Import Parent Directory Python
In Python, the parent directory refers to the directory that contains the current directory. It is a crucial concept in file and directory navigation, especially when working with modules and packages. By understanding how to access and import modules from the parent directory, you can effectively organize your code and utilize resources from different locations.
Accessing the Parent Directory Using the os Module
One way to access the parent directory in Python is by using the os module. The os module provides a wide range of functions for interacting with the operating system, including file operations.
To access the parent directory, you can make use of the os.path.dirname() function. This function returns the parent directory of a given path. For example:
“`python
import os
current_directory = os.getcwd()
parent_directory = os.path.dirname(current_directory)
“`
In this example, the current_directory variable stores the path of the current directory, while parent_directory stores the path of the parent directory.
Navigating to the Parent Directory Using the pathlib Module
Alternatively, you can use the pathlib module to navigate to the parent directory. The pathlib module provides an object-oriented approach to working with file system paths.
To navigate to the parent directory, you can make use of the parent attribute of a pathlib.Path object. For example:
“`python
from pathlib import Path
current_directory = Path.cwd()
parent_directory = current_directory.parent
“`
In this example, the current_directory variable stores a pathlib.Path object representing the current directory, while parent_directory stores the parent directory.
Importing Modules from the Parent Directory
Once you have accessed or navigated to the parent directory, you may need to import modules from it. This is useful when you want to reuse code or access resources that are located in the parent directory.
To import a module from the parent directory, you can modify the sys.path list to include the parent directory. For example:
“`python
import sys
parent_directory = os.path.dirname(os.getcwd())
sys.path.append(parent_directory)
from parent_module import some_function
“`
In this example, we first add the parent directory to the sys.path list using the append() method. Then, we can import the desired module using the regular import statement.
Handling Parent Directory Imports in Different Scenarios
Handling parent directory imports can become slightly more complex in certain scenarios. For instance, if you have a package structure with multiple levels of directories, you may need to adjust your import statements accordingly.
To import a module from a parent directory in a package structure, you can make use of relative imports. Using relative imports, you can specify the location of the module relative to the current file or package.
For example, if you have the following structure:
“`
– parent_directory/
– package/
– __init__.py
– module.py
– main.py
“`
And you want to import the module.py file in main.py, you can use the following import statement:
“`python
from ..package import module
“`
Here, the “..” represents the parent directory relative to the current file. This allows you to import modules from the parent directory in a structured manner.
Best Practices and Potential Issues with Parent Directory Imports
While importing modules from the parent directory can be convenient, it is important to follow best practices to ensure clean and maintainable code.
One common potential issue when importing modules from the parent directory is the “attempted relative import beyond top-level package” error. This occurs when you try to perform a relative import from a script that is not part of a package.
To avoid this error, it is recommended to use absolute imports whenever possible. Absolute imports specify the full path of the module, starting from the root package.
For example, instead of using a relative import, you can use the following absolute import statement:
“`python
from parent_directory.package import module
“`
This ensures that the import statement is unambiguous and can be used from any script or package within the project.
Another best practice is to use the “__all__” variable in your modules. The “__all__” variable allows you to specify the public interface of your module, defining which attributes should be visible when using the “from module import *” syntax.
By explicitly defining the “__all__” variable, you can control what gets imported and avoid polluting the global namespace.
In conclusion, understanding how to import modules from the parent directory in Python is essential for effective code organization and resource utilization. By using the os or pathlib modules, navigating to the parent directory becomes straightforward. However, it is important to follow best practices, handle potential issues, and use relative or absolute imports appropriately to maintain clean and maintainable code.
FAQs
Q: How can I import a module from another directory in Python?
A: To import a module from another directory, you can modify the sys.path list to include the desired directory. Then, you can use the regular import statement to import the module.
Q: How do I get the parent directory in Python?
A: You can use the os module and its os.path.dirname() function to get the parent directory of a given path.
Q: How do I import modules from the parent directory in Python3?
A: You can modify the sys.path list to include the parent directory, and then use the regular import statement to import the desired module.
Q: What does “attempted relative import beyond top-level package” mean?
A: This error occurs when you try to perform a relative import from a script that is not part of a package. To avoid this error, it is recommended to use absolute imports whenever possible.
Q: How do I import a function from the parent folder in Python?
A: You can modify the sys.path list to include the parent directory, and then use the regular import statement to import the desired function.
Q: Can I import a module from a sibling directory in Python?
A: Yes, you can import a module from a sibling directory by modifying the sys.path list to include the sibling directory, and then using the regular import statement to import the desired module.
Q: What is the “__all__” variable in Python?
A: The “__all__” variable is a list that defines the public interface of a module. It specifies which attributes should be visible when using the “from module import *” syntax.
Q: How do I import a module in Python from a specific path?
A: You can use the importlib module and its import_module() function to import a module from a specific path. Additionally, you can modify the sys.path list to include the desired path, and then use the regular import statement to import the module.
Python – Importing Your Modules (Part 2: Import From A Different Folder ~7 Mins! No Ads)
Keywords searched by users: import parent directory python Python import module from another directory, Get parent directory Python, Python3 import from parent directory, attempted relative import beyond top-level package, Import function from parent folder python, Python import from sibling directory, Python __all__, Import module Python from path
Categories: Top 38 Import Parent Directory Python
See more here: nhanvietluanvan.com
Python Import Module From Another Directory
Python is a versatile and powerful programming language used for a wide range of applications, from web development to scientific computing. It provides a vast number of built-in modules and libraries to simplify programming tasks. However, there may come a time when you need to import a module from another directory. In this article, we will explore different methods to achieve this and address some frequently asked questions regarding this topic.
Importing a module from another directory can be necessary if you have organized your code into different directories or if the module you want to import is located outside the current working directory. Python offers several ways to accomplish this task. We will discuss two common methods: modifying the sys.path and using the package structure.
1. Modifying the sys.path
The sys module in Python provides access to various system-specific parameters and functions. By adding the desired directory to the sys.path list, the Python interpreter will search for modules in that directory. This can be achieved by appending the desired path to sys.path. Consider the following example:
import sys
sys.path.append(‘/path/to/directory’)
In this code snippet, we import the sys module and append the desired directory path to sys.path. Once added, Python will search for modules in this directory when import statements are encountered. However, it is important to ensure that the specified path is correct and exists; otherwise, a ModuleNotFoundError will be raised.
2. Using the package structure
Python allows you to structure your code into packages, which are directories containing multiple modules. By utilizing the package structure, you can import modules from other directories simply by specifying the package name. To achieve this, follow these steps:
a. Create an empty __init__.py file in the directory you want to import from. This file is necessary for Python to treat the directory as a package.
b. In the file where you want to import the module, use the following syntax:
from package_name import module_name
or
import package_name.module_name
In the first syntax, you import the desired module directly into the current namespace. In the second syntax, you import the entire package and access the module using package_name.module_name. Both ways achieve the same result of importing the module from the desired directory.
Frequently Asked Questions:
Q: Can I import a module from a completely different directory?
A: Yes, you can import a module from a completely different directory using the sys.path method described earlier. By adding the desired directory to sys.path, the Python interpreter will search for modules in that directory when encountering import statements.
Q: What happens if I import a module with the same name from two different directories?
A: Python will import the module that appears first in the sys.path list. If two modules have the same name, it is important to ensure that their import order is correct to avoid conflicts.
Q: How can I import a module from a parent directory?
A: To import a module from a parent directory, you can use the package structure. By specifying the parent package name in the import statement, you can access modules from the parent directory.
Q: Is it considered good practice to modify the sys.path?
A: Modifying the sys.path should generally be avoided, as it can lead to confusion and potential conflicts. It is recommended to use the package structure whenever possible.
Q: Can I import a module from a specific subdirectory within a directory?
A: Yes, by properly structuring your code into packages and including the desired subdirectory structure, you can import modules from specific subdirectories within a directory.
In conclusion, Python provides various methods to import modules from another directory. By modifying the sys.path or utilizing the package structure, you can easily access modules located outside the current working directory. It is essential to pay attention to the correct directory structure and ensure that modules are imported in the appropriate order to prevent conflicts and errors.
Get Parent Directory Python
In Python, dealing with file and directory paths is a common task for many programmers. The ability to navigate through the file system and retrieve information about parent directories is essential when working with file management. In this article, we will explore the techniques and functions available in Python to get the parent directory of a given file or directory.
Understanding File Paths in Python
Before diving into the details of getting the parent directory, let’s first understand how file paths are represented in Python.
In Python, file paths can be represented as strings. The format of the file path string depends on the operating system you are using. In Windows, file paths are typically represented using backslashes (‘\\’) as the path separator, while in Unix-based systems (like Linux and macOS), forward slashes (‘/’) are used as path separators.
Python provides the `os` module, which includes several functions for working with file paths. Additionally, the `os.path` module offers specific functions dedicated to path manipulation, such as `os.path.dirname()`, which we will discuss in more detail later.
Get Parent Directory using `os.path.dirname()`
The `os.path.dirname()` function from the `os.path` module allows us to retrieve the directory name of a given file path. It essentially returns the parent directory.
Here is an example of how to use `os.path.dirname()` to get the parent directory:
“`python
import os
file_path = ‘/path/to/file.txt’
parent_directory = os.path.dirname(file_path)
print(parent_directory)
“`
Output:
“`
/path/to
“`
As shown in the example, the `os.path.dirname()` function extracts the parent directory ‘/path/to’ from the given file path ‘/path/to/file.txt’.
This function works for both Windows and Unix-based systems, as it automatically adjusts the path separator based on the operating system.
Get Parent Directory using `Path` object from `pathlib`
Starting from Python 3.4, the `pathlib` module was introduced, providing a more intuitive and object-oriented approach to working with file paths. The `Path` object can be utilized to get the parent directory using the `parent` property.
Here is an example using the `Path` object to retrieve the parent directory:
“`python
from pathlib import Path
file_path = Path(‘/path/to/file.txt’)
parent_directory = file_path.parent
print(parent_directory)
“`
Output:
“`
/path/to
“`
As demonstrated, the `Path` object’s `parent` property gives us direct access to the parent directory without the need for additional functions.
Frequently Asked Questions (FAQs):
Q: Can I get the parent directory of a directory using the same methods?
A: Yes, both `os.path.dirname()` and the `Path` object can be used to retrieve the parent directory of both files and directories.
Q: What happens if the given file path is already the root directory?
A: In such cases, the `os.path.dirname()` function will return the same root directory path, while the `Path` object’s `parent` property will return `None`. For example, if the file path is ‘/root/file.txt’, `dirname()` will return ‘/root’ while `parent` will be `None`.
Q: Is it possible to get the parent directory of a given file or directory without using any external libraries?
A: Yes, you can achieve this by using string manipulation techniques. For example, by splitting the path string at the path separator and rejoining the resulting list excluding the last element, you can obtain the parent directory. However, using `os.path` or `pathlib` modules provides a more consistent and reliable approach.
Q: Can I get the grandparent directory or any other ancestor directory using the same methods?
A: Yes, you can use either `os.path.dirname()` or the `Path` object’s `parent` property repeatedly for retrieving ancestor directories. For example, to get the grandparent directory, you can apply `os.path.dirname()` twice or access the `parent` property of the `Path` object twice.
Conclusion
Working with file paths and navigating through directories is crucial when dealing with file management tasks in Python. By utilizing functions like `os.path.dirname()` or the `Path` object’s `parent` property, you can easily retrieve the parent directory of a given file or directory. Understanding these techniques will greatly enhance your ability to work with file systems efficiently.
Images related to the topic import parent directory python
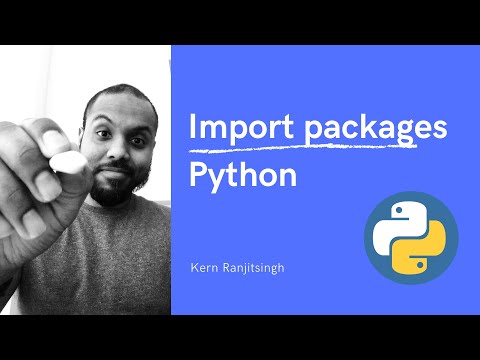
Found 30 images related to import parent directory python theme
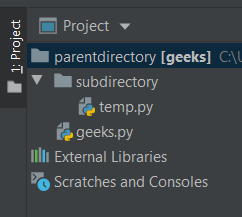
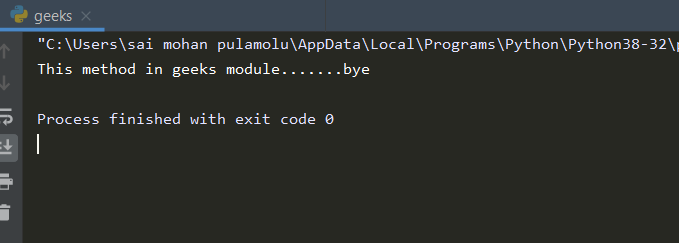

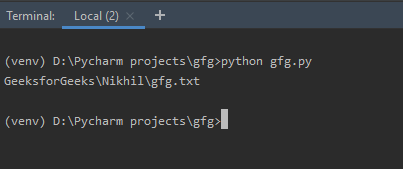
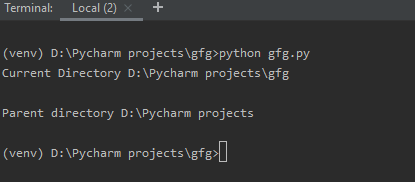
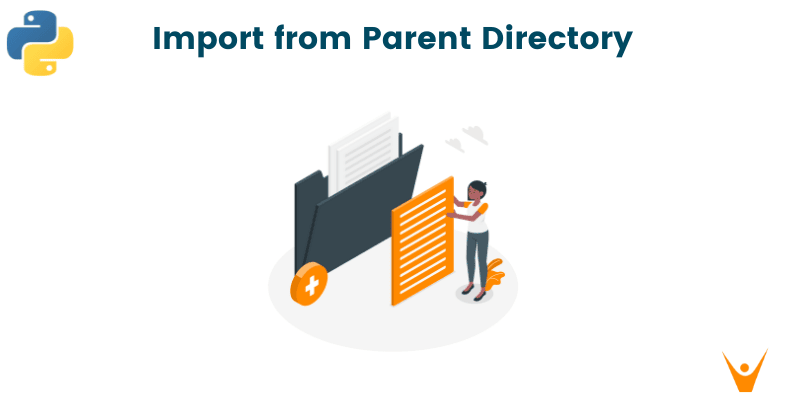
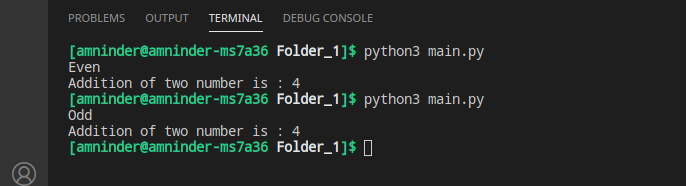
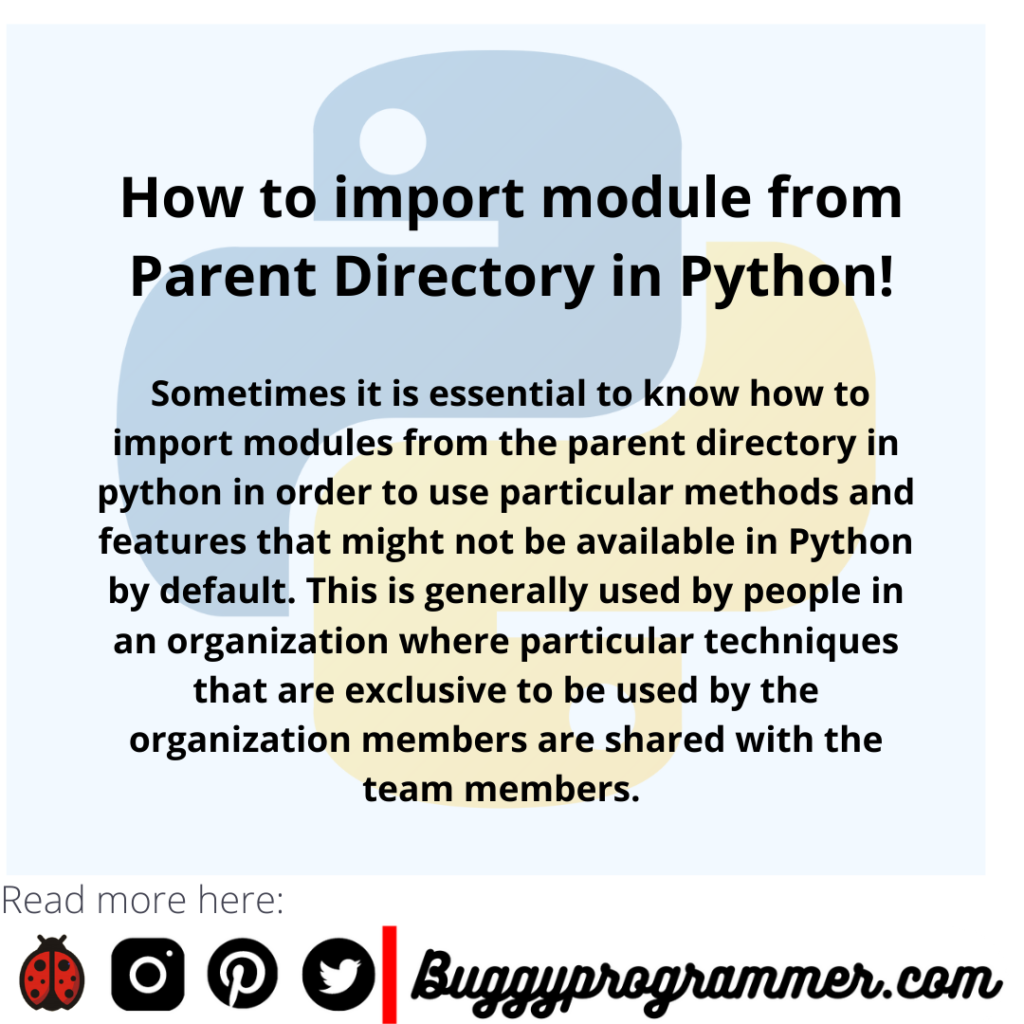



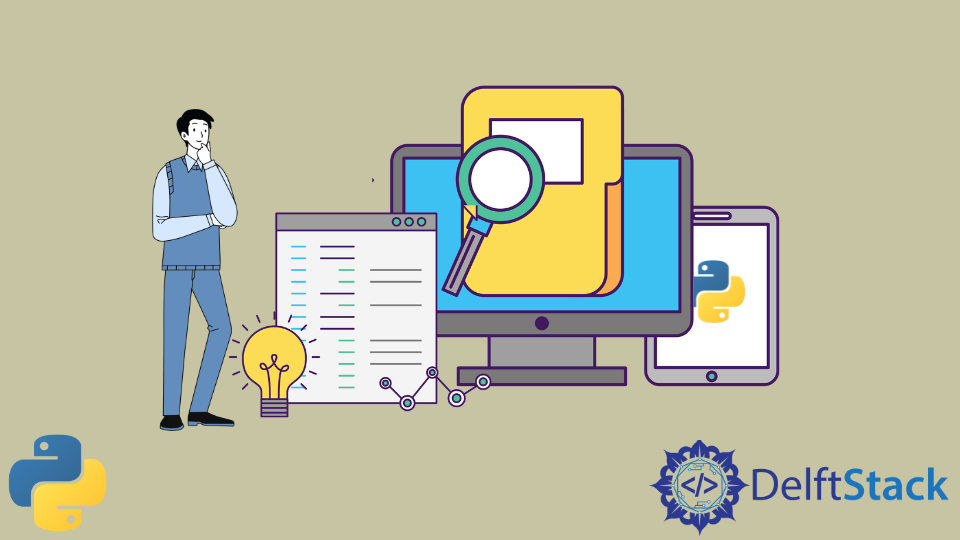
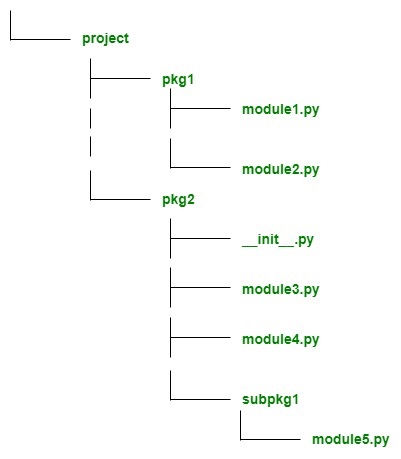
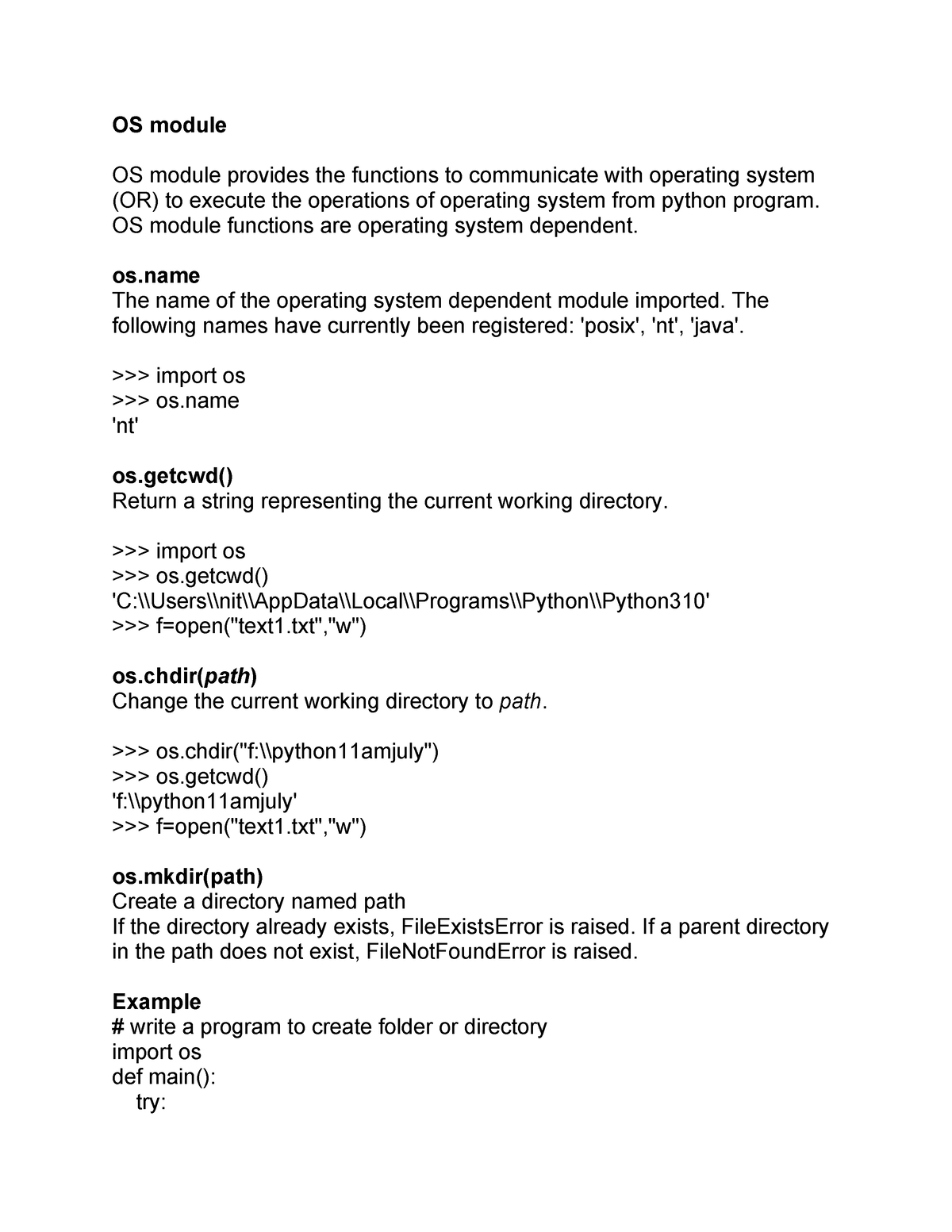

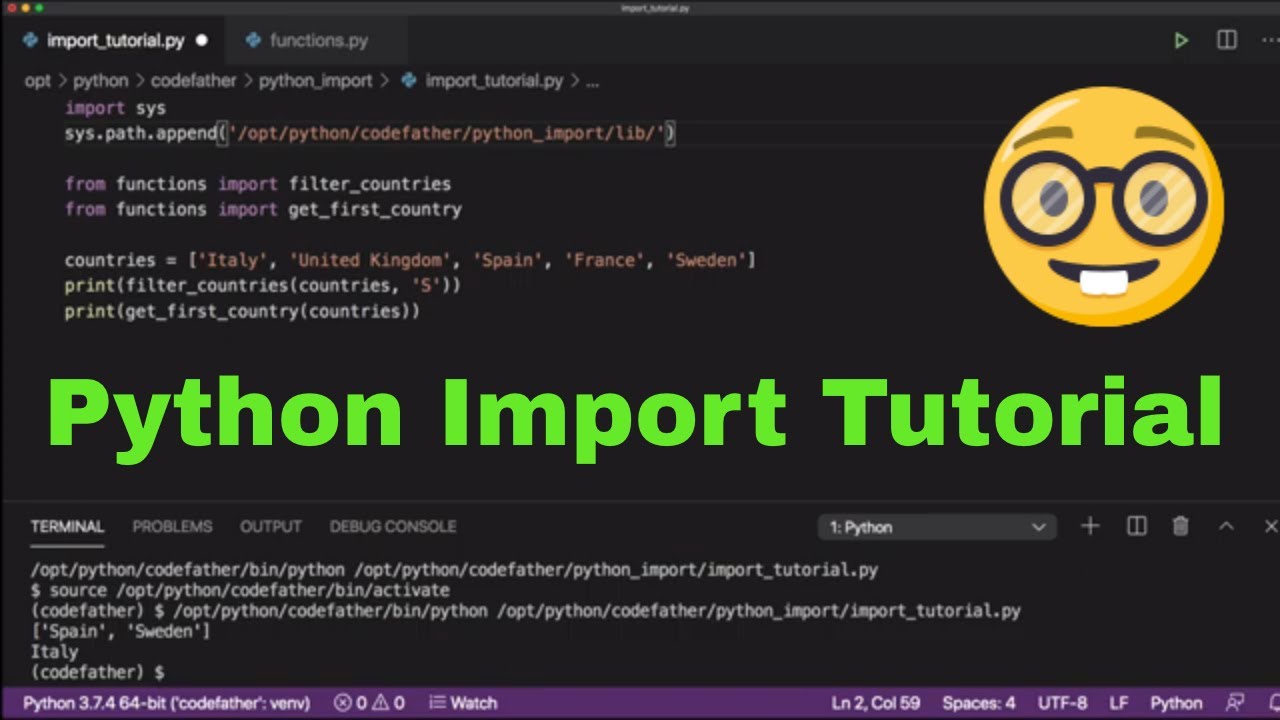

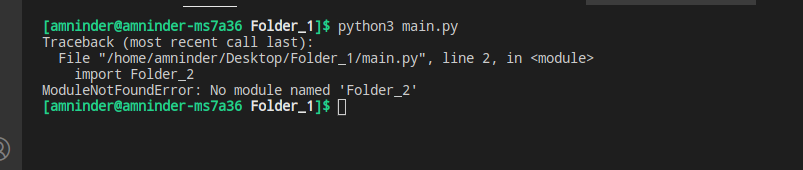


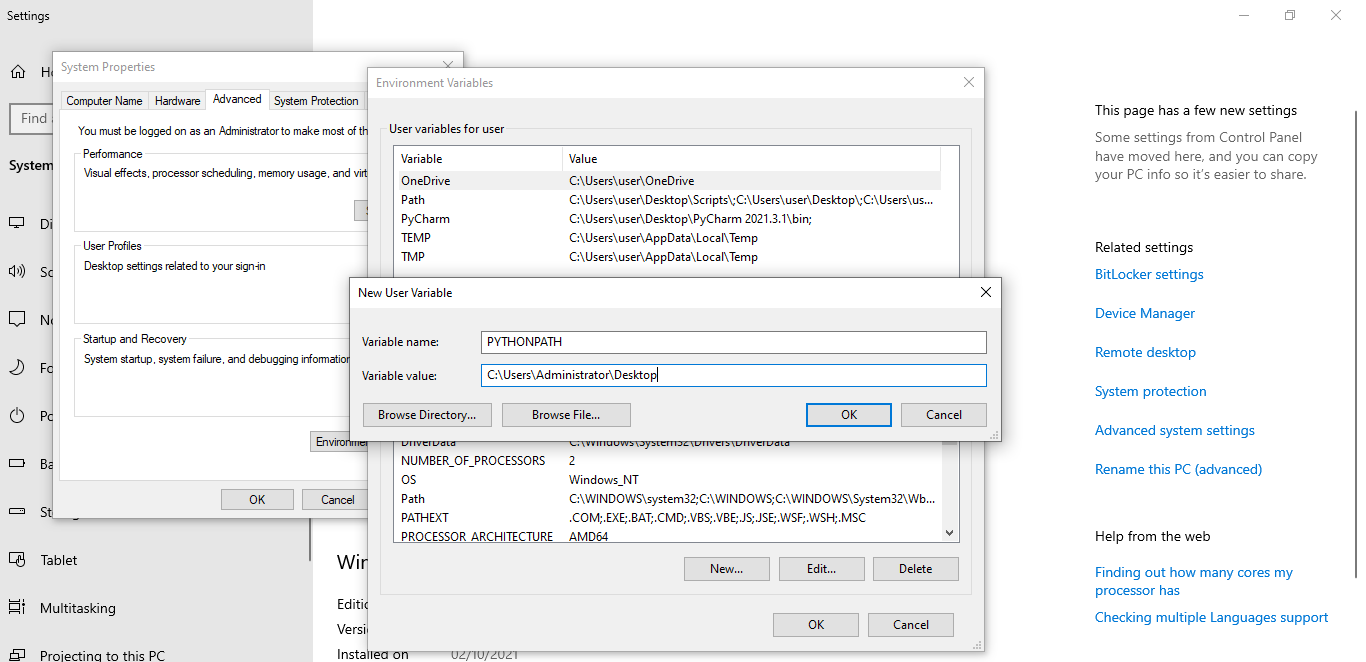
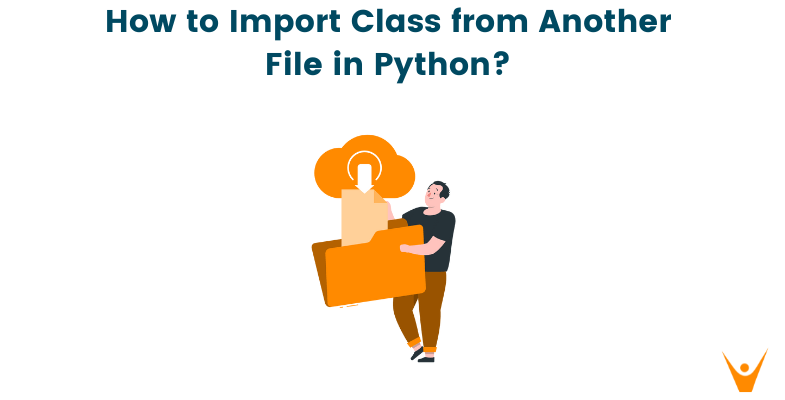

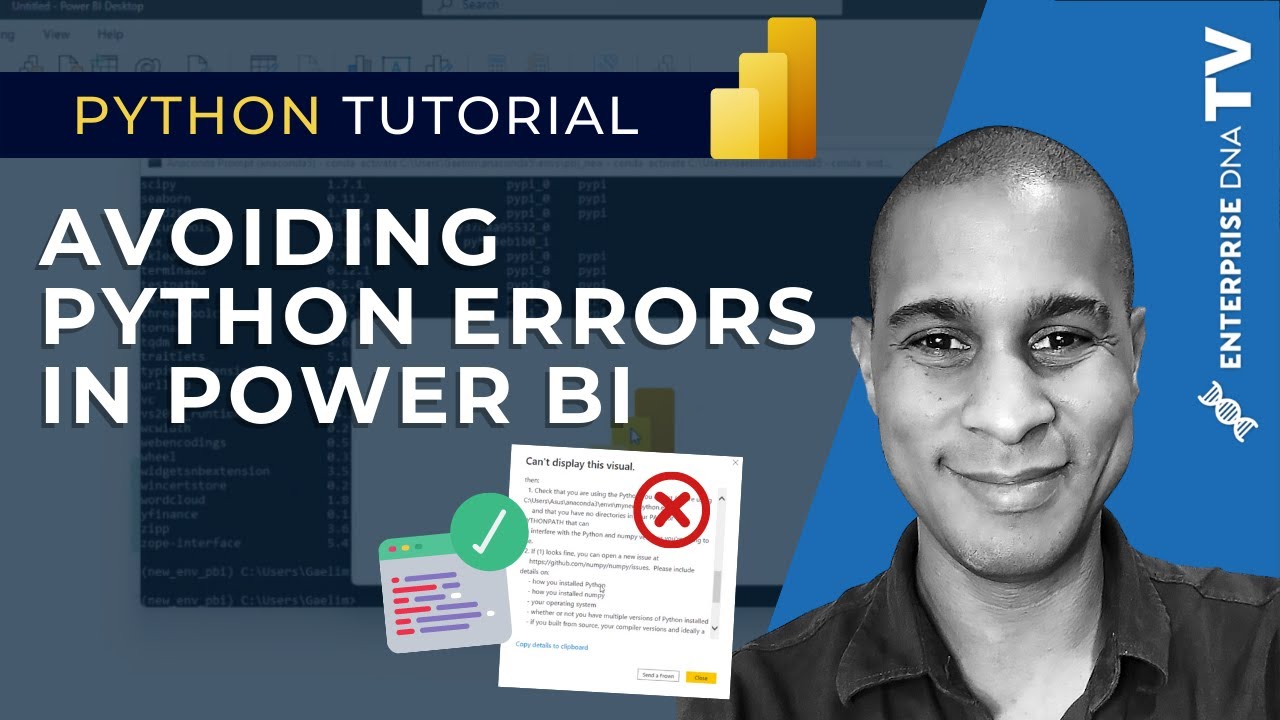
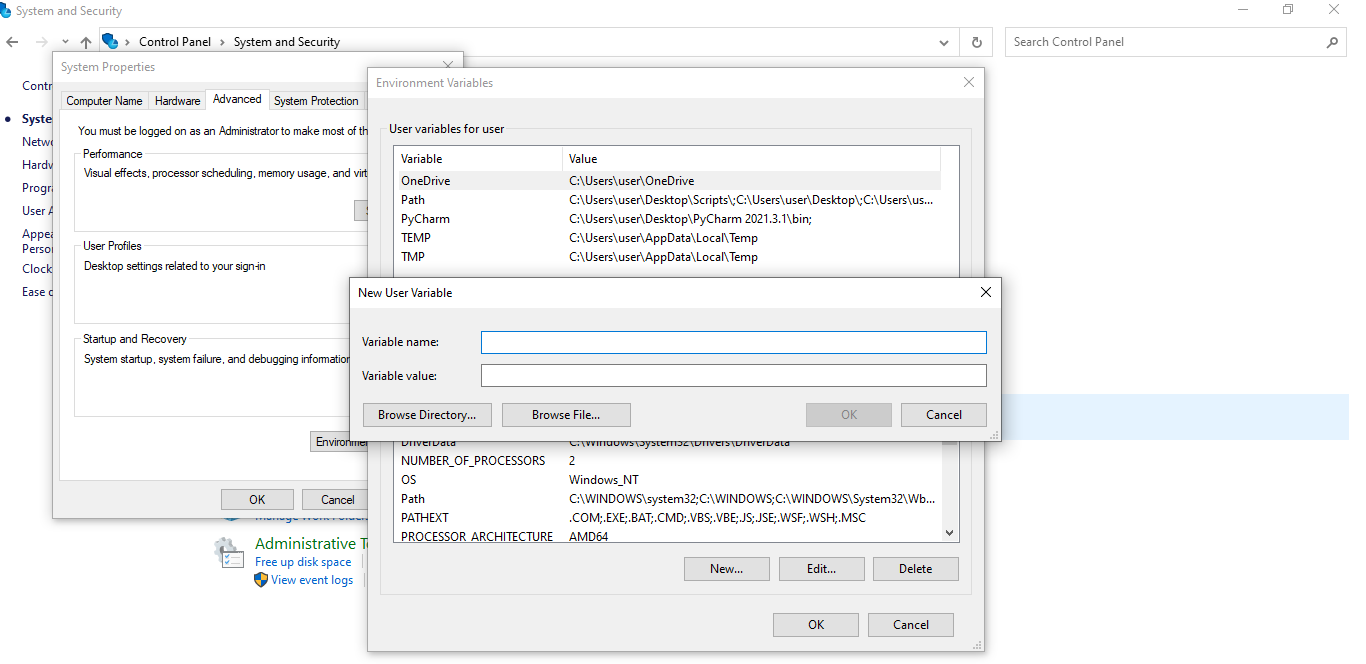
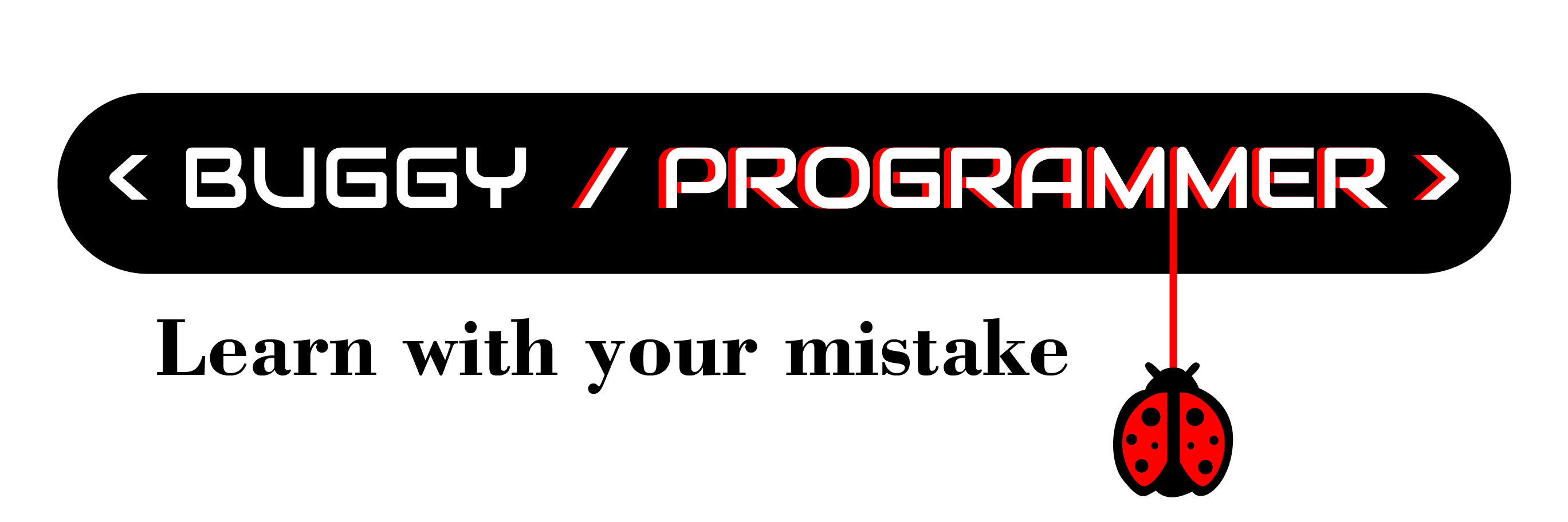
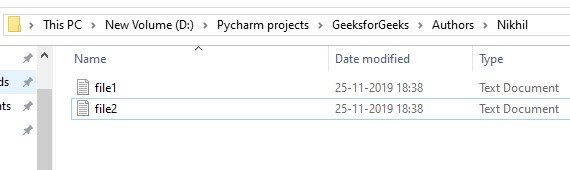

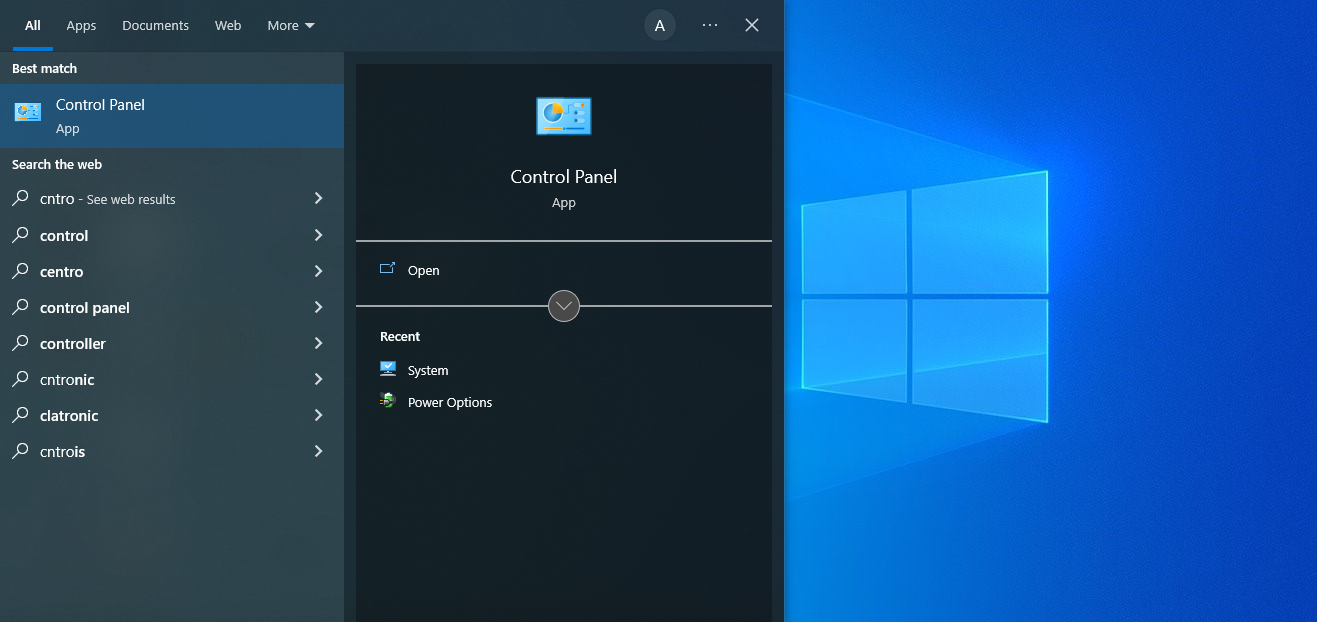
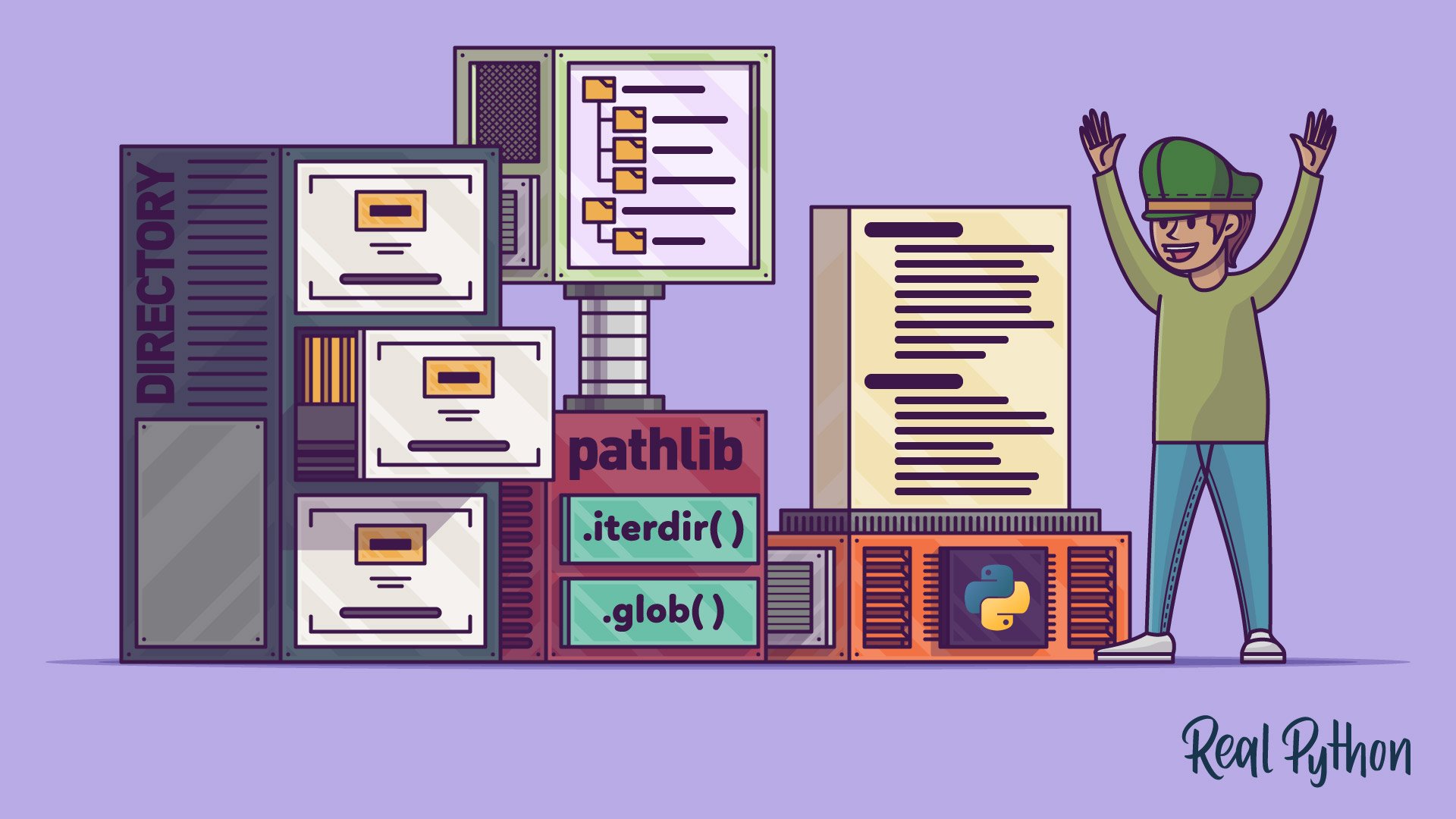
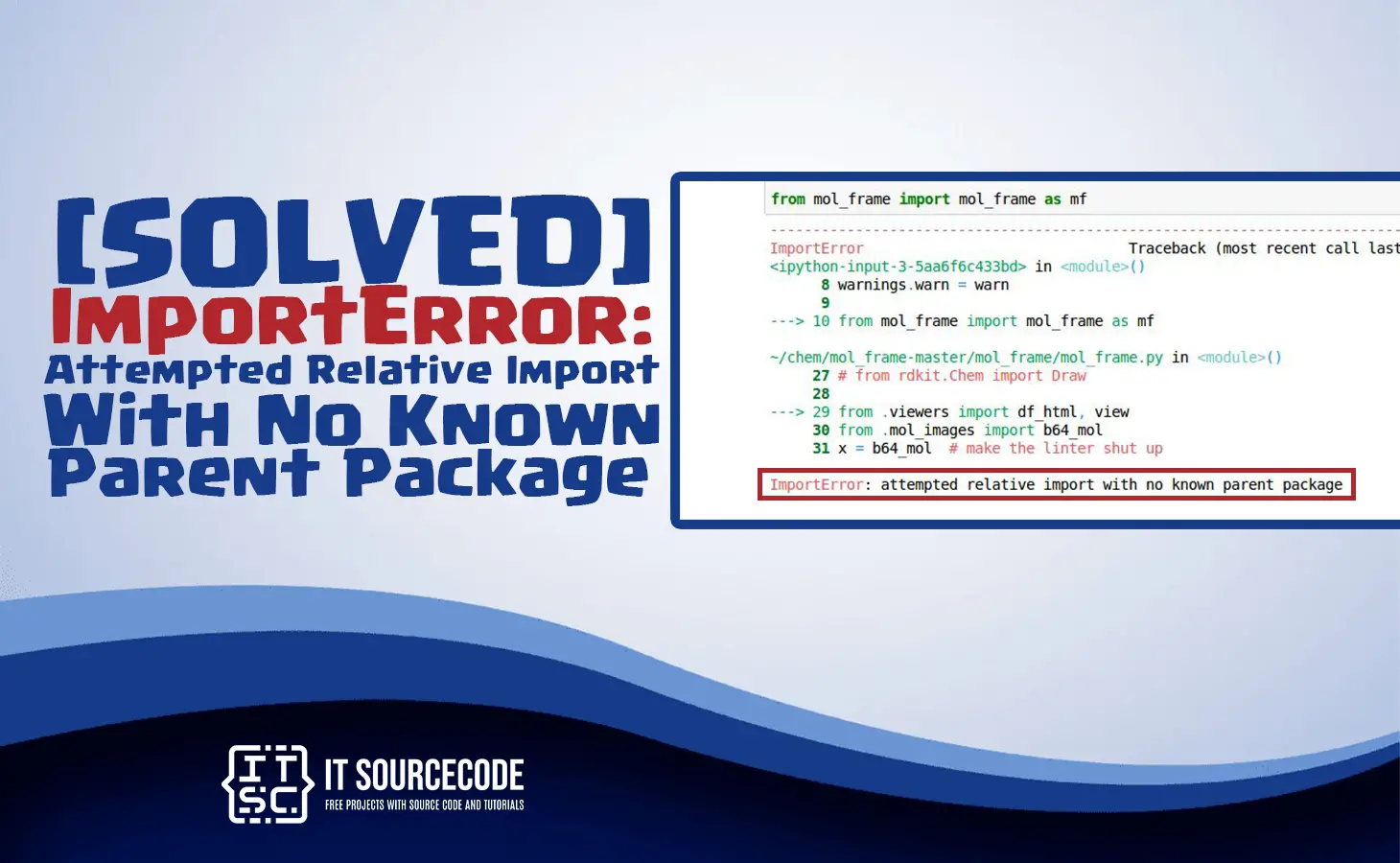
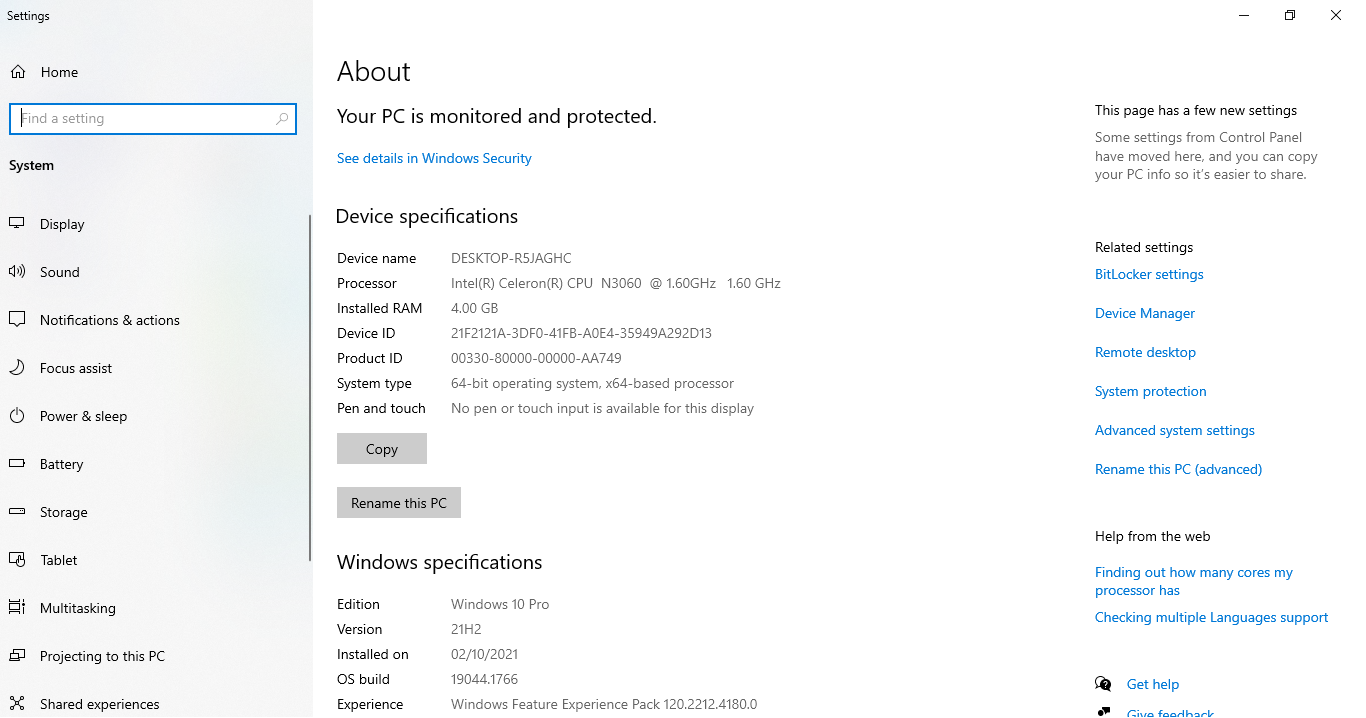

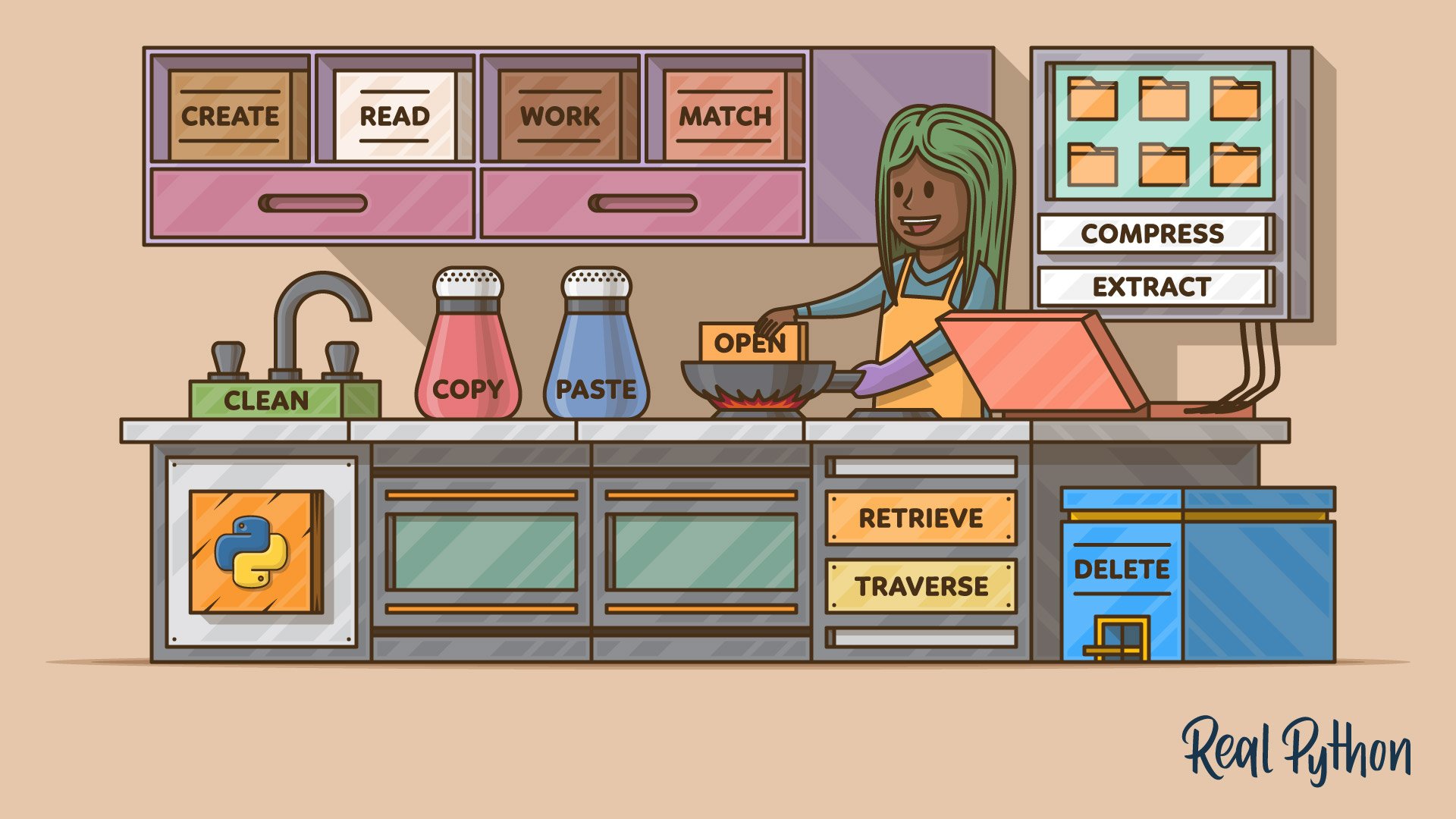
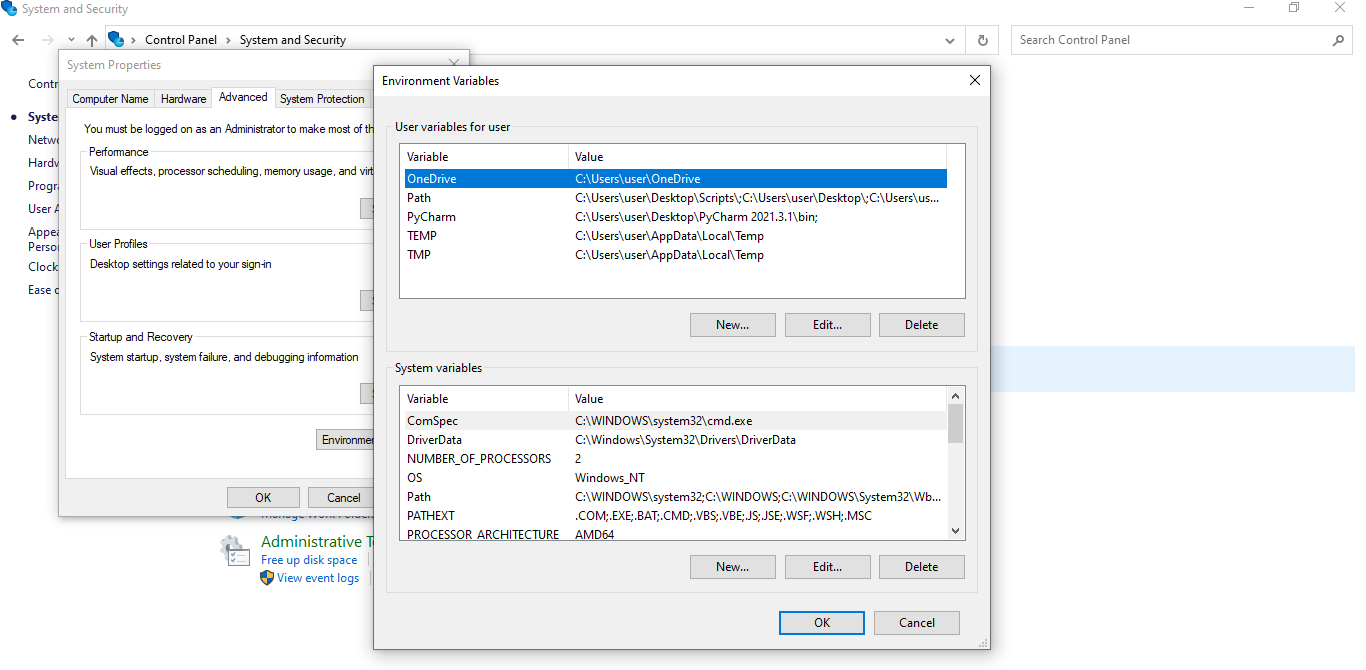

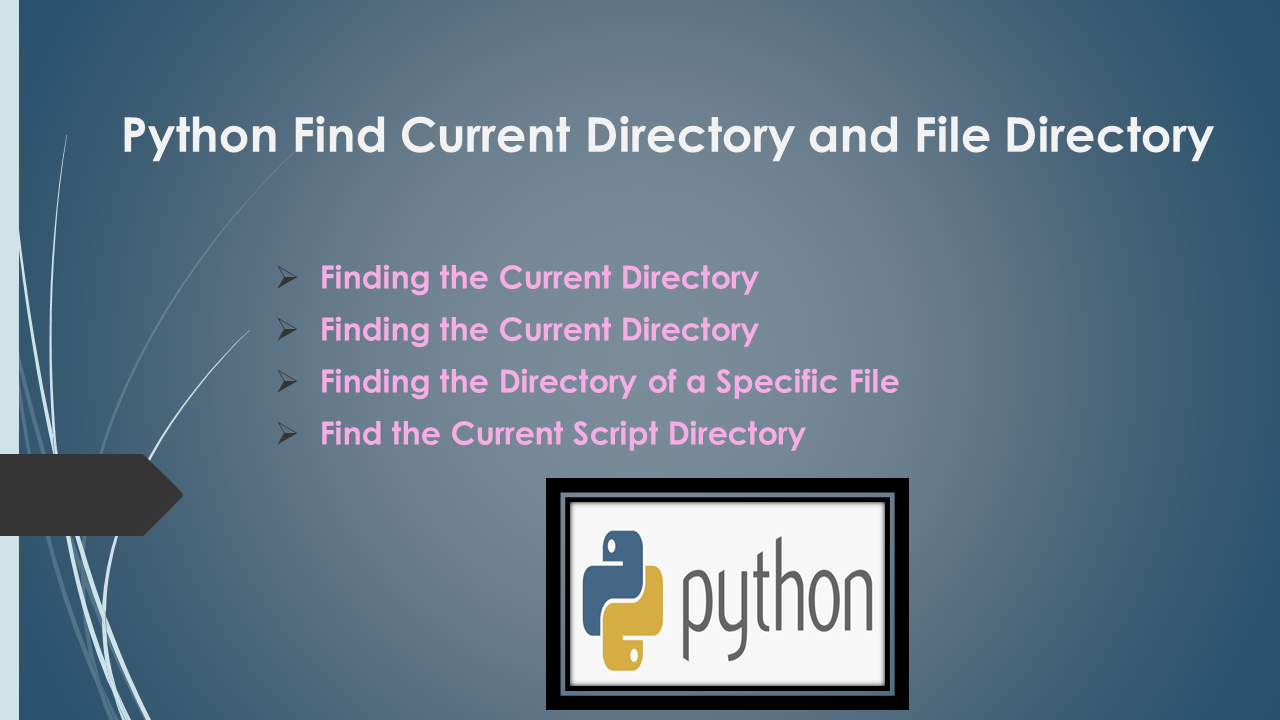
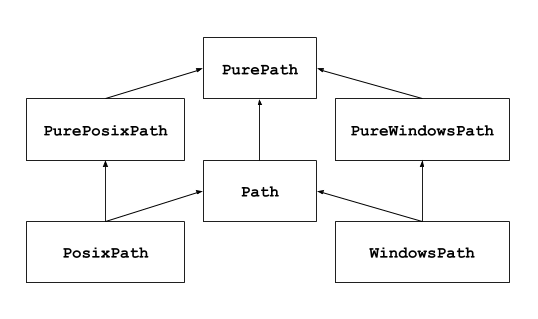

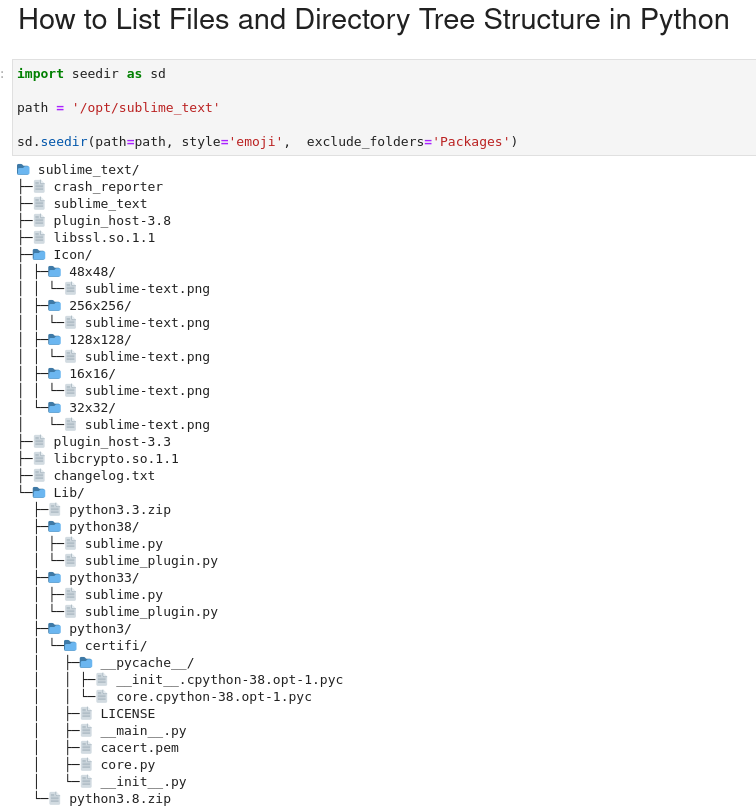


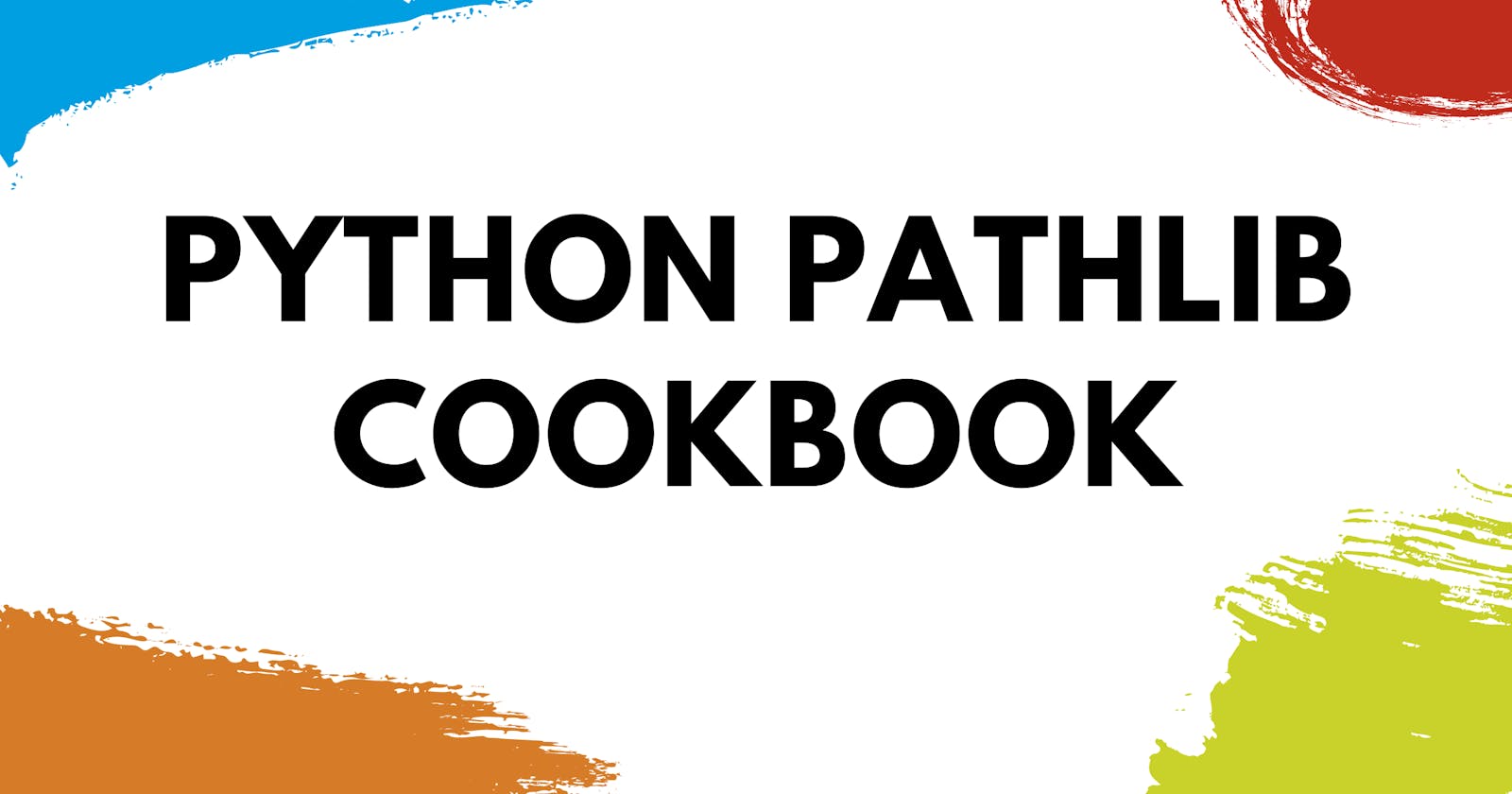
![FileNotFoundError: [Errno 2] No such file or directory: 'FSnm.png' - 🚀 Deployment - Streamlit Filenotfounderror: [Errno 2] No Such File Or Directory: 'Fsnm.Png' - 🚀 Deployment - Streamlit](https://global.discourse-cdn.com/business7/uploads/streamlit/optimized/2X/7/7a62f10889b442beaf7a1b533406adf4e2b4f636_2_1024x506.png)



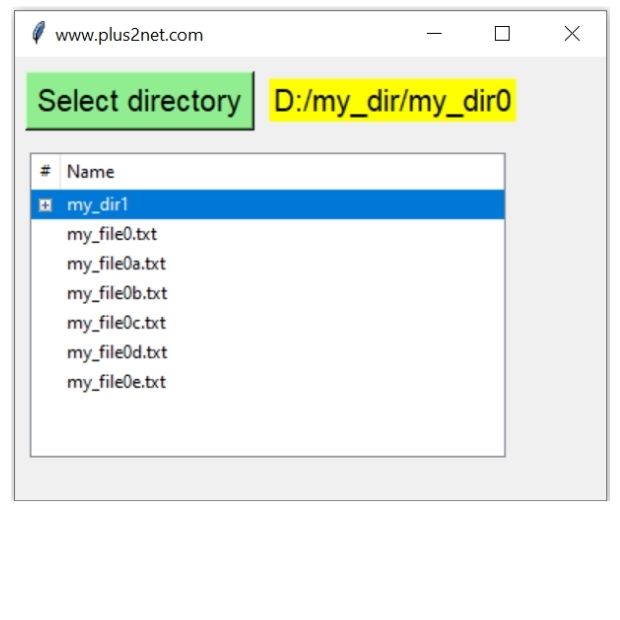
Article link: import parent directory python.
Learn more about the topic import parent directory python.
- Python – Import from parent directory – GeeksforGeeks
- Importing modules from parent folder – python – Stack Overflow
- How to Import File from Parent Directory in Python? (with code)
- Python Import From a Parent Directory: A Quick Guide
- How can I import a module from a parent directory in Python?
- How To Import Module From Parent Directory In Python In 2022?
- Python Import from Parent Directory in Simple Way
- Python Import Module from Parent Directory in 3 Easy Steps
- Import From Parent Folder · Python Cook Book
- Adding Parent Directory To Python Path – Krystian Safjan’s Blog
See more: nhanvietluanvan.com/luat-hoc