Golang Boolean To String
Introduction:
In Golang, boolean variables are used to represent binary values, typically denoting “true” or “false.” Understanding how to manipulate and convert these boolean values from their native boolean form to string format is a fundamental aspect of Golang programming. This article aims to provide an in-depth understanding of boolean variables in Golang and explore various methods for converting boolean to string.
Understanding boolean variables in Golang:
Boolean variables in Golang are used to store logical values, which can only be either true or false. They serve a crucial role in controlling the flow of programs and making decisions based on conditions. Boolean variables are primarily used in conditions such as if statements, loops, and logical operations.
How boolean variables are represented in Golang:
In Golang, boolean variables are represented using the `bool` data type. The `bool` data type can only have two possible values: `true` or `false`. For example, you can declare a boolean variable as follows:
“`go
var isTrue bool = true
var isFalse bool = false
“`
Converting boolean to string in Golang:
There are multiple ways to convert a boolean variable to its string representation in Golang. Let’s explore some of the commonly used approaches.
1. Using `strconv.FormatBool()` function:
The `strconv` package in Golang provides a convenient function called `FormatBool()` that converts a boolean value to its string representation. This function takes a boolean parameter and returns the corresponding string value.
“`go
import (
“strconv”
)
func main() {
isTrue := true
str := strconv.FormatBool(isTrue)
fmt.Println(str) // Output: “true”
}
“`
Using `strconv.FormatBool()` function is one of the easiest and most recommended ways to convert a boolean to its string representation. It ensures consistent and reliable conversions.
2. Using boolean string constants:
Golang provides built-in string constants that represent boolean values. These constants are `true` and `false`. You can directly assign them to a string variable for conversion purposes.
“`go
var str string
isTrue := true
str = fmt.Sprintf(“%t”, isTrue)
isFalse := false
str = fmt.Sprintf(“%t”, isFalse)
“`
Using boolean string constants provides a simple and straightforward approach for converting boolean to string. However, it lacks flexibility in case you want to customize the string representation.
3. Custom conversion using if-else statements:
Another way to convert a boolean to string in Golang is by using if-else statements to manually check the boolean value and assign the corresponding string representation.
“`go
var str string
isTrue := true
if isTrue {
str = “true”
} else {
str = “false”
}
“`
This approach allows you to define custom string representations for true and false cases. However, it can become cumbersome and less readable when dealing with multiple boolean variables.
Using strconv.FormatBool() function:
The `strconv.FormatBool()` function is a powerful tool for converting boolean variables to their string representation. It accepts a boolean value as input and returns the corresponding string representation.
“`go
isTrue := true
str := strconv.FormatBool(isTrue)
“`
Benefits of using `strconv.FormatBool()`:
– Provides a standardized conversion method for boolean to string
– Handles various edge cases and error scenarios gracefully
– Ensures consistency and reliability in string representations
Considerations when using `strconv.FormatBool()`:
– Ensure proper import of the `strconv` package
– Carefully handle the returned string value according to your application requirements
Using boolean string constants:
Golang offers the boolean string constants `true` and `false`, which can be directly used for conversion purposes. These constants are automatically converted to their respective string representations.
Introduction to boolean string constants in Golang:
Golang includes the boolean string constants “true” and “false” which directly represent the boolean values.
Available boolean string constants in Golang:
– `true`: The constant “true” represents the boolean value `true`.
– `false`: The constant “false” represents the boolean value `false`.
Pros of using boolean string constants:
– Simple and straightforward approach
– No additional libraries or functions required
Cons of using boolean string constants:
– Limited flexibility in customizing string representations
– Inability to handle non-boolean values
Custom conversion with if-else statements:
Custom conversion allows you to define your own logic for converting boolean variables to strings. By using if-else statements, you can handle true and false cases separately and assign the desired string representation.
Writing custom code to convert boolean to string using if-else statements:
“`go
var str string
isTrue := true
if isTrue {
str = “true”
} else {
str = “false”
}
“`
When using custom conversion methods, you gain complete control over the string representation. However, if you have multiple boolean variables, the code can become repetitive and less maintainable compared to other conversion approaches.
Handling invalid boolean values:
While converting boolean to string, it is essential to handle situations where the boolean value is not strictly true or false. If an invalid boolean value is encountered, an error or exception should be handled gracefully.
Common errors and exceptions while converting invalid boolean values:
– Invalid boolean values can include nil pointers or non-boolean types.
– Non-bool values passed to boolean conversion functions can result in unexpected behavior or errors.
Best practices for error handling and validation in boolean to string conversion:
– Ensure proper type checking and validation before conversion to avoid errors.
– Implement appropriate error handling strategies such as returning an error value or logging errors.
Performance considerations:
When working with large datasets or performance-critical applications, it is crucial to evaluate the performance impact of different boolean to string conversion methods.
Benchmarking the conversion approaches:
– Perform benchmarks to measure the execution time of different conversion approaches.
– Compare the performance of `strconv.FormatBool()` with custom conversion methods.
Optimizations and trade-offs for better performance:
– Use `strconv.FormatBool()` for optimal performance and reliable conversions.
– Avoid excessive if-else statements or nested conditions for custom conversion.
Use cases and practical applications:
Boolean to string conversion is frequently used in real-world scenarios, such as:
– Displaying boolean values in user interfaces
– Logging boolean variables
– Serializing boolean data for storage or transmission
Understanding boolean to string conversion is crucial for proper implementation and handling of boolean values in Golang.
In conclusion, converting boolean variables to their string representation is an essential task in Golang programming. This article has provided a comprehensive understanding of boolean variables in Golang and explored various methods for converting boolean to string. By using the `strconv.FormatBool()` function, boolean string constants, or custom conversion with if-else statements, you can effectively handle boolean to string conversion in your Golang projects.
Hands-On With Go: Converting Boolean To String |Packtpub.Com
How To Convert Bool To String In Golang?
In Go (Golang), data types play a vital role in representing the various kinds of values a program can store and manipulate. Among the commonly used data types, the boolean type is frequently employed for evaluating conditions and making decisions. However, there might be situations where you need to convert a boolean value to a string in order to perform certain operations or display the value in a specific format. This article will guide you through the process of converting a bool value to a string in Go, providing detailed explanations and examples to help you grasp the concept effectively.
Converting Bool to String
To convert a boolean value to a string in Golang, you can make use of the “strconv” package. This package offers a variety of functions to convert values between different data types, including booleans and strings. The “strconv” package provides the strconv.FormatBool function, specifically designed for converting boolean values to their corresponding string representation.
Here’s some sample code that demonstrates how to convert a bool value to a string:
“`go
package main
import (
“fmt”
“strconv”
)
func main() {
booleanValue := true
stringValue := strconv.FormatBool(booleanValue)
fmt.Println(“Boolean value as a string:”, stringValue)
}
“`
In the example above, we define a variable called “booleanValue” and assign it the value “true”. We then use the strconv.FormatBool function to convert this boolean value to its string representation and store it in the “stringValue” variable. Finally, we print the string value using the fmt.Println function to verify the conversion.
Output:
“`
Boolean value as a string: true
“`
As you can see, the boolean value “true” has been successfully converted to the string “true”. Similarly, you can convert a boolean value of “false” to the string “false” using the strconv.FormatBool function.
It is important to note that when you convert a boolean value to a string, the resulting string will always be in lowercase. Therefore, the output will be “true” or “false”, rather than “True” or “False”. This is the standard convention in Go for representing boolean values as strings.
Frequently Asked Questions (FAQs):
Q: Can I directly convert a bool value to a string without using the strconv package?
A: No, Golang does not provide a direct built-in function to convert a bool value to a string. Hence, you need to make use of the strconv package to achieve the conversion.
Q: What happens if I pass an invalid boolean value to strconv.FormatBool?
A: If you pass an invalid boolean value (i.e., a value other than true or false) to the strconv.FormatBool function, it will panic and throw a runtime error. Therefore, it is crucial to ensure that you only pass valid boolean values to this function.
Q: Can I convert a string representation of a boolean value to a bool type in Golang?
A: Yes, Golang provides the strconv.ParseBool function to convert string representations of boolean values back to a bool type. This function returns the parsed bool value and an error if any parsing errors occur. You can use this function to effectively convert a string back to a bool type.
Q: How can I format the converted string value as uppercase?
A: As mentioned earlier, strconv.FormatBool always returns the converted string value in lowercase. If you specifically need an uppercase representation, you can use the strings.ToUpper function to convert the resulting string to uppercase.
Conclusion:
Converting a boolean value to a string is a common task in Go (Golang), particularly when dealing with output formatting or while passing data to external systems that require values to be represented as strings. In this article, we explored how to convert a bool value to a string using the strconv package’s FormatBool function. By following the examples and explanations provided here, you can now efficiently convert boolean values to strings in your Go programs. Remember to handle potential errors and ensure valid boolean values are passed to the conversion function.
How Can I Convert Boolean To String?
There are several programming languages that support boolean values, such as Java, Python, JavaScript, and C++. Each language may have different ways of converting booleans to strings, so we will discuss a few approaches using a couple of popular languages.
One of the simplest ways to convert a boolean to a string is by using conditional statements. In Java, for example, you can achieve this by writing a simple if-else statement. Let’s say we have a boolean variable named “isTrue” that we want to convert to a string:
“`java
boolean isTrue = true;
String booleanString = isTrue ? “true” : “false”;
“`
In this code snippet, we use a ternary operator to check if the boolean is true or false. If it evaluates to true, the string value “true” is assigned to the “booleanString” variable; otherwise, it assigns the string value “false”. This approach ensures that the resulting string accurately represents the boolean value.
Another method to convert boolean to string is by using the toString() method. Many programming languages provide this method for objects, including booleans. In JavaScript, for instance, you can use this method to convert a boolean to a string directly:
“`javascript
let isTrue = true;
let booleanString = isTrue.toString();
“`
By calling the toString() method on a boolean variable, JavaScript automatically converts the boolean value to its corresponding string representation. It is worth noting that this method is applicable to most programming languages and is generally straightforward to use.
Additionally, some languages offer helper functions or utilities specifically designed for boolean-to-string conversion. In Python, the built-in str() function can convert a boolean to its string representation conveniently:
“`python
is_true = True
boolean_string = str(is_true)
“`
The str() function converts its argument to its string equivalent, making it easy to convert a boolean variable into a string with a single line of code.
Now, let’s address some frequently asked questions about boolean-to-string conversion:
Q: Can I directly concatenate a boolean and a string?
Yes, in many programming languages, you can concatenate a boolean and a string using the concatenation operator (+). This implicitly converts the boolean to a string before appending it to the existing string.
Q: How does boolean-to-string conversion work in programming languages that do not have native boolean data types?
In programming languages that do not have native boolean data types, such as C, boolean values are often represented as integers. In these cases, you can explicitly convert the boolean value to an integer and then convert the integer to a string using the appropriate conversion functions provided by the language.
Q: Are there any language-specific considerations when converting booleans to strings?
Yes, some languages may have specific conventions for representing boolean values as strings. For instance, in Python, the string representation of True and False is “True” and “False” (with an uppercase first letter), while in JavaScript, it is “true” and “false” (with a lowercase first letter). It’s crucial to consult the documentation or guidelines of the programming language you are using to ensure accurate conversion.
In conclusion, converting a boolean to a string is a fundamental task in programming. Whether you choose conditional statements, toString() methods, or dedicated conversion functions, there are various options available to achieve this conversion efficiently. Consider the programming language you are using, its specific conventions, and the requirements of your project to determine the most suitable approach for your needs.
Keywords searched by users: golang boolean to string Golang bool to string, Golang boolean to int, Int to bool golang, Golang slice to string, Convert string to time golang, Golang string to int, Convert string to struct golang, Array to string golang
Categories: Top 23 Golang Boolean To String
See more here: nhanvietluanvan.com
Golang Bool To String
Method 1: Using the strconv package
The strconv package in Go provides a set of functions for converting boolean values to strings. The most commonly used function for this purpose is the FormatBool() function. This function takes a boolean value as a parameter and returns its string representation. Here’s an example:
“`
import (
“fmt”
“strconv”
)
func main() {
b := true
s := strconv.FormatBool(b)
fmt.Println(s)
}
“`
Output:
“`
true
“`
Method 2: Using string concatenation
Another method to convert a boolean value to a string in Go is by using string concatenation. This approach involves concatenating the boolean value with an empty string, which automatically converts it to its string representation. Here’s an example:
“`
import “fmt”
func main() {
b := true
s := “” + fmt.Sprintf(“%v”, b)
fmt.Println(s)
}
“`
Output:
“`
true
“`
It’s worth noting that the fmt.Sprintf() function is used here to format the boolean value as a string before concatenating it.
Method 3: Using if-else statements
You can also use if-else statements to convert a boolean to a string in Go. This method involves checking the boolean value and assigning the corresponding string representation. Here’s an example:
“`
import “fmt”
func boolToString(b bool) string {
if b {
return “true”
}
return “false”
}
func main() {
b := true
s := boolToString(b)
fmt.Println(s)
}
“`
Output:
“`
true
“`
The advantage of this method is that it allows you to customize the string representation as per your requirements.
FAQs:
Q1: Can I convert a string to a boolean in Go?
A1: Yes, you can convert a string to a boolean in Go. The strconv package provides a function named ParseBool(), which takes a string as a parameter and returns its boolean representation. Here’s an example:
“`
import (
“fmt”
“strconv”
)
func main() {
s := “true”
b, _ := strconv.ParseBool(s)
fmt.Println(b)
}
“`
Output:
“`
true
“`
It’s important to note that the second return value of ParseBool() is an error, which you can handle if needed.
Q2: What is the default string representation for a boolean in Go?
A2: In Go, the default string representation for a boolean is “true” for true and “false” for false.
Q3: Are there any other built-in functions or libraries for converting a boolean to a string in Go?
A3: Apart from the strconv package, there are no other built-in functions or libraries specifically for converting a boolean to a string in Go. However, you can always create your own custom functions to handle specific requirements.
In conclusion, converting a boolean value to a string in Go is a straightforward process that can be accomplished using various methods. The choice of method depends on your specific requirements and preferences. Whether you choose to use the strconv package, string concatenation, or if-else statements, the end result remains the same – a convenient way to convert boolean values to their string representation.
Golang Boolean To Int
Converting a boolean value to an integer in Go is a straightforward process. Go provides a built-in type called ‘int’, which represents signed integers. To convert a boolean value to an integer, you simply need to use the ‘true’ value for ‘true’, and ‘false’ for ‘false’.
Let’s take a look at an example:
“`
package main
import (
“fmt”
)
func main() {
var b bool = true
i := 0
if b {
i = 1
}
fmt.Println(i) // Output: 1
}
“`
In this code snippet, we declare a boolean variable ‘b’ and initialize it with the value ‘true’. We also declare an integer variable ‘i’ and initialize it with 0. Using an ‘if’ statement, we check if the boolean variable ‘b’ is ‘true’. If it is, we set the integer variable ‘i’ to 1. Finally, we print the value of ‘i’, which should be 1 in this case.
Converting a boolean value to an integer can be useful in various scenarios in programming. Here are a few common use cases:
1. Conditional assignments: You may need to assign different integer values based on the result of a condition. Using boolean to integer conversion allows you to easily achieve this. For example, if a condition evaluates to ‘true’, you can assign a value of 1, otherwise, a value of 0.
2. Encoding flags: In some cases, you might need to encode a set of flags into a single integer value. Each flag can be represented by a boolean value, and by converting them to integers, you can combine them into a single value for compact storage.
3. Mathematical operations: Although boolean values are not directly compatible with mathematical operations, converting them to integers allows you to perform calculations and mathematical operations involving boolean conditions.
Now, let’s address some frequently asked questions about boolean to integer conversion in Go:
Q: Can I convert any non-zero value to ‘true’ and zero to ‘false’?
A: No, Go only considers ‘0’ as false, and any other non-zero value as true. So, converting non-zero integers to boolean will result in ‘true’, and ‘0’ will be converted to ‘false’.
Q: Can I directly use arithmetic operators on boolean values?
A: No, you cannot directly perform arithmetic operations on boolean values in Go. However, after converting them to integers, you can use arithmetic operators as needed.
Q: Is there any performance impact when converting boolean to integer?
A: Converting a boolean value to an integer in Go is a simple operation and does not have a significant impact on performance. It is an efficient process that can be executed quickly.
Q: Is there any alternative method to convert a boolean value to an integer?
A: Yes, you can also use a ternary operator to achieve the same result. For example: `i = int(boolValue)`, where ‘boolValue’ is a boolean variable.
In conclusion, Go provides a straightforward way to convert boolean values to integers. This feature can be utilized in various programming scenarios such as conditional assignments, encoding flags, and mathematical operations. Understanding the process of boolean to integer conversion in Go is essential for performing these tasks efficiently.
Int To Bool Golang
In the Go programming language, there are often scenarios where you may need to convert an integer (int) to a boolean (bool) value. Understanding how this conversion works and why it is necessary can be crucial in writing efficient and error-free code. In this article, we will explore the int to bool conversion in Golang, discuss its implementation, and address some frequently asked questions.
Understanding the Need for Int to Bool Conversion:
The int to bool conversion is primarily used when you want to evaluate an integer value and determine its truthiness based on a specific condition. While integers and booleans are distinct data types, Golang allows us to convert an int value to bool by following a few rules and conventions.
Implementation of Int to Bool Conversion:
In Golang, true is represented by the value 1, while false is represented by the value 0. Therefore, when converting an int to bool, any non-zero value is considered true, and a zero value is considered false. Golang supports this conversion implicitly, without requiring any explicit casting or function calls.
Here’s an example of converting an int to bool in Golang:
“`go
package main
import “fmt”
func main() {
var i int = 5
b := bool(i) // int to bool conversion
fmt.Println(“Converted bool value:”, b)
}
“`
In this code snippet, we create an int variable `i` with the value 5. We then convert `i` to a bool by assigning `bool(i)` to the variable `b`. Finally, we print the converted bool value, which will be true in this case.
Handling Integers Outside Range:
While any non-zero value is considered true during the int to bool conversion, it is essential to understand that Golang does not perform any range checks. If the integer value falls outside the range of 0 to 1, it may result in unexpected behavior.
For example, consider the following code:
“`go
package main
import “fmt”
func main() {
var i int = 100
b := bool(i) // int to bool conversion
fmt.Println(“Converted bool value:”, b)
}
“`
In this code snippet, we assign `i` with a value of 100. However, as Golang only considers 0 as false and any non-zero value as true, the converted bool value will be true. This can lead to logical errors if the intention was to evaluate the truthiness based on a narrower range. Therefore, it is essential to ensure that the integer values being converted are within the desired range.
FAQs:
1. Can I explicitly cast an int to bool in Golang?
No, Golang does not support explicit casting from int to bool. The conversion is implicit, meaning you can directly assign an int value to a bool variable without any casting functions.
2. What happens if I assign an int value outside the range to a bool variable?
If the int value is non-zero, the bool variable will be true. However, it is important to note that Golang does not perform any range check during the conversion. Therefore, assigning an int value greater than 1 or less than 0 to a bool variable may result in unexpected behavior.
3. How can I check if an int value is within a specific range before converting it to bool?
To check if an int value is within a specific range in Golang, you can simply use conditional statements or comparison operators. For example:
“`go
if i >= 0 && i <= 10 {
b := bool(i) // int to bool conversion within range
} else {
// Handle values outside the desired range
}
```
4. What is the preferred way to convert an int to bool in Golang?
The preferred way is to directly assign the int value to a bool variable. Golang handles the conversion implicitly. For example:
```go
var i int = 5
b := bool(i) // int to bool conversion
```
5. What happens if I assign 0 to a bool variable?
Assigning 0 to a bool variable will result in a false value. In Golang, 0 is considered false during bool evaluation.
To conclude, the int to bool conversion in Golang simplifies the process of evaluating integer values based on specific conditions. By knowing the implicit rules and taking care of the desired range, you can effectively convert an int to bool without the need for explicit casting.
Images related to the topic golang boolean to string
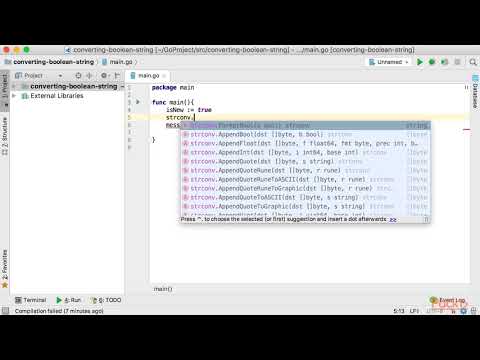
Found 29 images related to golang boolean to string theme
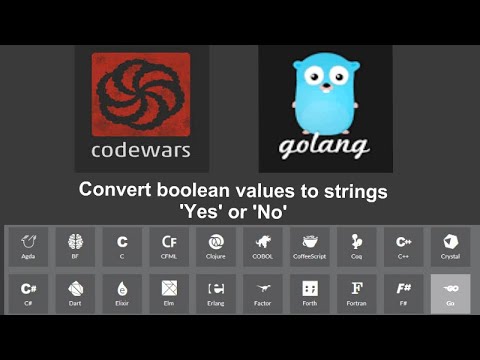

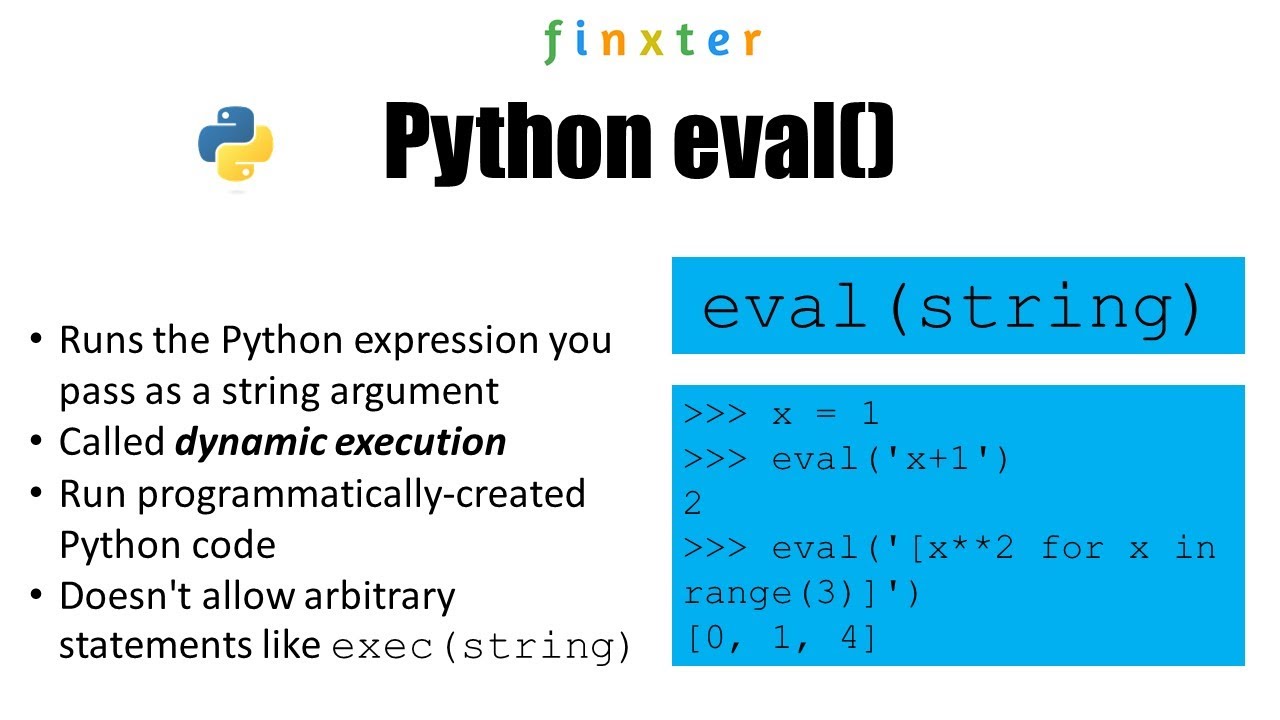
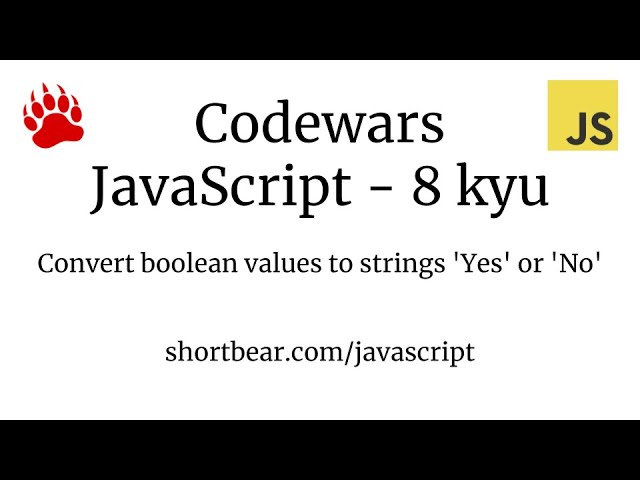

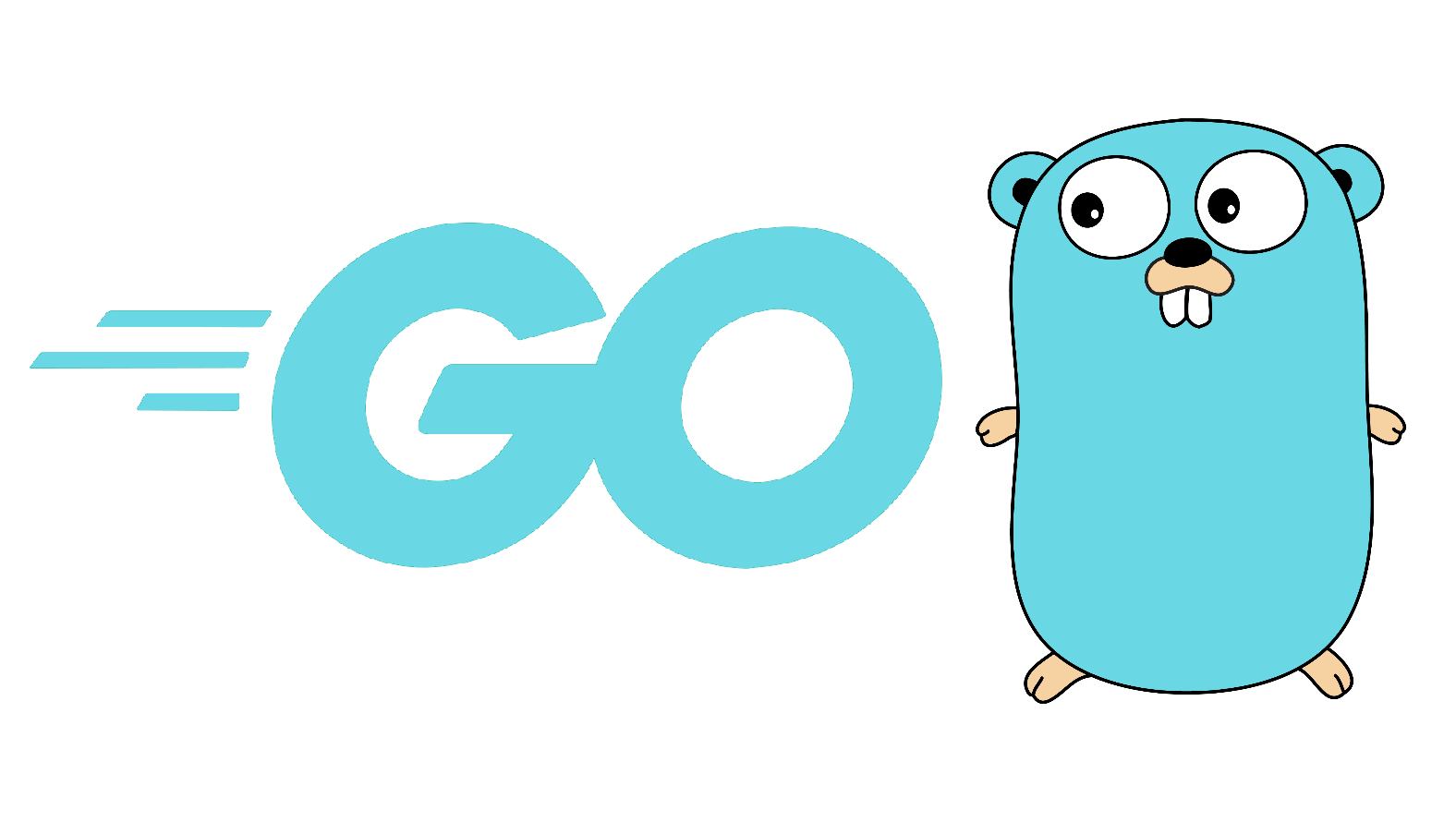
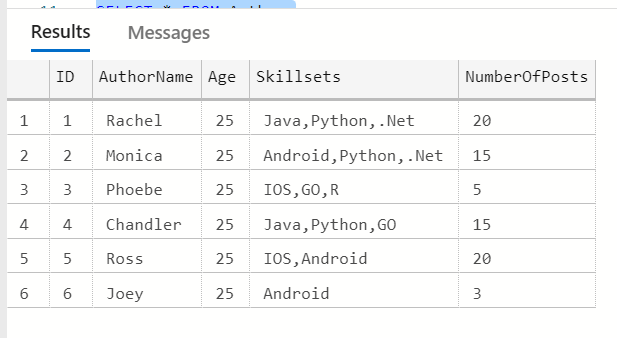



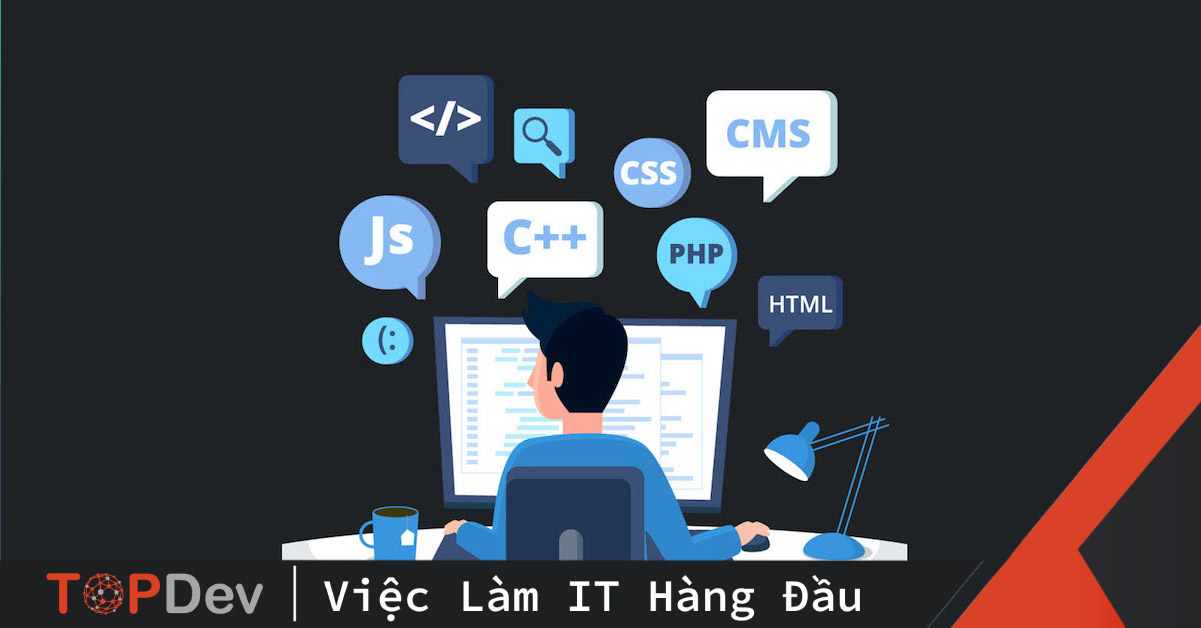
Article link: golang boolean to string.
Learn more about the topic golang boolean to string.
- How to convert a bool to a string in Go? – Stack Overflow
- Golang Program to convert Boolean to String – Tutorialspoint
- Java Convert boolean to String – javatpoint
- Golang Program to convert int type variables to String – Tutorialspoint
- How to convert a boolean value to string value in JavaScript
- Golang Program to convert Boolean to String – Tutorialspoint
- How to convert Boolean Type to String in Go?
- Convert string to bool in Go (Golang) – GOSAMPLES
- How to convert a Boolean to a string in Golang – Educative.io
- How to Convert Bool to String in Go – AskGolang
- how to convert bool to string in golang? – aGuideHub
- Different Ways to Convert the Boolean Type in String in Golang
- Concise Bool to String Conversion in Golang – MarketSplash
- How to convert Boolean to String type in GoLang – Code Destine