Golang Byte To String
What is a Byte?
Before diving into the conversion methods, let’s briefly understand what a byte is. In computer science, a byte is a unit of digital information that typically consists of eight bits. It is the fundamental building block of storage and processing in most modern computer systems. Bytes are commonly used to represent characters, numbers, and other data types.
Representation of a Byte in Go
In Golang, a byte is represented by the `byte` data type. This type is an alias for the `uint8` type, indicating an 8-bit unsigned integer. By default, when Go source code is UTF-8 encoded, a string literal is represented using a sequence of bytes.
Converting Byte to String in Go
There are multiple ways to convert a byte to a string in Golang. We will explore some of the most common approaches in the following sections.
1. Using the Built-in String() Function:
Golang provides a built-in function called `string()` that converts a sequence of bytes into a string. This function takes a byte slice as input and returns its corresponding string representation. Here’s an example:
“`go
byteSlice := []byte{71, 111, 108, 97, 110, 103}
str := string(byteSlice)
fmt.Println(str) // Output: Golang
“`
2. Converting Byte to String using the strconv Package:
The `strconv` package in Golang provides functions for converting various data types, including bytes, to strings. To convert a byte slice to a string, we can use the `strconv.Itoa()` function, which converts an integer to its string representation. Here’s an example:
“`go
byteSlice := []byte{49, 50, 51}
str := strconv.Itoa(int(byteSlice[0])) + strconv.Itoa(int(byteSlice[1])) + strconv.Itoa(int(byteSlice[2]))
fmt.Println(str) // Output: 123
“`
3. Converting Byte to String using the bytes Package:
The `bytes` package in Golang provides a method called `ToString()` that converts a byte slice to a string. This method takes a byte slice as input and returns its corresponding string representation. Here’s an example:
“`go
byteSlice := []byte{72, 101, 108, 108, 111}
str := string(byteSlice)
fmt.Println(str) // Output: Hello
“`
4. Converting Byte to String with Custom Implementations:
If the built-in functions and packages do not meet your specific requirements, you can create custom implementations to convert bytes to strings. This may involve iterating over the byte slice and manually converting each byte to its string representation. Here’s an example:
“`go
byteSlice := []byte{65, 66, 67}
var str string
for _, b := range byteSlice {
str += string(b)
}
fmt.Println(str) // Output: ABC
“`
Performance Considerations when Converting Byte to String:
When converting a large number of bytes to strings, performance can become a crucial factor. In such cases, using the `bytes.Buffer` type can offer better performance compared to concatenating strings using the `+` operator. The `bytes.Buffer` provides a more efficient way to build a string from a byte slice. Here’s an example:
“`go
byteSlice := []byte{103, 111, 108, 97, 110, 103}
var buffer bytes.Buffer
for _, b := range byteSlice {
buffer.WriteString(string(b))
}
str := buffer.String()
fmt.Println(str) // Output: Golang
“`
Frequently Asked Questions (FAQs):
1. How do I convert a byte array to a string in Golang?
To convert a byte array to a string in Golang, you can use the `string()` function or the `bytes.ToString()` method from the `bytes` package, as explained in the previous sections.
2. How can I convert a string to a byte array in Golang with UTF-8 encoding?
To convert a string to a byte array with UTF-8 encoding in Golang, you can use the `[]byte()` type conversion, which converts a string to a byte array. Here’s an example:
“`go
str := “Hello”
byteArray := []byte(str)
fmt.Println(byteArray) // Output: [72 101 108 108 111]
“`
3. How can I convert a byte to JSON in Golang?
To convert a byte to JSON in Golang, you can first convert the byte to a string and then use the `json.Marshal()` function to encode the string as JSON. Here’s an example:
“`go
byteSlice := []byte{123, 34, 110, 97, 109, 101, 34, 58, 34, 71, 111, 108, 97, 110, 103, 34, 125}
str := string(byteSlice)
jsonData, err := json.Marshal(str)
if err != nil {
fmt.Println(err)
} else {
fmt.Println(string(jsonData)) // Output: “Golang”
}
“`
4. How can I convert a byte to an integer in Golang?
To convert a byte to an integer in Golang, you can use type conversion or the `binary.BigEndian.Uint32()` function from the `encoding/binary` package. Here’s an example using type conversion:
“`go
b := byte(65)
num := int(b)
fmt.Println(num) // Output: 65
“`
5. How can I convert a Golang buffer to a string?
To convert a Golang buffer to a string, you can use the `String()` method of the `bytes.Buffer` type. Here’s an example:
“`go
var buffer bytes.Buffer
buffer.WriteString(“Hello”)
str := buffer.String()
fmt.Println(str) // Output: Hello
“`
6. How can I convert a byte to hexadecimal in Golang?
To convert a byte to hexadecimal in Golang, you can use the `%02x` format specifier with the `fmt.Sprintf()` function. Here’s an example:
“`go
b := byte(10)
hexStr := fmt.Sprintf(“%02x”, b)
fmt.Println(hexStr) // Output: 0a
“`
In conclusion, converting byte to string in Golang can be done using various methods, from built-in functions and packages to custom implementations. Depending on performance requirements, developers can choose the most suitable approach. Additionally, Golang provides convenient ways to convert byte to JSON, string to byte, string to byte array (UTF-8), byte to int, byte to string base64, Golang buffer to string, and byte to hex.
Strings (Slice Of Bytes) – Beginner Friendly Golang
Keywords searched by users: golang byte to string Golang byte to JSON, String to byte Golang, Golang string to byte array utf8, Byte to string, Byte to string base64 golang, Byte to int golang, Golang Buffer to string, Byte to hex golang
Categories: Top 90 Golang Byte To String
See more here: nhanvietluanvan.com
Golang Byte To Json
Introduction
The Go programming language (Golang) provides a powerful standard library for handling JSON data. Converting bytes to JSON is a common task when dealing with network communication or file handling. In this article, we will explore various techniques to convert bytes to JSON and manipulate the resulting data. So, let’s dive into the world of Golang byte to JSON conversion and learn how to handle it effectively.
Understanding JSON in Golang
JSON (JavaScript Object Notation) is a lightweight data interchange format widely used for transmitting structured data between a server and web application. Since JSON is text-based, it needs to be converted to a byte representation when transmitting or storing it. Golang provides built-in support for parsing JSON data through the “encoding/json” package in its standard library.
Converting Bytes to JSON in Golang
To convert bytes to JSON in Golang, we first need to unmarshal the byte array into a suitable data structure. The “encoding/json” package provides the Unmarshal function for this purpose. Consider the following code snippet:
“`
package main
import (
“encoding/json”
“fmt”
)
type Person struct {
Name string `json:”name”`
Age int `json:”age”`
}
func main() {
jsonBytes := []byte(`{“name”:”John Doe”,”age”:30}`)
var person Person
err := json.Unmarshal(jsonBytes, &person)
if err != nil {
fmt.Println(“Error:”, err)
return
}
fmt.Println(“Name:”, person.Name)
fmt.Println(“Age:”, person.Age)
}
“`
In the above example, we define a struct type “Person” to match the JSON structure. We create a byte array “jsonBytes” with some JSON content. After declaring a variable of type “Person”, we use the Unmarshal function to convert bytes to JSON and store the result in the “person” variable. Finally, we can access the individual fields of “person” to manipulate or display the data.
Handling Unknown JSON Structures
In some cases, we may not know the structure of the JSON data in advance and need a flexible approach to handle it. Golang provides the “json.RawMessage” type to unmarshal unknown JSON data into a byte array. Consider the following example:
“`
package main
import (
“encoding/json”
“fmt”
)
func main() {
jsonBytes := []byte(`{“name”:”John Doe”,”age”:30}`)
var jsonData json.RawMessage
err := json.Unmarshal(jsonBytes, &jsonData)
if err != nil {
fmt.Println(“Error:”, err)
return
}
var result map[string]interface{}
err = json.Unmarshal(jsonData, &result)
if err != nil {
fmt.Println(“Error:”, err)
return
}
fmt.Println(“Name:”, result[“name”])
fmt.Println(“Age:”, result[“age”])
}
“`
In the above example, we use the “json.RawMessage” type to store the JSON data as raw bytes. After unmarshaling the byte array, we can further process it by unmarshaling it into a map[string]interface{}. This approach allows us to extract values from unknown JSON structures by accessing them using their respective keys.
Frequently Asked Questions
Q1. What happens if the JSON structure doesn’t match the defined struct type during unmarshaling?
When unmarshaling JSON data into a struct, if the structure doesn’t match, Go will skip the field or assign the zero value to it. No error will be thrown for mismatched fields.
Q2. How can I handle optional fields in JSON while converting bytes to JSON in Golang?
To handle optional fields in JSON, you can use pointers as struct fields. If the JSON field is present, the pointer will be assigned the corresponding value; otherwise, it will be set to nil.
Q3. Can I convert JSON to bytes in Golang?
Yes, Golang provides the Marshal function in the “encoding/json” package to convert JSON data to bytes. It takes a value as input and returns its byte representation.
Q4. Is there a way to pretty-print JSON in Golang?
Yes, you can pretty-print JSON in Golang by using the MarshalIndent function from the “encoding/json” package. It accepts an additional parameter for specifying the indentation prefix and returns a formatted byte array.
Q5. Are there any libraries to simplify JSON handling in Golang?
Apart from the standard “encoding/json” package, there are several third-party libraries available for working with JSON in Golang, such as “jsoniter” and “easyjson”. These libraries provide additional features, improved performance, and ease of use compared to the standard library.
Conclusion
Converting bytes to JSON in Golang is a fundamental operation when dealing with network communication or file handling. By utilizing the “encoding/json” package, Go provides a robust way to parse and manipulate JSON data efficiently. Understanding the concepts discussed in this article will help you handle JSON data effectively and build robust applications in Golang.
String To Byte Golang
Converting a string to bytes in Golang revolves around understanding the underlying byte representation of the string. In Go, a string is a sequence of immutable bytes, making it easier to convert a string to bytes and vice versa compared to other programming languages. The byte type represents a uint8 value, which can hold any 8-bit number from 0 to 255.
There are several approaches to convert a string to bytes in Golang. Let’s explore some of them:
Method 1: Using the []byte Conversion
The simple and direct method to convert a string to bytes is by using the built-in conversion function []byte. This function returns a new byte slice containing a copy of the original string as bytes.
“`go
str := “Hello, World!”
bytes := []byte(str)
“`
In this method, the string “Hello, World!” is converted to a byte slice named `bytes`. Each character in the string is converted to its corresponding byte representation according to the UTF-8 encoding.
Method 2: Using the string and byte Conversion
Golang provides built-in functions to convert between characters (runes) and bytes using the `string` and `byte` conversion functions respectively. By iterating over each character in the string, we can extract its byte value and store it in a byte slice.
“`go
str := “Hello, World!”
bytes := make([]byte, len(str))
for i, ch := range str {
bytes[i] = byte(ch)
}
“`
In this method, the `make()` function is used to create a byte slice of the same length as the input string. By iterating over each Unicode character in the string, we extract the byte value using the byte conversion function.
Method 3: Using the Encodings Package
Golang’s standard library provides a dedicated package named `encoding` that includes various functionalities to encode and decode data in different formats, including strings and bytes. The `encoding` package has support for a wide range of encodings such as base64, JSON, hexadecimal, and more.
To convert a string to bytes using the `encoding` package, we can utilize the `ASCII` and `UTF-8` encodings.
“`go
import (
“fmt”
“golang.org/x/text/encoding”
“golang.org/x/text/encoding/charmap”
“golang.org/x/text/encoding/unicode”
)
func convertStringToBytes(s string, enc encoding.Encoding) ([]byte, error) {
b, err := enc.NewEncoder().Bytes([]byte(s))
if err != nil {
return nil, err
}
return b, nil
}
str := “Hello, World!”
bytes, err := convertStringToBytes(str, unicode.UTF8)
if err != nil {
fmt.Println(“Error:”, err)
return
}
“`
In this method, we create a function `convertStringToBytes` that takes a string and the desired encoding as input parameters. By utilizing the `encoding` package, we encode the input string into bytes using the specified encoding.
FAQs:
Q1: Are there any other encoding formats supported by Golang to convert a string to bytes?
A1: Yes, Golang’s `encoding` package supports various other encoding formats such as base64, JSON, hexadecimal, UTF-16, and more. You can choose the appropriate encoding based on your specific requirements.
Q2: Can we convert a byte slice back to a string in Golang?
A2: Yes, Golang provides a simple mechanism to convert a byte slice back to a string using the `string()` conversion function.
Q3: What happens when a non-UTF-8 string is converted to bytes?
A3: When converting a non-UTF-8 string to bytes, Golang will perform the conversion based on the underlying byte representation of the string. However, it’s important to note that manipulating and interpreting non-UTF-8 encoded strings can lead to unexpected behavior and should be used with caution.
Q4: Is there a performance difference between different string to byte conversion methods?
A4: The performance difference between different string to byte conversion methods is generally negligible. However, using the built-in `[]byte` conversion function is often considered the most efficient method.
In conclusion, converting a string to bytes in Golang is a simple process that can be achieved using built-in functions, methods, and the `encoding` package. By understanding the underlying byte representation of a string and leveraging the provided functionalities, developers can efficiently convert strings to bytes and utilize them in various programming scenarios. Happy coding!
Images related to the topic golang byte to string
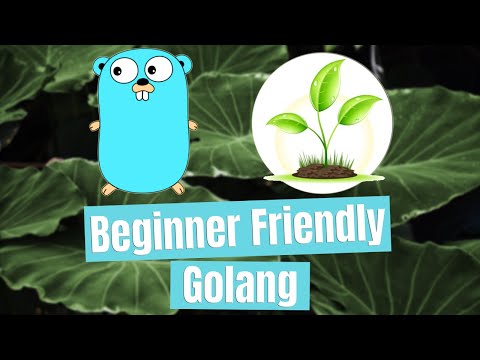
Found 49 images related to golang byte to string theme
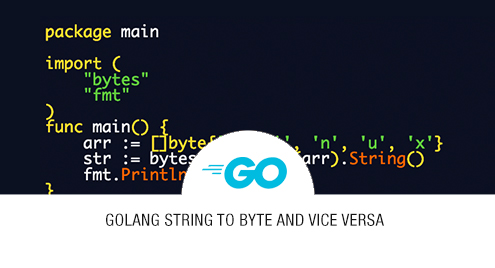
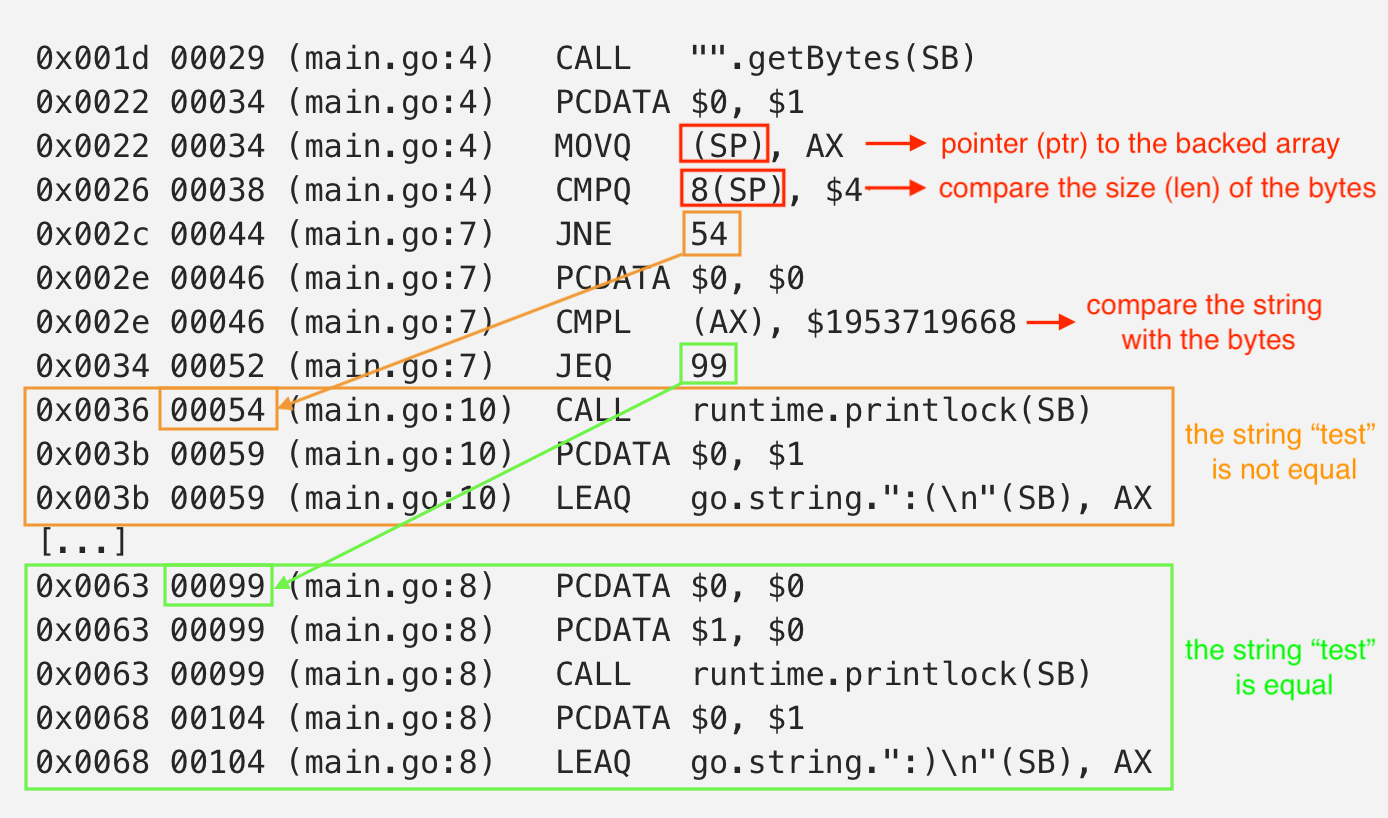

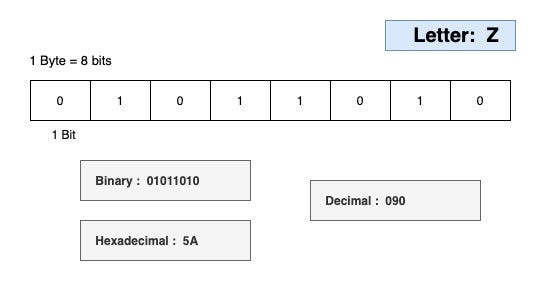
![Debug: []byte/[]uint8/[]rune should be displayed as strings · Issue #1385 · microsoft/vscode-go · GitHub Debug: []Byte/[]Uint8/[]Rune Should Be Displayed As Strings · Issue #1385 · Microsoft/Vscode-Go · Github](https://user-images.githubusercontent.com/63830/33410495-5fa6534e-d535-11e7-80ce-152523ade0cb.png)
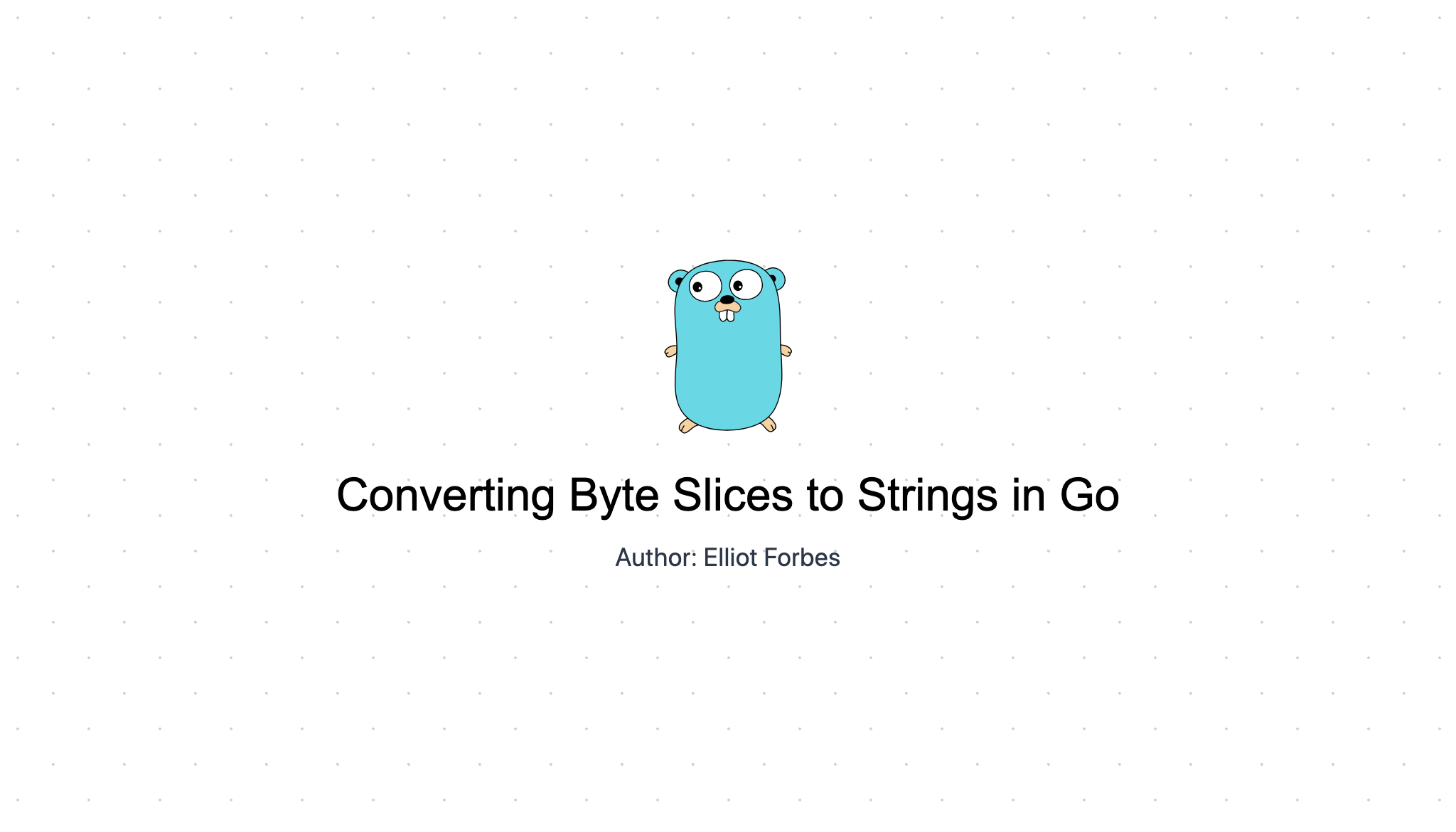

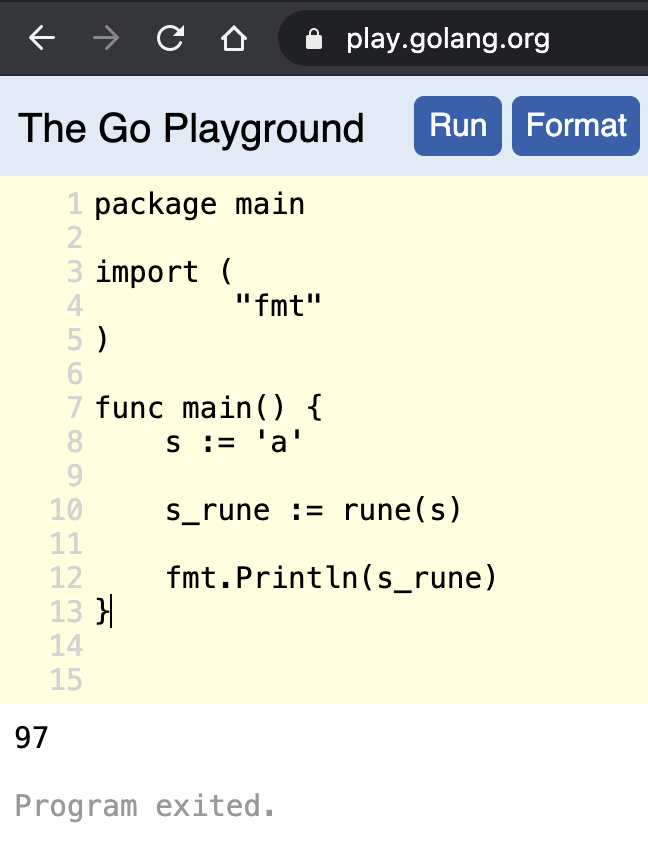
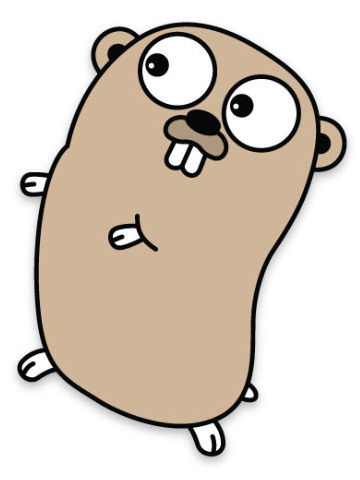
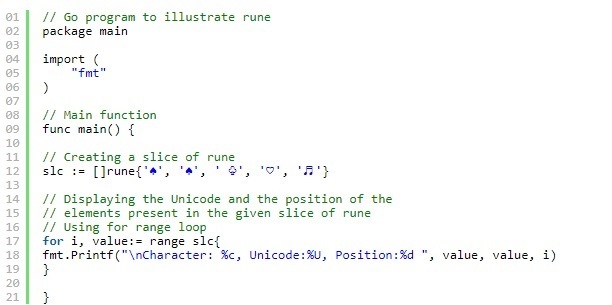



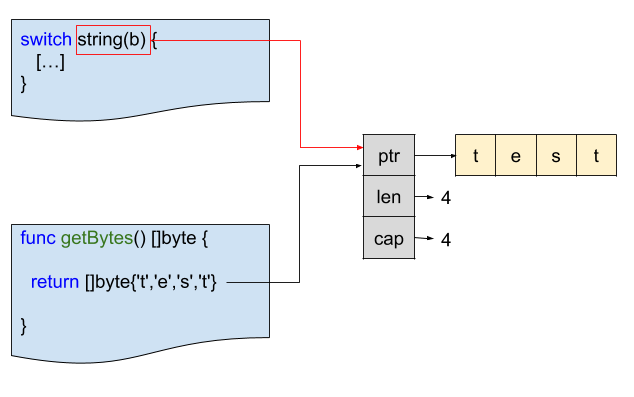
![golang で string を []byte にキャストするとメモリコピーが走ります - Qiita Golang で String を []Byte にキャストするとメモリコピーが走ります - Qiita](https://qiita-user-contents.imgix.net/https%3A%2F%2Fcdn.qiita.com%2Fassets%2Fpublic%2Farticle-ogp-background-9f5428127621718a910c8b63951390ad.png?ixlib=rb-4.0.0&w=1200&mark64=aHR0cHM6Ly9xaWl0YS11c2VyLWNvbnRlbnRzLmltZ2l4Lm5ldC9-dGV4dD9peGxpYj1yYi00LjAuMCZ3PTkxNiZ0eHQ9Z29sYW5nJTIwJUUzJTgxJUE3JTIwc3RyaW5nJTIwJUUzJTgyJTkyJTIwJTVCJTVEYnl0ZSUyMCVFMyU4MSVBQiVFMyU4MiVBRCVFMyU4MyVBMyVFMyU4MiVCOSVFMyU4MyU4OCVFMyU4MSU5OSVFMyU4MiU4QiVFMyU4MSVBOCVFMyU4MyVBMSVFMyU4MyVBMiVFMyU4MyVBQSVFMyU4MiVCMyVFMyU4MyU5NCVFMyU4MyVCQyVFMyU4MSU4QyVFOCVCNSVCMCVFMyU4MiU4QSVFMyU4MSVCRSVFMyU4MSU5OSZ0eHQtY29sb3I9JTIzMjEyMTIxJnR4dC1mb250PUhpcmFnaW5vJTIwU2FucyUyMFc2JnR4dC1zaXplPTU2JnR4dC1jbGlwPWVsbGlwc2lzJnR4dC1hbGlnbj1sZWZ0JTJDdG9wJnM9ODg5MDRhNzIzN2VlMTQ1NDU0MzNmMDMzMjczZTA5MzY&mark-x=142&mark-y=112&blend64=aHR0cHM6Ly9xaWl0YS11c2VyLWNvbnRlbnRzLmltZ2l4Lm5ldC9-dGV4dD9peGxpYj1yYi00LjAuMCZ3PTYxNiZ0eHQ9JTQwaWthd2FoYSZ0eHQtY29sb3I9JTIzMjEyMTIxJnR4dC1mb250PUhpcmFnaW5vJTIwU2FucyUyMFc2JnR4dC1zaXplPTM2JnR4dC1hbGlnbj1sZWZ0JTJDdG9wJnM9OWE3YzM5MmVjNjc1NTYzMWI0ZDcyOTkwODkwYjJjNTk&blend-x=142&blend-y=491&blend-mode=normal&s=0f4bd84e3345657ca6aff8c2ce7f47e2)
![Javarevisited: 2 Examples to Convert Byte[] Array to String in Java Javarevisited: 2 Examples To Convert Byte[] Array To String In Java](https://1.bp.blogspot.com/-8J4Yz4LWYPs/U_yr6-loLnI/AAAAAAAABzg/HBGWbD6A7Vo/w1200-h630-p-k-no-nu/Character%2BEncoding%2C%2BConverting%2BByte%2Barray%2Bto%2BString%2Bin%2BJava.png)

![Golang中[]byte与string转换全解析- 知乎 Golang中[]Byte与String转换全解析- 知乎](https://pic1.zhimg.com/v2-d9c505e50cffbb4b4caddf1302e84514_720w.jpg?source=172ae18b)
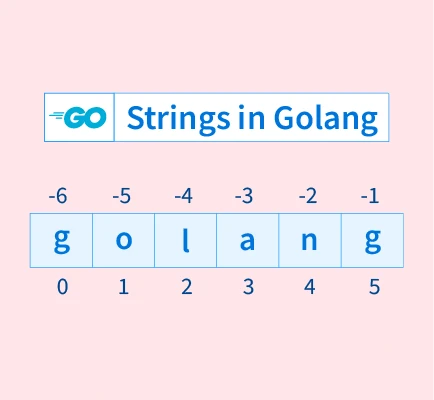
![Convert map[string]interface{}) to []byte In Go - YouTube Convert Map[String]Interface{}) To []Byte In Go - Youtube](https://i.ytimg.com/vi/i4I4la437AI/maxres2.jpg?sqp=-oaymwEoCIAKENAF8quKqQMcGADwAQH4AbYIgAKAD4oCDAgAEAEYWyBjKGUwDw==&rs=AOn4CLANut5MzuNKZ3TZixh0SIQdIoXOgA)

![Golang string []byte 高性能转换- Zhh Blog Golang String []Byte 高性能转换- Zhh Blog](https://www.huihongcloud.com/2020/06/17/go/Golang%20zero%20copy%20string%20bytes%20fast%20conversion/3888359.png)
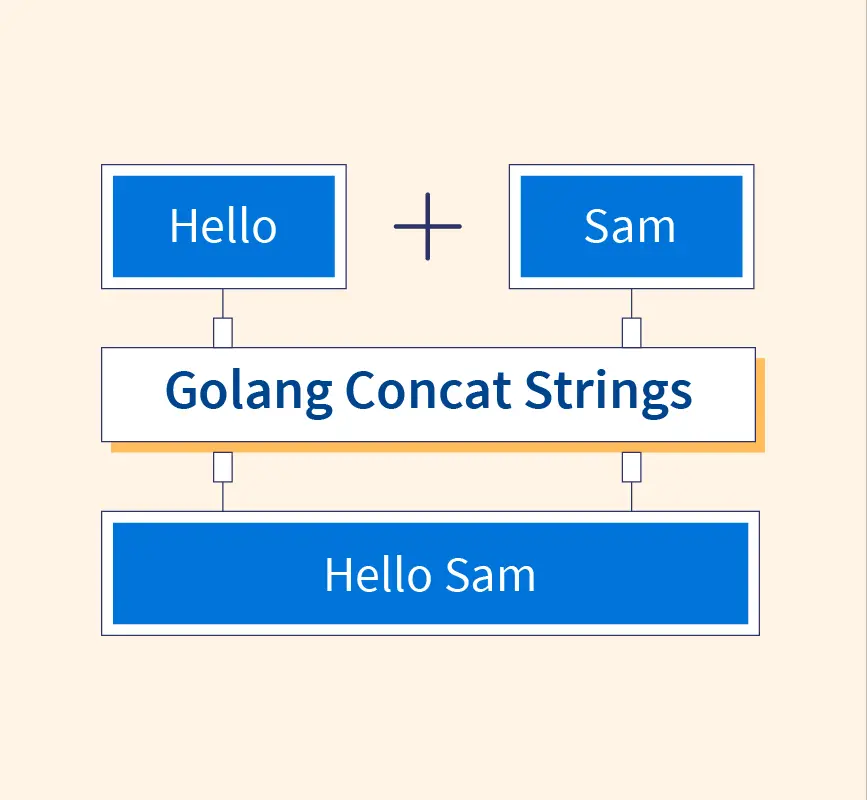
![Go 基础】[]byte 与string 的互转有新方法啦- 知乎 Go 基础】[]Byte 与String 的互转有新方法啦- 知乎](https://picx.zhimg.com/v2-9449e01d671afc1835038f9606ddab51_720w.jpg?source=172ae18b)
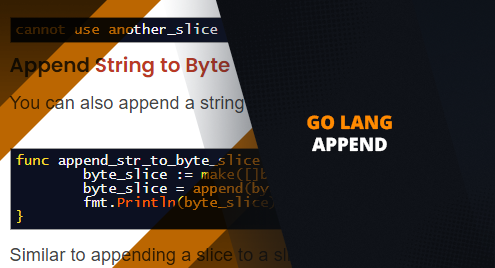
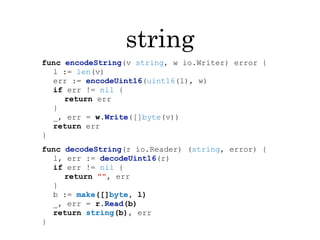


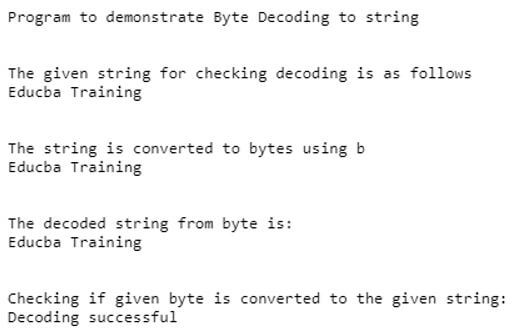

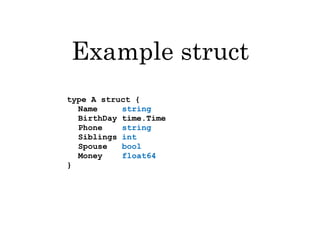
![Golang string []byte 高性能转换- Zhh Blog Golang String []Byte 高性能转换- Zhh Blog](https://www.huihongcloud.com/2020/06/17/go/Golang%20zero%20copy%20string%20bytes%20fast%20conversion/5759234.png)
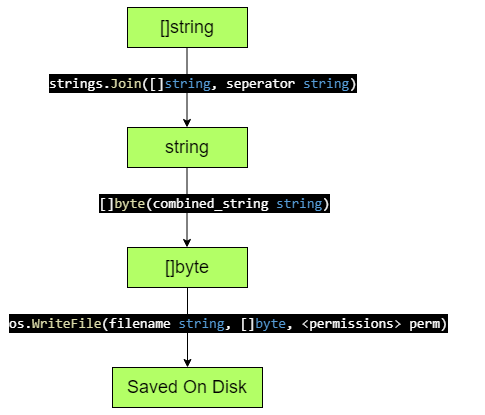
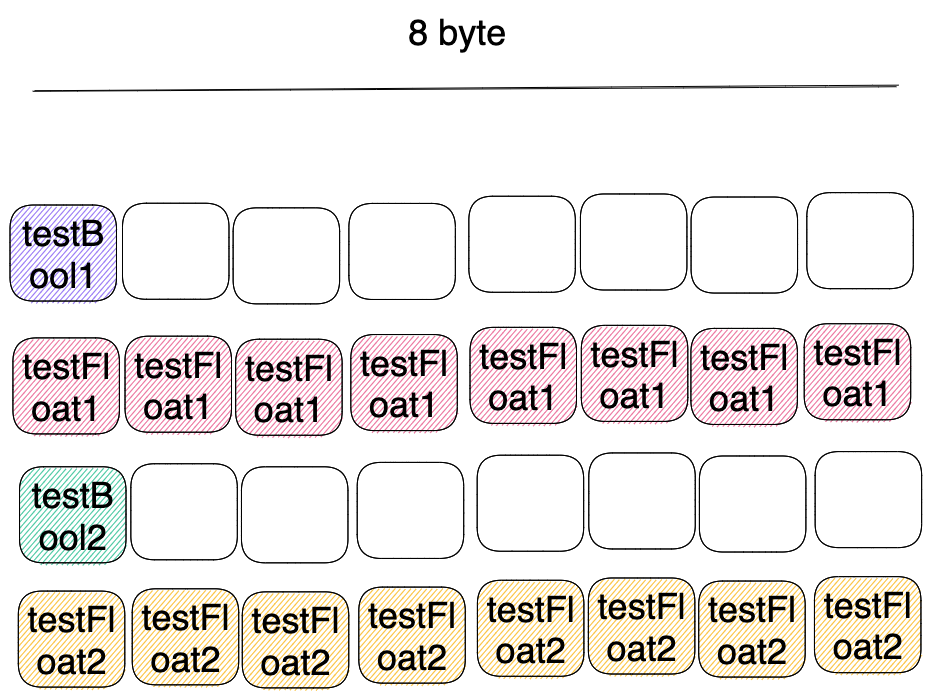
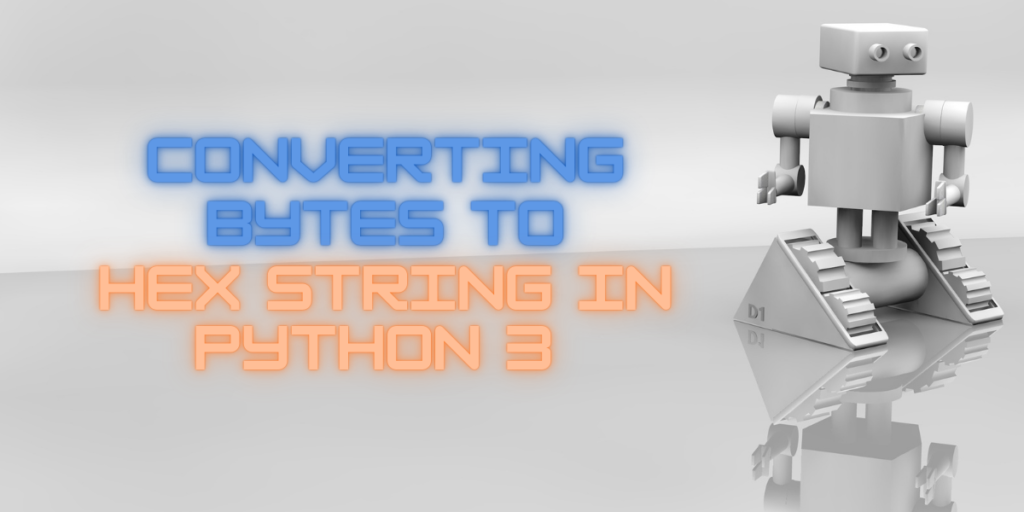
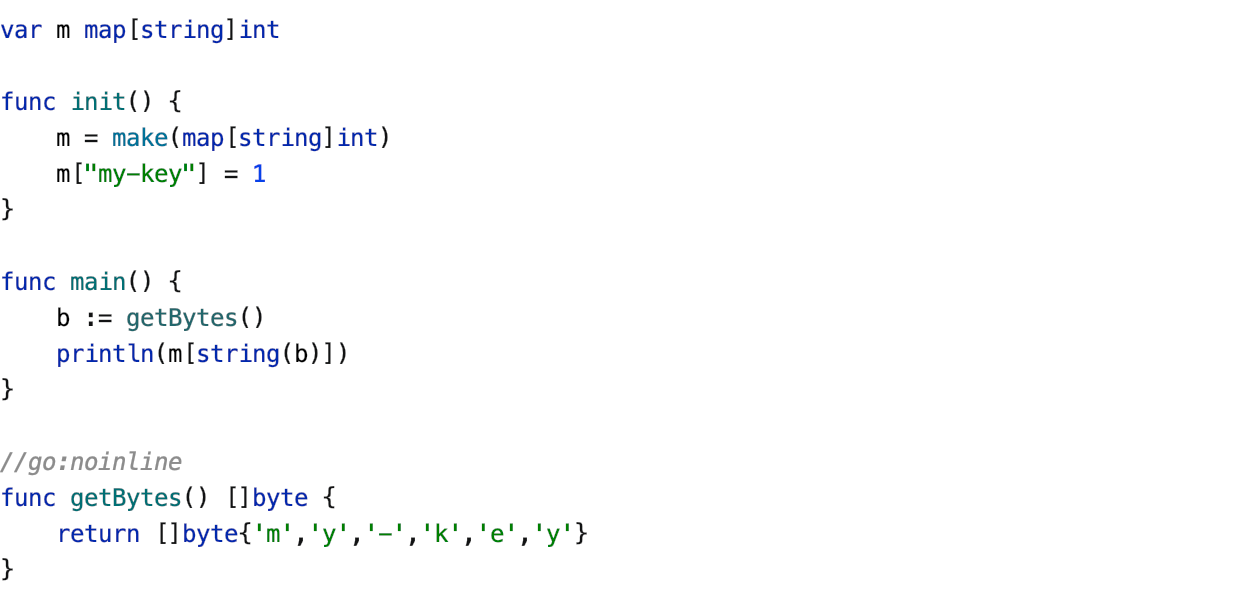


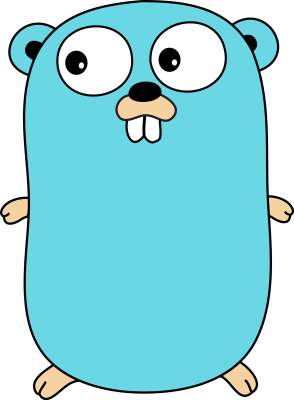
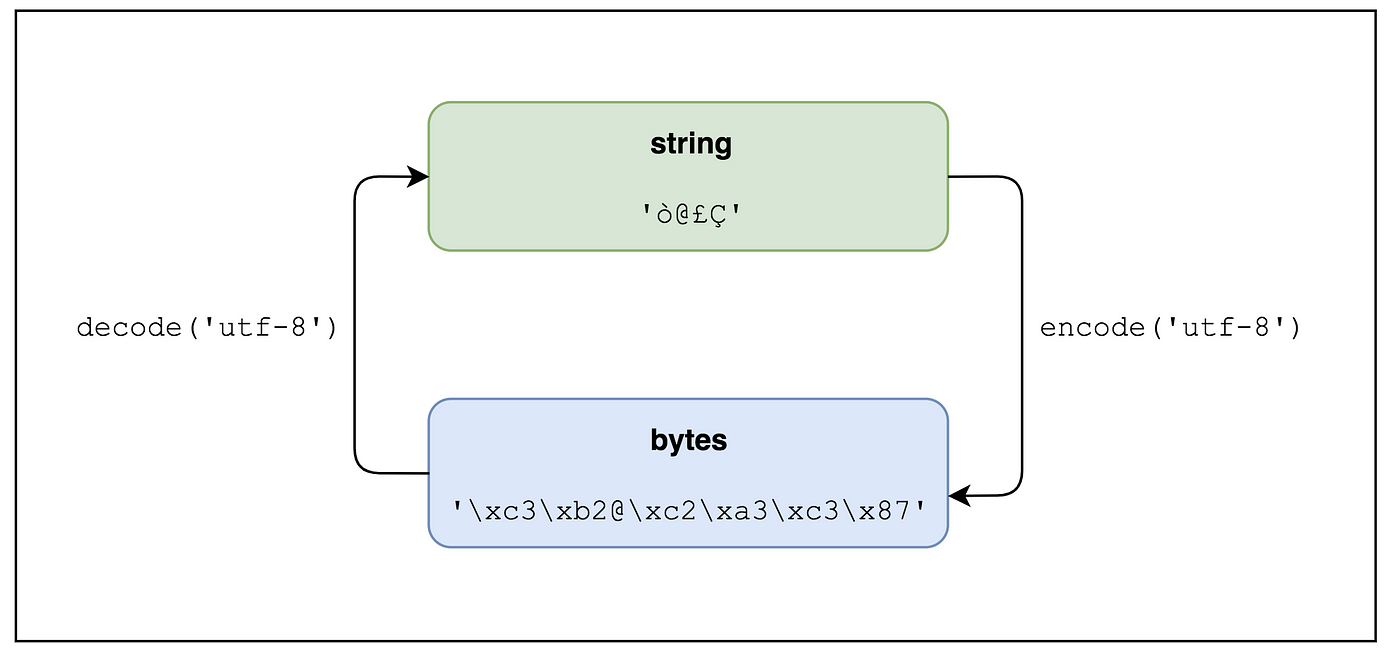
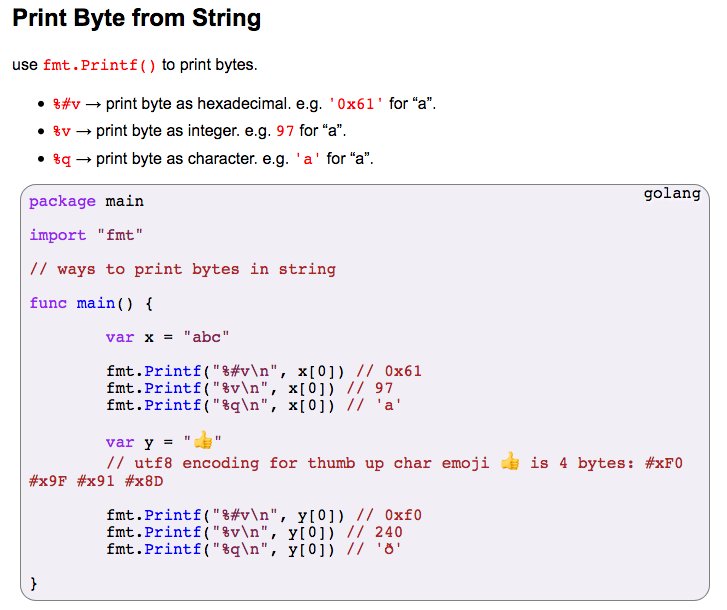

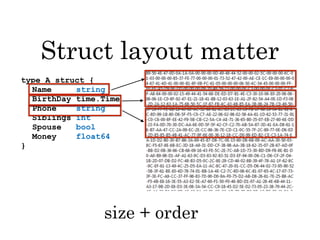
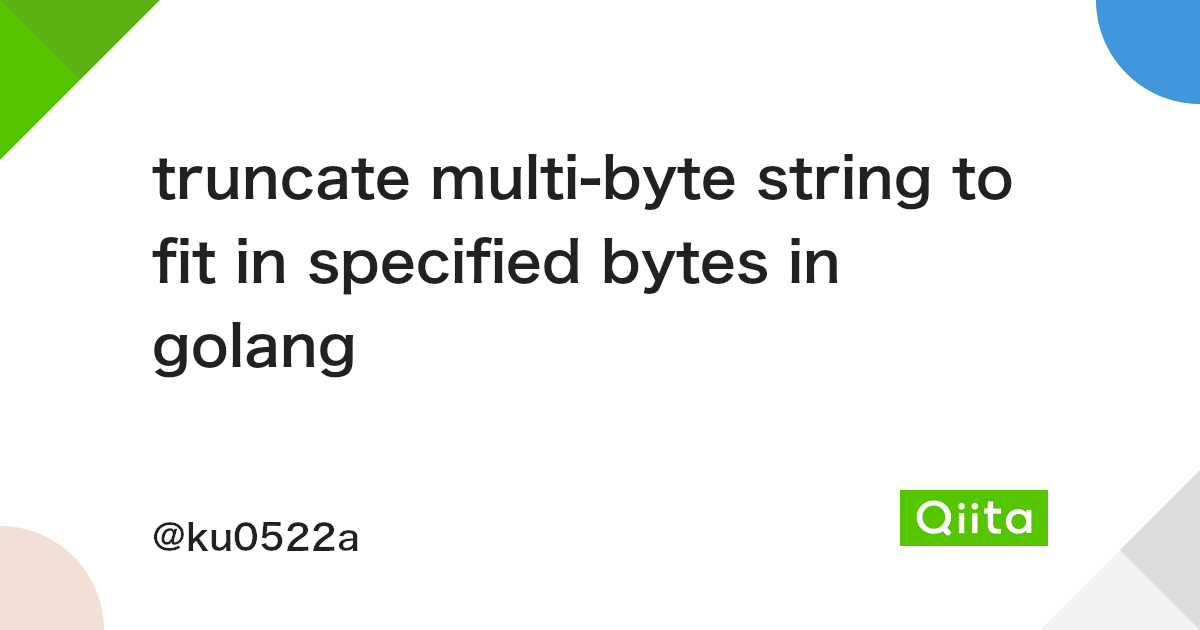
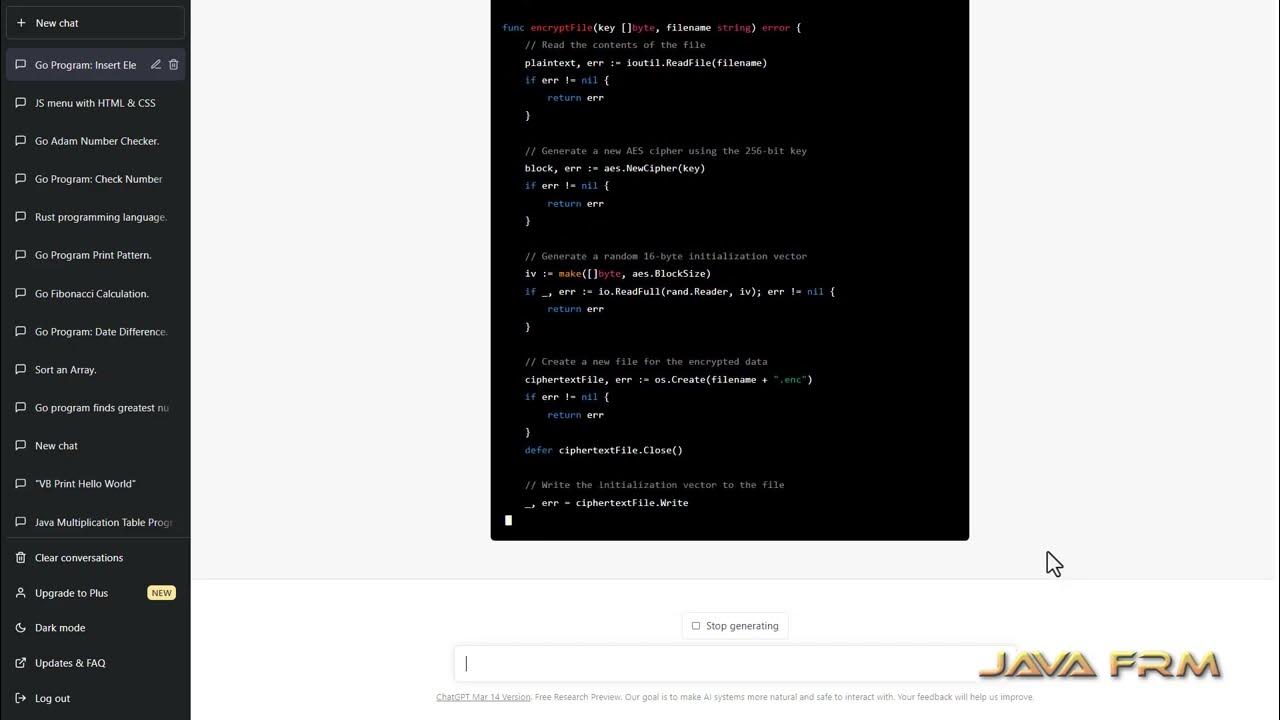
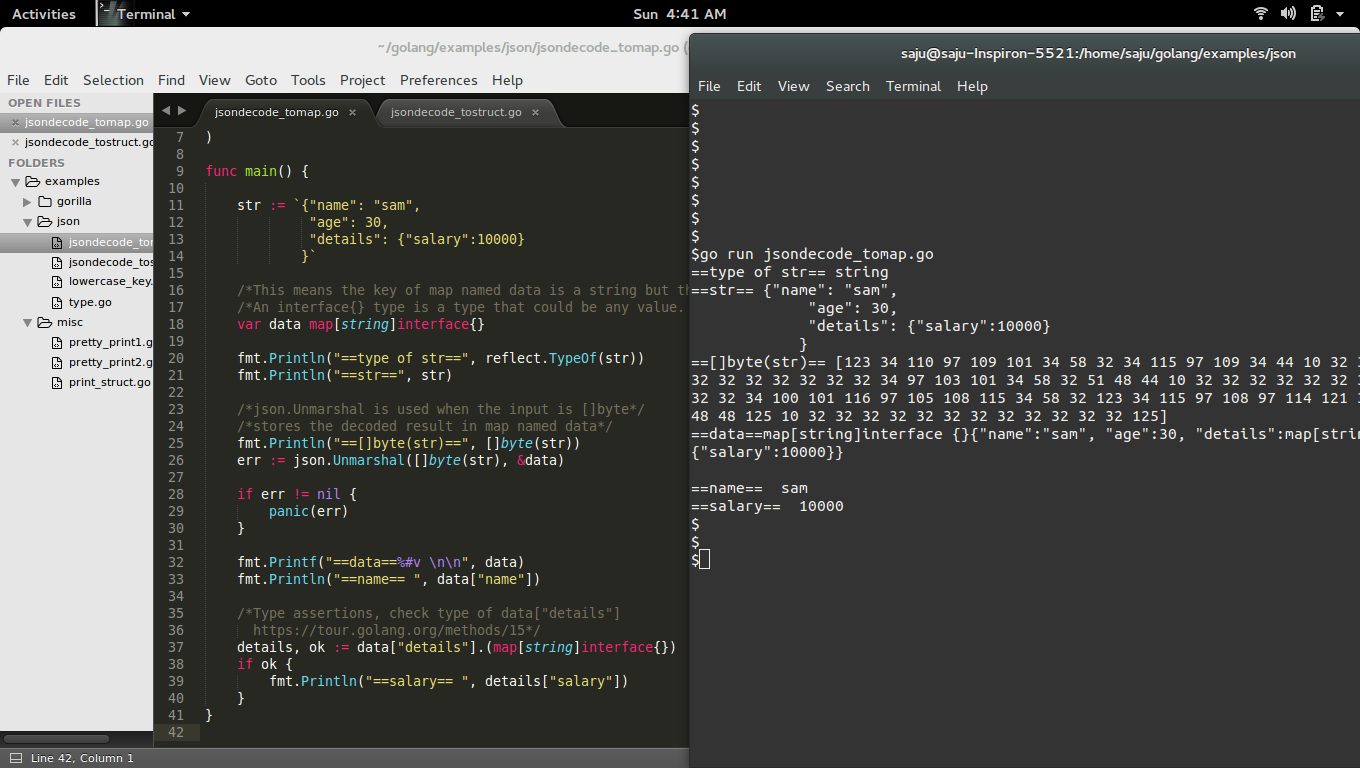
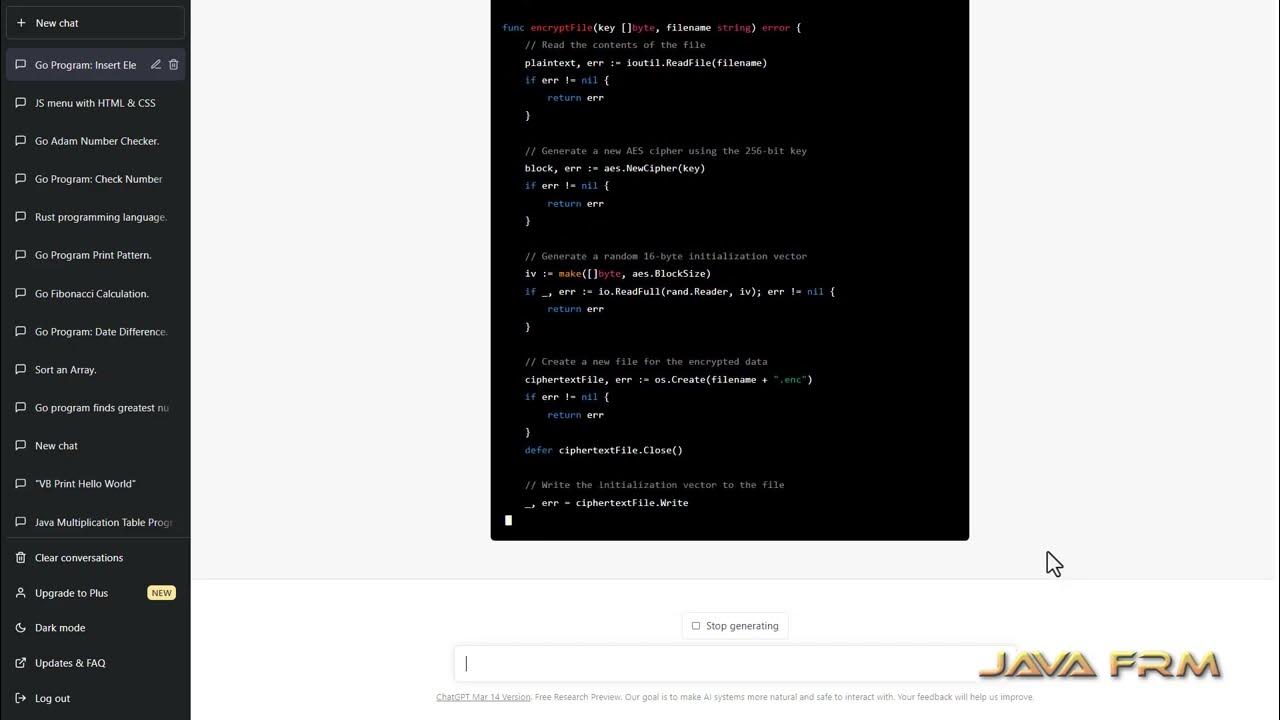
Article link: golang byte to string.
Learn more about the topic golang byte to string.
- How to convert byte array to string in Go – Stack Overflow
- Golang Byte Array to String
- How to Convert Byte Array to String in Golang – AskGolang
- Convert between byte array/slice and string · YourBasic Go
- Golang Byte to String – Tutorial Gateway
- Go Convert Byte Array to String – GeekBits
- Convert string to []byte or []byte to string in Go (Golang)
- Golang String to Byte and Vice Versa – Linux Hint
See more: nhanvietluanvan.com/luat-hoc