Golang Get Unique Values From Slice
1. Introduction to the concept of slices in Golang
Slices in Golang are an essential data structure that allows you to work with a dynamic collection of elements. A slice is a reference to an underlying array and provides a flexible way to store and manipulate data. It is similar to an array, but with an unspecified length.
2. Understanding the need for getting unique values from a slice
In many applications, you may come across scenarios where you need a slice with only unique values. Duplicate entries can lead to unexpected behavior and incorrect results. Therefore, it becomes crucial to remove duplicates and obtain a slice containing only distinct elements.
3. Exploring the common approach of using a map to get unique values
One of the most common approaches to remove duplicates from a slice in Golang is by utilizing a map. The concept revolves around using the elements of the slice as keys in a map. Since maps do not allow duplicate keys, this method automatically removes the duplicates.
4. Implementing a function to remove duplicates from a slice using a map
To implement this approach, you need to define a function that takes a slice as input and returns a new slice with unique values. Within the function, create an empty map and iterate over the elements of the input slice. Add each element to the map as a key and set the corresponding value to true.
5. Utilizing an empty struct as the value in the map to reduce memory usage
While implementing the map-based approach, you can take advantage of the fact that maps in Golang do not store the value associated with each key explicitly. Instead, they simply store a reference to the associated value. By using an empty struct as the value in the map, you can significantly reduce memory consumption.
6. Handling slices containing complex types and custom structs
The map-based approach can handle slices containing not only primitive types like integers or strings but also complex types and custom structs. As long as the elements of the slice are comparable, they can be used as keys in the map.
7. Considering the order of elements in the original slice while removing duplicates
When using the map-based approach, it’s important to note that the order of elements in the original slice may not be preserved in the resulting slice. Maps are inherently unordered collections, and therefore, the order of insertion is not guaranteed.
8. Evaluating the performance and efficiency of the map-based approach
The map-based approach for getting unique values from a slice generally offers good performance and efficiency. The time complexity of this approach is O(n) for inserting elements into the map, where n is the number of elements in the original slice. The space complexity is also O(n), where n is the number of unique elements.
9. Exploring an alternative method using a nested loop for getting unique values
Another method to obtain unique values from a slice is by using a nested loop. In this approach, you iterate over each element of the slice and compare it with the remaining elements to identify duplicates.
10. Comparing the map-based and nested loop approaches for different scenarios and use cases
Both the map-based and nested loop approaches have their own advantages and limitations. The map-based method excels in terms of performance and simplicity, especially for large slices. On the other hand, the nested loop approach may be more suitable for smaller slices or when preserving the order of elements is crucial.
FAQs
Q1. How can I get a unique slice in Golang?
A1. You can use the map-based approach mentioned in this article to obtain a unique slice by treating the elements as keys in a map.
Q2. Can I use the map-based approach for slices containing complex types or custom structs?
A2. Yes, the map-based approach can handle slices containing complex types and custom structs as long as the elements are comparable.
Q3. Is the order of elements preserved when using the map-based approach?
A3. No, the map-based approach does not guarantee the preservation of the order of elements in the original slice.
Q4. What is the time complexity of the map-based approach?
A4. The time complexity of the map-based approach is O(n) for inserting elements into the map, where n is the number of elements in the original slice.
Q5. Are there any alternate methods to obtain unique values from a slice?
A5. Yes, you can use a nested loop approach to identify and remove duplicates from a slice. However, it may have performance limitations compared to the map-based approach, especially for larger slices.
How To Remove A Value From Slice Based On Index In Golang
How To Remove Duplicate Values From Slice In Golang?
Golang, also known as Go, is a programming language developed by Google. It is known for its simplicity, efficiency, and built-in support for concurrency. When working with slices, it is common to encounter duplicate values. In this article, we will explore different methods to remove duplicate values from a slice in Golang.
Method 1: Using a Map
One way to remove duplicate values from a slice is by using a map. Maps in Golang are key-value pairs where each key is unique. We can leverage this property to remove duplicates from a slice.
Here’s an example:
“`go
func RemoveDuplicates(input []int) []int {
// Create an empty map
uniqueValues := make(map[int]bool)
// Iterate over the slice
for _, value := range input {
// Check if value exists in the map
if _, exists := uniqueValues[value]; !exists {
// Add value to the map
uniqueValues[value] = true
}
}
// Create a new slice to store unique values
result := make([]int, 0, len(uniqueValues))
// Append unique values to the new slice
for value := range uniqueValues {
result = append(result, value)
}
return result
}
“`
In this approach, we create an empty map called `uniqueValues` to keep track of unique values. We iterate over the input slice, and for each value, we check if it exists in the map. If it doesn’t, we add it to the map. Finally, we create a new slice called `result` and append all the unique values from the map to it. By doing this, we effectively remove any duplicate values from the original slice.
Method 2: Using a Slice
Another method to remove duplicate values from a slice is by using another slice. We initialize an empty slice and iterate over the input slice, checking if the value already exists in the new slice. If it doesn’t, we append it to the new slice. This approach maintains the order of the elements in the original slice.
Here’s an example:
“`go
func RemoveDuplicates(input []int) []int {
uniqueValues := []int{}
for _, value := range input {
found := false
for _, uniqueValue := range uniqueValues {
if uniqueValue == value {
found = true
break
}
}
if !found {
uniqueValues = append(uniqueValues, value)
}
}
return uniqueValues
}
“`
In this approach, we create an empty slice called `uniqueValues` to store the unique values. We iterate over the input slice and set a boolean variable called `found` to false. Then, we iterate over the `uniqueValues` slice and check if the current value already exists. If it does, we set `found` to true and break out of the loop. If the value is not found, we append it to the `uniqueValues` slice. Finally, we return the `uniqueValues` slice, which contains the unique values from the original slice.
FAQs:
Q: Can these methods be used for slices of other types, such as strings or structs?
A: Yes, these methods can be used for slices of any type, not just integers. You can replace `[]int` with the appropriate type in the function signature and modify the logic accordingly.
Q: Will the order of elements be preserved when removing duplicates using these methods?
A: Method 2, which uses a slice, preserves the order of the elements in the original slice. However, Method 1, which uses a map, does not guarantee the order of the elements since maps in Golang are not ordered.
Q: Are there any built-in functions or packages in Golang to remove duplicates from a slice?
A: Golang does not provide a built-in function or package specifically to remove duplicates from a slice. However, the aforementioned methods are efficient and simple to implement.
Q: How do I test the performance of these methods?
A: You can use the built-in `testing` package in Golang to write test cases for these methods. By creating a large input slice with duplicate values and measuring the execution time, you can assess the performance of each method.
Q: Can these methods be used for multidimensional slices?
A: Both methods can be used for multidimensional slices as well. You need to modify the type and logic accordingly when working with multidimensional data.
In summary, removing duplicate values from a slice in Golang can be achieved using a map or another slice. Both methods have their advantages, such as preserving order or efficiency. Choose the method that best suits your requirements and consider the FAQs section as a reference for any further questions.
How To Get One Element In Slice Golang?
In the Go programming language (Golang), a slice is a dynamically sized, flexible view of elements in an array. It provides a more powerful and convenient way to work with arrays. Slices are widely used in Go for various purposes, such as storing and manipulating collections of data. Often, you may need to access or retrieve a specific element from a slice. In this article, we will explore different approaches to get one element from a slice in Golang.
Using Index Position
The most straightforward way to get an element from a slice is by using its index position. Each element in a slice has an associated index starting from 0 for the first element. To access an element at a particular index, simply use the index position within square brackets after the slice variable.
For example, consider the following code snippet:
“`go
package main
import “fmt”
func main() {
nums := []int{1, 2, 3, 4, 5}
element := nums[2]
fmt.Println(element)
}
“`
In this snippet, we have a slice called `nums` with five elements. We want to retrieve the element at index 2, which is 3. By assigning `nums[2]` to the variable `element`, we can access and print the desired element using `fmt.Println(element)`. Running this code will output `3`.
Using Slicing
Another method to get one element from a slice is by utilizing Golang’s slicing feature. Slicing allows you to extract a portion of a slice by specifying a range of indices. By defining the start and end indices for the range, you can obtain a new slice that contains only a subset of the original elements.
To retrieve a single element using slicing, we need to specify the index of the desired element as both the start and end indices in the slice expression.
Consider the following example:
“`go
package main
import “fmt”
func main() {
nums := []int{1, 2, 3, 4, 5}
element := nums[2:3][0]
fmt.Println(element)
}
“`
In this snippet, we create a slice `nums` with the same elements as before. By defining a slice expression `nums[2:3]`, we can extract a sub-slice that contains only the element at index 2. Finally, by appending `[0]` to the expression, we obtain the element itself rather than a sub-slice. The output of this code will again be `3`.
Using the len() Function
There is another way to get one element from a slice using the len() function. The len() function returns the number of elements in a slice. Combining it with indexing, we can access the last element of a slice using `slice[len(slice)-1]`. Similarly, we can access the first element using `slice[0]`.
Consider the following example:
“`go
package main
import “fmt”
func main() {
nums := []int{1, 2, 3, 4, 5}
lastElement := nums[len(nums)-1]
fmt.Println(lastElement)
}
“`
In this code, `nums[len(nums)-1]` retrieves the last element of the `nums` slice. The output of this code will be `5`.
FAQs
Q: Can I get multiple elements from a slice using the same techniques?
A: Yes, the same techniques can be used to retrieve multiple elements from a slice. For example, you can access a range of elements by specifying the start and end indices in a slice expression.
Q: What happens if I try to access an index that is out of bounds?
A: If you attempt to access an index that is greater than or equal to the length of the slice, or less than zero, it will result in a runtime error. It is important to ensure that the index is within the valid range.
Q: Does Go have a built-in function to directly retrieve the minimum or maximum element from a slice?
A: No, there is no built-in function specifically for finding the minimum or maximum element. However, you can easily implement your own solutions using techniques such as looping through the slice or sorting the slice and selecting the first or last element.
Q: Are there any performance considerations when choosing between these methods?
A: When accessing a single element, all of the mentioned techniques have similar performance characteristics. However, if you need to repeatedly access elements in a slice, indexing directly by the index position is typically the most efficient approach.
In conclusion, Golang provides multiple methods to retrieve one element from a slice. Whether you prefer using index positions, slicing, or the len() function, all these approaches can effectively fetch the desired elements from the slice. Remember to handle index out of bounds errors and consider performance when selecting the most suitable method for your specific use case.
Keywords searched by users: golang get unique values from slice Golang unique slice, Golang remove duplicates from slice, Slice contains golang, Golang distinct array, Sort slice Golang, Golang find in array, Check duplicate in array Golang, Remove element in array golang
Categories: Top 10 Golang Get Unique Values From Slice
See more here: nhanvietluanvan.com
Golang Unique Slice
Introduction:
In the world of programming, there is often a need to store and manipulate collections of data efficiently. Go, also known as Golang, being a popular open-source programming language, provides many built-in functionalities to handle such requirements. One of these powerful features is the unique slice, which allows developers to streamline their data in an organized and optimized manner. In this article, we will delve into the concept of a unique slice in Golang, its implementation, and its benefits. So, let’s get started!
What is a Unique Slice in Golang?
A unique slice, as the name suggests, is a data structure in Go that enables the storage of a collection of unique elements. Unlike a traditional slice, which allows duplicate elements, a unique slice only allows each element to appear once. This unique property sets it apart and makes it a valuable asset for developers who aim for efficient data management while eliminating redundancy.
Implementing a Unique Slice:
In order to create a unique slice in Golang, the standard library provides a data structure called a map. A map is an unordered collection of key-value pairs, where each key must be unique. In the context of a unique slice, the map’s functionality is harnessed to handle unique elements of the slice efficiently.
To implement a unique slice, we start by declaring a map with the desired element type. For instance, let’s say we want to create a unique slice of integers:
“`
uniqueSlice := map[int]bool{}
“`
Here, the keys of the map represent unique integers, and the corresponding boolean value has no significance other than indicating the existence of the key. We can then use this map to store the unique elements of our collection.
Adding elements to the unique slice is trivial. We can simply assign a boolean value of `true` to each new element we want to add:
“`
uniqueSlice[42] = true
“`
This line of code adds the integer value 42 to the unique slice. If the element already exists in the map, it will be updated with `true`, ensuring its uniqueness.
Retrieving elements from the unique slice can be done using a `range` loop:
“`
for elem := range uniqueSlice {
fmt.Println(elem) // Prints the unique elements one by one
}
“`
Benefits of Using a Unique Slice:
1. Eliminating Redundancy: The primary advantage of using a unique slice is that it ensures the absence of duplicate elements. This makes the data more compact and efficient, saving both memory and processing time.
2. Simplified Operations: With a unique slice, developers can perform operations like union, intersection, difference, and merging of slices with ease. Since each element is unique, these operations become simpler and more predictable.
3. Enhanced Search Performance: Searching for an element within a unique slice becomes highly optimized thanks to the unique property. There is no need to loop through the entire slice since duplicates are absent, resulting in improved search performance.
FAQs (Frequently Asked Questions):
Q: Can a unique slice contain elements of any type?
A: Yes, a unique slice can store elements of any type that is allowed by the Go programming language.
Q: Is it possible to convert a traditional slice into a unique slice?
A: Absolutely! By iterating over the elements of a traditional slice and adding them one by one to the map with a boolean value of `true`, you can convert it into a unique slice.
Q: How does a unique slice differ from a set?
A: In some programming languages, sets are a dedicated data structure for storing unique elements. In Golang, a unique slice is implemented using a map, which provides similar functionality to a set.
Q: Are there any disadvantages to using a unique slice?
A: While unique slices offer numerous advantages, they also have some trade-offs. The primary disadvantage is that the order of elements is not preserved. If element ordering is critical, a traditional slice should be considered instead.
Conclusion:
Golang’s unique slice offers a practical approach to efficiently manage collections of data while ensuring the uniqueness of each element. By utilizing a map to store unique elements, developers can streamline their data, enhance search performance, and simplify various operations. Understanding the concept of a unique slice opens up new possibilities for organizing and manipulating data in a concise and optimized manner. So, embrace this powerful feature and take your data management skills to new heights with Golang’s unique slice.
Golang Remove Duplicates From Slice
Go, also known as Golang, is a powerful programming language that offers simplicity and efficiency while maintaining robustness. One common task when working with slices in Golang is removing duplicate elements. In this article, we will explore various approaches to remove duplicates from a slice in Go, from basic methods to more advanced techniques.
Understanding Slice in Golang
Before diving into the topic of removing duplicates, let’s briefly touch upon what a slice is in Go. A slice is a dynamically sized, flexible view of an underlying array. It provides a convenient way to work with sequences of data, allowing the addition and removal of elements as needed.
Golang Slice Example:
“`go
numbers := []int{1, 2, 3, 4, 5}
“`
In the example above, “numbers” is a slice containing five integers. Slices can contain elements of any data type, including structs, strings, and more.
Basic Approach: Using a Map
One straightforward way to remove duplicates from a slice in Go is by using a map. Maps are an unordered collection of key-value pairs. In this approach, the elements of the slice will be treated as keys in the map, ensuring uniqueness. Let’s see how this can be implemented:
“`go
numbers := []int{1, 2, 3, 4, 4, 5, 2}
unique := make(map[int]bool)
for _, num := range numbers {
unique[num] = true
}
result := []int{}
for k := range unique {
result = append(result, k)
}
“`
In the code snippet above, we start by creating an empty map called “unique” using the make() function. Then, we iterate through each element of the slice, and assign it as a key in the map with a value of true. This ensures that duplicate keys will be automatically discarded.
Finally, we create a new slice called “result” and append each key from the map to build the final slice. This approach gives us a slice containing only unique elements.
Advanced Approach: Using the Sort Package
The basic approach presented above does not preserve the original ordering of the elements. If maintaining the order is crucial, we can use the sort package in Go to achieve this.
To use this approach, we need to follow these steps:
1. Convert the slice to a set by inserting all elements into a map. The value can be set to an unused struct: `map[T]struct{}`.
2. Extract all keys from the map into a new slice.
3. Sort the new slice using the sort package.
4. Return the sorted slice.
Let’s look at an example code snippet demonstrating this advanced approach:
“`go
import (
“sort”
)
func removeDuplicates(numbers []int) []int {
set := make(map[int]struct{})
for _, num := range numbers {
set[num] = struct{}{}
}
unique := make([]int, 0, len(set))
for k := range set {
unique = append(unique, k)
}
sort.Ints(unique)
return unique
}
“`
The code snippet above introduces a function named “removeDuplicates” that takes a slice of integers as input and returns a sorted slice without any duplicates. It follows the steps mentioned earlier to achieve the desired result.
FAQs
Q: Why can’t we remove duplicates directly from the original slice?
A: Unlike some other programming languages, Go does not have built-in methods to directly remove duplicates from a slice. Thus, we need to use alternative methods, such as using maps or sort packages, to accomplish this task.
Q: Can we remove duplicates from slices of custom types?
A: Yes, the methods discussed in this article can be applied to slices containing elements of custom types as well. However, for custom types, you need to specify the equality criterion explicitly.
Q: How efficient are the methods discussed in this article?
A: The basic method using a map has a time complexity of O(n) since it iterates through each element once. The advanced method has a time complexity of O(n*log(n)) due to sorting. Both methods are efficient and suitable for most use cases.
Q: Is it possible to remove duplicates in place, without creating a new slice?
A: Yes, it is possible. However, it requires manipulation of the slice by reordering the values, potentially leading to a less readable and more complex code. The methods discussed in this article provide a clean and straightforward way to remove duplicates.
In conclusion, removing duplicates from a slice is a common task in Go, and there are multiple approaches to achieve this. The basic approach using a map allows for a simple implementation, while the advanced approach using the sort package enables preservation of the original order. By understanding and utilizing these methods, you can efficiently remove duplicates from slices in your Golang programs.
Slice Contains Golang
Slices in Go are analogous to arrays, but with dynamic sizes. They allow you to work with a contiguous section of an underlying array without the need to specify its length during initialization. This feature makes slices a vital part of Go programming, enabling efficient memory management and flexible data handling.
To declare a slice in Go, you use the following syntax:
“`go
var sliceName []type
“`
Here, `sliceName` is the name you choose for your slice, and `type` indicates the type of elements that the slice will hold. For example, if you want to create a slice of integers, you would use the following code:
“`go
var numbers []int
“`
One significant advantage of slices is their ability to grow or shrink dynamically. Unlike arrays, which have a fixed size, slices can expand or contract as needed. To add elements to a slice, you can use the `append()` function, which appends one or more elements to the end of the slice. For example:
“`go
numbers := []int{1, 2, 3}
numbers = append(numbers, 4, 5, 6)
“`
In this code snippet, we first initialize `numbers` as a slice containing the integers 1, 2, and 3. Then, using `append()`, we add the elements 4, 5, and 6 to the end of the `numbers` slice. The result will be a expanded slice with the values [1, 2, 3, 4, 5, 6].
Another important operation with slices is slicing itself. Slicing allows you to create a new slice from an existing one by specifying the range of indices to be included. The syntax for slicing is as follows:
“`go
sliced := sliceName[startIndex: endIndex]
“`
Here, `startIndex` is the index of the first element to include in the new slice, and `endIndex` is the index of the first element to exclude from the new slice. For instance:
“`go
numbers := []int{1, 2, 3, 4, 5, 6}
sliced := numbers[1:4]
“`
In the above code snippet, `sliced` will contain the numbers [2, 3, 4]. By default, the start index is inclusive, and the end index is exclusive, meaning the element at the end index itself is not included in the new slice.
FAQs:
Q1. What is the difference between arrays and slices in Go?
A1. Arrays have a fixed size determined during initialization, while slices have dynamic sizes that can be modified during runtime. Additionally, arrays are value types, meaning they are copied when passed as function arguments, whereas slices are reference types, so changes made to a slice inside a function will affect the original slice.
Q2. How are slices different from maps in Go?
A2. Slices are used to store ordered collections of elements, similar to arrays, but with dynamic sizes. On the other hand, maps are used to store key-value pairs, allowing efficient lookups based on keys. While both are powerful, Go data structures, they serve different purposes.
Q3. Can I have a slice of slices in Go?
A3. Yes, Go supports multi-dimensional slices. You can create a slice of slices by defining the initial slice as `[][]type`, where `type` represents the type of elements in the nested slices. This enables creating and manipulating two-dimensional arrays using slices.
Q4. Is there a limit to the number of elements I can add to a slice?
A4. Technically, there is no limit to the elements you can add to a slice. However, the underlying array that the slice points to has a limit owing to the available memory. If the number of elements exceeds this limit, you will need to create a new larger slice and copy the existing elements into it.
Q5. How can I check if a slice is empty?
A5. You can use the `len()` function to check if a slice has any elements. If the length returned by `len()` is zero, then the slice is empty. For example:
“`go
numbers := []int{}
if len(numbers) == 0 {
fmt.Println(“The slice is empty.”)
}
“`
In conclusion, slices are a powerful and dynamic data structure in Go that allow for more flexible array handling. With their ability to grow or shrink as needed, they provide efficient memory management and ease of use. Slices are widely used in Go programming, making them an essential topic to understand for any Go developer.
Images related to the topic golang get unique values from slice
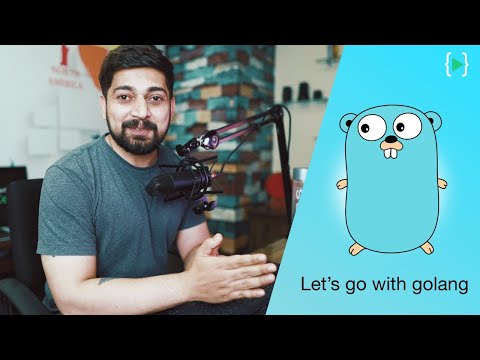
Found 18 images related to golang get unique values from slice theme
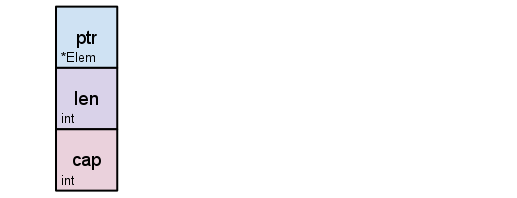
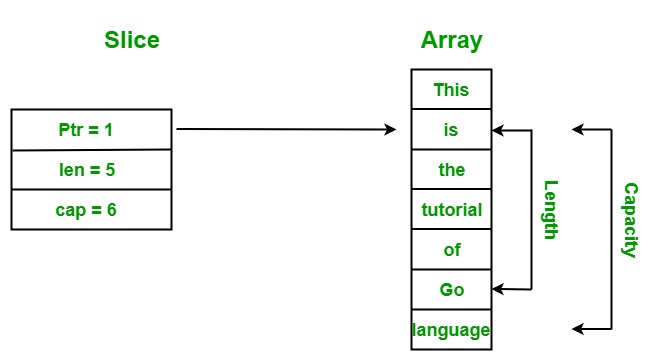
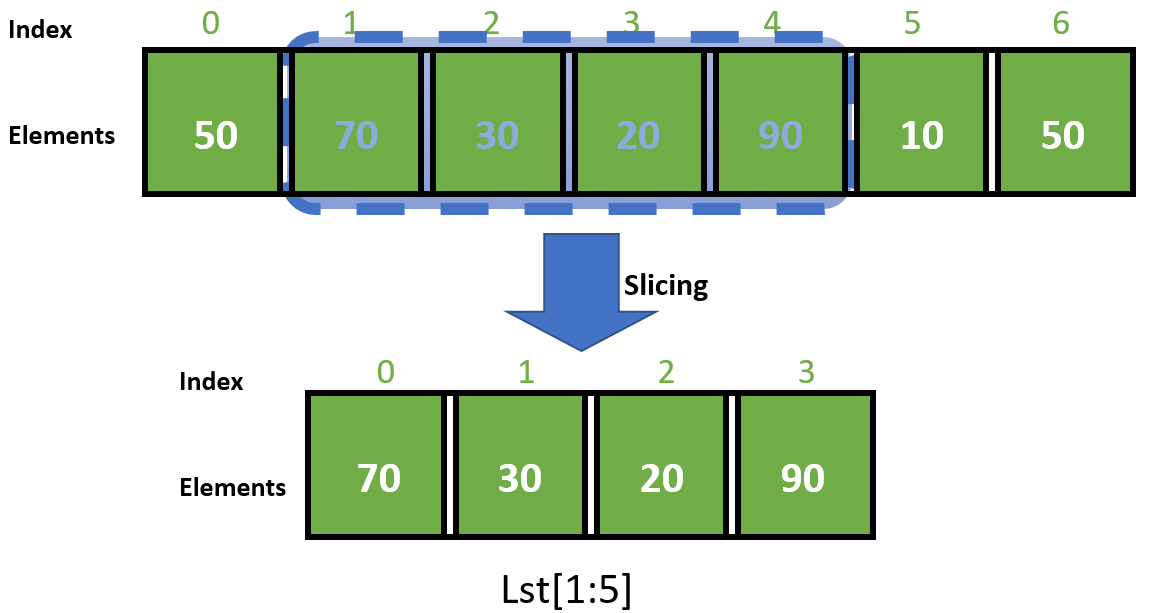

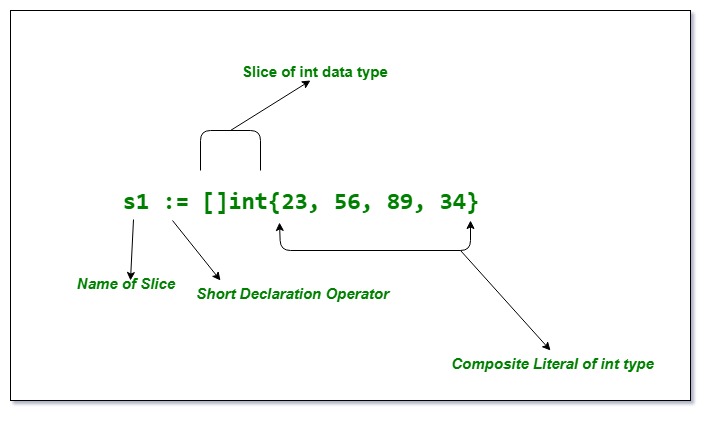
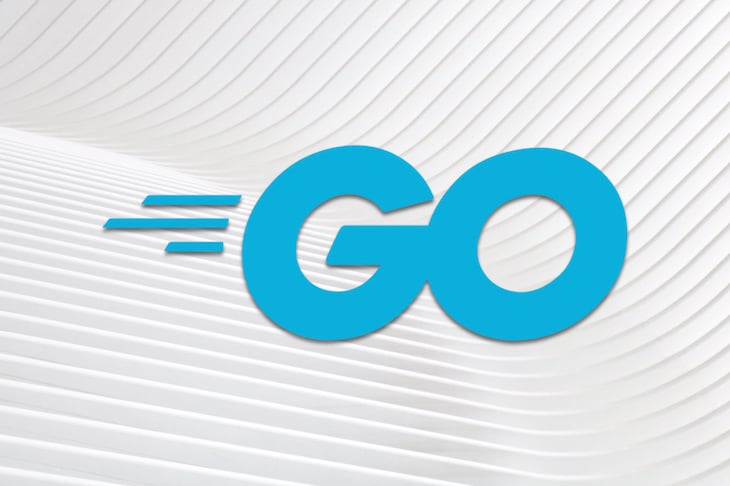
Article link: golang get unique values from slice.
Learn more about the topic golang get unique values from slice.
- How to Remove duplicate values from Slice? – Golang Programs
- How to get unique values from slice – Golang – OneLinerHub
- Return Unique items in a Go slice
- Finding Unique Items in a Go Slice or Array – Stack Overflow
- How to Remove Duplicate Values from Slice in Golang?
- Creating Unique Slices in Go – Kyle Banks
- Go code that ensures elements in a slice are unique · GitHub
- How to Remove Duplicate Values from Slice in Golang
- unique – Go Packages
- Removing duplicate elements from an array/slice in Golang – Souvik Haldar
- How to get the first element of a slices in golang? – aGuideHub
- How to Get First and Last Element of Slice in Golang? – GeeksforGeeks
- ️ Remove duplicates from any slice using Generics in Go
See more: nhanvietluanvan.com/luat-hoc