Golang Write To File
### File Handling in Go
File handling is an essential aspect of any programming language, and Golang offers a straightforward and convenient way to interact with files. There are several operations you can perform while writing to a file, such as opening a file, writing data to it, appending data to an existing file, creating a new file, and more. Let’s dive into each of these operations to understand how to write to a file in Golang effectively.
#### 1. Opening a File
Before we can write data to a file, we need to open it first. In Golang, the `os.OpenFile()` function is used to open a file in various modes such as read-only, write-only, or read-write. Here’s an example of opening a file for writing:
“`go
file, err := os.OpenFile(“example.txt”, os.O_WRONLY|os.O_CREATE, 0644)
if err != nil {
log.Fatal(err)
}
defer file.Close()
“`
In the example above, we use the `os.OpenFile()` function to open a file named “example.txt” in write-only mode. The second argument `os.O_WRONLY|os.O_CREATE` specifies that we want to open the file for writing and create it if it doesn’t exist. The third argument `0644` represents the file’s permissions.
#### 2. Writing Data to a File
Once we have opened the file, we can start writing data to it. Golang provides the `file.Write()` function to write bytes to a file. Here’s an example of writing a byte slice to a file:
“`go
data := []byte(“Hello, World!”)
_, err := file.Write(data)
if err != nil {
log.Fatal(err)
}
“`
In the above example, we pass a byte slice `data` containing the string “Hello, World!” to the `file.Write()` function. The `Write()` function returns the number of bytes written and an error if any.
#### 3. Writing Strings to a File
In addition to writing byte slices, Golang also allows us to write strings directly to a file using the `file.WriteString()` function. Here’s an example:
“`go
str := “This is a string.”
_, err := file.WriteString(str)
if err != nil {
log.Fatal(err)
}
“`
In the above example, we use the `file.WriteString()` function to write the string “This is a string.” to the file.
#### 4. Writing Byte Slices to a File
If we have data in the form of a byte slice, we can write it directly to a file using the `file.Write()` function. Here’s an example:
“`go
data := []byte{72, 101, 108, 108, 111, 44, 32, 87, 111, 114, 108, 100, 33}
_, err := file.Write(data)
if err != nil {
log.Fatal(err)
}
“`
In the above example, we have a byte slice `data` containing the ASCII values of the characters in the string “Hello, World!”. We pass this byte slice to the `file.Write()` function to write it to the file.
#### 5. Appending Data to a File
Sometimes, we may want to append data to an existing file instead of overwriting its contents. In Golang, this can be achieved using the `os.OpenFile()` function with the appropriate flag. Here’s an example:
“`go
file, err := os.OpenFile(“example.txt”, os.O_WRONLY|os.O_APPEND|os.O_CREATE, 0644)
if err != nil {
log.Fatal(err)
}
defer file.Close()
str := “This is appended text.”
_, err := file.WriteString(str)
if err != nil {
log.Fatal(err)
}
“`
In the above example, we pass the `os.O_APPEND` flag to `os.OpenFile()` to indicate that we want to append data to the file. We then use the `file.WriteString()` function to write the string “This is appended text.” to the file.
#### 6. Creating and Writing to a New File
If we want to create a new file and write data to it, we can use the `os.Create()` function. Here’s an example:
“`go
file, err := os.Create(“new_file.txt”)
if err != nil {
log.Fatal(err)
}
defer file.Close()
str := “This is new file text.”
_, err := file.WriteString(str)
if err != nil {
log.Fatal(err)
}
“`
In the above example, we use the `os.Create()` function to create a new file named “new_file.txt”. We then write the string “This is new file text.” to the file using the `file.WriteString()` function.
#### 7. Error Handling and File Closing
It is important to handle errors properly while performing file operations in Golang. In the examples provided above, we use the `log.Fatal(err)` function to log any errors encountered during file handling. Additionally, we use the `defer` statement to ensure that the file is closed after we are done with it.
### FAQs
Q1. How do I write to a file in Golang?
To write to a file in Golang, you need to open the file using the `os.OpenFile()` function, write data to it using functions like `file.Write()` or `file.WriteString()`, and then close the file using the `file.Close()` function.
Q2. How do I append text to a file in Golang?
To append text to a file in Golang, you need to open the file in append mode using the `os.OpenFile()` function with the `os.O_APPEND` flag, and then use functions like `file.Write()` or `file.WriteString()` to write the text to the file.
Q3. How do I write an array to a file in Golang?
To write an array to a file in Golang, you can convert the array to a string or a byte slice and then write it to the file using functions like `file.Write()` or `file.WriteString()`.
Q4. How do I overwrite a file in Golang?
To overwrite a file in Golang, you can open the file in write mode using the `os.OpenFile()` function with the `os.O_WRONLY` flag, and then write the new data to it using functions like `file.Write()` or `file.WriteString()`.
Q5. How do I append to a file in Golang?
To append data to a file in Golang, you need to open the file in append mode using the `os.OpenFile()` function with the `os.O_APPEND` flag, and then use functions like `file.Write()` or `file.WriteString()` to write the data to the file.
Q6. How do I write bytes to a file in Golang?
To write bytes to a file in Golang, you can use the `file.Write()` function and pass a byte slice containing the data you want to write.
Q7. How do I create a file if it doesn’t exist in Golang?
To create a file if it doesn’t exist in Golang, you can use the `os.OpenFile()` function with the `os.O_CREATE` flag. If the file doesn’t exist, it will be created.
Write Files With Go
Keywords searched by users: golang write to file Write to file go, Append text to file golang, Write array to file golang, Golang overwrite file, Append file golang, Byte to file golang, Golang create file if not exists, Open file Golang
Categories: Top 80 Golang Write To File
See more here: nhanvietluanvan.com
Write To File Go
What does it mean to write to a file?
Writing to a file refers to the process of sending data from a program or application and saving it to a file that resides on a storage device, such as a hard drive or SSD. The data can be text, numbers, or any other type of information that needs to be persisted for future use.
Why do we need to write to a file?
Writing to a file is essential for a wide range of applications. It allows us to store data, such as user preferences, configuration settings, and program output. By saving data to a file, we can preserve it between different program runs or even share it with other programs or users. Additionally, writing to a file allows us to create logs, generate reports, and analyze data, among other things.
How to write to a file in different programming languages?
The process of writing to a file may differ slightly depending on the programming language you are using. However, most languages provide similar functionalities and methods to accomplish this task. Let’s take a look at how to write to a file in a few popular programming languages:
1. Python:
In Python, you can use the `open()` function with the “w” mode to open a file for writing. After opening the file, you can use the `write()` method to write data to it. Finally, don’t forget to close the file using the `close()` method.
“`python
file = open(“filename.txt”, “w”)
file.write(“Hello, World!”)
file.close()
“`
2. Java:
In Java, you can use the `FileWriter` class to write data to a file. Create a `FileWriter` object, specify the file path, and then use the `write()` method to write data. Finally, close the file using the `close()` method.
“`java
import java.io.FileWriter;
import java.io.IOException;
public class FileWriterExample {
public static void main(String[] args) {
try {
FileWriter file = new FileWriter(“filename.txt”);
file.write(“Hello, World!”);
file.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
“`
3. C#:
In C#, you can use the `StreamWriter` class to write data to a file. Create a `StreamWriter` object, specify the file path, and then use the `Write()` or `WriteLine()` method to write data. Finally, close the file using the `Close()` method.
“`csharp
using System;
using System.IO;
class Program {
static void Main() {
using (StreamWriter file = new StreamWriter(“filename.txt”)) {
file.Write(“Hello, World!”);
}
}
}
“`
These are just a few examples, but the process of writing to a file in other programming languages will often follow a similar pattern. Consult the documentation or resources specific to your programming language for detailed instructions and best practices.
What are the different file writing modes?
When opening a file for writing, you can specify a mode that determines how the file should be treated. Some of the common file writing modes include:
1. “w” (Write):
Opens the file for writing. If the file already exists, it gets overwritten. If the file does not exist, a new file is created.
2. “a” (Append):
Opens the file for writing, but appends new data to the end of the existing file. If the file does not exist, a new file is created.
3. “x” (Exclusive creation):
Creates a new file for writing. If the file already exists, an exception is thrown.
These modes provide flexibility based on your specific use-case. Choose the appropriate mode based on whether you want to overwrite, append, or exclusively create a file.
What are file streams? How are they related to writing to a file?
File streams are a concept used in many programming languages to facilitate reading from and writing to files. A file stream represents a connection between the program and the file it is reading from or writing to. It provides a way to transfer data between the program’s memory and the file on the storage device.
When writing to a file, you typically create a file stream, open the file using the appropriate mode, write data to the stream, and then close the stream. The file stream ensures data integrity and manages the low-level I/O operations required for writing to the file.
Frequently Asked Questions (FAQs):
Q1. What happens if the file I am trying to write to already exists?
A1. If the file already exists and you open it in write mode (“w”), the existing file will be overwritten with the new data. If you want to append data to an existing file, use the append mode (“a”) instead.
Q2. How do I handle errors while writing to a file?
A2. Writing to a file may encounter errors due to various reasons, such as insufficient permissions, lack of available storage, or file system errors. It is essential to handle these errors gracefully by using try-catch blocks or proper exception handling mechanisms offered by your programming language.
Q3. Is it necessary to close the file after writing to it?
A3. Yes, it is good practice to close the file after writing to it. Closing the file ensures that any pending write operations are completed, releases system resources associated with the file, and reduces the risk of data corruption or loss. Many programming languages provide mechanisms like the `close()` method or using blocks to automatically close the file when it goes out of scope.
Q4. Can I write data to multiple files simultaneously?
A4. Yes, you can write data to multiple files simultaneously by creating separate file stream objects and opening each file individually. You can then write data to each file using the appropriate file stream.
In conclusion, writing to a file is a crucial skill for every programmer. Understanding how to write to a file, different file writing modes, and working with file streams can significantly enhance your ability to persist data and create valuable applications. The process of writing to a file may vary slightly between programming languages, but the fundamental concepts remain the same. By mastering this skill, you can improve the functionality and versatility of your programs.
Append Text To File Golang
Working with files is a common requirement when it comes to building applications. In this article, we will explore how to append text to a file using the Go programming language, more commonly known as Golang. We will cover various aspects of the process and provide examples to help you understand the concepts better.
Before we dive into the technicalities, let’s first briefly discuss what Golang is. Developed by Google, Golang is an open-source programming language designed for simplicity, readability, and efficiency. Its robust standard library makes it ideal for system-level programming and building scalable applications.
Appending text to a file is the process of adding new content to the end of an existing file without altering the existing content. Let’s get started with understanding the steps involved.
Step 1: Opening the File
To append text to a file, the first step is to open the file in append mode. Golang provides the `OpenFile` function to achieve this. Here’s an example:
“`
file, err := os.OpenFile(“example.txt”, os.O_WRONLY|os.O_APPEND|os.O_CREATE, 0644)
if err != nil {
log.Fatal(err)
}
defer file.Close()
“`
In the above code snippet, we use `OpenFile` to open a file called `example.txt` in write-only, append, and create mode if it doesn’t exist. The third argument, `0644`, sets the file permissions.
Step 2: Writing to the File
Once the file is opened, you can use the `WriteString` or `Write` method to append text to it. Let’s see an example of appending a simple string:
“`
text := “Hello, world!\n”
_, err = file.WriteString(text)
if err != nil {
log.Fatal(err)
}
“`
In the above code, we use the `WriteString` method to append the string “Hello, world!” to the file.
Step 3: Handling Errors
Error handling is essential to ensure the reliability of your application. Always check for errors after performing file operations. In the code snippets provided, we use the `log.Fatal(err)` function to terminate the program if an error occurs while opening the file or writing to it. However, in a real-world scenario, it’s recommended to handle errors more gracefully.
Step 4: Flushing the Buffer
After writing the content to the file, it’s a best practice to flush the buffer to ensure that the data is written immediately. We can achieve this by calling the `Sync` method:
“`
err = file.Sync()
if err != nil {
log.Fatal(err)
}
“`
Step 5: Closing the File
Lastly, it’s important to close the file properly to release system resources. We defer the `Close` method earlier, which ensures that it will be called automatically when the function returns:
“`
defer file.Close()
“`
Now that we have covered the basic steps to append text to a file in Golang, let’s address some frequently asked questions:
FAQs:
Q1: Can I append multiple lines of text at once?
Yes, you can append multiple lines of text in one go. Instead of using `WriteString` to append one line at a time, you can use `Write` and pass a byte slice containing your desired content.
Q2: Can I append text to a specific position within the file?
No, by default, appending text means adding it to the end of the file. If you want to insert text at a particular position, you would need to read the content, modify it, and then rewrite it to the file.
Q3: What happens if the file doesn’t exist?
If the file doesn’t exist, `OpenFile` with the `os.O_CREATE` flag will create a new file with the given name. However, if there are any errors related to permission or disk space, they will be returned.
Q4: How can I ensure that the file will only be opened in append mode?
The `os.OpenFile` function used in the examples above opens the file in append mode by specifying it in the second argument with the `os.O_APPEND` flag. By omitting the `os.O_TRUNC` flag, you prevent the file from being truncated and retain its existing content.
Conclusion:
Appending text to a file in Golang is a straightforward process that involves opening the file in append mode, writing the content, flushing the buffer, and closing the file. Remember to handle errors appropriately and release system resources by closing the file. By following these steps, you can effortlessly append text to a file in your Golang applications.
Images related to the topic golang write to file
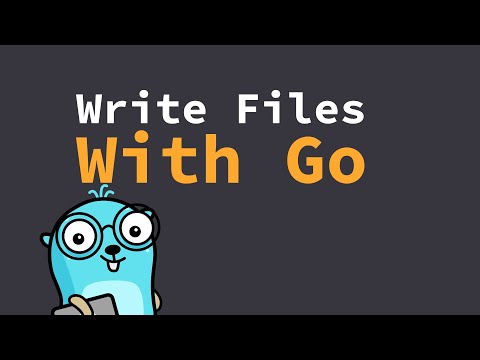
Found 5 images related to golang write to file theme
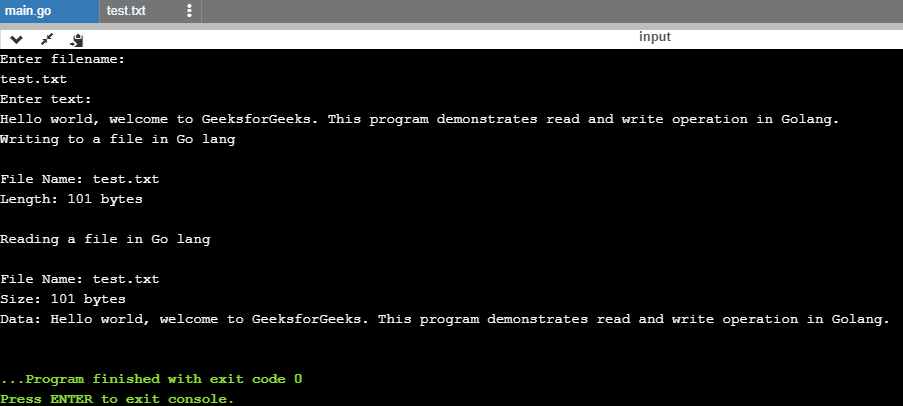
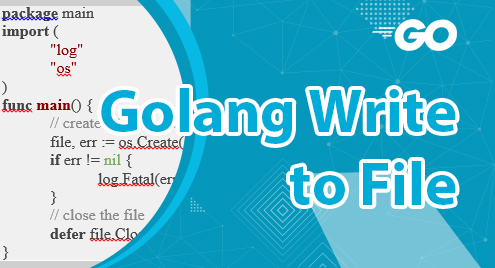

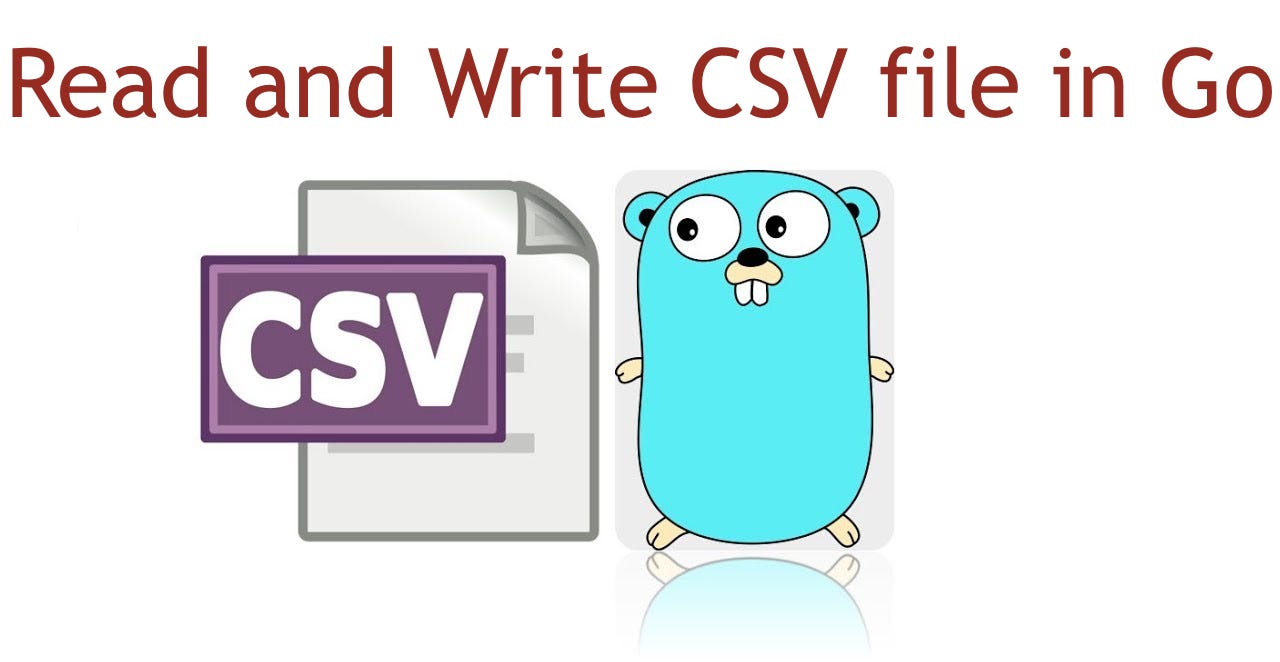
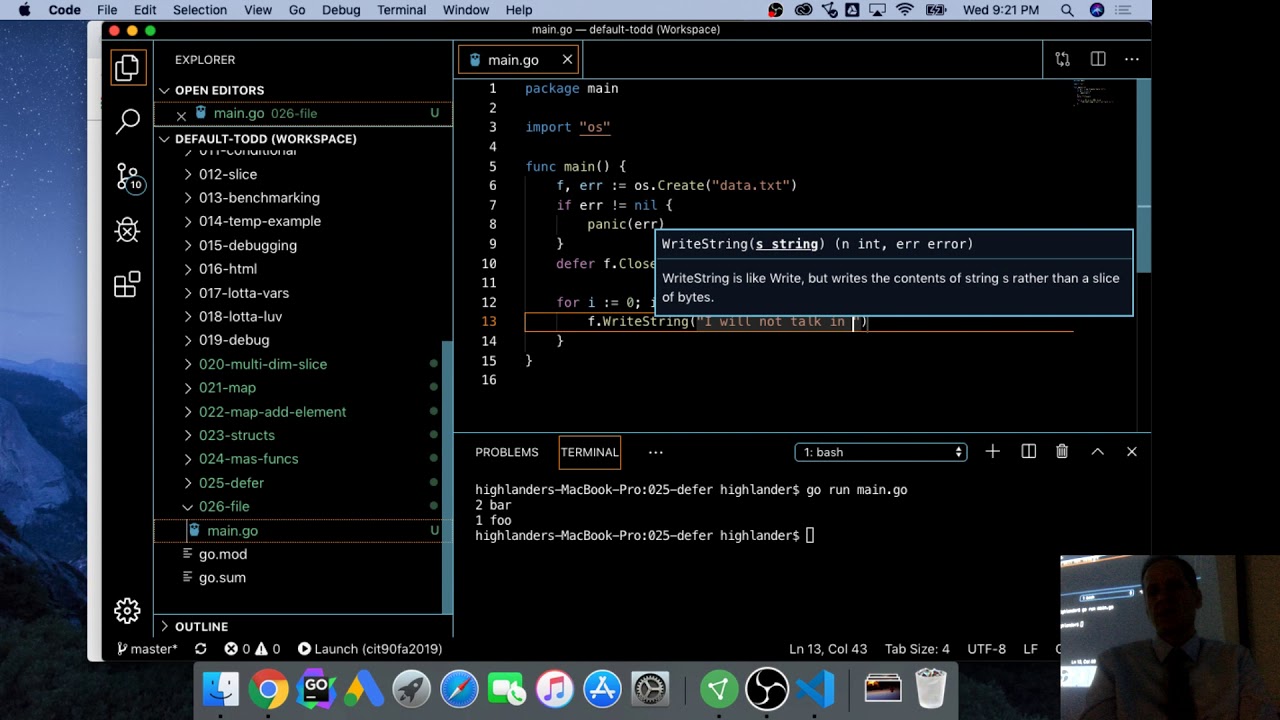
![How to write logs to file in Golang? [SOLVED] | GoLinuxCloud How To Write Logs To File In Golang? [Solved] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/syslog.jpg)
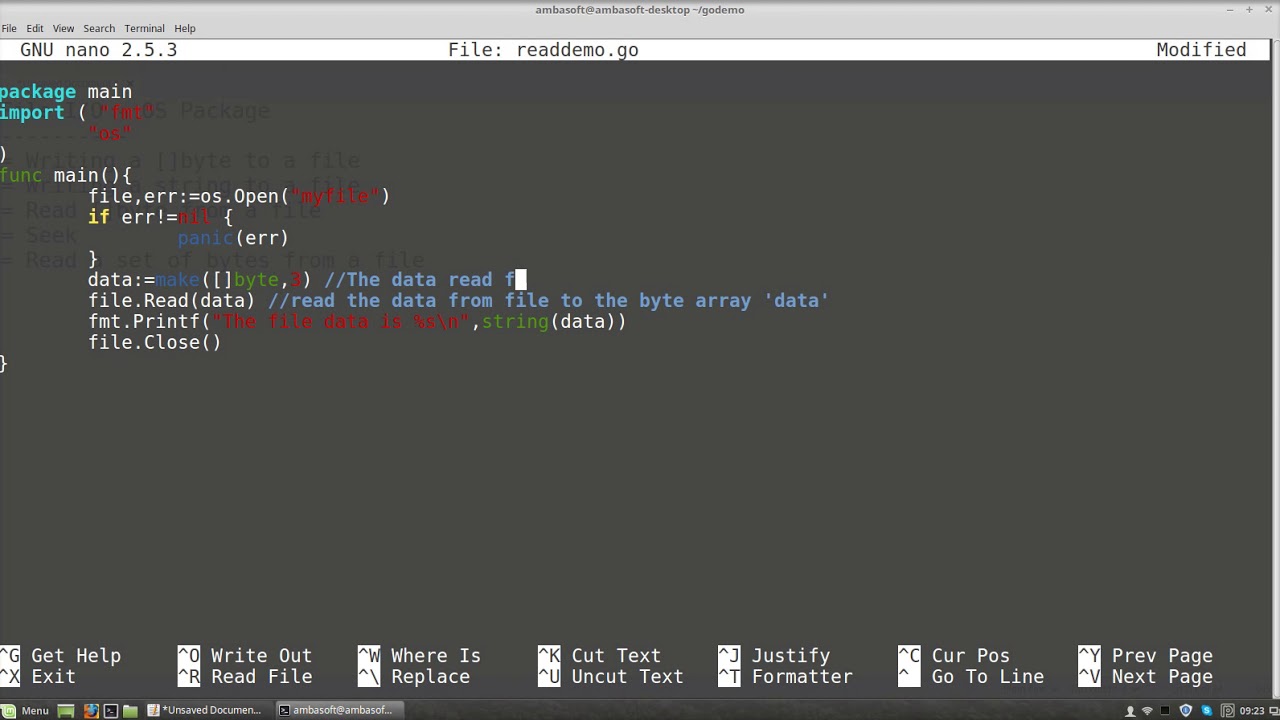
![How to write logs to file in Golang? [SOLVED] | GoLinuxCloud How To Write Logs To File In Golang? [Solved] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/syslog2.jpg)
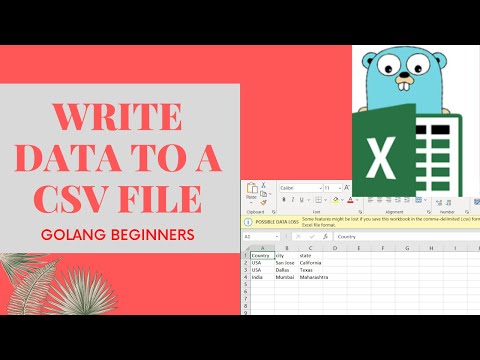
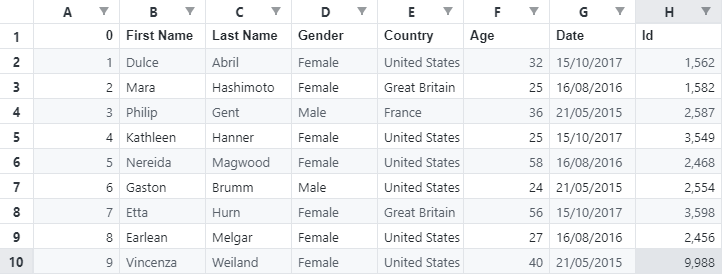

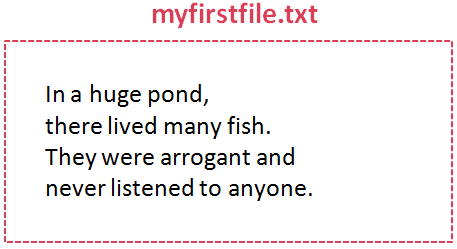
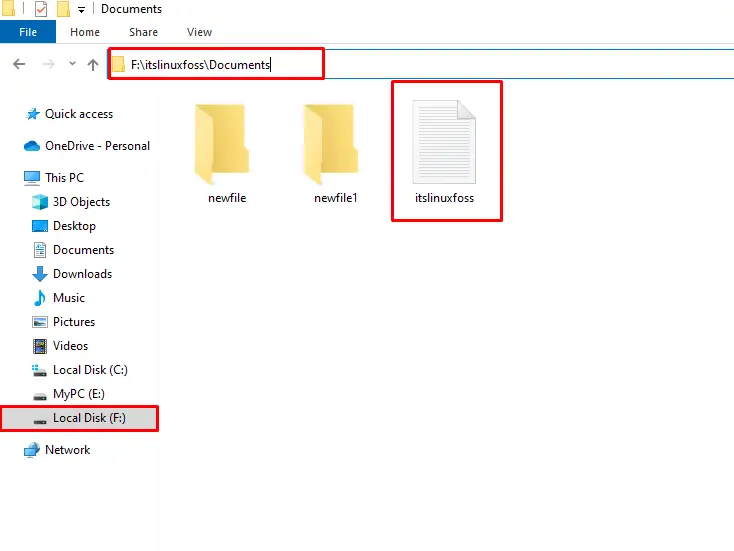
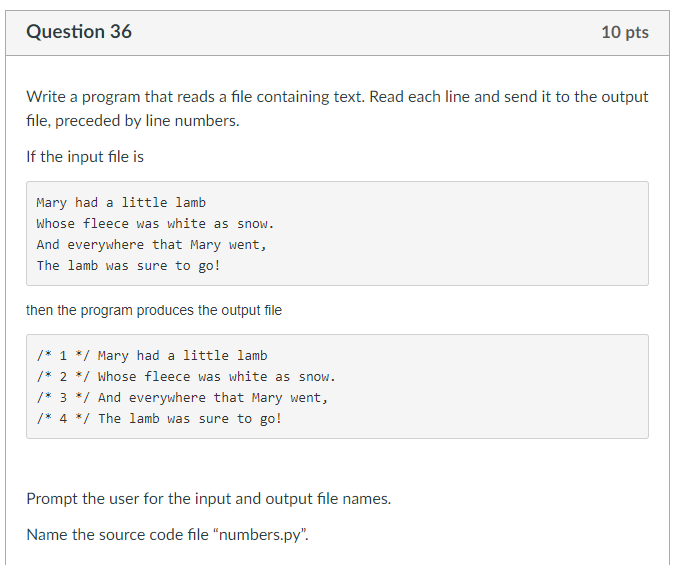
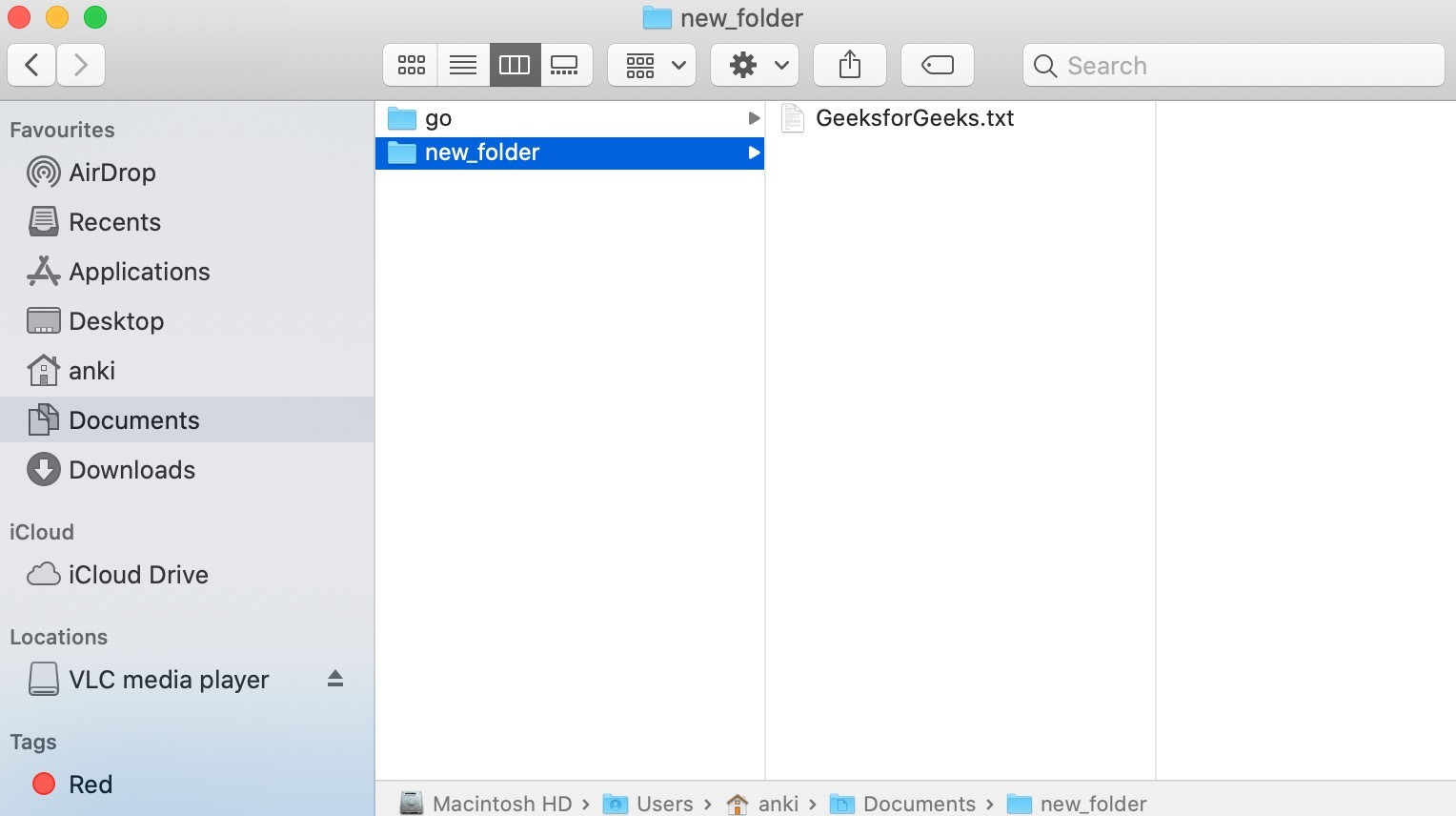

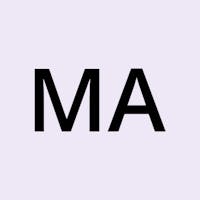


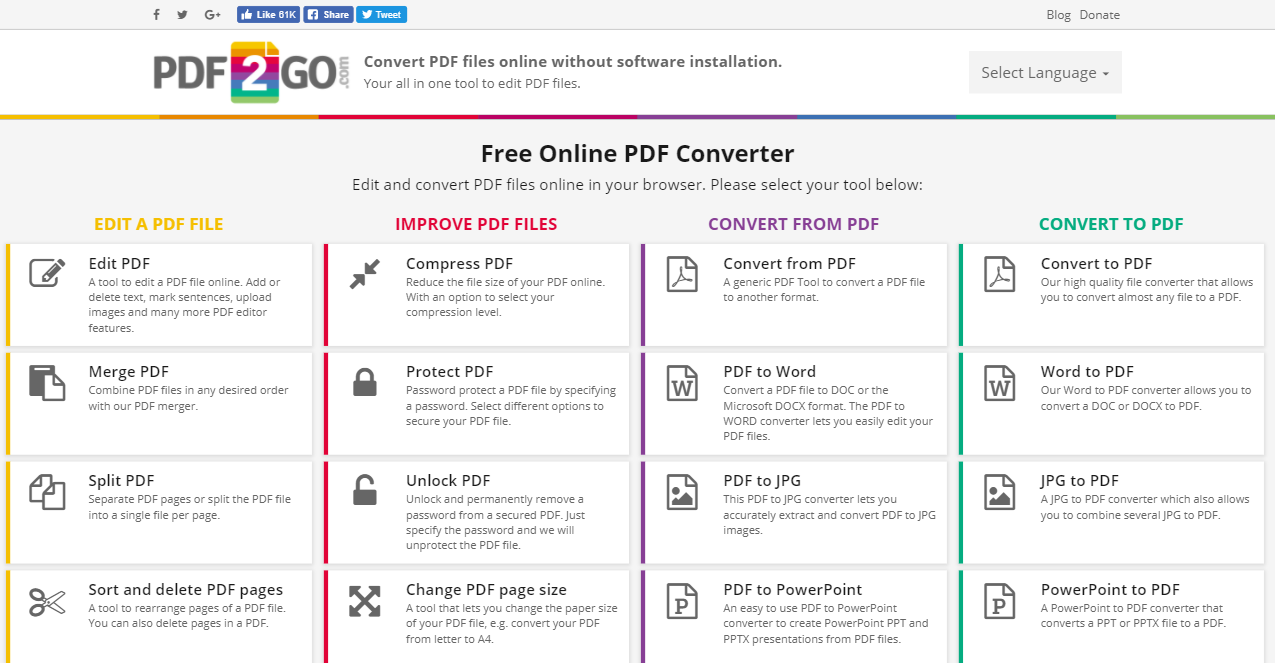

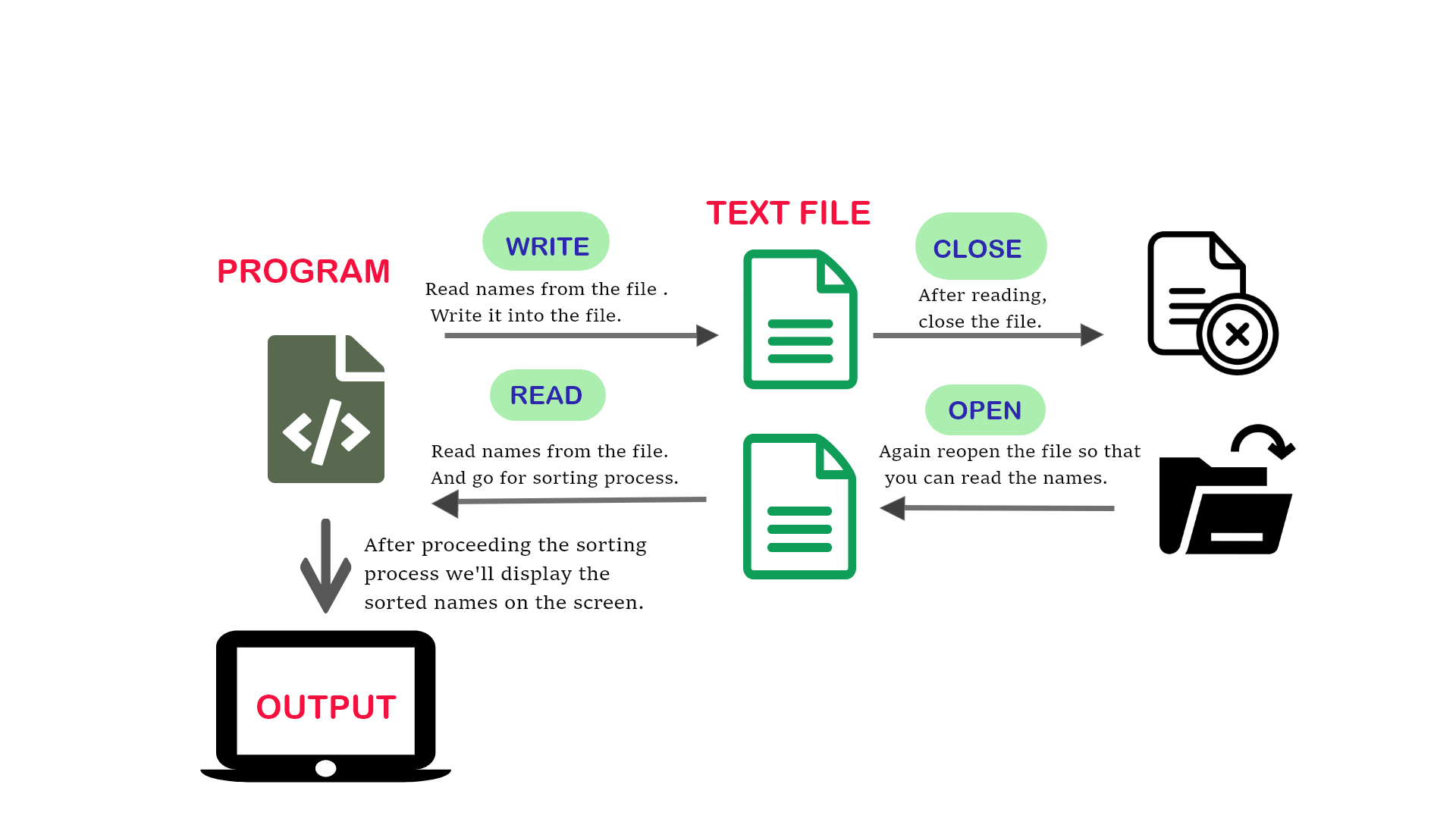

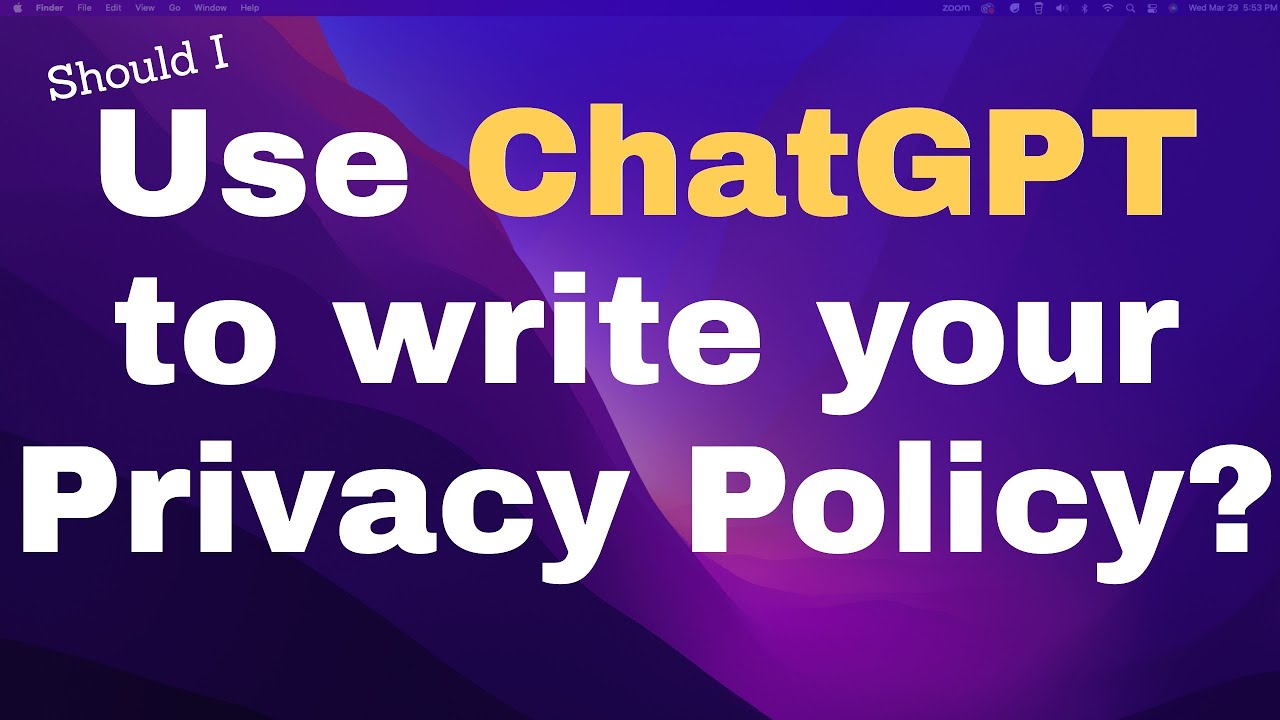

Article link: golang write to file.
Learn more about the topic golang write to file.
- Writing Files – Go by Example
- Go write file – writing files in Golang – ZetCode
- How to read/write from/to a file using Go – Stack Overflow
- Golang Write to File – Linux Hint
- Learn how to write a file in Golang | golangbot.com
- Read and write to files | golang-book – GitHub Pages
- Golang program to write into a file – Tutorialspoint
- How to Write a File in Golang – AppDividend
- Write to a file in Go (Golang) – GOSAMPLES
- Golang: File Write – Meet Gor
See more: nhanvietluanvan.com/luat-hoc