How To Remove Empty Row In R
Removing empty rows in R is a common task when working with dataframes, as these empty rows can affect data analysis and visualization. In this article, we will explore various methods to identify and remove empty rows in R, including using dplyr and tidyverse packages. We will also cover removing rows with NA values, empty cells, blank values, missing data, and null cells. Let’s dive in!
Identifying Empty Rows in a Dataframe
Before we remove empty rows, it is essential to identify them accurately. By understanding the structure of the data, we can pinpoint the rows with missing or empty values. In R, we can use functions such as is.na(), is.null(), is.empty(), and is.blank() to identify empty rows in a dataframe.
Removing Rows with NA Values
One common scenario is when we have missing values represented as NA in our dataframe. We can remove these rows using the complete.cases() function, which returns a logical vector representing rows with complete observations. Here’s an example:
“`R
data <- data[complete.cases(data), ]
```
In the above code, we overwrite the original dataframe with only the rows that have complete observations. This will effectively remove any rows with missing NA values.
Removing Rows with Empty Cells
Sometimes, empty cells in a dataframe can also indicate missing data. To remove rows with empty cells, we can use the complete() function from the tidyr package. This function will return a dataframe with all missing or empty cells filled in.
```R
library(tidyr)
data <- complete(data)
```
By calling the complete() function, we replace rows with missing values in any column of the dataframe with complete observations. This can help us deal with rows containing empty cells efficiently.
Removing Rows with Blank Values
In some cases, empty cells might be represented by blank values instead of NA. To remove rows with blank values, we need to first convert these blank values to NA and then use the complete.cases() function as described earlier. Here's how we can do it:
```R
data[data == ""] <- NA
data <- data[complete.cases(data), ]
```
The first line replaces all blank values in the dataframe with NA, and the second line removes the rows with missing values.
Deleting Rows with Missing Data
In situations where we want to remove rows with missing or incomplete data, we can use the na.omit() function. This function removes rows with any missing values and returns the modified dataframe:
```R
data <- na.omit(data)
```
By applying na.omit(), we can easily remove rows with any NA values from our dataframe.
Handling Rows with Null Cells
To remove rows with null cells, we can use the is.null() function to identify such values. Once identified, we can remove the rows using logical subsetting. Here's an example:
```R
data <- data[!is.null(data$column), ]
```
In the above code, we exclude rows with null values in a specific column from our dataframe.
Remove Empty Rows in R - FAQs
Q1: Can I use the dplyr package to remove empty rows in R?
Absolutely! The dplyr package is widely used for data manipulation tasks in R, including removing empty rows. You can use the filter() function from dplyr to filter out rows based on certain conditions. Here's an example that removes rows with NA values:
```R
library(dplyr)
data <- data %>%
filter(!is.na(column))
“`
Q2: What about removing empty rows using the tidyverse package?
The tidyverse package, which includes dplyr, provides a set of powerful tools for data manipulation. To remove empty rows using tidyverse, you can follow similar steps as mentioned earlier. Here’s an example using the drop_na() function from the tidyr package:
“`R
library(tidyverse)
data <- drop_na(data)
```
The drop_na() function removes any rows with missing values from your dataframe.
Q3: Is there a way to remove empty values in R without modifying the original dataframe?
Yes, you can create a new dataframe with non-empty rows that don't alter the original dataframe. Here's an example:
```R
new_data <- data[complete.cases(data), ]
```
In the above code, we create a new dataframe called new_data that only contains rows with complete observations.
Q4: Can I remove empty rows in an Excel file using R?
Yes, you can read an Excel file using a package like readxl, remove empty rows using the methods described in this article, and then write the modified dataframe back to an Excel file. Here's an example using the readxl and writexl packages:
```R
library(readxl)
library(writexl)
data <- read_excel("input.xlsx")
data <- data[complete.cases(data), ]
write_xlsx(data, "output.xlsx")
```
Q5: How can I remove empty columns in R?
To remove empty columns in R, we can use the colSums() function to calculate the sum of non-NA values in each column and then subset the original dataframe accordingly. Here's an example:
```R
data <- data[,colSums(!is.na(data)) > 0]
“`
In the above code, we select only the columns with a sum of non-NA values greater than zero.
Q6: How can I remove rows with blanks in a specific column?
To remove rows with blanks in a specific column, we can use logical subsetting. Here’s an example:
“`R
data <- data[data$column != "", ]
```
In the above code, we exclude rows with blanks (represented as "") in a specific column from our dataframe.
Q7: Is there a function to remove empty rows in R without explicitly specifying the conditions?
Yes, the remove_empty() function from the janitor package provides a simple approach to remove empty rows. Here's an example:
```R
library(janitor)
data <- remove_empty(data)
```
The remove_empty() function automatically detects and removes empty rows from the dataframe.
Q8: How can I delete a null row in R?
To delete a null row in R, you can use the is.null() function to identify the null rows and then subset the original dataframe excluding these rows. Here's an example:
```R
data <- data[!is.null(data), ]
```
By excluding null rows using logical subsetting, we can delete them from our dataframe effectively.
In conclusion, removing empty rows in R is essential for data cleaning and analysis tasks. By using the methods explained in this article, you can easily identify and remove empty rows, whether they contain NA values, empty cells, blank values, missing data, or null cells. Utilizing packages such as dplyr, tidyverse, janitor, and tidyr simplifies the process, making data manipulation in R more efficient and effective.
Remove Empty Rows Of Data Frame In R (2 Examples) | Apply, All, Rowsums, Is.Na \U0026 Ncol Functions
How To Remove All Null Rows In R?
Data cleaning is an essential step in any data analysis project. One common issue that data analysts frequently encounter is dealing with null values. Null values can present challenges in data analysis, as they can skew results and lead to inaccurate conclusions. Therefore, it is crucial to identify and remove null values from datasets before proceeding further. In this article, we will discuss how to remove all null rows in R, a powerful and widely used programming language for data analysis.
What are Null Values?
Null, or NA (Not Available), values represent missing or undefined data points in a dataset. Null values can occur for various reasons, such as measurement errors or incomplete data collection. These values, if left unhandled, can cause problems during data analysis, including biased or inaccurate results.
Identifying Null Values in R:
The first step in removing null rows in R is to identify the null values within a dataset. In R, you can use the function is.na() to check for null values. This function returns a logical vector of the same shape as the input, with TRUE values indicating null values and FALSE values indicating non-null values.
For example, consider a dataset called “mydata” with three variables: “A”, “B”, and “C”. To check for null values in this dataset, you can use the following code:
“`
null_rows <- apply(mydata, 1, function(x) any(is.na(x)))
```
In this code, the apply() function is used to iterate over each row of the dataset. Inside the apply() function, the function is.na() is applied to each row vector "x" to check for null values. The any() function is then used to determine if any null value is present in a row. The resulting logical vector "null_rows" will contain TRUE values for null rows and FALSE values for non-null rows.
Removing Null Rows in R:
Once you have identified the null rows, you can proceed to remove them from the dataset. To remove null rows in R, you can use the subsetting technique.
```
clean_data <- mydata[!null_rows, ]
```
In the above code, the negation operator "!" is used to select the rows that are not null. Consequently, the resulting dataframe "clean_data" will only contain non-null rows.
Alternatively, you can also use the complete.cases() function to remove the null rows.
```
clean_data <- mydata[complete.cases(mydata), ]
```
The complete.cases() function returns a logical vector indicating complete cases (rows without any missing value). By using this function as an index, you can subset the dataset to include only the complete cases.
Frequently Asked Questions:
Q: Can I use the na.omit() function to remove null rows instead of subsetting?
A: Yes, you can use the na.omit() function to remove null rows. The na.omit() function removes rows with any missing values from a dataframe. However, note that na.omit() will remove the entire row, including non-null values, if any missing value is present.
Q: What if I want to remove null rows for specific variables rather than the entire dataset?
A: You can modify the code accordingly to subset the dataset based on specific variable(s). For example, if you want to remove null rows only for variable "A", you can use the following code:
``` clean_data <- mydata[!is.na(mydata$A), ] ```
This code will remove rows with null values in variable "A" while retaining the rest of the dataset.
Q: How can I remove null rows from a list or a matrix?
A: The process is similar for lists and matrices. You can use the same approaches discussed above by applying the appropriate functions to the respective data structures.
Q: Are there any potential drawbacks of removing null rows?
A: Removing null rows can alter the size and structure of your dataset, potentially impacting subsequent analyses. It is essential to carefully consider the reasons for null values and the implications of their removal on your analysis. Additionally, removing null rows can lead to a loss of valuable information if the null values carry meaningful insights in your data.
Q: Is it possible to replace null values with other values instead of removing the entire row?
A: Yes, it is possible to replace null values with other values. R provides various functions, such as replace() or na.locf(), that allow you to replace missing values with specific values or with nearby non-null values.
Conclusion:
Null values can cause problems during data analysis, but R provides several methods to remove them effectively. By identifying the null rows using is.na(), you can subset the dataset to include only non-null rows. Additionally, the complete.cases() function can be used to subset the dataset using logical indexing. However, before removing null rows, it is crucial to consider their implications and potential loss of information. Understanding how to remove null rows in R will help ensure accurate and reliable data analysis results.
How To Remove Rows From Dataframe In R Based On Condition?
In data analysis using R, it is common to work with large datasets that may contain unwanted or irrelevant information. As a result, removing specific rows from a dataframe based on a certain condition becomes essential. This article will guide you on how to remove rows from a dataframe in R based on a condition efficiently and effectively.
1. Subset Method:
One straightforward approach to removing rows from a dataframe in R is by using the subset() function. This function allows you to create a new dataframe containing only the rows that match a specified condition. Here’s the general syntax:
new_dataframe <- subset(original_dataframe, condition) For example, let's say we have a dataframe called "data" with columns "age" and "gender". Suppose we want to remove all rows where the age is less than 18: new_data <- subset(data, age >= 18)
In this case, the new_dataframe will only include rows where the age is greater than or equal to 18.
2. Filter Method (dplyr):
Another efficient way to remove rows from a dataframe based on a condition is by using the filter() function from the dplyr package. This package provides a set of tools for data manipulation that are optimized for speed and ease of use. Here’s how to use the filter() function:
install.packages(“dplyr”) # If you haven’t installed it already
library(dplyr)
new_dataframe <- filter(original_dataframe, condition) Following our previous example, we can remove rows where the age is less than 18 using the filter() function: new_data <- filter(data, age >= 18)
The resulting new_dataframe will contain only the rows where the age is greater than or equal to 18.
3. Remove Rows with NA Values:
In some cases, you may need to remove rows from a dataframe that have missing or NA (Not Available) values in specific columns. The na.omit() function in R helps to achieve this by removing any rows that contain NA values. Here’s how to use it:
new_dataframe <- na.omit(original_dataframe)
For instance, let's consider a dataframe called "df" with columns "name" and "age". To remove rows with NA values in the "age" column:
new_df <- na.omit(df)
The resulting new_dataframe will exclude any row that has NA in the "age" column.
FAQs:
Q1. Can I directly modify the original dataframe when removing rows based on a condition?
A1. Yes, you can modify the original dataframe directly using the assignment operator "<-" and overwrite it with the filtered dataframe. For example:
- data <- data[data$age >= 18, ]
– df <- df[complete.cases(df$age), ]
Q2. How can I remove rows based on multiple conditions?
A2. To remove rows based on more than one condition, you can use logical operators such as & (AND) or | (OR). For example:
- new_data <- subset(data, age >= 18 & gender == “Female”)
– new_data <- filter(data, age >= 18 & gender == “Female”)
Q3. Is there any other way to remove rows based on a condition?
A3. Yes, there are multiple ways to achieve this. You can use the ‘which()’ function combined with negative indexing, or the ‘slice()’ function from the dplyr package. However, using subset() or filter() is generally more straightforward and widely used.
Q4. What should I do if I want to remove rows based on a condition across multiple columns?
A4. In such cases, you can use the apply() function in combination with the rowSums() function. Here’s an example to remove rows based on the sum of two columns being greater than a certain value:
– new_data <- data[rowSums(data[, c("col1", "col2")]) > 10, ]
Removing rows from a dataframe based on a condition is a common task in data analysis. Utilizing the subset() function, filter() function from the dplyr package, or the na.omit() function helps in achieving this efficiently. Remember, it is essential to explore the available options and select the one that suits your specific requirements.
Keywords searched by users: how to remove empty row in r remove empty rows in r: dplyr, remove empty rows in r tidyverse, remove empty values in r, remove empty rows in excel, r remove empty columns, r remove rows with blank in one column, remove_empty in r, Delete null row in r
Categories: Top 92 How To Remove Empty Row In R
See more here: nhanvietluanvan.com
Remove Empty Rows In R: Dplyr
Before we dive into the code, let’s first understand what an empty row is. An empty row, also known as a missing value or NA (Not Available), refers to a row in a dataset where one or more variables have missing values. These missing values can occur due to various reasons, such as data entry errors, data corruption, or incomplete data collection.
To demonstrate how to remove empty rows in R using dplyr, we will create a simple dataset. Suppose we have a dataset named “data” with three variables: “Name,” “Age,” and “City.” Let’s create a dataframe with some empty rows:
“`{r}
# Load the dplyr package
library(dplyr)
# Create a dataframe with empty rows
data <- data.frame(
Name = c("John", "Alice", NA, "Tom", NA),
Age = c(25, 30, NA, 35, NA),
City = c("New York", "London", NA, "Sydney", NA)
)
```
Now, let's move on to removing empty rows using dplyr. The dplyr package provides the "filter" function, which allows us to select rows based on certain conditions. In our case, we want to keep only those rows that do not have any missing values. We can achieve this by using the "complete.cases" function, which returns a logical vector indicating whether a row has complete cases or not. Here's how we can use these functions together to remove empty rows:
```{r}
# Remove empty rows using dplyr
filtered_data <- filter(data, complete.cases(data))
```
In the code above, we pass the "data" dataframe to the "filter" function and use "complete.cases(data)" as the condition. This condition filters out the rows that have any missing values, effectively removing the empty rows.
Now, let's print the filtered dataset to see the result:
```{r}
# Print the filtered dataset
print(filtered_data)
```
Output:
```{r}
Name Age City
1 John 25 New York
2 Alice 30 London
3 Tom 35 Sydney
```
As you can see, the empty rows have been successfully removed, and we are left with only the rows that have complete data.
Frequently Asked Questions (FAQs):
Q1: Can I remove only specific columns that have missing values instead of removing entire rows?
Yes, you can remove specific columns instead of removing entire rows. To achieve this, you can use the "select" function from the dplyr package to select only the columns you want to keep, followed by the "complete.cases" function to filter out the rows with missing values. Here's an example:
```{r}
# Remove specific columns with missing values
filtered_data <- data %>%
select(-Age) %>%
filter(complete.cases(.))
“`
In the code above, we use the pipe operator (%>%) to chain the “select” and “filter” functions. We exclude the “Age” column using the “select” function with the negative sign (-), and then apply the “complete.cases” function to filter out the rows with missing values.
Q2: Can I remove empty rows based on a specific variable condition?
Yes, you can remove empty rows based on specific variable conditions. To achieve this, you can use the “mutate” function from the dplyr package to create a new variable that represents the condition you want to filter on, and then use the “filter” function to remove the rows that don’t meet that condition. Here’s an example:
“`{r}
# Remove empty rows based on a condition
filtered_data <- data %>%
mutate(Age_condition = !is.na(Age)) %>%
filter(Age_condition) %>%
select(-Age_condition)
“`
In the code above, we use the “mutate” function to create a new variable named “Age_condition” that represents whether the “Age” variable is not missing. We then use the “filter” function to exclude the rows where “Age_condition” is FALSE, effectively removing the empty rows. Finally, we use the “select” function to remove the “Age_condition” variable from the filtered dataset.
Removing empty rows in R using dplyr is a straightforward process that can greatly improve the quality and reliability of your data analysis. By leveraging the power of dplyr’s data manipulation functions like “filter,” you can efficiently remove empty rows and focus on analyzing clean and complete data.
In conclusion, this article has covered how to remove empty rows in R using dplyr, discussing the step-by-step process and providing code examples. We have also addressed some frequently asked questions related to removing empty rows in R. With this knowledge, you can confidently handle empty rows in your datasets and enhance the accuracy of your data analysis and modeling endeavors.
Remove Empty Rows In R Tidyverse
In this article, we will discuss different approaches to remove empty rows in R tidyverse and address some frequently asked questions about this topic.
Before diving into the methods, let’s briefly understand what the R tidyverse is. The tidyverse is a popular collection of R packages designed for data cleaning, wrangling, and visualization. It provides a concise and consistent set of functions that work seamlessly together, making it easier to perform data manipulations.
Now, let’s explore the methods to remove empty rows in R tidyverse:
Method 1: Using the filter() function:
————————————–
The filter() function from the dplyr package allows us to select rows based on certain conditions. To remove empty rows, we can utilize this function along with is.na() or complete.cases().
Here’s an example code snippet that demonstrates this method:
“`R
library(dplyr)
# Create a sample dataframe with empty rows
data <- data.frame(
name = c("John", "", "Emma", "Alex"),
age = c(25, NA, "", 30),
stringsAsFactors = FALSE
)
# Remove empty rows using filter() and complete.cases()
filtered_data <- data %>% filter(complete.cases(.))
“`
In this example, we use the complete.cases() function within the filter() function to remove rows with any missing or empty values. The resulting dataframe, filtered_data, will contain only non-empty rows.
Method 2: Using the drop_na() function:
—————————————
The drop_na() function, also from the dplyr package, is specifically designed to remove rows with missing values.
Here’s how we can implement this method:
“`R
library(dplyr)
# Create a sample dataframe with empty rows
data <- data.frame(
name = c("John", "", "Emma", "Alex"),
age = c(25, NA, "", 30),
stringsAsFactors = FALSE
)
# Remove empty rows using drop_na()
filtered_data <- drop_na(data)
```
In this example, drop_na() removes rows that contain any missing or empty values, resulting in a dataframe named filtered_data that only contains non-empty rows.
Method 3: Using the complete() function:
---------------------------------------
The complete() function, available in the tidyr package, is primarily used for creating combinations of variables. However, by setting the na.rm argument to TRUE, we can effectively remove empty rows.
Here's an example of how to use complete() to remove empty rows:
```R
library(tidyr)
# Create a sample dataframe with empty rows
data <- data.frame(
name = c("John", "", "Emma", "Alex"),
age = c(25, NA, "", 30),
stringsAsFactors = FALSE
)
# Remove empty rows using complete() and na.rm = TRUE
filtered_data <- complete(data, na.rm = TRUE)
```
In this example, complete() removes rows with any missing or empty values by specifying na.rm = TRUE, resulting in the dataframe filtered_data.
Now, let's address some frequently asked questions about removing empty rows in R tidyverse:
FAQs:
------
Q1: Can we directly remove empty rows from the original dataframe?
A: Yes, by assigning the filtered dataframe to the original dataframe, we can directly remove empty rows. For example:
```R
data <- data %>% filter(complete.cases(.))
“`
Q2: What if we want to remove rows only if they have empty values in specific columns?
A: We can modify the conditions in the filter() function to target only specific columns. For example, to remove rows with empty values in the ‘age’ column:
“`R
filtered_data <- data %>% filter(!is.na(age))
“`
Q3: Are these methods case-sensitive?
A: No, these methods are not case-sensitive. Whether the empty values are represented as “”, NA, “NA”, or any other case, the methods will identify them as empty and remove those rows.
Q4: Is it possible to remove rows with a threshold of missing or empty values?
A: Yes, we can set a threshold by using the na.rm argument with the complete() function. For example, to remove rows with more than two empty values:
“`R
filtered_data <- complete(data, na.rm = 2)
```
Q5: Are there any alternatives to the tidyverse for removing empty rows?
A: Yes, there are other packages and functions available in R, such as base R functions like complete.cases() and na.omit(), which can be used to remove empty rows. However, the tidyverse offers a more comprehensive and consistent set of functions for data cleaning and manipulation.
In conclusion, removing empty rows in R tidyverse is an essential step in data cleaning. We covered different methods using the filter(), drop_na(), and complete() functions. These methods provide flexibility in targeting specific columns or setting thresholds. Understanding these techniques will help ensure data quality and integrity for further analysis and visualization.
Remove Empty Values In R
Empty values or missing data can often create challenges when working with datasets in R. These empty values can jeopardize the accuracy and reliability of our analysis and modeling. Therefore, it is essential to identify and remove these empty values to ensure the quality of our results. In this article, we will explore different methods to remove empty values in R, providing you with a comprehensive guide.
Understanding Empty Values in R:
Empty values in R are commonly represented by NA (Not Available) or NaN (Not a Number). These values occur when there is a lack of data or when data cannot be calculated mathematically. Identifying the presence of empty values is crucial before proceeding with any analysis or modeling tasks.
Method 1: Removing Rows or Columns Containing Empty Values:
The simplest approach to removing empty values in R is to remove entire rows or columns from our dataset containing NA or NaN values. This method ensures that we only work with complete cases. However, this approach should be used cautiously, as it may result in significant data loss.
To remove rows containing empty values, we can utilize the `complete.cases()` function. This function provides a logical vector that identifies complete cases, allowing us to subset our data accordingly.
Example:
“`R
complete_dataset <- dataset[complete.cases(dataset), ]
```
Similarly, to remove columns containing empty values, we can use the `na.omit()` function. This function omits any columns containing empty values from our data frame.
Example:
```R
empty_columns_removed_dataset <- na.omit(dataset)
```
Method 2: Replacing Empty Values with Suitable Alternatives:
Instead of removing rows or columns, we can also choose to replace empty values with suitable alternatives to retain the maximum amount of data. This approach is especially useful when the missing values follow a pattern or can be estimated based on other variables.
One common technique is to replace missing values with the mean, median, or mode of the rest of the data in the respective column. The `na.aggregate()` function from the "zoo" package is particularly well-suited for this purpose.
Example:
```R
library(zoo)
dataset_filled <- na.aggregate(dataset, FUN = mean) # Replace with mean
```
Alternatively, we can use sophisticated imputation methods, such as multiple imputation or regression imputation, to estimate missing values based on other variables' observed values. The "mice" package in R provides comprehensive functionalities for imputation.
Method 3: Removing or Replacing Values Based on Conditions:
Sometimes, we may need to remove or replace empty values based on certain conditions or rules. R facilitates this process by allowing us to define conditional statements and apply them selectively to the dataset.
To remove rows or columns based on specific conditions, we can use the `subset()` function in combination with logical operators.
Example:
```R
subset_dataset <- subset(dataset, variable > 0) # Remove rows where variable is greater than 0
“`
To replace empty values based on conditions, we can use the `ifelse()` function. This function allows us to replace values if a given condition is satisfied, and otherwise keeps the original value.
Example:
“`R
dataset_replaced <- ifelse(dataset == NA, "Not Available", dataset) # Replace NA with "Not Available"
```
FAQs:
Q1. Can we remove empty values for only specific variables in a dataset?
Yes, we can selectively remove empty values for specific variables by subsetting the dataset based on the desired conditions using logical operators.
Q2. How can we determine the number of empty values in a dataset?
The `is.na()` function in R identifies whether a value is NA or NaN. By applying this function to each element in the dataset, we can calculate the sum of empty values.
Q3. What should we consider before removing or replacing missing values?
Before removing or replacing missing values, it is crucial to understand the nature and patterns of missingness in the dataset. This analysis can highlight potential biases and guide us in selecting appropriate removal or imputation methods.
Q4. Can we incorporate machine learning algorithms to remove empty values?
Yes, machine learning algorithms can be utilized to handle missing values. Techniques like Expectation-Maximization and decision tree models can help impute missing values more accurately and efficiently.
Q5. Are there any risks associated with removing or replacing missing values?
Removing or replacing missing values might introduce bias in our dataset if the missing values are not random. Care should be taken to ensure that our data remains representative and unbiased after handling missing values.
Conclusion:
Handling empty values is an essential aspect of data manipulation and analysis in R. In this article, we presented several methods to remove empty values from a dataset, ranging from removing rows or columns containing empty values to replacing empty values with suitable alternatives. Each method has its advantages and limitations, and the choice depends on the nature of the dataset and the specific analysis goals. Prioritizing data quality and transparency, while selecting the appropriate method, ensures reliable and accurate results.
Images related to the topic how to remove empty row in r
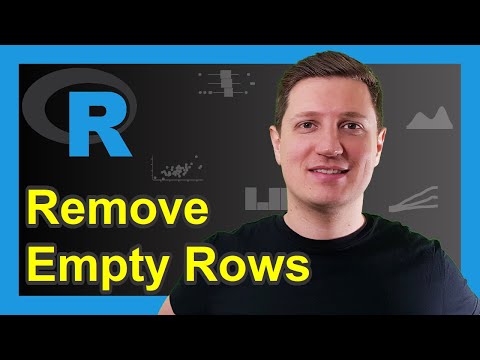
Found 20 images related to how to remove empty row in r theme
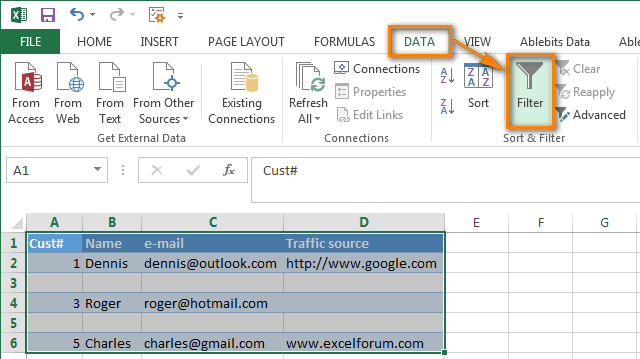
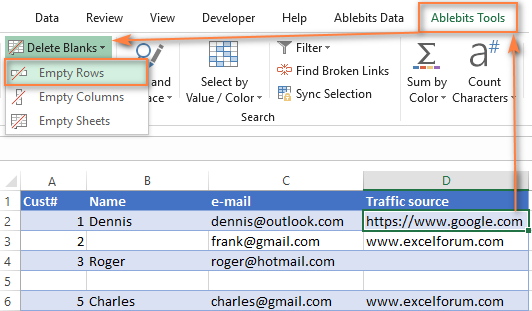
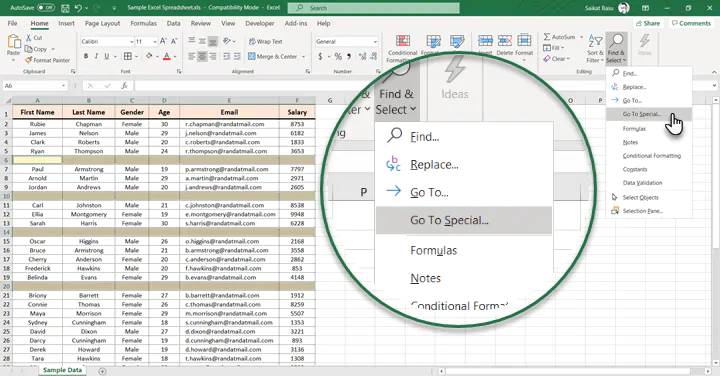
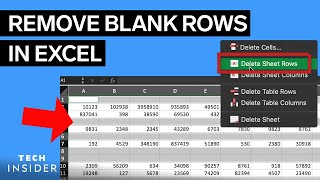

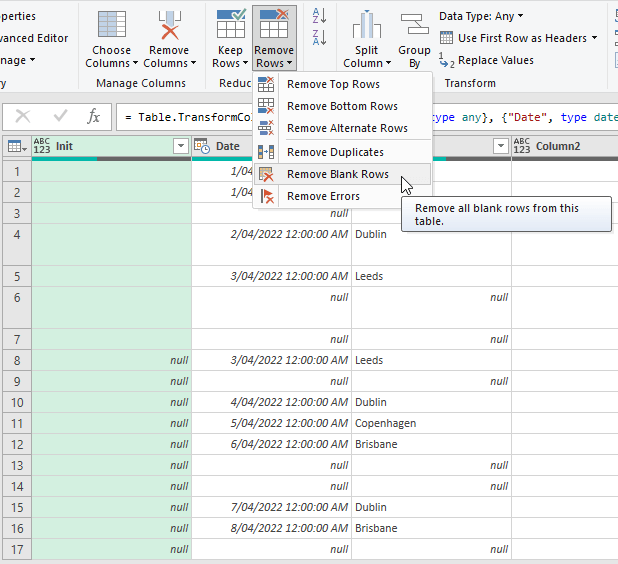
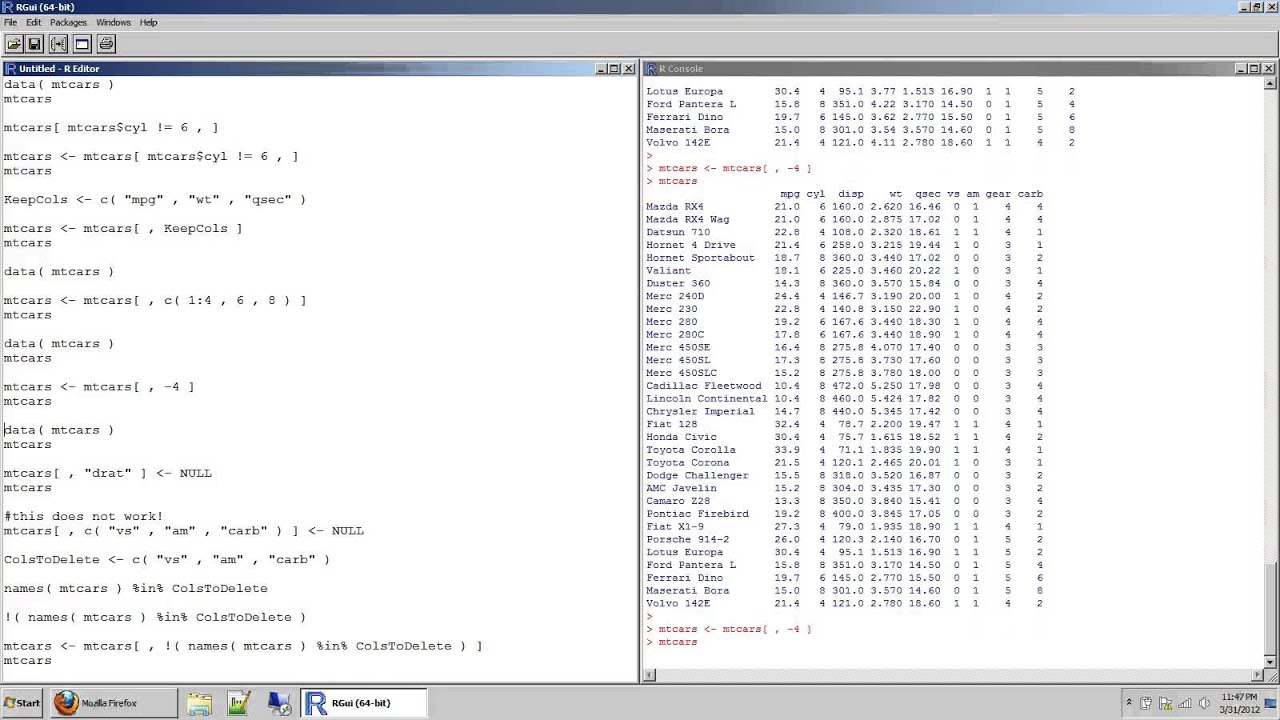

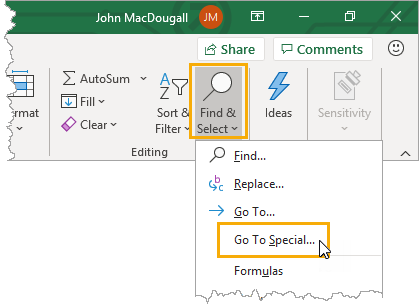
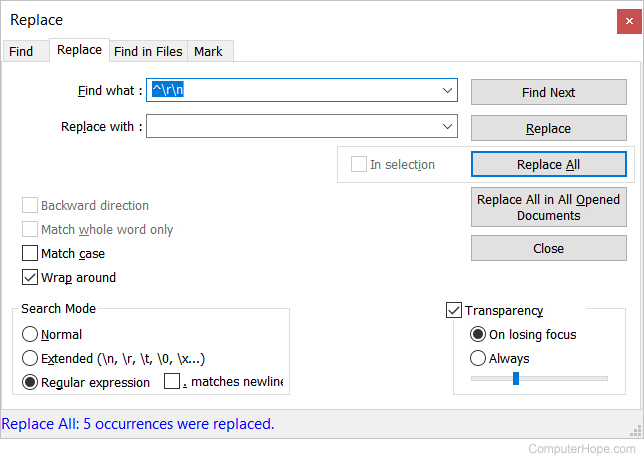
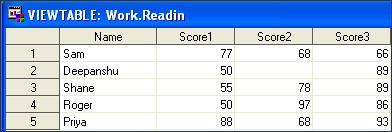


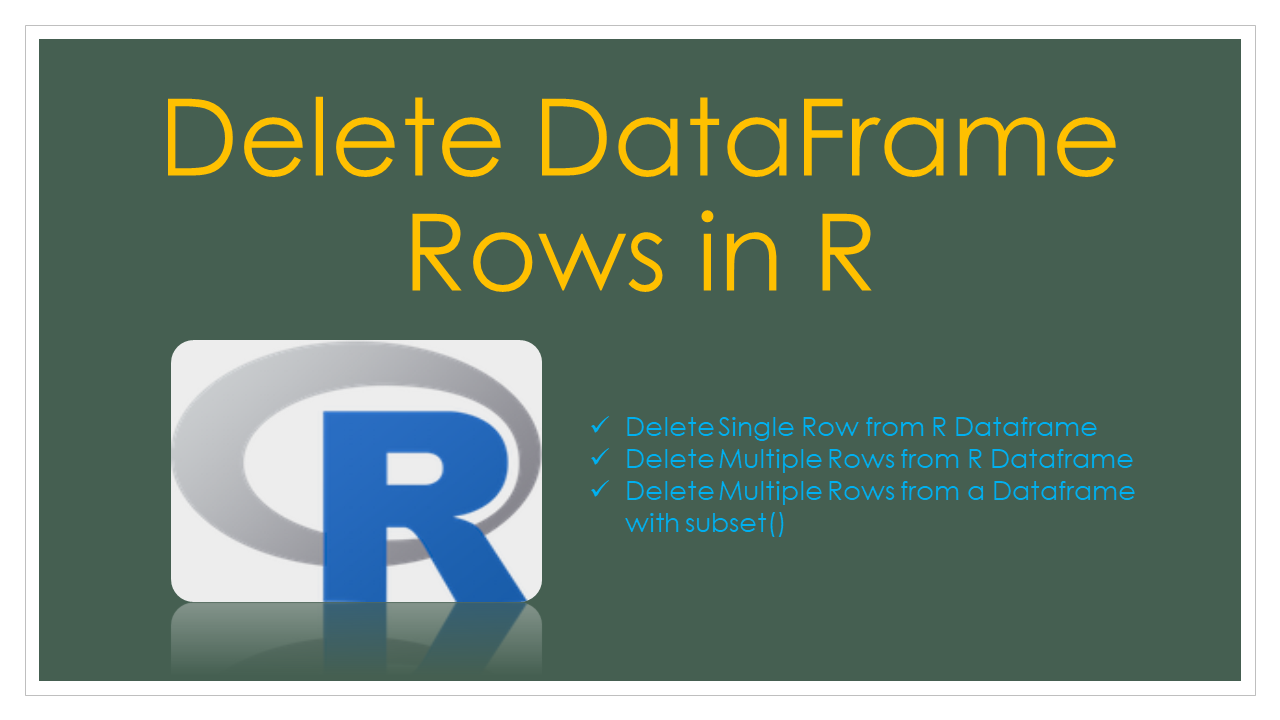
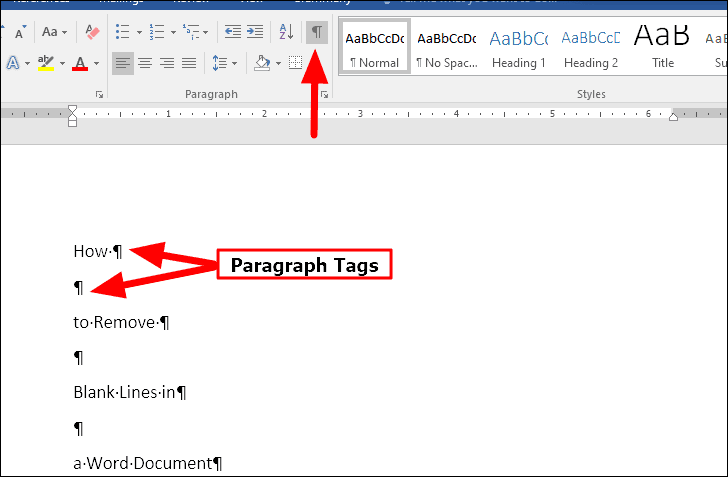
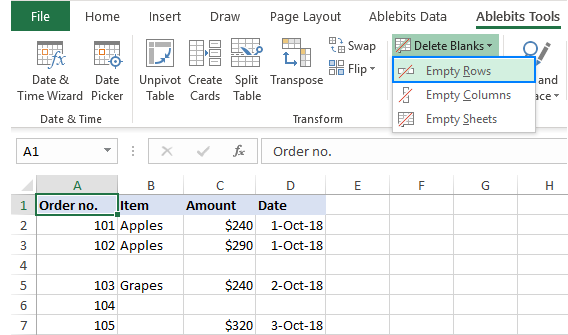


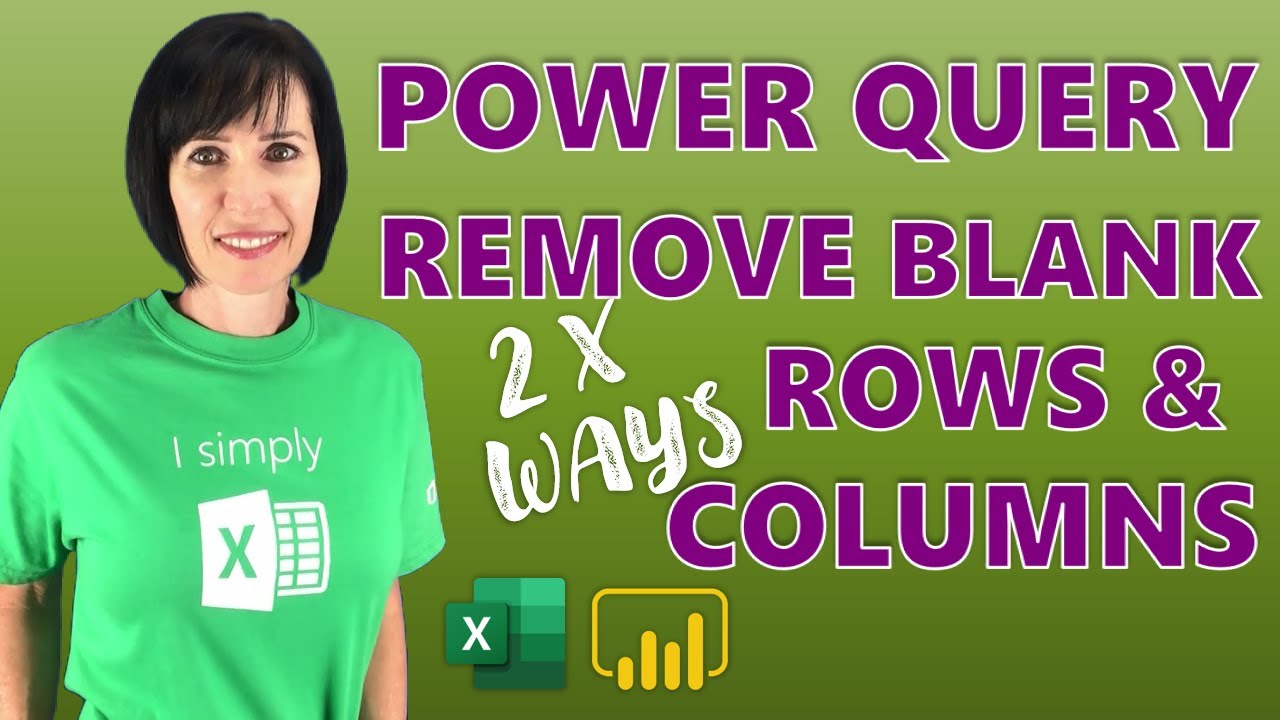
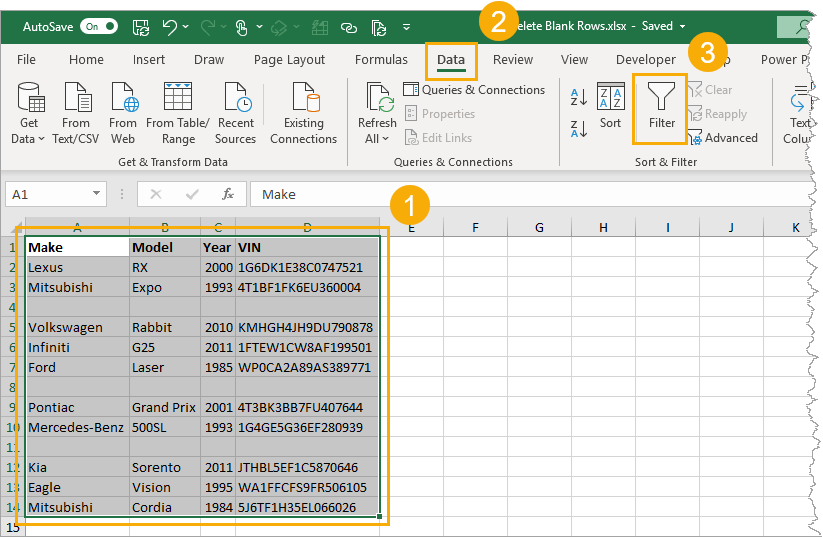
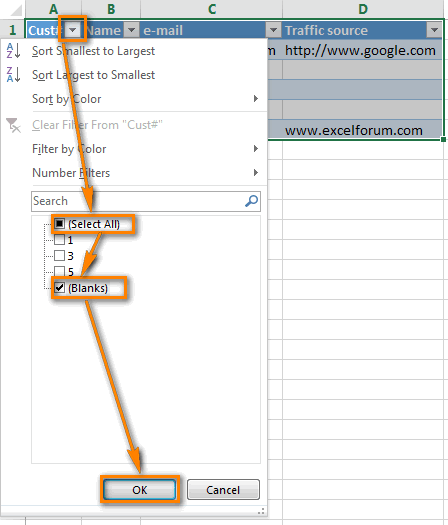
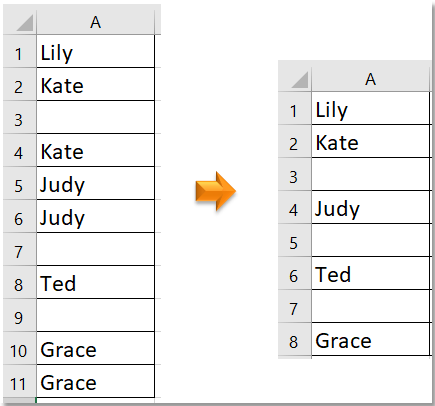

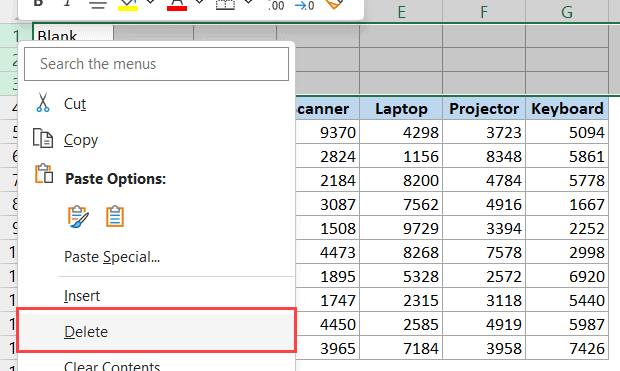




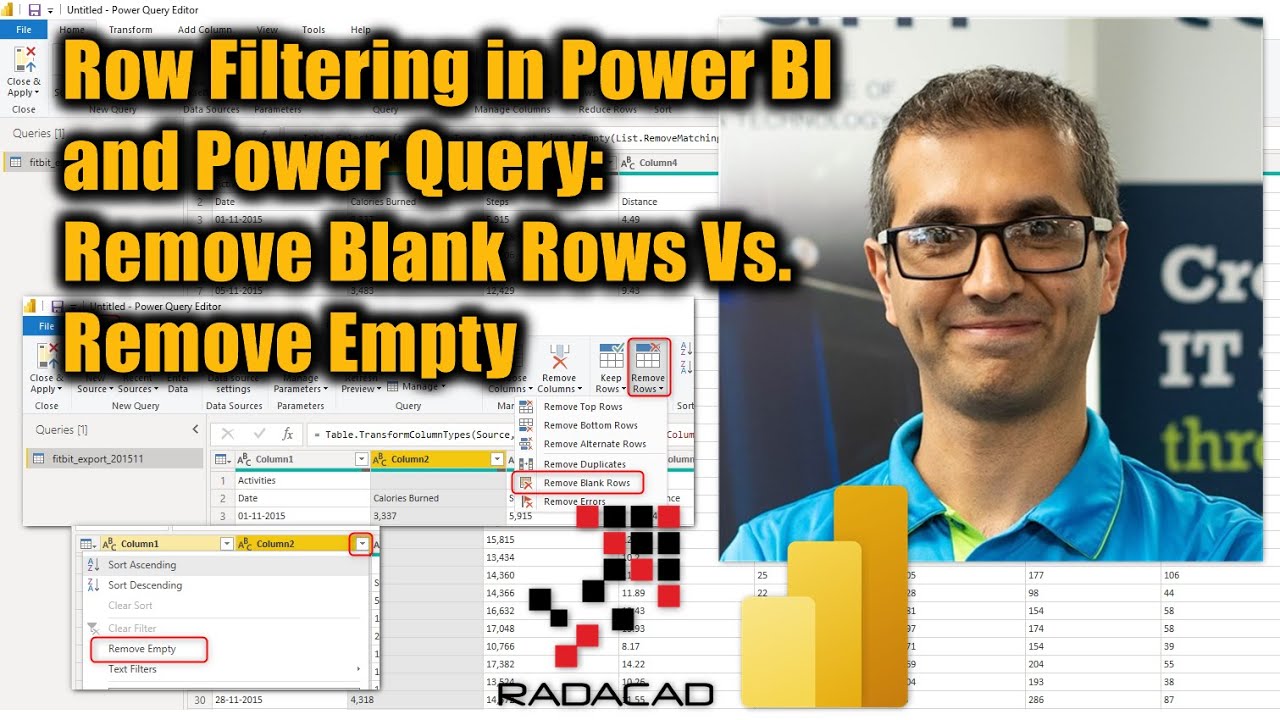
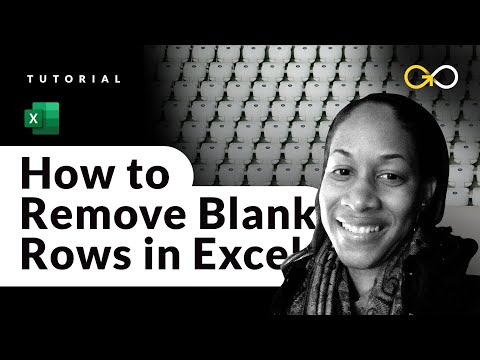
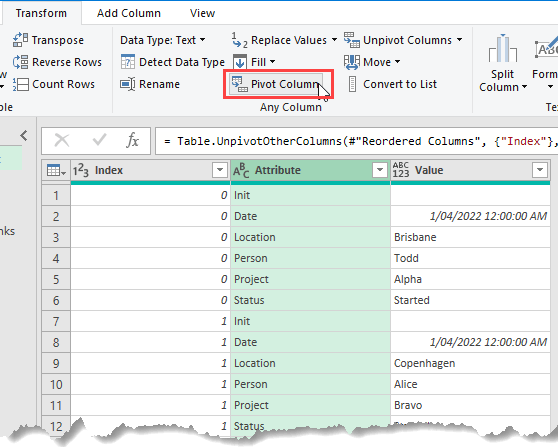
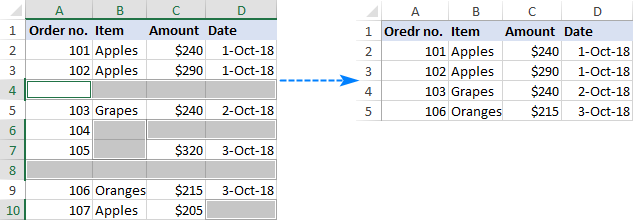

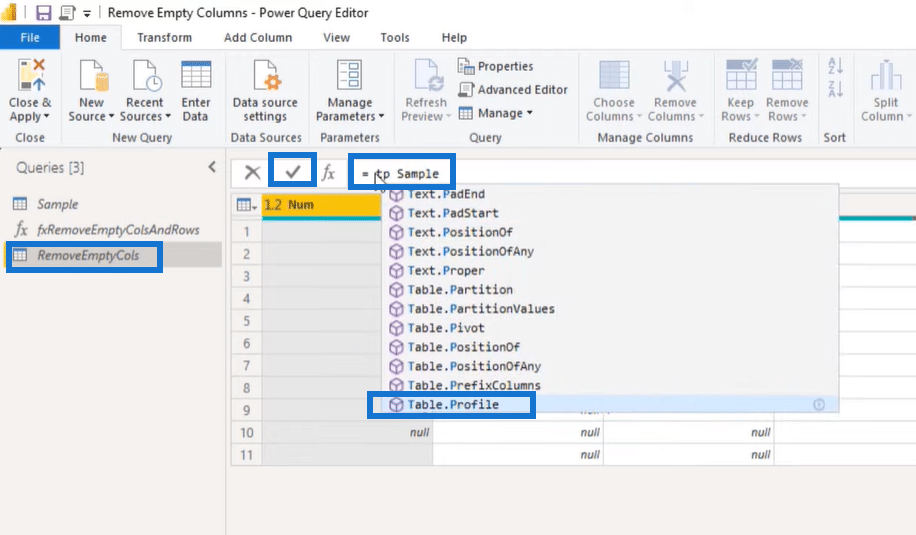
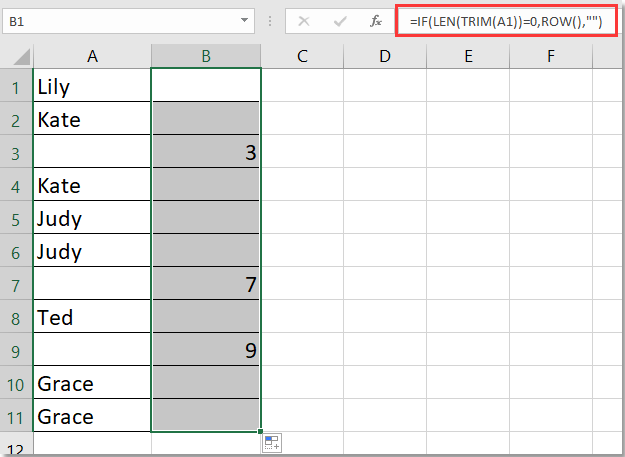

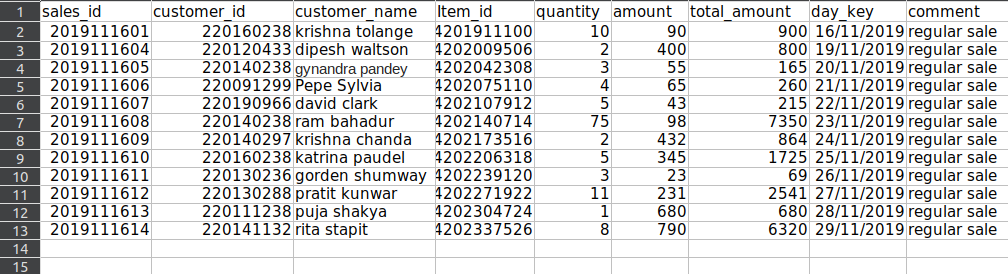
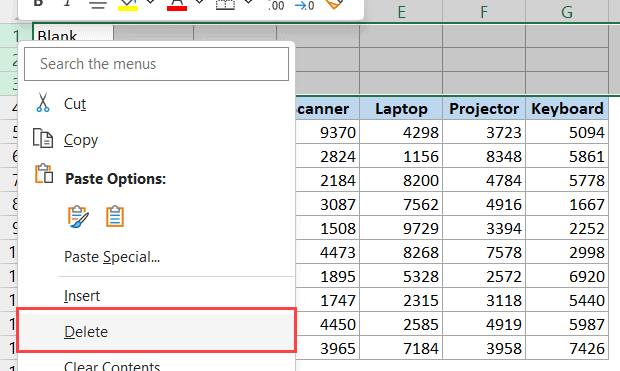


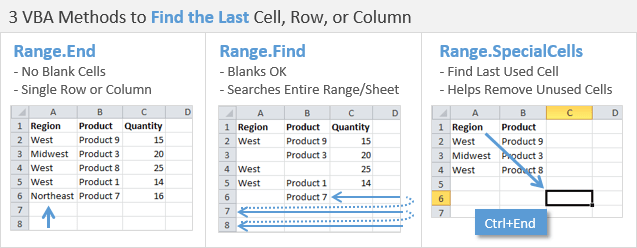


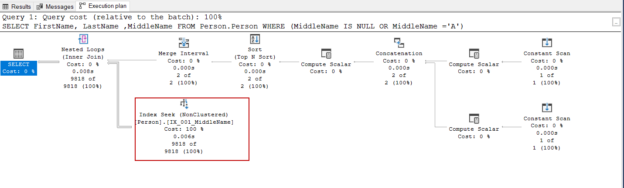
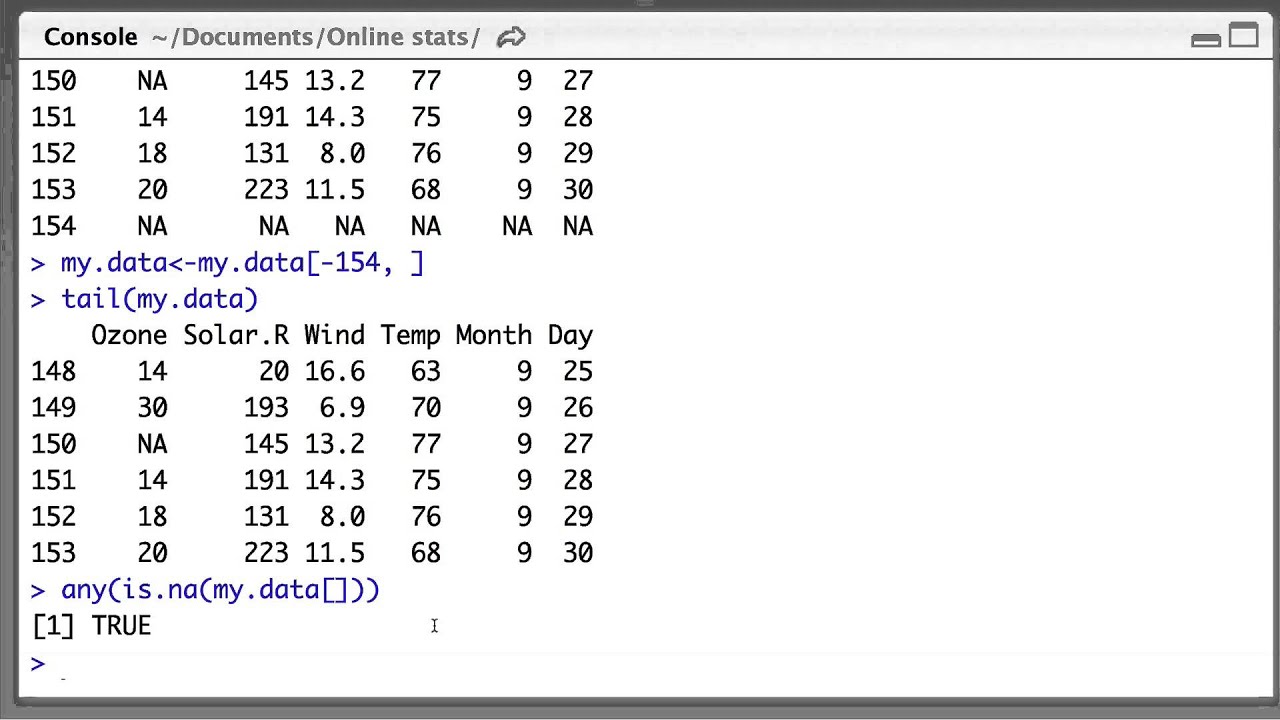
Article link: how to remove empty row in r.
Learn more about the topic how to remove empty row in r.
- Removing empty rows of a data file in R – Stack Overflow
- Remove Empty Rows of Data Frame in R (2 Examples)
- How to Remove Empty Rows from Data Frame in R – Statology
- How to remove empty rows from R dataframe? – GeeksforGeeks
- How to remove all rows having NA in R – Tutorialspoint
- How to Conditionally Remove Rows in R DataFrame? – GeeksforGeeks
- Remove empty rows and/or columns from a data.frame or matrix.
See more: nhanvietluanvan.com/luat-hoc