Get Computer Name C#
The computer name is a unique identifier for a computer on a network. It is used to identify a specific machine and can be useful in various scenarios. Let’s dive into some methods to retrieve the computer name in C#.
### Using the Environment Class to Get the Computer Name
The `Environment` class in C# provides various static methods and properties to retrieve information about the current environment. One such property is `Environment.MachineName`, which returns the name of the computer.
Here’s an example of how to obtain the computer name using the `Environment` class:
“`csharp
string computerName = Environment.MachineName;
Console.WriteLine(“Computer Name: ” + computerName);
“`
### Retrieving the Computer Name with WMI (Windows Management Instrumentation)
WMI (Windows Management Instrumentation) is a powerful technology that allows developers to retrieve various system information, including the computer name. In C#, you can use the `System.Management` namespace to interact with WMI and query system information.
Here’s an example of how to retrieve the computer name using WMI in C#:
“`csharp
using System.Management;
var query = new ObjectQuery(“SELECT * FROM Win32_ComputerSystem”);
var searcher = new ManagementObjectSearcher(query);
var collection = searcher.Get();
foreach (var item in collection)
{
string computerName = item[“Name”].ToString();
Console.WriteLine(“Computer Name: ” + computerName);
}
“`
### Getting the Computer Name using P/Invoke
P/Invoke (Platform Invocation Services) allows you to call unmanaged functions from managed code. In this case, we can make use of the `GetComputerName` function from the `kernel32.dll` to retrieve the computer name in C#.
Here’s an example of how to use P/Invoke to get the computer name in C#:
“`csharp
using System.Runtime.InteropServices;
public class Program
{
[DllImport(“kernel32.dll”)]
public static extern bool GetComputerName(StringBuilder lpBuffer, ref int nSize);
public static void Main()
{
StringBuilder sb = new StringBuilder(256);
int bufferSize = sb.Capacity;
if (GetComputerName(sb, ref bufferSize))
{
string computerName = sb.ToString();
Console.WriteLine(“Computer Name: ” + computerName);
}
}
}
“`
### Using the System.Net Namespace to Obtain the Computer Name
The `System.Net` namespace provides classes for network programming in .NET. One of these classes is `Dns`, which can be used to retrieve the computer name.
Here’s an example of how to use the `Dns` class to get the computer name in C#:
“`csharp
using System.Net;
string computerName = Dns.GetHostName();
Console.WriteLine(“Computer Name: ” + computerName);
“`
### Getting the Computer Name using the Registry
The computer name can also be obtained by reading the registry value of the computer name. In C#, you can use the `Microsoft.Win32` namespace to interact with the registry.
Here’s an example of how to retrieve the computer name using the registry in C#:
“`csharp
using Microsoft.Win32;
var key = Registry.LocalMachine.OpenSubKey(@”SYSTEM\CurrentControlSet\Control\ComputerName\ComputerName”);
string computerName = key.GetValue(“ComputerName”).ToString();
Console.WriteLine(“Computer Name: ” + computerName);
“`
### Retrieving the Computer Name with PowerShell
PowerShell is a powerful scripting language that can be used to automate administrative tasks in Windows. You can also use PowerShell to retrieve the computer name.
Here’s an example of how to obtain the computer name using PowerShell in C#:
“`csharp
using System.Diagnostics;
ProcessStartInfo psi = new ProcessStartInfo(“powershell”, “hostname”);
psi.RedirectStandardOutput = true;
psi.UseShellExecute = false;
psi.CreateNoWindow = true;
Process powershell = Process.Start(psi);
powershell.WaitForExit();
string computerName = powershell.StandardOutput.ReadToEnd().Trim();
Console.WriteLine(“Computer Name: ” + computerName);
“`
### Considerations and Best Practices when Obtaining the Computer Name in C#
When retrieving the computer name in C#, there are a few considerations and best practices to keep in mind:
1. Security: Some methods may require administrator privileges or the appropriate permissions to access system information. Ensure that your application has the necessary permissions to retrieve the computer name.
2. Error handling: When using methods like WMI, P/Invoke, or registry access, it is essential to handle exceptions and errors appropriately. Make sure to implement error handling mechanisms to handle potential exceptions.
3. Platform Compatibility: Different methods may vary in their compatibility with different versions of Windows. Ensure that the method you choose to retrieve the computer name is compatible with the target environment.
4. Performance: Some methods may have a higher overhead compared to others. Consider the performance implications of the method you choose, especially if you plan to retrieve the computer name frequently or in a performance-critical application.
### FAQs
#### Q: How can I retrieve the computer name in C#?
A: There are several methods to retrieve the computer name in C#. Some commonly used methods include using the `Environment.MachineName` property, using WMI, P/Invoke, the `System.Net.Dns` class, or reading the registry.
#### Q: Is administrator access required to retrieve the computer name in C#?
A: The level of access required may vary depending on the method used. Some methods, such as accessing the registry or using WMI, may require administrator privileges or appropriate permissions to retrieve the computer name.
#### Q: What should I do if I encounter an exception while retrieving the computer name?
A: It is essential to handle exceptions appropriately when retrieving the computer name. Implement error handling mechanisms to handle potential exceptions and provide suitable feedback or fallback options to the user.
In conclusion, retrieving the computer name in C# is a straightforward task that can be accomplished using various methods and libraries. Whether you choose to use the `Environment` class, WMI, P/Invoke, the `System.Net` namespace, the registry, or PowerShell, each method has its advantages and considerations. By understanding these different methods, you can choose the most suitable approach for your specific requirements and implement it effectively in your C# applications.
How To Find Your Computer Name On Windows 10
How To Get The Name Of Your Computer In C?
In C programming, interacting with the underlying system is a common requirement. One such task is to obtain the name of the computer on which the program is running. Fortunately, C provides various functions and libraries that allow us to accomplish this.
In this article, we will discuss different approaches to retrieve the name of the computer in C and explore code examples to illustrate each method. So, let’s dive in!
Method 1: Using the gethostname() function
The most straightforward way to obtain the computer’s name in C is by utilizing the gethostname() function. This function is part of the
“`c
#include
#include
int main() {
char hostname[256];
if (gethostname(hostname, sizeof(hostname)) != -1) {
printf(“Computer name: %s\n”, hostname);
}
return 0;
}
“`
The gethostname() function takes two arguments: the buffer to store the computer name and its size. Upon success, the function returns 0, while -1 represents a failure. This approach is widely supported and compatible across different platforms.
Method 2: Using the getenv() function
If you’re looking for an alternative way or need to access more system-related information, getenv() might be a useful function. This function is part of the
To obtain the computer’s name using getenv(), you can retrieve the value of the “HOSTNAME” or “COMPUTERNAME” environment variables. Here’s a code snippet to exemplify this approach:
“`c
#include
#include
int main() {
char* computerName = getenv(“HOSTNAME”);
if (!computerName) {
computerName = getenv(“COMPUTERNAME”);
}
if (computerName) {
printf(“Computer name: %s\n”, computerName);
}
return 0;
}
“`
By calling getenv() with “HOSTNAME,” it attempts to retrieve the computer’s name. If it fails, it falls back to “COMPUTERNAME.” However, note that the exact environment variable might differ across operating systems, so it’s crucial to check the appropriate variable for each system.
Method 3: Using system-specific functions
Sometimes, we need to use system-specific functions to obtain the computer name, as certain platforms may not provide standardized functions. Here, we will cover two common functions: gethostname() for Windows and uname() for Unix-like systems.
For Windows:
“`c
#include
#include
int main() {
char computerName[MAX_COMPUTERNAME_LENGTH + 1];
DWORD size = sizeof(computerName);
if (GetComputerNameA(computerName, &size)) {
printf(“Computer name: %s\n”, computerName);
}
return 0;
}
“`
Here, we utilize the GetComputerNameA() function from the windows.h header. It populates the computerName buffer with the computer’s name.
For Unix-like systems:
“`c
#include
#include
int main() {
struct utsname computerInfo;
if (uname(&computerInfo) != -1) {
printf(“Computer name: %s\n”, computerInfo.nodename);
}
return 0;
}
“`
In Unix-like systems, the uname() function from the sys/utsname.h header allows us to retrieve system information. Among other details, it stores the computer’s name in the nodename member of the utsname structure.
FAQs
Q: Can I obtain the computer name without using any external functions?
A: No, retrieving the computer name in C typically requires invoking system functions or accessing environment variables.
Q: Do the methods mentioned work on all operating systems?
A: While the gethostname() and getenv() methods are widely supported, certain functions like GetComputerNameA() and uname() are platform-specific. It’s crucial to use the appropriate function for each operating system.
Q: Are there any security risks associated with retrieving the computer name?
A: Generally, retrieving the computer name does not pose any direct security risks. However, it is important to handle sensitive information with care and adhere to security best practices while working with system-related functions.
Q: Can I change the computer name programmatically?
A: Changing the computer’s name programmatically is typically not feasible within a C program. Modifying the computer’s name usually requires administrative privileges and is better suited for system configuration purposes.
Q: Are there any performance considerations when using these methods?
A: The performance impact of retrieving the computer name is minimal. However, the time required to obtain the name may vary depending on the method used and the underlying system’s capabilities.
Conclusion
Obtaining the computer’s name in C can be achieved through various methods, such as using gethostname(), getenv(), or system-dependent functions. By leveraging these techniques, developers can incorporate system-specific details into their C programs efficiently.
Remember to choose the appropriate method based on the target platform, handle potential errors, and consider any relevant security implications. By mastering these techniques, you’ll enhance the capabilities of your C programs and access valuable system information.
How To Get Computer Name From Ip In Cmd?
In certain situations, you may find yourself needing to obtain the computer name associated with a specific IP address. This can be a useful task, especially when troubleshooting network issues or managing systems remotely. Fortunately, with the Command Prompt (CMD) utility in Windows, you can retrieve the hostname from an IP address quickly and effectively. In this article, we will guide you through the process step by step to help you accomplish this task effortlessly.
Step 1: Open Command Prompt
To begin, you need to open the Command Prompt utility on your Windows computer. There are multiple ways to access it, but the simplest way is to press the ‘Windows + R’ keys simultaneously to open the Run dialog box. Type ‘cmd’ in the box and hit Enter, and the Command Prompt window will appear.
Step 2: Use the ‘nslookup’ Command
The ‘nslookup’ command is a powerful tool that enables you to query DNS (Domain Name System) servers to retrieve information about domain names and IP addresses. In this case, we will use ‘nslookup’ to find the computer name from a given IP address.
Type ‘nslookup’ in the Command Prompt window, followed by a space. Then, type the IP address you want to retrieve the computer name for. For instance, if the IP address is ‘192.168.1.10’, the command should look as follows:
nslookup 192.168.1.10
Press Enter after typing the command, and the utility will display the information related to the IP address you provided. In the ‘Name’ field, the computer name will be listed, providing the desired result.
Step 3: Using the ‘ping’ Command for Comparison
Another method to obtain the computer name from an IP address is through the ‘ping’ command. While the primary purpose of ‘ping’ is to test network connectivity between systems, it can also assist in retrieving the hostname.
Open Command Prompt as before, and type ‘ping’ followed by a space and then the IP address you wish to obtain the computer name for. For example:
ping 192.168.1.10
Hit Enter to execute the command. You will notice that the Command Prompt will generate a series of responses, one of which will show the computer name within brackets. This computer name is enclosed within square brackets, such as ‘[DESKTOP-XXXX]’. By carefully inspecting these responses, you can locate the computer name associated with the given IP address.
FAQs Section:
Q1: Can I get the computer name from any IP address?
A1: You can only retrieve the computer name from an IP address that is within your network or that is connected to the network you are using.
Q2: Is it possible to obtain the computer name of a device on a different network?
A2: Obtaining the computer name of a device on a different network might not be feasible as your network typically does not have information on the naming conventions used by other networks.
Q3: Can I retrieve multiple computer names at once using CMD?
A3: Yes, you can retrieve multiple computer names by entering each IP address sequentially after the ‘nslookup’ or ‘ping’ command, separating them with spaces. The Command Prompt will display the corresponding computer names for each IP address entered.
Q4: Are there any limitations to using CMD to retrieve computer names?
A4: While CMD is a useful tool, it might not always provide accurate results in certain network configurations. Firewalls, security settings, or incorrect DNS configurations could affect the command’s functionality.
Q5: Is there an alternative method to retrieve computer names from IP addresses?
A5: Yes, there are various online tools and software available that provide this functionality. However, using CMD remains a convenient and reliable option, particularly for quick checks or troubleshooting within your network.
In conclusion, retrieving computer names from IP addresses using CMD can be a valuable skill when it comes to managing networks or troubleshooting connectivity issues. By utilizing the ‘nslookup’ or ‘ping’ command, you can easily obtain the computer names associated with specific IP addresses in a few simple steps. Remember to use this information responsibly and ensure you have the necessary privileges to perform these operations on the network you are working with.
Keywords searched by users: get computer name c# Get computer name C#, C++ get machine name, GetComputerName example, c gethostname example, C++ get hardware information, Gethostname function in C++, C++ get IP address, GetUserName c++
Categories: Top 94 Get Computer Name C#
See more here: nhanvietluanvan.com
Get Computer Name C#
Introduction (100 words)
———————————
In computer programming, retrieving the computer name is a useful operation that allows developers to identify the individual machines on a network. The process of getting the computer name can be accomplished through various programming languages, including C#. This article aims to explore the techniques and methods available to retrieve the computer name in C#, providing a comprehensive guide to assist both beginner and intermediate programmers in their development endeavors.
I. Understanding the Computer Name (150 words)
——————————————–
Before diving into the code, it’s important to grasp the concept of a computer name. The computer name is a unique identifier that distinguishes a particular machine on a network. It commonly consists of a combination of alphabetic, numeric, and special characters. The computer name is typically assigned by the user during the initial setup of the operating system or provided by network administrators.
II. Using C# to Get the Computer Name (400 words)
————————————————-
1. Environment class:
The simplest way to obtain the computer name in C# is by utilizing the Environment class from the System namespace. This class provides access to various system-related information, including the computer name.
Example code:
“`csharp
string computerName = Environment.MachineName;
“`
By calling the `MachineName` property, this code snippet retrieves the computer name as a string.
2. WMI (Windows Management Instrumentation):
Another way to retrieve the computer name in C# is through WMI, which provides a more comprehensive set of tools for interacting with the Windows operating system.
Example code:
“`csharp
using System.Management;
…
string computerName;
using (ManagementObjectSearcher searcher = new ManagementObjectSearcher(“SELECT * FROM Win32_ComputerSystem”))
{
ManagementObjectCollection collection = searcher.Get();
computerName = collection.Cast
}
“`
This code snippet utilizes the System.Management namespace and WMI to query the Win32_ComputerSystem class, retrieving the computer name as a string.
III. Best Practices (150 words)
——————————
When implementing the retrieval of computer names in C#, there are a few best practices that can enhance performance and minimize errors. Some guidelines to follow include:
– Handling exceptions: Exception handling should be employed when accessing system information, as it ensures a program can gracefully respond to unexpected issues, such as lack of permission or network connectivity problems.
– Memory management: Utilize the “using” statement, as demonstrated in the WMI example, to free up system resources when querying system information.
– Cross-platform compatibility: The techniques mentioned above work on Windows operating systems. However, when targeting other platforms such as Linux or macOS, alternate methods, like querying environment variables, need to be employed.
FAQs (217 words)
———————-
Q1. Can I retrieve the computer name in C# from a remote machine?
A1. Yes, you can retrieve the computer name of a remote machine using the System.Management namespace and WMI. However, this requires the necessary administrative privileges and connectivity to the remote machine.
Q2. What should I do if the computer name is not accessible?
A2. If the computer name is not accessible due to restricted security settings, such as permission issues or network restrictions, the application might throw exceptions. Ensure your application gracefully handles such situations, providing useful error messages.
Q3. Is the computer name guaranteed to be unique across a network?
A3. No, by default, the computer name is not guaranteed to be unique across a network. It is recommended to complement the computer name with additional identifiers to maintain uniqueness, such as an IP address or a MAC address.
Q4. Can I change the computer name programmatically using C#?
A4. Yes, you can change the computer name programmatically in C#. However, this operation requires administrative privileges and proper handling to ensure system stability and security. It is advisable to consult system documentation and security guidelines before making any modifications.
Conclusion (100 words)
————————
Retrieving the computer name is a fundamental task in network programming. This article extensively explored how to get the computer name using C#, providing two methods – the Environment class and WMI. By following the best practices, developers can ensure efficient and error-free retrieval of this essential system information. Remember, handling exceptions, managing system resources, and considering cross-platform compatibility will lead to more robust and versatile code. As you advance in your programming journey, leverage these methods to better identify and interact with machines on a network.
C++ Get Machine Name
Introduction:
In the world of programming, obtaining the machine name is a useful task for various purposes. C++ is a powerful language that allows developers to access system-level functionality, making it possible to retrieve the machine name programmatically. In this guide, we will explore different methods to get the machine name in C++, detailing step-by-step instructions and providing a thorough understanding of the topic.
Methods to Get Machine Name:
Method 1: Using the Win32 API
One common approach to obtaining the machine name is by utilizing the Win32 API, available on the Windows operating system. The Win32 API provides a comprehensive set of functions to access and retrieve system information. To get the machine name using this method, you need to include the relevant header files and utilize the GetComputerName function. Here’s the code snippet:
“`cpp
#include
#include
int main() {
char machineName[MAX_COMPUTERNAME_LENGTH + 1];
DWORD size = sizeof(machineName);
if (GetComputerName(machineName, &size)) {
std::cout << "Machine Name: " << machineName << std::endl;
} else {
std::cerr << "Failed to retrieve machine name." << std::endl;
}
return 0;
}
```
By executing the code above, you can retrieve the machine name and print it on the console.
Method 2: Using the Boost Library
Another method to obtain the machine name in C++ is by utilizing the Boost Library. Boost is a popular C++ library that provides various functionalities, including system-level access. To get the machine name using this method, you need to include the necessary Boost headers and utilize the boost::asio::ip::host_name function. Here's an example:
```cpp
#include
#include
int main() {
std::string machineName = boost::asio::ip::host_name();
std::cout << "Machine Name: " << machineName << std::endl;
return 0;
}
```
The code snippet above retrieves the machine name using the boost::asio::ip::host_name function and displays it on the console.
FAQs:
Q1. What is a machine name?
A1. A machine name is a unique identifier assigned to a computer or device on a network. It is used for identification and communication purposes.
Q2. Why would I need to get the machine name in C++?
A2. Obtaining the machine name can be useful for various purposes, such as network operations, system administration tasks, or customization based on the specific machine.
Q3. Are there any limitations or considerations when using these methods?
A3. When using the Win32 API method, it is essential to ensure that the program is running on a Windows system. The Boost Library method, on the other hand, requires the inclusion and configuration of the Boost Library in the development environment.
Q4. Can I retrieve the fully qualified domain name (FQDN) using these methods?
A4. Yes, both the Win32 API and Boost Library methods provide options to retrieve the FQDN along with the machine name.
Q5. Are there any security concerns when retrieving the machine name?
A5. No, retrieving the machine name does not pose any significant security risks. However, it is essential to handle the retrieved information with care and not disclose it unnecessarily.
Conclusion:
Retrieving the machine name in C++ is a valuable functionality that allows developers to access system-level information. In this comprehensive guide, we explored two methods to get the machine name: the Win32 API approach and the Boost Library method. By following the provided code snippets and instructions, developers can easily incorporate this functionality into their C++ applications. Remember to consider the limitations and security considerations when utilizing these methods and handle the retrieved information responsibly.
Images related to the topic get computer name c#
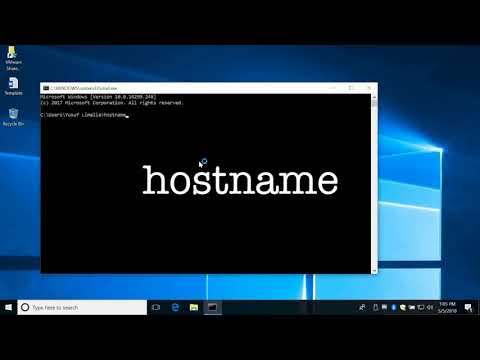
Article link: get computer name c#.
Learn more about the topic get computer name c#.
- Get Computer Name and logged user name – c++
- GetComputerNameA function (winbase.h) – Win32 apps
- how to find the computer name – C Board
- Getting Hostname in C Programs in Linux – SysTutorials
- How to find your computer name on Windows and Mac – IONOS
- GetComputerNameA function (winbase.h) – Win32 apps
- How to Find Your Computer Name – Drexel University
- `get computer name` C++ Examples – Program Creek
- Getting Hostname in C Programs in Linux – SysTutorials
- C Program to display hostname and IP address – GeeksforGeeks
- [Solved]-Get Computer Name and logged user name-C++
- Get Computer Name in C# | Delft Stack