Golang Read File Line By Line
Reading a File in Go:
Before we dive into reading a file line by line in Go, we need to open the file and specify the file mode for reading. This can be achieved using the `os` package. Here’s an example:
“`
func readFile(filepath string) error {
file, err := os.Open(filepath)
if err != nil {
return err
}
defer file.Close()
// File reading logic goes here
return nil
}
“`
By utilizing the `os.Open()` function, we can open the file at the specified `filepath`. If no error occurs, the file is opened successfully. However, it is important to handle any potential errors that may occur during the file opening process.
Checking for End of File:
Once the file is opened, we need to check for the end of the file condition to avoid reading beyond its contents. In Go, we can utilize `io.EOF` error to check for this. Here’s an example of how to implement a loop to read the file until the end is reached:
“`
scanner := bufio.NewScanner(file)
for scanner.Scan() {
// Line reading logic goes here
}
if err := scanner.Err(); err != nil {
return err
}
“`
By creating a new scanner using the `bufio.NewScanner()` function, we can read the file line by line. The loop will continue until `scanner.Scan()` returns false, indicating the end of the file. It is crucial to handle any errors that may occur during the file reading process.
Reading the File Line by Line:
To read the file line by line, we need to loop through each line using the scanner. Here’s an example of how to achieve this:
“`
scanner := bufio.NewScanner(file)
for scanner.Scan() {
line := scanner.Text()
// Line processing logic goes here
}
“`
By utilizing `scanner.Text()`, we can access the content of each line read by the scanner. It is important to note that the trailing newline character is not included in the returned line. This allows us to process the data from each line separately.
Processing Each Line:
Once we have access to each line’s content, we can implement the necessary logic to process the data. This may include parsing, filtering, or performing any required operations or manipulations on the line data. Here’s an example of how to process each line:
“`
scanner := bufio.NewScanner(file)
for scanner.Scan() {
line := scanner.Text()
// Line processing logic goes here
// Process the line data
}
“`
By adding code within the loop, we can perform any required operations on the line data. This may involve splitting the line into different fields, converting values, or storing the data for further processing.
Handling Errors:
During the line processing phase, it is crucial to check for any error occurrences. This can be achieved by checking the error returned by `scanner.Err()`. Here’s an example of how to handle errors:
“`
scanner := bufio.NewScanner(file)
for scanner.Scan() {
line := scanner.Text()
// Line processing logic goes here
if err := scanner.Err(); err != nil {
return err
}
}
“`
By including the error check within the loop, we can detect any errors and gracefully handle them based on the specific requirements. It is important to understand the potential errors that can occur during file reading, such as file not found, permission denied, or corrupt file format.
Closing the File:
To ensure proper file handling and resource management, it is important to close the opened file once we have finished reading it. This can be achieved using the `defer` keyword and the file’s `Close()` method. Here’s an example:
“`
func readFile(filepath string) error {
file, err := os.Open(filepath)
if err != nil {
return err
}
defer file.Close()
// File reading logic goes here
return nil
}
“`
By using `defer`, the `file.Close()` method will be called automatically when the function returns, even if an error occurs or an early return statement is encountered. This ensures that the file is closed properly, freeing up system resources.
Error Handling Best Practices:
When working with files in Go, it is important to implement robust error handling techniques. This includes using proper logging and reporting mechanisms for errors encountered during the file reading process. Additionally, it is crucial to handle panics, unexpected errors, and implement graceful error recovery mechanisms.
FAQs:
1. How can I read a file into a string in Go?
To read a file into a string in Go, you can use the `ioutil` package. Here’s an example:
“`
func readFileToString(filepath string) (string, error) {
data, err := ioutil.ReadFile(filepath)
if err != nil {
return “”, err
}
return string(data), nil
}
“`
By utilizing `ioutil.ReadFile()`, we can read the entire contents of the file into a byte slice. Then, by converting the byte slice to a string, we can obtain the file content as a string.
2. How can I read the first line of a file in Go?
To read the first line of a file in Go, you can use the same file reading approach mentioned earlier, but exit the loop after reading the first line. Here’s an example:
“`
scanner := bufio.NewScanner(file)
if scanner.Scan() {
line := scanner.Text()
// Process the first line
}
“`
By calling `scanner.Scan()` once before the loop, we ensure that only the first line is read.
3. How can I write a line to a file in Go?
To write a line to a file in Go, you can utilize the `File` type’s `WriteString()` method. Here’s an example:
“`
func writeLine(filepath string, line string) error {
file, err := os.OpenFile(filepath, os.O_APPEND|os.O_WRONLY, 0644)
if err != nil {
return err
}
defer file.Close()
_, err = file.WriteString(line + “\n”)
if err != nil {
return err
}
return nil
}
“`
By opening the file in append mode using `os.O_APPEND` and `os.O_WRONLY` flags, we can write the line to the end of the file. Additionally, we append the newline character `\n` to the line to ensure it is placed on a new line.
4. How can I append to a file in Go?
To append to a file in Go, you can use the `File` type’s `WriteString()` method in a similar manner to writing a line. Here’s an example:
“`
func appendToFile(filepath string, content string) error {
file, err := os.OpenFile(filepath, os.O_APPEND|os.O_WRONLY, 0644)
if err != nil {
return err
}
defer file.Close()
_, err = file.WriteString(content)
if err != nil {
return err
}
return nil
}
“`
By opening the file in append mode and using `WriteString()`, we can append the content to the end of the file without overwriting the existing data.
5. How can I create an `io.Reader` from a file in Go?
To create an `io.Reader` from a file in Go, you can use the `os.Open()` function combined with `bufio.NewReader()`. Here’s an example:
“`
file, err := os.Open(filepath)
if err != nil {
return err
}
defer file.Close()
reader := bufio.NewReader(file)
“`
By passing the opened file to `bufio.NewReader()`, we can create an `io.Reader` that can be used to read from the file.
In conclusion, reading a file line by line in Go can be achieved by utilizing the `os`, `bufio`, and `io/ioutil` packages. By following the steps outlined in this article and implementing best practices for error handling, you can efficiently process the contents of a file in a Go program.
Learn To Read Files With Go!
Keywords searched by users: golang read file line by line Golang read file line by line, Golang read file to string, Read text file Golang, Golang read first line of file, Write line golang, Append file golang, Io reader file golang, Open file Golang
Categories: Top 33 Golang Read File Line By Line
See more here: nhanvietluanvan.com
Golang Read File Line By Line
Reading a file line by line is a common requirement in various applications, such as log analysis, data extraction, and parsing. Golang provides a straightforward approach to accomplish this task, with built-in packages and functions that simplify the process.
Before diving into the code, it is important to understand the main steps involved in reading a file line by line:
1. Opening the File: The first step is to open the file we want to read. Golang provides the `os` package which offers a function called `Open` that allows us to open a file by specifying its path. The `Open` function returns a `*File` pointer and an `error` value.
2. Scanning the File: Once the file is opened, we can use the `bufio` package to create a new scanner that reads from the opened file. The scanner provides an easy way to read the file line by line, providing a simple and efficient solution.
3. Reading Line by Line: With the scanner created, we can use its `Scan` method to read each line of the file. After each call to `Scan`, the scanner moves to the next line automatically. To access the content of the line, we can use the `Text` method of the scanner, which returns the current line as a `string`.
4. Closing the File: Once we have finished reading the file, it is good practice to close it using the `Close` method of the file pointer obtained in the first step. This step ensures that system resources are properly released.
Now, let’s dive into the implementation of these steps with some example code:
“`go
package main
import (
“bufio”
“fmt”
“log”
“os”
)
func main() {
// Step 1: Opening the file
file, err := os.Open(“myFile.txt”)
if err != nil {
log.Fatal(err)
}
defer file.Close()
// Step 2: Creating a scanner
scanner := bufio.NewScanner(file)
// Step 3: Reading line by line
for scanner.Scan() {
line := scanner.Text()
fmt.Println(line)
}
// Step 4: Closing the file
err = scanner.Err()
if err != nil {
log.Fatal(err)
}
}
“`
In the code snippet above, we first open the file “myFile.txt” using the `os.Open` function. We handle any error that may occur during the process using the `log.Fatal` function, which terminates the program in case of an error.
Next, we create a scanner using the `bufio.NewScanner` function, passing in the opened file. The scanner provides a convenient way to iterate over the lines of the file in a loop.
Inside the loop, we call `scanner.Scan()` to advance to the next line. If there is a line available, we retrieve its content using `scanner.Text()`. In this example, we simply print the line, but you can process it in any desired way based on the requirements of your application.
Finally, we close the file and handle any potential errors that occurred during the scanning process using `scanner.Err()`. By following these steps, you can effectively read a file line by line in Golang.
Now, let’s address some frequently asked questions regarding file reading in Golang:
**Q1: How can I read a large file without consuming excessive memory?**
Reading a large file line by line can be memory-intensive if all lines are loaded into memory at once. Golang’s `bufio.Scanner` offers a memory-friendly solution, as it reads and processes each line sequentially without loading the entire file into memory.
**Q2: Can I specify the file encoding in Golang when reading?**
By default, Golang assumes that files are encoded in UTF-8. However, if your file is encoded in a different format, you can use the `golang.org/x/text/encoding` package to handle different encodings when reading the file.
**Q3: How can I optimize file reading for performance?**
Golang’s `bufio.Scanner` is already an efficient option. To further optimize file reading, you can adjust the buffer size used by the scanner. By default, the buffer size is 64 KB, but you can set a custom size using `scanner.Buffer([]byte, bufferSize)` method, where `bufferSize` is the desired size in bytes.
**Q4: What should I do if an error occurs while reading the file?**
You can use the `scanner.Err()` method to check for errors while scanning the file. If an error occurs, you can handle it accordingly. In the example code above, we use `log.Fatal` to terminate the program if any error occurs during the file reading process.
In conclusion, Golang provides a simple, efficient, and convenient way to read files line by line. By using the `os`, `bufio`, and `log` packages, developers can easily open, scan, read, and close files in their applications. Understanding the steps involved in file reading and addressing potential concerns will help you handle this common task effectively and with ease.
Golang Read File To String
Introduction:
The Go programming language, also known as Golang, has gained significant popularity among developers due to its simplicity, efficiency, and strong support for concurrency. In this article, we will explore one of the common tasks in programming – reading a file into a string using Golang. We will cover various techniques and concepts associated with this task, providing a comprehensive guide for developers.
Reading a File in Golang:
Before we dive into the techniques, it’s important to understand the basic process of reading a file in Golang. The built-in `os` package in Golang provides functions and types that allow us to interact with the operating system, including file operations.
To read a file in Golang, we need to follow a series of steps:
1. Open the file: The `os.Open()` function is used to open a file. It takes the file path as a parameter and returns a `*os.File` object and an error. It’s crucial to handle the error properly to ensure smooth execution of the program.
2. Read the file: Once the file is opened, we can read its contents. Golang provides several approaches to read the file, depending on the requirements. We will discuss these in detail later in this article.
3. Close the file: After reading the file, it’s important to close it using the `Close()` method on the `*os.File` object. This step is crucial to release resources and avoid potential issues.
Techniques for Reading File to String:
Now that we understand the basic steps involved in reading a file, let’s explore some techniques to convert the file contents into a string. We will cover two common approaches – using the `bufio` package and the `ioutil` package.
1. bufio Package:
The `bufio` package contains various buffered I/O operations that offer efficient ways to read files in Golang. To read a file to a string using this package, we can follow these steps:
a. Open the file: Use the `os.Open()` function to open the file as mentioned earlier.
b. Create a `Scanner` object: The `Scanner` type in the `bufio` package provides convenient methods for reading various types of data, including strings. Create a `Scanner` object using the `bufio.NewScanner()` function and pass the opened file as its parameter.
c. Read the file line by line: Use the `Scanner` object’s `Scan()` method in a loop to read the file contents line by line. Append each line to a string variable.
d. Close the file: Close the file using the `Close()` method on the `*os.File` object.
2. ioutil Package:
The `ioutil` package is another powerful option for reading files in Golang. It provides utility functions that simplify common I/O tasks. Here’s how we can read a file to string using the `ioutil` package:
a. Open the file: Use the `os.Open()` function to open the file, just like in the previous approach.
b. Read the file contents: The `ioutil` package’s `ReadFile()` function takes a file path as a parameter and returns the file’s contents as a `[]byte` slice and an error. We can use type conversion to convert the `[]byte` slice to a string.
c. Close the file: Close the file using the `Close()` method on the `*os.File` object.
FAQs:
Q1. What is the advantage of using bufio or ioutil package over manual file reading in Golang?
A. The `bufio` and `ioutil` packages provide higher-level abstractions and utility functions that simplify file reading operations. They handle many low-level details, such as buffering and error handling, allowing developers to focus on the core logic.
Q2. How does Golang handle file closing automatically?
A. Golang uses the concept of garbage collection. When a file is opened in Golang, the associated `*os.File` object is allocated memory. Once the file is closed, and there are no references to the object, the garbage collector frees the memory automatically.
Q3. What are the performance considerations when reading large files in Golang?
A. When reading large files, it’s usually more efficient to use `bufio` or `ioutil` techniques that read the file incrementally rather than loading the entire file into memory at once. This helps avoid potential memory exhaustion.
Q4. Can Golang handle file encoding when reading files to strings?
A. Golang doesn’t handle file encoding automatically when reading files. It treats the file contents as a byte stream. If your file has a specific encoding, you need to handle the encoding explicitly while converting the `[]byte` to a string.
Conclusion:
In this comprehensive guide, we explored the different techniques for reading a file into a string using Golang. We covered the basic process of reading a file, along with detailed explanations and examples of using the `bufio` and `ioutil` packages. Additionally, we addressed some frequently asked questions related to file reading in Golang. Armed with this knowledge, you can confidently handle file reading tasks in your Go programs, efficiently and effectively.
Images related to the topic golang read file line by line
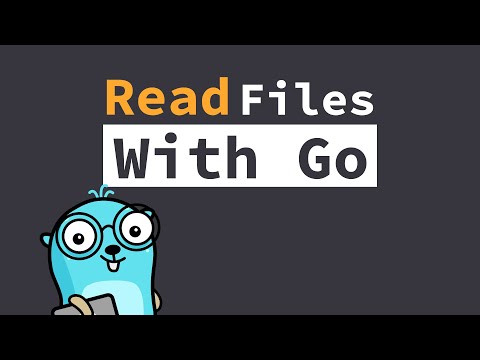
Found 22 images related to golang read file line by line theme
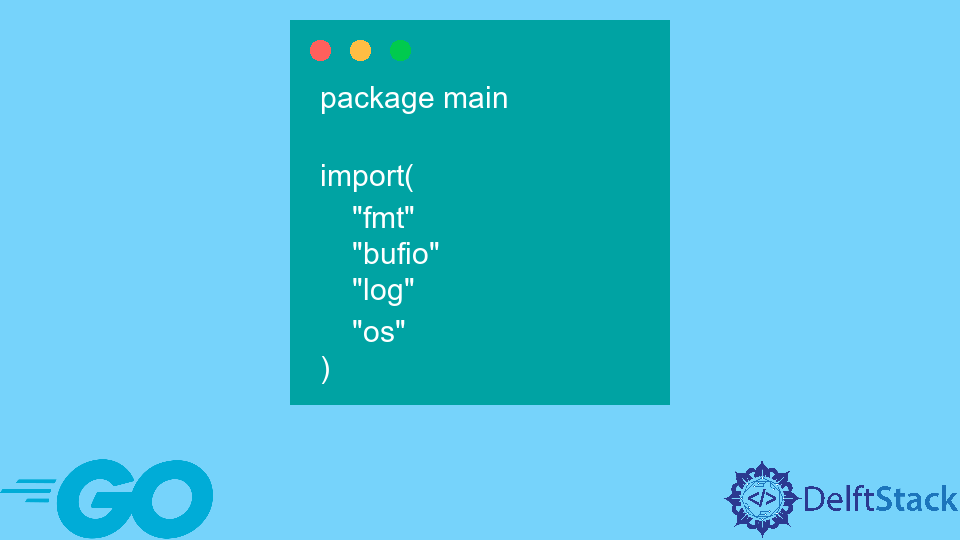
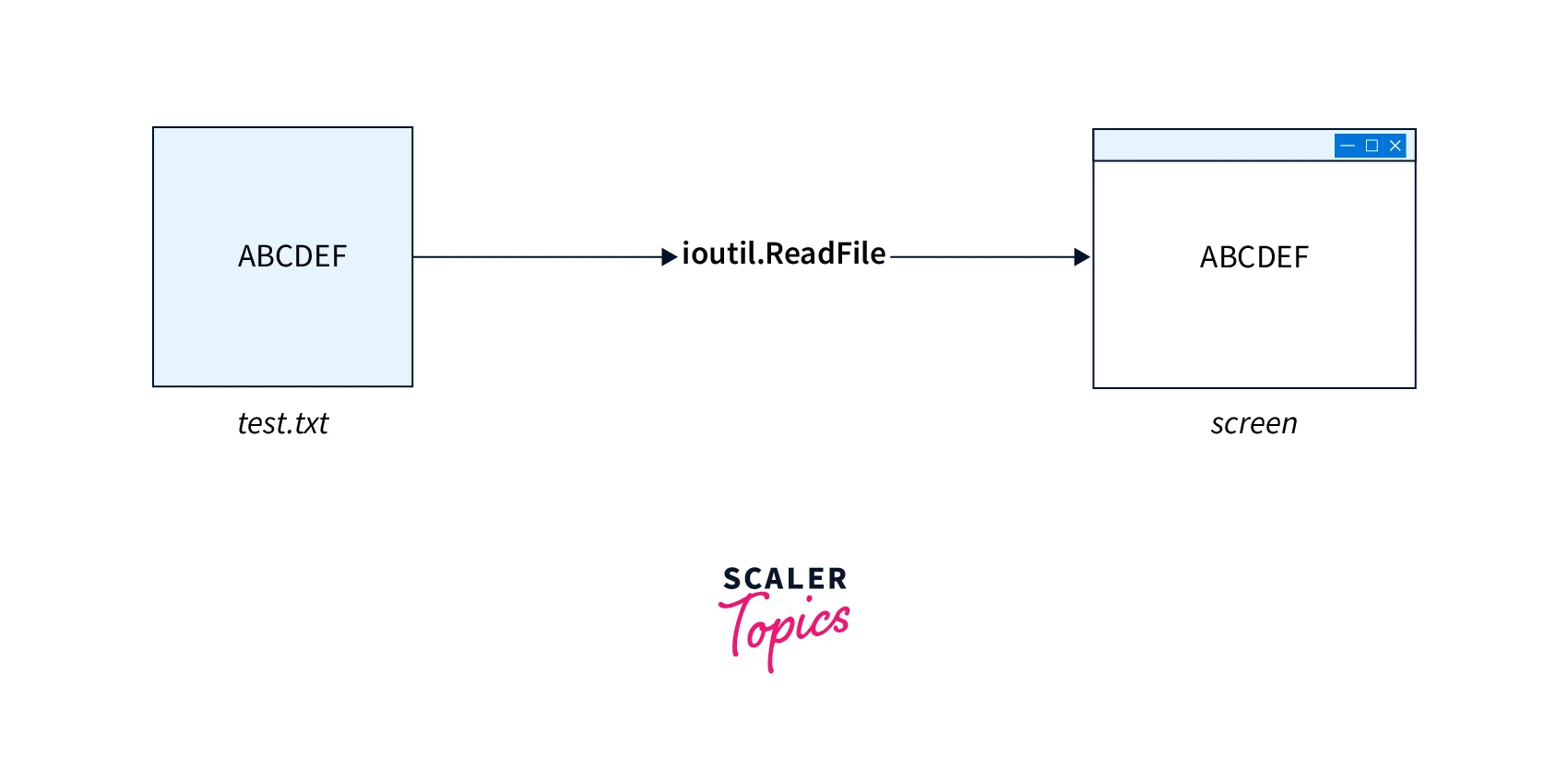
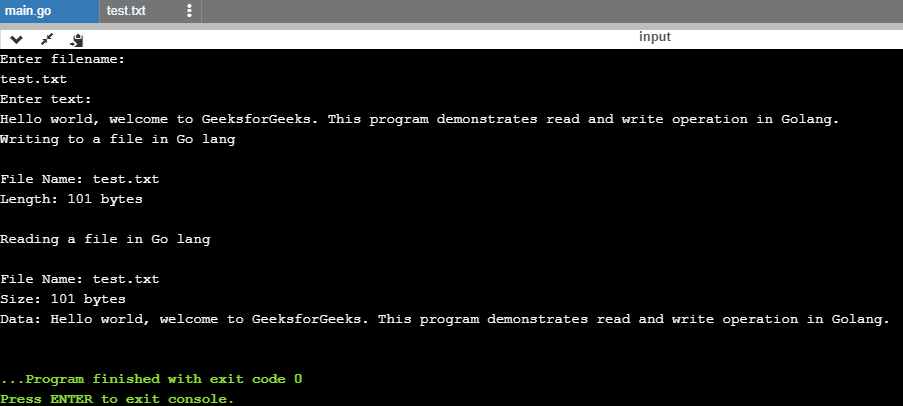
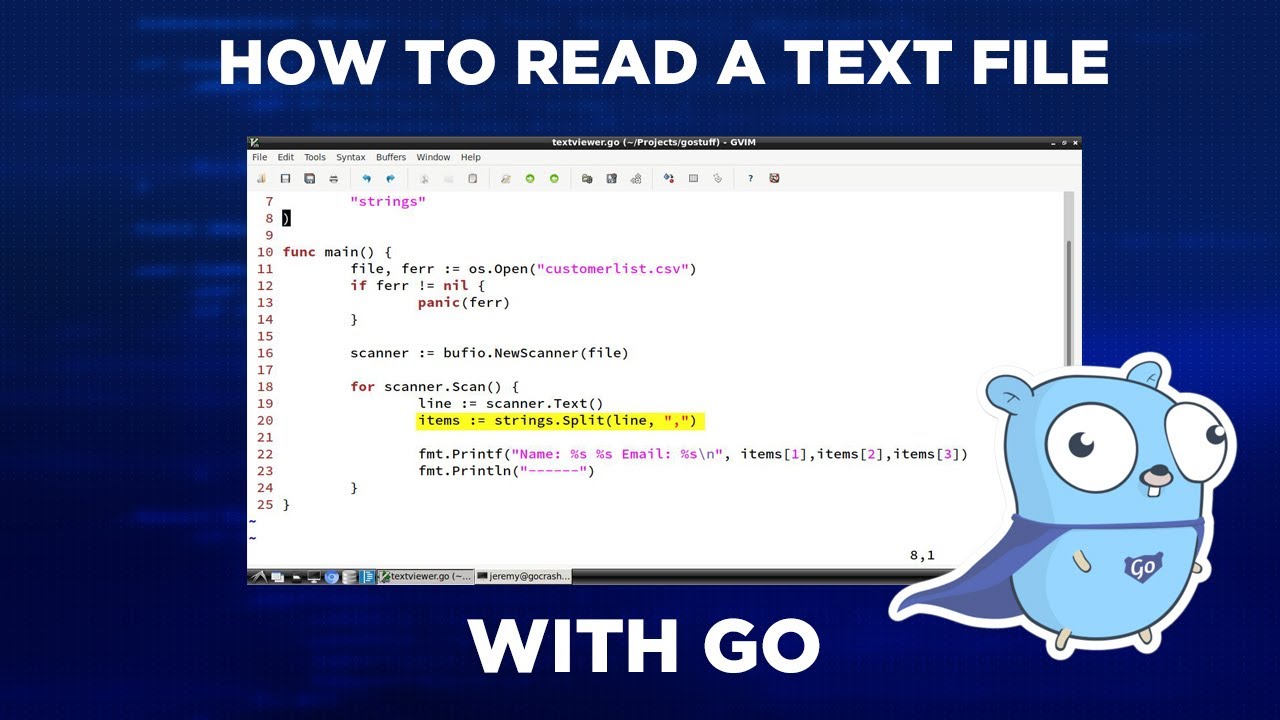
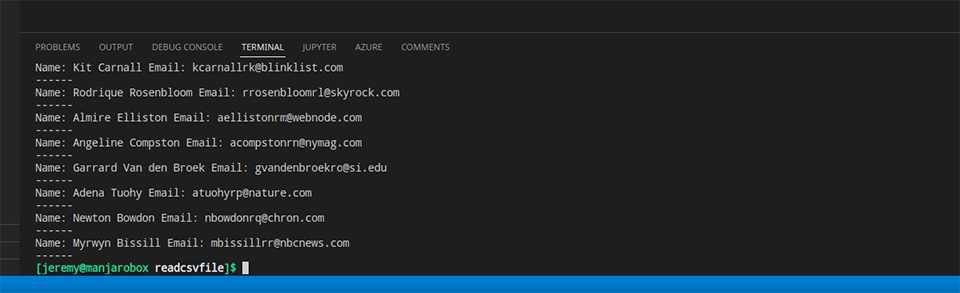
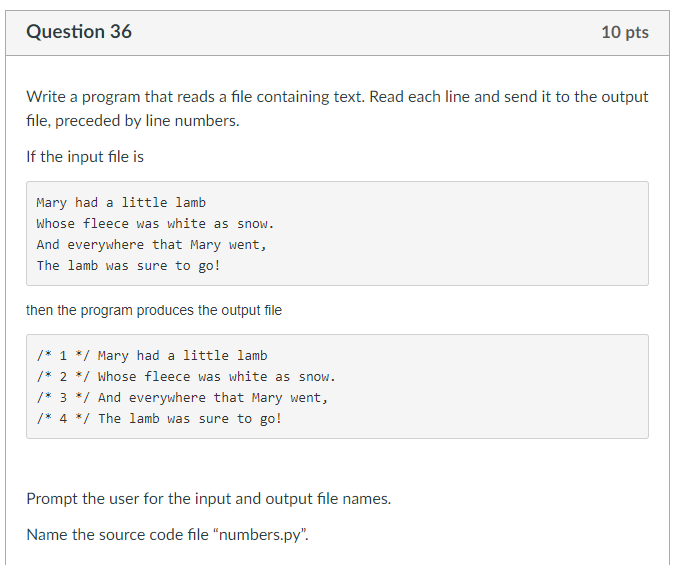
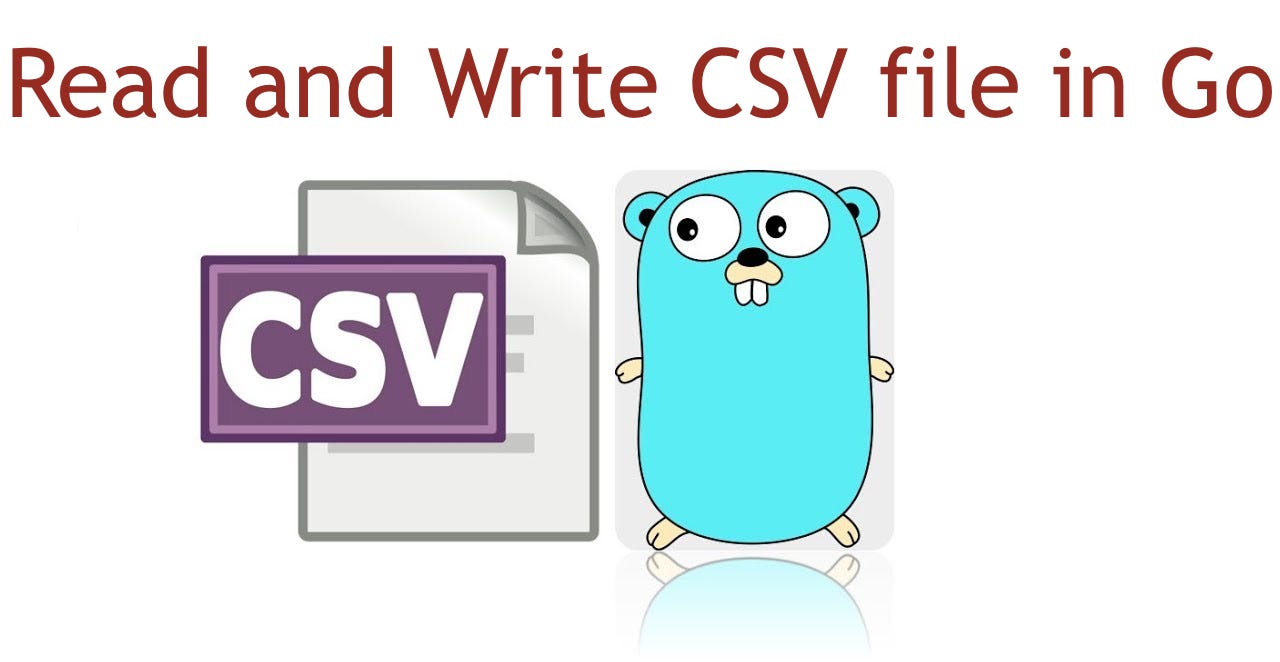

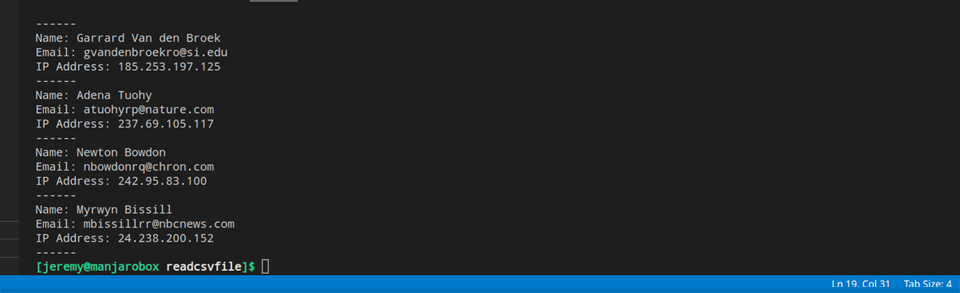
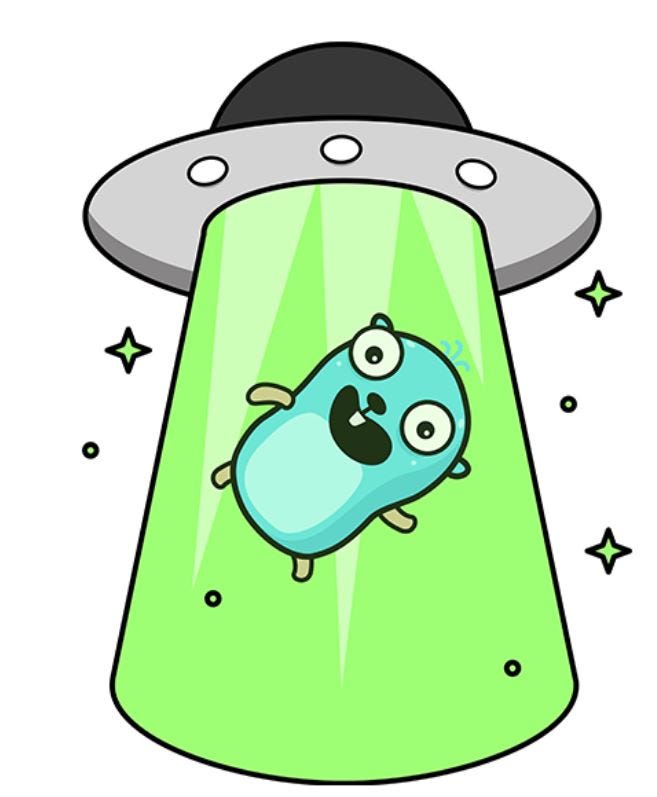
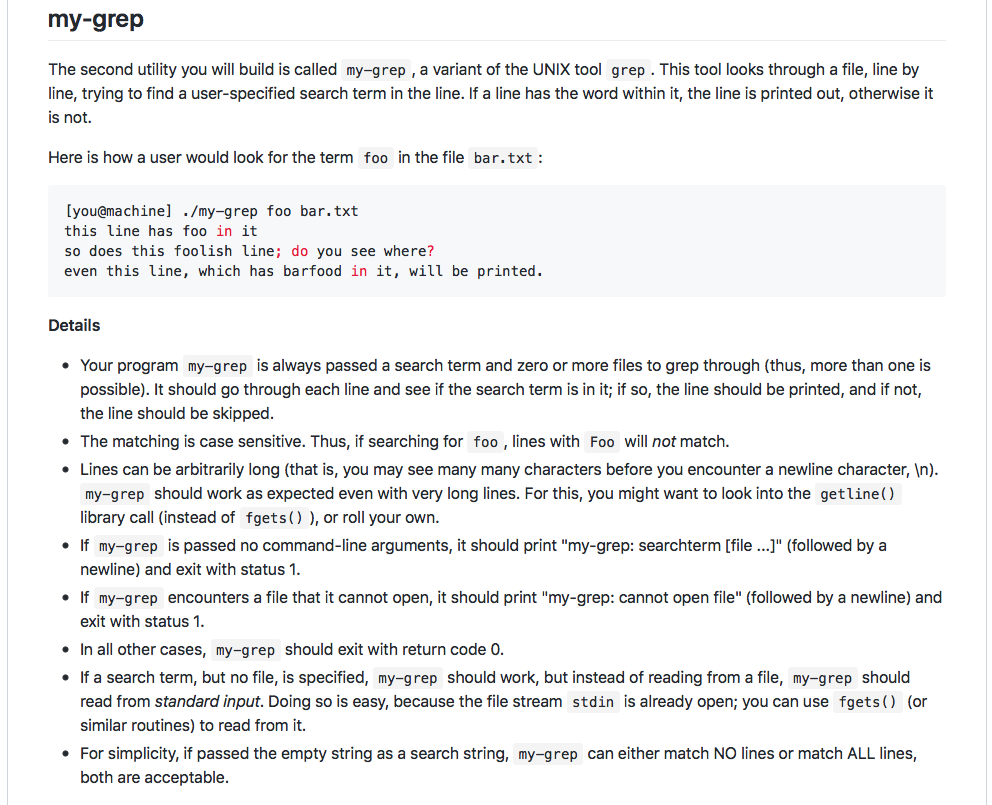
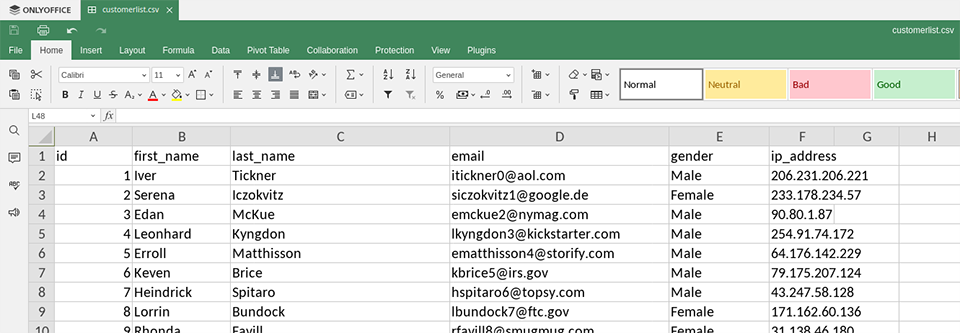
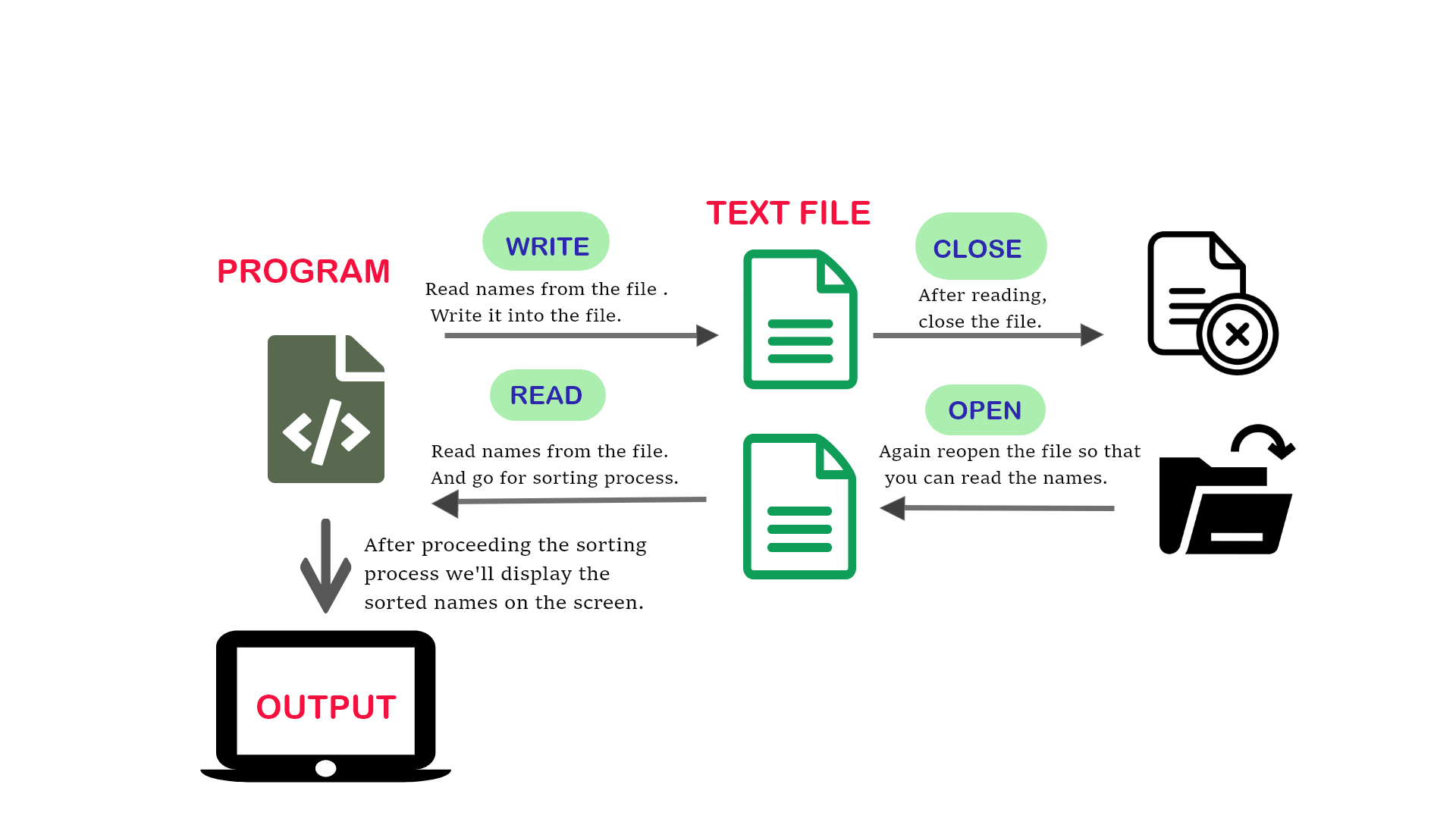
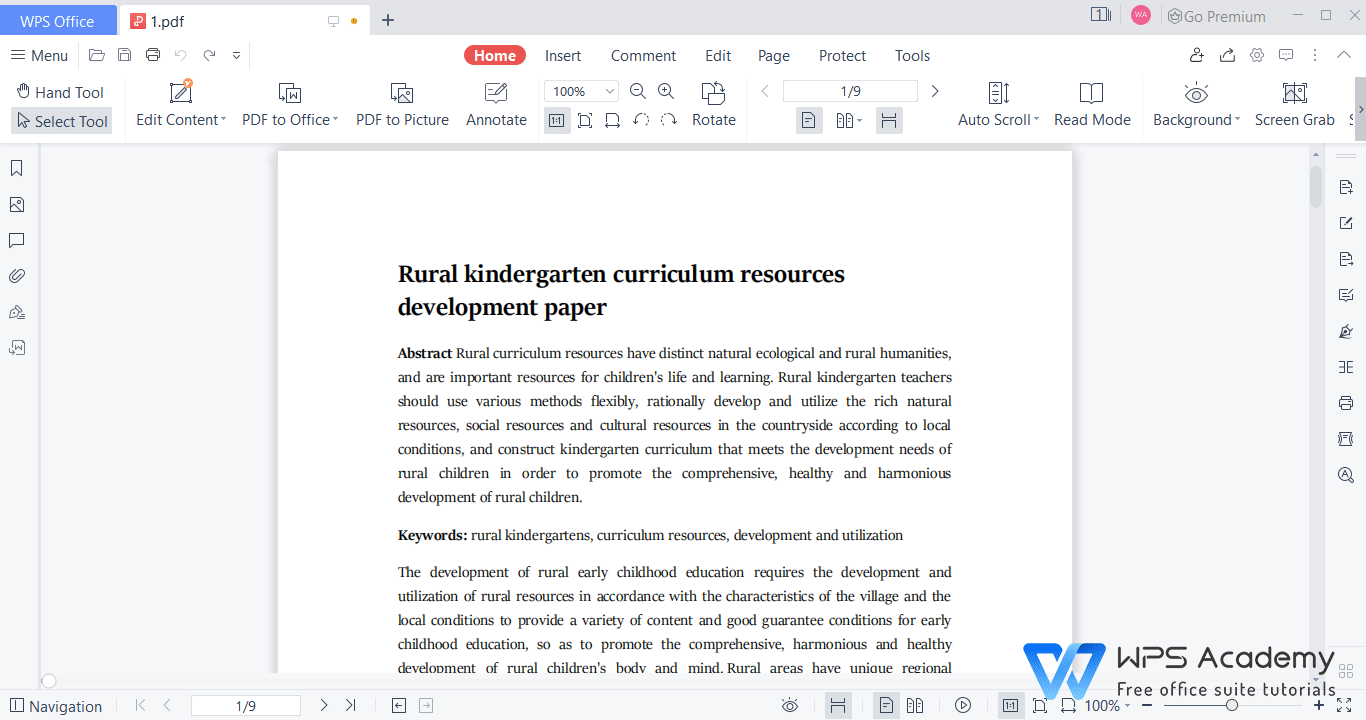
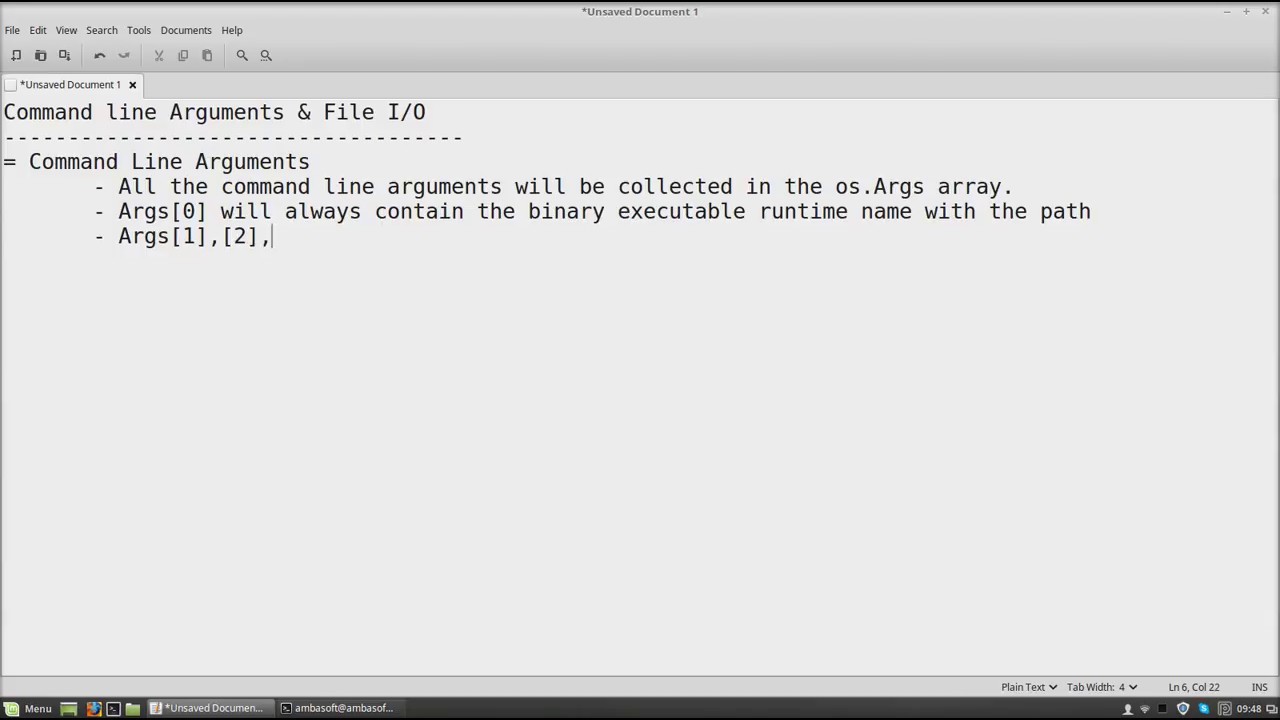
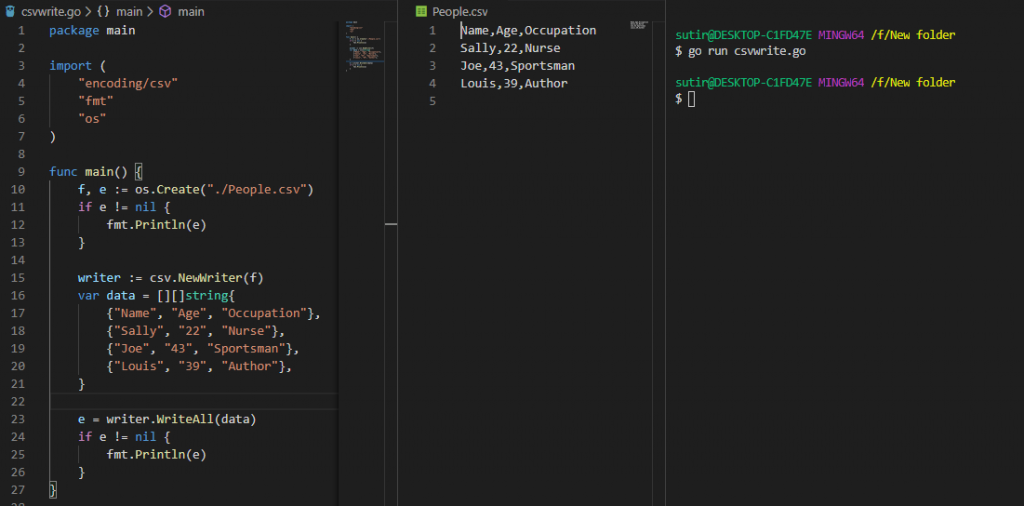
.thumb.jpg.e9898b7e912697eeb84679298182cda3.jpg)

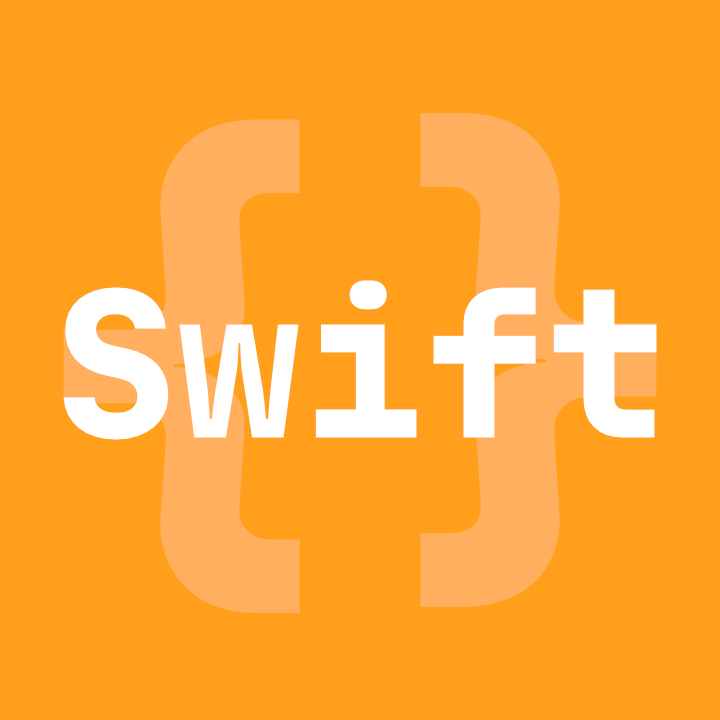
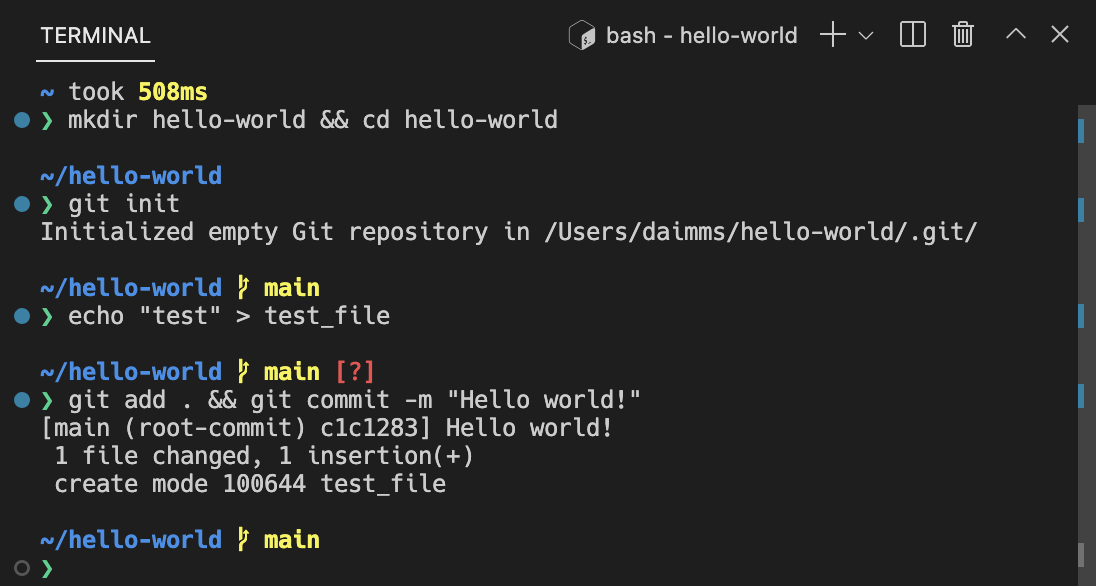
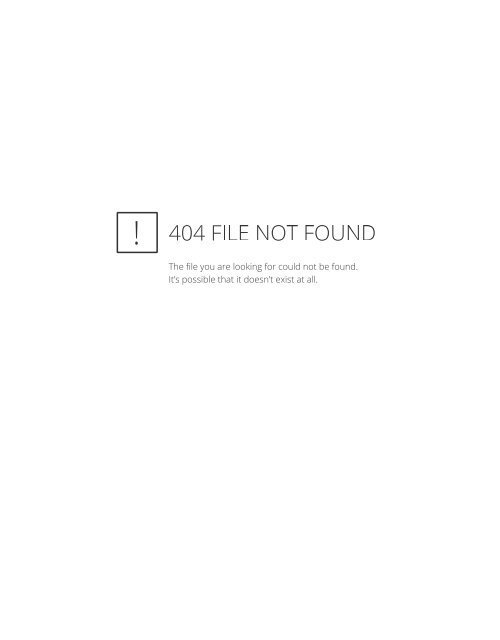

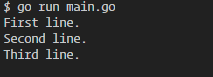

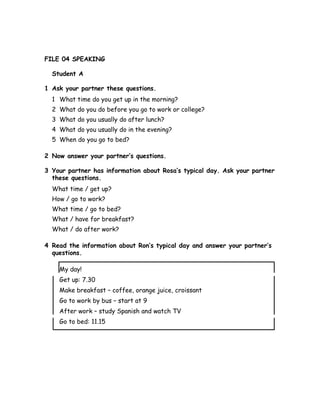


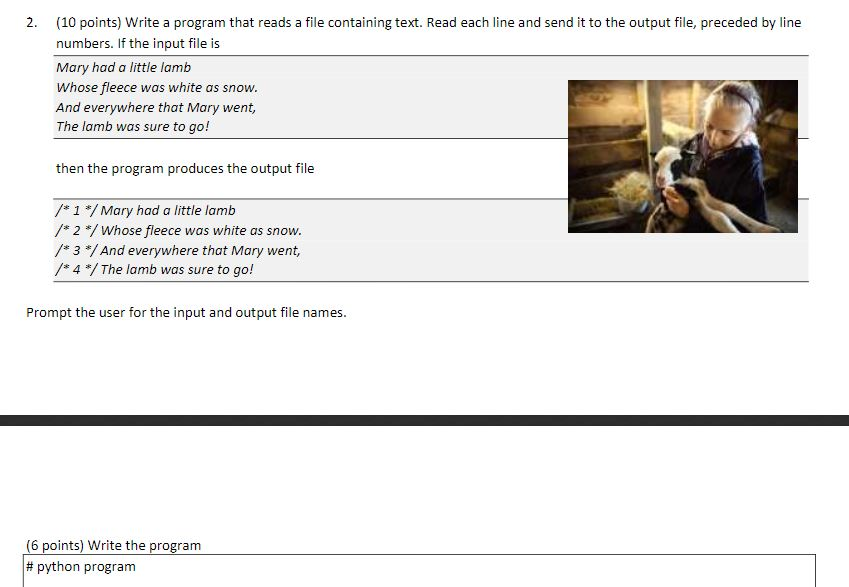
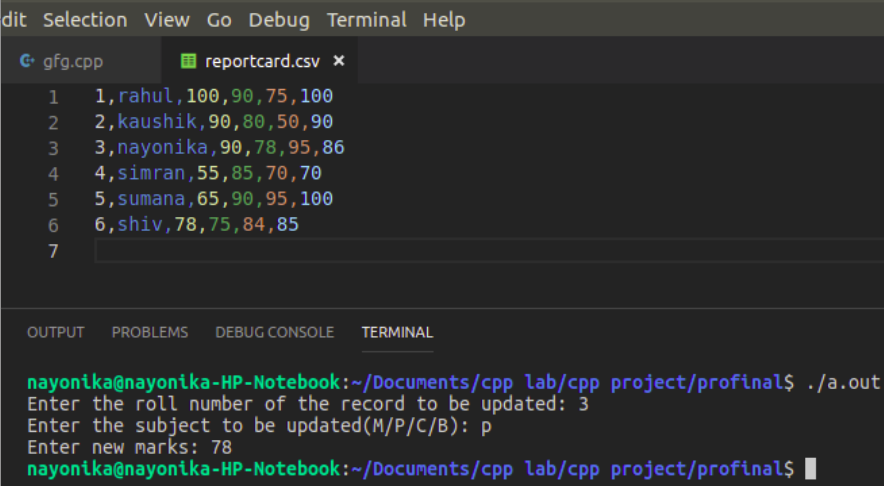
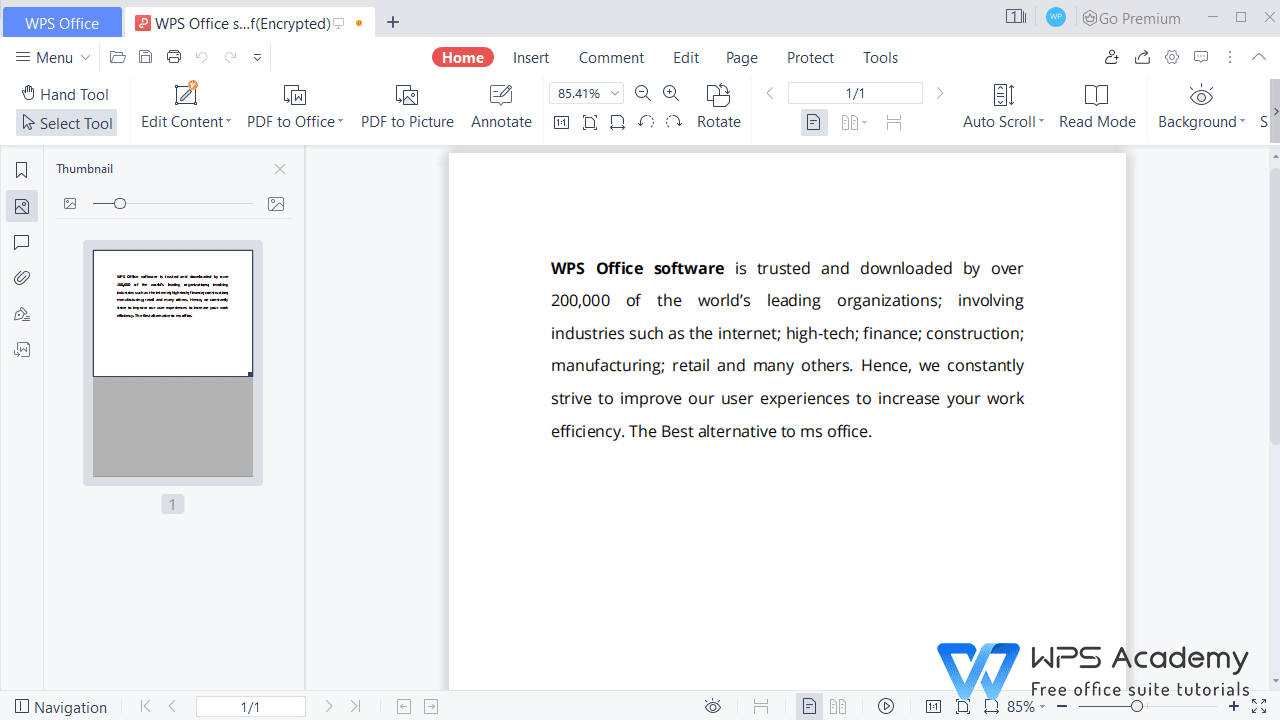
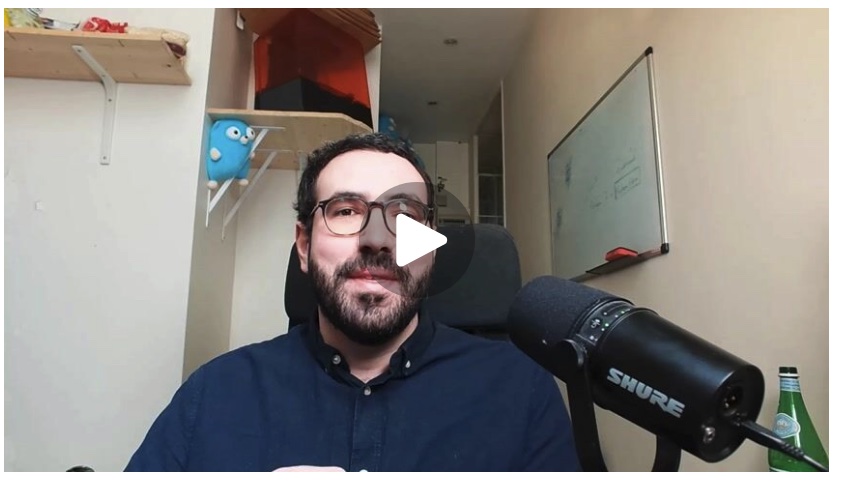
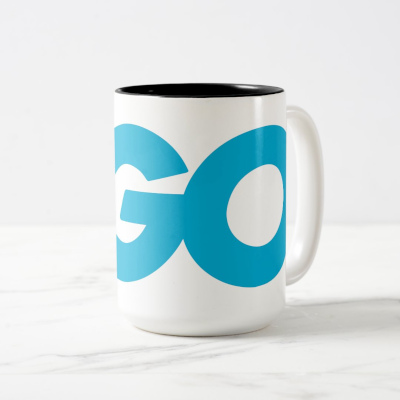
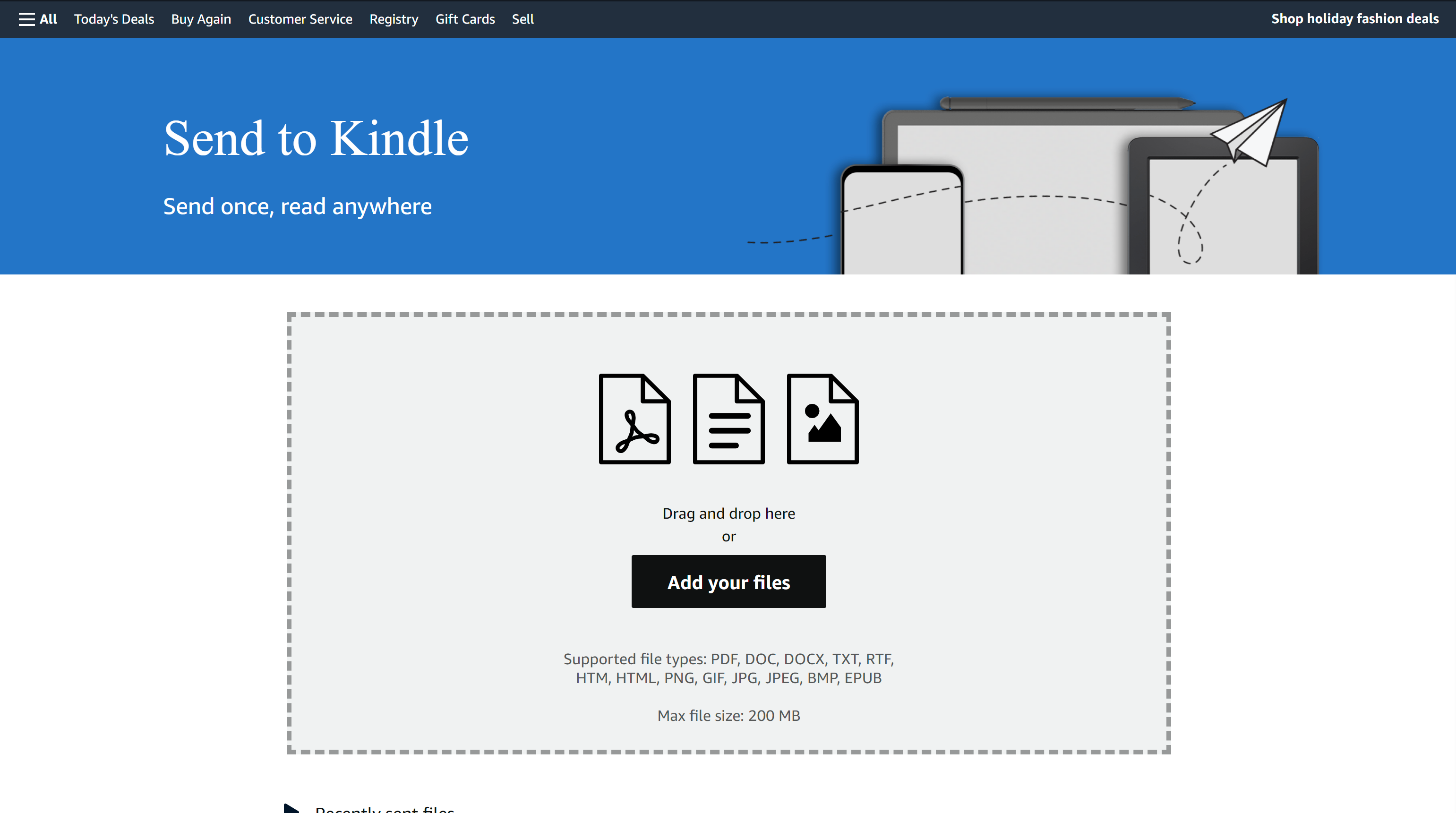


Article link: golang read file line by line.
Learn more about the topic golang read file line by line.
- Reading a file line by line in Go – string
- Golang Read File Line by Line
- Reading file line by line in Go
- How to Read File Line by Line in Golang?
- Golang program to read the content of a file line by line
- How to Read a File Line by Line to String in Golang?
- Golang read file line by line – 3 Simple ways
- Golang read file line by line to string – golangprograms.com
- How to Read a File Line by Line | Golang Project Structure
- Go: Read a file line by line – Programming With Swift