Function Components Cannot Be Given Refs.
In React, refs are a way to directly reference and interact with elements or components. They allow us to access methods and properties of these elements and components. However, there is an important limitation when it comes to using refs in function components.
Function components, also known as stateless components or functional components, have gained popularity in recent years due to their simplicity and reusability. While they have numerous advantages, one of their limitations is that they cannot be given refs like class components can.
Let’s explore why function components cannot be given refs and how we can work around this limitation.
Function Components vs Class Components:
Before diving into the limitations of refs in function components, let’s briefly compare function components with class components.
Traditionally, class components have been the primary way to create components in React. They have the ability to hold state, use lifecycle methods, and accept refs. However, class components can be more complex and harder to maintain compared to function components.
Function components, on the other hand, are simpler, more lightweight, and often preferred for their ease of use. They are purely functional and do not have state or lifecycle methods by default. However, with the introduction of React hooks, function components can now have state and use other features previously limited to class components.
State and Props in Function Components:
Function components can receive and use props just like class components. Props are a way to pass data from parent components to child components.
State, on the other hand, was not available in function components until the introduction of React hooks, specifically the useState hook. With the useState hook, function components can now have local state, making them more powerful and versatile.
UseRef Hook and Function Components:
To address the limitation of not being able to use refs in function components, React provides the useRef hook. The useRef hook allows us to create a mutable value that persists across renders, similar to instance variables in class components.
To illustrate how useRef can be used, let’s consider an example where we want to focus on an input element when a button is clicked:
“`jsx
import React, { useRef } from ‘react’;
function MyComponent() {
const inputRef = useRef(null);
const handleClick = () => {
inputRef.current.focus();
};
return (
);
}
“`
In this example, we create a reference using the useRef hook and assign it to the input element. When the button is clicked, the handleClick function is called, which in turn calls the focus method on the inputRef.current, focusing on the input element.
Callback Functions in Function Components:
An alternative approach to using useRef is using callback functions to pass data or references between components. Callback functions can be used to achieve the functionality of refs in function components.
To demonstrate how callback functions can be used, let’s consider an example where we want to get the dimensions of a component from its parent:
“`jsx
import React, { useState } from ‘react’;
function ParentComponent() {
const [childDimensions, setChildDimensions] = useState(null);
const handleChildDimensions = (dimensions) => {
setChildDimensions(dimensions);
};
return (
{childDimensions && (
Child component dimensions: {childDimensions.width}px x {childDimensions.height}px
)}
);
}
function ChildComponent({ onDimensions }) {
const [dimensions, setDimensions] = useState(null);
const refCallback = (node) => {
if (node) {
const { width, height } = node.getBoundingClientRect();
setDimensions({ width, height });
onDimensions({ width, height });
}
};
return
;
}
“`
In this example, the ParentComponent holds the state for the dimensions of the ChildComponent. The ChildComponent uses a refCallback function, which is called with the DOM node of the component. It then calculates the width and height using getBoundingClientRect and passes these dimensions back to the parent component using the onDimensions callback function.
Memoization in Function Components:
Another advantage of class components is the ability to utilize memoization techniques to optimize rendering. Memoization prevents unnecessary re-renders of components when the props they receive have not changed.
In function components, we can achieve similar optimization using the useMemo hook. The useMemo hook allows us to memoize expensive computations and only recompute them when the dependencies change.
Here is an example of using the useMemo hook:
“`jsx
import React, { useMemo } from ‘react’;
function MyComponent({ data }) {
const result = useMemo(() => expensiveComputation(data), [data]);
return
;
}
“`
In this example, the expensiveComputation function is only executed when the data prop changes, thanks to the dependency list provided as the second argument to useMemo.
Passing Props to Child Components in Function Components:
Passing props to child components in function components is done the same way as in class components. We can simply pass the props as attributes to the child component in JSX.
Here is an example:
“`jsx
import React from ‘react’;
function ParentComponent() {
const message = ‘Hello, World!’;
return
}
function ChildComponent({ message }) {
return
;
}
“`
In this example, the ParentComponent passes the message prop to the ChildComponent, which then displays it in a div element.
Lifecycle Methods in Function Components:
Class components in React have lifecycle methods that allow us to perform certain actions at specific points in the component’s lifecycle, such as mounting, updating, and unmounting.
Function components, by default, don’t have lifecycle methods. However, with the introduction of React hooks, we now have the useEffect hook, which allows us to replicate the behavior of lifecycle methods in function components.
The useEffect hook can be used to perform side effects, such as fetching data, subscribing to events, or cleaning up resources when the component mounts, updates, or unmounts.
Here is an example of using the useEffect hook to fetch data when the component mounts:
“`jsx
import React, { useState, useEffect } from ‘react’;
function MyComponent() {
const [data, setData] = useState(null);
useEffect(() => {
fetchData();
}, []);
const fetchData = async () => {
const response = await fetch(‘https://api.example.com/data’);
const data = await response.json();
setData(data);
};
return
;
}
“`
In this example, the fetchData function is called inside the useEffect hook, which only runs once when the component mounts, thanks to the empty dependency list provided as the second argument.
Rerendering in Function Components:
In React, components are rerendered when their state or props change, or when their parent components rerender. This allows the UI to be automatically updated to reflect these changes.
Function components, like class components, are rerendered according to these same principles. React intelligently decides which parts of the UI need to be updated to minimize unnecessary rendering and improve performance.
ForwardRef and Function Components:
React provides a way to create components that can accept refs with the forwardRef function. This allows us to pass a ref to a child component directly from its parent component.
Let’s consider an example where we want to pass a ref to a custom input component:
“`jsx
import React, { forwardRef } from ‘react’;
const InputComponent = forwardRef((props, ref) => {
return ;
});
function ParentComponent() {
const inputRef = useRef(null);
const handleClick = () => {
inputRef.current.focus();
};
return (
);
}
“`
In this example, we create an InputComponent using the forwardRef function. This allows the ref to be passed from the ParentComponent directly to the underlying input element in the InputComponent.
React.forwardRef is an essential tool when working with function components that require refs.
React callback ref and Ref of Child Components in Function Components:
In cases where we need to access the ref of a child component in a function component, we can use callback refs. Callback refs allow us to pass a function as the ref property, which will be called with the DOM node when the component mounts or unmounts.
Here is an example:
“`jsx
import React, { useRef } from ‘react’;
function ParentComponent() {
const childRef = useRef(null);
const handleClick = () => {
childRef.current.focus();
};
return (
);
}
const ChildComponent = React.forwardRef((props, ref) => {
return ;
});
“`
In this example, the ParentComponent creates a ref using the useRef hook and passes it to the ChildComponent. The ChildComponent then forwards the ref to the underlying input element. When the button is clicked, the handleClick function is called, which calls the focus method on the childRef.current, focusing on the input element.
FAQs:
Q: Why can’t function components be given refs?
A: Function components can’t be given refs directly because they don’t have instances like class components. Refs are used to reference and interact with instances of components or DOM elements, and since function components don’t have instances, refs don’t directly apply to them.
Q: What can I use instead of refs in function components?
A: Instead of using refs, React recommends using the useRef hook, which creates a mutable value that persists across renders. This can be useful for storing and accessing data or references within function components.
Q: Can I pass a ref from a child component to its parent component in a function component?
A: Yes, you can pass a ref from a child component to its parent by using callback refs. Callback refs allow you to pass a function as the ref property, which will be called with the DOM node when the component mounts or unmounts. This allows you to access the ref of a child component in a function component.
Q: Can function components memoize expensive computations?
A: Yes, function components can memoize expensive computations using the useMemo hook. The useMemo hook allows you to memoize the result of a computation and recompute it only when the dependencies change. This can help optimize performance by preventing unnecessary computations and re-renders.
Q: Can function components have lifecycle methods?
A: Function components don’t have lifecycle methods by default. However, with the introduction of React hooks, the useEffect hook can be used to replicate the behavior of lifecycle methods in function components. The useEffect hook allows you to perform side effects, such as fetching data or subscribing to events, when the component mounts, updates, or unmounts.
Warning: Function Components Cannot Be Given Refs Warning In React Using Forwardref
Keywords searched by users: function components cannot be given refs. forwardRef, React forwardRef, Function components cannot have string refs we recommend using useRef() instead, React ref functional component, Pass ref from child to parent react functional component, forwardRef React functional component, React callback ref, React Get ref of child
Categories: Top 52 Function Components Cannot Be Given Refs.
See more here: nhanvietluanvan.com
Forwardref
Introduction
React is a popular JavaScript library for building user interfaces. Its component-based architecture allows developers to break down complex UIs into reusable and manageable pieces. In the development process, it is common to pass references to child components to enable inter-component communication or manipulate their behavior. However, in some cases, passing a ref to a child component can be challenging. This is where forwardRef comes into play. In this article, we will explore forwardRef in React in depth, discussing its purpose, usage, and potential benefits.
What is forwardRef?
forwardRef is a feature introduced in React 16.3 that allows components to pass a ref from their parent component to one of their descendants. In simpler terms, it enables the forwarding of refs through intermediate components. This feature is especially useful when you want to access the DOM node or a React component instance of a child component.
Usage of forwardRef
To use forwardRef, you typically create a functional or class component as usual, and then wrap it with the forwardRef() function. This function takes in the component being wrapped and returns a new component capable of accepting a ref.
Let’s take a look at an example to better understand its usage:
“`jsx
const ChildComponent = forwardRef((props, ref) => {
return
});
const ParentComponent = () => {
const childRef = useRef(null);
useEffect(() => {
if (childRef.current) {
// Access child component’s DOM node or instance here
}
}, []);
return
}
“`
In this example, we have a ChildComponent that accepts a ref using forwardRef. The ref is then assigned to the containing `
Benefits of forwardRef
Using forwardRef brings several benefits to your React applications:
1. Simplifies component communication: With forwardRef, you can easily pass a ref through intermediary components without manually passing it down through each level. This simplifies the codebase, enhances component reusability, and improves maintainability.
2. Access to DOM nodes: By forwarding a ref to a child component, you can easily access and manipulate its underlying DOM node. This enables you to perform actions such as focusing or scrolling programmatically.
3. Access to component instances: In addition to accessing DOM nodes, forwardRef allows you to access the instances of child components. This can be useful in scenarios where you need to call methods or access state variables of a child component directly.
4. Higher-order components (HOC) compatibility: forwardRef is fully compatible with HOCs. You can use it seamlessly with other HOCs to enhance the functionality of your components.
FAQs about forwardRef:
Q1. Can forwardRef be used with functional components?
Yes, forwardRef can be used with both functional and class components. It is not limited to a specific component type.
Q2. Can forwardRef be used multiple times in a component tree?
Yes, you can use forwardRef multiple times in a component tree. Each instance of a component wrapped with forwardRef will receive a separate ref to access different child components.
Q3. Can forwardRef be used with custom hooks?
Yes, forwardRef can be used with custom hooks. You can wrap the custom hook in a higher-order component or pass it as a prop to the component using forwardRef.
Q4. What happens if I pass a ref to a non-DOM node component using forwardRef?
If you pass a ref to a non-DOM node component using forwardRef, the ref will be assigned to the child component’s instance. However, you won’t be able to access its underlying DOM node as there is none.
Q5. Are there any performance implications of using forwardRef?
No, there are no significant performance implications of using forwardRef. React’s reconciler handles ref forwarding efficiently, allowing you to use it without worrying about performance degradation.
Conclusion
forwardRef is a powerful feature in React that simplifies component communication and enables easier access to DOM nodes or component instances. By using forwardRef, you can eliminate the complexity of passing refs through intermediary components and improve the reusability and maintainability of your codebase. Remember to use it wisely and only when necessary. With its straightforward usage and the various benefits it brings, forwardRef is a valuable tool in any React developer’s toolkit.
React Forwardref
In this article, we will dive into the concept of React forwardRef and explore its uses and benefits. We will also address some frequently asked questions to help you gain a better understanding of this essential feature.
Understanding Refs in React
Refs in React provide a way to access the underlying DOM nodes or React components created by the render method. They are commonly used in cases where you need to manipulate a specific element directly, such as focusing an input field or measuring the dimensions of an element.
Traditionally, refs can be created and accessed within a component. However, there may be scenarios where you want to pass a ref from a parent component to its nested child component. This is where the forwardRef API comes in handy.
Introducing React forwardRef
React forwardRef is a higher-order function that allows components to propagate a ref to one of their children. It allows you to gain direct access to the child component’s DOM node or React component instance without exposing unnecessary details or breaking the encapsulation.
To use forwardRef, you need to define a component using the forwardRef function. This component receives two parameters: props and ref. Inside the component, you can use the ref parameter like any other prop.
Here’s an example of how to use forwardRef:
“`jsx
const ChildComponent = React.forwardRef((props, ref) => {
return ;
});
const ParentComponent = () => {
const inputRef = React.createRef();
const handleClick = () => {
inputRef.current.focus();
};
return (
);
};
“`
In this example, we define a ChildComponent using forwardRef. The ChildComponent accepts props and ref, and the input element within the component is assigned the ref as a prop. The ParentComponent then creates a ref using the createRef() method and passes it down to the ChildComponent.
When the button within the ParentComponent is clicked, the handleClick function is triggered, and it focuses the input field through the ref.
Benefits and Use Cases of forwardRef
The forwardRef API brings several benefits to React developers. It enables easy access to nested child components, allowing you to interact with them directly. Here are some common use cases where forwardRef proves to be extremely useful:
1. Wrapping Third-Party Libraries:
When working with third-party libraries that expect a ref to be passed to a component, forwardRef becomes essential. This way, you can seamlessly integrate those libraries into your React application while keeping the ref functionality intact.
2. Higher-Order Components (HOCs):
HOCs are a widely-used pattern in React for enhancing component behavior or reusability. forwardRef allows you to preserve the ref of the original component when using HOCs, ensuring that the ref functionality is maintained throughout the component hierarchy.
3. Custom Component APIs:
By utilizing forwardRef, you can define custom APIs for your components, exposing specific methods or properties to the parent component through the ref. This allows for more granular control and manipulation of the child component’s behavior.
4. Scrollable Containers:
When dealing with scrollable containers or virtualization libraries, forwardRef enables you to imperatively scroll or manipulate the container by accessing its underlying API methods through the ref.
Now, let’s address some frequently asked questions about React forwardRef:
FAQs
Q1. Can I use forwardRef with functional components?
Yes, forwardRef is compatible with functional components. You can use it by defining the component as a function and passing it as an argument to forwardRef.
Q2. Can a component have multiple forwarded refs?
No, a component can only have a single forwarded ref. If you need to pass multiple refs to child components, you can use the useRef() hook to create a container for the refs.
Q3. Are all props forwarded with forwardRef?
Yes, all props that are passed to the component using forwardRef are forwarded to the child component. This includes both standard props and any custom props defined by the parent component.
Q4. Can I forward the ref to multiple levels within the component hierarchy?
Yes, you can forward the ref to any level within the component hierarchy. Just pass the ref from one component to another until you reach the desired component that needs access to the ref.
In conclusion, React forwardRef is a powerful feature that allows components to pass down a ref to their nested child components. It simplifies the process of accessing and manipulating specific elements while preserving encapsulation and component reusability. By understanding the concept and benefits of forwardRef, you can enhance your React development workflow and create more efficient and interactive web applications.
Images related to the topic function components cannot be given refs.
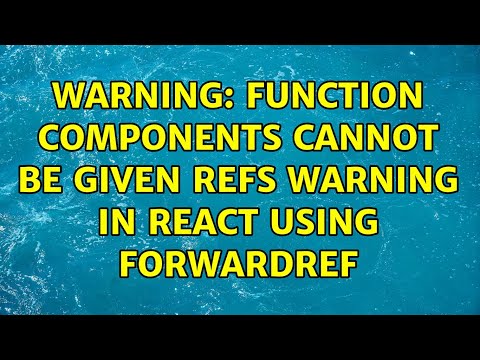
Found 21 images related to function components cannot be given refs. theme

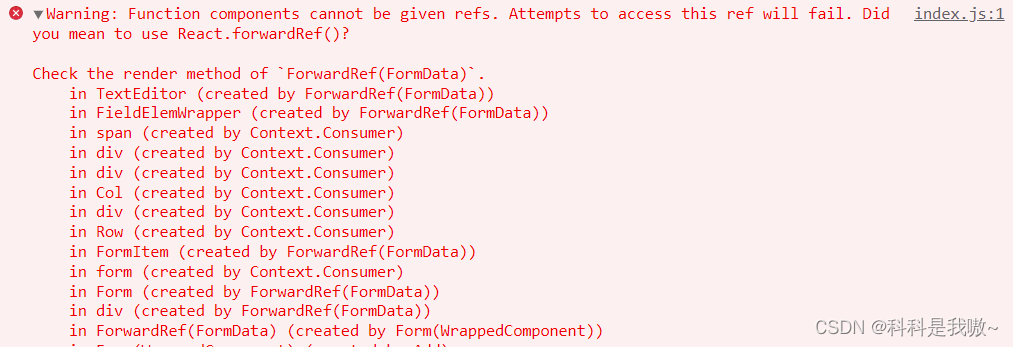

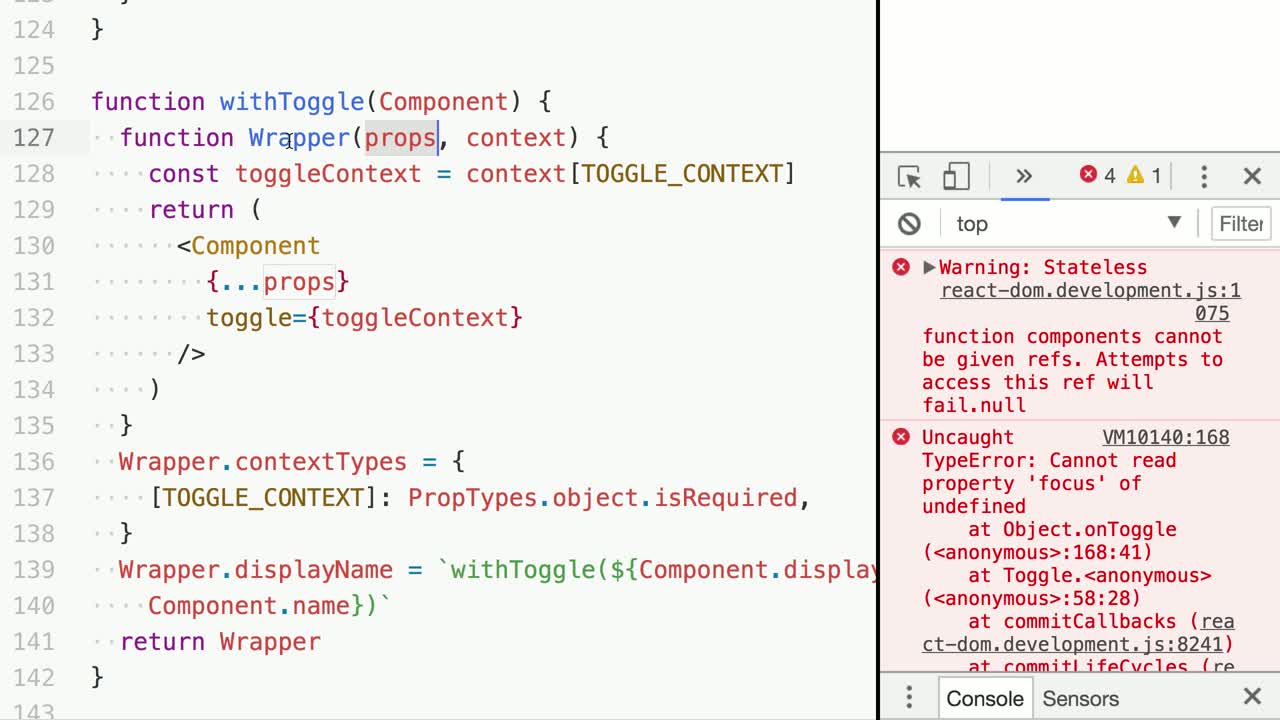
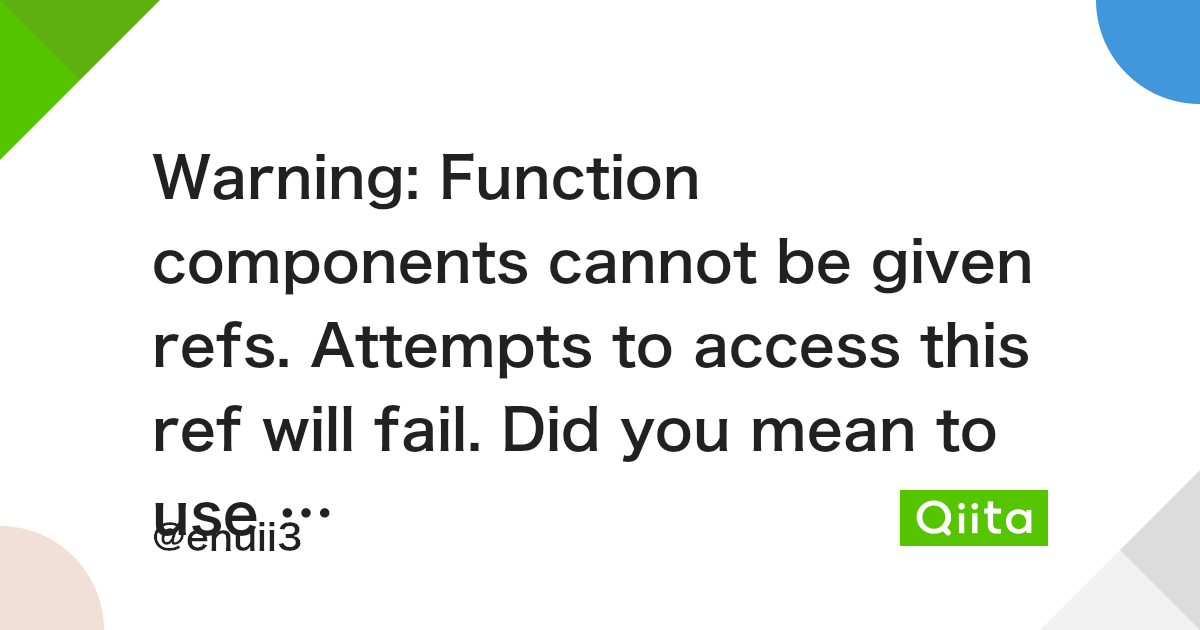

![react] Warning: Function components cannot be given refs. Attempts to access this ref will fail. Di_木木夕zzZ的博客-CSDN博客 React] Warning: Function Components Cannot Be Given Refs. Attempts To Access This Ref Will Fail. Di_木木夕Zzz的博客-Csdn博客](https://img-blog.csdnimg.cn/img_convert/4b6567c4a16f1bab5e5dc370c9a41331.png)

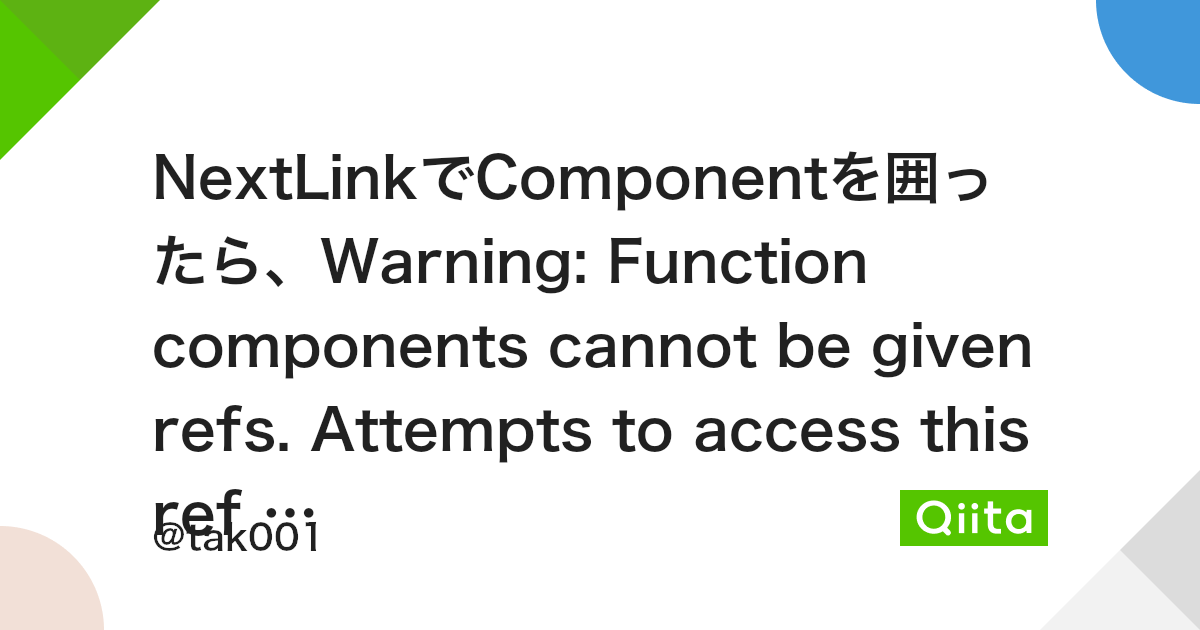

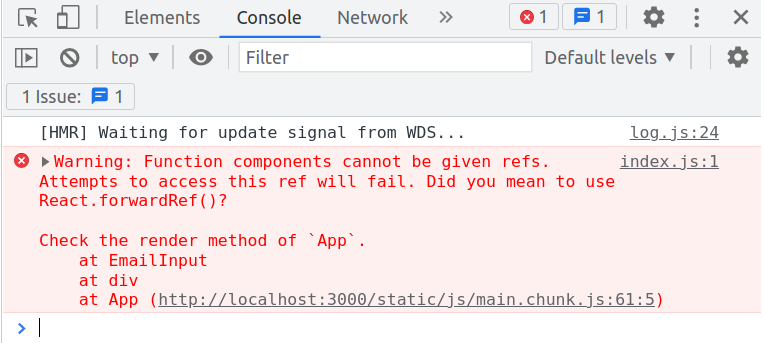


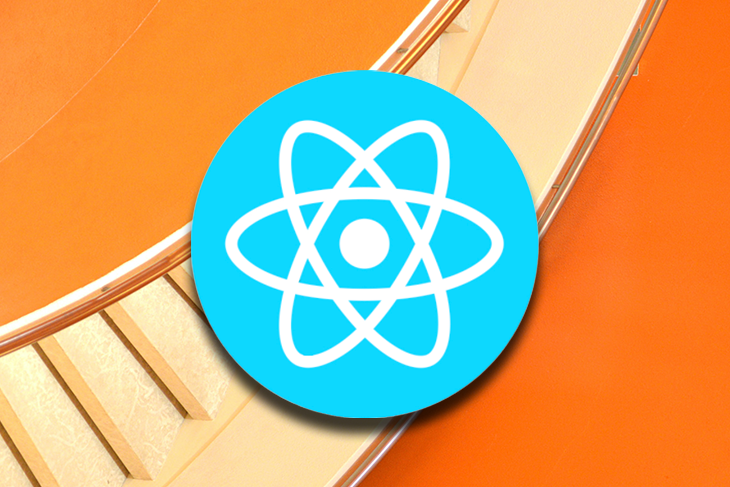
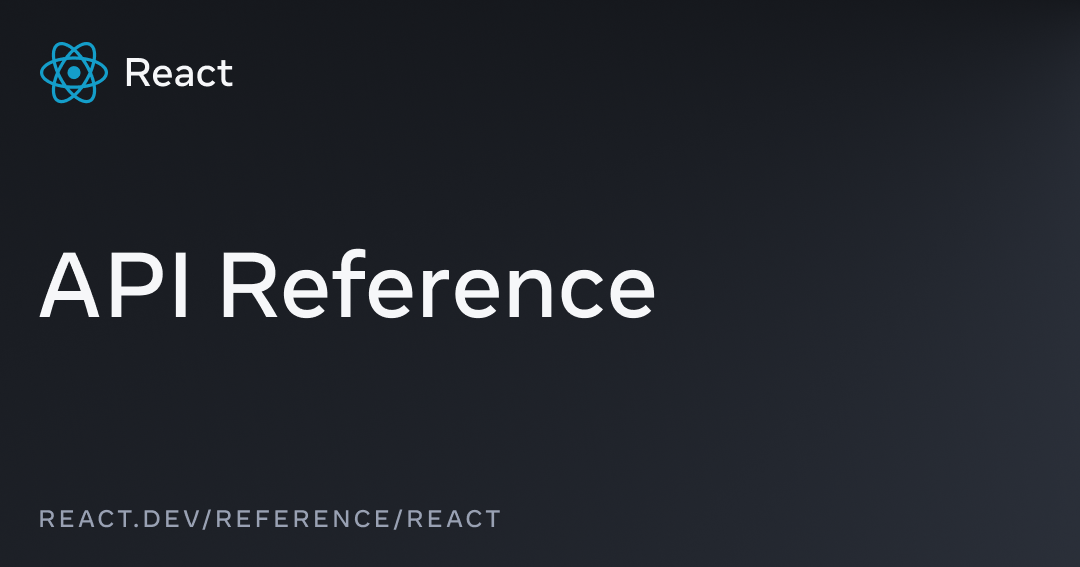

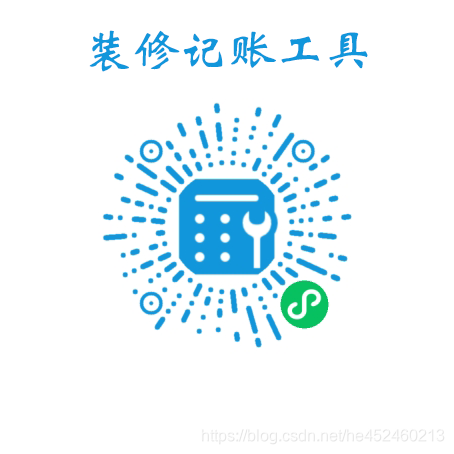
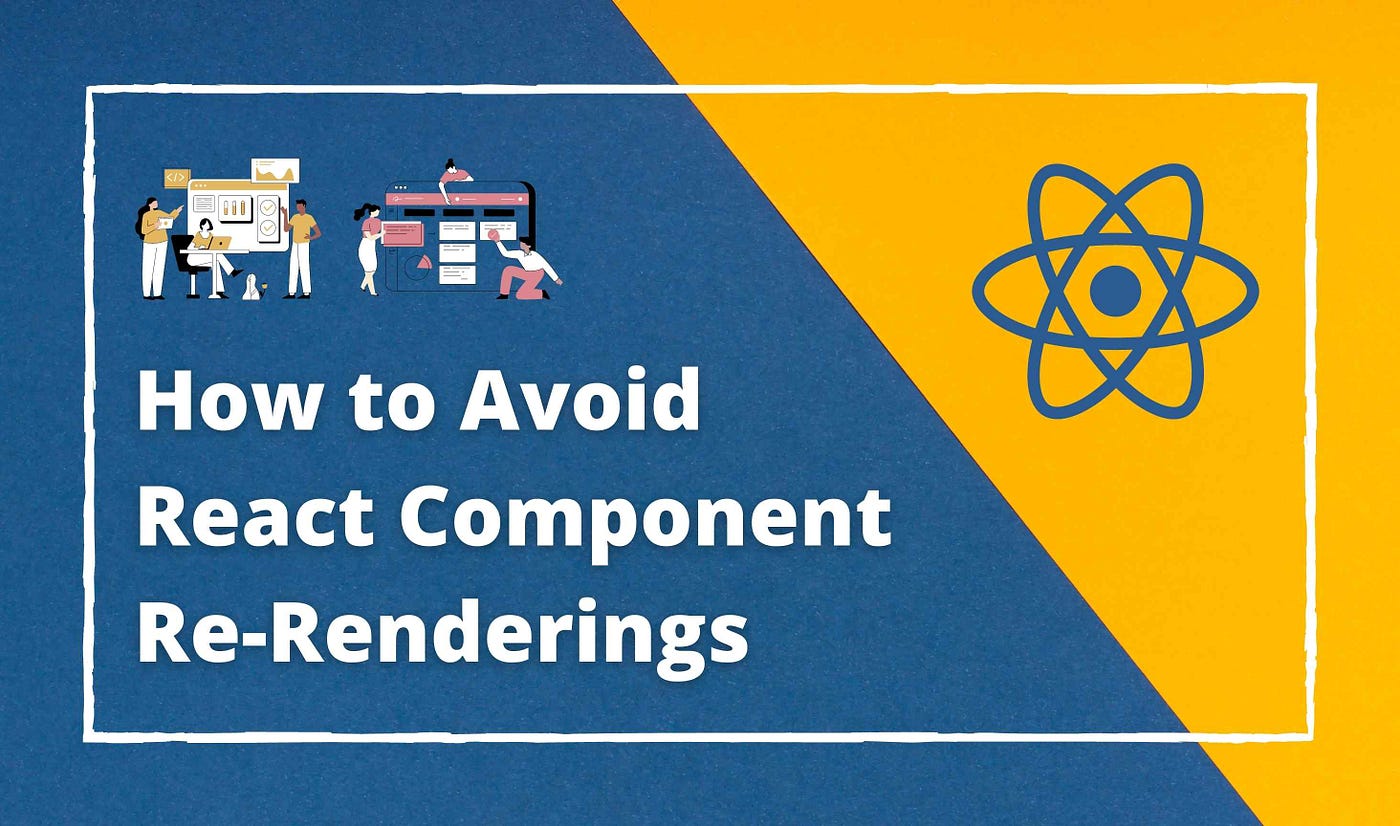
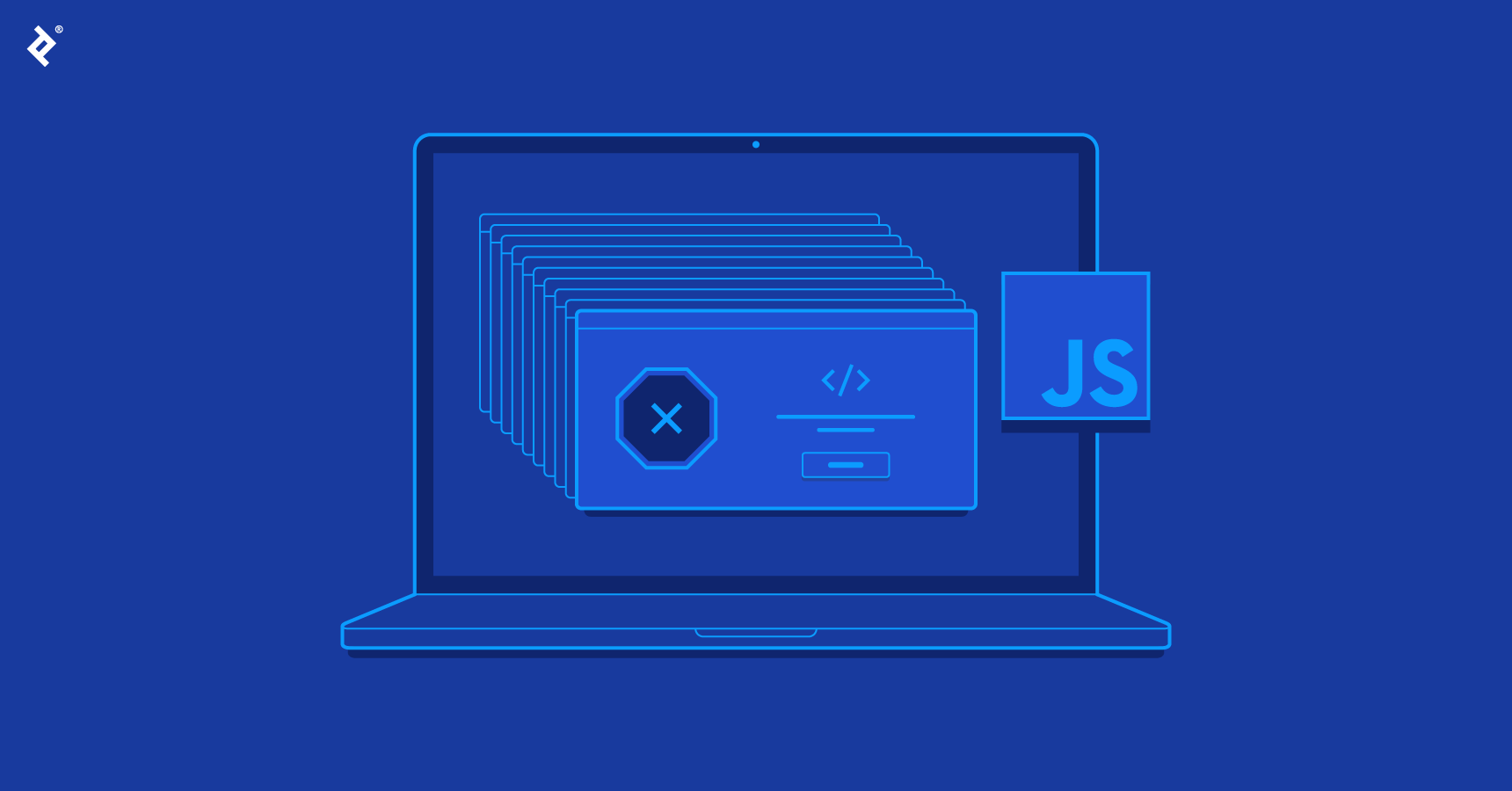
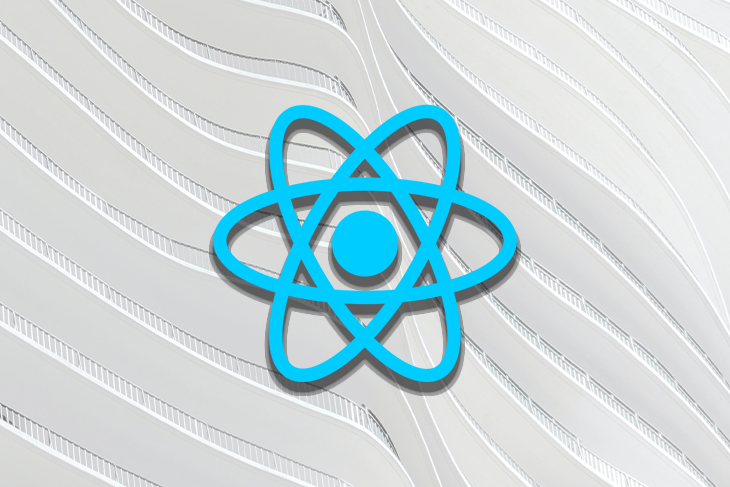

Article link: function components cannot be given refs..
Learn more about the topic function components cannot be given refs..
- How do I avoid ‘Function components cannot be given refs …
- Solved: Function components cannot be given refs … – GitHub
- Function components cannot be given refs … – bobbyhadz
- Function Components: Refs Cannot Be Assigned
- React forwardRef(): How to Pass Refs to Child Components
- ref prop should not be used on React function components
- UseRef, CreateRef, ForwardRef? What’s up with refs in React?
- Warning: Function components cannot be given refs-Reactjs
See more: nhanvietluanvan.com/luat-hoc