Generate 4 Digit Random Number In Python
1. Importing the necessary libraries:
In order to generate random numbers in Python, we need to import the “random” library. This library provides various functions and methods to generate random numbers, perform random sampling, and shuffle sequences. To import the library, we can use the “import” keyword, followed by the library name:
“`python
import random
“`
2. Understanding random number generation:
Random number generation is a vital aspect of many programming tasks. It involves generating numbers that lack any discernible pattern or predictability. Random numbers find applications in simulations, cryptography, games, and statistical analysis. In Python, random numbers can be categorized into two types: pseudo-random numbers and truly random numbers.
Pseudo-random numbers are generated using deterministic algorithms that mimic randomness. These algorithms rely on a starting value called a seed, which is used as a basis for generating subsequent numbers. Given the same seed, these algorithms will always produce the same sequence of numbers. However, changing the seed or using a random seed will result in a different sequence each time.
On the other hand, truly random numbers are generated from unpredictable physical processes, such as atmospheric noise or radioactive decay. These numbers are considered to be more random than pseudo-random numbers but require specialized hardware or external services to generate.
It is important to note that the random numbers generated by Python’s “random” library are pseudo-random numbers.
3. Generating a single random digit:
To generate a single random digit in Python, we can use the “random.randint()” function. This function takes two arguments: the lower and upper limits of the range within which the random digit should be generated. The function will return a random integer within this specified range.
Here’s an example of how to generate a single random digit between 0 and 9:
“`python
import random
random_digit = random.randint(0, 9)
print(random_digit)
“`
The “randint()” function will generate a random digit and assign it to the variable “random_digit”. The value will then be printed to the console.
4. Generating a four-digit random number:
To generate a four-digit random number, we can concatenate four randomly generated digits. We can achieve this by using a loop to generate each digit and appending it to a string. Here’s an example code snippet:
“`python
import random
random_number = “”
for i in range(4):
random_digit = random.randint(0, 9)
random_number += str(random_digit)
print(random_number)
“`
In this code, we initialize an empty string, “random_number”, which will store the generated four-digit number. The loop runs four times, generating a random digit each time using the “randint()” function. The result is concatenated to the “random_number” string using the “+=” operator. Finally, the four-digit number is printed.
5. Ensuring the uniqueness of the generated number:
When generating random numbers, there is a possibility of generating duplicate numbers. In certain cases, it is crucial to ensure the uniqueness of the generated number. To avoid duplicates, we can use various methods.
One approach is to use the “random.sample()” function instead of concatenating digits. This function generates a specified number of unique random elements from a given population without replacement. By using this function and treating the digits 0-9 as the population, we can generate a four-digit number without duplicates.
Another approach is to check against existing numbers by maintaining a list of generated numbers and comparing each new random number against this list. If a duplicate is encountered, a new random number is generated until a unique number is obtained.
6. Useful applications and further enhancements:
Random number generation has numerous applications. In simulations, random numbers are often used to model uncertain or stochastic events. In cryptography, random numbers are essential for generating cryptographic keys and ensuring the security of encrypted data. Random numbers can also be used in games, where unpredictable outcomes are necessary for engaging gameplay.
To enhance the generated numbers, you can add constraints, such as excluding certain digits or ensuring a specific order of digits. Additionally, you can generate multiple four-digit numbers at once by modifying the code to include a loop.
FAQs:
1. Can I generate a 6-digit unique random number in Python?
Yes, you can generate a 6-digit unique random number in Python by using similar techniques as explained for generating 4-digit numbers. Instead of generating four digits, you would generate six digits while ensuring uniqueness.
2. How can I generate a random number within a specific range in Python?
To generate a random number within a specific range in Python, you can use the “random.randint()” function. This function takes two arguments: the lower and upper limits of the desired range and returns a random integer within that range.
3. How can I write a program that generates and prints 50 random integers between 3 and 6 in Python?
Here’s an example code snippet to generate and print 50 random integers between 3 and 6 inclusively:
“`python
import random
for _ in range(50):
random_number = random.randint(3, 6)
print(random_number)
“`
4. How can I generate a random 4-digit number in JavaScript?
In JavaScript, you can generate a random 4-digit number using the “Math.random()” function and manipulating the resulting value. Here’s an example code snippet:
“`javascript
const randomNumber = Math.floor(1000 + Math.random() * 9000);
console.log(randomNumber);
“`
5. How can I write a program that prints 10 random numbers between 25 and 35 inclusively in Python?
Here’s an example code snippet to generate and print 10 random numbers between 25 and 35 inclusively:
“`python
import random
for _ in range(10):
random_number = random.randint(25, 35)
print(random_number)
“`
In conclusion, generating random numbers in Python is a fundamental requirement in various programming tasks. By understanding the concepts and utilizing the “random” library, we can easily generate single and multi-digit random numbers, ensure uniqueness, and apply them in practical applications.
Random Number Generator In Python
How To Generate A 4 Digit Random Number In Java?
In programming, generating random numbers is a common requirement across various applications. Java, being a widely-used programming language, provides several methods to generate random numbers. If you specifically need to generate a 4-digit random number, there are multiple approaches you can take. In this article, we will explore different methods and discuss their implementation in Java.
Method 1: Using Math.random()
One of the simplest ways to generate a random number in Java is by utilizing the `Math.random()` method. By default, this method returns a random number between 0.0 (inclusive) and 1.0 (exclusive). However, we can adapt it to generate a 4-digit random number.
Here is an example code snippet that demonstrates how to generate a 4-digit random number using `Math.random()`:
“`java
int randomNumber = (int) (Math.random() * 9000) + 1000;
“`
In this code, we multiply `Math.random()` by 9000 to get a number between 0.0 and 9000. Then, we add 1000 to the result to ensure the range of the random number is between 1000 and 9999.
Method 2: Using java.util.Random
Another approach is to use the `java.util.Random` class, which provides more flexibility and control over random number generation. This class allows us to specify the desired range of the random numbers.
Here is an example code snippet that demonstrates how to use `java.util.Random` to generate a 4-digit random number:
“`java
Random random = new Random();
int randomNumber = random.nextInt(9000) + 1000;
“`
In this code, we create an instance of `Random` and then use the `nextInt()` method to generate a random number within the specified range. Similar to the previous method, we add 1000 to ensure the generated number falls within the desired 4-digit range.
Method 3: Using ThreadLocalRandom
Starting from Java 7, another option for generating random numbers is the `ThreadLocalRandom` class. This class provides the advantage of being thread-safe, making it suitable for multi-threaded applications.
Here is an example code snippet that demonstrates how to generate a 4-digit random number using `ThreadLocalRandom`:
“`java
int randomNumber = ThreadLocalRandom.current().nextInt(1000, 10000);
“`
In this code, we use the `current()` method to obtain an instance of `ThreadLocalRandom` and then call `nextInt()` with the desired range of 1000 and 10000.
Method 4: Using RandomStringUtils
If you have Apache Commons Text library available in your project, you can use the `RandomStringUtils` class to generate random numbers. This method provides more flexibility, allowing you to generate random numbers with different lengths and character sets.
Here is an example code snippet that demonstrates how to use `RandomStringUtils` to generate a 4-digit random number:
“`java
String randomNumber = RandomStringUtils.randomNumeric(4);
“`
In this code, we utilize the `randomNumeric()` method to generate a random string consisting of four numeric characters.
Frequently Asked Questions (FAQs):
Q: Can I generate a 4-digit random number without any leading zeros?
A: Yes, all the methods mentioned above ensure that the generated random number will be a 4-digit number, always omitting leading zeros.
Q: How can I generate a unique 4-digit random number?
A: Generating a truly unique 4-digit random number is challenging since there are only 9000 possibilities. To achieve uniqueness, you would typically need to implement additional logic to keep track of used numbers and avoid repetition.
Q: How can I generate a 4-digit random number with unique digits?
A: To generate a 4-digit random number with unique digits, you can adapt the methods mentioned earlier by introducing additional logic. For instance, you could use a loop to continuously generate random numbers until a unique combination is obtained.
Q: Can I generate a 4-digit random number with a specific format?
A: Yes, you can manipulate the generated random number to fit a specific format by converting it to a string and applying formatting options like padding, substring, or concatenation.
In conclusion, generating a 4-digit random number in Java can be achieved through various methods. Whether you choose to use `Math.random()`, `java.util.Random`, `ThreadLocalRandom`, or `RandomStringUtils`, each approach offers its own advantages. By understanding these methods, you can easily generate random numbers to suit your project’s requirements.
How To Generate 4 Digit Random Number In C?
Generating a random number is a common requirement in many programming tasks. In C, there are several ways to generate random numbers, and generating a 4-digit random number is just one of the many possibilities. In this article, we will explore different methods to generate a 4-digit random number in C and explain the steps involved in each method.
Method 1: Using rand() function
The most basic method to generate a random number in C is by using the rand() function. The rand() function returns a pseudo-random number between 0 and RAND_MAX (a constant defined in the C library, typically representing a large integer value). To generate a 4-digit random number, we can make use of the rand() function along with some arithmetic operations.
Here is an example code snippet that generates a 4-digit random number using the rand() function:
“`c
#include
#include
#include
int main() {
srand(time(0)); // Seed the random number generator with current time
int randomNumber = rand() % 9000 + 1000; // Generate a number between 1000 and 9999
printf(“Random number: %d\n”, randomNumber);
return 0;
}
“`
In this code, we first seed the random number generator using the `srand()` function with a seed value obtained from the current time (`time(0)`). This helps ensure that we get different random numbers on each run.
Next, we use the modulus operator (`%`) to restrict the range of the generated number between 0 and 8999 (`rand() % 9000`). By adding 1000 to this result, we shift the range to 1000 and 9999, thus achieving a 4-digit random number.
Method 2: Using random() function
Another alternative to generate a random number in C is by using the `random()` function. Similar to the `rand()` function, `random()` returns a pseudo-random number, but with a larger range compared to `rand()`. We can utilize this function along with the modulo and addition operation to generate our desired 4-digit random number.
“`c
#include
#include
#include
int main() {
srandom(time(0)); // Seed the random number generator with current time
int randomNumber = random() % 9000 + 1000; // Generate a number between 1000 and 9999
printf(“Random number: %d\n”, randomNumber);
return 0;
}
“`
Note that the code is similar to the previous example, except for using `random()` instead of `rand()`, and `srandom()` instead of `srand()`.
Method 3: Using time() function
If you need a unique random number for each execution of your program within a short time span, you can leverage the `time()` function as a source of randomness. However, this method is not recommended for applications that require high security or long periods of time between each execution.
“`c
#include
#include
#include
int main() {
time_t t;
srand((unsigned) time(&t)); // Seed the random number generator with current time
int randomNumber = rand() % 9000 + 1000; // Generate a number between 1000 and 9999
printf(“Random number: %d\n”, randomNumber);
return 0;
}
“`
By using `srand((unsigned) time(&t))`, we provide the `srand()` function with a seed value based on the current system time. This ensures that each execution of the program within a short time frame generates a different random number.
FAQs:
Q: What is the purpose of using the time() function in generating random numbers?
A: By using the time() function, we can seed the random number generator with a value that changes with time, making the generated random numbers unique during each execution.
Q: Can I generate a 4-digit random number without restricting the range?
A: Yes, you can generate a 4-digit random number without restricting the range. Instead of using the modulo and addition operations, you can simply multiply the generated random number by 9000 and then add 1000 to shift the range to 1000 and 9999.
Q: How can I generate a random number with leading zeros?
A: If you want to include leading zeros in your 4-digit random number, you can generate a random number between 0 and 9999 and use formatting to display it as four digits.
In conclusion, generating a 4-digit random number in C can be done using various methods. The key is to seed the random number generator properly and restrict the range of the generated number. By following the examples and explanations provided in this article, you can easily generate a 4-digit random number in your C programs.
Keywords searched by users: generate 4 digit random number in python generate 6 digit unique random number in python, Python random number 4 digits, Python generate random number in range, Write a program that generates and prints 50 random integers each between 3 and 6, Random 6 number python, generate 4 random numbers python, Random 4 number js, Write a program that prints 10 random numbers between 25 and 35 inclusively
Categories: Top 82 Generate 4 Digit Random Number In Python
See more here: nhanvietluanvan.com
Generate 6 Digit Unique Random Number In Python
Introduction:
Python is a powerful programming language that provides various functionalities to generate random numbers. Oftentimes, it is necessary to generate unique random numbers for specific purposes. In this article, we will explore different approaches to generating 6-digit unique random numbers in Python and discuss various scenarios where this functionality is commonly used.
Methods to Generate Unique Random Numbers:
Method 1: Utilizing the random module
The random module in Python provides several functions to generate random numbers. One of them is the randint() function, which generates random integers within a specified range. By utilizing this function, we can generate 6-digit random numbers.
“`python
import random
def generate_unique_number():
return random.randint(100000, 999999)
“`
In the above code snippet, we import the random module and define a function called generate_unique_number(). This function utilizes the randint() function to generate a random integer between 100000 and 999999, inclusive. The resulting integer represents a 6-digit unique random number.
Method 2: Utilizing the secrets module (Python 3.6 onwards)
Starting from Python 3.6, the secrets module was introduced to provide a more secure way of generating random numbers. This module offers a function called randbelow() that generates random integers below a given limit.
“`python
import secrets
def generate_unique_number():
return str(secrets.randbelow(900000) + 100000)
“`
In this approach, we import the secrets module and define the generate_unique_number() function. Using the randbelow() function, we generate a random integer below the limit 900000, and then add 100000 to ensure a 6-digit number. Finally, we convert the resulting integer to a string format.
Method 3: Utilizing the uuid module
Another approach to generating unique random numbers is by utilizing the uuid module, which provides functions to generate universally unique identifiers (UUIDs). We can use the uuid4() function to generate a 6-digit unique random number.
“`python
import uuid
def generate_unique_number():
return str(uuid.uuid4().int % 1000000).zfill(6)
“`
In the above code snippet, we import the uuid module and define the generate_unique_number() function. The uuid4() function generates a random UUID. By applying the modulus operation with 1000000, we ensure the resulting number is within the range of 0 to 999999. Finally, the zfill(6) function pads the number with leading zeros to ensure it consists of 6 digits.
Usage Scenarios:
1. Generate unique codes: In various systems, unique codes are required for identification purposes. By generating 6-digit unique random numbers, we can create unique codes that are easy to use and remember.
2. Gaming applications: In gaming applications, it is often necessary to generate unique identification numbers for players or items. By utilizing the above methods, we can generate 6-digit unique random numbers to serve this purpose.
3. Password reset tokens: When implementing password reset functionality, unique tokens are required to ensure security. By generating unique random numbers, we can create these tokens efficiently.
FAQs:
Q1. Why do we need unique random numbers?
A: Unique random numbers are necessary to ensure that each number generated is distinct from the others. In various scenarios, such as assigning identification numbers or creating secure tokens, it is crucial to have unique values to avoid conflicts and ensure accuracy.
Q2. Can the methods provided guarantee uniqueness?
A: While these methods generate unique random numbers for a particular execution, there is still a possibility of collisions when generating a large number of random numbers. For applications where uniqueness is critical, additional checks or databases should be used to ensure uniqueness across a larger scale.
Q3. Is there a limit on the number of unique random numbers that can be generated?
A: It depends on the range of numbers used and the algorithm employed. For a 6-digit unique random number, there are a total of 900,000 possible combinations. With each generation, the number of available unique numbers decreases. Consequently, the total number of unique random numbers that can be generated is limited by the range and the frequency of generation.
Conclusion:
In Python, generating 6-digit unique random numbers can be accomplished using various approaches. By leveraging the random module, the secrets module, or the uuid module, developers can generate unique numbers to suit their specific needs. Whether for identification purposes, gaming applications, or security-related functionality like password reset tokens, these methods provide effective solutions. However, it is important to note that additional considerations and checks may be necessary to ensure uniqueness at a larger scale.
Python Random Number 4 Digits
Python is a versatile programming language that offers a wide range of functionalities. One such functionality is generating random numbers. In this article, we will delve into the specifics of generating random 4-digit numbers using Python and explore the various methods and possibilities. Whether you are a beginner or an experienced programmer, this guide will provide you with a comprehensive understanding of how to generate random 4-digit numbers in Python.
Generating random numbers is a common requirement in many programming scenarios. Python provides a built-in module called ‘random’ which offers a variety of functions to help with random number generation. To generate a 4-digit random number, we will focus on the ‘randint’ function from the ‘random’ module.
To begin, we need to import the ‘random’ module into our Python script. This can be achieved by adding the following line at the beginning of your code:
“`python
import random
“`
Once the ‘random’ module is imported, we can utilize the ‘randint’ function to generate a random 4-digit number. The ‘randint’ function takes two arguments: the lower bound and the upper bound between which the random number should be generated.
“`python
random_number = random.randint(1000, 9999)
“`
In this case, we have specified the lower bound as 1000 and the upper bound as 9999, ensuring that our generated number will always be a 4-digit number. The ‘randint’ function is inclusive of both the lower and upper bounds, so a number between 1000 and 9999, both inclusive, will be generated.
Additionally, if you want to generate a list of random 4-digit numbers, you can use a loop to repeat the process multiple times:
“`python
random_number_list = []
for _ in range(10):
random_number_list.append(random.randint(1000, 9999))
“`
In the example above, we have created an empty list called ‘random_number_list’ to store our generated numbers. The loop runs 10 times, and each time it generates a random 4-digit number using the ‘randint’ function and adds it to the list.
Now that we know how to generate random 4-digit numbers using Python, let’s address some frequently asked questions:
FAQs:
Q1. Can I generate random 4-digit numbers starting from zero?
Yes, you can generate random 4-digit numbers starting from zero. You would need to set the lower bound as 0 and the upper bound as 9999, like this: `random.randint(0, 9999)`. However, keep in mind that the generated number may start with one or more leading zeroes, which might affect its usability in certain scenarios.
Q2. How can I ensure that the generated 4-digit number has no repeated digits?
To ensure that the generated 4-digit number has no repeated digits, you can utilize the ‘sample’ function from the ‘random’ module. The ‘sample’ function allows you to generate a random sample without replacement. Here’s an example code snippet:
“`python
random_number = int(“”.join(random.sample(“0123456789”, 4)))
“`
In this example, the ‘sample’ function takes a string of digits (from 0 to 9) and generates a random sample of 4 non-repeating digits. The ‘join’ function is then used to concatenate the sampled digits into a single string, which is then converted to an integer using ‘int()’.
Q3. Can I generate random numbers with a different number of digits?
Absolutely! If you want to generate random numbers with a different number of digits, you can adjust the lower and upper bounds accordingly. For example, to generate a random 3-digit number, you would use `random.randint(100, 999)`. Likewise, for a 5-digit number, you can utilize `random.randint(10000, 99999)`.
In conclusion, generating random 4-digit numbers using Python is a straightforward task with the help of the ‘random’ module’s ‘randint’ function. By specifying the desired range, you can generate a single random number or create a list of random numbers. Additionally, you can customize the generation process to ensure no repeated digits or modify the function to generate numbers with different digit lengths. Python’s flexibility and powerful modules make it an excellent choice for all your random number generation needs.
Python Generate Random Number In Range
Python offers different approaches to generate random numbers within a range. Let’s explore some of the most commonly used methods in Python’s random module.
1. Using random.randint():
The random.randint() function is a straightforward method to generate random integers within a specific range. The range is inclusive, meaning that the boundaries are also a part of the possible outputs. It takes two arguments: the lowest and highest integers for the range.
Here’s an example to generate a random integer between 1 and 10 using random.randint():
“`python
import random
random_number = random.randint(1, 10)
print(random_number)
“`
The output will be a random integer between 1 and 10, including both 1 and 10.
2. Using random.randrange():
The random.randrange() function allows you to generate random integers from a range, excluding the upper limit. It is similar to random.randint(), but it only takes a single argument: the upper limit of the range.
Here’s an example to generate a random number between 1 and 9:
“`python
import random
random_number = random.randrange(1, 10)
print(random_number)
“`
The output will be a random integer between 1 and 9, excluding 10.
3. Using random.choice():
If you have a sequence, such as a list, you can use the random.choice() function to select a random element from that sequence. This approach is useful when you have a predefined set of values and want to randomly select one.
Here’s an example to generate a random number from a list:
“`python
import random
numbers = [1, 2, 3, 4, 5]
random_number = random.choice(numbers)
print(random_number)
“`
The output will be a random number from the numbers list.
Frequently Asked Questions (FAQs):
Q1. Can random.randrange() generate floating-point numbers?
No, random.randrange() function works only with integers. If you need to generate random floating-point numbers, you can use random.uniform() method instead.
Q2. How can I set a seed value for reproducibility?
To produce the same sequence of random numbers, you can set a seed value using the random.seed() function. The seed value can be any hashable object, such as an integer or string.
“`python
import random
random.seed(42)
random_number = random.randint(1, 100)
print(random_number)
“`
Executing the above code will always generate the same random number whenever the seed is set to 42.
Q3. How can I generate a list of random numbers within a range?
To generate a list of random numbers, you can use list comprehension with the desired range. For example, if you want to create a list of 10 random numbers between 1 and 100:
“`python
import random
random_numbers = [random.randint(1, 100) for _ in range(10)]
print(random_numbers)
“`
This will generate and print a list of 10 random numbers between 1 and 100.
Q4. Can I generate random numbers following a specific probability distribution?
Yes, Python provides several probability distributions, such as normal, exponential, and uniform, through the random module. You can use functions like random.normalvariate(), random.expovariate(), and random.uniform() to generate random numbers following those distributions.
“`python
import random
random_number = random.normalvariate(0, 1) # Generates a random number from a standard normal distribution
print(random_number)
“`
The output will be a random number following the standard normal distribution with mean 0 and standard deviation 1.
Conclusion:
In Python, generating random numbers within a range is a breeze with the help of the random module. We explored various techniques like using random.randint(), random.randrange(), and random.choice() to generate random numbers either inclusively or exclusively. Additionally, we covered some frequently asked questions related to setting a seed value for reproducibility, generating a list of random numbers, and generating numbers following specific probability distributions. With such flexibility and versatility offered by Python, you have all the tools you need to create applications that require random number generation.
Images related to the topic generate 4 digit random number in python
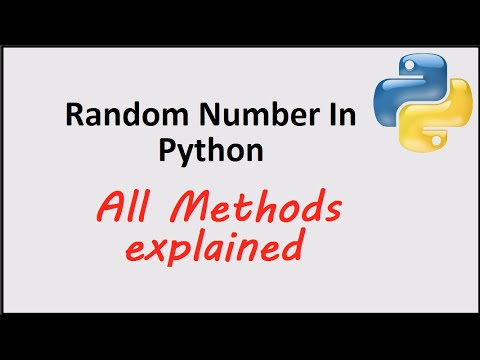
Found 38 images related to generate 4 digit random number in python theme
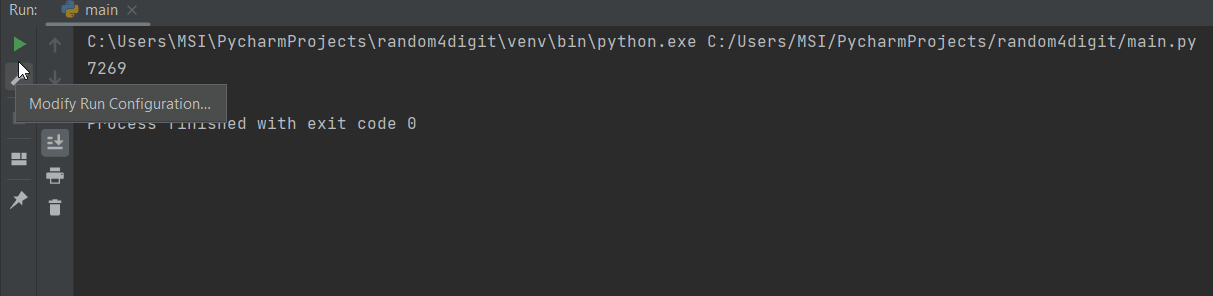





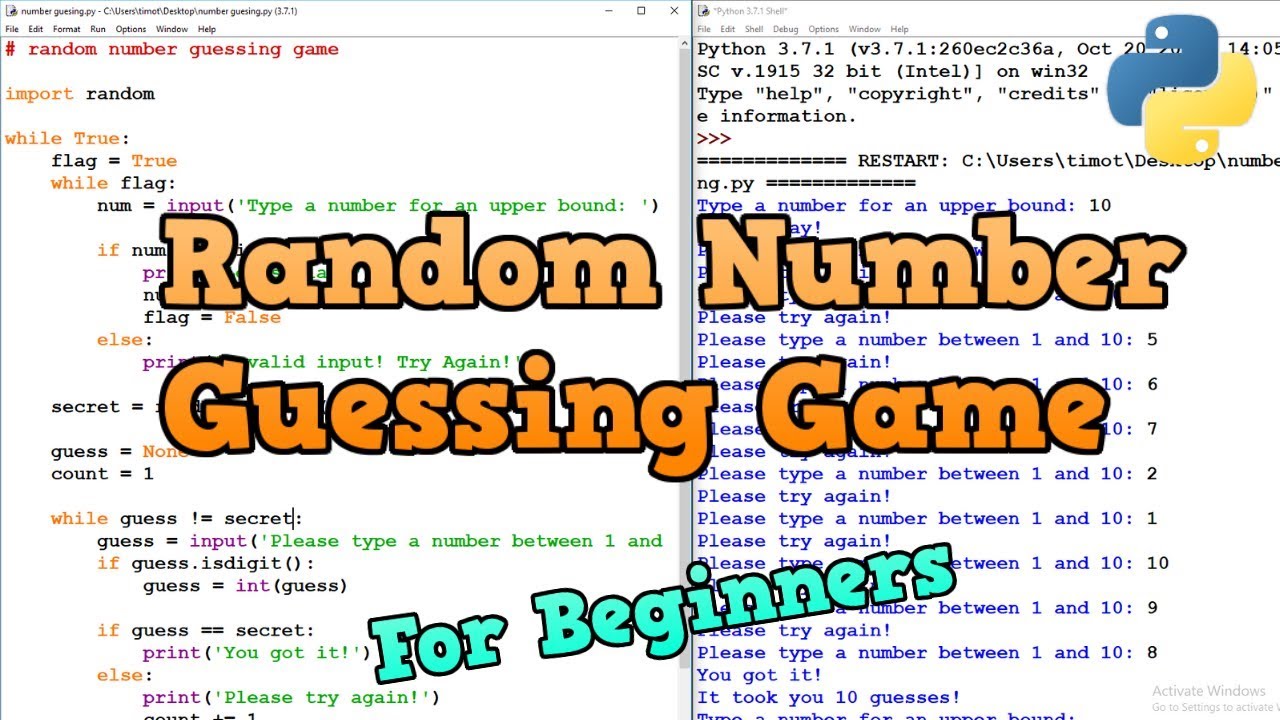
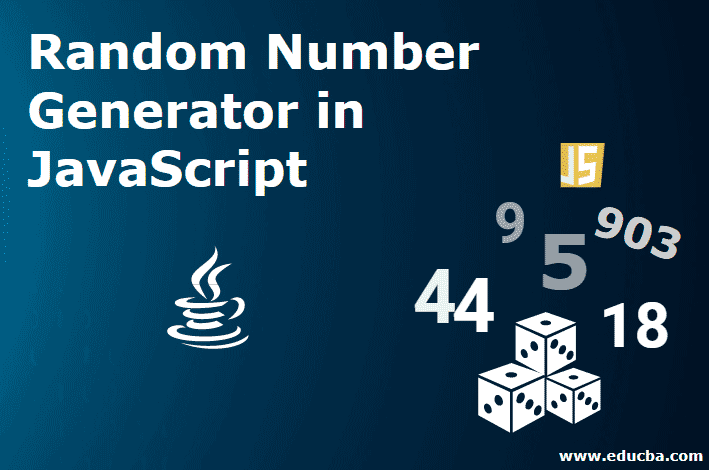
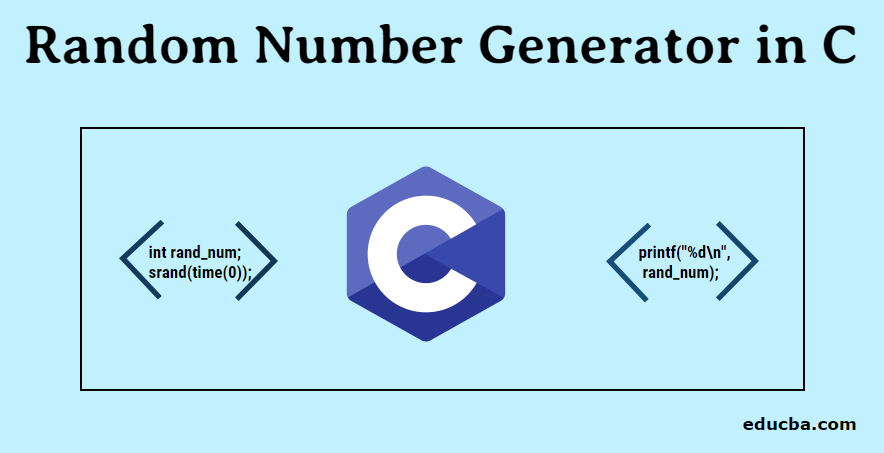


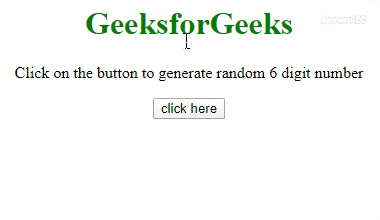
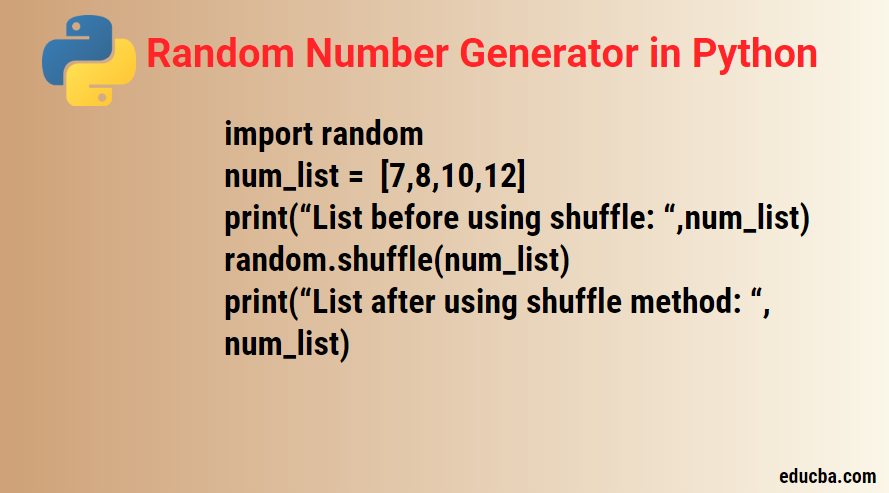
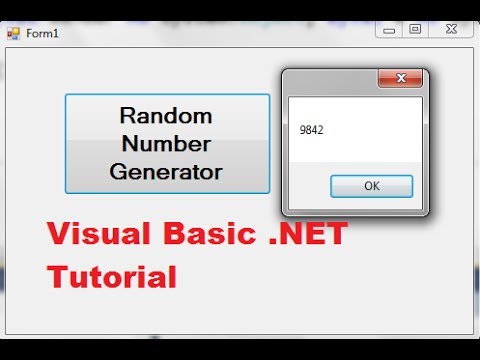
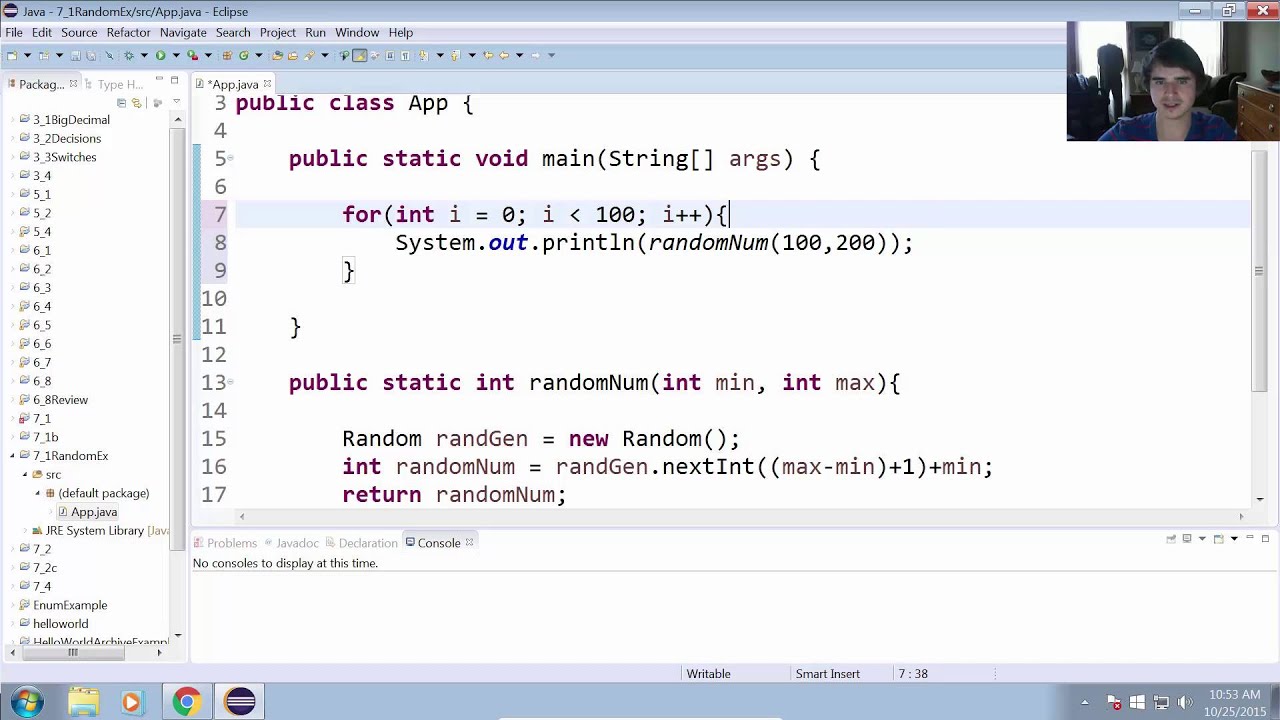
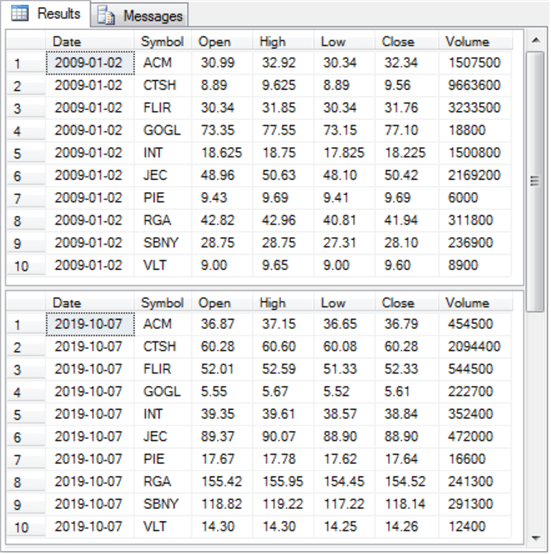
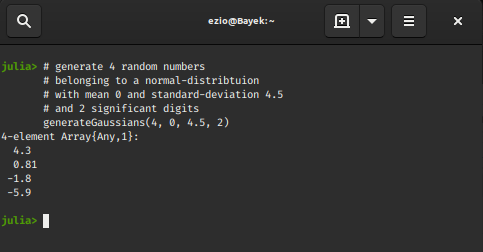
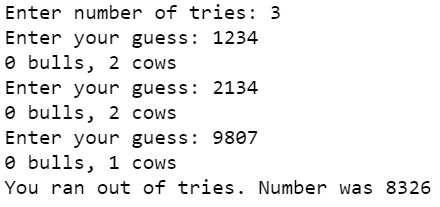
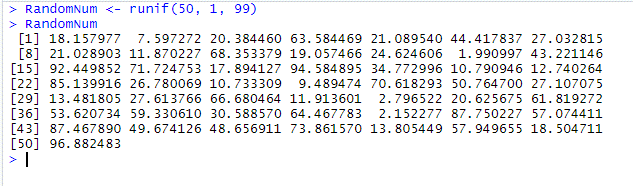


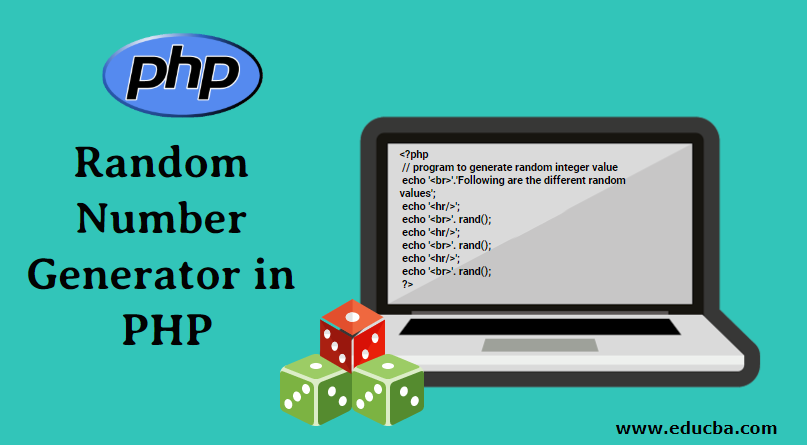
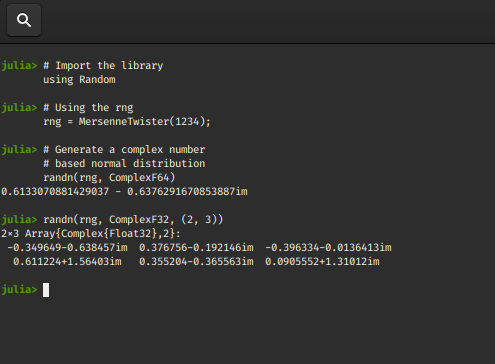
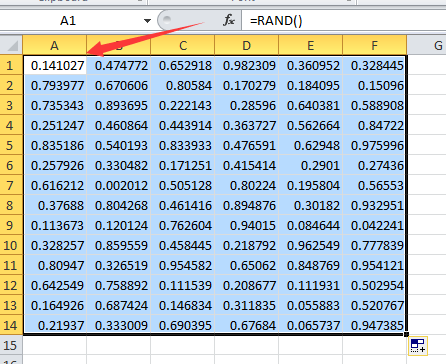
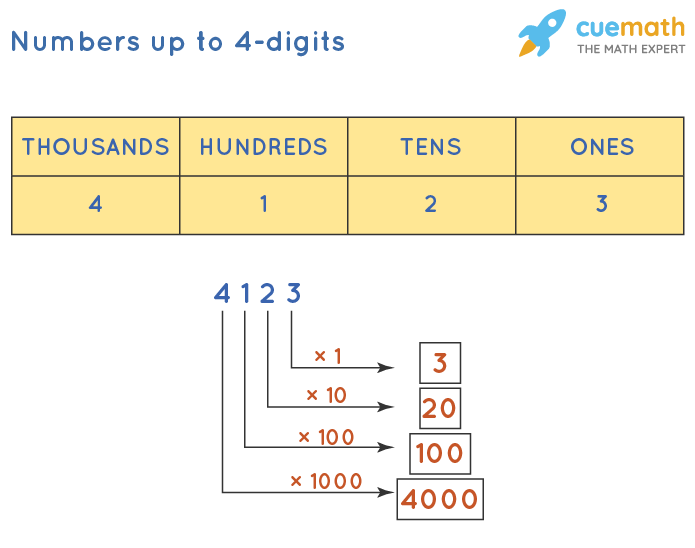
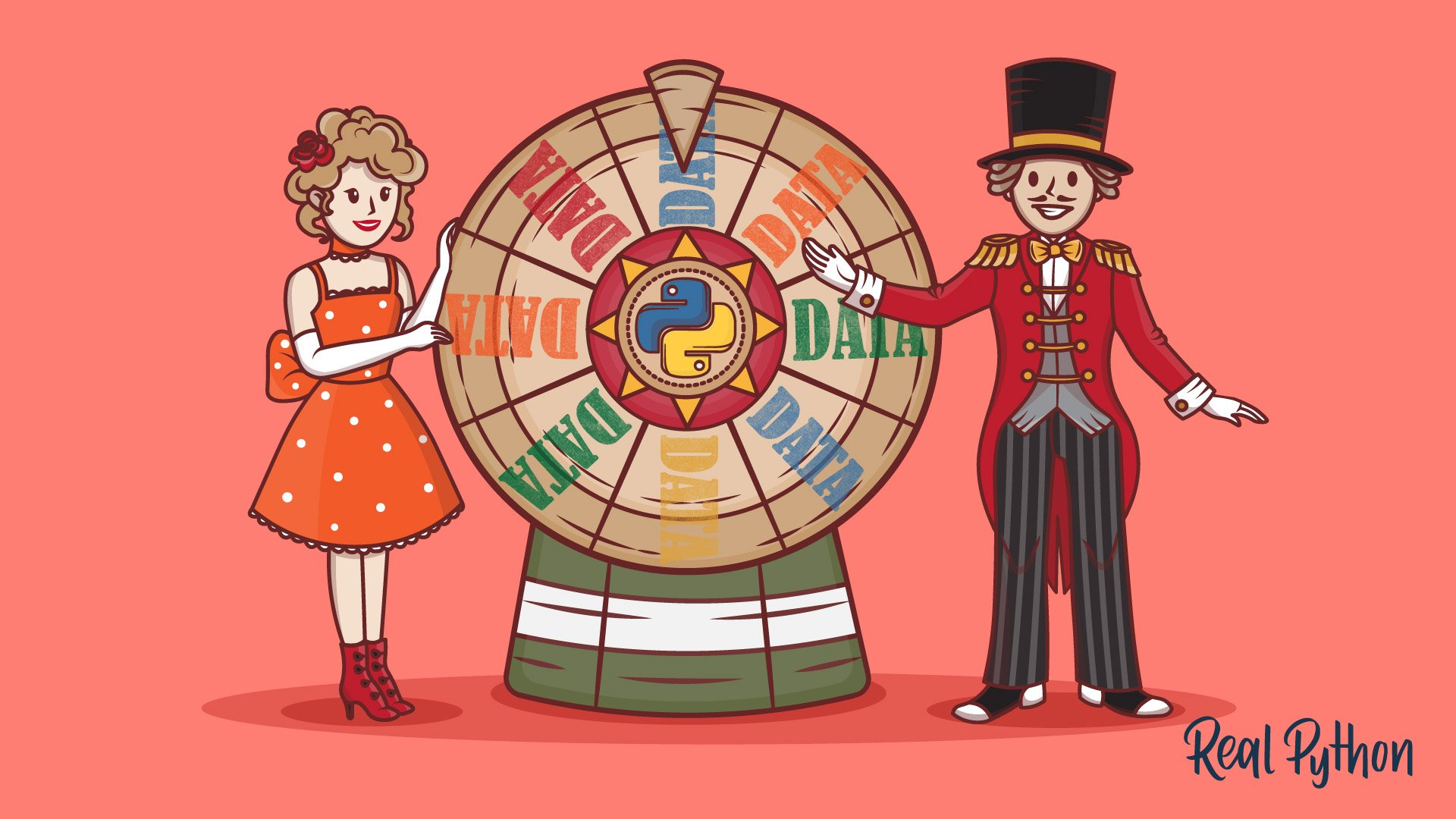
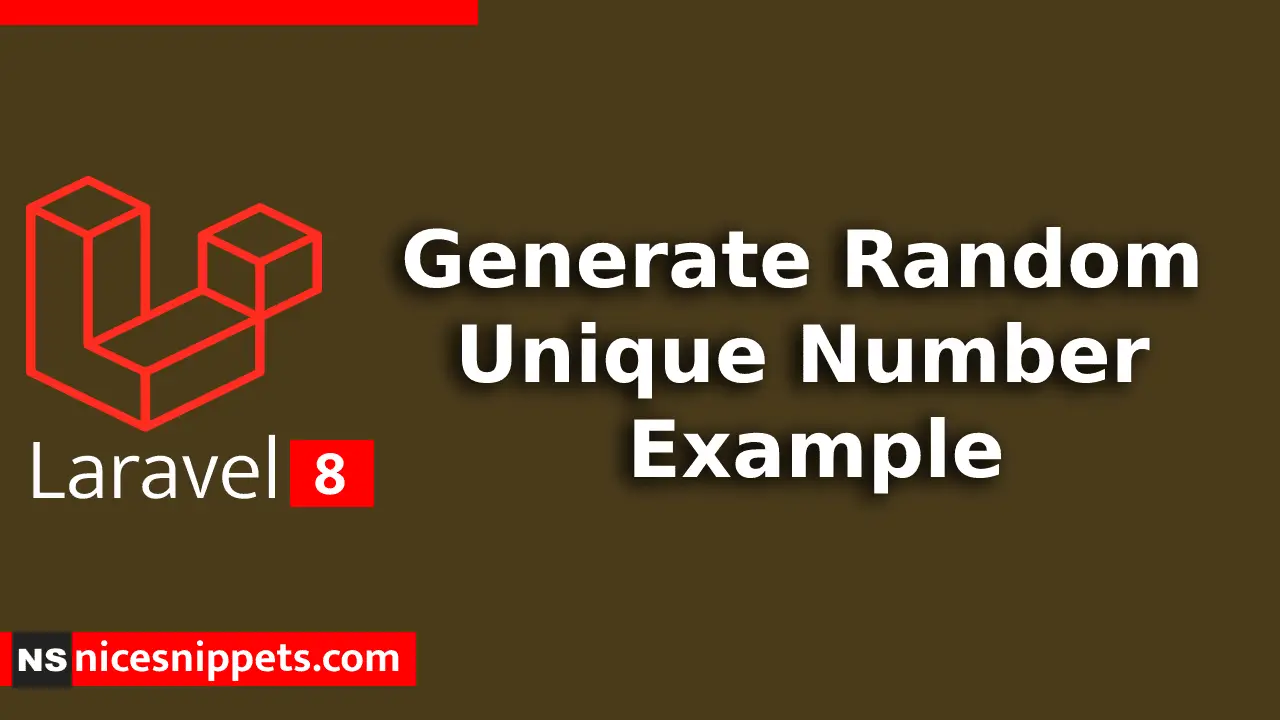
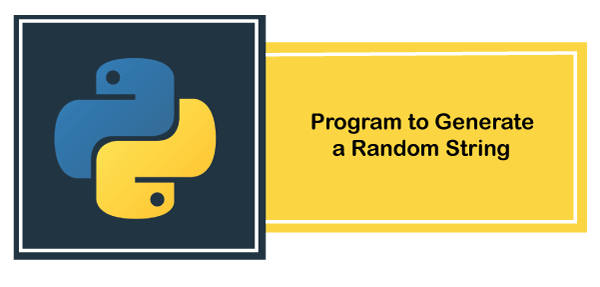
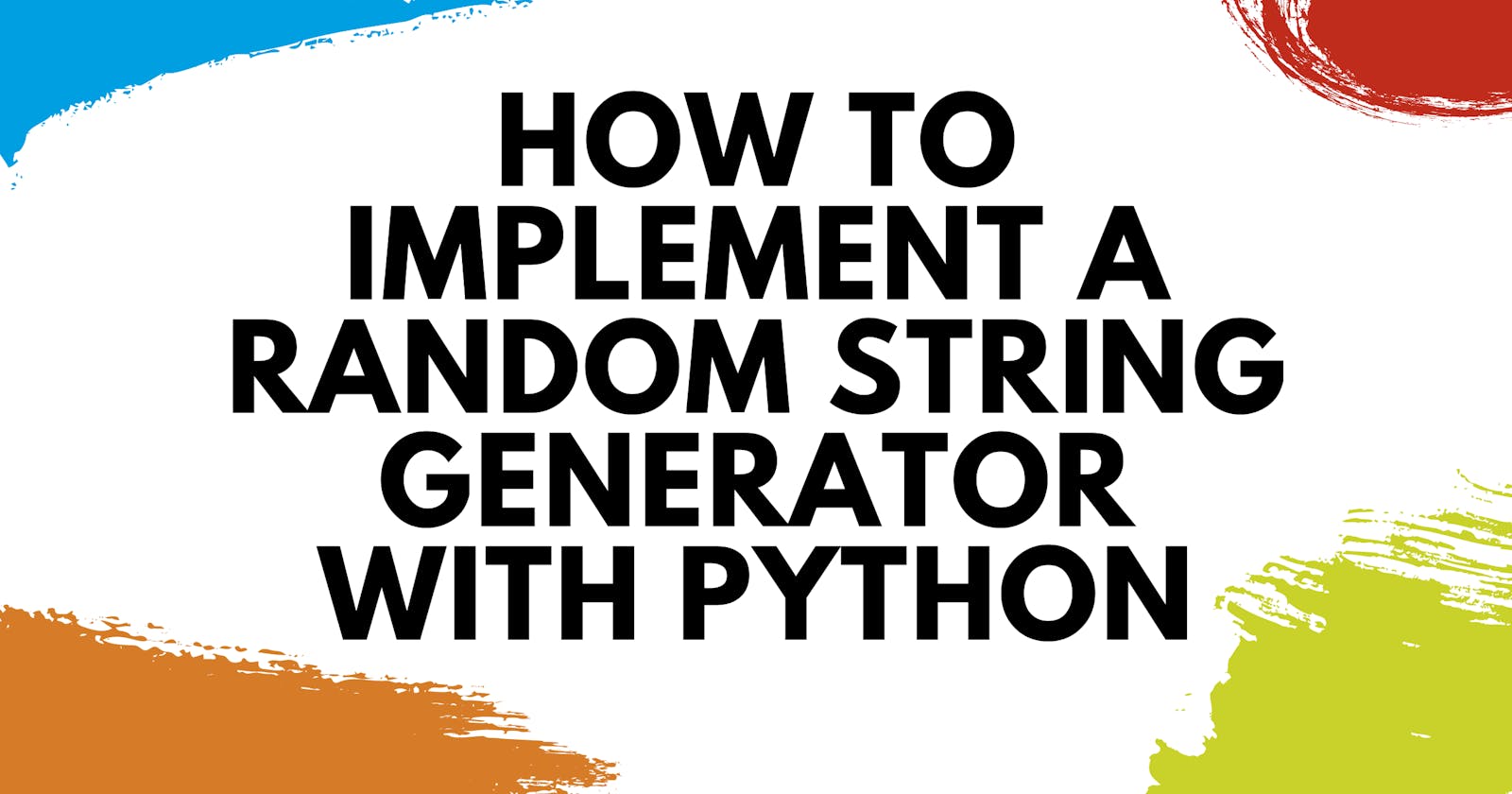
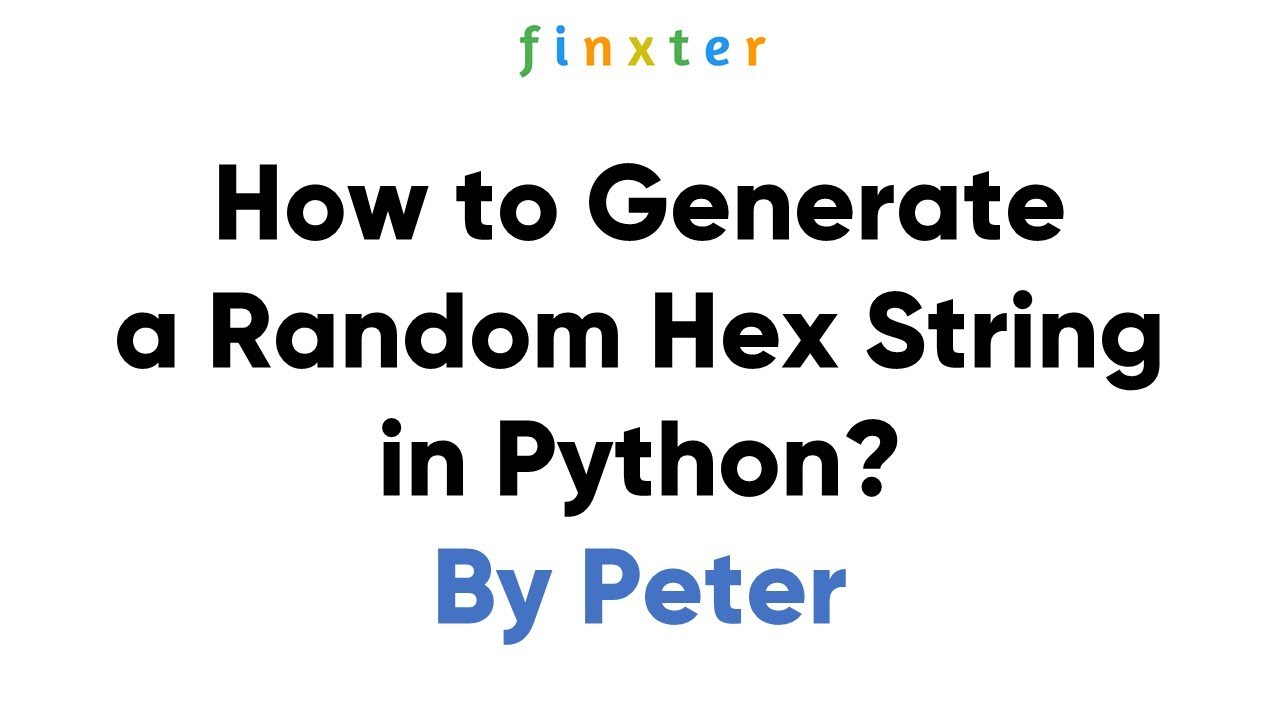
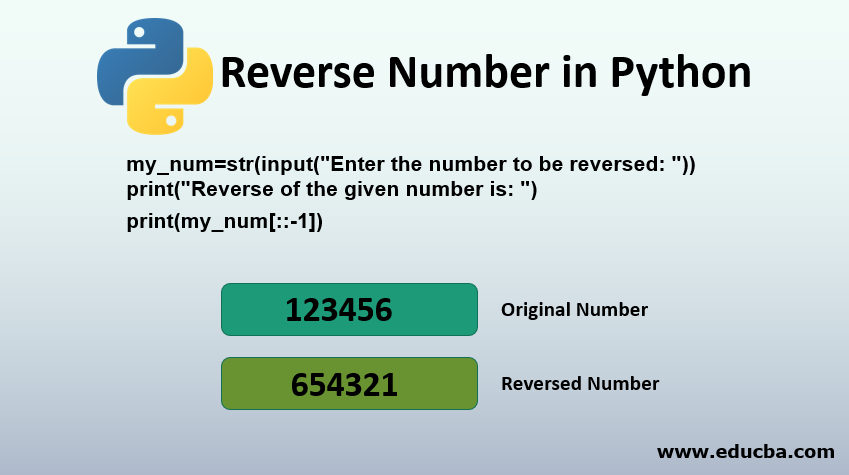
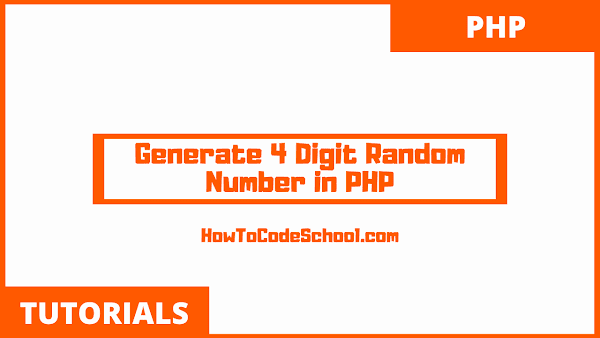
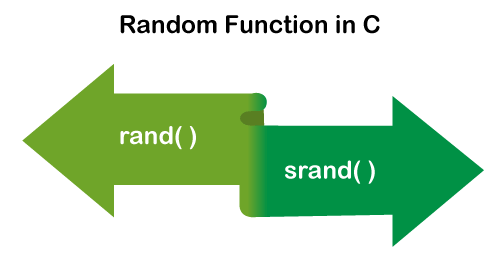
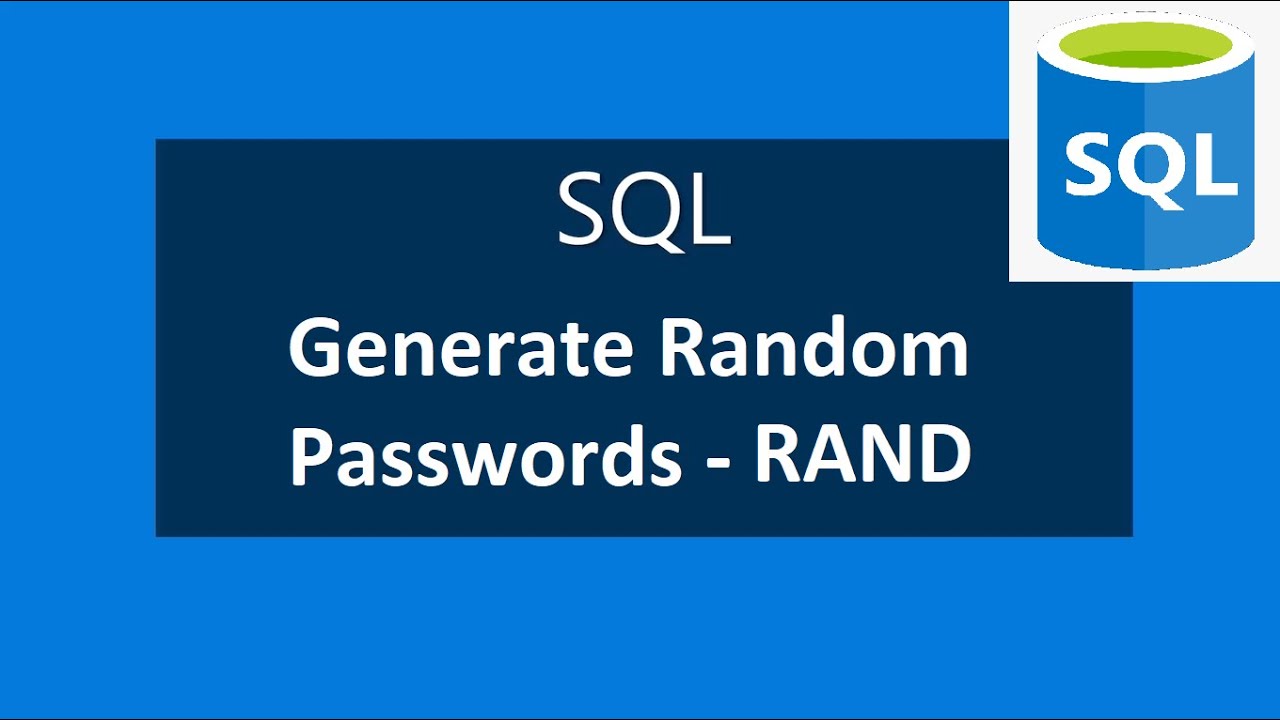
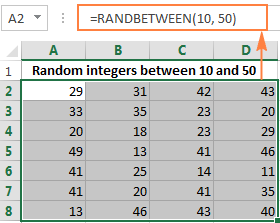
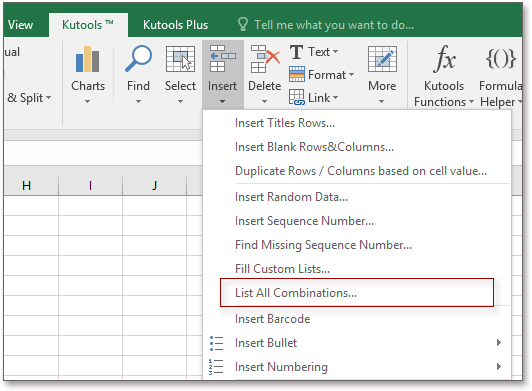
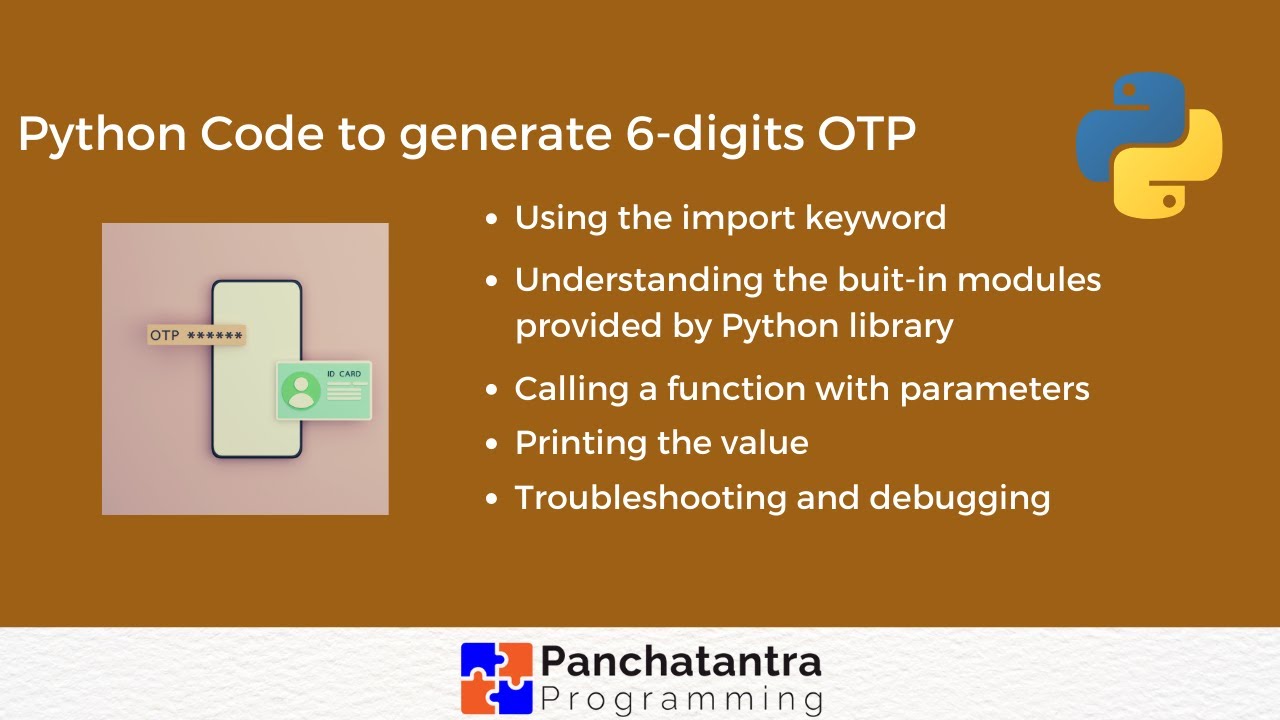

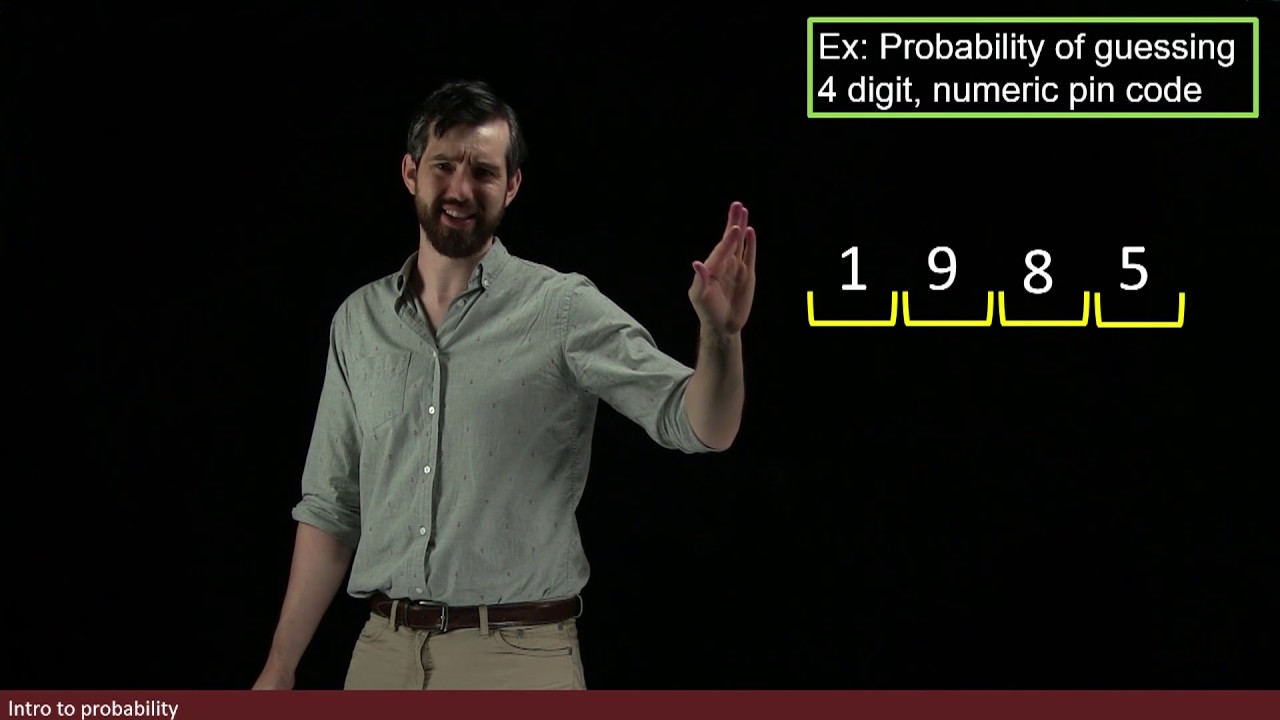
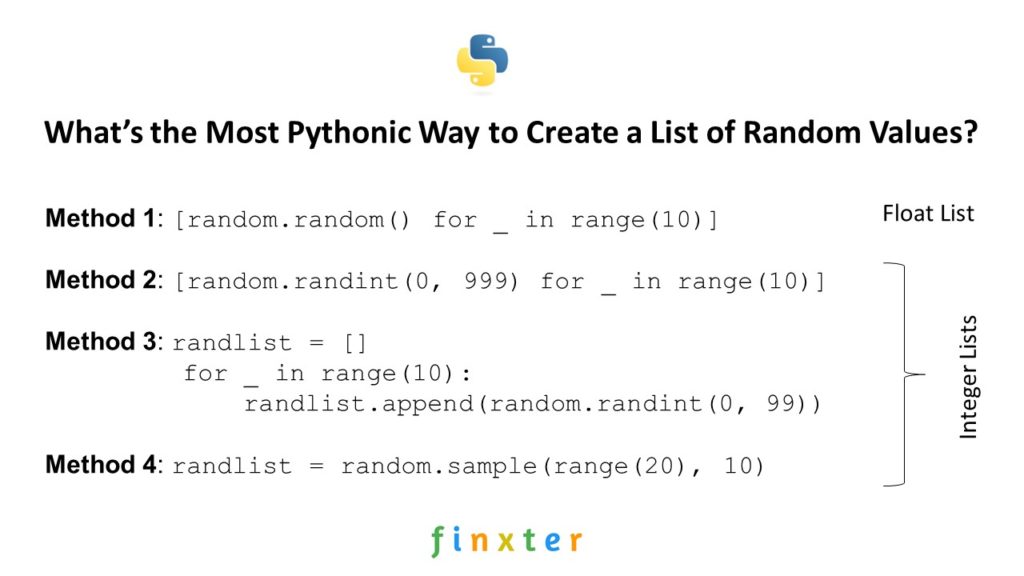
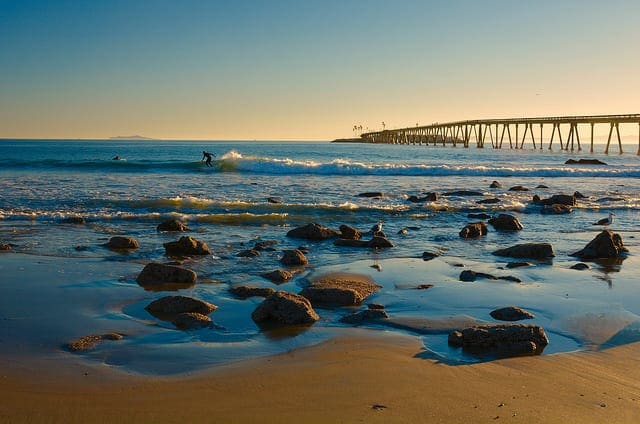
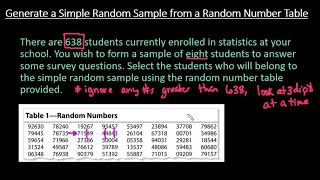

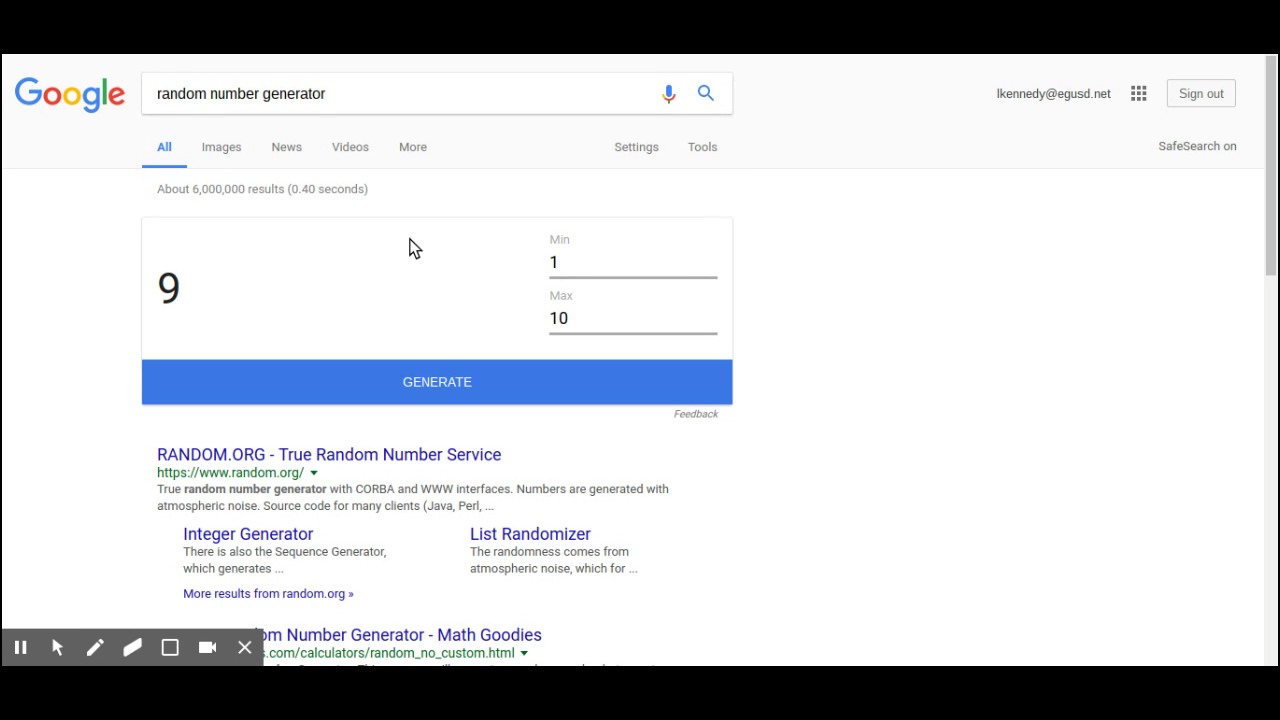

Article link: generate 4 digit random number in python.
Learn more about the topic generate 4 digit random number in python.
- Generate Random 4 Digit Number in Python | Delft Stack
- How do I generate a random 4 digit number and store it as a …
- How to generate random numbers in Java – Educative.io
- Random Function in C – Javatpoint
- How to Make a Random Number Generator in Python
- How do you generate a 4 digit random number? – Reviews.tn
- How do you generate a 4 digit random number in Python?
- How to have random number with 4 digit. | Data360 Analyze
- How To Generate 10 Digit Random Number In Python?
- 3 Best Ways to Generate a Random Number with a … – Finxter
- How to Generate a Random Number in Python – Mindmajix
- Random 4 Digit Number Generator