Golang Run Every 5 Minutes
Running code periodically is a common requirement in many applications. Whether it is for automation, job scheduling, or real-time data processing, the ability to execute code at regular intervals can greatly improve the efficiency and functionality of a program. In this article, we will explore the advantages of running Golang code periodically and discuss various methods to implement this functionality in your Go programs.
Advantages of Running Golang Code Periodically
1. Automation and Efficiency:
Automating repetitive tasks can save valuable time and resources. By running code periodically, you can automate tasks such as data backup, file processing, or database maintenance, freeing up your time for more important or complex tasks. This not only leads to increased efficiency but also reduces the risk of human errors associated with manual execution.
2. Job Scheduling:
Running code periodically is essential for scheduling tasks in an application. Whether you need to process incoming data, generate reports, send notifications, or perform other time-sensitive operations, the ability to schedule code execution at specific intervals ensures the smooth functioning of your application.
3. Real-time Data Processing:
In certain applications, real-time data processing is crucial. By running code every few minutes, you can continuously process and analyze incoming data streams, making informed decisions and taking appropriate actions promptly. This is particularly useful for applications like financial systems, monitoring systems, and real-time analytics platforms.
Implementing Golang Code to Run Every 5 Minutes
Now let’s dive into different methods you can use to implement periodic code execution in your Golang programs.
1. Using a Timer:
One way to run code every 5 minutes is by using the time package in Golang. You can start a timer with a duration of 5 minutes and execute the desired code when the timer expires. This method is simple and straightforward, but it may not be suitable for long-running tasks as it blocks the main Goroutine until the timer expires.
2. Utilizing Cron Jobs:
Cron jobs are a widely used method to schedule tasks in Unix-like operating systems. In Golang, you can utilize third-party libraries like “github.com/robfig/cron” to create and manage cron jobs. You can define a cron job that executes the desired code every 5 minutes, providing flexibility and control over your periodic tasks.
3. Deploying a Scheduled Task:
Another way to run code periodically is by deploying a scheduled task using external tools or services. For example, you can use Linux’s built-in task scheduler, cron, to execute your Golang program at regular intervals. Alternatively, you can leverage cloud-based services like AWS CloudWatch Events, Azure Scheduler, or Google Cloud Scheduler to schedule and run your code periodically.
Considerations for Continuous Code Execution
While implementing periodic code execution, it is important to consider a few aspects to ensure smooth and reliable operation.
1. Handling Errors and Failures:
Periodic code execution may encounter errors or failures due to various reasons such as network issues, resource unavailability, or unexpected conditions. It is crucial to handle these errors gracefully and implement appropriate error handling mechanisms to prevent the entire program from crashing or causing undesirable consequences.
2. Resource Management and Optimization:
Running code periodically can consume system resources, especially if the code involves heavy computations or I/O operations. It is vital to optimize resource usage and manage system resources efficiently to avoid performance issues or resource depletion. Utilize techniques like caching, efficient data structures, and concurrency to optimize the execution of your code.
3. Logging and Monitoring:
To ensure the successful execution and performance of your periodic tasks, it is recommended to implement logging and monitoring mechanisms. Proper logging helps in debugging and identifying issues, while monitoring tools can provide insights into the health and performance of your periodic code execution. Leverage popular logging libraries like “github.com/sirupsen/logrus” and monitoring tools like Prometheus or Grafana to gain visibility into the execution of your code.
FAQs
1. How can I run a Golang function periodically?
You can use a timer or utilize cron jobs to run a Golang function periodically. By defining the desired interval and executing the function when the timer expires or when the cron job triggers, you can achieve periodic code execution.
2. Can I run Golang code in parallel for periodic execution?
Yes, you can run Golang code in parallel for periodic execution. By utilizing Goroutines and concurrency patterns, you can execute the same or different functions concurrently at regular intervals.
3. How can I check if a Golang timer is running?
To check if a Golang timer is running, you can use the Timer’s “Stop” method. If the timer is running, calling “Stop” will stop the timer and return a boolean value indicating whether the timer was active or already expired.
4. What is the difference between Golang timer and ticker?
In Golang, a timer triggers the execution of a function once, after a specified duration, while a ticker repeatedly triggers the execution of a function at regular intervals. Depending on your requirements, you can choose either a timer or a ticker for periodic code execution.
In conclusion, running Golang code every 5 minutes can bring numerous advantages to your applications, including automation, efficient job scheduling, and real-time data processing. By using timers, cron jobs, or scheduled tasks, you can easily implement this functionality in your Golang programs. Just remember to handle errors, optimize resource usage, and implement logging and monitoring mechanisms for smooth and reliable execution.
Go Workspace \U0026 Runtime Explained In 5 Minutes
Keywords searched by users: golang run every 5 minutes golang run function periodically, Golang run parallel, golang check if timer is running, golang timer, golang timer loop, golang timer goroutine, golang schedule task, golang timer vs ticker
Categories: Top 11 Golang Run Every 5 Minutes
See more here: nhanvietluanvan.com
Golang Run Function Periodically
## Background
When it comes to scheduling periodic tasks in Go, there are several approaches available. The most straightforward method is to use the built-in time package, which provides convenient functions for managing time-based operations. One of the key components of this package is the *Ticker* type, which allows us to send time values at regular intervals by creating a ticker channel.
## Implementing Periodic Function Execution
Let’s take a closer look at how to implement periodic function execution in Go using the time package. First, we need to create a ticker by calling the `time.NewTicker()` function and passing the desired duration between function invocations as an argument. For example, if we want to execute a function every 5 seconds, we can create a ticker with `time.NewTicker(5 * time.Second)`.
Next, we need to create a goroutine that listens for ticker events and triggers the desired function. A goroutine is a concurrent lightweight thread of execution in Go that enables us to run multiple functions concurrently. We can start a goroutine by using the `go` keyword followed by the function call. In our case, the function call would be the desired task function that we want to execute periodically.
A typical implementation of periodic function execution using goroutines and tickers may look like this:
“`go
func main() {
ticker := time.NewTicker(5 * time.Second)
go func() {
for range ticker.C {
// Call your desired function here
// This function will run every 5 seconds
}
}()
// Keep the main goroutine alive
select {}
}
“`
In this example, we create a new ticker that emits time events every 5 seconds. Inside the goroutine, the function wrapped by the ticker’s channel (`ticker.C`) is executed whenever a tick is received. This allows us to run our desired function at the specified interval.
Lastly, we use a `select{}` statement outside the goroutine to prevent the main goroutine from exiting immediately. This ensures that the periodic execution continues until the program is terminated.
## FAQs
**Q: How precise is the periodic execution using tickers in Go?**
A: The precision of periodic function execution depends on various factors, including the underlying operating system and hardware. Go guarantees a minimum precision of 1 nanosecond, but in practice, the accuracy may vary slightly due to system limitations.
**Q: Can I change the interval of a running ticker?**
A: No, once a ticker is created, its interval cannot be changed. If you need to adjust the interval, you must stop the current ticker and create a new one with the desired interval.
**Q: What happens if the execution of a function takes longer than the ticker interval?**
A: The ticker does not stack tick events. If the execution of a function takes longer than the ticker interval, subsequent ticks will be dropped. It is important to keep the execution time of the function shorter than the interval to avoid scheduling conflicts.
**Q: How can I stop the periodic execution?**
A: To stop the periodic execution, you can call the `Stop()` method on the ticker. This will close the ticker channel and stop the emission of tick events.
**Q: Is it possible to pass arguments to the function being executed periodically?**
A: Absolutely! You can pass arguments to the function by using anonymous functions or closures. Simply create a new function that wraps the desired function and includes the necessary arguments, then call this wrapper function using the ticker’s channel.
## Conclusion
Periodic function execution is a common requirement in many software applications. Fortunately, Go provides a simple and efficient way to achieve this using tickers and goroutines. By creating a ticker with the desired interval and running a function inside a goroutine listening to ticker events, we can easily implement periodic execution in Go. Remember to consider the precision, execution duration, and other factors discussed above to ensure the reliability and effectiveness of your periodic function execution.
Golang Run Parallel
Introduction (approximately 80 words):
Parallelism is a crucial aspect of modern computing as it allows for the efficient utilization of available resources and enhanced program performance. In the world of programming languages, Golang, also known as Go, has emerged as a leading choice for developers seeking simplified yet powerful concurrent programming. In this article, we will delve into the concept of parallelism in Golang, exploring its benefits and practical implementation techniques.
Understanding Golang’s Concurrency and Parallelism (approximately 150 words):
Before diving into Golang’s parallel execution capabilities, it’s vital to understand the concepts of concurrency and parallelism. Concurrency refers to the ability of a program to handle multiple tasks simultaneously, whereas parallelism refers to the actual execution of these tasks simultaneously. Golang distinguishes itself by making concurrency a primary feature of the language, allowing developers to write concurrent programs with ease.
Goroutines: Lightweight Concurrent Units (approximately 200 words):
Goroutines are a fundamental construct in Golang that enables concurrent execution of functions or methods. Unlike traditional threads, which tend to be relatively heavy, Goroutines are lightweight and consume fewer resources. Developers can spin up thousands of Goroutines within the same address space without incurring significant overhead. Goroutines have automatic scheduling, allowing them to be efficiently executed in parallel without requiring manual management.
Concurrency via Channels (approximately 200 words):
Channels represent a key synchronization component in Golang that facilitates communication and data sharing between Goroutines. Acting as typed conduits, channels enable Goroutines to exchange data safely and synchronize their execution. By using channels, developers can orchestrate communication patterns and enforce data integrity. Furthermore, Golang offers various channel operations like blocking, unbuffered channels, and buffered channels, providing flexibility in handling concurrent tasks.
Parallel Execution with the ‘sync’ Package (approximately 150 words):
While Goroutines and channels lay the foundation for concurrent execution, Golang’s ‘sync’ package takes parallelism to the next level. This package provides synchronization primitives like mutexes, wait groups, and conditions, allowing developers to coordinate and control the execution of concurrent tasks. By leveraging these primitives, developers can effectively manage shared resources, synchronize Goroutine execution, and develop complex parallel algorithms.
Frequently Asked Questions: (approximately 145 words)
Q1. Is Golang suitable for all types of software development?
A1. Golang is well-suited for various domains, including web development, cloud-based applications, distributed systems, or even low-level systems programming.
Q2. What advantages does Golang’s concurrency model offer?
A2. Golang’s concurrency model provides high-level abstractions like Goroutines and channels, eliminating the complexities associated with traditional threading and locking mechanisms. This simplifies development, enhances code readability, and offers efficient use of system resources.
Q3. Can Golang applications efficiently utilize multicore processors?
A3. Yes, Golang inherently supports parallelism, making it ideal for exploiting the capabilities of modern multicore processors. It allows developers to distribute workloads effectively and leverage concurrent and parallel execution.
Q4. Are there any downsides to using Golang’s parallelism features?
A4. While Golang offers powerful tools for parallel execution, inexperienced developers may struggle with managing synchronization and avoiding race conditions. However, thorough understanding of Golang’s concurrency primitives can significantly mitigate these challenges.
Conclusion (approximately 100 words):
Golang’s parallelism capabilities have considerably simplified the development of concurrent software, providing high-level constructs like Goroutines and channels. By utilizing these features alongside the ‘sync’ package, developers can effortlessly harness parallel execution, leading to improved program performance and resource utilization. Whether developing web applications, cloud-based services, or low-level system programs, Golang empowers developers to build software that effectively harnesses the full potential of modern computing architectures.
Images related to the topic golang run every 5 minutes
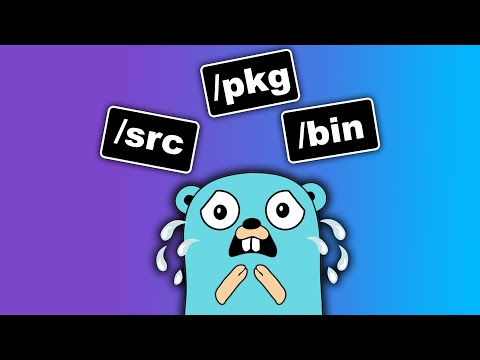
Found 32 images related to golang run every 5 minutes theme




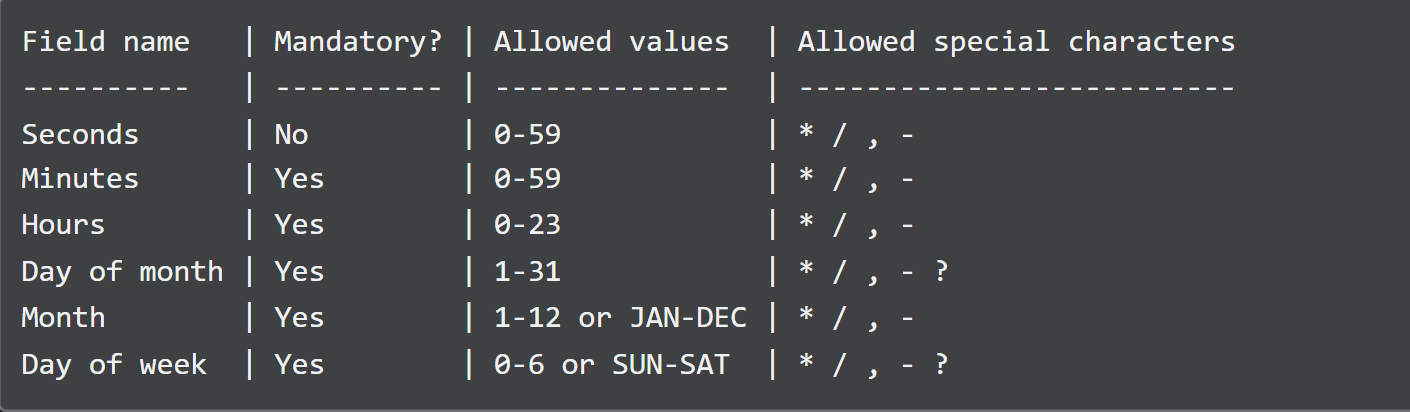
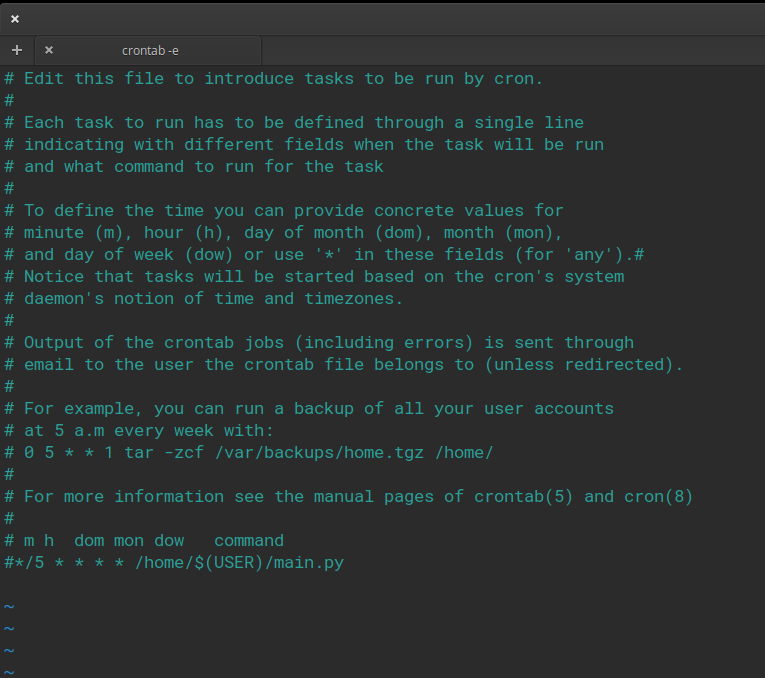
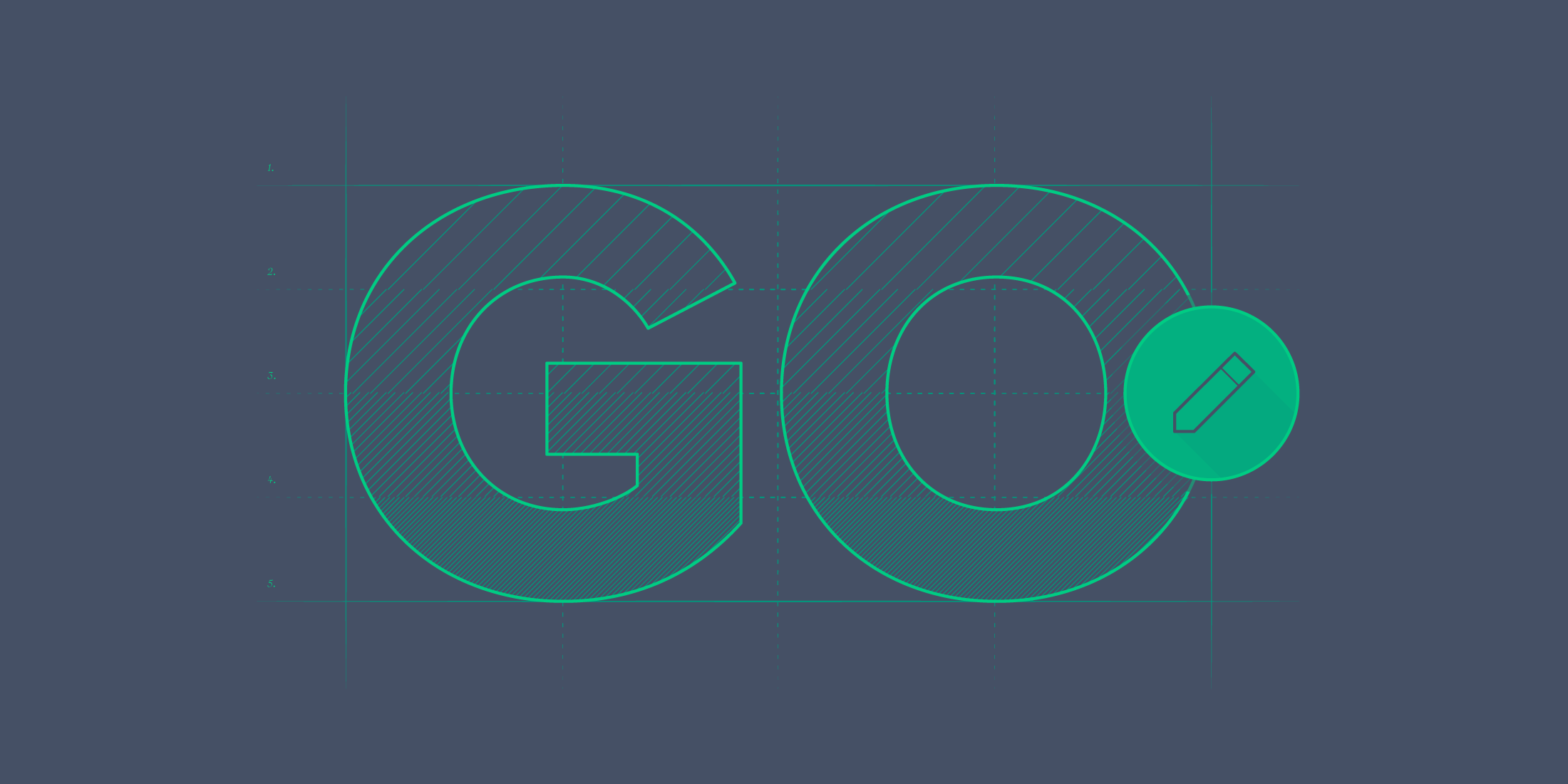

![How to Run Go[Golang] on Visual Studio Code - YouTube How To Run Go[Golang] On Visual Studio Code - Youtube](https://i.ytimg.com/vi/fbyobdxDQno/mqdefault.jpg)
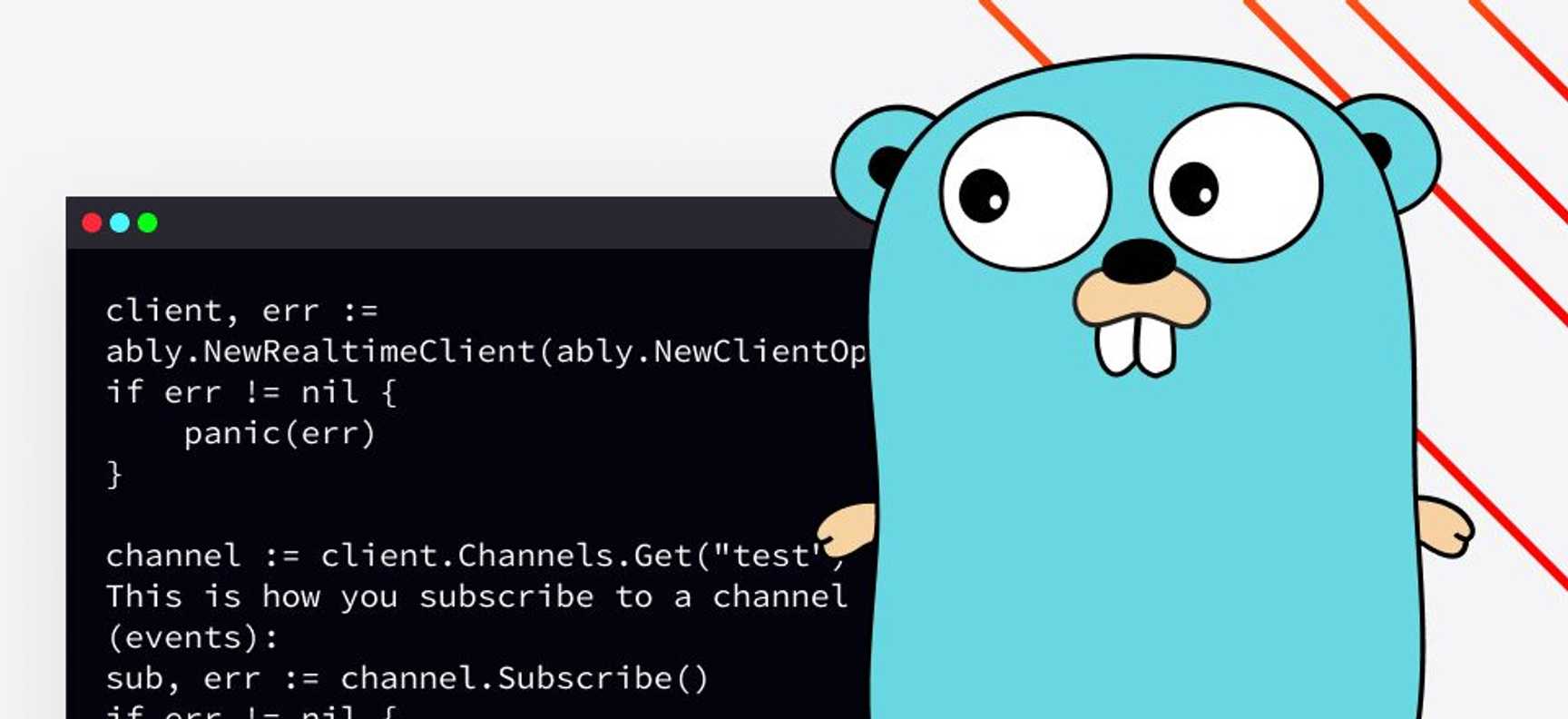
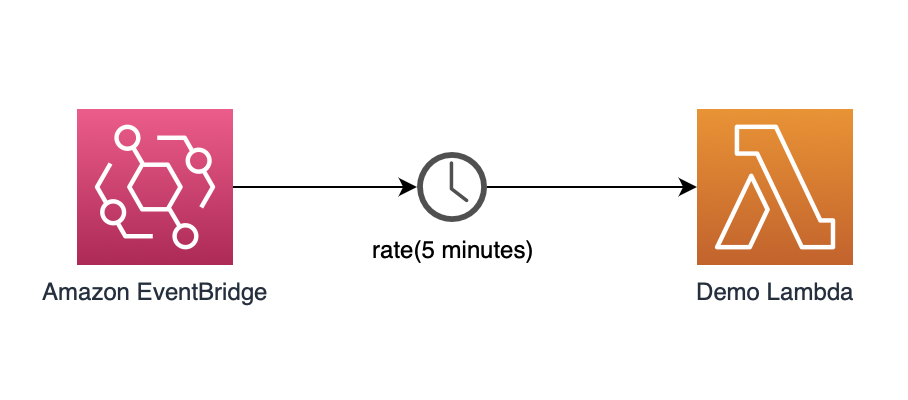
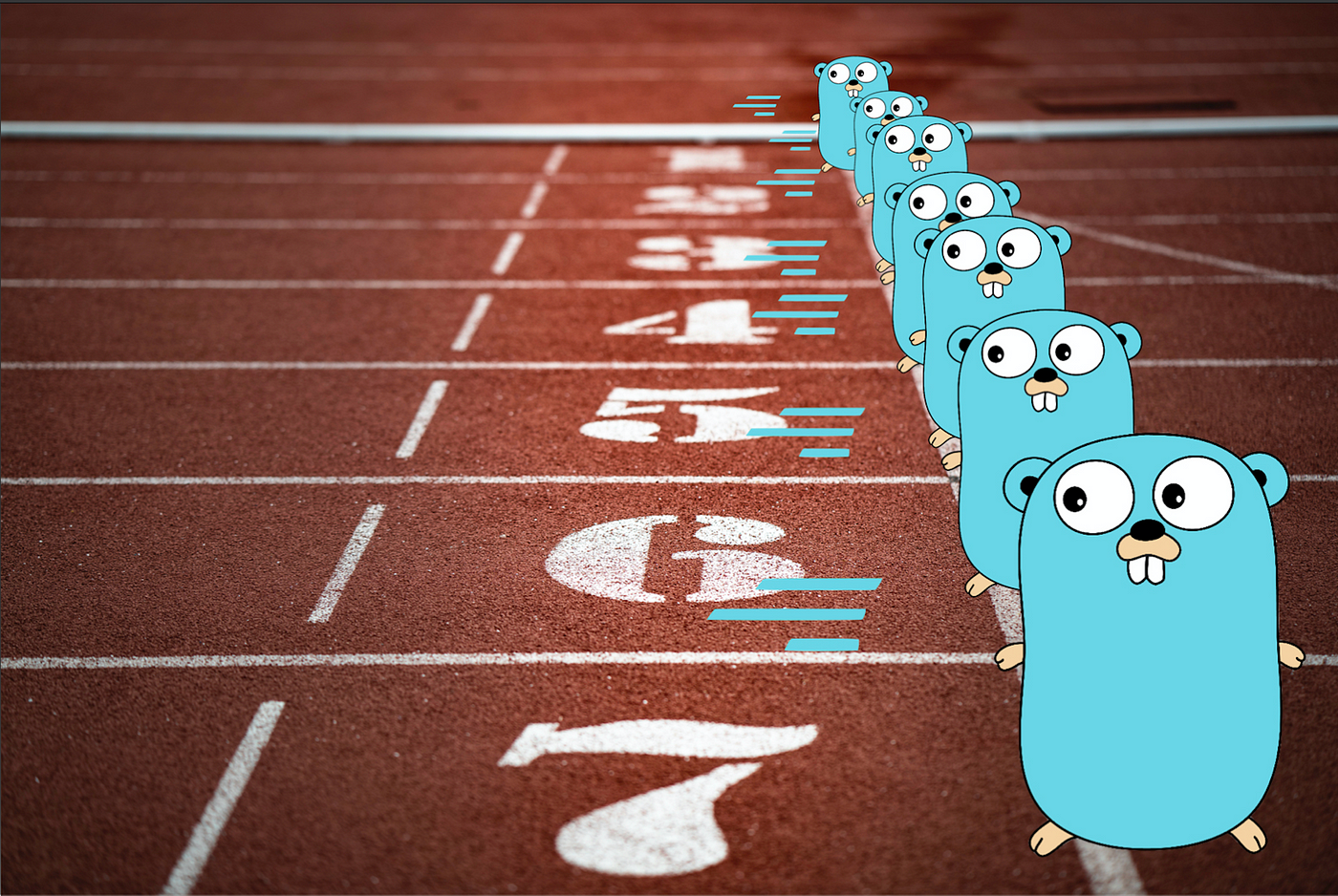
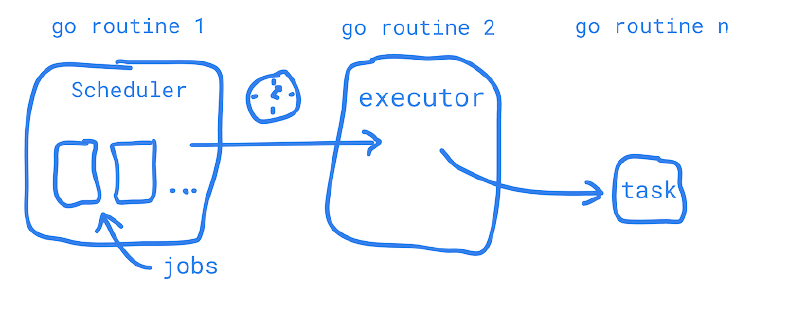
Article link: golang run every 5 minutes.
Learn more about the topic golang run every 5 minutes.
- How to do something every 5 minutes in Go? : r/golang – Reddit
- go – Is there a way to do repetitive tasks at intervals?
- How to do repetitive tasks at intervals GO? [SOLVED]
- Running background task every head of hour – Go Forum
- Timer and Ticker Using Golang – Level Up Coding
- gocron – Go Packages
- Cron job every 5 minutes – Crontab.guru
- How to Schedule Tasks using Chrono in Golang – ITNEXT
See more: nhanvietluanvan.com/luat-hoc