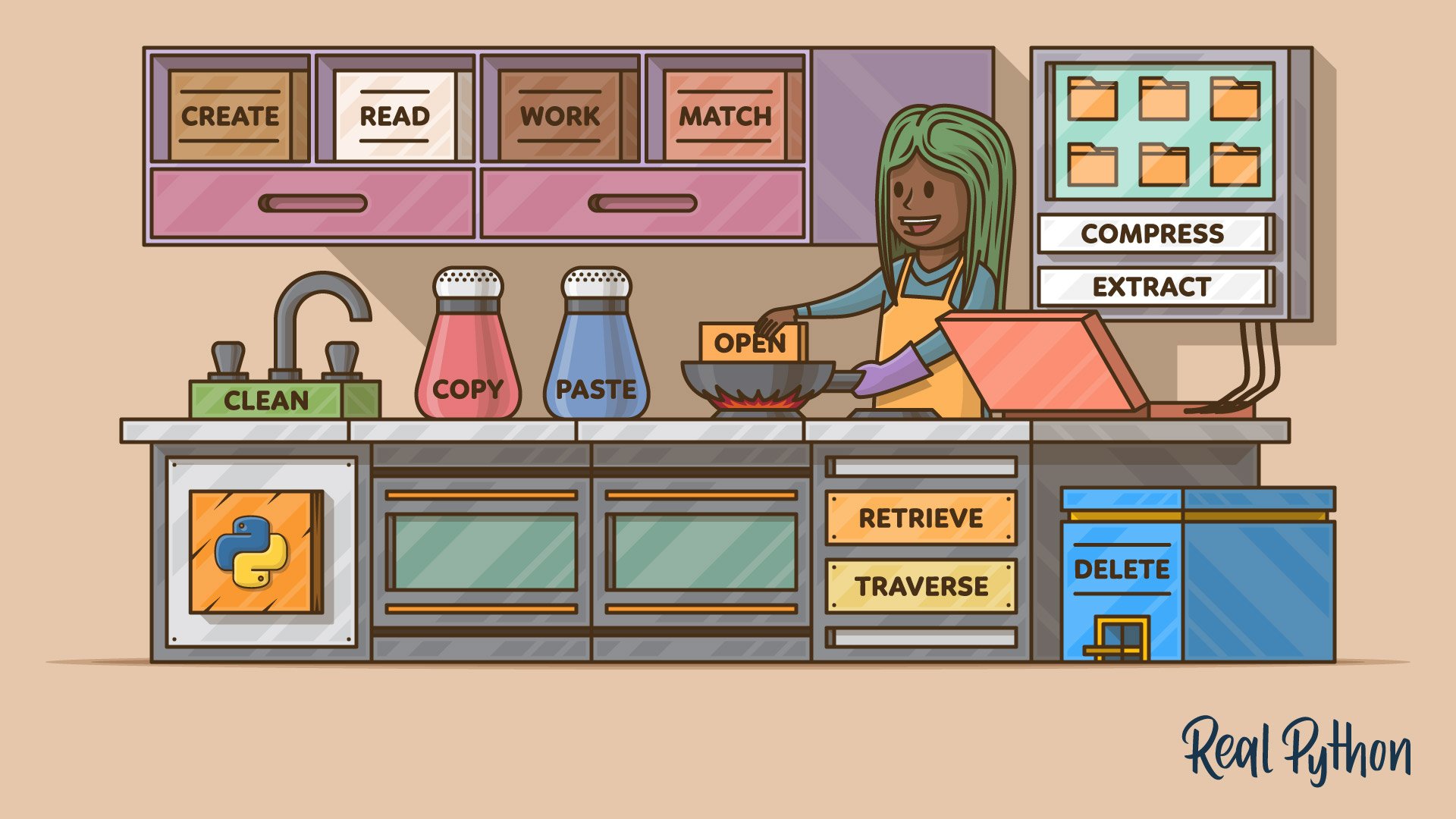



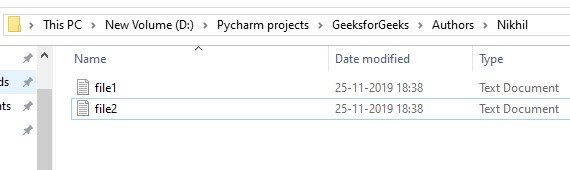
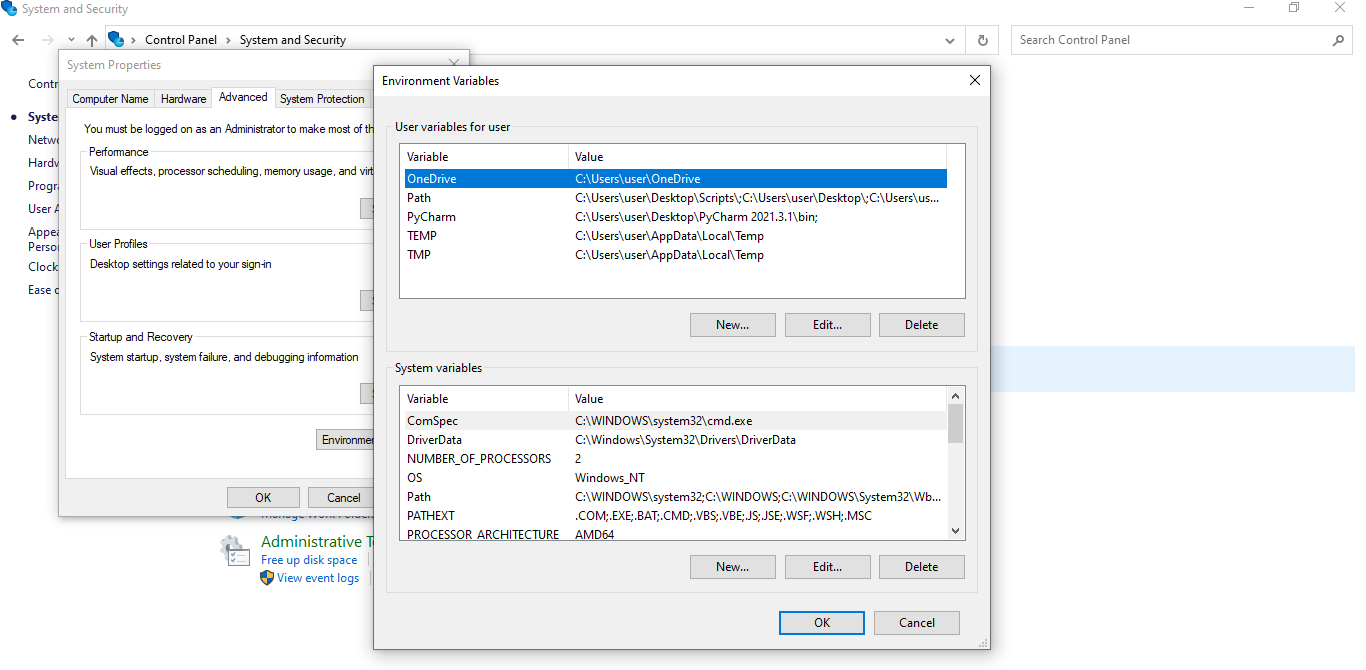
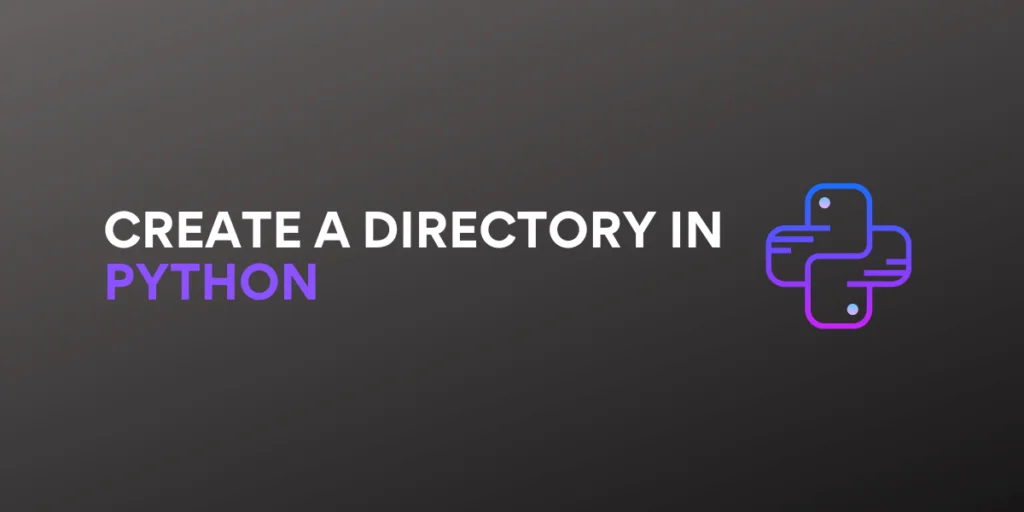
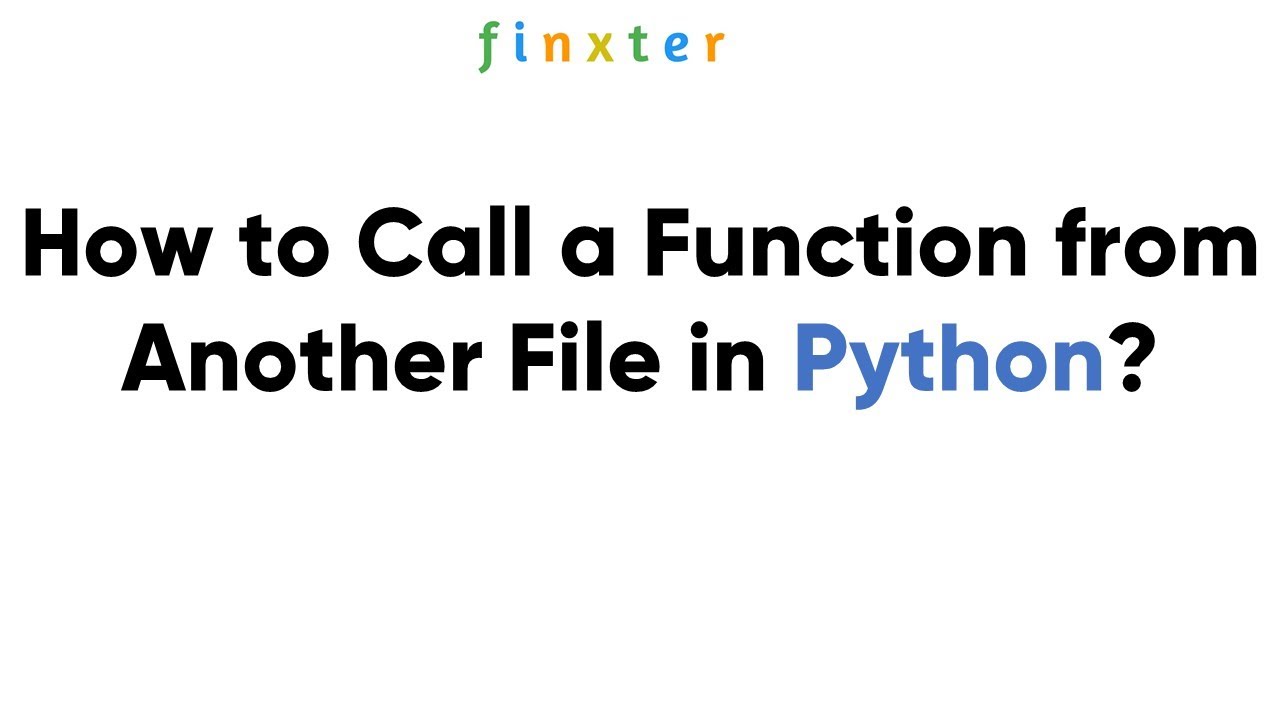

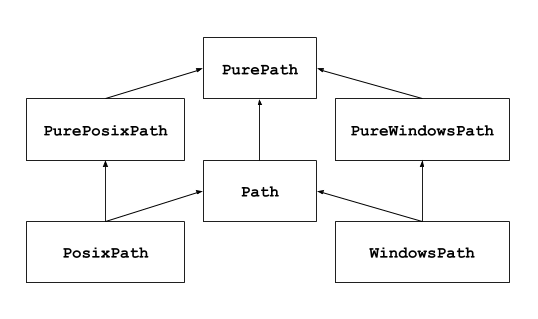
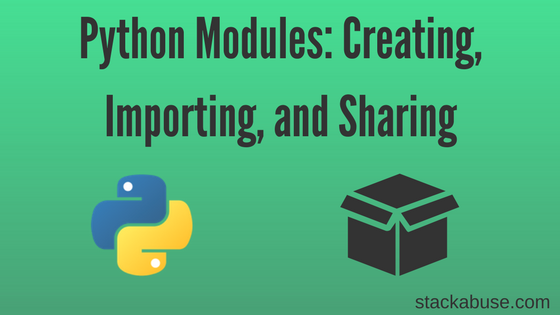

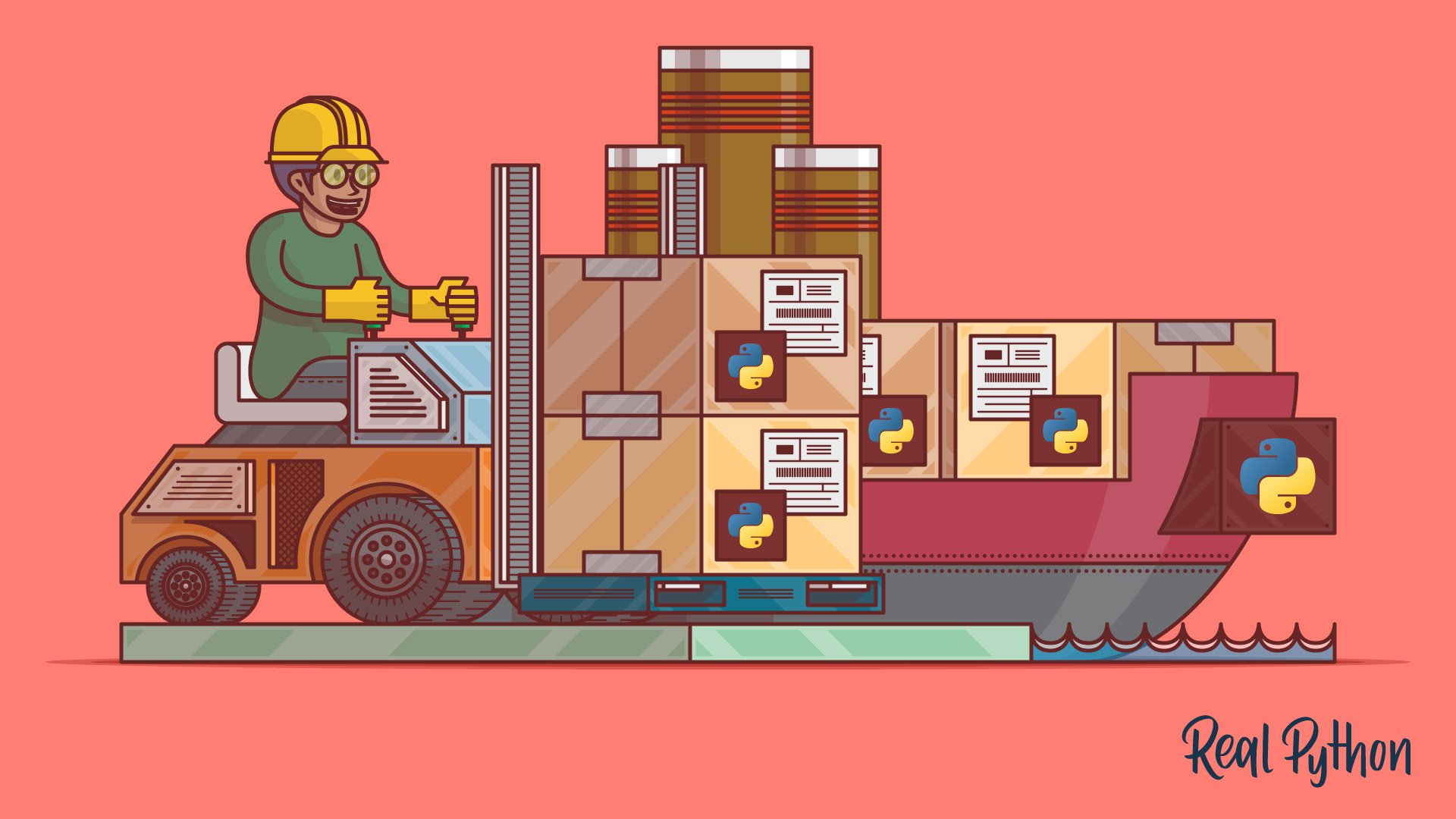
Article link: importing from parent directory python.
Learn more about the topic importing from parent directory python.
- Python – Import from parent directory – GeeksforGeeks
- python – Importing modules from parent folder – Stack Overflow
- How to Import File from Parent Directory in Python? (with code)
- How to Import File from Parent Directory in Python? (with code)
- Python – Import from parent directory – GeeksforGeeks
- Python Import From a Parent Directory: A Quick Guide
- How To Import Module From Parent Directory In Python In 2022?
- How can I import a module from a parent directory in Python?
- Python Import Module from Parent Directory in 3 Easy Steps
- Import From Parent Folder · Python Cook Book
- Import Modules From Parent Directory in Python | Delft Stack
- Python Import from Parent Directory in Simple Way
See more: nhanvietluanvan.com/luat-hoc