How To Make Python Code Go Back To A Line
Python is a versatile and powerful programming language that allows you to build various applications and solve complex problems. One common requirement when writing Python code is the ability to go back to a specific line or restart the code execution. In this article, we will explore different techniques to achieve this goal, including using loops, conditional statements, and recursion.
Initializing Variables
The first step in making Python code go back to a line is initializing the necessary variables. Variables will be used to control the program flow, store information, and determine when to go back to a specific line. For example, a variable called “restart” can be set to True initially, and its value can change based on certain conditions.
Understanding the Need for Looping
Looping is a fundamental concept in programming that allows executing a block of code multiple times. By utilizing loops, we can repeat specific sections of the code, which helps in going back to a line or restarting the code. There are two types of loops in Python: the ‘for’ loop and the ‘while’ loop.
Using ‘for’ Loop
The ‘for’ loop iterates over a sequence, such as a list, string, or range, and performs the code block for each item in the sequence. To make the code go back to a specific line, we can use a ‘for’ loop to repeat the execution until certain conditions are met. For example:
“`python
restart = True
for item in range(5):
# Code to be executed
if restart:
continue # Go back to the line ‘for item in range(5):’
# More code
“`
Using ‘while’ Loop
The ‘while’ loop repeats a block of code as long as a certain condition is true. To make the code go back to a specific line, we can use a ‘while’ loop together with conditional statements or break statements. For example:
“`python
restart = True
while restart:
# Code to be executed
if not restart:
break # Exit the loop and continue with the rest of the code
# More code
“`
Implementing Nested Loops
Nested loops allow us to create loops within loops. By using nested loops along with proper control flow, we can go back to a specific line or restart the code execution. The inner loop will execute multiple times before proceeding to the next iteration of the outer loop. For example:
“`python
restart = True
for i in range(3):
for j in range(2):
# Code to be executed
if restart:
break # Go back to the line ‘for j in range(2):’
# More code
if restart:
continue # Go back to the line ‘for i in range(3):’
# More code
“`
Adding Conditions with ‘if’ Statements
Conditional statements, such as ‘if’, ‘elif’, and ‘else’, allow us to execute specific code blocks based on certain conditions. By combining conditional statements with loops, we can control when to go back to a line or restart the code. For example:
“`python
restart = True
for item in range(5):
# Code to be executed
if restart:
continue # Go back to the line ‘for item in range(5):’
elif condition:
# Code to go back to a different line
else:
# Code to go back to another line
# More code
“`
Using ‘break’ Statement to Exit Loop
The ‘break’ statement is used to exit the current loop and resume execution at the next statement after the loop. By incorporating ‘break’ statements within loops, we can control the flow and make the code go back to a line. For example:
“`python
restart = True
for item in range(5):
# Code to be executed
if restart:
break # Go back to the line ‘for item in range(5):’
# More code
“`
Using ‘continue’ Statement to Skip Current Iteration
The ‘continue’ statement is used to skip the rest of the code within the current iteration and move to the next iteration. By using ‘continue’ statements within loops, we can go back to a specific line and restart the code execution. For example:
“`python
restart = True
for item in range(5):
# Code to be executed
if restart:
continue # Go back to the line ‘for item in range(5):’
# More code
“`
Utilizing ‘pass’ Statement
The ‘pass’ statement does nothing and serves as a placeholder when a statement is required syntactically but no action is needed. It can be used when going back to a line that needs to be skipped temporarily. For example:
“`python
restart = True
for item in range(5):
# Code to be executed
if restart:
pass # Go back to the line ‘for item in range(5):’
# More code
“`
Understanding the Concept of Recursion
Recursion is a programming technique where a function calls itself repeatedly until a certain condition is met. By utilizing recursion, we can achieve the desired behavior of going back to a specific line or restarting the code. However, careful consideration and proper termination conditions are essential to avoid infinite recursion.
In conclusion, there are several techniques to make Python code go back to a specific line or restart the code execution. These include using loops, conditional statements, and recursion. By understanding these concepts and implementing them in your code, you can control the program flow and achieve the desired outcome.
FAQs
Q: How to make code run again in Python?
A: To make code run again in Python, you can use loops or recursion to repeat the execution of the code block.
Q: How to go back to the start of a loop in Python?
A: In Python, you can use ‘continue’ statements or control flow constructs within loops to go back to the start of the loop.
Q: How to go back to a specific line in Python?
A: By utilizing loops and conditional statements, you can control the flow of your code and make it go back to a specific line when necessary.
Q: How to make an ‘else’ statement go back to ‘if’ in Python?
A: In Python, ‘else’ statements are executed when the preceding ‘if’ or ‘elif’ conditions are not true. To make it go back to ‘if’, you need to modify the code flow by applying loops or conditional statements.
Q: How to return to a specific line in Python code?
A: In Python, you can achieve this by utilizing appropriate loops, conditional statements, or by using the ‘pass’ statement as a placeholder.
Q: How to restart a Python script?
A: To restart a Python script automatically, you can incorporate loops or recursion to recreate the initial conditions and execute the script again.
Q: How to repeat a program in Python?
A: By using loops or recursion, you can repeat the execution of a program in Python multiple times.
By applying the techniques mentioned above, you can make your Python code go back to a specific line or restart the code execution, enabling you to build more dynamic and interactive programs.
Python Tutorial – Looping Your Code Back (To The Beginning Or Middle) Using A Procedure
Keywords searched by users: how to make python code go back to a line How to make code run again in Python, Python go back to start of loop, Go back to a line python, Python else go back to if, Return python code, Restart python script, Restart python script automatically, Repeat program Python
Categories: Top 39 How To Make Python Code Go Back To A Line
See more here: nhanvietluanvan.com
How To Make Code Run Again In Python
Python is a powerful and versatile programming language, widely used in various industries and applications. As a Python programmer, you may encounter situations where you need to rerun your code to troubleshoot errors, modify functionality, or improve performance. In this article, we will explore the various methods and techniques to make your code run again in Python.
1. Understand the Error Messages:
When your code fails to run, Python will often provide error messages that can help you identify the issue. It is crucial to carefully read and understand these error messages, as they often pinpoint the exact line of code causing the problem. Common error types include syntax errors, logic errors, and runtime errors. By analyzing the error messages, you can make the necessary corrections and rerun the code.
2. Debugging with Print Statements:
One of the simplest ways to debug and rerun your code is by strategically placing print statements throughout your code. These print statements can display intermediate values, variable states, or the execution flow. By observing the printed output, you can identify the stages where the code goes wrong. Print statements are especially useful in tracking down logical errors or unexpected variable values. Once you’ve identified the problematic section, you can modify and rerun the code.
3. Utilize Debuggers:
Python provides several debugging tools that can greatly aid in troubleshooting your code. The built-in debugger, pdb, is a powerful tool for step-by-step code execution, variable inspection, and setting breakpoints. By executing your code in debug mode, you can meticulously examine each function call, line of code, and variable value, making it easier to identify and resolve errors. Various integrated development environments (IDEs), such as PyCharm, also offer advanced debugging features that allow you to navigate and inspect your code during runtime.
4. Check for Indentation Errors:
Python relies heavily on proper indentation to define code blocks and structures, such as loops or conditionals. It is crucial to ensure that your code uses consistent and correct indentation. Otherwise, Python will raise an IndentationError. This error can often be solved by carefully checking the alignment of your code and adjusting the indentation accordingly. By fixing the indentation errors, you can rerun the code and proceed with its execution.
5. Compile-Time Errors:
Compile-time errors occur when Python is translating your code into machine-readable instructions. They typically indicate mistakes in syntax or unresolved dependencies. To address these errors, it is essential to carefully review the code and analyze any missing or incorrect syntax. Common issues include missing parentheses, quotation marks, or improper variable assignments. Once you’ve rectified the compile-time errors, you can rerun the code to confirm the desired execution.
6. Runtime Errors:
While compile-time errors occur before the code is executed, runtime errors happen during program execution. Common examples of runtime errors include division by zero, illegal memory access, and attempts to manipulate objects in invalid ways. When encountering a runtime error, Python will raise an exception and provide a traceback. The traceback reveals the sequence of function calls that led to the error, helping you identify the problematic part of your code. By fixing the root cause of the exception, you can rerun your code and aim for successful execution.
Frequently Asked Questions (FAQs):
Q: What if my code runs but produces incorrect results?
A: If your code runs without any errors but produces unexpected or incorrect results, there might be logical errors in your program. Consider stepping through your code using debugging tools, print statements, or inspecting variables to identify any inconsistencies.
Q: How can I rerun a specific section of my code without re-executing the entire script?
A: In most integrated development environments or text editors, you can highlight the specific section of code you want to rerun and execute it separately. This feature allows you to test and modify particular parts of your code without repeating unnecessary computations.
Q: What should I do if my code runs indefinitely, causing the program to hang?
A: Infinite loops can occur due to logical errors, incorrect loop conditions, or a lack of termination conditions. To avoid indefinite execution, make sure to define appropriate loop exit conditions or add conditional statements to break out of the loop when necessary.
Q: Are there tools to automatically identify and fix code issues?
A: Yes, various Python code linters are available that can analyze your code for potential errors, stylistic inconsistencies, and security vulnerabilities. Popular examples include pylint and flake8. Integrating these tools into your development workflow can help you catch and fix code-related issues before executing the program.
Conclusion:
Making code run again in Python demands a thorough understanding of error messages, using debuggers effectively, handling indentation errors, addressing compile-time and runtime errors, and finding logical inconsistencies. By following the strategies outlined in this comprehensive guide, you will be well-equipped to troubleshoot and rerun your Python code successfully. Remember, debugging and refining code is a fundamental aspect of software development, so embrace the process and continue learning from each iteration.
Python Go Back To Start Of Loop
The “go back to start of loop” functionality in Python is achieved using the “continue” statement. When encountered within a loop, the “continue” statement facilitates the loop to immediately jump back to the beginning, ignoring any remaining code within the loop’s body. This enables the loop to restart and go through the iterations from the start as if the conditions for continuing were met.
To better understand this concept, let’s consider an example where we want to print only the even numbers between 1 and 10. We can achieve this using a “for” loop in Python:
“`python
for num in range(1, 11):
if num % 2 != 0:
continue
print(num)
“`
Output:
“`
2
4
6
8
10
“`
In this code snippet, the range function creates a sequence of numbers from 1 to 10 (excluding 11), which is iterated using the “for” loop. Inside the loop, we use the modulo operator (%) to check if the number is odd. If it is odd, the “continue” statement is executed, causing the loop to go back to the start without printing the odd number. If the number is even, it is printed.
The “go back to start of loop” mechanism is not limited to “for” loops but can also be used in “while” loops. Consider the following example that prints all the numbers between 1 and 10 while skipping numbers divisible by 3:
“`python
num = 0
while num < 10: num += 1 if num % 3 == 0: continue print(num) ``` Output: ``` 1 2 4 5 7 8 10 ``` In this code snippet, we initialize the variable "num" to 0 and increment it by 1 in each iteration of the "while" loop. If the number is divisible by 3, the "continue" statement is executed, causing the loop to go back to the start without printing that number. Now, let's address some frequently asked questions related to the "go back to start of loop" mechanism in Python: Q: Can the "continue" statement be used in nested loops? A: Yes, the "continue" statement can be used in nested loops. When encountered, it only affects the innermost loop, allowing the program to skip the remainder of the current iteration and move on to the next one within that particular loop. Q: Are there any alternative ways to achieve the same functionality? A: Yes, besides using "continue," you can also utilize a combination of "if" statements and "break" statements to achieve similar results. However, the "continue" statement provides a more straightforward and concise approach for going back to the start of a loop in Python. Q: Can the "continue" statement be used outside of loops? A: No, the "continue" statement must be used within a loop. If encountered outside of a loop, it will result in a SyntaxError. Q: Is the "go back to start of loop" mechanism exclusive to Python? A: No, the concept of going back to the start of a loop exists in various programming languages, each with its own syntax and implementation. However, Python offers the "continue" statement as a simple and efficient way to accomplish this functionality. In conclusion, the "go back to start of loop" functionality in Python allows developers to enhance the control flow of their code. By using the "continue" statement within loops, you can effortlessly skip certain iterations and move back to the start of the loop. This mechanism can be applied to both "for" and "while" loops, and it offers a convenient way to handle specific conditions within loop iterations. Understanding and utilizing this feature effectively can greatly improve the performance and readability of your Python code.
Go Back To A Line Python
Python is a versatile and popular programming language that is widely used in various fields, including web development, data analysis, artificial intelligence, and more. One of the key features in Python is the ability to manipulate files, read data from them, and write data back into them. In this article, we will explore the concept of “go back to a line” in Python, which refers to the process of moving the file cursor to a specific line in a text file.
Understanding the file cursor
Before diving into the details of how to go back to a specific line in a text file, it is important to understand the concept of the file cursor. When you open a file for reading or writing in Python, a file object is created. This file object has a built-in cursor that keeps track of the current position in the file. Initially, the cursor is set to the beginning of the file.
To read data from a file, you can use the `readline()` or `readlines()` methods. These methods move the cursor forward in the file as data is being read. However, once the cursor has moved past a specific line, there is no direct way to move the cursor back to that line again. This is where the concept of “go back to a line” becomes useful.
Techniques to go back to a line
There are multiple techniques you can utilize to go back to a line in Python.
1. Using `seek()`: The `seek()` method in Python allows you to move the file cursor to a specific position. To go back to a line, you need to calculate the byte offset of that line and then use the `seek()` method to move the cursor to that offset. However, this technique only works with files that have a fixed line length, such as files with fixed-width columns.
2. Using `linecache`: The `linecache` module in Python provides a high-level interface to get lines from a file in memory. You can use this module to read the desired line or lines into memory and then perform operations on them. This technique is useful when dealing with large files or files with variable line lengths.
3. Using a loop: If you need to access multiple lines in a file, you can use a loop to iterate through the lines and stop at the desired line. This technique is straightforward and works well with files of any size.
Considerations and best practices
While going back to a specific line in a file can be useful in certain situations, it is important to consider some best practices to ensure efficient usage of system resources and avoid potential issues.
1. File size: If you need to frequently go back to lines in large files, it can be inefficient to read and process the entire file each time. Consider splitting the file into smaller, more manageable chunks or using techniques like binary file reading for faster access.
2. Line lengths: If the lines in your file have variable lengths, using the `seek()` method may not be practical. In such cases, techniques like `linecache` or iteration through the file using a loop may be more effective.
3. Memory consumption: When using techniques like `linecache` or reading a file with a loop, keep in mind that the lines are stored in memory. If you are working with very large files, memory consumption could become an issue. Consider using generators or processing the file line by line to reduce memory usage.
FAQs:
Q: Is it always necessary to go back to a line in a file?
A: No, it depends on the specific requirements of your program. If you only need to read the file sequentially, going back to a line may not be necessary.
Q: Can I modify data at a specific line in a file?
A: Yes, after moving the cursor back to a line, you can modify the data or overwrite it with new information.
Q: Are there other file operations I should be aware of?
A: Yes, Python provides various file operations like append, delete, and update that can be useful in manipulating file content.
Q: Can I go back to a line in a binary file?
A: Yes, you can use the same techniques mentioned above to go back to a specific line in a binary file.
Q: How can I determine the line number in a text file?
A: Python offers several approaches to calculate the line number, such as using a loop counter or regular expressions to match a specific pattern.
In conclusion, the ability to go back to a specific line in a text file can be immensely useful in certain scenarios, allowing you to efficiently perform operations on specific data points. By understanding the file cursor and utilizing appropriate techniques, you can harness the full power of Python’s file manipulation capabilities.
Images related to the topic how to make python code go back to a line
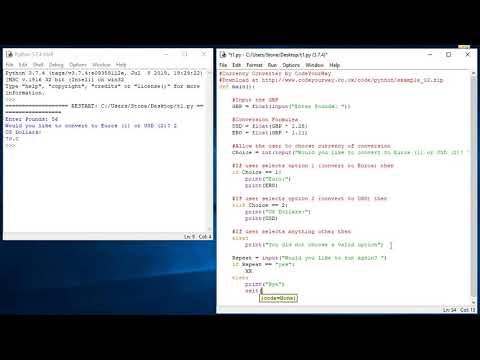
Found 35 images related to how to make python code go back to a line theme
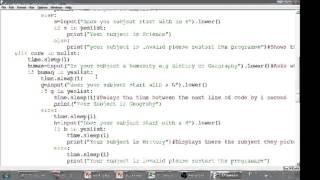

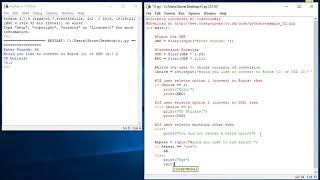
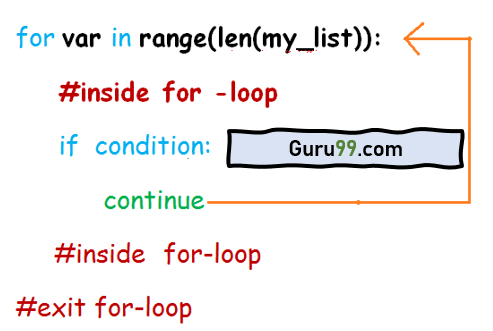

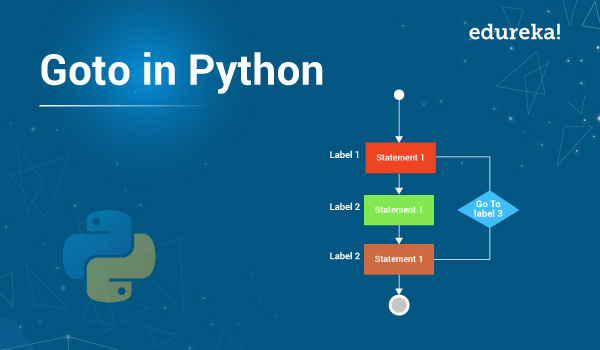
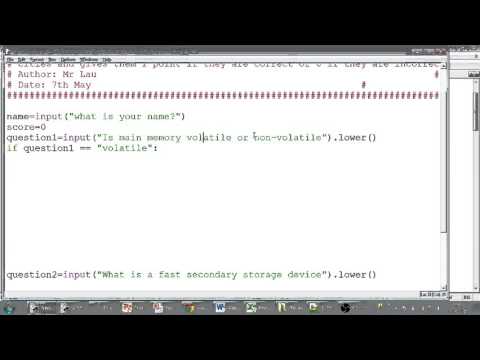
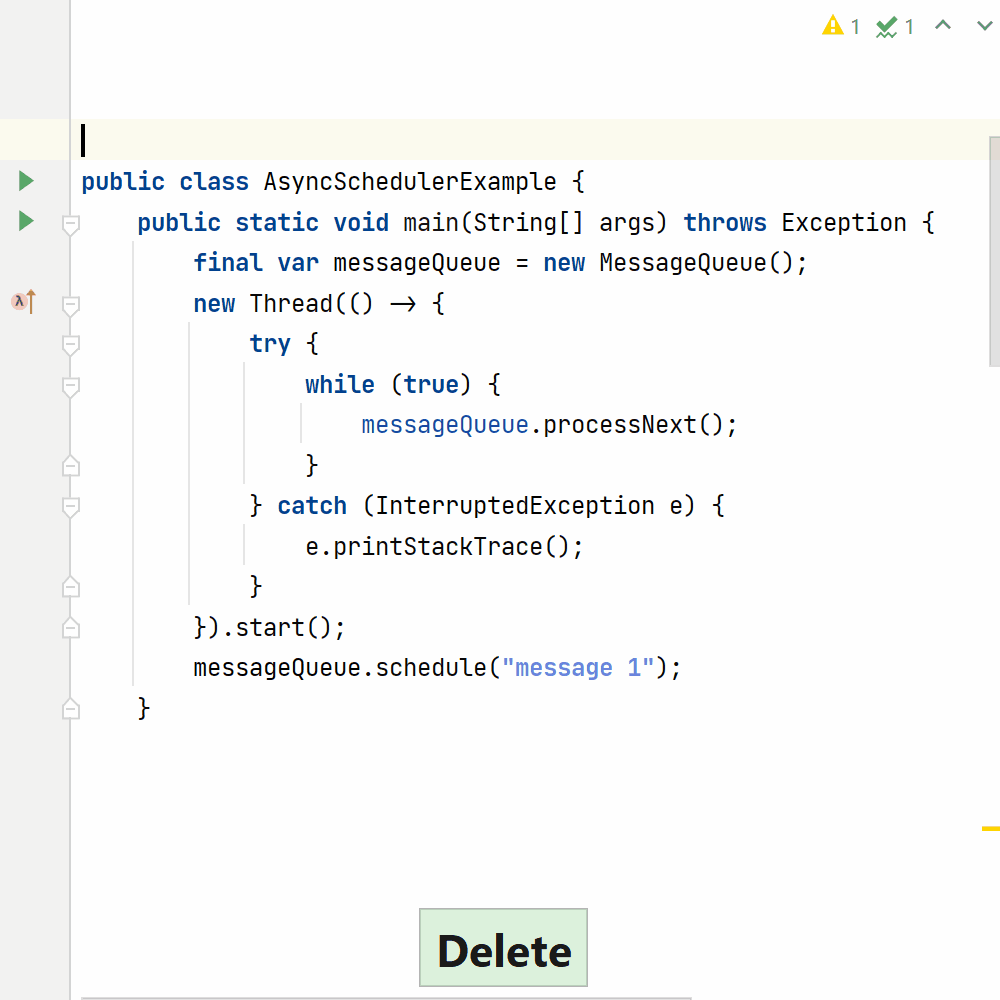


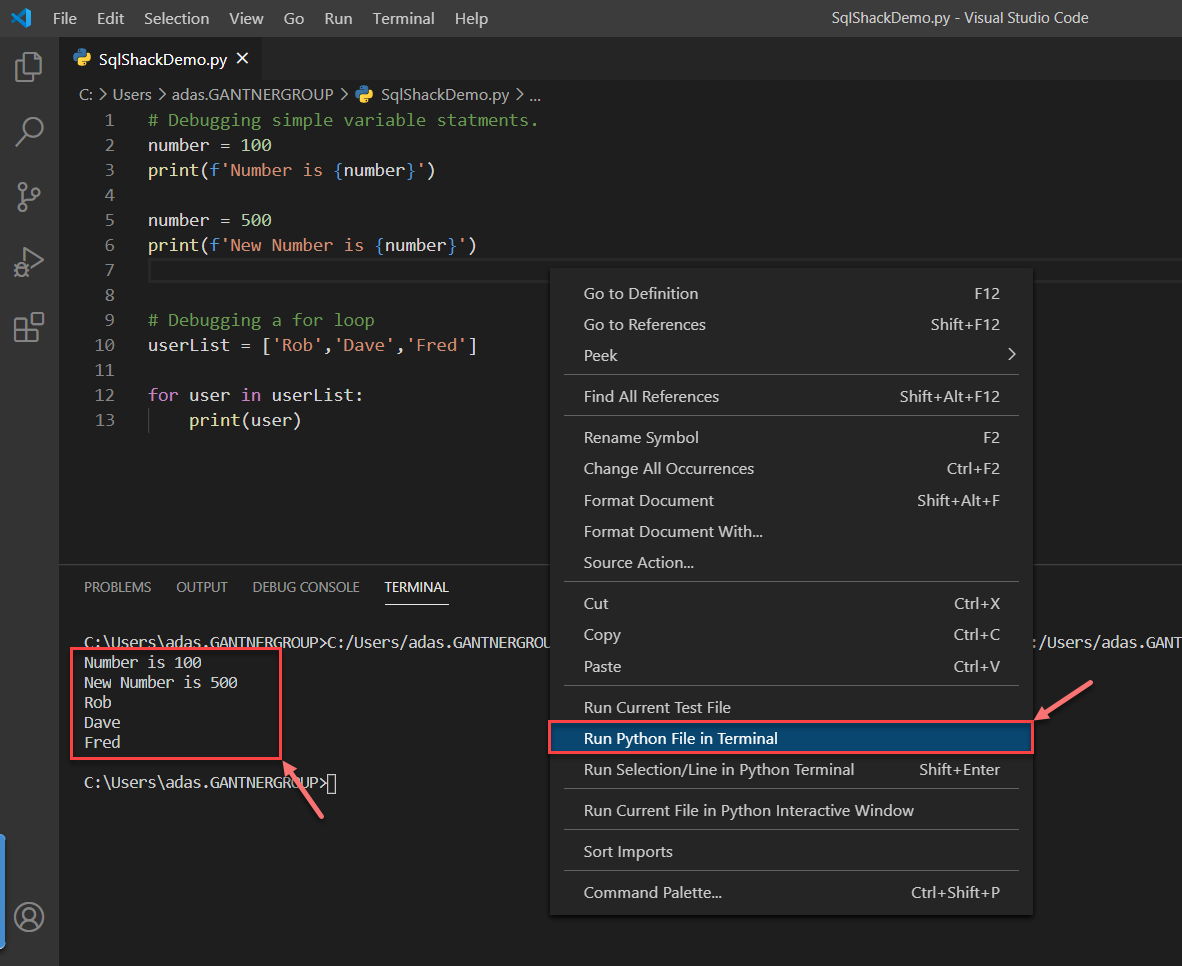
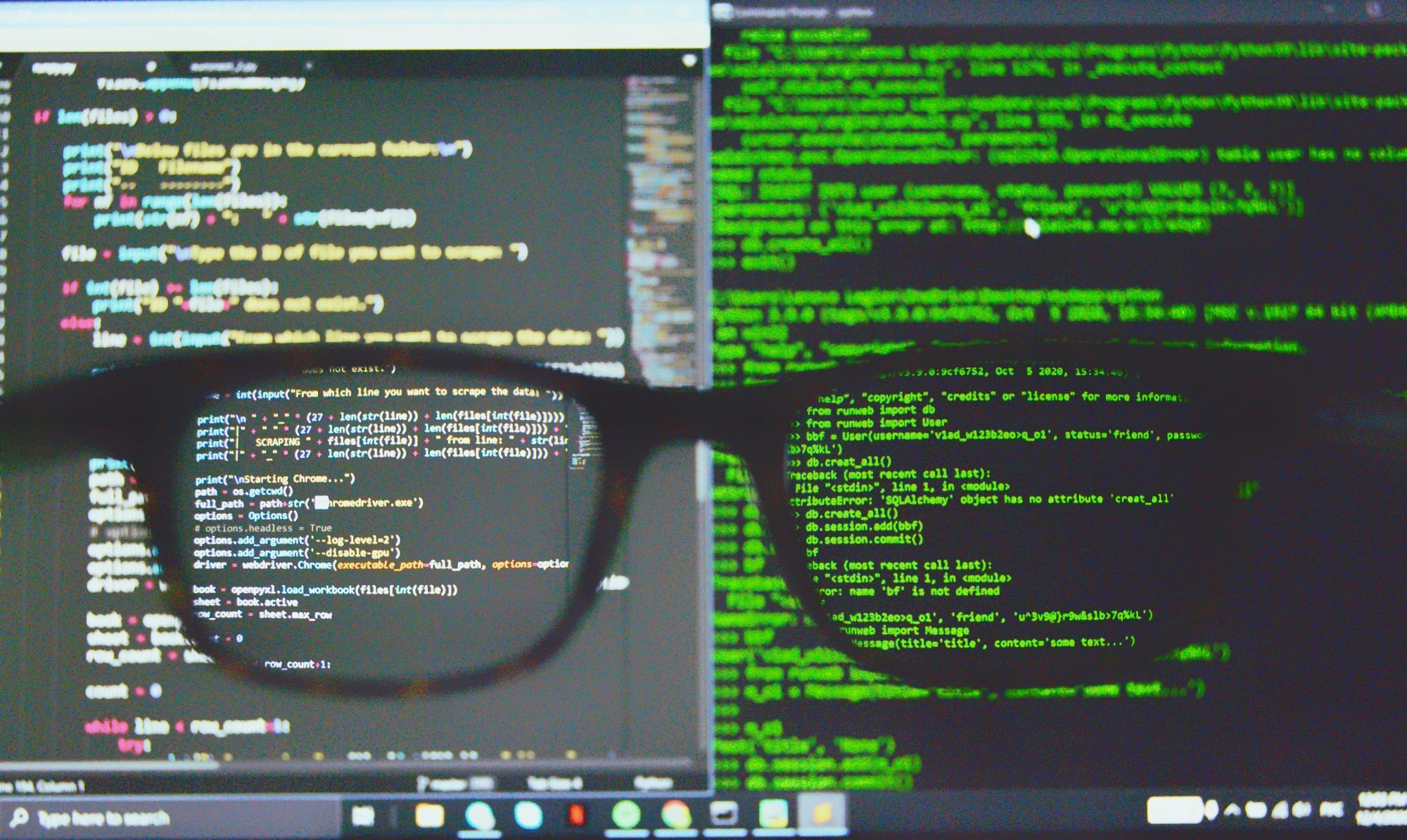
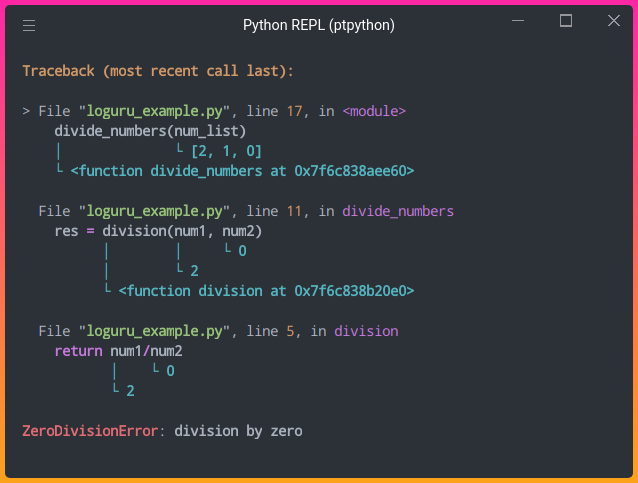
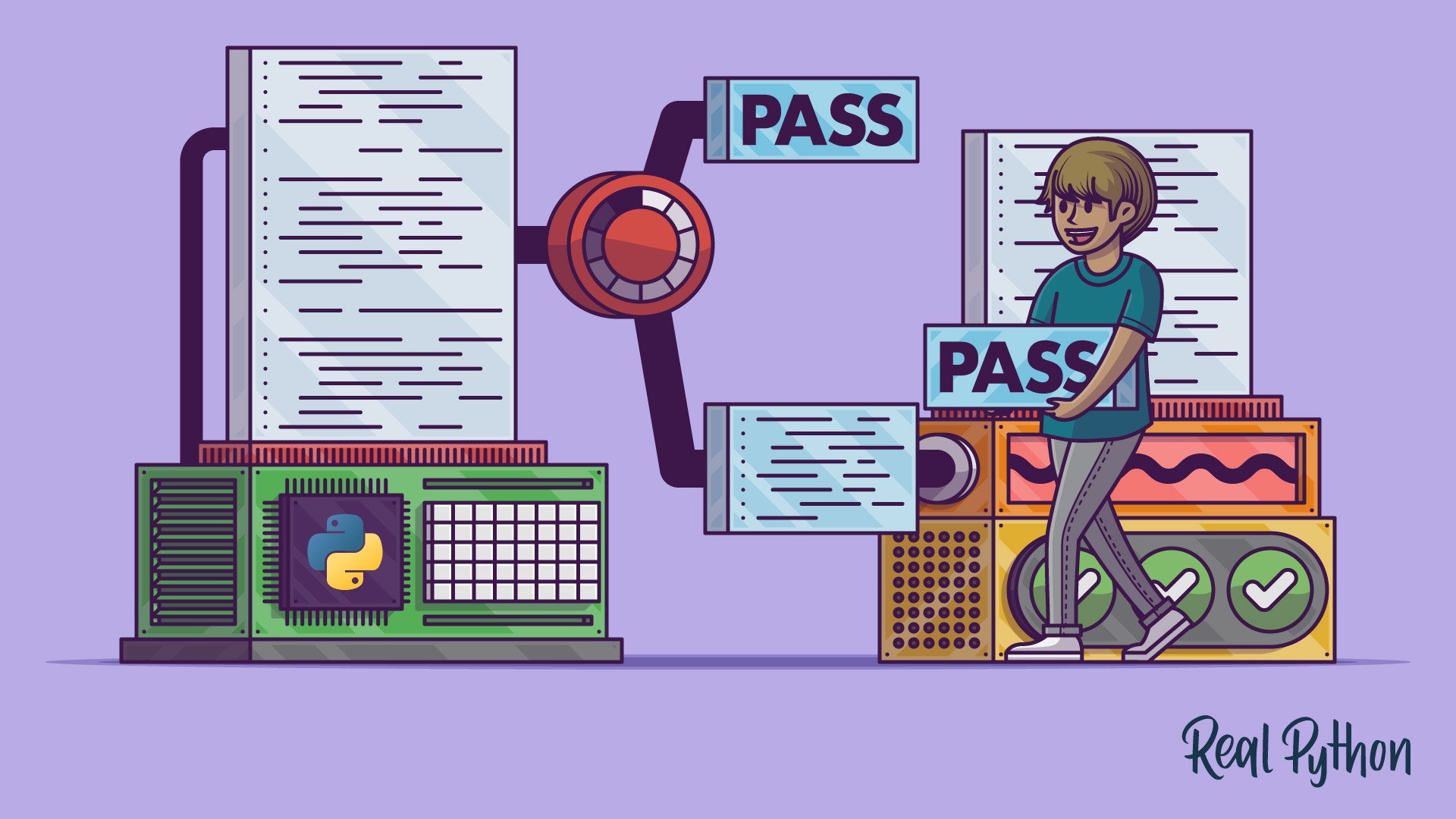

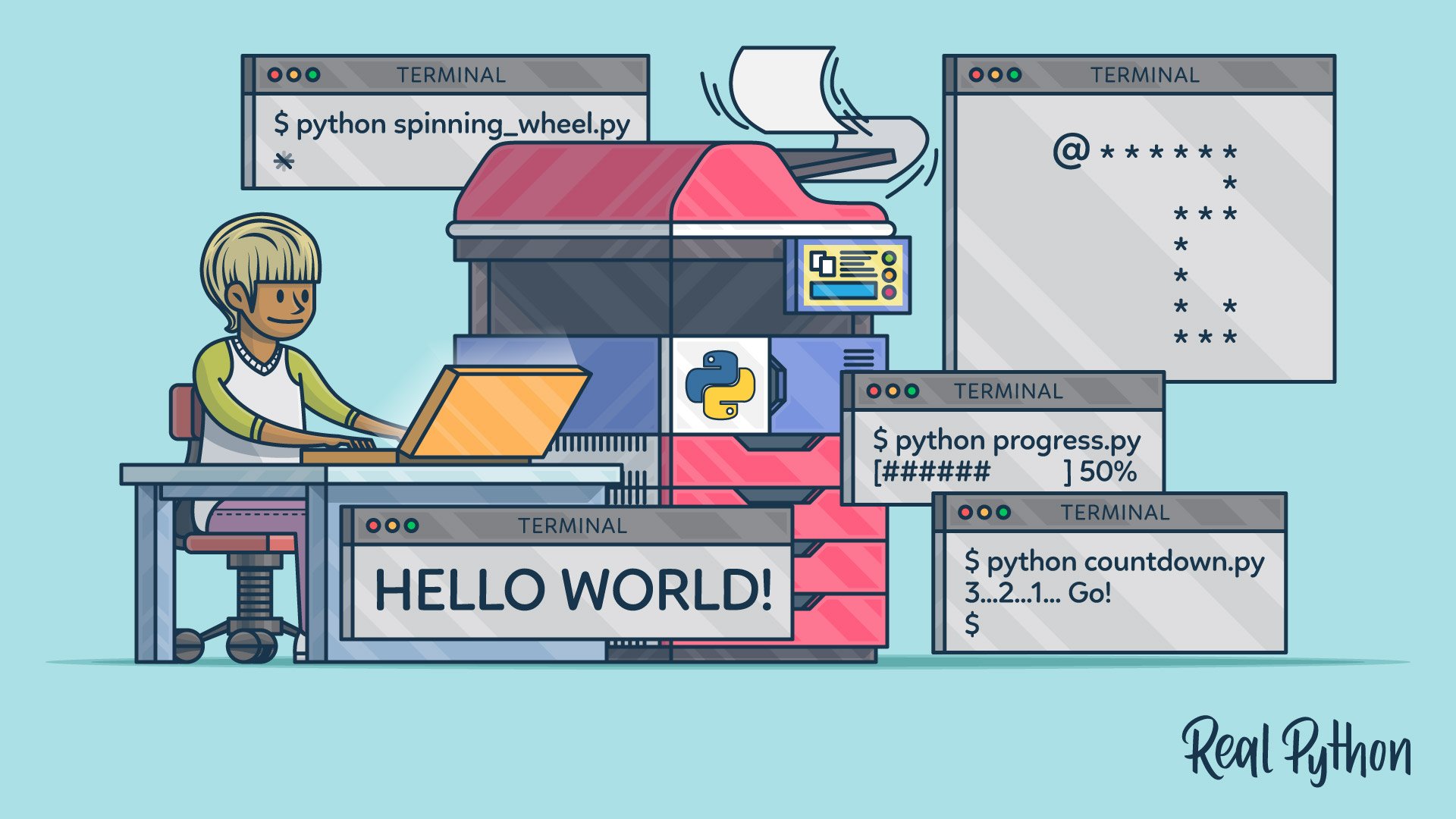
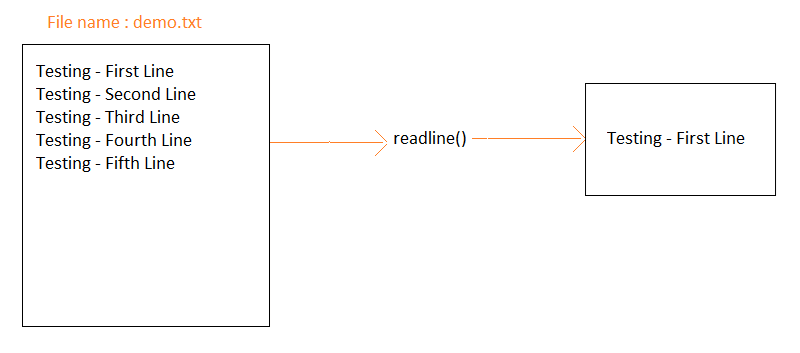
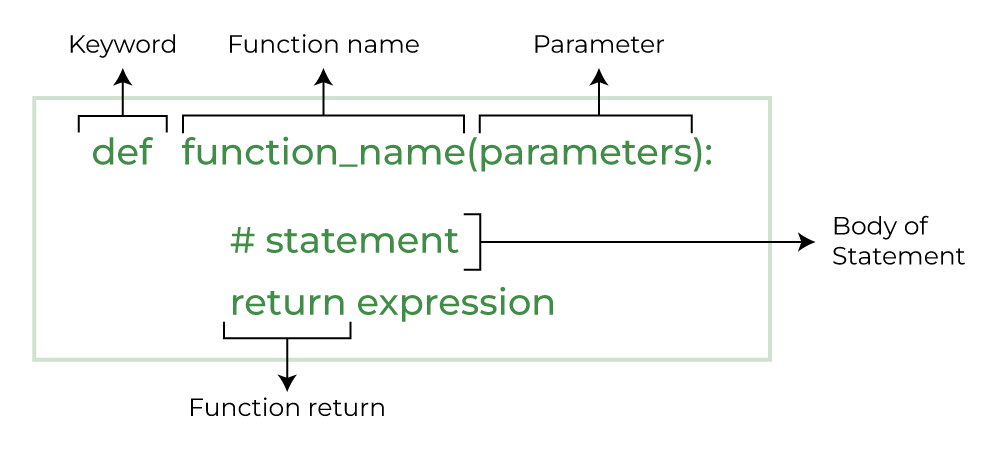
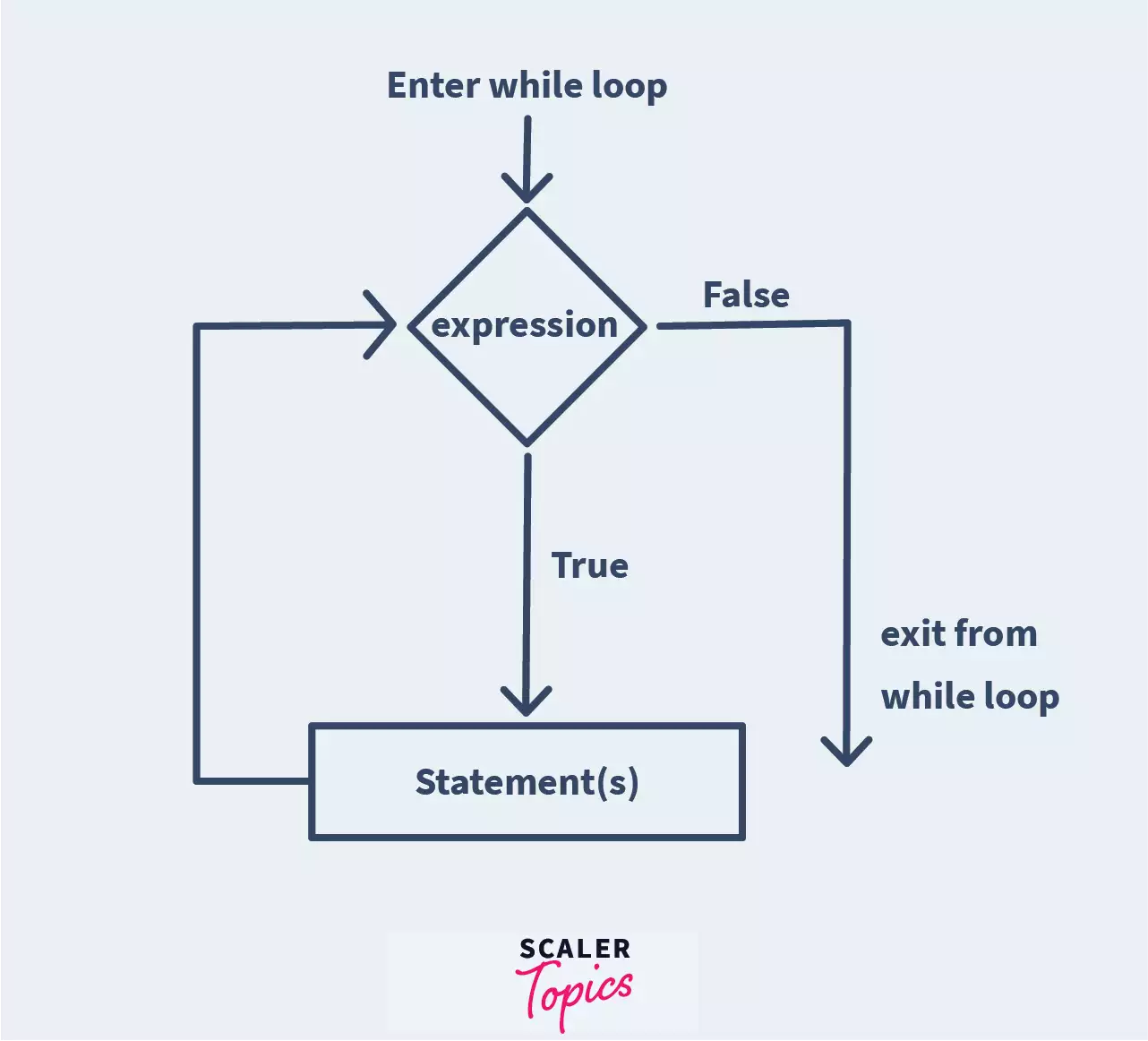

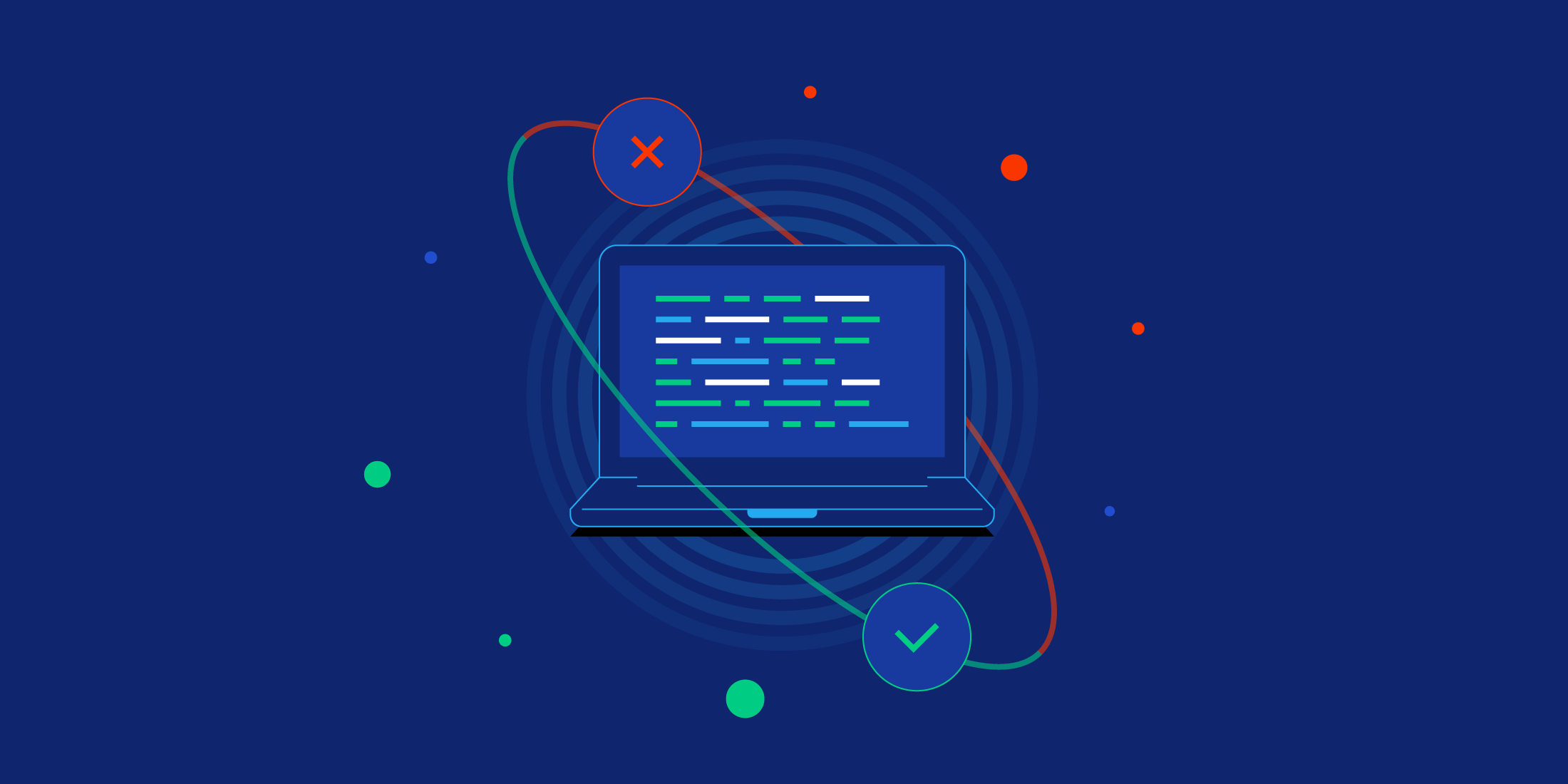
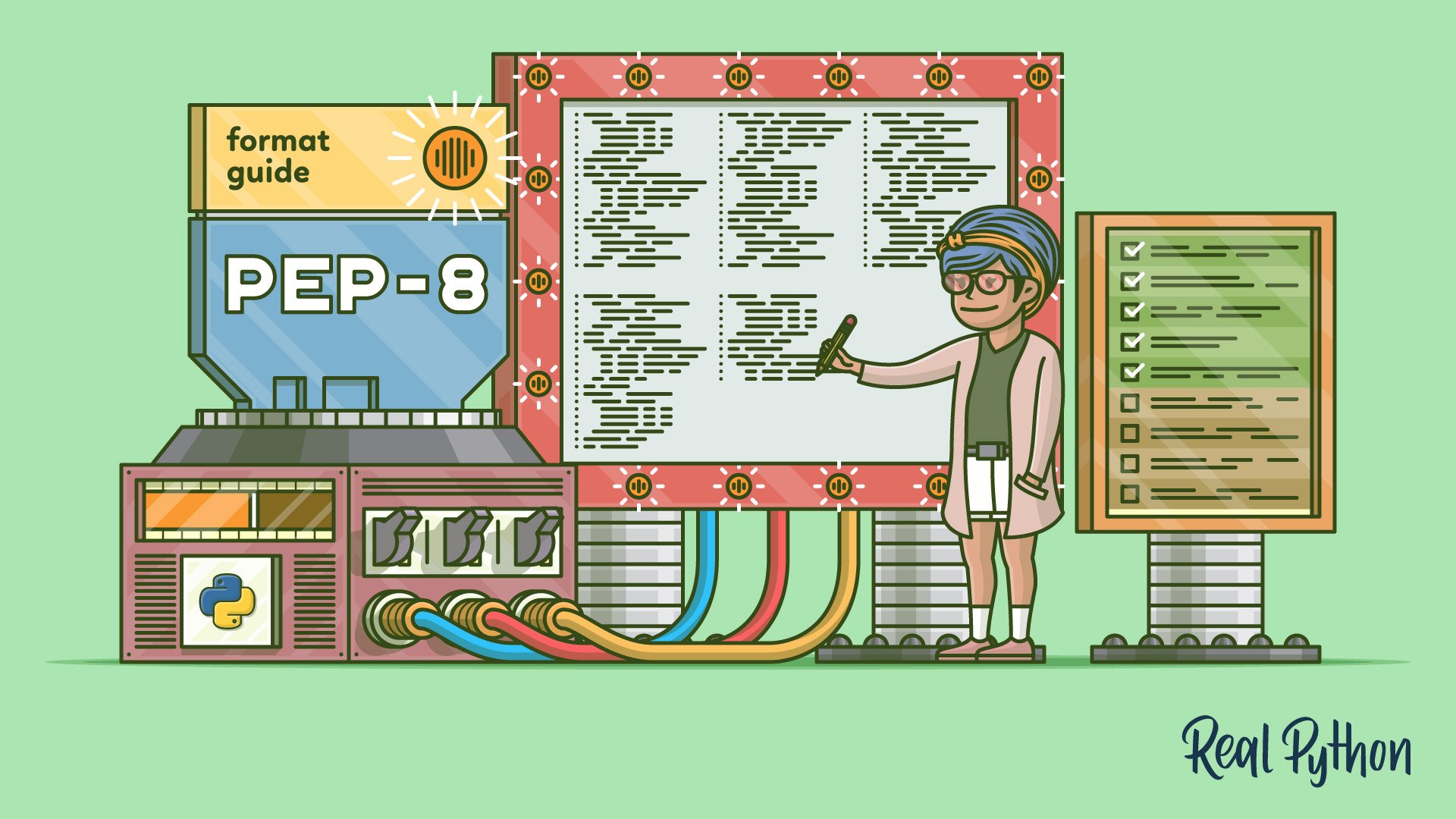

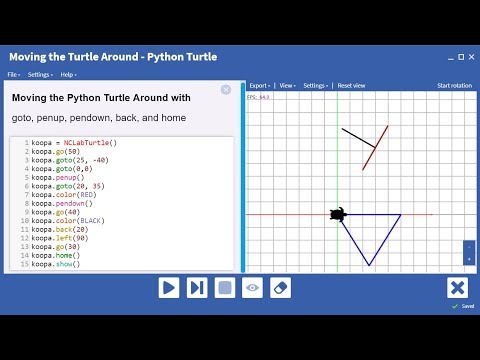
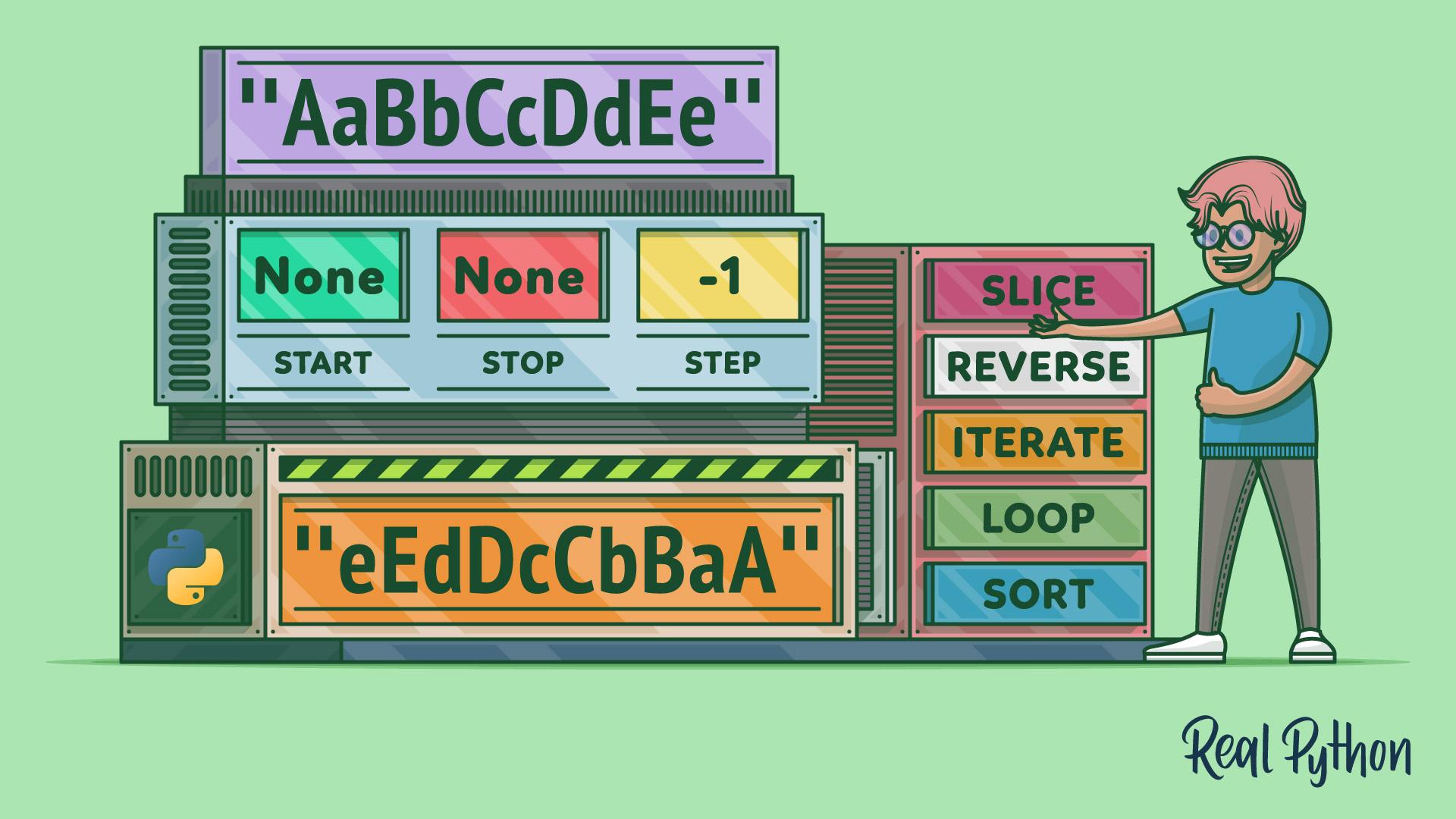

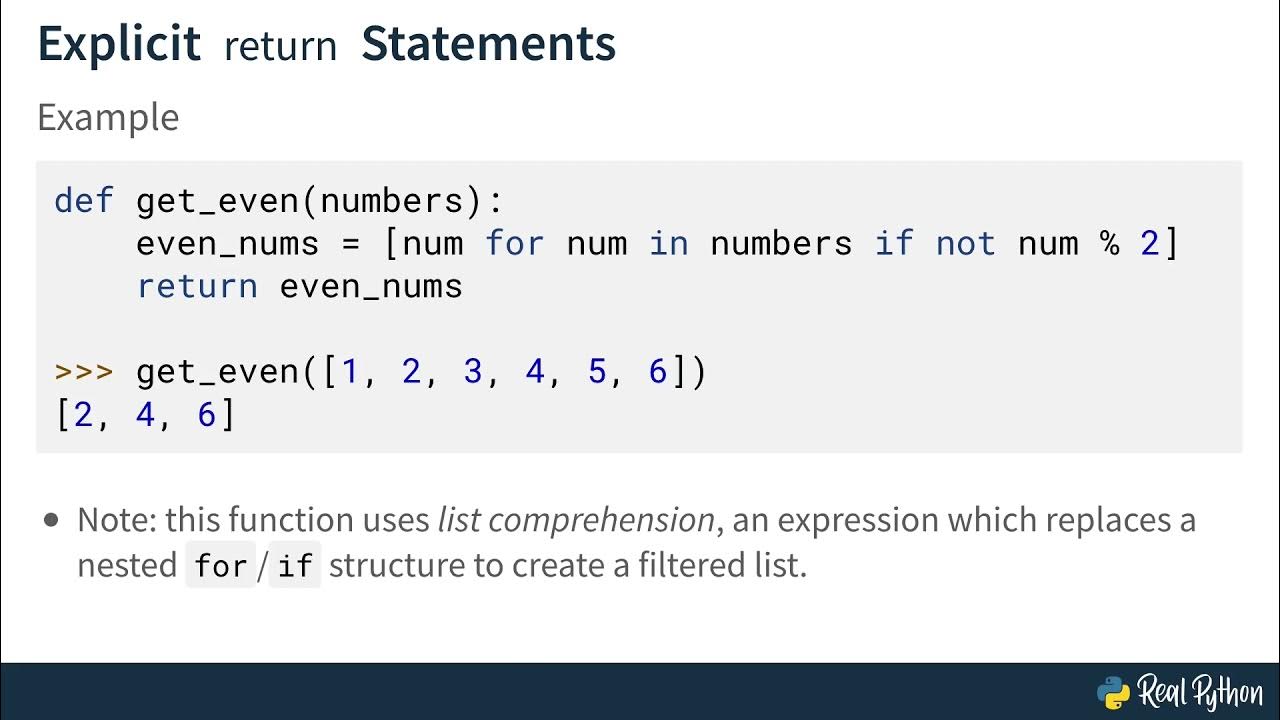

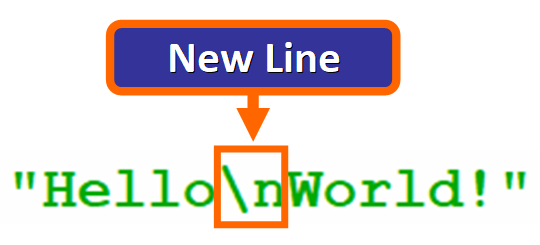
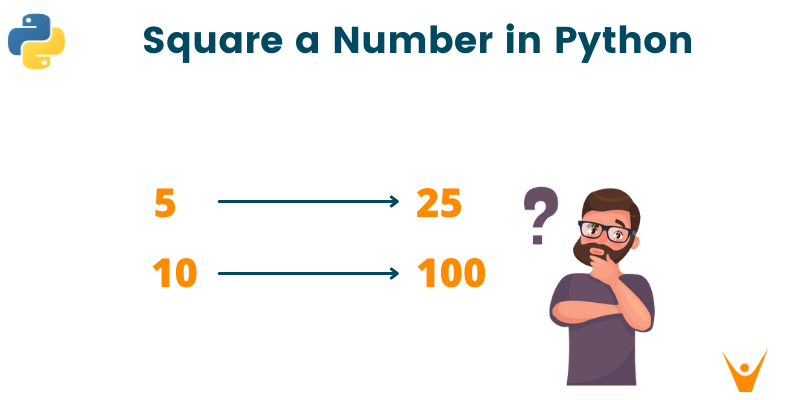

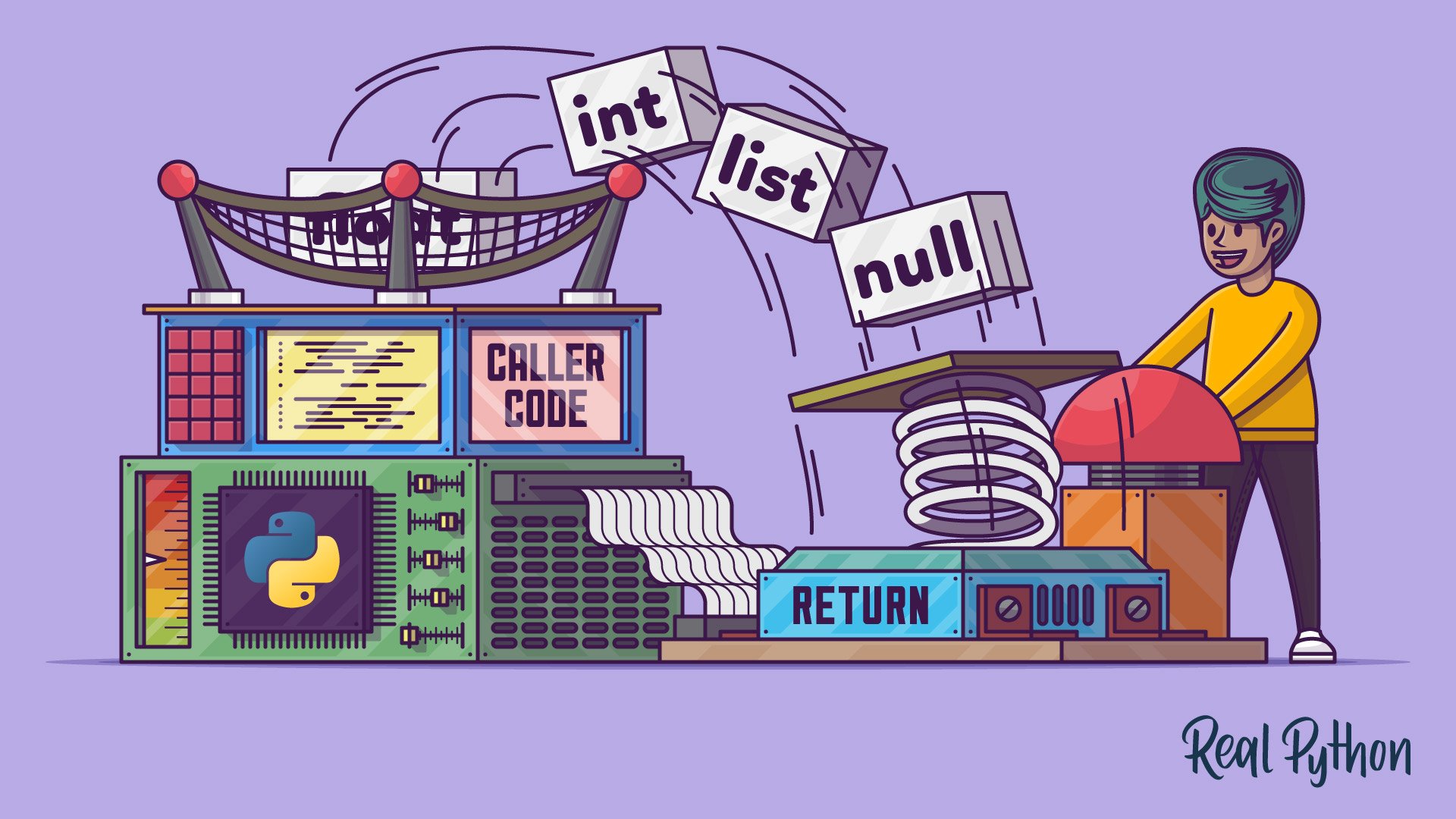
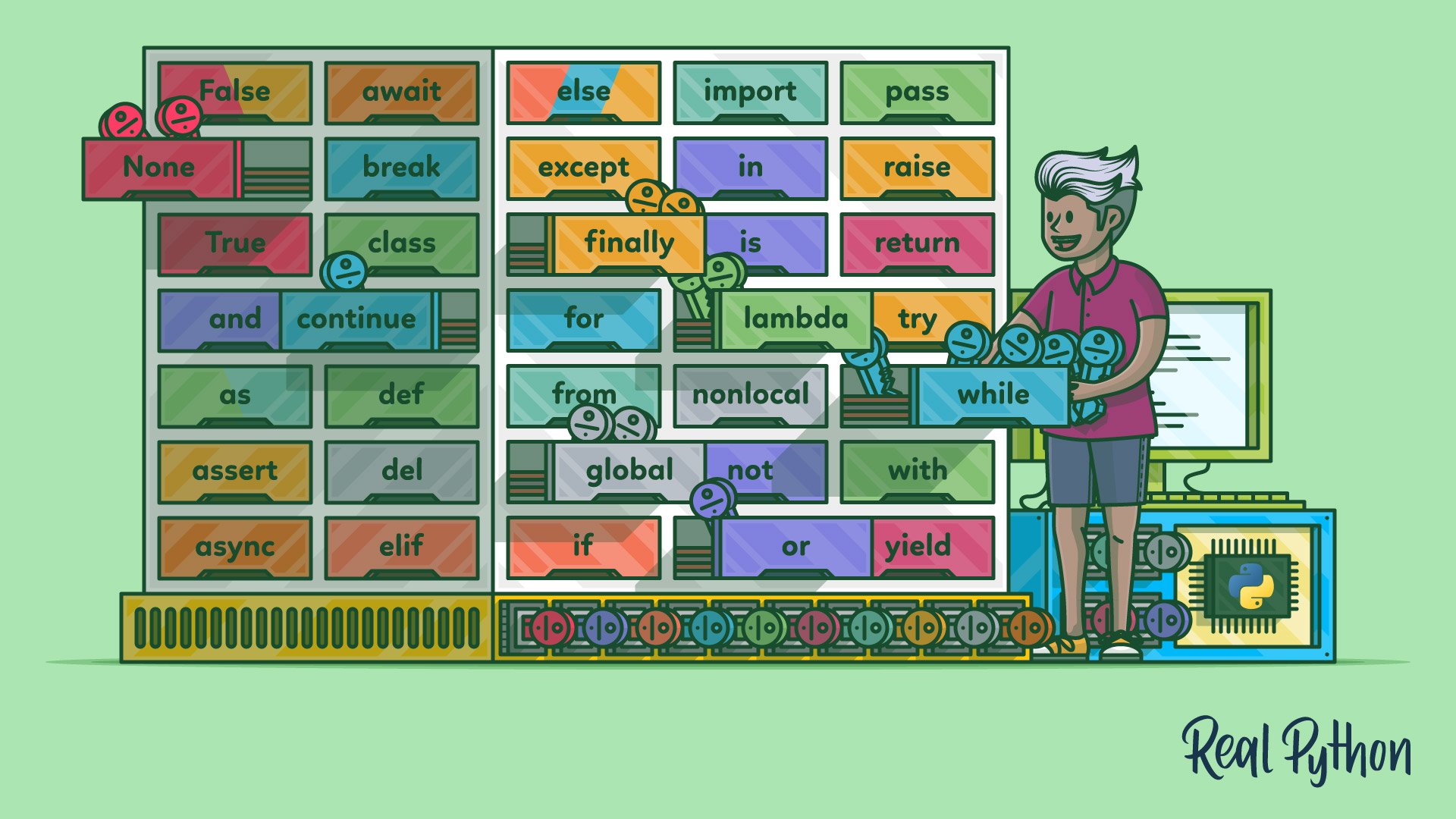
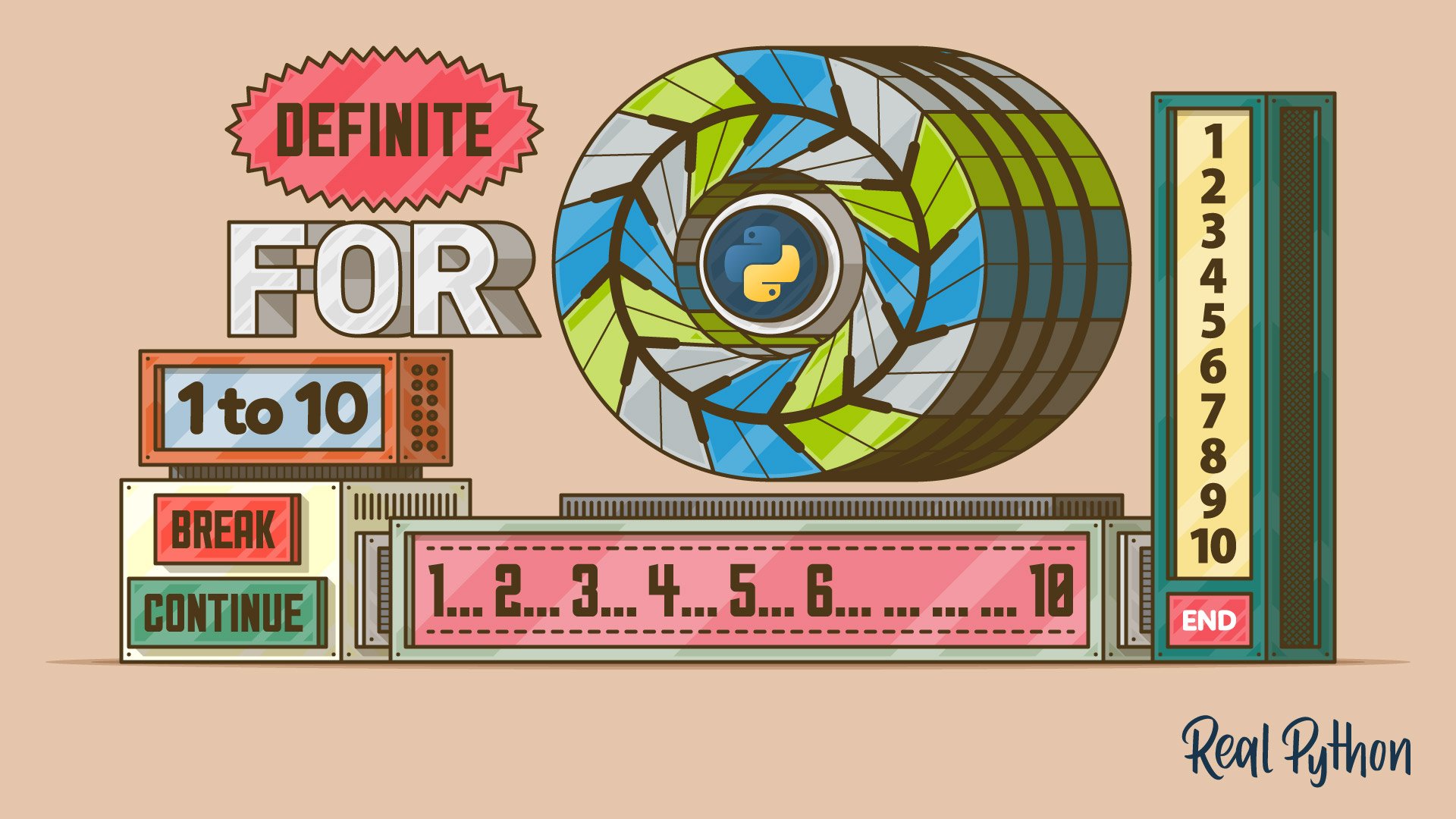
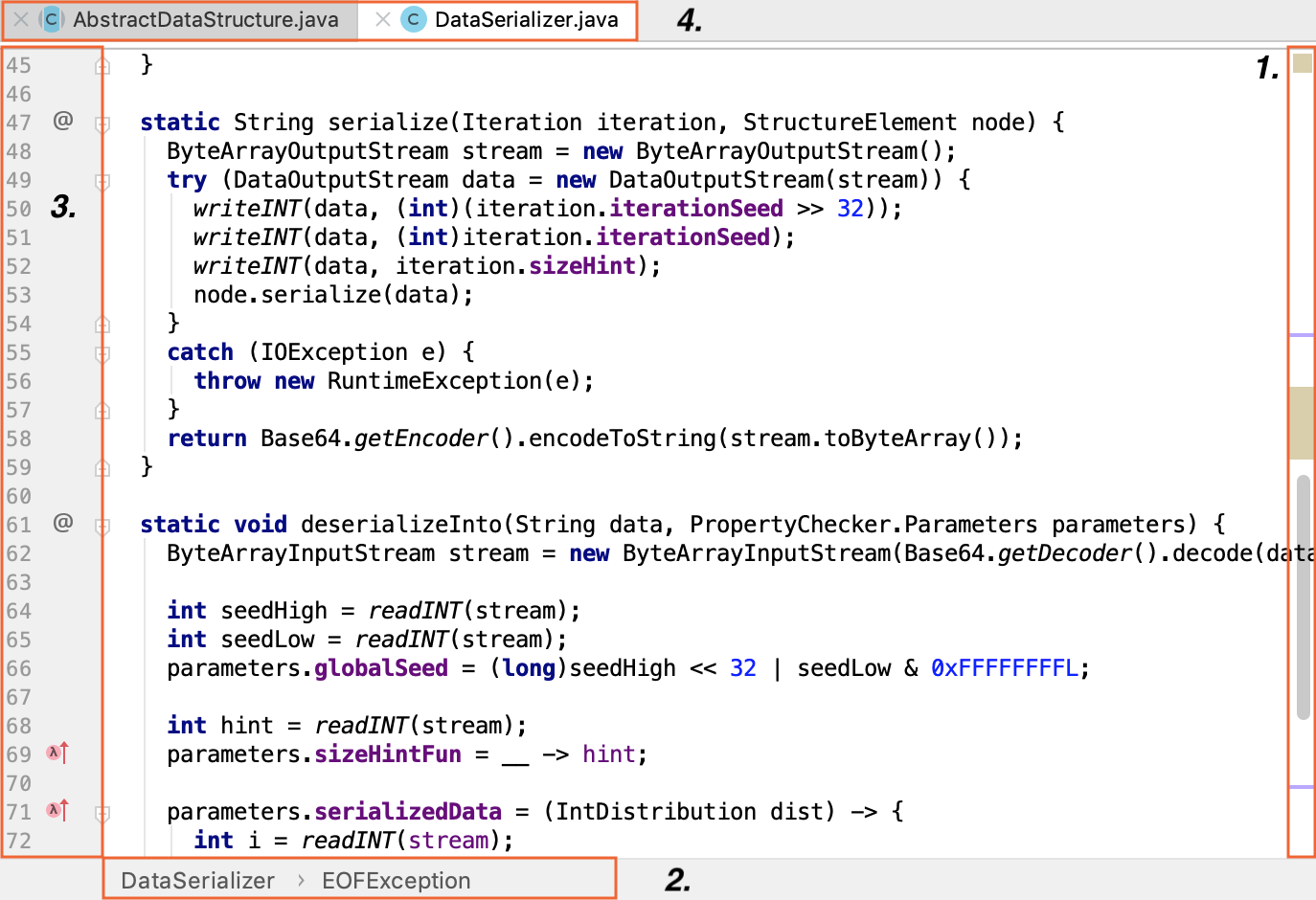
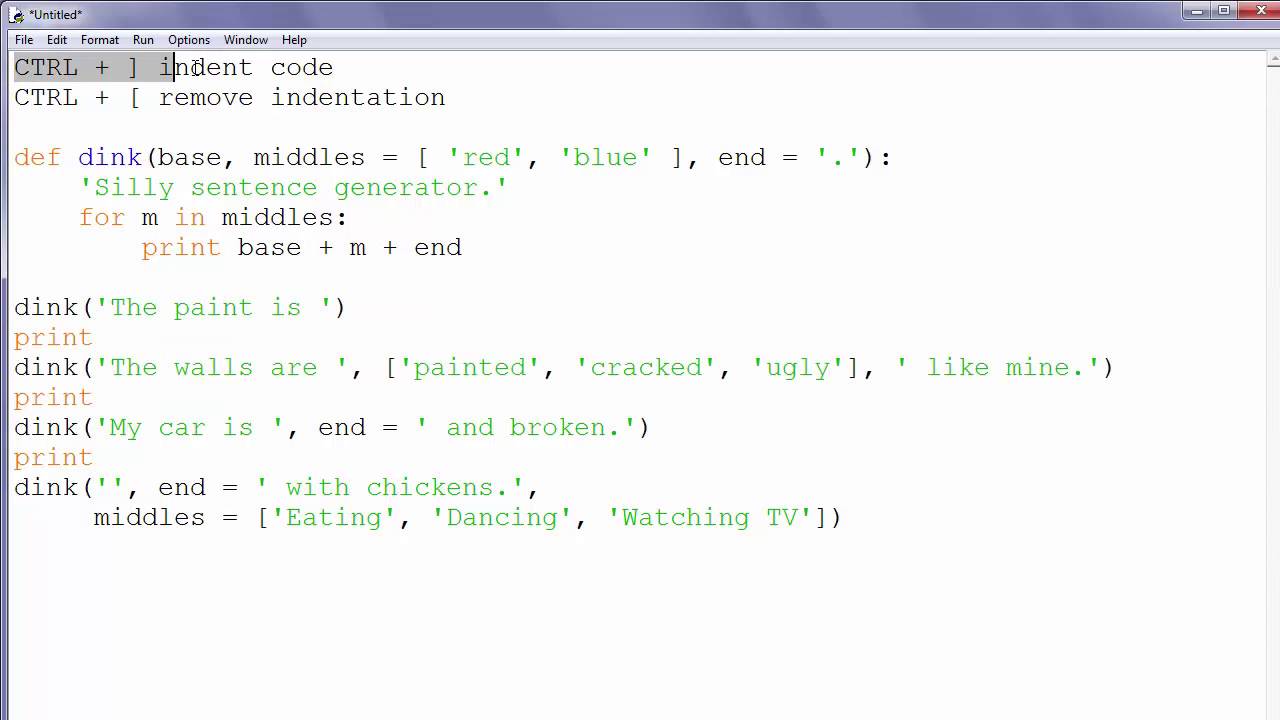
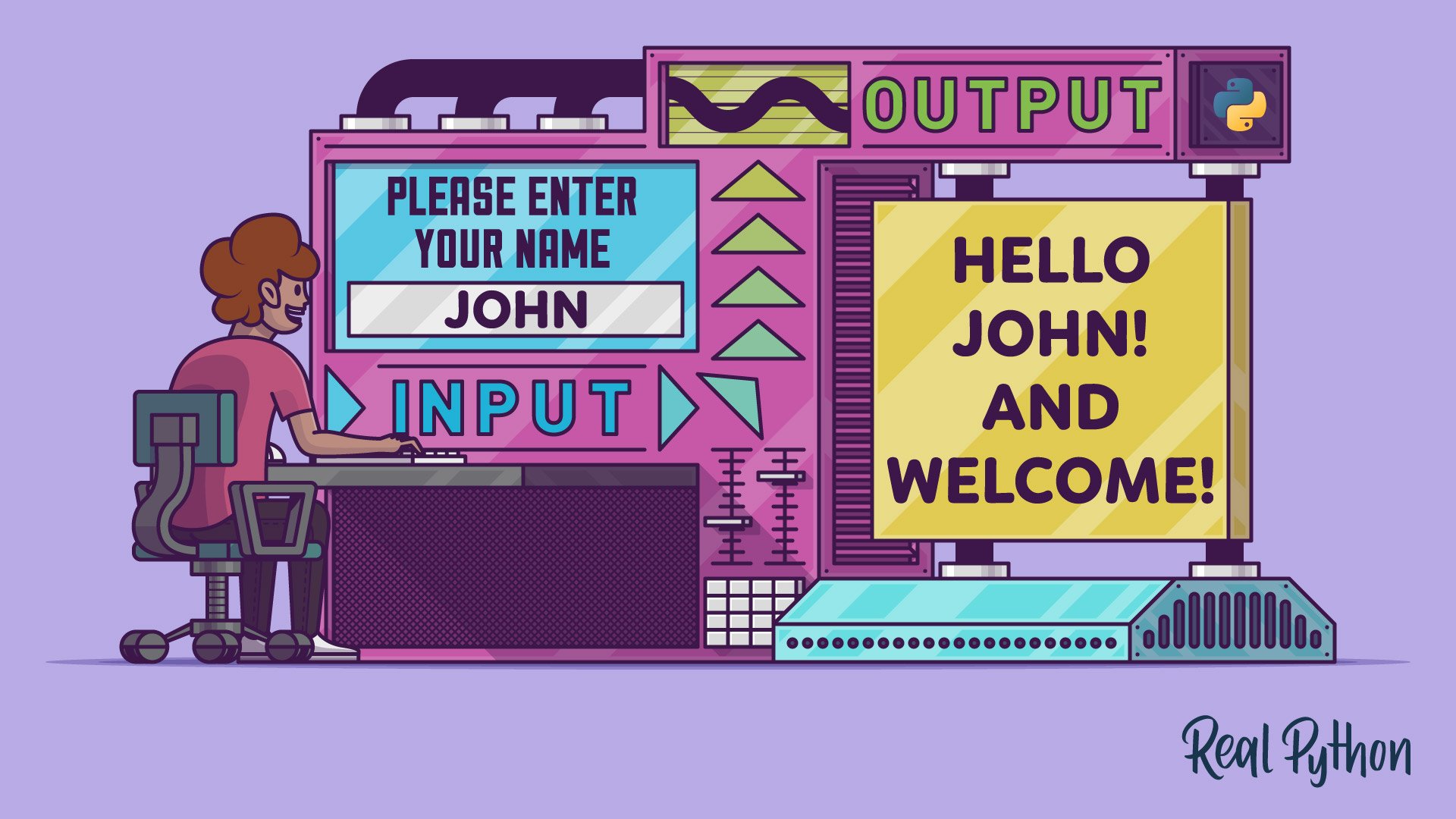
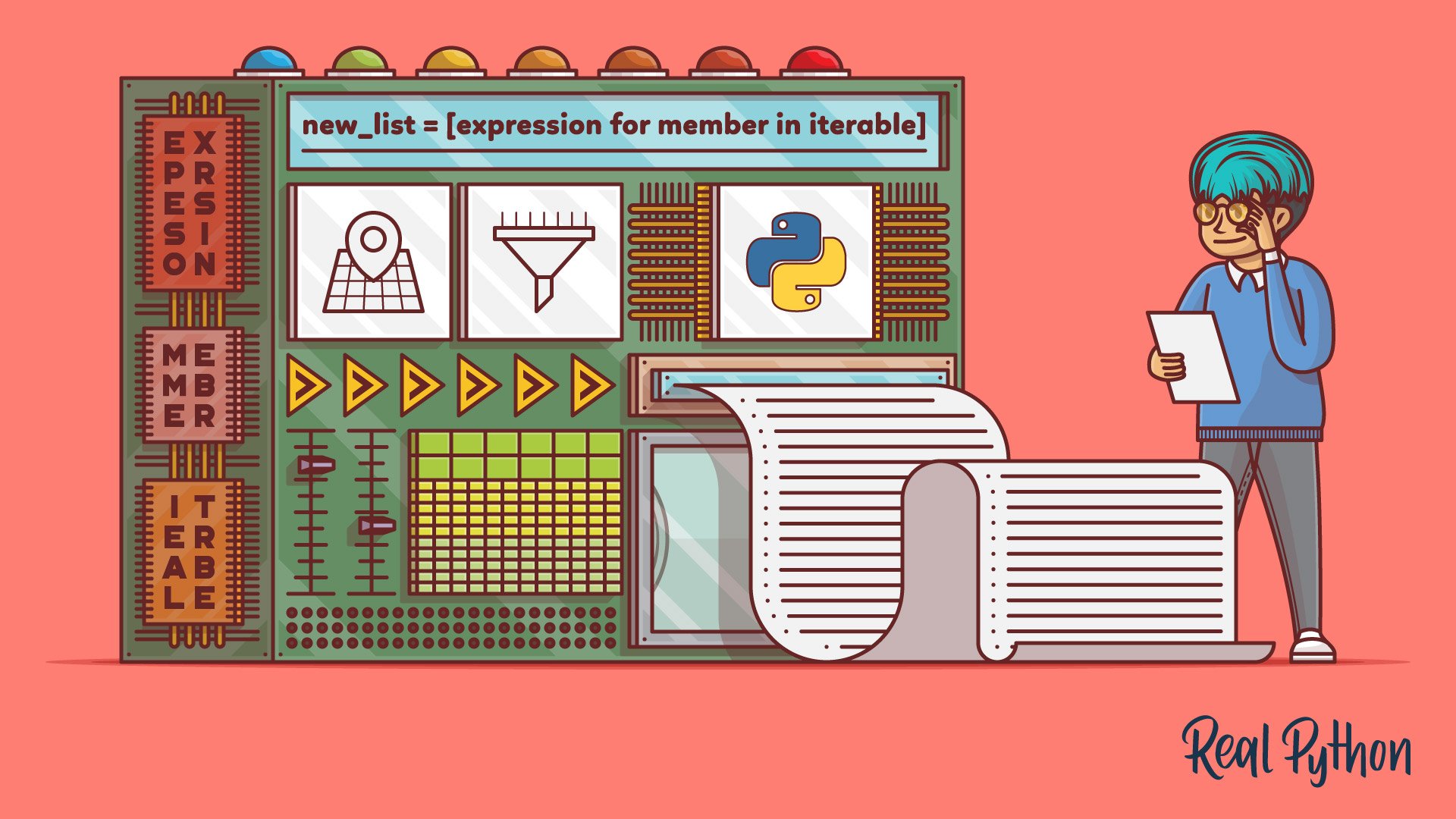
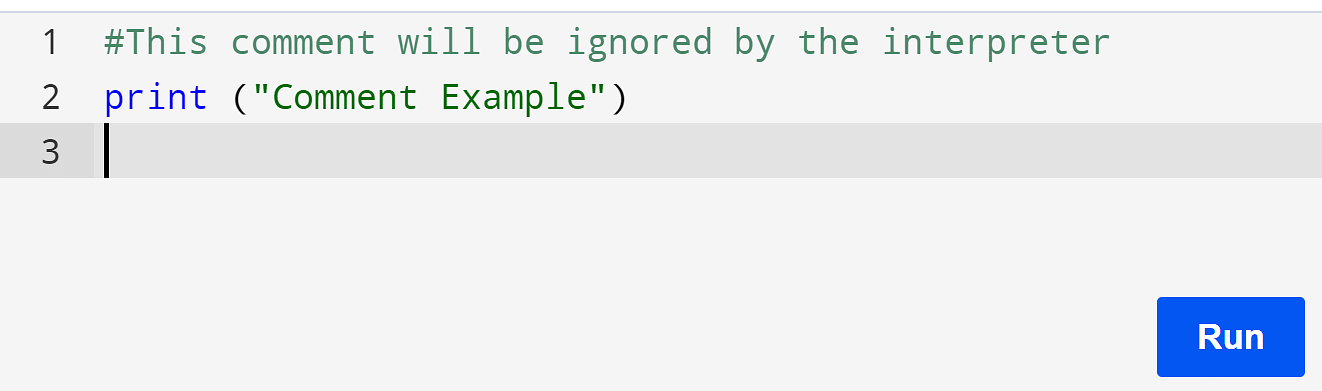

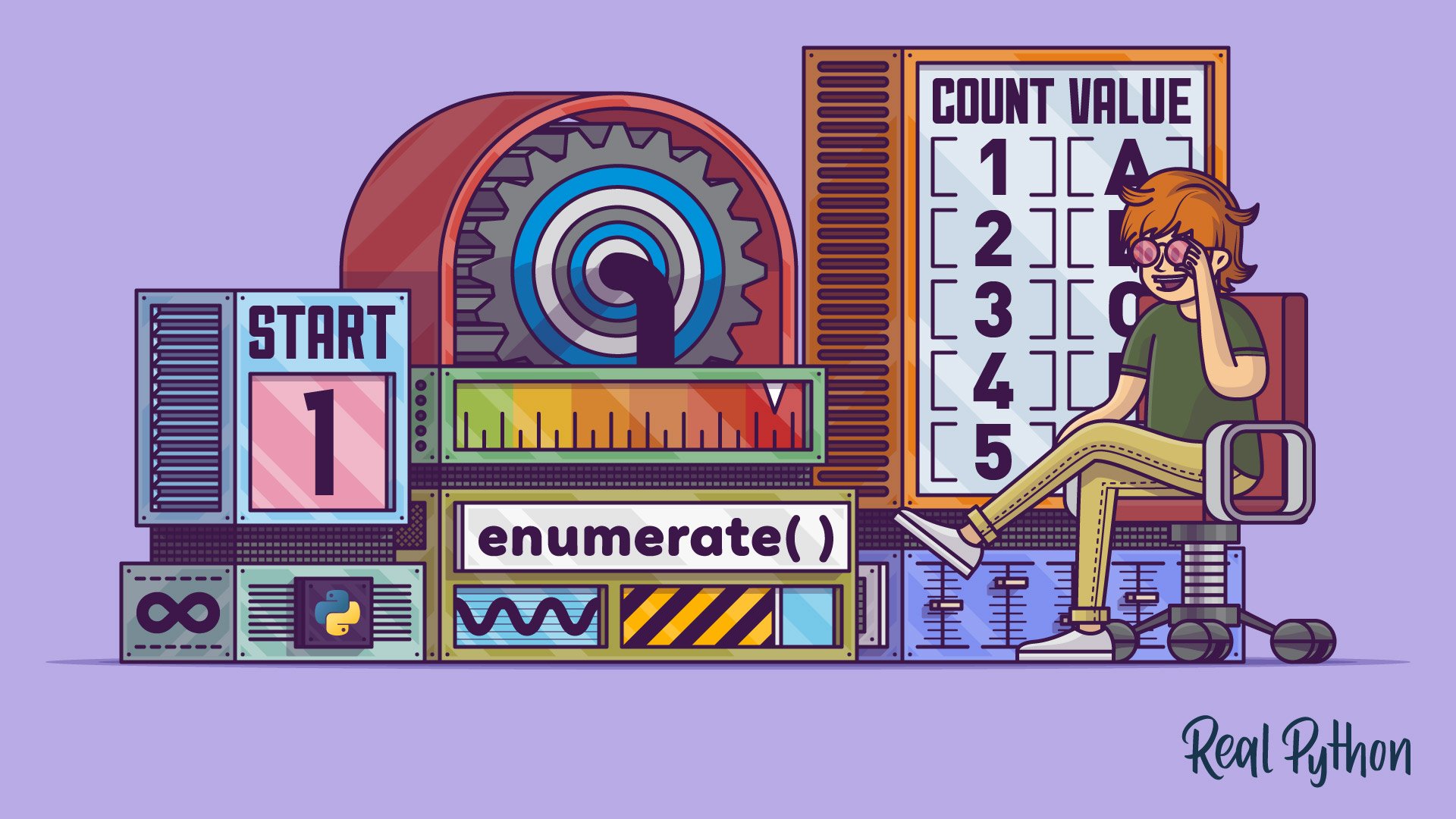
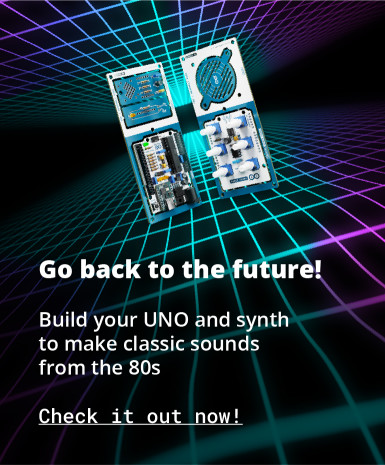
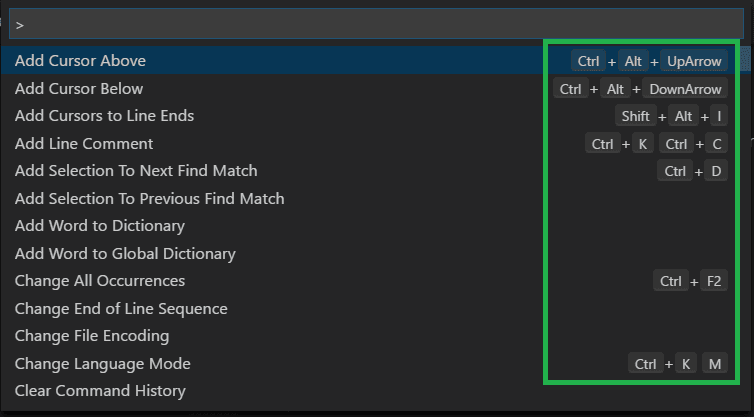
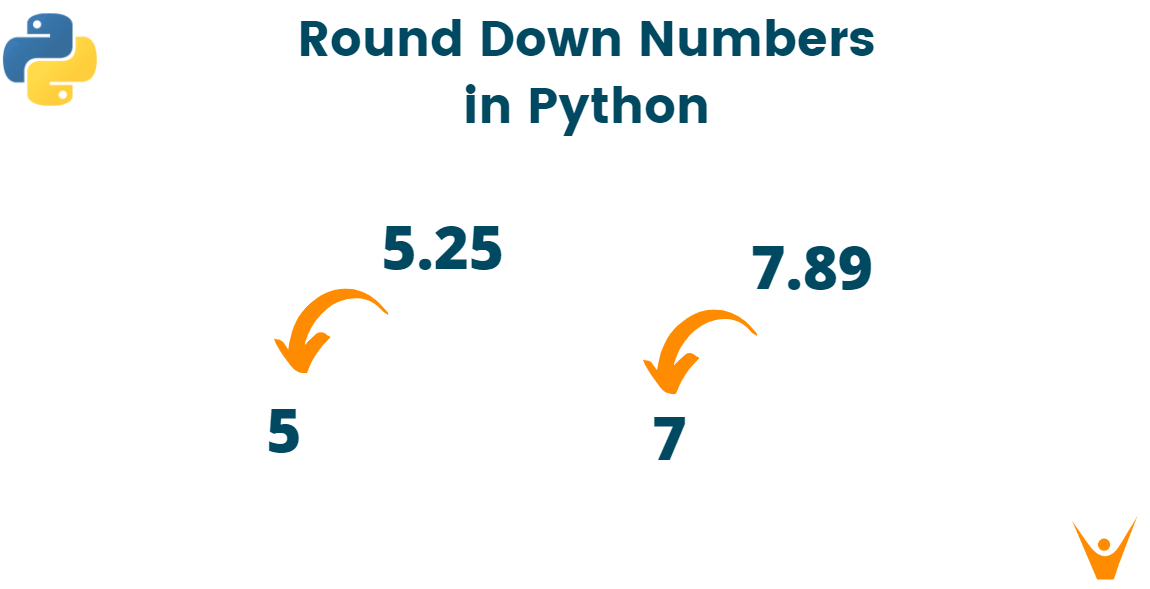
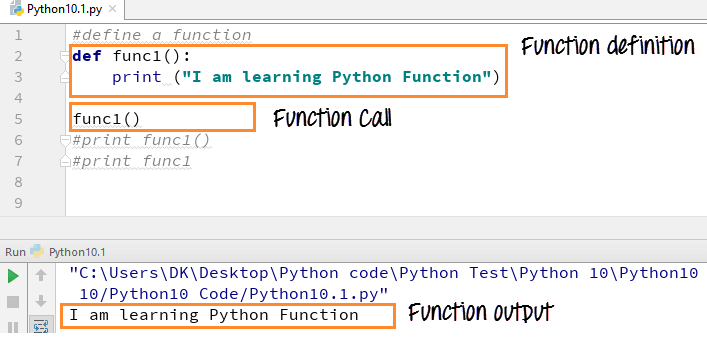
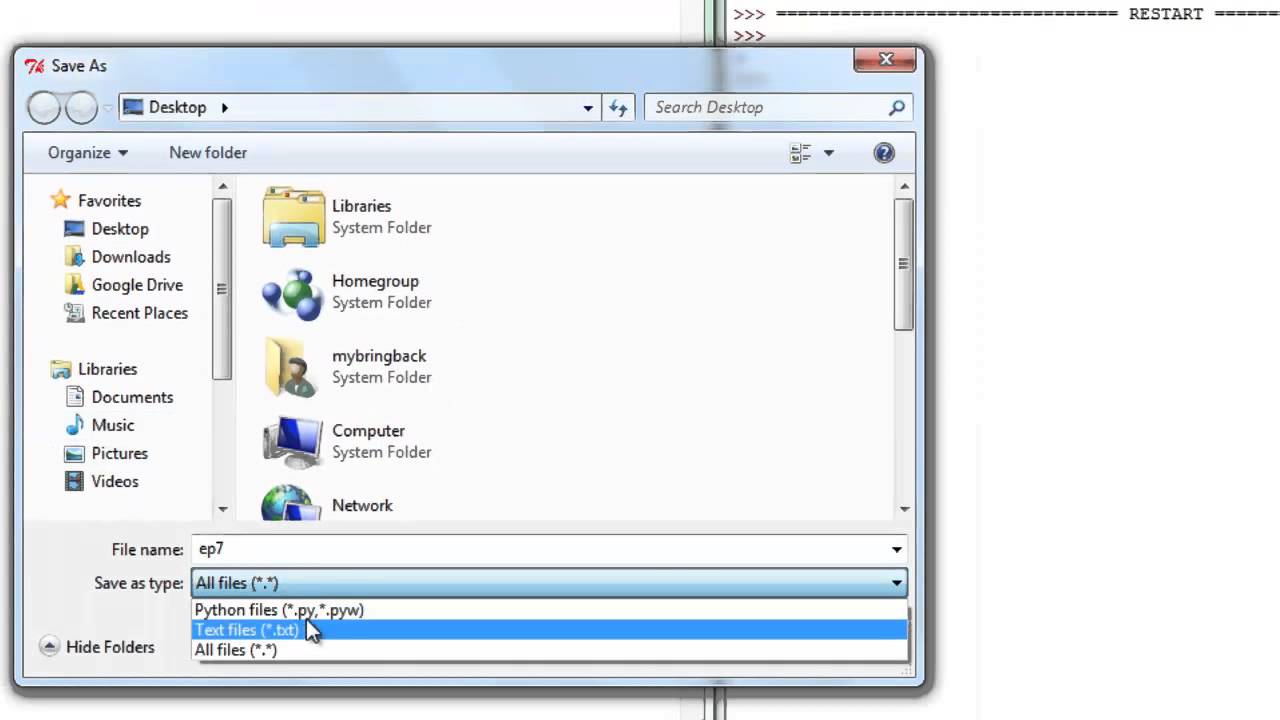
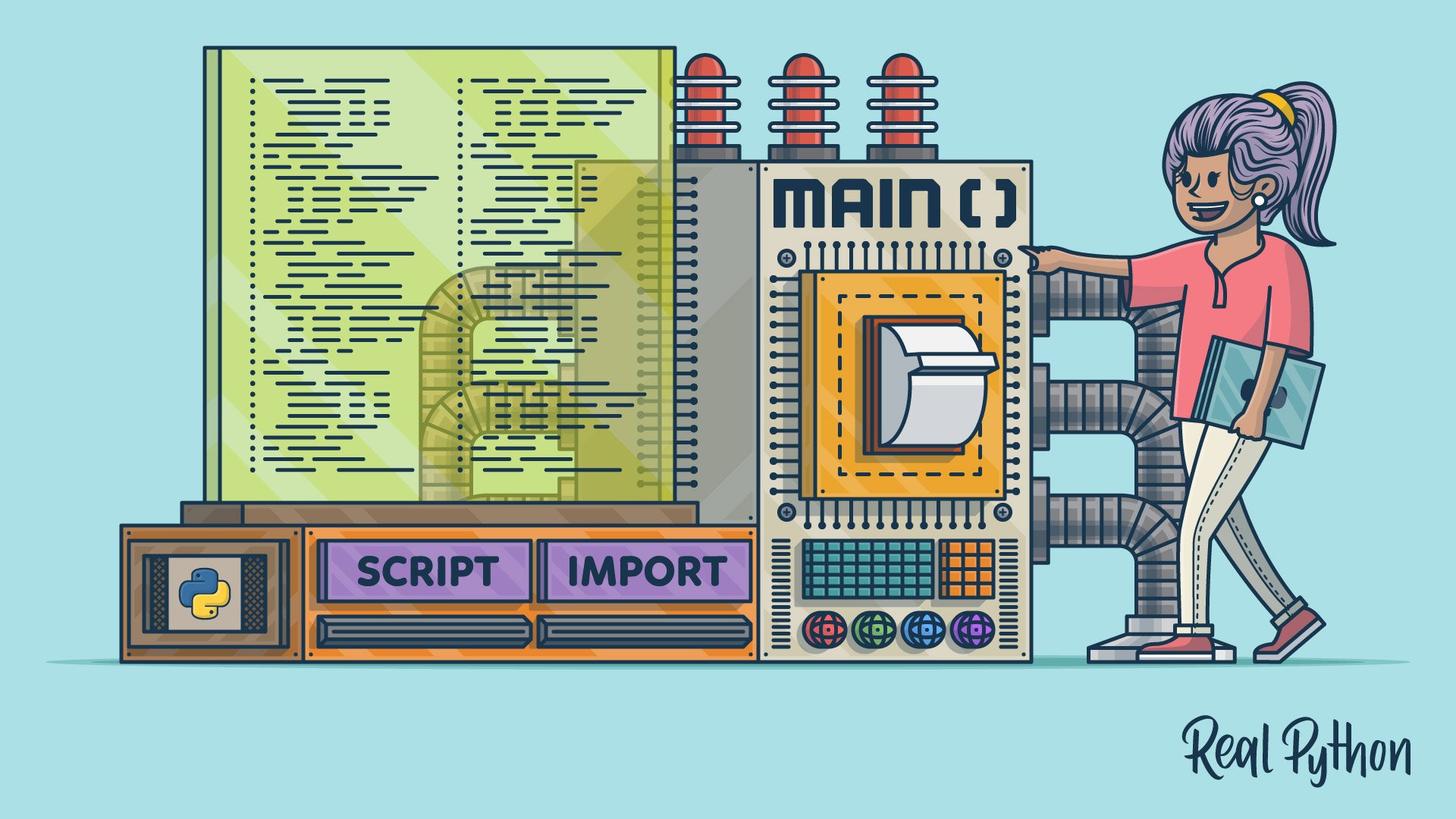
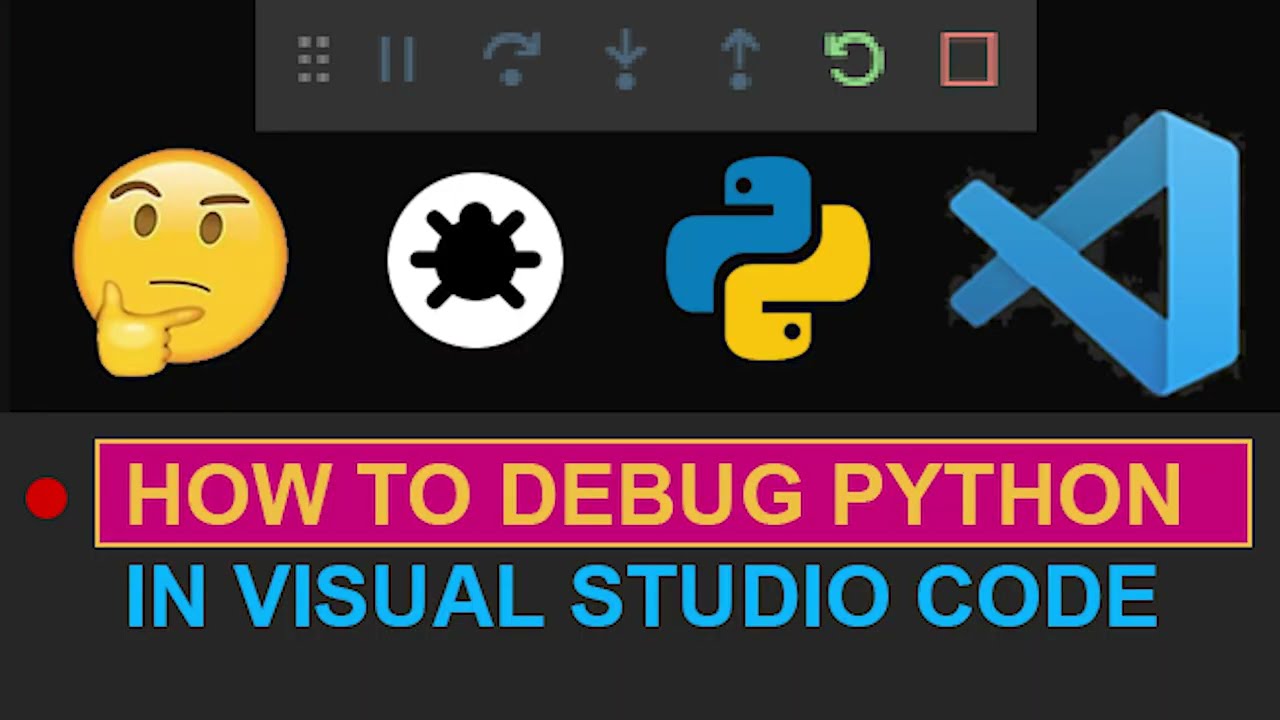
Article link: how to make python code go back to a line.
Learn more about the topic how to make python code go back to a line.
- 2 Ways How Loop Back to the Beginning of a Program in Python
- How to make program go back to the top of the code instead of …
- How to go back to a line of code…? – DaniWeb
- How can I go back to a specific code line? – Python-forum.io
- goto: is there a way to get python to go to a specific line and …
- break, continue, and return :: Learn Python by Nina Zakharenko
- Python break, continue and pass Statements – Tutorialspoint
- How to make my python script go back to a certain line
See more: nhanvietluanvan.com/luat-hoc